Welcome
Contents
Welcome#
We type code in cells and then run them.#
2 + 4
6
2 ** 4
16
days_in_week = 7
hours_in_week = days_in_week * 24
hours_in_week
168
We use libraries other people wrote#
# Some code to set up our notebook for data science!
from datascience import *
from cs104 import *
import numpy as np
%matplotlib inline
We write code to manipulate and analyze data#
hopkins_trees = Table.read_table("data/hopkins-trees.csv")
hopkins_trees.show(10)
plot | genus | species | common name | count |
---|---|---|---|---|
p00-1 | Acer | pensylvanicum | Maple, striped | 28 |
p00-1 | Acer | rubrum | Maple, red | 8 |
p00-1 | Acer | saccharum | Maple, sugar | 12 |
p00-1 | Amelanchier | canadensis | Shadbush | 17 |
p00-1 | Betula | alleghaniensis | Birch, yellow | 7 |
p00-1 | Betula | papyrifera | Birch, paper | 5 |
p00-1 | Fagus | grandifolia | Beech, American | 142 |
p00-1 | Ostrya | virginiana | Hophornbeam | 7 |
p00-1 | Prunus | serotina | Cherry, black | 11 |
p00-1 | Quercus | rubra | Oak, red | 6 |
... (3783 rows omitted)
hopkins_trees = hopkins_trees.drop("genus", "species")
hopkins_trees
plot | common name | count |
---|---|---|
p00-1 | Maple, striped | 28 |
p00-1 | Maple, red | 8 |
p00-1 | Maple, sugar | 12 |
p00-1 | Shadbush | 17 |
p00-1 | Birch, yellow | 7 |
p00-1 | Birch, paper | 5 |
p00-1 | Beech, American | 142 |
p00-1 | Hophornbeam | 7 |
p00-1 | Cherry, black | 11 |
p00-1 | Oak, red | 6 |
... (3783 rows omitted)
How many of each species?#
hopkins_trees.drop("plot").group("common name", sum).sort("count sum", descending=True)
common name | count sum |
---|---|
Beech, American | 42922 |
Maple, striped | 8939 |
Maple, red | 5564 |
Maple, sugar | 5193 |
Ash, white | 2523 |
Oak, red | 2283 |
Birch, black | 2144 |
Hophornbeam | 1613 |
Honeysuckle, Morrow's | 1608 |
Alder, speckled | 1564 |
... (67 rows omitted)
tree_counts = hopkins_trees.drop("plot").group("common name", sum)
tree_counts = tree_counts.sort("count sum", descending=True)
tree_counts.take(np.arange(0,10)).barh("common name")
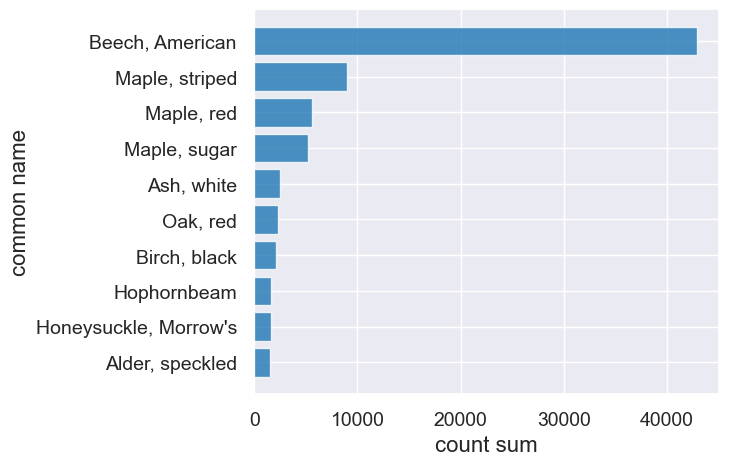
Where are all the red maples?#
red_maples = hopkins_trees.where("common name", "Maple, red")
red_maples.sort("count", descending=True)
plot | common name | count |
---|---|---|
p0621 | Maple, red | 106 |
p1236 | Maple, red | 81 |
p0821 | Maple, red | 76 |
p1032 | Maple, red | 72 |
p0629 | Maple, red | 65 |
p1133 | Maple, red | 64 |
p1141 | Maple, red | 64 |
p0630 | Maple, red | 63 |
p0622 | Maple, red | 62 |
p0940 | Maple, red | 61 |
... (341 rows omitted)
But where are those plots?#
plot_info = Table.read_table("data/hopkins-plots.csv").select("plot", "latitude", "longitude")
plot_info
plot | latitude | longitude |
---|---|---|
p00-1 | 42.7472 | -73.2759 |
p00-2 | 42.7472 | -73.2772 |
p0000 | 42.7472 | -73.2747 |
p0001 | 42.7472 | -73.2735 |
p0002 | 42.7472 | -73.2723 |
p0003 | 42.7472 | -73.271 |
p0004 | 42.7472 | -73.2698 |
p0005 | 42.7472 | -73.2686 |
p0006 | 42.7472 | -73.2673 |
p0007 | 42.7472 | -73.2661 |
... (413 rows omitted)
red_maples.join("plot", plot_info)
plot | common name | count | latitude | longitude |
---|---|---|---|---|
p00-1 | Maple, red | 8 | 42.7472 | -73.2759 |
p00-2 | Maple, red | 2 | 42.7472 | -73.2772 |
p0000 | Maple, red | 13 | 42.7472 | -73.2747 |
p0001 | Maple, red | 20 | 42.7472 | -73.2735 |
p0002 | Maple, red | 12 | 42.7472 | -73.2723 |
p0003 | Maple, red | 4 | 42.7472 | -73.271 |
p0004 | Maple, red | 2 | 42.7472 | -73.2698 |
p0005 | Maple, red | 5 | 42.7472 | -73.2686 |
p0006 | Maple, red | 3 | 42.7472 | -73.2673 |
p0007 | Maple, red | 6 | 42.7472 | -73.2661 |
... (341 rows omitted)
Visualization!#
trees_with_lat_lon = hopkins_trees.join("plot", plot_info)
def population_map(tree_name):
counts = trees_with_lat_lon.where("common name", tree_name)
counts = counts.select("latitude", "longitude", "count")
points = counts.with_columns("colors", "blue",
"areas", 1.0 * counts.column("count")).drop("count")
return Circle.map_table(points)
population_map("Maple, red")
Make this Notebook Trusted to load map: File -> Trust Notebook
Exploration, Hypotheses, and Drawing Conclusions#
all_tree_names = np.unique(np.sort(trees_with_lat_lon.column("common name")))
interact(population_map, tree_name=Choice(all_tree_names))
' <style>\n .interact-inline {\n display: flex; /* Aligns children (label, slider, readout) in a row */\n align-items: center; /* Centers the items vertically */\n font-family: var(--jp-ui-font-family);\n }\n\n .interact-label {\n width: 120px;\n overflow: hidden; /* Prevents the text from spilling out */\n text-overflow: ellipsis; /* Adds ellipses if the text overflows */\n white-space: nowrap; /* Keeps the text on a single line */\n text-align: right;\n margin-right: 10px; \n }\n\n .interact-readout {\n width: 100px;\n overflow: hidden;\n text-overflow: ellipsis;\n white-space: nowrap;\n padding-left: 20px;\n }\n\n .interact-output {\n margin-top: 10px;\n background-color: white important!;\n }\n </style>\n\n <div>\n <div class="interact-inline">\n <label class="interact-label" for="choice_i_1">tree_name</label>\n <select id="choice_i_1">\n <option data-value="Alder, speckled" value="Alder, speckled">Alder, speckled</option><option data-value="American chestnut" value="American chestnut">American chestnut</option><option data-value="Apple" value="Apple">Apple</option><option data-value="Arrow-wood" value="Arrow-wood">Arrow-wood</option><option data-value="Ash, American mountain" value="Ash, American mountain">Ash, American mountain</option><option data-value="Ash, white" value="Ash, white">Ash, white</option><option data-value="Aspen, big-toothed" value="Aspen, big-toothed">Aspen, big-toothed</option><option data-value="Aspen, quaking" value="Aspen, quaking">Aspen, quaking</option><option data-value="Aster, white wood" value="Aster, white wood">Aster, white wood</option><option data-value="Azalea, early" value="Azalea, early">Azalea, early</option><option data-value="Basswood, American" value="Basswood, American">Basswood, American</option><option data-value="Beech, American" value="Beech, American">Beech, American</option><option data-value="Birch, black" value="Birch, black">Birch, black</option><option data-value="Birch, gray" value="Birch, gray">Birch, gray</option><option data-value="Birch, paper" value="Birch, paper">Birch, paper</option><option data-value="Birch, yellow" value="Birch, yellow">Birch, yellow</option><option data-value="Bitternut hickory" value="Bitternut hickory">Bitternut hickory</option><option data-value="Bittersweet, Asiatic" value="Bittersweet, Asiatic">Bittersweet, Asiatic</option><option data-value="Bittersweet, climbing" value="Bittersweet, climbing">Bittersweet, climbing</option><option data-value="Box-elder" value="Box-elder">Box-elder</option><option data-value="Buckthorn, alder-leaved" value="Buckthorn, alder-leaved">Buckthorn, alder-leaved</option><option data-value="Buckthorn, common" value="Buckthorn, common">Buckthorn, common</option><option data-value="Buckthorn, glossy" value="Buckthorn, glossy">Buckthorn, glossy</option><option data-value="Burning bush" value="Burning bush">Burning bush</option><option data-value="Butternut" value="Butternut">Butternut</option><option data-value="Cherry, black" value="Cherry, black">Cherry, black</option><option data-value="Cherry, choke" value="Cherry, choke">Cherry, choke</option><option data-value="Cherry, pin" value="Cherry, pin">Cherry, pin</option><option data-value="Dogwood, alternate-leaved" value="Dogwood, alternate-leaved">Dogwood, alternate-leaved</option><option data-value="Dogwood, gray" value="Dogwood, gray">Dogwood, gray</option><option data-value="Elderberry, red" value="Elderberry, red">Elderberry, red</option><option data-value="Elm" value="Elm">Elm</option><option data-value="Elm, American" value="Elm, American">Elm, American</option><option data-value="Grape" value="Grape">Grape</option><option data-value="Hawthorn" value="Hawthorn">Hawthorn</option><option data-value="Hazelnut, American" value="Hazelnut, American">Hazelnut, American</option><option data-value="Hemlock, eastern" value="Hemlock, eastern">Hemlock, eastern</option><option data-value="Hickory, shagbark" value="Hickory, shagbark">Hickory, shagbark</option><option data-value="Holly, mountain" value="Holly, mountain">Holly, mountain</option><option data-value="Honeysuckle" value="Honeysuckle">Honeysuckle</option><option data-value="Honeysuckle, European fly" value="Honeysuckle, European fly">Honeysuckle, European fly</option><option data-value="Honeysuckle, Morrow\'s" value="Honeysuckle, Morrow\'s">Honeysuckle, Morrow\'s</option><option data-value="Honeysuckle, Tartarian" value="Honeysuckle, Tartarian">Honeysuckle, Tartarian</option><option data-value="Hophornbeam" value="Hophornbeam">Hophornbeam</option><option data-value="Japanese barberry" value="Japanese barberry">Japanese barberry</option><option data-value="Larch" value="Larch">Larch</option><option data-value="Locust, black" value="Locust, black">Locust, black</option><option data-value="Maleberry" value="Maleberry">Maleberry</option><option data-value="Maple, Norway" value="Maple, Norway">Maple, Norway</option><option data-value="Maple, mountain" value="Maple, mountain">Maple, mountain</option><option data-value="Maple, red" value="Maple, red">Maple, red</option><option data-value="Maple, striped" value="Maple, striped">Maple, striped</option><option data-value="Maple, sugar" value="Maple, sugar">Maple, sugar</option><option data-value="Musclewood" value="Musclewood">Musclewood</option><option data-value="Nannyberry" value="Nannyberry">Nannyberry</option><option data-value="Oak, black" value="Oak, black">Oak, black</option><option data-value="Oak, red" value="Oak, red">Oak, red</option><option data-value="Oak, white" value="Oak, white">Oak, white</option><option data-value="Olive, Russian" value="Olive, Russian">Olive, Russian</option><option data-value="Pine, red" value="Pine, red">Pine, red</option><option data-value="Pine, white" value="Pine, white">Pine, white</option><option data-value="Plantation spruce" value="Plantation spruce">Plantation spruce</option><option data-value="Plot converted to meadow" value="Plot converted to meadow">Plot converted to meadow</option><option data-value="Poplar species" value="Poplar species">Poplar species</option><option data-value="Poplar, hybrid in plantation" value="Poplar, hybrid in plantation">Poplar, hybrid in plantation</option><option data-value="Privet" value="Privet">Privet</option><option data-value="Rose" value="Rose">Rose</option><option data-value="Rose, multiflora" value="Rose, multiflora">Rose, multiflora</option><option data-value="Saphireberry" value="Saphireberry">Saphireberry</option><option data-value="Shadbush" value="Shadbush">Shadbush</option><option data-value="Spicebush" value="Spicebush">Spicebush</option><option data-value="Spruce, Norway" value="Spruce, Norway">Spruce, Norway</option><option data-value="Spruce, red" value="Spruce, red">Spruce, red</option><option data-value="Virginia creeper" value="Virginia creeper">Virginia creeper</option><option data-value="Willow, black" value="Willow, black">Willow, black</option><option data-value="Witch-hazel" value="Witch-hazel">Witch-hazel</option><option data-value="Withe-rod" value="Withe-rod">Withe-rod</option>\n </select>\n</div>\n\n <div class="interact-output" id="output_i_2"></div>\n </div>\n\n <script>\n\n var _choice_i_1 = document.getElementById(\'choice_i_1\');\n function i_1_value() { return _choice_i_1.value; }\n\n function createCSVLine(values) {\n return values.map(value => {\n let stringValue = value.toString();\n // Escape existing double quotes\n stringValue = stringValue.replace(/"/g, \'""\');\n // If the value contains a comma, newline or double quote, enclose it in double quotes\n if (stringValue.includes(\',\') || stringValue.includes(\'\\n\') || stringValue.includes(\'"\')) {\n stringValue = `"${stringValue}"`;\n }\n return stringValue;\n }).join(\',\');\n }\n\n var _output_i_2 = document.getElementById(\'output_i_2\');\n var _cache_i_2 = {\n "\\"Alder, speckled\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_636b41bf1ae788accbb9a71b09a8371b {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_636b41bf1ae788accbb9a71b09a8371b" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_636b41bf1ae788accbb9a71b09a8371b = L.map(\\n "map_636b41bf1ae788accbb9a71b09a8371b",\\n {\\n center: [42.73545109854018, -73.24026986949026],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_10c95c855b082b50945da631d136e201 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var circle_marker_a1756dd7acaf980d48b190d211251886 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.715176518808164, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_b7bdba836c5821c64c731b3323092b6c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e5b099ee124afc6500164ada4df29a0 = $(`<div id="html_1e5b099ee124afc6500164ada4df29a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b7bdba836c5821c64c731b3323092b6c.setContent(html_1e5b099ee124afc6500164ada4df29a0);\\n \\n \\n\\n circle_marker_a1756dd7acaf980d48b190d211251886.bindPopup(popup_b7bdba836c5821c64c731b3323092b6c)\\n ;\\n\\n \\n \\n \\n var circle_marker_66c4f9b106264f44f03db74c88a70c84 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_95188536f4d942f70a03c5a74c62c263 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5d00d0eeacadf4a479cc3ce2c33b9410 = $(`<div id="html_5d00d0eeacadf4a479cc3ce2c33b9410" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95188536f4d942f70a03c5a74c62c263.setContent(html_5d00d0eeacadf4a479cc3ce2c33b9410);\\n \\n \\n\\n circle_marker_66c4f9b106264f44f03db74c88a70c84.bindPopup(popup_95188536f4d942f70a03c5a74c62c263)\\n ;\\n\\n \\n \\n \\n var circle_marker_ae5d6fad1ca64a96dece054b2b39f5c0 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_b9b2ed795b4c7a41579a966d7783f5c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_424a9215b154a47ff7e00a1f2cf345bb = $(`<div id="html_424a9215b154a47ff7e00a1f2cf345bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b9b2ed795b4c7a41579a966d7783f5c8.setContent(html_424a9215b154a47ff7e00a1f2cf345bb);\\n \\n \\n\\n circle_marker_ae5d6fad1ca64a96dece054b2b39f5c0.bindPopup(popup_b9b2ed795b4c7a41579a966d7783f5c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_50021220b38854a1b93be5346c243cd3 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.778826859036359, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_83ef5480badcfb2d4c9a6267bf92a8da = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5da3a48c8e3bd871f14ddfddbc6abdc = $(`<div id="html_e5da3a48c8e3bd871f14ddfddbc6abdc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83ef5480badcfb2d4c9a6267bf92a8da.setContent(html_e5da3a48c8e3bd871f14ddfddbc6abdc);\\n \\n \\n\\n circle_marker_50021220b38854a1b93be5346c243cd3.bindPopup(popup_83ef5480badcfb2d4c9a6267bf92a8da)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f36b4ca97ea63169b6396f4fed90438 = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_3da7aeb7c6a0d9663c71784a23905e57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0bd18c352c9f8a53b9b19dae77876493 = $(`<div id="html_0bd18c352c9f8a53b9b19dae77876493" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3da7aeb7c6a0d9663c71784a23905e57.setContent(html_0bd18c352c9f8a53b9b19dae77876493);\\n \\n \\n\\n circle_marker_5f36b4ca97ea63169b6396f4fed90438.bindPopup(popup_3da7aeb7c6a0d9663c71784a23905e57)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3dc681f308370fa305bc194a100b42a = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_0288cb9a63127aff6166aad8bfbce1ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c649d65eb9026cf51bd2f2b20253e847 = $(`<div id="html_c649d65eb9026cf51bd2f2b20253e847" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0288cb9a63127aff6166aad8bfbce1ef.setContent(html_c649d65eb9026cf51bd2f2b20253e847);\\n \\n \\n\\n circle_marker_c3dc681f308370fa305bc194a100b42a.bindPopup(popup_0288cb9a63127aff6166aad8bfbce1ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a572cf37da3070fc68f6b6c373bb2eb = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_d6401e1e0f9c6d196ea770d95960c909 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a1d6b01fb8b4764f6f29a67a07a9eaf = $(`<div id="html_0a1d6b01fb8b4764f6f29a67a07a9eaf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d6401e1e0f9c6d196ea770d95960c909.setContent(html_0a1d6b01fb8b4764f6f29a67a07a9eaf);\\n \\n \\n\\n circle_marker_3a572cf37da3070fc68f6b6c373bb2eb.bindPopup(popup_d6401e1e0f9c6d196ea770d95960c909)\\n ;\\n\\n \\n \\n \\n var circle_marker_26fc5ddb0f98121a7a9e5a2ff9cd5c1e = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 11.084566571000565, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_2916465c18cedac6ee723ac0269ee58d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb38b318051e76ce4ea45162ad88649c = $(`<div id="html_fb38b318051e76ce4ea45162ad88649c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2916465c18cedac6ee723ac0269ee58d.setContent(html_fb38b318051e76ce4ea45162ad88649c);\\n \\n \\n\\n circle_marker_26fc5ddb0f98121a7a9e5a2ff9cd5c1e.bindPopup(popup_2916465c18cedac6ee723ac0269ee58d)\\n ;\\n\\n \\n \\n \\n var circle_marker_1752bb663dacee379a78be9e39e44b07 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_fe6a87be605c27092d97b6fab6afa8e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e80c03a3baf1b52254a9a31f06d16624 = $(`<div id="html_e80c03a3baf1b52254a9a31f06d16624" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe6a87be605c27092d97b6fab6afa8e2.setContent(html_e80c03a3baf1b52254a9a31f06d16624);\\n \\n \\n\\n circle_marker_1752bb663dacee379a78be9e39e44b07.bindPopup(popup_fe6a87be605c27092d97b6fab6afa8e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9662551f734250df5298570079a3701 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1073621666150775, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_828c466557f411065d623257431387f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76f777dcdc3caf93506ea24a7eee8ea1 = $(`<div id="html_76f777dcdc3caf93506ea24a7eee8ea1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_828c466557f411065d623257431387f6.setContent(html_76f777dcdc3caf93506ea24a7eee8ea1);\\n \\n \\n\\n circle_marker_e9662551f734250df5298570079a3701.bindPopup(popup_828c466557f411065d623257431387f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_8291646935ef330a6195df1f7b7bc619 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.232078956954491, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_253836bc42cbcb810e5d64da2e2fb1fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25f8f94055e558b84f0ee9f94edbc16b = $(`<div id="html_25f8f94055e558b84f0ee9f94edbc16b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_253836bc42cbcb810e5d64da2e2fb1fb.setContent(html_25f8f94055e558b84f0ee9f94edbc16b);\\n \\n \\n\\n circle_marker_8291646935ef330a6195df1f7b7bc619.bindPopup(popup_253836bc42cbcb810e5d64da2e2fb1fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_df273a9b72022e1a939fc451384e64aa = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.652426491681278, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_1f8ad4155cea086c78403261a9b49423 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c30b33019221a29125705873384984d = $(`<div id="html_0c30b33019221a29125705873384984d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f8ad4155cea086c78403261a9b49423.setContent(html_0c30b33019221a29125705873384984d);\\n \\n \\n\\n circle_marker_df273a9b72022e1a939fc451384e64aa.bindPopup(popup_1f8ad4155cea086c78403261a9b49423)\\n ;\\n\\n \\n \\n \\n var circle_marker_03e25b12819540044bf164079d053655 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_d294c1c8961cee3c1f5769d8cbc13d56 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8720a53c5f571e6affde652c900a27a = $(`<div id="html_d8720a53c5f571e6affde652c900a27a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d294c1c8961cee3c1f5769d8cbc13d56.setContent(html_d8720a53c5f571e6affde652c900a27a);\\n \\n \\n\\n circle_marker_03e25b12819540044bf164079d053655.bindPopup(popup_d294c1c8961cee3c1f5769d8cbc13d56)\\n ;\\n\\n \\n \\n \\n var circle_marker_025663551635572ee84617bdd6825981 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_eb67e8015112c9ac0bd030d18d2b08a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_684cb4b5a9a369530ad7f7daf279d3c1 = $(`<div id="html_684cb4b5a9a369530ad7f7daf279d3c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb67e8015112c9ac0bd030d18d2b08a8.setContent(html_684cb4b5a9a369530ad7f7daf279d3c1);\\n \\n \\n\\n circle_marker_025663551635572ee84617bdd6825981.bindPopup(popup_eb67e8015112c9ac0bd030d18d2b08a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_df8beee39a1d0be45f36dfc205397442 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_f6d57675ce539ede0017a9919ff541f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44f916865d43d64a708a07d25b135de3 = $(`<div id="html_44f916865d43d64a708a07d25b135de3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6d57675ce539ede0017a9919ff541f8.setContent(html_44f916865d43d64a708a07d25b135de3);\\n \\n \\n\\n circle_marker_df8beee39a1d0be45f36dfc205397442.bindPopup(popup_f6d57675ce539ede0017a9919ff541f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_57d362db6a579af92b8750d36ed26730 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_f043845215fc5603025ecab5e61f6dd8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0733e9ca5ccb4325e477dc822ece61da = $(`<div id="html_0733e9ca5ccb4325e477dc822ece61da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f043845215fc5603025ecab5e61f6dd8.setContent(html_0733e9ca5ccb4325e477dc822ece61da);\\n \\n \\n\\n circle_marker_57d362db6a579af92b8750d36ed26730.bindPopup(popup_f043845215fc5603025ecab5e61f6dd8)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5cdf074e4e823a475a951648c12753d = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_e168f0037973b015c616e2e5167c2e89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86e91dc42314ff9542ee3def3461b678 = $(`<div id="html_86e91dc42314ff9542ee3def3461b678" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e168f0037973b015c616e2e5167c2e89.setContent(html_86e91dc42314ff9542ee3def3461b678);\\n \\n \\n\\n circle_marker_d5cdf074e4e823a475a951648c12753d.bindPopup(popup_e168f0037973b015c616e2e5167c2e89)\\n ;\\n\\n \\n \\n \\n var circle_marker_395b7092bb750853fc5a709592353c92 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_636b41bf1ae788accbb9a71b09a8371b);\\n \\n \\n var popup_74bd66e0ae2b811361f7aa429963af1a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba79d1513b7f49123f9e230ffef91762 = $(`<div id="html_ba79d1513b7f49123f9e230ffef91762" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_74bd66e0ae2b811361f7aa429963af1a.setContent(html_ba79d1513b7f49123f9e230ffef91762);\\n \\n \\n\\n circle_marker_395b7092bb750853fc5a709592353c92.bindPopup(popup_74bd66e0ae2b811361f7aa429963af1a)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "American chestnut": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_aaaa0947dc784648579c7f620e1d3393 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_aaaa0947dc784648579c7f620e1d3393" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_aaaa0947dc784648579c7f620e1d3393 = L.map(\\n "map_aaaa0947dc784648579c7f620e1d3393",\\n {\\n center: [42.736358328997184, -73.25072597440742],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_0345d201e4725d212d212fda15d29b51 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var circle_marker_45ea8f2dcc9d70d8d6836e67e886bdde = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_57cab41639ee930054bd7c4bfa2cbd57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c95f876aeef9a8fe76f619a4771d2e08 = $(`<div id="html_c95f876aeef9a8fe76f619a4771d2e08" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_57cab41639ee930054bd7c4bfa2cbd57.setContent(html_c95f876aeef9a8fe76f619a4771d2e08);\\n \\n \\n\\n circle_marker_45ea8f2dcc9d70d8d6836e67e886bdde.bindPopup(popup_57cab41639ee930054bd7c4bfa2cbd57)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6171f34f0b96a67f1524b1265d88524 = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_8131efdbff2b8f78df61ed8047ad6f8e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95523ad067c8310ce6b0aa4b639779a7 = $(`<div id="html_95523ad067c8310ce6b0aa4b639779a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8131efdbff2b8f78df61ed8047ad6f8e.setContent(html_95523ad067c8310ce6b0aa4b639779a7);\\n \\n \\n\\n circle_marker_f6171f34f0b96a67f1524b1265d88524.bindPopup(popup_8131efdbff2b8f78df61ed8047ad6f8e)\\n ;\\n\\n \\n \\n \\n var circle_marker_06a9359e9505df391493c2b53f1813ff = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_3d0a94de88276c1bdf22fce8b2df0d4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3ad3ba2f6ec2a6bda8e63a5cf63e2f1 = $(`<div id="html_f3ad3ba2f6ec2a6bda8e63a5cf63e2f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d0a94de88276c1bdf22fce8b2df0d4c.setContent(html_f3ad3ba2f6ec2a6bda8e63a5cf63e2f1);\\n \\n \\n\\n circle_marker_06a9359e9505df391493c2b53f1813ff.bindPopup(popup_3d0a94de88276c1bdf22fce8b2df0d4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_1237edf907d49c685addb24dfc755c07 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_615989c2d129c5863e19afbfef06b24e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5312cbc450ceaef79d775ad01b3901b9 = $(`<div id="html_5312cbc450ceaef79d775ad01b3901b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_615989c2d129c5863e19afbfef06b24e.setContent(html_5312cbc450ceaef79d775ad01b3901b9);\\n \\n \\n\\n circle_marker_1237edf907d49c685addb24dfc755c07.bindPopup(popup_615989c2d129c5863e19afbfef06b24e)\\n ;\\n\\n \\n \\n \\n var circle_marker_86a28a1d3c22982d9acbc3457ffa8b5d = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_5f5f5a916e21f671c1d12e479c026dab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea21760fe5ac5a253e73d2491402aae1 = $(`<div id="html_ea21760fe5ac5a253e73d2491402aae1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f5f5a916e21f671c1d12e479c026dab.setContent(html_ea21760fe5ac5a253e73d2491402aae1);\\n \\n \\n\\n circle_marker_86a28a1d3c22982d9acbc3457ffa8b5d.bindPopup(popup_5f5f5a916e21f671c1d12e479c026dab)\\n ;\\n\\n \\n \\n \\n var circle_marker_39b0c24cb3d9be5e261c039c2cf28cd7 = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_a3de1f19e994ad88a390e618f628f9a7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9612aae98fc947096be7cde8abce03f = $(`<div id="html_a9612aae98fc947096be7cde8abce03f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3de1f19e994ad88a390e618f628f9a7.setContent(html_a9612aae98fc947096be7cde8abce03f);\\n \\n \\n\\n circle_marker_39b0c24cb3d9be5e261c039c2cf28cd7.bindPopup(popup_a3de1f19e994ad88a390e618f628f9a7)\\n ;\\n\\n \\n \\n \\n var circle_marker_d10767c69c8e15bde82de7bc8c565469 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_56de81eb48a9e79463bf931291ff69d6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ff2851b0bc3c9a16da453b1ed8ee974 = $(`<div id="html_3ff2851b0bc3c9a16da453b1ed8ee974" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_56de81eb48a9e79463bf931291ff69d6.setContent(html_3ff2851b0bc3c9a16da453b1ed8ee974);\\n \\n \\n\\n circle_marker_d10767c69c8e15bde82de7bc8c565469.bindPopup(popup_56de81eb48a9e79463bf931291ff69d6)\\n ;\\n\\n \\n \\n \\n var circle_marker_6584a90cde25d683dca4062fb471a814 = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_12bf999326d39ec9f1ab76bf01bd6e90 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0cc3ef704fedef9b61d12c14bbd31d61 = $(`<div id="html_0cc3ef704fedef9b61d12c14bbd31d61" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12bf999326d39ec9f1ab76bf01bd6e90.setContent(html_0cc3ef704fedef9b61d12c14bbd31d61);\\n \\n \\n\\n circle_marker_6584a90cde25d683dca4062fb471a814.bindPopup(popup_12bf999326d39ec9f1ab76bf01bd6e90)\\n ;\\n\\n \\n \\n \\n var circle_marker_c39f04235ea4eaca216f62a0e64a68c2 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_4a7c5a9c502108103b66914c65472203 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a57e46ca5dd9e8505ebf4482172086b = $(`<div id="html_4a57e46ca5dd9e8505ebf4482172086b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a7c5a9c502108103b66914c65472203.setContent(html_4a57e46ca5dd9e8505ebf4482172086b);\\n \\n \\n\\n circle_marker_c39f04235ea4eaca216f62a0e64a68c2.bindPopup(popup_4a7c5a9c502108103b66914c65472203)\\n ;\\n\\n \\n \\n \\n var circle_marker_711919161225f23b672e506038daa3b9 = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_e10767466360ba1732c04c7f48664607 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aca014b05e0640161c186b757d42ce53 = $(`<div id="html_aca014b05e0640161c186b757d42ce53" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e10767466360ba1732c04c7f48664607.setContent(html_aca014b05e0640161c186b757d42ce53);\\n \\n \\n\\n circle_marker_711919161225f23b672e506038daa3b9.bindPopup(popup_e10767466360ba1732c04c7f48664607)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a0f9cfac9d5d20ab837143dcbfaac54 = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_10236ca9eb8b3d107b4bb340720e8608 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_188e14390110950b7daf8400b6d78f79 = $(`<div id="html_188e14390110950b7daf8400b6d78f79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10236ca9eb8b3d107b4bb340720e8608.setContent(html_188e14390110950b7daf8400b6d78f79);\\n \\n \\n\\n circle_marker_3a0f9cfac9d5d20ab837143dcbfaac54.bindPopup(popup_10236ca9eb8b3d107b4bb340720e8608)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a4bf899dfc47cde101a2451f8aec7d3 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_eecfa00f30427db7f9176be7ec4cb098 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_972a5e85dd96d7fe7a228e13a06cdb09 = $(`<div id="html_972a5e85dd96d7fe7a228e13a06cdb09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eecfa00f30427db7f9176be7ec4cb098.setContent(html_972a5e85dd96d7fe7a228e13a06cdb09);\\n \\n \\n\\n circle_marker_8a4bf899dfc47cde101a2451f8aec7d3.bindPopup(popup_eecfa00f30427db7f9176be7ec4cb098)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a57ccf92f0fc6721f67fa63ddbd3ea3 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_3807612da6d49c8c481e3707c2d8432a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fce8c43940492cd140b858ace5c01278 = $(`<div id="html_fce8c43940492cd140b858ace5c01278" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3807612da6d49c8c481e3707c2d8432a.setContent(html_fce8c43940492cd140b858ace5c01278);\\n \\n \\n\\n circle_marker_0a57ccf92f0fc6721f67fa63ddbd3ea3.bindPopup(popup_3807612da6d49c8c481e3707c2d8432a)\\n ;\\n\\n \\n \\n \\n var circle_marker_34e523ef813c546dc00b14c9c08b36f3 = L.circleMarker(\\n [42.73274377588172, -73.25503076225394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_3b92faba58bdca1eb94ecf8b6b781dd4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42fcdf5cde40f220362f946fff77ecac = $(`<div id="html_42fcdf5cde40f220362f946fff77ecac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3b92faba58bdca1eb94ecf8b6b781dd4.setContent(html_42fcdf5cde40f220362f946fff77ecac);\\n \\n \\n\\n circle_marker_34e523ef813c546dc00b14c9c08b36f3.bindPopup(popup_3b92faba58bdca1eb94ecf8b6b781dd4)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c0f5011b7129874fd024140301e1479 = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_f149c9089381e0b5e2dd55f6b8717804 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d39c595d2765b661e8a84a9147ab6ae = $(`<div id="html_0d39c595d2765b661e8a84a9147ab6ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f149c9089381e0b5e2dd55f6b8717804.setContent(html_0d39c595d2765b661e8a84a9147ab6ae);\\n \\n \\n\\n circle_marker_5c0f5011b7129874fd024140301e1479.bindPopup(popup_f149c9089381e0b5e2dd55f6b8717804)\\n ;\\n\\n \\n \\n \\n var circle_marker_2091b57db6ff225f3f25f3794397a5c2 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_fe03e222e05a63d652695f3e7acbc4b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24c6d427e1eabaf481adfeb5235f889c = $(`<div id="html_24c6d427e1eabaf481adfeb5235f889c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe03e222e05a63d652695f3e7acbc4b4.setContent(html_24c6d427e1eabaf481adfeb5235f889c);\\n \\n \\n\\n circle_marker_2091b57db6ff225f3f25f3794397a5c2.bindPopup(popup_fe03e222e05a63d652695f3e7acbc4b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_e60cd0283f85e1980d7d02d507c16d24 = L.circleMarker(\\n [42.73093703750333, -73.26241163912951],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_34613c665f571169be941e316916540c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7fd5fb1be6ceaf5cae9e47617c8f0d47 = $(`<div id="html_7fd5fb1be6ceaf5cae9e47617c8f0d47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_34613c665f571169be941e316916540c.setContent(html_7fd5fb1be6ceaf5cae9e47617c8f0d47);\\n \\n \\n\\n circle_marker_e60cd0283f85e1980d7d02d507c16d24.bindPopup(popup_34613c665f571169be941e316916540c)\\n ;\\n\\n \\n \\n \\n var circle_marker_fbba186d3e4f05421839ff10aa0b598d = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_167d62a730f9ac4c720e5536f8d0b489 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76161a5f3083e62fc2c1c4231af0e924 = $(`<div id="html_76161a5f3083e62fc2c1c4231af0e924" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_167d62a730f9ac4c720e5536f8d0b489.setContent(html_76161a5f3083e62fc2c1c4231af0e924);\\n \\n \\n\\n circle_marker_fbba186d3e4f05421839ff10aa0b598d.bindPopup(popup_167d62a730f9ac4c720e5536f8d0b489)\\n ;\\n\\n \\n \\n \\n var circle_marker_5166bb80e539bf3ce9fa5471ec54b502 = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_1a426ad4a36def9d29c626f540926d17 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e724580e5270df463725bf8d938a34ba = $(`<div id="html_e724580e5270df463725bf8d938a34ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a426ad4a36def9d29c626f540926d17.setContent(html_e724580e5270df463725bf8d938a34ba);\\n \\n \\n\\n circle_marker_5166bb80e539bf3ce9fa5471ec54b502.bindPopup(popup_1a426ad4a36def9d29c626f540926d17)\\n ;\\n\\n \\n \\n \\n var circle_marker_f92d84727cfb6aa74a71719a0b7f1e07 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_2835247e8c6850aa41323c8fb9bbc04c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_23231af5979fb05e9f8b0839f367deb7 = $(`<div id="html_23231af5979fb05e9f8b0839f367deb7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2835247e8c6850aa41323c8fb9bbc04c.setContent(html_23231af5979fb05e9f8b0839f367deb7);\\n \\n \\n\\n circle_marker_f92d84727cfb6aa74a71719a0b7f1e07.bindPopup(popup_2835247e8c6850aa41323c8fb9bbc04c)\\n ;\\n\\n \\n \\n \\n var circle_marker_a144ee412ef6f12c79e89d8a99800142 = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_37de33db1faab74b0ee658a48d48ce79 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fee4c77322a60feba0381ddf919184c8 = $(`<div id="html_fee4c77322a60feba0381ddf919184c8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_37de33db1faab74b0ee658a48d48ce79.setContent(html_fee4c77322a60feba0381ddf919184c8);\\n \\n \\n\\n circle_marker_a144ee412ef6f12c79e89d8a99800142.bindPopup(popup_37de33db1faab74b0ee658a48d48ce79)\\n ;\\n\\n \\n \\n \\n var circle_marker_44526ab531d2cc27e1dbbdf4d2b98b33 = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_befa47c99e2261dc61314a806ecc2d4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a8a31958ce56b31350f668612d22a84 = $(`<div id="html_5a8a31958ce56b31350f668612d22a84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_befa47c99e2261dc61314a806ecc2d4c.setContent(html_5a8a31958ce56b31350f668612d22a84);\\n \\n \\n\\n circle_marker_44526ab531d2cc27e1dbbdf4d2b98b33.bindPopup(popup_befa47c99e2261dc61314a806ecc2d4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd272554fce41f3a6a2651bac81a00ea = L.circleMarker(\\n [42.72912939726166, -73.25380127326159],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_958e7aca8af8ce1e96b56c096a9fa15f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_52a394714b52a97f96860d5981cfae3d = $(`<div id="html_52a394714b52a97f96860d5981cfae3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_958e7aca8af8ce1e96b56c096a9fa15f.setContent(html_52a394714b52a97f96860d5981cfae3d);\\n \\n \\n\\n circle_marker_dd272554fce41f3a6a2651bac81a00ea.bindPopup(popup_958e7aca8af8ce1e96b56c096a9fa15f)\\n ;\\n\\n \\n \\n \\n var circle_marker_69e89964fc6961627725b3bfbcef0d5a = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_33854e4ca18bae25063819d616ed2ed4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4219cfdaa86f35be3863d3975093ebc8 = $(`<div id="html_4219cfdaa86f35be3863d3975093ebc8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33854e4ca18bae25063819d616ed2ed4.setContent(html_4219cfdaa86f35be3863d3975093ebc8);\\n \\n \\n\\n circle_marker_69e89964fc6961627725b3bfbcef0d5a.bindPopup(popup_33854e4ca18bae25063819d616ed2ed4)\\n ;\\n\\n \\n \\n \\n var circle_marker_bdc8a09c74330449a5a800613a363414 = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_612f40aff571f1169675258e58f4a712 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7144726831ed34b31108fdd159ea559d = $(`<div id="html_7144726831ed34b31108fdd159ea559d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_612f40aff571f1169675258e58f4a712.setContent(html_7144726831ed34b31108fdd159ea559d);\\n \\n \\n\\n circle_marker_bdc8a09c74330449a5a800613a363414.bindPopup(popup_612f40aff571f1169675258e58f4a712)\\n ;\\n\\n \\n \\n \\n var circle_marker_daaf3d7c83c9831d58f7b7b0dfdd4558 = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_cd906dcab305fb034258bf42d4d3fff3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb58401b5bd3cf6cfc5957c5bc127a65 = $(`<div id="html_cb58401b5bd3cf6cfc5957c5bc127a65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd906dcab305fb034258bf42d4d3fff3.setContent(html_cb58401b5bd3cf6cfc5957c5bc127a65);\\n \\n \\n\\n circle_marker_daaf3d7c83c9831d58f7b7b0dfdd4558.bindPopup(popup_cd906dcab305fb034258bf42d4d3fff3)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc3acb225f2050448cb56ddc8af6b49d = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_a3f8d22f9de97b0ed3284f9d8a67b74c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b1bfeb8565e13f884e8603ad8524cce = $(`<div id="html_0b1bfeb8565e13f884e8603ad8524cce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3f8d22f9de97b0ed3284f9d8a67b74c.setContent(html_0b1bfeb8565e13f884e8603ad8524cce);\\n \\n \\n\\n circle_marker_fc3acb225f2050448cb56ddc8af6b49d.bindPopup(popup_a3f8d22f9de97b0ed3284f9d8a67b74c)\\n ;\\n\\n \\n \\n \\n var circle_marker_78ced8fe597b1f98b63de2db502fb367 = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_80ae04e7536d5c307e2bebeb670ff1c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2d6d30736c912fcc3e81dab1a776f91 = $(`<div id="html_f2d6d30736c912fcc3e81dab1a776f91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80ae04e7536d5c307e2bebeb670ff1c0.setContent(html_f2d6d30736c912fcc3e81dab1a776f91);\\n \\n \\n\\n circle_marker_78ced8fe597b1f98b63de2db502fb367.bindPopup(popup_80ae04e7536d5c307e2bebeb670ff1c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f3dd321bfe46bbf650855f830eee5c1 = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_b631f1b58abefd9768230097db47cb38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57fd56109fd49b58ff662a1080b552a5 = $(`<div id="html_57fd56109fd49b58ff662a1080b552a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b631f1b58abefd9768230097db47cb38.setContent(html_57fd56109fd49b58ff662a1080b552a5);\\n \\n \\n\\n circle_marker_3f3dd321bfe46bbf650855f830eee5c1.bindPopup(popup_b631f1b58abefd9768230097db47cb38)\\n ;\\n\\n \\n \\n \\n var circle_marker_f391ab33eccc6fec42f9e0e1b2329d87 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_d37d80d26916c8c3822bf29dfcc261aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e21df8a0dadc91dfafe881d8ef6c2d40 = $(`<div id="html_e21df8a0dadc91dfafe881d8ef6c2d40" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d37d80d26916c8c3822bf29dfcc261aa.setContent(html_e21df8a0dadc91dfafe881d8ef6c2d40);\\n \\n \\n\\n circle_marker_f391ab33eccc6fec42f9e0e1b2329d87.bindPopup(popup_d37d80d26916c8c3822bf29dfcc261aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_39abf5fb9ddffb3545b6a64937425299 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_8d42e8df6ccb9486957b1a6485eed7f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5927fea00ab4554762b0edc2abefc908 = $(`<div id="html_5927fea00ab4554762b0edc2abefc908" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d42e8df6ccb9486957b1a6485eed7f6.setContent(html_5927fea00ab4554762b0edc2abefc908);\\n \\n \\n\\n circle_marker_39abf5fb9ddffb3545b6a64937425299.bindPopup(popup_8d42e8df6ccb9486957b1a6485eed7f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_370675cfd9b23e8b8e0830acccb32240 = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aaaa0947dc784648579c7f620e1d3393);\\n \\n \\n var popup_a063533c137f7d78794573e6eace1eae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96db9afaa9b1704e560c25d220c16dc2 = $(`<div id="html_96db9afaa9b1704e560c25d220c16dc2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a063533c137f7d78794573e6eace1eae.setContent(html_96db9afaa9b1704e560c25d220c16dc2);\\n \\n \\n\\n circle_marker_370675cfd9b23e8b8e0830acccb32240.bindPopup(popup_a063533c137f7d78794573e6eace1eae)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Apple": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_120983a05ef6714ef6c9815a64fb9405 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_120983a05ef6714ef6c9815a64fb9405" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_120983a05ef6714ef6c9815a64fb9405 = L.map(\\n "map_120983a05ef6714ef6c9815a64fb9405",\\n {\\n center: [42.73454782162162, -73.23227129205617],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_e2be1fcb4332393e8c229af137d97269 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var circle_marker_d117944774bda8e8a1bdb5fd02f510f2 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_b792aef0825e51f8a33d46fbb1878eef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe51ebc4c9a3b7732c4f57ffbc2873e2 = $(`<div id="html_fe51ebc4c9a3b7732c4f57ffbc2873e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b792aef0825e51f8a33d46fbb1878eef.setContent(html_fe51ebc4c9a3b7732c4f57ffbc2873e2);\\n \\n \\n\\n circle_marker_d117944774bda8e8a1bdb5fd02f510f2.bindPopup(popup_b792aef0825e51f8a33d46fbb1878eef)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf012885cb3d4bcb35c98753ed9d4341 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_0747bac93b9284dc009b548c6b8e85e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2effcd4e0963e2b61b0d8416c0249067 = $(`<div id="html_2effcd4e0963e2b61b0d8416c0249067" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0747bac93b9284dc009b548c6b8e85e7.setContent(html_2effcd4e0963e2b61b0d8416c0249067);\\n \\n \\n\\n circle_marker_bf012885cb3d4bcb35c98753ed9d4341.bindPopup(popup_0747bac93b9284dc009b548c6b8e85e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_f9c242991e06d8b47d1becd5f1eb2cd0 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_0a1678165c7fbb99d904568c342e7804 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1fe271c2ff2b1417b14990463a45360b = $(`<div id="html_1fe271c2ff2b1417b14990463a45360b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a1678165c7fbb99d904568c342e7804.setContent(html_1fe271c2ff2b1417b14990463a45360b);\\n \\n \\n\\n circle_marker_f9c242991e06d8b47d1becd5f1eb2cd0.bindPopup(popup_0a1678165c7fbb99d904568c342e7804)\\n ;\\n\\n \\n \\n \\n var circle_marker_0f05230deb90ecc5b3f0bf18c90e5096 = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_ed63bd7dd93c3cf6a2b11ebf5337bfd6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fbf5a8464953037ed2b783dff1bcd9f0 = $(`<div id="html_fbf5a8464953037ed2b783dff1bcd9f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed63bd7dd93c3cf6a2b11ebf5337bfd6.setContent(html_fbf5a8464953037ed2b783dff1bcd9f0);\\n \\n \\n\\n circle_marker_0f05230deb90ecc5b3f0bf18c90e5096.bindPopup(popup_ed63bd7dd93c3cf6a2b11ebf5337bfd6)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6c71ce605fb9287d493399459d4ce0b = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_baa1af5073bc3f3767a06ef838a6cf51 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fdaec7bce70584d0f74034526e46524e = $(`<div id="html_fdaec7bce70584d0f74034526e46524e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_baa1af5073bc3f3767a06ef838a6cf51.setContent(html_fdaec7bce70584d0f74034526e46524e);\\n \\n \\n\\n circle_marker_d6c71ce605fb9287d493399459d4ce0b.bindPopup(popup_baa1af5073bc3f3767a06ef838a6cf51)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a1a87f92874f203c2de518fc121f808 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_6fd7e1fbcb14ddede4176ef64b7e05ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f653cfbf1e3321c8102b8bbd7069cd0 = $(`<div id="html_7f653cfbf1e3321c8102b8bbd7069cd0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6fd7e1fbcb14ddede4176ef64b7e05ff.setContent(html_7f653cfbf1e3321c8102b8bbd7069cd0);\\n \\n \\n\\n circle_marker_3a1a87f92874f203c2de518fc121f808.bindPopup(popup_6fd7e1fbcb14ddede4176ef64b7e05ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_09fac70c70f093ad4e09b892736cde50 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_dddbe53d1e640f6ce5b38b4a392e0911 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_89abf2854a0f38d384e8f7701bc2345c = $(`<div id="html_89abf2854a0f38d384e8f7701bc2345c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dddbe53d1e640f6ce5b38b4a392e0911.setContent(html_89abf2854a0f38d384e8f7701bc2345c);\\n \\n \\n\\n circle_marker_09fac70c70f093ad4e09b892736cde50.bindPopup(popup_dddbe53d1e640f6ce5b38b4a392e0911)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e1709337f72d81147ee6b16d5ca8403 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_8da754c2e35695a16c4094e8f18fc819 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c43521f17625e12219d2cbf46e615dd7 = $(`<div id="html_c43521f17625e12219d2cbf46e615dd7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8da754c2e35695a16c4094e8f18fc819.setContent(html_c43521f17625e12219d2cbf46e615dd7);\\n \\n \\n\\n circle_marker_1e1709337f72d81147ee6b16d5ca8403.bindPopup(popup_8da754c2e35695a16c4094e8f18fc819)\\n ;\\n\\n \\n \\n \\n var circle_marker_43361ced4682e2ec45ef636c098b07f9 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_8e59f2d14274716db7af4b7cefbc4f9a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d96150655934576e748e80b00323063 = $(`<div id="html_3d96150655934576e748e80b00323063" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e59f2d14274716db7af4b7cefbc4f9a.setContent(html_3d96150655934576e748e80b00323063);\\n \\n \\n\\n circle_marker_43361ced4682e2ec45ef636c098b07f9.bindPopup(popup_8e59f2d14274716db7af4b7cefbc4f9a)\\n ;\\n\\n \\n \\n \\n var circle_marker_1894ef99a8a8a0e856495d70157164c5 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_e0b32c9c9f02610956b1d552cdcd3248 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_347c7089376ebb6ff75824de9adbfe0e = $(`<div id="html_347c7089376ebb6ff75824de9adbfe0e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0b32c9c9f02610956b1d552cdcd3248.setContent(html_347c7089376ebb6ff75824de9adbfe0e);\\n \\n \\n\\n circle_marker_1894ef99a8a8a0e856495d70157164c5.bindPopup(popup_e0b32c9c9f02610956b1d552cdcd3248)\\n ;\\n\\n \\n \\n \\n var circle_marker_270b01313bac2bd64de25fce8a0b2a80 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_120983a05ef6714ef6c9815a64fb9405);\\n \\n \\n var popup_3fb8c7817a65972197f6fa6ff02bc1f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e772a6b180b85368b6cfed8b574cd38a = $(`<div id="html_e772a6b180b85368b6cfed8b574cd38a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3fb8c7817a65972197f6fa6ff02bc1f9.setContent(html_e772a6b180b85368b6cfed8b574cd38a);\\n \\n \\n\\n circle_marker_270b01313bac2bd64de25fce8a0b2a80.bindPopup(popup_3fb8c7817a65972197f6fa6ff02bc1f9)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Arrow-wood": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_e53373adaee2e9dc2b168d302c1524de {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_e53373adaee2e9dc2b168d302c1524de" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_e53373adaee2e9dc2b168d302c1524de = L.map(\\n "map_e53373adaee2e9dc2b168d302c1524de",\\n {\\n center: [42.73454784790659, -73.23657792568366],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_eb97db58fea1725badd07c2ca9a982bc = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_e53373adaee2e9dc2b168d302c1524de);\\n \\n \\n var circle_marker_4067a61bcc8584d4868bf1d71919ef21 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_e53373adaee2e9dc2b168d302c1524de);\\n \\n \\n var popup_432a66cfc3fb812db193f34efb6035bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_391da33d04a3ebf650044e56df0247ec = $(`<div id="html_391da33d04a3ebf650044e56df0247ec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_432a66cfc3fb812db193f34efb6035bc.setContent(html_391da33d04a3ebf650044e56df0247ec);\\n \\n \\n\\n circle_marker_4067a61bcc8584d4868bf1d71919ef21.bindPopup(popup_432a66cfc3fb812db193f34efb6035bc)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Ash, American mountain\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_4f129ce3fdd3f01d84769ed16216adc8 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_4f129ce3fdd3f01d84769ed16216adc8" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_4f129ce3fdd3f01d84769ed16216adc8 = L.map(\\n "map_4f129ce3fdd3f01d84769ed16216adc8",\\n {\\n center: [42.73635790439397, -73.27409676708663],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_7889eceec46d09bd67bc2e8f02a2fc54 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_4f129ce3fdd3f01d84769ed16216adc8);\\n \\n \\n var circle_marker_19bde5ab9f729dfa8723988bc6c9aff5 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4f129ce3fdd3f01d84769ed16216adc8);\\n \\n \\n var popup_92a9f1a7858eb26b2c5c072df0583836 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_760069b07d3c2143749d4353748a4a18 = $(`<div id="html_760069b07d3c2143749d4353748a4a18" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_92a9f1a7858eb26b2c5c072df0583836.setContent(html_760069b07d3c2143749d4353748a4a18);\\n \\n \\n\\n circle_marker_19bde5ab9f729dfa8723988bc6c9aff5.bindPopup(popup_92a9f1a7858eb26b2c5c072df0583836)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6dbce4c1ecc157fc65a7b8dbbcf9b7b = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4f129ce3fdd3f01d84769ed16216adc8);\\n \\n \\n var popup_9b2ec6199879115018deb15d16d07407 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3777107b5870884902f34d903bf708f = $(`<div id="html_f3777107b5870884902f34d903bf708f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b2ec6199879115018deb15d16d07407.setContent(html_f3777107b5870884902f34d903bf708f);\\n \\n \\n\\n circle_marker_f6dbce4c1ecc157fc65a7b8dbbcf9b7b.bindPopup(popup_9b2ec6199879115018deb15d16d07407)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0c16a3569f166c686e306198a2ab8a7 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_4f129ce3fdd3f01d84769ed16216adc8);\\n \\n \\n var popup_3344d6eaa459d7571dcbed6c05ec9aa2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ee2ec28f987fd395ff9adcf49bd6da81 = $(`<div id="html_ee2ec28f987fd395ff9adcf49bd6da81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3344d6eaa459d7571dcbed6c05ec9aa2.setContent(html_ee2ec28f987fd395ff9adcf49bd6da81);\\n \\n \\n\\n circle_marker_b0c16a3569f166c686e306198a2ab8a7.bindPopup(popup_3344d6eaa459d7571dcbed6c05ec9aa2)\\n ;\\n\\n \\n \\n \\n var circle_marker_13143912c322294a8d3c23806254dad0 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4f129ce3fdd3f01d84769ed16216adc8);\\n \\n \\n var popup_2c6ab837202e0c233182739d141ae7c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6564f51377933a9bcc7d57541e54a202 = $(`<div id="html_6564f51377933a9bcc7d57541e54a202" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c6ab837202e0c233182739d141ae7c1.setContent(html_6564f51377933a9bcc7d57541e54a202);\\n \\n \\n\\n circle_marker_13143912c322294a8d3c23806254dad0.bindPopup(popup_2c6ab837202e0c233182739d141ae7c1)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Ash, white\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_aeb4c47ecdf1032f270fe2adc8c0e511 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_aeb4c47ecdf1032f270fe2adc8c0e511" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_aeb4c47ecdf1032f270fe2adc8c0e511 = L.map(\\n "map_aeb4c47ecdf1032f270fe2adc8c0e511",\\n {\\n center: [42.73545173466282, -73.24764986998687],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_5f75186deafcc97a8541b7f4220bfb28 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var circle_marker_5459906434a7dd37e554a115bafbc9d5 = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_1330b2737a2b64f13d794f4af122ab27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b55712b450e372688a5f8d2f197a409d = $(`<div id="html_b55712b450e372688a5f8d2f197a409d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1330b2737a2b64f13d794f4af122ab27.setContent(html_b55712b450e372688a5f8d2f197a409d);\\n \\n \\n\\n circle_marker_5459906434a7dd37e554a115bafbc9d5.bindPopup(popup_1330b2737a2b64f13d794f4af122ab27)\\n ;\\n\\n \\n \\n \\n var circle_marker_59f7b9bbc45fa4301f0e0eddda87ff05 = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ee184d3099e6e71b634e228c8663cc1c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_473721d12ef5f4206da4178053fa55c0 = $(`<div id="html_473721d12ef5f4206da4178053fa55c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee184d3099e6e71b634e228c8663cc1c.setContent(html_473721d12ef5f4206da4178053fa55c0);\\n \\n \\n\\n circle_marker_59f7b9bbc45fa4301f0e0eddda87ff05.bindPopup(popup_ee184d3099e6e71b634e228c8663cc1c)\\n ;\\n\\n \\n \\n \\n var circle_marker_60cf1675340c08b74236a6d89b624a0f = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_9fa09c923a3016a41e17dea6d0144516 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_051efc35d1cb7b8a5e840207fa0fa93b = $(`<div id="html_051efc35d1cb7b8a5e840207fa0fa93b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fa09c923a3016a41e17dea6d0144516.setContent(html_051efc35d1cb7b8a5e840207fa0fa93b);\\n \\n \\n\\n circle_marker_60cf1675340c08b74236a6d89b624a0f.bindPopup(popup_9fa09c923a3016a41e17dea6d0144516)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d4044db9d9cd10cf83e0a8608668949 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_faa2bbd1f387957d179a5b1fe6ffdc1c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65ca9f69da72633b02b3d2099f01eb79 = $(`<div id="html_65ca9f69da72633b02b3d2099f01eb79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_faa2bbd1f387957d179a5b1fe6ffdc1c.setContent(html_65ca9f69da72633b02b3d2099f01eb79);\\n \\n \\n\\n circle_marker_8d4044db9d9cd10cf83e0a8608668949.bindPopup(popup_faa2bbd1f387957d179a5b1fe6ffdc1c)\\n ;\\n\\n \\n \\n \\n var circle_marker_d7fba261d1e001df3c324346313ab350 = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6f96a2eaa2160d8b03285c9c289ba533 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6aaae6650d101466c7c4ad1a6e17ed7 = $(`<div id="html_c6aaae6650d101466c7c4ad1a6e17ed7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f96a2eaa2160d8b03285c9c289ba533.setContent(html_c6aaae6650d101466c7c4ad1a6e17ed7);\\n \\n \\n\\n circle_marker_d7fba261d1e001df3c324346313ab350.bindPopup(popup_6f96a2eaa2160d8b03285c9c289ba533)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5906b073d8d6adf90bdb519b5707ef1 = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_195a161ba92c72db828beb412d0bf9cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a0c4177c6f60f0ed6c70b5083ce98b9 = $(`<div id="html_3a0c4177c6f60f0ed6c70b5083ce98b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_195a161ba92c72db828beb412d0bf9cc.setContent(html_3a0c4177c6f60f0ed6c70b5083ce98b9);\\n \\n \\n\\n circle_marker_b5906b073d8d6adf90bdb519b5707ef1.bindPopup(popup_195a161ba92c72db828beb412d0bf9cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_260ea0251193a140363083242d701f6d = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_04b4b1089e56d82001dffa22adf031e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1289dbfba8806fd40c13929a64102881 = $(`<div id="html_1289dbfba8806fd40c13929a64102881" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04b4b1089e56d82001dffa22adf031e8.setContent(html_1289dbfba8806fd40c13929a64102881);\\n \\n \\n\\n circle_marker_260ea0251193a140363083242d701f6d.bindPopup(popup_04b4b1089e56d82001dffa22adf031e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_63f48d4e2e7fe035491da5ceceb186e9 = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_9010e49b51f2d3b80dd7bb9bcbddd2ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f232ab39ad964268f90df1936b0cc91f = $(`<div id="html_f232ab39ad964268f90df1936b0cc91f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9010e49b51f2d3b80dd7bb9bcbddd2ac.setContent(html_f232ab39ad964268f90df1936b0cc91f);\\n \\n \\n\\n circle_marker_63f48d4e2e7fe035491da5ceceb186e9.bindPopup(popup_9010e49b51f2d3b80dd7bb9bcbddd2ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_9914be4b5d1f55a0daff6b6a4da7d3a1 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3f1082ed200c54cc5aa9806314051687 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_efc7c8b10a21189c727f33b14e9e682e = $(`<div id="html_efc7c8b10a21189c727f33b14e9e682e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3f1082ed200c54cc5aa9806314051687.setContent(html_efc7c8b10a21189c727f33b14e9e682e);\\n \\n \\n\\n circle_marker_9914be4b5d1f55a0daff6b6a4da7d3a1.bindPopup(popup_3f1082ed200c54cc5aa9806314051687)\\n ;\\n\\n \\n \\n \\n var circle_marker_0576b27bed75820bba09762802fcdc54 = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_1e2e1ea41e60b1793e2485e7e832f8ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1df0a606eae320fb0341cd00a569385f = $(`<div id="html_1df0a606eae320fb0341cd00a569385f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1e2e1ea41e60b1793e2485e7e832f8ec.setContent(html_1df0a606eae320fb0341cd00a569385f);\\n \\n \\n\\n circle_marker_0576b27bed75820bba09762802fcdc54.bindPopup(popup_1e2e1ea41e60b1793e2485e7e832f8ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_f66be6967a2fdefa63d8c60ce4e14879 = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_691e9dd6a8cd5ccdffc3b446a20486a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e62f8298687f912b9bf8f38245b6a92 = $(`<div id="html_4e62f8298687f912b9bf8f38245b6a92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_691e9dd6a8cd5ccdffc3b446a20486a8.setContent(html_4e62f8298687f912b9bf8f38245b6a92);\\n \\n \\n\\n circle_marker_f66be6967a2fdefa63d8c60ce4e14879.bindPopup(popup_691e9dd6a8cd5ccdffc3b446a20486a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4dd6e6ec7861b57f721f6735dacf98a = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6e3344752390f0129b4b79613da484e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8640298edada0fa7a2549d572fb0191e = $(`<div id="html_8640298edada0fa7a2549d572fb0191e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e3344752390f0129b4b79613da484e8.setContent(html_8640298edada0fa7a2549d572fb0191e);\\n \\n \\n\\n circle_marker_a4dd6e6ec7861b57f721f6735dacf98a.bindPopup(popup_6e3344752390f0129b4b79613da484e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_7dc5b1b435eaa911d1341df33982b7a5 = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6bf68c4c89561f9a56b4604a91eb8659 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1223294ea5eefafc4d33bf2d9d4ca3b = $(`<div id="html_d1223294ea5eefafc4d33bf2d9d4ca3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6bf68c4c89561f9a56b4604a91eb8659.setContent(html_d1223294ea5eefafc4d33bf2d9d4ca3b);\\n \\n \\n\\n circle_marker_7dc5b1b435eaa911d1341df33982b7a5.bindPopup(popup_6bf68c4c89561f9a56b4604a91eb8659)\\n ;\\n\\n \\n \\n \\n var circle_marker_d7cdb632213761e41751da9ecfcabd0d = L.circleMarker(\\n [42.74358697111428, -73.26733253452443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f7048e17bd126372c2a222c234cecafe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d67c7601c305d88ca8b95d2a92949c69 = $(`<div id="html_d67c7601c305d88ca8b95d2a92949c69" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7048e17bd126372c2a222c234cecafe.setContent(html_d67c7601c305d88ca8b95d2a92949c69);\\n \\n \\n\\n circle_marker_d7cdb632213761e41751da9ecfcabd0d.bindPopup(popup_f7048e17bd126372c2a222c234cecafe)\\n ;\\n\\n \\n \\n \\n var circle_marker_a92a0ea1f0b97fb66d4753bd188d051b = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f6dbe638b276a34bd4e104c5e028f5ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d12df12272738a734e77f4108acf3b19 = $(`<div id="html_d12df12272738a734e77f4108acf3b19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6dbe638b276a34bd4e104c5e028f5ee.setContent(html_d12df12272738a734e77f4108acf3b19);\\n \\n \\n\\n circle_marker_a92a0ea1f0b97fb66d4753bd188d051b.bindPopup(popup_f6dbe638b276a34bd4e104c5e028f5ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b3747f0b770a17a64030ec7862d4ffe = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ed5b593831d77b8fe006c95e96ed1096 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3953357c8544306df52fdc0772cbbcf = $(`<div id="html_a3953357c8544306df52fdc0772cbbcf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed5b593831d77b8fe006c95e96ed1096.setContent(html_a3953357c8544306df52fdc0772cbbcf);\\n \\n \\n\\n circle_marker_9b3747f0b770a17a64030ec7862d4ffe.bindPopup(popup_ed5b593831d77b8fe006c95e96ed1096)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a85fad4a9b1d8b24488ade78c9e99fd = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3a6fd6602de65e7f4759889857a0a4c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13f02246704374c578a3c7e11d6b78d8 = $(`<div id="html_13f02246704374c578a3c7e11d6b78d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a6fd6602de65e7f4759889857a0a4c5.setContent(html_13f02246704374c578a3c7e11d6b78d8);\\n \\n \\n\\n circle_marker_1a85fad4a9b1d8b24488ade78c9e99fd.bindPopup(popup_3a6fd6602de65e7f4759889857a0a4c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_226d6d2e5ff6ab5323aaf7ed263df9f5 = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6470fb1ab604ea114838905f3a0c7623 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_106d0daaca7e1c1d388236edb5ea4839 = $(`<div id="html_106d0daaca7e1c1d388236edb5ea4839" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6470fb1ab604ea114838905f3a0c7623.setContent(html_106d0daaca7e1c1d388236edb5ea4839);\\n \\n \\n\\n circle_marker_226d6d2e5ff6ab5323aaf7ed263df9f5.bindPopup(popup_6470fb1ab604ea114838905f3a0c7623)\\n ;\\n\\n \\n \\n \\n var circle_marker_e723bb89f166adc0bc8adbe43da8e7ce = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_849587c2a741f51f49670906f051ea16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c31cd62fe5973e0125a0340c43907e26 = $(`<div id="html_c31cd62fe5973e0125a0340c43907e26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_849587c2a741f51f49670906f051ea16.setContent(html_c31cd62fe5973e0125a0340c43907e26);\\n \\n \\n\\n circle_marker_e723bb89f166adc0bc8adbe43da8e7ce.bindPopup(popup_849587c2a741f51f49670906f051ea16)\\n ;\\n\\n \\n \\n \\n var circle_marker_14e77295482697429a39ab232e6176c9 = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cc625292041f59ef244a14c84e60330f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d54ede0cbf8995edb17ebd49bcb7255 = $(`<div id="html_6d54ede0cbf8995edb17ebd49bcb7255" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc625292041f59ef244a14c84e60330f.setContent(html_6d54ede0cbf8995edb17ebd49bcb7255);\\n \\n \\n\\n circle_marker_14e77295482697429a39ab232e6176c9.bindPopup(popup_cc625292041f59ef244a14c84e60330f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1fa6330256808a593b0b046680b64d08 = L.circleMarker(\\n [42.7417798048184, -73.2611808954216],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_2c0229fc9bc568a50f1df1adf41d4464 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a084cd6f495f04f6f26ec8db1be94b18 = $(`<div id="html_a084cd6f495f04f6f26ec8db1be94b18" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c0229fc9bc568a50f1df1adf41d4464.setContent(html_a084cd6f495f04f6f26ec8db1be94b18);\\n \\n \\n\\n circle_marker_1fa6330256808a593b0b046680b64d08.bindPopup(popup_2c0229fc9bc568a50f1df1adf41d4464)\\n ;\\n\\n \\n \\n \\n var circle_marker_7bb2b3496c50bcd134aae6a375853656 = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_93347455ce3e1222d37da832055b606a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3cb231e01870ed86ba9a61b5467757e9 = $(`<div id="html_3cb231e01870ed86ba9a61b5467757e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93347455ce3e1222d37da832055b606a.setContent(html_3cb231e01870ed86ba9a61b5467757e9);\\n \\n \\n\\n circle_marker_7bb2b3496c50bcd134aae6a375853656.bindPopup(popup_93347455ce3e1222d37da832055b606a)\\n ;\\n\\n \\n \\n \\n var circle_marker_3da4d92b9b43626e9785ee75ac248efc = L.circleMarker(\\n [42.74177969946516, -73.25872026847817],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c0d7703d338df2ffe2b0f3cd87ca6f9f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_822e23edb0331d3d1f73157fe36a3e41 = $(`<div id="html_822e23edb0331d3d1f73157fe36a3e41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0d7703d338df2ffe2b0f3cd87ca6f9f.setContent(html_822e23edb0331d3d1f73157fe36a3e41);\\n \\n \\n\\n circle_marker_3da4d92b9b43626e9785ee75ac248efc.bindPopup(popup_c0d7703d338df2ffe2b0f3cd87ca6f9f)\\n ;\\n\\n \\n \\n \\n var circle_marker_62edf9aab0e565e44a9a0fef84767a8f = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_024756ea6dbdf8424afbb2923a7896b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a6f3aac2a03422ee955378e210fabdb = $(`<div id="html_4a6f3aac2a03422ee955378e210fabdb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_024756ea6dbdf8424afbb2923a7896b4.setContent(html_4a6f3aac2a03422ee955378e210fabdb);\\n \\n \\n\\n circle_marker_62edf9aab0e565e44a9a0fef84767a8f.bindPopup(popup_024756ea6dbdf8424afbb2923a7896b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_b84ea0f090593b16723f19401dd0584c = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_746a0914bd56bac76538fa31d13c48c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_231b0364e74e9c391c7fa24380c1109e = $(`<div id="html_231b0364e74e9c391c7fa24380c1109e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_746a0914bd56bac76538fa31d13c48c7.setContent(html_231b0364e74e9c391c7fa24380c1109e);\\n \\n \\n\\n circle_marker_b84ea0f090593b16723f19401dd0584c.bindPopup(popup_746a0914bd56bac76538fa31d13c48c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_c63b2fcdafb3d76cb40e30d1bd9c7704 = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_0a3514670ed8d641701817cd68e9e66e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_72c4758d0085d35a0ff3768cb950db13 = $(`<div id="html_72c4758d0085d35a0ff3768cb950db13" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a3514670ed8d641701817cd68e9e66e.setContent(html_72c4758d0085d35a0ff3768cb950db13);\\n \\n \\n\\n circle_marker_c63b2fcdafb3d76cb40e30d1bd9c7704.bindPopup(popup_0a3514670ed8d641701817cd68e9e66e)\\n ;\\n\\n \\n \\n \\n var circle_marker_88745eebc81f9c401b3361df49b5e54e = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_19b40cc5187bc064b444213420970b69 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67fc02d48737f20edde5e4e8b94bb103 = $(`<div id="html_67fc02d48737f20edde5e4e8b94bb103" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19b40cc5187bc064b444213420970b69.setContent(html_67fc02d48737f20edde5e4e8b94bb103);\\n \\n \\n\\n circle_marker_88745eebc81f9c401b3361df49b5e54e.bindPopup(popup_19b40cc5187bc064b444213420970b69)\\n ;\\n\\n \\n \\n \\n var circle_marker_f638bd1284f7b70514f3b610f943f7e7 = L.circleMarker(\\n [42.73997267144673, -73.26856266869262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_70a3ac3c4e6eb8be6b2747bbb4bcb956 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8a04f266353fcbc5633e9972dc933ea = $(`<div id="html_c8a04f266353fcbc5633e9972dc933ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_70a3ac3c4e6eb8be6b2747bbb4bcb956.setContent(html_c8a04f266353fcbc5633e9972dc933ea);\\n \\n \\n\\n circle_marker_f638bd1284f7b70514f3b610f943f7e7.bindPopup(popup_70a3ac3c4e6eb8be6b2747bbb4bcb956)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f817cf10ee936484e675edf5778df3e = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a3553e89fbaba74507cb9284e41e9f94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7cde0e66efab8202f46c8f42201d2efb = $(`<div id="html_7cde0e66efab8202f46c8f42201d2efb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3553e89fbaba74507cb9284e41e9f94.setContent(html_7cde0e66efab8202f46c8f42201d2efb);\\n \\n \\n\\n circle_marker_3f817cf10ee936484e675edf5778df3e.bindPopup(popup_a3553e89fbaba74507cb9284e41e9f94)\\n ;\\n\\n \\n \\n \\n var circle_marker_e61136a2b0ef17cd80beddaded0157f2 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d79ab32ca5808ffc88c4f7b7ab59e810 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_744ebc512bb9328231de6d056d76356c = $(`<div id="html_744ebc512bb9328231de6d056d76356c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d79ab32ca5808ffc88c4f7b7ab59e810.setContent(html_744ebc512bb9328231de6d056d76356c);\\n \\n \\n\\n circle_marker_e61136a2b0ef17cd80beddaded0157f2.bindPopup(popup_d79ab32ca5808ffc88c4f7b7ab59e810)\\n ;\\n\\n \\n \\n \\n var circle_marker_fce99c2414ec11354a99da4e7feb1928 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3e497ef5e25117d287ac6a9db9322f2c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a606c811510328bcc2207786a7cfc2c1 = $(`<div id="html_a606c811510328bcc2207786a7cfc2c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e497ef5e25117d287ac6a9db9322f2c.setContent(html_a606c811510328bcc2207786a7cfc2c1);\\n \\n \\n\\n circle_marker_fce99c2414ec11354a99da4e7feb1928.bindPopup(popup_3e497ef5e25117d287ac6a9db9322f2c)\\n ;\\n\\n \\n \\n \\n var circle_marker_90a2b5fd99fa14c28f18c86b239af55a = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ee4e6350afb2d977c35e86e5b9526e45 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5e62d5113ec6444ba844889db9ee15ad = $(`<div id="html_5e62d5113ec6444ba844889db9ee15ad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee4e6350afb2d977c35e86e5b9526e45.setContent(html_5e62d5113ec6444ba844889db9ee15ad);\\n \\n \\n\\n circle_marker_90a2b5fd99fa14c28f18c86b239af55a.bindPopup(popup_ee4e6350afb2d977c35e86e5b9526e45)\\n ;\\n\\n \\n \\n \\n var circle_marker_d3a097c775e5e358215fe4ff46c701cb = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3635d3994820ed667c23a442899ce70d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_71c523dc35f7c6ddfc019b49fac25899 = $(`<div id="html_71c523dc35f7c6ddfc019b49fac25899" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3635d3994820ed667c23a442899ce70d.setContent(html_71c523dc35f7c6ddfc019b49fac25899);\\n \\n \\n\\n circle_marker_d3a097c775e5e358215fe4ff46c701cb.bindPopup(popup_3635d3994820ed667c23a442899ce70d)\\n ;\\n\\n \\n \\n \\n var circle_marker_7b17a0e6ed448d27a69d5242d5faf820 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6e334cc755609076557614b3a036ed47 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4b9e0ca01af55540622e65158f00432 = $(`<div id="html_a4b9e0ca01af55540622e65158f00432" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e334cc755609076557614b3a036ed47.setContent(html_a4b9e0ca01af55540622e65158f00432);\\n \\n \\n\\n circle_marker_7b17a0e6ed448d27a69d5242d5faf820.bindPopup(popup_6e334cc755609076557614b3a036ed47)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec307d2a0bb6a7a11b188ac3dd6271d7 = L.circleMarker(\\n [42.73996598837205, -73.22550295499178],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_b77c9a738c221531726b05b5d9acde71 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2e09223f3344efdf19e66053fdf35e8 = $(`<div id="html_f2e09223f3344efdf19e66053fdf35e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b77c9a738c221531726b05b5d9acde71.setContent(html_f2e09223f3344efdf19e66053fdf35e8);\\n \\n \\n\\n circle_marker_ec307d2a0bb6a7a11b188ac3dd6271d7.bindPopup(popup_b77c9a738c221531726b05b5d9acde71)\\n ;\\n\\n \\n \\n \\n var circle_marker_34cb9a21327707f409e6ded3fbe9ddff = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_308801705be2122f375280780b7be0f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_860c898a0393940590361faf596e323a = $(`<div id="html_860c898a0393940590361faf596e323a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_308801705be2122f375280780b7be0f3.setContent(html_860c898a0393940590361faf596e323a);\\n \\n \\n\\n circle_marker_34cb9a21327707f409e6ded3fbe9ddff.bindPopup(popup_308801705be2122f375280780b7be0f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_62c5ad5252cac1a9ad057b8d4ed2cb4b = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_47ab9a30a366b2c58db1fccec4487956 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8b9c1aae1f48937a1a5dd65645680eb = $(`<div id="html_d8b9c1aae1f48937a1a5dd65645680eb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47ab9a30a366b2c58db1fccec4487956.setContent(html_d8b9c1aae1f48937a1a5dd65645680eb);\\n \\n \\n\\n circle_marker_62c5ad5252cac1a9ad057b8d4ed2cb4b.bindPopup(popup_47ab9a30a366b2c58db1fccec4487956)\\n ;\\n\\n \\n \\n \\n var circle_marker_f493b489dfa87ce743f91d7d96730f55 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a769fc95ab7b31d0093a1482267e08d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7db78c5e3e441517cf484c726c4c33bb = $(`<div id="html_7db78c5e3e441517cf484c726c4c33bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a769fc95ab7b31d0093a1482267e08d5.setContent(html_7db78c5e3e441517cf484c726c4c33bb);\\n \\n \\n\\n circle_marker_f493b489dfa87ce743f91d7d96730f55.bindPopup(popup_a769fc95ab7b31d0093a1482267e08d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_005cc5df52a295e3c2775c403d8400d7 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_67144b7412d58b2ef18f9bc13015ea6b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf76747deb766519b41904acb9b44cf7 = $(`<div id="html_bf76747deb766519b41904acb9b44cf7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_67144b7412d58b2ef18f9bc13015ea6b.setContent(html_bf76747deb766519b41904acb9b44cf7);\\n \\n \\n\\n circle_marker_005cc5df52a295e3c2775c403d8400d7.bindPopup(popup_67144b7412d58b2ef18f9bc13015ea6b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9c8046af924019ea42ef01cf482d9bfe = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_927d9d63c552232de893a54c827aabe9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e4e251f73c57dc2848daf9e103374197 = $(`<div id="html_e4e251f73c57dc2848daf9e103374197" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_927d9d63c552232de893a54c827aabe9.setContent(html_e4e251f73c57dc2848daf9e103374197);\\n \\n \\n\\n circle_marker_9c8046af924019ea42ef01cf482d9bfe.bindPopup(popup_927d9d63c552232de893a54c827aabe9)\\n ;\\n\\n \\n \\n \\n var circle_marker_d75ef19823b7c60ddc886dbcdf813b25 = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_767f3bb7e46c9e0644b99fa4b6206a59 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c8d642eaffedff9b925ca535fe59dce = $(`<div id="html_9c8d642eaffedff9b925ca535fe59dce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_767f3bb7e46c9e0644b99fa4b6206a59.setContent(html_9c8d642eaffedff9b925ca535fe59dce);\\n \\n \\n\\n circle_marker_d75ef19823b7c60ddc886dbcdf813b25.bindPopup(popup_767f3bb7e46c9e0644b99fa4b6206a59)\\n ;\\n\\n \\n \\n \\n var circle_marker_607922e02c83c4e36ea7db22ca31b7e8 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_bbffa70fbf8163b1bec46afa572536e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2387afa5243cd8b953ee803441656037 = $(`<div id="html_2387afa5243cd8b953ee803441656037" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bbffa70fbf8163b1bec46afa572536e2.setContent(html_2387afa5243cd8b953ee803441656037);\\n \\n \\n\\n circle_marker_607922e02c83c4e36ea7db22ca31b7e8.bindPopup(popup_bbffa70fbf8163b1bec46afa572536e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_62665e5e14e409f025a01c888da62a56 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.352372348458314, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_610802a47992b6ad3ba64f8fc345edf6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10d791aa9edc8ff977939d85a8f1b947 = $(`<div id="html_10d791aa9edc8ff977939d85a8f1b947" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_610802a47992b6ad3ba64f8fc345edf6.setContent(html_10d791aa9edc8ff977939d85a8f1b947);\\n \\n \\n\\n circle_marker_62665e5e14e409f025a01c888da62a56.bindPopup(popup_610802a47992b6ad3ba64f8fc345edf6)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba57eaa9d09c2e44b544e656dc2643e9 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_9b3658a5f716950ae47b42df7328acbc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4558e7cf837fb043a0d8284c8cf4263c = $(`<div id="html_4558e7cf837fb043a0d8284c8cf4263c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b3658a5f716950ae47b42df7328acbc.setContent(html_4558e7cf837fb043a0d8284c8cf4263c);\\n \\n \\n\\n circle_marker_ba57eaa9d09c2e44b544e656dc2643e9.bindPopup(popup_9b3658a5f716950ae47b42df7328acbc)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea6abc55e75e791aedee33b6c04a2d97 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ed995d06407139281c0f1fb6de74f84d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7819c264814556d39fd85bb7e99bf632 = $(`<div id="html_7819c264814556d39fd85bb7e99bf632" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed995d06407139281c0f1fb6de74f84d.setContent(html_7819c264814556d39fd85bb7e99bf632);\\n \\n \\n\\n circle_marker_ea6abc55e75e791aedee33b6c04a2d97.bindPopup(popup_ed995d06407139281c0f1fb6de74f84d)\\n ;\\n\\n \\n \\n \\n var circle_marker_7651faa1bfd7cfafb3f878339713adea = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_97893ac20e90574decf1118eaa6ff6ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0aa40f6ac7506d29b12cebd28409e09e = $(`<div id="html_0aa40f6ac7506d29b12cebd28409e09e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97893ac20e90574decf1118eaa6ff6ff.setContent(html_0aa40f6ac7506d29b12cebd28409e09e);\\n \\n \\n\\n circle_marker_7651faa1bfd7cfafb3f878339713adea.bindPopup(popup_97893ac20e90574decf1118eaa6ff6ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_29a4c9edb5b0e63e87de9107c87dbccb = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_650049a074d160b481ddbaf3a3d203be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_535b6829575d92a309f1c269608be96f = $(`<div id="html_535b6829575d92a309f1c269608be96f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_650049a074d160b481ddbaf3a3d203be.setContent(html_535b6829575d92a309f1c269608be96f);\\n \\n \\n\\n circle_marker_29a4c9edb5b0e63e87de9107c87dbccb.bindPopup(popup_650049a074d160b481ddbaf3a3d203be)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce4fd7084493c55aa3ddef0c94b06c54 = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_b958b7e0ec7b962d6a6a818eab7374d0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0455f3a87ea66f8ba7c65dc4f333c0a1 = $(`<div id="html_0455f3a87ea66f8ba7c65dc4f333c0a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b958b7e0ec7b962d6a6a818eab7374d0.setContent(html_0455f3a87ea66f8ba7c65dc4f333c0a1);\\n \\n \\n\\n circle_marker_ce4fd7084493c55aa3ddef0c94b06c54.bindPopup(popup_b958b7e0ec7b962d6a6a818eab7374d0)\\n ;\\n\\n \\n \\n \\n var circle_marker_5540785833bad0f01e3de12a0c127d88 = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c9d81c66a80e94cf5e3b9da2dfb60954 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b01e0e3ee3b79443c0c56f8de89773d = $(`<div id="html_1b01e0e3ee3b79443c0c56f8de89773d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9d81c66a80e94cf5e3b9da2dfb60954.setContent(html_1b01e0e3ee3b79443c0c56f8de89773d);\\n \\n \\n\\n circle_marker_5540785833bad0f01e3de12a0c127d88.bindPopup(popup_c9d81c66a80e94cf5e3b9da2dfb60954)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0c89f19e208f4c8cfd210f8e8e60bef = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ba20009da6365524eb3ab9af962131fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69e6a37f3f51f184cb9706397fb2999a = $(`<div id="html_69e6a37f3f51f184cb9706397fb2999a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba20009da6365524eb3ab9af962131fa.setContent(html_69e6a37f3f51f184cb9706397fb2999a);\\n \\n \\n\\n circle_marker_e0c89f19e208f4c8cfd210f8e8e60bef.bindPopup(popup_ba20009da6365524eb3ab9af962131fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_21e9a4f765628fdbbdcf0ce4cdbe968f = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cdafd3bb7844a17984f595e4f63ab77c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e42ee4ddb848884e18c19a850dc90e3 = $(`<div id="html_9e42ee4ddb848884e18c19a850dc90e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cdafd3bb7844a17984f595e4f63ab77c.setContent(html_9e42ee4ddb848884e18c19a850dc90e3);\\n \\n \\n\\n circle_marker_21e9a4f765628fdbbdcf0ce4cdbe968f.bindPopup(popup_cdafd3bb7844a17984f595e4f63ab77c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2dbb116d260ca002ce273518d266fc13 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c83bbf8bf0b6442e22c400b4adc03321 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c143a913f5db5bdb8a64534e13cb208f = $(`<div id="html_c143a913f5db5bdb8a64534e13cb208f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c83bbf8bf0b6442e22c400b4adc03321.setContent(html_c143a913f5db5bdb8a64534e13cb208f);\\n \\n \\n\\n circle_marker_2dbb116d260ca002ce273518d266fc13.bindPopup(popup_c83bbf8bf0b6442e22c400b4adc03321)\\n ;\\n\\n \\n \\n \\n var circle_marker_07b5a0528df2bb44c78cb8931ed3f05a = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_2df6bfbc6f4d4b1aaec248242e3db925 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9d7f374f3a95b081ce075f19c5f02f1 = $(`<div id="html_a9d7f374f3a95b081ce075f19c5f02f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2df6bfbc6f4d4b1aaec248242e3db925.setContent(html_a9d7f374f3a95b081ce075f19c5f02f1);\\n \\n \\n\\n circle_marker_07b5a0528df2bb44c78cb8931ed3f05a.bindPopup(popup_2df6bfbc6f4d4b1aaec248242e3db925)\\n ;\\n\\n \\n \\n \\n var circle_marker_7dd011c325165709a79d83310b78d10c = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ebf9ec002830a3d86c74ca9506d52d15 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cdc88250dd4aae70fe81143da9cffbad = $(`<div id="html_cdc88250dd4aae70fe81143da9cffbad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebf9ec002830a3d86c74ca9506d52d15.setContent(html_cdc88250dd4aae70fe81143da9cffbad);\\n \\n \\n\\n circle_marker_7dd011c325165709a79d83310b78d10c.bindPopup(popup_ebf9ec002830a3d86c74ca9506d52d15)\\n ;\\n\\n \\n \\n \\n var circle_marker_ae3f44615b79e841a66d8c2bca6e3aeb = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_48c2ddc9990117dd5345541cd6c65480 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e25488a82623c1710910e0e6aa64746 = $(`<div id="html_0e25488a82623c1710910e0e6aa64746" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_48c2ddc9990117dd5345541cd6c65480.setContent(html_0e25488a82623c1710910e0e6aa64746);\\n \\n \\n\\n circle_marker_ae3f44615b79e841a66d8c2bca6e3aeb.bindPopup(popup_48c2ddc9990117dd5345541cd6c65480)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a9cb173141be4aa05b1a98653a14391 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_1b64d2ddb29a5ebe608128a8632ae70d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dfaa3e333dfba5f71ce3336749e1a57d = $(`<div id="html_dfaa3e333dfba5f71ce3336749e1a57d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b64d2ddb29a5ebe608128a8632ae70d.setContent(html_dfaa3e333dfba5f71ce3336749e1a57d);\\n \\n \\n\\n circle_marker_6a9cb173141be4aa05b1a98653a14391.bindPopup(popup_1b64d2ddb29a5ebe608128a8632ae70d)\\n ;\\n\\n \\n \\n \\n var circle_marker_10de3486ca9f91abc7698aa08359b5b7 = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a368197377b956a40ac1b41a3b7c6286 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5209a191716d177c8d56a2dfff4a248c = $(`<div id="html_5209a191716d177c8d56a2dfff4a248c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a368197377b956a40ac1b41a3b7c6286.setContent(html_5209a191716d177c8d56a2dfff4a248c);\\n \\n \\n\\n circle_marker_10de3486ca9f91abc7698aa08359b5b7.bindPopup(popup_a368197377b956a40ac1b41a3b7c6286)\\n ;\\n\\n \\n \\n \\n var circle_marker_b785b12467cdf2a6fa8813a6d9ea2704 = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_40a49161a2514e181d1ea89dc19658fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76714e97b34d00fbc25266db47637233 = $(`<div id="html_76714e97b34d00fbc25266db47637233" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_40a49161a2514e181d1ea89dc19658fb.setContent(html_76714e97b34d00fbc25266db47637233);\\n \\n \\n\\n circle_marker_b785b12467cdf2a6fa8813a6d9ea2704.bindPopup(popup_40a49161a2514e181d1ea89dc19658fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b7821f42fc1ae96e642155313fb6506 = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d4bc429c6f1b12a482132ac3e1e2af38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19429d7bd411144584779f7b47c81ef3 = $(`<div id="html_19429d7bd411144584779f7b47c81ef3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d4bc429c6f1b12a482132ac3e1e2af38.setContent(html_19429d7bd411144584779f7b47c81ef3);\\n \\n \\n\\n circle_marker_4b7821f42fc1ae96e642155313fb6506.bindPopup(popup_d4bc429c6f1b12a482132ac3e1e2af38)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb0526665dab25e1c977b42321334349 = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ff78e508105aa214c288989be7680f33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c2a24d383b850a8b0229706af9746c01 = $(`<div id="html_c2a24d383b850a8b0229706af9746c01" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff78e508105aa214c288989be7680f33.setContent(html_c2a24d383b850a8b0229706af9746c01);\\n \\n \\n\\n circle_marker_cb0526665dab25e1c977b42321334349.bindPopup(popup_ff78e508105aa214c288989be7680f33)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d2261ff27103f114a12c6e77ebfba0c = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_9bb00347929cac0f78503e70694b1807 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2600a1d189c41afa8a28638e74d328b = $(`<div id="html_d2600a1d189c41afa8a28638e74d328b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9bb00347929cac0f78503e70694b1807.setContent(html_d2600a1d189c41afa8a28638e74d328b);\\n \\n \\n\\n circle_marker_3d2261ff27103f114a12c6e77ebfba0c.bindPopup(popup_9bb00347929cac0f78503e70694b1807)\\n ;\\n\\n \\n \\n \\n var circle_marker_170fd17830d95792c1cdcf67f39bd095 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.720348719413148, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_2c79f0c9160451be17e1171b5d44b4af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b74338482dc454259d67235fe78e5037 = $(`<div id="html_b74338482dc454259d67235fe78e5037" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c79f0c9160451be17e1171b5d44b4af.setContent(html_b74338482dc454259d67235fe78e5037);\\n \\n \\n\\n circle_marker_170fd17830d95792c1cdcf67f39bd095.bindPopup(popup_2c79f0c9160451be17e1171b5d44b4af)\\n ;\\n\\n \\n \\n \\n var circle_marker_d2c3ad23bbd34f3e780616ff39a0bb2b = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_24ebfb3fd45c8a55148cb79b60e79884 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2dd14cf27d86f19aeef0a0193b69230d = $(`<div id="html_2dd14cf27d86f19aeef0a0193b69230d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24ebfb3fd45c8a55148cb79b60e79884.setContent(html_2dd14cf27d86f19aeef0a0193b69230d);\\n \\n \\n\\n circle_marker_d2c3ad23bbd34f3e780616ff39a0bb2b.bindPopup(popup_24ebfb3fd45c8a55148cb79b60e79884)\\n ;\\n\\n \\n \\n \\n var circle_marker_9445f6780344511b9dabfe56f2b259d4 = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a9eb5c77ca50a3ce832c65d9082fa540 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da6089c1aabf5107415a50a18447af8f = $(`<div id="html_da6089c1aabf5107415a50a18447af8f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9eb5c77ca50a3ce832c65d9082fa540.setContent(html_da6089c1aabf5107415a50a18447af8f);\\n \\n \\n\\n circle_marker_9445f6780344511b9dabfe56f2b259d4.bindPopup(popup_a9eb5c77ca50a3ce832c65d9082fa540)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e5c20c23af7a503de6f809d108a293f = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_2bc1ed23f64b43fb48063c047bf7995e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36fa675da3db0355e7d11a156df6b459 = $(`<div id="html_36fa675da3db0355e7d11a156df6b459" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2bc1ed23f64b43fb48063c047bf7995e.setContent(html_36fa675da3db0355e7d11a156df6b459);\\n \\n \\n\\n circle_marker_7e5c20c23af7a503de6f809d108a293f.bindPopup(popup_2bc1ed23f64b43fb48063c047bf7995e)\\n ;\\n\\n \\n \\n \\n var circle_marker_5080d351c5af1aa754c717bdc050b375 = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_102122e495f5aadfd6d5d69792055876 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1174c977393f6179bd05dd061737d3ea = $(`<div id="html_1174c977393f6179bd05dd061737d3ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_102122e495f5aadfd6d5d69792055876.setContent(html_1174c977393f6179bd05dd061737d3ea);\\n \\n \\n\\n circle_marker_5080d351c5af1aa754c717bdc050b375.bindPopup(popup_102122e495f5aadfd6d5d69792055876)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e4afe8b5bc47045360996791e5f3b02 = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cf88f6ec3cc44a439cd5231499199205 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28993146eb6bcce42712f697d01d6e4b = $(`<div id="html_28993146eb6bcce42712f697d01d6e4b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf88f6ec3cc44a439cd5231499199205.setContent(html_28993146eb6bcce42712f697d01d6e4b);\\n \\n \\n\\n circle_marker_0e4afe8b5bc47045360996791e5f3b02.bindPopup(popup_cf88f6ec3cc44a439cd5231499199205)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5b57f24363ed5529f9e1ae2f8d4c0e8 = L.circleMarker(\\n [42.73635085311189, -73.22304483851624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_b21ef904b8246304184817a55f1a0a84 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa69676128426e7ba0aaed04db1d4b5b = $(`<div id="html_aa69676128426e7ba0aaed04db1d4b5b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b21ef904b8246304184817a55f1a0a84.setContent(html_aa69676128426e7ba0aaed04db1d4b5b);\\n \\n \\n\\n circle_marker_a5b57f24363ed5529f9e1ae2f8d4c0e8.bindPopup(popup_b21ef904b8246304184817a55f1a0a84)\\n ;\\n\\n \\n \\n \\n var circle_marker_efd476529c444597ceabf6c72ed0fe8a = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_54e2954f0a7e03c94ef432ab95ae3ccc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_73ad3aba95fb8ae0ff7e9ad22edf0717 = $(`<div id="html_73ad3aba95fb8ae0ff7e9ad22edf0717" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54e2954f0a7e03c94ef432ab95ae3ccc.setContent(html_73ad3aba95fb8ae0ff7e9ad22edf0717);\\n \\n \\n\\n circle_marker_efd476529c444597ceabf6c72ed0fe8a.bindPopup(popup_54e2954f0a7e03c94ef432ab95ae3ccc)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb61de880261ca9ab31a5801eb6c18bf = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f448bbe74633b3d817114047b055b070 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6a47ab240f75285f197531b77b7fc65 = $(`<div id="html_f6a47ab240f75285f197531b77b7fc65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f448bbe74633b3d817114047b055b070.setContent(html_f6a47ab240f75285f197531b77b7fc65);\\n \\n \\n\\n circle_marker_fb61de880261ca9ab31a5801eb6c18bf.bindPopup(popup_f448bbe74633b3d817114047b055b070)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a2e89f3404605b100f448a91ac44dfd = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ccd9091cc6e69c67997a9c6a4ead895a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0157c18569287202276df47304566ca0 = $(`<div id="html_0157c18569287202276df47304566ca0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ccd9091cc6e69c67997a9c6a4ead895a.setContent(html_0157c18569287202276df47304566ca0);\\n \\n \\n\\n circle_marker_0a2e89f3404605b100f448a91ac44dfd.bindPopup(popup_ccd9091cc6e69c67997a9c6a4ead895a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8e62139b683a18c2346ee6385529a92 = L.circleMarker(\\n [42.7345512252472, -73.26979251605432],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cec08b6eda51be664ce1ae679be26781 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2c1abece358dae35b1069c864e3054b = $(`<div id="html_b2c1abece358dae35b1069c864e3054b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cec08b6eda51be664ce1ae679be26781.setContent(html_b2c1abece358dae35b1069c864e3054b);\\n \\n \\n\\n circle_marker_a8e62139b683a18c2346ee6385529a92.bindPopup(popup_cec08b6eda51be664ce1ae679be26781)\\n ;\\n\\n \\n \\n \\n var circle_marker_b709697a18a21e0a6a631ce298aa4253 = L.circleMarker(\\n [42.73455127133177, -73.26856234600437],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_e025f88903ad696a8af56825cab8a7ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f8180516d3ba7e7dd6b429c26a7d89c = $(`<div id="html_3f8180516d3ba7e7dd6b429c26a7d89c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e025f88903ad696a8af56825cab8a7ae.setContent(html_3f8180516d3ba7e7dd6b429c26a7d89c);\\n \\n \\n\\n circle_marker_b709697a18a21e0a6a631ce298aa4253.bindPopup(popup_e025f88903ad696a8af56825cab8a7ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_3dfe26e831ff30caa120ca67096f2960 = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f426874fb68cabc8f520b537a1ad0844 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d3ae9c1d118a05bbabe378f1e0db86a = $(`<div id="html_9d3ae9c1d118a05bbabe378f1e0db86a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f426874fb68cabc8f520b537a1ad0844.setContent(html_9d3ae9c1d118a05bbabe378f1e0db86a);\\n \\n \\n\\n circle_marker_3dfe26e831ff30caa120ca67096f2960.bindPopup(popup_f426874fb68cabc8f520b537a1ad0844)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e14a1f8d681277783acb1e161ceff09 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4d2788b65cafc287b4877508c6b5b97d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38916b40615781ccf2509f1d3fd2d2fe = $(`<div id="html_38916b40615781ccf2509f1d3fd2d2fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4d2788b65cafc287b4877508c6b5b97d.setContent(html_38916b40615781ccf2509f1d3fd2d2fe);\\n \\n \\n\\n circle_marker_1e14a1f8d681277783acb1e161ceff09.bindPopup(popup_4d2788b65cafc287b4877508c6b5b97d)\\n ;\\n\\n \\n \\n \\n var circle_marker_706cdc3bdcb266d892097ccca711a189 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_083ead4e6ef95f50b206e17bfdd26e91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24422f3c566a29d177d76794e87013a1 = $(`<div id="html_24422f3c566a29d177d76794e87013a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_083ead4e6ef95f50b206e17bfdd26e91.setContent(html_24422f3c566a29d177d76794e87013a1);\\n \\n \\n\\n circle_marker_706cdc3bdcb266d892097ccca711a189.bindPopup(popup_083ead4e6ef95f50b206e17bfdd26e91)\\n ;\\n\\n \\n \\n \\n var circle_marker_e5584ffe8ff70bdb7993078e47e5fec9 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4d002e87988ccada4299d30972b17c19 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_632b91bc725b5ebdd464f053cb6359e9 = $(`<div id="html_632b91bc725b5ebdd464f053cb6359e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4d002e87988ccada4299d30972b17c19.setContent(html_632b91bc725b5ebdd464f053cb6359e9);\\n \\n \\n\\n circle_marker_e5584ffe8ff70bdb7993078e47e5fec9.bindPopup(popup_4d002e87988ccada4299d30972b17c19)\\n ;\\n\\n \\n \\n \\n var circle_marker_2273534883983d63a5830ed3211651bc = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_29955abe4a2ca4f09f51cd6982c5be92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6781a6f7e575232fb6df4ae9d4caacc6 = $(`<div id="html_6781a6f7e575232fb6df4ae9d4caacc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_29955abe4a2ca4f09f51cd6982c5be92.setContent(html_6781a6f7e575232fb6df4ae9d4caacc6);\\n \\n \\n\\n circle_marker_2273534883983d63a5830ed3211651bc.bindPopup(popup_29955abe4a2ca4f09f51cd6982c5be92)\\n ;\\n\\n \\n \\n \\n var circle_marker_00b804b60e56aed0e1a1cc619ee0b750 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_41f5ce64100faae63f829a41929eb908 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9da02e599a7aee3df76e41c536818811 = $(`<div id="html_9da02e599a7aee3df76e41c536818811" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41f5ce64100faae63f829a41929eb908.setContent(html_9da02e599a7aee3df76e41c536818811);\\n \\n \\n\\n circle_marker_00b804b60e56aed0e1a1cc619ee0b750.bindPopup(popup_41f5ce64100faae63f829a41929eb908)\\n ;\\n\\n \\n \\n \\n var circle_marker_310e32ab0bbaf4aaf771fba7a19de3c7 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.720348719413148, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_9794a5c5bf912bb3a14dd68fc266ea67 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1d2d69a88ea1f7592fe519b91b569e30 = $(`<div id="html_1d2d69a88ea1f7592fe519b91b569e30" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9794a5c5bf912bb3a14dd68fc266ea67.setContent(html_1d2d69a88ea1f7592fe519b91b569e30);\\n \\n \\n\\n circle_marker_310e32ab0bbaf4aaf771fba7a19de3c7.bindPopup(popup_9794a5c5bf912bb3a14dd68fc266ea67)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fae28aacdc19ae78df2cb5a146d0e2c = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.863230142835039, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_536fc05dd6cf9bfa7893216d45238ab8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_11816b8a09c203ea9b8414835a293776 = $(`<div id="html_11816b8a09c203ea9b8414835a293776" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_536fc05dd6cf9bfa7893216d45238ab8.setContent(html_11816b8a09c203ea9b8414835a293776);\\n \\n \\n\\n circle_marker_9fae28aacdc19ae78df2cb5a146d0e2c.bindPopup(popup_536fc05dd6cf9bfa7893216d45238ab8)\\n ;\\n\\n \\n \\n \\n var circle_marker_f244af8c50a7c93a805efafbfd0204fb = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.686510657907603, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3ca524772d1c83d78666460746f5e0f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e1a08b1654f8f87e2c7c2df931ef411 = $(`<div id="html_6e1a08b1654f8f87e2c7c2df931ef411" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ca524772d1c83d78666460746f5e0f3.setContent(html_6e1a08b1654f8f87e2c7c2df931ef411);\\n \\n \\n\\n circle_marker_f244af8c50a7c93a805efafbfd0204fb.bindPopup(popup_3ca524772d1c83d78666460746f5e0f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_f759a0f5c451df8b9ceb4cb625eeb129 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_fdd58af28c263c112ac434d3a5ca9ed0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a5fa2070a3090efeb3efac86c30ba29 = $(`<div id="html_0a5fa2070a3090efeb3efac86c30ba29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fdd58af28c263c112ac434d3a5ca9ed0.setContent(html_0a5fa2070a3090efeb3efac86c30ba29);\\n \\n \\n\\n circle_marker_f759a0f5c451df8b9ceb4cb625eeb129.bindPopup(popup_fdd58af28c263c112ac434d3a5ca9ed0)\\n ;\\n\\n \\n \\n \\n var circle_marker_1551dc274ca6e96c6ef3a515b4866d1d = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_7f52c126277b8de4f0032ba3ee7b82c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_52f22848ea5fe7fa9e6ba1e9e7ad37a9 = $(`<div id="html_52f22848ea5fe7fa9e6ba1e9e7ad37a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f52c126277b8de4f0032ba3ee7b82c4.setContent(html_52f22848ea5fe7fa9e6ba1e9e7ad37a9);\\n \\n \\n\\n circle_marker_1551dc274ca6e96c6ef3a515b4866d1d.bindPopup(popup_7f52c126277b8de4f0032ba3ee7b82c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8700cc589bea32e4a21146285886021 = L.circleMarker(\\n [42.7345450038305, -73.22673656679281],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_afc6dde8e2b90d1997bb83d7d48f3380 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c44036650d14890cf2bf30e83886ec31 = $(`<div id="html_c44036650d14890cf2bf30e83886ec31" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_afc6dde8e2b90d1997bb83d7d48f3380.setContent(html_c44036650d14890cf2bf30e83886ec31);\\n \\n \\n\\n circle_marker_c8700cc589bea32e4a21146285886021.bindPopup(popup_afc6dde8e2b90d1997bb83d7d48f3380)\\n ;\\n\\n \\n \\n \\n var circle_marker_e54197a93a9e80eef7b835659888e566 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_dc57053dc67729d9550f5a2f1be4b7d6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_094f4cf45a165b22bcd81e0b08e94111 = $(`<div id="html_094f4cf45a165b22bcd81e0b08e94111" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc57053dc67729d9550f5a2f1be4b7d6.setContent(html_094f4cf45a165b22bcd81e0b08e94111);\\n \\n \\n\\n circle_marker_e54197a93a9e80eef7b835659888e566.bindPopup(popup_dc57053dc67729d9550f5a2f1be4b7d6)\\n ;\\n\\n \\n \\n \\n var circle_marker_c44af360d3780121484646f6f0e29449 = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_dcf121dd79b367d808bd6f8a96e1dc0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc3e6561736d7e9853bca4f4141ba9ac = $(`<div id="html_bc3e6561736d7e9853bca4f4141ba9ac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dcf121dd79b367d808bd6f8a96e1dc0d.setContent(html_bc3e6561736d7e9853bca4f4141ba9ac);\\n \\n \\n\\n circle_marker_c44af360d3780121484646f6f0e29449.bindPopup(popup_dcf121dd79b367d808bd6f8a96e1dc0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d4f5b833899dbfe5dbcb413649f58d8 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_8c93e46d9d7349bf1de2c78ee20a569b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_75b7b27ad791458836d25b1a13bb196f = $(`<div id="html_75b7b27ad791458836d25b1a13bb196f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c93e46d9d7349bf1de2c78ee20a569b.setContent(html_75b7b27ad791458836d25b1a13bb196f);\\n \\n \\n\\n circle_marker_2d4f5b833899dbfe5dbcb413649f58d8.bindPopup(popup_8c93e46d9d7349bf1de2c78ee20a569b)\\n ;\\n\\n \\n \\n \\n var circle_marker_617cf95e53f5ec8c1d9225dc24e68f15 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a28279d6f06e2b51b28e7b5bf5e53d7e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6ac04f48f12fe80737abf6b95c54c0c = $(`<div id="html_c6ac04f48f12fe80737abf6b95c54c0c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a28279d6f06e2b51b28e7b5bf5e53d7e.setContent(html_c6ac04f48f12fe80737abf6b95c54c0c);\\n \\n \\n\\n circle_marker_617cf95e53f5ec8c1d9225dc24e68f15.bindPopup(popup_a28279d6f06e2b51b28e7b5bf5e53d7e)\\n ;\\n\\n \\n \\n \\n var circle_marker_9230ec6aa6ca873cde4c013b08980c50 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_93ee0967679406cae211604145b7a465 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b9103dfe6bf762630e54f489c308026 = $(`<div id="html_5b9103dfe6bf762630e54f489c308026" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93ee0967679406cae211604145b7a465.setContent(html_5b9103dfe6bf762630e54f489c308026);\\n \\n \\n\\n circle_marker_9230ec6aa6ca873cde4c013b08980c50.bindPopup(popup_93ee0967679406cae211604145b7a465)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d1f71b39f28f0630f9295eb3101c90a = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_dcfa6ec667edb50256b1bdaf6991ec25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_411609f336bca182205cd32c491f2e52 = $(`<div id="html_411609f336bca182205cd32c491f2e52" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dcfa6ec667edb50256b1bdaf6991ec25.setContent(html_411609f336bca182205cd32c491f2e52);\\n \\n \\n\\n circle_marker_7d1f71b39f28f0630f9295eb3101c90a.bindPopup(popup_dcfa6ec667edb50256b1bdaf6991ec25)\\n ;\\n\\n \\n \\n \\n var circle_marker_33fa7acd55ea0514e5391d939f2b2772 = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d687c5fba70668839bd0c42d99906b8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2eb6aa5b7a819eebd4df422ed0c02b8b = $(`<div id="html_2eb6aa5b7a819eebd4df422ed0c02b8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d687c5fba70668839bd0c42d99906b8d.setContent(html_2eb6aa5b7a819eebd4df422ed0c02b8b);\\n \\n \\n\\n circle_marker_33fa7acd55ea0514e5391d939f2b2772.bindPopup(popup_d687c5fba70668839bd0c42d99906b8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f2ab83d524ee82d16428df82f24ac57 = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_aebbfd2aca154ece4107109a89aef93c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8a3fba3e626a0f1280a360c0755bdec = $(`<div id="html_f8a3fba3e626a0f1280a360c0755bdec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aebbfd2aca154ece4107109a89aef93c.setContent(html_f8a3fba3e626a0f1280a360c0755bdec);\\n \\n \\n\\n circle_marker_9f2ab83d524ee82d16428df82f24ac57.bindPopup(popup_aebbfd2aca154ece4107109a89aef93c)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d4d3dba29691586d2d4569f406fed3f = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_222d42092d1c4584859b0883464d1b92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53e890529c6f939838fd52f4e8c4c91f = $(`<div id="html_53e890529c6f939838fd52f4e8c4c91f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_222d42092d1c4584859b0883464d1b92.setContent(html_53e890529c6f939838fd52f4e8c4c91f);\\n \\n \\n\\n circle_marker_3d4d3dba29691586d2d4569f406fed3f.bindPopup(popup_222d42092d1c4584859b0883464d1b92)\\n ;\\n\\n \\n \\n \\n var circle_marker_d9577df58ae7175d5f36a68e1c966589 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a1d9f2655b67e86151ff7e5c1325b116 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_72794cadc80989d78372c848d71e2649 = $(`<div id="html_72794cadc80989d78372c848d71e2649" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a1d9f2655b67e86151ff7e5c1325b116.setContent(html_72794cadc80989d78372c848d71e2649);\\n \\n \\n\\n circle_marker_d9577df58ae7175d5f36a68e1c966589.bindPopup(popup_a1d9f2655b67e86151ff7e5c1325b116)\\n ;\\n\\n \\n \\n \\n var circle_marker_c35eb71ae8c673e96586537c1ae56e7a = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_03a4e299fcc59dbcdc59957f7ea090a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b2d7ca137203142048d249e6c1959f7 = $(`<div id="html_5b2d7ca137203142048d249e6c1959f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03a4e299fcc59dbcdc59957f7ea090a3.setContent(html_5b2d7ca137203142048d249e6c1959f7);\\n \\n \\n\\n circle_marker_c35eb71ae8c673e96586537c1ae56e7a.bindPopup(popup_03a4e299fcc59dbcdc59957f7ea090a3)\\n ;\\n\\n \\n \\n \\n var circle_marker_cd33f80a1a2ef44b8fdc6302c32c0c98 = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_fd734ba39b31e1c8a06699bf39cea12d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6c6e906c4558dd3aaf67eebbf159b2dd = $(`<div id="html_6c6e906c4558dd3aaf67eebbf159b2dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd734ba39b31e1c8a06699bf39cea12d.setContent(html_6c6e906c4558dd3aaf67eebbf159b2dd);\\n \\n \\n\\n circle_marker_cd33f80a1a2ef44b8fdc6302c32c0c98.bindPopup(popup_fd734ba39b31e1c8a06699bf39cea12d)\\n ;\\n\\n \\n \\n \\n var circle_marker_66c6a46834e1ce4f1004995c654cf8bd = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cff82ffc384979d1bc3fcb54e27f9a1f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dacdaabb1493c01eb8d17fc0657296f5 = $(`<div id="html_dacdaabb1493c01eb8d17fc0657296f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cff82ffc384979d1bc3fcb54e27f9a1f.setContent(html_dacdaabb1493c01eb8d17fc0657296f5);\\n \\n \\n\\n circle_marker_66c6a46834e1ce4f1004995c654cf8bd.bindPopup(popup_cff82ffc384979d1bc3fcb54e27f9a1f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6828fa35b99627499a891b9bce910e62 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f2ccb2b76022ecd5dfc073fe34833ee1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6cf25dddb99fe99151aacb34ad14f98 = $(`<div id="html_f6cf25dddb99fe99151aacb34ad14f98" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2ccb2b76022ecd5dfc073fe34833ee1.setContent(html_f6cf25dddb99fe99151aacb34ad14f98);\\n \\n \\n\\n circle_marker_6828fa35b99627499a891b9bce910e62.bindPopup(popup_f2ccb2b76022ecd5dfc073fe34833ee1)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce313114325b9c5c54311894d7013170 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d06d89d66566d1c282624a54271bc578 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5369c9bb55895af4d9dd01fb04f39df4 = $(`<div id="html_5369c9bb55895af4d9dd01fb04f39df4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d06d89d66566d1c282624a54271bc578.setContent(html_5369c9bb55895af4d9dd01fb04f39df4);\\n \\n \\n\\n circle_marker_ce313114325b9c5c54311894d7013170.bindPopup(popup_d06d89d66566d1c282624a54271bc578)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a0f16ad54704c7184bd76993ec759b4 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_b21f4b7e4136187b91de3a3729b37f27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7230ab21d1bfaf3c8b4d892a55b717c5 = $(`<div id="html_7230ab21d1bfaf3c8b4d892a55b717c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b21f4b7e4136187b91de3a3729b37f27.setContent(html_7230ab21d1bfaf3c8b4d892a55b717c5);\\n \\n \\n\\n circle_marker_1a0f16ad54704c7184bd76993ec759b4.bindPopup(popup_b21f4b7e4136187b91de3a3729b37f27)\\n ;\\n\\n \\n \\n \\n var circle_marker_d8f4ec5ec1a67e73fc3555f0c09197b7 = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_82dd89348ac397907b2d99f09a776c1e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c1f1a445dbf5562409e50ee0fe77abc = $(`<div id="html_1c1f1a445dbf5562409e50ee0fe77abc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82dd89348ac397907b2d99f09a776c1e.setContent(html_1c1f1a445dbf5562409e50ee0fe77abc);\\n \\n \\n\\n circle_marker_d8f4ec5ec1a67e73fc3555f0c09197b7.bindPopup(popup_82dd89348ac397907b2d99f09a776c1e)\\n ;\\n\\n \\n \\n \\n var circle_marker_621b0cbbb0d032fff44edb499371da6a = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_9c3b2c5dba5652013fd577bacd603154 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d507b5d958293167e970ed35f8afaf85 = $(`<div id="html_d507b5d958293167e970ed35f8afaf85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9c3b2c5dba5652013fd577bacd603154.setContent(html_d507b5d958293167e970ed35f8afaf85);\\n \\n \\n\\n circle_marker_621b0cbbb0d032fff44edb499371da6a.bindPopup(popup_9c3b2c5dba5652013fd577bacd603154)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5ebfca97d9bcd079c4d16c72c1607c8 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_68dcae860a28bd84f45d6c33f4234ff2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6415cb800d1abe49b42a1494bd58813a = $(`<div id="html_6415cb800d1abe49b42a1494bd58813a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68dcae860a28bd84f45d6c33f4234ff2.setContent(html_6415cb800d1abe49b42a1494bd58813a);\\n \\n \\n\\n circle_marker_f5ebfca97d9bcd079c4d16c72c1607c8.bindPopup(popup_68dcae860a28bd84f45d6c33f4234ff2)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d5a74c338a3139dce498ca3a9a535c2 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.853342309955121, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_0cfff83bb40431bd2ee7af8d15470ffd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50aabbe0fbe59d77db632ec43478a4ef = $(`<div id="html_50aabbe0fbe59d77db632ec43478a4ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cfff83bb40431bd2ee7af8d15470ffd.setContent(html_50aabbe0fbe59d77db632ec43478a4ef);\\n \\n \\n\\n circle_marker_7d5a74c338a3139dce498ca3a9a535c2.bindPopup(popup_0cfff83bb40431bd2ee7af8d15470ffd)\\n ;\\n\\n \\n \\n \\n var circle_marker_b14a6b292eca6d005bf7cfc570a90f5f = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.282549314314218, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_46db7b269bd8c29aa3651b15f76ee22a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6c29d4fc34b8a4050e29a3c2e284f380 = $(`<div id="html_6c29d4fc34b8a4050e29a3c2e284f380" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46db7b269bd8c29aa3651b15f76ee22a.setContent(html_6c29d4fc34b8a4050e29a3c2e284f380);\\n \\n \\n\\n circle_marker_b14a6b292eca6d005bf7cfc570a90f5f.bindPopup(popup_46db7b269bd8c29aa3651b15f76ee22a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4c937a08e3102a980e0a3583d260031 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_11f48e9b67813772b0096ebd51df0397 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c54bb8c781afba3624bb79b61a715895 = $(`<div id="html_c54bb8c781afba3624bb79b61a715895" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11f48e9b67813772b0096ebd51df0397.setContent(html_c54bb8c781afba3624bb79b61a715895);\\n \\n \\n\\n circle_marker_e4c937a08e3102a980e0a3583d260031.bindPopup(popup_11f48e9b67813772b0096ebd51df0397)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a042aeb65ec329f758ccf76145e656f = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4dc05120c27416b07a6f9598328748a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a61f0dd93953404e92791fcc2cc8f2d = $(`<div id="html_3a61f0dd93953404e92791fcc2cc8f2d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4dc05120c27416b07a6f9598328748a1.setContent(html_3a61f0dd93953404e92791fcc2cc8f2d);\\n \\n \\n\\n circle_marker_6a042aeb65ec329f758ccf76145e656f.bindPopup(popup_4dc05120c27416b07a6f9598328748a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_56724dd7ed048b4ebfd6a414c67ebd1c = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cfbe80eb2d67e7e644b4aad16bda83ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce5b76eaef8e8b1678a45851bbe66502 = $(`<div id="html_ce5b76eaef8e8b1678a45851bbe66502" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfbe80eb2d67e7e644b4aad16bda83ce.setContent(html_ce5b76eaef8e8b1678a45851bbe66502);\\n \\n \\n\\n circle_marker_56724dd7ed048b4ebfd6a414c67ebd1c.bindPopup(popup_cfbe80eb2d67e7e644b4aad16bda83ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_b779a9311335b941d6cdb77e4ac8129d = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_2fc100d9becb6f607c21b2012f0af3b0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6585497733c82eb8d7d50e58a1b691e = $(`<div id="html_c6585497733c82eb8d7d50e58a1b691e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fc100d9becb6f607c21b2012f0af3b0.setContent(html_c6585497733c82eb8d7d50e58a1b691e);\\n \\n \\n\\n circle_marker_b779a9311335b941d6cdb77e4ac8129d.bindPopup(popup_2fc100d9becb6f607c21b2012f0af3b0)\\n ;\\n\\n \\n \\n \\n var circle_marker_42b1be9f7283f8d6aaf075f2a62db3c4 = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_7c8592874b0e99bb983b950a3d7e7efb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_979ef6411e856a0c6e4913dbdf46acd3 = $(`<div id="html_979ef6411e856a0c6e4913dbdf46acd3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c8592874b0e99bb983b950a3d7e7efb.setContent(html_979ef6411e856a0c6e4913dbdf46acd3);\\n \\n \\n\\n circle_marker_42b1be9f7283f8d6aaf075f2a62db3c4.bindPopup(popup_7c8592874b0e99bb983b950a3d7e7efb)\\n ;\\n\\n \\n \\n \\n var circle_marker_f78fcbf3d953511d991a1c156be61044 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_246a528315647dd814561d1ef1c7ea97 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_335a49dfc060d02e4d34aa738b1124b9 = $(`<div id="html_335a49dfc060d02e4d34aa738b1124b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_246a528315647dd814561d1ef1c7ea97.setContent(html_335a49dfc060d02e4d34aa738b1124b9);\\n \\n \\n\\n circle_marker_f78fcbf3d953511d991a1c156be61044.bindPopup(popup_246a528315647dd814561d1ef1c7ea97)\\n ;\\n\\n \\n \\n \\n var circle_marker_77b63650137bfeb8763ae38552f6d87e = L.circleMarker(\\n [42.730936958507606, -73.26979222927841],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_fc5767c30c48530723019b48d18af0e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1edbcf284dda802b6a4d040db5f57b9 = $(`<div id="html_b1edbcf284dda802b6a4d040db5f57b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc5767c30c48530723019b48d18af0e2.setContent(html_b1edbcf284dda802b6a4d040db5f57b9);\\n \\n \\n\\n circle_marker_77b63650137bfeb8763ae38552f6d87e.bindPopup(popup_fc5767c30c48530723019b48d18af0e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_20054a53ad092bf309fcd03f9195949c = L.circleMarker(\\n [42.73093700458845, -73.26856213092245],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_5f45e477392a3424848a068985cc1b91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0aa7ea65b437cfbe6229830472b5c89 = $(`<div id="html_c0aa7ea65b437cfbe6229830472b5c89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f45e477392a3424848a068985cc1b91.setContent(html_c0aa7ea65b437cfbe6229830472b5c89);\\n \\n \\n\\n circle_marker_20054a53ad092bf309fcd03f9195949c.bindPopup(popup_5f45e477392a3424848a068985cc1b91)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec447a0b717a1f8986672a17e46fcbc3 = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_31c74405dd6f3a474675d072966201f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ccdae0f84f6e3ea3a42c8296e3702f1f = $(`<div id="html_ccdae0f84f6e3ea3a42c8296e3702f1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31c74405dd6f3a474675d072966201f1.setContent(html_ccdae0f84f6e3ea3a42c8296e3702f1f);\\n \\n \\n\\n circle_marker_ec447a0b717a1f8986672a17e46fcbc3.bindPopup(popup_31c74405dd6f3a474675d072966201f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_1431b29dec645b584080f6f6f3eaa48b = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_72552208f34ee6a595e0e41706579ced = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c14a15a04bc53ae56e288cc18f4c2993 = $(`<div id="html_c14a15a04bc53ae56e288cc18f4c2993" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72552208f34ee6a595e0e41706579ced.setContent(html_c14a15a04bc53ae56e288cc18f4c2993);\\n \\n \\n\\n circle_marker_1431b29dec645b584080f6f6f3eaa48b.bindPopup(popup_72552208f34ee6a595e0e41706579ced)\\n ;\\n\\n \\n \\n \\n var circle_marker_7520a1ff0b58db6c96de8e2bbf99d27d = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cc1cb943c552b3baadb69fba4fccc3f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00564552cb05f68072953176fca7ec1f = $(`<div id="html_00564552cb05f68072953176fca7ec1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc1cb943c552b3baadb69fba4fccc3f0.setContent(html_00564552cb05f68072953176fca7ec1f);\\n \\n \\n\\n circle_marker_7520a1ff0b58db6c96de8e2bbf99d27d.bindPopup(popup_cc1cb943c552b3baadb69fba4fccc3f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_dad757b59fe66edc25b6ce7c5f1fdcd4 = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c37f67725ba00bb5798a92d06a3121d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57d0ae21752dc8b81e86ecf365464736 = $(`<div id="html_57d0ae21752dc8b81e86ecf365464736" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c37f67725ba00bb5798a92d06a3121d7.setContent(html_57d0ae21752dc8b81e86ecf365464736);\\n \\n \\n\\n circle_marker_dad757b59fe66edc25b6ce7c5f1fdcd4.bindPopup(popup_c37f67725ba00bb5798a92d06a3121d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_142ec2be9a2dc9cc2cb7a4f61d2b9bdb = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3a56c6d8fea1fc976a4032d948d66c8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d4014edbd443bc5446d79735d8c1faa = $(`<div id="html_6d4014edbd443bc5446d79735d8c1faa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a56c6d8fea1fc976a4032d948d66c8f.setContent(html_6d4014edbd443bc5446d79735d8c1faa);\\n \\n \\n\\n circle_marker_142ec2be9a2dc9cc2cb7a4f61d2b9bdb.bindPopup(popup_3a56c6d8fea1fc976a4032d948d66c8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3a7683f158792293d90a5c968e89987 = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_b7ddbffd1f600a0f8abe4b74520c1bf9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_408d7a640a9b419ff8f44e213041040a = $(`<div id="html_408d7a640a9b419ff8f44e213041040a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b7ddbffd1f600a0f8abe4b74520c1bf9.setContent(html_408d7a640a9b419ff8f44e213041040a);\\n \\n \\n\\n circle_marker_f3a7683f158792293d90a5c968e89987.bindPopup(popup_b7ddbffd1f600a0f8abe4b74520c1bf9)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8a149fdc278cf0d77eee5f7dfe26105 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4115163798060095, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_dcfe4eecba2ed4fef953964fdb2d768d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1e8b0c084c03380f32765f27a2a539c = $(`<div id="html_a1e8b0c084c03380f32765f27a2a539c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dcfe4eecba2ed4fef953964fdb2d768d.setContent(html_a1e8b0c084c03380f32765f27a2a539c);\\n \\n \\n\\n circle_marker_f8a149fdc278cf0d77eee5f7dfe26105.bindPopup(popup_dcfe4eecba2ed4fef953964fdb2d768d)\\n ;\\n\\n \\n \\n \\n var circle_marker_77ab9db8c5082d307d4ea1b14ff8c12b = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_1bbb18762dedc43cff2319fbffe53c7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4734655964ee8a8e9e77ad3dd0b3672 = $(`<div id="html_a4734655964ee8a8e9e77ad3dd0b3672" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1bbb18762dedc43cff2319fbffe53c7b.setContent(html_a4734655964ee8a8e9e77ad3dd0b3672);\\n \\n \\n\\n circle_marker_77ab9db8c5082d307d4ea1b14ff8c12b.bindPopup(popup_1bbb18762dedc43cff2319fbffe53c7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_f97ea64429c2f121d478615b8a1859dd = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_dfdf40d0ed6d627c06999be367248e06 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba3c190822063273becae20280f73634 = $(`<div id="html_ba3c190822063273becae20280f73634" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dfdf40d0ed6d627c06999be367248e06.setContent(html_ba3c190822063273becae20280f73634);\\n \\n \\n\\n circle_marker_f97ea64429c2f121d478615b8a1859dd.bindPopup(popup_dfdf40d0ed6d627c06999be367248e06)\\n ;\\n\\n \\n \\n \\n var circle_marker_374d6d88ba4dec97559210d7e95bc2a6 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d09f8a422c3aa082b49405b454a4dadc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8dbe79ff81ea9cf20cdde035a6e4748 = $(`<div id="html_f8dbe79ff81ea9cf20cdde035a6e4748" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d09f8a422c3aa082b49405b454a4dadc.setContent(html_f8dbe79ff81ea9cf20cdde035a6e4748);\\n \\n \\n\\n circle_marker_374d6d88ba4dec97559210d7e95bc2a6.bindPopup(popup_d09f8a422c3aa082b49405b454a4dadc)\\n ;\\n\\n \\n \\n \\n var circle_marker_c43a38dd4045143e2f58dd0ee947b937 = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_e45429e07e35981bd2ef933af44fa4b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e95b4866cb88abc1966892fb6a49f35 = $(`<div id="html_2e95b4866cb88abc1966892fb6a49f35" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e45429e07e35981bd2ef933af44fa4b5.setContent(html_2e95b4866cb88abc1966892fb6a49f35);\\n \\n \\n\\n circle_marker_c43a38dd4045143e2f58dd0ee947b937.bindPopup(popup_e45429e07e35981bd2ef933af44fa4b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_4415a427f071a7f71c4ed2334c6c77cc = L.circleMarker(\\n [42.73093113915656, -73.22796888742165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_db1775a9d949752a1869e85fc85e2fc4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74de617b506134632bd5673d9c5a5417 = $(`<div id="html_74de617b506134632bd5673d9c5a5417" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db1775a9d949752a1869e85fc85e2fc4.setContent(html_74de617b506134632bd5673d9c5a5417);\\n \\n \\n\\n circle_marker_4415a427f071a7f71c4ed2334c6c77cc.bindPopup(popup_db1775a9d949752a1869e85fc85e2fc4)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a0b29786f5ec93ab0098343fc6bc877 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6f050b02dfe305b74d170bcbd037c999 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ce9ba3436172b53cda4c6fe77e33834 = $(`<div id="html_4ce9ba3436172b53cda4c6fe77e33834" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f050b02dfe305b74d170bcbd037c999.setContent(html_4ce9ba3436172b53cda4c6fe77e33834);\\n \\n \\n\\n circle_marker_5a0b29786f5ec93ab0098343fc6bc877.bindPopup(popup_6f050b02dfe305b74d170bcbd037c999)\\n ;\\n\\n \\n \\n \\n var circle_marker_2eb486ea76d6461ae01bca9b147fffc8 = L.circleMarker(\\n [42.73093032286755, -73.22550869120535],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_8e0a15a5f4a99659584bf59c7caa10fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4bb963abc18ec96da477a78cf744610b = $(`<div id="html_4bb963abc18ec96da477a78cf744610b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e0a15a5f4a99659584bf59c7caa10fb.setContent(html_4bb963abc18ec96da477a78cf744610b);\\n \\n \\n\\n circle_marker_2eb486ea76d6461ae01bca9b147fffc8.bindPopup(popup_8e0a15a5f4a99659584bf59c7caa10fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_66f1a65180b935474eaf7f5b03f0479f = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_09131e1fb6028d2f44d96a9c8691e645 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_269f45d2a7fcaeb36fc277410cbf3216 = $(`<div id="html_269f45d2a7fcaeb36fc277410cbf3216" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09131e1fb6028d2f44d96a9c8691e645.setContent(html_269f45d2a7fcaeb36fc277410cbf3216);\\n \\n \\n\\n circle_marker_66f1a65180b935474eaf7f5b03f0479f.bindPopup(popup_09131e1fb6028d2f44d96a9c8691e645)\\n ;\\n\\n \\n \\n \\n var circle_marker_1f71ce1240b0fa5d259b4805a5d819f7 = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4cc857b8f39ff46ba0299329cab64482 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_739ebd8d1354b9c73fa8f7d67855c8ef = $(`<div id="html_739ebd8d1354b9c73fa8f7d67855c8ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4cc857b8f39ff46ba0299329cab64482.setContent(html_739ebd8d1354b9c73fa8f7d67855c8ef);\\n \\n \\n\\n circle_marker_1f71ce1240b0fa5d259b4805a5d819f7.bindPopup(popup_4cc857b8f39ff46ba0299329cab64482)\\n ;\\n\\n \\n \\n \\n var circle_marker_58f130d97ec45376731600fe15d5aca6 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6340adf0cb880f7fa2d683368a9de0d6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d30a3afb27b7b2e27992f6404864da89 = $(`<div id="html_d30a3afb27b7b2e27992f6404864da89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6340adf0cb880f7fa2d683368a9de0d6.setContent(html_d30a3afb27b7b2e27992f6404864da89);\\n \\n \\n\\n circle_marker_58f130d97ec45376731600fe15d5aca6.bindPopup(popup_6340adf0cb880f7fa2d683368a9de0d6)\\n ;\\n\\n \\n \\n \\n var circle_marker_30624b8b2c897e1065a5c513bb786b11 = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d1ff0d91db499e898081b3997646943d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e497ef5f30d8d42053c2cd17054aad08 = $(`<div id="html_e497ef5f30d8d42053c2cd17054aad08" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d1ff0d91db499e898081b3997646943d.setContent(html_e497ef5f30d8d42053c2cd17054aad08);\\n \\n \\n\\n circle_marker_30624b8b2c897e1065a5c513bb786b11.bindPopup(popup_d1ff0d91db499e898081b3997646943d)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba381d23761cfaca288744856bdefbd9 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_192695d0e9999531bdf117990906ae95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1148bce1c764ac4f4e56fdbbe88e0f8d = $(`<div id="html_1148bce1c764ac4f4e56fdbbe88e0f8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_192695d0e9999531bdf117990906ae95.setContent(html_1148bce1c764ac4f4e56fdbbe88e0f8d);\\n \\n \\n\\n circle_marker_ba381d23761cfaca288744856bdefbd9.bindPopup(popup_192695d0e9999531bdf117990906ae95)\\n ;\\n\\n \\n \\n \\n var circle_marker_e98ff45f5309e6b1c1248fb771ac75ef = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_8d10902fb1e1c050eacaa7204f636a6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe25fd453d9b503c965aa4ee57d3640f = $(`<div id="html_fe25fd453d9b503c965aa4ee57d3640f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d10902fb1e1c050eacaa7204f636a6a.setContent(html_fe25fd453d9b503c965aa4ee57d3640f);\\n \\n \\n\\n circle_marker_e98ff45f5309e6b1c1248fb771ac75ef.bindPopup(popup_8d10902fb1e1c050eacaa7204f636a6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_9aafea05d7c34107ed3cc56c4712e1dc = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_5418477aa26ea2b4967ebd4eccf58dbe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_79bd0652038afb23b0e70803b4fa196c = $(`<div id="html_79bd0652038afb23b0e70803b4fa196c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5418477aa26ea2b4967ebd4eccf58dbe.setContent(html_79bd0652038afb23b0e70803b4fa196c);\\n \\n \\n\\n circle_marker_9aafea05d7c34107ed3cc56c4712e1dc.bindPopup(popup_5418477aa26ea2b4967ebd4eccf58dbe)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5e7ad85540ee52218c0028896e50f59 = L.circleMarker(\\n [42.72912990413034, -73.26733196087964],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_5f1f31f751b0c3f7bf2bd5146c972400 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a1cc9c2aaf91e396f88e051222988fe = $(`<div id="html_7a1cc9c2aaf91e396f88e051222988fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f1f31f751b0c3f7bf2bd5146c972400.setContent(html_7a1cc9c2aaf91e396f88e051222988fe);\\n \\n \\n\\n circle_marker_b5e7ad85540ee52218c0028896e50f59.bindPopup(popup_5f1f31f751b0c3f7bf2bd5146c972400)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d6d007cb94d7acec6e9f5737450cc94 = L.circleMarker(\\n [42.72912993046118, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f2999c679867bf848ea8d7f9c36266a7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2300bd463d23e71345b1360dd092ad04 = $(`<div id="html_2300bd463d23e71345b1360dd092ad04" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2999c679867bf848ea8d7f9c36266a7.setContent(html_2300bd463d23e71345b1360dd092ad04);\\n \\n \\n\\n circle_marker_9d6d007cb94d7acec6e9f5737450cc94.bindPopup(popup_f2999c679867bf848ea8d7f9c36266a7)\\n ;\\n\\n \\n \\n \\n var circle_marker_522586275ce32b0e7246b534461421be = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_307b4cc467611141b818c0fc2a9a45a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_75101c7c5142dccd9c5a06573b955ff7 = $(`<div id="html_75101c7c5142dccd9c5a06573b955ff7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_307b4cc467611141b818c0fc2a9a45a5.setContent(html_75101c7c5142dccd9c5a06573b955ff7);\\n \\n \\n\\n circle_marker_522586275ce32b0e7246b534461421be.bindPopup(popup_307b4cc467611141b818c0fc2a9a45a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_28c175959858ffdd1ab14e089bbbd488 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_726d54f544227a5253f8fd0efe45ac87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e2557ffd128b7f09563754a87b0f9a2 = $(`<div id="html_4e2557ffd128b7f09563754a87b0f9a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_726d54f544227a5253f8fd0efe45ac87.setContent(html_4e2557ffd128b7f09563754a87b0f9a2);\\n \\n \\n\\n circle_marker_28c175959858ffdd1ab14e089bbbd488.bindPopup(popup_726d54f544227a5253f8fd0efe45ac87)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf194ad1470e2a325a7241d00664f721 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_391099567bddb0e4f1c877de0d3c8abc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1a35bc10ffc99062bee6b318279103e = $(`<div id="html_a1a35bc10ffc99062bee6b318279103e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_391099567bddb0e4f1c877de0d3c8abc.setContent(html_a1a35bc10ffc99062bee6b318279103e);\\n \\n \\n\\n circle_marker_bf194ad1470e2a325a7241d00664f721.bindPopup(popup_391099567bddb0e4f1c877de0d3c8abc)\\n ;\\n\\n \\n \\n \\n var circle_marker_beadc6fbd8d546b8c9bef66c67cf4608 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_5e4607e82d3fd50bc725e272ec12ce35 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56e1d7a2bd77a7e934a1230be1ac46e8 = $(`<div id="html_56e1d7a2bd77a7e934a1230be1ac46e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e4607e82d3fd50bc725e272ec12ce35.setContent(html_56e1d7a2bd77a7e934a1230be1ac46e8);\\n \\n \\n\\n circle_marker_beadc6fbd8d546b8c9bef66c67cf4608.bindPopup(popup_5e4607e82d3fd50bc725e272ec12ce35)\\n ;\\n\\n \\n \\n \\n var circle_marker_324b366c84c9f106e85f715199b225db = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ca0ee2f8dcfafc83b3042bc2ecef5d5d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a33835dc68c0cd27034bd4096465d54a = $(`<div id="html_a33835dc68c0cd27034bd4096465d54a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca0ee2f8dcfafc83b3042bc2ecef5d5d.setContent(html_a33835dc68c0cd27034bd4096465d54a);\\n \\n \\n\\n circle_marker_324b366c84c9f106e85f715199b225db.bindPopup(popup_ca0ee2f8dcfafc83b3042bc2ecef5d5d)\\n ;\\n\\n \\n \\n \\n var circle_marker_1fb0d693dc1d0e3ab59abfcd7ca98f0b = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_611d623614e0c2283580d41db457b04c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_122535136da46ed20c381b72a96c65e7 = $(`<div id="html_122535136da46ed20c381b72a96c65e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_611d623614e0c2283580d41db457b04c.setContent(html_122535136da46ed20c381b72a96c65e7);\\n \\n \\n\\n circle_marker_1fb0d693dc1d0e3ab59abfcd7ca98f0b.bindPopup(popup_611d623614e0c2283580d41db457b04c)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0675ca818961aef1e9ef63e7efe374c = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_79bcd8a3593013ec90a0c54842e9124b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_305dcf9f22f1c552875c188426ac264e = $(`<div id="html_305dcf9f22f1c552875c188426ac264e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79bcd8a3593013ec90a0c54842e9124b.setContent(html_305dcf9f22f1c552875c188426ac264e);\\n \\n \\n\\n circle_marker_d0675ca818961aef1e9ef63e7efe374c.bindPopup(popup_79bcd8a3593013ec90a0c54842e9124b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3395e2d7744b2aec391a896f87132e67 = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_83a00355ea06415a8ac10b0babc0160a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_171ab4696f3225971fcf73acb7d61d5e = $(`<div id="html_171ab4696f3225971fcf73acb7d61d5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83a00355ea06415a8ac10b0babc0160a.setContent(html_171ab4696f3225971fcf73acb7d61d5e);\\n \\n \\n\\n circle_marker_3395e2d7744b2aec391a896f87132e67.bindPopup(popup_83a00355ea06415a8ac10b0babc0160a)\\n ;\\n\\n \\n \\n \\n var circle_marker_1dace7ac50d2519731608ff093059e53 = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4e0ff1385b11c7fab7bb32db30810b4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5de8493a075ab7ef3d7b5d71b7621a0a = $(`<div id="html_5de8493a075ab7ef3d7b5d71b7621a0a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e0ff1385b11c7fab7bb32db30810b4b.setContent(html_5de8493a075ab7ef3d7b5d71b7621a0a);\\n \\n \\n\\n circle_marker_1dace7ac50d2519731608ff093059e53.bindPopup(popup_4e0ff1385b11c7fab7bb32db30810b4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_5da3a2bd3cb6f97704bc870698578e7b = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_e46168a1c73092d6ef145b7875c738c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6a230e4c78068888827b5ac7d139c27 = $(`<div id="html_c6a230e4c78068888827b5ac7d139c27" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e46168a1c73092d6ef145b7875c738c4.setContent(html_c6a230e4c78068888827b5ac7d139c27);\\n \\n \\n\\n circle_marker_5da3a2bd3cb6f97704bc870698578e7b.bindPopup(popup_e46168a1c73092d6ef145b7875c738c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_652d38ea154e752dea460f3742befb36 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ef102ff73c1df5ce0feebe59a121f8a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c684366b09806d4dbbaeee72121c1ab7 = $(`<div id="html_c684366b09806d4dbbaeee72121c1ab7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef102ff73c1df5ce0feebe59a121f8a5.setContent(html_c684366b09806d4dbbaeee72121c1ab7);\\n \\n \\n\\n circle_marker_652d38ea154e752dea460f3742befb36.bindPopup(popup_ef102ff73c1df5ce0feebe59a121f8a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_967b752f49ae9377f06019a51e3cba9f = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_bb8bcc1cd2c2c5b527d08b944b9df73d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49df2124639be6bd9d2d1d7da2d4076a = $(`<div id="html_49df2124639be6bd9d2d1d7da2d4076a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb8bcc1cd2c2c5b527d08b944b9df73d.setContent(html_49df2124639be6bd9d2d1d7da2d4076a);\\n \\n \\n\\n circle_marker_967b752f49ae9377f06019a51e3cba9f.bindPopup(popup_bb8bcc1cd2c2c5b527d08b944b9df73d)\\n ;\\n\\n \\n \\n \\n var circle_marker_1225449e9d3119b2b10656ecb7c2b41a = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_91bbf2900fa715a8539651514792c3b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_637d54cc204ca54864e744deb11c7e09 = $(`<div id="html_637d54cc204ca54864e744deb11c7e09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91bbf2900fa715a8539651514792c3b8.setContent(html_637d54cc204ca54864e744deb11c7e09);\\n \\n \\n\\n circle_marker_1225449e9d3119b2b10656ecb7c2b41a.bindPopup(popup_91bbf2900fa715a8539651514792c3b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a5a2ded6d2000777c4a344e596eb517 = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ecd51b6529870d6491dcf1a5d84b7653 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b4268458b38be3f186c133f7f1fd23b = $(`<div id="html_2b4268458b38be3f186c133f7f1fd23b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ecd51b6529870d6491dcf1a5d84b7653.setContent(html_2b4268458b38be3f186c133f7f1fd23b);\\n \\n \\n\\n circle_marker_5a5a2ded6d2000777c4a344e596eb517.bindPopup(popup_ecd51b6529870d6491dcf1a5d84b7653)\\n ;\\n\\n \\n \\n \\n var circle_marker_3bec9c39e42cfe63aceea8228684dcbd = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_737b28ca500582fe272600a228dab10c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad37c35222a4fbeef28e1e787ce29269 = $(`<div id="html_ad37c35222a4fbeef28e1e787ce29269" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_737b28ca500582fe272600a228dab10c.setContent(html_ad37c35222a4fbeef28e1e787ce29269);\\n \\n \\n\\n circle_marker_3bec9c39e42cfe63aceea8228684dcbd.bindPopup(popup_737b28ca500582fe272600a228dab10c)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f7209aa6e5e8019dbe4e19e358d443d = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_edb529705e49504669e6c3cb37dd8087 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_47ede430a5241713871c25732750ca34 = $(`<div id="html_47ede430a5241713871c25732750ca34" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_edb529705e49504669e6c3cb37dd8087.setContent(html_47ede430a5241713871c25732750ca34);\\n \\n \\n\\n circle_marker_9f7209aa6e5e8019dbe4e19e358d443d.bindPopup(popup_edb529705e49504669e6c3cb37dd8087)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e04568d2a74932beeb52c41e0a6b290 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_70628fdf73ee6b133c38bc2da33d85a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bbebcd2aef4bb89f347840804b694f9c = $(`<div id="html_bbebcd2aef4bb89f347840804b694f9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_70628fdf73ee6b133c38bc2da33d85a4.setContent(html_bbebcd2aef4bb89f347840804b694f9c);\\n \\n \\n\\n circle_marker_9e04568d2a74932beeb52c41e0a6b290.bindPopup(popup_70628fdf73ee6b133c38bc2da33d85a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_abc8802c7899dffe1801348d7a96f7c0 = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_810dd01853a5ddf76908c76ed3a7124d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_becdb42514fe4d828a2734e02aae029f = $(`<div id="html_becdb42514fe4d828a2734e02aae029f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_810dd01853a5ddf76908c76ed3a7124d.setContent(html_becdb42514fe4d828a2734e02aae029f);\\n \\n \\n\\n circle_marker_abc8802c7899dffe1801348d7a96f7c0.bindPopup(popup_810dd01853a5ddf76908c76ed3a7124d)\\n ;\\n\\n \\n \\n \\n var circle_marker_51cf7a653675dde2e287439a5a1382e5 = L.circleMarker(\\n [42.72732247454739, -73.27348202255521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c305abab5c0b5668d32a7b9d0f065ea8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd01f758d3022e6204527e5537d50994 = $(`<div id="html_bd01f758d3022e6204527e5537d50994" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c305abab5c0b5668d32a7b9d0f065ea8.setContent(html_bd01f758d3022e6204527e5537d50994);\\n \\n \\n\\n circle_marker_51cf7a653675dde2e287439a5a1382e5.bindPopup(popup_c305abab5c0b5668d32a7b9d0f065ea8)\\n ;\\n\\n \\n \\n \\n var circle_marker_c9fafb0c1dcf8d90c0f35cca1ec6ab0a = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_bca9ecd2bf252f2885479c10de57df23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3320d7e8eda0a0be9ead2b0022bee353 = $(`<div id="html_3320d7e8eda0a0be9ead2b0022bee353" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bca9ecd2bf252f2885479c10de57df23.setContent(html_3320d7e8eda0a0be9ead2b0022bee353);\\n \\n \\n\\n circle_marker_c9fafb0c1dcf8d90c0f35cca1ec6ab0a.bindPopup(popup_bca9ecd2bf252f2885479c10de57df23)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fa5e8efa53b48f14a3d59a84fb74bff = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4e13ae3becabfb09a8b9dd4f851d2ec5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bde4b0f4abb6f6879d5b90709f6de724 = $(`<div id="html_bde4b0f4abb6f6879d5b90709f6de724" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e13ae3becabfb09a8b9dd4f851d2ec5.setContent(html_bde4b0f4abb6f6879d5b90709f6de724);\\n \\n \\n\\n circle_marker_9fa5e8efa53b48f14a3d59a84fb74bff.bindPopup(popup_4e13ae3becabfb09a8b9dd4f851d2ec5)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9c25cdfab0111da69e66e8394c912b3 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_8168532e18539c6892f4a8318d67f143 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cae5dad668683e5683f2ba6679eafb8b = $(`<div id="html_cae5dad668683e5683f2ba6679eafb8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8168532e18539c6892f4a8318d67f143.setContent(html_cae5dad668683e5683f2ba6679eafb8b);\\n \\n \\n\\n circle_marker_e9c25cdfab0111da69e66e8394c912b3.bindPopup(popup_8168532e18539c6892f4a8318d67f143)\\n ;\\n\\n \\n \\n \\n var circle_marker_29dacf0f5a764bf3ea2bffafe87eade3 = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_560f2cd5c9d37f444866e12cc59e001f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_686eca0ca90cff316d05aef37888484a = $(`<div id="html_686eca0ca90cff316d05aef37888484a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_560f2cd5c9d37f444866e12cc59e001f.setContent(html_686eca0ca90cff316d05aef37888484a);\\n \\n \\n\\n circle_marker_29dacf0f5a764bf3ea2bffafe87eade3.bindPopup(popup_560f2cd5c9d37f444866e12cc59e001f)\\n ;\\n\\n \\n \\n \\n var circle_marker_40d3b42383081273f9902b0d470bb5c9 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f1a5365704a604b71db3a2128457b299 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5569edbb44a6d8d5fc400f84f25b2d8b = $(`<div id="html_5569edbb44a6d8d5fc400f84f25b2d8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1a5365704a604b71db3a2128457b299.setContent(html_5569edbb44a6d8d5fc400f84f25b2d8b);\\n \\n \\n\\n circle_marker_40d3b42383081273f9902b0d470bb5c9.bindPopup(popup_f1a5365704a604b71db3a2128457b299)\\n ;\\n\\n \\n \\n \\n var circle_marker_49427b09f8e1579c587ab4c839080ec5 = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d534d59ac797012593001cd79ff42bb6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5cae8d1be8d886dd249c54674960f993 = $(`<div id="html_5cae8d1be8d886dd249c54674960f993" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d534d59ac797012593001cd79ff42bb6.setContent(html_5cae8d1be8d886dd249c54674960f993);\\n \\n \\n\\n circle_marker_49427b09f8e1579c587ab4c839080ec5.bindPopup(popup_d534d59ac797012593001cd79ff42bb6)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fa9a46eff36a75ea695eafe11f74c23 = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_759e8c81dd1f4e6d60465f36a9ef5ce0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_feced49e0d793a62eb500b88d32018da = $(`<div id="html_feced49e0d793a62eb500b88d32018da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_759e8c81dd1f4e6d60465f36a9ef5ce0.setContent(html_feced49e0d793a62eb500b88d32018da);\\n \\n \\n\\n circle_marker_3fa9a46eff36a75ea695eafe11f74c23.bindPopup(popup_759e8c81dd1f4e6d60465f36a9ef5ce0)\\n ;\\n\\n \\n \\n \\n var circle_marker_333b8c09a65f47163d707bb0effac5ef = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_014df2623bb575b64b94fb21145f06e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dcb6f6eacad56e9c796f26ffe902f4b2 = $(`<div id="html_dcb6f6eacad56e9c796f26ffe902f4b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_014df2623bb575b64b94fb21145f06e0.setContent(html_dcb6f6eacad56e9c796f26ffe902f4b2);\\n \\n \\n\\n circle_marker_333b8c09a65f47163d707bb0effac5ef.bindPopup(popup_014df2623bb575b64b94fb21145f06e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_354f8d4f474b5c73f10015e716abd4ae = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a814949a2724322c523bfd3b227c34d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8f21041d135433e922963206332afdc = $(`<div id="html_f8f21041d135433e922963206332afdc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a814949a2724322c523bfd3b227c34d5.setContent(html_f8f21041d135433e922963206332afdc);\\n \\n \\n\\n circle_marker_354f8d4f474b5c73f10015e716abd4ae.bindPopup(popup_a814949a2724322c523bfd3b227c34d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a62da47cfa6282a435a625fd84564d5f = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_043f612e33d8346a4c15c2642e342889 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b06851823a0f0a6ceac0b8d6ea4e1968 = $(`<div id="html_b06851823a0f0a6ceac0b8d6ea4e1968" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_043f612e33d8346a4c15c2642e342889.setContent(html_b06851823a0f0a6ceac0b8d6ea4e1968);\\n \\n \\n\\n circle_marker_a62da47cfa6282a435a625fd84564d5f.bindPopup(popup_043f612e33d8346a4c15c2642e342889)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee672f97169cbb9899ce16249e9419f5 = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_93652c7fb332669167dbf0e23c9b3540 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e38f7654360c3738f476aa6c6f6fbc87 = $(`<div id="html_e38f7654360c3738f476aa6c6f6fbc87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93652c7fb332669167dbf0e23c9b3540.setContent(html_e38f7654360c3738f476aa6c6f6fbc87);\\n \\n \\n\\n circle_marker_ee672f97169cbb9899ce16249e9419f5.bindPopup(popup_93652c7fb332669167dbf0e23c9b3540)\\n ;\\n\\n \\n \\n \\n var circle_marker_55a7011bce9495349b7659742cbb9295 = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_efb7bc766f2ed122c6996a1b049221be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2f227756ee922579eeeda517d7b2e159 = $(`<div id="html_2f227756ee922579eeeda517d7b2e159" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_efb7bc766f2ed122c6996a1b049221be.setContent(html_2f227756ee922579eeeda517d7b2e159);\\n \\n \\n\\n circle_marker_55a7011bce9495349b7659742cbb9295.bindPopup(popup_efb7bc766f2ed122c6996a1b049221be)\\n ;\\n\\n \\n \\n \\n var circle_marker_3907ccc8798491379f61f9c61a50019d = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_dc27882e20087006459e56e66ef673fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8889f1f95db47340889743e86b2bc829 = $(`<div id="html_8889f1f95db47340889743e86b2bc829" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc27882e20087006459e56e66ef673fd.setContent(html_8889f1f95db47340889743e86b2bc829);\\n \\n \\n\\n circle_marker_3907ccc8798491379f61f9c61a50019d.bindPopup(popup_dc27882e20087006459e56e66ef673fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_c649f22b2969f080c002fc7af55d5551 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_85a2b4364c1800302570339a7639c8e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd44702b2333d408a9bd808f77ff6583 = $(`<div id="html_dd44702b2333d408a9bd808f77ff6583" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_85a2b4364c1800302570339a7639c8e3.setContent(html_dd44702b2333d408a9bd808f77ff6583);\\n \\n \\n\\n circle_marker_c649f22b2969f080c002fc7af55d5551.bindPopup(popup_85a2b4364c1800302570339a7639c8e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c7846d9e2fd8e9aabedd833e76e3178 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_df8f1a2d5621fa3e3d0d533ea1feaa87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49e7a030a9757e4ee20fb2f026d1659c = $(`<div id="html_49e7a030a9757e4ee20fb2f026d1659c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df8f1a2d5621fa3e3d0d533ea1feaa87.setContent(html_49e7a030a9757e4ee20fb2f026d1659c);\\n \\n \\n\\n circle_marker_8c7846d9e2fd8e9aabedd833e76e3178.bindPopup(popup_df8f1a2d5621fa3e3d0d533ea1feaa87)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5d6f51dfbec56b56538789b8d540eb1 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c830487fdeae6967f5a18b5194f1d9ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39b43eebe427185b74a63ca95cfb33db = $(`<div id="html_39b43eebe427185b74a63ca95cfb33db" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c830487fdeae6967f5a18b5194f1d9ba.setContent(html_39b43eebe427185b74a63ca95cfb33db);\\n \\n \\n\\n circle_marker_b5d6f51dfbec56b56538789b8d540eb1.bindPopup(popup_c830487fdeae6967f5a18b5194f1d9ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a4cb8d182f8d0a5ea1c6f5665e7d672 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_e8d240118b8981c158795356bf35e590 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df9cc3228a5062e22875cd8d8e3cd6a3 = $(`<div id="html_df9cc3228a5062e22875cd8d8e3cd6a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8d240118b8981c158795356bf35e590.setContent(html_df9cc3228a5062e22875cd8d8e3cd6a3);\\n \\n \\n\\n circle_marker_0a4cb8d182f8d0a5ea1c6f5665e7d672.bindPopup(popup_e8d240118b8981c158795356bf35e590)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b8191b1101c3133dc5af4c00fc144f7 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_81916d47967fb95eb80d940fcddf5111 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b990ca36bc77d3b385740a734aa4abfd = $(`<div id="html_b990ca36bc77d3b385740a734aa4abfd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81916d47967fb95eb80d940fcddf5111.setContent(html_b990ca36bc77d3b385740a734aa4abfd);\\n \\n \\n\\n circle_marker_1b8191b1101c3133dc5af4c00fc144f7.bindPopup(popup_81916d47967fb95eb80d940fcddf5111)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e831cba889e4e18092709024c889c9c = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4ed59d43e0573d401aeebb9e3f7321eb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2aabbe168b1adc80281aa42a525cd3e3 = $(`<div id="html_2aabbe168b1adc80281aa42a525cd3e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ed59d43e0573d401aeebb9e3f7321eb.setContent(html_2aabbe168b1adc80281aa42a525cd3e3);\\n \\n \\n\\n circle_marker_2e831cba889e4e18092709024c889c9c.bindPopup(popup_4ed59d43e0573d401aeebb9e3f7321eb)\\n ;\\n\\n \\n \\n \\n var circle_marker_35fd295466b4df51077f6a806011bbc0 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c626a5c0b216dac79cf59c34aadfcbe1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6aa1efc3b7546dfbd0139a4321072c9 = $(`<div id="html_f6aa1efc3b7546dfbd0139a4321072c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c626a5c0b216dac79cf59c34aadfcbe1.setContent(html_f6aa1efc3b7546dfbd0139a4321072c9);\\n \\n \\n\\n circle_marker_35fd295466b4df51077f6a806011bbc0.bindPopup(popup_c626a5c0b216dac79cf59c34aadfcbe1)\\n ;\\n\\n \\n \\n \\n var circle_marker_6cf47c0e3f51013ecf1ab5926d09005a = L.circleMarker(\\n [42.72731562880686, -73.22428095863906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_d83a3206413507546cb8791049ea61bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d9018dbab7faa447c378a2e0268189f = $(`<div id="html_4d9018dbab7faa447c378a2e0268189f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d83a3206413507546cb8791049ea61bd.setContent(html_4d9018dbab7faa447c378a2e0268189f);\\n \\n \\n\\n circle_marker_6cf47c0e3f51013ecf1ab5926d09005a.bindPopup(popup_d83a3206413507546cb8791049ea61bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_8fa69ef4098efef6a93e6572e1fb6dd0 = L.circleMarker(\\n [42.72731518778323, -73.22305093225523],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_e09e2c1d9d7176080fc219b5a11a4094 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a23b52ea82a29929293fcc99ea268ee = $(`<div id="html_6a23b52ea82a29929293fcc99ea268ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e09e2c1d9d7176080fc219b5a11a4094.setContent(html_6a23b52ea82a29929293fcc99ea268ee);\\n \\n \\n\\n circle_marker_8fa69ef4098efef6a93e6572e1fb6dd0.bindPopup(popup_e09e2c1d9d7176080fc219b5a11a4094)\\n ;\\n\\n \\n \\n \\n var circle_marker_ac5489b7cc3ffffdbe60198eba1ea5b2 = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3abf6823e1fe5566df3c2b6ed3850054 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b8c4e71a1fddd42d758919632a3d3b2 = $(`<div id="html_1b8c4e71a1fddd42d758919632a3d3b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3abf6823e1fe5566df3c2b6ed3850054.setContent(html_1b8c4e71a1fddd42d758919632a3d3b2);\\n \\n \\n\\n circle_marker_ac5489b7cc3ffffdbe60198eba1ea5b2.bindPopup(popup_3abf6823e1fe5566df3c2b6ed3850054)\\n ;\\n\\n \\n \\n \\n var circle_marker_997b6b89959fdf91af05dc328c074d82 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_cab04093a91723167fa240eac5b1561e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_696d8e7e19cb25c8b19d05097ef9cd85 = $(`<div id="html_696d8e7e19cb25c8b19d05097ef9cd85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cab04093a91723167fa240eac5b1561e.setContent(html_696d8e7e19cb25c8b19d05097ef9cd85);\\n \\n \\n\\n circle_marker_997b6b89959fdf91af05dc328c074d82.bindPopup(popup_cab04093a91723167fa240eac5b1561e)\\n ;\\n\\n \\n \\n \\n var circle_marker_9137ddc7ca63e229aa3506f6932d6b01 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_2d87cc3551e1b7b1e954a0d8176f9df7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9624a29cd2d60929fa54c5c29dba4e95 = $(`<div id="html_9624a29cd2d60929fa54c5c29dba4e95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d87cc3551e1b7b1e954a0d8176f9df7.setContent(html_9624a29cd2d60929fa54c5c29dba4e95);\\n \\n \\n\\n circle_marker_9137ddc7ca63e229aa3506f6932d6b01.bindPopup(popup_2d87cc3551e1b7b1e954a0d8176f9df7)\\n ;\\n\\n \\n \\n \\n var circle_marker_2bf00644e2c1dffc615a7f933b5a807c = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f75810597ecb9ed769560699ddd4f4f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9be6049bf01a80a00f5fab36b94dad5 = $(`<div id="html_f9be6049bf01a80a00f5fab36b94dad5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f75810597ecb9ed769560699ddd4f4f1.setContent(html_f9be6049bf01a80a00f5fab36b94dad5);\\n \\n \\n\\n circle_marker_2bf00644e2c1dffc615a7f933b5a807c.bindPopup(popup_f75810597ecb9ed769560699ddd4f4f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_e49ed8b8a75ec5ee8900166361591e61 = L.circleMarker(\\n [42.725515657130885, -73.26610182668713],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_698b2fcc9527baa1f92fcaaa48b096b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c9d9ecfab30aa0bd4d00f1aa6628903 = $(`<div id="html_8c9d9ecfab30aa0bd4d00f1aa6628903" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_698b2fcc9527baa1f92fcaaa48b096b3.setContent(html_8c9d9ecfab30aa0bd4d00f1aa6628903);\\n \\n \\n\\n circle_marker_e49ed8b8a75ec5ee8900166361591e61.bindPopup(popup_698b2fcc9527baa1f92fcaaa48b096b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_42fc17097c0bc38e7954e7d7ca22c095 = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_0e26de3d8ac743584054fac9f55fbf9d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1d97c499bf7c167b3eed1858da02561 = $(`<div id="html_c1d97c499bf7c167b3eed1858da02561" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e26de3d8ac743584054fac9f55fbf9d.setContent(html_c1d97c499bf7c167b3eed1858da02561);\\n \\n \\n\\n circle_marker_42fc17097c0bc38e7954e7d7ca22c095.bindPopup(popup_0e26de3d8ac743584054fac9f55fbf9d)\\n ;\\n\\n \\n \\n \\n var circle_marker_667f067aab2a295d0dc4c2a1de5ad562 = L.circleMarker(\\n [42.72551560447347, -73.26118186332953],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_eba0567b933f2e16c516e45d44af897d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c1c6dac2f2d5dc4c4486da1041acfa9 = $(`<div id="html_1c1c6dac2f2d5dc4c4486da1041acfa9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eba0567b933f2e16c516e45d44af897d.setContent(html_1c1c6dac2f2d5dc4c4486da1041acfa9);\\n \\n \\n\\n circle_marker_667f067aab2a295d0dc4c2a1de5ad562.bindPopup(popup_eba0567b933f2e16c516e45d44af897d)\\n ;\\n\\n \\n \\n \\n var circle_marker_76cca6d416e22575806380ec39aab5dd = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ce27aa677d6ef4ce80ac39a90fd4a83c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0595ea10c63c35cc6105f4c8b198d94b = $(`<div id="html_0595ea10c63c35cc6105f4c8b198d94b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce27aa677d6ef4ce80ac39a90fd4a83c.setContent(html_0595ea10c63c35cc6105f4c8b198d94b);\\n \\n \\n\\n circle_marker_76cca6d416e22575806380ec39aab5dd.bindPopup(popup_ce27aa677d6ef4ce80ac39a90fd4a83c)\\n ;\\n\\n \\n \\n \\n var circle_marker_167192b914ad0361d85090097a5d2c4b = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c9bc9bcfb1ada964cb23507a4fd2e07b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a67eab291390518294f6a65908b1f24 = $(`<div id="html_5a67eab291390518294f6a65908b1f24" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9bc9bcfb1ada964cb23507a4fd2e07b.setContent(html_5a67eab291390518294f6a65908b1f24);\\n \\n \\n\\n circle_marker_167192b914ad0361d85090097a5d2c4b.bindPopup(popup_c9bc9bcfb1ada964cb23507a4fd2e07b)\\n ;\\n\\n \\n \\n \\n var circle_marker_b8bbd708f9e55f39c23ce2fa9650fd1e = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_0588685c4234c8ca319532708f34ed9e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_71fec5bc3451fbcfdc4b7c457ea593e9 = $(`<div id="html_71fec5bc3451fbcfdc4b7c457ea593e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0588685c4234c8ca319532708f34ed9e.setContent(html_71fec5bc3451fbcfdc4b7c457ea593e9);\\n \\n \\n\\n circle_marker_b8bbd708f9e55f39c23ce2fa9650fd1e.bindPopup(popup_0588685c4234c8ca319532708f34ed9e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e167c90f3628312739771f39cf26e0ad = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f054d7767beffba7482958f33dd5ae3c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b00cecc0245fa7e10404255263efcd2 = $(`<div id="html_9b00cecc0245fa7e10404255263efcd2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f054d7767beffba7482958f33dd5ae3c.setContent(html_9b00cecc0245fa7e10404255263efcd2);\\n \\n \\n\\n circle_marker_e167c90f3628312739771f39cf26e0ad.bindPopup(popup_f054d7767beffba7482958f33dd5ae3c)\\n ;\\n\\n \\n \\n \\n var circle_marker_bd3f64e87443365aed4e7171d21013d5 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f24b484efbd30d2b5cd31c8a91592ae1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7396be0cf8c7df9daf07c8e694983cfa = $(`<div id="html_7396be0cf8c7df9daf07c8e694983cfa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f24b484efbd30d2b5cd31c8a91592ae1.setContent(html_7396be0cf8c7df9daf07c8e694983cfa);\\n \\n \\n\\n circle_marker_bd3f64e87443365aed4e7171d21013d5.bindPopup(popup_f24b484efbd30d2b5cd31c8a91592ae1)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0f559e5dbaf32155cfd6dd625688066 = L.circleMarker(\\n [42.725513531087856, -73.24273200123443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_60fda49e4f377a9c46037c3338b398a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b3c57bc8d70263ab0c7a97e88acff153 = $(`<div id="html_b3c57bc8d70263ab0c7a97e88acff153" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_60fda49e4f377a9c46037c3338b398a0.setContent(html_b3c57bc8d70263ab0c7a97e88acff153);\\n \\n \\n\\n circle_marker_b0f559e5dbaf32155cfd6dd625688066.bindPopup(popup_60fda49e4f377a9c46037c3338b398a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_7bca0a5dfa3ef2542a2db4a41b9c262e = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_974c8e474f72773532b2c49aebc7dba9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f78f14574882735fac9b6f95dd534be4 = $(`<div id="html_f78f14574882735fac9b6f95dd534be4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_974c8e474f72773532b2c49aebc7dba9.setContent(html_f78f14574882735fac9b6f95dd534be4);\\n \\n \\n\\n circle_marker_7bca0a5dfa3ef2542a2db4a41b9c262e.bindPopup(popup_974c8e474f72773532b2c49aebc7dba9)\\n ;\\n\\n \\n \\n \\n var circle_marker_5086f07b628b11bdc6b27e7c1f997371 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_1283957840426e351b81152bbfbbde94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67016aadcac32d29b9550ca8803f9a88 = $(`<div id="html_67016aadcac32d29b9550ca8803f9a88" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1283957840426e351b81152bbfbbde94.setContent(html_67016aadcac32d29b9550ca8803f9a88);\\n \\n \\n\\n circle_marker_5086f07b628b11bdc6b27e7c1f997371.bindPopup(popup_1283957840426e351b81152bbfbbde94)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e6015ec7d18fd1c906be4c74cbf5ad8 = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_ad920deb7c5200636a742160dc0b47de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_947e1db5396ce0c74be631566da95409 = $(`<div id="html_947e1db5396ce0c74be631566da95409" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad920deb7c5200636a742160dc0b47de.setContent(html_947e1db5396ce0c74be631566da95409);\\n \\n \\n\\n circle_marker_4e6015ec7d18fd1c906be4c74cbf5ad8.bindPopup(popup_ad920deb7c5200636a742160dc0b47de)\\n ;\\n\\n \\n \\n \\n var circle_marker_35ed64dc662daaef6f3908e5c87a791f = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c154472f7c0c1e8477115d9b22ff547e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_420fd3a2d547b579b8d232c1f877fbaa = $(`<div id="html_420fd3a2d547b579b8d232c1f877fbaa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c154472f7c0c1e8477115d9b22ff547e.setContent(html_420fd3a2d547b579b8d232c1f877fbaa);\\n \\n \\n\\n circle_marker_35ed64dc662daaef6f3908e5c87a791f.bindPopup(popup_c154472f7c0c1e8477115d9b22ff547e)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d6f847221a18bb27611b24205342e23 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.656366395715726, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_89e2a96f2e08e49455fc879e405a06ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_80850ab438b29ce3710cdfda818814e8 = $(`<div id="html_80850ab438b29ce3710cdfda818814e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89e2a96f2e08e49455fc879e405a06ac.setContent(html_80850ab438b29ce3710cdfda818814e8);\\n \\n \\n\\n circle_marker_0d6f847221a18bb27611b24205342e23.bindPopup(popup_89e2a96f2e08e49455fc879e405a06ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_83a9e4096b5524a17ca9ece5eeabda38 = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_c732ad9c946c3d1be777cf63fe12414f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6564e525c0ed326a20da0623a261a866 = $(`<div id="html_6564e525c0ed326a20da0623a261a866" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c732ad9c946c3d1be777cf63fe12414f.setContent(html_6564e525c0ed326a20da0623a261a866);\\n \\n \\n\\n circle_marker_83a9e4096b5524a17ca9ece5eeabda38.bindPopup(popup_c732ad9c946c3d1be777cf63fe12414f)\\n ;\\n\\n \\n \\n \\n var circle_marker_c7277c6518069c805bf1b3e1ee207c9c = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_09fc670dbc7fa6583d43c3e167cc0669 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8a30dda31a1010153179586d87ebec0 = $(`<div id="html_a8a30dda31a1010153179586d87ebec0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09fc670dbc7fa6583d43c3e167cc0669.setContent(html_a8a30dda31a1010153179586d87ebec0);\\n \\n \\n\\n circle_marker_c7277c6518069c805bf1b3e1ee207c9c.bindPopup(popup_09fc670dbc7fa6583d43c3e167cc0669)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9f499840f0cfd8cd9766d05302773df = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_eba058fcd2567a4907889e2f3d2c08b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb6f2695bcd95c18745e5e2bc0cf8cae = $(`<div id="html_cb6f2695bcd95c18745e5e2bc0cf8cae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eba058fcd2567a4907889e2f3d2c08b4.setContent(html_cb6f2695bcd95c18745e5e2bc0cf8cae);\\n \\n \\n\\n circle_marker_e9f499840f0cfd8cd9766d05302773df.bindPopup(popup_eba058fcd2567a4907889e2f3d2c08b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d0bb0bfffadaa7c70adb2564f3e5c46 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_51e8ad4a8ab9cbaf2c29c0fc1d680437 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb0b088428b4b4ebd272f157c6619339 = $(`<div id="html_bb0b088428b4b4ebd272f157c6619339" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51e8ad4a8ab9cbaf2c29c0fc1d680437.setContent(html_bb0b088428b4b4ebd272f157c6619339);\\n \\n \\n\\n circle_marker_7d0bb0bfffadaa7c70adb2564f3e5c46.bindPopup(popup_51e8ad4a8ab9cbaf2c29c0fc1d680437)\\n ;\\n\\n \\n \\n \\n var circle_marker_f9c7c529154246bb4bfeb328131a6a16 = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_a3b66e14ecaa2f3f023e4dba633f22d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f5465b88087cc3ecc80e5f6be9e3434 = $(`<div id="html_4f5465b88087cc3ecc80e5f6be9e3434" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3b66e14ecaa2f3f023e4dba633f22d7.setContent(html_4f5465b88087cc3ecc80e5f6be9e3434);\\n \\n \\n\\n circle_marker_f9c7c529154246bb4bfeb328131a6a16.bindPopup(popup_a3b66e14ecaa2f3f023e4dba633f22d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_6fe91b8158b0b2c75ebc7ad7a51f00a2 = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_b43f507fdd098fe72dbefa20dea469cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3bc7085733c97f722ec567453bf12cd = $(`<div id="html_f3bc7085733c97f722ec567453bf12cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b43f507fdd098fe72dbefa20dea469cf.setContent(html_f3bc7085733c97f722ec567453bf12cd);\\n \\n \\n\\n circle_marker_6fe91b8158b0b2c75ebc7ad7a51f00a2.bindPopup(popup_b43f507fdd098fe72dbefa20dea469cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_34858d93e22f900b114a88b891e947ee = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_20dcc39bf957fc321bb45565d54e9f81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf43ca81a4e17050bdc7c85d4d1ee183 = $(`<div id="html_bf43ca81a4e17050bdc7c85d4d1ee183" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20dcc39bf957fc321bb45565d54e9f81.setContent(html_bf43ca81a4e17050bdc7c85d4d1ee183);\\n \\n \\n\\n circle_marker_34858d93e22f900b114a88b891e947ee.bindPopup(popup_20dcc39bf957fc321bb45565d54e9f81)\\n ;\\n\\n \\n \\n \\n var circle_marker_cfddae9fb406284c3e407b200f0a8dac = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_4f483803dc67cddc388a454feb30ad41 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cfe9d89dc52c6ee676abda1b2a2ecb9c = $(`<div id="html_cfe9d89dc52c6ee676abda1b2a2ecb9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f483803dc67cddc388a454feb30ad41.setContent(html_cfe9d89dc52c6ee676abda1b2a2ecb9c);\\n \\n \\n\\n circle_marker_cfddae9fb406284c3e407b200f0a8dac.bindPopup(popup_4f483803dc67cddc388a454feb30ad41)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4f53fd96e5747ddc41ec84c2ecc347a = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_fbd58849a48b70a1fb7b2424e06d5a4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d7a2a61a133b84d185a34efec41e9b1 = $(`<div id="html_3d7a2a61a133b84d185a34efec41e9b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fbd58849a48b70a1fb7b2424e06d5a4e.setContent(html_3d7a2a61a133b84d185a34efec41e9b1);\\n \\n \\n\\n circle_marker_a4f53fd96e5747ddc41ec84c2ecc347a.bindPopup(popup_fbd58849a48b70a1fb7b2424e06d5a4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_17fef50c6005a20090a0af64d43a8cb7 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_55acdaf34344b69204dd28f03c0d428a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2a2ff37efb9142dcb2a81828d988eb0 = $(`<div id="html_e2a2ff37efb9142dcb2a81828d988eb0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_55acdaf34344b69204dd28f03c0d428a.setContent(html_e2a2ff37efb9142dcb2a81828d988eb0);\\n \\n \\n\\n circle_marker_17fef50c6005a20090a0af64d43a8cb7.bindPopup(popup_55acdaf34344b69204dd28f03c0d428a)\\n ;\\n\\n \\n \\n \\n var circle_marker_43ba29a6ab5421077bc2d44b5eb79aed = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_6e84d4393087a1926f3107817f2b042b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43199a68ae837a3555ecd94e61017747 = $(`<div id="html_43199a68ae837a3555ecd94e61017747" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e84d4393087a1926f3107817f2b042b.setContent(html_43199a68ae837a3555ecd94e61017747);\\n \\n \\n\\n circle_marker_43ba29a6ab5421077bc2d44b5eb79aed.bindPopup(popup_6e84d4393087a1926f3107817f2b042b)\\n ;\\n\\n \\n \\n \\n var circle_marker_ddff3d8a6adbc80063907f94b95eeea6 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_3002018aeae7c13704c0589755f1b6ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b27af866f2fd2fc960a46e21f40c564 = $(`<div id="html_8b27af866f2fd2fc960a46e21f40c564" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3002018aeae7c13704c0589755f1b6ab.setContent(html_8b27af866f2fd2fc960a46e21f40c564);\\n \\n \\n\\n circle_marker_ddff3d8a6adbc80063907f94b95eeea6.bindPopup(popup_3002018aeae7c13704c0589755f1b6ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_703257d53764ba412dc19fdd2a479c30 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_28f1997357570575a07e8da8d4a416c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_658dbe4cccbe5ab8957f910625e4b02d = $(`<div id="html_658dbe4cccbe5ab8957f910625e4b02d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_28f1997357570575a07e8da8d4a416c8.setContent(html_658dbe4cccbe5ab8957f910625e4b02d);\\n \\n \\n\\n circle_marker_703257d53764ba412dc19fdd2a479c30.bindPopup(popup_28f1997357570575a07e8da8d4a416c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_8fcd07473a528234363bc0694c5fb91a = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_aeb4c47ecdf1032f270fe2adc8c0e511);\\n \\n \\n var popup_f7192c3ef9b91d4b6ecd272e6f22685b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edf6720603b8ae15bb90566847de02a5 = $(`<div id="html_edf6720603b8ae15bb90566847de02a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7192c3ef9b91d4b6ecd272e6f22685b.setContent(html_edf6720603b8ae15bb90566847de02a5);\\n \\n \\n\\n circle_marker_8fcd07473a528234363bc0694c5fb91a.bindPopup(popup_f7192c3ef9b91d4b6ecd272e6f22685b)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Aspen, big-toothed\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_952d260262d53c5f8c195ac46aa15218 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_952d260262d53c5f8c195ac46aa15218" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_952d260262d53c5f8c195ac46aa15218 = L.map(\\n "map_952d260262d53c5f8c195ac46aa15218",\\n {\\n center: [42.729129690214265, -73.23965471433218],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_a7221bfe65c285f3bbcbe700660f86c7 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var circle_marker_42cd5d89136902ae3cc1661a052b3eea = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_d1319ee53752845cd6a0fc6fe569ef2d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9338f03e89ddc01991b75cf389e01a50 = $(`<div id="html_9338f03e89ddc01991b75cf389e01a50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d1319ee53752845cd6a0fc6fe569ef2d.setContent(html_9338f03e89ddc01991b75cf389e01a50);\\n \\n \\n\\n circle_marker_42cd5d89136902ae3cc1661a052b3eea.bindPopup(popup_d1319ee53752845cd6a0fc6fe569ef2d)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb00e8a117be4e4b7c0a59df399b5e1f = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_9a25b0541861d9fbe420b0aee0c7c346 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da760e52cf1a1fc2d46af2f77312d32b = $(`<div id="html_da760e52cf1a1fc2d46af2f77312d32b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a25b0541861d9fbe420b0aee0c7c346.setContent(html_da760e52cf1a1fc2d46af2f77312d32b);\\n \\n \\n\\n circle_marker_cb00e8a117be4e4b7c0a59df399b5e1f.bindPopup(popup_9a25b0541861d9fbe420b0aee0c7c346)\\n ;\\n\\n \\n \\n \\n var circle_marker_84ba9d57452f4450b34cd28b3aaab0ac = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_11da2bde5dced77fea9db8e9a2184fda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30cc78604e3569b7b1bc41f53a286fd8 = $(`<div id="html_30cc78604e3569b7b1bc41f53a286fd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11da2bde5dced77fea9db8e9a2184fda.setContent(html_30cc78604e3569b7b1bc41f53a286fd8);\\n \\n \\n\\n circle_marker_84ba9d57452f4450b34cd28b3aaab0ac.bindPopup(popup_11da2bde5dced77fea9db8e9a2184fda)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd14708da5e67852b3f5d1af4ea3e4a0 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_3ad1db94da7f3bc84d0688357dbc24e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_122e88144cfb3a1001d7c5fc145ad9c9 = $(`<div id="html_122e88144cfb3a1001d7c5fc145ad9c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ad1db94da7f3bc84d0688357dbc24e5.setContent(html_122e88144cfb3a1001d7c5fc145ad9c9);\\n \\n \\n\\n circle_marker_dd14708da5e67852b3f5d1af4ea3e4a0.bindPopup(popup_3ad1db94da7f3bc84d0688357dbc24e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_bdaccac513c3c4c2856d129ac7ead70d = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_d139a9e0e1c41b5e851ca5d606bdac54 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df28b70cc6c1e96e7329490815b8c0c4 = $(`<div id="html_df28b70cc6c1e96e7329490815b8c0c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d139a9e0e1c41b5e851ca5d606bdac54.setContent(html_df28b70cc6c1e96e7329490815b8c0c4);\\n \\n \\n\\n circle_marker_bdaccac513c3c4c2856d129ac7ead70d.bindPopup(popup_d139a9e0e1c41b5e851ca5d606bdac54)\\n ;\\n\\n \\n \\n \\n var circle_marker_1dd53a88b6f17b26e6ad27125da2d118 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_e3991b15bd6e71ea1687ffc3b149965f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_975a128d3111e391f93060d81e97a9d6 = $(`<div id="html_975a128d3111e391f93060d81e97a9d6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3991b15bd6e71ea1687ffc3b149965f.setContent(html_975a128d3111e391f93060d81e97a9d6);\\n \\n \\n\\n circle_marker_1dd53a88b6f17b26e6ad27125da2d118.bindPopup(popup_e3991b15bd6e71ea1687ffc3b149965f)\\n ;\\n\\n \\n \\n \\n var circle_marker_87d0ff1e0c4d214e1555e5df371b78cf = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_944bc298c213098f1101b82e71f991ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b8be8d5e1045cb0de11b675fae5e7e50 = $(`<div id="html_b8be8d5e1045cb0de11b675fae5e7e50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_944bc298c213098f1101b82e71f991ec.setContent(html_b8be8d5e1045cb0de11b675fae5e7e50);\\n \\n \\n\\n circle_marker_87d0ff1e0c4d214e1555e5df371b78cf.bindPopup(popup_944bc298c213098f1101b82e71f991ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_651fb779a61e5dd1a4f365478d95fe71 = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_692aca66669bbf1171d92ef9d4678312 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_010b381fe7703d332b6275ffd978e7d6 = $(`<div id="html_010b381fe7703d332b6275ffd978e7d6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_692aca66669bbf1171d92ef9d4678312.setContent(html_010b381fe7703d332b6275ffd978e7d6);\\n \\n \\n\\n circle_marker_651fb779a61e5dd1a4f365478d95fe71.bindPopup(popup_692aca66669bbf1171d92ef9d4678312)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fda25b31266cf9acf87f18482244424 = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_d2db8b056c365da5a7edf164e282a882 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_742cb9c5f1b892f2782b41daddc08e7c = $(`<div id="html_742cb9c5f1b892f2782b41daddc08e7c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2db8b056c365da5a7edf164e282a882.setContent(html_742cb9c5f1b892f2782b41daddc08e7c);\\n \\n \\n\\n circle_marker_9fda25b31266cf9acf87f18482244424.bindPopup(popup_d2db8b056c365da5a7edf164e282a882)\\n ;\\n\\n \\n \\n \\n var circle_marker_183b4571c563daab681c3d7e2778bf01 = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_7202568ba4502c2759683632cde55f30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_45b4ededb8f497ef600cd0f3c712de2c = $(`<div id="html_45b4ededb8f497ef600cd0f3c712de2c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7202568ba4502c2759683632cde55f30.setContent(html_45b4ededb8f497ef600cd0f3c712de2c);\\n \\n \\n\\n circle_marker_183b4571c563daab681c3d7e2778bf01.bindPopup(popup_7202568ba4502c2759683632cde55f30)\\n ;\\n\\n \\n \\n \\n var circle_marker_8252967810b2ac4e62d4a966597ce63d = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_98a3903fc24c8cd887809d71a4933dab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_525658bf3139ea48dac4bc7da92ac024 = $(`<div id="html_525658bf3139ea48dac4bc7da92ac024" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98a3903fc24c8cd887809d71a4933dab.setContent(html_525658bf3139ea48dac4bc7da92ac024);\\n \\n \\n\\n circle_marker_8252967810b2ac4e62d4a966597ce63d.bindPopup(popup_98a3903fc24c8cd887809d71a4933dab)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba23458e9c0b6a85291fe662874261a3 = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_0e17a339de04be9679dfaec2e5d7eef4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad94d26e47ef68138ff4bb3f4cbb2410 = $(`<div id="html_ad94d26e47ef68138ff4bb3f4cbb2410" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e17a339de04be9679dfaec2e5d7eef4.setContent(html_ad94d26e47ef68138ff4bb3f4cbb2410);\\n \\n \\n\\n circle_marker_ba23458e9c0b6a85291fe662874261a3.bindPopup(popup_0e17a339de04be9679dfaec2e5d7eef4)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a5c4987fb7dc8d75955aed2d6f07a38 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_9a6c2b240a55ff4eac8db3b8a47dde58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67c9bc3e3df27caf044058a68b9ef5aa = $(`<div id="html_67c9bc3e3df27caf044058a68b9ef5aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a6c2b240a55ff4eac8db3b8a47dde58.setContent(html_67c9bc3e3df27caf044058a68b9ef5aa);\\n \\n \\n\\n circle_marker_7a5c4987fb7dc8d75955aed2d6f07a38.bindPopup(popup_9a6c2b240a55ff4eac8db3b8a47dde58)\\n ;\\n\\n \\n \\n \\n var circle_marker_d41fbf654e890dea0af5b68cd702704f = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_c2ee1bd8873e44722d0200ebaea10164 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f3556485c78a78c4843b3e404b32484 = $(`<div id="html_1f3556485c78a78c4843b3e404b32484" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2ee1bd8873e44722d0200ebaea10164.setContent(html_1f3556485c78a78c4843b3e404b32484);\\n \\n \\n\\n circle_marker_d41fbf654e890dea0af5b68cd702704f.bindPopup(popup_c2ee1bd8873e44722d0200ebaea10164)\\n ;\\n\\n \\n \\n \\n var circle_marker_59f1731342d4f383fb0f003f897b8c34 = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_952d260262d53c5f8c195ac46aa15218);\\n \\n \\n var popup_262b1f1fc256cce81dfb03a438b1cb87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2209c93936c3a0ecaed287ee08e007b = $(`<div id="html_b2209c93936c3a0ecaed287ee08e007b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_262b1f1fc256cce81dfb03a438b1cb87.setContent(html_b2209c93936c3a0ecaed287ee08e007b);\\n \\n \\n\\n circle_marker_59f1731342d4f383fb0f003f897b8c34.bindPopup(popup_262b1f1fc256cce81dfb03a438b1cb87)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Aspen, quaking\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_b890d893d58bbbcce1b20a5270edad95 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_b890d893d58bbbcce1b20a5270edad95" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_b890d893d58bbbcce1b20a5270edad95 = L.map(\\n "map_b890d893d58bbbcce1b20a5270edad95",\\n {\\n center: [42.73454877005139, -73.24580637107132],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_0a8707e1b9983b993f082765b783d592 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var circle_marker_9172dc244bdc9817814c429fd1759037 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_2bd278e3b54eae75b1d23c2b00b702dc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8fdee480abf2aa5d1cc294009b1f76e = $(`<div id="html_c8fdee480abf2aa5d1cc294009b1f76e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2bd278e3b54eae75b1d23c2b00b702dc.setContent(html_c8fdee480abf2aa5d1cc294009b1f76e);\\n \\n \\n\\n circle_marker_9172dc244bdc9817814c429fd1759037.bindPopup(popup_2bd278e3b54eae75b1d23c2b00b702dc)\\n ;\\n\\n \\n \\n \\n var circle_marker_d100674b5ee8312a6e109ba6d084e412 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_b9eb607d688d4b3af7318384ef7f0a91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c65cbd8fffbf2b774e649e7426af3d1 = $(`<div id="html_7c65cbd8fffbf2b774e649e7426af3d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b9eb607d688d4b3af7318384ef7f0a91.setContent(html_7c65cbd8fffbf2b774e649e7426af3d1);\\n \\n \\n\\n circle_marker_d100674b5ee8312a6e109ba6d084e412.bindPopup(popup_b9eb607d688d4b3af7318384ef7f0a91)\\n ;\\n\\n \\n \\n \\n var circle_marker_e742ad1e243cf07ce492c3d66e403be4 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_51a93613c95287ac47b5128e1170ba82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6fa2574359aa2bc8a32cc5da3d1f2f86 = $(`<div id="html_6fa2574359aa2bc8a32cc5da3d1f2f86" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51a93613c95287ac47b5128e1170ba82.setContent(html_6fa2574359aa2bc8a32cc5da3d1f2f86);\\n \\n \\n\\n circle_marker_e742ad1e243cf07ce492c3d66e403be4.bindPopup(popup_51a93613c95287ac47b5128e1170ba82)\\n ;\\n\\n \\n \\n \\n var circle_marker_16f8703e8822286dc313cdd57024768c = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_2539cc26f50a5529476540822cc574c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d644d7c5fe1d93f2f7a12ac437db97e2 = $(`<div id="html_d644d7c5fe1d93f2f7a12ac437db97e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2539cc26f50a5529476540822cc574c4.setContent(html_d644d7c5fe1d93f2f7a12ac437db97e2);\\n \\n \\n\\n circle_marker_16f8703e8822286dc313cdd57024768c.bindPopup(popup_2539cc26f50a5529476540822cc574c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_75e01a627c5e5006883a9470f40270e2 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_8ea496be9054ee470861e9cdc996a97e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4da8600a89b9fff8ec8f46a699388a4b = $(`<div id="html_4da8600a89b9fff8ec8f46a699388a4b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ea496be9054ee470861e9cdc996a97e.setContent(html_4da8600a89b9fff8ec8f46a699388a4b);\\n \\n \\n\\n circle_marker_75e01a627c5e5006883a9470f40270e2.bindPopup(popup_8ea496be9054ee470861e9cdc996a97e)\\n ;\\n\\n \\n \\n \\n var circle_marker_33d71549cc1f6bd63c237d6e320932b2 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_198b9c59b8a3c36be06ac741d35c54ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1ec4a0c95db8d6f91df916ca48a0c07 = $(`<div id="html_b1ec4a0c95db8d6f91df916ca48a0c07" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_198b9c59b8a3c36be06ac741d35c54ff.setContent(html_b1ec4a0c95db8d6f91df916ca48a0c07);\\n \\n \\n\\n circle_marker_33d71549cc1f6bd63c237d6e320932b2.bindPopup(popup_198b9c59b8a3c36be06ac741d35c54ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_d222455318ce0ba59c1d876bcd27a72b = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_7f5076aefd8ea5046e392ec458216252 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc66c9ebea91b1ff83f7bad051dfb498 = $(`<div id="html_cc66c9ebea91b1ff83f7bad051dfb498" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f5076aefd8ea5046e392ec458216252.setContent(html_cc66c9ebea91b1ff83f7bad051dfb498);\\n \\n \\n\\n circle_marker_d222455318ce0ba59c1d876bcd27a72b.bindPopup(popup_7f5076aefd8ea5046e392ec458216252)\\n ;\\n\\n \\n \\n \\n var circle_marker_db3fc2b32a6d86a0a44f96badf105cad = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_0b6764afd0c394cfc6fa337ba1b70127 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c0b98dee1c8f713ee3279cc96ce2393 = $(`<div id="html_0c0b98dee1c8f713ee3279cc96ce2393" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b6764afd0c394cfc6fa337ba1b70127.setContent(html_0c0b98dee1c8f713ee3279cc96ce2393);\\n \\n \\n\\n circle_marker_db3fc2b32a6d86a0a44f96badf105cad.bindPopup(popup_0b6764afd0c394cfc6fa337ba1b70127)\\n ;\\n\\n \\n \\n \\n var circle_marker_50e5da26f93e6fe592b98a4ff1775de3 = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_b963667bd84f741f2382db7136dcd018 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5dd71ca4d19be1f7d5a68df250279d17 = $(`<div id="html_5dd71ca4d19be1f7d5a68df250279d17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b963667bd84f741f2382db7136dcd018.setContent(html_5dd71ca4d19be1f7d5a68df250279d17);\\n \\n \\n\\n circle_marker_50e5da26f93e6fe592b98a4ff1775de3.bindPopup(popup_b963667bd84f741f2382db7136dcd018)\\n ;\\n\\n \\n \\n \\n var circle_marker_c49c1ee8142d3adf29a4e78741ad0150 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_a59f15b83a76822b71addf2911840cc6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9fe6532581a940c5002b5f2664c46265 = $(`<div id="html_9fe6532581a940c5002b5f2664c46265" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a59f15b83a76822b71addf2911840cc6.setContent(html_9fe6532581a940c5002b5f2664c46265);\\n \\n \\n\\n circle_marker_c49c1ee8142d3adf29a4e78741ad0150.bindPopup(popup_a59f15b83a76822b71addf2911840cc6)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec1b586ed0b3fb7c8c93b86bb81fd527 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_2dd7f899c8cfa5a26c2a96a30aeefd27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8e437a45c19992a52fd21f158d7277e = $(`<div id="html_c8e437a45c19992a52fd21f158d7277e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2dd7f899c8cfa5a26c2a96a30aeefd27.setContent(html_c8e437a45c19992a52fd21f158d7277e);\\n \\n \\n\\n circle_marker_ec1b586ed0b3fb7c8c93b86bb81fd527.bindPopup(popup_2dd7f899c8cfa5a26c2a96a30aeefd27)\\n ;\\n\\n \\n \\n \\n var circle_marker_182f56f7d3077f59ebdca1db3a3bcede = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_4dd87819ab81a2cf98761d13b91b14e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e93f7417c3361ef6eda732ec60493b4e = $(`<div id="html_e93f7417c3361ef6eda732ec60493b4e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4dd87819ab81a2cf98761d13b91b14e5.setContent(html_e93f7417c3361ef6eda732ec60493b4e);\\n \\n \\n\\n circle_marker_182f56f7d3077f59ebdca1db3a3bcede.bindPopup(popup_4dd87819ab81a2cf98761d13b91b14e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_7657330f6ef2f4d29b5de926da28e370 = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_b021633c07b785af56c6e19482369ef9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60ac3c49c04b338606f9d74e60570e6b = $(`<div id="html_60ac3c49c04b338606f9d74e60570e6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b021633c07b785af56c6e19482369ef9.setContent(html_60ac3c49c04b338606f9d74e60570e6b);\\n \\n \\n\\n circle_marker_7657330f6ef2f4d29b5de926da28e370.bindPopup(popup_b021633c07b785af56c6e19482369ef9)\\n ;\\n\\n \\n \\n \\n var circle_marker_1eb82193fe28983fc6e1511f034a590a = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_7b2def43cdc44955e8bf716240122f05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_392cdc8d181d4e9c1865c1fbd77828e5 = $(`<div id="html_392cdc8d181d4e9c1865c1fbd77828e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7b2def43cdc44955e8bf716240122f05.setContent(html_392cdc8d181d4e9c1865c1fbd77828e5);\\n \\n \\n\\n circle_marker_1eb82193fe28983fc6e1511f034a590a.bindPopup(popup_7b2def43cdc44955e8bf716240122f05)\\n ;\\n\\n \\n \\n \\n var circle_marker_67d40dd4a47e4a4d0f0e2d9da1619640 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_374534052a3c59aa992a59aca0ecb79c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ddf21f42ef0cc1ebbf02357298facd7 = $(`<div id="html_3ddf21f42ef0cc1ebbf02357298facd7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_374534052a3c59aa992a59aca0ecb79c.setContent(html_3ddf21f42ef0cc1ebbf02357298facd7);\\n \\n \\n\\n circle_marker_67d40dd4a47e4a4d0f0e2d9da1619640.bindPopup(popup_374534052a3c59aa992a59aca0ecb79c)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e1c0f8e1526ddd5ddb53001ff8dd7f9 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_435f4f82182277c47303b03c2fddceb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0484c309933b23228f61debde911e0b6 = $(`<div id="html_0484c309933b23228f61debde911e0b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_435f4f82182277c47303b03c2fddceb2.setContent(html_0484c309933b23228f61debde911e0b6);\\n \\n \\n\\n circle_marker_4e1c0f8e1526ddd5ddb53001ff8dd7f9.bindPopup(popup_435f4f82182277c47303b03c2fddceb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_32bf03c325e33b1cdb8e358cbb3d1a33 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_946115a081d2a54e80a9cd6d7cce27d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_578e31133bdb23197ccfb17f046eb0bd = $(`<div id="html_578e31133bdb23197ccfb17f046eb0bd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_946115a081d2a54e80a9cd6d7cce27d9.setContent(html_578e31133bdb23197ccfb17f046eb0bd);\\n \\n \\n\\n circle_marker_32bf03c325e33b1cdb8e358cbb3d1a33.bindPopup(popup_946115a081d2a54e80a9cd6d7cce27d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_11ed172781dcf4059576397027ee1e53 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_1cad70aad0848c4ed668fffbbd5c2a8c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba4c01f2ad89a59f85d55ee3cba593b3 = $(`<div id="html_ba4c01f2ad89a59f85d55ee3cba593b3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1cad70aad0848c4ed668fffbbd5c2a8c.setContent(html_ba4c01f2ad89a59f85d55ee3cba593b3);\\n \\n \\n\\n circle_marker_11ed172781dcf4059576397027ee1e53.bindPopup(popup_1cad70aad0848c4ed668fffbbd5c2a8c)\\n ;\\n\\n \\n \\n \\n var circle_marker_f142e40d0bd16ad11f1006c6616ba2f7 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_c5dd1516ba0f656fee3c79ee49bdda8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d452dcb8b696ae2337af0847b124a09 = $(`<div id="html_6d452dcb8b696ae2337af0847b124a09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c5dd1516ba0f656fee3c79ee49bdda8b.setContent(html_6d452dcb8b696ae2337af0847b124a09);\\n \\n \\n\\n circle_marker_f142e40d0bd16ad11f1006c6616ba2f7.bindPopup(popup_c5dd1516ba0f656fee3c79ee49bdda8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_0327397a50ee3640b7bd4120816f916a = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_bb012c0694c5359972101fa7508633f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a7c38d198fb2be65ce819e01c03b2530 = $(`<div id="html_a7c38d198fb2be65ce819e01c03b2530" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb012c0694c5359972101fa7508633f3.setContent(html_a7c38d198fb2be65ce819e01c03b2530);\\n \\n \\n\\n circle_marker_0327397a50ee3640b7bd4120816f916a.bindPopup(popup_bb012c0694c5359972101fa7508633f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_898473398fc833b8d17295e7b0cef2ef = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_f372d6b6dc90626b2a93d6780af43232 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e524e7c52f85bbcc8ba75117743333da = $(`<div id="html_e524e7c52f85bbcc8ba75117743333da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f372d6b6dc90626b2a93d6780af43232.setContent(html_e524e7c52f85bbcc8ba75117743333da);\\n \\n \\n\\n circle_marker_898473398fc833b8d17295e7b0cef2ef.bindPopup(popup_f372d6b6dc90626b2a93d6780af43232)\\n ;\\n\\n \\n \\n \\n var circle_marker_127ab51e8f464d37bce1ce19a4ab275c = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_ef48bbbc24c6832fef1855d642a027d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2d04cc1f34ced568723894644ac28b1 = $(`<div id="html_b2d04cc1f34ced568723894644ac28b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef48bbbc24c6832fef1855d642a027d7.setContent(html_b2d04cc1f34ced568723894644ac28b1);\\n \\n \\n\\n circle_marker_127ab51e8f464d37bce1ce19a4ab275c.bindPopup(popup_ef48bbbc24c6832fef1855d642a027d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_995cdec06df807c8ed9d5f56a13169ac = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_3845a0372b81dac78eeb03424025d561 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6cfe6da9ab1566f686f385fd86adb7b0 = $(`<div id="html_6cfe6da9ab1566f686f385fd86adb7b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3845a0372b81dac78eeb03424025d561.setContent(html_6cfe6da9ab1566f686f385fd86adb7b0);\\n \\n \\n\\n circle_marker_995cdec06df807c8ed9d5f56a13169ac.bindPopup(popup_3845a0372b81dac78eeb03424025d561)\\n ;\\n\\n \\n \\n \\n var circle_marker_eff95f044c7f2d7d0d2aa13bd3c083cc = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_b63e77ee0c00a06771ca5619533b45b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd5b97e38fceb0b2657372f81ac36603 = $(`<div id="html_dd5b97e38fceb0b2657372f81ac36603" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b63e77ee0c00a06771ca5619533b45b7.setContent(html_dd5b97e38fceb0b2657372f81ac36603);\\n \\n \\n\\n circle_marker_eff95f044c7f2d7d0d2aa13bd3c083cc.bindPopup(popup_b63e77ee0c00a06771ca5619533b45b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_b13e2ad69421c6c2577ddc16afc6a9eb = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_cbdee6eaa071c8dac6b9906e525acba3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fdfbaed1c3ee04d93b47c2b395803f0 = $(`<div id="html_8fdfbaed1c3ee04d93b47c2b395803f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cbdee6eaa071c8dac6b9906e525acba3.setContent(html_8fdfbaed1c3ee04d93b47c2b395803f0);\\n \\n \\n\\n circle_marker_b13e2ad69421c6c2577ddc16afc6a9eb.bindPopup(popup_cbdee6eaa071c8dac6b9906e525acba3)\\n ;\\n\\n \\n \\n \\n var circle_marker_a31e70143ae65f991d396d39dcf11247 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_aec21a27e30aab5ba637de15a1386dae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5b32a5b1aa0e5ee05cef74d373d0fcd = $(`<div id="html_e5b32a5b1aa0e5ee05cef74d373d0fcd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aec21a27e30aab5ba637de15a1386dae.setContent(html_e5b32a5b1aa0e5ee05cef74d373d0fcd);\\n \\n \\n\\n circle_marker_a31e70143ae65f991d396d39dcf11247.bindPopup(popup_aec21a27e30aab5ba637de15a1386dae)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d190f5c909390fb178013eea4386388 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_6159ba6116a0383a997548f67c382d5d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f79919cfc10df231a2c380a6099a36b = $(`<div id="html_7f79919cfc10df231a2c380a6099a36b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6159ba6116a0383a997548f67c382d5d.setContent(html_7f79919cfc10df231a2c380a6099a36b);\\n \\n \\n\\n circle_marker_0d190f5c909390fb178013eea4386388.bindPopup(popup_6159ba6116a0383a997548f67c382d5d)\\n ;\\n\\n \\n \\n \\n var circle_marker_76b0b8739fc0e8089db377a55fcf51f7 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_00e00db44de9a0bd99ca7759a69e387c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2875e676fb4222147eae931eecbc85b6 = $(`<div id="html_2875e676fb4222147eae931eecbc85b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00e00db44de9a0bd99ca7759a69e387c.setContent(html_2875e676fb4222147eae931eecbc85b6);\\n \\n \\n\\n circle_marker_76b0b8739fc0e8089db377a55fcf51f7.bindPopup(popup_00e00db44de9a0bd99ca7759a69e387c)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3a313d9b6936f9d31459db79dd0740e = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_8c0cd8dbd4d3e8545aa56260fc0d18dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d28efee8af5a93d84bb3fcd3fe9d4cf4 = $(`<div id="html_d28efee8af5a93d84bb3fcd3fe9d4cf4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c0cd8dbd4d3e8545aa56260fc0d18dd.setContent(html_d28efee8af5a93d84bb3fcd3fe9d4cf4);\\n \\n \\n\\n circle_marker_f3a313d9b6936f9d31459db79dd0740e.bindPopup(popup_8c0cd8dbd4d3e8545aa56260fc0d18dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_c79a70c9d4c1d653aaed6595972fe711 = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_693bb066c8031287751e10af97328704 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14f11566344cd541291e2dbcf26be53e = $(`<div id="html_14f11566344cd541291e2dbcf26be53e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_693bb066c8031287751e10af97328704.setContent(html_14f11566344cd541291e2dbcf26be53e);\\n \\n \\n\\n circle_marker_c79a70c9d4c1d653aaed6595972fe711.bindPopup(popup_693bb066c8031287751e10af97328704)\\n ;\\n\\n \\n \\n \\n var circle_marker_66f387b2316401079977fe62894c032a = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_bef1dac39518e5f7a7b50c4e68edfe03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_650c9a82aa649c1b8d9cf964fd6f855f = $(`<div id="html_650c9a82aa649c1b8d9cf964fd6f855f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bef1dac39518e5f7a7b50c4e68edfe03.setContent(html_650c9a82aa649c1b8d9cf964fd6f855f);\\n \\n \\n\\n circle_marker_66f387b2316401079977fe62894c032a.bindPopup(popup_bef1dac39518e5f7a7b50c4e68edfe03)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7bc170b05081528736b4e91f38b1d1f = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_c9bf873cd82d7650985cf32c3b403e21 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7987d1abce745b5271a9cdc31a4da79 = $(`<div id="html_c7987d1abce745b5271a9cdc31a4da79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9bf873cd82d7650985cf32c3b403e21.setContent(html_c7987d1abce745b5271a9cdc31a4da79);\\n \\n \\n\\n circle_marker_a7bc170b05081528736b4e91f38b1d1f.bindPopup(popup_c9bf873cd82d7650985cf32c3b403e21)\\n ;\\n\\n \\n \\n \\n var circle_marker_e531c42da1a7c42a95043ad62f2ec8b7 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_00b9a50249b3c3536aa3789a1df637dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dcf717d49f8e5a241da43dd6876d4770 = $(`<div id="html_dcf717d49f8e5a241da43dd6876d4770" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00b9a50249b3c3536aa3789a1df637dd.setContent(html_dcf717d49f8e5a241da43dd6876d4770);\\n \\n \\n\\n circle_marker_e531c42da1a7c42a95043ad62f2ec8b7.bindPopup(popup_00b9a50249b3c3536aa3789a1df637dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_eae09915ff169662d9519db6cc0b7024 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_6f15d00623026fa7e85c9c1c3a169dc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d0507ed6835c1115f405686d84320eb0 = $(`<div id="html_d0507ed6835c1115f405686d84320eb0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f15d00623026fa7e85c9c1c3a169dc1.setContent(html_d0507ed6835c1115f405686d84320eb0);\\n \\n \\n\\n circle_marker_eae09915ff169662d9519db6cc0b7024.bindPopup(popup_6f15d00623026fa7e85c9c1c3a169dc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ad12e504df841066dbdcf37cb6236b0 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_3528aed8d143955e54e41c3aecc903d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_391633dd7ebd2448235eaa3c912ed8fa = $(`<div id="html_391633dd7ebd2448235eaa3c912ed8fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3528aed8d143955e54e41c3aecc903d5.setContent(html_391633dd7ebd2448235eaa3c912ed8fa);\\n \\n \\n\\n circle_marker_9ad12e504df841066dbdcf37cb6236b0.bindPopup(popup_3528aed8d143955e54e41c3aecc903d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c13e37460e3628727ba3a912b76d6abf = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_7d674163f4f183bfe93f48e9d63e7a08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3dd25d7f13869f8523717f24d980dcb8 = $(`<div id="html_3dd25d7f13869f8523717f24d980dcb8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d674163f4f183bfe93f48e9d63e7a08.setContent(html_3dd25d7f13869f8523717f24d980dcb8);\\n \\n \\n\\n circle_marker_c13e37460e3628727ba3a912b76d6abf.bindPopup(popup_7d674163f4f183bfe93f48e9d63e7a08)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a3e874657f5de441dc2d399bf99e75d = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_fd48b04f608a27e9b77791f03c7f67cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76d15cdaa41adf61f872551e498aa324 = $(`<div id="html_76d15cdaa41adf61f872551e498aa324" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd48b04f608a27e9b77791f03c7f67cf.setContent(html_76d15cdaa41adf61f872551e498aa324);\\n \\n \\n\\n circle_marker_9a3e874657f5de441dc2d399bf99e75d.bindPopup(popup_fd48b04f608a27e9b77791f03c7f67cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_7b1031fc9f3f0179d290d441a63581be = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_3167224738492afbfed98ba124117b77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81995e5a7b0f7c9665f13553afe5536f = $(`<div id="html_81995e5a7b0f7c9665f13553afe5536f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3167224738492afbfed98ba124117b77.setContent(html_81995e5a7b0f7c9665f13553afe5536f);\\n \\n \\n\\n circle_marker_7b1031fc9f3f0179d290d441a63581be.bindPopup(popup_3167224738492afbfed98ba124117b77)\\n ;\\n\\n \\n \\n \\n var circle_marker_8ea757a6d6855ad8a192d3c752a11e91 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_bff7374de2f793eb1df29785ab97339f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c395d8999385dc7ed17d63b21b20df48 = $(`<div id="html_c395d8999385dc7ed17d63b21b20df48" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bff7374de2f793eb1df29785ab97339f.setContent(html_c395d8999385dc7ed17d63b21b20df48);\\n \\n \\n\\n circle_marker_8ea757a6d6855ad8a192d3c752a11e91.bindPopup(popup_bff7374de2f793eb1df29785ab97339f)\\n ;\\n\\n \\n \\n \\n var circle_marker_ccb412824958502f03ffba6457c2c871 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_402b45dc57c05a9d3f58c9ab22c86564 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_585c9c52595f630b04bdf7d291ec549d = $(`<div id="html_585c9c52595f630b04bdf7d291ec549d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_402b45dc57c05a9d3f58c9ab22c86564.setContent(html_585c9c52595f630b04bdf7d291ec549d);\\n \\n \\n\\n circle_marker_ccb412824958502f03ffba6457c2c871.bindPopup(popup_402b45dc57c05a9d3f58c9ab22c86564)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4304315d758203a51b85a1c11f6e394 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_a0ae653ef47742b05efa1da0a98bbac7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78d6058beb5f87a11662cfe4dce4152e = $(`<div id="html_78d6058beb5f87a11662cfe4dce4152e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0ae653ef47742b05efa1da0a98bbac7.setContent(html_78d6058beb5f87a11662cfe4dce4152e);\\n \\n \\n\\n circle_marker_f4304315d758203a51b85a1c11f6e394.bindPopup(popup_a0ae653ef47742b05efa1da0a98bbac7)\\n ;\\n\\n \\n \\n \\n var circle_marker_83f357a66898f726c2c382e52b951ab0 = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_8a144c063ce6c541ce7c8d73186b5d95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9fd160cefa830d76af661a573b6dbedb = $(`<div id="html_9fd160cefa830d76af661a573b6dbedb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a144c063ce6c541ce7c8d73186b5d95.setContent(html_9fd160cefa830d76af661a573b6dbedb);\\n \\n \\n\\n circle_marker_83f357a66898f726c2c382e52b951ab0.bindPopup(popup_8a144c063ce6c541ce7c8d73186b5d95)\\n ;\\n\\n \\n \\n \\n var circle_marker_d02bdf0c4217c36ffcee37c9668e45f4 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_6536e16d5f4107e3d75df424f247c7c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41c0a2372d41963fa248cda73faa2660 = $(`<div id="html_41c0a2372d41963fa248cda73faa2660" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6536e16d5f4107e3d75df424f247c7c7.setContent(html_41c0a2372d41963fa248cda73faa2660);\\n \\n \\n\\n circle_marker_d02bdf0c4217c36ffcee37c9668e45f4.bindPopup(popup_6536e16d5f4107e3d75df424f247c7c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_18b48c82133b2e00e3eb6d7079c26d0d = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_6c5627ffc900cb15c10795714fe0c1f7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82cd105cc99269e190b6b0bb8c6e67fa = $(`<div id="html_82cd105cc99269e190b6b0bb8c6e67fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c5627ffc900cb15c10795714fe0c1f7.setContent(html_82cd105cc99269e190b6b0bb8c6e67fa);\\n \\n \\n\\n circle_marker_18b48c82133b2e00e3eb6d7079c26d0d.bindPopup(popup_6c5627ffc900cb15c10795714fe0c1f7)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa50f4336767b558544fb49c946d2a3e = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_541610ce6678eb6f7775b93453bfb6d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_545d0cc825e48132a07d719cdb93f9af = $(`<div id="html_545d0cc825e48132a07d719cdb93f9af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_541610ce6678eb6f7775b93453bfb6d3.setContent(html_545d0cc825e48132a07d719cdb93f9af);\\n \\n \\n\\n circle_marker_aa50f4336767b558544fb49c946d2a3e.bindPopup(popup_541610ce6678eb6f7775b93453bfb6d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e2614d28d4efac14fef3d1e2859d721 = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_b890d893d58bbbcce1b20a5270edad95);\\n \\n \\n var popup_6ff372c8efa9fa98755d5fb8edb8aa3d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b0f814d9fa54e98c1c3bf6e8e55ed1a = $(`<div id="html_2b0f814d9fa54e98c1c3bf6e8e55ed1a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ff372c8efa9fa98755d5fb8edb8aa3d.setContent(html_2b0f814d9fa54e98c1c3bf6e8e55ed1a);\\n \\n \\n\\n circle_marker_9e2614d28d4efac14fef3d1e2859d721.bindPopup(popup_6ff372c8efa9fa98755d5fb8edb8aa3d)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Aster, white wood\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_7e748737339317554fb167c88df10e80 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_7e748737339317554fb167c88df10e80" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_7e748737339317554fb167c88df10e80 = L.map(\\n "map_7e748737339317554fb167c88df10e80",\\n {\\n center: [42.73093294947496, -73.23411937822746],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_a20248d31848eda916a58136eab0fd45 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_7e748737339317554fb167c88df10e80);\\n \\n \\n var circle_marker_c12f9e24f1d509422eb32d98509daa17 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_7e748737339317554fb167c88df10e80);\\n \\n \\n var popup_59dbdee69b48f10fff920940abb6ebd9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba61bdaf128697c6ed27490ac4174eef = $(`<div id="html_ba61bdaf128697c6ed27490ac4174eef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_59dbdee69b48f10fff920940abb6ebd9.setContent(html_ba61bdaf128697c6ed27490ac4174eef);\\n \\n \\n\\n circle_marker_c12f9e24f1d509422eb32d98509daa17.bindPopup(popup_59dbdee69b48f10fff920940abb6ebd9)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Azalea, early\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_3779f3a2d92bf7e4f75f33f1ff66ac31 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_3779f3a2d92bf7e4f75f33f1ff66ac31" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_3779f3a2d92bf7e4f75f33f1ff66ac31 = L.map(\\n "map_3779f3a2d92bf7e4f75f33f1ff66ac31",\\n {\\n center: [42.73635827299586, -73.27102329584875],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_2f5253cd77acbfe9df6abee34a6433fc = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_3779f3a2d92bf7e4f75f33f1ff66ac31);\\n \\n \\n var circle_marker_68577d6ecc1c6ce45a85ca5331ffbaf1 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3779f3a2d92bf7e4f75f33f1ff66ac31);\\n \\n \\n var popup_f2213b0bf84ba0e63f53e24e0d67c689 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0de0637bdec5ee2962dd88a9e3710c79 = $(`<div id="html_0de0637bdec5ee2962dd88a9e3710c79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2213b0bf84ba0e63f53e24e0d67c689.setContent(html_0de0637bdec5ee2962dd88a9e3710c79);\\n \\n \\n\\n circle_marker_68577d6ecc1c6ce45a85ca5331ffbaf1.bindPopup(popup_f2213b0bf84ba0e63f53e24e0d67c689)\\n ;\\n\\n \\n \\n \\n var circle_marker_645a373bbafd2dcc78d41ec6601bb850 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3779f3a2d92bf7e4f75f33f1ff66ac31);\\n \\n \\n var popup_32eeb913ffda3d9bfebb4464cfdd380b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3e8192cdca5ab6f71bb1dc81e49ffc5 = $(`<div id="html_f3e8192cdca5ab6f71bb1dc81e49ffc5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_32eeb913ffda3d9bfebb4464cfdd380b.setContent(html_f3e8192cdca5ab6f71bb1dc81e49ffc5);\\n \\n \\n\\n circle_marker_645a373bbafd2dcc78d41ec6601bb850.bindPopup(popup_32eeb913ffda3d9bfebb4464cfdd380b)\\n ;\\n\\n \\n \\n \\n var circle_marker_41a2264c70caa4ddf65ad1e8cbf9d3ac = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3779f3a2d92bf7e4f75f33f1ff66ac31);\\n \\n \\n var popup_25d72d4322343d0ccd2d67c9347861bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7f5b3e52a31788e9d9786fb569870e9 = $(`<div id="html_c7f5b3e52a31788e9d9786fb569870e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_25d72d4322343d0ccd2d67c9347861bc.setContent(html_c7f5b3e52a31788e9d9786fb569870e9);\\n \\n \\n\\n circle_marker_41a2264c70caa4ddf65ad1e8cbf9d3ac.bindPopup(popup_25d72d4322343d0ccd2d67c9347861bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_61b59cdf20e247e4c015ad70cf231562 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3779f3a2d92bf7e4f75f33f1ff66ac31);\\n \\n \\n var popup_b5349020809107776cc188a6f6712517 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_130a7ca2633b1c0dbd7a6105a0c0f8a8 = $(`<div id="html_130a7ca2633b1c0dbd7a6105a0c0f8a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5349020809107776cc188a6f6712517.setContent(html_130a7ca2633b1c0dbd7a6105a0c0f8a8);\\n \\n \\n\\n circle_marker_61b59cdf20e247e4c015ad70cf231562.bindPopup(popup_b5349020809107776cc188a6f6712517)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Basswood, American\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_960c0b956d564214f0c9a37df6ec9d42 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_960c0b956d564214f0c9a37df6ec9d42" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_960c0b956d564214f0c9a37df6ec9d42 = L.map(\\n "map_960c0b956d564214f0c9a37df6ec9d42",\\n {\\n center: [42.73635671967905, -73.24519139416742],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_6a9513b9816cedc75be81849eefa8d10 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var circle_marker_f5f4e52d1273f321c8b68160adddf023 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_50b2102dacb702d724f29fbc7cbf15c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5968f4dbaa211fd21471b7ba6af3003c = $(`<div id="html_5968f4dbaa211fd21471b7ba6af3003c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_50b2102dacb702d724f29fbc7cbf15c5.setContent(html_5968f4dbaa211fd21471b7ba6af3003c);\\n \\n \\n\\n circle_marker_f5f4e52d1273f321c8b68160adddf023.bindPopup(popup_50b2102dacb702d724f29fbc7cbf15c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c57bd1210580881f5ed6357973efc443 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_693fc2d1ccd2704a730b6ce42c10c0fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_192d199d18eb1b6b873332c78835d29c = $(`<div id="html_192d199d18eb1b6b873332c78835d29c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_693fc2d1ccd2704a730b6ce42c10c0fe.setContent(html_192d199d18eb1b6b873332c78835d29c);\\n \\n \\n\\n circle_marker_c57bd1210580881f5ed6357973efc443.bindPopup(popup_693fc2d1ccd2704a730b6ce42c10c0fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_1f822e3981242b5918ba5881b47f5021 = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_b5ac8a4e05a316cb0d042b332743da41 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d6e99689273c0204a4a4daf1bf87325 = $(`<div id="html_8d6e99689273c0204a4a4daf1bf87325" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5ac8a4e05a316cb0d042b332743da41.setContent(html_8d6e99689273c0204a4a4daf1bf87325);\\n \\n \\n\\n circle_marker_1f822e3981242b5918ba5881b47f5021.bindPopup(popup_b5ac8a4e05a316cb0d042b332743da41)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b3e897f8a86fb0b4b7ef45b8988890d = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_81b8c0737c4100b20642417f141f386a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2fc3975c9295a789ebe8f1dc62592d8 = $(`<div id="html_a2fc3975c9295a789ebe8f1dc62592d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81b8c0737c4100b20642417f141f386a.setContent(html_a2fc3975c9295a789ebe8f1dc62592d8);\\n \\n \\n\\n circle_marker_5b3e897f8a86fb0b4b7ef45b8988890d.bindPopup(popup_81b8c0737c4100b20642417f141f386a)\\n ;\\n\\n \\n \\n \\n var circle_marker_db55669446b3b2e1579fce2b093adb7a = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_76df5efd98aca044bc827cc3f7753947 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e9c9c1d77b51b5263fdbaee8f645a112 = $(`<div id="html_e9c9c1d77b51b5263fdbaee8f645a112" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76df5efd98aca044bc827cc3f7753947.setContent(html_e9c9c1d77b51b5263fdbaee8f645a112);\\n \\n \\n\\n circle_marker_db55669446b3b2e1579fce2b093adb7a.bindPopup(popup_76df5efd98aca044bc827cc3f7753947)\\n ;\\n\\n \\n \\n \\n var circle_marker_f14fe8d17e596f3d1ba811337968402f = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_70949737e4e2b43d6765704ee8846b58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7af396a3db3f3d590bfaf1c6ab30a627 = $(`<div id="html_7af396a3db3f3d590bfaf1c6ab30a627" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_70949737e4e2b43d6765704ee8846b58.setContent(html_7af396a3db3f3d590bfaf1c6ab30a627);\\n \\n \\n\\n circle_marker_f14fe8d17e596f3d1ba811337968402f.bindPopup(popup_70949737e4e2b43d6765704ee8846b58)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea49d10fb76bd20b40800404c4947d9a = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_4ce90169816728317536b4739c4094c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4766303883037a5722f43ccaf22b048d = $(`<div id="html_4766303883037a5722f43ccaf22b048d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ce90169816728317536b4739c4094c5.setContent(html_4766303883037a5722f43ccaf22b048d);\\n \\n \\n\\n circle_marker_ea49d10fb76bd20b40800404c4947d9a.bindPopup(popup_4ce90169816728317536b4739c4094c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_9267af571ed478c9762db29e45e1fa15 = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_e28d9319b86c1350a4d4f5d09d0a3536 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9f035f3c3d95b2fc04b4ce16bc2fe703 = $(`<div id="html_9f035f3c3d95b2fc04b4ce16bc2fe703" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e28d9319b86c1350a4d4f5d09d0a3536.setContent(html_9f035f3c3d95b2fc04b4ce16bc2fe703);\\n \\n \\n\\n circle_marker_9267af571ed478c9762db29e45e1fa15.bindPopup(popup_e28d9319b86c1350a4d4f5d09d0a3536)\\n ;\\n\\n \\n \\n \\n var circle_marker_c99eefef76dcf57edefd581ff506f191 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_19f0bf3a25f49f4c60e7918f4d8dca2c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c9ab9c7be0edc660770e88c626aa5771 = $(`<div id="html_c9ab9c7be0edc660770e88c626aa5771" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19f0bf3a25f49f4c60e7918f4d8dca2c.setContent(html_c9ab9c7be0edc660770e88c626aa5771);\\n \\n \\n\\n circle_marker_c99eefef76dcf57edefd581ff506f191.bindPopup(popup_19f0bf3a25f49f4c60e7918f4d8dca2c)\\n ;\\n\\n \\n \\n \\n var circle_marker_50d4c6ffc2ec7a111073fd4213a0fae2 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_615732adc49fa778a51598407abf1499 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46a85ae0b3ca5bef1e0058d0dde9d3ff = $(`<div id="html_46a85ae0b3ca5bef1e0058d0dde9d3ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_615732adc49fa778a51598407abf1499.setContent(html_46a85ae0b3ca5bef1e0058d0dde9d3ff);\\n \\n \\n\\n circle_marker_50d4c6ffc2ec7a111073fd4213a0fae2.bindPopup(popup_615732adc49fa778a51598407abf1499)\\n ;\\n\\n \\n \\n \\n var circle_marker_a49c2147a2e19cda8a010bb99e309508 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_a34a31636ac89a316ed0b8d2f07029fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6150dd54c32d07ab5e2a9102bce5db8c = $(`<div id="html_6150dd54c32d07ab5e2a9102bce5db8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a34a31636ac89a316ed0b8d2f07029fb.setContent(html_6150dd54c32d07ab5e2a9102bce5db8c);\\n \\n \\n\\n circle_marker_a49c2147a2e19cda8a010bb99e309508.bindPopup(popup_a34a31636ac89a316ed0b8d2f07029fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_d222ef9c198a4ef0302379aaed8ab005 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_0d0a1bf122808efb69ac46c59f97d620 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb6b747032d84d0f7b284522fad2c31b = $(`<div id="html_eb6b747032d84d0f7b284522fad2c31b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d0a1bf122808efb69ac46c59f97d620.setContent(html_eb6b747032d84d0f7b284522fad2c31b);\\n \\n \\n\\n circle_marker_d222ef9c198a4ef0302379aaed8ab005.bindPopup(popup_0d0a1bf122808efb69ac46c59f97d620)\\n ;\\n\\n \\n \\n \\n var circle_marker_f605a0f0e843922f90915e3e299e5729 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_1a5f06c02988e94f301f719086b62cff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c823c47e4dbebb4b80f7138139cd1d8 = $(`<div id="html_7c823c47e4dbebb4b80f7138139cd1d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a5f06c02988e94f301f719086b62cff.setContent(html_7c823c47e4dbebb4b80f7138139cd1d8);\\n \\n \\n\\n circle_marker_f605a0f0e843922f90915e3e299e5729.bindPopup(popup_1a5f06c02988e94f301f719086b62cff)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c1baccda162291c6fb9560a7e80b856 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_36808ff6b5656e2eaa3fbf32bf123732 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab2d4316f67ce3aa84b0acddad2e2727 = $(`<div id="html_ab2d4316f67ce3aa84b0acddad2e2727" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36808ff6b5656e2eaa3fbf32bf123732.setContent(html_ab2d4316f67ce3aa84b0acddad2e2727);\\n \\n \\n\\n circle_marker_1c1baccda162291c6fb9560a7e80b856.bindPopup(popup_36808ff6b5656e2eaa3fbf32bf123732)\\n ;\\n\\n \\n \\n \\n var circle_marker_2009df5b5474271e4992f98726dd0f16 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_0c4c56c07d5590a399ca5235f640a684 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c1b141c41cb061c72ce6970e4b449a8 = $(`<div id="html_0c1b141c41cb061c72ce6970e4b449a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c4c56c07d5590a399ca5235f640a684.setContent(html_0c1b141c41cb061c72ce6970e4b449a8);\\n \\n \\n\\n circle_marker_2009df5b5474271e4992f98726dd0f16.bindPopup(popup_0c4c56c07d5590a399ca5235f640a684)\\n ;\\n\\n \\n \\n \\n var circle_marker_87da3da0ef29026b01456a617ddc43b9 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_ab1cbf691cef2cbc6392946bc2491b2a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e12a3d2db8a451232d480457babc61d7 = $(`<div id="html_e12a3d2db8a451232d480457babc61d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ab1cbf691cef2cbc6392946bc2491b2a.setContent(html_e12a3d2db8a451232d480457babc61d7);\\n \\n \\n\\n circle_marker_87da3da0ef29026b01456a617ddc43b9.bindPopup(popup_ab1cbf691cef2cbc6392946bc2491b2a)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ae4e677377899f0b4fb3ad381617b68 = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_9cf897776130914510a694a993bd920b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c4ad3866ecbab0e7185b1edcb03cc4c2 = $(`<div id="html_c4ad3866ecbab0e7185b1edcb03cc4c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9cf897776130914510a694a993bd920b.setContent(html_c4ad3866ecbab0e7185b1edcb03cc4c2);\\n \\n \\n\\n circle_marker_3ae4e677377899f0b4fb3ad381617b68.bindPopup(popup_9cf897776130914510a694a993bd920b)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcffc31899b87d67a0d4a6171d3b1148 = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_77c4490cf807a116d09f6a37fb5f4b82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_050fdfc5bec165173ea780e03f68c27d = $(`<div id="html_050fdfc5bec165173ea780e03f68c27d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77c4490cf807a116d09f6a37fb5f4b82.setContent(html_050fdfc5bec165173ea780e03f68c27d);\\n \\n \\n\\n circle_marker_bcffc31899b87d67a0d4a6171d3b1148.bindPopup(popup_77c4490cf807a116d09f6a37fb5f4b82)\\n ;\\n\\n \\n \\n \\n var circle_marker_4312cf6d03a1081ff872a152ec7a1f02 = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_159cb8268b896d15c762501d3f7d7867 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15569873cf542620649d31338fafbd92 = $(`<div id="html_15569873cf542620649d31338fafbd92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_159cb8268b896d15c762501d3f7d7867.setContent(html_15569873cf542620649d31338fafbd92);\\n \\n \\n\\n circle_marker_4312cf6d03a1081ff872a152ec7a1f02.bindPopup(popup_159cb8268b896d15c762501d3f7d7867)\\n ;\\n\\n \\n \\n \\n var circle_marker_20076a09bfbbacacc0fb00ebd19f2ab2 = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_e3f79657a69b0fbe44bba13efd6af91d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26d72edff5d51d5f0eab31a63523bd68 = $(`<div id="html_26d72edff5d51d5f0eab31a63523bd68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3f79657a69b0fbe44bba13efd6af91d.setContent(html_26d72edff5d51d5f0eab31a63523bd68);\\n \\n \\n\\n circle_marker_20076a09bfbbacacc0fb00ebd19f2ab2.bindPopup(popup_e3f79657a69b0fbe44bba13efd6af91d)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cc71074783c98171a131a7f79f6fa0b = L.circleMarker(\\n [42.729122320849, -73.22304971370495],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_ad96d2679ddb0466364c74b194e7235a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0cee734eb436e21461f9394622b5f19d = $(`<div id="html_0cee734eb436e21461f9394622b5f19d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad96d2679ddb0466364c74b194e7235a.setContent(html_0cee734eb436e21461f9394622b5f19d);\\n \\n \\n\\n circle_marker_3cc71074783c98171a131a7f79f6fa0b.bindPopup(popup_ad96d2679ddb0466364c74b194e7235a)\\n ;\\n\\n \\n \\n \\n var circle_marker_80ba24f3a1685a8665aade1cef74806e = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_55c820b3a84c3cfa322f1a4787d4348f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1fee86f6cf81abb0bc475173765c80c2 = $(`<div id="html_1fee86f6cf81abb0bc475173765c80c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_55c820b3a84c3cfa322f1a4787d4348f.setContent(html_1fee86f6cf81abb0bc475173765c80c2);\\n \\n \\n\\n circle_marker_80ba24f3a1685a8665aade1cef74806e.bindPopup(popup_55c820b3a84c3cfa322f1a4787d4348f)\\n ;\\n\\n \\n \\n \\n var circle_marker_368c40a42602bc6f6be4903097cca397 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_6487fa6f26779e1add638621507e4b95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed9677111ca39d44edf6eece51a9ee81 = $(`<div id="html_ed9677111ca39d44edf6eece51a9ee81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6487fa6f26779e1add638621507e4b95.setContent(html_ed9677111ca39d44edf6eece51a9ee81);\\n \\n \\n\\n circle_marker_368c40a42602bc6f6be4903097cca397.bindPopup(popup_6487fa6f26779e1add638621507e4b95)\\n ;\\n\\n \\n \\n \\n var circle_marker_2dddc5b64a2a701e4a3399065c235cff = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_f0a5ebdd2b6a2c62c8c18b3fb0c850b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8f9d847657d5cc4a40c6e6f0aa40f31 = $(`<div id="html_f8f9d847657d5cc4a40c6e6f0aa40f31" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0a5ebdd2b6a2c62c8c18b3fb0c850b6.setContent(html_f8f9d847657d5cc4a40c6e6f0aa40f31);\\n \\n \\n\\n circle_marker_2dddc5b64a2a701e4a3399065c235cff.bindPopup(popup_f0a5ebdd2b6a2c62c8c18b3fb0c850b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_4447ba37f12fc59f4da3de82f855504c = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_f6aea348702f54475cd877b3a0aad1e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_27cec37322e629719d69e29e7fbfa6a4 = $(`<div id="html_27cec37322e629719d69e29e7fbfa6a4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6aea348702f54475cd877b3a0aad1e7.setContent(html_27cec37322e629719d69e29e7fbfa6a4);\\n \\n \\n\\n circle_marker_4447ba37f12fc59f4da3de82f855504c.bindPopup(popup_f6aea348702f54475cd877b3a0aad1e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f2fb90d2191e8fc830107b423790419 = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_53ff61c7af8459aba9318a71c2832926 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2f5cc0d8e37116365910063afe9111f = $(`<div id="html_a2f5cc0d8e37116365910063afe9111f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_53ff61c7af8459aba9318a71c2832926.setContent(html_a2f5cc0d8e37116365910063afe9111f);\\n \\n \\n\\n circle_marker_9f2fb90d2191e8fc830107b423790419.bindPopup(popup_53ff61c7af8459aba9318a71c2832926)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec9d9a4f7bf0c67498356c126c92ea65 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_9e0e05d89ef517fe0847db3bda641c27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c18415304ef997fca23f0b653b139d39 = $(`<div id="html_c18415304ef997fca23f0b653b139d39" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e0e05d89ef517fe0847db3bda641c27.setContent(html_c18415304ef997fca23f0b653b139d39);\\n \\n \\n\\n circle_marker_ec9d9a4f7bf0c67498356c126c92ea65.bindPopup(popup_9e0e05d89ef517fe0847db3bda641c27)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8237eb8240e0a2e16ec92eb2bad5a62 = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_960c0b956d564214f0c9a37df6ec9d42);\\n \\n \\n var popup_dd27b786056bbe3bfc8ee4d94773dddc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a25008ba07b1373b4a3f8b4318ea3afe = $(`<div id="html_a25008ba07b1373b4a3f8b4318ea3afe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd27b786056bbe3bfc8ee4d94773dddc.setContent(html_a25008ba07b1373b4a3f8b4318ea3afe);\\n \\n \\n\\n circle_marker_a8237eb8240e0a2e16ec92eb2bad5a62.bindPopup(popup_dd27b786056bbe3bfc8ee4d94773dddc)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Beech, American\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_85a446a94185c64bc7506a690a637c85 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_85a446a94185c64bc7506a690a637c85" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_85a446a94185c64bc7506a690a637c85 = L.map(\\n "map_85a446a94185c64bc7506a690a637c85",\\n {\\n center: [42.73545212957737, -73.24826491897292],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_98d84bd1b923f52670b8247c91a2e71a = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var circle_marker_ccaeeaeac9aa932a1f9e588e7c034e45 = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.723094811029982, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e25cd0d9f07b93fd59e74598176ce7bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_731eaf50247e35760c7c2e11d2ad7666 = $(`<div id="html_731eaf50247e35760c7c2e11d2ad7666" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e25cd0d9f07b93fd59e74598176ce7bd.setContent(html_731eaf50247e35760c7c2e11d2ad7666);\\n \\n \\n\\n circle_marker_ccaeeaeac9aa932a1f9e588e7c034e45.bindPopup(popup_e25cd0d9f07b93fd59e74598176ce7bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d122ab414a8ef615ecc4505f2ee5ba0 = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.607802401865856, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ef1c7a75262bd5139e5100d5c31f8cb7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ee0d23a5e2d5abc3cea8c64fec9284b = $(`<div id="html_6ee0d23a5e2d5abc3cea8c64fec9284b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef1c7a75262bd5139e5100d5c31f8cb7.setContent(html_6ee0d23a5e2d5abc3cea8c64fec9284b);\\n \\n \\n\\n circle_marker_7d122ab414a8ef615ecc4505f2ee5ba0.bindPopup(popup_ef1c7a75262bd5139e5100d5c31f8cb7)\\n ;\\n\\n \\n \\n \\n var circle_marker_01411b2070bee641d436ce7e169bf9de = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 13.049742875180646, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_dc84560e4418d246ead8205508646155 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e70f62dc3e609fc80a1c8a762cfbdc8b = $(`<div id="html_e70f62dc3e609fc80a1c8a762cfbdc8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc84560e4418d246ead8205508646155.setContent(html_e70f62dc3e609fc80a1c8a762cfbdc8b);\\n \\n \\n\\n circle_marker_01411b2070bee641d436ce7e169bf9de.bindPopup(popup_dc84560e4418d246ead8205508646155)\\n ;\\n\\n \\n \\n \\n var circle_marker_88b9bbaf7e39299a9f1d51d6c9091e27 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.124020118074668, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6ba498bef9988a497c86805c6fd2bdfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63c585ab168c60cf0f7ded95342b11e5 = $(`<div id="html_63c585ab168c60cf0f7ded95342b11e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ba498bef9988a497c86805c6fd2bdfe.setContent(html_63c585ab168c60cf0f7ded95342b11e5);\\n \\n \\n\\n circle_marker_88b9bbaf7e39299a9f1d51d6c9091e27.bindPopup(popup_6ba498bef9988a497c86805c6fd2bdfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_011fed005d408c33d1a1dab2a8882f1f = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.593479713983122, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_590399808f68a219756ec862cde09974 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ecb046f9870c03871fb03dfb86dd6ca = $(`<div id="html_8ecb046f9870c03871fb03dfb86dd6ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_590399808f68a219756ec862cde09974.setContent(html_8ecb046f9870c03871fb03dfb86dd6ca);\\n \\n \\n\\n circle_marker_011fed005d408c33d1a1dab2a8882f1f.bindPopup(popup_590399808f68a219756ec862cde09974)\\n ;\\n\\n \\n \\n \\n var circle_marker_78e01c151933efc565ab58d3541b3963 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.797255174810172, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_71889f7e3b0f4111008b28de1f86c545 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7d94ac64d6d7fcbab8d3ffc8978056c = $(`<div id="html_c7d94ac64d6d7fcbab8d3ffc8978056c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71889f7e3b0f4111008b28de1f86c545.setContent(html_c7d94ac64d6d7fcbab8d3ffc8978056c);\\n \\n \\n\\n circle_marker_78e01c151933efc565ab58d3541b3963.bindPopup(popup_71889f7e3b0f4111008b28de1f86c545)\\n ;\\n\\n \\n \\n \\n var circle_marker_74421001d9e209382dee0bfaedbeb1c5 = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.058239062071396, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a348dfed6598d677091f00728568c4e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_026a22011e2024b8f328c66b53a3d84f = $(`<div id="html_026a22011e2024b8f328c66b53a3d84f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a348dfed6598d677091f00728568c4e5.setContent(html_026a22011e2024b8f328c66b53a3d84f);\\n \\n \\n\\n circle_marker_74421001d9e209382dee0bfaedbeb1c5.bindPopup(popup_a348dfed6598d677091f00728568c4e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_f946da2fed72666d0cb22638c0666770 = L.circleMarker(\\n [42.747201204933376, -73.2685630990657],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.338999347593866, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8b431df64f3bc1eb3e4f1f70690ecc8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8846c0bc109cb59296cf79e0d010b134 = $(`<div id="html_8846c0bc109cb59296cf79e0d010b134" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b431df64f3bc1eb3e4f1f70690ecc8d.setContent(html_8846c0bc109cb59296cf79e0d010b134);\\n \\n \\n\\n circle_marker_f946da2fed72666d0cb22638c0666770.bindPopup(popup_8b431df64f3bc1eb3e4f1f70690ecc8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_d3870efde0d601c4128dc5806fc4f32a = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.333012368467128, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_58b03f9364a76e5de7e31eebdb32ac6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44421871f65a422ce986b914f5b2e5c8 = $(`<div id="html_44421871f65a422ce986b914f5b2e5c8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58b03f9364a76e5de7e31eebdb32ac6a.setContent(html_44421871f65a422ce986b914f5b2e5c8);\\n \\n \\n\\n circle_marker_d3870efde0d601c4128dc5806fc4f32a.bindPopup(popup_58b03f9364a76e5de7e31eebdb32ac6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3b377e05d0d485aa72b1aa1b3204556 = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.51351666838205, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_13f362873a98f2032f60ee7b02173ff4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4be8ee2a3d8de54475ad10f0365e83bf = $(`<div id="html_4be8ee2a3d8de54475ad10f0365e83bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13f362873a98f2032f60ee7b02173ff4.setContent(html_4be8ee2a3d8de54475ad10f0365e83bf);\\n \\n \\n\\n circle_marker_f3b377e05d0d485aa72b1aa1b3204556.bindPopup(popup_13f362873a98f2032f60ee7b02173ff4)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fd0643e33c98d74a27ca828ba71f23a = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.370193722368317, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3c8b5074eb792d4db72205066012028e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be6852b2609bee849605eb6aa506e73f = $(`<div id="html_be6852b2609bee849605eb6aa506e73f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c8b5074eb792d4db72205066012028e.setContent(html_be6852b2609bee849605eb6aa506e73f);\\n \\n \\n\\n circle_marker_3fd0643e33c98d74a27ca828ba71f23a.bindPopup(popup_3c8b5074eb792d4db72205066012028e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6aa1f9cced73514652afea31bd4e2d6a = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.158763278359629, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0761f787e90c1b8446070f34bfb12ba0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d27a66a7e1b10a2e546b044575968c1c = $(`<div id="html_d27a66a7e1b10a2e546b044575968c1c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0761f787e90c1b8446070f34bfb12ba0.setContent(html_d27a66a7e1b10a2e546b044575968c1c);\\n \\n \\n\\n circle_marker_6aa1f9cced73514652afea31bd4e2d6a.bindPopup(popup_0761f787e90c1b8446070f34bfb12ba0)\\n ;\\n\\n \\n \\n \\n var circle_marker_af8b25cbcd45fee8dd173713c0aea1c3 = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.530966601401967, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a898d1e9519d029bdf74c55ea79ae58a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f69788dc99dec3fd9b6aad7f0f774afd = $(`<div id="html_f69788dc99dec3fd9b6aad7f0f774afd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a898d1e9519d029bdf74c55ea79ae58a.setContent(html_f69788dc99dec3fd9b6aad7f0f774afd);\\n \\n \\n\\n circle_marker_af8b25cbcd45fee8dd173713c0aea1c3.bindPopup(popup_a898d1e9519d029bdf74c55ea79ae58a)\\n ;\\n\\n \\n \\n \\n var circle_marker_025e98e6a1a3be991bd6bcc2680381f4 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.3078313050504, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3ae92d7736c90aad958de2207720684e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d98cb99ca4828e7f897e1ce1264c15a = $(`<div id="html_9d98cb99ca4828e7f897e1ce1264c15a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ae92d7736c90aad958de2207720684e.setContent(html_9d98cb99ca4828e7f897e1ce1264c15a);\\n \\n \\n\\n circle_marker_025e98e6a1a3be991bd6bcc2680381f4.bindPopup(popup_3ae92d7736c90aad958de2207720684e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1cbbe95363d9924d3de7104112366b3 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a18dc602f492617d7efc95be27ce4e00 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6aafbf19b929b7154e2005a577f35d58 = $(`<div id="html_6aafbf19b929b7154e2005a577f35d58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a18dc602f492617d7efc95be27ce4e00.setContent(html_6aafbf19b929b7154e2005a577f35d58);\\n \\n \\n\\n circle_marker_b1cbbe95363d9924d3de7104112366b3.bindPopup(popup_a18dc602f492617d7efc95be27ce4e00)\\n ;\\n\\n \\n \\n \\n var circle_marker_cbba140ca5742b08ea4725f18b08d3b6 = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.878478774854901, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7451d12d5180e0ef4bf06d40c00d5916 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_98358c56df0ab8a66168680bb774d35a = $(`<div id="html_98358c56df0ab8a66168680bb774d35a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7451d12d5180e0ef4bf06d40c00d5916.setContent(html_98358c56df0ab8a66168680bb774d35a);\\n \\n \\n\\n circle_marker_cbba140ca5742b08ea4725f18b08d3b6.bindPopup(popup_7451d12d5180e0ef4bf06d40c00d5916)\\n ;\\n\\n \\n \\n \\n var circle_marker_6807e7e490ab70b50e6021d1e9abd97b = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4700209598571305, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ccdc8744d9af1c895d33fba9630b605f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d065a5c526b00f076b1d7e8886f2255c = $(`<div id="html_d065a5c526b00f076b1d7e8886f2255c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ccdc8744d9af1c895d33fba9630b605f.setContent(html_d065a5c526b00f076b1d7e8886f2255c);\\n \\n \\n\\n circle_marker_6807e7e490ab70b50e6021d1e9abd97b.bindPopup(popup_ccdc8744d9af1c895d33fba9630b605f)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c0fb28f477ac0e19f7c289e4bcba5bc = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.583497844237541, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_28e37269a5bd6aa341e774826837e4b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c28be9ee1599304ccfc2b87c6c71afee = $(`<div id="html_c28be9ee1599304ccfc2b87c6c71afee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_28e37269a5bd6aa341e774826837e4b2.setContent(html_c28be9ee1599304ccfc2b87c6c71afee);\\n \\n \\n\\n circle_marker_2c0fb28f477ac0e19f7c289e4bcba5bc.bindPopup(popup_28e37269a5bd6aa341e774826837e4b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec448c082ca4fd788273487cdc83f28c = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4c3f14202d848cc4a7f54aa33e2add8a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b8b5c7ca9fb6113dd915edde8d6609b = $(`<div id="html_7b8b5c7ca9fb6113dd915edde8d6609b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c3f14202d848cc4a7f54aa33e2add8a.setContent(html_7b8b5c7ca9fb6113dd915edde8d6609b);\\n \\n \\n\\n circle_marker_ec448c082ca4fd788273487cdc83f28c.bindPopup(popup_4c3f14202d848cc4a7f54aa33e2add8a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c403bf753cfa3968a91e1389ff0ee8a3 = L.circleMarker(\\n [42.74539359743371, -73.27594530262718],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.291859587312052, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5a38cbae5fa37cebd848384f9527f813 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8338c6e661027afb3caef818bbb06ce = $(`<div id="html_f8338c6e661027afb3caef818bbb06ce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a38cbae5fa37cebd848384f9527f813.setContent(html_f8338c6e661027afb3caef818bbb06ce);\\n \\n \\n\\n circle_marker_c403bf753cfa3968a91e1389ff0ee8a3.bindPopup(popup_5a38cbae5fa37cebd848384f9527f813)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9ece581f6cef73746520f664a2c6e55 = L.circleMarker(\\n [42.74539347231661, -73.2771756878084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 11.213046198183495, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_121e91ba3e7c8ed977b3d717acf89ff6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_834eaf9c3da698f4e5e1072b730f33cd = $(`<div id="html_834eaf9c3da698f4e5e1072b730f33cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_121e91ba3e7c8ed977b3d717acf89ff6.setContent(html_834eaf9c3da698f4e5e1072b730f33cd);\\n \\n \\n\\n circle_marker_e9ece581f6cef73746520f664a2c6e55.bindPopup(popup_121e91ba3e7c8ed977b3d717acf89ff6)\\n ;\\n\\n \\n \\n \\n var circle_marker_15aa7d67cfe924e258823d3b91951aa0 = L.circleMarker(\\n [42.74539370938061, -73.27471491744124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.869286963719311, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4c0c972ab371bc6ca15448bcd4302be9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fa144fc2417ddc67296cf347d145e0f7 = $(`<div id="html_fa144fc2417ddc67296cf347d145e0f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c0c972ab371bc6ca15448bcd4302be9.setContent(html_fa144fc2417ddc67296cf347d145e0f7);\\n \\n \\n\\n circle_marker_15aa7d67cfe924e258823d3b91951aa0.bindPopup(popup_4c0c972ab371bc6ca15448bcd4302be9)\\n ;\\n\\n \\n \\n \\n var circle_marker_96e6375ee024c337da49c7cb0449090d = L.circleMarker(\\n [42.74539380815728, -73.27348453225115],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.140011726956917, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4a84a9b638cf345de09b300a9334a93f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a08eb8c69f8fa69a404a281a4b760961 = $(`<div id="html_a08eb8c69f8fa69a404a281a4b760961" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a84a9b638cf345de09b300a9334a93f.setContent(html_a08eb8c69f8fa69a404a281a4b760961);\\n \\n \\n\\n circle_marker_96e6375ee024c337da49c7cb0449090d.bindPopup(popup_4a84a9b638cf345de09b300a9334a93f)\\n ;\\n\\n \\n \\n \\n var circle_marker_32a71612fc6c6d0850719994853e8f6d = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.287732724180174, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ef331ca8718f92c30bc422ad1252e379 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d0077b6d3354ce485ce65b31177396ea = $(`<div id="html_d0077b6d3354ce485ce65b31177396ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef331ca8718f92c30bc422ad1252e379.setContent(html_d0077b6d3354ce485ce65b31177396ea);\\n \\n \\n\\n circle_marker_32a71612fc6c6d0850719994853e8f6d.bindPopup(popup_ef331ca8718f92c30bc422ad1252e379)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba2b9ceb31a4208e41cb3c256906ad6f = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.296739856991561, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d430cd6bb8115917d43f45b61c374244 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_989dbe0a39cb9f911d3825a1a410d490 = $(`<div id="html_989dbe0a39cb9f911d3825a1a410d490" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d430cd6bb8115917d43f45b61c374244.setContent(html_989dbe0a39cb9f911d3825a1a410d490);\\n \\n \\n\\n circle_marker_ba2b9ceb31a4208e41cb3c256906ad6f.bindPopup(popup_d430cd6bb8115917d43f45b61c374244)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c88323e76cce04fd2c757a8d725da57 = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b94e330c095d18933c14da0c881057a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_84f47ba649733a8b58cf6124e18c9fe8 = $(`<div id="html_84f47ba649733a8b58cf6124e18c9fe8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b94e330c095d18933c14da0c881057a0.setContent(html_84f47ba649733a8b58cf6124e18c9fe8);\\n \\n \\n\\n circle_marker_6c88323e76cce04fd2c757a8d725da57.bindPopup(popup_b94e330c095d18933c14da0c881057a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_81f0316ba8fc78f350bcf3cfd944523a = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1111a0419798c9acfab52b85e88b6a13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_951fdf3324345a263988b8a8c77dc267 = $(`<div id="html_951fdf3324345a263988b8a8c77dc267" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1111a0419798c9acfab52b85e88b6a13.setContent(html_951fdf3324345a263988b8a8c77dc267);\\n \\n \\n\\n circle_marker_81f0316ba8fc78f350bcf3cfd944523a.bindPopup(popup_1111a0419798c9acfab52b85e88b6a13)\\n ;\\n\\n \\n \\n \\n var circle_marker_2faa2439c1127fc354a175e1db5a3fdf = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.432750982580687, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c93cc91fd64e1101458def467a9323e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_feae0a2cdb3421438f2b90610df87e89 = $(`<div id="html_feae0a2cdb3421438f2b90610df87e89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c93cc91fd64e1101458def467a9323e9.setContent(html_feae0a2cdb3421438f2b90610df87e89);\\n \\n \\n\\n circle_marker_2faa2439c1127fc354a175e1db5a3fdf.bindPopup(popup_c93cc91fd64e1101458def467a9323e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff53d54c1d9b7dce9b097109af48d33a = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.382025607773058, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e680218df7e1abacc8365a69e14c6544 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e7ce94df797eadb2171672615facedc = $(`<div id="html_2e7ce94df797eadb2171672615facedc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e680218df7e1abacc8365a69e14c6544.setContent(html_2e7ce94df797eadb2171672615facedc);\\n \\n \\n\\n circle_marker_ff53d54c1d9b7dce9b097109af48d33a.bindPopup(popup_e680218df7e1abacc8365a69e14c6544)\\n ;\\n\\n \\n \\n \\n var circle_marker_41223303a5f247aa0548358f2c3a39c0 = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.673807981960539, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_dbe60ab618c73b39acf75e19b4d3c642 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0d88ff02f05a07f0fd7e014ef2f29cb = $(`<div id="html_b0d88ff02f05a07f0fd7e014ef2f29cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dbe60ab618c73b39acf75e19b4d3c642.setContent(html_b0d88ff02f05a07f0fd7e014ef2f29cb);\\n \\n \\n\\n circle_marker_41223303a5f247aa0548358f2c3a39c0.bindPopup(popup_dbe60ab618c73b39acf75e19b4d3c642)\\n ;\\n\\n \\n \\n \\n var circle_marker_6796bd46565ee58cf85bf36f69f07d2e = L.circleMarker(\\n [42.74539412424261, -73.26364145064247],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.225151993843567, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a47a7f14c0b2260d7e8e35e7bd551a68 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da16f55ecc0eb7d78ba059d7a7dda11c = $(`<div id="html_da16f55ecc0eb7d78ba059d7a7dda11c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a47a7f14c0b2260d7e8e35e7bd551a68.setContent(html_da16f55ecc0eb7d78ba059d7a7dda11c);\\n \\n \\n\\n circle_marker_6796bd46565ee58cf85bf36f69f07d2e.bindPopup(popup_a47a7f14c0b2260d7e8e35e7bd551a68)\\n ;\\n\\n \\n \\n \\n var circle_marker_72a5d748d5a3c1f897e117de57a1264e = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.989422804014327, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2aec1c19724025ce0c9969f2940abf13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a14433da9b1b66beb101210835783382 = $(`<div id="html_a14433da9b1b66beb101210835783382" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2aec1c19724025ce0c9969f2940abf13.setContent(html_a14433da9b1b66beb101210835783382);\\n \\n \\n\\n circle_marker_72a5d748d5a3c1f897e117de57a1264e.bindPopup(popup_2aec1c19724025ce0c9969f2940abf13)\\n ;\\n\\n \\n \\n \\n var circle_marker_214bf495492ed9e18272f11ec5b1a527 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ede696a97435b5c4f0d184d04199ad31 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c14427626ac3efa693eb8987e76d7b07 = $(`<div id="html_c14427626ac3efa693eb8987e76d7b07" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ede696a97435b5c4f0d184d04199ad31.setContent(html_c14427626ac3efa693eb8987e76d7b07);\\n \\n \\n\\n circle_marker_214bf495492ed9e18272f11ec5b1a527.bindPopup(popup_ede696a97435b5c4f0d184d04199ad31)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ed42b6fd3c322ecc6ee487087e8a788 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.222008245644752, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b8c810c808023abee144f25c87cb1a9b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a96160d02d8123946765f35e9af2338 = $(`<div id="html_1a96160d02d8123946765f35e9af2338" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b8c810c808023abee144f25c87cb1a9b.setContent(html_1a96160d02d8123946765f35e9af2338);\\n \\n \\n\\n circle_marker_6ed42b6fd3c322ecc6ee487087e8a788.bindPopup(popup_b8c810c808023abee144f25c87cb1a9b)\\n ;\\n\\n \\n \\n \\n var circle_marker_622b318a0fe8682983205f42dc68a398 = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c7402db24544838080563c25092917bb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_91c306d425695c7dcd92db1a0719c134 = $(`<div id="html_91c306d425695c7dcd92db1a0719c134" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c7402db24544838080563c25092917bb.setContent(html_91c306d425695c7dcd92db1a0719c134);\\n \\n \\n\\n circle_marker_622b318a0fe8682983205f42dc68a398.bindPopup(popup_c7402db24544838080563c25092917bb)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c86f3cf70bff66b87e56c2f19209231 = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ed17c5b6fa8849953dbaa6cb7ac43e60 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fbcc5c13aec0562b3db91fd8316d6d66 = $(`<div id="html_fbcc5c13aec0562b3db91fd8316d6d66" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed17c5b6fa8849953dbaa6cb7ac43e60.setContent(html_fbcc5c13aec0562b3db91fd8316d6d66);\\n \\n \\n\\n circle_marker_7c86f3cf70bff66b87e56c2f19209231.bindPopup(popup_ed17c5b6fa8849953dbaa6cb7ac43e60)\\n ;\\n\\n \\n \\n \\n var circle_marker_f07d4d28f57de3be57e7fe115e0a7936 = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_619d550e36972fcb2a3b5ffe166712ad = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1ff6a1a4913a7e7e6d72d4dd670e4be = $(`<div id="html_c1ff6a1a4913a7e7e6d72d4dd670e4be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_619d550e36972fcb2a3b5ffe166712ad.setContent(html_c1ff6a1a4913a7e7e6d72d4dd670e4be);\\n \\n \\n\\n circle_marker_f07d4d28f57de3be57e7fe115e0a7936.bindPopup(popup_619d550e36972fcb2a3b5ffe166712ad)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a702f181763d9f9c5c9bc0fdebfb30f = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b1edfbaa854aa87ceae09ddd0c104e8c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4c05b11504c7c3eb798b3f7fb366199 = $(`<div id="html_a4c05b11504c7c3eb798b3f7fb366199" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1edfbaa854aa87ceae09ddd0c104e8c.setContent(html_a4c05b11504c7c3eb798b3f7fb366199);\\n \\n \\n\\n circle_marker_5a702f181763d9f9c5c9bc0fdebfb30f.bindPopup(popup_b1edfbaa854aa87ceae09ddd0c104e8c)\\n ;\\n\\n \\n \\n \\n var circle_marker_a1ffd8d616a608ec8556740a2aacab1f = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.756324418739616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_88dbc8633596884b61c587601422d4df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a16d9d59f7251fb22e34993d895cc72 = $(`<div id="html_0a16d9d59f7251fb22e34993d895cc72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88dbc8633596884b61c587601422d4df.setContent(html_0a16d9d59f7251fb22e34993d895cc72);\\n \\n \\n\\n circle_marker_a1ffd8d616a608ec8556740a2aacab1f.bindPopup(popup_88dbc8633596884b61c587601422d4df)\\n ;\\n\\n \\n \\n \\n var circle_marker_22602c175dc1cfee4c27d01c79fc7d28 = L.circleMarker(\\n [42.74358657602363, -73.27471463051425],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.530966601401967, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_db3d11e8aa1f2826b0369fb9ee005d3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b0b7e14bd7ec81d2243cbd877dad372 = $(`<div id="html_9b0b7e14bd7ec81d2243cbd877dad372" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db3d11e8aa1f2826b0369fb9ee005d3e.setContent(html_9b0b7e14bd7ec81d2243cbd877dad372);\\n \\n \\n\\n circle_marker_22602c175dc1cfee4c27d01c79fc7d28.bindPopup(popup_db3d11e8aa1f2826b0369fb9ee005d3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_9649f43cf9af952f8b983840d1356c4e = L.circleMarker(\\n [42.74358667479628, -73.27348428119001],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.32636489145962, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a49eb5b959bdb85b51c18517b848a2dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c40f033aeaf66da07d24047a8ca8cc3 = $(`<div id="html_8c40f033aeaf66da07d24047a8ca8cc3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a49eb5b959bdb85b51c18517b848a2dd.setContent(html_8c40f033aeaf66da07d24047a8ca8cc3);\\n \\n \\n\\n circle_marker_9649f43cf9af952f8b983840d1356c4e.bindPopup(popup_a49eb5b959bdb85b51c18517b848a2dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_720639b08588500e007cf07b59455766 = L.circleMarker(\\n [42.74358676039927, -73.27225393186212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.3078313050504, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3a8f8c12ac9f4e7d988896fcd4c08c49 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5d46987cfad2b00cf9caa309a8855a94 = $(`<div id="html_5d46987cfad2b00cf9caa309a8855a94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a8f8c12ac9f4e7d988896fcd4c08c49.setContent(html_5d46987cfad2b00cf9caa309a8855a94);\\n \\n \\n\\n circle_marker_720639b08588500e007cf07b59455766.bindPopup(popup_3a8f8c12ac9f4e7d988896fcd4c08c49)\\n ;\\n\\n \\n \\n \\n var circle_marker_eeb91f2df723db5052000e9fe01a94f4 = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.222008245644752, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1572a1c313e5588aad39491c99912944 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_88d2e631dcefb8bdb258bae180bf8ae1 = $(`<div id="html_88d2e631dcefb8bdb258bae180bf8ae1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1572a1c313e5588aad39491c99912944.setContent(html_88d2e631dcefb8bdb258bae180bf8ae1);\\n \\n \\n\\n circle_marker_eeb91f2df723db5052000e9fe01a94f4.bindPopup(popup_1572a1c313e5588aad39491c99912944)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f5cd3d381585a88750af4538b853a96 = L.circleMarker(\\n [42.74358689209615, -73.26979323319746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.420755373261929, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_68fa386a2bc9b05b09b868f7e915d568 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70c14587608df14c2d0df58d4935d242 = $(`<div id="html_70c14587608df14c2d0df58d4935d242" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68fa386a2bc9b05b09b868f7e915d568.setContent(html_70c14587608df14c2d0df58d4935d242);\\n \\n \\n\\n circle_marker_6f5cd3d381585a88750af4538b853a96.bindPopup(popup_68fa386a2bc9b05b09b868f7e915d568)\\n ;\\n\\n \\n \\n \\n var circle_marker_0f41a1342b5c04060b3e3102606d2da5 = L.circleMarker(\\n [42.743586938190056, -73.26856288386173],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1a802019cdbae9539fe7e2b2fd79db67 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5d7e74f5e5296c36d44279680cbd353c = $(`<div id="html_5d7e74f5e5296c36d44279680cbd353c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a802019cdbae9539fe7e2b2fd79db67.setContent(html_5d7e74f5e5296c36d44279680cbd353c);\\n \\n \\n\\n circle_marker_0f41a1342b5c04060b3e3102606d2da5.bindPopup(popup_1a802019cdbae9539fe7e2b2fd79db67)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c20651071aec0071e7805618f742790 = L.circleMarker(\\n [42.74358697111428, -73.26733253452443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fb94f0f42946dde544d19a5f677918ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_955759cc7e71bc898720c66956193cef = $(`<div id="html_955759cc7e71bc898720c66956193cef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb94f0f42946dde544d19a5f677918ec.setContent(html_955759cc7e71bc898720c66956193cef);\\n \\n \\n\\n circle_marker_3c20651071aec0071e7805618f742790.bindPopup(popup_fb94f0f42946dde544d19a5f677918ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_5fbbfe68180507dc63573568800a3e42 = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.231677632421503, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_69b4f009602bf7d33e25e86b4bbc51f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be2d1d63d57e3b97d99f5ca11ec7f668 = $(`<div id="html_be2d1d63d57e3b97d99f5ca11ec7f668" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69b4f009602bf7d33e25e86b4bbc51f3.setContent(html_be2d1d63d57e3b97d99f5ca11ec7f668);\\n \\n \\n\\n circle_marker_5fbbfe68180507dc63573568800a3e42.bindPopup(popup_69b4f009602bf7d33e25e86b4bbc51f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d9f0c4a3cfbb7addd20bb06e855e01d4 = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.158763278359629, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8ec3011a4255af84ae474611cecd1e8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c1f8105b6360cccde01a502a73c4683 = $(`<div id="html_5c1f8105b6360cccde01a502a73c4683" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ec3011a4255af84ae474611cecd1e8b.setContent(html_5c1f8105b6360cccde01a502a73c4683);\\n \\n \\n\\n circle_marker_d9f0c4a3cfbb7addd20bb06e855e01d4.bindPopup(popup_8ec3011a4255af84ae474611cecd1e8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_afa86f2589cd8aeef599cc85dcaa9ea4 = L.circleMarker(\\n [42.74358699086881, -73.26364148650833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bae2b4b1b083d9f93534132d73dcc4e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d5d54afc61030f1f68f2980cdb6c5cb = $(`<div id="html_4d5d54afc61030f1f68f2980cdb6c5cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bae2b4b1b083d9f93534132d73dcc4e5.setContent(html_4d5d54afc61030f1f68f2980cdb6c5cb);\\n \\n \\n\\n circle_marker_afa86f2589cd8aeef599cc85dcaa9ea4.bindPopup(popup_bae2b4b1b083d9f93534132d73dcc4e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ac1b9ced4dea367da314e55b76ec4ef = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.076507779746499, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3e6759970a2a8ae861e013b6bf3a2bf7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b48a1aa4dbc2f75766104fcbc684e86 = $(`<div id="html_5b48a1aa4dbc2f75766104fcbc684e86" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e6759970a2a8ae861e013b6bf3a2bf7.setContent(html_5b48a1aa4dbc2f75766104fcbc684e86);\\n \\n \\n\\n circle_marker_6ac1b9ced4dea367da314e55b76ec4ef.bindPopup(popup_3e6759970a2a8ae861e013b6bf3a2bf7)\\n ;\\n\\n \\n \\n \\n var circle_marker_aaaef45c8e5e947eacdf6c84dfe7ec30 = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.170882945826411, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7e9474688cbb77e20a7312472db0887b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bff49bdf9d65535860dfdc63d8ea7168 = $(`<div id="html_bff49bdf9d65535860dfdc63d8ea7168" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e9474688cbb77e20a7312472db0887b.setContent(html_bff49bdf9d65535860dfdc63d8ea7168);\\n \\n \\n\\n circle_marker_aaaef45c8e5e947eacdf6c84dfe7ec30.bindPopup(popup_7e9474688cbb77e20a7312472db0887b)\\n ;\\n\\n \\n \\n \\n var circle_marker_c862ab4eebb07180da03c41b47b5d47d = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.890312181373172, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_99fc48abdc9b34cb8bb8da3f222a0663 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be8736d052aab9c92fe6cd7fa05a383d = $(`<div id="html_be8736d052aab9c92fe6cd7fa05a383d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99fc48abdc9b34cb8bb8da3f222a0663.setContent(html_be8736d052aab9c92fe6cd7fa05a383d);\\n \\n \\n\\n circle_marker_c862ab4eebb07180da03c41b47b5d47d.bindPopup(popup_99fc48abdc9b34cb8bb8da3f222a0663)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc045cc9c7e6959674f39ddc44e6b3a7 = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.917270272703197, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e3cf41acd00e48c30367ca261d728ccd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_496b7f86984bd4cff22379c08fa68f6f = $(`<div id="html_496b7f86984bd4cff22379c08fa68f6f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3cf41acd00e48c30367ca261d728ccd.setContent(html_496b7f86984bd4cff22379c08fa68f6f);\\n \\n \\n\\n circle_marker_dc045cc9c7e6959674f39ddc44e6b3a7.bindPopup(popup_e3cf41acd00e48c30367ca261d728ccd)\\n ;\\n\\n \\n \\n \\n var circle_marker_051a0c1b11067bf618d6f59699693c8a = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2cfa6ef5ae163a6fd0fd25d83f615a12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b36e32b04be190c53573a38919dafb04 = $(`<div id="html_b36e32b04be190c53573a38919dafb04" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2cfa6ef5ae163a6fd0fd25d83f615a12.setContent(html_b36e32b04be190c53573a38919dafb04);\\n \\n \\n\\n circle_marker_051a0c1b11067bf618d6f59699693c8a.bindPopup(popup_2cfa6ef5ae163a6fd0fd25d83f615a12)\\n ;\\n\\n \\n \\n \\n var circle_marker_0988d149feba830c4879bf93fe035fa5 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_afbee48e53e8559ca69d9dae8a711776 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_328ff4a29c7d9cffcafca9300eb98615 = $(`<div id="html_328ff4a29c7d9cffcafca9300eb98615" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_afbee48e53e8559ca69d9dae8a711776.setContent(html_328ff4a29c7d9cffcafca9300eb98615);\\n \\n \\n\\n circle_marker_0988d149feba830c4879bf93fe035fa5.bindPopup(popup_afbee48e53e8559ca69d9dae8a711776)\\n ;\\n\\n \\n \\n \\n var circle_marker_a998455c40b22bf39c34c29be53d595a = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e5084bfb556e26f02925d8437632cdd5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8215e94fc97a8b207c1b7d30ecb1449 = $(`<div id="html_c8215e94fc97a8b207c1b7d30ecb1449" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5084bfb556e26f02925d8437632cdd5.setContent(html_c8215e94fc97a8b207c1b7d30ecb1449);\\n \\n \\n\\n circle_marker_a998455c40b22bf39c34c29be53d595a.bindPopup(popup_e5084bfb556e26f02925d8437632cdd5)\\n ;\\n\\n \\n \\n \\n var circle_marker_30ac2f40a4b26c805b8b74cb3f1bd92a = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d06ea66884f67ffef06447ad7264ba59 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32e4acca35602ae75083e314c5ce328f = $(`<div id="html_32e4acca35602ae75083e314c5ce328f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d06ea66884f67ffef06447ad7264ba59.setContent(html_32e4acca35602ae75083e314c5ce328f);\\n \\n \\n\\n circle_marker_30ac2f40a4b26c805b8b74cb3f1bd92a.bindPopup(popup_d06ea66884f67ffef06447ad7264ba59)\\n ;\\n\\n \\n \\n \\n var circle_marker_5800056c2e12d327dbd8fe0c3874453d = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ee3b0477d1068b3df777eefd5a0f978f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_863c4584bdab22f82a20079722cdcefb = $(`<div id="html_863c4584bdab22f82a20079722cdcefb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee3b0477d1068b3df777eefd5a0f978f.setContent(html_863c4584bdab22f82a20079722cdcefb);\\n \\n \\n\\n circle_marker_5800056c2e12d327dbd8fe0c3874453d.bindPopup(popup_ee3b0477d1068b3df777eefd5a0f978f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8d299ef2e794bbafcf0bfb824086537 = L.circleMarker(\\n [42.7417795414353, -73.27348403014923],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.387831891992912, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_749acab9accd5ff1b0b014d8a38ec08e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13f4eb04b51fc29d583f0a6b92083a3b = $(`<div id="html_13f4eb04b51fc29d583f0a6b92083a3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_749acab9accd5ff1b0b014d8a38ec08e.setContent(html_13f4eb04b51fc29d583f0a6b92083a3b);\\n \\n \\n\\n circle_marker_f8d299ef2e794bbafcf0bfb824086537.bindPopup(popup_749acab9accd5ff1b0b014d8a38ec08e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ebf43f0941be29b57baf27614efa5dc6 = L.circleMarker(\\n [42.74177962703481, -73.2722537166843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.377736139048903, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_843c3f87b5bd55e0c15f3b47b8b31533 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4e72023170ed76268781114c6ffc6f1 = $(`<div id="html_a4e72023170ed76268781114c6ffc6f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_843c3f87b5bd55e0c15f3b47b8b31533.setContent(html_a4e72023170ed76268781114c6ffc6f1);\\n \\n \\n\\n circle_marker_ebf43f0941be29b57baf27614efa5dc6.bindPopup(popup_843c3f87b5bd55e0c15f3b47b8b31533)\\n ;\\n\\n \\n \\n \\n var circle_marker_d72ff03c4f2008740e974ebfdc6889c6 = L.circleMarker(\\n [42.74177969946516, -73.27102340321625],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.478115991081385, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f1621d9fc8f5a5f8b3303b31a699b03f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b7174c9d313b315827a70c9c4c3fea1 = $(`<div id="html_9b7174c9d313b315827a70c9c4c3fea1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1621d9fc8f5a5f8b3303b31a699b03f.setContent(html_9b7174c9d313b315827a70c9c4c3fea1);\\n \\n \\n\\n circle_marker_d72ff03c4f2008740e974ebfdc6889c6.bindPopup(popup_f1621d9fc8f5a5f8b3303b31a699b03f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f87c68541c174b827fc181c70b4fe50f = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.890312181373172, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_219aadc4a4f447aa07e5719bb2d4d813 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_028d7552d65a3eddfe0aba90df4e0145 = $(`<div id="html_028d7552d65a3eddfe0aba90df4e0145" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_219aadc4a4f447aa07e5719bb2d4d813.setContent(html_028d7552d65a3eddfe0aba90df4e0145);\\n \\n \\n\\n circle_marker_f87c68541c174b827fc181c70b4fe50f.bindPopup(popup_219aadc4a4f447aa07e5719bb2d4d813)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee42d7a2c1adf497865745e71a37d3df = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4f4f8ad2a47b3c7443b3a238fc2eb603 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a893d5ea049d9acb980d6b02cd3046eb = $(`<div id="html_a893d5ea049d9acb980d6b02cd3046eb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f4f8ad2a47b3c7443b3a238fc2eb603.setContent(html_a893d5ea049d9acb980d6b02cd3046eb);\\n \\n \\n\\n circle_marker_ee42d7a2c1adf497865745e71a37d3df.bindPopup(popup_4f4f8ad2a47b3c7443b3a238fc2eb603)\\n ;\\n\\n \\n \\n \\n var circle_marker_765d2a55d1ed545391f841240db92791 = L.circleMarker(\\n [42.74177983774129, -73.26733246279849],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.234075889688375, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6768c754cd67748b69c7444c9966df83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7804f0d20ca8446aa9cf5580d8554551 = $(`<div id="html_7804f0d20ca8446aa9cf5580d8554551" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6768c754cd67748b69c7444c9966df83.setContent(html_7804f0d20ca8446aa9cf5580d8554551);\\n \\n \\n\\n circle_marker_765d2a55d1ed545391f841240db92791.bindPopup(popup_6768c754cd67748b69c7444c9966df83)\\n ;\\n\\n \\n \\n \\n var circle_marker_673d99da19621eaef270fc6c13d9eed3 = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.918778161951929, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fac8a28375cdc047b4804c61b5532740 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb9ac325385814553ac00888429a2776 = $(`<div id="html_cb9ac325385814553ac00888429a2776" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fac8a28375cdc047b4804c61b5532740.setContent(html_cb9ac325385814553ac00888429a2776);\\n \\n \\n\\n circle_marker_673d99da19621eaef270fc6c13d9eed3.bindPopup(popup_fac8a28375cdc047b4804c61b5532740)\\n ;\\n\\n \\n \\n \\n var circle_marker_21d7a32db6d3584ffa739f5e05d3bfda = L.circleMarker(\\n [42.7417798640796, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.382025607773058, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a4a6da9095fbead393bb27933b657c8e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_726d7f00b0f2a3a7e13457e4ca2cdd82 = $(`<div id="html_726d7f00b0f2a3a7e13457e4ca2cdd82" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4a6da9095fbead393bb27933b657c8e.setContent(html_726d7f00b0f2a3a7e13457e4ca2cdd82);\\n \\n \\n\\n circle_marker_21d7a32db6d3584ffa739f5e05d3bfda.bindPopup(popup_a4a6da9095fbead393bb27933b657c8e)\\n ;\\n\\n \\n \\n \\n var circle_marker_1885b4260a15cf6578ae9a3fafa951a6 = L.circleMarker(\\n [42.74177985749502, -73.26364152237132],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.997464891746821, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_56baa6320c1d9bb05050e9c23058ca76 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fab3d28c69ca0ee24a1d381f25ab8667 = $(`<div id="html_fab3d28c69ca0ee24a1d381f25ab8667" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_56baa6320c1d9bb05050e9c23058ca76.setContent(html_fab3d28c69ca0ee24a1d381f25ab8667);\\n \\n \\n\\n circle_marker_1885b4260a15cf6578ae9a3fafa951a6.bindPopup(popup_56baa6320c1d9bb05050e9c23058ca76)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c905e666201e260ec7b5378fee47f5f = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.781222885281108, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3303732b2eb2b553899edc813f7f9e33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_62be5e4f7d2f07148c847305c451b876 = $(`<div id="html_62be5e4f7d2f07148c847305c451b876" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3303732b2eb2b553899edc813f7f9e33.setContent(html_62be5e4f7d2f07148c847305c451b876);\\n \\n \\n\\n circle_marker_0c905e666201e260ec7b5378fee47f5f.bindPopup(popup_3303732b2eb2b553899edc813f7f9e33)\\n ;\\n\\n \\n \\n \\n var circle_marker_d21bbf0716378dbfa6baa5d0d2ec7902 = L.circleMarker(\\n [42.7417798048184, -73.2611808954216],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.499039842323396, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8b04794b59559fb8618d5c8ed79b0fb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bdd36559bd5d2b8599c74979b18f777 = $(`<div id="html_1bdd36559bd5d2b8599c74979b18f777" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b04794b59559fb8618d5c8ed79b0fb1.setContent(html_1bdd36559bd5d2b8599c74979b18f777);\\n \\n \\n\\n circle_marker_d21bbf0716378dbfa6baa5d0d2ec7902.bindPopup(popup_8b04794b59559fb8618d5c8ed79b0fb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6303ccb749126ecf73ef8d4a0d2f2a1 = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.432750982580687, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0da039573cf4e30d8deb92296d384772 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1cb708b1992b2c2de3c20f02255b2b9 = $(`<div id="html_c1cb708b1992b2c2de3c20f02255b2b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0da039573cf4e30d8deb92296d384772.setContent(html_c1cb708b1992b2c2de3c20f02255b2b9);\\n \\n \\n\\n circle_marker_e6303ccb749126ecf73ef8d4a0d2f2a1.bindPopup(popup_0da039573cf4e30d8deb92296d384772)\\n ;\\n\\n \\n \\n \\n var circle_marker_58f8a3d75831d2f92e939b38f8504010 = L.circleMarker(\\n [42.74177969946516, -73.25872026847817],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.950743503369154, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_54bd4a253667550efc07290640acac6b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_98e72807fb5edc0ffda2045525dcc412 = $(`<div id="html_98e72807fb5edc0ffda2045525dcc412" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54bd4a253667550efc07290640acac6b.setContent(html_98e72807fb5edc0ffda2045525dcc412);\\n \\n \\n\\n circle_marker_58f8a3d75831d2f92e939b38f8504010.bindPopup(popup_54bd4a253667550efc07290640acac6b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3625d2b47e247a8502878a025456a2ba = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b6d2231b7975efb629b5132b2ff2a5c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c29a5f88af5f7cdd17a76a9c62aebbb5 = $(`<div id="html_c29a5f88af5f7cdd17a76a9c62aebbb5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b6d2231b7975efb629b5132b2ff2a5c7.setContent(html_c29a5f88af5f7cdd17a76a9c62aebbb5);\\n \\n \\n\\n circle_marker_3625d2b47e247a8502878a025456a2ba.bindPopup(popup_b6d2231b7975efb629b5132b2ff2a5c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3b4f2bb2b8c53dd3015b047085cc59c = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.781222885281108, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7317bfe026151793588ce20117e459f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ca71049b1bff56e053d56b4202f0cf67 = $(`<div id="html_ca71049b1bff56e053d56b4202f0cf67" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7317bfe026151793588ce20117e459f2.setContent(html_ca71049b1bff56e053d56b4202f0cf67);\\n \\n \\n\\n circle_marker_f3b4f2bb2b8c53dd3015b047085cc59c.bindPopup(popup_7317bfe026151793588ce20117e459f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_7410e766246fb8d651c6d2cad2f21500 = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e65a85201418963e91acc494c0d982d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edadd6c6656c49b71e71225ade609a35 = $(`<div id="html_edadd6c6656c49b71e71225ade609a35" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e65a85201418963e91acc494c0d982d9.setContent(html_edadd6c6656c49b71e71225ade609a35);\\n \\n \\n\\n circle_marker_7410e766246fb8d651c6d2cad2f21500.bindPopup(popup_e65a85201418963e91acc494c0d982d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_5ea5a97dc599b65ac2c2131c4cefe870 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.764051215546116, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_be93cb4309f6181b4bf5e7ceabb01402 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce4b1c685a51d600b7e4ec5b9cf47e87 = $(`<div id="html_ce4b1c685a51d600b7e4ec5b9cf47e87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be93cb4309f6181b4bf5e7ceabb01402.setContent(html_ce4b1c685a51d600b7e4ec5b9cf47e87);\\n \\n \\n\\n circle_marker_5ea5a97dc599b65ac2c2131c4cefe870.bindPopup(popup_be93cb4309f6181b4bf5e7ceabb01402)\\n ;\\n\\n \\n \\n \\n var circle_marker_864583f273b115a0a9610a2a32ad8507 = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.201570947860099, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0ed055167be17a4a574abdbd9a792de5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58768ff28a8f064a4685c631e9a098ef = $(`<div id="html_58768ff28a8f064a4685c631e9a098ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0ed055167be17a4a574abdbd9a792de5.setContent(html_58768ff28a8f064a4685c631e9a098ef);\\n \\n \\n\\n circle_marker_864583f273b115a0a9610a2a32ad8507.bindPopup(popup_0ed055167be17a4a574abdbd9a792de5)\\n ;\\n\\n \\n \\n \\n var circle_marker_470f24450dc7bc27a69e269285d13d51 = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.997417539138688, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_be8db58b42afb84d17fabd2530f97f85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00f317e9ed9e09f55fa8cd85d15aaa45 = $(`<div id="html_00f317e9ed9e09f55fa8cd85d15aaa45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be8db58b42afb84d17fabd2530f97f85.setContent(html_00f317e9ed9e09f55fa8cd85d15aaa45);\\n \\n \\n\\n circle_marker_470f24450dc7bc27a69e269285d13d51.bindPopup(popup_be8db58b42afb84d17fabd2530f97f85)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1cd1409ac3033ff7196a371a562d341 = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f9e7f47544d3d00760ac2ff5ede6ed0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_573f113e4d618b08adf534bcc8f8b631 = $(`<div id="html_573f113e4d618b08adf534bcc8f8b631" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9e7f47544d3d00760ac2ff5ede6ed0d.setContent(html_573f113e4d618b08adf534bcc8f8b631);\\n \\n \\n\\n circle_marker_f1cd1409ac3033ff7196a371a562d341.bindPopup(popup_f9e7f47544d3d00760ac2ff5ede6ed0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_d700fe7529be8c99acc5892e59b95898 = L.circleMarker(\\n [42.73997267144673, -73.26856266869262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.4820448144285745, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f8c1f0602571311810a7a2c8f1b3e7fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53fe7157b628a5e7856184e0ca567c81 = $(`<div id="html_53fe7157b628a5e7856184e0ca567c81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f8c1f0602571311810a7a2c8f1b3e7fe.setContent(html_53fe7157b628a5e7856184e0ca567c81);\\n \\n \\n\\n circle_marker_d700fe7529be8c99acc5892e59b95898.bindPopup(popup_f8c1f0602571311810a7a2c8f1b3e7fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1de18cd4cda885e7bee6830b0b29bea = L.circleMarker(\\n [42.73997270436829, -73.26733239107837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.652426491681278, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f9878519e82477534766c2dcad319232 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6bab192319ba0fe870b50c4af4ce098d = $(`<div id="html_6bab192319ba0fe870b50c4af4ce098d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9878519e82477534766c2dcad319232.setContent(html_6bab192319ba0fe870b50c4af4ce098d);\\n \\n \\n\\n circle_marker_d1de18cd4cda885e7bee6830b0b29bea.bindPopup(popup_f9878519e82477534766c2dcad319232)\\n ;\\n\\n \\n \\n \\n var circle_marker_709f7b7b9b9f10b91ab69f508f3ce51e = L.circleMarker(\\n [42.73997272412122, -73.26610211346305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.257165172872317, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e3eb3f0ca9c556b269b56b6785385c6c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dbf384247a6f8c7942770ed0dbb965f4 = $(`<div id="html_dbf384247a6f8c7942770ed0dbb965f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3eb3f0ca9c556b269b56b6785385c6c.setContent(html_dbf384247a6f8c7942770ed0dbb965f4);\\n \\n \\n\\n circle_marker_709f7b7b9b9f10b91ab69f508f3ce51e.bindPopup(popup_e3eb3f0ca9c556b269b56b6785385c6c)\\n ;\\n\\n \\n \\n \\n var circle_marker_0415f1c6c2f724f2cf1ea7c7288d062c = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.442433223290478, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2033fcfc7578c93bff6c05fc3abe466c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eafb58eee9fee0397eb0fd8c2ec4d86b = $(`<div id="html_eafb58eee9fee0397eb0fd8c2ec4d86b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2033fcfc7578c93bff6c05fc3abe466c.setContent(html_eafb58eee9fee0397eb0fd8c2ec4d86b);\\n \\n \\n\\n circle_marker_0415f1c6c2f724f2cf1ea7c7288d062c.bindPopup(popup_2033fcfc7578c93bff6c05fc3abe466c)\\n ;\\n\\n \\n \\n \\n var circle_marker_c369ea4da07b1e403927543399c36eab = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.023896332520351, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8cd303fcfc2738606c80377a90700bee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_657fd2cb75260ea2c1c62fd9683f7d2e = $(`<div id="html_657fd2cb75260ea2c1c62fd9683f7d2e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8cd303fcfc2738606c80377a90700bee.setContent(html_657fd2cb75260ea2c1c62fd9683f7d2e);\\n \\n \\n\\n circle_marker_c369ea4da07b1e403927543399c36eab.bindPopup(popup_8cd303fcfc2738606c80377a90700bee)\\n ;\\n\\n \\n \\n \\n var circle_marker_f021f699e0700968e0872ec9169d99d3 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.206085419025319, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_810f3fe2bf46240d3d3ee4a734ee458e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_592169584ce6a7ecee078a1cecff633c = $(`<div id="html_592169584ce6a7ecee078a1cecff633c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_810f3fe2bf46240d3d3ee4a734ee458e.setContent(html_592169584ce6a7ecee078a1cecff633c);\\n \\n \\n\\n circle_marker_f021f699e0700968e0872ec9169d99d3.bindPopup(popup_810f3fe2bf46240d3d3ee4a734ee458e)\\n ;\\n\\n \\n \\n \\n var circle_marker_37a15ff647242752840d44e714f1eee1 = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fc3f9c77c355c1bce7420cee8f8155bf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86c1b4b2d30e1be88e832fbec1eb020c = $(`<div id="html_86c1b4b2d30e1be88e832fbec1eb020c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc3f9c77c355c1bce7420cee8f8155bf.setContent(html_86c1b4b2d30e1be88e832fbec1eb020c);\\n \\n \\n\\n circle_marker_37a15ff647242752840d44e714f1eee1.bindPopup(popup_fc3f9c77c355c1bce7420cee8f8155bf)\\n ;\\n\\n \\n \\n \\n var circle_marker_62e8f1ad6f2b0c0b1840f2d451e35b1f = L.circleMarker(\\n [42.73997262535657, -73.25995072538909],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.1809610472257885, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_82388dd3a8e74aea86303a16f31dc14a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78fd5cb0fbe5745b50463d6d7dc1b6a7 = $(`<div id="html_78fd5cb0fbe5745b50463d6d7dc1b6a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82388dd3a8e74aea86303a16f31dc14a.setContent(html_78fd5cb0fbe5745b50463d6d7dc1b6a7);\\n \\n \\n\\n circle_marker_62e8f1ad6f2b0c0b1840f2d451e35b1f.bindPopup(popup_82388dd3a8e74aea86303a16f31dc14a)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e69fb6799f77dc15a07d2e19c9036ad = L.circleMarker(\\n [42.73997256609777, -73.25872044777847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.399277020331919, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_45fa0615b914e9b84a9b7b8a1dcd0844 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_568ce15504df3c0bc36ed6aea011aef6 = $(`<div id="html_568ce15504df3c0bc36ed6aea011aef6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_45fa0615b914e9b84a9b7b8a1dcd0844.setContent(html_568ce15504df3c0bc36ed6aea011aef6);\\n \\n \\n\\n circle_marker_6e69fb6799f77dc15a07d2e19c9036ad.bindPopup(popup_45fa0615b914e9b84a9b7b8a1dcd0844)\\n ;\\n\\n \\n \\n \\n var circle_marker_59e517de5e31e95b135d0bdba301fc53 = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_cff53ddb3c3a6c42a6dcc8124201c0be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d71a63fd09c2ab6ddcf7f153a6772953 = $(`<div id="html_d71a63fd09c2ab6ddcf7f153a6772953" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cff53ddb3c3a6c42a6dcc8124201c0be.setContent(html_d71a63fd09c2ab6ddcf7f153a6772953);\\n \\n \\n\\n circle_marker_59e517de5e31e95b135d0bdba301fc53.bindPopup(popup_cff53ddb3c3a6c42a6dcc8124201c0be)\\n ;\\n\\n \\n \\n \\n var circle_marker_fddd00f4d3be50d72422ff3acba1b52f = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_cf867148b1eecfd841dcf51e5ab691a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a69552532f9adae9cee633714050e22b = $(`<div id="html_a69552532f9adae9cee633714050e22b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf867148b1eecfd841dcf51e5ab691a9.setContent(html_a69552532f9adae9cee633714050e22b);\\n \\n \\n\\n circle_marker_fddd00f4d3be50d72422ff3acba1b52f.bindPopup(popup_cf867148b1eecfd841dcf51e5ab691a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_179a35c0f5cd48c1c38c897bc7790f8c = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_dae4616bd7c8882da5d7ff7a418bbdb6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_948d44a384651608f9419b3aa4921db5 = $(`<div id="html_948d44a384651608f9419b3aa4921db5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dae4616bd7c8882da5d7ff7a418bbdb6.setContent(html_948d44a384651608f9419b3aa4921db5);\\n \\n \\n\\n circle_marker_179a35c0f5cd48c1c38c897bc7790f8c.bindPopup(popup_dae4616bd7c8882da5d7ff7a418bbdb6)\\n ;\\n\\n \\n \\n \\n var circle_marker_b205d3b829d4cf1416f9e9c23d392041 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1ded789726d509649566cbe887712982 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9ac9fc3df8720eaed81ef64bedc066c = $(`<div id="html_a9ac9fc3df8720eaed81ef64bedc066c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ded789726d509649566cbe887712982.setContent(html_a9ac9fc3df8720eaed81ef64bedc066c);\\n \\n \\n\\n circle_marker_b205d3b829d4cf1416f9e9c23d392041.bindPopup(popup_1ded789726d509649566cbe887712982)\\n ;\\n\\n \\n \\n \\n var circle_marker_66d99b5eea1940720f3609d5c3e3ef82 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3c84ddf8b10139ea3a734781c230bb48 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8486d109fcaa72db9a21dec9b7092ceb = $(`<div id="html_8486d109fcaa72db9a21dec9b7092ceb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c84ddf8b10139ea3a734781c230bb48.setContent(html_8486d109fcaa72db9a21dec9b7092ceb);\\n \\n \\n\\n circle_marker_66d99b5eea1940720f3609d5c3e3ef82.bindPopup(popup_3c84ddf8b10139ea3a734781c230bb48)\\n ;\\n\\n \\n \\n \\n var circle_marker_c819b54843ea7d5efd336424f09b329f = L.circleMarker(\\n [42.73996556039193, -73.22427267765188],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3d614fb2b643aa0c615e0c1fb978412f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c5111b7bd11ee270feb2985c261ab3eb = $(`<div id="html_c5111b7bd11ee270feb2985c261ab3eb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d614fb2b643aa0c615e0c1fb978412f.setContent(html_c5111b7bd11ee270feb2985c261ab3eb);\\n \\n \\n\\n circle_marker_c819b54843ea7d5efd336424f09b329f.bindPopup(popup_3d614fb2b643aa0c615e0c1fb978412f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b77436074488287524c4e738a99b2a43 = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bfad1b352de831f77b85a3c7d8f3cbdb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d018895a816a2f90891653c63671b09 = $(`<div id="html_9d018895a816a2f90891653c63671b09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfad1b352de831f77b85a3c7d8f3cbdb.setContent(html_9d018895a816a2f90891653c63671b09);\\n \\n \\n\\n circle_marker_b77436074488287524c4e738a99b2a43.bindPopup(popup_bfad1b352de831f77b85a3c7d8f3cbdb)\\n ;\\n\\n \\n \\n \\n var circle_marker_249fb376cea48d4d7db4215316b79131 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2ca2b11cc4a377355fff251f93d8a5ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01351c587a9d14cd3bf51e68b91958d3 = $(`<div id="html_01351c587a9d14cd3bf51e68b91958d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ca2b11cc4a377355fff251f93d8a5ea.setContent(html_01351c587a9d14cd3bf51e68b91958d3);\\n \\n \\n\\n circle_marker_249fb376cea48d4d7db4215316b79131.bindPopup(popup_2ca2b11cc4a377355fff251f93d8a5ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ee8dc304525c78aca3f99dc15b97667 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2c33edfacc210d7e89cb9f80b47da0e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf76670afb05beee1f9469ec53fd56e3 = $(`<div id="html_bf76670afb05beee1f9469ec53fd56e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c33edfacc210d7e89cb9f80b47da0e8.setContent(html_bf76670afb05beee1f9469ec53fd56e3);\\n \\n \\n\\n circle_marker_3ee8dc304525c78aca3f99dc15b97667.bindPopup(popup_2c33edfacc210d7e89cb9f80b47da0e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_85762d795a709c2a810e071061d2fec9 = L.circleMarker(\\n [42.73816527471333, -73.27348352812871],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 13.991446672986724, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3cd7639bbf91c8990a6638e10615894b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69180bea22ba095892cc5652aa750722 = $(`<div id="html_69180bea22ba095892cc5652aa750722" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3cd7639bbf91c8990a6638e10615894b.setContent(html_69180bea22ba095892cc5652aa750722);\\n \\n \\n\\n circle_marker_85762d795a709c2a810e071061d2fec9.bindPopup(popup_3cd7639bbf91c8990a6638e10615894b)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce50b540a37dc416303e0c72e3fcc99e = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.352372348458314, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f23ace23b052b878dce8e5b18e37aa01 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6bb862f18d8967cbcd02fccd5754b433 = $(`<div id="html_6bb862f18d8967cbcd02fccd5754b433" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f23ace23b052b878dce8e5b18e37aa01.setContent(html_6bb862f18d8967cbcd02fccd5754b433);\\n \\n \\n\\n circle_marker_ce50b540a37dc416303e0c72e3fcc99e.bindPopup(popup_f23ace23b052b878dce8e5b18e37aa01)\\n ;\\n\\n \\n \\n \\n var circle_marker_b47039fe24d64c53c45e088a46bb70fc = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.269074295019226, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f2b23f246a622b078f092e2c23bb2663 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46a543d45e9ac91cb2a70c81844c14de = $(`<div id="html_46a543d45e9ac91cb2a70c81844c14de" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2b23f246a622b078f092e2c23bb2663.setContent(html_46a543d45e9ac91cb2a70c81844c14de);\\n \\n \\n\\n circle_marker_b47039fe24d64c53c45e088a46bb70fc.bindPopup(popup_f2b23f246a622b078f092e2c23bb2663)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a859ea45349cec870dc80d434f4a30f = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4115163798060095, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_98e8e7e2a8b87f9314a7188b4737211e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92a83abb2651ffdf6d8771c6de5b2944 = $(`<div id="html_92a83abb2651ffdf6d8771c6de5b2944" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98e8e7e2a8b87f9314a7188b4737211e.setContent(html_92a83abb2651ffdf6d8771c6de5b2944);\\n \\n \\n\\n circle_marker_9a859ea45349cec870dc80d434f4a30f.bindPopup(popup_98e8e7e2a8b87f9314a7188b4737211e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e9a5dfc0840fd4ca36bc12edf682f15 = L.circleMarker(\\n [42.73816553807507, -73.26856256112116],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_051963823c9bfe38704098f61f1f161b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad0d0198849717b8da9950d685ec7309 = $(`<div id="html_ad0d0198849717b8da9950d685ec7309" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_051963823c9bfe38704098f61f1f161b.setContent(html_ad0d0198849717b8da9950d685ec7309);\\n \\n \\n\\n circle_marker_6e9a5dfc0840fd4ca36bc12edf682f15.bindPopup(popup_051963823c9bfe38704098f61f1f161b)\\n ;\\n\\n \\n \\n \\n var circle_marker_09c092cdf0c9bde8ed1537b28d940637 = L.circleMarker(\\n [42.7381655709953, -73.26733231936404],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e3de34b32dd5473035138e46a7182278 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76c3448ae9110c0a946acca4b236f79c = $(`<div id="html_76c3448ae9110c0a946acca4b236f79c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3de34b32dd5473035138e46a7182278.setContent(html_76c3448ae9110c0a946acca4b236f79c);\\n \\n \\n\\n circle_marker_09c092cdf0c9bde8ed1537b28d940637.bindPopup(popup_e3de34b32dd5473035138e46a7182278)\\n ;\\n\\n \\n \\n \\n var circle_marker_d777671709a444178d1a3188c9a611cd = L.circleMarker(\\n [42.73816559074744, -73.2661020776059],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9d5c2eae070bf7f375f70a9cf93901f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53a543d8720db9435164d15fd6c1470c = $(`<div id="html_53a543d8720db9435164d15fd6c1470c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d5c2eae070bf7f375f70a9cf93901f8.setContent(html_53a543d8720db9435164d15fd6c1470c);\\n \\n \\n\\n circle_marker_d777671709a444178d1a3188c9a611cd.bindPopup(popup_9d5c2eae070bf7f375f70a9cf93901f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_446ec539ba68998640b34420ce8c8459 = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_742ad14d6a18c43a24e04405c1e6bd9f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae104987ff3c9d1a37592cfada33f584 = $(`<div id="html_ae104987ff3c9d1a37592cfada33f584" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_742ad14d6a18c43a24e04405c1e6bd9f.setContent(html_ae104987ff3c9d1a37592cfada33f584);\\n \\n \\n\\n circle_marker_446ec539ba68998640b34420ce8c8459.bindPopup(popup_742ad14d6a18c43a24e04405c1e6bd9f)\\n ;\\n\\n \\n \\n \\n var circle_marker_445efad3942a0f2fc1e93e2d1e66a6c2 = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.014626706796775, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f61d34187cffe5aba46c8f8698457d29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3aaec907f92c88af4d6e0e9a96cfafee = $(`<div id="html_3aaec907f92c88af4d6e0e9a96cfafee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f61d34187cffe5aba46c8f8698457d29.setContent(html_3aaec907f92c88af4d6e0e9a96cfafee);\\n \\n \\n\\n circle_marker_445efad3942a0f2fc1e93e2d1e66a6c2.bindPopup(popup_f61d34187cffe5aba46c8f8698457d29)\\n ;\\n\\n \\n \\n \\n var circle_marker_4defc1fb1662c6a04e3189ede68d908d = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.222008245644752, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a4f1c7d2d6ca8fcdea29989b0754f87b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53d5ad169ba166f9b3590d0582f4381c = $(`<div id="html_53d5ad169ba166f9b3590d0582f4381c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4f1c7d2d6ca8fcdea29989b0754f87b.setContent(html_53d5ad169ba166f9b3590d0582f4381c);\\n \\n \\n\\n circle_marker_4defc1fb1662c6a04e3189ede68d908d.bindPopup(popup_a4f1c7d2d6ca8fcdea29989b0754f87b)\\n ;\\n\\n \\n \\n \\n var circle_marker_efcbae798e2a67754e2954d28be10fef = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_06e9bc977455a7059963003d298319a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ba00e98c4506ec66193cdf2a2af4b9c = $(`<div id="html_3ba00e98c4506ec66193cdf2a2af4b9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06e9bc977455a7059963003d298319a8.setContent(html_3ba00e98c4506ec66193cdf2a2af4b9c);\\n \\n \\n\\n circle_marker_efcbae798e2a67754e2954d28be10fef.bindPopup(popup_06e9bc977455a7059963003d298319a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_50aa6dab66f008bc505b9730c921472c = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.33015937350939, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f4496c3ddef93d34423d4709122d7865 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81bc927e1dd02ada4e415a79c20accb0 = $(`<div id="html_81bc927e1dd02ada4e415a79c20accb0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4496c3ddef93d34423d4709122d7865.setContent(html_81bc927e1dd02ada4e415a79c20accb0);\\n \\n \\n\\n circle_marker_50aa6dab66f008bc505b9730c921472c.bindPopup(popup_f4496c3ddef93d34423d4709122d7865)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa9060d148236c4adb283a91f85240b6 = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.2909362219603215, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_395c2e03520f2fd2dea4956c2bfc4878 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d77fe1e252f1181e7b78d426745eb45 = $(`<div id="html_4d77fe1e252f1181e7b78d426745eb45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_395c2e03520f2fd2dea4956c2bfc4878.setContent(html_4d77fe1e252f1181e7b78d426745eb45);\\n \\n \\n\\n circle_marker_aa9060d148236c4adb283a91f85240b6.bindPopup(popup_395c2e03520f2fd2dea4956c2bfc4878)\\n ;\\n\\n \\n \\n \\n var circle_marker_3dd34d28553bfa2a1f102ac343134cab = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.556358677747903, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6992101fbadd3171373caa4caa78a602 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_362eeffcc699926304829a68c6281db0 = $(`<div id="html_362eeffcc699926304829a68c6281db0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6992101fbadd3171373caa4caa78a602.setContent(html_362eeffcc699926304829a68c6281db0);\\n \\n \\n\\n circle_marker_3dd34d28553bfa2a1f102ac343134cab.bindPopup(popup_6992101fbadd3171373caa4caa78a602)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9685743d9d18ca144944ae816c6c331 = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.225151993843567, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f4e42c6756f6e2040a513d30cd032ca9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_966b6a3de1e0f780e50e6f6baa4d581d = $(`<div id="html_966b6a3de1e0f780e50e6f6baa4d581d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4e42c6756f6e2040a513d30cd032ca9.setContent(html_966b6a3de1e0f780e50e6f6baa4d581d);\\n \\n \\n\\n circle_marker_a9685743d9d18ca144944ae816c6c331.bindPopup(popup_f4e42c6756f6e2040a513d30cd032ca9)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c1fbcaea4cb6de9ce67581a70d8efe6 = L.circleMarker(\\n [42.73816517595268, -73.25502990182166],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.590394548326596, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_26185c1a742b1881ddaacc3c3ccc47d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e4de119d46646dbc0fac585ae491a59 = $(`<div id="html_4e4de119d46646dbc0fac585ae491a59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_26185c1a742b1881ddaacc3c3ccc47d4.setContent(html_4e4de119d46646dbc0fac585ae491a59);\\n \\n \\n\\n circle_marker_7c1fbcaea4cb6de9ce67581a70d8efe6.bindPopup(popup_26185c1a742b1881ddaacc3c3ccc47d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_5dad4cdde5bd01e78311d8c54f5f930c = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6785f9269dbfdefce0fe74dca3160525 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a19fd82316974a148fd1be884882af9a = $(`<div id="html_a19fd82316974a148fd1be884882af9a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6785f9269dbfdefce0fe74dca3160525.setContent(html_a19fd82316974a148fd1be884882af9a);\\n \\n \\n\\n circle_marker_5dad4cdde5bd01e78311d8c54f5f930c.bindPopup(popup_6785f9269dbfdefce0fe74dca3160525)\\n ;\\n\\n \\n \\n \\n var circle_marker_38334ac7c778a2f1a7697720d932681c = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9e4aec319e197aac616ee9c9e899e293 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e0aae3604c92005aeecd69dee5884c9 = $(`<div id="html_7e0aae3604c92005aeecd69dee5884c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e4aec319e197aac616ee9c9e899e293.setContent(html_7e0aae3604c92005aeecd69dee5884c9);\\n \\n \\n\\n circle_marker_38334ac7c778a2f1a7697720d932681c.bindPopup(popup_9e4aec319e197aac616ee9c9e899e293)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea6ff505c3e3963cf6b35eb3f6444bb0 = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1f652d4720133b405a247a3997848b2d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81c23a18d40a2950877d3b0a8fdb1e55 = $(`<div id="html_81c23a18d40a2950877d3b0a8fdb1e55" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f652d4720133b405a247a3997848b2d.setContent(html_81c23a18d40a2950877d3b0a8fdb1e55);\\n \\n \\n\\n circle_marker_ea6ff505c3e3963cf6b35eb3f6444bb0.bindPopup(popup_1f652d4720133b405a247a3997848b2d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1f51d5e9f1465414bd5ce555e9202af = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3dea2b11c98d83613234255fab2a4f6d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96623382cbf0e7efa110544dcd1425ca = $(`<div id="html_96623382cbf0e7efa110544dcd1425ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3dea2b11c98d83613234255fab2a4f6d.setContent(html_96623382cbf0e7efa110544dcd1425ca);\\n \\n \\n\\n circle_marker_c1f51d5e9f1465414bd5ce555e9202af.bindPopup(popup_3dea2b11c98d83613234255fab2a4f6d)\\n ;\\n\\n \\n \\n \\n var circle_marker_27201b748c01da67466fed699993730b = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.406461512053774, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_46cfd6d07328c8b479d662e68e6cbf49 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea70519f1ef911a0f8dd0fda470d9c62 = $(`<div id="html_ea70519f1ef911a0f8dd0fda470d9c62" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46cfd6d07328c8b479d662e68e6cbf49.setContent(html_ea70519f1ef911a0f8dd0fda470d9c62);\\n \\n \\n\\n circle_marker_27201b748c01da67466fed699993730b.bindPopup(popup_46cfd6d07328c8b479d662e68e6cbf49)\\n ;\\n\\n \\n \\n \\n var circle_marker_259301ce72c0b028d249667f5bb4f437 = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0533e7cfb76bb99c677488334e768c83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b078e9763d89085c03460ad654bc8d1 = $(`<div id="html_9b078e9763d89085c03460ad654bc8d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0533e7cfb76bb99c677488334e768c83.setContent(html_9b078e9763d89085c03460ad654bc8d1);\\n \\n \\n\\n circle_marker_259301ce72c0b028d249667f5bb4f437.bindPopup(popup_0533e7cfb76bb99c677488334e768c83)\\n ;\\n\\n \\n \\n \\n var circle_marker_5619c59f9660501ddb57d407b1624c3b = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3a8053387dc5aa49f59312c689a50f6b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c773018c19e2e0c6411ced7ceeebb938 = $(`<div id="html_c773018c19e2e0c6411ced7ceeebb938" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a8053387dc5aa49f59312c689a50f6b.setContent(html_c773018c19e2e0c6411ced7ceeebb938);\\n \\n \\n\\n circle_marker_5619c59f9660501ddb57d407b1624c3b.bindPopup(popup_3a8053387dc5aa49f59312c689a50f6b)\\n ;\\n\\n \\n \\n \\n var circle_marker_75cb6fdb23bd7b40295982472c84f316 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_83e5b4ddd3493158b359b6f8baf6d11c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c0953b46b344abc3fe1481597500374 = $(`<div id="html_1c0953b46b344abc3fe1481597500374" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83e5b4ddd3493158b359b6f8baf6d11c.setContent(html_1c0953b46b344abc3fe1481597500374);\\n \\n \\n\\n circle_marker_75cb6fdb23bd7b40295982472c84f316.bindPopup(popup_83e5b4ddd3493158b359b6f8baf6d11c)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f61a81617cf10cb2f127d489a6a0fb2 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6a19f4fb77a7293dd05392f73d4f2b4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3bcb5bfba0504e935edb18b30f91a45 = $(`<div id="html_e3bcb5bfba0504e935edb18b30f91a45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a19f4fb77a7293dd05392f73d4f2b4f.setContent(html_e3bcb5bfba0504e935edb18b30f91a45);\\n \\n \\n\\n circle_marker_6f61a81617cf10cb2f127d489a6a0fb2.bindPopup(popup_6a19f4fb77a7293dd05392f73d4f2b4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_486d57e49be381d673e843b3707c03c1 = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_af17707b42afb0d95a207b764b1f6703 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_149feb34a763ac864d26854384866d5e = $(`<div id="html_149feb34a763ac864d26854384866d5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af17707b42afb0d95a207b764b1f6703.setContent(html_149feb34a763ac864d26854384866d5e);\\n \\n \\n\\n circle_marker_486d57e49be381d673e843b3707c03c1.bindPopup(popup_af17707b42afb0d95a207b764b1f6703)\\n ;\\n\\n \\n \\n \\n var circle_marker_c14773d0fd74c79740eaca1a9f2f824d = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7c948a28852e849e832f96ba27754a2c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fa2b8c3ef78f25b31de941bc3ccfd6a2 = $(`<div id="html_fa2b8c3ef78f25b31de941bc3ccfd6a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c948a28852e849e832f96ba27754a2c.setContent(html_fa2b8c3ef78f25b31de941bc3ccfd6a2);\\n \\n \\n\\n circle_marker_c14773d0fd74c79740eaca1a9f2f824d.bindPopup(popup_7c948a28852e849e832f96ba27754a2c)\\n ;\\n\\n \\n \\n \\n var circle_marker_f0e7b9f51de56a526009dd3f781eaa1b = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.507891862878783, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b290922a2e98ab4fff10a832877e1382 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_486caf8c045871c540d3e638d0e9a9ca = $(`<div id="html_486caf8c045871c540d3e638d0e9a9ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b290922a2e98ab4fff10a832877e1382.setContent(html_486caf8c045871c540d3e638d0e9a9ca);\\n \\n \\n\\n circle_marker_f0e7b9f51de56a526009dd3f781eaa1b.bindPopup(popup_b290922a2e98ab4fff10a832877e1382)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec31f684a38505e45894898fcaea8511 = L.circleMarker(\\n [42.736358404703424, -73.2685624535584],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.403141895720198, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bb20754a1a82a4f3c34f0185cca601f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b8bd4cc829811778fc5372227b30b09 = $(`<div id="html_4b8bd4cc829811778fc5372227b30b09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb20754a1a82a4f3c34f0185cca601f1.setContent(html_4b8bd4cc829811778fc5372227b30b09);\\n \\n \\n\\n circle_marker_ec31f684a38505e45894898fcaea8511.bindPopup(popup_bb20754a1a82a4f3c34f0185cca601f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_1143b11e1cf3e4285e8cb60e80ce1c1a = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.214724333230155, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0fc98ebf1ccdad997f419bf3eac7c2d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd6dd2a87bbfec4f267555eb068900d7 = $(`<div id="html_bd6dd2a87bbfec4f267555eb068900d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0fc98ebf1ccdad997f419bf3eac7c2d3.setContent(html_bd6dd2a87bbfec4f267555eb068900d7);\\n \\n \\n\\n circle_marker_1143b11e1cf3e4285e8cb60e80ce1c1a.bindPopup(popup_0fc98ebf1ccdad997f419bf3eac7c2d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_e86ae670f8dfd52b2659803bfea3c58e = L.circleMarker(\\n [42.73635845737364, -73.26610204175164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1dd81808e44ac5c276facbdb58fb3461 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19eaaea93e345915a4d0489a79049cf2 = $(`<div id="html_19eaaea93e345915a4d0489a79049cf2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1dd81808e44ac5c276facbdb58fb3461.setContent(html_19eaaea93e345915a4d0489a79049cf2);\\n \\n \\n\\n circle_marker_e86ae670f8dfd52b2659803bfea3c58e.bindPopup(popup_1dd81808e44ac5c276facbdb58fb3461)\\n ;\\n\\n \\n \\n \\n var circle_marker_610d04191a597f66e26d824af7055d33 = L.circleMarker(\\n [42.73635846395741, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.723094811029982, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c3f4d63da6d479067849f47db457ba7e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b9b78a1218cc5e80d8c960eab85c0283 = $(`<div id="html_b9b78a1218cc5e80d8c960eab85c0283" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3f4d63da6d479067849f47db457ba7e.setContent(html_b9b78a1218cc5e80d8c960eab85c0283);\\n \\n \\n\\n circle_marker_610d04191a597f66e26d824af7055d33.bindPopup(popup_c3f4d63da6d479067849f47db457ba7e)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ec21e7345655388cd1d4324ca6de874 = L.circleMarker(\\n [42.73635845737364, -73.26364162994278],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.156394191823509, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9abbd3e47d32dde11fc6c384bb470149 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e0a6faa9bce0a9e0ca0346ef5e67d3ca = $(`<div id="html_e0a6faa9bce0a9e0ca0346ef5e67d3ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9abbd3e47d32dde11fc6c384bb470149.setContent(html_e0a6faa9bce0a9e0ca0346ef5e67d3ca);\\n \\n \\n\\n circle_marker_2ec21e7345655388cd1d4324ca6de874.bindPopup(popup_9abbd3e47d32dde11fc6c384bb470149)\\n ;\\n\\n \\n \\n \\n var circle_marker_c2e5e44fb818cbf86768e8136154b92f = L.circleMarker(\\n [42.73635843762231, -73.26241142403887],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.694520051971082, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5cd7fab49b9fda52cdabd50ee990a183 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d38687ec9aa719ce4d87888a9034e512 = $(`<div id="html_d38687ec9aa719ce4d87888a9034e512" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5cd7fab49b9fda52cdabd50ee990a183.setContent(html_d38687ec9aa719ce4d87888a9034e512);\\n \\n \\n\\n circle_marker_c2e5e44fb818cbf86768e8136154b92f.bindPopup(popup_5cd7fab49b9fda52cdabd50ee990a183)\\n ;\\n\\n \\n \\n \\n var circle_marker_f558727f0274f11289f284f493605bf9 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.54834217738561, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_77172b94ebbc911c589b690cf7edb02a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6019edd1e8eed2eed28803524da762bf = $(`<div id="html_6019edd1e8eed2eed28803524da762bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77172b94ebbc911c589b690cf7edb02a.setContent(html_6019edd1e8eed2eed28803524da762bf);\\n \\n \\n\\n circle_marker_f558727f0274f11289f284f493605bf9.bindPopup(popup_77172b94ebbc911c589b690cf7edb02a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1063b0f1a1d4944d8463fc822499837 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.579524642479541, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ee1e57ea5c60fb75c8d1d75a0613d435 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0de3b59a1360a99be137caa79da52031 = $(`<div id="html_0de3b59a1360a99be137caa79da52031" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee1e57ea5c60fb75c8d1d75a0613d435.setContent(html_0de3b59a1360a99be137caa79da52031);\\n \\n \\n\\n circle_marker_f1063b0f1a1d4944d8463fc822499837.bindPopup(popup_ee1e57ea5c60fb75c8d1d75a0613d435)\\n ;\\n\\n \\n \\n \\n var circle_marker_976069379bd7a97be344feea86318a6d = L.circleMarker(\\n [42.73635829936299, -73.25872080633552],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.776816725043752, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_13558612b9f10d85b0c7e7969838e2fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a20433d0fa2063d9eb0c5c7480f4d8a = $(`<div id="html_7a20433d0fa2063d9eb0c5c7480f4d8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13558612b9f10d85b0c7e7969838e2fc.setContent(html_7a20433d0fa2063d9eb0c5c7480f4d8a);\\n \\n \\n\\n circle_marker_976069379bd7a97be344feea86318a6d.bindPopup(popup_13558612b9f10d85b0c7e7969838e2fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6fcce8e66984caecfe20ec781988db9 = L.circleMarker(\\n [42.73635822694145, -73.25749060043893],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.970821321441846, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f53b2d17f1cad25cb2a7ae4a91cd8832 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5adaad960dd7fd1116118728b32ba493 = $(`<div id="html_5adaad960dd7fd1116118728b32ba493" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f53b2d17f1cad25cb2a7ae4a91cd8832.setContent(html_5adaad960dd7fd1116118728b32ba493);\\n \\n \\n\\n circle_marker_b6fcce8e66984caecfe20ec781988db9.bindPopup(popup_f53b2d17f1cad25cb2a7ae4a91cd8832)\\n ;\\n\\n \\n \\n \\n var circle_marker_0bc83449444d1893e36555fb41f17ff3 = L.circleMarker(\\n [42.73635814135235, -73.25626039454548],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.93287767191607, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bfa9b3c758f7150d298c2c86d2cfde1d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b79c171145c359f7c2edad33f43c76c7 = $(`<div id="html_b79c171145c359f7c2edad33f43c76c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfa9b3c758f7150d298c2c86d2cfde1d.setContent(html_b79c171145c359f7c2edad33f43c76c7);\\n \\n \\n\\n circle_marker_0bc83449444d1893e36555fb41f17ff3.bindPopup(popup_bfa9b3c758f7150d298c2c86d2cfde1d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a7b172069f297619a50ae213e906483 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.076507779746499, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6948c8a82540fbc7344bbddabe385740 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9ebb43fc8dfd85a994ce81d30d82327 = $(`<div id="html_a9ebb43fc8dfd85a994ce81d30d82327" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6948c8a82540fbc7344bbddabe385740.setContent(html_a9ebb43fc8dfd85a994ce81d30d82327);\\n \\n \\n\\n circle_marker_0a7b172069f297619a50ae213e906483.bindPopup(popup_6948c8a82540fbc7344bbddabe385740)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f731054a46fbd835d1f4df67db2187c = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.068428945128219, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8859112d3977acdc805d0eaeef11e608 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4311b359a4ba217c48235e1ccf23dfc7 = $(`<div id="html_4311b359a4ba217c48235e1ccf23dfc7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8859112d3977acdc805d0eaeef11e608.setContent(html_4311b359a4ba217c48235e1ccf23dfc7);\\n \\n \\n\\n circle_marker_6f731054a46fbd835d1f4df67db2187c.bindPopup(popup_8859112d3977acdc805d0eaeef11e608)\\n ;\\n\\n \\n \\n \\n var circle_marker_930aeab20cf66faad6484e169e0c418b = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.3078313050504, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e7779c735f3c92ecb45062311ae94b0a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67227047650c1fdb5a328f30b22f828d = $(`<div id="html_67227047650c1fdb5a328f30b22f828d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e7779c735f3c92ecb45062311ae94b0a.setContent(html_67227047650c1fdb5a328f30b22f828d);\\n \\n \\n\\n circle_marker_930aeab20cf66faad6484e169e0c418b.bindPopup(popup_e7779c735f3c92ecb45062311ae94b0a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c4bee39f5a6c2c9f289793b2fd47c2c = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4e739894eee33a820a68fb0da5dfd110 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce0b3f3fa8b1246c728399743ee59fc6 = $(`<div id="html_ce0b3f3fa8b1246c728399743ee59fc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e739894eee33a820a68fb0da5dfd110.setContent(html_ce0b3f3fa8b1246c728399743ee59fc6);\\n \\n \\n\\n circle_marker_8c4bee39f5a6c2c9f289793b2fd47c2c.bindPopup(popup_4e739894eee33a820a68fb0da5dfd110)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc0b09084fdf4b6e0710343e1c0be8e1 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.232078956954491, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_89d27a6164460c1d54fe462e1357f2b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d5e9c9e7a95b46a31cfb6435cbfaf72 = $(`<div id="html_9d5e9c9e7a95b46a31cfb6435cbfaf72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89d27a6164460c1d54fe462e1357f2b8.setContent(html_9d5e9c9e7a95b46a31cfb6435cbfaf72);\\n \\n \\n\\n circle_marker_fc0b09084fdf4b6e0710343e1c0be8e1.bindPopup(popup_89d27a6164460c1d54fe462e1357f2b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_6907f553c747d936288225d7f26f75fa = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.253382073025046, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_79080f53f4153cbb638c944ca1aad958 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ef0365b20401a89b5695e1920ff112f6 = $(`<div id="html_ef0365b20401a89b5695e1920ff112f6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79080f53f4153cbb638c944ca1aad958.setContent(html_ef0365b20401a89b5695e1920ff112f6);\\n \\n \\n\\n circle_marker_6907f553c747d936288225d7f26f75fa.bindPopup(popup_79080f53f4153cbb638c944ca1aad958)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b1bbb7c3be4b98dab3aa5c64b32840d = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.720348719413148, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4bf98bb0a66bfffa878a54821352775a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cfc9295bac66dda4f8e56aa35b1f75b1 = $(`<div id="html_cfc9295bac66dda4f8e56aa35b1f75b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4bf98bb0a66bfffa878a54821352775a.setContent(html_cfc9295bac66dda4f8e56aa35b1f75b1);\\n \\n \\n\\n circle_marker_8b1bbb7c3be4b98dab3aa5c64b32840d.bindPopup(popup_4bf98bb0a66bfffa878a54821352775a)\\n ;\\n\\n \\n \\n \\n var circle_marker_7988e2f4063d2e238ae341c730745d6a = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3a02fb7fb34be70e0b2b1662a1b6a43a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63aef0afdf33550168e242478fb0282b = $(`<div id="html_63aef0afdf33550168e242478fb0282b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a02fb7fb34be70e0b2b1662a1b6a43a.setContent(html_63aef0afdf33550168e242478fb0282b);\\n \\n \\n\\n circle_marker_7988e2f4063d2e238ae341c730745d6a.bindPopup(popup_3a02fb7fb34be70e0b2b1662a1b6a43a)\\n ;\\n\\n \\n \\n \\n var circle_marker_9668c9352b03176e9685de4bc0198a3d = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.820437914901168, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d4a11b2e303e51f0895b8c02d54affe5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_416100d0b94cf44a0287f09adb6f730a = $(`<div id="html_416100d0b94cf44a0287f09adb6f730a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d4a11b2e303e51f0895b8c02d54affe5.setContent(html_416100d0b94cf44a0287f09adb6f730a);\\n \\n \\n\\n circle_marker_9668c9352b03176e9685de4bc0198a3d.bindPopup(popup_d4a11b2e303e51f0895b8c02d54affe5)\\n ;\\n\\n \\n \\n \\n var circle_marker_98f6e384200cc5bbe19659653710d1f8 = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2c31bdd7df9fae0d83c401b1da6e1496 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b88455443a8a863ceebad5ab2438b5d = $(`<div id="html_0b88455443a8a863ceebad5ab2438b5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c31bdd7df9fae0d83c401b1da6e1496.setContent(html_0b88455443a8a863ceebad5ab2438b5d);\\n \\n \\n\\n circle_marker_98f6e384200cc5bbe19659653710d1f8.bindPopup(popup_2c31bdd7df9fae0d83c401b1da6e1496)\\n ;\\n\\n \\n \\n \\n var circle_marker_14f34f344aa51087cb92943ab81f0225 = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.478115991081385, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_31d89cb4dfc3a45d5b58090ca9553d93 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d5c0e7ce298cef9c4e8a7b88ccf26bea = $(`<div id="html_d5c0e7ce298cef9c4e8a7b88ccf26bea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31d89cb4dfc3a45d5b58090ca9553d93.setContent(html_d5c0e7ce298cef9c4e8a7b88ccf26bea);\\n \\n \\n\\n circle_marker_14f34f344aa51087cb92943ab81f0225.bindPopup(popup_31d89cb4dfc3a45d5b58090ca9553d93)\\n ;\\n\\n \\n \\n \\n var circle_marker_00921878fb5dd467b89612aa0a778457 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ec3aa5d4b9b22d3eda0d0aa05a83242c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49c002cdd6e52e04bc83149372820b8c = $(`<div id="html_49c002cdd6e52e04bc83149372820b8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec3aa5d4b9b22d3eda0d0aa05a83242c.setContent(html_49c002cdd6e52e04bc83149372820b8c);\\n \\n \\n\\n circle_marker_00921878fb5dd467b89612aa0a778457.bindPopup(popup_ec3aa5d4b9b22d3eda0d0aa05a83242c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2274c3ab560019a8f084d5aacebcdd40 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e7f24a0505934f5ea147e99d3dd3c233 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f98d35eba4452e0cc00597616446b1a6 = $(`<div id="html_f98d35eba4452e0cc00597616446b1a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e7f24a0505934f5ea147e99d3dd3c233.setContent(html_f98d35eba4452e0cc00597616446b1a6);\\n \\n \\n\\n circle_marker_2274c3ab560019a8f084d5aacebcdd40.bindPopup(popup_e7f24a0505934f5ea147e99d3dd3c233)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c350cce4c1de0ea5e053604ed1d96fa = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0af09d4659e8476358d636090854933b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2ff4fab1774dcb6cbe9da2de0851e92e = $(`<div id="html_2ff4fab1774dcb6cbe9da2de0851e92e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0af09d4659e8476358d636090854933b.setContent(html_2ff4fab1774dcb6cbe9da2de0851e92e);\\n \\n \\n\\n circle_marker_6c350cce4c1de0ea5e053604ed1d96fa.bindPopup(popup_0af09d4659e8476358d636090854933b)\\n ;\\n\\n \\n \\n \\n var circle_marker_b634d66accb275217c06e7e21183b921 = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_80dc5f9471ac1d4e9eb78b35fcbc0343 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_258f8fb285d1d18b1700ec67b867d943 = $(`<div id="html_258f8fb285d1d18b1700ec67b867d943" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80dc5f9471ac1d4e9eb78b35fcbc0343.setContent(html_258f8fb285d1d18b1700ec67b867d943);\\n \\n \\n\\n circle_marker_b634d66accb275217c06e7e21183b921.bindPopup(popup_80dc5f9471ac1d4e9eb78b35fcbc0343)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0960ded97d46db611e57e03a026e533 = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5414791071f1f403cf8da1e9e96a1d4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3622b9ece0bf2aa69f367c8c8c6f5f39 = $(`<div id="html_3622b9ece0bf2aa69f367c8c8c6f5f39" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5414791071f1f403cf8da1e9e96a1d4b.setContent(html_3622b9ece0bf2aa69f367c8c8c6f5f39);\\n \\n \\n\\n circle_marker_e0960ded97d46db611e57e03a026e533.bindPopup(popup_5414791071f1f403cf8da1e9e96a1d4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ab53223ce988dc1ef34f1860f7896b6 = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_90ed912c0aea2f6300d36714a1bbc4a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe39f96f690b3aa7b55f574f7fa0cd92 = $(`<div id="html_fe39f96f690b3aa7b55f574f7fa0cd92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90ed912c0aea2f6300d36714a1bbc4a6.setContent(html_fe39f96f690b3aa7b55f574f7fa0cd92);\\n \\n \\n\\n circle_marker_1ab53223ce988dc1ef34f1860f7896b6.bindPopup(popup_90ed912c0aea2f6300d36714a1bbc4a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_646ca1ff53c4fa4cdbc5c40e493c7dd7 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_64c72e4de87cbdc07d7e72287a67e6d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_065e982b67b7e12c796e3c16ee07dfaa = $(`<div id="html_065e982b67b7e12c796e3c16ee07dfaa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64c72e4de87cbdc07d7e72287a67e6d3.setContent(html_065e982b67b7e12c796e3c16ee07dfaa);\\n \\n \\n\\n circle_marker_646ca1ff53c4fa4cdbc5c40e493c7dd7.bindPopup(popup_64c72e4de87cbdc07d7e72287a67e6d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a5dd86bfe74db0ec2d60a6362e2ecbf = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.686510657907603, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_50110b603fb62993fd081d81942cbb8e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24a0d28806b3432f945ba133eef08991 = $(`<div id="html_24a0d28806b3432f945ba133eef08991" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_50110b603fb62993fd081d81942cbb8e.setContent(html_24a0d28806b3432f945ba133eef08991);\\n \\n \\n\\n circle_marker_1a5dd86bfe74db0ec2d60a6362e2ecbf.bindPopup(popup_50110b603fb62993fd081d81942cbb8e)\\n ;\\n\\n \\n \\n \\n var circle_marker_aebb817b5deef96c6553a37d22e63f5d = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8ba3d6af83cb20f70612c1eed221133b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ead1bc342e14b540a5be7abdaa6333f = $(`<div id="html_5ead1bc342e14b540a5be7abdaa6333f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ba3d6af83cb20f70612c1eed221133b.setContent(html_5ead1bc342e14b540a5be7abdaa6333f);\\n \\n \\n\\n circle_marker_aebb817b5deef96c6553a37d22e63f5d.bindPopup(popup_8ba3d6af83cb20f70612c1eed221133b)\\n ;\\n\\n \\n \\n \\n var circle_marker_851a5eae0a206ae598bc4474f789a966 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6bf7a7bab83594442a5ddf110cf1e144 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a0f7a56dfda695bfc836cfa3b9b83b84 = $(`<div id="html_a0f7a56dfda695bfc836cfa3b9b83b84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6bf7a7bab83594442a5ddf110cf1e144.setContent(html_a0f7a56dfda695bfc836cfa3b9b83b84);\\n \\n \\n\\n circle_marker_851a5eae0a206ae598bc4474f789a966.bindPopup(popup_6bf7a7bab83594442a5ddf110cf1e144)\\n ;\\n\\n \\n \\n \\n var circle_marker_54ccce0146658901b19e61e1a1eaf8fb = L.circleMarker(\\n [42.7345512252472, -73.26979251605432],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.349243383340204, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a2773b1f5c4b4bb25712371724af9ec4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1cb3786bf2d4dd9ff0b9ea290d89257 = $(`<div id="html_b1cb3786bf2d4dd9ff0b9ea290d89257" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a2773b1f5c4b4bb25712371724af9ec4.setContent(html_b1cb3786bf2d4dd9ff0b9ea290d89257);\\n \\n \\n\\n circle_marker_54ccce0146658901b19e61e1a1eaf8fb.bindPopup(popup_a2773b1f5c4b4bb25712371724af9ec4)\\n ;\\n\\n \\n \\n \\n var circle_marker_d2d49a26415ac9c470706ec7050cd70f = L.circleMarker(\\n [42.73455127133177, -73.26856234600437],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_20f664fa87418c151877792dd97bb974 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0a17ded9a1b2382a948accc03e4ab98 = $(`<div id="html_c0a17ded9a1b2382a948accc03e4ab98" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20f664fa87418c151877792dd97bb974.setContent(html_c0a17ded9a1b2382a948accc03e4ab98);\\n \\n \\n\\n circle_marker_d2d49a26415ac9c470706ec7050cd70f.bindPopup(popup_20f664fa87418c151877792dd97bb974)\\n ;\\n\\n \\n \\n \\n var circle_marker_8589c53af0b67571c2ce477c627f0425 = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.1141599576634995, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4a348b469f5816f46c53a019f93b2af9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40082a8e3ddf613a5775d7c2feffa265 = $(`<div id="html_40082a8e3ddf613a5775d7c2feffa265" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a348b469f5816f46c53a019f93b2af9.setContent(html_40082a8e3ddf613a5775d7c2feffa265);\\n \\n \\n\\n circle_marker_8589c53af0b67571c2ce477c627f0425.bindPopup(popup_4a348b469f5816f46c53a019f93b2af9)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6dfc787cb1d6f856fdd3d94678ef23d = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.478115991081385, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_239332ceb75bc3715c5e42100484d7fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e8a76baa832454d0ff2a824d4820021 = $(`<div id="html_7e8a76baa832454d0ff2a824d4820021" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_239332ceb75bc3715c5e42100484d7fe.setContent(html_7e8a76baa832454d0ff2a824d4820021);\\n \\n \\n\\n circle_marker_f6dfc787cb1d6f856fdd3d94678ef23d.bindPopup(popup_239332ceb75bc3715c5e42100484d7fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_d369962f82d3fbe9ffc6e8654e840b45 = L.circleMarker(\\n [42.734551330583365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.149617481968136, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0440190ef01adfe792b4c4ed758a8cd2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09829ea563e95a5a792c54a4def6548b = $(`<div id="html_09829ea563e95a5a792c54a4def6548b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0440190ef01adfe792b4c4ed758a8cd2.setContent(html_09829ea563e95a5a792c54a4def6548b);\\n \\n \\n\\n circle_marker_d369962f82d3fbe9ffc6e8654e840b45.bindPopup(popup_0440190ef01adfe792b4c4ed758a8cd2)\\n ;\\n\\n \\n \\n \\n var circle_marker_55554a636db8c3f7597672de51f9f517 = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bd93b0d3b86c9743bc74df14cfebed74 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b079dc626cd6db05b175c74af24abac1 = $(`<div id="html_b079dc626cd6db05b175c74af24abac1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd93b0d3b86c9743bc74df14cfebed74.setContent(html_b079dc626cd6db05b175c74af24abac1);\\n \\n \\n\\n circle_marker_55554a636db8c3f7597672de51f9f517.bindPopup(popup_bd93b0d3b86c9743bc74df14cfebed74)\\n ;\\n\\n \\n \\n \\n var circle_marker_19daeaf3d120a32892ae3b4ab55c66fd = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1073621666150775, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_464ae4b7644932584ba2362a3eb5f578 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_116a7cd40392ca1e9ce8e595f1ae72ae = $(`<div id="html_116a7cd40392ca1e9ce8e595f1ae72ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_464ae4b7644932584ba2362a3eb5f578.setContent(html_116a7cd40392ca1e9ce8e595f1ae72ae);\\n \\n \\n\\n circle_marker_19daeaf3d120a32892ae3b4ab55c66fd.bindPopup(popup_464ae4b7644932584ba2362a3eb5f578)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1d60976b63ba213c0900fc188791fc3 = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.463526651802308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fa0fac1f7b8489ae81bf13f1cd8d4d55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6031de3b96af1e197885eb756385a368 = $(`<div id="html_6031de3b96af1e197885eb756385a368" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa0fac1f7b8489ae81bf13f1cd8d4d55.setContent(html_6031de3b96af1e197885eb756385a368);\\n \\n \\n\\n circle_marker_b1d60976b63ba213c0900fc188791fc3.bindPopup(popup_fa0fac1f7b8489ae81bf13f1cd8d4d55)\\n ;\\n\\n \\n \\n \\n var circle_marker_362e7189874555a3c8a6126a0bf61a8d = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.253382073025046, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6f303ced2ceda95c859f4e6e46737ed4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_156eb175a214b3c4d60a2d3fa746f269 = $(`<div id="html_156eb175a214b3c4d60a2d3fa746f269" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f303ced2ceda95c859f4e6e46737ed4.setContent(html_156eb175a214b3c4d60a2d3fa746f269);\\n \\n \\n\\n circle_marker_362e7189874555a3c8a6126a0bf61a8d.bindPopup(popup_6f303ced2ceda95c859f4e6e46737ed4)\\n ;\\n\\n \\n \\n \\n var circle_marker_ac1ed71000e38152328ea8688fc676c0 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.735777827895049, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_058f521e40c4177601e1003f652cd82c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7fda75ad447cd65bac730225c282b1b2 = $(`<div id="html_7fda75ad447cd65bac730225c282b1b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_058f521e40c4177601e1003f652cd82c.setContent(html_7fda75ad447cd65bac730225c282b1b2);\\n \\n \\n\\n circle_marker_ac1ed71000e38152328ea8688fc676c0.bindPopup(popup_058f521e40c4177601e1003f652cd82c)\\n ;\\n\\n \\n \\n \\n var circle_marker_5cfff35fe7c70199f57255558b7764f2 = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.898654169668588, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5cdc1976ba42f85c16dcb9911285acb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc77a174d1aab94abbc942d21ef542e6 = $(`<div id="html_bc77a174d1aab94abbc942d21ef542e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5cdc1976ba42f85c16dcb9911285acb2.setContent(html_bc77a174d1aab94abbc942d21ef542e6);\\n \\n \\n\\n circle_marker_5cfff35fe7c70199f57255558b7764f2.bindPopup(popup_5cdc1976ba42f85c16dcb9911285acb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3229afd991a8b97beb9f9a790d0c879 = L.circleMarker(\\n [42.73455100799136, -73.25626064550488],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.804569629897316, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5b57e115da8765d5e999f7b4e0a1e7d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d086f44a705f354c465726e70ad7373b = $(`<div id="html_d086f44a705f354c465726e70ad7373b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5b57e115da8765d5e999f7b4e0a1e7d7.setContent(html_d086f44a705f354c465726e70ad7373b);\\n \\n \\n\\n circle_marker_a3229afd991a8b97beb9f9a790d0c879.bindPopup(popup_5b57e115da8765d5e999f7b4e0a1e7d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_31c16fb06959eac508d6fd9bfaddb6ab = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.686510657907603, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_cfd07c000e9b368fc28e152709e95c14 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46691487a7e1336462afa61eb072387e = $(`<div id="html_46691487a7e1336462afa61eb072387e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfd07c000e9b368fc28e152709e95c14.setContent(html_46691487a7e1336462afa61eb072387e);\\n \\n \\n\\n circle_marker_31c16fb06959eac508d6fd9bfaddb6ab.bindPopup(popup_cfd07c000e9b368fc28e152709e95c14)\\n ;\\n\\n \\n \\n \\n var circle_marker_545fade8d84d694d61f86628f9203c1d = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9fad3b8a25fd81b993a66f3ca3eef1bf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3211efe20163f1bc3259fe1a3506d2f2 = $(`<div id="html_3211efe20163f1bc3259fe1a3506d2f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fad3b8a25fd81b993a66f3ca3eef1bf.setContent(html_3211efe20163f1bc3259fe1a3506d2f2);\\n \\n \\n\\n circle_marker_545fade8d84d694d61f86628f9203c1d.bindPopup(popup_9fad3b8a25fd81b993a66f3ca3eef1bf)\\n ;\\n\\n \\n \\n \\n var circle_marker_a0e6d510fdcb974d13ff5e7d21939efc = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_54d5bdc90aa2cc4e9da80c277cada889 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6124028e40872d7feb3a1b74f1817979 = $(`<div id="html_6124028e40872d7feb3a1b74f1817979" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54d5bdc90aa2cc4e9da80c277cada889.setContent(html_6124028e40872d7feb3a1b74f1817979);\\n \\n \\n\\n circle_marker_a0e6d510fdcb974d13ff5e7d21939efc.bindPopup(popup_54d5bdc90aa2cc4e9da80c277cada889)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba5cf016d8b2c5ecd9d0bcac890862f5 = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.698035485213008, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5ae0021bd73a7222778ac945263312e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0511a69b764bca48adf3c84165f9a06a = $(`<div id="html_0511a69b764bca48adf3c84165f9a06a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ae0021bd73a7222778ac945263312e8.setContent(html_0511a69b764bca48adf3c84165f9a06a);\\n \\n \\n\\n circle_marker_ba5cf016d8b2c5ecd9d0bcac890862f5.bindPopup(popup_5ae0021bd73a7222778ac945263312e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_8ed8710d2cef08694c14425c1d33526c = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_40445ab9ac349b9c80a93fe52a9b79db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1876655b97c54e439d8fcd9c2e8fecc6 = $(`<div id="html_1876655b97c54e439d8fcd9c2e8fecc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_40445ab9ac349b9c80a93fe52a9b79db.setContent(html_1876655b97c54e439d8fcd9c2e8fecc6);\\n \\n \\n\\n circle_marker_8ed8710d2cef08694c14425c1d33526c.bindPopup(popup_40445ab9ac349b9c80a93fe52a9b79db)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a0f6cb08db21b22187d9387926e86b8 = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.154581744998688, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_128e5ea22290998066c0660ee286e016 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2425d874dcacb0647eda0172fa7a752 = $(`<div id="html_a2425d874dcacb0647eda0172fa7a752" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_128e5ea22290998066c0660ee286e016.setContent(html_a2425d874dcacb0647eda0172fa7a752);\\n \\n \\n\\n circle_marker_1a0f6cb08db21b22187d9387926e86b8.bindPopup(popup_128e5ea22290998066c0660ee286e016)\\n ;\\n\\n \\n \\n \\n var circle_marker_65ff80428940347483945f15c44954bd = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.180387232371033, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5b1a80e67d785a3b510acab0ccbaa599 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68ece715a3960eb0b63331a5d6e6c0aa = $(`<div id="html_68ece715a3960eb0b63331a5d6e6c0aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5b1a80e67d785a3b510acab0ccbaa599.setContent(html_68ece715a3960eb0b63331a5d6e6c0aa);\\n \\n \\n\\n circle_marker_65ff80428940347483945f15c44954bd.bindPopup(popup_5b1a80e67d785a3b510acab0ccbaa599)\\n ;\\n\\n \\n \\n \\n var circle_marker_27ccbe2abbb8f6c61f62a8ed00963b8a = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e5aacfb4c8f90e10a2ce5201f02a390b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fce4075d0642442967f5d7bb1ab64814 = $(`<div id="html_fce4075d0642442967f5d7bb1ab64814" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5aacfb4c8f90e10a2ce5201f02a390b.setContent(html_fce4075d0642442967f5d7bb1ab64814);\\n \\n \\n\\n circle_marker_27ccbe2abbb8f6c61f62a8ed00963b8a.bindPopup(popup_e5aacfb4c8f90e10a2ce5201f02a390b)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c8e62d2f5a1c9c2dd6fd0072be73a3f = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5527ba3262256b0a2a21e0df74c9f7c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_27f9f65c288e2e9b9f79d0b1f72bfa26 = $(`<div id="html_27f9f65c288e2e9b9f79d0b1f72bfa26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5527ba3262256b0a2a21e0df74c9f7c8.setContent(html_27f9f65c288e2e9b9f79d0b1f72bfa26);\\n \\n \\n\\n circle_marker_6c8e62d2f5a1c9c2dd6fd0072be73a3f.bindPopup(popup_5527ba3262256b0a2a21e0df74c9f7c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_c51a76946f6e59913c9ab81889674071 = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.432750982580687, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_25bd36aa04d5ebe8a6498577cb125f2e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_987b26b2ca6709ec0aa5b0719d1274b6 = $(`<div id="html_987b26b2ca6709ec0aa5b0719d1274b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_25bd36aa04d5ebe8a6498577cb125f2e.setContent(html_987b26b2ca6709ec0aa5b0719d1274b6);\\n \\n \\n\\n circle_marker_c51a76946f6e59913c9ab81889674071.bindPopup(popup_25bd36aa04d5ebe8a6498577cb125f2e)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cbcdc9828490d358bb19f49840b851e = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.259537945889915, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_05998e8cc2ffc3e105682f988dbfe814 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_065e95108c0d57f27c2cccd1739cca3a = $(`<div id="html_065e95108c0d57f27c2cccd1739cca3a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05998e8cc2ffc3e105682f988dbfe814.setContent(html_065e95108c0d57f27c2cccd1739cca3a);\\n \\n \\n\\n circle_marker_3cbcdc9828490d358bb19f49840b851e.bindPopup(popup_05998e8cc2ffc3e105682f988dbfe814)\\n ;\\n\\n \\n \\n \\n var circle_marker_d80edd86813836098fcc004b08c77c1e = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_46d385797fa815d7ec1acf5bf5f3f03a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78f4aac2c3839bc912879b0ec684c0d0 = $(`<div id="html_78f4aac2c3839bc912879b0ec684c0d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46d385797fa815d7ec1acf5bf5f3f03a.setContent(html_78f4aac2c3839bc912879b0ec684c0d0);\\n \\n \\n\\n circle_marker_d80edd86813836098fcc004b08c77c1e.bindPopup(popup_46d385797fa815d7ec1acf5bf5f3f03a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5342ed87b49b47827ee98169df1a7cd2 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_76057ba0e7a26746f4093915447a7aea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3d8cd0367bd9089aafe940ebec05b96 = $(`<div id="html_d3d8cd0367bd9089aafe940ebec05b96" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76057ba0e7a26746f4093915447a7aea.setContent(html_d3d8cd0367bd9089aafe940ebec05b96);\\n \\n \\n\\n circle_marker_5342ed87b49b47827ee98169df1a7cd2.bindPopup(popup_76057ba0e7a26746f4093915447a7aea)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3bfc33aecadb592799e7c889ceb6200 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_58261aeaf8405ccb06d4de93b93ce48e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d97770f05cc02c2cc18713a8ff9176e = $(`<div id="html_9d97770f05cc02c2cc18713a8ff9176e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58261aeaf8405ccb06d4de93b93ce48e.setContent(html_9d97770f05cc02c2cc18713a8ff9176e);\\n \\n \\n\\n circle_marker_c3bfc33aecadb592799e7c889ceb6200.bindPopup(popup_58261aeaf8405ccb06d4de93b93ce48e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f965d66a01f9951935029d1631d65e0f = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_385a64dd142d14042da1ca88de1c1e2b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9a53b6b7cda2c5b639ccca5aed2d3f9 = $(`<div id="html_d9a53b6b7cda2c5b639ccca5aed2d3f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_385a64dd142d14042da1ca88de1c1e2b.setContent(html_d9a53b6b7cda2c5b639ccca5aed2d3f9);\\n \\n \\n\\n circle_marker_f965d66a01f9951935029d1631d65e0f.bindPopup(popup_385a64dd142d14042da1ca88de1c1e2b)\\n ;\\n\\n \\n \\n \\n var circle_marker_996f9343dc233ff6fde455bd50ec40dd = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3c097aceb271bbeb919951d72cf81ea9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d61d3452202fdc8fbb26990d358f16ac = $(`<div id="html_d61d3452202fdc8fbb26990d358f16ac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c097aceb271bbeb919951d72cf81ea9.setContent(html_d61d3452202fdc8fbb26990d358f16ac);\\n \\n \\n\\n circle_marker_996f9343dc233ff6fde455bd50ec40dd.bindPopup(popup_3c097aceb271bbeb919951d72cf81ea9)\\n ;\\n\\n \\n \\n \\n var circle_marker_19a3f4b87fe1a9cdcd22d19dbcfbb40b = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.272643200907131, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5550592f03b98d8fe09c37f7fd3935ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3713967a05f108b14fe4566b9dc596dc = $(`<div id="html_3713967a05f108b14fe4566b9dc596dc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5550592f03b98d8fe09c37f7fd3935ca.setContent(html_3713967a05f108b14fe4566b9dc596dc);\\n \\n \\n\\n circle_marker_19a3f4b87fe1a9cdcd22d19dbcfbb40b.bindPopup(popup_5550592f03b98d8fe09c37f7fd3935ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_491313e430cc4306c566c4e7f7b2d967 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7e6b265a73ce08080efdcf8a2a809480 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7313dfc7a932957e986c786952e384a = $(`<div id="html_f7313dfc7a932957e986c786952e384a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e6b265a73ce08080efdcf8a2a809480.setContent(html_f7313dfc7a932957e986c786952e384a);\\n \\n \\n\\n circle_marker_491313e430cc4306c566c4e7f7b2d967.bindPopup(popup_7e6b265a73ce08080efdcf8a2a809480)\\n ;\\n\\n \\n \\n \\n var circle_marker_13739e6295c0d09d001a768150cdc4c2 = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.149617481968136, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_661c0c4f3116f6d64f0a10101d7c3c04 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2f96aaca63300863e6159ef77d8c947e = $(`<div id="html_2f96aaca63300863e6159ef77d8c947e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_661c0c4f3116f6d64f0a10101d7c3c04.setContent(html_2f96aaca63300863e6159ef77d8c947e);\\n \\n \\n\\n circle_marker_13739e6295c0d09d001a768150cdc4c2.bindPopup(popup_661c0c4f3116f6d64f0a10101d7c3c04)\\n ;\\n\\n \\n \\n \\n var circle_marker_d2d4d13953b9df394a8e0596ed8b53c3 = L.circleMarker(\\n [42.7327440918774, -73.26979237266056],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 11.821084554078958, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bab5f8a66a25c5f48079aae68e4623a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_162167b724e20cf467d6301aca2ee500 = $(`<div id="html_162167b724e20cf467d6301aca2ee500" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bab5f8a66a25c5f48079aae68e4623a1.setContent(html_162167b724e20cf467d6301aca2ee500);\\n \\n \\n\\n circle_marker_d2d4d13953b9df394a8e0596ed8b53c3.bindPopup(popup_bab5f8a66a25c5f48079aae68e4623a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_330c5e6ad166b4e1dfe65109436ad341 = L.circleMarker(\\n [42.7327441379601, -73.26856223845904],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 13.434347973244154, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_880ce280ca25df8eadf59e7af0249ff4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50ad35e3a853143bc0d87baf10ca5cc7 = $(`<div id="html_50ad35e3a853143bc0d87baf10ca5cc7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_880ce280ca25df8eadf59e7af0249ff4.setContent(html_50ad35e3a853143bc0d87baf10ca5cc7);\\n \\n \\n\\n circle_marker_330c5e6ad166b4e1dfe65109436ad341.bindPopup(popup_880ce280ca25df8eadf59e7af0249ff4)\\n ;\\n\\n \\n \\n \\n var circle_marker_c517d0cf3b21ae79dbca7bec4efbfbb0 = L.circleMarker(\\n [42.73274417087632, -73.26733210425597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.117274569709014, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_000d51a7789b841ca71fd7e367b5074e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_216e7bc568abecdcf78584b1ce4aa069 = $(`<div id="html_216e7bc568abecdcf78584b1ce4aa069" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_000d51a7789b841ca71fd7e367b5074e.setContent(html_216e7bc568abecdcf78584b1ce4aa069);\\n \\n \\n\\n circle_marker_c517d0cf3b21ae79dbca7bec4efbfbb0.bindPopup(popup_000d51a7789b841ca71fd7e367b5074e)\\n ;\\n\\n \\n \\n \\n var circle_marker_c78f7b73d42ac7fffdae6c15e9ba7183 = L.circleMarker(\\n [42.73274419062606, -73.26610197005185],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.652426491681278, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_569d5f29121e1a9f6883c027ca84d736 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b5c9b73680d560e7b7175608897f1ff6 = $(`<div id="html_b5c9b73680d560e7b7175608897f1ff6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_569d5f29121e1a9f6883c027ca84d736.setContent(html_b5c9b73680d560e7b7175608897f1ff6);\\n \\n \\n\\n circle_marker_c78f7b73d42ac7fffdae6c15e9ba7183.bindPopup(popup_569d5f29121e1a9f6883c027ca84d736)\\n ;\\n\\n \\n \\n \\n var circle_marker_ccc081c71f2154ca35ff599800c84a5d = L.circleMarker(\\n [42.7327441972093, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.383076486422923, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fb13029c026adaff64b0f7ee0b7fb557 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc1e0c72c17d44cbe698e9324e5e5abb = $(`<div id="html_fc1e0c72c17d44cbe698e9324e5e5abb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb13029c026adaff64b0f7ee0b7fb557.setContent(html_fc1e0c72c17d44cbe698e9324e5e5abb);\\n \\n \\n\\n circle_marker_ccc081c71f2154ca35ff599800c84a5d.bindPopup(popup_fb13029c026adaff64b0f7ee0b7fb557)\\n ;\\n\\n \\n \\n \\n var circle_marker_d00f8d6f6d168a58a4d32527a01319d6 = L.circleMarker(\\n [42.73274419062606, -73.26364170164257],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.48482063701911, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_aff0d9d253ee42b1f85bf5839296f368 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d54bb4e5bebf09604840b0a1296ec092 = $(`<div id="html_d54bb4e5bebf09604840b0a1296ec092" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aff0d9d253ee42b1f85bf5839296f368.setContent(html_d54bb4e5bebf09604840b0a1296ec092);\\n \\n \\n\\n circle_marker_d00f8d6f6d168a58a4d32527a01319d6.bindPopup(popup_aff0d9d253ee42b1f85bf5839296f368)\\n ;\\n\\n \\n \\n \\n var circle_marker_1327913a47ca5fae7091461043d5e9d2 = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.389986074159536, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_84be87f0cfb7cf2a71df82d108ffb3f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9f961394729289941ece02b63dd3b922 = $(`<div id="html_9f961394729289941ece02b63dd3b922" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84be87f0cfb7cf2a71df82d108ffb3f4.setContent(html_9f961394729289941ece02b63dd3b922);\\n \\n \\n\\n circle_marker_1327913a47ca5fae7091461043d5e9d2.bindPopup(popup_84be87f0cfb7cf2a71df82d108ffb3f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_295aa580b1ff9c4d5adcbee58796fca4 = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.399277020331919, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_72951616648752e369230cf13ed7171c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_666d3e60879bfb0a3128b1e5ef2f7c63 = $(`<div id="html_666d3e60879bfb0a3128b1e5ef2f7c63" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72951616648752e369230cf13ed7171c.setContent(html_666d3e60879bfb0a3128b1e5ef2f7c63);\\n \\n \\n\\n circle_marker_295aa580b1ff9c4d5adcbee58796fca4.bindPopup(popup_72951616648752e369230cf13ed7171c)\\n ;\\n\\n \\n \\n \\n var circle_marker_9590b5b7d82d48a9dd745e6340031f9b = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.136496464611085, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_58a217f90cd9899af3cf2da60ceee0aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25b6dd68170f5c234404a54766e8dc3a = $(`<div id="html_25b6dd68170f5c234404a54766e8dc3a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58a217f90cd9899af3cf2da60ceee0aa.setContent(html_25b6dd68170f5c234404a54766e8dc3a);\\n \\n \\n\\n circle_marker_9590b5b7d82d48a9dd745e6340031f9b.bindPopup(popup_58a217f90cd9899af3cf2da60ceee0aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e44d835c7cad10dd6cfe626618974d2 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.698035485213008, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_996501af6daec6202f17fd5d3416aa5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_665560861d78d1db15621e930965a8da = $(`<div id="html_665560861d78d1db15621e930965a8da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_996501af6daec6202f17fd5d3416aa5e.setContent(html_665560861d78d1db15621e930965a8da);\\n \\n \\n\\n circle_marker_9e44d835c7cad10dd6cfe626618974d2.bindPopup(popup_996501af6daec6202f17fd5d3416aa5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f261affe8f1524973f0004800161d737 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.920620580763856, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6435b2a20829761b2dd6d5ced55ee795 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5eb4215516b48ce01928a55978c84d9b = $(`<div id="html_5eb4215516b48ce01928a55978c84d9b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6435b2a20829761b2dd6d5ced55ee795.setContent(html_5eb4215516b48ce01928a55978c84d9b);\\n \\n \\n\\n circle_marker_f261affe8f1524973f0004800161d737.bindPopup(popup_6435b2a20829761b2dd6d5ced55ee795)\\n ;\\n\\n \\n \\n \\n var circle_marker_908852d7ee1856b6228106e1ea2d7430 = L.circleMarker(\\n [42.73274377588172, -73.25503076225394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.82078894558317, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fd4ba83a1a484c1f69e47359610c8c77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3bc1c2f76bdc3caa9811d8d312e7de2 = $(`<div id="html_d3bc1c2f76bdc3caa9811d8d312e7de2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd4ba83a1a484c1f69e47359610c8c77.setContent(html_d3bc1c2f76bdc3caa9811d8d312e7de2);\\n \\n \\n\\n circle_marker_908852d7ee1856b6228106e1ea2d7430.bindPopup(popup_fd4ba83a1a484c1f69e47359610c8c77)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a6d405ccde9a95ef6c04fe517e5b991 = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.878478774854901, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e8a5b0cd8843bbb0c3efde1a69a02026 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_deba75c3e74e59e69eaeb8527b873606 = $(`<div id="html_deba75c3e74e59e69eaeb8527b873606" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8a5b0cd8843bbb0c3efde1a69a02026.setContent(html_deba75c3e74e59e69eaeb8527b873606);\\n \\n \\n\\n circle_marker_1a6d405ccde9a95ef6c04fe517e5b991.bindPopup(popup_e8a5b0cd8843bbb0c3efde1a69a02026)\\n ;\\n\\n \\n \\n \\n var circle_marker_6510b89e0c9f698ff41ad41b706d32d3 = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.781222885281108, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_40f86db7f5e3a8d439ea832e9fd778b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14fb6c237e5966310376f31894384f52 = $(`<div id="html_14fb6c237e5966310376f31894384f52" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_40f86db7f5e3a8d439ea832e9fd778b7.setContent(html_14fb6c237e5966310376f31894384f52);\\n \\n \\n\\n circle_marker_6510b89e0c9f698ff41ad41b706d32d3.bindPopup(popup_40f86db7f5e3a8d439ea832e9fd778b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_388880fb982f89d0716332c979112448 = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.406461512053774, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c6dbb6c53e7a271c5305c5071108ae92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_675bdbbeb35ad2376938a7efca3e7336 = $(`<div id="html_675bdbbeb35ad2376938a7efca3e7336" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6dbb6c53e7a271c5305c5071108ae92.setContent(html_675bdbbeb35ad2376938a7efca3e7336);\\n \\n \\n\\n circle_marker_388880fb982f89d0716332c979112448.bindPopup(popup_c6dbb6c53e7a271c5305c5071108ae92)\\n ;\\n\\n \\n \\n \\n var circle_marker_efaa7a2b0c6d6e21d3869ecbb37c31aa = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1214e610e86599ea9885a6f6b66734e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f21eb37d4a00f2a44a64cddb7be59f9 = $(`<div id="html_5f21eb37d4a00f2a44a64cddb7be59f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1214e610e86599ea9885a6f6b66734e5.setContent(html_5f21eb37d4a00f2a44a64cddb7be59f9);\\n \\n \\n\\n circle_marker_efaa7a2b0c6d6e21d3869ecbb37c31aa.bindPopup(popup_1214e610e86599ea9885a6f6b66734e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_35fa3842c38ae29dc02e85ffd08a341b = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a4d27c9bb1cb34b802b91da9e5909cc2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_552cd9416a0cb76ba200cad540a17c4b = $(`<div id="html_552cd9416a0cb76ba200cad540a17c4b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4d27c9bb1cb34b802b91da9e5909cc2.setContent(html_552cd9416a0cb76ba200cad540a17c4b);\\n \\n \\n\\n circle_marker_35fa3842c38ae29dc02e85ffd08a341b.bindPopup(popup_a4d27c9bb1cb34b802b91da9e5909cc2)\\n ;\\n\\n \\n \\n \\n var circle_marker_24bfb5ab33bb2da0fd8d04c78dc87886 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_65ebda3112b50e59830cea57ace1df37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3729e30f134329f8ed2b365c138e9240 = $(`<div id="html_3729e30f134329f8ed2b365c138e9240" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65ebda3112b50e59830cea57ace1df37.setContent(html_3729e30f134329f8ed2b365c138e9240);\\n \\n \\n\\n circle_marker_24bfb5ab33bb2da0fd8d04c78dc87886.bindPopup(popup_65ebda3112b50e59830cea57ace1df37)\\n ;\\n\\n \\n \\n \\n var circle_marker_524456aab290d8d993c4cdcc6b525ca1 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_672da72fe668685558dd14e5601e0aa9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1406865da34242a84e29472b26c7a64 = $(`<div id="html_d1406865da34242a84e29472b26c7a64" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_672da72fe668685558dd14e5601e0aa9.setContent(html_d1406865da34242a84e29472b26c7a64);\\n \\n \\n\\n circle_marker_524456aab290d8d993c4cdcc6b525ca1.bindPopup(popup_672da72fe668685558dd14e5601e0aa9)\\n ;\\n\\n \\n \\n \\n var circle_marker_75847e8deea0f33d04ca0766ce29d3b4 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_02aff764394987c70ba777eb5b0bdf4d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d71d650e0225f21dab2907ae0f31e74d = $(`<div id="html_d71d650e0225f21dab2907ae0f31e74d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_02aff764394987c70ba777eb5b0bdf4d.setContent(html_d71d650e0225f21dab2907ae0f31e74d);\\n \\n \\n\\n circle_marker_75847e8deea0f33d04ca0766ce29d3b4.bindPopup(popup_02aff764394987c70ba777eb5b0bdf4d)\\n ;\\n\\n \\n \\n \\n var circle_marker_295f5b4aa3aedad0755dbe815895fa32 = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3320f1b5fd2fe92d0e3c127e4a92c9fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2efa81b1cf095e336a505ae6eacf3453 = $(`<div id="html_2efa81b1cf095e336a505ae6eacf3453" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3320f1b5fd2fe92d0e3c127e4a92c9fb.setContent(html_2efa81b1cf095e336a505ae6eacf3453);\\n \\n \\n\\n circle_marker_295f5b4aa3aedad0755dbe815895fa32.bindPopup(popup_3320f1b5fd2fe92d0e3c127e4a92c9fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_6afb907ea722001842631574a2cefc8a = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_319a3d85490f333f0a4cffc8cc99ca5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c4c8a0f4692d4b957f1ac114a8998546 = $(`<div id="html_c4c8a0f4692d4b957f1ac114a8998546" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_319a3d85490f333f0a4cffc8cc99ca5a.setContent(html_c4c8a0f4692d4b957f1ac114a8998546);\\n \\n \\n\\n circle_marker_6afb907ea722001842631574a2cefc8a.bindPopup(popup_319a3d85490f333f0a4cffc8cc99ca5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e47a9a515c1b30fa6f9fb53d3cec4a3 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.949327084834294, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_941e6630cd1e91f56b4d684217ce196f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63f5f40e4d39c60522eeb7040c73691d = $(`<div id="html_63f5f40e4d39c60522eeb7040c73691d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_941e6630cd1e91f56b4d684217ce196f.setContent(html_63f5f40e4d39c60522eeb7040c73691d);\\n \\n \\n\\n circle_marker_8e47a9a515c1b30fa6f9fb53d3cec4a3.bindPopup(popup_941e6630cd1e91f56b4d684217ce196f)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee8b5d1fd099dd499581c05569246b02 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f4e29e42739d0b98e44437545f502a38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5715e04e471d991f1e36b5b37d58dd9f = $(`<div id="html_5715e04e471d991f1e36b5b37d58dd9f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4e29e42739d0b98e44437545f502a38.setContent(html_5715e04e471d991f1e36b5b37d58dd9f);\\n \\n \\n\\n circle_marker_ee8b5d1fd099dd499581c05569246b02.bindPopup(popup_f4e29e42739d0b98e44437545f502a38)\\n ;\\n\\n \\n \\n \\n var circle_marker_85b92bf1691703baa08acbf82921c582 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d5bb63aea9bb2de244485ffdcc64c51b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33dea714d8707bed109b6b4787fa87c9 = $(`<div id="html_33dea714d8707bed109b6b4787fa87c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5bb63aea9bb2de244485ffdcc64c51b.setContent(html_33dea714d8707bed109b6b4787fa87c9);\\n \\n \\n\\n circle_marker_85b92bf1691703baa08acbf82921c582.bindPopup(popup_d5bb63aea9bb2de244485ffdcc64c51b)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ffd5dc390a2592c7d74b99c4f8cf50a = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ce58cc2c1618f8114e109f1293866cef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55653c516610a6bf46d9d7f5274f5f5f = $(`<div id="html_55653c516610a6bf46d9d7f5274f5f5f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce58cc2c1618f8114e109f1293866cef.setContent(html_55653c516610a6bf46d9d7f5274f5f5f);\\n \\n \\n\\n circle_marker_1ffd5dc390a2592c7d74b99c4f8cf50a.bindPopup(popup_ce58cc2c1618f8114e109f1293866cef)\\n ;\\n\\n \\n \\n \\n var circle_marker_d36ec74bf5170305a6100af8efee81d4 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b84ca8e30d12a797da3adaa5a34e3b13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f4f4f55d29eaf398beb562e456996249 = $(`<div id="html_f4f4f55d29eaf398beb562e456996249" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b84ca8e30d12a797da3adaa5a34e3b13.setContent(html_f4f4f55d29eaf398beb562e456996249);\\n \\n \\n\\n circle_marker_d36ec74bf5170305a6100af8efee81d4.bindPopup(popup_b84ca8e30d12a797da3adaa5a34e3b13)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a3a3270adcf1c5238300b6d05e32feb = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e8d6a3107aa0b8292aec7b8586aba8cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_52d90db4fc280b013e363576b65d1225 = $(`<div id="html_52d90db4fc280b013e363576b65d1225" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8d6a3107aa0b8292aec7b8586aba8cc.setContent(html_52d90db4fc280b013e363576b65d1225);\\n \\n \\n\\n circle_marker_5a3a3270adcf1c5238300b6d05e32feb.bindPopup(popup_e8d6a3107aa0b8292aec7b8586aba8cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_a07f90b042c3fb0ce45ad5e7b7d1f9ff = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_1931ec116ab7d877173efce138d2e7ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30d4963b7b5645bf4352156aefd4cea4 = $(`<div id="html_30d4963b7b5645bf4352156aefd4cea4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1931ec116ab7d877173efce138d2e7ec.setContent(html_30d4963b7b5645bf4352156aefd4cea4);\\n \\n \\n\\n circle_marker_a07f90b042c3fb0ce45ad5e7b7d1f9ff.bindPopup(popup_1931ec116ab7d877173efce138d2e7ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_b3e96d417d90054a23a3ea9b9df78181 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_89e54eab1cf2c78470bc0ee5356d129b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1a5582b8c9b524566f3b779fdff0551 = $(`<div id="html_d1a5582b8c9b524566f3b779fdff0551" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89e54eab1cf2c78470bc0ee5356d129b.setContent(html_d1a5582b8c9b524566f3b779fdff0551);\\n \\n \\n\\n circle_marker_b3e96d417d90054a23a3ea9b9df78181.bindPopup(popup_89e54eab1cf2c78470bc0ee5356d129b)\\n ;\\n\\n \\n \\n \\n var circle_marker_49a684397d0615769502229bce352c2f = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_650c40ba0f1690104bf24d92fb1b03a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5475cc35183b0ad4ca7ce568d2b1eac4 = $(`<div id="html_5475cc35183b0ad4ca7ce568d2b1eac4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_650c40ba0f1690104bf24d92fb1b03a9.setContent(html_5475cc35183b0ad4ca7ce568d2b1eac4);\\n \\n \\n\\n circle_marker_49a684397d0615769502229bce352c2f.bindPopup(popup_650c40ba0f1690104bf24d92fb1b03a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9608ad101fdbb5a29fad41604d58bf00 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.863662517577698, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8d0441235461d37651d436252da50806 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c2323a056d030e6e3c9d90af94d530c4 = $(`<div id="html_c2323a056d030e6e3c9d90af94d530c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d0441235461d37651d436252da50806.setContent(html_c2323a056d030e6e3c9d90af94d530c4);\\n \\n \\n\\n circle_marker_9608ad101fdbb5a29fad41604d58bf00.bindPopup(popup_8d0441235461d37651d436252da50806)\\n ;\\n\\n \\n \\n \\n var circle_marker_f400a807801ed964ef9aba147bfc2db7 = L.circleMarker(\\n [42.73093682684808, -73.27225242598358],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.387831891992912, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e5ed360a05d58d5062f096b5728a0bb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86006c03292ae22268f1218b5878d56b = $(`<div id="html_86006c03292ae22268f1218b5878d56b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5ed360a05d58d5062f096b5728a0bb1.setContent(html_86006c03292ae22268f1218b5878d56b);\\n \\n \\n\\n circle_marker_f400a807801ed964ef9aba147bfc2db7.bindPopup(popup_e5ed360a05d58d5062f096b5728a0bb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_8dace0b3155bd8d6e7edba028a01a789 = L.circleMarker(\\n [42.730936899260826, -73.2710223276323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.023896332520351, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4e6971955f5c4e90702fee5c1b83bdd2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b7fb018f3806e5a8da0d7fb1bcbc4082 = $(`<div id="html_b7fb018f3806e5a8da0d7fb1bcbc4082" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e6971955f5c4e90702fee5c1b83bdd2.setContent(html_b7fb018f3806e5a8da0d7fb1bcbc4082);\\n \\n \\n\\n circle_marker_8dace0b3155bd8d6e7edba028a01a789.bindPopup(popup_4e6971955f5c4e90702fee5c1b83bdd2)\\n ;\\n\\n \\n \\n \\n var circle_marker_05bd72aef2250c438310d14dde5d52a4 = L.circleMarker(\\n [42.730936958507606, -73.26979222927841],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.117274569709014, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_90c39a1b5ca20ae4264e4ae168063010 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_795daee05f55af5055dd386f02ce4a13 = $(`<div id="html_795daee05f55af5055dd386f02ce4a13" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90c39a1b5ca20ae4264e4ae168063010.setContent(html_795daee05f55af5055dd386f02ce4a13);\\n \\n \\n\\n circle_marker_05bd72aef2250c438310d14dde5d52a4.bindPopup(popup_90c39a1b5ca20ae4264e4ae168063010)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc3b9f7e424035b41e509fa77adb9721 = L.circleMarker(\\n [42.73093700458845, -73.26856213092245],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.686510657907603, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3e508f822fbf053f83b2bb1fd5924d26 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9934c5dfee123a8542206cd02f0293da = $(`<div id="html_9934c5dfee123a8542206cd02f0293da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e508f822fbf053f83b2bb1fd5924d26.setContent(html_9934c5dfee123a8542206cd02f0293da);\\n \\n \\n\\n circle_marker_dc3b9f7e424035b41e509fa77adb9721.bindPopup(popup_3e508f822fbf053f83b2bb1fd5924d26)\\n ;\\n\\n \\n \\n \\n var circle_marker_75be5b7a113cc04e785cfaed6c51e08b = L.circleMarker(\\n [42.73093703750333, -73.26733203256491],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.373021315815206, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e448989f714558197bcc7d62fedc042a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_18f36e94582f7af45a9df16802fce3f2 = $(`<div id="html_18f36e94582f7af45a9df16802fce3f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e448989f714558197bcc7d62fedc042a.setContent(html_18f36e94582f7af45a9df16802fce3f2);\\n \\n \\n\\n circle_marker_75be5b7a113cc04e785cfaed6c51e08b.bindPopup(popup_e448989f714558197bcc7d62fedc042a)\\n ;\\n\\n \\n \\n \\n var circle_marker_3694cd9cb6369c5d12dd8787c0efc85a = L.circleMarker(\\n [42.73093705725226, -73.26610193420632],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.114761669619748, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8bc8ed3977211b1f16bbcc19c508a43e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_052b79e2a0d6d62fe6ac4f66d6475f63 = $(`<div id="html_052b79e2a0d6d62fe6ac4f66d6475f63" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8bc8ed3977211b1f16bbcc19c508a43e.setContent(html_052b79e2a0d6d62fe6ac4f66d6475f63);\\n \\n \\n\\n circle_marker_3694cd9cb6369c5d12dd8787c0efc85a.bindPopup(popup_8bc8ed3977211b1f16bbcc19c508a43e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b4985941656ced100e5dce1a7b081249 = L.circleMarker(\\n [42.73093706383524, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.07796550300118, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5ce6154ba7fb1f9a4ae7d2e1b2f74084 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d1e5bab10a3e3dcc9c69b7d82f6e1b6 = $(`<div id="html_8d1e5bab10a3e3dcc9c69b7d82f6e1b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ce6154ba7fb1f9a4ae7d2e1b2f74084.setContent(html_8d1e5bab10a3e3dcc9c69b7d82f6e1b6);\\n \\n \\n\\n circle_marker_b4985941656ced100e5dce1a7b081249.bindPopup(popup_5ce6154ba7fb1f9a4ae7d2e1b2f74084)\\n ;\\n\\n \\n \\n \\n var circle_marker_d83907ad7ee9944a522da8390fe30db7 = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.998767850296815, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c3b5eb47142dc810db7f778253979ee0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_763f1f5bdf23163206327baa10257cf2 = $(`<div id="html_763f1f5bdf23163206327baa10257cf2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3b5eb47142dc810db7f778253979ee0.setContent(html_763f1f5bdf23163206327baa10257cf2);\\n \\n \\n\\n circle_marker_d83907ad7ee9944a522da8390fe30db7.bindPopup(popup_c3b5eb47142dc810db7f778253979ee0)\\n ;\\n\\n \\n \\n \\n var circle_marker_7dec73b401547cc22869533270eda323 = L.circleMarker(\\n [42.73093703750333, -73.26241163912951],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.442171739215616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3b98a4f6bbb5359383b133dfc5c3ebf7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33634829a9261ecc2eac70bd2f3bcad6 = $(`<div id="html_33634829a9261ecc2eac70bd2f3bcad6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3b98a4f6bbb5359383b133dfc5c3ebf7.setContent(html_33634829a9261ecc2eac70bd2f3bcad6);\\n \\n \\n\\n circle_marker_7dec73b401547cc22869533270eda323.bindPopup(popup_3b98a4f6bbb5359383b133dfc5c3ebf7)\\n ;\\n\\n \\n \\n \\n var circle_marker_34f912c5654203022c554a45ba3e6769 = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.991702346646203, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_cf2ea60f544fad26a02b4e6dffb548ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_942efa56c3cae7fbac7064daeb5abbe1 = $(`<div id="html_942efa56c3cae7fbac7064daeb5abbe1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf2ea60f544fad26a02b4e6dffb548ca.setContent(html_942efa56c3cae7fbac7064daeb5abbe1);\\n \\n \\n\\n circle_marker_34f912c5654203022c554a45ba3e6769.bindPopup(popup_cf2ea60f544fad26a02b4e6dffb548ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_ef2a80d378b7408c8d68991d5ce4a606 = L.circleMarker(\\n [42.730936958507606, -73.259951442416],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.0270333367641, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bc1f0d11eb3d6e32afee3025b61ae904 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2efb5eb920e7ca68ce56f89c15e0d526 = $(`<div id="html_2efb5eb920e7ca68ce56f89c15e0d526" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc1f0d11eb3d6e32afee3025b61ae904.setContent(html_2efb5eb920e7ca68ce56f89c15e0d526);\\n \\n \\n\\n circle_marker_ef2a80d378b7408c8d68991d5ce4a606.bindPopup(popup_bc1f0d11eb3d6e32afee3025b61ae904)\\n ;\\n\\n \\n \\n \\n var circle_marker_4c4e3f979b22d39855f1431d337d5194 = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.746726148605861, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7d7570fa269d2a247cb885f0b64e1c70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8359fc74cf287f7bbb46d5532893d072 = $(`<div id="html_8359fc74cf287f7bbb46d5532893d072" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d7570fa269d2a247cb885f0b64e1c70.setContent(html_8359fc74cf287f7bbb46d5532893d072);\\n \\n \\n\\n circle_marker_4c4e3f979b22d39855f1431d337d5194.bindPopup(popup_7d7570fa269d2a247cb885f0b64e1c70)\\n ;\\n\\n \\n \\n \\n var circle_marker_156294493886ef2717e48c8a8cf7aae5 = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.699380116989518, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_43b7e6a63807b846074f3a6ae6962c02 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07d2e653c0e5a8a18a9dd5541edd6fde = $(`<div id="html_07d2e653c0e5a8a18a9dd5541edd6fde" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43b7e6a63807b846074f3a6ae6962c02.setContent(html_07d2e653c0e5a8a18a9dd5541edd6fde);\\n \\n \\n\\n circle_marker_156294493886ef2717e48c8a8cf7aae5.bindPopup(popup_43b7e6a63807b846074f3a6ae6962c02)\\n ;\\n\\n \\n \\n \\n var circle_marker_53ffe642a3a6930f7c9388f9ad93490a = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4115163798060095, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6fb90e98b7f4464b6cf43fa9fd2c5b87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3bdcbdd2a8134f7a5baf8f12db28c93d = $(`<div id="html_3bdcbdd2a8134f7a5baf8f12db28c93d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6fb90e98b7f4464b6cf43fa9fd2c5b87.setContent(html_3bdcbdd2a8134f7a5baf8f12db28c93d);\\n \\n \\n\\n circle_marker_53ffe642a3a6930f7c9388f9ad93490a.bindPopup(popup_6fb90e98b7f4464b6cf43fa9fd2c5b87)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d88ce26416e743bb9bd3d2f06b54bf3 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.440847306724618, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_39923d0af86e7876acfb2855ac516149 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01cbaa552c720f489536c063bc713af8 = $(`<div id="html_01cbaa552c720f489536c063bc713af8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39923d0af86e7876acfb2855ac516149.setContent(html_01cbaa552c720f489536c063bc713af8);\\n \\n \\n\\n circle_marker_5d88ce26416e743bb9bd3d2f06b54bf3.bindPopup(popup_39923d0af86e7876acfb2855ac516149)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ea30619483dc3372d78a5d2dab90dd2 = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.673807981960539, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_78bc20a10d5f38c9b296bcc1f184eb10 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d18f30028f38fe3b3bb6f23c7f4e42a6 = $(`<div id="html_d18f30028f38fe3b3bb6f23c7f4e42a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_78bc20a10d5f38c9b296bcc1f184eb10.setContent(html_d18f30028f38fe3b3bb6f23c7f4e42a6);\\n \\n \\n\\n circle_marker_1ea30619483dc3372d78a5d2dab90dd2.bindPopup(popup_78bc20a10d5f38c9b296bcc1f184eb10)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c946965a411fe16970e68e1333ab04e = L.circleMarker(\\n [42.73093640553757, -73.2525708523423],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.23618154310838, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_db24d3b2f597145824482d7efccaf940 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_353dd77631b677e537f23f6668b21c30 = $(`<div id="html_353dd77631b677e537f23f6668b21c30" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db24d3b2f597145824482d7efccaf940.setContent(html_353dd77631b677e537f23f6668b21c30);\\n \\n \\n\\n circle_marker_2c946965a411fe16970e68e1333ab04e.bindPopup(popup_db24d3b2f597145824482d7efccaf940)\\n ;\\n\\n \\n \\n \\n var circle_marker_c351ec2c07c670dd1629cf5350f17577 = L.circleMarker(\\n [42.73093626729506, -73.25134075401192],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.124020118074668, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e7ecacc7da47296b13f5031df8a85d1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92ec1f5d31c7785fbb6597db95648607 = $(`<div id="html_92ec1f5d31c7785fbb6597db95648607" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e7ecacc7da47296b13f5031df8a85d1b.setContent(html_92ec1f5d31c7785fbb6597db95648607);\\n \\n \\n\\n circle_marker_c351ec2c07c670dd1629cf5350f17577.bindPopup(popup_e7ecacc7da47296b13f5031df8a85d1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_98db1d548a707b9408202c8627bf01bd = L.circleMarker(\\n [42.73093611588659, -73.2501106556873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.6488625410045, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f4b5e3e63a980553bf8c56221cbc197e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_801dfe4ddd3f567045468aca29ddce60 = $(`<div id="html_801dfe4ddd3f567045468aca29ddce60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4b5e3e63a980553bf8c56221cbc197e.setContent(html_801dfe4ddd3f567045468aca29ddce60);\\n \\n \\n\\n circle_marker_98db1d548a707b9408202c8627bf01bd.bindPopup(popup_f4b5e3e63a980553bf8c56221cbc197e)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fd4b833569e3f70c958c368ccb9211a = L.circleMarker(\\n [42.73093595131219, -73.24888055736892],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.001408606295148, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4f06379544764410338bcd766526cf4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1818e1ec1bc0b86366987bf383b4c77e = $(`<div id="html_1818e1ec1bc0b86366987bf383b4c77e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f06379544764410338bcd766526cf4b.setContent(html_1818e1ec1bc0b86366987bf383b4c77e);\\n \\n \\n\\n circle_marker_9fd4b833569e3f70c958c368ccb9211a.bindPopup(popup_4f06379544764410338bcd766526cf4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_4fe564ab5070011c40a36911f83dd2a3 = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_97d1a7f137faaeff1f2abfc8f6476fb6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_485d34ab27653f1eb6d546cd1b38da7e = $(`<div id="html_485d34ab27653f1eb6d546cd1b38da7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97d1a7f137faaeff1f2abfc8f6476fb6.setContent(html_485d34ab27653f1eb6d546cd1b38da7e);\\n \\n \\n\\n circle_marker_4fe564ab5070011c40a36911f83dd2a3.bindPopup(popup_97d1a7f137faaeff1f2abfc8f6476fb6)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff1973cb4cdf31da0b8993ffbd171cbd = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5473579f5324c768ba52360e2ab1612f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_393ccc38dede683074965a40c2e578dd = $(`<div id="html_393ccc38dede683074965a40c2e578dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5473579f5324c768ba52360e2ab1612f.setContent(html_393ccc38dede683074965a40c2e578dd);\\n \\n \\n\\n circle_marker_ff1973cb4cdf31da0b8993ffbd171cbd.bindPopup(popup_5473579f5324c768ba52360e2ab1612f)\\n ;\\n\\n \\n \\n \\n var circle_marker_81da60103cbc7f3b9a7ea3dfd717e451 = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.970821321441846, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_535c1b41eb638a6221c2e1600fdc20df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_565b9810e33ccc559f459a9240797a06 = $(`<div id="html_565b9810e33ccc559f459a9240797a06" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_535c1b41eb638a6221c2e1600fdc20df.setContent(html_565b9810e33ccc559f459a9240797a06);\\n \\n \\n\\n circle_marker_81da60103cbc7f3b9a7ea3dfd717e451.bindPopup(popup_535c1b41eb638a6221c2e1600fdc20df)\\n ;\\n\\n \\n \\n \\n var circle_marker_b9b4267e698eb6ee605477398ec43235 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.352372348458314, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_545c6b61ee28fd660f91fc4fdf4588c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4c13df25b8077bdb10375ced2c297da5 = $(`<div id="html_4c13df25b8077bdb10375ced2c297da5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_545c6b61ee28fd660f91fc4fdf4588c4.setContent(html_4c13df25b8077bdb10375ced2c297da5);\\n \\n \\n\\n circle_marker_b9b4267e698eb6ee605477398ec43235.bindPopup(popup_545c6b61ee28fd660f91fc4fdf4588c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_49c3eafaad0335c4390103df49a3ad2a = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.51907589177983, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0d7bee483552d05bfb8960ccef90a077 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64c5f8ec85fe923d46f4ca3b94a86d18 = $(`<div id="html_64c5f8ec85fe923d46f4ca3b94a86d18" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d7bee483552d05bfb8960ccef90a077.setContent(html_64c5f8ec85fe923d46f4ca3b94a86d18);\\n \\n \\n\\n circle_marker_49c3eafaad0335c4390103df49a3ad2a.bindPopup(popup_0d7bee483552d05bfb8960ccef90a077)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b2cc4a1369625b5498a3d83eccb2b19 = L.circleMarker(\\n [42.730934687380696, -73.24149996761956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.944106103225343, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ad4e864d6d644c779810ba218d8977af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec35d22d155ff76ea5669cb21d7d5bc6 = $(`<div id="html_ec35d22d155ff76ea5669cb21d7d5bc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad4e864d6d644c779810ba218d8977af.setContent(html_ec35d22d155ff76ea5669cb21d7d5bc6);\\n \\n \\n\\n circle_marker_8b2cc4a1369625b5498a3d83eccb2b19.bindPopup(popup_ad4e864d6d644c779810ba218d8977af)\\n ;\\n\\n \\n \\n \\n var circle_marker_adb9c6d85cabcd912446af15d219a5cb = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_14ba899e3f672fc3e5c176535f4d693f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64a517011f9476351fefa744a1e7e2f1 = $(`<div id="html_64a517011f9476351fefa744a1e7e2f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14ba899e3f672fc3e5c176535f4d693f.setContent(html_64a517011f9476351fefa744a1e7e2f1);\\n \\n \\n\\n circle_marker_adb9c6d85cabcd912446af15d219a5cb.bindPopup(popup_14ba899e3f672fc3e5c176535f4d693f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1600aed7878ada656fa46f43d425ba34 = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.3336224206596095, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_684415d9f0b81bc618e57b037777ec61 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4bda21b5fc288e40604d8d72b0243d0c = $(`<div id="html_4bda21b5fc288e40604d8d72b0243d0c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_684415d9f0b81bc618e57b037777ec61.setContent(html_4bda21b5fc288e40604d8d72b0243d0c);\\n \\n \\n\\n circle_marker_1600aed7878ada656fa46f43d425ba34.bindPopup(popup_684415d9f0b81bc618e57b037777ec61)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0a16e34036be23d91968d1cb050e5b7 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f9dc7538a87118d1b2a37d64007d001b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d6dbf0705cdd0479b64619d1b902f01 = $(`<div id="html_6d6dbf0705cdd0479b64619d1b902f01" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9dc7538a87118d1b2a37d64007d001b.setContent(html_6d6dbf0705cdd0479b64619d1b902f01);\\n \\n \\n\\n circle_marker_c0a16e34036be23d91968d1cb050e5b7.bindPopup(popup_f9dc7538a87118d1b2a37d64007d001b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9c9ad80b7d58e47c2a284b76cac8baf6 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_71b29e3c25ae4257ee3b87dc8c013138 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_689a8e31342cc4d9d44a9415ad81e27f = $(`<div id="html_689a8e31342cc4d9d44a9415ad81e27f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71b29e3c25ae4257ee3b87dc8c013138.setContent(html_689a8e31342cc4d9d44a9415ad81e27f);\\n \\n \\n\\n circle_marker_9c9ad80b7d58e47c2a284b76cac8baf6.bindPopup(popup_71b29e3c25ae4257ee3b87dc8c013138)\\n ;\\n\\n \\n \\n \\n var circle_marker_881d326f5ed0d76a41dcba9f30bfcfa1 = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_688242d3c2ed1ca3574c063503a9b175 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_928b217f2681dbfc1a14f44d0e260c53 = $(`<div id="html_928b217f2681dbfc1a14f44d0e260c53" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_688242d3c2ed1ca3574c063503a9b175.setContent(html_928b217f2681dbfc1a14f44d0e260c53);\\n \\n \\n\\n circle_marker_881d326f5ed0d76a41dcba9f30bfcfa1.bindPopup(popup_688242d3c2ed1ca3574c063503a9b175)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d93873d064eaa7d5349084fff875a22 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.753945931439391, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4a14b80c66f2b889e883b75821d5cf10 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b4605104e9b0af593a064ec6958dee2 = $(`<div id="html_7b4605104e9b0af593a064ec6958dee2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a14b80c66f2b889e883b75821d5cf10.setContent(html_7b4605104e9b0af593a064ec6958dee2);\\n \\n \\n\\n circle_marker_3d93873d064eaa7d5349084fff875a22.bindPopup(popup_4a14b80c66f2b889e883b75821d5cf10)\\n ;\\n\\n \\n \\n \\n var circle_marker_578d4763341fd549f77618a8f4e12117 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7c539d3fec48936b4a4c334a5261eb85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f150aa5b79273cdc0f2c0d62a63d231 = $(`<div id="html_7f150aa5b79273cdc0f2c0d62a63d231" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c539d3fec48936b4a4c334a5261eb85.setContent(html_7f150aa5b79273cdc0f2c0d62a63d231);\\n \\n \\n\\n circle_marker_578d4763341fd549f77618a8f4e12117.bindPopup(popup_7c539d3fec48936b4a4c334a5261eb85)\\n ;\\n\\n \\n \\n \\n var circle_marker_57cdf456ffd64bd863e4ea86c25c4451 = L.circleMarker(\\n [42.73092945391477, -73.2230484950559],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_29ce5579dd04e7aea7736e31107c73a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c82f6466ef1655a874d2dabf0dbec870 = $(`<div id="html_c82f6466ef1655a874d2dabf0dbec870" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_29ce5579dd04e7aea7736e31107c73a0.setContent(html_c82f6466ef1655a874d2dabf0dbec870);\\n \\n \\n\\n circle_marker_57cdf456ffd64bd863e4ea86c25c4451.bindPopup(popup_29ce5579dd04e7aea7736e31107c73a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_000af3d9149c7c67ee34ba9528bfec9d = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_794d62903b9beb8080261fb4881a28cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7923c7445015ea6e381674bcaa0f7222 = $(`<div id="html_7923c7445015ea6e381674bcaa0f7222" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_794d62903b9beb8080261fb4881a28cc.setContent(html_7923c7445015ea6e381674bcaa0f7222);\\n \\n \\n\\n circle_marker_000af3d9149c7c67ee34ba9528bfec9d.bindPopup(popup_794d62903b9beb8080261fb4881a28cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_1849cd224272441244e22314d3a946a9 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f62162a164c93f9de2b9cc7ae2c565ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf5cc14baae483cb1a2324514dc99cd4 = $(`<div id="html_bf5cc14baae483cb1a2324514dc99cd4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f62162a164c93f9de2b9cc7ae2c565ef.setContent(html_bf5cc14baae483cb1a2324514dc99cd4);\\n \\n \\n\\n circle_marker_1849cd224272441244e22314d3a946a9.bindPopup(popup_f62162a164c93f9de2b9cc7ae2c565ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d3dd994fcff2e05067f38eccb0c103f = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f5f4b7a75085df7eb603bd827dabbbf6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9797ad1151178f3a60b3f4f8eba68506 = $(`<div id="html_9797ad1151178f3a60b3f4f8eba68506" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5f4b7a75085df7eb603bd827dabbbf6.setContent(html_9797ad1151178f3a60b3f4f8eba68506);\\n \\n \\n\\n circle_marker_1d3dd994fcff2e05067f38eccb0c103f.bindPopup(popup_f5f4b7a75085df7eb603bd827dabbbf6)\\n ;\\n\\n \\n \\n \\n var circle_marker_e919cab3af727c1ddf4f11a025abfd1e = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0de24774d0521482ef37f3431352c12c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4437096796f35a2d3d14327cf08b011f = $(`<div id="html_4437096796f35a2d3d14327cf08b011f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0de24774d0521482ef37f3431352c12c.setContent(html_4437096796f35a2d3d14327cf08b011f);\\n \\n \\n\\n circle_marker_e919cab3af727c1ddf4f11a025abfd1e.bindPopup(popup_0de24774d0521482ef37f3431352c12c)\\n ;\\n\\n \\n \\n \\n var circle_marker_96de707e7cf5352f192d40187b80e2cd = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9a308a71c013e550b41c08ce84903be8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_019872a2e72b8a6441a3d666525bfe3f = $(`<div id="html_019872a2e72b8a6441a3d666525bfe3f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a308a71c013e550b41c08ce84903be8.setContent(html_019872a2e72b8a6441a3d666525bfe3f);\\n \\n \\n\\n circle_marker_96de707e7cf5352f192d40187b80e2cd.bindPopup(popup_9a308a71c013e550b41c08ce84903be8)\\n ;\\n\\n \\n \\n \\n var circle_marker_4dfe645b71a29d93facda61a563cc3a6 = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.938444016277748, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_efabff18c46af9821a537937b774a8de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_35492ffbd378eea1014792cbf3564a49 = $(`<div id="html_35492ffbd378eea1014792cbf3564a49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_efabff18c46af9821a537937b774a8de.setContent(html_35492ffbd378eea1014792cbf3564a49);\\n \\n \\n\\n circle_marker_4dfe645b71a29d93facda61a563cc3a6.bindPopup(popup_efabff18c46af9821a537937b774a8de)\\n ;\\n\\n \\n \\n \\n var circle_marker_ccd01bbb3604497a5789bf760839e667 = L.circleMarker(\\n [42.729129693483614, -73.27225221092779],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.569397566060481, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a65d87807a2920c4b9ca2a6f9dbdecfa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d09d6836d41859bac2d40e41f326cd4c = $(`<div id="html_d09d6836d41859bac2d40e41f326cd4c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a65d87807a2920c4b9ca2a6f9dbdecfa.setContent(html_d09d6836d41859bac2d40e41f326cd4c);\\n \\n \\n\\n circle_marker_ccd01bbb3604497a5789bf760839e667.bindPopup(popup_a65d87807a2920c4b9ca2a6f9dbdecfa)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d031668ef2e46f6268d45d2aba47cbc = L.circleMarker(\\n [42.72912976589343, -73.27102214841915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.574939283076915, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e4acf5c5b84386af3b05e8c89faff4e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_167848593e2b352b63b4140cc79ef377 = $(`<div id="html_167848593e2b352b63b4140cc79ef377" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4acf5c5b84386af3b05e8c89faff4e0.setContent(html_167848593e2b352b63b4140cc79ef377);\\n \\n \\n\\n circle_marker_3d031668ef2e46f6268d45d2aba47cbc.bindPopup(popup_e4acf5c5b84386af3b05e8c89faff4e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_b2e87b51b5adb47e217024990cc2437e = L.circleMarker(\\n [42.72912982513782, -73.2697920859079],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.368283871884005, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a3eea632d07b255565c7fe5c8a088870 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa5cc7a27f7d1ab4bd762d3e72276401 = $(`<div id="html_aa5cc7a27f7d1ab4bd762d3e72276401" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3eea632d07b255565c7fe5c8a088870.setContent(html_aa5cc7a27f7d1ab4bd762d3e72276401);\\n \\n \\n\\n circle_marker_b2e87b51b5adb47e217024990cc2437e.bindPopup(popup_a3eea632d07b255565c7fe5c8a088870)\\n ;\\n\\n \\n \\n \\n var circle_marker_21034930b389cb7ad9a5f00fb9198e14 = L.circleMarker(\\n [42.729129871216784, -73.26856202339455],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.304852983362556, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a87062831980e95e673e2687d08e0cfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c9af478da2b1fbfbfd757c38c226554 = $(`<div id="html_9c9af478da2b1fbfbfd757c38c226554" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a87062831980e95e673e2687d08e0cfe.setContent(html_9c9af478da2b1fbfbfd757c38c226554);\\n \\n \\n\\n circle_marker_21034930b389cb7ad9a5f00fb9198e14.bindPopup(popup_a87062831980e95e673e2687d08e0cfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e97a753283dbd1381403f316467720e = L.circleMarker(\\n [42.72912990413034, -73.26733196087964],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.781222885281108, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a464f409b6bdc975c41b3052256e3406 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c3e45b474e0cac951ef7edfbb5e64d73 = $(`<div id="html_c3e45b474e0cac951ef7edfbb5e64d73" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a464f409b6bdc975c41b3052256e3406.setContent(html_c3e45b474e0cac951ef7edfbb5e64d73);\\n \\n \\n\\n circle_marker_3e97a753283dbd1381403f316467720e.bindPopup(popup_a464f409b6bdc975c41b3052256e3406)\\n ;\\n\\n \\n \\n \\n var circle_marker_757aa95cd1994915e47b41f6d4931a2e = L.circleMarker(\\n [42.72912992387847, -73.26610189836369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.140011726956917, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_64f8147ff4509a3b00776543fc6995cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32ce4cdb7ec3c9f9a3104d060245b153 = $(`<div id="html_32ce4cdb7ec3c9f9a3104d060245b153" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64f8147ff4509a3b00776543fc6995cd.setContent(html_32ce4cdb7ec3c9f9a3104d060245b153);\\n \\n \\n\\n circle_marker_757aa95cd1994915e47b41f6d4931a2e.bindPopup(popup_64f8147ff4509a3b00776543fc6995cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e4f6b22aeb6bddac1d5d9c79e8587a3 = L.circleMarker(\\n [42.72912993046118, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.944106103225343, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c03bea7a3667ea306a177f291a641e78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d4e91eadbe072781d86a41d3f9d2120 = $(`<div id="html_0d4e91eadbe072781d86a41d3f9d2120" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c03bea7a3667ea306a177f291a641e78.setContent(html_0d4e91eadbe072781d86a41d3f9d2120);\\n \\n \\n\\n circle_marker_8e4f6b22aeb6bddac1d5d9c79e8587a3.bindPopup(popup_c03bea7a3667ea306a177f291a641e78)\\n ;\\n\\n \\n \\n \\n var circle_marker_98c7d94d7d06be2afcf0cad69b71f392 = L.circleMarker(\\n [42.72912992387847, -73.26364177333073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.770275002573076, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_dd821304634e1978ed7098be09fadbf4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a8b865a4df6456a5cdc208e96c1836a = $(`<div id="html_6a8b865a4df6456a5cdc208e96c1836a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd821304634e1978ed7098be09fadbf4.setContent(html_6a8b865a4df6456a5cdc208e96c1836a);\\n \\n \\n\\n circle_marker_98c7d94d7d06be2afcf0cad69b71f392.bindPopup(popup_dd821304634e1978ed7098be09fadbf4)\\n ;\\n\\n \\n \\n \\n var circle_marker_981774b21fd0be7d0d7545b9696ed080 = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.156394191823509, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a3cc94bbe4ed1ddc58a6521155364c59 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e136a4cb65fda21d9b0e8e35bf0dbd2 = $(`<div id="html_8e136a4cb65fda21d9b0e8e35bf0dbd2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3cc94bbe4ed1ddc58a6521155364c59.setContent(html_8e136a4cb65fda21d9b0e8e35bf0dbd2);\\n \\n \\n\\n circle_marker_981774b21fd0be7d0d7545b9696ed080.bindPopup(popup_a3cc94bbe4ed1ddc58a6521155364c59)\\n ;\\n\\n \\n \\n \\n var circle_marker_cbe5859f282a6c4e8f88e483776f4c28 = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.720348719413148, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fe0c88f917486bd7b529c5e5abbf23c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d9efa09651ea953a7d8d725aa617408 = $(`<div id="html_3d9efa09651ea953a7d8d725aa617408" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe0c88f917486bd7b529c5e5abbf23c9.setContent(html_3d9efa09651ea953a7d8d725aa617408);\\n \\n \\n\\n circle_marker_cbe5859f282a6c4e8f88e483776f4c28.bindPopup(popup_fe0c88f917486bd7b529c5e5abbf23c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_7808d5de0eb94f77a7e9103666635c35 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.746726148605861, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f487d9059bdfce46481a2333472283bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ab9c2e84b2d4d02f5485d0bc152ac1d = $(`<div id="html_3ab9c2e84b2d4d02f5485d0bc152ac1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f487d9059bdfce46481a2333472283bc.setContent(html_3ab9c2e84b2d4d02f5485d0bc152ac1d);\\n \\n \\n\\n circle_marker_7808d5de0eb94f77a7e9103666635c35.bindPopup(popup_f487d9059bdfce46481a2333472283bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_82b2f518b86c8d1d55634104ab90ac32 = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.54834217738561, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6d59376f9b4a0bb1a4f19a7a863b3917 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_34802b6c398ae1bcbf1546c89db639fb = $(`<div id="html_34802b6c398ae1bcbf1546c89db639fb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d59376f9b4a0bb1a4f19a7a863b3917.setContent(html_34802b6c398ae1bcbf1546c89db639fb);\\n \\n \\n\\n circle_marker_82b2f518b86c8d1d55634104ab90ac32.bindPopup(popup_6d59376f9b4a0bb1a4f19a7a863b3917)\\n ;\\n\\n \\n \\n \\n var circle_marker_931e8969d2dbc6e4f60cca1f61b31817 = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.527227892173502, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_480ef8d45f22c065e5b5b32fcd7dd365 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f8d3c8d0b108d706dddc2acc8a4512a = $(`<div id="html_3f8d3c8d0b108d706dddc2acc8a4512a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_480ef8d45f22c065e5b5b32fcd7dd365.setContent(html_3f8d3c8d0b108d706dddc2acc8a4512a);\\n \\n \\n\\n circle_marker_931e8969d2dbc6e4f60cca1f61b31817.bindPopup(popup_480ef8d45f22c065e5b5b32fcd7dd365)\\n ;\\n\\n \\n \\n \\n var circle_marker_75211e0874e409ff45c62f61cdff51df = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.555290583552474, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_76fd2f1a889b81f355657b5fd2b4af33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69f270d2b1bdc8d4028903b27bc996f8 = $(`<div id="html_69f270d2b1bdc8d4028903b27bc996f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76fd2f1a889b81f355657b5fd2b4af33.setContent(html_69f270d2b1bdc8d4028903b27bc996f8);\\n \\n \\n\\n circle_marker_75211e0874e409ff45c62f61cdff51df.bindPopup(popup_76fd2f1a889b81f355657b5fd2b4af33)\\n ;\\n\\n \\n \\n \\n var circle_marker_6570627cf21e640b8b49e4bf871f9b69 = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.820437914901168, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_448d6bc5d1cc5d75a3da2b69c6df8f10 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8b1cb3bb71535429ef3ffc37ee6d16b = $(`<div id="html_a8b1cb3bb71535429ef3ffc37ee6d16b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_448d6bc5d1cc5d75a3da2b69c6df8f10.setContent(html_a8b1cb3bb71535429ef3ffc37ee6d16b);\\n \\n \\n\\n circle_marker_6570627cf21e640b8b49e4bf871f9b69.bindPopup(popup_448d6bc5d1cc5d75a3da2b69c6df8f10)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5d96494ffc87b09b9452743e75611c6 = L.circleMarker(\\n [42.72912939726166, -73.25380127326159],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.024103669400641, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8c3f62b912da86b5a327ec977a187812 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a4b4497829d4d1166567c615d049646 = $(`<div id="html_8a4b4497829d4d1166567c615d049646" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c3f62b912da86b5a327ec977a187812.setContent(html_8a4b4497829d4d1166567c615d049646);\\n \\n \\n\\n circle_marker_f5d96494ffc87b09b9452743e75611c6.bindPopup(popup_8c3f62b912da86b5a327ec977a187812)\\n ;\\n\\n \\n \\n \\n var circle_marker_62f07f95c4880de0a5056dbdb6f3fb55 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.694520051971082, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4feedcc7843a09a7827017e3e4670ff1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_582095e2497f3aabccc6de716d02a6f9 = $(`<div id="html_582095e2497f3aabccc6de716d02a6f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4feedcc7843a09a7827017e3e4670ff1.setContent(html_582095e2497f3aabccc6de716d02a6f9);\\n \\n \\n\\n circle_marker_62f07f95c4880de0a5056dbdb6f3fb55.bindPopup(popup_4feedcc7843a09a7827017e3e4670ff1)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a4f31c6fc2ec5ffbb86ffd51e5b6a0b = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.723094811029982, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_092e720c52b5f6b337f14bce202d75f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f19bedd79aa73c115d3356cc5031b9c6 = $(`<div id="html_f19bedd79aa73c115d3356cc5031b9c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_092e720c52b5f6b337f14bce202d75f5.setContent(html_f19bedd79aa73c115d3356cc5031b9c6);\\n \\n \\n\\n circle_marker_2a4f31c6fc2ec5ffbb86ffd51e5b6a0b.bindPopup(popup_092e720c52b5f6b337f14bce202d75f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a623cc6949138eed0489f5f3aa57740c = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.51907589177983, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d5cb02ca37677bffa18c5b1da250e684 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b57de06d649793a7a19282d7e1a59df = $(`<div id="html_9b57de06d649793a7a19282d7e1a59df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5cb02ca37677bffa18c5b1da250e684.setContent(html_9b57de06d649793a7a19282d7e1a59df);\\n \\n \\n\\n circle_marker_a623cc6949138eed0489f5f3aa57740c.bindPopup(popup_d5cb02ca37677bffa18c5b1da250e684)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b8285c8717b12482d84f84b2675c744 = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.54834217738561, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bcbef6d1e9749c2e18a07b419392d9af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed7def60ea2366ff905cc84082132ffc = $(`<div id="html_ed7def60ea2366ff905cc84082132ffc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bcbef6d1e9749c2e18a07b419392d9af.setContent(html_ed7def60ea2366ff905cc84082132ffc);\\n \\n \\n\\n circle_marker_3b8285c8717b12482d84f84b2675c744.bindPopup(popup_bcbef6d1e9749c2e18a07b419392d9af)\\n ;\\n\\n \\n \\n \\n var circle_marker_bc012483625ae2ef9bf83d8dbce9a6cb = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.917270272703197, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6708d5d4ac856fbc46e62f835d685b0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_acee956f704e6d5a7b7b0891d4e9d661 = $(`<div id="html_acee956f704e6d5a7b7b0891d4e9d661" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6708d5d4ac856fbc46e62f835d685b0d.setContent(html_acee956f704e6d5a7b7b0891d4e9d661);\\n \\n \\n\\n circle_marker_bc012483625ae2ef9bf83d8dbce9a6cb.bindPopup(popup_6708d5d4ac856fbc46e62f835d685b0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6fbc9e95e9e60f4ac01da60f2132a65 = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.917270272703197, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_baaf35bc3ecad0b2c86ea4b4b34bf34d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39af862b9782a5dd47dc9c478969dddd = $(`<div id="html_39af862b9782a5dd47dc9c478969dddd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_baaf35bc3ecad0b2c86ea4b4b34bf34d.setContent(html_39af862b9782a5dd47dc9c478969dddd);\\n \\n \\n\\n circle_marker_d6fbc9e95e9e60f4ac01da60f2132a65.bindPopup(popup_baaf35bc3ecad0b2c86ea4b4b34bf34d)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6d45f3f55aef0ea6cc04e31a7524fd5 = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.356025796273888, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f7e38483c17d1244fc85503b38c4594e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0ee7ad5c4a6d7410b3ff93f1735ae59 = $(`<div id="html_b0ee7ad5c4a6d7410b3ff93f1735ae59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7e38483c17d1244fc85503b38c4594e.setContent(html_b0ee7ad5c4a6d7410b3ff93f1735ae59);\\n \\n \\n\\n circle_marker_a6d45f3f55aef0ea6cc04e31a7524fd5.bindPopup(popup_f7e38483c17d1244fc85503b38c4594e)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb99407e21b8e3d10990abd8b99669e5 = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.333012368467128, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3d11b1ad90416a3c089b87d8d919222a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e6f9f58359ba12a99d66b9d2c6c4265 = $(`<div id="html_9e6f9f58359ba12a99d66b9d2c6c4265" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d11b1ad90416a3c089b87d8d919222a.setContent(html_9e6f9f58359ba12a99d66b9d2c6c4265);\\n \\n \\n\\n circle_marker_cb99407e21b8e3d10990abd8b99669e5.bindPopup(popup_3d11b1ad90416a3c089b87d8d919222a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8fcc5131bc2035069506e29a8c51f3ee = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.311031542587394, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9a6008dda8112618a0b29aeb7f400eec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f20e66a72f8805c1eed90896d9b7bfd = $(`<div id="html_5f20e66a72f8805c1eed90896d9b7bfd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a6008dda8112618a0b29aeb7f400eec.setContent(html_5f20e66a72f8805c1eed90896d9b7bfd);\\n \\n \\n\\n circle_marker_8fcc5131bc2035069506e29a8c51f3ee.bindPopup(popup_9a6008dda8112618a0b29aeb7f400eec)\\n ;\\n\\n \\n \\n \\n var circle_marker_e72c4736a8924bc577dc8ca9644869a7 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.091753098990329, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_ac0f854d17e05f3d9d61c54bdf5dd87c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8d914234167c9187ef17377c3fe2669 = $(`<div id="html_d8d914234167c9187ef17377c3fe2669" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac0f854d17e05f3d9d61c54bdf5dd87c.setContent(html_d8d914234167c9187ef17377c3fe2669);\\n \\n \\n\\n circle_marker_e72c4736a8924bc577dc8ca9644869a7.bindPopup(popup_ac0f854d17e05f3d9d61c54bdf5dd87c)\\n ;\\n\\n \\n \\n \\n var circle_marker_51fe72b2199b81527f3dc122e268bbcf = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.001408606295148, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d3b0fce63bee6bcac59a2fde7b244f4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_abbdaa36e256ab094ceaa29e0f7af945 = $(`<div id="html_abbdaa36e256ab094ceaa29e0f7af945" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3b0fce63bee6bcac59a2fde7b244f4b.setContent(html_abbdaa36e256ab094ceaa29e0f7af945);\\n \\n \\n\\n circle_marker_51fe72b2199b81527f3dc122e268bbcf.bindPopup(popup_d3b0fce63bee6bcac59a2fde7b244f4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_0814415b5a07905099b6db6f2b76006f = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.90988298942671, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2dab7b4098fb77b774599a5728387f0a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c3bb2c1f1a343d743a86cf7c4645d4c = $(`<div id="html_0c3bb2c1f1a343d743a86cf7c4645d4c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2dab7b4098fb77b774599a5728387f0a.setContent(html_0c3bb2c1f1a343d743a86cf7c4645d4c);\\n \\n \\n\\n circle_marker_0814415b5a07905099b6db6f2b76006f.bindPopup(popup_2dab7b4098fb77b774599a5728387f0a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e292ee6be12c92e03a5b08ba17f26a90 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.069275219628611, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_65af6deaace11c6f8bba21aa14d6c518 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f523dfeda614f746336735976b7971ff = $(`<div id="html_f523dfeda614f746336735976b7971ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65af6deaace11c6f8bba21aa14d6c518.setContent(html_f523dfeda614f746336735976b7971ff);\\n \\n \\n\\n circle_marker_e292ee6be12c92e03a5b08ba17f26a90.bindPopup(popup_65af6deaace11c6f8bba21aa14d6c518)\\n ;\\n\\n \\n \\n \\n var circle_marker_6135ace28740185e420085a15eb793c6 = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.044647077096718, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_29e55e0006c28bd644c5c695dc851a3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3786d679f7fd0dab33c36841b14a2c96 = $(`<div id="html_3786d679f7fd0dab33c36841b14a2c96" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_29e55e0006c28bd644c5c695dc851a3e.setContent(html_3786d679f7fd0dab33c36841b14a2c96);\\n \\n \\n\\n circle_marker_6135ace28740185e420085a15eb793c6.bindPopup(popup_29e55e0006c28bd644c5c695dc851a3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_155c87b6442f09c7f3304c70668a7f15 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_508ddf5075568b646e0689a80870d84e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb7288bb5baffa3aaa69a1763f4d11ee = $(`<div id="html_cb7288bb5baffa3aaa69a1763f4d11ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_508ddf5075568b646e0689a80870d84e.setContent(html_cb7288bb5baffa3aaa69a1763f4d11ee);\\n \\n \\n\\n circle_marker_155c87b6442f09c7f3304c70668a7f15.bindPopup(popup_508ddf5075568b646e0689a80870d84e)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b37fd157d461fee107cff3944527ae2 = L.circleMarker(\\n [42.7291258162676, -73.23412027429306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.6036697681803656, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_56495846821e2941e49ffd18bdda23e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bed963b5922d1102e38c11ff27178b12 = $(`<div id="html_bed963b5922d1102e38c11ff27178b12" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_56495846821e2941e49ffd18bdda23e1.setContent(html_bed963b5922d1102e38c11ff27178b12);\\n \\n \\n\\n circle_marker_1b37fd157d461fee107cff3944527ae2.bindPopup(popup_56495846821e2941e49ffd18bdda23e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd895c5c14b059dc00ec44a6131b0afd = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0a75348937ffb264046a304498d2fdb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69bdb6458bd4a56a06068cd718eb084a = $(`<div id="html_69bdb6458bd4a56a06068cd718eb084a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a75348937ffb264046a304498d2fdb2.setContent(html_69bdb6458bd4a56a06068cd718eb084a);\\n \\n \\n\\n circle_marker_dd895c5c14b059dc00ec44a6131b0afd.bindPopup(popup_0a75348937ffb264046a304498d2fdb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1be72d6e18388115717862239e8623c = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.890312181373172, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5ef5da7c33f0c6b527b30cb297311869 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_216767586f77ebdb52e446e4498221ce = $(`<div id="html_216767586f77ebdb52e446e4498221ce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ef5da7c33f0c6b527b30cb297311869.setContent(html_216767586f77ebdb52e446e4498221ce);\\n \\n \\n\\n circle_marker_c1be72d6e18388115717862239e8623c.bindPopup(popup_5ef5da7c33f0c6b527b30cb297311869)\\n ;\\n\\n \\n \\n \\n var circle_marker_25da8b0ff05ceb8c7efb6c01da98c920 = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_34e441463be120a1637599bb1f65daaa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae129f142533a3f777fcb8afc2846d32 = $(`<div id="html_ae129f142533a3f777fcb8afc2846d32" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_34e441463be120a1637599bb1f65daaa.setContent(html_ae129f142533a3f777fcb8afc2846d32);\\n \\n \\n\\n circle_marker_25da8b0ff05ceb8c7efb6c01da98c920.bindPopup(popup_34e441463be120a1637599bb1f65daaa)\\n ;\\n\\n \\n \\n \\n var circle_marker_6051f108dc267453418e9b7043a7ec3e = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_09083fe95636f1136c1b959dd5183927 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36026694bda146fdf02fea051f093d63 = $(`<div id="html_36026694bda146fdf02fea051f093d63" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09083fe95636f1136c1b959dd5183927.setContent(html_36026694bda146fdf02fea051f093d63);\\n \\n \\n\\n circle_marker_6051f108dc267453418e9b7043a7ec3e.bindPopup(popup_09083fe95636f1136c1b959dd5183927)\\n ;\\n\\n \\n \\n \\n var circle_marker_a32659bb2010b82812baa6d1ec704a60 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.370193722368317, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e5a19a49004499ec13ac6a3f1a10fc22 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f3ca39ce318c9f79025f63c54567a29 = $(`<div id="html_0f3ca39ce318c9f79025f63c54567a29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5a19a49004499ec13ac6a3f1a10fc22.setContent(html_0f3ca39ce318c9f79025f63c54567a29);\\n \\n \\n\\n circle_marker_a32659bb2010b82812baa6d1ec704a60.bindPopup(popup_e5a19a49004499ec13ac6a3f1a10fc22)\\n ;\\n\\n \\n \\n \\n var circle_marker_5943e20baeab5d60865df0fe69372e6d = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_66408027c0f1ee358fdbe4bc77a2396d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e69620af8acc7b8cd09a4ba68885faa0 = $(`<div id="html_e69620af8acc7b8cd09a4ba68885faa0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_66408027c0f1ee358fdbe4bc77a2396d.setContent(html_e69620af8acc7b8cd09a4ba68885faa0);\\n \\n \\n\\n circle_marker_5943e20baeab5d60865df0fe69372e6d.bindPopup(popup_66408027c0f1ee358fdbe4bc77a2396d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c52ab66aa76023130aa52a4efba546b8 = L.circleMarker(\\n [42.729122320849, -73.22304971370495],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7d6d125b00ec32d5f90324f4f8205e81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f796d5c209f6abd332c26017807b3c97 = $(`<div id="html_f796d5c209f6abd332c26017807b3c97" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d6d125b00ec32d5f90324f4f8205e81.setContent(html_f796d5c209f6abd332c26017807b3c97);\\n \\n \\n\\n circle_marker_c52ab66aa76023130aa52a4efba546b8.bindPopup(popup_7d6d125b00ec32d5f90324f4f8205e81)\\n ;\\n\\n \\n \\n \\n var circle_marker_bddceb54dd658a1e57005da07a838053 = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_046adba57c515885f165f16d45559983 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1b45fd71d092c4108a7e71ba4605b7f = $(`<div id="html_b1b45fd71d092c4108a7e71ba4605b7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_046adba57c515885f165f16d45559983.setContent(html_b1b45fd71d092c4108a7e71ba4605b7f);\\n \\n \\n\\n circle_marker_bddceb54dd658a1e57005da07a838053.bindPopup(popup_046adba57c515885f165f16d45559983)\\n ;\\n\\n \\n \\n \\n var circle_marker_bbec938881f4448a579d72fb302efc26 = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_526d6977ea505784957386e7df557c5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14c26225eba393a65302c01ce0fb5459 = $(`<div id="html_14c26225eba393a65302c01ce0fb5459" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_526d6977ea505784957386e7df557c5e.setContent(html_14c26225eba393a65302c01ce0fb5459);\\n \\n \\n\\n circle_marker_bbec938881f4448a579d72fb302efc26.bindPopup(popup_526d6977ea505784957386e7df557c5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f99d9aa40c1226c70fa1d45ac1e2e10 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_79cef5f1c36de5c969fb5dcbbde9d4c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd594a5bf65c5ea6b42eda699fae34f8 = $(`<div id="html_fd594a5bf65c5ea6b42eda699fae34f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79cef5f1c36de5c969fb5dcbbde9d4c5.setContent(html_fd594a5bf65c5ea6b42eda699fae34f8);\\n \\n \\n\\n circle_marker_5f99d9aa40c1226c70fa1d45ac1e2e10.bindPopup(popup_79cef5f1c36de5c969fb5dcbbde9d4c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d1801beb1a69f6a17ef807b61614010 = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c3c64c97e07b758c0224a4ac0af1cec1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ed51567681ab483a09b6abf42032ff0 = $(`<div id="html_4ed51567681ab483a09b6abf42032ff0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3c64c97e07b758c0224a4ac0af1cec1.setContent(html_4ed51567681ab483a09b6abf42032ff0);\\n \\n \\n\\n circle_marker_5d1801beb1a69f6a17ef807b61614010.bindPopup(popup_c3c64c97e07b758c0224a4ac0af1cec1)\\n ;\\n\\n \\n \\n \\n var circle_marker_f9f1a19acbe80d419a004db5e50f2a97 = L.circleMarker(\\n [42.72732247454739, -73.27348202255521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.722159296752494, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6d9df4ebd3b978375857b5541062dd3b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bba27053eedce4e032a6b25cced12194 = $(`<div id="html_bba27053eedce4e032a6b25cced12194" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d9df4ebd3b978375857b5541062dd3b.setContent(html_bba27053eedce4e032a6b25cced12194);\\n \\n \\n\\n circle_marker_f9f1a19acbe80d419a004db5e50f2a97.bindPopup(popup_6d9df4ebd3b978375857b5541062dd3b)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d530bd6d8e36fc99be5eaf0a24b6fdc = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.389986074159536, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e6531587c32e13d915f476d39b4ef78e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd4f6d319b2b16d7846e68b406b74ab2 = $(`<div id="html_dd4f6d319b2b16d7846e68b406b74ab2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6531587c32e13d915f476d39b4ef78e.setContent(html_dd4f6d319b2b16d7846e68b406b74ab2);\\n \\n \\n\\n circle_marker_7d530bd6d8e36fc99be5eaf0a24b6fdc.bindPopup(popup_e6531587c32e13d915f476d39b4ef78e)\\n ;\\n\\n \\n \\n \\n var circle_marker_56d39694c2406918764e4537e99fedca = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.837972188868215, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bde15a912042c9145fc54a17c20bafc7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42c0fbbb1f0c4490d7d82e7d0cf3b6f9 = $(`<div id="html_42c0fbbb1f0c4490d7d82e7d0cf3b6f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bde15a912042c9145fc54a17c20bafc7.setContent(html_42c0fbbb1f0c4490d7d82e7d0cf3b6f9);\\n \\n \\n\\n circle_marker_56d39694c2406918764e4537e99fedca.bindPopup(popup_bde15a912042c9145fc54a17c20bafc7)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f169e7f2e23a3f7978790256136e3c0 = L.circleMarker(\\n [42.72732269176802, -73.26979194254899],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 10.645107772184812, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_99cb01c5c4c5eba9b3ecb07b3dd268c3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00a551bd6d3859b4dc884d072d093575 = $(`<div id="html_00a551bd6d3859b4dc884d072d093575" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99cb01c5c4c5eba9b3ecb07b3dd268c3.setContent(html_00a551bd6d3859b4dc884d072d093575);\\n \\n \\n\\n circle_marker_3f169e7f2e23a3f7978790256136e3c0.bindPopup(popup_99cb01c5c4c5eba9b3ecb07b3dd268c3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d29fe4119016a84167a7685f6dace8da = L.circleMarker(\\n [42.727322737845135, -73.26856191587537],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.442171739215616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_80b4479acd4dbe45dc709d035d24082b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a05921cecb0887faf092643bd84e7f5 = $(`<div id="html_4a05921cecb0887faf092643bd84e7f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80b4479acd4dbe45dc709d035d24082b.setContent(html_4a05921cecb0887faf092643bd84e7f5);\\n \\n \\n\\n circle_marker_d29fe4119016a84167a7685f6dace8da.bindPopup(popup_80b4479acd4dbe45dc709d035d24082b)\\n ;\\n\\n \\n \\n \\n var circle_marker_fdbd17a23d748ce1d731b188d3ff291e = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.735777827895049, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_99cc198803603cde51212a300643d318 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dca8d608486449d6e5034465a50902dc = $(`<div id="html_dca8d608486449d6e5034465a50902dc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99cc198803603cde51212a300643d318.setContent(html_dca8d608486449d6e5034465a50902dc);\\n \\n \\n\\n circle_marker_fdbd17a23d748ce1d731b188d3ff291e.bindPopup(popup_99cc198803603cde51212a300643d318)\\n ;\\n\\n \\n \\n \\n var circle_marker_1bd484f179fa0ea2c0d60666ef64acda = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.45754080179648, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d0b7f7ec875042ac2e15ed886729371e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f4deb7726c72e7f3cd946054bfc3c60 = $(`<div id="html_8f4deb7726c72e7f3cd946054bfc3c60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0b7f7ec875042ac2e15ed886729371e.setContent(html_8f4deb7726c72e7f3cd946054bfc3c60);\\n \\n \\n\\n circle_marker_1bd484f179fa0ea2c0d60666ef64acda.bindPopup(popup_d0b7f7ec875042ac2e15ed886729371e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b9faf0b46689215161b9c9f463a2c97b = L.circleMarker(\\n [42.727322797087126, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.837972188868215, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a7034ec3ecb7611f5bb10408d64de6dc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab01b0be7cc4ed645758c4978d98cdfe = $(`<div id="html_ab01b0be7cc4ed645758c4978d98cdfe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7034ec3ecb7611f5bb10408d64de6dc.setContent(html_ab01b0be7cc4ed645758c4978d98cdfe);\\n \\n \\n\\n circle_marker_b9faf0b46689215161b9c9f463a2c97b.bindPopup(popup_a7034ec3ecb7611f5bb10408d64de6dc)\\n ;\\n\\n \\n \\n \\n var circle_marker_0568706e1600fffe186d053da5e06c80 = L.circleMarker(\\n [42.72732279050467, -73.26364180917045],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.6036697681803656, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_614f57dc776c0d1a466161c4ef4644e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4bfc4ae8bf4d230fd3460ae74818c1cc = $(`<div id="html_4bfc4ae8bf4d230fd3460ae74818c1cc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_614f57dc776c0d1a466161c4ef4644e5.setContent(html_4bfc4ae8bf4d230fd3460ae74818c1cc);\\n \\n \\n\\n circle_marker_0568706e1600fffe186d053da5e06c80.bindPopup(popup_614f57dc776c0d1a466161c4ef4644e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d426c47be7c40d9a1a42553b7175590 = L.circleMarker(\\n [42.72732277075734, -73.26241178249424],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.377736139048903, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_84d7c45b59a97fabd996caaea63fc206 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c3e41e76b6d229097f8077d3a3ba912 = $(`<div id="html_7c3e41e76b6d229097f8077d3a3ba912" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84d7c45b59a97fabd996caaea63fc206.setContent(html_7c3e41e76b6d229097f8077d3a3ba912);\\n \\n \\n\\n circle_marker_1d426c47be7c40d9a1a42553b7175590.bindPopup(popup_84d7c45b59a97fabd996caaea63fc206)\\n ;\\n\\n \\n \\n \\n var circle_marker_e5d0f9529a652f7b33151e0cb2d9cd21 = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.432750982580687, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4e6357175424f30ae8932706a896dad8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_45c8bd299459d5c75c67d89c180cbf5a = $(`<div id="html_45c8bd299459d5c75c67d89c180cbf5a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e6357175424f30ae8932706a896dad8.setContent(html_45c8bd299459d5c75c67d89c180cbf5a);\\n \\n \\n\\n circle_marker_e5d0f9529a652f7b33151e0cb2d9cd21.bindPopup(popup_4e6357175424f30ae8932706a896dad8)\\n ;\\n\\n \\n \\n \\n var circle_marker_30360793058ec61e4e70f1c0454a3a72 = L.circleMarker(\\n [42.72732269176802, -73.25995172914543],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.33015937350939, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_15e0d0912281fc92a6df0527be337056 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea8f01e3313a5d0298b668e451657125 = $(`<div id="html_ea8f01e3313a5d0298b668e451657125" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_15e0d0912281fc92a6df0527be337056.setContent(html_ea8f01e3313a5d0298b668e451657125);\\n \\n \\n\\n circle_marker_30360793058ec61e4e70f1c0454a3a72.bindPopup(popup_15e0d0912281fc92a6df0527be337056)\\n ;\\n\\n \\n \\n \\n var circle_marker_19b96334af903c1703186a33f15a5c70 = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.001408606295148, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_f5c8f3ba853358e38e4e30b969dba231 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb7f69789d4dd11def2278716004de3b = $(`<div id="html_cb7f69789d4dd11def2278716004de3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5c8f3ba853358e38e4e30b969dba231.setContent(html_cb7f69789d4dd11def2278716004de3b);\\n \\n \\n\\n circle_marker_19b96334af903c1703186a33f15a5c70.bindPopup(popup_f5c8f3ba853358e38e4e30b969dba231)\\n ;\\n\\n \\n \\n \\n var circle_marker_4ddbf84680a42f7855076742eccbbf23 = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.136496464611085, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a83be187668c8a1f566d003103f4f4a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_18ce9271fc358da98f0b0905d71e9cbf = $(`<div id="html_18ce9271fc358da98f0b0905d71e9cbf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a83be187668c8a1f566d003103f4f4a9.setContent(html_18ce9271fc358da98f0b0905d71e9cbf);\\n \\n \\n\\n circle_marker_4ddbf84680a42f7855076742eccbbf23.bindPopup(popup_a83be187668c8a1f566d003103f4f4a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cd77c20f30c84c826d5c457597eee60 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.463526651802308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_82a4e2bcc4c5f6c854b511e4a53d22c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb5e353b33c85d7cca502b10da9a368d = $(`<div id="html_fb5e353b33c85d7cca502b10da9a368d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82a4e2bcc4c5f6c854b511e4a53d22c6.setContent(html_fb5e353b33c85d7cca502b10da9a368d);\\n \\n \\n\\n circle_marker_3cd77c20f30c84c826d5c457597eee60.bindPopup(popup_82a4e2bcc4c5f6c854b511e4a53d22c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c5fdc74a11d18af49db1a0acb4e0370 = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_cc2351c7a14186fa65cf149261a000d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb4ca288764e14c1e8e7d27643f7400e = $(`<div id="html_eb4ca288764e14c1e8e7d27643f7400e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc2351c7a14186fa65cf149261a000d5.setContent(html_eb4ca288764e14c1e8e7d27643f7400e);\\n \\n \\n\\n circle_marker_7c5fdc74a11d18af49db1a0acb4e0370.bindPopup(popup_cc2351c7a14186fa65cf149261a000d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fcb1065686d843016c26b4287207371 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.753627391751592, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_bc99381c84d3d78b367c51fab38f11d6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ffe2076fd3c82cc32046426fc14038df = $(`<div id="html_ffe2076fd3c82cc32046426fc14038df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc99381c84d3d78b367c51fab38f11d6.setContent(html_ffe2076fd3c82cc32046426fc14038df);\\n \\n \\n\\n circle_marker_3fcb1065686d843016c26b4287207371.bindPopup(popup_bc99381c84d3d78b367c51fab38f11d6)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0d0381f297f9b3032c3d2adaf0fabfd = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.613615477764332, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_5f3c374199490fb6f85ada5fb6e5b1b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0fd0a37f885624956d26efccbfbb8cfc = $(`<div id="html_0fd0a37f885624956d26efccbfbb8cfc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f3c374199490fb6f85ada5fb6e5b1b2.setContent(html_0fd0a37f885624956d26efccbfbb8cfc);\\n \\n \\n\\n circle_marker_b0d0381f297f9b3032c3d2adaf0fabfd.bindPopup(popup_5f3c374199490fb6f85ada5fb6e5b1b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b8d140f47e6a3c289a78baa9255f090 = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e472e7329a14085c67b1ecb9e4fe7c25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2df914b1f38da9ed3402bb72265cb543 = $(`<div id="html_2df914b1f38da9ed3402bb72265cb543" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e472e7329a14085c67b1ecb9e4fe7c25.setContent(html_2df914b1f38da9ed3402bb72265cb543);\\n \\n \\n\\n circle_marker_3b8d140f47e6a3c289a78baa9255f090.bindPopup(popup_e472e7329a14085c67b1ecb9e4fe7c25)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7c9ec166400d9d7a3b0c46d6d01ae79 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.590394548326596, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7ed96410c761947249459c0a9566d6cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_396c417a15f7983ecce916c420fa1fd8 = $(`<div id="html_396c417a15f7983ecce916c420fa1fd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7ed96410c761947249459c0a9566d6cc.setContent(html_396c417a15f7983ecce916c420fa1fd8);\\n \\n \\n\\n circle_marker_a7c9ec166400d9d7a3b0c46d6d01ae79.bindPopup(popup_7ed96410c761947249459c0a9566d6cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0318ab0e55dff17b7d61c213ac0b8de = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.253382073025046, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_abe014be09106a71f5b07fb6ea823f08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_218e94975f7291e3070606a7bc46d4c0 = $(`<div id="html_218e94975f7291e3070606a7bc46d4c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_abe014be09106a71f5b07fb6ea823f08.setContent(html_218e94975f7291e3070606a7bc46d4c0);\\n \\n \\n\\n circle_marker_b0318ab0e55dff17b7d61c213ac0b8de.bindPopup(popup_abe014be09106a71f5b07fb6ea823f08)\\n ;\\n\\n \\n \\n \\n var circle_marker_78ee6bcc8e80fba643ef28905d665c91 = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.333012368467128, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c3f8c62dba27d8ce91e696eda05a34ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a727bb00b2600772d512384fb17e75d9 = $(`<div id="html_a727bb00b2600772d512384fb17e75d9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3f8c62dba27d8ce91e696eda05a34ea.setContent(html_a727bb00b2600772d512384fb17e75d9);\\n \\n \\n\\n circle_marker_78ee6bcc8e80fba643ef28905d665c91.bindPopup(popup_c3f8c62dba27d8ce91e696eda05a34ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_5cefd28bc26b470404f78562de30bf8b = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9de00754bcbeb24cdc5eb10992cb1bd7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70b748c574dae3272b5503528d31610e = $(`<div id="html_70b748c574dae3272b5503528d31610e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9de00754bcbeb24cdc5eb10992cb1bd7.setContent(html_70b748c574dae3272b5503528d31610e);\\n \\n \\n\\n circle_marker_5cefd28bc26b470404f78562de30bf8b.bindPopup(popup_9de00754bcbeb24cdc5eb10992cb1bd7)\\n ;\\n\\n \\n \\n \\n var circle_marker_0fa12ae0f93160bcc471e4e542d50865 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_00c262282b7a0006b825739a9864511f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b990494b1c86a2eac16163af36fa2048 = $(`<div id="html_b990494b1c86a2eac16163af36fa2048" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00c262282b7a0006b825739a9864511f.setContent(html_b990494b1c86a2eac16163af36fa2048);\\n \\n \\n\\n circle_marker_0fa12ae0f93160bcc471e4e542d50865.bindPopup(popup_00c262282b7a0006b825739a9864511f)\\n ;\\n\\n \\n \\n \\n var circle_marker_94b2003f6b0a518c172da08ec00e8246 = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.506054213402069, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c48fffa38e0ef3e8ee11b77297c6bbc3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec955e9597dc305632bc8d39410f7dd9 = $(`<div id="html_ec955e9597dc305632bc8d39410f7dd9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c48fffa38e0ef3e8ee11b77297c6bbc3.setContent(html_ec955e9597dc305632bc8d39410f7dd9);\\n \\n \\n\\n circle_marker_94b2003f6b0a518c172da08ec00e8246.bindPopup(popup_c48fffa38e0ef3e8ee11b77297c6bbc3)\\n ;\\n\\n \\n \\n \\n var circle_marker_99d45f0111405acdecbae8a40087e9c1 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c2a5a9da86ed31a7f8904eed245f7038 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_567f60dba934a21f73f2fe0ed56ee00e = $(`<div id="html_567f60dba934a21f73f2fe0ed56ee00e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2a5a9da86ed31a7f8904eed245f7038.setContent(html_567f60dba934a21f73f2fe0ed56ee00e);\\n \\n \\n\\n circle_marker_99d45f0111405acdecbae8a40087e9c1.bindPopup(popup_c2a5a9da86ed31a7f8904eed245f7038)\\n ;\\n\\n \\n \\n \\n var circle_marker_22c1569d991ebef0ab49f9d70a373aab = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_92ef0236328efbfc981577ce43bc2af5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f948b5243fbe13aae77fe55671db071b = $(`<div id="html_f948b5243fbe13aae77fe55671db071b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_92ef0236328efbfc981577ce43bc2af5.setContent(html_f948b5243fbe13aae77fe55671db071b);\\n \\n \\n\\n circle_marker_22c1569d991ebef0ab49f9d70a373aab.bindPopup(popup_92ef0236328efbfc981577ce43bc2af5)\\n ;\\n\\n \\n \\n \\n var circle_marker_86536a5f6bea5f4ab64503cb970377ed = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_3a526f1c70fb9e3f4789b43fa0ca2de2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56445bbcf9a8b7786d99fcca30079298 = $(`<div id="html_56445bbcf9a8b7786d99fcca30079298" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a526f1c70fb9e3f4789b43fa0ca2de2.setContent(html_56445bbcf9a8b7786d99fcca30079298);\\n \\n \\n\\n circle_marker_86536a5f6bea5f4ab64503cb970377ed.bindPopup(popup_3a526f1c70fb9e3f4789b43fa0ca2de2)\\n ;\\n\\n \\n \\n \\n var circle_marker_45df6050601a0ee758b67224e16cfa51 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.898654169668588, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_41a2b4ea2f77a66a93a86edc397f5a5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9be92018ccd48d4fee6ac003cd32cf8 = $(`<div id="html_f9be92018ccd48d4fee6ac003cd32cf8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41a2b4ea2f77a66a93a86edc397f5a5a.setContent(html_f9be92018ccd48d4fee6ac003cd32cf8);\\n \\n \\n\\n circle_marker_45df6050601a0ee758b67224e16cfa51.bindPopup(popup_41a2b4ea2f77a66a93a86edc397f5a5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c028b29176325d5339dd7ffbd7d085d = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.044647077096718, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_d96974e7283d3a2eaad60264782a7ce9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc27f445f9eabedd13991399b24c1265 = $(`<div id="html_cc27f445f9eabedd13991399b24c1265" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d96974e7283d3a2eaad60264782a7ce9.setContent(html_cc27f445f9eabedd13991399b24c1265);\\n \\n \\n\\n circle_marker_7c028b29176325d5339dd7ffbd7d085d.bindPopup(popup_d96974e7283d3a2eaad60264782a7ce9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9cb4ef51d73da3bcbe7ae9c640361cea = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.797255174810172, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c89a76df2a7dea3cea27c61a1943f3f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b774285c1ed3a8b3ebe8c60b732216d1 = $(`<div id="html_b774285c1ed3a8b3ebe8c60b732216d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c89a76df2a7dea3cea27c61a1943f3f0.setContent(html_b774285c1ed3a8b3ebe8c60b732216d1);\\n \\n \\n\\n circle_marker_9cb4ef51d73da3bcbe7ae9c640361cea.bindPopup(popup_c89a76df2a7dea3cea27c61a1943f3f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_368b1a64ef393efab5953dc9f5d8f3d3 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.33015937350939, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b10971ccafc9f90e9d136b270b406467 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5fd96047cc4a48be5d44794a4f550cdc = $(`<div id="html_5fd96047cc4a48be5d44794a4f550cdc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b10971ccafc9f90e9d136b270b406467.setContent(html_5fd96047cc4a48be5d44794a4f550cdc);\\n \\n \\n\\n circle_marker_368b1a64ef393efab5953dc9f5d8f3d3.bindPopup(popup_b10971ccafc9f90e9d136b270b406467)\\n ;\\n\\n \\n \\n \\n var circle_marker_42f701c1379e0bafddc0716b7705b1d7 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4115163798060095, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fa313c0082e581987ab27405c20c96f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2fca43325fb1ec7346bb2f538ba05e6 = $(`<div id="html_e2fca43325fb1ec7346bb2f538ba05e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa313c0082e581987ab27405c20c96f8.setContent(html_e2fca43325fb1ec7346bb2f538ba05e6);\\n \\n \\n\\n circle_marker_42f701c1379e0bafddc0716b7705b1d7.bindPopup(popup_fa313c0082e581987ab27405c20c96f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_38660faa6f1a058c28b463bd6fd9c993 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9f3229b3cf0836ff8a2a79e6b6fa4620 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5eb8b15d1bd1325b3d75e721f415cfe1 = $(`<div id="html_5eb8b15d1bd1325b3d75e721f415cfe1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f3229b3cf0836ff8a2a79e6b6fa4620.setContent(html_5eb8b15d1bd1325b3d75e721f415cfe1);\\n \\n \\n\\n circle_marker_38660faa6f1a058c28b463bd6fd9c993.bindPopup(popup_9f3229b3cf0836ff8a2a79e6b6fa4620)\\n ;\\n\\n \\n \\n \\n var circle_marker_866ee0479df16d04ed22f9c78f8ecf72 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2fcaa62102a1fd2c15c0258e36af428c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3809cf11a2da45d6ff84fffb9c78d76c = $(`<div id="html_3809cf11a2da45d6ff84fffb9c78d76c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fcaa62102a1fd2c15c0258e36af428c.setContent(html_3809cf11a2da45d6ff84fffb9c78d76c);\\n \\n \\n\\n circle_marker_866ee0479df16d04ed22f9c78f8ecf72.bindPopup(popup_2fcaa62102a1fd2c15c0258e36af428c)\\n ;\\n\\n \\n \\n \\n var circle_marker_0fa71b2ad0d5736366e4d7d18cce785a = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9fe84c6dad709954d9a5848fa9cd642c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6be8d3d81d055152ce77015562cedc4 = $(`<div id="html_b6be8d3d81d055152ce77015562cedc4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fe84c6dad709954d9a5848fa9cd642c.setContent(html_b6be8d3d81d055152ce77015562cedc4);\\n \\n \\n\\n circle_marker_0fa71b2ad0d5736366e4d7d18cce785a.bindPopup(popup_9fe84c6dad709954d9a5848fa9cd642c)\\n ;\\n\\n \\n \\n \\n var circle_marker_c19acdc650557032a1038c9c23aaa73e = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7d91c1911bd7f85d5e312e3c7917390b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d7c779f59e3f3e1ca0d1199ef1f73a28 = $(`<div id="html_d7c779f59e3f3e1ca0d1199ef1f73a28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d91c1911bd7f85d5e312e3c7917390b.setContent(html_d7c779f59e3f3e1ca0d1199ef1f73a28);\\n \\n \\n\\n circle_marker_c19acdc650557032a1038c9c23aaa73e.bindPopup(popup_7d91c1911bd7f85d5e312e3c7917390b)\\n ;\\n\\n \\n \\n \\n var circle_marker_d30031826778f2cd22e4d94d5ba381d2 = L.circleMarker(\\n [42.72731562880686, -73.22428095863906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fc58a81ffc62fbbb704f871a52099192 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_90e3720e342f4e8ae62679b998778e7e = $(`<div id="html_90e3720e342f4e8ae62679b998778e7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc58a81ffc62fbbb704f871a52099192.setContent(html_90e3720e342f4e8ae62679b998778e7e);\\n \\n \\n\\n circle_marker_d30031826778f2cd22e4d94d5ba381d2.bindPopup(popup_fc58a81ffc62fbbb704f871a52099192)\\n ;\\n\\n \\n \\n \\n var circle_marker_f891cf8a46ae5581ed7420f62d71bdd7 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_031197dbe9a8da06de8e7c292332ee3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d0ed8da821ca89c04203664e8c839aa2 = $(`<div id="html_d0ed8da821ca89c04203664e8c839aa2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_031197dbe9a8da06de8e7c292332ee3f.setContent(html_d0ed8da821ca89c04203664e8c839aa2);\\n \\n \\n\\n circle_marker_f891cf8a46ae5581ed7420f62d71bdd7.bindPopup(popup_031197dbe9a8da06de8e7c292332ee3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d094a83ab137973ba33a6fc0cd609fd = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_03afa7ea04ab807903c52e7baed0de29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e7fe7932a307bcdb1982b20f23cd4bd = $(`<div id="html_6e7fe7932a307bcdb1982b20f23cd4bd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03afa7ea04ab807903c52e7baed0de29.setContent(html_6e7fe7932a307bcdb1982b20f23cd4bd);\\n \\n \\n\\n circle_marker_0d094a83ab137973ba33a6fc0cd609fd.bindPopup(popup_03afa7ea04ab807903c52e7baed0de29)\\n ;\\n\\n \\n \\n \\n var circle_marker_af0ec9871952fda7873d5978e2381c80 = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.812923024107548, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_31ce06e97347ccffdf5416dcf7c552aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_540526b5619e1ebe62ede4e2caadc369 = $(`<div id="html_540526b5619e1ebe62ede4e2caadc369" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31ce06e97347ccffdf5416dcf7c552aa.setContent(html_540526b5619e1ebe62ede4e2caadc369);\\n \\n \\n\\n circle_marker_af0ec9871952fda7873d5978e2381c80.bindPopup(popup_31ce06e97347ccffdf5416dcf7c552aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a3c1599ea652dc0d564943415ec1574 = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.640875829802336, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_42b7e91a990a3dde6f30012bf6795721 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3cdb8f54f10714e09d2baa6b5acfd4ba = $(`<div id="html_3cdb8f54f10714e09d2baa6b5acfd4ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_42b7e91a990a3dde6f30012bf6795721.setContent(html_3cdb8f54f10714e09d2baa6b5acfd4ba);\\n \\n \\n\\n circle_marker_0a3c1599ea652dc0d564943415ec1574.bindPopup(popup_42b7e91a990a3dde6f30012bf6795721)\\n ;\\n\\n \\n \\n \\n var circle_marker_6bb638465a247526a1c9c708d15a991a = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_4d04d718133be5d26000df7c91d3e600 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e3a6baab94fce323dcfa902e3c87311 = $(`<div id="html_6e3a6baab94fce323dcfa902e3c87311" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4d04d718133be5d26000df7c91d3e600.setContent(html_6e3a6baab94fce323dcfa902e3c87311);\\n \\n \\n\\n circle_marker_6bb638465a247526a1c9c708d15a991a.bindPopup(popup_4d04d718133be5d26000df7c91d3e600)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a1e4e4ab6e48a6d94399b67db480627 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.13220620552083, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_6ec03efd71a88a72a1da95ed862407a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ac84cc647bb2093c5a236740e4d86ec = $(`<div id="html_5ac84cc647bb2093c5a236740e4d86ec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ec03efd71a88a72a1da95ed862407a0.setContent(html_5ac84cc647bb2093c5a236740e4d86ec);\\n \\n \\n\\n circle_marker_7a1e4e4ab6e48a6d94399b67db480627.bindPopup(popup_6ec03efd71a88a72a1da95ed862407a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_ada9fe8ff9b32602c8ec39e6e4b9210d = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.652426491681278, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e301ab65700c97520afaa3a6effc1221 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b84b0a8c666a5b83b1b7f0139819272 = $(`<div id="html_0b84b0a8c666a5b83b1b7f0139819272" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e301ab65700c97520afaa3a6effc1221.setContent(html_0b84b0a8c666a5b83b1b7f0139819272);\\n \\n \\n\\n circle_marker_ada9fe8ff9b32602c8ec39e6e4b9210d.bindPopup(popup_e301ab65700c97520afaa3a6effc1221)\\n ;\\n\\n \\n \\n \\n var circle_marker_f66f758d191fe2069c3c369c95321876 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.83698151873187, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_22eed7a2da633298dfb896d8f7562001 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9052934d48fe7ee9cd9c46e4a1e70464 = $(`<div id="html_9052934d48fe7ee9cd9c46e4a1e70464" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22eed7a2da633298dfb896d8f7562001.setContent(html_9052934d48fe7ee9cd9c46e4a1e70464);\\n \\n \\n\\n circle_marker_f66f758d191fe2069c3c369c95321876.bindPopup(popup_22eed7a2da633298dfb896d8f7562001)\\n ;\\n\\n \\n \\n \\n var circle_marker_161362d559b58aa374df5dc0d730f984 = L.circleMarker(\\n [42.725515657130885, -73.26610182668713],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.735777827895049, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c0b4d29b998895572f7fc8638d8b8655 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d7e2c01c52c3bdc2c4885b5adef81e0f = $(`<div id="html_d7e2c01c52c3bdc2c4885b5adef81e0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0b4d29b998895572f7fc8638d8b8655.setContent(html_d7e2c01c52c3bdc2c4885b5adef81e0f);\\n \\n \\n\\n circle_marker_161362d559b58aa374df5dc0d730f984.bindPopup(popup_c0b4d29b998895572f7fc8638d8b8655)\\n ;\\n\\n \\n \\n \\n var circle_marker_300f08750153817a1f9a3cd8731324b7 = L.circleMarker(\\n [42.72551566371306, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.352372348458314, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e290f462fb728548c8be85a349e1b1b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5592a5269822c537e6f16e3c8feb4984 = $(`<div id="html_5592a5269822c537e6f16e3c8feb4984" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e290f462fb728548c8be85a349e1b1b7.setContent(html_5592a5269822c537e6f16e3c8feb4984);\\n \\n \\n\\n circle_marker_300f08750153817a1f9a3cd8731324b7.bindPopup(popup_e290f462fb728548c8be85a349e1b1b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_7955f371ead43505522a2ebb5ab9e893 = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.440847306724618, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_969bcec9388d7b67a17289576241bcc5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_704d8d7c591aa8675acb2f6398142848 = $(`<div id="html_704d8d7c591aa8675acb2f6398142848" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_969bcec9388d7b67a17289576241bcc5.setContent(html_704d8d7c591aa8675acb2f6398142848);\\n \\n \\n\\n circle_marker_7955f371ead43505522a2ebb5ab9e893.bindPopup(popup_969bcec9388d7b67a17289576241bcc5)\\n ;\\n\\n \\n \\n \\n var circle_marker_6fbe8396bb5388915c37e1022bd0279c = L.circleMarker(\\n [42.72551563738435, -73.26241185416787],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.399277020331919, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_16531e3a4cdfcf21ce8c7f4d7e30a068 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f01edf998ab76aa99eb85cd1aafb1b28 = $(`<div id="html_f01edf998ab76aa99eb85cd1aafb1b28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16531e3a4cdfcf21ce8c7f4d7e30a068.setContent(html_f01edf998ab76aa99eb85cd1aafb1b28);\\n \\n \\n\\n circle_marker_6fbe8396bb5388915c37e1022bd0279c.bindPopup(popup_16531e3a4cdfcf21ce8c7f4d7e30a068)\\n ;\\n\\n \\n \\n \\n var circle_marker_b39257cea3ba16bd32ac26e8519a69f9 = L.circleMarker(\\n [42.72551560447347, -73.26118186332953],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.046265044040321, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_69b91683a5df9fe74b83b8a518e0662c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2afc3213b234f327e1b9bc82e7a454cd = $(`<div id="html_2afc3213b234f327e1b9bc82e7a454cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69b91683a5df9fe74b83b8a518e0662c.setContent(html_2afc3213b234f327e1b9bc82e7a454cd);\\n \\n \\n\\n circle_marker_b39257cea3ba16bd32ac26e8519a69f9.bindPopup(popup_69b91683a5df9fe74b83b8a518e0662c)\\n ;\\n\\n \\n \\n \\n var circle_marker_98c8d6df34847f6a233440a7aab2118b = L.circleMarker(\\n [42.72551555839823, -73.25995187249272],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.050259243299904, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c2e1007bce0c7903498a8532c528b5b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70d86dbc412c86b8ba5a34c3cafc3dce = $(`<div id="html_70d86dbc412c86b8ba5a34c3cafc3dce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2e1007bce0c7903498a8532c528b5b4.setContent(html_70d86dbc412c86b8ba5a34c3cafc3dce);\\n \\n \\n\\n circle_marker_98c8d6df34847f6a233440a7aab2118b.bindPopup(popup_c2e1007bce0c7903498a8532c528b5b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6757377727221f638bc9b0b33b64436 = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.406461512053774, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7929b48aae9efa8a737f590e40e97dd8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_921bda355f1ea4cffad0b7e8c6facbc1 = $(`<div id="html_921bda355f1ea4cffad0b7e8c6facbc1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7929b48aae9efa8a737f590e40e97dd8.setContent(html_921bda355f1ea4cffad0b7e8c6facbc1);\\n \\n \\n\\n circle_marker_b6757377727221f638bc9b0b33b64436.bindPopup(popup_7929b48aae9efa8a737f590e40e97dd8)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4b258e0c791a9800a746c8fd874cb92 = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.970821321441846, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b31cf69282a129291ffb11f5a27dc7e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1efdabe56a918875952bb9b83e370c3e = $(`<div id="html_1efdabe56a918875952bb9b83e370c3e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b31cf69282a129291ffb11f5a27dc7e9.setContent(html_1efdabe56a918875952bb9b83e370c3e);\\n \\n \\n\\n circle_marker_f4b258e0c791a9800a746c8fd874cb92.bindPopup(popup_b31cf69282a129291ffb11f5a27dc7e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_361e9dbbcc6dfd5124f260962fec1d98 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.853342309955121, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_23fcddd9b8612d11f3d7f9eef63bafd9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24d86a7527b3b4a73cf1bf34bdc86f84 = $(`<div id="html_24d86a7527b3b4a73cf1bf34bdc86f84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23fcddd9b8612d11f3d7f9eef63bafd9.setContent(html_24d86a7527b3b4a73cf1bf34bdc86f84);\\n \\n \\n\\n circle_marker_361e9dbbcc6dfd5124f260962fec1d98.bindPopup(popup_23fcddd9b8612d11f3d7f9eef63bafd9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b48f94916c1c69e9fc34a1d1fe29008 = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_c64d6ecdc59c4c16f3ea03afac6202a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b79cdec4ead1c06ec35d48f05d6c591 = $(`<div id="html_8b79cdec4ead1c06ec35d48f05d6c591" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c64d6ecdc59c4c16f3ea03afac6202a4.setContent(html_8b79cdec4ead1c06ec35d48f05d6c591);\\n \\n \\n\\n circle_marker_9b48f94916c1c69e9fc34a1d1fe29008.bindPopup(popup_c64d6ecdc59c4c16f3ea03afac6202a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_d7f8057792a0e2257ec892b84d513f54 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8d403091a120b67dddc079f4a6d1c116 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eea167336d4c9a8d507e3cc355493840 = $(`<div id="html_eea167336d4c9a8d507e3cc355493840" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d403091a120b67dddc079f4a6d1c116.setContent(html_eea167336d4c9a8d507e3cc355493840);\\n \\n \\n\\n circle_marker_d7f8057792a0e2257ec892b84d513f54.bindPopup(popup_8d403091a120b67dddc079f4a6d1c116)\\n ;\\n\\n \\n \\n \\n var circle_marker_74e86c3459ef132d1beee4b45aa16336 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_0478ffe310f8497c34940883cea23b42 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a733b4b7b62158c18abc89bf2755de5d = $(`<div id="html_a733b4b7b62158c18abc89bf2755de5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0478ffe310f8497c34940883cea23b42.setContent(html_a733b4b7b62158c18abc89bf2755de5d);\\n \\n \\n\\n circle_marker_74e86c3459ef132d1beee4b45aa16336.bindPopup(popup_0478ffe310f8497c34940883cea23b42)\\n ;\\n\\n \\n \\n \\n var circle_marker_f591e0ea8647b30b1633cdfdf760f649 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_885095eb2935b88b624b3d9de0379d94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e32ea2170ec5f82dec9d37999871bea3 = $(`<div id="html_e32ea2170ec5f82dec9d37999871bea3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_885095eb2935b88b624b3d9de0379d94.setContent(html_e32ea2170ec5f82dec9d37999871bea3);\\n \\n \\n\\n circle_marker_f591e0ea8647b30b1633cdfdf760f649.bindPopup(popup_885095eb2935b88b624b3d9de0379d94)\\n ;\\n\\n \\n \\n \\n var circle_marker_8624de3bdf3873d5f2473e01bdfba1c6 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a306e48de24ba50b1bb845fc5a5c7ed1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7432101c24a32681edcb3d383c311fea = $(`<div id="html_7432101c24a32681edcb3d383c311fea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a306e48de24ba50b1bb845fc5a5c7ed1.setContent(html_7432101c24a32681edcb3d383c311fea);\\n \\n \\n\\n circle_marker_8624de3bdf3873d5f2473e01bdfba1c6.bindPopup(popup_a306e48de24ba50b1bb845fc5a5c7ed1)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4a7bb945ec8a6beb047915de8ed947e = L.circleMarker(\\n [42.725513531087856, -73.24273200123443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2b55647d4848598cee7850e809b5af58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d364872203ca44bd3895aabef488b84b = $(`<div id="html_d364872203ca44bd3895aabef488b84b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b55647d4848598cee7850e809b5af58.setContent(html_d364872203ca44bd3895aabef488b84b);\\n \\n \\n\\n circle_marker_f4a7bb945ec8a6beb047915de8ed947e.bindPopup(popup_2b55647d4848598cee7850e809b5af58)\\n ;\\n\\n \\n \\n \\n var circle_marker_a06f822a3f600bf867ea89cf64ccf96b = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_7a25857d664641265db09d86c98e5c34 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46c0336d49093fe27f6b683225b1efd3 = $(`<div id="html_46c0336d49093fe27f6b683225b1efd3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a25857d664641265db09d86c98e5c34.setContent(html_46c0336d49093fe27f6b683225b1efd3);\\n \\n \\n\\n circle_marker_a06f822a3f600bf867ea89cf64ccf96b.bindPopup(popup_7a25857d664641265db09d86c98e5c34)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8663e76431ae497bbce04909f59cf92 = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_8d757aa75939d3870eb20740edb5f165 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c9097d4ce929f3f93979835b50b663d0 = $(`<div id="html_c9097d4ce929f3f93979835b50b663d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d757aa75939d3870eb20740edb5f165.setContent(html_c9097d4ce929f3f93979835b50b663d0);\\n \\n \\n\\n circle_marker_a8663e76431ae497bbce04909f59cf92.bindPopup(popup_8d757aa75939d3870eb20740edb5f165)\\n ;\\n\\n \\n \\n \\n var circle_marker_a800925310d76ca7c9a01aafb861d215 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_de449d1609308da25fc09908b78a4652 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cfb245626035520b60707998640b460c = $(`<div id="html_cfb245626035520b60707998640b460c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de449d1609308da25fc09908b78a4652.setContent(html_cfb245626035520b60707998640b460c);\\n \\n \\n\\n circle_marker_a800925310d76ca7c9a01aafb861d215.bindPopup(popup_de449d1609308da25fc09908b78a4652)\\n ;\\n\\n \\n \\n \\n var circle_marker_f137c9420f9d2f60a6c280127000ec46 = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_04a5ab7ed42fc89458e5aa98729ac0b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_334e4f9885c03ab1801d12e8a92b4578 = $(`<div id="html_334e4f9885c03ab1801d12e8a92b4578" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04a5ab7ed42fc89458e5aa98729ac0b6.setContent(html_334e4f9885c03ab1801d12e8a92b4578);\\n \\n \\n\\n circle_marker_f137c9420f9d2f60a6c280127000ec46.bindPopup(popup_04a5ab7ed42fc89458e5aa98729ac0b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d65bfe072e873597b6d0c382a60933d = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_aea3c501725fa3097e291dc600943640 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e86461b5c5e0c15516821932484eb12 = $(`<div id="html_7e86461b5c5e0c15516821932484eb12" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aea3c501725fa3097e291dc600943640.setContent(html_7e86461b5c5e0c15516821932484eb12);\\n \\n \\n\\n circle_marker_2d65bfe072e873597b6d0c382a60933d.bindPopup(popup_aea3c501725fa3097e291dc600943640)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ae6fa552d183f081b97bf4ac1dc7a8f = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_a98c4b030cd7dc35750dfd271f6a119e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4e0c6c826a3adf7a8c23319dd1f1e05 = $(`<div id="html_a4e0c6c826a3adf7a8c23319dd1f1e05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a98c4b030cd7dc35750dfd271f6a119e.setContent(html_a4e0c6c826a3adf7a8c23319dd1f1e05);\\n \\n \\n\\n circle_marker_7ae6fa552d183f081b97bf4ac1dc7a8f.bindPopup(popup_a98c4b030cd7dc35750dfd271f6a119e)\\n ;\\n\\n \\n \\n \\n var circle_marker_c36c702d71f57679566d2cc6109639d6 = L.circleMarker(\\n [42.72551121416183, -73.23289207553623],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.136496464611085, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_80e8e9397b4059feafda5bc6efc06168 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f0f512c2a6668d1e5fed61d4fc8b373 = $(`<div id="html_7f0f512c2a6668d1e5fed61d4fc8b373" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80e8e9397b4059feafda5bc6efc06168.setContent(html_7f0f512c2a6668d1e5fed61d4fc8b373);\\n \\n \\n\\n circle_marker_c36c702d71f57679566d2cc6109639d6.bindPopup(popup_80e8e9397b4059feafda5bc6efc06168)\\n ;\\n\\n \\n \\n \\n var circle_marker_4c7abaff4a096efd43693fe47feaf448 = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4115163798060095, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_b394975e204439c0903e9ecd0cd8d032 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bfdf481032626bc0b2310aa3521ca302 = $(`<div id="html_bfdf481032626bc0b2310aa3521ca302" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b394975e204439c0903e9ecd0cd8d032.setContent(html_bfdf481032626bc0b2310aa3521ca302);\\n \\n \\n\\n circle_marker_4c7abaff4a096efd43693fe47feaf448.bindPopup(popup_b394975e204439c0903e9ecd0cd8d032)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7161206031536043be7e4d509881b2d = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2e2dada14e45aaf70ffad16948d86d46 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58ad7dda9b39e4afcf462012b557b700 = $(`<div id="html_58ad7dda9b39e4afcf462012b557b700" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e2dada14e45aaf70ffad16948d86d46.setContent(html_58ad7dda9b39e4afcf462012b557b700);\\n \\n \\n\\n circle_marker_b7161206031536043be7e4d509881b2d.bindPopup(popup_2e2dada14e45aaf70ffad16948d86d46)\\n ;\\n\\n \\n \\n \\n var circle_marker_cdd15e8d286e75ad2b396bb2167ce66a = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_155acb95a40150fdf8220af56757825c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fbffee837502c76b8f070fa8c7286482 = $(`<div id="html_fbffee837502c76b8f070fa8c7286482" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_155acb95a40150fdf8220af56757825c.setContent(html_fbffee837502c76b8f070fa8c7286482);\\n \\n \\n\\n circle_marker_cdd15e8d286e75ad2b396bb2167ce66a.bindPopup(popup_155acb95a40150fdf8220af56757825c)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5b5200905d88ba0fa20a8daa79f3ac5 = L.circleMarker(\\n [42.72370842502844, -73.2599520158284],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.349243383340204, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_2e56cf8f0a3d2d78fa5bcad9e0cd6e0c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e1b73ecfa1f77a07346248b5a125e0f9 = $(`<div id="html_e1b73ecfa1f77a07346248b5a125e0f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e56cf8f0a3d2d78fa5bcad9e0cd6e0c.setContent(html_e1b73ecfa1f77a07346248b5a125e0f9);\\n \\n \\n\\n circle_marker_a5b5200905d88ba0fa20a8daa79f3ac5.bindPopup(popup_2e56cf8f0a3d2d78fa5bcad9e0cd6e0c)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1844db63a943ad1ac075bd37eb780f0 = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.257165172872317, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_467e30b27400cc6738528c43cc73dea7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5089c13398172f4d1fc01f802e63e2b2 = $(`<div id="html_5089c13398172f4d1fc01f802e63e2b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_467e30b27400cc6738528c43cc73dea7.setContent(html_5089c13398172f4d1fc01f802e63e2b2);\\n \\n \\n\\n circle_marker_b1844db63a943ad1ac075bd37eb780f0.bindPopup(popup_467e30b27400cc6738528c43cc73dea7)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1d2df97656c7b887cf91fc708e89183 = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.206085419025319, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_e8cd90d968ec9270bd5d2fcc352415ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06198cec074728ea5fd47128b3152967 = $(`<div id="html_06198cec074728ea5fd47128b3152967" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8cd90d968ec9270bd5d2fcc352415ef.setContent(html_06198cec074728ea5fd47128b3152967);\\n \\n \\n\\n circle_marker_d1d2df97656c7b887cf91fc708e89183.bindPopup(popup_e8cd90d968ec9270bd5d2fcc352415ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3c1a3383f5d07ad78a740dad9ae2bc8 = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4115163798060095, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_62831337135e780d802919504f258a31 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f193e15ed4aabc3363d86404455c4ded = $(`<div id="html_f193e15ed4aabc3363d86404455c4ded" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62831337135e780d802919504f258a31.setContent(html_f193e15ed4aabc3363d86404455c4ded);\\n \\n \\n\\n circle_marker_e3c1a3383f5d07ad78a740dad9ae2bc8.bindPopup(popup_62831337135e780d802919504f258a31)\\n ;\\n\\n \\n \\n \\n var circle_marker_84693ff94b2f85158c89c7667320e08b = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_fb3c5ce1d58b212dcb7317992595f27a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3c8c4689b09bedd022b4b1ed48f7e8f = $(`<div id="html_f3c8c4689b09bedd022b4b1ed48f7e8f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb3c5ce1d58b212dcb7317992595f27a.setContent(html_f3c8c4689b09bedd022b4b1ed48f7e8f);\\n \\n \\n\\n circle_marker_84693ff94b2f85158c89c7667320e08b.bindPopup(popup_fb3c5ce1d58b212dcb7317992595f27a)\\n ;\\n\\n \\n \\n \\n var circle_marker_945657a3180f7e113bf34a3f83af3c26 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_76a7b296bc4e5be87e8ff3996e0f9df0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_568f1c180ddc199977255cc4fe90bcfe = $(`<div id="html_568f1c180ddc199977255cc4fe90bcfe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76a7b296bc4e5be87e8ff3996e0f9df0.setContent(html_568f1c180ddc199977255cc4fe90bcfe);\\n \\n \\n\\n circle_marker_945657a3180f7e113bf34a3f83af3c26.bindPopup(popup_76a7b296bc4e5be87e8ff3996e0f9df0)\\n ;\\n\\n \\n \\n \\n var circle_marker_0867a3306612c896f24b20f53b0a512d = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_9511c5a4a0bc67570136f83bbb9ae825 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1398c876c56f3e8b543f235e39f5065 = $(`<div id="html_c1398c876c56f3e8b543f235e39f5065" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9511c5a4a0bc67570136f83bbb9ae825.setContent(html_c1398c876c56f3e8b543f235e39f5065);\\n \\n \\n\\n circle_marker_0867a3306612c896f24b20f53b0a512d.bindPopup(popup_9511c5a4a0bc67570136f83bbb9ae825)\\n ;\\n\\n \\n \\n \\n var circle_marker_5013f76e51bb8d672864a041f8d56284 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_85a446a94185c64bc7506a690a637c85);\\n \\n \\n var popup_56ab14206398ea867030682499680c8c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9aa0d268e6dda4a3331920190638cd0 = $(`<div id="html_d9aa0d268e6dda4a3331920190638cd0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_56ab14206398ea867030682499680c8c.setContent(html_d9aa0d268e6dda4a3331920190638cd0);\\n \\n \\n\\n circle_marker_5013f76e51bb8d672864a041f8d56284.bindPopup(popup_56ab14206398ea867030682499680c8c)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Birch, black\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_edde0508db6948fcada72369e97ee22c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_edde0508db6948fcada72369e97ee22c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_edde0508db6948fcada72369e97ee22c = L.map(\\n "map_edde0508db6948fcada72369e97ee22c",\\n {\\n center: [42.73545212957737, -73.24703638002094],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_64ba08892da543efc492bbb237573eed = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var circle_marker_a253029655174dfed7cdb4e83294ff20 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2ae094cdc7573262c7448b007a0da675 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65386064065fed7c36d2ff7fba47c831 = $(`<div id="html_65386064065fed7c36d2ff7fba47c831" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ae094cdc7573262c7448b007a0da675.setContent(html_65386064065fed7c36d2ff7fba47c831);\\n \\n \\n\\n circle_marker_a253029655174dfed7cdb4e83294ff20.bindPopup(popup_2ae094cdc7573262c7448b007a0da675)\\n ;\\n\\n \\n \\n \\n var circle_marker_95b001f44925f199c16c4f969f643ac6 = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6e25aac0424daa9524da19ce9f1b152e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48562693f40ee8343180190d97f0a6f4 = $(`<div id="html_48562693f40ee8343180190d97f0a6f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e25aac0424daa9524da19ce9f1b152e.setContent(html_48562693f40ee8343180190d97f0a6f4);\\n \\n \\n\\n circle_marker_95b001f44925f199c16c4f969f643ac6.bindPopup(popup_6e25aac0424daa9524da19ce9f1b152e)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d1fc680bad828b17357aeb820733686 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3355200e8c942ae1792578317f519447 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ab5dc127cc2932a64bf4f765afba409 = $(`<div id="html_4ab5dc127cc2932a64bf4f765afba409" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3355200e8c942ae1792578317f519447.setContent(html_4ab5dc127cc2932a64bf4f765afba409);\\n \\n \\n\\n circle_marker_8d1fc680bad828b17357aeb820733686.bindPopup(popup_3355200e8c942ae1792578317f519447)\\n ;\\n\\n \\n \\n \\n var circle_marker_8940f5cdfbc7dc674416f043ce716dee = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c231607b46e4cdc786967fd62937b86a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d28a0a3398915841354c0a03928f22c = $(`<div id="html_7d28a0a3398915841354c0a03928f22c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c231607b46e4cdc786967fd62937b86a.setContent(html_7d28a0a3398915841354c0a03928f22c);\\n \\n \\n\\n circle_marker_8940f5cdfbc7dc674416f043ce716dee.bindPopup(popup_c231607b46e4cdc786967fd62937b86a)\\n ;\\n\\n \\n \\n \\n var circle_marker_db64ef8a628f8d5c4a2d2500cb8d27d9 = L.circleMarker(\\n [42.747201204933376, -73.2685630990657],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9fb750fb49b51c15afaa665bdebbac25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e6046e652d887dd6d6781c6f8b7df536 = $(`<div id="html_e6046e652d887dd6d6781c6f8b7df536" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fb750fb49b51c15afaa665bdebbac25.setContent(html_e6046e652d887dd6d6781c6f8b7df536);\\n \\n \\n\\n circle_marker_db64ef8a628f8d5c4a2d2500cb8d27d9.bindPopup(popup_9fb750fb49b51c15afaa665bdebbac25)\\n ;\\n\\n \\n \\n \\n var circle_marker_39a36bc2125a6c3475bce4ee53ab57ee = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c989f663795b19aec698c3ca5e006b41 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd9c33c7e27f81c858eb48778e0e8704 = $(`<div id="html_cd9c33c7e27f81c858eb48778e0e8704" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c989f663795b19aec698c3ca5e006b41.setContent(html_cd9c33c7e27f81c858eb48778e0e8704);\\n \\n \\n\\n circle_marker_39a36bc2125a6c3475bce4ee53ab57ee.bindPopup(popup_c989f663795b19aec698c3ca5e006b41)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ba4a630ba15ab0d9f4a7e89bad1eacb = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_64f46dd89764e0b7f892e3fd48daca29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3745789f22c10aaa993a2962bb09d88 = $(`<div id="html_e3745789f22c10aaa993a2962bb09d88" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64f46dd89764e0b7f892e3fd48daca29.setContent(html_e3745789f22c10aaa993a2962bb09d88);\\n \\n \\n\\n circle_marker_9ba4a630ba15ab0d9f4a7e89bad1eacb.bindPopup(popup_64f46dd89764e0b7f892e3fd48daca29)\\n ;\\n\\n \\n \\n \\n var circle_marker_4aaad7dbaddb6ca3aad1ac6d991af628 = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_981b8e5158de54938c8b9d2663424e7a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bbfe7fcbee8e446ab492b59bba0d869b = $(`<div id="html_bbfe7fcbee8e446ab492b59bba0d869b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_981b8e5158de54938c8b9d2663424e7a.setContent(html_bbfe7fcbee8e446ab492b59bba0d869b);\\n \\n \\n\\n circle_marker_4aaad7dbaddb6ca3aad1ac6d991af628.bindPopup(popup_981b8e5158de54938c8b9d2663424e7a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f23b77a8d4f173cb70264410d081e86a = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c75a76856b4c3be1c741a81ce3fc03de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e5fb1a27c08b1d8b886288bde981fe0 = $(`<div id="html_9e5fb1a27c08b1d8b886288bde981fe0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c75a76856b4c3be1c741a81ce3fc03de.setContent(html_9e5fb1a27c08b1d8b886288bde981fe0);\\n \\n \\n\\n circle_marker_f23b77a8d4f173cb70264410d081e86a.bindPopup(popup_c75a76856b4c3be1c741a81ce3fc03de)\\n ;\\n\\n \\n \\n \\n var circle_marker_49528d38effb1bb37d81fc19863a74d2 = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_bb12fff3afe35c706c661f00be4cdce9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65003a86ecd24ea557ca0dd054c8f6d9 = $(`<div id="html_65003a86ecd24ea557ca0dd054c8f6d9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb12fff3afe35c706c661f00be4cdce9.setContent(html_65003a86ecd24ea557ca0dd054c8f6d9);\\n \\n \\n\\n circle_marker_49528d38effb1bb37d81fc19863a74d2.bindPopup(popup_bb12fff3afe35c706c661f00be4cdce9)\\n ;\\n\\n \\n \\n \\n var circle_marker_95599cca6ab0d70c02f9a664e508416d = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ffa30e86285408e3b85f7260ef835459 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c522725670ee2142ac000b9b6193bfdf = $(`<div id="html_c522725670ee2142ac000b9b6193bfdf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ffa30e86285408e3b85f7260ef835459.setContent(html_c522725670ee2142ac000b9b6193bfdf);\\n \\n \\n\\n circle_marker_95599cca6ab0d70c02f9a664e508416d.bindPopup(popup_ffa30e86285408e3b85f7260ef835459)\\n ;\\n\\n \\n \\n \\n var circle_marker_ae9934db2197b17bf8588b036377b013 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f93fa5f63d2925121c9383bba74f8439 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b5556beb804dcb8363c3381ff9f553e = $(`<div id="html_9b5556beb804dcb8363c3381ff9f553e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f93fa5f63d2925121c9383bba74f8439.setContent(html_9b5556beb804dcb8363c3381ff9f553e);\\n \\n \\n\\n circle_marker_ae9934db2197b17bf8588b036377b013.bindPopup(popup_f93fa5f63d2925121c9383bba74f8439)\\n ;\\n\\n \\n \\n \\n var circle_marker_b177e82a5968712b036938b3c542e153 = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_bd7c78de90b62a4aa69f69d162eac798 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f0d2507926e7d5ab116810fe4e5790ef = $(`<div id="html_f0d2507926e7d5ab116810fe4e5790ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd7c78de90b62a4aa69f69d162eac798.setContent(html_f0d2507926e7d5ab116810fe4e5790ef);\\n \\n \\n\\n circle_marker_b177e82a5968712b036938b3c542e153.bindPopup(popup_bd7c78de90b62a4aa69f69d162eac798)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d217599be1ecbe8260eece82bdaf11e = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9c3f622b368a4e4635e58cfc8d7d1a55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2e6552392947c1d51a1035ad2716b6a = $(`<div id="html_a2e6552392947c1d51a1035ad2716b6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9c3f622b368a4e4635e58cfc8d7d1a55.setContent(html_a2e6552392947c1d51a1035ad2716b6a);\\n \\n \\n\\n circle_marker_5d217599be1ecbe8260eece82bdaf11e.bindPopup(popup_9c3f622b368a4e4635e58cfc8d7d1a55)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b10dc5508e6b962571e2fdc3b9a613e = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_21ac22296d376cfb9f413b1cd24ba6d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00da57c391275d25d29854fe87192c82 = $(`<div id="html_00da57c391275d25d29854fe87192c82" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_21ac22296d376cfb9f413b1cd24ba6d1.setContent(html_00da57c391275d25d29854fe87192c82);\\n \\n \\n\\n circle_marker_8b10dc5508e6b962571e2fdc3b9a613e.bindPopup(popup_21ac22296d376cfb9f413b1cd24ba6d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_025cc470caae1341040de0ee1fd59518 = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f0e0dcab3fb2d73a56d32a0fde121341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3bd93819eaeaf2c794c87ba0eaa59aa0 = $(`<div id="html_3bd93819eaeaf2c794c87ba0eaa59aa0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0e0dcab3fb2d73a56d32a0fde121341.setContent(html_3bd93819eaeaf2c794c87ba0eaa59aa0);\\n \\n \\n\\n circle_marker_025cc470caae1341040de0ee1fd59518.bindPopup(popup_f0e0dcab3fb2d73a56d32a0fde121341)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7b91f659c14593d1f99a6cc910ce639 = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a39216edd4b287153454f1a33e73c700 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ebfa52eeff64021a8abc1972358c3eda = $(`<div id="html_ebfa52eeff64021a8abc1972358c3eda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a39216edd4b287153454f1a33e73c700.setContent(html_ebfa52eeff64021a8abc1972358c3eda);\\n \\n \\n\\n circle_marker_e7b91f659c14593d1f99a6cc910ce639.bindPopup(popup_a39216edd4b287153454f1a33e73c700)\\n ;\\n\\n \\n \\n \\n var circle_marker_4daaa8f4f62a34aac1f15622addc9780 = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ce16e46689b4d081a71a79fd8b93abac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_729d6e74609a964aa406a6774eb3278a = $(`<div id="html_729d6e74609a964aa406a6774eb3278a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce16e46689b4d081a71a79fd8b93abac.setContent(html_729d6e74609a964aa406a6774eb3278a);\\n \\n \\n\\n circle_marker_4daaa8f4f62a34aac1f15622addc9780.bindPopup(popup_ce16e46689b4d081a71a79fd8b93abac)\\n ;\\n\\n \\n \\n \\n var circle_marker_230909c5dead5d71be7ba8c33bc11820 = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_df3595343f6d2144c21e9af920f5cdcf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a2ee09911681c47f3d2026d0e915ffd = $(`<div id="html_5a2ee09911681c47f3d2026d0e915ffd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df3595343f6d2144c21e9af920f5cdcf.setContent(html_5a2ee09911681c47f3d2026d0e915ffd);\\n \\n \\n\\n circle_marker_230909c5dead5d71be7ba8c33bc11820.bindPopup(popup_df3595343f6d2144c21e9af920f5cdcf)\\n ;\\n\\n \\n \\n \\n var circle_marker_936ad2b0f5f17993532a0e85e96d8523 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_fde74c508feff1fbeec643773b6ffb8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ccca6e0edf00a9425365d9148bd287e = $(`<div id="html_7ccca6e0edf00a9425365d9148bd287e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fde74c508feff1fbeec643773b6ffb8d.setContent(html_7ccca6e0edf00a9425365d9148bd287e);\\n \\n \\n\\n circle_marker_936ad2b0f5f17993532a0e85e96d8523.bindPopup(popup_fde74c508feff1fbeec643773b6ffb8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0407ed7aeab0c44afd7abc59fcdaccc4 = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3b4eeddd16f098a6c59d7f86fcb6bb39 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8545d3f9a50d1bf4dcd09391dbe45c56 = $(`<div id="html_8545d3f9a50d1bf4dcd09391dbe45c56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3b4eeddd16f098a6c59d7f86fcb6bb39.setContent(html_8545d3f9a50d1bf4dcd09391dbe45c56);\\n \\n \\n\\n circle_marker_0407ed7aeab0c44afd7abc59fcdaccc4.bindPopup(popup_3b4eeddd16f098a6c59d7f86fcb6bb39)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1e035233fdd7555daeed1abbaf45a9e = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_84fb7d6320391705b1e81e02a5f599be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97b3b01a9c93105efe322b11e0bd38b9 = $(`<div id="html_97b3b01a9c93105efe322b11e0bd38b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84fb7d6320391705b1e81e02a5f599be.setContent(html_97b3b01a9c93105efe322b11e0bd38b9);\\n \\n \\n\\n circle_marker_e1e035233fdd7555daeed1abbaf45a9e.bindPopup(popup_84fb7d6320391705b1e81e02a5f599be)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8c9037ab7e0cba5e638c6baa87f38f0 = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2bf7a48c5e378c8ea6c7c57021f36ebe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_541e4243624b385cd1cc8c534cbe49aa = $(`<div id="html_541e4243624b385cd1cc8c534cbe49aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2bf7a48c5e378c8ea6c7c57021f36ebe.setContent(html_541e4243624b385cd1cc8c534cbe49aa);\\n \\n \\n\\n circle_marker_c8c9037ab7e0cba5e638c6baa87f38f0.bindPopup(popup_2bf7a48c5e378c8ea6c7c57021f36ebe)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1cef9855883d6bdb8f4435a262a4b5a = L.circleMarker(\\n [42.74539412424261, -73.26364145064247],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f5da074bfbcc3e926ae0b115fe1c8b56 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ea46d617bf4c029e1e087b803016087 = $(`<div id="html_7ea46d617bf4c029e1e087b803016087" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5da074bfbcc3e926ae0b115fe1c8b56.setContent(html_7ea46d617bf4c029e1e087b803016087);\\n \\n \\n\\n circle_marker_b1cef9855883d6bdb8f4435a262a4b5a.bindPopup(popup_f5da074bfbcc3e926ae0b115fe1c8b56)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f114c8ce2061be807c8f7250516650f = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_168453232dbe60f678ea10dac096dc85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56b601b98dd677b5f62a092b98bf5396 = $(`<div id="html_56b601b98dd677b5f62a092b98bf5396" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_168453232dbe60f678ea10dac096dc85.setContent(html_56b601b98dd677b5f62a092b98bf5396);\\n \\n \\n\\n circle_marker_6f114c8ce2061be807c8f7250516650f.bindPopup(popup_168453232dbe60f678ea10dac096dc85)\\n ;\\n\\n \\n \\n \\n var circle_marker_03ba4a7e4b6667b7e46e28d1ae5c4be4 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5b51fb3dbd976aefe7bfb5d4e7deaf88 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92e439d96478f0f7cd23d5d827086243 = $(`<div id="html_92e439d96478f0f7cd23d5d827086243" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5b51fb3dbd976aefe7bfb5d4e7deaf88.setContent(html_92e439d96478f0f7cd23d5d827086243);\\n \\n \\n\\n circle_marker_03ba4a7e4b6667b7e46e28d1ae5c4be4.bindPopup(popup_5b51fb3dbd976aefe7bfb5d4e7deaf88)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e8c4adead5a5b9bb1424f8464808a0d = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_987bdbc17c0b27b2b369ff3325a020aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7eac19a880fabad89e2706701660061d = $(`<div id="html_7eac19a880fabad89e2706701660061d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_987bdbc17c0b27b2b369ff3325a020aa.setContent(html_7eac19a880fabad89e2706701660061d);\\n \\n \\n\\n circle_marker_9e8c4adead5a5b9bb1424f8464808a0d.bindPopup(popup_987bdbc17c0b27b2b369ff3325a020aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_1f1fd4c5397ef6272e5e7b1cd4de5aaf = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e645ec39aa51f2b63a68c3acfeaf856f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ffa6c6e5075203092ee62a136eee444 = $(`<div id="html_9ffa6c6e5075203092ee62a136eee444" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e645ec39aa51f2b63a68c3acfeaf856f.setContent(html_9ffa6c6e5075203092ee62a136eee444);\\n \\n \\n\\n circle_marker_1f1fd4c5397ef6272e5e7b1cd4de5aaf.bindPopup(popup_e645ec39aa51f2b63a68c3acfeaf856f)\\n ;\\n\\n \\n \\n \\n var circle_marker_75bec47abb2f23f1f0c1ca8cc2ff856d = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_80800a802570c5662e818d07d32f6fa9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e44d72a9d1de31061fa856867ba683f8 = $(`<div id="html_e44d72a9d1de31061fa856867ba683f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80800a802570c5662e818d07d32f6fa9.setContent(html_e44d72a9d1de31061fa856867ba683f8);\\n \\n \\n\\n circle_marker_75bec47abb2f23f1f0c1ca8cc2ff856d.bindPopup(popup_80800a802570c5662e818d07d32f6fa9)\\n ;\\n\\n \\n \\n \\n var circle_marker_060c927b71410c78f4ec7886cacf5b50 = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9826d6e36f82ec0aa909aa75a4f4de77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be8ec912ff76a69f05c6d741e30c42f1 = $(`<div id="html_be8ec912ff76a69f05c6d741e30c42f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9826d6e36f82ec0aa909aa75a4f4de77.setContent(html_be8ec912ff76a69f05c6d741e30c42f1);\\n \\n \\n\\n circle_marker_060c927b71410c78f4ec7886cacf5b50.bindPopup(popup_9826d6e36f82ec0aa909aa75a4f4de77)\\n ;\\n\\n \\n \\n \\n var circle_marker_63995604287fd002471227f96934b795 = L.circleMarker(\\n [42.74358676039927, -73.27225393186212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_95404e19684ccbc5225ccce5b8f4ad5f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_12bad408c05fce52530d93f42f4156b7 = $(`<div id="html_12bad408c05fce52530d93f42f4156b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95404e19684ccbc5225ccce5b8f4ad5f.setContent(html_12bad408c05fce52530d93f42f4156b7);\\n \\n \\n\\n circle_marker_63995604287fd002471227f96934b795.bindPopup(popup_95404e19684ccbc5225ccce5b8f4ad5f)\\n ;\\n\\n \\n \\n \\n var circle_marker_406a8694505cb081853ff1cf6d47e3b6 = L.circleMarker(\\n [42.74358689209615, -73.26979323319746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f22e8297152cdf64358c7867c771be13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6f75944833dea6c31311345ef9e1249 = $(`<div id="html_b6f75944833dea6c31311345ef9e1249" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f22e8297152cdf64358c7867c771be13.setContent(html_b6f75944833dea6c31311345ef9e1249);\\n \\n \\n\\n circle_marker_406a8694505cb081853ff1cf6d47e3b6.bindPopup(popup_f22e8297152cdf64358c7867c771be13)\\n ;\\n\\n \\n \\n \\n var circle_marker_30d06a8876a599ec811b832167fab690 = L.circleMarker(\\n [42.743586938190056, -73.26856288386173],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_962f1fc40d5f11fcccee7cf3c8e8b85b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9b7d20e2c31319472d93911a25b5bc9 = $(`<div id="html_d9b7d20e2c31319472d93911a25b5bc9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_962f1fc40d5f11fcccee7cf3c8e8b85b.setContent(html_d9b7d20e2c31319472d93911a25b5bc9);\\n \\n \\n\\n circle_marker_30d06a8876a599ec811b832167fab690.bindPopup(popup_962f1fc40d5f11fcccee7cf3c8e8b85b)\\n ;\\n\\n \\n \\n \\n var circle_marker_7635bcf4ff8ebe78c3f90a65df1fa261 = L.circleMarker(\\n [42.74358697111428, -73.26733253452443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3e8e6a8e1f5477e3c86e4cd59d324939 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7716e246dff47bb224b99d44164d392 = $(`<div id="html_e7716e246dff47bb224b99d44164d392" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e8e6a8e1f5477e3c86e4cd59d324939.setContent(html_e7716e246dff47bb224b99d44164d392);\\n \\n \\n\\n circle_marker_7635bcf4ff8ebe78c3f90a65df1fa261.bindPopup(popup_3e8e6a8e1f5477e3c86e4cd59d324939)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5acbc1f5e520dfd6e76c57d8c011d48 = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c4eb5fd0159a23498d73a6e3bab8856a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_936594f91e429e86474684a05be16a0f = $(`<div id="html_936594f91e429e86474684a05be16a0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4eb5fd0159a23498d73a6e3bab8856a.setContent(html_936594f91e429e86474684a05be16a0f);\\n \\n \\n\\n circle_marker_d5acbc1f5e520dfd6e76c57d8c011d48.bindPopup(popup_c4eb5fd0159a23498d73a6e3bab8856a)\\n ;\\n\\n \\n \\n \\n var circle_marker_49dfb044854c81c12fb311ec9aa52f8d = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_de170c93f4c1b78394017bebdfa8ccd5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7319b77de02f4c57d104fe4d900a0863 = $(`<div id="html_7319b77de02f4c57d104fe4d900a0863" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de170c93f4c1b78394017bebdfa8ccd5.setContent(html_7319b77de02f4c57d104fe4d900a0863);\\n \\n \\n\\n circle_marker_49dfb044854c81c12fb311ec9aa52f8d.bindPopup(popup_de170c93f4c1b78394017bebdfa8ccd5)\\n ;\\n\\n \\n \\n \\n var circle_marker_857276452cf32ccb3af6338230ec6bc2 = L.circleMarker(\\n [42.74358699086881, -73.26364148650833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e3367c184230cb886f57da3393c803e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60b8fbf87c8c825dd111d5f849d64a1d = $(`<div id="html_60b8fbf87c8c825dd111d5f849d64a1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3367c184230cb886f57da3393c803e4.setContent(html_60b8fbf87c8c825dd111d5f849d64a1d);\\n \\n \\n\\n circle_marker_857276452cf32ccb3af6338230ec6bc2.bindPopup(popup_e3367c184230cb886f57da3393c803e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_a11b3e407962540de4baa035998b81d0 = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_598e8c597dfd54c0a61d286b1185c138 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2d1ce608936c2078193f9020021be3e = $(`<div id="html_e2d1ce608936c2078193f9020021be3e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_598e8c597dfd54c0a61d286b1185c138.setContent(html_e2d1ce608936c2078193f9020021be3e);\\n \\n \\n\\n circle_marker_a11b3e407962540de4baa035998b81d0.bindPopup(popup_598e8c597dfd54c0a61d286b1185c138)\\n ;\\n\\n \\n \\n \\n var circle_marker_439f1352834ff1b6a7113b6764eea08f = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ac2fde8a00752bf644467472478fb83d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b13fc8857892c419e61543de9f274e2f = $(`<div id="html_b13fc8857892c419e61543de9f274e2f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac2fde8a00752bf644467472478fb83d.setContent(html_b13fc8857892c419e61543de9f274e2f);\\n \\n \\n\\n circle_marker_439f1352834ff1b6a7113b6764eea08f.bindPopup(popup_ac2fde8a00752bf644467472478fb83d)\\n ;\\n\\n \\n \\n \\n var circle_marker_2856598aee15c15ef6e9f0146a5494e3 = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6c7680256cf9594a1cb152c83f7e379f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2b0419f44375c59dbb25b532a7d0b71 = $(`<div id="html_d2b0419f44375c59dbb25b532a7d0b71" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c7680256cf9594a1cb152c83f7e379f.setContent(html_d2b0419f44375c59dbb25b532a7d0b71);\\n \\n \\n\\n circle_marker_2856598aee15c15ef6e9f0146a5494e3.bindPopup(popup_6c7680256cf9594a1cb152c83f7e379f)\\n ;\\n\\n \\n \\n \\n var circle_marker_698bdf45cac42929f6f8053cfdc2d567 = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_cc28f7dbe8ed7fc399bdfb2d5fafa024 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06ef9ded33590eec8af89d1eb08cbfdd = $(`<div id="html_06ef9ded33590eec8af89d1eb08cbfdd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc28f7dbe8ed7fc399bdfb2d5fafa024.setContent(html_06ef9ded33590eec8af89d1eb08cbfdd);\\n \\n \\n\\n circle_marker_698bdf45cac42929f6f8053cfdc2d567.bindPopup(popup_cc28f7dbe8ed7fc399bdfb2d5fafa024)\\n ;\\n\\n \\n \\n \\n var circle_marker_4824f423ed445e7ac3d2e00f95a178c2 = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c535706352aabae18405d5a3627de9b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_200f57835bd946f85e7e6d6e668d6dd4 = $(`<div id="html_200f57835bd946f85e7e6d6e668d6dd4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c535706352aabae18405d5a3627de9b7.setContent(html_200f57835bd946f85e7e6d6e668d6dd4);\\n \\n \\n\\n circle_marker_4824f423ed445e7ac3d2e00f95a178c2.bindPopup(popup_c535706352aabae18405d5a3627de9b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_880ea5f644c855823ddc26f16c6fc967 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_02199fcdc283104c437164c8b3bbd736 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c923fd7f08ea9587c252f2540924837 = $(`<div id="html_9c923fd7f08ea9587c252f2540924837" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_02199fcdc283104c437164c8b3bbd736.setContent(html_9c923fd7f08ea9587c252f2540924837);\\n \\n \\n\\n circle_marker_880ea5f644c855823ddc26f16c6fc967.bindPopup(popup_02199fcdc283104c437164c8b3bbd736)\\n ;\\n\\n \\n \\n \\n var circle_marker_a81c8c3ea4e2ac37f0f15a311a6eb04f = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8b5fa194ceb5f8c111aaeec728bfd8ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19a63a69feccc79f777f10ff2a8fc1b9 = $(`<div id="html_19a63a69feccc79f777f10ff2a8fc1b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b5fa194ceb5f8c111aaeec728bfd8ab.setContent(html_19a63a69feccc79f777f10ff2a8fc1b9);\\n \\n \\n\\n circle_marker_a81c8c3ea4e2ac37f0f15a311a6eb04f.bindPopup(popup_8b5fa194ceb5f8c111aaeec728bfd8ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca12d7c516a28374ef6dddafdd381786 = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e88fbf051f9e336c20b207a11882a726 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_29d0be33c492ac53e14a0191f89b20c6 = $(`<div id="html_29d0be33c492ac53e14a0191f89b20c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e88fbf051f9e336c20b207a11882a726.setContent(html_29d0be33c492ac53e14a0191f89b20c6);\\n \\n \\n\\n circle_marker_ca12d7c516a28374ef6dddafdd381786.bindPopup(popup_e88fbf051f9e336c20b207a11882a726)\\n ;\\n\\n \\n \\n \\n var circle_marker_a412e785be6f1393dbf746314e8b949c = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_94ac693e99da012655f0b755554e9055 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ef7370f4bbfe5df79f9ab8fde6ce510 = $(`<div id="html_0ef7370f4bbfe5df79f9ab8fde6ce510" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94ac693e99da012655f0b755554e9055.setContent(html_0ef7370f4bbfe5df79f9ab8fde6ce510);\\n \\n \\n\\n circle_marker_a412e785be6f1393dbf746314e8b949c.bindPopup(popup_94ac693e99da012655f0b755554e9055)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6f1f7722358ec08bcb0dcc1aa4db456 = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b0636798ad8da4fa035646374979efdf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5dfb687ecddf0deda505d7e2473983d8 = $(`<div id="html_5dfb687ecddf0deda505d7e2473983d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0636798ad8da4fa035646374979efdf.setContent(html_5dfb687ecddf0deda505d7e2473983d8);\\n \\n \\n\\n circle_marker_d6f1f7722358ec08bcb0dcc1aa4db456.bindPopup(popup_b0636798ad8da4fa035646374979efdf)\\n ;\\n\\n \\n \\n \\n var circle_marker_294b52bb2a637cec38841b7e24370c2f = L.circleMarker(\\n [42.74177983774129, -73.26733246279849],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8c4e4163fe26847246da017416a1d11f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d88822fb771666bd29e314a039f33021 = $(`<div id="html_d88822fb771666bd29e314a039f33021" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c4e4163fe26847246da017416a1d11f.setContent(html_d88822fb771666bd29e314a039f33021);\\n \\n \\n\\n circle_marker_294b52bb2a637cec38841b7e24370c2f.bindPopup(popup_8c4e4163fe26847246da017416a1d11f)\\n ;\\n\\n \\n \\n \\n var circle_marker_d67b70c8f6443eea2664011ccc55a4f0 = L.circleMarker(\\n [42.74177985749502, -73.26364152237132],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_fa9d848ab85308564a58ff17f7751c30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_deca56a780614218ca0c2ce2f95a6f7f = $(`<div id="html_deca56a780614218ca0c2ce2f95a6f7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa9d848ab85308564a58ff17f7751c30.setContent(html_deca56a780614218ca0c2ce2f95a6f7f);\\n \\n \\n\\n circle_marker_d67b70c8f6443eea2664011ccc55a4f0.bindPopup(popup_fa9d848ab85308564a58ff17f7751c30)\\n ;\\n\\n \\n \\n \\n var circle_marker_bec0cefd43f6f5ed916d65736c91722e = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9d6aecffbfb6c69f4fd9accb387efeeb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69d8b4ac5c1374bd1bb797ef520aa22d = $(`<div id="html_69d8b4ac5c1374bd1bb797ef520aa22d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d6aecffbfb6c69f4fd9accb387efeeb.setContent(html_69d8b4ac5c1374bd1bb797ef520aa22d);\\n \\n \\n\\n circle_marker_bec0cefd43f6f5ed916d65736c91722e.bindPopup(popup_9d6aecffbfb6c69f4fd9accb387efeeb)\\n ;\\n\\n \\n \\n \\n var circle_marker_f33e317fb1ee708af82dd7e06a326fe3 = L.circleMarker(\\n [42.7417798048184, -73.2611808954216],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b41b024ce812ff17810cd6f1bbce6338 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_80e5d986c8391d0364eca4595282fada = $(`<div id="html_80e5d986c8391d0364eca4595282fada" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b41b024ce812ff17810cd6f1bbce6338.setContent(html_80e5d986c8391d0364eca4595282fada);\\n \\n \\n\\n circle_marker_f33e317fb1ee708af82dd7e06a326fe3.bindPopup(popup_b41b024ce812ff17810cd6f1bbce6338)\\n ;\\n\\n \\n \\n \\n var circle_marker_1db69b4ac0f20153be3955b8f8e93bea = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6b8f5cf6f7644a14ac5e78dde2aa881b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d8b643ec2cf3b9c618b1d576207e030 = $(`<div id="html_7d8b643ec2cf3b9c618b1d576207e030" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b8f5cf6f7644a14ac5e78dde2aa881b.setContent(html_7d8b643ec2cf3b9c618b1d576207e030);\\n \\n \\n\\n circle_marker_1db69b4ac0f20153be3955b8f8e93bea.bindPopup(popup_6b8f5cf6f7644a14ac5e78dde2aa881b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0d407da315cc32a69351e656fcea380 = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9e98636ee6cc02963f96584df6535c1a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63d080d19f24cf114f0d79c8562ce74c = $(`<div id="html_63d080d19f24cf114f0d79c8562ce74c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e98636ee6cc02963f96584df6535c1a.setContent(html_63d080d19f24cf114f0d79c8562ce74c);\\n \\n \\n\\n circle_marker_e0d407da315cc32a69351e656fcea380.bindPopup(popup_9e98636ee6cc02963f96584df6535c1a)\\n ;\\n\\n \\n \\n \\n var circle_marker_552147b82ebb06e67e3c14b18069201f = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_47cae2c85d858df440891103120b1f62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b5baebc1ecbbc74143850827f96e359e = $(`<div id="html_b5baebc1ecbbc74143850827f96e359e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47cae2c85d858df440891103120b1f62.setContent(html_b5baebc1ecbbc74143850827f96e359e);\\n \\n \\n\\n circle_marker_552147b82ebb06e67e3c14b18069201f.bindPopup(popup_47cae2c85d858df440891103120b1f62)\\n ;\\n\\n \\n \\n \\n var circle_marker_d8c97ec2eca3fb1a398bcc29de27e3f3 = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_cd56b68abcf90d8e85a7a02072a2ed83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d5b93ccfe3591ddaeae7161ca59f48db = $(`<div id="html_d5b93ccfe3591ddaeae7161ca59f48db" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd56b68abcf90d8e85a7a02072a2ed83.setContent(html_d5b93ccfe3591ddaeae7161ca59f48db);\\n \\n \\n\\n circle_marker_d8c97ec2eca3fb1a398bcc29de27e3f3.bindPopup(popup_cd56b68abcf90d8e85a7a02072a2ed83)\\n ;\\n\\n \\n \\n \\n var circle_marker_da3f86e9221705384364a86fbb8f6db7 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f7cddb81b8ace9d3cff86e22ac449c94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd852773afe92ee0cc4d4860d43314ea = $(`<div id="html_bd852773afe92ee0cc4d4860d43314ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7cddb81b8ace9d3cff86e22ac449c94.setContent(html_bd852773afe92ee0cc4d4860d43314ea);\\n \\n \\n\\n circle_marker_da3f86e9221705384364a86fbb8f6db7.bindPopup(popup_f7cddb81b8ace9d3cff86e22ac449c94)\\n ;\\n\\n \\n \\n \\n var circle_marker_165a801ffccfb17f36fcbb1780372487 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1b25ae60c1d41635907c35d2c15d1eb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_465d53e1c4081491fb13fcdaa2d1e01a = $(`<div id="html_465d53e1c4081491fb13fcdaa2d1e01a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b25ae60c1d41635907c35d2c15d1eb1.setContent(html_465d53e1c4081491fb13fcdaa2d1e01a);\\n \\n \\n\\n circle_marker_165a801ffccfb17f36fcbb1780372487.bindPopup(popup_1b25ae60c1d41635907c35d2c15d1eb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ad0f0e53afb5f465a7b79f3a45bf4e1 = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5a65a49c93ec13112422eaaa42d59d4a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2664f09d7cf68d659b9451dd6ff4a1d2 = $(`<div id="html_2664f09d7cf68d659b9451dd6ff4a1d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a65a49c93ec13112422eaaa42d59d4a.setContent(html_2664f09d7cf68d659b9451dd6ff4a1d2);\\n \\n \\n\\n circle_marker_3ad0f0e53afb5f465a7b79f3a45bf4e1.bindPopup(popup_5a65a49c93ec13112422eaaa42d59d4a)\\n ;\\n\\n \\n \\n \\n var circle_marker_da1c4018deb8a6423a5bf91419cefed5 = L.circleMarker(\\n [42.73997262535657, -73.25995072538909],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ff152c95442f18ebec27d23cfff53a78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_068a492b28dba80937b8e57b76daa578 = $(`<div id="html_068a492b28dba80937b8e57b76daa578" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff152c95442f18ebec27d23cfff53a78.setContent(html_068a492b28dba80937b8e57b76daa578);\\n \\n \\n\\n circle_marker_da1c4018deb8a6423a5bf91419cefed5.bindPopup(popup_ff152c95442f18ebec27d23cfff53a78)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a966beafaefd025ff92881d5ef71d6a = L.circleMarker(\\n [42.73997256609777, -73.25872044777847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0dbf7919b192e2c987148e28cfa05c37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9cd4c3b1c854adb7f3c6cc35389b757 = $(`<div id="html_a9cd4c3b1c854adb7f3c6cc35389b757" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0dbf7919b192e2c987148e28cfa05c37.setContent(html_a9cd4c3b1c854adb7f3c6cc35389b757);\\n \\n \\n\\n circle_marker_8a966beafaefd025ff92881d5ef71d6a.bindPopup(popup_0dbf7919b192e2c987148e28cfa05c37)\\n ;\\n\\n \\n \\n \\n var circle_marker_7670821a731cfb4e2da7f8d5bd674f79 = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_73ad5c4ed98d39ab89f3a84a1ea7ac25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40e281a86d147bc266195248d94f154a = $(`<div id="html_40e281a86d147bc266195248d94f154a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73ad5c4ed98d39ab89f3a84a1ea7ac25.setContent(html_40e281a86d147bc266195248d94f154a);\\n \\n \\n\\n circle_marker_7670821a731cfb4e2da7f8d5bd674f79.bindPopup(popup_73ad5c4ed98d39ab89f3a84a1ea7ac25)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa511991f540d202943b7efb4efb6c65 = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_20ab15a53a2f79a3ddadb8c74da9dbbf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0fe7aea746822d104f101a500c9f7b8a = $(`<div id="html_0fe7aea746822d104f101a500c9f7b8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20ab15a53a2f79a3ddadb8c74da9dbbf.setContent(html_0fe7aea746822d104f101a500c9f7b8a);\\n \\n \\n\\n circle_marker_fa511991f540d202943b7efb4efb6c65.bindPopup(popup_20ab15a53a2f79a3ddadb8c74da9dbbf)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e6d4ab6aa865b718f246379df1a7351 = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ac733ea2db14770bb9f4fa85f445a64f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_575f3ff33d3da4a0dc26ae534b6f96df = $(`<div id="html_575f3ff33d3da4a0dc26ae534b6f96df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac733ea2db14770bb9f4fa85f445a64f.setContent(html_575f3ff33d3da4a0dc26ae534b6f96df);\\n \\n \\n\\n circle_marker_6e6d4ab6aa865b718f246379df1a7351.bindPopup(popup_ac733ea2db14770bb9f4fa85f445a64f)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a5a331f7c273e3f3a2a990e7da9cfdc = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0eac3808b3779632548d84e99336962b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e17485b179eca9ce8346d2fb4fd2d2b4 = $(`<div id="html_e17485b179eca9ce8346d2fb4fd2d2b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0eac3808b3779632548d84e99336962b.setContent(html_e17485b179eca9ce8346d2fb4fd2d2b4);\\n \\n \\n\\n circle_marker_5a5a331f7c273e3f3a2a990e7da9cfdc.bindPopup(popup_0eac3808b3779632548d84e99336962b)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f8b8c27f986c428f7469b5e41439087 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_fe5142eed95e22445516b878a039934a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e97795ce608fc067dd6a715d216d8f84 = $(`<div id="html_e97795ce608fc067dd6a715d216d8f84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe5142eed95e22445516b878a039934a.setContent(html_e97795ce608fc067dd6a715d216d8f84);\\n \\n \\n\\n circle_marker_5f8b8c27f986c428f7469b5e41439087.bindPopup(popup_fe5142eed95e22445516b878a039934a)\\n ;\\n\\n \\n \\n \\n var circle_marker_7252556a1f1b0e10ff4af6386fd23be6 = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_855d8faa4876fd376f702c7360b0f1fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ee5bf013f907bbbc43fb7c58aca1a351 = $(`<div id="html_ee5bf013f907bbbc43fb7c58aca1a351" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_855d8faa4876fd376f702c7360b0f1fd.setContent(html_ee5bf013f907bbbc43fb7c58aca1a351);\\n \\n \\n\\n circle_marker_7252556a1f1b0e10ff4af6386fd23be6.bindPopup(popup_855d8faa4876fd376f702c7360b0f1fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_11f37752c2007886d8d078b272b37b66 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ffe028bf221c21d4dfe37075b5c508a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_75ed137c99d1680e50efb1c01569543d = $(`<div id="html_75ed137c99d1680e50efb1c01569543d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ffe028bf221c21d4dfe37075b5c508a8.setContent(html_75ed137c99d1680e50efb1c01569543d);\\n \\n \\n\\n circle_marker_11f37752c2007886d8d078b272b37b66.bindPopup(popup_ffe028bf221c21d4dfe37075b5c508a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7e67d6f52b791d0a463d28019d9fdd8 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a2706dd6e7a59cc1f10acd3934d4c704 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68b14e2105e02d84bc2a2d7c2758d82a = $(`<div id="html_68b14e2105e02d84bc2a2d7c2758d82a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a2706dd6e7a59cc1f10acd3934d4c704.setContent(html_68b14e2105e02d84bc2a2d7c2758d82a);\\n \\n \\n\\n circle_marker_b7e67d6f52b791d0a463d28019d9fdd8.bindPopup(popup_a2706dd6e7a59cc1f10acd3934d4c704)\\n ;\\n\\n \\n \\n \\n var circle_marker_8ee776a830c68add96d7c8ea62f0d9d7 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_7bebb95f3fdc97935b84f9324cb39b4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3787c87042a254c33c06ccd8fd53bcd5 = $(`<div id="html_3787c87042a254c33c06ccd8fd53bcd5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7bebb95f3fdc97935b84f9324cb39b4c.setContent(html_3787c87042a254c33c06ccd8fd53bcd5);\\n \\n \\n\\n circle_marker_8ee776a830c68add96d7c8ea62f0d9d7.bindPopup(popup_7bebb95f3fdc97935b84f9324cb39b4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5003c6b73f0949e5a83a8e8fc9a2014 = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_216c4126d566b23aa5e46154a2ab6363 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9270e0f26f494d2c7841e93ee226a803 = $(`<div id="html_9270e0f26f494d2c7841e93ee226a803" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_216c4126d566b23aa5e46154a2ab6363.setContent(html_9270e0f26f494d2c7841e93ee226a803);\\n \\n \\n\\n circle_marker_d5003c6b73f0949e5a83a8e8fc9a2014.bindPopup(popup_216c4126d566b23aa5e46154a2ab6363)\\n ;\\n\\n \\n \\n \\n var circle_marker_eaaae0e9de2e6d6e7917fdcd1b5d062a = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a332e0e4482f07f46c190b7746c98afa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c38f5d82aa23b1aec70bf56753d68ed5 = $(`<div id="html_c38f5d82aa23b1aec70bf56753d68ed5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a332e0e4482f07f46c190b7746c98afa.setContent(html_c38f5d82aa23b1aec70bf56753d68ed5);\\n \\n \\n\\n circle_marker_eaaae0e9de2e6d6e7917fdcd1b5d062a.bindPopup(popup_a332e0e4482f07f46c190b7746c98afa)\\n ;\\n\\n \\n \\n \\n var circle_marker_0161cf83ce394849cee92782075b05c1 = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_72e7877df861eb1a8a50f35eceb10f1e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1f4df4fc6e22cb16f668ca5bdfdb3b4 = $(`<div id="html_b1f4df4fc6e22cb16f668ca5bdfdb3b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72e7877df861eb1a8a50f35eceb10f1e.setContent(html_b1f4df4fc6e22cb16f668ca5bdfdb3b4);\\n \\n \\n\\n circle_marker_0161cf83ce394849cee92782075b05c1.bindPopup(popup_72e7877df861eb1a8a50f35eceb10f1e)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a7237a048d4eba98a7978dbb1ddd157 = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c88cf08791c4c66a2510df7e2f86b378 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ebcf2054a9246541ee1d2a676489babc = $(`<div id="html_ebcf2054a9246541ee1d2a676489babc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c88cf08791c4c66a2510df7e2f86b378.setContent(html_ebcf2054a9246541ee1d2a676489babc);\\n \\n \\n\\n circle_marker_9a7237a048d4eba98a7978dbb1ddd157.bindPopup(popup_c88cf08791c4c66a2510df7e2f86b378)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6984d9e54e2e953310fcd4f37800a4a = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_77af152bb27a71e7a93ad1e3f1a3d381 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4885fb9cd8548351eba1d773d4f66671 = $(`<div id="html_4885fb9cd8548351eba1d773d4f66671" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77af152bb27a71e7a93ad1e3f1a3d381.setContent(html_4885fb9cd8548351eba1d773d4f66671);\\n \\n \\n\\n circle_marker_d6984d9e54e2e953310fcd4f37800a4a.bindPopup(popup_77af152bb27a71e7a93ad1e3f1a3d381)\\n ;\\n\\n \\n \\n \\n var circle_marker_316888ae42578e8e96eaeb6bdb900be3 = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d8d50c179f3c20ed35004b08becbd5b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a14897fa26d0016ec59b0dcd53df3e5a = $(`<div id="html_a14897fa26d0016ec59b0dcd53df3e5a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8d50c179f3c20ed35004b08becbd5b4.setContent(html_a14897fa26d0016ec59b0dcd53df3e5a);\\n \\n \\n\\n circle_marker_316888ae42578e8e96eaeb6bdb900be3.bindPopup(popup_d8d50c179f3c20ed35004b08becbd5b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_440780b39624dc91ab9996f693abfc9f = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5ce5a490d491588c86e607da7bf02daf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_861b03a9749830525130a13f012f47bc = $(`<div id="html_861b03a9749830525130a13f012f47bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ce5a490d491588c86e607da7bf02daf.setContent(html_861b03a9749830525130a13f012f47bc);\\n \\n \\n\\n circle_marker_440780b39624dc91ab9996f693abfc9f.bindPopup(popup_5ce5a490d491588c86e607da7bf02daf)\\n ;\\n\\n \\n \\n \\n var circle_marker_cce2987973a3693285e3f080974be5a6 = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e6624e0c02592ee8ba48f2af34b8a376 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_709b7989fa24c938a31e1357dc17f16f = $(`<div id="html_709b7989fa24c938a31e1357dc17f16f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6624e0c02592ee8ba48f2af34b8a376.setContent(html_709b7989fa24c938a31e1357dc17f16f);\\n \\n \\n\\n circle_marker_cce2987973a3693285e3f080974be5a6.bindPopup(popup_e6624e0c02592ee8ba48f2af34b8a376)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d901d018cafae65b7c2c4a4fc8405ed = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2d7a675fefcfa74de0491a4d22c0c98e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_480fa719a40a2e756633676cf33b55af = $(`<div id="html_480fa719a40a2e756633676cf33b55af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d7a675fefcfa74de0491a4d22c0c98e.setContent(html_480fa719a40a2e756633676cf33b55af);\\n \\n \\n\\n circle_marker_2d901d018cafae65b7c2c4a4fc8405ed.bindPopup(popup_2d7a675fefcfa74de0491a4d22c0c98e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d3b4ee3cb2517ad2c67fbde3bb1d2e06 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1d4e3eda373d89c5d01d4b6753800d4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5e7c5dd3e2887ef647ee34e7ec610d52 = $(`<div id="html_5e7c5dd3e2887ef647ee34e7ec610d52" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d4e3eda373d89c5d01d4b6753800d4f.setContent(html_5e7c5dd3e2887ef647ee34e7ec610d52);\\n \\n \\n\\n circle_marker_d3b4ee3cb2517ad2c67fbde3bb1d2e06.bindPopup(popup_1d4e3eda373d89c5d01d4b6753800d4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1735b415c5e04466859a8c8e57551318 = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_642410c1368728cc6f40453d10ca7c68 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f2442400230b28a17fa4437039875d3 = $(`<div id="html_3f2442400230b28a17fa4437039875d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_642410c1368728cc6f40453d10ca7c68.setContent(html_3f2442400230b28a17fa4437039875d3);\\n \\n \\n\\n circle_marker_1735b415c5e04466859a8c8e57551318.bindPopup(popup_642410c1368728cc6f40453d10ca7c68)\\n ;\\n\\n \\n \\n \\n var circle_marker_d4264ffc999274125883ebb235481b53 = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.370193722368317, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f49f5bef1db6e865a850ef8bf750ad05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83f640279a5dc0e71e9ce3f9aca1bec8 = $(`<div id="html_83f640279a5dc0e71e9ce3f9aca1bec8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f49f5bef1db6e865a850ef8bf750ad05.setContent(html_83f640279a5dc0e71e9ce3f9aca1bec8);\\n \\n \\n\\n circle_marker_d4264ffc999274125883ebb235481b53.bindPopup(popup_f49f5bef1db6e865a850ef8bf750ad05)\\n ;\\n\\n \\n \\n \\n var circle_marker_0aa7ce0d8bb38df4016939c489c9a68a = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f99f48bd421f7f66abb015d0ce294f14 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0c2f51f8edd36e7a19956b03139b1bc = $(`<div id="html_c0c2f51f8edd36e7a19956b03139b1bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f99f48bd421f7f66abb015d0ce294f14.setContent(html_c0c2f51f8edd36e7a19956b03139b1bc);\\n \\n \\n\\n circle_marker_0aa7ce0d8bb38df4016939c489c9a68a.bindPopup(popup_f99f48bd421f7f66abb015d0ce294f14)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f93e63ef2167e6dd0a26a65c6e472a5 = L.circleMarker(\\n [42.73635845737364, -73.26364162994278],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_309ed5b2cdb98d1eac07af3eefba42fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb80a78f841975dedf505dce28e04294 = $(`<div id="html_eb80a78f841975dedf505dce28e04294" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_309ed5b2cdb98d1eac07af3eefba42fc.setContent(html_eb80a78f841975dedf505dce28e04294);\\n \\n \\n\\n circle_marker_2f93e63ef2167e6dd0a26a65c6e472a5.bindPopup(popup_309ed5b2cdb98d1eac07af3eefba42fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6e12d2db264374127fa03519784ad51 = L.circleMarker(\\n [42.73635843762231, -73.26241142403887],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_920b406e89963607b73603657f93c0b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14f80f1dfac7695166bf4fbb45ed835b = $(`<div id="html_14f80f1dfac7695166bf4fbb45ed835b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_920b406e89963607b73603657f93c0b2.setContent(html_14f80f1dfac7695166bf4fbb45ed835b);\\n \\n \\n\\n circle_marker_d6e12d2db264374127fa03519784ad51.bindPopup(popup_920b406e89963607b73603657f93c0b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_cbc9d0a9727eb0b7c918e7c6afeb2c88 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_52f44e80873d7618011ec3d824843360 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d19f04e6ca3346c43a942f3eac9bee4 = $(`<div id="html_3d19f04e6ca3346c43a942f3eac9bee4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52f44e80873d7618011ec3d824843360.setContent(html_3d19f04e6ca3346c43a942f3eac9bee4);\\n \\n \\n\\n circle_marker_cbc9d0a9727eb0b7c918e7c6afeb2c88.bindPopup(popup_52f44e80873d7618011ec3d824843360)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fd778236a699a1cd2e7192ddd657d07 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a2e4e347ca13e981961096f9b38e76c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_18e73cea56a038c082a12914ca1e6c5c = $(`<div id="html_18e73cea56a038c082a12914ca1e6c5c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a2e4e347ca13e981961096f9b38e76c0.setContent(html_18e73cea56a038c082a12914ca1e6c5c);\\n \\n \\n\\n circle_marker_9fd778236a699a1cd2e7192ddd657d07.bindPopup(popup_a2e4e347ca13e981961096f9b38e76c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_86ada2f0d60926797d5689bd137fd04c = L.circleMarker(\\n [42.73635829936299, -73.25872080633552],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c2d2a54b52b12d082164939fe29bd8b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5daddd0dd3ea423e332c3a78f4308d87 = $(`<div id="html_5daddd0dd3ea423e332c3a78f4308d87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2d2a54b52b12d082164939fe29bd8b5.setContent(html_5daddd0dd3ea423e332c3a78f4308d87);\\n \\n \\n\\n circle_marker_86ada2f0d60926797d5689bd137fd04c.bindPopup(popup_c2d2a54b52b12d082164939fe29bd8b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3f97dab4d3d1577c5090239027790f7 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e960be25560af418e1474852ef6099d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3afda4c38c8d10f97d4bf803929e79a = $(`<div id="html_e3afda4c38c8d10f97d4bf803929e79a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e960be25560af418e1474852ef6099d3.setContent(html_e3afda4c38c8d10f97d4bf803929e79a);\\n \\n \\n\\n circle_marker_a3f97dab4d3d1577c5090239027790f7.bindPopup(popup_e960be25560af418e1474852ef6099d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8005faee2575e06b57373480497f2eb = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_210490bfc20f71f2c39481aa0df0663b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68dbaad22ba45379aa64d77b0b8ccf7f = $(`<div id="html_68dbaad22ba45379aa64d77b0b8ccf7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_210490bfc20f71f2c39481aa0df0663b.setContent(html_68dbaad22ba45379aa64d77b0b8ccf7f);\\n \\n \\n\\n circle_marker_c8005faee2575e06b57373480497f2eb.bindPopup(popup_210490bfc20f71f2c39481aa0df0663b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c5f0a8fc4a4e222a739b55f22374512 = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8758750928991e2f02174ba3e439345d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_badfbcda733dd259caa85a982c29c6f5 = $(`<div id="html_badfbcda733dd259caa85a982c29c6f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8758750928991e2f02174ba3e439345d.setContent(html_badfbcda733dd259caa85a982c29c6f5);\\n \\n \\n\\n circle_marker_3c5f0a8fc4a4e222a739b55f22374512.bindPopup(popup_8758750928991e2f02174ba3e439345d)\\n ;\\n\\n \\n \\n \\n var circle_marker_56d606fd1c495e49c3741de8a50bedcc = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_215d1a45fb5ada68602fd045f430966d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_905c683d90e57b88f1e8bfd4ea707166 = $(`<div id="html_905c683d90e57b88f1e8bfd4ea707166" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_215d1a45fb5ada68602fd045f430966d.setContent(html_905c683d90e57b88f1e8bfd4ea707166);\\n \\n \\n\\n circle_marker_56d606fd1c495e49c3741de8a50bedcc.bindPopup(popup_215d1a45fb5ada68602fd045f430966d)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c744b2a27478d5c8902c930fc900ded = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_da303cb262209ec6da0b4fd9425e1315 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6da513023059a0bf150e5ec95ec24088 = $(`<div id="html_6da513023059a0bf150e5ec95ec24088" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_da303cb262209ec6da0b4fd9425e1315.setContent(html_6da513023059a0bf150e5ec95ec24088);\\n \\n \\n\\n circle_marker_2c744b2a27478d5c8902c930fc900ded.bindPopup(popup_da303cb262209ec6da0b4fd9425e1315)\\n ;\\n\\n \\n \\n \\n var circle_marker_0209b0816deef2fd696d0d6debd180bc = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_58c5800b73fb789449860f88b5673a83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c59a4bec490e47f9c4232d1734db0cdd = $(`<div id="html_c59a4bec490e47f9c4232d1734db0cdd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58c5800b73fb789449860f88b5673a83.setContent(html_c59a4bec490e47f9c4232d1734db0cdd);\\n \\n \\n\\n circle_marker_0209b0816deef2fd696d0d6debd180bc.bindPopup(popup_58c5800b73fb789449860f88b5673a83)\\n ;\\n\\n \\n \\n \\n var circle_marker_124ddb85f5cd3f4af146c72f670d5c9e = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d1e6a1e60eec55589c41445269c91312 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab03051fd30636093a8ad609b1aeb5d5 = $(`<div id="html_ab03051fd30636093a8ad609b1aeb5d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d1e6a1e60eec55589c41445269c91312.setContent(html_ab03051fd30636093a8ad609b1aeb5d5);\\n \\n \\n\\n circle_marker_124ddb85f5cd3f4af146c72f670d5c9e.bindPopup(popup_d1e6a1e60eec55589c41445269c91312)\\n ;\\n\\n \\n \\n \\n var circle_marker_29b5b1dc2868188aa30c5524fd8600cc = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8297886706635be929ba91725aa36420 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ae47d23cfa3a5cffd94251496c64c49 = $(`<div id="html_3ae47d23cfa3a5cffd94251496c64c49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8297886706635be929ba91725aa36420.setContent(html_3ae47d23cfa3a5cffd94251496c64c49);\\n \\n \\n\\n circle_marker_29b5b1dc2868188aa30c5524fd8600cc.bindPopup(popup_8297886706635be929ba91725aa36420)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3f56f215618c334a1f2efe2f3e94251 = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_64a701a9ec749d7723c0a9514706573b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0601a50932698808d2a038ef288d873d = $(`<div id="html_0601a50932698808d2a038ef288d873d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64a701a9ec749d7723c0a9514706573b.setContent(html_0601a50932698808d2a038ef288d873d);\\n \\n \\n\\n circle_marker_f3f56f215618c334a1f2efe2f3e94251.bindPopup(popup_64a701a9ec749d7723c0a9514706573b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b0dcfb23f73c0bde38ba9f6933c6c52 = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3070ef538dc761d6c0131a7c80efa670 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_809452d6df42afce38a742f7ecc5f246 = $(`<div id="html_809452d6df42afce38a742f7ecc5f246" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3070ef538dc761d6c0131a7c80efa670.setContent(html_809452d6df42afce38a742f7ecc5f246);\\n \\n \\n\\n circle_marker_9b0dcfb23f73c0bde38ba9f6933c6c52.bindPopup(popup_3070ef538dc761d6c0131a7c80efa670)\\n ;\\n\\n \\n \\n \\n var circle_marker_66194c8a14120dd343aac8e91f837139 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_eca29a44d73fba6586f68984543e9f51 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14d1b8061599f6b1409164f125654c8d = $(`<div id="html_14d1b8061599f6b1409164f125654c8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eca29a44d73fba6586f68984543e9f51.setContent(html_14d1b8061599f6b1409164f125654c8d);\\n \\n \\n\\n circle_marker_66194c8a14120dd343aac8e91f837139.bindPopup(popup_eca29a44d73fba6586f68984543e9f51)\\n ;\\n\\n \\n \\n \\n var circle_marker_d320461ff8f654586b35d871ed3082de = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2edbad6e17f96ae699f3523e4bd65ded = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9a75abf4c68825ea9037fdcf8c32600 = $(`<div id="html_d9a75abf4c68825ea9037fdcf8c32600" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2edbad6e17f96ae699f3523e4bd65ded.setContent(html_d9a75abf4c68825ea9037fdcf8c32600);\\n \\n \\n\\n circle_marker_d320461ff8f654586b35d871ed3082de.bindPopup(popup_2edbad6e17f96ae699f3523e4bd65ded)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a0cdb80cb3b2f9cd1827ddf7becdfd7 = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_387892dacc2ecfef79a2f84a7b8bb8a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b0b15022947ea8d69e8820f3dd5a355 = $(`<div id="html_4b0b15022947ea8d69e8820f3dd5a355" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_387892dacc2ecfef79a2f84a7b8bb8a0.setContent(html_4b0b15022947ea8d69e8820f3dd5a355);\\n \\n \\n\\n circle_marker_8a0cdb80cb3b2f9cd1827ddf7becdfd7.bindPopup(popup_387892dacc2ecfef79a2f84a7b8bb8a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_6aeafdc0854c56369367ec2dda3ff462 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5f117cb1aa9aa9ce8b3ac898bacb5c08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92f23f3884040857deaad66e292571e6 = $(`<div id="html_92f23f3884040857deaad66e292571e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f117cb1aa9aa9ce8b3ac898bacb5c08.setContent(html_92f23f3884040857deaad66e292571e6);\\n \\n \\n\\n circle_marker_6aeafdc0854c56369367ec2dda3ff462.bindPopup(popup_5f117cb1aa9aa9ce8b3ac898bacb5c08)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ed02ab1caf0e27041bd60a2a983652e = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_75b286978a778d15f051cee218e4ecd2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3092ad0a3f5ee194247cb342fc8437e5 = $(`<div id="html_3092ad0a3f5ee194247cb342fc8437e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75b286978a778d15f051cee218e4ecd2.setContent(html_3092ad0a3f5ee194247cb342fc8437e5);\\n \\n \\n\\n circle_marker_1ed02ab1caf0e27041bd60a2a983652e.bindPopup(popup_75b286978a778d15f051cee218e4ecd2)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7faaa7195872deb7626f4728a2add61 = L.circleMarker(\\n [42.734551330583365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3a7af3bf7b62c342e358f1c36e6274fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c44bd543ce53ad22ac6b800be6143049 = $(`<div id="html_c44bd543ce53ad22ac6b800be6143049" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a7af3bf7b62c342e358f1c36e6274fc.setContent(html_c44bd543ce53ad22ac6b800be6143049);\\n \\n \\n\\n circle_marker_e7faaa7195872deb7626f4728a2add61.bindPopup(popup_3a7af3bf7b62c342e358f1c36e6274fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_1552429008c8470cf8e5a938b7a15a84 = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_850de907bf5f55a1f01d2d54004a5ccd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_794d933cbfe6d0d9e1bf4fc6faeb02fe = $(`<div id="html_794d933cbfe6d0d9e1bf4fc6faeb02fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_850de907bf5f55a1f01d2d54004a5ccd.setContent(html_794d933cbfe6d0d9e1bf4fc6faeb02fe);\\n \\n \\n\\n circle_marker_1552429008c8470cf8e5a938b7a15a84.bindPopup(popup_850de907bf5f55a1f01d2d54004a5ccd)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b8ba5fdd72db86470fea21b61a413e3 = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e3c9c59870380d180c7faed13f52a215 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19bc4c6d44759279dbff3f078bcf32d8 = $(`<div id="html_19bc4c6d44759279dbff3f078bcf32d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3c9c59870380d180c7faed13f52a215.setContent(html_19bc4c6d44759279dbff3f078bcf32d8);\\n \\n \\n\\n circle_marker_2b8ba5fdd72db86470fea21b61a413e3.bindPopup(popup_e3c9c59870380d180c7faed13f52a215)\\n ;\\n\\n \\n \\n \\n var circle_marker_33cbab477ac7d8a43a45df2f0404513a = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ee1b895b91225e5753094a2c6672cced = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd2b7f029a04e454b48348bd5b632b65 = $(`<div id="html_bd2b7f029a04e454b48348bd5b632b65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee1b895b91225e5753094a2c6672cced.setContent(html_bd2b7f029a04e454b48348bd5b632b65);\\n \\n \\n\\n circle_marker_33cbab477ac7d8a43a45df2f0404513a.bindPopup(popup_ee1b895b91225e5753094a2c6672cced)\\n ;\\n\\n \\n \\n \\n var circle_marker_b2843859bd60a0f0988dbb3f9821c125 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1a14b64f150583833b8795e89e2da158 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3e9d8c731fa33c3391c8341c0cf3b500 = $(`<div id="html_3e9d8c731fa33c3391c8341c0cf3b500" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a14b64f150583833b8795e89e2da158.setContent(html_3e9d8c731fa33c3391c8341c0cf3b500);\\n \\n \\n\\n circle_marker_b2843859bd60a0f0988dbb3f9821c125.bindPopup(popup_1a14b64f150583833b8795e89e2da158)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd7abc98a60e623f44f5267b4aa97196 = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5ae7827fc9fafa2fb75b7e5429d1ae6d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f5aff634a7c9f844854f3ed722f9d1bc = $(`<div id="html_f5aff634a7c9f844854f3ed722f9d1bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ae7827fc9fafa2fb75b7e5429d1ae6d.setContent(html_f5aff634a7c9f844854f3ed722f9d1bc);\\n \\n \\n\\n circle_marker_dd7abc98a60e623f44f5267b4aa97196.bindPopup(popup_5ae7827fc9fafa2fb75b7e5429d1ae6d)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e3590eb9e94adbb9eaa6edf56ee22b6 = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6eeef318abc730cf7ca7f1b2647ef622 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c16e5069c2d822372ab41dce477b4fbe = $(`<div id="html_c16e5069c2d822372ab41dce477b4fbe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6eeef318abc730cf7ca7f1b2647ef622.setContent(html_c16e5069c2d822372ab41dce477b4fbe);\\n \\n \\n\\n circle_marker_8e3590eb9e94adbb9eaa6edf56ee22b6.bindPopup(popup_6eeef318abc730cf7ca7f1b2647ef622)\\n ;\\n\\n \\n \\n \\n var circle_marker_872638298ed4e9e9a549cdec2f03c4d6 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_03efa26ccf5af62c3c3d31e004397017 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_153af78fce65965f7eaddbcaa218f90d = $(`<div id="html_153af78fce65965f7eaddbcaa218f90d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03efa26ccf5af62c3c3d31e004397017.setContent(html_153af78fce65965f7eaddbcaa218f90d);\\n \\n \\n\\n circle_marker_872638298ed4e9e9a549cdec2f03c4d6.bindPopup(popup_03efa26ccf5af62c3c3d31e004397017)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e0ae4364f2b6460954896624708623b = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1f13d854670f2d4845b6a8361be2c624 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d271885a9c6932016e8ea4aa3e2145ef = $(`<div id="html_d271885a9c6932016e8ea4aa3e2145ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f13d854670f2d4845b6a8361be2c624.setContent(html_d271885a9c6932016e8ea4aa3e2145ef);\\n \\n \\n\\n circle_marker_6e0ae4364f2b6460954896624708623b.bindPopup(popup_1f13d854670f2d4845b6a8361be2c624)\\n ;\\n\\n \\n \\n \\n var circle_marker_47cb527dbcc125fec6fe336f4da0d5da = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e9370260959d847ae6e15521006ba0a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b13d745fad816f82ba78dee7678561f = $(`<div id="html_4b13d745fad816f82ba78dee7678561f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9370260959d847ae6e15521006ba0a6.setContent(html_4b13d745fad816f82ba78dee7678561f);\\n \\n \\n\\n circle_marker_47cb527dbcc125fec6fe336f4da0d5da.bindPopup(popup_e9370260959d847ae6e15521006ba0a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e02d928125ba52b42bd96a713121277 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a9c9c3216abfd6cfbde0007f1c236196 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09b11ecc5a74fe058056348d23ef82dd = $(`<div id="html_09b11ecc5a74fe058056348d23ef82dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9c9c3216abfd6cfbde0007f1c236196.setContent(html_09b11ecc5a74fe058056348d23ef82dd);\\n \\n \\n\\n circle_marker_1e02d928125ba52b42bd96a713121277.bindPopup(popup_a9c9c3216abfd6cfbde0007f1c236196)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d0330976e85f6ed57e0c4d5235e63eb = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8b202854705020499fac86db33595251 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_130d3909a9d2d2992f2d5bda10e876a1 = $(`<div id="html_130d3909a9d2d2992f2d5bda10e876a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b202854705020499fac86db33595251.setContent(html_130d3909a9d2d2992f2d5bda10e876a1);\\n \\n \\n\\n circle_marker_1d0330976e85f6ed57e0c4d5235e63eb.bindPopup(popup_8b202854705020499fac86db33595251)\\n ;\\n\\n \\n \\n \\n var circle_marker_101aaf73a8ee571ed119357f535c6149 = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0c0f9db605c64a57c4cde99a87847559 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ea71d25a307466bc0c8018429a1bfdc = $(`<div id="html_5ea71d25a307466bc0c8018429a1bfdc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c0f9db605c64a57c4cde99a87847559.setContent(html_5ea71d25a307466bc0c8018429a1bfdc);\\n \\n \\n\\n circle_marker_101aaf73a8ee571ed119357f535c6149.bindPopup(popup_0c0f9db605c64a57c4cde99a87847559)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcc125ed01eb6e1b529613a2d2157b3e = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_746951be6e64249d4340dbd352c46689 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83fb6762b7f94ca565c248353fc00f34 = $(`<div id="html_83fb6762b7f94ca565c248353fc00f34" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_746951be6e64249d4340dbd352c46689.setContent(html_83fb6762b7f94ca565c248353fc00f34);\\n \\n \\n\\n circle_marker_bcc125ed01eb6e1b529613a2d2157b3e.bindPopup(popup_746951be6e64249d4340dbd352c46689)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7545dd8db1e4bfa26ed74a250d04733 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_4b9d4924d1597bb05057e1877c26eb6b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d582f14ca1c7c6fe4cf6c8f6b4d45216 = $(`<div id="html_d582f14ca1c7c6fe4cf6c8f6b4d45216" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4b9d4924d1597bb05057e1877c26eb6b.setContent(html_d582f14ca1c7c6fe4cf6c8f6b4d45216);\\n \\n \\n\\n circle_marker_f7545dd8db1e4bfa26ed74a250d04733.bindPopup(popup_4b9d4924d1597bb05057e1877c26eb6b)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c1799644cc9bc5f38f14c69dfb6c1af = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_707b87d11014145b15337eb12cc056a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_308b319774469a5f67693fe89bdb046c = $(`<div id="html_308b319774469a5f67693fe89bdb046c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_707b87d11014145b15337eb12cc056a9.setContent(html_308b319774469a5f67693fe89bdb046c);\\n \\n \\n\\n circle_marker_2c1799644cc9bc5f38f14c69dfb6c1af.bindPopup(popup_707b87d11014145b15337eb12cc056a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb46ad9d5e61fb4f250db34b96422d92 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2885bf995c99818b67c1a7260e82a234 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e80d0817f9efc51aba091a58ec20d93 = $(`<div id="html_8e80d0817f9efc51aba091a58ec20d93" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2885bf995c99818b67c1a7260e82a234.setContent(html_8e80d0817f9efc51aba091a58ec20d93);\\n \\n \\n\\n circle_marker_cb46ad9d5e61fb4f250db34b96422d92.bindPopup(popup_2885bf995c99818b67c1a7260e82a234)\\n ;\\n\\n \\n \\n \\n var circle_marker_b732d6f4462c6befa5b079d0724c3547 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3699333068c716d7381c56486ba29eb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7061211bf5ee92c4fdfceb6632730dcf = $(`<div id="html_7061211bf5ee92c4fdfceb6632730dcf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3699333068c716d7381c56486ba29eb2.setContent(html_7061211bf5ee92c4fdfceb6632730dcf);\\n \\n \\n\\n circle_marker_b732d6f4462c6befa5b079d0724c3547.bindPopup(popup_3699333068c716d7381c56486ba29eb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a3d8a8fddf36a01751ea6e7969a2e9b = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9fd91f87fc36daf1eecb27c6da7625db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44af92148360e66e37e494bbf5c06980 = $(`<div id="html_44af92148360e66e37e494bbf5c06980" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fd91f87fc36daf1eecb27c6da7625db.setContent(html_44af92148360e66e37e494bbf5c06980);\\n \\n \\n\\n circle_marker_0a3d8a8fddf36a01751ea6e7969a2e9b.bindPopup(popup_9fd91f87fc36daf1eecb27c6da7625db)\\n ;\\n\\n \\n \\n \\n var circle_marker_c78a1c0ac54006164b86be33a061bc68 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_4dafe05016e7bbd7171e32e0abc864cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fde0a7fa3f14441ed8ec75ce426958c7 = $(`<div id="html_fde0a7fa3f14441ed8ec75ce426958c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4dafe05016e7bbd7171e32e0abc864cd.setContent(html_fde0a7fa3f14441ed8ec75ce426958c7);\\n \\n \\n\\n circle_marker_c78a1c0ac54006164b86be33a061bc68.bindPopup(popup_4dafe05016e7bbd7171e32e0abc864cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_334302f4eff6a4a204f8a2bb4985e931 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c6dffdd21689a6e600068b32c40ebed9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c7d4d3980ee5d3efd9156244332f4e1 = $(`<div id="html_9c7d4d3980ee5d3efd9156244332f4e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6dffdd21689a6e600068b32c40ebed9.setContent(html_9c7d4d3980ee5d3efd9156244332f4e1);\\n \\n \\n\\n circle_marker_334302f4eff6a4a204f8a2bb4985e931.bindPopup(popup_c6dffdd21689a6e600068b32c40ebed9)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c56c5b92c1f06722006b11c41661c0e = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b87ce9f3d42f2623b420e6e76505928a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec8b5c2389d09d45fb7a5391745f44d0 = $(`<div id="html_ec8b5c2389d09d45fb7a5391745f44d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b87ce9f3d42f2623b420e6e76505928a.setContent(html_ec8b5c2389d09d45fb7a5391745f44d0);\\n \\n \\n\\n circle_marker_6c56c5b92c1f06722006b11c41661c0e.bindPopup(popup_b87ce9f3d42f2623b420e6e76505928a)\\n ;\\n\\n \\n \\n \\n var circle_marker_503242098674b35590679a56d6c57870 = L.circleMarker(\\n [42.73274419062606, -73.26364170164257],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d0d3740427f6dedbba124bfc321d918d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f5f9a3a9492fa027f9c5b8a24e1635f4 = $(`<div id="html_f5f9a3a9492fa027f9c5b8a24e1635f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0d3740427f6dedbba124bfc321d918d.setContent(html_f5f9a3a9492fa027f9c5b8a24e1635f4);\\n \\n \\n\\n circle_marker_503242098674b35590679a56d6c57870.bindPopup(popup_d0d3740427f6dedbba124bfc321d918d)\\n ;\\n\\n \\n \\n \\n var circle_marker_f2fbc028b0ecfff11509c0fa855bfb84 = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_827f038c6e947f9250d88cabdc1c6f8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d07acb5e40c9dade378ae067241bfd1b = $(`<div id="html_d07acb5e40c9dade378ae067241bfd1b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_827f038c6e947f9250d88cabdc1c6f8b.setContent(html_d07acb5e40c9dade378ae067241bfd1b);\\n \\n \\n\\n circle_marker_f2fbc028b0ecfff11509c0fa855bfb84.bindPopup(popup_827f038c6e947f9250d88cabdc1c6f8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_a16a85d8d26fff97f2ee8dbadc240bea = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9993a4fb05cff742caee83a4eca0639f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4110031e0c377ba4e386362f83147775 = $(`<div id="html_4110031e0c377ba4e386362f83147775" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9993a4fb05cff742caee83a4eca0639f.setContent(html_4110031e0c377ba4e386362f83147775);\\n \\n \\n\\n circle_marker_a16a85d8d26fff97f2ee8dbadc240bea.bindPopup(popup_9993a4fb05cff742caee83a4eca0639f)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e85f4aec8fb43f362e72fecc23c9dc5 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6a16fadbf2fb35b453edb037ca124375 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d74d5b5bc614e0d858f284699abf4c0f = $(`<div id="html_d74d5b5bc614e0d858f284699abf4c0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a16fadbf2fb35b453edb037ca124375.setContent(html_d74d5b5bc614e0d858f284699abf4c0f);\\n \\n \\n\\n circle_marker_9e85f4aec8fb43f362e72fecc23c9dc5.bindPopup(popup_6a16fadbf2fb35b453edb037ca124375)\\n ;\\n\\n \\n \\n \\n var circle_marker_b71d0a57e1c8fcd6f0a96d204caa8f84 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_df1931c4aac493df00f8d9252096bc80 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3cb12b57f74ebc62cce9b08e39bc709 = $(`<div id="html_a3cb12b57f74ebc62cce9b08e39bc709" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df1931c4aac493df00f8d9252096bc80.setContent(html_a3cb12b57f74ebc62cce9b08e39bc709);\\n \\n \\n\\n circle_marker_b71d0a57e1c8fcd6f0a96d204caa8f84.bindPopup(popup_df1931c4aac493df00f8d9252096bc80)\\n ;\\n\\n \\n \\n \\n var circle_marker_50bcb3db347c9bbb3581b19cc9b37f69 = L.circleMarker(\\n [42.73274377588172, -73.25503076225394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_73fb1e521109219e0122acea04f06d13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3da6f0fe9a7454a44088161f6f0ca10f = $(`<div id="html_3da6f0fe9a7454a44088161f6f0ca10f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73fb1e521109219e0122acea04f06d13.setContent(html_3da6f0fe9a7454a44088161f6f0ca10f);\\n \\n \\n\\n circle_marker_50bcb3db347c9bbb3581b19cc9b37f69.bindPopup(popup_73fb1e521109219e0122acea04f06d13)\\n ;\\n\\n \\n \\n \\n var circle_marker_bc66473ed6558998340279a4b3730627 = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1f38ac0ec050900cb78730f19f7eeaf8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b88d2db43987bb1b1e37c2ba93693bb = $(`<div id="html_3b88d2db43987bb1b1e37c2ba93693bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f38ac0ec050900cb78730f19f7eeaf8.setContent(html_3b88d2db43987bb1b1e37c2ba93693bb);\\n \\n \\n\\n circle_marker_bc66473ed6558998340279a4b3730627.bindPopup(popup_1f38ac0ec050900cb78730f19f7eeaf8)\\n ;\\n\\n \\n \\n \\n var circle_marker_49d8cfd4bd3f7ea9ad87609d6a871cbb = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ac3cae2d52d2170423b5d764ce056e00 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5462c42f68fd80a57ae1fcc9fc684e09 = $(`<div id="html_5462c42f68fd80a57ae1fcc9fc684e09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac3cae2d52d2170423b5d764ce056e00.setContent(html_5462c42f68fd80a57ae1fcc9fc684e09);\\n \\n \\n\\n circle_marker_49d8cfd4bd3f7ea9ad87609d6a871cbb.bindPopup(popup_ac3cae2d52d2170423b5d764ce056e00)\\n ;\\n\\n \\n \\n \\n var circle_marker_06c05c96a4655ae19a98d41f957d790a = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_99518bf7c18bb5fca06b5a4764e371e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3335b3a706d73c3ef59e41db7a927665 = $(`<div id="html_3335b3a706d73c3ef59e41db7a927665" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99518bf7c18bb5fca06b5a4764e371e1.setContent(html_3335b3a706d73c3ef59e41db7a927665);\\n \\n \\n\\n circle_marker_06c05c96a4655ae19a98d41f957d790a.bindPopup(popup_99518bf7c18bb5fca06b5a4764e371e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_870fa451a391841b021d92b409b2cba8 = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5e5e5a8388744e8ceae0119aae9fe6c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da010bc3f66c667cbf987b1a508b3216 = $(`<div id="html_da010bc3f66c667cbf987b1a508b3216" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e5e5a8388744e8ceae0119aae9fe6c1.setContent(html_da010bc3f66c667cbf987b1a508b3216);\\n \\n \\n\\n circle_marker_870fa451a391841b021d92b409b2cba8.bindPopup(popup_5e5e5a8388744e8ceae0119aae9fe6c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_13a9ac580f31b2b58ece11e1868378fa = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5e650f91052a27ef9740a80c30408567 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26d96fa8ced4122ded684fa39d45eb6e = $(`<div id="html_26d96fa8ced4122ded684fa39d45eb6e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e650f91052a27ef9740a80c30408567.setContent(html_26d96fa8ced4122ded684fa39d45eb6e);\\n \\n \\n\\n circle_marker_13a9ac580f31b2b58ece11e1868378fa.bindPopup(popup_5e650f91052a27ef9740a80c30408567)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d8a39d49ccde5c334548030cdb52ea9 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3e2dbe71b7fe04bd8cd60e72b722140d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f3c3acadb37173e93658014dabeb3e3 = $(`<div id="html_4f3c3acadb37173e93658014dabeb3e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e2dbe71b7fe04bd8cd60e72b722140d.setContent(html_4f3c3acadb37173e93658014dabeb3e3);\\n \\n \\n\\n circle_marker_1d8a39d49ccde5c334548030cdb52ea9.bindPopup(popup_3e2dbe71b7fe04bd8cd60e72b722140d)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4ebae2651ee65d16192b48304c6d476 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_caf2d66555e0d941f96d11cbcb0fbfaf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_070aa7943817020f80b776ecb085cd76 = $(`<div id="html_070aa7943817020f80b776ecb085cd76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_caf2d66555e0d941f96d11cbcb0fbfaf.setContent(html_070aa7943817020f80b776ecb085cd76);\\n \\n \\n\\n circle_marker_f4ebae2651ee65d16192b48304c6d476.bindPopup(popup_caf2d66555e0d941f96d11cbcb0fbfaf)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4b538ae645930c8c4ec056ac46e64a2 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_fc151f7d567de8e431db863fc520ab85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5efda73ebbd02b533e739288343f173 = $(`<div id="html_e5efda73ebbd02b533e739288343f173" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc151f7d567de8e431db863fc520ab85.setContent(html_e5efda73ebbd02b533e739288343f173);\\n \\n \\n\\n circle_marker_e4b538ae645930c8c4ec056ac46e64a2.bindPopup(popup_fc151f7d567de8e431db863fc520ab85)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ecbd0493f34f761fdde447ed6f0eef9 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1b81614d2521f653fc6be1fff44e434a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e45250b4a31da10ed8a052b6d609f6bc = $(`<div id="html_e45250b4a31da10ed8a052b6d609f6bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b81614d2521f653fc6be1fff44e434a.setContent(html_e45250b4a31da10ed8a052b6d609f6bc);\\n \\n \\n\\n circle_marker_9ecbd0493f34f761fdde447ed6f0eef9.bindPopup(popup_1b81614d2521f653fc6be1fff44e434a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5cfc32d64810d5e4f6c07d730e92b495 = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_45c33c792a3944a061b00295e7a6b5f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e74a624f1f7cb82981a7d8ee6267f43 = $(`<div id="html_6e74a624f1f7cb82981a7d8ee6267f43" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_45c33c792a3944a061b00295e7a6b5f1.setContent(html_6e74a624f1f7cb82981a7d8ee6267f43);\\n \\n \\n\\n circle_marker_5cfc32d64810d5e4f6c07d730e92b495.bindPopup(popup_45c33c792a3944a061b00295e7a6b5f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c19985c010d63083fb898090a868946 = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6a96e3b567d4205e4fa4bd61e6222ce7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b0ae770770e9d9839dc3ca34543e1a3 = $(`<div id="html_4b0ae770770e9d9839dc3ca34543e1a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a96e3b567d4205e4fa4bd61e6222ce7.setContent(html_4b0ae770770e9d9839dc3ca34543e1a3);\\n \\n \\n\\n circle_marker_0c19985c010d63083fb898090a868946.bindPopup(popup_6a96e3b567d4205e4fa4bd61e6222ce7)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7e377fd11034761a771ffcb95ddcd28 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d5f452483fb28b8ee4935c193d3153bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a0c608abf49b6d0faa84e44e976f35e1 = $(`<div id="html_a0c608abf49b6d0faa84e44e976f35e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5f452483fb28b8ee4935c193d3153bd.setContent(html_a0c608abf49b6d0faa84e44e976f35e1);\\n \\n \\n\\n circle_marker_f7e377fd11034761a771ffcb95ddcd28.bindPopup(popup_d5f452483fb28b8ee4935c193d3153bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7d8b240926c2fbf4bd4134e451efdef = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e09f4a55c7e2b734982be614a62dc966 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04e2b71def35f5921f178003d00508ba = $(`<div id="html_04e2b71def35f5921f178003d00508ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e09f4a55c7e2b734982be614a62dc966.setContent(html_04e2b71def35f5921f178003d00508ba);\\n \\n \\n\\n circle_marker_e7d8b240926c2fbf4bd4134e451efdef.bindPopup(popup_e09f4a55c7e2b734982be614a62dc966)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf1901eceb9df3e9dcadd624b2704d18 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2c460bf6d2a455e238a805fbec5cca55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_366a03355e4b26e4427ba31a1e16fde7 = $(`<div id="html_366a03355e4b26e4427ba31a1e16fde7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c460bf6d2a455e238a805fbec5cca55.setContent(html_366a03355e4b26e4427ba31a1e16fde7);\\n \\n \\n\\n circle_marker_cf1901eceb9df3e9dcadd624b2704d18.bindPopup(popup_2c460bf6d2a455e238a805fbec5cca55)\\n ;\\n\\n \\n \\n \\n var circle_marker_b51ef1e3d63d1a3b6e0b10667ea89a79 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_39568371cfd12df901426035e1617ad4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d95f9ec6bd1fea0a4db265420e073a6 = $(`<div id="html_8d95f9ec6bd1fea0a4db265420e073a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39568371cfd12df901426035e1617ad4.setContent(html_8d95f9ec6bd1fea0a4db265420e073a6);\\n \\n \\n\\n circle_marker_b51ef1e3d63d1a3b6e0b10667ea89a79.bindPopup(popup_39568371cfd12df901426035e1617ad4)\\n ;\\n\\n \\n \\n \\n var circle_marker_8302fce63c766965a258f863df69b5c6 = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_25bc502f956cd2ae570e6fac8043374c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff26d1f7aeed4ba5736e1e7f2af6cb8a = $(`<div id="html_ff26d1f7aeed4ba5736e1e7f2af6cb8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_25bc502f956cd2ae570e6fac8043374c.setContent(html_ff26d1f7aeed4ba5736e1e7f2af6cb8a);\\n \\n \\n\\n circle_marker_8302fce63c766965a258f863df69b5c6.bindPopup(popup_25bc502f956cd2ae570e6fac8043374c)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f1e648d2e744356fbc51215937406c5 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_af720d0bb1b2493b1f054b69e3ad297d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fac05eecfe6a715108cc6b834d168c5a = $(`<div id="html_fac05eecfe6a715108cc6b834d168c5a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af720d0bb1b2493b1f054b69e3ad297d.setContent(html_fac05eecfe6a715108cc6b834d168c5a);\\n \\n \\n\\n circle_marker_5f1e648d2e744356fbc51215937406c5.bindPopup(popup_af720d0bb1b2493b1f054b69e3ad297d)\\n ;\\n\\n \\n \\n \\n var circle_marker_35a96463397340c56f55bbd12d7f0e06 = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_23a07f0c3437133b3c8790f0f4622829 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9ea25ad0e8e1d7c3e8c21ed33826bad = $(`<div id="html_f9ea25ad0e8e1d7c3e8c21ed33826bad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23a07f0c3437133b3c8790f0f4622829.setContent(html_f9ea25ad0e8e1d7c3e8c21ed33826bad);\\n \\n \\n\\n circle_marker_35a96463397340c56f55bbd12d7f0e06.bindPopup(popup_23a07f0c3437133b3c8790f0f4622829)\\n ;\\n\\n \\n \\n \\n var circle_marker_afa959f89653b36f78cf973e2af69c1a = L.circleMarker(\\n [42.73093703750333, -73.26733203256491],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8f8e374f8ca26ef3d2a654b0be7a498b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39a04f4c032edce493e9740a9235df9a = $(`<div id="html_39a04f4c032edce493e9740a9235df9a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f8e374f8ca26ef3d2a654b0be7a498b.setContent(html_39a04f4c032edce493e9740a9235df9a);\\n \\n \\n\\n circle_marker_afa959f89653b36f78cf973e2af69c1a.bindPopup(popup_8f8e374f8ca26ef3d2a654b0be7a498b)\\n ;\\n\\n \\n \\n \\n var circle_marker_8cbe5e642ba771c1bce315827b3ab464 = L.circleMarker(\\n [42.73093703750333, -73.26241163912951],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d5589cf495c5c3f05d127af8a167bf5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cee1c68e48b4f2992c8f9fd2c6c087fd = $(`<div id="html_cee1c68e48b4f2992c8f9fd2c6c087fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5589cf495c5c3f05d127af8a167bf5c.setContent(html_cee1c68e48b4f2992c8f9fd2c6c087fd);\\n \\n \\n\\n circle_marker_8cbe5e642ba771c1bce315827b3ab464.bindPopup(popup_d5589cf495c5c3f05d127af8a167bf5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_475439d9c4f1717e8e974e2fc09ee3cc = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8343c42ac3e1ec9b532d51244fd7bf3b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2ac8d1fe6ea59641c9ac15e265bbb15b = $(`<div id="html_2ac8d1fe6ea59641c9ac15e265bbb15b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8343c42ac3e1ec9b532d51244fd7bf3b.setContent(html_2ac8d1fe6ea59641c9ac15e265bbb15b);\\n \\n \\n\\n circle_marker_475439d9c4f1717e8e974e2fc09ee3cc.bindPopup(popup_8343c42ac3e1ec9b532d51244fd7bf3b)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa200268982cad63b4c6f4ae6c33954d = L.circleMarker(\\n [42.730936958507606, -73.259951442416],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1c360f8789a8157b4b8fe5163de6e957 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab29c8fdcf94fadca6ab1dfc472aa1e9 = $(`<div id="html_ab29c8fdcf94fadca6ab1dfc472aa1e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1c360f8789a8157b4b8fe5163de6e957.setContent(html_ab29c8fdcf94fadca6ab1dfc472aa1e9);\\n \\n \\n\\n circle_marker_aa200268982cad63b4c6f4ae6c33954d.bindPopup(popup_1c360f8789a8157b4b8fe5163de6e957)\\n ;\\n\\n \\n \\n \\n var circle_marker_afe6e37799b92fdd2f045c44a5eceb3e = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2c8fe48b6e4bb934f4f01e928ba8cdd6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e1a4bb9f642e983dbc9f3b69ece06446 = $(`<div id="html_e1a4bb9f642e983dbc9f3b69ece06446" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c8fe48b6e4bb934f4f01e928ba8cdd6.setContent(html_e1a4bb9f642e983dbc9f3b69ece06446);\\n \\n \\n\\n circle_marker_afe6e37799b92fdd2f045c44a5eceb3e.bindPopup(popup_2c8fe48b6e4bb934f4f01e928ba8cdd6)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ae146fbb9a0fab3ef098b2ad1a5e951 = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a8154a067edf6be82313594cd95983c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d7ed6470e93e92b11b1940375ae083b = $(`<div id="html_8d7ed6470e93e92b11b1940375ae083b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8154a067edf6be82313594cd95983c6.setContent(html_8d7ed6470e93e92b11b1940375ae083b);\\n \\n \\n\\n circle_marker_1ae146fbb9a0fab3ef098b2ad1a5e951.bindPopup(popup_a8154a067edf6be82313594cd95983c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_1be8508647c8c5a2005bf63ad6e5236a = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_34993b81e80fd1433ec243cac40b411d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c633aa51eacca4dba058d00b1b5d1c5f = $(`<div id="html_c633aa51eacca4dba058d00b1b5d1c5f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_34993b81e80fd1433ec243cac40b411d.setContent(html_c633aa51eacca4dba058d00b1b5d1c5f);\\n \\n \\n\\n circle_marker_1be8508647c8c5a2005bf63ad6e5236a.bindPopup(popup_34993b81e80fd1433ec243cac40b411d)\\n ;\\n\\n \\n \\n \\n var circle_marker_144d044beeb00e387f8c79250c6630fd = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_08089e23ba102e57719ee199a7757592 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed56c240101d164a7aea5b1aed06688a = $(`<div id="html_ed56c240101d164a7aea5b1aed06688a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_08089e23ba102e57719ee199a7757592.setContent(html_ed56c240101d164a7aea5b1aed06688a);\\n \\n \\n\\n circle_marker_144d044beeb00e387f8c79250c6630fd.bindPopup(popup_08089e23ba102e57719ee199a7757592)\\n ;\\n\\n \\n \\n \\n var circle_marker_1396a7075679164c1ce0acbca2f0e0c6 = L.circleMarker(\\n [42.73093640553757, -73.2525708523423],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_db5b552ab90483d1b9f053d67441b974 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_791d4eb68ac80509538f5b4719ca89b2 = $(`<div id="html_791d4eb68ac80509538f5b4719ca89b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db5b552ab90483d1b9f053d67441b974.setContent(html_791d4eb68ac80509538f5b4719ca89b2);\\n \\n \\n\\n circle_marker_1396a7075679164c1ce0acbca2f0e0c6.bindPopup(popup_db5b552ab90483d1b9f053d67441b974)\\n ;\\n\\n \\n \\n \\n var circle_marker_f977bf2ec18e180a15b9ec1c9814ddf2 = L.circleMarker(\\n [42.73093626729506, -73.25134075401192],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b3b8c40de264b0a0037421e448832ff6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7dcd4c2c2bd7068bac373057dfbb13cb = $(`<div id="html_7dcd4c2c2bd7068bac373057dfbb13cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3b8c40de264b0a0037421e448832ff6.setContent(html_7dcd4c2c2bd7068bac373057dfbb13cb);\\n \\n \\n\\n circle_marker_f977bf2ec18e180a15b9ec1c9814ddf2.bindPopup(popup_b3b8c40de264b0a0037421e448832ff6)\\n ;\\n\\n \\n \\n \\n var circle_marker_c73dd6d814f769bf6d860bd7278713f5 = L.circleMarker(\\n [42.73093611588659, -73.2501106556873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a7c449d4fadbe93f1297972da2044209 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2413fdc6d5eb19809cf8bc6b4d40f912 = $(`<div id="html_2413fdc6d5eb19809cf8bc6b4d40f912" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7c449d4fadbe93f1297972da2044209.setContent(html_2413fdc6d5eb19809cf8bc6b4d40f912);\\n \\n \\n\\n circle_marker_c73dd6d814f769bf6d860bd7278713f5.bindPopup(popup_a7c449d4fadbe93f1297972da2044209)\\n ;\\n\\n \\n \\n \\n var circle_marker_ad951e2f8b28d1b44f2ebc446627840f = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_11a70f913e19e0f29fcc75a2abeccad9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33f3dbf8e19b440fa658a05071cb0346 = $(`<div id="html_33f3dbf8e19b440fa658a05071cb0346" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11a70f913e19e0f29fcc75a2abeccad9.setContent(html_33f3dbf8e19b440fa658a05071cb0346);\\n \\n \\n\\n circle_marker_ad951e2f8b28d1b44f2ebc446627840f.bindPopup(popup_11a70f913e19e0f29fcc75a2abeccad9)\\n ;\\n\\n \\n \\n \\n var circle_marker_a192847a35151d4f68736f2b4dda1984 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ddf3ff394408b52fbdf12511b242af6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_85c33ecbdadf768c5a7131664d5bfb08 = $(`<div id="html_85c33ecbdadf768c5a7131664d5bfb08" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ddf3ff394408b52fbdf12511b242af6a.setContent(html_85c33ecbdadf768c5a7131664d5bfb08);\\n \\n \\n\\n circle_marker_a192847a35151d4f68736f2b4dda1984.bindPopup(popup_ddf3ff394408b52fbdf12511b242af6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a10c0343bd9fe6b87f512763adbc2a05 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_056acc329a73aa1de8369fbd90e02b81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4db7d4c80b42b084f2c6926f99796e42 = $(`<div id="html_4db7d4c80b42b084f2c6926f99796e42" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_056acc329a73aa1de8369fbd90e02b81.setContent(html_4db7d4c80b42b084f2c6926f99796e42);\\n \\n \\n\\n circle_marker_a10c0343bd9fe6b87f512763adbc2a05.bindPopup(popup_056acc329a73aa1de8369fbd90e02b81)\\n ;\\n\\n \\n \\n \\n var circle_marker_d801e5c574a423d29a299958b43a4a21 = L.circleMarker(\\n [42.730934687380696, -73.24149996761956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.322553886092406, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e8f42dab8b8e595cc7c39d13609edbfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ed302956c83f28b756ced7962c70c9e = $(`<div id="html_6ed302956c83f28b756ced7962c70c9e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8f42dab8b8e595cc7c39d13609edbfe.setContent(html_6ed302956c83f28b756ced7962c70c9e);\\n \\n \\n\\n circle_marker_d801e5c574a423d29a299958b43a4a21.bindPopup(popup_e8f42dab8b8e595cc7c39d13609edbfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_bc9b467e7877ea83ddf4e9e158da33bb = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9b7500c56478d92fb7507e95e9f65a22 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ca11064f8bf7857832436ea9c343bce = $(`<div id="html_7ca11064f8bf7857832436ea9c343bce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b7500c56478d92fb7507e95e9f65a22.setContent(html_7ca11064f8bf7857832436ea9c343bce);\\n \\n \\n\\n circle_marker_bc9b467e7877ea83ddf4e9e158da33bb.bindPopup(popup_9b7500c56478d92fb7507e95e9f65a22)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d2befed434a1cbaf025a2f735dbfdf0 = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_39be8775c6251b139fa7d126cb64ca59 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_703a86b1e0cfe9a758eb2f55cdeb23cd = $(`<div id="html_703a86b1e0cfe9a758eb2f55cdeb23cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39be8775c6251b139fa7d126cb64ca59.setContent(html_703a86b1e0cfe9a758eb2f55cdeb23cd);\\n \\n \\n\\n circle_marker_5d2befed434a1cbaf025a2f735dbfdf0.bindPopup(popup_39be8775c6251b139fa7d126cb64ca59)\\n ;\\n\\n \\n \\n \\n var circle_marker_54258fed846725182862c8056f42803d = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0a44fb6730c031e1f884c8731035f7e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3db162dfcdeaffa0af8cfba2369595a0 = $(`<div id="html_3db162dfcdeaffa0af8cfba2369595a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a44fb6730c031e1f884c8731035f7e3.setContent(html_3db162dfcdeaffa0af8cfba2369595a0);\\n \\n \\n\\n circle_marker_54258fed846725182862c8056f42803d.bindPopup(popup_0a44fb6730c031e1f884c8731035f7e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d3fd470239ba020392048d3d0b6cdeee = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5975e3beeb6e756b41880be3b531a910 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2ab9ce07bae260df4fd3ccd36c399b0b = $(`<div id="html_2ab9ce07bae260df4fd3ccd36c399b0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5975e3beeb6e756b41880be3b531a910.setContent(html_2ab9ce07bae260df4fd3ccd36c399b0b);\\n \\n \\n\\n circle_marker_d3fd470239ba020392048d3d0b6cdeee.bindPopup(popup_5975e3beeb6e756b41880be3b531a910)\\n ;\\n\\n \\n \\n \\n var circle_marker_f0f12d43f703b78628fb31ad8509e692 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_39ef779935dd2aa717bc9b86f6c55cd4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf30a377bdf3805479895f6e5dfac8c9 = $(`<div id="html_bf30a377bdf3805479895f6e5dfac8c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39ef779935dd2aa717bc9b86f6c55cd4.setContent(html_bf30a377bdf3805479895f6e5dfac8c9);\\n \\n \\n\\n circle_marker_f0f12d43f703b78628fb31ad8509e692.bindPopup(popup_39ef779935dd2aa717bc9b86f6c55cd4)\\n ;\\n\\n \\n \\n \\n var circle_marker_211e383c6522f693d5a878fd23693d6c = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_db0623d0226e627cc51f2a132ac44b41 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43cd2ae53d16b4da771b98a318ec5daa = $(`<div id="html_43cd2ae53d16b4da771b98a318ec5daa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db0623d0226e627cc51f2a132ac44b41.setContent(html_43cd2ae53d16b4da771b98a318ec5daa);\\n \\n \\n\\n circle_marker_211e383c6522f693d5a878fd23693d6c.bindPopup(popup_db0623d0226e627cc51f2a132ac44b41)\\n ;\\n\\n \\n \\n \\n var circle_marker_a0b43513669db9115070f67a2a2256ce = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_808c49f4c6c6ad7a5bfd67ea78bb89e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ecf2ce7dac30f85f4e9ba3179bd1476 = $(`<div id="html_8ecf2ce7dac30f85f4e9ba3179bd1476" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_808c49f4c6c6ad7a5bfd67ea78bb89e2.setContent(html_8ecf2ce7dac30f85f4e9ba3179bd1476);\\n \\n \\n\\n circle_marker_a0b43513669db9115070f67a2a2256ce.bindPopup(popup_808c49f4c6c6ad7a5bfd67ea78bb89e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_e25399c83ccb6ff066581d93f252ba06 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_7b5e069d6d7e384cb6172347148cca55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87fb9ea2c457cb84c27b5261b6fb63cb = $(`<div id="html_87fb9ea2c457cb84c27b5261b6fb63cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7b5e069d6d7e384cb6172347148cca55.setContent(html_87fb9ea2c457cb84c27b5261b6fb63cb);\\n \\n \\n\\n circle_marker_e25399c83ccb6ff066581d93f252ba06.bindPopup(popup_7b5e069d6d7e384cb6172347148cca55)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7dd32b3444d1b646200fa37912614fe = L.circleMarker(\\n [42.73092945391477, -73.2230484950559],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_dc5c293e09eb62af5195bd7f30ba58f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_16253a85e0dad8d20c2f23bb5131eafa = $(`<div id="html_16253a85e0dad8d20c2f23bb5131eafa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc5c293e09eb62af5195bd7f30ba58f3.setContent(html_16253a85e0dad8d20c2f23bb5131eafa);\\n \\n \\n\\n circle_marker_a7dd32b3444d1b646200fa37912614fe.bindPopup(popup_dc5c293e09eb62af5195bd7f30ba58f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d4a3a89f854358c11d36d5067ad2ed8b = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8c5677f1de2c4347b402ba00c9cabfe4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_511883d0903185586ab5155d1c5eb4ea = $(`<div id="html_511883d0903185586ab5155d1c5eb4ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c5677f1de2c4347b402ba00c9cabfe4.setContent(html_511883d0903185586ab5155d1c5eb4ea);\\n \\n \\n\\n circle_marker_d4a3a89f854358c11d36d5067ad2ed8b.bindPopup(popup_8c5677f1de2c4347b402ba00c9cabfe4)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b75a0639665f908c1daba910e57139d = L.circleMarker(\\n [42.72912992387847, -73.26364177333073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_539daf6dba27c18ce7d16a5c067c85a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ec067cf501ca7c0b2531ecb3b796c37 = $(`<div id="html_8ec067cf501ca7c0b2531ecb3b796c37" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_539daf6dba27c18ce7d16a5c067c85a8.setContent(html_8ec067cf501ca7c0b2531ecb3b796c37);\\n \\n \\n\\n circle_marker_1b75a0639665f908c1daba910e57139d.bindPopup(popup_539daf6dba27c18ce7d16a5c067c85a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_a55115970debed3b75b653b2e743d0f1 = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a05debc1213dcdbcd5b6f775108999ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6848f306effdd14123fdfbf13f17242a = $(`<div id="html_6848f306effdd14123fdfbf13f17242a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a05debc1213dcdbcd5b6f775108999ee.setContent(html_6848f306effdd14123fdfbf13f17242a);\\n \\n \\n\\n circle_marker_a55115970debed3b75b653b2e743d0f1.bindPopup(popup_a05debc1213dcdbcd5b6f775108999ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e4643163dc3930aa8aa64cbb1543940 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3cc23ccdf4b80619db8b5e7477208f96 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6021660da831b77773132be369d393ea = $(`<div id="html_6021660da831b77773132be369d393ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3cc23ccdf4b80619db8b5e7477208f96.setContent(html_6021660da831b77773132be369d393ea);\\n \\n \\n\\n circle_marker_7e4643163dc3930aa8aa64cbb1543940.bindPopup(popup_3cc23ccdf4b80619db8b5e7477208f96)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3bb85f684416ea21fdd3654afd58027 = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e745ce5d5106c1a66f86c31c07fa90e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04be43db77054bb3ffc0fb11cb27d361 = $(`<div id="html_04be43db77054bb3ffc0fb11cb27d361" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e745ce5d5106c1a66f86c31c07fa90e2.setContent(html_04be43db77054bb3ffc0fb11cb27d361);\\n \\n \\n\\n circle_marker_f3bb85f684416ea21fdd3654afd58027.bindPopup(popup_e745ce5d5106c1a66f86c31c07fa90e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcf99817ae0f23fb6ab36238128f74cf = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c3f54dc7bd1e4e1336042234fe517e00 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4c257a7ab3d5e6d1cc3f7643f63518b6 = $(`<div id="html_4c257a7ab3d5e6d1cc3f7643f63518b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3f54dc7bd1e4e1336042234fe517e00.setContent(html_4c257a7ab3d5e6d1cc3f7643f63518b6);\\n \\n \\n\\n circle_marker_bcf99817ae0f23fb6ab36238128f74cf.bindPopup(popup_c3f54dc7bd1e4e1336042234fe517e00)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9cbda44dbd3a8a1f85b69d2b9f39801 = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d64ecff2053b5291d2759e16ce68d483 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1cb217077496b50a30b510101969070e = $(`<div id="html_1cb217077496b50a30b510101969070e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d64ecff2053b5291d2759e16ce68d483.setContent(html_1cb217077496b50a30b510101969070e);\\n \\n \\n\\n circle_marker_e9cbda44dbd3a8a1f85b69d2b9f39801.bindPopup(popup_d64ecff2053b5291d2759e16ce68d483)\\n ;\\n\\n \\n \\n \\n var circle_marker_14d88410c08ca2b1d5fa3f96a623f931 = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_75b0f4eb6f82f35b370b91112b0cfe6c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_036d70030b334339790ce6d12aa558f9 = $(`<div id="html_036d70030b334339790ce6d12aa558f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75b0f4eb6f82f35b370b91112b0cfe6c.setContent(html_036d70030b334339790ce6d12aa558f9);\\n \\n \\n\\n circle_marker_14d88410c08ca2b1d5fa3f96a623f931.bindPopup(popup_75b0f4eb6f82f35b370b91112b0cfe6c)\\n ;\\n\\n \\n \\n \\n var circle_marker_326a93a3c9099db3707420d260b19081 = L.circleMarker(\\n [42.72912939726166, -73.25380127326159],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c1c8603396514397530c5c63ae49a4ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81a318efa224b3966cee1a534c9dadea = $(`<div id="html_81a318efa224b3966cee1a534c9dadea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c1c8603396514397530c5c63ae49a4ca.setContent(html_81a318efa224b3966cee1a534c9dadea);\\n \\n \\n\\n circle_marker_326a93a3c9099db3707420d260b19081.bindPopup(popup_c1c8603396514397530c5c63ae49a4ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c4c679f6306b933435da789e0db81b9 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9ac570b68546f0843b060443ad594295 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff4c823508747c36c12176ed55a9e8ff = $(`<div id="html_ff4c823508747c36c12176ed55a9e8ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ac570b68546f0843b060443ad594295.setContent(html_ff4c823508747c36c12176ed55a9e8ff);\\n \\n \\n\\n circle_marker_8c4c679f6306b933435da789e0db81b9.bindPopup(popup_9ac570b68546f0843b060443ad594295)\\n ;\\n\\n \\n \\n \\n var circle_marker_755cb39ad142005cc9f688926d95c480 = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_93c20111534fd4bb42dcd526f16f3173 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d823ef1f00aa7e3839fdd352c0311aac = $(`<div id="html_d823ef1f00aa7e3839fdd352c0311aac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93c20111534fd4bb42dcd526f16f3173.setContent(html_d823ef1f00aa7e3839fdd352c0311aac);\\n \\n \\n\\n circle_marker_755cb39ad142005cc9f688926d95c480.bindPopup(popup_93c20111534fd4bb42dcd526f16f3173)\\n ;\\n\\n \\n \\n \\n var circle_marker_1235fba22b6d763f57df2329193feb1f = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0a62a05d81fb58dc723a5bb3124cfdb9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d010cab1a40a52ef173208033a6b5a34 = $(`<div id="html_d010cab1a40a52ef173208033a6b5a34" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a62a05d81fb58dc723a5bb3124cfdb9.setContent(html_d010cab1a40a52ef173208033a6b5a34);\\n \\n \\n\\n circle_marker_1235fba22b6d763f57df2329193feb1f.bindPopup(popup_0a62a05d81fb58dc723a5bb3124cfdb9)\\n ;\\n\\n \\n \\n \\n var circle_marker_18ee533e3c8b402ab5577ed70cc0eee0 = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_96128ebca6107831d936fefb7aa0ef90 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26ded09ad9e3ede39e0e90b378a2e9b1 = $(`<div id="html_26ded09ad9e3ede39e0e90b378a2e9b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96128ebca6107831d936fefb7aa0ef90.setContent(html_26ded09ad9e3ede39e0e90b378a2e9b1);\\n \\n \\n\\n circle_marker_18ee533e3c8b402ab5577ed70cc0eee0.bindPopup(popup_96128ebca6107831d936fefb7aa0ef90)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc7aa756faf561c406ed2d954b3b7df9 = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5421710f8b828b91c55bdcc5024154a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe9bf7bb304cfed4cb764b713a1a63ef = $(`<div id="html_fe9bf7bb304cfed4cb764b713a1a63ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5421710f8b828b91c55bdcc5024154a3.setContent(html_fe9bf7bb304cfed4cb764b713a1a63ef);\\n \\n \\n\\n circle_marker_fc7aa756faf561c406ed2d954b3b7df9.bindPopup(popup_5421710f8b828b91c55bdcc5024154a3)\\n ;\\n\\n \\n \\n \\n var circle_marker_35bb58cdaf0e6fe752d7a62f4ad1ecce = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5be8ae2e5bd1b36c2d17acf2f19f3fa6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ca2ca2c6a6252a33ba457befe62fba22 = $(`<div id="html_ca2ca2c6a6252a33ba457befe62fba22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5be8ae2e5bd1b36c2d17acf2f19f3fa6.setContent(html_ca2ca2c6a6252a33ba457befe62fba22);\\n \\n \\n\\n circle_marker_35bb58cdaf0e6fe752d7a62f4ad1ecce.bindPopup(popup_5be8ae2e5bd1b36c2d17acf2f19f3fa6)\\n ;\\n\\n \\n \\n \\n var circle_marker_0113e7345e7b86e2869b26a0fe31df78 = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1fc2b07dd7b1d209d8234a8b86da6cf6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_179be7071e1d1577779ab5668fa06564 = $(`<div id="html_179be7071e1d1577779ab5668fa06564" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1fc2b07dd7b1d209d8234a8b86da6cf6.setContent(html_179be7071e1d1577779ab5668fa06564);\\n \\n \\n\\n circle_marker_0113e7345e7b86e2869b26a0fe31df78.bindPopup(popup_1fc2b07dd7b1d209d8234a8b86da6cf6)\\n ;\\n\\n \\n \\n \\n var circle_marker_89d0f7bd32f82b5ad6c95899969c1d8d = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9ab1ba6fa643bb47fa54b5075b752337 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c56d9ff54166c991f7d5564d46bbb11f = $(`<div id="html_c56d9ff54166c991f7d5564d46bbb11f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ab1ba6fa643bb47fa54b5075b752337.setContent(html_c56d9ff54166c991f7d5564d46bbb11f);\\n \\n \\n\\n circle_marker_89d0f7bd32f82b5ad6c95899969c1d8d.bindPopup(popup_9ab1ba6fa643bb47fa54b5075b752337)\\n ;\\n\\n \\n \\n \\n var circle_marker_267bfef8119bd2914be853c6e7fcc76d = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_05bd88636e397471ffe3aa3208d64d2e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_207f0dbd62a4241ae5ccffde81fe56f5 = $(`<div id="html_207f0dbd62a4241ae5ccffde81fe56f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05bd88636e397471ffe3aa3208d64d2e.setContent(html_207f0dbd62a4241ae5ccffde81fe56f5);\\n \\n \\n\\n circle_marker_267bfef8119bd2914be853c6e7fcc76d.bindPopup(popup_05bd88636e397471ffe3aa3208d64d2e)\\n ;\\n\\n \\n \\n \\n var circle_marker_37896718154017026bfc217335e7fa09 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_bd99014ffa5390628e36b8134f046355 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3146c7153da40d0660fc3451bde5d827 = $(`<div id="html_3146c7153da40d0660fc3451bde5d827" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd99014ffa5390628e36b8134f046355.setContent(html_3146c7153da40d0660fc3451bde5d827);\\n \\n \\n\\n circle_marker_37896718154017026bfc217335e7fa09.bindPopup(popup_bd99014ffa5390628e36b8134f046355)\\n ;\\n\\n \\n \\n \\n var circle_marker_5facecc16bd4c9d09c059bf7c02c4828 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5517b19ee0c14034242646a726738896 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2ba5392b2d0129dcd196535b45485706 = $(`<div id="html_2ba5392b2d0129dcd196535b45485706" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5517b19ee0c14034242646a726738896.setContent(html_2ba5392b2d0129dcd196535b45485706);\\n \\n \\n\\n circle_marker_5facecc16bd4c9d09c059bf7c02c4828.bindPopup(popup_5517b19ee0c14034242646a726738896)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc960e239824cde779d0809fd50efc37 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_441b8853afe0d087e30225d8c3782709 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e071bcefbb5dc5f7efd97649dada3d08 = $(`<div id="html_e071bcefbb5dc5f7efd97649dada3d08" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_441b8853afe0d087e30225d8c3782709.setContent(html_e071bcefbb5dc5f7efd97649dada3d08);\\n \\n \\n\\n circle_marker_fc960e239824cde779d0809fd50efc37.bindPopup(popup_441b8853afe0d087e30225d8c3782709)\\n ;\\n\\n \\n \\n \\n var circle_marker_48a77c639d0f5806597a676ae45a16eb = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f0c50d488772a69d55148f5aed1a9977 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25cced4e72eb26ce0ac0602c99a545b5 = $(`<div id="html_25cced4e72eb26ce0ac0602c99a545b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0c50d488772a69d55148f5aed1a9977.setContent(html_25cced4e72eb26ce0ac0602c99a545b5);\\n \\n \\n\\n circle_marker_48a77c639d0f5806597a676ae45a16eb.bindPopup(popup_f0c50d488772a69d55148f5aed1a9977)\\n ;\\n\\n \\n \\n \\n var circle_marker_62dab9aa49969555c55a561b88a5bfbc = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e22c0a4e3a97e228f0f3adf86219ef78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_249baedbdf4409dd0dea6b697eeaf0d2 = $(`<div id="html_249baedbdf4409dd0dea6b697eeaf0d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e22c0a4e3a97e228f0f3adf86219ef78.setContent(html_249baedbdf4409dd0dea6b697eeaf0d2);\\n \\n \\n\\n circle_marker_62dab9aa49969555c55a561b88a5bfbc.bindPopup(popup_e22c0a4e3a97e228f0f3adf86219ef78)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b7c17117b114a1cb251f2b6f2d29f72 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ebadbf865bbf3de043d4acdb15533008 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0868eecc75914df08d4883d853af49d2 = $(`<div id="html_0868eecc75914df08d4883d853af49d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebadbf865bbf3de043d4acdb15533008.setContent(html_0868eecc75914df08d4883d853af49d2);\\n \\n \\n\\n circle_marker_8b7c17117b114a1cb251f2b6f2d29f72.bindPopup(popup_ebadbf865bbf3de043d4acdb15533008)\\n ;\\n\\n \\n \\n \\n var circle_marker_52d0c59ca0121bf2109fbf7b6757cd5c = L.circleMarker(\\n [42.7291258162676, -73.23412027429306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c6a12a24d1d57d7897e11cfeb3894ffd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab98cb9544fe9fa50d489e4f50b2805e = $(`<div id="html_ab98cb9544fe9fa50d489e4f50b2805e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6a12a24d1d57d7897e11cfeb3894ffd.setContent(html_ab98cb9544fe9fa50d489e4f50b2805e);\\n \\n \\n\\n circle_marker_52d0c59ca0121bf2109fbf7b6757cd5c.bindPopup(popup_c6a12a24d1d57d7897e11cfeb3894ffd)\\n ;\\n\\n \\n \\n \\n var circle_marker_e492450fd735f91084d441458317cc11 = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ae9b6fb8e8d126392833edcb6b1fbdf0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e6956405113706dbfc32e352991fcb49 = $(`<div id="html_e6956405113706dbfc32e352991fcb49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ae9b6fb8e8d126392833edcb6b1fbdf0.setContent(html_e6956405113706dbfc32e352991fcb49);\\n \\n \\n\\n circle_marker_e492450fd735f91084d441458317cc11.bindPopup(popup_ae9b6fb8e8d126392833edcb6b1fbdf0)\\n ;\\n\\n \\n \\n \\n var circle_marker_74aefc028109c167e77e3e275f548c0f = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_297759c59364f947b4cc34616664f308 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e676fccfca3e9c5e55f2eac3ad7ac20 = $(`<div id="html_2e676fccfca3e9c5e55f2eac3ad7ac20" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_297759c59364f947b4cc34616664f308.setContent(html_2e676fccfca3e9c5e55f2eac3ad7ac20);\\n \\n \\n\\n circle_marker_74aefc028109c167e77e3e275f548c0f.bindPopup(popup_297759c59364f947b4cc34616664f308)\\n ;\\n\\n \\n \\n \\n var circle_marker_66ba52a807619ba451b4dd2ead81617f = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b14d18602958265da51591f21930e920 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eaa4577d1248ceac81a86ea577136ebf = $(`<div id="html_eaa4577d1248ceac81a86ea577136ebf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b14d18602958265da51591f21930e920.setContent(html_eaa4577d1248ceac81a86ea577136ebf);\\n \\n \\n\\n circle_marker_66ba52a807619ba451b4dd2ead81617f.bindPopup(popup_b14d18602958265da51591f21930e920)\\n ;\\n\\n \\n \\n \\n var circle_marker_6bf4ccc82c5c395feca4a7ef589b9b53 = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_075644999f0b7d206f9bc428522c0255 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_739a1552a4863d541b7daceeb9c37358 = $(`<div id="html_739a1552a4863d541b7daceeb9c37358" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_075644999f0b7d206f9bc428522c0255.setContent(html_739a1552a4863d541b7daceeb9c37358);\\n \\n \\n\\n circle_marker_6bf4ccc82c5c395feca4a7ef589b9b53.bindPopup(popup_075644999f0b7d206f9bc428522c0255)\\n ;\\n\\n \\n \\n \\n var circle_marker_0dd2d4ef50e2defb21fc21e251b08186 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1644caec1f77d6ba3c6266b5bd72c540 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d08d84a171697ce04ad4bb12204f9680 = $(`<div id="html_d08d84a171697ce04ad4bb12204f9680" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1644caec1f77d6ba3c6266b5bd72c540.setContent(html_d08d84a171697ce04ad4bb12204f9680);\\n \\n \\n\\n circle_marker_0dd2d4ef50e2defb21fc21e251b08186.bindPopup(popup_1644caec1f77d6ba3c6266b5bd72c540)\\n ;\\n\\n \\n \\n \\n var circle_marker_97d88ff5e9e56aa71c4342d104fb63d4 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6809f5f6bff67ff0c58836ff59771123 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f0773e3271b1b43cc4803752b632514 = $(`<div id="html_4f0773e3271b1b43cc4803752b632514" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6809f5f6bff67ff0c58836ff59771123.setContent(html_4f0773e3271b1b43cc4803752b632514);\\n \\n \\n\\n circle_marker_97d88ff5e9e56aa71c4342d104fb63d4.bindPopup(popup_6809f5f6bff67ff0c58836ff59771123)\\n ;\\n\\n \\n \\n \\n var circle_marker_7817faaf322bbd4a0bc86d76638a3273 = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_72df548afd6a943016bdfd53f1562a03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cfc053b6816c0561f443d5b549a2e6f = $(`<div id="html_9cfc053b6816c0561f443d5b549a2e6f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72df548afd6a943016bdfd53f1562a03.setContent(html_9cfc053b6816c0561f443d5b549a2e6f);\\n \\n \\n\\n circle_marker_7817faaf322bbd4a0bc86d76638a3273.bindPopup(popup_72df548afd6a943016bdfd53f1562a03)\\n ;\\n\\n \\n \\n \\n var circle_marker_aac0b3cad84e34a400734f0701f31f9d = L.circleMarker(\\n [42.729122320849, -73.22304971370495],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_94ec0143f4806ddbdde5952e8855e8c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a79924e81dd4dc7b5e456531ec31c2b2 = $(`<div id="html_a79924e81dd4dc7b5e456531ec31c2b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94ec0143f4806ddbdde5952e8855e8c2.setContent(html_a79924e81dd4dc7b5e456531ec31c2b2);\\n \\n \\n\\n circle_marker_aac0b3cad84e34a400734f0701f31f9d.bindPopup(popup_94ec0143f4806ddbdde5952e8855e8c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_67e0156d323ad7f54fd8a47ebf742bc2 = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_41f8936cbf0f0d1b571f28d93bf32342 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74f4ce643e84c8ef53c35c4a3ae7655f = $(`<div id="html_74f4ce643e84c8ef53c35c4a3ae7655f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41f8936cbf0f0d1b571f28d93bf32342.setContent(html_74f4ce643e84c8ef53c35c4a3ae7655f);\\n \\n \\n\\n circle_marker_67e0156d323ad7f54fd8a47ebf742bc2.bindPopup(popup_41f8936cbf0f0d1b571f28d93bf32342)\\n ;\\n\\n \\n \\n \\n var circle_marker_f744b75c0c6846c2afe5ad2683d0cf5b = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_161483bb878e538f6447147d2e526310 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6fcec05092235156eb5e87b94123efc1 = $(`<div id="html_6fcec05092235156eb5e87b94123efc1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_161483bb878e538f6447147d2e526310.setContent(html_6fcec05092235156eb5e87b94123efc1);\\n \\n \\n\\n circle_marker_f744b75c0c6846c2afe5ad2683d0cf5b.bindPopup(popup_161483bb878e538f6447147d2e526310)\\n ;\\n\\n \\n \\n \\n var circle_marker_19b1eed447eb0d5fb5e4df25da1295fc = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0f556529fb82ef063148fe738b726652 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b313ed30b2fd60ae81e76dd080343e0 = $(`<div id="html_6b313ed30b2fd60ae81e76dd080343e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f556529fb82ef063148fe738b726652.setContent(html_6b313ed30b2fd60ae81e76dd080343e0);\\n \\n \\n\\n circle_marker_19b1eed447eb0d5fb5e4df25da1295fc.bindPopup(popup_0f556529fb82ef063148fe738b726652)\\n ;\\n\\n \\n \\n \\n var circle_marker_69744c0465c65354e481e9ded4161502 = L.circleMarker(\\n [42.72732269176802, -73.25995172914543],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_019d2e5ed7faaecb501de973d5d12d5f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7333dcb76945fc036c663f0db23ff41 = $(`<div id="html_c7333dcb76945fc036c663f0db23ff41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_019d2e5ed7faaecb501de973d5d12d5f.setContent(html_c7333dcb76945fc036c663f0db23ff41);\\n \\n \\n\\n circle_marker_69744c0465c65354e481e9ded4161502.bindPopup(popup_019d2e5ed7faaecb501de973d5d12d5f)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff3827e75ee5402a318a089dda28bc3e = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_5aa9a8426ee00e2dc5723936950ec282 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ae3c7b2003a455dd93d02f386749f6b = $(`<div id="html_8ae3c7b2003a455dd93d02f386749f6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5aa9a8426ee00e2dc5723936950ec282.setContent(html_8ae3c7b2003a455dd93d02f386749f6b);\\n \\n \\n\\n circle_marker_ff3827e75ee5402a318a089dda28bc3e.bindPopup(popup_5aa9a8426ee00e2dc5723936950ec282)\\n ;\\n\\n \\n \\n \\n var circle_marker_c9893eb765171a163aee20bb124623d5 = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a4fc40fa52cb4b7a99bcb277a33253e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a91df92fbb8dcd6b7f148f98c31a69d = $(`<div id="html_4a91df92fbb8dcd6b7f148f98c31a69d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4fc40fa52cb4b7a99bcb277a33253e3.setContent(html_4a91df92fbb8dcd6b7f148f98c31a69d);\\n \\n \\n\\n circle_marker_c9893eb765171a163aee20bb124623d5.bindPopup(popup_a4fc40fa52cb4b7a99bcb277a33253e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_4610dd4bdd9488d7a39f1c3768abdb63 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_20163a2883a3bad9e54c84c0954b9a3c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7302adfa189c3a9910230abb11fa29fd = $(`<div id="html_7302adfa189c3a9910230abb11fa29fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20163a2883a3bad9e54c84c0954b9a3c.setContent(html_7302adfa189c3a9910230abb11fa29fd);\\n \\n \\n\\n circle_marker_4610dd4bdd9488d7a39f1c3768abdb63.bindPopup(popup_20163a2883a3bad9e54c84c0954b9a3c)\\n ;\\n\\n \\n \\n \\n var circle_marker_1078b692fbb12e22029f22e88968fdc2 = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_493d108168b1e9fa4ce9f116edb258e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6d12008b37ae3afe3942e0c9227103e = $(`<div id="html_b6d12008b37ae3afe3942e0c9227103e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_493d108168b1e9fa4ce9f116edb258e7.setContent(html_b6d12008b37ae3afe3942e0c9227103e);\\n \\n \\n\\n circle_marker_1078b692fbb12e22029f22e88968fdc2.bindPopup(popup_493d108168b1e9fa4ce9f116edb258e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e84433fcf46814862d04cb596235313 = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3cfc3c36cabe81ebe507dd40d4bd36bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_294ce74f8b2f5f4c93b92660a19b70d5 = $(`<div id="html_294ce74f8b2f5f4c93b92660a19b70d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3cfc3c36cabe81ebe507dd40d4bd36bc.setContent(html_294ce74f8b2f5f4c93b92660a19b70d5);\\n \\n \\n\\n circle_marker_7e84433fcf46814862d04cb596235313.bindPopup(popup_3cfc3c36cabe81ebe507dd40d4bd36bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_68de83e719a9a349fe7fd8f37af489c8 = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_355ddca12b092877599891b72bc971cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_51c0a0ca5097f0b922ac89d169a5603f = $(`<div id="html_51c0a0ca5097f0b922ac89d169a5603f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_355ddca12b092877599891b72bc971cb.setContent(html_51c0a0ca5097f0b922ac89d169a5603f);\\n \\n \\n\\n circle_marker_68de83e719a9a349fe7fd8f37af489c8.bindPopup(popup_355ddca12b092877599891b72bc971cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb52df5d0f20832362538f33aef06281 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_2c3830f6c8589fa5317aa94125d8cb7d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67215175d89849aaba320530db3ecf9d = $(`<div id="html_67215175d89849aaba320530db3ecf9d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c3830f6c8589fa5317aa94125d8cb7d.setContent(html_67215175d89849aaba320530db3ecf9d);\\n \\n \\n\\n circle_marker_fb52df5d0f20832362538f33aef06281.bindPopup(popup_2c3830f6c8589fa5317aa94125d8cb7d)\\n ;\\n\\n \\n \\n \\n var circle_marker_213d88d1b4d3720641d9e13d471c11da = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_4cc38fb95e61aff47f6dfb93168b7b24 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87adf18df560f70c4c89d073cf70a41a = $(`<div id="html_87adf18df560f70c4c89d073cf70a41a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4cc38fb95e61aff47f6dfb93168b7b24.setContent(html_87adf18df560f70c4c89d073cf70a41a);\\n \\n \\n\\n circle_marker_213d88d1b4d3720641d9e13d471c11da.bindPopup(popup_4cc38fb95e61aff47f6dfb93168b7b24)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f3f1330f8a136077da53428970087be = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_045a577fe7be7e78a0291906bedb9043 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c3766e87a6a2e4f0458e9bfae3a4aeb = $(`<div id="html_1c3766e87a6a2e4f0458e9bfae3a4aeb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_045a577fe7be7e78a0291906bedb9043.setContent(html_1c3766e87a6a2e4f0458e9bfae3a4aeb);\\n \\n \\n\\n circle_marker_9f3f1330f8a136077da53428970087be.bindPopup(popup_045a577fe7be7e78a0291906bedb9043)\\n ;\\n\\n \\n \\n \\n var circle_marker_b11bf8e2b59a83092d57303edfe63cf4 = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a85f892e474318887046eef6e1b16ac0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13fdc76a1be77d0499e4a14a35941ad5 = $(`<div id="html_13fdc76a1be77d0499e4a14a35941ad5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a85f892e474318887046eef6e1b16ac0.setContent(html_13fdc76a1be77d0499e4a14a35941ad5);\\n \\n \\n\\n circle_marker_b11bf8e2b59a83092d57303edfe63cf4.bindPopup(popup_a85f892e474318887046eef6e1b16ac0)\\n ;\\n\\n \\n \\n \\n var circle_marker_e748d4b26881d42468603c11f1da6e45 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_148a2968686c8fbd7f90bbcaf8d818d2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_357db06f0c9a3d31c7dbe4aa43f52834 = $(`<div id="html_357db06f0c9a3d31c7dbe4aa43f52834" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_148a2968686c8fbd7f90bbcaf8d818d2.setContent(html_357db06f0c9a3d31c7dbe4aa43f52834);\\n \\n \\n\\n circle_marker_e748d4b26881d42468603c11f1da6e45.bindPopup(popup_148a2968686c8fbd7f90bbcaf8d818d2)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e9fef72e47f7b0d3ebabb8828fac9ae = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_67e9d142bd594e6defdba345551443f7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3cc6af2dfbb321c18dca02a70744c5c2 = $(`<div id="html_3cc6af2dfbb321c18dca02a70744c5c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_67e9d142bd594e6defdba345551443f7.setContent(html_3cc6af2dfbb321c18dca02a70744c5c2);\\n \\n \\n\\n circle_marker_0e9fef72e47f7b0d3ebabb8828fac9ae.bindPopup(popup_67e9d142bd594e6defdba345551443f7)\\n ;\\n\\n \\n \\n \\n var circle_marker_5997dfed51dd31b1c743657297168a54 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6b0fe3eaca49f74deec5d0ff1c575770 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d8ae8a726ededbaed48195f6581316f = $(`<div id="html_7d8ae8a726ededbaed48195f6581316f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b0fe3eaca49f74deec5d0ff1c575770.setContent(html_7d8ae8a726ededbaed48195f6581316f);\\n \\n \\n\\n circle_marker_5997dfed51dd31b1c743657297168a54.bindPopup(popup_6b0fe3eaca49f74deec5d0ff1c575770)\\n ;\\n\\n \\n \\n \\n var circle_marker_3aafae1b655623230d5e42e69d1e8ffd = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d8eb56707ae9f6de2a0373e77502850d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40d0f9d81f249389a1b9ea4a4906f366 = $(`<div id="html_40d0f9d81f249389a1b9ea4a4906f366" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8eb56707ae9f6de2a0373e77502850d.setContent(html_40d0f9d81f249389a1b9ea4a4906f366);\\n \\n \\n\\n circle_marker_3aafae1b655623230d5e42e69d1e8ffd.bindPopup(popup_d8eb56707ae9f6de2a0373e77502850d)\\n ;\\n\\n \\n \\n \\n var circle_marker_8dd2965b3f7ea3e6ef3d07592b07e92d = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_6dc25f7d806a0ff880bf30590dc92633 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_203c99676d2717ef6d44004688d484d7 = $(`<div id="html_203c99676d2717ef6d44004688d484d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6dc25f7d806a0ff880bf30590dc92633.setContent(html_203c99676d2717ef6d44004688d484d7);\\n \\n \\n\\n circle_marker_8dd2965b3f7ea3e6ef3d07592b07e92d.bindPopup(popup_6dc25f7d806a0ff880bf30590dc92633)\\n ;\\n\\n \\n \\n \\n var circle_marker_e053be7c82ec99e487ef780930646987 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d72b8b277f59eb6dd4174664d785a9c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39d1f68ceea0f2d9b0725a3c167b02f8 = $(`<div id="html_39d1f68ceea0f2d9b0725a3c167b02f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d72b8b277f59eb6dd4174664d785a9c1.setContent(html_39d1f68ceea0f2d9b0725a3c167b02f8);\\n \\n \\n\\n circle_marker_e053be7c82ec99e487ef780930646987.bindPopup(popup_d72b8b277f59eb6dd4174664d785a9c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_3bcaaa94d0fe26a2baf4fd8ea1246be2 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_33a70e6133e12e150df934fc5025ca82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74f0fe62d89cdb417030d679bd2ed9bb = $(`<div id="html_74f0fe62d89cdb417030d679bd2ed9bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33a70e6133e12e150df934fc5025ca82.setContent(html_74f0fe62d89cdb417030d679bd2ed9bb);\\n \\n \\n\\n circle_marker_3bcaaa94d0fe26a2baf4fd8ea1246be2.bindPopup(popup_33a70e6133e12e150df934fc5025ca82)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe67d5152820f2dc4087a7e070fbeed6 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_8b0be5f4a1771f2baa6c2665a5649541 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_554d70f317552a8456aa82c5e4a1272c = $(`<div id="html_554d70f317552a8456aa82c5e4a1272c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b0be5f4a1771f2baa6c2665a5649541.setContent(html_554d70f317552a8456aa82c5e4a1272c);\\n \\n \\n\\n circle_marker_fe67d5152820f2dc4087a7e070fbeed6.bindPopup(popup_8b0be5f4a1771f2baa6c2665a5649541)\\n ;\\n\\n \\n \\n \\n var circle_marker_dbf4142d82b02c074ddb1f59ece0c9c5 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_e02e1b945a6367d9a5863373f3f6e5b0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c78b55654dcaf54318730f4b969ad06c = $(`<div id="html_c78b55654dcaf54318730f4b969ad06c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e02e1b945a6367d9a5863373f3f6e5b0.setContent(html_c78b55654dcaf54318730f4b969ad06c);\\n \\n \\n\\n circle_marker_dbf4142d82b02c074ddb1f59ece0c9c5.bindPopup(popup_e02e1b945a6367d9a5863373f3f6e5b0)\\n ;\\n\\n \\n \\n \\n var circle_marker_d03e0689800b4b6f7da0787d9cf07a64 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_7bb68f56ce7d99771f2f3e435568db04 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e86612494e4d18e8ec863b4b12e0402 = $(`<div id="html_8e86612494e4d18e8ec863b4b12e0402" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7bb68f56ce7d99771f2f3e435568db04.setContent(html_8e86612494e4d18e8ec863b4b12e0402);\\n \\n \\n\\n circle_marker_d03e0689800b4b6f7da0787d9cf07a64.bindPopup(popup_7bb68f56ce7d99771f2f3e435568db04)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c5a28a2f808b307b19fcde9f9ca2f20 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_20c88c9dc4a705b6b6c960dafdf124da = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9540dda3417d057aedbea6dd1f01f31 = $(`<div id="html_d9540dda3417d057aedbea6dd1f01f31" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20c88c9dc4a705b6b6c960dafdf124da.setContent(html_d9540dda3417d057aedbea6dd1f01f31);\\n \\n \\n\\n circle_marker_1c5a28a2f808b307b19fcde9f9ca2f20.bindPopup(popup_20c88c9dc4a705b6b6c960dafdf124da)\\n ;\\n\\n \\n \\n \\n var circle_marker_d4536ef3c51703e0449f5ea91af84965 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3fcdf7f908a16f4bb3a21e917b84d138 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83472ca0865f718328a87ae1ac310f76 = $(`<div id="html_83472ca0865f718328a87ae1ac310f76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3fcdf7f908a16f4bb3a21e917b84d138.setContent(html_83472ca0865f718328a87ae1ac310f76);\\n \\n \\n\\n circle_marker_d4536ef3c51703e0449f5ea91af84965.bindPopup(popup_3fcdf7f908a16f4bb3a21e917b84d138)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6368d0eec25265cce94dbc1ab1438aa = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_f4200acb1059ef2d75ae7903c63d2749 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e80c200901d6f76db0bb94527655046 = $(`<div id="html_9e80c200901d6f76db0bb94527655046" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4200acb1059ef2d75ae7903c63d2749.setContent(html_9e80c200901d6f76db0bb94527655046);\\n \\n \\n\\n circle_marker_d6368d0eec25265cce94dbc1ab1438aa.bindPopup(popup_f4200acb1059ef2d75ae7903c63d2749)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b7908e47c13e6fdb304c9299a632a36 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b7e69a531a77a9c9c79156d46ca5cee2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2f2e6ab3e2702b75c2fdbac90df0fe3 = $(`<div id="html_e2f2e6ab3e2702b75c2fdbac90df0fe3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b7e69a531a77a9c9c79156d46ca5cee2.setContent(html_e2f2e6ab3e2702b75c2fdbac90df0fe3);\\n \\n \\n\\n circle_marker_0b7908e47c13e6fdb304c9299a632a36.bindPopup(popup_b7e69a531a77a9c9c79156d46ca5cee2)\\n ;\\n\\n \\n \\n \\n var circle_marker_77916d7fb007447b754456871beac2b6 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_0bb6d05cbec473d4f13c120dd4f9f1da = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f0442384e3cf8bb5a3254ecd6c40811 = $(`<div id="html_8f0442384e3cf8bb5a3254ecd6c40811" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0bb6d05cbec473d4f13c120dd4f9f1da.setContent(html_8f0442384e3cf8bb5a3254ecd6c40811);\\n \\n \\n\\n circle_marker_77916d7fb007447b754456871beac2b6.bindPopup(popup_0bb6d05cbec473d4f13c120dd4f9f1da)\\n ;\\n\\n \\n \\n \\n var circle_marker_def010c67daf8b52d1d390a7ed8898b4 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_77b86d10fb36b10079ce43ff09be3934 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a31ea48283d3b29499bb749c1f6b1168 = $(`<div id="html_a31ea48283d3b29499bb749c1f6b1168" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77b86d10fb36b10079ce43ff09be3934.setContent(html_a31ea48283d3b29499bb749c1f6b1168);\\n \\n \\n\\n circle_marker_def010c67daf8b52d1d390a7ed8898b4.bindPopup(popup_77b86d10fb36b10079ce43ff09be3934)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d2830cb32e300820d5dd6156ef705e9 = L.circleMarker(\\n [42.72551563738435, -73.26241185416787],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c80052ba62b6835e4c9b80a3dcbcbff1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e0336af97ae1670de8f534de50644562 = $(`<div id="html_e0336af97ae1670de8f534de50644562" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c80052ba62b6835e4c9b80a3dcbcbff1.setContent(html_e0336af97ae1670de8f534de50644562);\\n \\n \\n\\n circle_marker_7d2830cb32e300820d5dd6156ef705e9.bindPopup(popup_c80052ba62b6835e4c9b80a3dcbcbff1)\\n ;\\n\\n \\n \\n \\n var circle_marker_76322a66944bb891a2e542d45d8d595b = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_bd8d640a141263329261d7bdcd82fe5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a047cd8e50c0f39c351eb0330c57b73 = $(`<div id="html_5a047cd8e50c0f39c351eb0330c57b73" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd8d640a141263329261d7bdcd82fe5a.setContent(html_5a047cd8e50c0f39c351eb0330c57b73);\\n \\n \\n\\n circle_marker_76322a66944bb891a2e542d45d8d595b.bindPopup(popup_bd8d640a141263329261d7bdcd82fe5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_55321d651146a8961e5becc9da24a6fd = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_b18c22d11df042607b2cc305bcea2479 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a14d2c9264d850a0d52d0bd3820e4aec = $(`<div id="html_a14d2c9264d850a0d52d0bd3820e4aec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b18c22d11df042607b2cc305bcea2479.setContent(html_a14d2c9264d850a0d52d0bd3820e4aec);\\n \\n \\n\\n circle_marker_55321d651146a8961e5becc9da24a6fd.bindPopup(popup_b18c22d11df042607b2cc305bcea2479)\\n ;\\n\\n \\n \\n \\n var circle_marker_d214787c2253b1cb9145185304ba0de6 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a81bdd5b166a3ffea2e7bdf16307305f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c791c83e5370d6d313a334566992bb59 = $(`<div id="html_c791c83e5370d6d313a334566992bb59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a81bdd5b166a3ffea2e7bdf16307305f.setContent(html_c791c83e5370d6d313a334566992bb59);\\n \\n \\n\\n circle_marker_d214787c2253b1cb9145185304ba0de6.bindPopup(popup_a81bdd5b166a3ffea2e7bdf16307305f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1b86520af98925122954f129f89dc24 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_237e6ef1a0d03e1896d73c9b82ec0e5d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab39f90d7186d05664783a8ac9b9f464 = $(`<div id="html_ab39f90d7186d05664783a8ac9b9f464" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_237e6ef1a0d03e1896d73c9b82ec0e5d.setContent(html_ab39f90d7186d05664783a8ac9b9f464);\\n \\n \\n\\n circle_marker_b1b86520af98925122954f129f89dc24.bindPopup(popup_237e6ef1a0d03e1896d73c9b82ec0e5d)\\n ;\\n\\n \\n \\n \\n var circle_marker_a70385e1de94d8f4de2049286708e9b0 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_14233292a3d2bf78852900ceb3453ea5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15a2f4b72858afcb5d6605dbe44f256b = $(`<div id="html_15a2f4b72858afcb5d6605dbe44f256b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14233292a3d2bf78852900ceb3453ea5.setContent(html_15a2f4b72858afcb5d6605dbe44f256b);\\n \\n \\n\\n circle_marker_a70385e1de94d8f4de2049286708e9b0.bindPopup(popup_14233292a3d2bf78852900ceb3453ea5)\\n ;\\n\\n \\n \\n \\n var circle_marker_d2f374d684c9f8fa30f5b088c5e83f77 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ba773c53b1780905df43ed8699c17f66 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_af16bc1e8dc2e7fae0f9adba6c62cbf0 = $(`<div id="html_af16bc1e8dc2e7fae0f9adba6c62cbf0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba773c53b1780905df43ed8699c17f66.setContent(html_af16bc1e8dc2e7fae0f9adba6c62cbf0);\\n \\n \\n\\n circle_marker_d2f374d684c9f8fa30f5b088c5e83f77.bindPopup(popup_ba773c53b1780905df43ed8699c17f66)\\n ;\\n\\n \\n \\n \\n var circle_marker_879ecc5048da8442ec69b96954678abd = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_c686c2ff705a49d482a48b6683c4d790 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04b4c58a8faea03e287c0b4876070d58 = $(`<div id="html_04b4c58a8faea03e287c0b4876070d58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c686c2ff705a49d482a48b6683c4d790.setContent(html_04b4c58a8faea03e287c0b4876070d58);\\n \\n \\n\\n circle_marker_879ecc5048da8442ec69b96954678abd.bindPopup(popup_c686c2ff705a49d482a48b6683c4d790)\\n ;\\n\\n \\n \\n \\n var circle_marker_8019cd58798253262eca93a4ad543881 = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_9f47359bd77a4944258e47c9a89f76ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_174a1144f1154b9417181ca1683fb914 = $(`<div id="html_174a1144f1154b9417181ca1683fb914" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f47359bd77a4944258e47c9a89f76ce.setContent(html_174a1144f1154b9417181ca1683fb914);\\n \\n \\n\\n circle_marker_8019cd58798253262eca93a4ad543881.bindPopup(popup_9f47359bd77a4944258e47c9a89f76ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d335abbe4e1e6ec3697dac84579cb0a = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_d007010d88b75a9052223f4a805bbf8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6c4056614dd2f2da333de30aaebad05 = $(`<div id="html_d6c4056614dd2f2da333de30aaebad05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d007010d88b75a9052223f4a805bbf8b.setContent(html_d6c4056614dd2f2da333de30aaebad05);\\n \\n \\n\\n circle_marker_0d335abbe4e1e6ec3697dac84579cb0a.bindPopup(popup_d007010d88b75a9052223f4a805bbf8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_42de5ff0a9c8bec70e3113a71c4ced7b = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_bb5ded6d92a8b2ad495089ec40e570f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cbcfaddfcff1e12cc5601ecc8c8dd3b1 = $(`<div id="html_cbcfaddfcff1e12cc5601ecc8c8dd3b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb5ded6d92a8b2ad495089ec40e570f8.setContent(html_cbcfaddfcff1e12cc5601ecc8c8dd3b1);\\n \\n \\n\\n circle_marker_42de5ff0a9c8bec70e3113a71c4ced7b.bindPopup(popup_bb5ded6d92a8b2ad495089ec40e570f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_b4c40a4e5ba1d6a66af12e864c557a46 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_51c2b4a0896dfa1117c525b71c4f1303 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d114c1c8ccea51de02f9722b4af580b1 = $(`<div id="html_d114c1c8ccea51de02f9722b4af580b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51c2b4a0896dfa1117c525b71c4f1303.setContent(html_d114c1c8ccea51de02f9722b4af580b1);\\n \\n \\n\\n circle_marker_b4c40a4e5ba1d6a66af12e864c557a46.bindPopup(popup_51c2b4a0896dfa1117c525b71c4f1303)\\n ;\\n\\n \\n \\n \\n var circle_marker_50a7e5ca3cb64ee30640e5ebd13a9727 = L.circleMarker(\\n [42.72551121416183, -73.23289207553623],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_fbedbe4fdd63ae03d90790fd96fca6b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db5ced901324673c64643efec5fe2f4c = $(`<div id="html_db5ced901324673c64643efec5fe2f4c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fbedbe4fdd63ae03d90790fd96fca6b8.setContent(html_db5ced901324673c64643efec5fe2f4c);\\n \\n \\n\\n circle_marker_50a7e5ca3cb64ee30640e5ebd13a9727.bindPopup(popup_fbedbe4fdd63ae03d90790fd96fca6b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_b98ed9b9405a825ee0d43d115d24f611 = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_a22571ab4f3d270fc8617b3afdf360bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c90c7eec76d7e63cc62f83e0ed613f39 = $(`<div id="html_c90c7eec76d7e63cc62f83e0ed613f39" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a22571ab4f3d270fc8617b3afdf360bc.setContent(html_c90c7eec76d7e63cc62f83e0ed613f39);\\n \\n \\n\\n circle_marker_b98ed9b9405a825ee0d43d115d24f611.bindPopup(popup_a22571ab4f3d270fc8617b3afdf360bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_a54504ffd456affc27c939fb1a63d446 = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_1ac3df453412c76bb7849acf2d230151 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a377a31ebea8579ed5e3dea3c0bbc1f = $(`<div id="html_6a377a31ebea8579ed5e3dea3c0bbc1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ac3df453412c76bb7849acf2d230151.setContent(html_6a377a31ebea8579ed5e3dea3c0bbc1f);\\n \\n \\n\\n circle_marker_a54504ffd456affc27c939fb1a63d446.bindPopup(popup_1ac3df453412c76bb7849acf2d230151)\\n ;\\n\\n \\n \\n \\n var circle_marker_bdb8a18815bb6dfa790f72053f9e056c = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_557cfe6d2005255d9c44c87b7058204c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36c0f4a734339a826410ab47aa8937c6 = $(`<div id="html_36c0f4a734339a826410ab47aa8937c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_557cfe6d2005255d9c44c87b7058204c.setContent(html_36c0f4a734339a826410ab47aa8937c6);\\n \\n \\n\\n circle_marker_bdb8a18815bb6dfa790f72053f9e056c.bindPopup(popup_557cfe6d2005255d9c44c87b7058204c)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6109683f177344e4ee28890aa02fb0b = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_3bf8f05db726c4d3318b5965647884c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31fdee20826b43bbbd7f84ce9c2d6457 = $(`<div id="html_31fdee20826b43bbbd7f84ce9c2d6457" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3bf8f05db726c4d3318b5965647884c6.setContent(html_31fdee20826b43bbbd7f84ce9c2d6457);\\n \\n \\n\\n circle_marker_a6109683f177344e4ee28890aa02fb0b.bindPopup(popup_3bf8f05db726c4d3318b5965647884c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_b959b4811aa8eba69584d61ea4485b05 = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ddb55285e42f0be734ab6c7d56bc0af9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a3134f1154cb507f3761ae48e2d2543 = $(`<div id="html_8a3134f1154cb507f3761ae48e2d2543" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ddb55285e42f0be734ab6c7d56bc0af9.setContent(html_8a3134f1154cb507f3761ae48e2d2543);\\n \\n \\n\\n circle_marker_b959b4811aa8eba69584d61ea4485b05.bindPopup(popup_ddb55285e42f0be734ab6c7d56bc0af9)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9d368d611d1e38f4eb400c6e2592e36 = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_07c7f9880a514a0addc665a76b269afb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68466d5cf25e00e1a15957bccd1703d9 = $(`<div id="html_68466d5cf25e00e1a15957bccd1703d9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07c7f9880a514a0addc665a76b269afb.setContent(html_68466d5cf25e00e1a15957bccd1703d9);\\n \\n \\n\\n circle_marker_e9d368d611d1e38f4eb400c6e2592e36.bindPopup(popup_07c7f9880a514a0addc665a76b269afb)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d7920cb8bc132cf444ee2371f0882b8 = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_ebe7951bbf9294285b49a0319d9e2897 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1590f84acf1e4a98c3da6acb3b2848d = $(`<div id="html_f1590f84acf1e4a98c3da6acb3b2848d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebe7951bbf9294285b49a0319d9e2897.setContent(html_f1590f84acf1e4a98c3da6acb3b2848d);\\n \\n \\n\\n circle_marker_7d7920cb8bc132cf444ee2371f0882b8.bindPopup(popup_ebe7951bbf9294285b49a0319d9e2897)\\n ;\\n\\n \\n \\n \\n var circle_marker_00b1157e95af3b375f150b0ba762c35f = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_fd04cb60f5646d04155040b6b54e1eca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edd6241dca2acd520ead35105f055455 = $(`<div id="html_edd6241dca2acd520ead35105f055455" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd04cb60f5646d04155040b6b54e1eca.setContent(html_edd6241dca2acd520ead35105f055455);\\n \\n \\n\\n circle_marker_00b1157e95af3b375f150b0ba762c35f.bindPopup(popup_fd04cb60f5646d04155040b6b54e1eca)\\n ;\\n\\n \\n \\n \\n var circle_marker_158d3b5fe09d0b2e69966f15ffb42300 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_54c5ab9224e9697275fb1baad4f418a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6564d42e8a2de8b8182ebe1a8764679a = $(`<div id="html_6564d42e8a2de8b8182ebe1a8764679a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54c5ab9224e9697275fb1baad4f418a8.setContent(html_6564d42e8a2de8b8182ebe1a8764679a);\\n \\n \\n\\n circle_marker_158d3b5fe09d0b2e69966f15ffb42300.bindPopup(popup_54c5ab9224e9697275fb1baad4f418a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c9b816d30376f44ea820063a2384546 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_edde0508db6948fcada72369e97ee22c);\\n \\n \\n var popup_4abfb5d165dbe1187cef39d300208e83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a209b3ab0bf0f5ca3b225a3f24add1d = $(`<div id="html_1a209b3ab0bf0f5ca3b225a3f24add1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4abfb5d165dbe1187cef39d300208e83.setContent(html_1a209b3ab0bf0f5ca3b225a3f24add1d);\\n \\n \\n\\n circle_marker_2c9b816d30376f44ea820063a2384546.bindPopup(popup_4abfb5d165dbe1187cef39d300208e83)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Birch, gray\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_93db2e13136fd383c3147578bb432469 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_93db2e13136fd383c3147578bb432469" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_93db2e13136fd383c3147578bb432469 = L.map(\\n "map_93db2e13136fd383c3147578bb432469",\\n {\\n center: [42.73274261051959, -73.25441641288887],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_ddfbf5290206c8f46fec99effdb1e5ce = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var circle_marker_6758a245b409a41f3841f1fccd91749c = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_c933b9f8c4372eeab3ab331a0ce63b87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_772a9af19331286c60d5bdc954115d87 = $(`<div id="html_772a9af19331286c60d5bdc954115d87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c933b9f8c4372eeab3ab331a0ce63b87.setContent(html_772a9af19331286c60d5bdc954115d87);\\n \\n \\n\\n circle_marker_6758a245b409a41f3841f1fccd91749c.bindPopup(popup_c933b9f8c4372eeab3ab331a0ce63b87)\\n ;\\n\\n \\n \\n \\n var circle_marker_14346d82e46b1864132b71afbcc60ef9 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_427bc59443b049b374885ce3ceb1f0c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60b9227c5f46781eb4a99a6fa46d5a22 = $(`<div id="html_60b9227c5f46781eb4a99a6fa46d5a22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_427bc59443b049b374885ce3ceb1f0c6.setContent(html_60b9227c5f46781eb4a99a6fa46d5a22);\\n \\n \\n\\n circle_marker_14346d82e46b1864132b71afbcc60ef9.bindPopup(popup_427bc59443b049b374885ce3ceb1f0c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba8ac1c5f8de2e5e454adec9c348b0c7 = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_235d184e7f2585289f7f92e8a035d882 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fea67c3e5233d205e2663681279dc62c = $(`<div id="html_fea67c3e5233d205e2663681279dc62c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_235d184e7f2585289f7f92e8a035d882.setContent(html_fea67c3e5233d205e2663681279dc62c);\\n \\n \\n\\n circle_marker_ba8ac1c5f8de2e5e454adec9c348b0c7.bindPopup(popup_235d184e7f2585289f7f92e8a035d882)\\n ;\\n\\n \\n \\n \\n var circle_marker_89cc3e6b5cecef7654e2358428c1a264 = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_8eaabe25e9c31344b15604058e8fb3a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30d786e40a634e2a6cdab950b0c087e6 = $(`<div id="html_30d786e40a634e2a6cdab950b0c087e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8eaabe25e9c31344b15604058e8fb3a9.setContent(html_30d786e40a634e2a6cdab950b0c087e6);\\n \\n \\n\\n circle_marker_89cc3e6b5cecef7654e2358428c1a264.bindPopup(popup_8eaabe25e9c31344b15604058e8fb3a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_859044d6b97bc6ac5fd1d41d3bcb7b39 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_260c8e770ba4c731337598843145fc44 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a620f0afcb89a4e2d0a59d305b024b69 = $(`<div id="html_a620f0afcb89a4e2d0a59d305b024b69" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_260c8e770ba4c731337598843145fc44.setContent(html_a620f0afcb89a4e2d0a59d305b024b69);\\n \\n \\n\\n circle_marker_859044d6b97bc6ac5fd1d41d3bcb7b39.bindPopup(popup_260c8e770ba4c731337598843145fc44)\\n ;\\n\\n \\n \\n \\n var circle_marker_671619ea3a9e218e3a83ea677cb23ff5 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_03a398db8a788b66e8964efeaac8ef3c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f86152af5a9ea1c90f7d5711332e1ba = $(`<div id="html_8f86152af5a9ea1c90f7d5711332e1ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03a398db8a788b66e8964efeaac8ef3c.setContent(html_8f86152af5a9ea1c90f7d5711332e1ba);\\n \\n \\n\\n circle_marker_671619ea3a9e218e3a83ea677cb23ff5.bindPopup(popup_03a398db8a788b66e8964efeaac8ef3c)\\n ;\\n\\n \\n \\n \\n var circle_marker_b9920f5e9abb7b8d786320a1a417d3d2 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_513373c9a523e235e02166ccd895c04f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4e9d814da8b1e500fc8ee8e2267f5b6 = $(`<div id="html_d4e9d814da8b1e500fc8ee8e2267f5b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_513373c9a523e235e02166ccd895c04f.setContent(html_d4e9d814da8b1e500fc8ee8e2267f5b6);\\n \\n \\n\\n circle_marker_b9920f5e9abb7b8d786320a1a417d3d2.bindPopup(popup_513373c9a523e235e02166ccd895c04f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e401a8e9ba00801c047aa74b532048cc = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_a78f7ca10bedd8455a964b59d3640327 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4c70441f461f00d660b22abee7b967d5 = $(`<div id="html_4c70441f461f00d660b22abee7b967d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a78f7ca10bedd8455a964b59d3640327.setContent(html_4c70441f461f00d660b22abee7b967d5);\\n \\n \\n\\n circle_marker_e401a8e9ba00801c047aa74b532048cc.bindPopup(popup_a78f7ca10bedd8455a964b59d3640327)\\n ;\\n\\n \\n \\n \\n var circle_marker_97a3de11b5f2e434b73db0fab2a3f1da = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_4e29491a604323b436cd86ccff60c438 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_faf9bb53f971424078fe7d87558cb710 = $(`<div id="html_faf9bb53f971424078fe7d87558cb710" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e29491a604323b436cd86ccff60c438.setContent(html_faf9bb53f971424078fe7d87558cb710);\\n \\n \\n\\n circle_marker_97a3de11b5f2e434b73db0fab2a3f1da.bindPopup(popup_4e29491a604323b436cd86ccff60c438)\\n ;\\n\\n \\n \\n \\n var circle_marker_054e73612fc5bda610b69025bab5a468 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_fd1b62924239ed698806a23f34b7c233 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_469a39245c50481033a0250bd84c800f = $(`<div id="html_469a39245c50481033a0250bd84c800f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd1b62924239ed698806a23f34b7c233.setContent(html_469a39245c50481033a0250bd84c800f);\\n \\n \\n\\n circle_marker_054e73612fc5bda610b69025bab5a468.bindPopup(popup_fd1b62924239ed698806a23f34b7c233)\\n ;\\n\\n \\n \\n \\n var circle_marker_1cb76ae759355fe3c87801788cebad0c = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_6894bd90137ec3d8413f20c4fa525378 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55425be58ae2bfad64d5a2aa62de1a9d = $(`<div id="html_55425be58ae2bfad64d5a2aa62de1a9d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6894bd90137ec3d8413f20c4fa525378.setContent(html_55425be58ae2bfad64d5a2aa62de1a9d);\\n \\n \\n\\n circle_marker_1cb76ae759355fe3c87801788cebad0c.bindPopup(popup_6894bd90137ec3d8413f20c4fa525378)\\n ;\\n\\n \\n \\n \\n var circle_marker_139d671469f669fa56185b8e14345b1e = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_417a0247e842bb6a6a8ba1a051d77114 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2ecdf2a97adfa99b630e22309f37da4 = $(`<div id="html_b2ecdf2a97adfa99b630e22309f37da4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_417a0247e842bb6a6a8ba1a051d77114.setContent(html_b2ecdf2a97adfa99b630e22309f37da4);\\n \\n \\n\\n circle_marker_139d671469f669fa56185b8e14345b1e.bindPopup(popup_417a0247e842bb6a6a8ba1a051d77114)\\n ;\\n\\n \\n \\n \\n var circle_marker_e2dfb8ec0b10207956eae6c0db9573c4 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_d0eacb523b06888fa555839411730dbb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_365f8e6c7e607577dd258c2240d0a2d4 = $(`<div id="html_365f8e6c7e607577dd258c2240d0a2d4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0eacb523b06888fa555839411730dbb.setContent(html_365f8e6c7e607577dd258c2240d0a2d4);\\n \\n \\n\\n circle_marker_e2dfb8ec0b10207956eae6c0db9573c4.bindPopup(popup_d0eacb523b06888fa555839411730dbb)\\n ;\\n\\n \\n \\n \\n var circle_marker_8765a1710b1dbf67308109e26be30981 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_07772d9373483e27530fd9fac8146b1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c90f309a2360f0ed14db3b26ec4ef490 = $(`<div id="html_c90f309a2360f0ed14db3b26ec4ef490" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07772d9373483e27530fd9fac8146b1b.setContent(html_c90f309a2360f0ed14db3b26ec4ef490);\\n \\n \\n\\n circle_marker_8765a1710b1dbf67308109e26be30981.bindPopup(popup_07772d9373483e27530fd9fac8146b1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_ed83e09753e59f00ccdecf20c2acf915 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_9389e469e62968bf425e4ccffdfe11b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d35a0200267a0ed1ac82ff149caf65f1 = $(`<div id="html_d35a0200267a0ed1ac82ff149caf65f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9389e469e62968bf425e4ccffdfe11b7.setContent(html_d35a0200267a0ed1ac82ff149caf65f1);\\n \\n \\n\\n circle_marker_ed83e09753e59f00ccdecf20c2acf915.bindPopup(popup_9389e469e62968bf425e4ccffdfe11b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_098052506c23ff5b09fcc4c987df41a6 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_255933c09f4598cd5791d85ff12fcc91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b214fbfaba803057a8ad8fbc4acd8441 = $(`<div id="html_b214fbfaba803057a8ad8fbc4acd8441" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_255933c09f4598cd5791d85ff12fcc91.setContent(html_b214fbfaba803057a8ad8fbc4acd8441);\\n \\n \\n\\n circle_marker_098052506c23ff5b09fcc4c987df41a6.bindPopup(popup_255933c09f4598cd5791d85ff12fcc91)\\n ;\\n\\n \\n \\n \\n var circle_marker_56884f7a5f3ef27124194679a6c976a4 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_93db2e13136fd383c3147578bb432469);\\n \\n \\n var popup_9aa5a16d92ecfc7246b31ac3b147b7a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_043b1d0cde5599bc8758c8add706952f = $(`<div id="html_043b1d0cde5599bc8758c8add706952f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9aa5a16d92ecfc7246b31ac3b147b7a6.setContent(html_043b1d0cde5599bc8758c8add706952f);\\n \\n \\n\\n circle_marker_56884f7a5f3ef27124194679a6c976a4.bindPopup(popup_9aa5a16d92ecfc7246b31ac3b147b7a6)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Birch, paper\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_47fe858b732d032490389b3501e3637c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_47fe858b732d032490389b3501e3637c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_47fe858b732d032490389b3501e3637c = L.map(\\n "map_47fe858b732d032490389b3501e3637c",\\n {\\n center: [42.73545173137012, -73.24826559998263],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_fe3ba69c13652aa71a574cdddef07d5d = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var circle_marker_3bde3484d947624feb248e1bbfdcb042 = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2b1a26fcd714a0515bc45f518e1bebb9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3458e95ee8162cf8476ab5a211b57d83 = $(`<div id="html_3458e95ee8162cf8476ab5a211b57d83" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b1a26fcd714a0515bc45f518e1bebb9.setContent(html_3458e95ee8162cf8476ab5a211b57d83);\\n \\n \\n\\n circle_marker_3bde3484d947624feb248e1bbfdcb042.bindPopup(popup_2b1a26fcd714a0515bc45f518e1bebb9)\\n ;\\n\\n \\n \\n \\n var circle_marker_08aab8af2a3993a15307f3809f85117e = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1c8e56b4ec40ab71c7ce7f875b53a5fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea961de5734cb8e9bd74e82f5a177e76 = $(`<div id="html_ea961de5734cb8e9bd74e82f5a177e76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1c8e56b4ec40ab71c7ce7f875b53a5fd.setContent(html_ea961de5734cb8e9bd74e82f5a177e76);\\n \\n \\n\\n circle_marker_08aab8af2a3993a15307f3809f85117e.bindPopup(popup_1c8e56b4ec40ab71c7ce7f875b53a5fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_e37090b6ce85635a6e506a642583878f = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ec9e59160732b4c0395942b18243aa93 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0091491ae95b66c2b34f62fff8fdb056 = $(`<div id="html_0091491ae95b66c2b34f62fff8fdb056" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec9e59160732b4c0395942b18243aa93.setContent(html_0091491ae95b66c2b34f62fff8fdb056);\\n \\n \\n\\n circle_marker_e37090b6ce85635a6e506a642583878f.bindPopup(popup_ec9e59160732b4c0395942b18243aa93)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f187b899dbff75a0a5c1705ea16e99d = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_d3224580fd180983fcbf7d102a3d5f14 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb5c8f6813e19739edf54308adfefef5 = $(`<div id="html_fb5c8f6813e19739edf54308adfefef5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3224580fd180983fcbf7d102a3d5f14.setContent(html_fb5c8f6813e19739edf54308adfefef5);\\n \\n \\n\\n circle_marker_5f187b899dbff75a0a5c1705ea16e99d.bindPopup(popup_d3224580fd180983fcbf7d102a3d5f14)\\n ;\\n\\n \\n \\n \\n var circle_marker_af002c01dc0fd7eabf3b8c56282ca3ea = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c6d85ded9801d19708b887eaa8d846a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a08dbf4ac0b7622a28d4092abe0911ec = $(`<div id="html_a08dbf4ac0b7622a28d4092abe0911ec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6d85ded9801d19708b887eaa8d846a2.setContent(html_a08dbf4ac0b7622a28d4092abe0911ec);\\n \\n \\n\\n circle_marker_af002c01dc0fd7eabf3b8c56282ca3ea.bindPopup(popup_c6d85ded9801d19708b887eaa8d846a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_888fcc73f7a77a6f00e1f3c9a9362227 = L.circleMarker(\\n [42.747201204933376, -73.2685630990657],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c6bdaeb50dba3cfc64ad61bf76b7f9e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37a2d0423d4af17697db69b47aba7ee6 = $(`<div id="html_37a2d0423d4af17697db69b47aba7ee6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6bdaeb50dba3cfc64ad61bf76b7f9e4.setContent(html_37a2d0423d4af17697db69b47aba7ee6);\\n \\n \\n\\n circle_marker_888fcc73f7a77a6f00e1f3c9a9362227.bindPopup(popup_c6bdaeb50dba3cfc64ad61bf76b7f9e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf6fcadfd2e4f63cc3c5b64bffc458ff = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_d20123b6edbe9a367553d7db98140bc0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a084ec2ce867974dafd3179ab27a5206 = $(`<div id="html_a084ec2ce867974dafd3179ab27a5206" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d20123b6edbe9a367553d7db98140bc0.setContent(html_a084ec2ce867974dafd3179ab27a5206);\\n \\n \\n\\n circle_marker_cf6fcadfd2e4f63cc3c5b64bffc458ff.bindPopup(popup_d20123b6edbe9a367553d7db98140bc0)\\n ;\\n\\n \\n \\n \\n var circle_marker_4771f815c556314dbbb86c30801e1454 = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_02ed266e626644129f878b29cb83f406 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d44cae233345f25fa8f068b497b75f4a = $(`<div id="html_d44cae233345f25fa8f068b497b75f4a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_02ed266e626644129f878b29cb83f406.setContent(html_d44cae233345f25fa8f068b497b75f4a);\\n \\n \\n\\n circle_marker_4771f815c556314dbbb86c30801e1454.bindPopup(popup_02ed266e626644129f878b29cb83f406)\\n ;\\n\\n \\n \\n \\n var circle_marker_e20a32851a33835f3f454508dcb8265a = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_eb6d7557c9be1019c3055aac63a1ea4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fad198047cb24ba94d30ffdf9cde9ebf = $(`<div id="html_fad198047cb24ba94d30ffdf9cde9ebf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb6d7557c9be1019c3055aac63a1ea4b.setContent(html_fad198047cb24ba94d30ffdf9cde9ebf);\\n \\n \\n\\n circle_marker_e20a32851a33835f3f454508dcb8265a.bindPopup(popup_eb6d7557c9be1019c3055aac63a1ea4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_03e0fd4f1fc33f1d5d83f6809b6c4306 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_41a949e12fc2d0934122cf4ebeba468e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8d48b4f5c85f0171df58771775c4bd4 = $(`<div id="html_a8d48b4f5c85f0171df58771775c4bd4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41a949e12fc2d0934122cf4ebeba468e.setContent(html_a8d48b4f5c85f0171df58771775c4bd4);\\n \\n \\n\\n circle_marker_03e0fd4f1fc33f1d5d83f6809b6c4306.bindPopup(popup_41a949e12fc2d0934122cf4ebeba468e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a33e3190a8b7a1d1169d8ba0cbaddc0f = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_454af5535037638f0b488360313b07d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d164904c59df56891d3d17f06a6a3fa = $(`<div id="html_4d164904c59df56891d3d17f06a6a3fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_454af5535037638f0b488360313b07d4.setContent(html_4d164904c59df56891d3d17f06a6a3fa);\\n \\n \\n\\n circle_marker_a33e3190a8b7a1d1169d8ba0cbaddc0f.bindPopup(popup_454af5535037638f0b488360313b07d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_42a181c1eb0c5f047707ee9103e94994 = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5e2815ca63f2967d9cfb68c75ced3fed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e6da0a972087fbacd82d24ba9bf666e0 = $(`<div id="html_e6da0a972087fbacd82d24ba9bf666e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e2815ca63f2967d9cfb68c75ced3fed.setContent(html_e6da0a972087fbacd82d24ba9bf666e0);\\n \\n \\n\\n circle_marker_42a181c1eb0c5f047707ee9103e94994.bindPopup(popup_5e2815ca63f2967d9cfb68c75ced3fed)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a27699ccd209a86ebea82e551b36c4d = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_4e47a3518d48eac5ca352183b20c030b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cc061d4aac42dc9a76da94b607034f3 = $(`<div id="html_9cc061d4aac42dc9a76da94b607034f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e47a3518d48eac5ca352183b20c030b.setContent(html_9cc061d4aac42dc9a76da94b607034f3);\\n \\n \\n\\n circle_marker_0a27699ccd209a86ebea82e551b36c4d.bindPopup(popup_4e47a3518d48eac5ca352183b20c030b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e397e52657177cb3d7e691bad648e1a4 = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_d2222c7555014a2a83788e8023dfc791 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9814b695516a7d61ecc740b1c9e3254f = $(`<div id="html_9814b695516a7d61ecc740b1c9e3254f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2222c7555014a2a83788e8023dfc791.setContent(html_9814b695516a7d61ecc740b1c9e3254f);\\n \\n \\n\\n circle_marker_e397e52657177cb3d7e691bad648e1a4.bindPopup(popup_d2222c7555014a2a83788e8023dfc791)\\n ;\\n\\n \\n \\n \\n var circle_marker_444b2f303d082130f30ec65b07e981de = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_af0d827efd692478358c5403dd524961 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cf5ae6aa4d858ae6c521e30204a46e8 = $(`<div id="html_9cf5ae6aa4d858ae6c521e30204a46e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af0d827efd692478358c5403dd524961.setContent(html_9cf5ae6aa4d858ae6c521e30204a46e8);\\n \\n \\n\\n circle_marker_444b2f303d082130f30ec65b07e981de.bindPopup(popup_af0d827efd692478358c5403dd524961)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3fb9da112fa421b6f14892b79dcef5a = L.circleMarker(\\n [42.74539359743371, -73.27594530262718],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_97f65ab6ad397db935761d52ab34ddd2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c98b7f84f5ffc1f8207e27735312f78 = $(`<div id="html_8c98b7f84f5ffc1f8207e27735312f78" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97f65ab6ad397db935761d52ab34ddd2.setContent(html_8c98b7f84f5ffc1f8207e27735312f78);\\n \\n \\n\\n circle_marker_e3fb9da112fa421b6f14892b79dcef5a.bindPopup(popup_97f65ab6ad397db935761d52ab34ddd2)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4a762676aa7d233ae8701dbe2cef8c9 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_cdad88dac6e4e7edeef2e71b2e797cf2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4dc2385e7ac4446af4d0c7600a466e34 = $(`<div id="html_4dc2385e7ac4446af4d0c7600a466e34" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cdad88dac6e4e7edeef2e71b2e797cf2.setContent(html_4dc2385e7ac4446af4d0c7600a466e34);\\n \\n \\n\\n circle_marker_e4a762676aa7d233ae8701dbe2cef8c9.bindPopup(popup_cdad88dac6e4e7edeef2e71b2e797cf2)\\n ;\\n\\n \\n \\n \\n var circle_marker_ab046cfc39943fb0bd73fa53e877ee9b = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a54f4e603228f84b5b36a03df6c4b536 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_90620d8eea8f05ac943c40e63136ea2e = $(`<div id="html_90620d8eea8f05ac943c40e63136ea2e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a54f4e603228f84b5b36a03df6c4b536.setContent(html_90620d8eea8f05ac943c40e63136ea2e);\\n \\n \\n\\n circle_marker_ab046cfc39943fb0bd73fa53e877ee9b.bindPopup(popup_a54f4e603228f84b5b36a03df6c4b536)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6ddf0fd354e48abc35a21dc3fc11379 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8fa13ac747503f8ff023fbf404db5c4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e28dfa0913a82866e86695d72b0b508b = $(`<div id="html_e28dfa0913a82866e86695d72b0b508b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fa13ac747503f8ff023fbf404db5c4e.setContent(html_e28dfa0913a82866e86695d72b0b508b);\\n \\n \\n\\n circle_marker_a6ddf0fd354e48abc35a21dc3fc11379.bindPopup(popup_8fa13ac747503f8ff023fbf404db5c4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_0281c6b5e5902cb233d924b0b4b20ed4 = L.circleMarker(\\n [42.74539412424261, -73.26364145064247],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_56a3adeb4ba2ae52f5aef0d8d64318bf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c06f8339729ef99d4cadf8c7fed125df = $(`<div id="html_c06f8339729ef99d4cadf8c7fed125df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_56a3adeb4ba2ae52f5aef0d8d64318bf.setContent(html_c06f8339729ef99d4cadf8c7fed125df);\\n \\n \\n\\n circle_marker_0281c6b5e5902cb233d924b0b4b20ed4.bindPopup(popup_56a3adeb4ba2ae52f5aef0d8d64318bf)\\n ;\\n\\n \\n \\n \\n var circle_marker_20ec2ea35255f7338c9a972d4ce9eb08 = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_14340dfce4ab0d0fac7f087d894c146d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fcff9b1e3002f941afe6d9ca5a3845ee = $(`<div id="html_fcff9b1e3002f941afe6d9ca5a3845ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14340dfce4ab0d0fac7f087d894c146d.setContent(html_fcff9b1e3002f941afe6d9ca5a3845ee);\\n \\n \\n\\n circle_marker_20ec2ea35255f7338c9a972d4ce9eb08.bindPopup(popup_14340dfce4ab0d0fac7f087d894c146d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c9aa8abc465478c82adc855b7822f023 = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_50a61761c00b25bf3c220abcf9e426a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1d078c3b4ddfcfa68dcace805a65523d = $(`<div id="html_1d078c3b4ddfcfa68dcace805a65523d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_50a61761c00b25bf3c220abcf9e426a9.setContent(html_1d078c3b4ddfcfa68dcace805a65523d);\\n \\n \\n\\n circle_marker_c9aa8abc465478c82adc855b7822f023.bindPopup(popup_50a61761c00b25bf3c220abcf9e426a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_141e9bb00c257794da00604899479f0e = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_877bc95ef95804b5d954e4db52326740 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7fb753fe461b5ca4f7d89483f4b4720c = $(`<div id="html_7fb753fe461b5ca4f7d89483f4b4720c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_877bc95ef95804b5d954e4db52326740.setContent(html_7fb753fe461b5ca4f7d89483f4b4720c);\\n \\n \\n\\n circle_marker_141e9bb00c257794da00604899479f0e.bindPopup(popup_877bc95ef95804b5d954e4db52326740)\\n ;\\n\\n \\n \\n \\n var circle_marker_4def60037b6b87acc5e8dcb8a1244dd8 = L.circleMarker(\\n [42.74358667479628, -73.27348428119001],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_bd190440c179bca2d1daac84c1cf12ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bcd781899c9aaad95e43c8b1e0a94581 = $(`<div id="html_bcd781899c9aaad95e43c8b1e0a94581" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd190440c179bca2d1daac84c1cf12ce.setContent(html_bcd781899c9aaad95e43c8b1e0a94581);\\n \\n \\n\\n circle_marker_4def60037b6b87acc5e8dcb8a1244dd8.bindPopup(popup_bd190440c179bca2d1daac84c1cf12ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_a48ccbff58d661c65ec85b3717a45811 = L.circleMarker(\\n [42.743586938190056, -73.26856288386173],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_64c923f2954f5c6d225ad5682752db91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67e6e3b991c34ded1d65fd8c1595b7b6 = $(`<div id="html_67e6e3b991c34ded1d65fd8c1595b7b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64c923f2954f5c6d225ad5682752db91.setContent(html_67e6e3b991c34ded1d65fd8c1595b7b6);\\n \\n \\n\\n circle_marker_a48ccbff58d661c65ec85b3717a45811.bindPopup(popup_64c923f2954f5c6d225ad5682752db91)\\n ;\\n\\n \\n \\n \\n var circle_marker_277d9650570f7a00eeb19c85cf13fc5b = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_679434ce068dad1f6f593c7c48dd5560 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9eff41b51977c3b90b540dcc891018e3 = $(`<div id="html_9eff41b51977c3b90b540dcc891018e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_679434ce068dad1f6f593c7c48dd5560.setContent(html_9eff41b51977c3b90b540dcc891018e3);\\n \\n \\n\\n circle_marker_277d9650570f7a00eeb19c85cf13fc5b.bindPopup(popup_679434ce068dad1f6f593c7c48dd5560)\\n ;\\n\\n \\n \\n \\n var circle_marker_5890da8565f1b6a0df64a24af66eb4cd = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_f4676e2a404c78506beed5ebbdec635e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_585264f0ebbeb525599ace93c095d757 = $(`<div id="html_585264f0ebbeb525599ace93c095d757" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4676e2a404c78506beed5ebbdec635e.setContent(html_585264f0ebbeb525599ace93c095d757);\\n \\n \\n\\n circle_marker_5890da8565f1b6a0df64a24af66eb4cd.bindPopup(popup_f4676e2a404c78506beed5ebbdec635e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0987dbfa51689a2f6490edb7e063efe = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_b3c9da6640c008c2d2bd4a6becbd5a6c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6662c5ea13669dd3939426cb81b73f1 = $(`<div id="html_d6662c5ea13669dd3939426cb81b73f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3c9da6640c008c2d2bd4a6becbd5a6c.setContent(html_d6662c5ea13669dd3939426cb81b73f1);\\n \\n \\n\\n circle_marker_d0987dbfa51689a2f6490edb7e063efe.bindPopup(popup_b3c9da6640c008c2d2bd4a6becbd5a6c)\\n ;\\n\\n \\n \\n \\n var circle_marker_08a3c9fa7e36ed8ba3d7c70789c2901c = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_55de5fd9bf3aa224be15fefd0cf542f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3195d2c1165f3a0ec977d4c45d40b75 = $(`<div id="html_a3195d2c1165f3a0ec977d4c45d40b75" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_55de5fd9bf3aa224be15fefd0cf542f0.setContent(html_a3195d2c1165f3a0ec977d4c45d40b75);\\n \\n \\n\\n circle_marker_08a3c9fa7e36ed8ba3d7c70789c2901c.bindPopup(popup_55de5fd9bf3aa224be15fefd0cf542f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1d7adec0b7109a663a2055a7f99d54e = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a957f28cb20d942bd4eef0f403cd2abc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57d956845aa3f3b6db1015bdf45f8b1b = $(`<div id="html_57d956845aa3f3b6db1015bdf45f8b1b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a957f28cb20d942bd4eef0f403cd2abc.setContent(html_57d956845aa3f3b6db1015bdf45f8b1b);\\n \\n \\n\\n circle_marker_f1d7adec0b7109a663a2055a7f99d54e.bindPopup(popup_a957f28cb20d942bd4eef0f403cd2abc)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b7e5e694606b17af03d8465cecdd205 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_463e913bb4a74fa03e7422bad477bdb3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3c47786e4cd568a3b95518407f6f079f = $(`<div id="html_3c47786e4cd568a3b95518407f6f079f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_463e913bb4a74fa03e7422bad477bdb3.setContent(html_3c47786e4cd568a3b95518407f6f079f);\\n \\n \\n\\n circle_marker_0b7e5e694606b17af03d8465cecdd205.bindPopup(popup_463e913bb4a74fa03e7422bad477bdb3)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7abe570d354bdc97043b7d6db19afcf = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_bae1b346238c7dc58e0eb1969ed58161 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce835f405919d77d3882a761bde1a8f7 = $(`<div id="html_ce835f405919d77d3882a761bde1a8f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bae1b346238c7dc58e0eb1969ed58161.setContent(html_ce835f405919d77d3882a761bde1a8f7);\\n \\n \\n\\n circle_marker_a7abe570d354bdc97043b7d6db19afcf.bindPopup(popup_bae1b346238c7dc58e0eb1969ed58161)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d1f18dd714fd3ed32f5beb425d9614b = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5e6a13200627626b0b0ab4d7a328592f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c0dd29b0ce545e52a2aa0a270f070b5 = $(`<div id="html_5c0dd29b0ce545e52a2aa0a270f070b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e6a13200627626b0b0ab4d7a328592f.setContent(html_5c0dd29b0ce545e52a2aa0a270f070b5);\\n \\n \\n\\n circle_marker_3d1f18dd714fd3ed32f5beb425d9614b.bindPopup(popup_5e6a13200627626b0b0ab4d7a328592f)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb171bfb21b8c84a296bffc3a3139786 = L.circleMarker(\\n [42.7417795414353, -73.27348403014923],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_7fcc29dd30a59702dcd03b54901fc575 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1fd1f44b8dac770432ae89dcc1511137 = $(`<div id="html_1fd1f44b8dac770432ae89dcc1511137" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7fcc29dd30a59702dcd03b54901fc575.setContent(html_1fd1f44b8dac770432ae89dcc1511137);\\n \\n \\n\\n circle_marker_cb171bfb21b8c84a296bffc3a3139786.bindPopup(popup_7fcc29dd30a59702dcd03b54901fc575)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee887b754f5963d94f177b173498959b = L.circleMarker(\\n [42.7417798640796, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8943b8c1bef73e415999fe9719c718b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ca0bc4969a540cab8c43e4aa03071c3 = $(`<div id="html_7ca0bc4969a540cab8c43e4aa03071c3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8943b8c1bef73e415999fe9719c718b2.setContent(html_7ca0bc4969a540cab8c43e4aa03071c3);\\n \\n \\n\\n circle_marker_ee887b754f5963d94f177b173498959b.bindPopup(popup_8943b8c1bef73e415999fe9719c718b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_26a7b437b663b405bb52c8f7f8906dde = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_7a37187db5abfb494b2f5bb7cce225d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_936b5fac5d6e41ffb54433c72480e62e = $(`<div id="html_936b5fac5d6e41ffb54433c72480e62e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a37187db5abfb494b2f5bb7cce225d7.setContent(html_936b5fac5d6e41ffb54433c72480e62e);\\n \\n \\n\\n circle_marker_26a7b437b663b405bb52c8f7f8906dde.bindPopup(popup_7a37187db5abfb494b2f5bb7cce225d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_549cb99708fba9c4cd6b6463c81a57e3 = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_4c3ba936203863cc813d8e68db4f4cb9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c91100bfe7df46cec66d3829bcd2b064 = $(`<div id="html_c91100bfe7df46cec66d3829bcd2b064" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c3ba936203863cc813d8e68db4f4cb9.setContent(html_c91100bfe7df46cec66d3829bcd2b064);\\n \\n \\n\\n circle_marker_549cb99708fba9c4cd6b6463c81a57e3.bindPopup(popup_4c3ba936203863cc813d8e68db4f4cb9)\\n ;\\n\\n \\n \\n \\n var circle_marker_e168f39d82169ffeae0bcdc257e73010 = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_296d72b57ea36be72b70dcffeaacdd2a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ebc2050fb520d7ed2f84e737e0f2d22f = $(`<div id="html_ebc2050fb520d7ed2f84e737e0f2d22f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_296d72b57ea36be72b70dcffeaacdd2a.setContent(html_ebc2050fb520d7ed2f84e737e0f2d22f);\\n \\n \\n\\n circle_marker_e168f39d82169ffeae0bcdc257e73010.bindPopup(popup_296d72b57ea36be72b70dcffeaacdd2a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8bd1209748bffd733faadaa158844ef6 = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2fc8568809026ad96fe8b682ef80a242 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_986756e3379182fa6d03bf44a67ac0a4 = $(`<div id="html_986756e3379182fa6d03bf44a67ac0a4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fc8568809026ad96fe8b682ef80a242.setContent(html_986756e3379182fa6d03bf44a67ac0a4);\\n \\n \\n\\n circle_marker_8bd1209748bffd733faadaa158844ef6.bindPopup(popup_2fc8568809026ad96fe8b682ef80a242)\\n ;\\n\\n \\n \\n \\n var circle_marker_89ad1a73eb05fc3efa509a2942514474 = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c570d691aa96da1cf203ba16b6149e5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7633ccf9e4150b6dc02aab93bc1bc2a = $(`<div id="html_f7633ccf9e4150b6dc02aab93bc1bc2a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c570d691aa96da1cf203ba16b6149e5a.setContent(html_f7633ccf9e4150b6dc02aab93bc1bc2a);\\n \\n \\n\\n circle_marker_89ad1a73eb05fc3efa509a2942514474.bindPopup(popup_c570d691aa96da1cf203ba16b6149e5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_4c07a0b0180d6bb17f00e8f905ad4d3b = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2f1416cb04c6c6ff4a506383505ffc15 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2dc37440584ddfd45b432297cc6760c6 = $(`<div id="html_2dc37440584ddfd45b432297cc6760c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f1416cb04c6c6ff4a506383505ffc15.setContent(html_2dc37440584ddfd45b432297cc6760c6);\\n \\n \\n\\n circle_marker_4c07a0b0180d6bb17f00e8f905ad4d3b.bindPopup(popup_2f1416cb04c6c6ff4a506383505ffc15)\\n ;\\n\\n \\n \\n \\n var circle_marker_be26031debae7670d8496bc8df4b18fc = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_cbefe8efcd1d29aa9b7553859c523e94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_292ede71456428bf960bd2fbb4ab73f6 = $(`<div id="html_292ede71456428bf960bd2fbb4ab73f6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cbefe8efcd1d29aa9b7553859c523e94.setContent(html_292ede71456428bf960bd2fbb4ab73f6);\\n \\n \\n\\n circle_marker_be26031debae7670d8496bc8df4b18fc.bindPopup(popup_cbefe8efcd1d29aa9b7553859c523e94)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b0ab0238aa260c77665e9bad8d22f4f = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1d8dfef55aa8b85f4900e53735579d8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d8e91a0b9419ab0b521db9139bbe640 = $(`<div id="html_7d8e91a0b9419ab0b521db9139bbe640" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d8dfef55aa8b85f4900e53735579d8d.setContent(html_7d8e91a0b9419ab0b521db9139bbe640);\\n \\n \\n\\n circle_marker_1b0ab0238aa260c77665e9bad8d22f4f.bindPopup(popup_1d8dfef55aa8b85f4900e53735579d8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d094b0281e40fe73d81e2b983822ce0 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_b35590b7943b283f23314fc8db36cbab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_235ca6f85e0b37babdba78dbc73dc15b = $(`<div id="html_235ca6f85e0b37babdba78dbc73dc15b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b35590b7943b283f23314fc8db36cbab.setContent(html_235ca6f85e0b37babdba78dbc73dc15b);\\n \\n \\n\\n circle_marker_6d094b0281e40fe73d81e2b983822ce0.bindPopup(popup_b35590b7943b283f23314fc8db36cbab)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f7cd9155247b092faa068487afe0cce = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_84d7ed341cb25696253c09740df500ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1e3a99118c610c9970e8fd8472564fd = $(`<div id="html_f1e3a99118c610c9970e8fd8472564fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84d7ed341cb25696253c09740df500ea.setContent(html_f1e3a99118c610c9970e8fd8472564fd);\\n \\n \\n\\n circle_marker_8f7cd9155247b092faa068487afe0cce.bindPopup(popup_84d7ed341cb25696253c09740df500ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_3450055931141b8a862cfbf0d88b1614 = L.circleMarker(\\n [42.73816527471333, -73.27348352812871],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c18270ae3b1b9704757ebbd0eb17efb4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64ba16bbe15e00454a853e1c3c6dab05 = $(`<div id="html_64ba16bbe15e00454a853e1c3c6dab05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c18270ae3b1b9704757ebbd0eb17efb4.setContent(html_64ba16bbe15e00454a853e1c3c6dab05);\\n \\n \\n\\n circle_marker_3450055931141b8a862cfbf0d88b1614.bindPopup(popup_c18270ae3b1b9704757ebbd0eb17efb4)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3dab11aa6c7dfb742a8721ecd9e875c = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_dafba4d2612743be128577601fe4481d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_84cb2bca1fb60bbbf18db6c636e6b7c7 = $(`<div id="html_84cb2bca1fb60bbbf18db6c636e6b7c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dafba4d2612743be128577601fe4481d.setContent(html_84cb2bca1fb60bbbf18db6c636e6b7c7);\\n \\n \\n\\n circle_marker_c3dab11aa6c7dfb742a8721ecd9e875c.bindPopup(popup_dafba4d2612743be128577601fe4481d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a8bc9aee9a774c26a64083356a767d7 = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_166687bdd22f5d5247d6fe72f90131c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8720b63e947a9e49c483e681604d5c85 = $(`<div id="html_8720b63e947a9e49c483e681604d5c85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_166687bdd22f5d5247d6fe72f90131c1.setContent(html_8720b63e947a9e49c483e681604d5c85);\\n \\n \\n\\n circle_marker_0a8bc9aee9a774c26a64083356a767d7.bindPopup(popup_166687bdd22f5d5247d6fe72f90131c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_438b0ca96e71074f57a5b1fdf1647070 = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_05f0697188de9fc5ef5d686702f5e7ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60519f2f9abfd41db6cba42326a83a28 = $(`<div id="html_60519f2f9abfd41db6cba42326a83a28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05f0697188de9fc5ef5d686702f5e7ef.setContent(html_60519f2f9abfd41db6cba42326a83a28);\\n \\n \\n\\n circle_marker_438b0ca96e71074f57a5b1fdf1647070.bindPopup(popup_05f0697188de9fc5ef5d686702f5e7ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_17e35ceaffae943bab4842323a46e7ad = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_94f3c64ca8b328c26f4639eb743acb93 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6d9eaddd2663ecc20b841c5f853514d = $(`<div id="html_a6d9eaddd2663ecc20b841c5f853514d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94f3c64ca8b328c26f4639eb743acb93.setContent(html_a6d9eaddd2663ecc20b841c5f853514d);\\n \\n \\n\\n circle_marker_17e35ceaffae943bab4842323a46e7ad.bindPopup(popup_94f3c64ca8b328c26f4639eb743acb93)\\n ;\\n\\n \\n \\n \\n var circle_marker_68b7446c7442e176d0cd8be82ca4fdaa = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_521c01ccb25ecb68b8f482932868c737 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b080f570c010edf0f71f3ac38896c8d3 = $(`<div id="html_b080f570c010edf0f71f3ac38896c8d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_521c01ccb25ecb68b8f482932868c737.setContent(html_b080f570c010edf0f71f3ac38896c8d3);\\n \\n \\n\\n circle_marker_68b7446c7442e176d0cd8be82ca4fdaa.bindPopup(popup_521c01ccb25ecb68b8f482932868c737)\\n ;\\n\\n \\n \\n \\n var circle_marker_558aa1c71e4b4eb9503eebb14522c88f = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8044f50ee6ce5bddc6297ca9d987f395 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b55ae74a9a540ff124e8ee08d1a40df4 = $(`<div id="html_b55ae74a9a540ff124e8ee08d1a40df4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8044f50ee6ce5bddc6297ca9d987f395.setContent(html_b55ae74a9a540ff124e8ee08d1a40df4);\\n \\n \\n\\n circle_marker_558aa1c71e4b4eb9503eebb14522c88f.bindPopup(popup_8044f50ee6ce5bddc6297ca9d987f395)\\n ;\\n\\n \\n \\n \\n var circle_marker_b21f621e8e5c2fd3ac9f4042d53dab74 = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5c47e2cb55a11b613981db6c5df0ac5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4054f20388a6ec1ae4c86fd617077123 = $(`<div id="html_4054f20388a6ec1ae4c86fd617077123" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c47e2cb55a11b613981db6c5df0ac5c.setContent(html_4054f20388a6ec1ae4c86fd617077123);\\n \\n \\n\\n circle_marker_b21f621e8e5c2fd3ac9f4042d53dab74.bindPopup(popup_5c47e2cb55a11b613981db6c5df0ac5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_625d35f168860105336f740f15e0ff4e = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1dd63022d4a875abcb7b79d2c9eb0eb7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21c006b3e2931daf71d5ed3380791db7 = $(`<div id="html_21c006b3e2931daf71d5ed3380791db7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1dd63022d4a875abcb7b79d2c9eb0eb7.setContent(html_21c006b3e2931daf71d5ed3380791db7);\\n \\n \\n\\n circle_marker_625d35f168860105336f740f15e0ff4e.bindPopup(popup_1dd63022d4a875abcb7b79d2c9eb0eb7)\\n ;\\n\\n \\n \\n \\n var circle_marker_a47aadfb977e704063c03aff4d23bf54 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c53c5d768f57bbb2c48a131774087585 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5a3f234a8aa37d4a9f48338514ad0f7 = $(`<div id="html_a5a3f234a8aa37d4a9f48338514ad0f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c53c5d768f57bbb2c48a131774087585.setContent(html_a5a3f234a8aa37d4a9f48338514ad0f7);\\n \\n \\n\\n circle_marker_a47aadfb977e704063c03aff4d23bf54.bindPopup(popup_c53c5d768f57bbb2c48a131774087585)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3a713e7b1fee7edaae15845bed00f1b = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_cf62016d0fe0f339b6980678f4419ca9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_af0374c7caabc7ef26d37a1bbf5b0110 = $(`<div id="html_af0374c7caabc7ef26d37a1bbf5b0110" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf62016d0fe0f339b6980678f4419ca9.setContent(html_af0374c7caabc7ef26d37a1bbf5b0110);\\n \\n \\n\\n circle_marker_c3a713e7b1fee7edaae15845bed00f1b.bindPopup(popup_cf62016d0fe0f339b6980678f4419ca9)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf5c991111354edb5b8f28f776d1599c = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_92d8bbcb3252e24a9f930bb76eb7b57b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e23cccbcd540560156b32a382b25a951 = $(`<div id="html_e23cccbcd540560156b32a382b25a951" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_92d8bbcb3252e24a9f930bb76eb7b57b.setContent(html_e23cccbcd540560156b32a382b25a951);\\n \\n \\n\\n circle_marker_cf5c991111354edb5b8f28f776d1599c.bindPopup(popup_92d8bbcb3252e24a9f930bb76eb7b57b)\\n ;\\n\\n \\n \\n \\n var circle_marker_b3ac07c26f1e54143bc7c289c97b69d1 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_4932ad7a88855833841ce8c85417f32a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bc3734705bfe7ee7b30b7c2d0ac1ca0 = $(`<div id="html_1bc3734705bfe7ee7b30b7c2d0ac1ca0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4932ad7a88855833841ce8c85417f32a.setContent(html_1bc3734705bfe7ee7b30b7c2d0ac1ca0);\\n \\n \\n\\n circle_marker_b3ac07c26f1e54143bc7c289c97b69d1.bindPopup(popup_4932ad7a88855833841ce8c85417f32a)\\n ;\\n\\n \\n \\n \\n var circle_marker_93d4dae40fbeae67c7817e247f864a24 = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2ec3112ed0274ce69268584132288c83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b8657c6a65677669c4bec2b5aab7bf93 = $(`<div id="html_b8657c6a65677669c4bec2b5aab7bf93" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ec3112ed0274ce69268584132288c83.setContent(html_b8657c6a65677669c4bec2b5aab7bf93);\\n \\n \\n\\n circle_marker_93d4dae40fbeae67c7817e247f864a24.bindPopup(popup_2ec3112ed0274ce69268584132288c83)\\n ;\\n\\n \\n \\n \\n var circle_marker_d09c392a2f15c86d2aeb16f016d207de = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_42a358fe80ec6fcf6a8074be4b66e397 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e72d4fbe862d7f466049002bbea5580b = $(`<div id="html_e72d4fbe862d7f466049002bbea5580b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_42a358fe80ec6fcf6a8074be4b66e397.setContent(html_e72d4fbe862d7f466049002bbea5580b);\\n \\n \\n\\n circle_marker_d09c392a2f15c86d2aeb16f016d207de.bindPopup(popup_42a358fe80ec6fcf6a8074be4b66e397)\\n ;\\n\\n \\n \\n \\n var circle_marker_aaa7045b87cc78f05c40cc1cb73ceb7f = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5e773ebf904fa639d8272a17b7574bfa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_61f3e40ae27ecb6093ae7b57f4095df7 = $(`<div id="html_61f3e40ae27ecb6093ae7b57f4095df7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e773ebf904fa639d8272a17b7574bfa.setContent(html_61f3e40ae27ecb6093ae7b57f4095df7);\\n \\n \\n\\n circle_marker_aaa7045b87cc78f05c40cc1cb73ceb7f.bindPopup(popup_5e773ebf904fa639d8272a17b7574bfa)\\n ;\\n\\n \\n \\n \\n var circle_marker_68d6abedc4d79aaf9d402dcc329a017a = L.circleMarker(\\n [42.73635845737364, -73.26610204175164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_23d8f840cca92f82d10e53849dd68f5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33ecc54cf07578ebe7fca7ea141c1633 = $(`<div id="html_33ecc54cf07578ebe7fca7ea141c1633" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23d8f840cca92f82d10e53849dd68f5c.setContent(html_33ecc54cf07578ebe7fca7ea141c1633);\\n \\n \\n\\n circle_marker_68d6abedc4d79aaf9d402dcc329a017a.bindPopup(popup_23d8f840cca92f82d10e53849dd68f5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_3dac848209b88291c54c7c4888aef7f6 = L.circleMarker(\\n [42.73635846395741, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2468dfb08d5f52982915090739bec589 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41e21565909504cc8f2635009ec2b49b = $(`<div id="html_41e21565909504cc8f2635009ec2b49b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2468dfb08d5f52982915090739bec589.setContent(html_41e21565909504cc8f2635009ec2b49b);\\n \\n \\n\\n circle_marker_3dac848209b88291c54c7c4888aef7f6.bindPopup(popup_2468dfb08d5f52982915090739bec589)\\n ;\\n\\n \\n \\n \\n var circle_marker_d2b1c964b1c6b83eb763d998a17af376 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_605964316396069f99d83aa194ab6de1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95ab09c1e1897cfcdefaaa374d4247f0 = $(`<div id="html_95ab09c1e1897cfcdefaaa374d4247f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_605964316396069f99d83aa194ab6de1.setContent(html_95ab09c1e1897cfcdefaaa374d4247f0);\\n \\n \\n\\n circle_marker_d2b1c964b1c6b83eb763d998a17af376.bindPopup(popup_605964316396069f99d83aa194ab6de1)\\n ;\\n\\n \\n \\n \\n var circle_marker_20bbde3842ecb0db7928c6218a510512 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5032a24886666c9dbab7f21f347d3b02 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d33f3119412763de10cceaf5336af937 = $(`<div id="html_d33f3119412763de10cceaf5336af937" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5032a24886666c9dbab7f21f347d3b02.setContent(html_d33f3119412763de10cceaf5336af937);\\n \\n \\n\\n circle_marker_20bbde3842ecb0db7928c6218a510512.bindPopup(popup_5032a24886666c9dbab7f21f347d3b02)\\n ;\\n\\n \\n \\n \\n var circle_marker_0dedfff254c88d533c4f7041d21ba242 = L.circleMarker(\\n [42.73635814135235, -73.25626039454548],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5c6e14f99d93907182b9416b3b6f34a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a7081bba6e019860499b3ae944f4c97d = $(`<div id="html_a7081bba6e019860499b3ae944f4c97d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c6e14f99d93907182b9416b3b6f34a4.setContent(html_a7081bba6e019860499b3ae944f4c97d);\\n \\n \\n\\n circle_marker_0dedfff254c88d533c4f7041d21ba242.bindPopup(popup_5c6e14f99d93907182b9416b3b6f34a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_afebcd76880a121ded13cf61740ad613 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_46636d517d85fb7fc6c11674301cefc7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_27d53ac0f5cb3f206a9d08311f3c813d = $(`<div id="html_27d53ac0f5cb3f206a9d08311f3c813d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46636d517d85fb7fc6c11674301cefc7.setContent(html_27d53ac0f5cb3f206a9d08311f3c813d);\\n \\n \\n\\n circle_marker_afebcd76880a121ded13cf61740ad613.bindPopup(popup_46636d517d85fb7fc6c11674301cefc7)\\n ;\\n\\n \\n \\n \\n var circle_marker_4870d6b0699ce96d45903f53be6c5f40 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_227362b576b91ce3036e13a6de865340 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b7147c64d75abd4ebeb11d62e100e540 = $(`<div id="html_b7147c64d75abd4ebeb11d62e100e540" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_227362b576b91ce3036e13a6de865340.setContent(html_b7147c64d75abd4ebeb11d62e100e540);\\n \\n \\n\\n circle_marker_4870d6b0699ce96d45903f53be6c5f40.bindPopup(popup_227362b576b91ce3036e13a6de865340)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d973ca024f8064c5a7a4be91a3e7c57 = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_141af3d5cc14e03cd2b57f0c0f7ce570 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c2465de118fc10f3282b401f6564ab1 = $(`<div id="html_2c2465de118fc10f3282b401f6564ab1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_141af3d5cc14e03cd2b57f0c0f7ce570.setContent(html_2c2465de118fc10f3282b401f6564ab1);\\n \\n \\n\\n circle_marker_8d973ca024f8064c5a7a4be91a3e7c57.bindPopup(popup_141af3d5cc14e03cd2b57f0c0f7ce570)\\n ;\\n\\n \\n \\n \\n var circle_marker_964dec29326e7622f9b7591020fb5337 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_cfa220e3216708b9697b4b78a968b5ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_493a4024bbe1c205e5335f5940d0bb4d = $(`<div id="html_493a4024bbe1c205e5335f5940d0bb4d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfa220e3216708b9697b4b78a968b5ee.setContent(html_493a4024bbe1c205e5335f5940d0bb4d);\\n \\n \\n\\n circle_marker_964dec29326e7622f9b7591020fb5337.bindPopup(popup_cfa220e3216708b9697b4b78a968b5ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_256403040aaecf7d92a00406c0c01380 = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_e9b4eca1f5b9e2ba8b819c0f278ce3a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67deddd1bf66d6c53a753618c97c959f = $(`<div id="html_67deddd1bf66d6c53a753618c97c959f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9b4eca1f5b9e2ba8b819c0f278ce3a0.setContent(html_67deddd1bf66d6c53a753618c97c959f);\\n \\n \\n\\n circle_marker_256403040aaecf7d92a00406c0c01380.bindPopup(popup_e9b4eca1f5b9e2ba8b819c0f278ce3a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4b96fa602ee16b6cbb7609e933a7eb1 = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a6d928e58d28ced69f1fc7cdb40cbe3a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44d2e4da5d0f8f415b65b6ae06de8d23 = $(`<div id="html_44d2e4da5d0f8f415b65b6ae06de8d23" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a6d928e58d28ced69f1fc7cdb40cbe3a.setContent(html_44d2e4da5d0f8f415b65b6ae06de8d23);\\n \\n \\n\\n circle_marker_c4b96fa602ee16b6cbb7609e933a7eb1.bindPopup(popup_a6d928e58d28ced69f1fc7cdb40cbe3a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e09f24adfecda6375e25c82e5d96cf35 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_41833d74cb638115f233b9ed7bf5fc23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0fae75b28c8d0a81ce2254a51a95c3b4 = $(`<div id="html_0fae75b28c8d0a81ce2254a51a95c3b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41833d74cb638115f233b9ed7bf5fc23.setContent(html_0fae75b28c8d0a81ce2254a51a95c3b4);\\n \\n \\n\\n circle_marker_e09f24adfecda6375e25c82e5d96cf35.bindPopup(popup_41833d74cb638115f233b9ed7bf5fc23)\\n ;\\n\\n \\n \\n \\n var circle_marker_b12afc68f4fa52674edc24715ea5a806 = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_891018ad5e6594f945f747fdb25eabb0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bcf8be3d0175150f38491b8be01c5c80 = $(`<div id="html_bcf8be3d0175150f38491b8be01c5c80" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_891018ad5e6594f945f747fdb25eabb0.setContent(html_bcf8be3d0175150f38491b8be01c5c80);\\n \\n \\n\\n circle_marker_b12afc68f4fa52674edc24715ea5a806.bindPopup(popup_891018ad5e6594f945f747fdb25eabb0)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0a87a523550e8f0bed8c770be3d7d9d = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a53e1dd89039305cb0af1f8f945d9582 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b36a86aec20e4274cde2a823d2dfe0e7 = $(`<div id="html_b36a86aec20e4274cde2a823d2dfe0e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a53e1dd89039305cb0af1f8f945d9582.setContent(html_b36a86aec20e4274cde2a823d2dfe0e7);\\n \\n \\n\\n circle_marker_d0a87a523550e8f0bed8c770be3d7d9d.bindPopup(popup_a53e1dd89039305cb0af1f8f945d9582)\\n ;\\n\\n \\n \\n \\n var circle_marker_39fd1438dec59fc808e01fd7b367c3b2 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_133a1225adab5ab2c1b567d46652af62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ff8b8f90d39b7be710aa45fd93d9e09 = $(`<div id="html_0ff8b8f90d39b7be710aa45fd93d9e09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_133a1225adab5ab2c1b567d46652af62.setContent(html_0ff8b8f90d39b7be710aa45fd93d9e09);\\n \\n \\n\\n circle_marker_39fd1438dec59fc808e01fd7b367c3b2.bindPopup(popup_133a1225adab5ab2c1b567d46652af62)\\n ;\\n\\n \\n \\n \\n var circle_marker_8be9a017400d4899df5b8068af8c8025 = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1ec6b344256b911b0b69f1d737826eee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8d2f4716f227f7511b8e12692d9f756 = $(`<div id="html_c8d2f4716f227f7511b8e12692d9f756" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ec6b344256b911b0b69f1d737826eee.setContent(html_c8d2f4716f227f7511b8e12692d9f756);\\n \\n \\n\\n circle_marker_8be9a017400d4899df5b8068af8c8025.bindPopup(popup_1ec6b344256b911b0b69f1d737826eee)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb9c15a97eb76f5bd3cbfd617e9e1177 = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_aaaf4f2043e4ccb24e578e14dcc47ed0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f0d8930ff02f60b5e303f928d997ef60 = $(`<div id="html_f0d8930ff02f60b5e303f928d997ef60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aaaf4f2043e4ccb24e578e14dcc47ed0.setContent(html_f0d8930ff02f60b5e303f928d997ef60);\\n \\n \\n\\n circle_marker_eb9c15a97eb76f5bd3cbfd617e9e1177.bindPopup(popup_aaaf4f2043e4ccb24e578e14dcc47ed0)\\n ;\\n\\n \\n \\n \\n var circle_marker_68f685c3f715f2397f0f7437d5dd1b84 = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_eebf7d3b7a53d69bcc4f89cd15fd10aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99c2cc76695b29a3c2bfbd7781af7b33 = $(`<div id="html_99c2cc76695b29a3c2bfbd7781af7b33" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eebf7d3b7a53d69bcc4f89cd15fd10aa.setContent(html_99c2cc76695b29a3c2bfbd7781af7b33);\\n \\n \\n\\n circle_marker_68f685c3f715f2397f0f7437d5dd1b84.bindPopup(popup_eebf7d3b7a53d69bcc4f89cd15fd10aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_26ecf1d5a158d8f574c2392a2cfe49fe = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_22a8ea367918eb3117ea0cbc0ece9967 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67c23aa42bb8f119044a9a51671e055d = $(`<div id="html_67c23aa42bb8f119044a9a51671e055d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22a8ea367918eb3117ea0cbc0ece9967.setContent(html_67c23aa42bb8f119044a9a51671e055d);\\n \\n \\n\\n circle_marker_26ecf1d5a158d8f574c2392a2cfe49fe.bindPopup(popup_22a8ea367918eb3117ea0cbc0ece9967)\\n ;\\n\\n \\n \\n \\n var circle_marker_896ccadb321f9b7e3bd86c1455dc70f6 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_81168a869bf4d91b818b251e26d1d1ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd30c0da6bfd7e61a02b40aebeb61be1 = $(`<div id="html_bd30c0da6bfd7e61a02b40aebeb61be1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81168a869bf4d91b818b251e26d1d1ec.setContent(html_bd30c0da6bfd7e61a02b40aebeb61be1);\\n \\n \\n\\n circle_marker_896ccadb321f9b7e3bd86c1455dc70f6.bindPopup(popup_81168a869bf4d91b818b251e26d1d1ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_76c62bb23927a11cad44df151f70dd53 = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_9191d2d80511f0f8a2019072b8aeae17 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f742b6633f4fdcd1cfd0a4ed1e6a2cea = $(`<div id="html_f742b6633f4fdcd1cfd0a4ed1e6a2cea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9191d2d80511f0f8a2019072b8aeae17.setContent(html_f742b6633f4fdcd1cfd0a4ed1e6a2cea);\\n \\n \\n\\n circle_marker_76c62bb23927a11cad44df151f70dd53.bindPopup(popup_9191d2d80511f0f8a2019072b8aeae17)\\n ;\\n\\n \\n \\n \\n var circle_marker_773d67859e45be316242ade56a3a0b5a = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ac5118cf77853a89bce30f588341b8f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d332118b6b0ef2528bdc2d1a40bc667 = $(`<div id="html_9d332118b6b0ef2528bdc2d1a40bc667" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac5118cf77853a89bce30f588341b8f5.setContent(html_9d332118b6b0ef2528bdc2d1a40bc667);\\n \\n \\n\\n circle_marker_773d67859e45be316242ade56a3a0b5a.bindPopup(popup_ac5118cf77853a89bce30f588341b8f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a90f4811bf2bc910f242183a22b927d0 = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_09cbb2cce6b180ceef0a595cfe6972a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_622f73696f3ba6b37792ecf70f97fa06 = $(`<div id="html_622f73696f3ba6b37792ecf70f97fa06" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09cbb2cce6b180ceef0a595cfe6972a1.setContent(html_622f73696f3ba6b37792ecf70f97fa06);\\n \\n \\n\\n circle_marker_a90f4811bf2bc910f242183a22b927d0.bindPopup(popup_09cbb2cce6b180ceef0a595cfe6972a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_835c97d8c5d796738f2ca3eb8bb1c60f = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_3ca2cd1c8d20a7a2bc54e60d34403df7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ade60fc5baf6d1ed8b88afb12771bee7 = $(`<div id="html_ade60fc5baf6d1ed8b88afb12771bee7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ca2cd1c8d20a7a2bc54e60d34403df7.setContent(html_ade60fc5baf6d1ed8b88afb12771bee7);\\n \\n \\n\\n circle_marker_835c97d8c5d796738f2ca3eb8bb1c60f.bindPopup(popup_3ca2cd1c8d20a7a2bc54e60d34403df7)\\n ;\\n\\n \\n \\n \\n var circle_marker_3bb94ad5785e2fd999a9139fd267bdc4 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_b689580e0493204e2354a67f93bc1f8a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42a56f0d6a89cbf2e04be95ac97fa02e = $(`<div id="html_42a56f0d6a89cbf2e04be95ac97fa02e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b689580e0493204e2354a67f93bc1f8a.setContent(html_42a56f0d6a89cbf2e04be95ac97fa02e);\\n \\n \\n\\n circle_marker_3bb94ad5785e2fd999a9139fd267bdc4.bindPopup(popup_b689580e0493204e2354a67f93bc1f8a)\\n ;\\n\\n \\n \\n \\n var circle_marker_efeceb017f3df97339d86e4b273800e3 = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5491aff54964f14b07d122d3b8e9b520 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ba87b8ca9ea305c7be126c536d4b8e2 = $(`<div id="html_1ba87b8ca9ea305c7be126c536d4b8e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5491aff54964f14b07d122d3b8e9b520.setContent(html_1ba87b8ca9ea305c7be126c536d4b8e2);\\n \\n \\n\\n circle_marker_efeceb017f3df97339d86e4b273800e3.bindPopup(popup_5491aff54964f14b07d122d3b8e9b520)\\n ;\\n\\n \\n \\n \\n var circle_marker_b98a6937b92b5f55de087fe46fafc698 = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_3426bf9dcaa460500bab3048ced68646 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ade8e9c21d4802004a91574e2bbef59 = $(`<div id="html_1ade8e9c21d4802004a91574e2bbef59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3426bf9dcaa460500bab3048ced68646.setContent(html_1ade8e9c21d4802004a91574e2bbef59);\\n \\n \\n\\n circle_marker_b98a6937b92b5f55de087fe46fafc698.bindPopup(popup_3426bf9dcaa460500bab3048ced68646)\\n ;\\n\\n \\n \\n \\n var circle_marker_4f714e104a988eb3700e45a3a1cd3719 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ef9086b44e538e945963b3546d733161 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41b861a01fee0501b33b83acef344094 = $(`<div id="html_41b861a01fee0501b33b83acef344094" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef9086b44e538e945963b3546d733161.setContent(html_41b861a01fee0501b33b83acef344094);\\n \\n \\n\\n circle_marker_4f714e104a988eb3700e45a3a1cd3719.bindPopup(popup_ef9086b44e538e945963b3546d733161)\\n ;\\n\\n \\n \\n \\n var circle_marker_5540615f14a25f208f7500df18176e45 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ee9ec7ab99472c3e6dab9c66e292ecde = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8cf9e05fc9cf143044c4e89956ef4a5e = $(`<div id="html_8cf9e05fc9cf143044c4e89956ef4a5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee9ec7ab99472c3e6dab9c66e292ecde.setContent(html_8cf9e05fc9cf143044c4e89956ef4a5e);\\n \\n \\n\\n circle_marker_5540615f14a25f208f7500df18176e45.bindPopup(popup_ee9ec7ab99472c3e6dab9c66e292ecde)\\n ;\\n\\n \\n \\n \\n var circle_marker_76aa3d02c4bcd33a1e54e7ff211f8172 = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_0c6b67d5d84abb76eb379ebcf47140d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_208676c70185e58863bdf7766c045f41 = $(`<div id="html_208676c70185e58863bdf7766c045f41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c6b67d5d84abb76eb379ebcf47140d4.setContent(html_208676c70185e58863bdf7766c045f41);\\n \\n \\n\\n circle_marker_76aa3d02c4bcd33a1e54e7ff211f8172.bindPopup(popup_0c6b67d5d84abb76eb379ebcf47140d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1c4b7769dcfec9f417758891348359c = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c1fe966e2f09d7ddad526689185faf15 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e12bf42b45709aba5b168e01e779ebd7 = $(`<div id="html_e12bf42b45709aba5b168e01e779ebd7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c1fe966e2f09d7ddad526689185faf15.setContent(html_e12bf42b45709aba5b168e01e779ebd7);\\n \\n \\n\\n circle_marker_f1c4b7769dcfec9f417758891348359c.bindPopup(popup_c1fe966e2f09d7ddad526689185faf15)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb2ef1d38871f6c2e67761f28231a24d = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_bc93da1e26e0180d33e7a87f7742f79f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2b63679b0f32a59e390578dde3c58a3 = $(`<div id="html_f2b63679b0f32a59e390578dde3c58a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc93da1e26e0180d33e7a87f7742f79f.setContent(html_f2b63679b0f32a59e390578dde3c58a3);\\n \\n \\n\\n circle_marker_eb2ef1d38871f6c2e67761f28231a24d.bindPopup(popup_bc93da1e26e0180d33e7a87f7742f79f)\\n ;\\n\\n \\n \\n \\n var circle_marker_a40de5ce6c121bfd5483709f10c89bf4 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_e2951caca0c55b4d4b038c59949abdfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_567c1580ed3819e6e1e3a95760b6bd8a = $(`<div id="html_567c1580ed3819e6e1e3a95760b6bd8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2951caca0c55b4d4b038c59949abdfe.setContent(html_567c1580ed3819e6e1e3a95760b6bd8a);\\n \\n \\n\\n circle_marker_a40de5ce6c121bfd5483709f10c89bf4.bindPopup(popup_e2951caca0c55b4d4b038c59949abdfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_22c7cb0e49498780e0eba3f495c508a4 = L.circleMarker(\\n [42.7327441379601, -73.26856223845904],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2f2d5c65befbe8f0bdfebba7125cc4e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_822f38b79025e0030945ca197cba539c = $(`<div id="html_822f38b79025e0030945ca197cba539c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f2d5c65befbe8f0bdfebba7125cc4e3.setContent(html_822f38b79025e0030945ca197cba539c);\\n \\n \\n\\n circle_marker_22c7cb0e49498780e0eba3f495c508a4.bindPopup(popup_2f2d5c65befbe8f0bdfebba7125cc4e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_97e0e84980d0a045e8ec99e57af5cc3c = L.circleMarker(\\n [42.73274417087632, -73.26733210425597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5490f09cf7f84553705319993dcec466 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c243d793b0cce7d1f8cc43b5d9e23772 = $(`<div id="html_c243d793b0cce7d1f8cc43b5d9e23772" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5490f09cf7f84553705319993dcec466.setContent(html_c243d793b0cce7d1f8cc43b5d9e23772);\\n \\n \\n\\n circle_marker_97e0e84980d0a045e8ec99e57af5cc3c.bindPopup(popup_5490f09cf7f84553705319993dcec466)\\n ;\\n\\n \\n \\n \\n var circle_marker_486bd67cc5a00db99b60d39fde3881e2 = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_aa7cb46aa341f3db2bc583cebc785f58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2aa7a247c3094334d91b514c624b15f = $(`<div id="html_a2aa7a247c3094334d91b514c624b15f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aa7cb46aa341f3db2bc583cebc785f58.setContent(html_a2aa7a247c3094334d91b514c624b15f);\\n \\n \\n\\n circle_marker_486bd67cc5a00db99b60d39fde3881e2.bindPopup(popup_aa7cb46aa341f3db2bc583cebc785f58)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a3c677a3c5f9933a4a88250704da60b = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_b8a4684b06bdeeb73923245bb5c2f61a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d53bf9dad95c6530f9125aaa9ced0704 = $(`<div id="html_d53bf9dad95c6530f9125aaa9ced0704" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b8a4684b06bdeeb73923245bb5c2f61a.setContent(html_d53bf9dad95c6530f9125aaa9ced0704);\\n \\n \\n\\n circle_marker_3a3c677a3c5f9933a4a88250704da60b.bindPopup(popup_b8a4684b06bdeeb73923245bb5c2f61a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5e6e188e62ea548b6bccc32494296d3 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ed3dc0cf36d2838496ef9a0179a274f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74cf6807d19dce81575d71d404d39800 = $(`<div id="html_74cf6807d19dce81575d71d404d39800" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed3dc0cf36d2838496ef9a0179a274f6.setContent(html_74cf6807d19dce81575d71d404d39800);\\n \\n \\n\\n circle_marker_a5e6e188e62ea548b6bccc32494296d3.bindPopup(popup_ed3dc0cf36d2838496ef9a0179a274f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_12ce055a1288599be31690eb59a60adb = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ed63439b19de8a5741e1c2318d161761 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_133c34e8fc14426d31b3b1e16a80aaef = $(`<div id="html_133c34e8fc14426d31b3b1e16a80aaef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed63439b19de8a5741e1c2318d161761.setContent(html_133c34e8fc14426d31b3b1e16a80aaef);\\n \\n \\n\\n circle_marker_12ce055a1288599be31690eb59a60adb.bindPopup(popup_ed63439b19de8a5741e1c2318d161761)\\n ;\\n\\n \\n \\n \\n var circle_marker_4491ab167369a153e4d8a9fda4d4f254 = L.circleMarker(\\n [42.73274377588172, -73.25503076225394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_96ad9d85b7a61fa292d91428898b6037 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad03ec60cff85b24beb3f4eb79e13f43 = $(`<div id="html_ad03ec60cff85b24beb3f4eb79e13f43" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96ad9d85b7a61fa292d91428898b6037.setContent(html_ad03ec60cff85b24beb3f4eb79e13f43);\\n \\n \\n\\n circle_marker_4491ab167369a153e4d8a9fda4d4f254.bindPopup(popup_96ad9d85b7a61fa292d91428898b6037)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7a5bfacc8627c223103b1080bca269b = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c22f44073d7c2ac89cbf8c0f5738dc33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9ae74df94d83c4cb48b2df6fc7611cb = $(`<div id="html_d9ae74df94d83c4cb48b2df6fc7611cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c22f44073d7c2ac89cbf8c0f5738dc33.setContent(html_d9ae74df94d83c4cb48b2df6fc7611cb);\\n \\n \\n\\n circle_marker_f7a5bfacc8627c223103b1080bca269b.bindPopup(popup_c22f44073d7c2ac89cbf8c0f5738dc33)\\n ;\\n\\n \\n \\n \\n var circle_marker_f67a16fcb62b9c942abba1c8f9990ae2 = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_adabc70087f03eb782ef1160a7fc04b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4db2a9d6797acf0c7d5f1790f2088050 = $(`<div id="html_4db2a9d6797acf0c7d5f1790f2088050" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_adabc70087f03eb782ef1160a7fc04b3.setContent(html_4db2a9d6797acf0c7d5f1790f2088050);\\n \\n \\n\\n circle_marker_f67a16fcb62b9c942abba1c8f9990ae2.bindPopup(popup_adabc70087f03eb782ef1160a7fc04b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6d934b603f373012f879df16aa13af1 = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_698427a87a7c0539fc12cc0efa35bdda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f05f8056f99c3ae648ec0d210510fb29 = $(`<div id="html_f05f8056f99c3ae648ec0d210510fb29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_698427a87a7c0539fc12cc0efa35bdda.setContent(html_f05f8056f99c3ae648ec0d210510fb29);\\n \\n \\n\\n circle_marker_e6d934b603f373012f879df16aa13af1.bindPopup(popup_698427a87a7c0539fc12cc0efa35bdda)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd16863b6a91edb98ace0e60bd79babf = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c654471f93f6642a3065c0cfcbdcdd35 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f01d23061c1859dbb08790bfbf05526 = $(`<div id="html_1f01d23061c1859dbb08790bfbf05526" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c654471f93f6642a3065c0cfcbdcdd35.setContent(html_1f01d23061c1859dbb08790bfbf05526);\\n \\n \\n\\n circle_marker_fd16863b6a91edb98ace0e60bd79babf.bindPopup(popup_c654471f93f6642a3065c0cfcbdcdd35)\\n ;\\n\\n \\n \\n \\n var circle_marker_154e094d82404d99bcfe4a67f1cdb071 = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_0469fdf1758b51224c1c5a7db18944d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2cba517d2b3460cf3684033c6b95fd0 = $(`<div id="html_f2cba517d2b3460cf3684033c6b95fd0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0469fdf1758b51224c1c5a7db18944d7.setContent(html_f2cba517d2b3460cf3684033c6b95fd0);\\n \\n \\n\\n circle_marker_154e094d82404d99bcfe4a67f1cdb071.bindPopup(popup_0469fdf1758b51224c1c5a7db18944d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e27b6fd26f2c826b11b4ad24b9a710a = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ca16cd87a2a84e189d0b2b3bb2a31613 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14d65e426c4ce6d4e2606c2ea241bfa5 = $(`<div id="html_14d65e426c4ce6d4e2606c2ea241bfa5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca16cd87a2a84e189d0b2b3bb2a31613.setContent(html_14d65e426c4ce6d4e2606c2ea241bfa5);\\n \\n \\n\\n circle_marker_9e27b6fd26f2c826b11b4ad24b9a710a.bindPopup(popup_ca16cd87a2a84e189d0b2b3bb2a31613)\\n ;\\n\\n \\n \\n \\n var circle_marker_151442156241dc4ba972a5b9e84b3807 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_6280a00456fb8041cc745be1643076ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e295ea8eb8030d8f51fbaca003b70fbb = $(`<div id="html_e295ea8eb8030d8f51fbaca003b70fbb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6280a00456fb8041cc745be1643076ce.setContent(html_e295ea8eb8030d8f51fbaca003b70fbb);\\n \\n \\n\\n circle_marker_151442156241dc4ba972a5b9e84b3807.bindPopup(popup_6280a00456fb8041cc745be1643076ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_854ad86ca9ed68517e8fc88ae38edd7a = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_9ea6232549beedec3e48162b8015b201 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6103d05decba8cc3be39576eb3a68d1f = $(`<div id="html_6103d05decba8cc3be39576eb3a68d1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ea6232549beedec3e48162b8015b201.setContent(html_6103d05decba8cc3be39576eb3a68d1f);\\n \\n \\n\\n circle_marker_854ad86ca9ed68517e8fc88ae38edd7a.bindPopup(popup_9ea6232549beedec3e48162b8015b201)\\n ;\\n\\n \\n \\n \\n var circle_marker_534deb14c3adb0d2af14aeb93bdcd372 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_e0c174224cb10bb674d69f887ffb6ab9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33f7ea4c2f5ea984e749a687b60fc711 = $(`<div id="html_33f7ea4c2f5ea984e749a687b60fc711" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0c174224cb10bb674d69f887ffb6ab9.setContent(html_33f7ea4c2f5ea984e749a687b60fc711);\\n \\n \\n\\n circle_marker_534deb14c3adb0d2af14aeb93bdcd372.bindPopup(popup_e0c174224cb10bb674d69f887ffb6ab9)\\n ;\\n\\n \\n \\n \\n var circle_marker_dad7a9ad06f19202a49e42ce516d2b55 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_59628ba31b9e989d2fa27dabddd507ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2420f54ec752878caa7cdf58585a4207 = $(`<div id="html_2420f54ec752878caa7cdf58585a4207" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_59628ba31b9e989d2fa27dabddd507ba.setContent(html_2420f54ec752878caa7cdf58585a4207);\\n \\n \\n\\n circle_marker_dad7a9ad06f19202a49e42ce516d2b55.bindPopup(popup_59628ba31b9e989d2fa27dabddd507ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_114671a2616f69580986e1dc592cc39b = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_da39e0aa756f4263c70efe9067d2e1e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5fb6dbb16717c198f3d9f2899bd6c793 = $(`<div id="html_5fb6dbb16717c198f3d9f2899bd6c793" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_da39e0aa756f4263c70efe9067d2e1e8.setContent(html_5fb6dbb16717c198f3d9f2899bd6c793);\\n \\n \\n\\n circle_marker_114671a2616f69580986e1dc592cc39b.bindPopup(popup_da39e0aa756f4263c70efe9067d2e1e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a1cb92a4fef435bc80ec2b30f27e519 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_6b9bd306072838a7766e8afb6c74fa0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60071d511f2524c991cff62631d5844a = $(`<div id="html_60071d511f2524c991cff62631d5844a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b9bd306072838a7766e8afb6c74fa0d.setContent(html_60071d511f2524c991cff62631d5844a);\\n \\n \\n\\n circle_marker_2a1cb92a4fef435bc80ec2b30f27e519.bindPopup(popup_6b9bd306072838a7766e8afb6c74fa0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_63366e021a1587971d0043dbec1c2369 = L.circleMarker(\\n [42.730936958507606, -73.26979222927841],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_d354b22a45a1784a1e061a4672e35a0e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ccc4382cd5b4e4daf5f090b39150c5f = $(`<div id="html_1ccc4382cd5b4e4daf5f090b39150c5f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d354b22a45a1784a1e061a4672e35a0e.setContent(html_1ccc4382cd5b4e4daf5f090b39150c5f);\\n \\n \\n\\n circle_marker_63366e021a1587971d0043dbec1c2369.bindPopup(popup_d354b22a45a1784a1e061a4672e35a0e)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a5bfede6ad2b994ff250e8497dfafda = L.circleMarker(\\n [42.73093705725226, -73.26610193420632],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ff80f445b817cfa84080a566d4fb9c32 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_914b6859228f93b434eef666d26573ef = $(`<div id="html_914b6859228f93b434eef666d26573ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff80f445b817cfa84080a566d4fb9c32.setContent(html_914b6859228f93b434eef666d26573ef);\\n \\n \\n\\n circle_marker_4a5bfede6ad2b994ff250e8497dfafda.bindPopup(popup_ff80f445b817cfa84080a566d4fb9c32)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4a651f70ce4f1fd429c147778666007 = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c46b5967c0eb775594c1a0f39ba17f6c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87dd0ca5c61cea9b472aedcf39387f2f = $(`<div id="html_87dd0ca5c61cea9b472aedcf39387f2f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c46b5967c0eb775594c1a0f39ba17f6c.setContent(html_87dd0ca5c61cea9b472aedcf39387f2f);\\n \\n \\n\\n circle_marker_a4a651f70ce4f1fd429c147778666007.bindPopup(popup_c46b5967c0eb775594c1a0f39ba17f6c)\\n ;\\n\\n \\n \\n \\n var circle_marker_92c961a29c1195aaa951aaf84385f307 = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_99d32b6afa1a15f8d2297fa8b9882635 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_853e645a1c49cfa5a024f656a1fb0d5e = $(`<div id="html_853e645a1c49cfa5a024f656a1fb0d5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99d32b6afa1a15f8d2297fa8b9882635.setContent(html_853e645a1c49cfa5a024f656a1fb0d5e);\\n \\n \\n\\n circle_marker_92c961a29c1195aaa951aaf84385f307.bindPopup(popup_99d32b6afa1a15f8d2297fa8b9882635)\\n ;\\n\\n \\n \\n \\n var circle_marker_c115663d9d280e0bad39a1fe833a9bba = L.circleMarker(\\n [42.730936958507606, -73.259951442416],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_43a5a7a8e05afbd139efa5b4f5f76672 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b23d3e2a6b680f5149319eedd86a3534 = $(`<div id="html_b23d3e2a6b680f5149319eedd86a3534" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43a5a7a8e05afbd139efa5b4f5f76672.setContent(html_b23d3e2a6b680f5149319eedd86a3534);\\n \\n \\n\\n circle_marker_c115663d9d280e0bad39a1fe833a9bba.bindPopup(popup_43a5a7a8e05afbd139efa5b4f5f76672)\\n ;\\n\\n \\n \\n \\n var circle_marker_d269f2ccd821660a88f16c6cd86a6843 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_f456e6eaae9e1f6e4c4aafb1ae735fe7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9caa2a1afb779435c27d15dfdfaeff8c = $(`<div id="html_9caa2a1afb779435c27d15dfdfaeff8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f456e6eaae9e1f6e4c4aafb1ae735fe7.setContent(html_9caa2a1afb779435c27d15dfdfaeff8c);\\n \\n \\n\\n circle_marker_d269f2ccd821660a88f16c6cd86a6843.bindPopup(popup_f456e6eaae9e1f6e4c4aafb1ae735fe7)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7f0d7c521f901a9dbd1142052b552bf = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_12cf261f7f8fd0d2f4100bc6f7f6b7fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a5270a7d8757f10a3e7ad1cfefab4ba = $(`<div id="html_8a5270a7d8757f10a3e7ad1cfefab4ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12cf261f7f8fd0d2f4100bc6f7f6b7fe.setContent(html_8a5270a7d8757f10a3e7ad1cfefab4ba);\\n \\n \\n\\n circle_marker_e7f0d7c521f901a9dbd1142052b552bf.bindPopup(popup_12cf261f7f8fd0d2f4100bc6f7f6b7fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a1b0a1745e9fba08ef4f852cd9ccd63 = L.circleMarker(\\n [42.73093640553757, -73.2525708523423],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_bcbadeea3948f8d78c92e67af8a8d28c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a869774574b37916aa013c1dbd1f590 = $(`<div id="html_1a869774574b37916aa013c1dbd1f590" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bcbadeea3948f8d78c92e67af8a8d28c.setContent(html_1a869774574b37916aa013c1dbd1f590);\\n \\n \\n\\n circle_marker_4a1b0a1745e9fba08ef4f852cd9ccd63.bindPopup(popup_bcbadeea3948f8d78c92e67af8a8d28c)\\n ;\\n\\n \\n \\n \\n var circle_marker_6855e2b67724dd96854548e1a046f844 = L.circleMarker(\\n [42.73093595131219, -73.24888055736892],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_4f5512cab0e04bccde6d0d9dde658bb6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_93a33e7beaed21962349b331d961b55c = $(`<div id="html_93a33e7beaed21962349b331d961b55c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f5512cab0e04bccde6d0d9dde658bb6.setContent(html_93a33e7beaed21962349b331d961b55c);\\n \\n \\n\\n circle_marker_6855e2b67724dd96854548e1a046f844.bindPopup(popup_4f5512cab0e04bccde6d0d9dde658bb6)\\n ;\\n\\n \\n \\n \\n var circle_marker_901e586fd18ae2e6381317fa8c46428a = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a4088fed7a3f57c293ce3c9730c8628a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b5a6168f685b09ac9e21ff3bfd3787e = $(`<div id="html_7b5a6168f685b09ac9e21ff3bfd3787e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4088fed7a3f57c293ce3c9730c8628a.setContent(html_7b5a6168f685b09ac9e21ff3bfd3787e);\\n \\n \\n\\n circle_marker_901e586fd18ae2e6381317fa8c46428a.bindPopup(popup_a4088fed7a3f57c293ce3c9730c8628a)\\n ;\\n\\n \\n \\n \\n var circle_marker_571ad3f2defaab7cd8f247f922a892e9 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_9dba9e34f3bd91b7212fafc0a7008af0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bce25a1b0b2cb504535f7e517112113e = $(`<div id="html_bce25a1b0b2cb504535f7e517112113e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9dba9e34f3bd91b7212fafc0a7008af0.setContent(html_bce25a1b0b2cb504535f7e517112113e);\\n \\n \\n\\n circle_marker_571ad3f2defaab7cd8f247f922a892e9.bindPopup(popup_9dba9e34f3bd91b7212fafc0a7008af0)\\n ;\\n\\n \\n \\n \\n var circle_marker_3558414c4daf7c351f46bc41a02d9b6e = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_e4d2eeba006384ab354a4e8795972eac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6eafa3f824a8ece347ff7bff1c0f7949 = $(`<div id="html_6eafa3f824a8ece347ff7bff1c0f7949" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4d2eeba006384ab354a4e8795972eac.setContent(html_6eafa3f824a8ece347ff7bff1c0f7949);\\n \\n \\n\\n circle_marker_3558414c4daf7c351f46bc41a02d9b6e.bindPopup(popup_e4d2eeba006384ab354a4e8795972eac)\\n ;\\n\\n \\n \\n \\n var circle_marker_9add953736c6b33089b73d640885f6b6 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_6a86871455124265840771853d093f24 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d05d1924d2ba61555ba055badbe19c9e = $(`<div id="html_d05d1924d2ba61555ba055badbe19c9e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a86871455124265840771853d093f24.setContent(html_d05d1924d2ba61555ba055badbe19c9e);\\n \\n \\n\\n circle_marker_9add953736c6b33089b73d640885f6b6.bindPopup(popup_6a86871455124265840771853d093f24)\\n ;\\n\\n \\n \\n \\n var circle_marker_563af4d5360948f7f7f73933bdf16dfe = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_87c32d791ac82d4494beec36aa170f81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b98197db3ae10679995013614f62881 = $(`<div id="html_9b98197db3ae10679995013614f62881" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_87c32d791ac82d4494beec36aa170f81.setContent(html_9b98197db3ae10679995013614f62881);\\n \\n \\n\\n circle_marker_563af4d5360948f7f7f73933bdf16dfe.bindPopup(popup_87c32d791ac82d4494beec36aa170f81)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d725b19c726d4729dac4d224aa953dc = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_aaaf03251a02362fe66dc5b1c0ff4bb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78cc9e8462705041966aac563a4b0c01 = $(`<div id="html_78cc9e8462705041966aac563a4b0c01" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aaaf03251a02362fe66dc5b1c0ff4bb1.setContent(html_78cc9e8462705041966aac563a4b0c01);\\n \\n \\n\\n circle_marker_5d725b19c726d4729dac4d224aa953dc.bindPopup(popup_aaaf03251a02362fe66dc5b1c0ff4bb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_8070418da931b42349686553626929e0 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_81e6c1da07f6f94b6ad69313117325c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8455168bd7ba16cdf9ba85cd9d4c5313 = $(`<div id="html_8455168bd7ba16cdf9ba85cd9d4c5313" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81e6c1da07f6f94b6ad69313117325c9.setContent(html_8455168bd7ba16cdf9ba85cd9d4c5313);\\n \\n \\n\\n circle_marker_8070418da931b42349686553626929e0.bindPopup(popup_81e6c1da07f6f94b6ad69313117325c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9bea7d97d4baa3e4a6817fffd6ab5186 = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_e2bddde303e1b26e38befb7dba30398f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5dfcdcce9d55a309ecf3297bf4a60597 = $(`<div id="html_5dfcdcce9d55a309ecf3297bf4a60597" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2bddde303e1b26e38befb7dba30398f.setContent(html_5dfcdcce9d55a309ecf3297bf4a60597);\\n \\n \\n\\n circle_marker_9bea7d97d4baa3e4a6817fffd6ab5186.bindPopup(popup_e2bddde303e1b26e38befb7dba30398f)\\n ;\\n\\n \\n \\n \\n var circle_marker_32d3e1dd644d7a9881845213cf6548cc = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_dcffb10a11eb5b00253ea8333eb8bb2f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55503fa63f6c818fca6aa8bf1d56e602 = $(`<div id="html_55503fa63f6c818fca6aa8bf1d56e602" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dcffb10a11eb5b00253ea8333eb8bb2f.setContent(html_55503fa63f6c818fca6aa8bf1d56e602);\\n \\n \\n\\n circle_marker_32d3e1dd644d7a9881845213cf6548cc.bindPopup(popup_dcffb10a11eb5b00253ea8333eb8bb2f)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc829662081d6c8d83358ec22db97344 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_caff1d8b13802e6a984f8c815fe19c2d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f7b1377cc581ffc2acdc07ae80e3a38 = $(`<div id="html_7f7b1377cc581ffc2acdc07ae80e3a38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_caff1d8b13802e6a984f8c815fe19c2d.setContent(html_7f7b1377cc581ffc2acdc07ae80e3a38);\\n \\n \\n\\n circle_marker_cc829662081d6c8d83358ec22db97344.bindPopup(popup_caff1d8b13802e6a984f8c815fe19c2d)\\n ;\\n\\n \\n \\n \\n var circle_marker_e986def5ef8d35b8ecde6df8e47324f4 = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_15627dd3a30aa2d8b2214750f9544981 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5092727f505b0d5e4a2e3ba4397bafdd = $(`<div id="html_5092727f505b0d5e4a2e3ba4397bafdd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_15627dd3a30aa2d8b2214750f9544981.setContent(html_5092727f505b0d5e4a2e3ba4397bafdd);\\n \\n \\n\\n circle_marker_e986def5ef8d35b8ecde6df8e47324f4.bindPopup(popup_15627dd3a30aa2d8b2214750f9544981)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8de353225f239c6dba567dfb32ef297 = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_54d8b41ebe506813ca013f1bc485e9fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17bf44e5ec63ca68886bda8e642eb5cf = $(`<div id="html_17bf44e5ec63ca68886bda8e642eb5cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54d8b41ebe506813ca013f1bc485e9fd.setContent(html_17bf44e5ec63ca68886bda8e642eb5cf);\\n \\n \\n\\n circle_marker_e8de353225f239c6dba567dfb32ef297.bindPopup(popup_54d8b41ebe506813ca013f1bc485e9fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b2fdfb545841831a641d53aad89f640 = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_bb9e74f60e87d14a88a3bcfd1a69b906 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a563d4614ae6fbbd68c78f146aaa9f2 = $(`<div id="html_9a563d4614ae6fbbd68c78f146aaa9f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb9e74f60e87d14a88a3bcfd1a69b906.setContent(html_9a563d4614ae6fbbd68c78f146aaa9f2);\\n \\n \\n\\n circle_marker_0b2fdfb545841831a641d53aad89f640.bindPopup(popup_bb9e74f60e87d14a88a3bcfd1a69b906)\\n ;\\n\\n \\n \\n \\n var circle_marker_6421739cc82ff0ec9f06fd1c24393fcd = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_0593f81c7ae6dfa7756d6cd3e168d35e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_73f12e66809585adbc5bf137f0ef85c7 = $(`<div id="html_73f12e66809585adbc5bf137f0ef85c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0593f81c7ae6dfa7756d6cd3e168d35e.setContent(html_73f12e66809585adbc5bf137f0ef85c7);\\n \\n \\n\\n circle_marker_6421739cc82ff0ec9f06fd1c24393fcd.bindPopup(popup_0593f81c7ae6dfa7756d6cd3e168d35e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba7c8eff83672d17244d36e49f3b1632 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_0cb66c6fbf7d79ce6c15cff8ef50cb39 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad1db91d46cd2145e474ba3783c42a7e = $(`<div id="html_ad1db91d46cd2145e474ba3783c42a7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cb66c6fbf7d79ce6c15cff8ef50cb39.setContent(html_ad1db91d46cd2145e474ba3783c42a7e);\\n \\n \\n\\n circle_marker_ba7c8eff83672d17244d36e49f3b1632.bindPopup(popup_0cb66c6fbf7d79ce6c15cff8ef50cb39)\\n ;\\n\\n \\n \\n \\n var circle_marker_f9abef37c0f34eff2be6f35b8a5394b3 = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_41f7c7017dc7a51a5efaf2ebf749b64f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33bf8c6b12b4e35f95470b9941cfa47c = $(`<div id="html_33bf8c6b12b4e35f95470b9941cfa47c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41f7c7017dc7a51a5efaf2ebf749b64f.setContent(html_33bf8c6b12b4e35f95470b9941cfa47c);\\n \\n \\n\\n circle_marker_f9abef37c0f34eff2be6f35b8a5394b3.bindPopup(popup_41f7c7017dc7a51a5efaf2ebf749b64f)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f77b928d04b4ae98b69faf6023e5dc2 = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8868d77f487ea03c377c72987821c727 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c291d293c7c4cb26f1abdc4e4089fcb = $(`<div id="html_0c291d293c7c4cb26f1abdc4e4089fcb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8868d77f487ea03c377c72987821c727.setContent(html_0c291d293c7c4cb26f1abdc4e4089fcb);\\n \\n \\n\\n circle_marker_2f77b928d04b4ae98b69faf6023e5dc2.bindPopup(popup_8868d77f487ea03c377c72987821c727)\\n ;\\n\\n \\n \\n \\n var circle_marker_87b67731356be751a8e28f03c0244e6b = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_3388891622c180f9855a2486fd644dbc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e084c22ea0691992ca6deee2328e4adf = $(`<div id="html_e084c22ea0691992ca6deee2328e4adf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3388891622c180f9855a2486fd644dbc.setContent(html_e084c22ea0691992ca6deee2328e4adf);\\n \\n \\n\\n circle_marker_87b67731356be751a8e28f03c0244e6b.bindPopup(popup_3388891622c180f9855a2486fd644dbc)\\n ;\\n\\n \\n \\n \\n var circle_marker_67b5cd70d3c086785064342a33bab686 = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_83e5cfd8ec2a16dec039d156849746b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_badec21cf90c875ed9e1aed01e8c14bf = $(`<div id="html_badec21cf90c875ed9e1aed01e8c14bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83e5cfd8ec2a16dec039d156849746b3.setContent(html_badec21cf90c875ed9e1aed01e8c14bf);\\n \\n \\n\\n circle_marker_67b5cd70d3c086785064342a33bab686.bindPopup(popup_83e5cfd8ec2a16dec039d156849746b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ee9d2876e56ba5b2c555d1088ae36d8 = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_bf78e2ec729448f108092d65a36ac51d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_825d96474dd3e407c11ebaf3186ca258 = $(`<div id="html_825d96474dd3e407c11ebaf3186ca258" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bf78e2ec729448f108092d65a36ac51d.setContent(html_825d96474dd3e407c11ebaf3186ca258);\\n \\n \\n\\n circle_marker_3ee9d2876e56ba5b2c555d1088ae36d8.bindPopup(popup_bf78e2ec729448f108092d65a36ac51d)\\n ;\\n\\n \\n \\n \\n var circle_marker_19f1fcb8554a73e2ebfd86e717c18341 = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_9a37b5d5b324817a1e9783d60756aa8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_216c835596f3e3b3fac1130359936763 = $(`<div id="html_216c835596f3e3b3fac1130359936763" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a37b5d5b324817a1e9783d60756aa8f.setContent(html_216c835596f3e3b3fac1130359936763);\\n \\n \\n\\n circle_marker_19f1fcb8554a73e2ebfd86e717c18341.bindPopup(popup_9a37b5d5b324817a1e9783d60756aa8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_61d36879c78d82021b197dd97c1d47ec = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_93bdbb786e9c90ef93b0a0948be0d643 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_874730c9667287c5742c1c5387e7c28a = $(`<div id="html_874730c9667287c5742c1c5387e7c28a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93bdbb786e9c90ef93b0a0948be0d643.setContent(html_874730c9667287c5742c1c5387e7c28a);\\n \\n \\n\\n circle_marker_61d36879c78d82021b197dd97c1d47ec.bindPopup(popup_93bdbb786e9c90ef93b0a0948be0d643)\\n ;\\n\\n \\n \\n \\n var circle_marker_69df0f8150bb656f7059d69f6207681f = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_588901e70e84d85bce7391000c1d2c08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_133faa713c6b6297d51cfdffda37cfa4 = $(`<div id="html_133faa713c6b6297d51cfdffda37cfa4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_588901e70e84d85bce7391000c1d2c08.setContent(html_133faa713c6b6297d51cfdffda37cfa4);\\n \\n \\n\\n circle_marker_69df0f8150bb656f7059d69f6207681f.bindPopup(popup_588901e70e84d85bce7391000c1d2c08)\\n ;\\n\\n \\n \\n \\n var circle_marker_18f0e0eb2d346284188fbc916c4dfe0d = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_5e349744e0d0bce2e4006d94d29ff1e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ca973d5d893941743afad48644ac419 = $(`<div id="html_5ca973d5d893941743afad48644ac419" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e349744e0d0bce2e4006d94d29ff1e8.setContent(html_5ca973d5d893941743afad48644ac419);\\n \\n \\n\\n circle_marker_18f0e0eb2d346284188fbc916c4dfe0d.bindPopup(popup_5e349744e0d0bce2e4006d94d29ff1e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_71592d4b67ee0732a7b4544fb7a5ffca = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_d3045c3e124044f7b2a69248719852f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1987609cfb4a001c1bbb6cb6dc0accdd = $(`<div id="html_1987609cfb4a001c1bbb6cb6dc0accdd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3045c3e124044f7b2a69248719852f1.setContent(html_1987609cfb4a001c1bbb6cb6dc0accdd);\\n \\n \\n\\n circle_marker_71592d4b67ee0732a7b4544fb7a5ffca.bindPopup(popup_d3045c3e124044f7b2a69248719852f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_572fb378cc079a844761d5264f93f57d = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_4ea9102b610ae0499508b20e574a5f67 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2c471a9917e6666018399b24f352026 = $(`<div id="html_d2c471a9917e6666018399b24f352026" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ea9102b610ae0499508b20e574a5f67.setContent(html_d2c471a9917e6666018399b24f352026);\\n \\n \\n\\n circle_marker_572fb378cc079a844761d5264f93f57d.bindPopup(popup_4ea9102b610ae0499508b20e574a5f67)\\n ;\\n\\n \\n \\n \\n var circle_marker_64852b8408310bd45e452cc7c371c6ed = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_62657e6f1dbd361fcdf7e4e4b1a604fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6bb17034614fde5149ee3eb474313ed2 = $(`<div id="html_6bb17034614fde5149ee3eb474313ed2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62657e6f1dbd361fcdf7e4e4b1a604fa.setContent(html_6bb17034614fde5149ee3eb474313ed2);\\n \\n \\n\\n circle_marker_64852b8408310bd45e452cc7c371c6ed.bindPopup(popup_62657e6f1dbd361fcdf7e4e4b1a604fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6064963ed3540f8993396d5a196bf1b = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_f92e3c10306e258639531e2897b5affc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9158fc4a3515c7818b364212d0ca8a3b = $(`<div id="html_9158fc4a3515c7818b364212d0ca8a3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f92e3c10306e258639531e2897b5affc.setContent(html_9158fc4a3515c7818b364212d0ca8a3b);\\n \\n \\n\\n circle_marker_d6064963ed3540f8993396d5a196bf1b.bindPopup(popup_f92e3c10306e258639531e2897b5affc)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9f993b31a2fdd5a88e5be9627ebd0bf = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_85b14937669738e65a93b2757e251bff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_02d0df1ca9ede496574d788f14e769d7 = $(`<div id="html_02d0df1ca9ede496574d788f14e769d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_85b14937669738e65a93b2757e251bff.setContent(html_02d0df1ca9ede496574d788f14e769d7);\\n \\n \\n\\n circle_marker_e9f993b31a2fdd5a88e5be9627ebd0bf.bindPopup(popup_85b14937669738e65a93b2757e251bff)\\n ;\\n\\n \\n \\n \\n var circle_marker_15d80cc34146a9c074a186055bf0234b = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_81ae65b514510fe1dfed37ae2c2f9b63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f1db73249d12a42e11204c6c271518f = $(`<div id="html_7f1db73249d12a42e11204c6c271518f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81ae65b514510fe1dfed37ae2c2f9b63.setContent(html_7f1db73249d12a42e11204c6c271518f);\\n \\n \\n\\n circle_marker_15d80cc34146a9c074a186055bf0234b.bindPopup(popup_81ae65b514510fe1dfed37ae2c2f9b63)\\n ;\\n\\n \\n \\n \\n var circle_marker_5673f171a758a2c9daba5ece07868334 = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_2ba6892312cf2e9459505f72d1b3924c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c09b6f4721f0af4d0ca602fc6e55ba66 = $(`<div id="html_c09b6f4721f0af4d0ca602fc6e55ba66" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ba6892312cf2e9459505f72d1b3924c.setContent(html_c09b6f4721f0af4d0ca602fc6e55ba66);\\n \\n \\n\\n circle_marker_5673f171a758a2c9daba5ece07868334.bindPopup(popup_2ba6892312cf2e9459505f72d1b3924c)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf136c4da75f20b1be9e2bdd8d2e55b6 = L.circleMarker(\\n [42.72732269176802, -73.26979194254899],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_7fb03b8f00d9b6f20b739db679217cea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d818cfa313aa8723053ba1edd3732f3 = $(`<div id="html_3d818cfa313aa8723053ba1edd3732f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7fb03b8f00d9b6f20b739db679217cea.setContent(html_3d818cfa313aa8723053ba1edd3732f3);\\n \\n \\n\\n circle_marker_bf136c4da75f20b1be9e2bdd8d2e55b6.bindPopup(popup_7fb03b8f00d9b6f20b739db679217cea)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba07948fe60488a8bfd1cbfcfe4a0066 = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_0a0a9d2ac1253e13229872e5e3d6e06e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_766bece8c3c5ebe0653794c2d710dca4 = $(`<div id="html_766bece8c3c5ebe0653794c2d710dca4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a0a9d2ac1253e13229872e5e3d6e06e.setContent(html_766bece8c3c5ebe0653794c2d710dca4);\\n \\n \\n\\n circle_marker_ba07948fe60488a8bfd1cbfcfe4a0066.bindPopup(popup_0a0a9d2ac1253e13229872e5e3d6e06e)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e7048b592ab7d554da086eeb868b21c = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8dd59fa85c68744e8791c7d79f97b92d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_158f301fce1963ac94b3cdc1bef49937 = $(`<div id="html_158f301fce1963ac94b3cdc1bef49937" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8dd59fa85c68744e8791c7d79f97b92d.setContent(html_158f301fce1963ac94b3cdc1bef49937);\\n \\n \\n\\n circle_marker_8e7048b592ab7d554da086eeb868b21c.bindPopup(popup_8dd59fa85c68744e8791c7d79f97b92d)\\n ;\\n\\n \\n \\n \\n var circle_marker_681ccf4a8fbcb4000bc2c97a6fc0df12 = L.circleMarker(\\n [42.72732279050467, -73.26364180917045],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c5dc9dd58318fe1566d075222a93fe0c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f37f0724909e86bacc4214a14f6d56fc = $(`<div id="html_f37f0724909e86bacc4214a14f6d56fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c5dc9dd58318fe1566d075222a93fe0c.setContent(html_f37f0724909e86bacc4214a14f6d56fc);\\n \\n \\n\\n circle_marker_681ccf4a8fbcb4000bc2c97a6fc0df12.bindPopup(popup_c5dc9dd58318fe1566d075222a93fe0c)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c1d89fde2955866343285e5323b24bd = L.circleMarker(\\n [42.72732277075734, -73.26241178249424],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a5865a254626da07a898febc80f3e02d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14251f7be3d18583668f438b0e8fe425 = $(`<div id="html_14251f7be3d18583668f438b0e8fe425" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a5865a254626da07a898febc80f3e02d.setContent(html_14251f7be3d18583668f438b0e8fe425);\\n \\n \\n\\n circle_marker_0c1d89fde2955866343285e5323b24bd.bindPopup(popup_a5865a254626da07a898febc80f3e02d)\\n ;\\n\\n \\n \\n \\n var circle_marker_8484122c90a63dd54550fc5661d08daf = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_a18f59e1f9066e8661618af9abd851ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a45a3d303375c820c6adb11b3cdac6e5 = $(`<div id="html_a45a3d303375c820c6adb11b3cdac6e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a18f59e1f9066e8661618af9abd851ac.setContent(html_a45a3d303375c820c6adb11b3cdac6e5);\\n \\n \\n\\n circle_marker_8484122c90a63dd54550fc5661d08daf.bindPopup(popup_a18f59e1f9066e8661618af9abd851ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_e17f6bedfc474184410f5e20f431f819 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c4621e5dc1068e1627172241e9c73593 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19eebc3e66440694a369e1adbcebece6 = $(`<div id="html_19eebc3e66440694a369e1adbcebece6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4621e5dc1068e1627172241e9c73593.setContent(html_19eebc3e66440694a369e1adbcebece6);\\n \\n \\n\\n circle_marker_e17f6bedfc474184410f5e20f431f819.bindPopup(popup_c4621e5dc1068e1627172241e9c73593)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5bb7a116c9da5d8f35ea950468293a3 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_19f64bda668a60f3a64bcd7b64e11373 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d7008178bee64c7ef873a1125ea68bb1 = $(`<div id="html_d7008178bee64c7ef873a1125ea68bb1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19f64bda668a60f3a64bcd7b64e11373.setContent(html_d7008178bee64c7ef873a1125ea68bb1);\\n \\n \\n\\n circle_marker_f5bb7a116c9da5d8f35ea950468293a3.bindPopup(popup_19f64bda668a60f3a64bcd7b64e11373)\\n ;\\n\\n \\n \\n \\n var circle_marker_c75c32134fd3daaa8954bb365bb3e2f4 = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_433c6736e8d2df900140d6a8d531240c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e1fe3646dbc3c9da21124125ba557d5 = $(`<div id="html_9e1fe3646dbc3c9da21124125ba557d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_433c6736e8d2df900140d6a8d531240c.setContent(html_9e1fe3646dbc3c9da21124125ba557d5);\\n \\n \\n\\n circle_marker_c75c32134fd3daaa8954bb365bb3e2f4.bindPopup(popup_433c6736e8d2df900140d6a8d531240c)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7030361e0d254c612e3e2a8887db99b = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_70becdd21948cdb7b36b26a17e6a1433 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_039022fbbb34ca9dc9410393b5940ef2 = $(`<div id="html_039022fbbb34ca9dc9410393b5940ef2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_70becdd21948cdb7b36b26a17e6a1433.setContent(html_039022fbbb34ca9dc9410393b5940ef2);\\n \\n \\n\\n circle_marker_e7030361e0d254c612e3e2a8887db99b.bindPopup(popup_70becdd21948cdb7b36b26a17e6a1433)\\n ;\\n\\n \\n \\n \\n var circle_marker_c7df8fb5969ccbe0e291f7e592a55cbd = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_f6ce3de386a403c9e8fe2148629ddfa0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5cd2cb547e934476cc008a9ae10d5a57 = $(`<div id="html_5cd2cb547e934476cc008a9ae10d5a57" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6ce3de386a403c9e8fe2148629ddfa0.setContent(html_5cd2cb547e934476cc008a9ae10d5a57);\\n \\n \\n\\n circle_marker_c7df8fb5969ccbe0e291f7e592a55cbd.bindPopup(popup_f6ce3de386a403c9e8fe2148629ddfa0)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce5f99fa6a8ccbedbd5f8c99633aa3a3 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_97ea82e5658c4547ca2639a9e0926c81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2608d87f1231fe22a426288a9913524 = $(`<div id="html_a2608d87f1231fe22a426288a9913524" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97ea82e5658c4547ca2639a9e0926c81.setContent(html_a2608d87f1231fe22a426288a9913524);\\n \\n \\n\\n circle_marker_ce5f99fa6a8ccbedbd5f8c99633aa3a3.bindPopup(popup_97ea82e5658c4547ca2639a9e0926c81)\\n ;\\n\\n \\n \\n \\n var circle_marker_612e9f6db1e2f2c560e987f95fa8466f = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_6e66b432a84f459e60048527fae0f870 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_88f9fce8c62e097ac758b11cc968ad1a = $(`<div id="html_88f9fce8c62e097ac758b11cc968ad1a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e66b432a84f459e60048527fae0f870.setContent(html_88f9fce8c62e097ac758b11cc968ad1a);\\n \\n \\n\\n circle_marker_612e9f6db1e2f2c560e987f95fa8466f.bindPopup(popup_6e66b432a84f459e60048527fae0f870)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0e60ca1870196dba17ebf159a81b4a1 = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_6db03ed8390bfad7488d1f81d6594dc9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b3525d0fcaa74e74cb4c27ad21c3f9b = $(`<div id="html_4b3525d0fcaa74e74cb4c27ad21c3f9b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6db03ed8390bfad7488d1f81d6594dc9.setContent(html_4b3525d0fcaa74e74cb4c27ad21c3f9b);\\n \\n \\n\\n circle_marker_b0e60ca1870196dba17ebf159a81b4a1.bindPopup(popup_6db03ed8390bfad7488d1f81d6594dc9)\\n ;\\n\\n \\n \\n \\n var circle_marker_35ed5cee97dbb4fff5a24d4c3399183f = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_f329dbc91481794ca21a9549cbf027b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c3a78212c543d5fc32e087fd2d1dbb68 = $(`<div id="html_c3a78212c543d5fc32e087fd2d1dbb68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f329dbc91481794ca21a9549cbf027b2.setContent(html_c3a78212c543d5fc32e087fd2d1dbb68);\\n \\n \\n\\n circle_marker_35ed5cee97dbb4fff5a24d4c3399183f.bindPopup(popup_f329dbc91481794ca21a9549cbf027b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_b77cf26f5066d2dc508e8dccc0cee511 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ac660f213320b2e4af80f26d6d641aff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_356a43882bfae948f2d20f3b7b6ff9d4 = $(`<div id="html_356a43882bfae948f2d20f3b7b6ff9d4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac660f213320b2e4af80f26d6d641aff.setContent(html_356a43882bfae948f2d20f3b7b6ff9d4);\\n \\n \\n\\n circle_marker_b77cf26f5066d2dc508e8dccc0cee511.bindPopup(popup_ac660f213320b2e4af80f26d6d641aff)\\n ;\\n\\n \\n \\n \\n var circle_marker_832ab4b42964db2c76a946825436bfe7 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_c9c2696955d0d138b0f562f5a0fda8e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6a5905f5461b26d6cee334b00874b50 = $(`<div id="html_c6a5905f5461b26d6cee334b00874b50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9c2696955d0d138b0f562f5a0fda8e7.setContent(html_c6a5905f5461b26d6cee334b00874b50);\\n \\n \\n\\n circle_marker_832ab4b42964db2c76a946825436bfe7.bindPopup(popup_c9c2696955d0d138b0f562f5a0fda8e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_638718178988a7ec539d5768ef824a18 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1a5de658353c993bfce7635f70f88f3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49089c4e9ce98adada0061724da91977 = $(`<div id="html_49089c4e9ce98adada0061724da91977" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a5de658353c993bfce7635f70f88f3f.setContent(html_49089c4e9ce98adada0061724da91977);\\n \\n \\n\\n circle_marker_638718178988a7ec539d5768ef824a18.bindPopup(popup_1a5de658353c993bfce7635f70f88f3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b4751c7be488673db7e51d99932c44ea = L.circleMarker(\\n [42.72551566371306, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_41f3bc9d848bae7d3d0c30b2de1c0801 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_912b89959302e83bd524d8a5e506f22f = $(`<div id="html_912b89959302e83bd524d8a5e506f22f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41f3bc9d848bae7d3d0c30b2de1c0801.setContent(html_912b89959302e83bd524d8a5e506f22f);\\n \\n \\n\\n circle_marker_b4751c7be488673db7e51d99932c44ea.bindPopup(popup_41f3bc9d848bae7d3d0c30b2de1c0801)\\n ;\\n\\n \\n \\n \\n var circle_marker_a43c67987c507f94b21d0b2f8d361444 = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8fc826439607d863607e0a54f4ffe757 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14f78e924049f3cb3d9f137dddb8c01f = $(`<div id="html_14f78e924049f3cb3d9f137dddb8c01f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fc826439607d863607e0a54f4ffe757.setContent(html_14f78e924049f3cb3d9f137dddb8c01f);\\n \\n \\n\\n circle_marker_a43c67987c507f94b21d0b2f8d361444.bindPopup(popup_8fc826439607d863607e0a54f4ffe757)\\n ;\\n\\n \\n \\n \\n var circle_marker_7949dc895dab2734d2e2979f59b1c814 = L.circleMarker(\\n [42.72551555839823, -73.25995187249272],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_fe4c3957ba7c346e4a74097cb7d20858 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_422c057839260b574a3a8bbc6bee7d03 = $(`<div id="html_422c057839260b574a3a8bbc6bee7d03" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe4c3957ba7c346e4a74097cb7d20858.setContent(html_422c057839260b574a3a8bbc6bee7d03);\\n \\n \\n\\n circle_marker_7949dc895dab2734d2e2979f59b1c814.bindPopup(popup_fe4c3957ba7c346e4a74097cb7d20858)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ad4e2901a095fda7421c35ae20e1d3a = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_d7ee421e21da67042baeddac83baa98c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cd21fc4519a2bafad4769c211f8ec95 = $(`<div id="html_9cd21fc4519a2bafad4769c211f8ec95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d7ee421e21da67042baeddac83baa98c.setContent(html_9cd21fc4519a2bafad4769c211f8ec95);\\n \\n \\n\\n circle_marker_0ad4e2901a095fda7421c35ae20e1d3a.bindPopup(popup_d7ee421e21da67042baeddac83baa98c)\\n ;\\n\\n \\n \\n \\n var circle_marker_15940bdd11e0254b3c30cc19ee66a091 = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8d3a459442f048312f754ab0d581faf9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_830ab5883a9de29d2a8899ec7745a4c4 = $(`<div id="html_830ab5883a9de29d2a8899ec7745a4c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d3a459442f048312f754ab0d581faf9.setContent(html_830ab5883a9de29d2a8899ec7745a4c4);\\n \\n \\n\\n circle_marker_15940bdd11e0254b3c30cc19ee66a091.bindPopup(popup_8d3a459442f048312f754ab0d581faf9)\\n ;\\n\\n \\n \\n \\n var circle_marker_577c9aefce7c9753a3a9baa2ebaaec49 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_f698d854772117aa1f4d88d392bdb42b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76894c6b45a86e087f8d61c8c2683415 = $(`<div id="html_76894c6b45a86e087f8d61c8c2683415" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f698d854772117aa1f4d88d392bdb42b.setContent(html_76894c6b45a86e087f8d61c8c2683415);\\n \\n \\n\\n circle_marker_577c9aefce7c9753a3a9baa2ebaaec49.bindPopup(popup_f698d854772117aa1f4d88d392bdb42b)\\n ;\\n\\n \\n \\n \\n var circle_marker_693eed8f46b12e13c1bdd842b8b52720 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_ce914a5f5e8c5bcd90dc6a2c67d2584b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28a5f1ee7d30c7c81613e4918a05359e = $(`<div id="html_28a5f1ee7d30c7c81613e4918a05359e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce914a5f5e8c5bcd90dc6a2c67d2584b.setContent(html_28a5f1ee7d30c7c81613e4918a05359e);\\n \\n \\n\\n circle_marker_693eed8f46b12e13c1bdd842b8b52720.bindPopup(popup_ce914a5f5e8c5bcd90dc6a2c67d2584b)\\n ;\\n\\n \\n \\n \\n var circle_marker_388f56711db52ecd5f4aac6d57446684 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_b61872b7aab45c0794d258a987c84a23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b918d951a202dd43ee07dc9110b884b8 = $(`<div id="html_b918d951a202dd43ee07dc9110b884b8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b61872b7aab45c0794d258a987c84a23.setContent(html_b918d951a202dd43ee07dc9110b884b8);\\n \\n \\n\\n circle_marker_388f56711db52ecd5f4aac6d57446684.bindPopup(popup_b61872b7aab45c0794d258a987c84a23)\\n ;\\n\\n \\n \\n \\n var circle_marker_aaf11d2d6439f0b9b69eaf974eea7656 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_525eb792dd5f988c3145f5a008bc7c5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_80a78ca766b1dcea73835ce88c3d503f = $(`<div id="html_80a78ca766b1dcea73835ce88c3d503f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_525eb792dd5f988c3145f5a008bc7c5c.setContent(html_80a78ca766b1dcea73835ce88c3d503f);\\n \\n \\n\\n circle_marker_aaf11d2d6439f0b9b69eaf974eea7656.bindPopup(popup_525eb792dd5f988c3145f5a008bc7c5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_25d1b6ab62c68ec7abdb535e8680cd7d = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_19770f664076b62b71d175d2e4a823d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa4e9f0dc655004c7560d26a3ca76d87 = $(`<div id="html_aa4e9f0dc655004c7560d26a3ca76d87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19770f664076b62b71d175d2e4a823d9.setContent(html_aa4e9f0dc655004c7560d26a3ca76d87);\\n \\n \\n\\n circle_marker_25d1b6ab62c68ec7abdb535e8680cd7d.bindPopup(popup_19770f664076b62b71d175d2e4a823d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba25e5429a9a5000d320dec0a014e050 = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_3d7548742fec2d6d904794166e0fd009 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b8e674040fac95feb2580ef3e09e85fa = $(`<div id="html_b8e674040fac95feb2580ef3e09e85fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d7548742fec2d6d904794166e0fd009.setContent(html_b8e674040fac95feb2580ef3e09e85fa);\\n \\n \\n\\n circle_marker_ba25e5429a9a5000d320dec0a014e050.bindPopup(popup_3d7548742fec2d6d904794166e0fd009)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b485e199b4fb84e815e42eda2a06c39 = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1adec5c7b2a3d1efd8791f95bf0796fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a59a44290f8610dc630711d634028372 = $(`<div id="html_a59a44290f8610dc630711d634028372" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1adec5c7b2a3d1efd8791f95bf0796fb.setContent(html_a59a44290f8610dc630711d634028372);\\n \\n \\n\\n circle_marker_0b485e199b4fb84e815e42eda2a06c39.bindPopup(popup_1adec5c7b2a3d1efd8791f95bf0796fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_07b3f8ffbc4ff506e0641e1b6a8fb235 = L.circleMarker(\\n [42.72370842502844, -73.2599520158284],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_0c4c8de1179d7b66a2c5be0d554c8634 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8ec2d4d200b418e15afb7aba470cfe2 = $(`<div id="html_f8ec2d4d200b418e15afb7aba470cfe2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c4c8de1179d7b66a2c5be0d554c8634.setContent(html_f8ec2d4d200b418e15afb7aba470cfe2);\\n \\n \\n\\n circle_marker_07b3f8ffbc4ff506e0641e1b6a8fb235.bindPopup(popup_0c4c8de1179d7b66a2c5be0d554c8634)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8ca861c2f79e37dfed5627a6c14cead = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_1755c82cf90f4b4d31bf60368b9d0806 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9620749af4e7fbd40fd81328dace4fb1 = $(`<div id="html_9620749af4e7fbd40fd81328dace4fb1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1755c82cf90f4b4d31bf60368b9d0806.setContent(html_9620749af4e7fbd40fd81328dace4fb1);\\n \\n \\n\\n circle_marker_a8ca861c2f79e37dfed5627a6c14cead.bindPopup(popup_1755c82cf90f4b4d31bf60368b9d0806)\\n ;\\n\\n \\n \\n \\n var circle_marker_c71fb5efea56810c6ace6a81ce52c088 = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_e871b4217e421a66ee5f9d5081e8c983 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6daa7ccc946b75feb41ebb7070320b23 = $(`<div id="html_6daa7ccc946b75feb41ebb7070320b23" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e871b4217e421a66ee5f9d5081e8c983.setContent(html_6daa7ccc946b75feb41ebb7070320b23);\\n \\n \\n\\n circle_marker_c71fb5efea56810c6ace6a81ce52c088.bindPopup(popup_e871b4217e421a66ee5f9d5081e8c983)\\n ;\\n\\n \\n \\n \\n var circle_marker_dbb2affcfb47b5038fac8b7ac21d1c88 = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_638ddd4539d405ff92d92625c6adbf86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8b9f9307f1716e739fcf4f16b9dcda5 = $(`<div id="html_f8b9f9307f1716e739fcf4f16b9dcda5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_638ddd4539d405ff92d92625c6adbf86.setContent(html_f8b9f9307f1716e739fcf4f16b9dcda5);\\n \\n \\n\\n circle_marker_dbb2affcfb47b5038fac8b7ac21d1c88.bindPopup(popup_638ddd4539d405ff92d92625c6adbf86)\\n ;\\n\\n \\n \\n \\n var circle_marker_e17e14e20f33bd608f1e6f6963410805 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_8eeb4916ea930e929890a8ca17f2d8f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_abf4b0c7bf56a6c127ca06c6ac52ea4d = $(`<div id="html_abf4b0c7bf56a6c127ca06c6ac52ea4d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8eeb4916ea930e929890a8ca17f2d8f4.setContent(html_abf4b0c7bf56a6c127ca06c6ac52ea4d);\\n \\n \\n\\n circle_marker_e17e14e20f33bd608f1e6f6963410805.bindPopup(popup_8eeb4916ea930e929890a8ca17f2d8f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_d4ab7b6a4b0c509572e63785188886fb = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_47fe858b732d032490389b3501e3637c);\\n \\n \\n var popup_251138e85f11959d867601e09baad8c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0907a9df930fcceb3460cc9c1a382628 = $(`<div id="html_0907a9df930fcceb3460cc9c1a382628" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_251138e85f11959d867601e09baad8c5.setContent(html_0907a9df930fcceb3460cc9c1a382628);\\n \\n \\n\\n circle_marker_d4ab7b6a4b0c509572e63785188886fb.bindPopup(popup_251138e85f11959d867601e09baad8c5)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Birch, yellow\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_ba69442bb6470fcbc989272505656bb0 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_ba69442bb6470fcbc989272505656bb0" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_ba69442bb6470fcbc989272505656bb0 = L.map(\\n "map_ba69442bb6470fcbc989272505656bb0",\\n {\\n center: [42.735454627410334, -73.24703686421026],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_94685db95ec6290c20fc33c58662537b = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var circle_marker_9cf8ea9bcbf03fde4d75171a8edd17a5 = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0d65349d9b0c85e08971fd8950f5f5ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9318abf88dc65a29b90d011e47661681 = $(`<div id="html_9318abf88dc65a29b90d011e47661681" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d65349d9b0c85e08971fd8950f5f5ea.setContent(html_9318abf88dc65a29b90d011e47661681);\\n \\n \\n\\n circle_marker_9cf8ea9bcbf03fde4d75171a8edd17a5.bindPopup(popup_0d65349d9b0c85e08971fd8950f5f5ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_575a77aa209da3cc384c14058aa57749 = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_4782c5692a56475c50f994da2a3903c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4a107e0b458997f6fb063d5facde164 = $(`<div id="html_d4a107e0b458997f6fb063d5facde164" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4782c5692a56475c50f994da2a3903c2.setContent(html_d4a107e0b458997f6fb063d5facde164);\\n \\n \\n\\n circle_marker_575a77aa209da3cc384c14058aa57749.bindPopup(popup_4782c5692a56475c50f994da2a3903c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ed1c33117e4f9ed10669f658ff23658 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_e9a08276e44e30c0ee4677f46c6148d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_34793d13f8fb3582b5e3af4012f5d7a0 = $(`<div id="html_34793d13f8fb3582b5e3af4012f5d7a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9a08276e44e30c0ee4677f46c6148d1.setContent(html_34793d13f8fb3582b5e3af4012f5d7a0);\\n \\n \\n\\n circle_marker_0ed1c33117e4f9ed10669f658ff23658.bindPopup(popup_e9a08276e44e30c0ee4677f46c6148d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_91b57f84e6ad54b70f3e9fcdb4b3df48 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_8606f03ffe3b8c5e9695f2b8c8ca454a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c79580421bc3e8a5fcad98373b7a1e7 = $(`<div id="html_5c79580421bc3e8a5fcad98373b7a1e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8606f03ffe3b8c5e9695f2b8c8ca454a.setContent(html_5c79580421bc3e8a5fcad98373b7a1e7);\\n \\n \\n\\n circle_marker_91b57f84e6ad54b70f3e9fcdb4b3df48.bindPopup(popup_8606f03ffe3b8c5e9695f2b8c8ca454a)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd53b60c876bd3874ef4325313c905ce = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_ad25fd1e939f69766d67f9a8f4f4fa83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d18f53226303e3b0de74dc9a6d560e95 = $(`<div id="html_d18f53226303e3b0de74dc9a6d560e95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad25fd1e939f69766d67f9a8f4f4fa83.setContent(html_d18f53226303e3b0de74dc9a6d560e95);\\n \\n \\n\\n circle_marker_dd53b60c876bd3874ef4325313c905ce.bindPopup(popup_ad25fd1e939f69766d67f9a8f4f4fa83)\\n ;\\n\\n \\n \\n \\n var circle_marker_55f2a9b7010493c05c0bc505af7620f8 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_4a52e56e253193cb4382ca0337597e0f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96b91ab6b3a078c11f76e06058524592 = $(`<div id="html_96b91ab6b3a078c11f76e06058524592" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a52e56e253193cb4382ca0337597e0f.setContent(html_96b91ab6b3a078c11f76e06058524592);\\n \\n \\n\\n circle_marker_55f2a9b7010493c05c0bc505af7620f8.bindPopup(popup_4a52e56e253193cb4382ca0337597e0f)\\n ;\\n\\n \\n \\n \\n var circle_marker_ae8782e5e245b0f91381f00a9d366da7 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_7d018cbcbf823d42e8e3e8bc480dfa82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96c76a2ea8e83a17d33a507b7784eef0 = $(`<div id="html_96c76a2ea8e83a17d33a507b7784eef0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d018cbcbf823d42e8e3e8bc480dfa82.setContent(html_96c76a2ea8e83a17d33a507b7784eef0);\\n \\n \\n\\n circle_marker_ae8782e5e245b0f91381f00a9d366da7.bindPopup(popup_7d018cbcbf823d42e8e3e8bc480dfa82)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b424aed229437ad9727747e6afca161 = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5f29c3db775f112f2bc491973464e755 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65cf0a564329debe34e03ae9f6c9c48f = $(`<div id="html_65cf0a564329debe34e03ae9f6c9c48f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f29c3db775f112f2bc491973464e755.setContent(html_65cf0a564329debe34e03ae9f6c9c48f);\\n \\n \\n\\n circle_marker_0b424aed229437ad9727747e6afca161.bindPopup(popup_5f29c3db775f112f2bc491973464e755)\\n ;\\n\\n \\n \\n \\n var circle_marker_11467af138578d63df167aa4ead8e38e = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_416fd7ef9ede037474172d4b991ba3e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06a60cf78bf13eccadb11f693fdc50c2 = $(`<div id="html_06a60cf78bf13eccadb11f693fdc50c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_416fd7ef9ede037474172d4b991ba3e7.setContent(html_06a60cf78bf13eccadb11f693fdc50c2);\\n \\n \\n\\n circle_marker_11467af138578d63df167aa4ead8e38e.bindPopup(popup_416fd7ef9ede037474172d4b991ba3e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_85d65327d5544d15601b5e47b23b3fa7 = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_503b425b063339dd2ed0e4d493dc1e4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_710985524fb349c74d2e52b0e054f43a = $(`<div id="html_710985524fb349c74d2e52b0e054f43a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_503b425b063339dd2ed0e4d493dc1e4f.setContent(html_710985524fb349c74d2e52b0e054f43a);\\n \\n \\n\\n circle_marker_85d65327d5544d15601b5e47b23b3fa7.bindPopup(popup_503b425b063339dd2ed0e4d493dc1e4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_96d26ba2e412506cb511cd9118ddd0b1 = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6e430b22103585ce8eb478bf4dd0408d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_22538e2cd315642b0e101c2cee46f09e = $(`<div id="html_22538e2cd315642b0e101c2cee46f09e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e430b22103585ce8eb478bf4dd0408d.setContent(html_22538e2cd315642b0e101c2cee46f09e);\\n \\n \\n\\n circle_marker_96d26ba2e412506cb511cd9118ddd0b1.bindPopup(popup_6e430b22103585ce8eb478bf4dd0408d)\\n ;\\n\\n \\n \\n \\n var circle_marker_39ffdb0e25dd3807bf835752de4db954 = L.circleMarker(\\n [42.74539359743371, -73.27594530262718],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d85b12b615ed8950c470e4b6fcc6de2c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_903da8b2d0ff12898bbe4e986bd63136 = $(`<div id="html_903da8b2d0ff12898bbe4e986bd63136" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d85b12b615ed8950c470e4b6fcc6de2c.setContent(html_903da8b2d0ff12898bbe4e986bd63136);\\n \\n \\n\\n circle_marker_39ffdb0e25dd3807bf835752de4db954.bindPopup(popup_d85b12b615ed8950c470e4b6fcc6de2c)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f4527df563f04436dc94a5a2cdf3bcc = L.circleMarker(\\n [42.74539370938061, -73.27471491744124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_91891dd4599e7552c14dc815cad6598e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ef12a363f2fd210af9ffb9861e122a1 = $(`<div id="html_0ef12a363f2fd210af9ffb9861e122a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91891dd4599e7552c14dc815cad6598e.setContent(html_0ef12a363f2fd210af9ffb9861e122a1);\\n \\n \\n\\n circle_marker_8f4527df563f04436dc94a5a2cdf3bcc.bindPopup(popup_91891dd4599e7552c14dc815cad6598e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5b966b1692e497a9a2108553f24aca4 = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0394d9f0a2193cac946ce40f3bbe9dc8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43b2d3fa0d13a3e21205db781b5b350d = $(`<div id="html_43b2d3fa0d13a3e21205db781b5b350d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0394d9f0a2193cac946ce40f3bbe9dc8.setContent(html_43b2d3fa0d13a3e21205db781b5b350d);\\n \\n \\n\\n circle_marker_b5b966b1692e497a9a2108553f24aca4.bindPopup(popup_0394d9f0a2193cac946ce40f3bbe9dc8)\\n ;\\n\\n \\n \\n \\n var circle_marker_79fb5b41b97c738d2857e8d7b937a45f = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_bc3a05707ce96c8f728c8f9306b25826 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5272da3688af56ddec92aac908368cf = $(`<div id="html_a5272da3688af56ddec92aac908368cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc3a05707ce96c8f728c8f9306b25826.setContent(html_a5272da3688af56ddec92aac908368cf);\\n \\n \\n\\n circle_marker_79fb5b41b97c738d2857e8d7b937a45f.bindPopup(popup_bc3a05707ce96c8f728c8f9306b25826)\\n ;\\n\\n \\n \\n \\n var circle_marker_f264a5313ab04e5fe3513f8c532d39c3 = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2fe292341f80cebef0bbdebee9bbc681 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b67fca9dd9001fa21a90195d42874de = $(`<div id="html_3b67fca9dd9001fa21a90195d42874de" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fe292341f80cebef0bbdebee9bbc681.setContent(html_3b67fca9dd9001fa21a90195d42874de);\\n \\n \\n\\n circle_marker_f264a5313ab04e5fe3513f8c532d39c3.bindPopup(popup_2fe292341f80cebef0bbdebee9bbc681)\\n ;\\n\\n \\n \\n \\n var circle_marker_26df5812988f5087d703a056c05fca32 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c00f09a161460b263b59796bc5e6e0ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6c75919ca8600a32a08b6b22db266822 = $(`<div id="html_6c75919ca8600a32a08b6b22db266822" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c00f09a161460b263b59796bc5e6e0ab.setContent(html_6c75919ca8600a32a08b6b22db266822);\\n \\n \\n\\n circle_marker_26df5812988f5087d703a056c05fca32.bindPopup(popup_c00f09a161460b263b59796bc5e6e0ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_a94bec770274f4535ada56e13929621d = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0d30ace60c13978f4eb9fbf513d8e245 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ef49a86bf4444586a9ef215a7a69a1d = $(`<div id="html_0ef49a86bf4444586a9ef215a7a69a1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d30ace60c13978f4eb9fbf513d8e245.setContent(html_0ef49a86bf4444586a9ef215a7a69a1d);\\n \\n \\n\\n circle_marker_a94bec770274f4535ada56e13929621d.bindPopup(popup_0d30ace60c13978f4eb9fbf513d8e245)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4d4e9a5f177f5e8a75e8ad45582a912 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_52c1e118cdff61621640b7a0f9b2fdab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2fd7f48e7ff3966032c18024bc32d7a3 = $(`<div id="html_2fd7f48e7ff3966032c18024bc32d7a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52c1e118cdff61621640b7a0f9b2fdab.setContent(html_2fd7f48e7ff3966032c18024bc32d7a3);\\n \\n \\n\\n circle_marker_e4d4e9a5f177f5e8a75e8ad45582a912.bindPopup(popup_52c1e118cdff61621640b7a0f9b2fdab)\\n ;\\n\\n \\n \\n \\n var circle_marker_c5598012272e146e37c507beeb867e0a = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_a7a77640deb6d2e7ae75f945d78e4428 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17706cdca03fd3061919d480fab410dc = $(`<div id="html_17706cdca03fd3061919d480fab410dc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7a77640deb6d2e7ae75f945d78e4428.setContent(html_17706cdca03fd3061919d480fab410dc);\\n \\n \\n\\n circle_marker_c5598012272e146e37c507beeb867e0a.bindPopup(popup_a7a77640deb6d2e7ae75f945d78e4428)\\n ;\\n\\n \\n \\n \\n var circle_marker_9efa01820f923cb1af5e210465d1befb = L.circleMarker(\\n [42.74539412424261, -73.26364145064247],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_4120f7f31cc5ccb80b265aaebbf9da63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d5c7d98a8f365bfc60e2e0951a2efb88 = $(`<div id="html_d5c7d98a8f365bfc60e2e0951a2efb88" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4120f7f31cc5ccb80b265aaebbf9da63.setContent(html_d5c7d98a8f365bfc60e2e0951a2efb88);\\n \\n \\n\\n circle_marker_9efa01820f923cb1af5e210465d1befb.bindPopup(popup_4120f7f31cc5ccb80b265aaebbf9da63)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc76502d21484528fbd6d5cd8c97af13 = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c57ffd1b0033f80f885bae3e28dd0fd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ac61d129487c29db6585856cf1463b4 = $(`<div id="html_9ac61d129487c29db6585856cf1463b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c57ffd1b0033f80f885bae3e28dd0fd0.setContent(html_9ac61d129487c29db6585856cf1463b4);\\n \\n \\n\\n circle_marker_dc76502d21484528fbd6d5cd8c97af13.bindPopup(popup_c57ffd1b0033f80f885bae3e28dd0fd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_0943bdc922fbc8b65aa3cc5a7c00c52c = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_be635c98a43972f91930f4d7f69537fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81bc007da9b47426d045967535d7e811 = $(`<div id="html_81bc007da9b47426d045967535d7e811" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be635c98a43972f91930f4d7f69537fa.setContent(html_81bc007da9b47426d045967535d7e811);\\n \\n \\n\\n circle_marker_0943bdc922fbc8b65aa3cc5a7c00c52c.bindPopup(popup_be635c98a43972f91930f4d7f69537fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_6aadb7120bc3a4be329fdc110da7ce9b = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_a4f25eefebf092aff39aeec02e8cce16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_22081a95c104192fc037afcd3ee12e4c = $(`<div id="html_22081a95c104192fc037afcd3ee12e4c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4f25eefebf092aff39aeec02e8cce16.setContent(html_22081a95c104192fc037afcd3ee12e4c);\\n \\n \\n\\n circle_marker_6aadb7120bc3a4be329fdc110da7ce9b.bindPopup(popup_a4f25eefebf092aff39aeec02e8cce16)\\n ;\\n\\n \\n \\n \\n var circle_marker_91d78cec037d6e13f72703353c1d01d1 = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_b19f55e31d258971bf185447abc94768 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b192e2b968caa467b9b3167a037f1db3 = $(`<div id="html_b192e2b968caa467b9b3167a037f1db3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b19f55e31d258971bf185447abc94768.setContent(html_b192e2b968caa467b9b3167a037f1db3);\\n \\n \\n\\n circle_marker_91d78cec037d6e13f72703353c1d01d1.bindPopup(popup_b19f55e31d258971bf185447abc94768)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3106b336086b8ef917606e812fbc88e = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_eb948f60ebeed6c66ddd50fadee5cbbe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bdb013b35c53c5ca7ee370696aff7206 = $(`<div id="html_bdb013b35c53c5ca7ee370696aff7206" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb948f60ebeed6c66ddd50fadee5cbbe.setContent(html_bdb013b35c53c5ca7ee370696aff7206);\\n \\n \\n\\n circle_marker_f3106b336086b8ef917606e812fbc88e.bindPopup(popup_eb948f60ebeed6c66ddd50fadee5cbbe)\\n ;\\n\\n \\n \\n \\n var circle_marker_f976292c926b10757dc7c794580b9447 = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_21399436b1552381162c8de5e412a192 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b8060bd4699c1e2539bf629e4400a212 = $(`<div id="html_b8060bd4699c1e2539bf629e4400a212" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_21399436b1552381162c8de5e412a192.setContent(html_b8060bd4699c1e2539bf629e4400a212);\\n \\n \\n\\n circle_marker_f976292c926b10757dc7c794580b9447.bindPopup(popup_21399436b1552381162c8de5e412a192)\\n ;\\n\\n \\n \\n \\n var circle_marker_73c228bcb323e2492441b55b83ee2479 = L.circleMarker(\\n [42.74358657602363, -73.27471463051425],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0c8616afdcb23a4257bc78f13bec6192 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ac84a8040fd26fd051833700729b368 = $(`<div id="html_1ac84a8040fd26fd051833700729b368" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c8616afdcb23a4257bc78f13bec6192.setContent(html_1ac84a8040fd26fd051833700729b368);\\n \\n \\n\\n circle_marker_73c228bcb323e2492441b55b83ee2479.bindPopup(popup_0c8616afdcb23a4257bc78f13bec6192)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d6e4fa5c44b04bcd7665c5e158cba0e = L.circleMarker(\\n [42.74358667479628, -73.27348428119001],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5a99b50003b105e7818815fb93222d7e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b371c7030a9385e4b380984c2194f289 = $(`<div id="html_b371c7030a9385e4b380984c2194f289" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a99b50003b105e7818815fb93222d7e.setContent(html_b371c7030a9385e4b380984c2194f289);\\n \\n \\n\\n circle_marker_0d6e4fa5c44b04bcd7665c5e158cba0e.bindPopup(popup_5a99b50003b105e7818815fb93222d7e)\\n ;\\n\\n \\n \\n \\n var circle_marker_5da6c9b7bf27be50bb57876f47831870 = L.circleMarker(\\n [42.74358676039927, -73.27225393186212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_ea1133baaaa486978338449df871f1ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6c9e0b1342599cd7dd8e884715e0874 = $(`<div id="html_d6c9e0b1342599cd7dd8e884715e0874" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ea1133baaaa486978338449df871f1ac.setContent(html_d6c9e0b1342599cd7dd8e884715e0874);\\n \\n \\n\\n circle_marker_5da6c9b7bf27be50bb57876f47831870.bindPopup(popup_ea1133baaaa486978338449df871f1ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_70cb769964ad0b425a3f777567ca9dbd = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_894a30b6825f982a8b1c584bc6c543b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2813d70c2e36c45cc5d7ec6b2ef86e19 = $(`<div id="html_2813d70c2e36c45cc5d7ec6b2ef86e19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_894a30b6825f982a8b1c584bc6c543b2.setContent(html_2813d70c2e36c45cc5d7ec6b2ef86e19);\\n \\n \\n\\n circle_marker_70cb769964ad0b425a3f777567ca9dbd.bindPopup(popup_894a30b6825f982a8b1c584bc6c543b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_7682bfa4af0a383dcd2b44af9a5a1131 = L.circleMarker(\\n [42.74358697111428, -73.26733253452443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c2dc7d1e940100fe398cfebd7820cb5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00d3e784906bb030abd9c8717c260014 = $(`<div id="html_00d3e784906bb030abd9c8717c260014" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2dc7d1e940100fe398cfebd7820cb5c.setContent(html_00d3e784906bb030abd9c8717c260014);\\n \\n \\n\\n circle_marker_7682bfa4af0a383dcd2b44af9a5a1131.bindPopup(popup_c2dc7d1e940100fe398cfebd7820cb5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_af856ab48fe57b2267cfe5534520cbd1 = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1c41ccb6abd5cb0a060a403c70986bf9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6cb1be2a157a9e7eda37c1cd689f3fed = $(`<div id="html_6cb1be2a157a9e7eda37c1cd689f3fed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1c41ccb6abd5cb0a060a403c70986bf9.setContent(html_6cb1be2a157a9e7eda37c1cd689f3fed);\\n \\n \\n\\n circle_marker_af856ab48fe57b2267cfe5534520cbd1.bindPopup(popup_1c41ccb6abd5cb0a060a403c70986bf9)\\n ;\\n\\n \\n \\n \\n var circle_marker_ade1c177cb41228a7515fe14bc09f51e = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_52cab2a8be686257488e56fa5ffbebbb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7b199e18703a0d3d8702333918f5ea4 = $(`<div id="html_f7b199e18703a0d3d8702333918f5ea4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52cab2a8be686257488e56fa5ffbebbb.setContent(html_f7b199e18703a0d3d8702333918f5ea4);\\n \\n \\n\\n circle_marker_ade1c177cb41228a7515fe14bc09f51e.bindPopup(popup_52cab2a8be686257488e56fa5ffbebbb)\\n ;\\n\\n \\n \\n \\n var circle_marker_82b279a162c6bbdb867be99b7de9fba7 = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_95a2f13d477ed1a1bb78acc49956188c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e05a6c471e50794f195e2bc549d8c5c = $(`<div id="html_9e05a6c471e50794f195e2bc549d8c5c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95a2f13d477ed1a1bb78acc49956188c.setContent(html_9e05a6c471e50794f195e2bc549d8c5c);\\n \\n \\n\\n circle_marker_82b279a162c6bbdb867be99b7de9fba7.bindPopup(popup_95a2f13d477ed1a1bb78acc49956188c)\\n ;\\n\\n \\n \\n \\n var circle_marker_809e7e6bdb405bbbc134e4d69dffaff3 = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_a831e73552af7710ed1095f948f1de0e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd3dea637bc10cd8df09c705ff397a37 = $(`<div id="html_cd3dea637bc10cd8df09c705ff397a37" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a831e73552af7710ed1095f948f1de0e.setContent(html_cd3dea637bc10cd8df09c705ff397a37);\\n \\n \\n\\n circle_marker_809e7e6bdb405bbbc134e4d69dffaff3.bindPopup(popup_a831e73552af7710ed1095f948f1de0e)\\n ;\\n\\n \\n \\n \\n var circle_marker_42e8cce4cb6113a79d88c26a92797ae5 = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_04aa09f46bc9cb3df3eae666ab70561e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_192a4228acb395c886505e6e935d2e79 = $(`<div id="html_192a4228acb395c886505e6e935d2e79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04aa09f46bc9cb3df3eae666ab70561e.setContent(html_192a4228acb395c886505e6e935d2e79);\\n \\n \\n\\n circle_marker_42e8cce4cb6113a79d88c26a92797ae5.bindPopup(popup_04aa09f46bc9cb3df3eae666ab70561e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e843b152c488eaa9a6a0d0586fc7f55f = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_b9fc259ff22a48c17027be798277c372 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2471b249cce644124afb06b6c8fb8291 = $(`<div id="html_2471b249cce644124afb06b6c8fb8291" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b9fc259ff22a48c17027be798277c372.setContent(html_2471b249cce644124afb06b6c8fb8291);\\n \\n \\n\\n circle_marker_e843b152c488eaa9a6a0d0586fc7f55f.bindPopup(popup_b9fc259ff22a48c17027be798277c372)\\n ;\\n\\n \\n \\n \\n var circle_marker_2dd5008c64d7523a6af6537225cd2a71 = L.circleMarker(\\n [42.7417795414353, -73.27348403014923],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_e6a420c0e88f94c8549ba1268b9a17bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97c7eda7bfa79623ba32f7cef2be5e1f = $(`<div id="html_97c7eda7bfa79623ba32f7cef2be5e1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6a420c0e88f94c8549ba1268b9a17bc.setContent(html_97c7eda7bfa79623ba32f7cef2be5e1f);\\n \\n \\n\\n circle_marker_2dd5008c64d7523a6af6537225cd2a71.bindPopup(popup_e6a420c0e88f94c8549ba1268b9a17bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_a53941c0bca80ae552db6bdc145c5775 = L.circleMarker(\\n [42.74177969946516, -73.27102340321625],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_7e4b3a56849dc6dab58995a93fa633a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30ba60046418bd39bd5bab6a4ac8a279 = $(`<div id="html_30ba60046418bd39bd5bab6a4ac8a279" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e4b3a56849dc6dab58995a93fa633a1.setContent(html_30ba60046418bd39bd5bab6a4ac8a279);\\n \\n \\n\\n circle_marker_a53941c0bca80ae552db6bdc145c5775.bindPopup(popup_7e4b3a56849dc6dab58995a93fa633a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_32558ac719771d47bcd057336b9769ed = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_4da5fe5c1eb6e180865e10765ab58fa0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe595acc99ae48b68ee447ad805090a2 = $(`<div id="html_fe595acc99ae48b68ee447ad805090a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4da5fe5c1eb6e180865e10765ab58fa0.setContent(html_fe595acc99ae48b68ee447ad805090a2);\\n \\n \\n\\n circle_marker_32558ac719771d47bcd057336b9769ed.bindPopup(popup_4da5fe5c1eb6e180865e10765ab58fa0)\\n ;\\n\\n \\n \\n \\n var circle_marker_76a472276542e7ca35873a5a700dbe9b = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d40a4d36bd224fa8d09b239b01b33b52 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5602b349c0f46056975d9c78908dc414 = $(`<div id="html_5602b349c0f46056975d9c78908dc414" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d40a4d36bd224fa8d09b239b01b33b52.setContent(html_5602b349c0f46056975d9c78908dc414);\\n \\n \\n\\n circle_marker_76a472276542e7ca35873a5a700dbe9b.bindPopup(popup_d40a4d36bd224fa8d09b239b01b33b52)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6f799b055ce060707c26964bbaf110e = L.circleMarker(\\n [42.74177983774129, -73.26733246279849],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_fd28b9278f9311fd1db9e3d8fa14d63b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87af7f10b4e0c5a61e85b661f15cbd87 = $(`<div id="html_87af7f10b4e0c5a61e85b661f15cbd87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd28b9278f9311fd1db9e3d8fa14d63b.setContent(html_87af7f10b4e0c5a61e85b661f15cbd87);\\n \\n \\n\\n circle_marker_e6f799b055ce060707c26964bbaf110e.bindPopup(popup_fd28b9278f9311fd1db9e3d8fa14d63b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3df1f258ac73584c4a19bae4865da514 = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_35b5be31417339d1be05e18a15b3fbea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97331bc6b3297367dfbdda5687800399 = $(`<div id="html_97331bc6b3297367dfbdda5687800399" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35b5be31417339d1be05e18a15b3fbea.setContent(html_97331bc6b3297367dfbdda5687800399);\\n \\n \\n\\n circle_marker_3df1f258ac73584c4a19bae4865da514.bindPopup(popup_35b5be31417339d1be05e18a15b3fbea)\\n ;\\n\\n \\n \\n \\n var circle_marker_761cb6698dade6545ae2c0bd1d60b5f0 = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0e36e251422e6b113f565c9e030fc472 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4435395400a0c55c436127184a676ff8 = $(`<div id="html_4435395400a0c55c436127184a676ff8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e36e251422e6b113f565c9e030fc472.setContent(html_4435395400a0c55c436127184a676ff8);\\n \\n \\n\\n circle_marker_761cb6698dade6545ae2c0bd1d60b5f0.bindPopup(popup_0e36e251422e6b113f565c9e030fc472)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b3cfd2cdfe5bd4ee7b4fe54469950c1 = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_32283c1427ff79c2284c27b3ac9285f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8dd46c553eabb73f3efb45299a0a90c = $(`<div id="html_a8dd46c553eabb73f3efb45299a0a90c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_32283c1427ff79c2284c27b3ac9285f0.setContent(html_a8dd46c553eabb73f3efb45299a0a90c);\\n \\n \\n\\n circle_marker_9b3cfd2cdfe5bd4ee7b4fe54469950c1.bindPopup(popup_32283c1427ff79c2284c27b3ac9285f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_91e5dc280d4ec7e7a376065d19c2ac6b = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_85f752ded2c4acccddaf820b151362e6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00e148d791fe310fa49da195188bc58a = $(`<div id="html_00e148d791fe310fa49da195188bc58a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_85f752ded2c4acccddaf820b151362e6.setContent(html_00e148d791fe310fa49da195188bc58a);\\n \\n \\n\\n circle_marker_91e5dc280d4ec7e7a376065d19c2ac6b.bindPopup(popup_85f752ded2c4acccddaf820b151362e6)\\n ;\\n\\n \\n \\n \\n var circle_marker_2fffc257b457266228f1b8fba5f27974 = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_61c61ed679bfbdede1e955cb894a8da8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15e2122090f71173386eba51c43762b3 = $(`<div id="html_15e2122090f71173386eba51c43762b3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61c61ed679bfbdede1e955cb894a8da8.setContent(html_15e2122090f71173386eba51c43762b3);\\n \\n \\n\\n circle_marker_2fffc257b457266228f1b8fba5f27974.bindPopup(popup_61c61ed679bfbdede1e955cb894a8da8)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a6ea621e5601a674db265c43ec35a68 = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_10be0e770b9f03cac3a33d8fe3e53446 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_753da4a7c82502232f34485893491eaa = $(`<div id="html_753da4a7c82502232f34485893491eaa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10be0e770b9f03cac3a33d8fe3e53446.setContent(html_753da4a7c82502232f34485893491eaa);\\n \\n \\n\\n circle_marker_8a6ea621e5601a674db265c43ec35a68.bindPopup(popup_10be0e770b9f03cac3a33d8fe3e53446)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8ebbcb76c32491f89f16ad18f633dc6 = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_f10fb1e97b26c5c54dfd0b94b96fa6b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69088a9f31d58f7f8f568bf512f2a5f9 = $(`<div id="html_69088a9f31d58f7f8f568bf512f2a5f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f10fb1e97b26c5c54dfd0b94b96fa6b8.setContent(html_69088a9f31d58f7f8f568bf512f2a5f9);\\n \\n \\n\\n circle_marker_c8ebbcb76c32491f89f16ad18f633dc6.bindPopup(popup_f10fb1e97b26c5c54dfd0b94b96fa6b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_88a360e0782df0998f89a14eea77804e = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c43b36e7538e786ceca54242091217fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1577612e2c312891af25875cb210d3f = $(`<div id="html_f1577612e2c312891af25875cb210d3f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c43b36e7538e786ceca54242091217fb.setContent(html_f1577612e2c312891af25875cb210d3f);\\n \\n \\n\\n circle_marker_88a360e0782df0998f89a14eea77804e.bindPopup(popup_c43b36e7538e786ceca54242091217fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_d11903647f423df1eadb601f1307aca9 = L.circleMarker(\\n [42.73997267144673, -73.26856266869262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5bd380d7e4d56c7bb6c10baeaf19172d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b929e2ca87424dca00280d4ab113d71 = $(`<div id="html_8b929e2ca87424dca00280d4ab113d71" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5bd380d7e4d56c7bb6c10baeaf19172d.setContent(html_8b929e2ca87424dca00280d4ab113d71);\\n \\n \\n\\n circle_marker_d11903647f423df1eadb601f1307aca9.bindPopup(popup_5bd380d7e4d56c7bb6c10baeaf19172d)\\n ;\\n\\n \\n \\n \\n var circle_marker_02d7a185d0c8e8c0fb6505e0be2348eb = L.circleMarker(\\n [42.73997272412122, -73.26610211346305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_8f45bfbeeffb7e3b190c01106adf7e7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a561d3df6032d63725c7163f4ff726aa = $(`<div id="html_a561d3df6032d63725c7163f4ff726aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f45bfbeeffb7e3b190c01106adf7e7b.setContent(html_a561d3df6032d63725c7163f4ff726aa);\\n \\n \\n\\n circle_marker_02d7a185d0c8e8c0fb6505e0be2348eb.bindPopup(popup_8f45bfbeeffb7e3b190c01106adf7e7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_51224b61b52852f3853d31bf111c7259 = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_3a4e79a15c2953ead3f12b3e1d3e3d48 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56ad38faf0eae24dc94846626afb25a3 = $(`<div id="html_56ad38faf0eae24dc94846626afb25a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a4e79a15c2953ead3f12b3e1d3e3d48.setContent(html_56ad38faf0eae24dc94846626afb25a3);\\n \\n \\n\\n circle_marker_51224b61b52852f3853d31bf111c7259.bindPopup(popup_3a4e79a15c2953ead3f12b3e1d3e3d48)\\n ;\\n\\n \\n \\n \\n var circle_marker_a2dde47c2f8592e677e51df9a5b173d9 = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_dc7501c2724b5c0998c5e145d87d961a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e15cf319b40367530775aa86073e6a62 = $(`<div id="html_e15cf319b40367530775aa86073e6a62" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc7501c2724b5c0998c5e145d87d961a.setContent(html_e15cf319b40367530775aa86073e6a62);\\n \\n \\n\\n circle_marker_a2dde47c2f8592e677e51df9a5b173d9.bindPopup(popup_dc7501c2724b5c0998c5e145d87d961a)\\n ;\\n\\n \\n \\n \\n var circle_marker_020d8e6cc0587dc36021880be04e1563 = L.circleMarker(\\n [42.73997262535657, -73.25995072538909],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_acfbf194aad1c6173c6d46363eaac580 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97a7d497e29f0a3c5e95c3a64d3dae12 = $(`<div id="html_97a7d497e29f0a3c5e95c3a64d3dae12" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_acfbf194aad1c6173c6d46363eaac580.setContent(html_97a7d497e29f0a3c5e95c3a64d3dae12);\\n \\n \\n\\n circle_marker_020d8e6cc0587dc36021880be04e1563.bindPopup(popup_acfbf194aad1c6173c6d46363eaac580)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d2fbc2ec5112b37510def96fffb1057 = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2f48bacc55b4675a761d099fd9c6bad2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ccac1a38b33286b37547672505010a1c = $(`<div id="html_ccac1a38b33286b37547672505010a1c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f48bacc55b4675a761d099fd9c6bad2.setContent(html_ccac1a38b33286b37547672505010a1c);\\n \\n \\n\\n circle_marker_0d2fbc2ec5112b37510def96fffb1057.bindPopup(popup_2f48bacc55b4675a761d099fd9c6bad2)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0a849324103ce04d3eef9198a005e7e = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_9313d9375d7aacee080e84661c3f36c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ee968287d7a6848c68095b48e0dde42f = $(`<div id="html_ee968287d7a6848c68095b48e0dde42f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9313d9375d7aacee080e84661c3f36c8.setContent(html_ee968287d7a6848c68095b48e0dde42f);\\n \\n \\n\\n circle_marker_b0a849324103ce04d3eef9198a005e7e.bindPopup(popup_9313d9375d7aacee080e84661c3f36c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf9b39a2b0ee66a95f966e87d4a9b38b = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_a0c455f40b3b97ff5952267e69798822 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b93fa736595ce6cdab7ca9ad4db0bb4a = $(`<div id="html_b93fa736595ce6cdab7ca9ad4db0bb4a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0c455f40b3b97ff5952267e69798822.setContent(html_b93fa736595ce6cdab7ca9ad4db0bb4a);\\n \\n \\n\\n circle_marker_bf9b39a2b0ee66a95f966e87d4a9b38b.bindPopup(popup_a0c455f40b3b97ff5952267e69798822)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f7022730fa69ffd07b3986bec073948 = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.989422804014327, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1dd2bd40866684de8a5b54cc7887df28 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40d91168f6bbfc99af3f54351cc89c28 = $(`<div id="html_40d91168f6bbfc99af3f54351cc89c28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1dd2bd40866684de8a5b54cc7887df28.setContent(html_40d91168f6bbfc99af3f54351cc89c28);\\n \\n \\n\\n circle_marker_5f7022730fa69ffd07b3986bec073948.bindPopup(popup_1dd2bd40866684de8a5b54cc7887df28)\\n ;\\n\\n \\n \\n \\n var circle_marker_994bec56c5749a843e211f894305dea0 = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_270be14976ee384f5746110fe4d27b95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46b02e5de9124e7a8fde1c701a424198 = $(`<div id="html_46b02e5de9124e7a8fde1c701a424198" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_270be14976ee384f5746110fe4d27b95.setContent(html_46b02e5de9124e7a8fde1c701a424198);\\n \\n \\n\\n circle_marker_994bec56c5749a843e211f894305dea0.bindPopup(popup_270be14976ee384f5746110fe4d27b95)\\n ;\\n\\n \\n \\n \\n var circle_marker_b09922f5d2b23cda3c20c48ebd374f12 = L.circleMarker(\\n [42.73816553807507, -73.26856256112116],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_360506354727e82797a5da016bffe1b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d7bf0ad63a843576c52d46c07d2b46d4 = $(`<div id="html_d7bf0ad63a843576c52d46c07d2b46d4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_360506354727e82797a5da016bffe1b9.setContent(html_d7bf0ad63a843576c52d46c07d2b46d4);\\n \\n \\n\\n circle_marker_b09922f5d2b23cda3c20c48ebd374f12.bindPopup(popup_360506354727e82797a5da016bffe1b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8c29681316648637c78d4293afca6c1 = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_cff010655248b8dce26b2675d9841dac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8631d3ec5aef3978b918d813a66c1ce2 = $(`<div id="html_8631d3ec5aef3978b918d813a66c1ce2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cff010655248b8dce26b2675d9841dac.setContent(html_8631d3ec5aef3978b918d813a66c1ce2);\\n \\n \\n\\n circle_marker_f8c29681316648637c78d4293afca6c1.bindPopup(popup_cff010655248b8dce26b2675d9841dac)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a5ac61691b2959cbe8a3085b557009f = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_941bbc179009faa55edf511ce4665dba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_740d307490b206f94fc227597f59af41 = $(`<div id="html_740d307490b206f94fc227597f59af41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_941bbc179009faa55edf511ce4665dba.setContent(html_740d307490b206f94fc227597f59af41);\\n \\n \\n\\n circle_marker_7a5ac61691b2959cbe8a3085b557009f.bindPopup(popup_941bbc179009faa55edf511ce4665dba)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7a087cf700bba5e8572c2037ffdca49 = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_404c8af8b5de448625bcd532619c94dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a57c45663be3416298250170c3b0e6e = $(`<div id="html_1a57c45663be3416298250170c3b0e6e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_404c8af8b5de448625bcd532619c94dd.setContent(html_1a57c45663be3416298250170c3b0e6e);\\n \\n \\n\\n circle_marker_b7a087cf700bba5e8572c2037ffdca49.bindPopup(popup_404c8af8b5de448625bcd532619c94dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_ac94852fcd70fa9a563a8c20d0e4e8b5 = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_f06d302f7efa6e947c0c96671e3ec4f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_633faa89b36b14708495d78dc9132bc6 = $(`<div id="html_633faa89b36b14708495d78dc9132bc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f06d302f7efa6e947c0c96671e3ec4f5.setContent(html_633faa89b36b14708495d78dc9132bc6);\\n \\n \\n\\n circle_marker_ac94852fcd70fa9a563a8c20d0e4e8b5.bindPopup(popup_f06d302f7efa6e947c0c96671e3ec4f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a48d69fa81953420c00cfad88bc6e24 = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_7dfaa2f160bf638dbd50f92a952a86e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d83343ef2cd9f2fcc5e176c41764a8fc = $(`<div id="html_d83343ef2cd9f2fcc5e176c41764a8fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7dfaa2f160bf638dbd50f92a952a86e0.setContent(html_d83343ef2cd9f2fcc5e176c41764a8fc);\\n \\n \\n\\n circle_marker_7a48d69fa81953420c00cfad88bc6e24.bindPopup(popup_7dfaa2f160bf638dbd50f92a952a86e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f7f2003a970abb1247663a94738dac1 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_bbd5cf7c2de71b8a455b739586b0f498 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7234020358405b87cd1cabe6987068f2 = $(`<div id="html_7234020358405b87cd1cabe6987068f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bbd5cf7c2de71b8a455b739586b0f498.setContent(html_7234020358405b87cd1cabe6987068f2);\\n \\n \\n\\n circle_marker_3f7f2003a970abb1247663a94738dac1.bindPopup(popup_bbd5cf7c2de71b8a455b739586b0f498)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3b9b8d476648c91c523858bf3420c6f = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6c421f2b20d44f420c057048cd76acc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc3cf8a0342f8415db3ee6de47ab7eac = $(`<div id="html_cc3cf8a0342f8415db3ee6de47ab7eac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c421f2b20d44f420c057048cd76acc1.setContent(html_cc3cf8a0342f8415db3ee6de47ab7eac);\\n \\n \\n\\n circle_marker_e3b9b8d476648c91c523858bf3420c6f.bindPopup(popup_6c421f2b20d44f420c057048cd76acc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_8403f96eec6a1adc34a36542a1336a45 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_807c1f098b06b43514db11b79943ded5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_083e9dbcee59802104fdd747dee4550d = $(`<div id="html_083e9dbcee59802104fdd747dee4550d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_807c1f098b06b43514db11b79943ded5.setContent(html_083e9dbcee59802104fdd747dee4550d);\\n \\n \\n\\n circle_marker_8403f96eec6a1adc34a36542a1336a45.bindPopup(popup_807c1f098b06b43514db11b79943ded5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c27b13da67eb8d5f2b994ebaa03b7eed = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6ff7d288f6fec8d9cd971b774ce947cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a976948f45e2467595ad042ec840e62d = $(`<div id="html_a976948f45e2467595ad042ec840e62d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ff7d288f6fec8d9cd971b774ce947cf.setContent(html_a976948f45e2467595ad042ec840e62d);\\n \\n \\n\\n circle_marker_c27b13da67eb8d5f2b994ebaa03b7eed.bindPopup(popup_6ff7d288f6fec8d9cd971b774ce947cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_43a976da46803a9ddd9bf915b4b67bec = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_9fe933bd11b115b7437901be7c839b57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eae04ec357f2188aea7b53193e4ee20a = $(`<div id="html_eae04ec357f2188aea7b53193e4ee20a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fe933bd11b115b7437901be7c839b57.setContent(html_eae04ec357f2188aea7b53193e4ee20a);\\n \\n \\n\\n circle_marker_43a976da46803a9ddd9bf915b4b67bec.bindPopup(popup_9fe933bd11b115b7437901be7c839b57)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb8af235bd4ff0a8086d977a2e1abe2f = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_339afb5a85a6b468c85df9e7a4d17bfa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc5977f2f9431dbcc2d9615b7c4ed3c8 = $(`<div id="html_cc5977f2f9431dbcc2d9615b7c4ed3c8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_339afb5a85a6b468c85df9e7a4d17bfa.setContent(html_cc5977f2f9431dbcc2d9615b7c4ed3c8);\\n \\n \\n\\n circle_marker_cb8af235bd4ff0a8086d977a2e1abe2f.bindPopup(popup_339afb5a85a6b468c85df9e7a4d17bfa)\\n ;\\n\\n \\n \\n \\n var circle_marker_28b8fa9e5caeb9df908dc44c05fd0f48 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_dac5a7677570eb2a8d64d42e35553af3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_834e9e775b02b708d8703444b7f0d341 = $(`<div id="html_834e9e775b02b708d8703444b7f0d341" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dac5a7677570eb2a8d64d42e35553af3.setContent(html_834e9e775b02b708d8703444b7f0d341);\\n \\n \\n\\n circle_marker_28b8fa9e5caeb9df908dc44c05fd0f48.bindPopup(popup_dac5a7677570eb2a8d64d42e35553af3)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c8489e13550e9d8598b5d1d03af3a1f = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_aca7cb4cdf51918dfc0e2bd141ffc1df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28ac4587dd74506c9486bdc7a9947403 = $(`<div id="html_28ac4587dd74506c9486bdc7a9947403" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aca7cb4cdf51918dfc0e2bd141ffc1df.setContent(html_28ac4587dd74506c9486bdc7a9947403);\\n \\n \\n\\n circle_marker_0c8489e13550e9d8598b5d1d03af3a1f.bindPopup(popup_aca7cb4cdf51918dfc0e2bd141ffc1df)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fbfdb865bdc05d25db0d69e8d6a88e1 = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_02e14cfbf02758cd7a21d288bafde192 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e51d2cf1bc156ab774645030fb0b39f = $(`<div id="html_6e51d2cf1bc156ab774645030fb0b39f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_02e14cfbf02758cd7a21d288bafde192.setContent(html_6e51d2cf1bc156ab774645030fb0b39f);\\n \\n \\n\\n circle_marker_3fbfdb865bdc05d25db0d69e8d6a88e1.bindPopup(popup_02e14cfbf02758cd7a21d288bafde192)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f6d5e4d849afc75acfcc8c2c5c96aa6 = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6b4c26a8280cfe7e7a80ba3211a2c3e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b96a07bdc693449223fe7dbf57ab4b87 = $(`<div id="html_b96a07bdc693449223fe7dbf57ab4b87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b4c26a8280cfe7e7a80ba3211a2c3e3.setContent(html_b96a07bdc693449223fe7dbf57ab4b87);\\n \\n \\n\\n circle_marker_9f6d5e4d849afc75acfcc8c2c5c96aa6.bindPopup(popup_6b4c26a8280cfe7e7a80ba3211a2c3e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_f94261b25f21d137c6e7282c95f9f77e = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d4ed7b6ea46bc01588d28b228f993659 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74b59dc9146d4c413f89b479542dd026 = $(`<div id="html_74b59dc9146d4c413f89b479542dd026" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d4ed7b6ea46bc01588d28b228f993659.setContent(html_74b59dc9146d4c413f89b479542dd026);\\n \\n \\n\\n circle_marker_f94261b25f21d137c6e7282c95f9f77e.bindPopup(popup_d4ed7b6ea46bc01588d28b228f993659)\\n ;\\n\\n \\n \\n \\n var circle_marker_67962a68e02dd2c7c51d4823f787943e = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1cfae0ba4e040816a20fad74fc42837f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c98804548e1a841ab095908e0e38a37c = $(`<div id="html_c98804548e1a841ab095908e0e38a37c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1cfae0ba4e040816a20fad74fc42837f.setContent(html_c98804548e1a841ab095908e0e38a37c);\\n \\n \\n\\n circle_marker_67962a68e02dd2c7c51d4823f787943e.bindPopup(popup_1cfae0ba4e040816a20fad74fc42837f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1227f1ad15d9c53a18d35ed0e16f773 = L.circleMarker(\\n [42.7345512252472, -73.26979251605432],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6848cf514b6768d6d35dd04056d137e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf674378d846df8633bfd64cd96133e0 = $(`<div id="html_cf674378d846df8633bfd64cd96133e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6848cf514b6768d6d35dd04056d137e4.setContent(html_cf674378d846df8633bfd64cd96133e0);\\n \\n \\n\\n circle_marker_e1227f1ad15d9c53a18d35ed0e16f773.bindPopup(popup_6848cf514b6768d6d35dd04056d137e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_1213532a349b6531e960d0545fa6cf0b = L.circleMarker(\\n [42.73455127133177, -73.26856234600437],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0bda800efe5a2cfce7f7b707b7c3193e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e8c65116b97cc95ec5bb623a3e0080f = $(`<div id="html_7e8c65116b97cc95ec5bb623a3e0080f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0bda800efe5a2cfce7f7b707b7c3193e.setContent(html_7e8c65116b97cc95ec5bb623a3e0080f);\\n \\n \\n\\n circle_marker_1213532a349b6531e960d0545fa6cf0b.bindPopup(popup_0bda800efe5a2cfce7f7b707b7c3193e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7e276fa1900c26d0c06391f3a8ffb76 = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2913b80509839ddda7a85731737ba0e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2c53f2b4360d620fe8d6c1522284dcb = $(`<div id="html_a2c53f2b4360d620fe8d6c1522284dcb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2913b80509839ddda7a85731737ba0e4.setContent(html_a2c53f2b4360d620fe8d6c1522284dcb);\\n \\n \\n\\n circle_marker_b7e276fa1900c26d0c06391f3a8ffb76.bindPopup(popup_2913b80509839ddda7a85731737ba0e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_127c70effbb23f97f7b4792aadea105b = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_89df5b94a2686cae0f254cd1b7e709cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d42039ec7c96d38f44591e8b5c35bd6 = $(`<div id="html_6d42039ec7c96d38f44591e8b5c35bd6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89df5b94a2686cae0f254cd1b7e709cc.setContent(html_6d42039ec7c96d38f44591e8b5c35bd6);\\n \\n \\n\\n circle_marker_127c70effbb23f97f7b4792aadea105b.bindPopup(popup_89df5b94a2686cae0f254cd1b7e709cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_98034d0b28e56682e8beb39608d9e1d1 = L.circleMarker(\\n [42.734551330583365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_b19999f06978df39fe7731badae0d82a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ba511463954b743985e36fdba5d034c = $(`<div id="html_9ba511463954b743985e36fdba5d034c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b19999f06978df39fe7731badae0d82a.setContent(html_9ba511463954b743985e36fdba5d034c);\\n \\n \\n\\n circle_marker_98034d0b28e56682e8beb39608d9e1d1.bindPopup(popup_b19999f06978df39fe7731badae0d82a)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce2daac11e1938f9b8004c45d51a03cd = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_4a2a4edbba1cb464084bce82047ac9e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d7b6d8b5c69ae471db0a2ccc07dd15e = $(`<div id="html_6d7b6d8b5c69ae471db0a2ccc07dd15e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a2a4edbba1cb464084bce82047ac9e0.setContent(html_6d7b6d8b5c69ae471db0a2ccc07dd15e);\\n \\n \\n\\n circle_marker_ce2daac11e1938f9b8004c45d51a03cd.bindPopup(popup_4a2a4edbba1cb464084bce82047ac9e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d096aec1458c600771c5d856f7c05c9 = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1fa857bba9691c4758d0dc9cc4225c76 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21c95f931756d1de988939bf8bf3cf0c = $(`<div id="html_21c95f931756d1de988939bf8bf3cf0c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1fa857bba9691c4758d0dc9cc4225c76.setContent(html_21c95f931756d1de988939bf8bf3cf0c);\\n \\n \\n\\n circle_marker_9d096aec1458c600771c5d856f7c05c9.bindPopup(popup_1fa857bba9691c4758d0dc9cc4225c76)\\n ;\\n\\n \\n \\n \\n var circle_marker_83d3e0b9738427efe6025a8f7a139db1 = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_481af6a7c7b4f13bcaa07e1d16f5ffb4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cf4cee5feed176d3ce4a013bbd04c87 = $(`<div id="html_9cf4cee5feed176d3ce4a013bbd04c87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_481af6a7c7b4f13bcaa07e1d16f5ffb4.setContent(html_9cf4cee5feed176d3ce4a013bbd04c87);\\n \\n \\n\\n circle_marker_83d3e0b9738427efe6025a8f7a139db1.bindPopup(popup_481af6a7c7b4f13bcaa07e1d16f5ffb4)\\n ;\\n\\n \\n \\n \\n var circle_marker_7025bf96e7fd2eb6b01df2529e14cb52 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6c6f5148e43adeb7e5d521b24f394559 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_551bbb9639aa2b20c63530bf79936f14 = $(`<div id="html_551bbb9639aa2b20c63530bf79936f14" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c6f5148e43adeb7e5d521b24f394559.setContent(html_551bbb9639aa2b20c63530bf79936f14);\\n \\n \\n\\n circle_marker_7025bf96e7fd2eb6b01df2529e14cb52.bindPopup(popup_6c6f5148e43adeb7e5d521b24f394559)\\n ;\\n\\n \\n \\n \\n var circle_marker_2917b7e58f79f7da3dbbda420171004e = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_79e445e42aec527e3cc9fd160aae98d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40a82cc663664249d467f2a0bb7ac26b = $(`<div id="html_40a82cc663664249d467f2a0bb7ac26b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79e445e42aec527e3cc9fd160aae98d1.setContent(html_40a82cc663664249d467f2a0bb7ac26b);\\n \\n \\n\\n circle_marker_2917b7e58f79f7da3dbbda420171004e.bindPopup(popup_79e445e42aec527e3cc9fd160aae98d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_42e7ffe4ec61f9d500feff74f7408426 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_b10530fba12cce2d43ac5e94503e47f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a61d07684115aac1edae4870a357c7d0 = $(`<div id="html_a61d07684115aac1edae4870a357c7d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b10530fba12cce2d43ac5e94503e47f8.setContent(html_a61d07684115aac1edae4870a357c7d0);\\n \\n \\n\\n circle_marker_42e7ffe4ec61f9d500feff74f7408426.bindPopup(popup_b10530fba12cce2d43ac5e94503e47f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_429e3cfb2175623b093941080a27203c = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c7bef4452bf145ecd1cc3d130f1da2e6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2f600f7f69faf7adc6b4b7c2bfdcec3b = $(`<div id="html_2f600f7f69faf7adc6b4b7c2bfdcec3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c7bef4452bf145ecd1cc3d130f1da2e6.setContent(html_2f600f7f69faf7adc6b4b7c2bfdcec3b);\\n \\n \\n\\n circle_marker_429e3cfb2175623b093941080a27203c.bindPopup(popup_c7bef4452bf145ecd1cc3d130f1da2e6)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c6f39178fa60a0bdb3412478058652f = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_df20a2400c235ba872fcfa45803196fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_039332f425ed5f53806b799edb5778da = $(`<div id="html_039332f425ed5f53806b799edb5778da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df20a2400c235ba872fcfa45803196fc.setContent(html_039332f425ed5f53806b799edb5778da);\\n \\n \\n\\n circle_marker_3c6f39178fa60a0bdb3412478058652f.bindPopup(popup_df20a2400c235ba872fcfa45803196fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ce785bc3749d1e5829cf69c6c07acbf = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_bd1c686b930d8034880fe2ff30f1f9d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_daad0601de803c7a6b4a2073ed0cd4aa = $(`<div id="html_daad0601de803c7a6b4a2073ed0cd4aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd1c686b930d8034880fe2ff30f1f9d1.setContent(html_daad0601de803c7a6b4a2073ed0cd4aa);\\n \\n \\n\\n circle_marker_7ce785bc3749d1e5829cf69c6c07acbf.bindPopup(popup_bd1c686b930d8034880fe2ff30f1f9d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_0fe16706b03503f74402bd0b1d6dabc4 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_23f448730035ccf1526b0a7a0e2e4479 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2379ff95f8e1f2e246ab789c62c0d561 = $(`<div id="html_2379ff95f8e1f2e246ab789c62c0d561" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23f448730035ccf1526b0a7a0e2e4479.setContent(html_2379ff95f8e1f2e246ab789c62c0d561);\\n \\n \\n\\n circle_marker_0fe16706b03503f74402bd0b1d6dabc4.bindPopup(popup_23f448730035ccf1526b0a7a0e2e4479)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd94dc12433986200f499e1a772bf031 = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_25d5abd5f6ab3ef6e3361e9774dfe78e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5c160fb0bbf014b928f6c6d1c3b3dd9 = $(`<div id="html_e5c160fb0bbf014b928f6c6d1c3b3dd9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_25d5abd5f6ab3ef6e3361e9774dfe78e.setContent(html_e5c160fb0bbf014b928f6c6d1c3b3dd9);\\n \\n \\n\\n circle_marker_dd94dc12433986200f499e1a772bf031.bindPopup(popup_25d5abd5f6ab3ef6e3361e9774dfe78e)\\n ;\\n\\n \\n \\n \\n var circle_marker_707f280a944b2da19001272930316145 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_43b8fb4952d24ac115e8bc10c926a178 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56326d1f1135bfc4a556beab797bf68a = $(`<div id="html_56326d1f1135bfc4a556beab797bf68a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43b8fb4952d24ac115e8bc10c926a178.setContent(html_56326d1f1135bfc4a556beab797bf68a);\\n \\n \\n\\n circle_marker_707f280a944b2da19001272930316145.bindPopup(popup_43b8fb4952d24ac115e8bc10c926a178)\\n ;\\n\\n \\n \\n \\n var circle_marker_c9c851d00e44c6b64571d8fc3a592be2 = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_f5832673ca9a6ac798def9db7757d4e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ddb4656f393f6494a1cacbf887b4296 = $(`<div id="html_5ddb4656f393f6494a1cacbf887b4296" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5832673ca9a6ac798def9db7757d4e4.setContent(html_5ddb4656f393f6494a1cacbf887b4296);\\n \\n \\n\\n circle_marker_c9c851d00e44c6b64571d8fc3a592be2.bindPopup(popup_f5832673ca9a6ac798def9db7757d4e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_619a747c07244ef5281dab10c06d9157 = L.circleMarker(\\n [42.7327440918774, -73.26979237266056],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_97f52e8bc42f107d82fda741b81b18d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db6c9a25d23c1a764d773ae09bb608ed = $(`<div id="html_db6c9a25d23c1a764d773ae09bb608ed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97f52e8bc42f107d82fda741b81b18d9.setContent(html_db6c9a25d23c1a764d773ae09bb608ed);\\n \\n \\n\\n circle_marker_619a747c07244ef5281dab10c06d9157.bindPopup(popup_97f52e8bc42f107d82fda741b81b18d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_f98a7c4a77e81b897d9802dfbbd975f4 = L.circleMarker(\\n [42.73274419062606, -73.26610197005185],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_22f2ca5987dd1b3e9b4534ea4ea227c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f46f0cf19ca50d0f1cd381fce3de1629 = $(`<div id="html_f46f0cf19ca50d0f1cd381fce3de1629" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22f2ca5987dd1b3e9b4534ea4ea227c2.setContent(html_f46f0cf19ca50d0f1cd381fce3de1629);\\n \\n \\n\\n circle_marker_f98a7c4a77e81b897d9802dfbbd975f4.bindPopup(popup_22f2ca5987dd1b3e9b4534ea4ea227c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_457a903facaadd13c10dcd79bf4738d6 = L.circleMarker(\\n [42.73274419062606, -73.26364170164257],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2936dcd9b43d6c7b7f095c2ca23c5b54 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b7413a5553f7c24750f4530a5e750f0 = $(`<div id="html_8b7413a5553f7c24750f4530a5e750f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2936dcd9b43d6c7b7f095c2ca23c5b54.setContent(html_8b7413a5553f7c24750f4530a5e750f0);\\n \\n \\n\\n circle_marker_457a903facaadd13c10dcd79bf4738d6.bindPopup(popup_2936dcd9b43d6c7b7f095c2ca23c5b54)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0ebc55f557d8274fe09d71e992f3007 = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6df5a4b6616d7f72a4a35b5047b226af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_290b0da75f47ee1c69f47ee9ed2f2b17 = $(`<div id="html_290b0da75f47ee1c69f47ee9ed2f2b17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6df5a4b6616d7f72a4a35b5047b226af.setContent(html_290b0da75f47ee1c69f47ee9ed2f2b17);\\n \\n \\n\\n circle_marker_b0ebc55f557d8274fe09d71e992f3007.bindPopup(popup_6df5a4b6616d7f72a4a35b5047b226af)\\n ;\\n\\n \\n \\n \\n var circle_marker_799efcf0d74860c212fea219a6c754fe = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_f1f03227b9c72508a1b6e4e5aa31a822 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_265c984b12a47d6ebf247cda9703299b = $(`<div id="html_265c984b12a47d6ebf247cda9703299b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1f03227b9c72508a1b6e4e5aa31a822.setContent(html_265c984b12a47d6ebf247cda9703299b);\\n \\n \\n\\n circle_marker_799efcf0d74860c212fea219a6c754fe.bindPopup(popup_f1f03227b9c72508a1b6e4e5aa31a822)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5605b9e4c54c020823ec8be3809e4ff = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_669ecea95fd2c37a78290080b5ffdf16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5568f9716be37d6e5102a17560868f76 = $(`<div id="html_5568f9716be37d6e5102a17560868f76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_669ecea95fd2c37a78290080b5ffdf16.setContent(html_5568f9716be37d6e5102a17560868f76);\\n \\n \\n\\n circle_marker_d5605b9e4c54c020823ec8be3809e4ff.bindPopup(popup_669ecea95fd2c37a78290080b5ffdf16)\\n ;\\n\\n \\n \\n \\n var circle_marker_8192e80c1d16aeabf799dfad482ad431 = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d8f14683ad0f23144d163daf354cb996 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07fcad6bc0cdff8f30a4762bdf1b8669 = $(`<div id="html_07fcad6bc0cdff8f30a4762bdf1b8669" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8f14683ad0f23144d163daf354cb996.setContent(html_07fcad6bc0cdff8f30a4762bdf1b8669);\\n \\n \\n\\n circle_marker_8192e80c1d16aeabf799dfad482ad431.bindPopup(popup_d8f14683ad0f23144d163daf354cb996)\\n ;\\n\\n \\n \\n \\n var circle_marker_ecf17f06f3e147f14997961991de02bf = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_791f2255f1526732cf28fe492e947ad1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce1ca05813b4027c60932bf24d5a1148 = $(`<div id="html_ce1ca05813b4027c60932bf24d5a1148" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_791f2255f1526732cf28fe492e947ad1.setContent(html_ce1ca05813b4027c60932bf24d5a1148);\\n \\n \\n\\n circle_marker_ecf17f06f3e147f14997961991de02bf.bindPopup(popup_791f2255f1526732cf28fe492e947ad1)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9dcb93fc5193b854a1ce4bb5d68db5e = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_58ae9a8f3f6552ac28f6bc622dd7fb50 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ae9bc1d5643605fac1a2894b6d89696 = $(`<div id="html_8ae9bc1d5643605fac1a2894b6d89696" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58ae9a8f3f6552ac28f6bc622dd7fb50.setContent(html_8ae9bc1d5643605fac1a2894b6d89696);\\n \\n \\n\\n circle_marker_a9dcb93fc5193b854a1ce4bb5d68db5e.bindPopup(popup_58ae9a8f3f6552ac28f6bc622dd7fb50)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8d9c702f379702a945ce43fd788bc31 = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_aec7148f9c2da74a5db921847bb066a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ebfa7f644f98d63ded3e25a407e7aa4a = $(`<div id="html_ebfa7f644f98d63ded3e25a407e7aa4a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aec7148f9c2da74a5db921847bb066a3.setContent(html_ebfa7f644f98d63ded3e25a407e7aa4a);\\n \\n \\n\\n circle_marker_f8d9c702f379702a945ce43fd788bc31.bindPopup(popup_aec7148f9c2da74a5db921847bb066a3)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e91258a374d51364289c5c96466c577 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_47696e952662a6dd15661a3fb56b3ba1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_23c94667b59735f111dbecef28342cd3 = $(`<div id="html_23c94667b59735f111dbecef28342cd3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47696e952662a6dd15661a3fb56b3ba1.setContent(html_23c94667b59735f111dbecef28342cd3);\\n \\n \\n\\n circle_marker_4e91258a374d51364289c5c96466c577.bindPopup(popup_47696e952662a6dd15661a3fb56b3ba1)\\n ;\\n\\n \\n \\n \\n var circle_marker_106d83acbfbb4755e795e9aae04d3704 = L.circleMarker(\\n [42.73093682684808, -73.27225242598358],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d09c5a4c887f52a7b4d986558f09cc9f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_455d4122ade14e9b2e88dff51ebb04a3 = $(`<div id="html_455d4122ade14e9b2e88dff51ebb04a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d09c5a4c887f52a7b4d986558f09cc9f.setContent(html_455d4122ade14e9b2e88dff51ebb04a3);\\n \\n \\n\\n circle_marker_106d83acbfbb4755e795e9aae04d3704.bindPopup(popup_d09c5a4c887f52a7b4d986558f09cc9f)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe9aeb121b49bcd9e9bc3bd33746749a = L.circleMarker(\\n [42.730936899260826, -73.2710223276323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_69fff3c5ac725893073b73f6c8d9b7ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc0881727662d6b3b7f4bca2b9df7cf1 = $(`<div id="html_fc0881727662d6b3b7f4bca2b9df7cf1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69fff3c5ac725893073b73f6c8d9b7ff.setContent(html_fc0881727662d6b3b7f4bca2b9df7cf1);\\n \\n \\n\\n circle_marker_fe9aeb121b49bcd9e9bc3bd33746749a.bindPopup(popup_69fff3c5ac725893073b73f6c8d9b7ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_0310c0eb6d8c8e2c67cb1d306b1ed147 = L.circleMarker(\\n [42.730936958507606, -73.26979222927841],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_051399c803ea4ed53e579657b1551338 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56aed7448802841d3f58f311c78d6e91 = $(`<div id="html_56aed7448802841d3f58f311c78d6e91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_051399c803ea4ed53e579657b1551338.setContent(html_56aed7448802841d3f58f311c78d6e91);\\n \\n \\n\\n circle_marker_0310c0eb6d8c8e2c67cb1d306b1ed147.bindPopup(popup_051399c803ea4ed53e579657b1551338)\\n ;\\n\\n \\n \\n \\n var circle_marker_47734ec0b0e7eaa10468167232f03b4b = L.circleMarker(\\n [42.73093703750333, -73.26733203256491],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_25a83e5d9702bce2013608afe0fd3233 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57862467c2c5070547682c2c5faa5f41 = $(`<div id="html_57862467c2c5070547682c2c5faa5f41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_25a83e5d9702bce2013608afe0fd3233.setContent(html_57862467c2c5070547682c2c5faa5f41);\\n \\n \\n\\n circle_marker_47734ec0b0e7eaa10468167232f03b4b.bindPopup(popup_25a83e5d9702bce2013608afe0fd3233)\\n ;\\n\\n \\n \\n \\n var circle_marker_19ac2d8a5ae94a7e45c6005e80d7b877 = L.circleMarker(\\n [42.73093705725226, -73.26610193420632],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_114058937edb9614f7d2e71922f80200 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60bf48396cfe7a80b0eb0a464d1e938a = $(`<div id="html_60bf48396cfe7a80b0eb0a464d1e938a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_114058937edb9614f7d2e71922f80200.setContent(html_60bf48396cfe7a80b0eb0a464d1e938a);\\n \\n \\n\\n circle_marker_19ac2d8a5ae94a7e45c6005e80d7b877.bindPopup(popup_114058937edb9614f7d2e71922f80200)\\n ;\\n\\n \\n \\n \\n var circle_marker_217e96a40b8e262c75fb9b86a5d3ae12 = L.circleMarker(\\n [42.73093706383524, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_386a08c867ebe10db1c21053ff045886 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_719f15a0891ae69ad544a63a9386dae5 = $(`<div id="html_719f15a0891ae69ad544a63a9386dae5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_386a08c867ebe10db1c21053ff045886.setContent(html_719f15a0891ae69ad544a63a9386dae5);\\n \\n \\n\\n circle_marker_217e96a40b8e262c75fb9b86a5d3ae12.bindPopup(popup_386a08c867ebe10db1c21053ff045886)\\n ;\\n\\n \\n \\n \\n var circle_marker_3de0161148cb91387a0d067ebccac667 = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_dfd0ed97cdbe5e33d7ae15798e43450f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c016d5993f601af9d5729e5cfc430e59 = $(`<div id="html_c016d5993f601af9d5729e5cfc430e59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dfd0ed97cdbe5e33d7ae15798e43450f.setContent(html_c016d5993f601af9d5729e5cfc430e59);\\n \\n \\n\\n circle_marker_3de0161148cb91387a0d067ebccac667.bindPopup(popup_dfd0ed97cdbe5e33d7ae15798e43450f)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c0e9008b2040dba788b6b5d76d7d428 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5826fdd7d9fcae8a8b13e677d675e0c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b54e5e0015ffe75f2eb3a8d9457214a = $(`<div id="html_8b54e5e0015ffe75f2eb3a8d9457214a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5826fdd7d9fcae8a8b13e677d675e0c1.setContent(html_8b54e5e0015ffe75f2eb3a8d9457214a);\\n \\n \\n\\n circle_marker_5c0e9008b2040dba788b6b5d76d7d428.bindPopup(popup_5826fdd7d9fcae8a8b13e677d675e0c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d282c4dd8759d015a79996855d6882d = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5bf505463f5844ba7b3dff3e4912e53e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6085530332e36dea9f4b28fa99089113 = $(`<div id="html_6085530332e36dea9f4b28fa99089113" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5bf505463f5844ba7b3dff3e4912e53e.setContent(html_6085530332e36dea9f4b28fa99089113);\\n \\n \\n\\n circle_marker_7d282c4dd8759d015a79996855d6882d.bindPopup(popup_5bf505463f5844ba7b3dff3e4912e53e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b919fe2e2cd361b9359d4928dcbc36b6 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_7382ab1612690ffb5c551329dd67b044 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c7a0e93bf6442d8968178400b3fc77f = $(`<div id="html_7c7a0e93bf6442d8968178400b3fc77f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7382ab1612690ffb5c551329dd67b044.setContent(html_7c7a0e93bf6442d8968178400b3fc77f);\\n \\n \\n\\n circle_marker_b919fe2e2cd361b9359d4928dcbc36b6.bindPopup(popup_7382ab1612690ffb5c551329dd67b044)\\n ;\\n\\n \\n \\n \\n var circle_marker_1dd7944f87447d11082dd4e59364a562 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0cc03765e009846619ef1755572d3c05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a28f538a2539bd43586276d6204c4b25 = $(`<div id="html_a28f538a2539bd43586276d6204c4b25" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cc03765e009846619ef1755572d3c05.setContent(html_a28f538a2539bd43586276d6204c4b25);\\n \\n \\n\\n circle_marker_1dd7944f87447d11082dd4e59364a562.bindPopup(popup_0cc03765e009846619ef1755572d3c05)\\n ;\\n\\n \\n \\n \\n var circle_marker_09703b7dc1707cef1505cf4e52156a4c = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_3c27e97f2a0fbfd0a38e14235791d16f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f4643d2d26abeeeda7763d2b748872a0 = $(`<div id="html_f4643d2d26abeeeda7763d2b748872a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c27e97f2a0fbfd0a38e14235791d16f.setContent(html_f4643d2d26abeeeda7763d2b748872a0);\\n \\n \\n\\n circle_marker_09703b7dc1707cef1505cf4e52156a4c.bindPopup(popup_3c27e97f2a0fbfd0a38e14235791d16f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6865ad5e75d39c5432d9cc3f8adf645 = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_9f95291fde934bec64ec4774c5630003 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd78033ea3177fcc0255ad645631de72 = $(`<div id="html_bd78033ea3177fcc0255ad645631de72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f95291fde934bec64ec4774c5630003.setContent(html_bd78033ea3177fcc0255ad645631de72);\\n \\n \\n\\n circle_marker_e6865ad5e75d39c5432d9cc3f8adf645.bindPopup(popup_9f95291fde934bec64ec4774c5630003)\\n ;\\n\\n \\n \\n \\n var circle_marker_02b470f42cfbba0d985ef40060df75e0 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_75f1d2f2465faab0710ced08c6ea2149 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e645902581aa6a16f8ab46f9637b79fb = $(`<div id="html_e645902581aa6a16f8ab46f9637b79fb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75f1d2f2465faab0710ced08c6ea2149.setContent(html_e645902581aa6a16f8ab46f9637b79fb);\\n \\n \\n\\n circle_marker_02b470f42cfbba0d985ef40060df75e0.bindPopup(popup_75f1d2f2465faab0710ced08c6ea2149)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6b13031ccec01a5fd5dd8bf23f0c137 = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1da85abe212d4e5e373bcaece03e6bd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6c161584e720e9f603df1670a0522a0 = $(`<div id="html_b6c161584e720e9f603df1670a0522a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1da85abe212d4e5e373bcaece03e6bd0.setContent(html_b6c161584e720e9f603df1670a0522a0);\\n \\n \\n\\n circle_marker_f6b13031ccec01a5fd5dd8bf23f0c137.bindPopup(popup_1da85abe212d4e5e373bcaece03e6bd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_e546d0de7d4276cdc167028c1ec4c0c5 = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.8678889139475245, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_cdea6188e574fe3c1621557a62c111b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_62c5d9b591256d2885137d11d03f319d = $(`<div id="html_62c5d9b591256d2885137d11d03f319d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cdea6188e574fe3c1621557a62c111b3.setContent(html_62c5d9b591256d2885137d11d03f319d);\\n \\n \\n\\n circle_marker_e546d0de7d4276cdc167028c1ec4c0c5.bindPopup(popup_cdea6188e574fe3c1621557a62c111b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a7ffdd6bc7bc4b9bf85a55a4e9dfbbd = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_81ea2411843516d77c35b64e2de15517 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_951f4ddd38855b424a09d6a04bfb4ce6 = $(`<div id="html_951f4ddd38855b424a09d6a04bfb4ce6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81ea2411843516d77c35b64e2de15517.setContent(html_951f4ddd38855b424a09d6a04bfb4ce6);\\n \\n \\n\\n circle_marker_3a7ffdd6bc7bc4b9bf85a55a4e9dfbbd.bindPopup(popup_81ea2411843516d77c35b64e2de15517)\\n ;\\n\\n \\n \\n \\n var circle_marker_e909a5629558ea9b691ce6cf29e0ab67 = L.circleMarker(\\n [42.72912976589343, -73.27102214841915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_304424852e6d1b63615995fbf1040173 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b69bf478836cfab60235e4ee3e02ab1e = $(`<div id="html_b69bf478836cfab60235e4ee3e02ab1e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_304424852e6d1b63615995fbf1040173.setContent(html_b69bf478836cfab60235e4ee3e02ab1e);\\n \\n \\n\\n circle_marker_e909a5629558ea9b691ce6cf29e0ab67.bindPopup(popup_304424852e6d1b63615995fbf1040173)\\n ;\\n\\n \\n \\n \\n var circle_marker_e581acadf881a9149b3f54f358caa308 = L.circleMarker(\\n [42.72912982513782, -73.2697920859079],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c64b0835bb485ad6f3fe24637fd76c94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b5cbf631b0f510e03339482a7bc31a0 = $(`<div id="html_2b5cbf631b0f510e03339482a7bc31a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c64b0835bb485ad6f3fe24637fd76c94.setContent(html_2b5cbf631b0f510e03339482a7bc31a0);\\n \\n \\n\\n circle_marker_e581acadf881a9149b3f54f358caa308.bindPopup(popup_c64b0835bb485ad6f3fe24637fd76c94)\\n ;\\n\\n \\n \\n \\n var circle_marker_214920187505b4a435219e08c5433c62 = L.circleMarker(\\n [42.729129871216784, -73.26856202339455],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_3559c3a00f891b72d30febcaeba2c6c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56d508170e5231abd7695fe8a89644b5 = $(`<div id="html_56d508170e5231abd7695fe8a89644b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3559c3a00f891b72d30febcaeba2c6c7.setContent(html_56d508170e5231abd7695fe8a89644b5);\\n \\n \\n\\n circle_marker_214920187505b4a435219e08c5433c62.bindPopup(popup_3559c3a00f891b72d30febcaeba2c6c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_5955be34b48696887cca1d6720532ea8 = L.circleMarker(\\n [42.72912990413034, -73.26733196087964],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_cd4ca8bace47d247395025c8868a4766 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0025449ccd3f3423e53627a52b3372a = $(`<div id="html_b0025449ccd3f3423e53627a52b3372a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd4ca8bace47d247395025c8868a4766.setContent(html_b0025449ccd3f3423e53627a52b3372a);\\n \\n \\n\\n circle_marker_5955be34b48696887cca1d6720532ea8.bindPopup(popup_cd4ca8bace47d247395025c8868a4766)\\n ;\\n\\n \\n \\n \\n var circle_marker_bedfdde5ced023acff46eb5a5f485ab2 = L.circleMarker(\\n [42.72912992387847, -73.26610189836369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2c76e977d7dbd3481e3a0b35bf097887 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33717b11db0918a12d0755ab080fd5d2 = $(`<div id="html_33717b11db0918a12d0755ab080fd5d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c76e977d7dbd3481e3a0b35bf097887.setContent(html_33717b11db0918a12d0755ab080fd5d2);\\n \\n \\n\\n circle_marker_bedfdde5ced023acff46eb5a5f485ab2.bindPopup(popup_2c76e977d7dbd3481e3a0b35bf097887)\\n ;\\n\\n \\n \\n \\n var circle_marker_596c42a7152c841045a232b30b122f54 = L.circleMarker(\\n [42.72912993046118, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5ee30b72f60904dd650c27feadd35b1e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_773fb59c8731e0c67a39a3f087d58861 = $(`<div id="html_773fb59c8731e0c67a39a3f087d58861" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ee30b72f60904dd650c27feadd35b1e.setContent(html_773fb59c8731e0c67a39a3f087d58861);\\n \\n \\n\\n circle_marker_596c42a7152c841045a232b30b122f54.bindPopup(popup_5ee30b72f60904dd650c27feadd35b1e)\\n ;\\n\\n \\n \\n \\n var circle_marker_98a92b692ef2923b6312dd3b4a9d53d3 = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_96f4e2144d5f423ca354f182641b107b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99950c6edde2a35367b29d9f8fbf37a0 = $(`<div id="html_99950c6edde2a35367b29d9f8fbf37a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96f4e2144d5f423ca354f182641b107b.setContent(html_99950c6edde2a35367b29d9f8fbf37a0);\\n \\n \\n\\n circle_marker_98a92b692ef2923b6312dd3b4a9d53d3.bindPopup(popup_96f4e2144d5f423ca354f182641b107b)\\n ;\\n\\n \\n \\n \\n var circle_marker_105c85ef24a42a4b9afa820ff49253dc = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_8ff3899e8d991a4dd0ab4c9eb6fa8208 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b164a0b2cbde1a8b58137da080d6806 = $(`<div id="html_9b164a0b2cbde1a8b58137da080d6806" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ff3899e8d991a4dd0ab4c9eb6fa8208.setContent(html_9b164a0b2cbde1a8b58137da080d6806);\\n \\n \\n\\n circle_marker_105c85ef24a42a4b9afa820ff49253dc.bindPopup(popup_8ff3899e8d991a4dd0ab4c9eb6fa8208)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e8da93edeec78d7a68515f37f2d2ca6 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_24049d45e8e24876114a53f821af2e3a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f21edbf6c00a50db8796e81cbdb52b0 = $(`<div id="html_8f21edbf6c00a50db8796e81cbdb52b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24049d45e8e24876114a53f821af2e3a.setContent(html_8f21edbf6c00a50db8796e81cbdb52b0);\\n \\n \\n\\n circle_marker_2e8da93edeec78d7a68515f37f2d2ca6.bindPopup(popup_24049d45e8e24876114a53f821af2e3a)\\n ;\\n\\n \\n \\n \\n var circle_marker_b167c623ab5d30795c59864fa4acb18a = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_9b2996277ba7daf1d9f189e5ab0e6315 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a146ac83cafe703e0eae0903880cd68 = $(`<div id="html_1a146ac83cafe703e0eae0903880cd68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b2996277ba7daf1d9f189e5ab0e6315.setContent(html_1a146ac83cafe703e0eae0903880cd68);\\n \\n \\n\\n circle_marker_b167c623ab5d30795c59864fa4acb18a.bindPopup(popup_9b2996277ba7daf1d9f189e5ab0e6315)\\n ;\\n\\n \\n \\n \\n var circle_marker_d32e5f71dfbdd166313847e5d8ff0555 = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_49af2fb03047538e7bb8bedef7c3d2df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5c70a3b2d42b44f23f870654669ba19 = $(`<div id="html_a5c70a3b2d42b44f23f870654669ba19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_49af2fb03047538e7bb8bedef7c3d2df.setContent(html_a5c70a3b2d42b44f23f870654669ba19);\\n \\n \\n\\n circle_marker_d32e5f71dfbdd166313847e5d8ff0555.bindPopup(popup_49af2fb03047538e7bb8bedef7c3d2df)\\n ;\\n\\n \\n \\n \\n var circle_marker_222cd162a3d262532c9de1ed7911e8ca = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_15578b190d605b17d10163daf796ea26 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_72d4cc8c778316eb2b92b382cc2423b1 = $(`<div id="html_72d4cc8c778316eb2b92b382cc2423b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_15578b190d605b17d10163daf796ea26.setContent(html_72d4cc8c778316eb2b92b382cc2423b1);\\n \\n \\n\\n circle_marker_222cd162a3d262532c9de1ed7911e8ca.bindPopup(popup_15578b190d605b17d10163daf796ea26)\\n ;\\n\\n \\n \\n \\n var circle_marker_efddd609b41fa6200ea683dc337f0e68 = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_4a94def2762d1f876840b9595599d697 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8bb0a1ae4599eebb25e8ec34e2948050 = $(`<div id="html_8bb0a1ae4599eebb25e8ec34e2948050" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a94def2762d1f876840b9595599d697.setContent(html_8bb0a1ae4599eebb25e8ec34e2948050);\\n \\n \\n\\n circle_marker_efddd609b41fa6200ea683dc337f0e68.bindPopup(popup_4a94def2762d1f876840b9595599d697)\\n ;\\n\\n \\n \\n \\n var circle_marker_feab091b63b5e2e930b85e8d1490c621 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_e8e10acee4b8077e7ebd3d9aad770750 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8798153b40a9b2e48652b8f683154890 = $(`<div id="html_8798153b40a9b2e48652b8f683154890" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8e10acee4b8077e7ebd3d9aad770750.setContent(html_8798153b40a9b2e48652b8f683154890);\\n \\n \\n\\n circle_marker_feab091b63b5e2e930b85e8d1490c621.bindPopup(popup_e8e10acee4b8077e7ebd3d9aad770750)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b4465edb627d71524aa05dccae0661e = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_04abc12d228ddef94b48be05a7507d87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2d8e0359c79722f600283c909b0e4d1d = $(`<div id="html_2d8e0359c79722f600283c909b0e4d1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04abc12d228ddef94b48be05a7507d87.setContent(html_2d8e0359c79722f600283c909b0e4d1d);\\n \\n \\n\\n circle_marker_3b4465edb627d71524aa05dccae0661e.bindPopup(popup_04abc12d228ddef94b48be05a7507d87)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7170b8155913a0dd30c90921c96e720 = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_33a6d0ad2d684a08a0074d184f6b4019 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d238e267a2aa107e1f617952ee0dc0a9 = $(`<div id="html_d238e267a2aa107e1f617952ee0dc0a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33a6d0ad2d684a08a0074d184f6b4019.setContent(html_d238e267a2aa107e1f617952ee0dc0a9);\\n \\n \\n\\n circle_marker_a7170b8155913a0dd30c90921c96e720.bindPopup(popup_33a6d0ad2d684a08a0074d184f6b4019)\\n ;\\n\\n \\n \\n \\n var circle_marker_183d062f8912989bf4720fc54b56cfbd = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_aa3637036199747ac51bcfabcea82595 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad2a73eddafa1375404f3fc664d85021 = $(`<div id="html_ad2a73eddafa1375404f3fc664d85021" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aa3637036199747ac51bcfabcea82595.setContent(html_ad2a73eddafa1375404f3fc664d85021);\\n \\n \\n\\n circle_marker_183d062f8912989bf4720fc54b56cfbd.bindPopup(popup_aa3637036199747ac51bcfabcea82595)\\n ;\\n\\n \\n \\n \\n var circle_marker_454602da9a7c810ad1e6ce93c163aa4b = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d3abc8d4e62ee98fafd7441d583a6213 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_379ba8bd1a4c9f809f1e87a6b85c410f = $(`<div id="html_379ba8bd1a4c9f809f1e87a6b85c410f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3abc8d4e62ee98fafd7441d583a6213.setContent(html_379ba8bd1a4c9f809f1e87a6b85c410f);\\n \\n \\n\\n circle_marker_454602da9a7c810ad1e6ce93c163aa4b.bindPopup(popup_d3abc8d4e62ee98fafd7441d583a6213)\\n ;\\n\\n \\n \\n \\n var circle_marker_f762c759ce17105d462b5ef253360643 = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_46000766da9799f6aeca938700f6510d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e5c3246b4af30f40a7533dde02535be = $(`<div id="html_2e5c3246b4af30f40a7533dde02535be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46000766da9799f6aeca938700f6510d.setContent(html_2e5c3246b4af30f40a7533dde02535be);\\n \\n \\n\\n circle_marker_f762c759ce17105d462b5ef253360643.bindPopup(popup_46000766da9799f6aeca938700f6510d)\\n ;\\n\\n \\n \\n \\n var circle_marker_125fb28b8a66752f0076c77612703ef0 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d3b304f20977178cd466d6754ac0c2d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e10bfe70ab30ebe07d9700a6824d1b6 = $(`<div id="html_1e10bfe70ab30ebe07d9700a6824d1b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3b304f20977178cd466d6754ac0c2d9.setContent(html_1e10bfe70ab30ebe07d9700a6824d1b6);\\n \\n \\n\\n circle_marker_125fb28b8a66752f0076c77612703ef0.bindPopup(popup_d3b304f20977178cd466d6754ac0c2d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e738831e8a938a39499496972fff955 = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_65e0ebfcb59cddc8f06bf2bae9a56058 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5bc51777153bf86f4ad81d2d4201d313 = $(`<div id="html_5bc51777153bf86f4ad81d2d4201d313" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65e0ebfcb59cddc8f06bf2bae9a56058.setContent(html_5bc51777153bf86f4ad81d2d4201d313);\\n \\n \\n\\n circle_marker_6e738831e8a938a39499496972fff955.bindPopup(popup_65e0ebfcb59cddc8f06bf2bae9a56058)\\n ;\\n\\n \\n \\n \\n var circle_marker_101b0da2885f526c5b76576aa7fddb20 = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_76b2fb232771d4e80c79bba137e0b1e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b57016848c970a1a3c8ad818556f6efa = $(`<div id="html_b57016848c970a1a3c8ad818556f6efa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76b2fb232771d4e80c79bba137e0b1e1.setContent(html_b57016848c970a1a3c8ad818556f6efa);\\n \\n \\n\\n circle_marker_101b0da2885f526c5b76576aa7fddb20.bindPopup(popup_76b2fb232771d4e80c79bba137e0b1e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_132642acd4f23d74fae6061396131c31 = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_3d95ecc6b2107af10992a43e2509b16c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a9db19075988f72539b97f3f4e9d6a2 = $(`<div id="html_3a9db19075988f72539b97f3f4e9d6a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d95ecc6b2107af10992a43e2509b16c.setContent(html_3a9db19075988f72539b97f3f4e9d6a2);\\n \\n \\n\\n circle_marker_132642acd4f23d74fae6061396131c31.bindPopup(popup_3d95ecc6b2107af10992a43e2509b16c)\\n ;\\n\\n \\n \\n \\n var circle_marker_42b64a9360e30cccfa7be92ff6eb923c = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_f7094614197b485026c4f5c677c91176 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b10bb07bc8eae6b273feeab653419531 = $(`<div id="html_b10bb07bc8eae6b273feeab653419531" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7094614197b485026c4f5c677c91176.setContent(html_b10bb07bc8eae6b273feeab653419531);\\n \\n \\n\\n circle_marker_42b64a9360e30cccfa7be92ff6eb923c.bindPopup(popup_f7094614197b485026c4f5c677c91176)\\n ;\\n\\n \\n \\n \\n var circle_marker_214c4851efcc08d2198b31e5f2c1025c = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_682e03440937fb8d68c0622ec77cbfd9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1390432d68443849c62cd29be8b95a31 = $(`<div id="html_1390432d68443849c62cd29be8b95a31" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_682e03440937fb8d68c0622ec77cbfd9.setContent(html_1390432d68443849c62cd29be8b95a31);\\n \\n \\n\\n circle_marker_214c4851efcc08d2198b31e5f2c1025c.bindPopup(popup_682e03440937fb8d68c0622ec77cbfd9)\\n ;\\n\\n \\n \\n \\n var circle_marker_5208fecd10e3b3914bfddedb638c11e3 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5596448d1368d256560e89c3f37dbc8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1264b27774aa96ec5591f44ff635b23 = $(`<div id="html_b1264b27774aa96ec5591f44ff635b23" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5596448d1368d256560e89c3f37dbc8d.setContent(html_b1264b27774aa96ec5591f44ff635b23);\\n \\n \\n\\n circle_marker_5208fecd10e3b3914bfddedb638c11e3.bindPopup(popup_5596448d1368d256560e89c3f37dbc8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a0bf77720592ddc9072d9d945c19ff6 = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_8d0e3e75efa272d619960add3e9fb600 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_642c058128609325a0016348cd028804 = $(`<div id="html_642c058128609325a0016348cd028804" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d0e3e75efa272d619960add3e9fb600.setContent(html_642c058128609325a0016348cd028804);\\n \\n \\n\\n circle_marker_0a0bf77720592ddc9072d9d945c19ff6.bindPopup(popup_8d0e3e75efa272d619960add3e9fb600)\\n ;\\n\\n \\n \\n \\n var circle_marker_88000dd5cb3da5e3f8b2d3eb6717459f = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_9a311239eda4c35b8b10f78b4e36ef33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1bb9b07e59e9b7779fff386e41df425 = $(`<div id="html_d1bb9b07e59e9b7779fff386e41df425" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a311239eda4c35b8b10f78b4e36ef33.setContent(html_d1bb9b07e59e9b7779fff386e41df425);\\n \\n \\n\\n circle_marker_88000dd5cb3da5e3f8b2d3eb6717459f.bindPopup(popup_9a311239eda4c35b8b10f78b4e36ef33)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c8b0562133e031c012f1ecacb909065 = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_3fbd5b623955e9b4f8ccdbb879e2f4d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a5718468ea9ce465db757bb7579f3ce = $(`<div id="html_8a5718468ea9ce465db757bb7579f3ce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3fbd5b623955e9b4f8ccdbb879e2f4d9.setContent(html_8a5718468ea9ce465db757bb7579f3ce);\\n \\n \\n\\n circle_marker_3c8b0562133e031c012f1ecacb909065.bindPopup(popup_3fbd5b623955e9b4f8ccdbb879e2f4d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_a92d14f52b916fae2570d0caefc49ed4 = L.circleMarker(\\n [42.727322737845135, -73.26856191587537],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_58d65e893c494e5066f1bbcef8b837c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a167fd426f375b79e8d620d9d74e1a76 = $(`<div id="html_a167fd426f375b79e8d620d9d74e1a76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58d65e893c494e5066f1bbcef8b837c1.setContent(html_a167fd426f375b79e8d620d9d74e1a76);\\n \\n \\n\\n circle_marker_a92d14f52b916fae2570d0caefc49ed4.bindPopup(popup_58d65e893c494e5066f1bbcef8b837c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_08cb191871156ca2b3ae06607bcbd955 = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_e925f372f08b948c0b9beb976b702922 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f547e32e2fb2c16f2aeda8679af2ecdf = $(`<div id="html_f547e32e2fb2c16f2aeda8679af2ecdf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e925f372f08b948c0b9beb976b702922.setContent(html_f547e32e2fb2c16f2aeda8679af2ecdf);\\n \\n \\n\\n circle_marker_08cb191871156ca2b3ae06607bcbd955.bindPopup(popup_e925f372f08b948c0b9beb976b702922)\\n ;\\n\\n \\n \\n \\n var circle_marker_034b6775e790c4cc52a856bfeae3a48f = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_99f1b00ad272adb3cdd300dafa06ba65 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a438785b94379d0cd4478e22c088e0e1 = $(`<div id="html_a438785b94379d0cd4478e22c088e0e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99f1b00ad272adb3cdd300dafa06ba65.setContent(html_a438785b94379d0cd4478e22c088e0e1);\\n \\n \\n\\n circle_marker_034b6775e790c4cc52a856bfeae3a48f.bindPopup(popup_99f1b00ad272adb3cdd300dafa06ba65)\\n ;\\n\\n \\n \\n \\n var circle_marker_159547c99dcbaed2370dc408dc921f0e = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c150410e6a6605ac3bf380ddb4958e34 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6282aaf799de1cb2917958941d7775a6 = $(`<div id="html_6282aaf799de1cb2917958941d7775a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c150410e6a6605ac3bf380ddb4958e34.setContent(html_6282aaf799de1cb2917958941d7775a6);\\n \\n \\n\\n circle_marker_159547c99dcbaed2370dc408dc921f0e.bindPopup(popup_c150410e6a6605ac3bf380ddb4958e34)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ad89f2257fc76a1a0fd087228dc45a6 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c3a66bb5f91c097fb72e7aced6a33349 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ffe883350dfe19222e79a35f244367b = $(`<div id="html_0ffe883350dfe19222e79a35f244367b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3a66bb5f91c097fb72e7aced6a33349.setContent(html_0ffe883350dfe19222e79a35f244367b);\\n \\n \\n\\n circle_marker_0ad89f2257fc76a1a0fd087228dc45a6.bindPopup(popup_c3a66bb5f91c097fb72e7aced6a33349)\\n ;\\n\\n \\n \\n \\n var circle_marker_03dc9274c761cbb9588d94175299eb0f = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6ad51af29bb954ac6862060289f275c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e8d84845295144bb2cc7d64a8dcde41e = $(`<div id="html_e8d84845295144bb2cc7d64a8dcde41e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ad51af29bb954ac6862060289f275c6.setContent(html_e8d84845295144bb2cc7d64a8dcde41e);\\n \\n \\n\\n circle_marker_03dc9274c761cbb9588d94175299eb0f.bindPopup(popup_6ad51af29bb954ac6862060289f275c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_df6d417922c6a529f395981a739bc68c = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d75ddc38c796c23ebf99d8ca23d03fc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_814a54cd40ce04e57ffaba3efd3e1595 = $(`<div id="html_814a54cd40ce04e57ffaba3efd3e1595" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d75ddc38c796c23ebf99d8ca23d03fc1.setContent(html_814a54cd40ce04e57ffaba3efd3e1595);\\n \\n \\n\\n circle_marker_df6d417922c6a529f395981a739bc68c.bindPopup(popup_d75ddc38c796c23ebf99d8ca23d03fc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_e85b263440d418be9d4adb14be163e3e = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_392b0bc4b1191cb9fbbcdb577e1149ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a52e771e5ff18dfe6f16cde5194b24ff = $(`<div id="html_a52e771e5ff18dfe6f16cde5194b24ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_392b0bc4b1191cb9fbbcdb577e1149ef.setContent(html_a52e771e5ff18dfe6f16cde5194b24ff);\\n \\n \\n\\n circle_marker_e85b263440d418be9d4adb14be163e3e.bindPopup(popup_392b0bc4b1191cb9fbbcdb577e1149ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_85c21a31e9109258f5fb0bac685d234c = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_b6782dc0cd7b4bdbc628567cf58ccd38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64299b0adbbb07475b2692eb14750327 = $(`<div id="html_64299b0adbbb07475b2692eb14750327" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b6782dc0cd7b4bdbc628567cf58ccd38.setContent(html_64299b0adbbb07475b2692eb14750327);\\n \\n \\n\\n circle_marker_85c21a31e9109258f5fb0bac685d234c.bindPopup(popup_b6782dc0cd7b4bdbc628567cf58ccd38)\\n ;\\n\\n \\n \\n \\n var circle_marker_bc639a4cba29a7a1cc24f519998e9130 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_406e650fdaa08fbfd0b3716b39ca50a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de893d0b5d099a940ef33ebfe165ed31 = $(`<div id="html_de893d0b5d099a940ef33ebfe165ed31" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_406e650fdaa08fbfd0b3716b39ca50a5.setContent(html_de893d0b5d099a940ef33ebfe165ed31);\\n \\n \\n\\n circle_marker_bc639a4cba29a7a1cc24f519998e9130.bindPopup(popup_406e650fdaa08fbfd0b3716b39ca50a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3fec42184faf1fa11df7d334edd180a = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_305c04231da09bbdc95ab74d9d1fd146 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5619f9a6a4ad470f57ea4555bb450bfb = $(`<div id="html_5619f9a6a4ad470f57ea4555bb450bfb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_305c04231da09bbdc95ab74d9d1fd146.setContent(html_5619f9a6a4ad470f57ea4555bb450bfb);\\n \\n \\n\\n circle_marker_c3fec42184faf1fa11df7d334edd180a.bindPopup(popup_305c04231da09bbdc95ab74d9d1fd146)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e74f3bf767cb524a4dea6f442e426de = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d1e578b9462a1d2643cdfd71e531e3fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a07099cb154daeb76cc554d19409cf8c = $(`<div id="html_a07099cb154daeb76cc554d19409cf8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d1e578b9462a1d2643cdfd71e531e3fe.setContent(html_a07099cb154daeb76cc554d19409cf8c);\\n \\n \\n\\n circle_marker_6e74f3bf767cb524a4dea6f442e426de.bindPopup(popup_d1e578b9462a1d2643cdfd71e531e3fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_8ee10ca88dcd189c24152697b7854994 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_046d3c9d53658f03d0f1668187aa2dd3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c839953ae8848b87caf3d9e2b94f472b = $(`<div id="html_c839953ae8848b87caf3d9e2b94f472b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_046d3c9d53658f03d0f1668187aa2dd3.setContent(html_c839953ae8848b87caf3d9e2b94f472b);\\n \\n \\n\\n circle_marker_8ee10ca88dcd189c24152697b7854994.bindPopup(popup_046d3c9d53658f03d0f1668187aa2dd3)\\n ;\\n\\n \\n \\n \\n var circle_marker_89032a1a3fab07a780359fa9ae2f6cc4 = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_ac90d911c3e31dc6d066bf6ade2f8e6f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_868413bbb35a91211f7e599962df6340 = $(`<div id="html_868413bbb35a91211f7e599962df6340" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac90d911c3e31dc6d066bf6ade2f8e6f.setContent(html_868413bbb35a91211f7e599962df6340);\\n \\n \\n\\n circle_marker_89032a1a3fab07a780359fa9ae2f6cc4.bindPopup(popup_ac90d911c3e31dc6d066bf6ade2f8e6f)\\n ;\\n\\n \\n \\n \\n var circle_marker_86af89f30010119cec70609241838580 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_e355ef361c160e4adc829e6683f612d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8340c090b64824c3fa00e9e683bfda6 = $(`<div id="html_a8340c090b64824c3fa00e9e683bfda6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e355ef361c160e4adc829e6683f612d4.setContent(html_a8340c090b64824c3fa00e9e683bfda6);\\n \\n \\n\\n circle_marker_86af89f30010119cec70609241838580.bindPopup(popup_e355ef361c160e4adc829e6683f612d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_013353b1519081b743494ee7f8c7267d = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_5d5e6dc925ca21a6a259fb0fe566ea44 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dac1df9f280aab9199036e69cd0890f8 = $(`<div id="html_dac1df9f280aab9199036e69cd0890f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d5e6dc925ca21a6a259fb0fe566ea44.setContent(html_dac1df9f280aab9199036e69cd0890f8);\\n \\n \\n\\n circle_marker_013353b1519081b743494ee7f8c7267d.bindPopup(popup_5d5e6dc925ca21a6a259fb0fe566ea44)\\n ;\\n\\n \\n \\n \\n var circle_marker_fcfa12af0875e2eac2d4f8f70bf54820 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2c6fe6ad4cf1b8628d3577cf4ee6e529 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc3ae9a5935b454cd997a841ed7e4a92 = $(`<div id="html_cc3ae9a5935b454cd997a841ed7e4a92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c6fe6ad4cf1b8628d3577cf4ee6e529.setContent(html_cc3ae9a5935b454cd997a841ed7e4a92);\\n \\n \\n\\n circle_marker_fcfa12af0875e2eac2d4f8f70bf54820.bindPopup(popup_2c6fe6ad4cf1b8628d3577cf4ee6e529)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1f086f0015002398b88d5163aa54e72 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_d805774bbb7d48b8f1fe887590395418 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e606bfb4fabb53cbc5dfbbffd197e685 = $(`<div id="html_e606bfb4fabb53cbc5dfbbffd197e685" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d805774bbb7d48b8f1fe887590395418.setContent(html_e606bfb4fabb53cbc5dfbbffd197e685);\\n \\n \\n\\n circle_marker_d1f086f0015002398b88d5163aa54e72.bindPopup(popup_d805774bbb7d48b8f1fe887590395418)\\n ;\\n\\n \\n \\n \\n var circle_marker_334a8d46727d961456517bde43bff551 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_76a176b0d5d0d38f21e87f488a09cc87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c5c852163fa33fa0ba16e334c770bce = $(`<div id="html_9c5c852163fa33fa0ba16e334c770bce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76a176b0d5d0d38f21e87f488a09cc87.setContent(html_9c5c852163fa33fa0ba16e334c770bce);\\n \\n \\n\\n circle_marker_334a8d46727d961456517bde43bff551.bindPopup(popup_76a176b0d5d0d38f21e87f488a09cc87)\\n ;\\n\\n \\n \\n \\n var circle_marker_e69b4aed2b4f4250157f93326f40b749 = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1684de203195047a1db19caa36214093 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b3a9e27cfa7029eb4e595c92d3cbe9e = $(`<div id="html_8b3a9e27cfa7029eb4e595c92d3cbe9e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1684de203195047a1db19caa36214093.setContent(html_8b3a9e27cfa7029eb4e595c92d3cbe9e);\\n \\n \\n\\n circle_marker_e69b4aed2b4f4250157f93326f40b749.bindPopup(popup_1684de203195047a1db19caa36214093)\\n ;\\n\\n \\n \\n \\n var circle_marker_3dc843e7112783046071078de7637a53 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_1c48d7b815a7722792b1e6c564e10055 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bee9d37b417607908f4ebd882b929543 = $(`<div id="html_bee9d37b417607908f4ebd882b929543" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1c48d7b815a7722792b1e6c564e10055.setContent(html_bee9d37b417607908f4ebd882b929543);\\n \\n \\n\\n circle_marker_3dc843e7112783046071078de7637a53.bindPopup(popup_1c48d7b815a7722792b1e6c564e10055)\\n ;\\n\\n \\n \\n \\n var circle_marker_6bffa4fe6414eacdfa269cac36cc12c5 = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_0a2bd52fad244333112202902ad07dda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ed20dbb64f5ffeddf3ca68a1d521e7f = $(`<div id="html_4ed20dbb64f5ffeddf3ca68a1d521e7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a2bd52fad244333112202902ad07dda.setContent(html_4ed20dbb64f5ffeddf3ca68a1d521e7f);\\n \\n \\n\\n circle_marker_6bffa4fe6414eacdfa269cac36cc12c5.bindPopup(popup_0a2bd52fad244333112202902ad07dda)\\n ;\\n\\n \\n \\n \\n var circle_marker_59dacc993da654a17e051d7ab7c83e19 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_56e2c760f0de667d1b23c7c08f163f24 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37deb9891416f10afec5cacc98b93f43 = $(`<div id="html_37deb9891416f10afec5cacc98b93f43" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_56e2c760f0de667d1b23c7c08f163f24.setContent(html_37deb9891416f10afec5cacc98b93f43);\\n \\n \\n\\n circle_marker_59dacc993da654a17e051d7ab7c83e19.bindPopup(popup_56e2c760f0de667d1b23c7c08f163f24)\\n ;\\n\\n \\n \\n \\n var circle_marker_6bd9e6826793613a8e48e342459eff33 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_767b6adca523c5e3d4594a3deca3f4ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b719e729bc80eb1c67151d52d6bbd331 = $(`<div id="html_b719e729bc80eb1c67151d52d6bbd331" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_767b6adca523c5e3d4594a3deca3f4ca.setContent(html_b719e729bc80eb1c67151d52d6bbd331);\\n \\n \\n\\n circle_marker_6bd9e6826793613a8e48e342459eff33.bindPopup(popup_767b6adca523c5e3d4594a3deca3f4ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_cd6942c68a0e0f6dcf759d1bbf9743ec = L.circleMarker(\\n [42.725515657130885, -73.26610182668713],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_13d4c849d8ebc27ac6b130a1fbcd3988 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_29cab4564c3905e971aa9822ec0bdcbc = $(`<div id="html_29cab4564c3905e971aa9822ec0bdcbc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13d4c849d8ebc27ac6b130a1fbcd3988.setContent(html_29cab4564c3905e971aa9822ec0bdcbc);\\n \\n \\n\\n circle_marker_cd6942c68a0e0f6dcf759d1bbf9743ec.bindPopup(popup_13d4c849d8ebc27ac6b130a1fbcd3988)\\n ;\\n\\n \\n \\n \\n var circle_marker_435ac248c49a031cca6e3fec48553f1f = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_9d304a1e4362ddcc364e1e6813dea2b0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd299e979057d942b6e79c5e1a2bcde0 = $(`<div id="html_dd299e979057d942b6e79c5e1a2bcde0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d304a1e4362ddcc364e1e6813dea2b0.setContent(html_dd299e979057d942b6e79c5e1a2bcde0);\\n \\n \\n\\n circle_marker_435ac248c49a031cca6e3fec48553f1f.bindPopup(popup_9d304a1e4362ddcc364e1e6813dea2b0)\\n ;\\n\\n \\n \\n \\n var circle_marker_c24c295a2b5dd33e556b1c163cb35691 = L.circleMarker(\\n [42.72551555839823, -73.25995187249272],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_854bb94230551cda82ad944a8841566e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_696b21aab752f50568449fcacbb53f56 = $(`<div id="html_696b21aab752f50568449fcacbb53f56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_854bb94230551cda82ad944a8841566e.setContent(html_696b21aab752f50568449fcacbb53f56);\\n \\n \\n\\n circle_marker_c24c295a2b5dd33e556b1c163cb35691.bindPopup(popup_854bb94230551cda82ad944a8841566e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3adc7cb497dccba60d6721f5b392dc5 = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_78e334d06025ed300ce37542b77c5055 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a10d0342f12e53a8ff2d637a9f4b607f = $(`<div id="html_a10d0342f12e53a8ff2d637a9f4b607f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_78e334d06025ed300ce37542b77c5055.setContent(html_a10d0342f12e53a8ff2d637a9f4b607f);\\n \\n \\n\\n circle_marker_e3adc7cb497dccba60d6721f5b392dc5.bindPopup(popup_78e334d06025ed300ce37542b77c5055)\\n ;\\n\\n \\n \\n \\n var circle_marker_a03b5f16ec6c7a64b8bad8cdb24612f1 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_917a1ec1f6b1885ba5ddd5fbf9d26233 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5092ef01825e56fdcd2fdf7c987fb215 = $(`<div id="html_5092ef01825e56fdcd2fdf7c987fb215" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_917a1ec1f6b1885ba5ddd5fbf9d26233.setContent(html_5092ef01825e56fdcd2fdf7c987fb215);\\n \\n \\n\\n circle_marker_a03b5f16ec6c7a64b8bad8cdb24612f1.bindPopup(popup_917a1ec1f6b1885ba5ddd5fbf9d26233)\\n ;\\n\\n \\n \\n \\n var circle_marker_bb35663f479dd4de0bf249ba4c9557ba = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_820960dcaff70f83e1b214d40c04af23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a67f789cb2197140b69c3efdec3f8a5 = $(`<div id="html_8a67f789cb2197140b69c3efdec3f8a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_820960dcaff70f83e1b214d40c04af23.setContent(html_8a67f789cb2197140b69c3efdec3f8a5);\\n \\n \\n\\n circle_marker_bb35663f479dd4de0bf249ba4c9557ba.bindPopup(popup_820960dcaff70f83e1b214d40c04af23)\\n ;\\n\\n \\n \\n \\n var circle_marker_de780f7781f52a36ac4410aebc79ce30 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_29ce64b0f6014a8c7ad639169775e2ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ccbf859b58d2e3b50457a9e09088ed9d = $(`<div id="html_ccbf859b58d2e3b50457a9e09088ed9d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_29ce64b0f6014a8c7ad639169775e2ca.setContent(html_ccbf859b58d2e3b50457a9e09088ed9d);\\n \\n \\n\\n circle_marker_de780f7781f52a36ac4410aebc79ce30.bindPopup(popup_29ce64b0f6014a8c7ad639169775e2ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_39d76e5f6155209f113437cde2ce01e1 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_836a130a30217e331c458d4ee1ac8a39 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de600d8bc7e48bf35f80c29f8c24f0d5 = $(`<div id="html_de600d8bc7e48bf35f80c29f8c24f0d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_836a130a30217e331c458d4ee1ac8a39.setContent(html_de600d8bc7e48bf35f80c29f8c24f0d5);\\n \\n \\n\\n circle_marker_39d76e5f6155209f113437cde2ce01e1.bindPopup(popup_836a130a30217e331c458d4ee1ac8a39)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba8207d7739e1e42a80f0c479a130919 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_57be970dd162280120d8a0bf22a21942 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f2fbef1769d63018cd19b71d763ed45 = $(`<div id="html_8f2fbef1769d63018cd19b71d763ed45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_57be970dd162280120d8a0bf22a21942.setContent(html_8f2fbef1769d63018cd19b71d763ed45);\\n \\n \\n\\n circle_marker_ba8207d7739e1e42a80f0c479a130919.bindPopup(popup_57be970dd162280120d8a0bf22a21942)\\n ;\\n\\n \\n \\n \\n var circle_marker_da56b93d9ff8b91edd43630ada60111a = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c596f62fb75d5ac43f1131b67c39016e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b150f5b8f046b80cf75773c2e2eeffb = $(`<div id="html_5b150f5b8f046b80cf75773c2e2eeffb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c596f62fb75d5ac43f1131b67c39016e.setContent(html_5b150f5b8f046b80cf75773c2e2eeffb);\\n \\n \\n\\n circle_marker_da56b93d9ff8b91edd43630ada60111a.bindPopup(popup_c596f62fb75d5ac43f1131b67c39016e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff78189039646ce8f3b4c3c29b52083f = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_12d74912ad50880f217f11181c295450 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e765fa8f95d152c1baaf7849d37f2997 = $(`<div id="html_e765fa8f95d152c1baaf7849d37f2997" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12d74912ad50880f217f11181c295450.setContent(html_e765fa8f95d152c1baaf7849d37f2997);\\n \\n \\n\\n circle_marker_ff78189039646ce8f3b4c3c29b52083f.bindPopup(popup_12d74912ad50880f217f11181c295450)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c51efd00230e7dd8d347bc4c84bf266 = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_2a548aa77c9ebcdca8dc34c6024d1ece = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cdcb5945f061339860df34788601862a = $(`<div id="html_cdcb5945f061339860df34788601862a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a548aa77c9ebcdca8dc34c6024d1ece.setContent(html_cdcb5945f061339860df34788601862a);\\n \\n \\n\\n circle_marker_5c51efd00230e7dd8d347bc4c84bf266.bindPopup(popup_2a548aa77c9ebcdca8dc34c6024d1ece)\\n ;\\n\\n \\n \\n \\n var circle_marker_52cb0fdecef8c3eba50e9c1d3cbd05ad = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_c9e8a89dc557c70ae605c7e315d57061 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f6d6de5cc7a653485a20381f096336e = $(`<div id="html_5f6d6de5cc7a653485a20381f096336e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9e8a89dc557c70ae605c7e315d57061.setContent(html_5f6d6de5cc7a653485a20381f096336e);\\n \\n \\n\\n circle_marker_52cb0fdecef8c3eba50e9c1d3cbd05ad.bindPopup(popup_c9e8a89dc557c70ae605c7e315d57061)\\n ;\\n\\n \\n \\n \\n var circle_marker_46cc503ed2d7d4ec088e39d7f16151e3 = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_8ca7546a75266a3fa1c5145cb4242cf9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44e036f020bd0247e59b85925a2d6319 = $(`<div id="html_44e036f020bd0247e59b85925a2d6319" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ca7546a75266a3fa1c5145cb4242cf9.setContent(html_44e036f020bd0247e59b85925a2d6319);\\n \\n \\n\\n circle_marker_46cc503ed2d7d4ec088e39d7f16151e3.bindPopup(popup_8ca7546a75266a3fa1c5145cb4242cf9)\\n ;\\n\\n \\n \\n \\n var circle_marker_fde6988e4ca1d5e9c490e78d11541764 = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_6da6c91cf7f5e459b4f2b33e1e09912d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea475ee192bda85fea9884ba8c7bad90 = $(`<div id="html_ea475ee192bda85fea9884ba8c7bad90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6da6c91cf7f5e459b4f2b33e1e09912d.setContent(html_ea475ee192bda85fea9884ba8c7bad90);\\n \\n \\n\\n circle_marker_fde6988e4ca1d5e9c490e78d11541764.bindPopup(popup_6da6c91cf7f5e459b4f2b33e1e09912d)\\n ;\\n\\n \\n \\n \\n var circle_marker_eedd83e921ccad816128891c912b7719 = L.circleMarker(\\n [42.72370842502844, -73.2599520158284],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_ada076fff6742aa63fa7db26456f5316 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_407286ff9030c6f14352fe40f6a2bfc9 = $(`<div id="html_407286ff9030c6f14352fe40f6a2bfc9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ada076fff6742aa63fa7db26456f5316.setContent(html_407286ff9030c6f14352fe40f6a2bfc9);\\n \\n \\n\\n circle_marker_eedd83e921ccad816128891c912b7719.bindPopup(popup_ada076fff6742aa63fa7db26456f5316)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b2935d6123f74c2f8f76fd71ea45460 = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_81c4cc4a65ef2fd10cecb8458a135561 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a79e38dc45c31160927ee5a5b13afb67 = $(`<div id="html_a79e38dc45c31160927ee5a5b13afb67" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81c4cc4a65ef2fd10cecb8458a135561.setContent(html_a79e38dc45c31160927ee5a5b13afb67);\\n \\n \\n\\n circle_marker_1b2935d6123f74c2f8f76fd71ea45460.bindPopup(popup_81c4cc4a65ef2fd10cecb8458a135561)\\n ;\\n\\n \\n \\n \\n var circle_marker_47ca21cbade9f51c38ea97e95b991241 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_ba69442bb6470fcbc989272505656bb0);\\n \\n \\n var popup_db33c1f857be982446d3b62e7db34341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2dc312186d9d86ce785d28474d91894 = $(`<div id="html_e2dc312186d9d86ce785d28474d91894" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db33c1f857be982446d3b62e7db34341.setContent(html_e2dc312186d9d86ce785d28474d91894);\\n \\n \\n\\n circle_marker_47ca21cbade9f51c38ea97e95b991241.bindPopup(popup_db33c1f857be982446d3b62e7db34341)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Bitternut hickory": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_71c11555b5d0e4615bf906907516696b {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_71c11555b5d0e4615bf906907516696b" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_71c11555b5d0e4615bf906907516696b = L.map(\\n "map_71c11555b5d0e4615bf906907516696b",\\n {\\n center: [42.73183626942616, -73.23165975617428],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_fa25502a9786a5ee8c0c47697113a63f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var circle_marker_2d6bdf335d471ed584e13780e49b09b4 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_cfd993448b5c2ab6d994a8b0b8b1353f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68190fcc6f47438b8225a59653091920 = $(`<div id="html_68190fcc6f47438b8225a59653091920" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfd993448b5c2ab6d994a8b0b8b1353f.setContent(html_68190fcc6f47438b8225a59653091920);\\n \\n \\n\\n circle_marker_2d6bdf335d471ed584e13780e49b09b4.bindPopup(popup_cfd993448b5c2ab6d994a8b0b8b1353f)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9f200852fbc2f09cbfab604ada454e6 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_91cbbeab6694bb1aa6ddd17982762e62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b76d98348a5859eef0752b77a23981d3 = $(`<div id="html_b76d98348a5859eef0752b77a23981d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91cbbeab6694bb1aa6ddd17982762e62.setContent(html_b76d98348a5859eef0752b77a23981d3);\\n \\n \\n\\n circle_marker_a9f200852fbc2f09cbfab604ada454e6.bindPopup(popup_91cbbeab6694bb1aa6ddd17982762e62)\\n ;\\n\\n \\n \\n \\n var circle_marker_022d2de061e5fc1598df39d2c230bcb5 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_6f8a63ce8a6b55870c0002efc5fe0ee9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea4eca149c5a26a6eb89393d7cab9320 = $(`<div id="html_ea4eca149c5a26a6eb89393d7cab9320" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f8a63ce8a6b55870c0002efc5fe0ee9.setContent(html_ea4eca149c5a26a6eb89393d7cab9320);\\n \\n \\n\\n circle_marker_022d2de061e5fc1598df39d2c230bcb5.bindPopup(popup_6f8a63ce8a6b55870c0002efc5fe0ee9)\\n ;\\n\\n \\n \\n \\n var circle_marker_51e6b83a58207df6b937a58834583065 = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_f5f89a45a34b54d7840af10b008dd09d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edc4491f0e5a7d5498843e5814539eab = $(`<div id="html_edc4491f0e5a7d5498843e5814539eab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5f89a45a34b54d7840af10b008dd09d.setContent(html_edc4491f0e5a7d5498843e5814539eab);\\n \\n \\n\\n circle_marker_51e6b83a58207df6b937a58834583065.bindPopup(popup_f5f89a45a34b54d7840af10b008dd09d)\\n ;\\n\\n \\n \\n \\n var circle_marker_254db0160904c626f147d76c46645c4f = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_12a49e283c8c57b130e4a940f1978530 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a03ef0ea256cc70a3992eb0592b3a7c = $(`<div id="html_8a03ef0ea256cc70a3992eb0592b3a7c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12a49e283c8c57b130e4a940f1978530.setContent(html_8a03ef0ea256cc70a3992eb0592b3a7c);\\n \\n \\n\\n circle_marker_254db0160904c626f147d76c46645c4f.bindPopup(popup_12a49e283c8c57b130e4a940f1978530)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd2c37ef1bb991b2dd03e7a41b52ebf6 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_be3b6877953c73a472c6741a78548844 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ffca0482ac40f95b3e145d2a21cb3dcd = $(`<div id="html_ffca0482ac40f95b3e145d2a21cb3dcd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be3b6877953c73a472c6741a78548844.setContent(html_ffca0482ac40f95b3e145d2a21cb3dcd);\\n \\n \\n\\n circle_marker_dd2c37ef1bb991b2dd03e7a41b52ebf6.bindPopup(popup_be3b6877953c73a472c6741a78548844)\\n ;\\n\\n \\n \\n \\n var circle_marker_024ccf3219ee1e15d182366a6b447244 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_e8c049325dd73615901c3be672f2b4e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_213b0084d7d580e7c75150f5c807e021 = $(`<div id="html_213b0084d7d580e7c75150f5c807e021" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8c049325dd73615901c3be672f2b4e9.setContent(html_213b0084d7d580e7c75150f5c807e021);\\n \\n \\n\\n circle_marker_024ccf3219ee1e15d182366a6b447244.bindPopup(popup_e8c049325dd73615901c3be672f2b4e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_2937bda7d0be5ce41e71c73b370b725f = L.circleMarker(\\n [42.73635085311189, -73.22304483851624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_ade7a4fa13703fcf90bbb5a79253d2b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aafd0327cc67509eec521592264c13bd = $(`<div id="html_aafd0327cc67509eec521592264c13bd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ade7a4fa13703fcf90bbb5a79253d2b6.setContent(html_aafd0327cc67509eec521592264c13bd);\\n \\n \\n\\n circle_marker_2937bda7d0be5ce41e71c73b370b725f.bindPopup(popup_ade7a4fa13703fcf90bbb5a79253d2b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c3b77e78638a7d7d0519d3da150691f = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_e54b6a4f4e48eec7d4e9aa739b1d0f08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57f1eefe3ba9db4cd349e37142e54b16 = $(`<div id="html_57f1eefe3ba9db4cd349e37142e54b16" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e54b6a4f4e48eec7d4e9aa739b1d0f08.setContent(html_57f1eefe3ba9db4cd349e37142e54b16);\\n \\n \\n\\n circle_marker_8c3b77e78638a7d7d0519d3da150691f.bindPopup(popup_e54b6a4f4e48eec7d4e9aa739b1d0f08)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e1c7c57ebf58455e864766e638a8bd5 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_7754822740c086cbe8a8c4568355bc8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_90a15356d024e12481661354c35e1ccd = $(`<div id="html_90a15356d024e12481661354c35e1ccd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7754822740c086cbe8a8c4568355bc8b.setContent(html_90a15356d024e12481661354c35e1ccd);\\n \\n \\n\\n circle_marker_4e1c7c57ebf58455e864766e638a8bd5.bindPopup(popup_7754822740c086cbe8a8c4568355bc8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_1f08203e8c4ab06eeff57c9b65aa3f31 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_5c91af2d220a98f6642432fe7be3ab75 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5f8971ca6118d22a13c73401676f227 = $(`<div id="html_e5f8971ca6118d22a13c73401676f227" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c91af2d220a98f6642432fe7be3ab75.setContent(html_e5f8971ca6118d22a13c73401676f227);\\n \\n \\n\\n circle_marker_1f08203e8c4ab06eeff57c9b65aa3f31.bindPopup(popup_5c91af2d220a98f6642432fe7be3ab75)\\n ;\\n\\n \\n \\n \\n var circle_marker_601f3a1abbdbd9d4a7903a45bdb80e8f = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_61ef521730f4bb82f147e751a80f1b01 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c117dd8f7d20c88f0c798de68f60e4fc = $(`<div id="html_c117dd8f7d20c88f0c798de68f60e4fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61ef521730f4bb82f147e751a80f1b01.setContent(html_c117dd8f7d20c88f0c798de68f60e4fc);\\n \\n \\n\\n circle_marker_601f3a1abbdbd9d4a7903a45bdb80e8f.bindPopup(popup_61ef521730f4bb82f147e751a80f1b01)\\n ;\\n\\n \\n \\n \\n var circle_marker_0300a8283764af752beda215d5d9697d = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_5d09c07107c6965896e8dcd05653e3ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_931cca167585deb6d619ebbc476dd951 = $(`<div id="html_931cca167585deb6d619ebbc476dd951" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d09c07107c6965896e8dcd05653e3ae.setContent(html_931cca167585deb6d619ebbc476dd951);\\n \\n \\n\\n circle_marker_0300a8283764af752beda215d5d9697d.bindPopup(popup_5d09c07107c6965896e8dcd05653e3ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_c690c0310fcf99dbc2ab194f5be68f39 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_b33de39e73972685f96c8b29ce03800d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b44f78e4b711709b362634a11becee9 = $(`<div id="html_6b44f78e4b711709b362634a11becee9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b33de39e73972685f96c8b29ce03800d.setContent(html_6b44f78e4b711709b362634a11becee9);\\n \\n \\n\\n circle_marker_c690c0310fcf99dbc2ab194f5be68f39.bindPopup(popup_b33de39e73972685f96c8b29ce03800d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8366d4bdf00e297ba042c4d7cfa56ae = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_db0fb41357ac9d35f78724e0157984a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f72e7f295b8a76f27bbb9844dee708f7 = $(`<div id="html_f72e7f295b8a76f27bbb9844dee708f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db0fb41357ac9d35f78724e0157984a6.setContent(html_f72e7f295b8a76f27bbb9844dee708f7);\\n \\n \\n\\n circle_marker_c8366d4bdf00e297ba042c4d7cfa56ae.bindPopup(popup_db0fb41357ac9d35f78724e0157984a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b05c141555f86af5c2212604f40ae94 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_6b6f912915e960dd96387df30a95cd5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac4e435adfbd0669b3dedbb951b1dce8 = $(`<div id="html_ac4e435adfbd0669b3dedbb951b1dce8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b6f912915e960dd96387df30a95cd5e.setContent(html_ac4e435adfbd0669b3dedbb951b1dce8);\\n \\n \\n\\n circle_marker_6b05c141555f86af5c2212604f40ae94.bindPopup(popup_6b6f912915e960dd96387df30a95cd5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_1cbee3c076a0f0157078af7dfb5f8fa0 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_a307dd2050f3a4a07efcccb136cf488a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_efa2b0a2a2e1124cba458cf190799755 = $(`<div id="html_efa2b0a2a2e1124cba458cf190799755" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a307dd2050f3a4a07efcccb136cf488a.setContent(html_efa2b0a2a2e1124cba458cf190799755);\\n \\n \\n\\n circle_marker_1cbee3c076a0f0157078af7dfb5f8fa0.bindPopup(popup_a307dd2050f3a4a07efcccb136cf488a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d5275db56510f9ba99fc26aee27c513 = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_48d7524bcc3e1b373bcf93713433de83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d69f9e6ab47df150008eff73d2ddf02f = $(`<div id="html_d69f9e6ab47df150008eff73d2ddf02f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_48d7524bcc3e1b373bcf93713433de83.setContent(html_d69f9e6ab47df150008eff73d2ddf02f);\\n \\n \\n\\n circle_marker_5d5275db56510f9ba99fc26aee27c513.bindPopup(popup_48d7524bcc3e1b373bcf93713433de83)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0c344a764a58caf6a3eafeb29b9156e = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_45f11296a2d7976b2188b9a47eab2e03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b4e3917ad22228fe5e1175ab1c555c3 = $(`<div id="html_7b4e3917ad22228fe5e1175ab1c555c3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_45f11296a2d7976b2188b9a47eab2e03.setContent(html_7b4e3917ad22228fe5e1175ab1c555c3);\\n \\n \\n\\n circle_marker_b0c344a764a58caf6a3eafeb29b9156e.bindPopup(popup_45f11296a2d7976b2188b9a47eab2e03)\\n ;\\n\\n \\n \\n \\n var circle_marker_6345a59395c58fde1cdaaee70476bc15 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_1cfa685b6eae74fc9ade7b7010aa401a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b3ace9a1eea2a5baf8ce4edbb73473b = $(`<div id="html_4b3ace9a1eea2a5baf8ce4edbb73473b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1cfa685b6eae74fc9ade7b7010aa401a.setContent(html_4b3ace9a1eea2a5baf8ce4edbb73473b);\\n \\n \\n\\n circle_marker_6345a59395c58fde1cdaaee70476bc15.bindPopup(popup_1cfa685b6eae74fc9ade7b7010aa401a)\\n ;\\n\\n \\n \\n \\n var circle_marker_615a057588e0dc326e86c1fc41b93ed0 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_5d08902f124371ed7e3607981bc8e722 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a0665c988e07de37a57659b141f5432 = $(`<div id="html_0a0665c988e07de37a57659b141f5432" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d08902f124371ed7e3607981bc8e722.setContent(html_0a0665c988e07de37a57659b141f5432);\\n \\n \\n\\n circle_marker_615a057588e0dc326e86c1fc41b93ed0.bindPopup(popup_5d08902f124371ed7e3607981bc8e722)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb4aea4a73353e0ea23071174caf65be = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_b1a4036c4da33622a05736d77fe34ff8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9aea96946555c2db24d38e20addb2d6f = $(`<div id="html_9aea96946555c2db24d38e20addb2d6f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1a4036c4da33622a05736d77fe34ff8.setContent(html_9aea96946555c2db24d38e20addb2d6f);\\n \\n \\n\\n circle_marker_eb4aea4a73353e0ea23071174caf65be.bindPopup(popup_b1a4036c4da33622a05736d77fe34ff8)\\n ;\\n\\n \\n \\n \\n var circle_marker_d49245894f88746e676061bdb301116e = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_94c84ea4637ba0fc69e4d99a23b11faa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc2dfe610b597b7294a07240b39f855f = $(`<div id="html_cc2dfe610b597b7294a07240b39f855f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94c84ea4637ba0fc69e4d99a23b11faa.setContent(html_cc2dfe610b597b7294a07240b39f855f);\\n \\n \\n\\n circle_marker_d49245894f88746e676061bdb301116e.bindPopup(popup_94c84ea4637ba0fc69e4d99a23b11faa)\\n ;\\n\\n \\n \\n \\n var circle_marker_f69ea8a16bdafe6e67ae92de6d49fb7f = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_c4b03102ebfeb40c582895b31103f7d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_611fe16f168e88e76f08af83dcd4e2a1 = $(`<div id="html_611fe16f168e88e76f08af83dcd4e2a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4b03102ebfeb40c582895b31103f7d1.setContent(html_611fe16f168e88e76f08af83dcd4e2a1);\\n \\n \\n\\n circle_marker_f69ea8a16bdafe6e67ae92de6d49fb7f.bindPopup(popup_c4b03102ebfeb40c582895b31103f7d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_151cb91eccb1b3569ac87c0197fb5717 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_0dab0b6de07cc2e7c909bcd56ca28686 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_008b1b48e45260f2b6df994a8d823976 = $(`<div id="html_008b1b48e45260f2b6df994a8d823976" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0dab0b6de07cc2e7c909bcd56ca28686.setContent(html_008b1b48e45260f2b6df994a8d823976);\\n \\n \\n\\n circle_marker_151cb91eccb1b3569ac87c0197fb5717.bindPopup(popup_0dab0b6de07cc2e7c909bcd56ca28686)\\n ;\\n\\n \\n \\n \\n var circle_marker_bffe48325e71ebbe8f394dee6595b537 = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_51f1dc783ad9593160aac7cf148cdf43 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3592498a830fd1ac61248f11b3e241ca = $(`<div id="html_3592498a830fd1ac61248f11b3e241ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51f1dc783ad9593160aac7cf148cdf43.setContent(html_3592498a830fd1ac61248f11b3e241ca);\\n \\n \\n\\n circle_marker_bffe48325e71ebbe8f394dee6595b537.bindPopup(popup_51f1dc783ad9593160aac7cf148cdf43)\\n ;\\n\\n \\n \\n \\n var circle_marker_05bea16f768244f98da63a225bea3361 = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_51d99cb58a4473e506e8333e8530cebf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65252d19b97d7e0e5f7ffc5ba3d57ef9 = $(`<div id="html_65252d19b97d7e0e5f7ffc5ba3d57ef9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51d99cb58a4473e506e8333e8530cebf.setContent(html_65252d19b97d7e0e5f7ffc5ba3d57ef9);\\n \\n \\n\\n circle_marker_05bea16f768244f98da63a225bea3361.bindPopup(popup_51d99cb58a4473e506e8333e8530cebf)\\n ;\\n\\n \\n \\n \\n var circle_marker_2338f95ca5e0d003b04563213f2c89ad = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_9155fd49bf91de724379663b5954eb4a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17e04cd258d73d2ba852c414ee357abf = $(`<div id="html_17e04cd258d73d2ba852c414ee357abf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9155fd49bf91de724379663b5954eb4a.setContent(html_17e04cd258d73d2ba852c414ee357abf);\\n \\n \\n\\n circle_marker_2338f95ca5e0d003b04563213f2c89ad.bindPopup(popup_9155fd49bf91de724379663b5954eb4a)\\n ;\\n\\n \\n \\n \\n var circle_marker_d4d8c3aad4491860606d2e64b66ac187 = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_f6a31cf9a05f46ac45600737e2a16d12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_761d989e96484bbfa89ca0fb1226786b = $(`<div id="html_761d989e96484bbfa89ca0fb1226786b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6a31cf9a05f46ac45600737e2a16d12.setContent(html_761d989e96484bbfa89ca0fb1226786b);\\n \\n \\n\\n circle_marker_d4d8c3aad4491860606d2e64b66ac187.bindPopup(popup_f6a31cf9a05f46ac45600737e2a16d12)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a5038fd9a6396fb6d20925d988a2993 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_3e5770da8313d7bc759e0e34ce83b17f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8aec3a67eb8c885951f947cc57d3343b = $(`<div id="html_8aec3a67eb8c885951f947cc57d3343b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e5770da8313d7bc759e0e34ce83b17f.setContent(html_8aec3a67eb8c885951f947cc57d3343b);\\n \\n \\n\\n circle_marker_0a5038fd9a6396fb6d20925d988a2993.bindPopup(popup_3e5770da8313d7bc759e0e34ce83b17f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6207c37bedc9a7b150a8045d78408e56 = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_d569e1dfb4917cc21ca40e2020f65034 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ff205badc566ed24c6e9fa177b9093d = $(`<div id="html_7ff205badc566ed24c6e9fa177b9093d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d569e1dfb4917cc21ca40e2020f65034.setContent(html_7ff205badc566ed24c6e9fa177b9093d);\\n \\n \\n\\n circle_marker_6207c37bedc9a7b150a8045d78408e56.bindPopup(popup_d569e1dfb4917cc21ca40e2020f65034)\\n ;\\n\\n \\n \\n \\n var circle_marker_38ffb5bbfd2b4f422a6345b3562aeb6e = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_b82e386f715b60f098365dfbddcd7e90 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d54e51544a97678a734628b5e113ad25 = $(`<div id="html_d54e51544a97678a734628b5e113ad25" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b82e386f715b60f098365dfbddcd7e90.setContent(html_d54e51544a97678a734628b5e113ad25);\\n \\n \\n\\n circle_marker_38ffb5bbfd2b4f422a6345b3562aeb6e.bindPopup(popup_b82e386f715b60f098365dfbddcd7e90)\\n ;\\n\\n \\n \\n \\n var circle_marker_badaffe34705b8e27d36e0c61d302246 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_3f11aead112465daed2cba0e19d1ac69 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d38729fe8b21840e78575bb83ffaf62f = $(`<div id="html_d38729fe8b21840e78575bb83ffaf62f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3f11aead112465daed2cba0e19d1ac69.setContent(html_d38729fe8b21840e78575bb83ffaf62f);\\n \\n \\n\\n circle_marker_badaffe34705b8e27d36e0c61d302246.bindPopup(popup_3f11aead112465daed2cba0e19d1ac69)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb53e890e72cb8108fcbc597c43bfae1 = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_3b498450066e2ca42702d2439a11e582 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74510cfac25b794e376e5f7a57d6cede = $(`<div id="html_74510cfac25b794e376e5f7a57d6cede" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3b498450066e2ca42702d2439a11e582.setContent(html_74510cfac25b794e376e5f7a57d6cede);\\n \\n \\n\\n circle_marker_cb53e890e72cb8108fcbc597c43bfae1.bindPopup(popup_3b498450066e2ca42702d2439a11e582)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ee2cd64985c8bca9d097da1786acf3b = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_f0cb1e8a214b0ae628d257b31e251aa9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc97211cff0851b9ba9b1daad36257b6 = $(`<div id="html_dc97211cff0851b9ba9b1daad36257b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0cb1e8a214b0ae628d257b31e251aa9.setContent(html_dc97211cff0851b9ba9b1daad36257b6);\\n \\n \\n\\n circle_marker_9ee2cd64985c8bca9d097da1786acf3b.bindPopup(popup_f0cb1e8a214b0ae628d257b31e251aa9)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d82ecd05c1982fbeb77220403328b56 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_73fb0533ff3e696cdbb3f103ba4959c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3976d8a881fb6fe45ee6199da2fd67f = $(`<div id="html_a3976d8a881fb6fe45ee6199da2fd67f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73fb0533ff3e696cdbb3f103ba4959c6.setContent(html_a3976d8a881fb6fe45ee6199da2fd67f);\\n \\n \\n\\n circle_marker_7d82ecd05c1982fbeb77220403328b56.bindPopup(popup_73fb0533ff3e696cdbb3f103ba4959c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_71625e1b67bb25d38831b9957a3f6928 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_b801e3dd88d54d187f1ab3edea71a420 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_386e222fa9c9b0b966944052adc920d5 = $(`<div id="html_386e222fa9c9b0b966944052adc920d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b801e3dd88d54d187f1ab3edea71a420.setContent(html_386e222fa9c9b0b966944052adc920d5);\\n \\n \\n\\n circle_marker_71625e1b67bb25d38831b9957a3f6928.bindPopup(popup_b801e3dd88d54d187f1ab3edea71a420)\\n ;\\n\\n \\n \\n \\n var circle_marker_639bd3036a80d747b01712a0b0b04241 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_e0b98f778d3dc38d1b2aa80b5f75d47d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b62eddd4cf08373f24447d353ba9596 = $(`<div id="html_5b62eddd4cf08373f24447d353ba9596" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0b98f778d3dc38d1b2aa80b5f75d47d.setContent(html_5b62eddd4cf08373f24447d353ba9596);\\n \\n \\n\\n circle_marker_639bd3036a80d747b01712a0b0b04241.bindPopup(popup_e0b98f778d3dc38d1b2aa80b5f75d47d)\\n ;\\n\\n \\n \\n \\n var circle_marker_47c5bdfb74f30774baa47a1353666ac7 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_12043878e63bead674969c9f2e9bb167 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f8916ed0ecf55a65521e1a4056429ea = $(`<div id="html_0f8916ed0ecf55a65521e1a4056429ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12043878e63bead674969c9f2e9bb167.setContent(html_0f8916ed0ecf55a65521e1a4056429ea);\\n \\n \\n\\n circle_marker_47c5bdfb74f30774baa47a1353666ac7.bindPopup(popup_12043878e63bead674969c9f2e9bb167)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c1a8dfd77a67ceb4984f65702d36019 = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_a0301d86ce7c2be52096d213efb6f27d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b11d0c0f66e778d5d49b4c9e70df8e1d = $(`<div id="html_b11d0c0f66e778d5d49b4c9e70df8e1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0301d86ce7c2be52096d213efb6f27d.setContent(html_b11d0c0f66e778d5d49b4c9e70df8e1d);\\n \\n \\n\\n circle_marker_6c1a8dfd77a67ceb4984f65702d36019.bindPopup(popup_a0301d86ce7c2be52096d213efb6f27d)\\n ;\\n\\n \\n \\n \\n var circle_marker_20d226846ae14359b79c8afaafd82ee2 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_7dc7b29cdc44146d60e7001184dda7b0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad28754c31c6e6befb7c90bbfb20848b = $(`<div id="html_ad28754c31c6e6befb7c90bbfb20848b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7dc7b29cdc44146d60e7001184dda7b0.setContent(html_ad28754c31c6e6befb7c90bbfb20848b);\\n \\n \\n\\n circle_marker_20d226846ae14359b79c8afaafd82ee2.bindPopup(popup_7dc7b29cdc44146d60e7001184dda7b0)\\n ;\\n\\n \\n \\n \\n var circle_marker_5964cb9130973bbc9b79769961b5aa90 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_694faed7ea8da2ee028b33323465ff05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6d1a3e40a2ba7c0bac4c6e3d64d6e8c = $(`<div id="html_b6d1a3e40a2ba7c0bac4c6e3d64d6e8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_694faed7ea8da2ee028b33323465ff05.setContent(html_b6d1a3e40a2ba7c0bac4c6e3d64d6e8c);\\n \\n \\n\\n circle_marker_5964cb9130973bbc9b79769961b5aa90.bindPopup(popup_694faed7ea8da2ee028b33323465ff05)\\n ;\\n\\n \\n \\n \\n var circle_marker_10624ea1d8859e53c4695e282533cfd9 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_fa61e093b603abf7a0c33b60f8ac18da = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aad570617f052d6a884f9f8691d01f46 = $(`<div id="html_aad570617f052d6a884f9f8691d01f46" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa61e093b603abf7a0c33b60f8ac18da.setContent(html_aad570617f052d6a884f9f8691d01f46);\\n \\n \\n\\n circle_marker_10624ea1d8859e53c4695e282533cfd9.bindPopup(popup_fa61e093b603abf7a0c33b60f8ac18da)\\n ;\\n\\n \\n \\n \\n var circle_marker_0131e832679dd35c43fa9b4a86188a16 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_3510d8c592cab2d4d9f947ec1deaecb6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6909ca68d492ed4eebea6b5fe848a58 = $(`<div id="html_f6909ca68d492ed4eebea6b5fe848a58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3510d8c592cab2d4d9f947ec1deaecb6.setContent(html_f6909ca68d492ed4eebea6b5fe848a58);\\n \\n \\n\\n circle_marker_0131e832679dd35c43fa9b4a86188a16.bindPopup(popup_3510d8c592cab2d4d9f947ec1deaecb6)\\n ;\\n\\n \\n \\n \\n var circle_marker_fbdff4f38b721f26fbb8aaf97b472246 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_71c11555b5d0e4615bf906907516696b);\\n \\n \\n var popup_65162390625ef48dc776593ff3f82813 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3cf1b546045348e0d6f609a37507d139 = $(`<div id="html_3cf1b546045348e0d6f609a37507d139" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65162390625ef48dc776593ff3f82813.setContent(html_3cf1b546045348e0d6f609a37507d139);\\n \\n \\n\\n circle_marker_fbdff4f38b721f26fbb8aaf97b472246.bindPopup(popup_65162390625ef48dc776593ff3f82813)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Bittersweet, Asiatic\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_7bae73a41af52185a692ad6190936f2d {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_7bae73a41af52185a692ad6190936f2d" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_7bae73a41af52185a692ad6190936f2d = L.map(\\n "map_7bae73a41af52185a692ad6190936f2d",\\n {\\n center: [42.72370220512386, -73.22674323325023],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_28fb6ff92f12cbcbe9f1d6d29b0cac38 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_7bae73a41af52185a692ad6190936f2d);\\n \\n \\n var circle_marker_d3f7988dad9330190c260169157a7ce0 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_7bae73a41af52185a692ad6190936f2d);\\n \\n \\n var popup_0e938907e6eb065194f2ae77f3a39b6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7ca85d76aafce24f75172b0e604c323 = $(`<div id="html_e7ca85d76aafce24f75172b0e604c323" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e938907e6eb065194f2ae77f3a39b6a.setContent(html_e7ca85d76aafce24f75172b0e604c323);\\n \\n \\n\\n circle_marker_d3f7988dad9330190c260169157a7ce0.bindPopup(popup_0e938907e6eb065194f2ae77f3a39b6a)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Bittersweet, climbing\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_321bccef63a8de6487d5974d37b979b1 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_321bccef63a8de6487d5974d37b979b1" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_321bccef63a8de6487d5974d37b979b1 = L.map(\\n "map_321bccef63a8de6487d5974d37b979b1",\\n {\\n center: [42.727316250822454, -73.2242814962688],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_302ab29247b762e88fe15be2fa442cc9 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_321bccef63a8de6487d5974d37b979b1);\\n \\n \\n var circle_marker_04f0c4246d99fcbdfe35f78c74a10af1 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_321bccef63a8de6487d5974d37b979b1);\\n \\n \\n var popup_4f47a7425918456c8eaad7964d7d27cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_12e7f3dfb27e3f05d4a01692caf16418 = $(`<div id="html_12e7f3dfb27e3f05d4a01692caf16418" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f47a7425918456c8eaad7964d7d27cb.setContent(html_12e7f3dfb27e3f05d4a01692caf16418);\\n \\n \\n\\n circle_marker_04f0c4246d99fcbdfe35f78c74a10af1.bindPopup(popup_4f47a7425918456c8eaad7964d7d27cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_2cf45a33343258e3404d9c4e0bf18bd0 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_321bccef63a8de6487d5974d37b979b1);\\n \\n \\n var popup_a7f4171a19c03acb66565bbfb56b1735 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f05fe83faa3a44342f0970cdeac529a = $(`<div id="html_4f05fe83faa3a44342f0970cdeac529a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7f4171a19c03acb66565bbfb56b1735.setContent(html_4f05fe83faa3a44342f0970cdeac529a);\\n \\n \\n\\n circle_marker_2cf45a33343258e3404d9c4e0bf18bd0.bindPopup(popup_a7f4171a19c03acb66565bbfb56b1735)\\n ;\\n\\n \\n \\n \\n var circle_marker_94627bed9cfa6f7e2c5cedf8191ad1b2 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_321bccef63a8de6487d5974d37b979b1);\\n \\n \\n var popup_82a4c68da9cb742420b83fddbe0f9a46 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_afb95880a37737dd119c95854abfcef5 = $(`<div id="html_afb95880a37737dd119c95854abfcef5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82a4c68da9cb742420b83fddbe0f9a46.setContent(html_afb95880a37737dd119c95854abfcef5);\\n \\n \\n\\n circle_marker_94627bed9cfa6f7e2c5cedf8191ad1b2.bindPopup(popup_82a4c68da9cb742420b83fddbe0f9a46)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Box-elder": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_090de3475fcc747104e35363e6ae450c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_090de3475fcc747104e35363e6ae450c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_090de3475fcc747104e35363e6ae450c = L.map(\\n "map_090de3475fcc747104e35363e6ae450c",\\n {\\n center: [42.7318329610091, -73.22243443162179],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_4cffe173531071986a7e442980d9121d = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_090de3475fcc747104e35363e6ae450c);\\n \\n \\n var circle_marker_4d37b1e500828482f067bd1310af84a0 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_090de3475fcc747104e35363e6ae450c);\\n \\n \\n var popup_9b7ce6c47a2a43a7a0ecd1f7d58adb9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8a758a21d36f3177d6352772ac72b5d = $(`<div id="html_a8a758a21d36f3177d6352772ac72b5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b7ce6c47a2a43a7a0ecd1f7d58adb9c.setContent(html_a8a758a21d36f3177d6352772ac72b5d);\\n \\n \\n\\n circle_marker_4d37b1e500828482f067bd1310af84a0.bindPopup(popup_9b7ce6c47a2a43a7a0ecd1f7d58adb9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_03e08aa9ce257bb768f65bd61e8d9889 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_090de3475fcc747104e35363e6ae450c);\\n \\n \\n var popup_2d65abca5e7cb89dd576b35a225d39da = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f24e6cd592c6063be8782c195ba1df4 = $(`<div id="html_6f24e6cd592c6063be8782c195ba1df4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d65abca5e7cb89dd576b35a225d39da.setContent(html_6f24e6cd592c6063be8782c195ba1df4);\\n \\n \\n\\n circle_marker_03e08aa9ce257bb768f65bd61e8d9889.bindPopup(popup_2d65abca5e7cb89dd576b35a225d39da)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ad285f0f4d9d655ee379e3b25594f72 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_090de3475fcc747104e35363e6ae450c);\\n \\n \\n var popup_62afe47bfc81c6a97af7ea9b945b0621 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4f661a1b64baa24154fb7c69c85e27a = $(`<div id="html_d4f661a1b64baa24154fb7c69c85e27a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62afe47bfc81c6a97af7ea9b945b0621.setContent(html_d4f661a1b64baa24154fb7c69c85e27a);\\n \\n \\n\\n circle_marker_2ad285f0f4d9d655ee379e3b25594f72.bindPopup(popup_62afe47bfc81c6a97af7ea9b945b0621)\\n ;\\n\\n \\n \\n \\n var circle_marker_91f3ce4f8af3ff9aa022b09eeb1cfa64 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_090de3475fcc747104e35363e6ae450c);\\n \\n \\n var popup_68d4a611d60a27315be227785784e8d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d86280a2bc664478b670da3f95c0c03 = $(`<div id="html_6d86280a2bc664478b670da3f95c0c03" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68d4a611d60a27315be227785784e8d9.setContent(html_6d86280a2bc664478b670da3f95c0c03);\\n \\n \\n\\n circle_marker_91f3ce4f8af3ff9aa022b09eeb1cfa64.bindPopup(popup_68d4a611d60a27315be227785784e8d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_1119d7f433718f2b3977265de4309bbe = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_090de3475fcc747104e35363e6ae450c);\\n \\n \\n var popup_2107ca096117337e4817fed6fbc708e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ea39ff735a67f4bc010cc29ce9dd667 = $(`<div id="html_5ea39ff735a67f4bc010cc29ce9dd667" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2107ca096117337e4817fed6fbc708e7.setContent(html_5ea39ff735a67f4bc010cc29ce9dd667);\\n \\n \\n\\n circle_marker_1119d7f433718f2b3977265de4309bbe.bindPopup(popup_2107ca096117337e4817fed6fbc708e7)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Buckthorn, alder-leaved\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_1a74b118823f2dc94b8adbaad5abc0fb {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_1a74b118823f2dc94b8adbaad5abc0fb" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_1a74b118823f2dc94b8adbaad5abc0fb = L.map(\\n "map_1a74b118823f2dc94b8adbaad5abc0fb",\\n {\\n center: [42.72912517451705, -73.23227534216484],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_b8202c2ba48e88d67441cecc77ce7764 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_1a74b118823f2dc94b8adbaad5abc0fb);\\n \\n \\n var circle_marker_f37ed486fbc625ac3c2a3b94e3f2b684 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_1a74b118823f2dc94b8adbaad5abc0fb);\\n \\n \\n var popup_8e7c7dd7889c9ee8efa2b267de1a0b64 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ad986b32ce38cc10b2bf06d765aae29 = $(`<div id="html_0ad986b32ce38cc10b2bf06d765aae29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e7c7dd7889c9ee8efa2b267de1a0b64.setContent(html_0ad986b32ce38cc10b2bf06d765aae29);\\n \\n \\n\\n circle_marker_f37ed486fbc625ac3c2a3b94e3f2b684.bindPopup(popup_8e7c7dd7889c9ee8efa2b267de1a0b64)\\n ;\\n\\n \\n \\n \\n var circle_marker_a89192decc22dbc68596b5a79b06389b = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_1a74b118823f2dc94b8adbaad5abc0fb);\\n \\n \\n var popup_9f5112361486abcb2f3be5bac9b25a6d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edd584d3676f1a69cee239ab63878e41 = $(`<div id="html_edd584d3676f1a69cee239ab63878e41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f5112361486abcb2f3be5bac9b25a6d.setContent(html_edd584d3676f1a69cee239ab63878e41);\\n \\n \\n\\n circle_marker_a89192decc22dbc68596b5a79b06389b.bindPopup(popup_9f5112361486abcb2f3be5bac9b25a6d)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Buckthorn, common\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_15bfcf45423a71d89ed67dc7a8b5a09f {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_15bfcf45423a71d89ed67dc7a8b5a09f" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_15bfcf45423a71d89ed67dc7a8b5a09f = L.map(\\n "map_15bfcf45423a71d89ed67dc7a8b5a09f",\\n {\\n center: [42.73183738520744, -73.2310445098941],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_706c852d1533db9b3acabdebc57275bb = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var circle_marker_dcef12d12023eb42c240bc4844c0d11f = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_b778de620d313fcedc1c7702324ae415 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9619dd681b78f3f681c42f84664049d1 = $(`<div id="html_9619dd681b78f3f681c42f84664049d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b778de620d313fcedc1c7702324ae415.setContent(html_9619dd681b78f3f681c42f84664049d1);\\n \\n \\n\\n circle_marker_dcef12d12023eb42c240bc4844c0d11f.bindPopup(popup_b778de620d313fcedc1c7702324ae415)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e318f2363e74e0bcb0e9b27a1868466 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.282549314314218, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_056f32d7ef418aeeaa716c53e5c8d768 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_023987f0428978f96e1cf4f038392690 = $(`<div id="html_023987f0428978f96e1cf4f038392690" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_056f32d7ef418aeeaa716c53e5c8d768.setContent(html_023987f0428978f96e1cf4f038392690);\\n \\n \\n\\n circle_marker_5e318f2363e74e0bcb0e9b27a1868466.bindPopup(popup_056f32d7ef418aeeaa716c53e5c8d768)\\n ;\\n\\n \\n \\n \\n var circle_marker_35a64920a7108080cc8156ac24875bdd = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_e280535111ffbb748b248def632c39f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e598afdf6a7ed8c1fb52cbfe94b937bf = $(`<div id="html_e598afdf6a7ed8c1fb52cbfe94b937bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e280535111ffbb748b248def632c39f0.setContent(html_e598afdf6a7ed8c1fb52cbfe94b937bf);\\n \\n \\n\\n circle_marker_35a64920a7108080cc8156ac24875bdd.bindPopup(popup_e280535111ffbb748b248def632c39f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e464464a6ff9ca00a9fd05dce5034ef = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_2ad9ccfc2c0364eb4920f8b40c8c2e1c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3fcee1dc6b7df233310dc5e4c501c977 = $(`<div id="html_3fcee1dc6b7df233310dc5e4c501c977" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ad9ccfc2c0364eb4920f8b40c8c2e1c.setContent(html_3fcee1dc6b7df233310dc5e4c501c977);\\n \\n \\n\\n circle_marker_7e464464a6ff9ca00a9fd05dce5034ef.bindPopup(popup_2ad9ccfc2c0364eb4920f8b40c8c2e1c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2cb0914aa02542a276468808e1a64c67 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.050259243299904, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_10f21565daf99633cd9bf7c791253872 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7449919c280dc8002fe801d1617a6996 = $(`<div id="html_7449919c280dc8002fe801d1617a6996" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10f21565daf99633cd9bf7c791253872.setContent(html_7449919c280dc8002fe801d1617a6996);\\n \\n \\n\\n circle_marker_2cb0914aa02542a276468808e1a64c67.bindPopup(popup_10f21565daf99633cd9bf7c791253872)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f66b26c68cf6678934102783a654fdf = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_5122191c24592db4f39e42b1aa28aa5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_efdfdc21bdba71c041a8553dcf2a82fd = $(`<div id="html_efdfdc21bdba71c041a8553dcf2a82fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5122191c24592db4f39e42b1aa28aa5e.setContent(html_efdfdc21bdba71c041a8553dcf2a82fd);\\n \\n \\n\\n circle_marker_5f66b26c68cf6678934102783a654fdf.bindPopup(popup_5122191c24592db4f39e42b1aa28aa5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb4db016201e77d89e7faa34d62de2d0 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_952a7bd4c70bf3b8988d0c7d49b97533 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e2cdb357df5037f7777afd3a5a70b54 = $(`<div id="html_1e2cdb357df5037f7777afd3a5a70b54" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_952a7bd4c70bf3b8988d0c7d49b97533.setContent(html_1e2cdb357df5037f7777afd3a5a70b54);\\n \\n \\n\\n circle_marker_cb4db016201e77d89e7faa34d62de2d0.bindPopup(popup_952a7bd4c70bf3b8988d0c7d49b97533)\\n ;\\n\\n \\n \\n \\n var circle_marker_7279fd668021516ff4ee331a7cd26233 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.753945931439391, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_580907bd0f7335e611d3bec9d5015442 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ee50121cb27a3d69042ddead080cf3d = $(`<div id="html_3ee50121cb27a3d69042ddead080cf3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_580907bd0f7335e611d3bec9d5015442.setContent(html_3ee50121cb27a3d69042ddead080cf3d);\\n \\n \\n\\n circle_marker_7279fd668021516ff4ee331a7cd26233.bindPopup(popup_580907bd0f7335e611d3bec9d5015442)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ca42cf05ab5ca0b89548016b9f8102b = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.950743503369154, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_e1e656a3cc2ab031d8cc6ea38787f67b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d414006995de44399976060ebddd0cc4 = $(`<div id="html_d414006995de44399976060ebddd0cc4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1e656a3cc2ab031d8cc6ea38787f67b.setContent(html_d414006995de44399976060ebddd0cc4);\\n \\n \\n\\n circle_marker_7ca42cf05ab5ca0b89548016b9f8102b.bindPopup(popup_e1e656a3cc2ab031d8cc6ea38787f67b)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8c11ab893955107ecd3209f7a2ed6e8 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.978639737521917, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_8bb89aef90158f46041ac126222a379c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a590da95523ba6b10b467b753ba08df3 = $(`<div id="html_a590da95523ba6b10b467b753ba08df3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8bb89aef90158f46041ac126222a379c.setContent(html_a590da95523ba6b10b467b753ba08df3);\\n \\n \\n\\n circle_marker_a8c11ab893955107ecd3209f7a2ed6e8.bindPopup(popup_8bb89aef90158f46041ac126222a379c)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e75baa1faf00d6f52a6b9c99f887663 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.725898905580716, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_06eadc6de9f910beabe39254605943b0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09e6ba2558f7ff779775881a6bd598be = $(`<div id="html_09e6ba2558f7ff779775881a6bd598be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06eadc6de9f910beabe39254605943b0.setContent(html_09e6ba2558f7ff779775881a6bd598be);\\n \\n \\n\\n circle_marker_8e75baa1faf00d6f52a6b9c99f887663.bindPopup(popup_06eadc6de9f910beabe39254605943b0)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e6afe17c84e8b3157538440c6c55675 = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_f149e04de429ec267b6ee386542b4ad7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb11dfea16dca6223bbddc59eac65d44 = $(`<div id="html_fb11dfea16dca6223bbddc59eac65d44" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f149e04de429ec267b6ee386542b4ad7.setContent(html_fb11dfea16dca6223bbddc59eac65d44);\\n \\n \\n\\n circle_marker_9e6afe17c84e8b3157538440c6c55675.bindPopup(popup_f149e04de429ec267b6ee386542b4ad7)\\n ;\\n\\n \\n \\n \\n var circle_marker_2098966d825b5f139faa5c0f7c53c026 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_0f60395009b946038bee9b62d42d8660 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_684ef338bad8d69147a4696255fc0465 = $(`<div id="html_684ef338bad8d69147a4696255fc0465" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f60395009b946038bee9b62d42d8660.setContent(html_684ef338bad8d69147a4696255fc0465);\\n \\n \\n\\n circle_marker_2098966d825b5f139faa5c0f7c53c026.bindPopup(popup_0f60395009b946038bee9b62d42d8660)\\n ;\\n\\n \\n \\n \\n var circle_marker_88593dc3a2ce21e164638bb6055d88ca = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_6e5be4a893d5f425bdd0b32ea1c031e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b5a4465a087295fe7e5eff7f2ff6a20 = $(`<div id="html_3b5a4465a087295fe7e5eff7f2ff6a20" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e5be4a893d5f425bdd0b32ea1c031e1.setContent(html_3b5a4465a087295fe7e5eff7f2ff6a20);\\n \\n \\n\\n circle_marker_88593dc3a2ce21e164638bb6055d88ca.bindPopup(popup_6e5be4a893d5f425bdd0b32ea1c031e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_25d6e2bf7928d74ae36bf3ef6ca6245e = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_e205d8f52feb8c577ff335202a68b120 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b621ab8a88ad9e9c59d62db0ed05ce41 = $(`<div id="html_b621ab8a88ad9e9c59d62db0ed05ce41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e205d8f52feb8c577ff335202a68b120.setContent(html_b621ab8a88ad9e9c59d62db0ed05ce41);\\n \\n \\n\\n circle_marker_25d6e2bf7928d74ae36bf3ef6ca6245e.bindPopup(popup_e205d8f52feb8c577ff335202a68b120)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c72a0a1ed1538125de009ff55a0a2bc = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_d96b9db13a076e4d646c01d5d27cf1a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b51dc33e71aba17200b7f056648ed15 = $(`<div id="html_7b51dc33e71aba17200b7f056648ed15" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d96b9db13a076e4d646c01d5d27cf1a1.setContent(html_7b51dc33e71aba17200b7f056648ed15);\\n \\n \\n\\n circle_marker_0c72a0a1ed1538125de009ff55a0a2bc.bindPopup(popup_d96b9db13a076e4d646c01d5d27cf1a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_4465629c367b98f1955e6ce156ebdd9c = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.61809077155419, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_7706336d13dfd149a64873c4697d6f7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_35f01927bf740fdf55b2210823c8b6a3 = $(`<div id="html_35f01927bf740fdf55b2210823c8b6a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7706336d13dfd149a64873c4697d6f7b.setContent(html_35f01927bf740fdf55b2210823c8b6a3);\\n \\n \\n\\n circle_marker_4465629c367b98f1955e6ce156ebdd9c.bindPopup(popup_7706336d13dfd149a64873c4697d6f7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3bd565775e6f13dbab4d3a3ab211db5a = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.886025119029199, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_3232f8ef30928e12c012591cd973a6b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e1ac6496dc783b16fbefb3a878bddaf = $(`<div id="html_8e1ac6496dc783b16fbefb3a878bddaf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3232f8ef30928e12c012591cd973a6b5.setContent(html_8e1ac6496dc783b16fbefb3a878bddaf);\\n \\n \\n\\n circle_marker_3bd565775e6f13dbab4d3a3ab211db5a.bindPopup(popup_3232f8ef30928e12c012591cd973a6b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_ed244b91db03da93f231829e173c226c = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_06531a0b7bd9c9911da9061ef960358e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_948eb0dd003869ec4a688027385e34ab = $(`<div id="html_948eb0dd003869ec4a688027385e34ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06531a0b7bd9c9911da9061ef960358e.setContent(html_948eb0dd003869ec4a688027385e34ab);\\n \\n \\n\\n circle_marker_ed244b91db03da93f231829e173c226c.bindPopup(popup_06531a0b7bd9c9911da9061ef960358e)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a30696d826e001d34acfc74c37173cc = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_a84ffcb974851e9fe948d5a546ef1382 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33c365db86a216eb2d332d6d38687da8 = $(`<div id="html_33c365db86a216eb2d332d6d38687da8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a84ffcb974851e9fe948d5a546ef1382.setContent(html_33c365db86a216eb2d332d6d38687da8);\\n \\n \\n\\n circle_marker_5a30696d826e001d34acfc74c37173cc.bindPopup(popup_a84ffcb974851e9fe948d5a546ef1382)\\n ;\\n\\n \\n \\n \\n var circle_marker_2881c44c38d7c9a9ce2309a20a964476 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_8e89943473c818b22b91e8e55c88093a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43d14ea908f483b020dfddb1298be0f4 = $(`<div id="html_43d14ea908f483b020dfddb1298be0f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e89943473c818b22b91e8e55c88093a.setContent(html_43d14ea908f483b020dfddb1298be0f4);\\n \\n \\n\\n circle_marker_2881c44c38d7c9a9ce2309a20a964476.bindPopup(popup_8e89943473c818b22b91e8e55c88093a)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb1b27d0bd7908a0e15859657e1598c4 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_fe42f48d1eb1f79ce43a46898576242b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1d01b0b874597155f5cb4f1731388ce7 = $(`<div id="html_1d01b0b874597155f5cb4f1731388ce7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe42f48d1eb1f79ce43a46898576242b.setContent(html_1d01b0b874597155f5cb4f1731388ce7);\\n \\n \\n\\n circle_marker_cb1b27d0bd7908a0e15859657e1598c4.bindPopup(popup_fe42f48d1eb1f79ce43a46898576242b)\\n ;\\n\\n \\n \\n \\n var circle_marker_cfe2057e0c1222fdc4ea04be918d8ae8 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_92833f2b95ca2671288f628156083860 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99dfcdd1830e3f042aa2ac2df2a11e23 = $(`<div id="html_99dfcdd1830e3f042aa2ac2df2a11e23" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_92833f2b95ca2671288f628156083860.setContent(html_99dfcdd1830e3f042aa2ac2df2a11e23);\\n \\n \\n\\n circle_marker_cfe2057e0c1222fdc4ea04be918d8ae8.bindPopup(popup_92833f2b95ca2671288f628156083860)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f353200cbafeaf053c671be382c1f9e = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_ce5db4584c69b955a78894ff2fceaae8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1842e2660fc721ddfd7fa2a6593c5ddd = $(`<div id="html_1842e2660fc721ddfd7fa2a6593c5ddd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce5db4584c69b955a78894ff2fceaae8.setContent(html_1842e2660fc721ddfd7fa2a6593c5ddd);\\n \\n \\n\\n circle_marker_6f353200cbafeaf053c671be382c1f9e.bindPopup(popup_ce5db4584c69b955a78894ff2fceaae8)\\n ;\\n\\n \\n \\n \\n var circle_marker_3461ab3a8ac973bfb4178dc5690c2778 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_35d70654f4e45ec2aef25062b6ed4556 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_08982a9124bc53436ea2e7b68cef920a = $(`<div id="html_08982a9124bc53436ea2e7b68cef920a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35d70654f4e45ec2aef25062b6ed4556.setContent(html_08982a9124bc53436ea2e7b68cef920a);\\n \\n \\n\\n circle_marker_3461ab3a8ac973bfb4178dc5690c2778.bindPopup(popup_35d70654f4e45ec2aef25062b6ed4556)\\n ;\\n\\n \\n \\n \\n var circle_marker_083fa8abbe310a9df077ffae9addce14 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_f4de6d99b2e4f138827df73b58506f98 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e13aaaf90144ede41370885e06afbfe = $(`<div id="html_0e13aaaf90144ede41370885e06afbfe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4de6d99b2e4f138827df73b58506f98.setContent(html_0e13aaaf90144ede41370885e06afbfe);\\n \\n \\n\\n circle_marker_083fa8abbe310a9df077ffae9addce14.bindPopup(popup_f4de6d99b2e4f138827df73b58506f98)\\n ;\\n\\n \\n \\n \\n var circle_marker_c75be3d01088877fbfb0ebdde95aafb1 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_94d2952cd4184596f37066b401aa88e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad507b64632d936b6ed444780c76d53f = $(`<div id="html_ad507b64632d936b6ed444780c76d53f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94d2952cd4184596f37066b401aa88e7.setContent(html_ad507b64632d936b6ed444780c76d53f);\\n \\n \\n\\n circle_marker_c75be3d01088877fbfb0ebdde95aafb1.bindPopup(popup_94d2952cd4184596f37066b401aa88e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a1a8648c65be8a498a300f5afdcc9b1 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_cfe31219e6044890b2e15a807a084159 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_157886df48ac34663c2e698b6b695144 = $(`<div id="html_157886df48ac34663c2e698b6b695144" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfe31219e6044890b2e15a807a084159.setContent(html_157886df48ac34663c2e698b6b695144);\\n \\n \\n\\n circle_marker_2a1a8648c65be8a498a300f5afdcc9b1.bindPopup(popup_cfe31219e6044890b2e15a807a084159)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ccfdb577f25a63ae0e62d0c20c24964 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_432cd4706e758a7c1bc8e30313a3253e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0360599081121c60cea58e2265ad032e = $(`<div id="html_0360599081121c60cea58e2265ad032e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_432cd4706e758a7c1bc8e30313a3253e.setContent(html_0360599081121c60cea58e2265ad032e);\\n \\n \\n\\n circle_marker_6ccfdb577f25a63ae0e62d0c20c24964.bindPopup(popup_432cd4706e758a7c1bc8e30313a3253e)\\n ;\\n\\n \\n \\n \\n var circle_marker_41eebb2448032323d9ddeb4dd4d2fbfa = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_cc138398badfb21896d162ab7947e8ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a108750588c916eeea713c46e1a5f1e = $(`<div id="html_6a108750588c916eeea713c46e1a5f1e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc138398badfb21896d162ab7947e8ae.setContent(html_6a108750588c916eeea713c46e1a5f1e);\\n \\n \\n\\n circle_marker_41eebb2448032323d9ddeb4dd4d2fbfa.bindPopup(popup_cc138398badfb21896d162ab7947e8ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_9591ff2cacd43e7ab70b3149a9649e98 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.180387232371033, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_9361e06258e6fcf52fbbe7d4a5bdd990 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ccc6e26a503877223048bccc84e4e9d = $(`<div id="html_9ccc6e26a503877223048bccc84e4e9d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9361e06258e6fcf52fbbe7d4a5bdd990.setContent(html_9ccc6e26a503877223048bccc84e4e9d);\\n \\n \\n\\n circle_marker_9591ff2cacd43e7ab70b3149a9649e98.bindPopup(popup_9361e06258e6fcf52fbbe7d4a5bdd990)\\n ;\\n\\n \\n \\n \\n var circle_marker_0afef382f2202fb8e88f8d79d0fbf9b4 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_792079deb397de5796aa9f7363f8eb1f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8299f3b906e8570e2cad8163242d41bb = $(`<div id="html_8299f3b906e8570e2cad8163242d41bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_792079deb397de5796aa9f7363f8eb1f.setContent(html_8299f3b906e8570e2cad8163242d41bb);\\n \\n \\n\\n circle_marker_0afef382f2202fb8e88f8d79d0fbf9b4.bindPopup(popup_792079deb397de5796aa9f7363f8eb1f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f7bcfa2e9548a8573102781b127f41c = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_c0137dd52e851fdd3830b2dceced8eb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19eb7c9be92c3be7417bd08162efb52e = $(`<div id="html_19eb7c9be92c3be7417bd08162efb52e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0137dd52e851fdd3830b2dceced8eb8.setContent(html_19eb7c9be92c3be7417bd08162efb52e);\\n \\n \\n\\n circle_marker_6f7bcfa2e9548a8573102781b127f41c.bindPopup(popup_c0137dd52e851fdd3830b2dceced8eb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6d9fa7cd02d04b01d84cbcdf4b31352 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_102b4d5de0c0ff5d8e9bbda6ef873cd7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1dd35afa64ca6af6cb18bc98ec89709b = $(`<div id="html_1dd35afa64ca6af6cb18bc98ec89709b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_102b4d5de0c0ff5d8e9bbda6ef873cd7.setContent(html_1dd35afa64ca6af6cb18bc98ec89709b);\\n \\n \\n\\n circle_marker_a6d9fa7cd02d04b01d84cbcdf4b31352.bindPopup(popup_102b4d5de0c0ff5d8e9bbda6ef873cd7)\\n ;\\n\\n \\n \\n \\n var circle_marker_16640c547887e571ef5d0e37616cbec0 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_d0214a7a7cfab52b9d00c68df991cc20 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dff2c6df9f8468697f55b70f6b259f40 = $(`<div id="html_dff2c6df9f8468697f55b70f6b259f40" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0214a7a7cfab52b9d00c68df991cc20.setContent(html_dff2c6df9f8468697f55b70f6b259f40);\\n \\n \\n\\n circle_marker_16640c547887e571ef5d0e37616cbec0.bindPopup(popup_d0214a7a7cfab52b9d00c68df991cc20)\\n ;\\n\\n \\n \\n \\n var circle_marker_4c137b5c49d12e114df171d7acda2ffa = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_784ad51c40f066a00bb320586e1123e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b5e902cf3844ea2d51898c30176a719a = $(`<div id="html_b5e902cf3844ea2d51898c30176a719a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_784ad51c40f066a00bb320586e1123e7.setContent(html_b5e902cf3844ea2d51898c30176a719a);\\n \\n \\n\\n circle_marker_4c137b5c49d12e114df171d7acda2ffa.bindPopup(popup_784ad51c40f066a00bb320586e1123e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f1e94ac14614362daa9838d46b9926d = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_7998f921a43b1d1bbb2955cb8f47af19 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70b4c3031b8b22abad23babeec92cc12 = $(`<div id="html_70b4c3031b8b22abad23babeec92cc12" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7998f921a43b1d1bbb2955cb8f47af19.setContent(html_70b4c3031b8b22abad23babeec92cc12);\\n \\n \\n\\n circle_marker_9f1e94ac14614362daa9838d46b9926d.bindPopup(popup_7998f921a43b1d1bbb2955cb8f47af19)\\n ;\\n\\n \\n \\n \\n var circle_marker_479e1ca80030eec30533e65488f80582 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_f57848ae22fa9e2eae8c28372ffef5f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d789240e4937191dfaa13c7c422154f0 = $(`<div id="html_d789240e4937191dfaa13c7c422154f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f57848ae22fa9e2eae8c28372ffef5f8.setContent(html_d789240e4937191dfaa13c7c422154f0);\\n \\n \\n\\n circle_marker_479e1ca80030eec30533e65488f80582.bindPopup(popup_f57848ae22fa9e2eae8c28372ffef5f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca11e3c9e97bf8852d6a340d16969bb4 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_397c1c4db51f0f5d6e9efc8d9a162375 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe0e677114102ccf6c0c20d98e0ccebf = $(`<div id="html_fe0e677114102ccf6c0c20d98e0ccebf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_397c1c4db51f0f5d6e9efc8d9a162375.setContent(html_fe0e677114102ccf6c0c20d98e0ccebf);\\n \\n \\n\\n circle_marker_ca11e3c9e97bf8852d6a340d16969bb4.bindPopup(popup_397c1c4db51f0f5d6e9efc8d9a162375)\\n ;\\n\\n \\n \\n \\n var circle_marker_6eac7ba59ee482d4f9033abacba92a20 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_2803b88803feb0c0caf60fe0d38bbfd5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2be3fa51e2fe591941d6a427d70bce6c = $(`<div id="html_2be3fa51e2fe591941d6a427d70bce6c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2803b88803feb0c0caf60fe0d38bbfd5.setContent(html_2be3fa51e2fe591941d6a427d70bce6c);\\n \\n \\n\\n circle_marker_6eac7ba59ee482d4f9033abacba92a20.bindPopup(popup_2803b88803feb0c0caf60fe0d38bbfd5)\\n ;\\n\\n \\n \\n \\n var circle_marker_f406717374fd7605e0608ab4f7535f5f = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_922b00eb7b9724781fe310ce5dfeb3c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_207b3623869fd7662e8ca981214033f3 = $(`<div id="html_207b3623869fd7662e8ca981214033f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_922b00eb7b9724781fe310ce5dfeb3c9.setContent(html_207b3623869fd7662e8ca981214033f3);\\n \\n \\n\\n circle_marker_f406717374fd7605e0608ab4f7535f5f.bindPopup(popup_922b00eb7b9724781fe310ce5dfeb3c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_44840b41e7d275204301a699420e9419 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_81a689ec65971f08d1e816b8e8a840d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e7e56b8eee8373b362329ba9a32f152 = $(`<div id="html_8e7e56b8eee8373b362329ba9a32f152" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81a689ec65971f08d1e816b8e8a840d1.setContent(html_8e7e56b8eee8373b362329ba9a32f152);\\n \\n \\n\\n circle_marker_44840b41e7d275204301a699420e9419.bindPopup(popup_81a689ec65971f08d1e816b8e8a840d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_4f3c28f01981be3478b0960f7f1d7a13 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_6cf0fcc00675e5b5a4efa50bee6fdd8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67fd850c9d8b12322b807e6b125f476c = $(`<div id="html_67fd850c9d8b12322b807e6b125f476c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6cf0fcc00675e5b5a4efa50bee6fdd8f.setContent(html_67fd850c9d8b12322b807e6b125f476c);\\n \\n \\n\\n circle_marker_4f3c28f01981be3478b0960f7f1d7a13.bindPopup(popup_6cf0fcc00675e5b5a4efa50bee6fdd8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_51f4d97a1ff56d969a3e9635cf82441c = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_6bd8be32160baf3afea8ea1c49541beb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e577e5da631886bdde2117389a07d778 = $(`<div id="html_e577e5da631886bdde2117389a07d778" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6bd8be32160baf3afea8ea1c49541beb.setContent(html_e577e5da631886bdde2117389a07d778);\\n \\n \\n\\n circle_marker_51f4d97a1ff56d969a3e9635cf82441c.bindPopup(popup_6bd8be32160baf3afea8ea1c49541beb)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e5c9c0144fd8975fc845024bb958cd9 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_7e0cd86c66e802aa637c91337ef6dc08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3507abfc8002bdae457e4d7143ec8525 = $(`<div id="html_3507abfc8002bdae457e4d7143ec8525" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e0cd86c66e802aa637c91337ef6dc08.setContent(html_3507abfc8002bdae457e4d7143ec8525);\\n \\n \\n\\n circle_marker_2e5c9c0144fd8975fc845024bb958cd9.bindPopup(popup_7e0cd86c66e802aa637c91337ef6dc08)\\n ;\\n\\n \\n \\n \\n var circle_marker_081bfc94e5df32384c74abca2c8da6b9 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_15bfcf45423a71d89ed67dc7a8b5a09f);\\n \\n \\n var popup_8fe2fd33d067d10d53ca6785bc69e158 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc16e95cbb0a54fecf0792459f81dae7 = $(`<div id="html_bc16e95cbb0a54fecf0792459f81dae7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fe2fd33d067d10d53ca6785bc69e158.setContent(html_bc16e95cbb0a54fecf0792459f81dae7);\\n \\n \\n\\n circle_marker_081bfc94e5df32384c74abca2c8da6b9.bindPopup(popup_8fe2fd33d067d10d53ca6785bc69e158)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Buckthorn, glossy\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_9f279ab10f0cf9933bf96724ca861fb0 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_9f279ab10f0cf9933bf96724ca861fb0" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_9f279ab10f0cf9933bf96724ca861fb0 = L.map(\\n "map_9f279ab10f0cf9933bf96724ca861fb0",\\n {\\n center: [42.730932159748164, -73.25257248411089],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_bfb7f9e1a23ef632699f3279a8658d0f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var circle_marker_590db341f7c88c04e939ab90e3ea150b = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_fc007aa9761493bf35826ad8308ebd76 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c94c4b98c2b74317859a9bd9cfb99503 = $(`<div id="html_c94c4b98c2b74317859a9bd9cfb99503" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc007aa9761493bf35826ad8308ebd76.setContent(html_c94c4b98c2b74317859a9bd9cfb99503);\\n \\n \\n\\n circle_marker_590db341f7c88c04e939ab90e3ea150b.bindPopup(popup_fc007aa9761493bf35826ad8308ebd76)\\n ;\\n\\n \\n \\n \\n var circle_marker_a79e780e317a2ac8a880e04ef0399d6b = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_35e6b3a4e3598a6ac77713bb737a8efe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_72ab934d70622c6e9c544b3b4425d7f5 = $(`<div id="html_72ab934d70622c6e9c544b3b4425d7f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35e6b3a4e3598a6ac77713bb737a8efe.setContent(html_72ab934d70622c6e9c544b3b4425d7f5);\\n \\n \\n\\n circle_marker_a79e780e317a2ac8a880e04ef0399d6b.bindPopup(popup_35e6b3a4e3598a6ac77713bb737a8efe)\\n ;\\n\\n \\n \\n \\n var circle_marker_541a05f1da652701e7edef14cc35dc78 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_6377dc0039f2524b1eae8eeb4e70be54 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95c4f49ff7ed1d85d44ec2e7bb113d42 = $(`<div id="html_95c4f49ff7ed1d85d44ec2e7bb113d42" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6377dc0039f2524b1eae8eeb4e70be54.setContent(html_95c4f49ff7ed1d85d44ec2e7bb113d42);\\n \\n \\n\\n circle_marker_541a05f1da652701e7edef14cc35dc78.bindPopup(popup_6377dc0039f2524b1eae8eeb4e70be54)\\n ;\\n\\n \\n \\n \\n var circle_marker_89f8546298c0a65195b27e97b06828e7 = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_d6b72625e439b6ca69972c3c532b1dc5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_520e63d6e1174915fe7850590e1177f1 = $(`<div id="html_520e63d6e1174915fe7850590e1177f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d6b72625e439b6ca69972c3c532b1dc5.setContent(html_520e63d6e1174915fe7850590e1177f1);\\n \\n \\n\\n circle_marker_89f8546298c0a65195b27e97b06828e7.bindPopup(popup_d6b72625e439b6ca69972c3c532b1dc5)\\n ;\\n\\n \\n \\n \\n var circle_marker_2cea8cc15d8c281e09da7764af1e8e0b = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_bbcf08bfa8ebde2d19def86e24430533 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c253d3661ecbdf79894013582d80677a = $(`<div id="html_c253d3661ecbdf79894013582d80677a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bbcf08bfa8ebde2d19def86e24430533.setContent(html_c253d3661ecbdf79894013582d80677a);\\n \\n \\n\\n circle_marker_2cea8cc15d8c281e09da7764af1e8e0b.bindPopup(popup_bbcf08bfa8ebde2d19def86e24430533)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a58f1d549b3f72f26988849b5001907 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_16017333c8527c715929b857407dec9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a8f27fe55419cf7b21b3917cd3be51b = $(`<div id="html_7a8f27fe55419cf7b21b3917cd3be51b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16017333c8527c715929b857407dec9c.setContent(html_7a8f27fe55419cf7b21b3917cd3be51b);\\n \\n \\n\\n circle_marker_8a58f1d549b3f72f26988849b5001907.bindPopup(popup_16017333c8527c715929b857407dec9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_51097ed8b332d229ca86fcba55703aec = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.154581744998688, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_0505670199f132d8f4269c9bc8ff291d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f5dff8726e52a8cc3f7e42d47c71b3ff = $(`<div id="html_f5dff8726e52a8cc3f7e42d47c71b3ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0505670199f132d8f4269c9bc8ff291d.setContent(html_f5dff8726e52a8cc3f7e42d47c71b3ff);\\n \\n \\n\\n circle_marker_51097ed8b332d229ca86fcba55703aec.bindPopup(popup_0505670199f132d8f4269c9bc8ff291d)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7fc6d7360b738b3f266040bfde447b1 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_89321cb94d14911318bcd9c5a38ac840 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be7e03f3ca21b4b36d8a1ad1b01a41d5 = $(`<div id="html_be7e03f3ca21b4b36d8a1ad1b01a41d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89321cb94d14911318bcd9c5a38ac840.setContent(html_be7e03f3ca21b4b36d8a1ad1b01a41d5);\\n \\n \\n\\n circle_marker_e7fc6d7360b738b3f266040bfde447b1.bindPopup(popup_89321cb94d14911318bcd9c5a38ac840)\\n ;\\n\\n \\n \\n \\n var circle_marker_5853a8031fd6a6ebae2f7b8d2f550ed0 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_ee99d2ffe1faf753b07323765e9950f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed412ef497fa205c11bfdc5ae8d53074 = $(`<div id="html_ed412ef497fa205c11bfdc5ae8d53074" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee99d2ffe1faf753b07323765e9950f9.setContent(html_ed412ef497fa205c11bfdc5ae8d53074);\\n \\n \\n\\n circle_marker_5853a8031fd6a6ebae2f7b8d2f550ed0.bindPopup(popup_ee99d2ffe1faf753b07323765e9950f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9816cffcbaa4c2652c8ea684c57faf95 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_3176435b002e1f1ed4320473bc95fc07 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_612789b997b86da11d1760ce4e4e95da = $(`<div id="html_612789b997b86da11d1760ce4e4e95da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3176435b002e1f1ed4320473bc95fc07.setContent(html_612789b997b86da11d1760ce4e4e95da);\\n \\n \\n\\n circle_marker_9816cffcbaa4c2652c8ea684c57faf95.bindPopup(popup_3176435b002e1f1ed4320473bc95fc07)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7b4f3c418a4252d6cbba6b9c4052b87 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_8e2c7dd44e75897ed631253e443cbf1d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae36dff4813b85a5519cad934e30d930 = $(`<div id="html_ae36dff4813b85a5519cad934e30d930" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e2c7dd44e75897ed631253e443cbf1d.setContent(html_ae36dff4813b85a5519cad934e30d930);\\n \\n \\n\\n circle_marker_a7b4f3c418a4252d6cbba6b9c4052b87.bindPopup(popup_8e2c7dd44e75897ed631253e443cbf1d)\\n ;\\n\\n \\n \\n \\n var circle_marker_e357f01f69471e8774d536179d5ee392 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_c8768bd283e2c9d8178be0e7b10ae02b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc675b1708ef871a899da131d5a3bdb9 = $(`<div id="html_bc675b1708ef871a899da131d5a3bdb9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8768bd283e2c9d8178be0e7b10ae02b.setContent(html_bc675b1708ef871a899da131d5a3bdb9);\\n \\n \\n\\n circle_marker_e357f01f69471e8774d536179d5ee392.bindPopup(popup_c8768bd283e2c9d8178be0e7b10ae02b)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b0468d1f0d81f8b5ec6d3e41e213c6c = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_8e8d2612d604879610b051660b2f036c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60059951359f6faace74a42acff2db8c = $(`<div id="html_60059951359f6faace74a42acff2db8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e8d2612d604879610b051660b2f036c.setContent(html_60059951359f6faace74a42acff2db8c);\\n \\n \\n\\n circle_marker_4b0468d1f0d81f8b5ec6d3e41e213c6c.bindPopup(popup_8e8d2612d604879610b051660b2f036c)\\n ;\\n\\n \\n \\n \\n var circle_marker_43937c262bed74b5dcc51a7e75fa41c5 = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_915d62502a38a0f4a84cbd72c7afc91d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7644391f3bf3a136fdd258fbfc8c2ea8 = $(`<div id="html_7644391f3bf3a136fdd258fbfc8c2ea8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_915d62502a38a0f4a84cbd72c7afc91d.setContent(html_7644391f3bf3a136fdd258fbfc8c2ea8);\\n \\n \\n\\n circle_marker_43937c262bed74b5dcc51a7e75fa41c5.bindPopup(popup_915d62502a38a0f4a84cbd72c7afc91d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c26c1c25e4d4f1a83b1f9d357d67469b = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_3c87b8a4e7f4e6299ca19000b91f43a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e8370de3cc433b14ae796c3ef800c05b = $(`<div id="html_e8370de3cc433b14ae796c3ef800c05b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c87b8a4e7f4e6299ca19000b91f43a4.setContent(html_e8370de3cc433b14ae796c3ef800c05b);\\n \\n \\n\\n circle_marker_c26c1c25e4d4f1a83b1f9d357d67469b.bindPopup(popup_3c87b8a4e7f4e6299ca19000b91f43a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_6975e756aaeb483664be6d3364b63bd6 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_8b25a49708c8a39f8d6b43aca42476d2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1964b9b0539ce84f1111862a5445c167 = $(`<div id="html_1964b9b0539ce84f1111862a5445c167" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b25a49708c8a39f8d6b43aca42476d2.setContent(html_1964b9b0539ce84f1111862a5445c167);\\n \\n \\n\\n circle_marker_6975e756aaeb483664be6d3364b63bd6.bindPopup(popup_8b25a49708c8a39f8d6b43aca42476d2)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e1bbea165a43a9b58a65431b5f3e6f0 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_9f279ab10f0cf9933bf96724ca861fb0);\\n \\n \\n var popup_0f471cdc1831e77f82e1da1818104503 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_951826e4889935621f5cf10b77e95206 = $(`<div id="html_951826e4889935621f5cf10b77e95206" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f471cdc1831e77f82e1da1818104503.setContent(html_951826e4889935621f5cf10b77e95206);\\n \\n \\n\\n circle_marker_1e1bbea165a43a9b58a65431b5f3e6f0.bindPopup(popup_0f471cdc1831e77f82e1da1818104503)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Burning bush": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_a88647fd4d81467e9d17d8f6be379d5f {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_a88647fd4d81467e9d17d8f6be379d5f" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_a88647fd4d81467e9d17d8f6be379d5f = L.map(\\n "map_a88647fd4d81467e9d17d8f6be379d5f",\\n {\\n center: [42.72641268425542, -73.22366702061295],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_efd9fec43243c98a77559a9a06a08fbd = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_a88647fd4d81467e9d17d8f6be379d5f);\\n \\n \\n var circle_marker_d623198851c89ae3e96a121d39961431 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_a88647fd4d81467e9d17d8f6be379d5f);\\n \\n \\n var popup_4f5abc28bd03d459446c84faa45bf5ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96b54b45a38a39531c6922070f392d5f = $(`<div id="html_96b54b45a38a39531c6922070f392d5f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f5abc28bd03d459446c84faa45bf5ab.setContent(html_96b54b45a38a39531c6922070f392d5f);\\n \\n \\n\\n circle_marker_d623198851c89ae3e96a121d39961431.bindPopup(popup_4f5abc28bd03d459446c84faa45bf5ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_1affdded3439e689d4931c983ae5264a = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_a88647fd4d81467e9d17d8f6be379d5f);\\n \\n \\n var popup_a35f70a70924c32bac2b906e25100ed1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_93e64da7081b1e7fe510c1bb6435ad75 = $(`<div id="html_93e64da7081b1e7fe510c1bb6435ad75" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a35f70a70924c32bac2b906e25100ed1.setContent(html_93e64da7081b1e7fe510c1bb6435ad75);\\n \\n \\n\\n circle_marker_1affdded3439e689d4931c983ae5264a.bindPopup(popup_a35f70a70924c32bac2b906e25100ed1)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b31814be8f083c56ee054a998eae76a = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_a88647fd4d81467e9d17d8f6be379d5f);\\n \\n \\n var popup_bd4bce0b803f385ea9e5149aa0162ead = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b5867117992c1a341e40026d7716521 = $(`<div id="html_7b5867117992c1a341e40026d7716521" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd4bce0b803f385ea9e5149aa0162ead.setContent(html_7b5867117992c1a341e40026d7716521);\\n \\n \\n\\n circle_marker_3b31814be8f083c56ee054a998eae76a.bindPopup(popup_bd4bce0b803f385ea9e5149aa0162ead)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Butternut": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_707a2e3a9c1e78259b8b9c18bd73f4c2 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_707a2e3a9c1e78259b8b9c18bd73f4c2" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_707a2e3a9c1e78259b8b9c18bd73f4c2 = L.map(\\n "map_707a2e3a9c1e78259b8b9c18bd73f4c2",\\n {\\n center: [42.729122501918184, -73.2236648344234],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_f3d705fb84ecfe41e57a3f8a82d9a374 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_707a2e3a9c1e78259b8b9c18bd73f4c2);\\n \\n \\n var circle_marker_b68b6b662c66dcdf32026d0e7395795d = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_707a2e3a9c1e78259b8b9c18bd73f4c2);\\n \\n \\n var popup_58742863c75e1a1c6689057997baa634 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed4a6991a1ab7b0b5b7b8ca42f2e68aa = $(`<div id="html_ed4a6991a1ab7b0b5b7b8ca42f2e68aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58742863c75e1a1c6689057997baa634.setContent(html_ed4a6991a1ab7b0b5b7b8ca42f2e68aa);\\n \\n \\n\\n circle_marker_b68b6b662c66dcdf32026d0e7395795d.bindPopup(popup_58742863c75e1a1c6689057997baa634)\\n ;\\n\\n \\n \\n \\n var circle_marker_40d7c05759983387e096ab311e0be79c = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_707a2e3a9c1e78259b8b9c18bd73f4c2);\\n \\n \\n var popup_75a8437134ef72ae705e1428981518c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13ea41eb63815222fd3b5f87fe807930 = $(`<div id="html_13ea41eb63815222fd3b5f87fe807930" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75a8437134ef72ae705e1428981518c6.setContent(html_13ea41eb63815222fd3b5f87fe807930);\\n \\n \\n\\n circle_marker_40d7c05759983387e096ab311e0be79c.bindPopup(popup_75a8437134ef72ae705e1428981518c6)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Cherry, black\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_f2f4d52e36e0f8060a1c373f1c3d6035 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_f2f4d52e36e0f8060a1c373f1c3d6035" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_f2f4d52e36e0f8060a1c373f1c3d6035 = L.map(\\n "map_f2f4d52e36e0f8060a1c373f1c3d6035",\\n {\\n center: [42.73545146795501, -73.24764986998687],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_2ea36c96f0f2369affb41a20c2cb31ef = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var circle_marker_1f5a296c057cb778ea0961af1a9d6d6e = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_ca7957841f568a0aedcd8ed45d7b6e57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0163ba7b422fba9f83cd75fb94086536 = $(`<div id="html_0163ba7b422fba9f83cd75fb94086536" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca7957841f568a0aedcd8ed45d7b6e57.setContent(html_0163ba7b422fba9f83cd75fb94086536);\\n \\n \\n\\n circle_marker_1f5a296c057cb778ea0961af1a9d6d6e.bindPopup(popup_ca7957841f568a0aedcd8ed45d7b6e57)\\n ;\\n\\n \\n \\n \\n var circle_marker_09e542333bd1ead364ecea239a3b5b3c = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_b64945cbd63eb0fabc47478dd4c4db12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9d2f69817336919a64b5470853d5078 = $(`<div id="html_a9d2f69817336919a64b5470853d5078" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b64945cbd63eb0fabc47478dd4c4db12.setContent(html_a9d2f69817336919a64b5470853d5078);\\n \\n \\n\\n circle_marker_09e542333bd1ead364ecea239a3b5b3c.bindPopup(popup_b64945cbd63eb0fabc47478dd4c4db12)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5cb4aa2c863c6b869f63d851f7611a4 = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e25d528166447815060f551a2c9a50d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b929f763d8f6b7fdd5a306ef5fd2541 = $(`<div id="html_3b929f763d8f6b7fdd5a306ef5fd2541" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e25d528166447815060f551a2c9a50d4.setContent(html_3b929f763d8f6b7fdd5a306ef5fd2541);\\n \\n \\n\\n circle_marker_f5cb4aa2c863c6b869f63d851f7611a4.bindPopup(popup_e25d528166447815060f551a2c9a50d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_34f21f1752641d79113ce8bde827bb82 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_a4c51a38ce81066c50fa78e5b1cbd01f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6331a0f59590eca38307d1648f918b3d = $(`<div id="html_6331a0f59590eca38307d1648f918b3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4c51a38ce81066c50fa78e5b1cbd01f.setContent(html_6331a0f59590eca38307d1648f918b3d);\\n \\n \\n\\n circle_marker_34f21f1752641d79113ce8bde827bb82.bindPopup(popup_a4c51a38ce81066c50fa78e5b1cbd01f)\\n ;\\n\\n \\n \\n \\n var circle_marker_08adc3e3b6db6c9e6db32c8b43b94fdb = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.068428945128219, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_479ec1b89059df91616642a4263c1a73 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac9e16a173cbb3e4c6eb529096ae9c20 = $(`<div id="html_ac9e16a173cbb3e4c6eb529096ae9c20" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_479ec1b89059df91616642a4263c1a73.setContent(html_ac9e16a173cbb3e4c6eb529096ae9c20);\\n \\n \\n\\n circle_marker_08adc3e3b6db6c9e6db32c8b43b94fdb.bindPopup(popup_479ec1b89059df91616642a4263c1a73)\\n ;\\n\\n \\n \\n \\n var circle_marker_5233ad996369aa040d899eb3542f2456 = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_bc2858d090fcff54586bff54816a4d7e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a0921f233fbeb23a8880b7905aa66972 = $(`<div id="html_a0921f233fbeb23a8880b7905aa66972" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc2858d090fcff54586bff54816a4d7e.setContent(html_a0921f233fbeb23a8880b7905aa66972);\\n \\n \\n\\n circle_marker_5233ad996369aa040d899eb3542f2456.bindPopup(popup_bc2858d090fcff54586bff54816a4d7e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ad3470b64c12808b14ef6005eb123ca4 = L.circleMarker(\\n [42.7417795414353, -73.27348403014923],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_750ac784dccf3d4dff12f8f4de621d30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2ba4b58ac789c93bb39e9690048b460c = $(`<div id="html_2ba4b58ac789c93bb39e9690048b460c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_750ac784dccf3d4dff12f8f4de621d30.setContent(html_2ba4b58ac789c93bb39e9690048b460c);\\n \\n \\n\\n circle_marker_ad3470b64c12808b14ef6005eb123ca4.bindPopup(popup_750ac784dccf3d4dff12f8f4de621d30)\\n ;\\n\\n \\n \\n \\n var circle_marker_32a0b506eedf1ec11ec13b137a256946 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_4f1b6859a49da46bd3f19cfa10d25266 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b980705c20410d765a73ea19b917aa4f = $(`<div id="html_b980705c20410d765a73ea19b917aa4f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f1b6859a49da46bd3f19cfa10d25266.setContent(html_b980705c20410d765a73ea19b917aa4f);\\n \\n \\n\\n circle_marker_32a0b506eedf1ec11ec13b137a256946.bindPopup(popup_4f1b6859a49da46bd3f19cfa10d25266)\\n ;\\n\\n \\n \\n \\n var circle_marker_20bb53f4dbb6243afb59dcd10772f0af = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_03221afa92564730ffd2a33cbb87b1ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ade69bbe7935194e7bb73bde56121e2 = $(`<div id="html_7ade69bbe7935194e7bb73bde56121e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03221afa92564730ffd2a33cbb87b1ce.setContent(html_7ade69bbe7935194e7bb73bde56121e2);\\n \\n \\n\\n circle_marker_20bb53f4dbb6243afb59dcd10772f0af.bindPopup(popup_03221afa92564730ffd2a33cbb87b1ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_15bd1e1e0b6da549dfe0dbe101c3f2a4 = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_d944f7ba737362dc2ead06d9681c5fb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b7826b54ab324f0cc858b98e63a039df = $(`<div id="html_b7826b54ab324f0cc858b98e63a039df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d944f7ba737362dc2ead06d9681c5fb1.setContent(html_b7826b54ab324f0cc858b98e63a039df);\\n \\n \\n\\n circle_marker_15bd1e1e0b6da549dfe0dbe101c3f2a4.bindPopup(popup_d944f7ba737362dc2ead06d9681c5fb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e5c067ec9e0774773e129a67a6d0a2b = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_86b7c6a410f6206dc4223ba259acf4a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4dac0969baffa8d5c5cf9c2ffedb9397 = $(`<div id="html_4dac0969baffa8d5c5cf9c2ffedb9397" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86b7c6a410f6206dc4223ba259acf4a4.setContent(html_4dac0969baffa8d5c5cf9c2ffedb9397);\\n \\n \\n\\n circle_marker_0e5c067ec9e0774773e129a67a6d0a2b.bindPopup(popup_86b7c6a410f6206dc4223ba259acf4a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d430ceeb08547aad7ece388262b955d = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_0cc2e74381ec5b93d2350bed73aaa873 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7a343d886f3b4ac2db77703a3e2160f = $(`<div id="html_c7a343d886f3b4ac2db77703a3e2160f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cc2e74381ec5b93d2350bed73aaa873.setContent(html_c7a343d886f3b4ac2db77703a3e2160f);\\n \\n \\n\\n circle_marker_2d430ceeb08547aad7ece388262b955d.bindPopup(popup_0cc2e74381ec5b93d2350bed73aaa873)\\n ;\\n\\n \\n \\n \\n var circle_marker_3acb878538a0a35c98a93ddf253b7680 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_349e5a0fa87740e1cc82be6400cc2623 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e50dd2fd741ef7ed021fde720d59844 = $(`<div id="html_1e50dd2fd741ef7ed021fde720d59844" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_349e5a0fa87740e1cc82be6400cc2623.setContent(html_1e50dd2fd741ef7ed021fde720d59844);\\n \\n \\n\\n circle_marker_3acb878538a0a35c98a93ddf253b7680.bindPopup(popup_349e5a0fa87740e1cc82be6400cc2623)\\n ;\\n\\n \\n \\n \\n var circle_marker_872e5c50db88da11e16a31124621a7c7 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_2dd8941209e0d81d1d3d8a758fb24df3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dfc718a3ac65007db3ef70c457744546 = $(`<div id="html_dfc718a3ac65007db3ef70c457744546" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2dd8941209e0d81d1d3d8a758fb24df3.setContent(html_dfc718a3ac65007db3ef70c457744546);\\n \\n \\n\\n circle_marker_872e5c50db88da11e16a31124621a7c7.bindPopup(popup_2dd8941209e0d81d1d3d8a758fb24df3)\\n ;\\n\\n \\n \\n \\n var circle_marker_0efc7400599926ed2c0a30b494048991 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_9d560acfd29bf414ec6605e2b86377b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6593e4ebf08547de44acb4f90ed18fce = $(`<div id="html_6593e4ebf08547de44acb4f90ed18fce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d560acfd29bf414ec6605e2b86377b3.setContent(html_6593e4ebf08547de44acb4f90ed18fce);\\n \\n \\n\\n circle_marker_0efc7400599926ed2c0a30b494048991.bindPopup(popup_9d560acfd29bf414ec6605e2b86377b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_54d833b9de86332175778694d3a1f761 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_8be7062683ed02fe133fe7deb4b63aef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ddd8bed05533dd80d04254b1cffee4c = $(`<div id="html_9ddd8bed05533dd80d04254b1cffee4c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8be7062683ed02fe133fe7deb4b63aef.setContent(html_9ddd8bed05533dd80d04254b1cffee4c);\\n \\n \\n\\n circle_marker_54d833b9de86332175778694d3a1f761.bindPopup(popup_8be7062683ed02fe133fe7deb4b63aef)\\n ;\\n\\n \\n \\n \\n var circle_marker_da691b3caf0f17413071e729c838cd65 = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_0310cdece5fffb90cb4a66ae3fc78ba6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e32feaa31e8a9ac650f9ea76f8bc3238 = $(`<div id="html_e32feaa31e8a9ac650f9ea76f8bc3238" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0310cdece5fffb90cb4a66ae3fc78ba6.setContent(html_e32feaa31e8a9ac650f9ea76f8bc3238);\\n \\n \\n\\n circle_marker_da691b3caf0f17413071e729c838cd65.bindPopup(popup_0310cdece5fffb90cb4a66ae3fc78ba6)\\n ;\\n\\n \\n \\n \\n var circle_marker_988368da17c0272633b1f4f296dc20ca = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_41fb1a1a7635c1d5625d0661665e9ebb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b99efcbe0b3e7e7f6e153bc4c845476a = $(`<div id="html_b99efcbe0b3e7e7f6e153bc4c845476a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41fb1a1a7635c1d5625d0661665e9ebb.setContent(html_b99efcbe0b3e7e7f6e153bc4c845476a);\\n \\n \\n\\n circle_marker_988368da17c0272633b1f4f296dc20ca.bindPopup(popup_41fb1a1a7635c1d5625d0661665e9ebb)\\n ;\\n\\n \\n \\n \\n var circle_marker_baa61a8455df4f1054a285ef367495f9 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_9339da4e5c6e997f3cc56f5181fa9d47 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c05dd9072637a017f06086d6ce036c8c = $(`<div id="html_c05dd9072637a017f06086d6ce036c8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9339da4e5c6e997f3cc56f5181fa9d47.setContent(html_c05dd9072637a017f06086d6ce036c8c);\\n \\n \\n\\n circle_marker_baa61a8455df4f1054a285ef367495f9.bindPopup(popup_9339da4e5c6e997f3cc56f5181fa9d47)\\n ;\\n\\n \\n \\n \\n var circle_marker_dfa6708d77c098bbbc1d8ca6a0b8a8b7 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_1afc8ee7b0c775dc0cb48af22ae4b278 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25ea6664f79ef2a6bc84ef00d8ec2c59 = $(`<div id="html_25ea6664f79ef2a6bc84ef00d8ec2c59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1afc8ee7b0c775dc0cb48af22ae4b278.setContent(html_25ea6664f79ef2a6bc84ef00d8ec2c59);\\n \\n \\n\\n circle_marker_dfa6708d77c098bbbc1d8ca6a0b8a8b7.bindPopup(popup_1afc8ee7b0c775dc0cb48af22ae4b278)\\n ;\\n\\n \\n \\n \\n var circle_marker_d3cd2b0baa9566005b043e27076cbcbf = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_f985251f67b3c3a9fd83582390bfa783 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_caa00e0be9cf1f37e4d877ac57aa64e8 = $(`<div id="html_caa00e0be9cf1f37e4d877ac57aa64e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f985251f67b3c3a9fd83582390bfa783.setContent(html_caa00e0be9cf1f37e4d877ac57aa64e8);\\n \\n \\n\\n circle_marker_d3cd2b0baa9566005b043e27076cbcbf.bindPopup(popup_f985251f67b3c3a9fd83582390bfa783)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1aa174865da5f844f89dd60aa20071e = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_b4955371ef87a2ddfb90833ebc93c336 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_45ab5d26fb70eb5fc5121a71f1c7c792 = $(`<div id="html_45ab5d26fb70eb5fc5121a71f1c7c792" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b4955371ef87a2ddfb90833ebc93c336.setContent(html_45ab5d26fb70eb5fc5121a71f1c7c792);\\n \\n \\n\\n circle_marker_b1aa174865da5f844f89dd60aa20071e.bindPopup(popup_b4955371ef87a2ddfb90833ebc93c336)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e651bde2e008383d8536a41759c4509 = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_7aeb880d339ba513345c8e8ccffa4649 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_077ef64b1e07e68800729ac9fe9e5eb6 = $(`<div id="html_077ef64b1e07e68800729ac9fe9e5eb6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7aeb880d339ba513345c8e8ccffa4649.setContent(html_077ef64b1e07e68800729ac9fe9e5eb6);\\n \\n \\n\\n circle_marker_1e651bde2e008383d8536a41759c4509.bindPopup(popup_7aeb880d339ba513345c8e8ccffa4649)\\n ;\\n\\n \\n \\n \\n var circle_marker_a13b173acf8a46a4cd67c97f511144ba = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_644db8d2a18d5ea850f6ee1b42f49a92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86857de31c7b0d55acd8b0c69a50844d = $(`<div id="html_86857de31c7b0d55acd8b0c69a50844d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_644db8d2a18d5ea850f6ee1b42f49a92.setContent(html_86857de31c7b0d55acd8b0c69a50844d);\\n \\n \\n\\n circle_marker_a13b173acf8a46a4cd67c97f511144ba.bindPopup(popup_644db8d2a18d5ea850f6ee1b42f49a92)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d399e1e54dcfdcb1f78dc3a702cc873 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_12c3c03c39e64662e6ff55418108e6cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c852aba281d8e34649452b0f1d990040 = $(`<div id="html_c852aba281d8e34649452b0f1d990040" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12c3c03c39e64662e6ff55418108e6cf.setContent(html_c852aba281d8e34649452b0f1d990040);\\n \\n \\n\\n circle_marker_9d399e1e54dcfdcb1f78dc3a702cc873.bindPopup(popup_12c3c03c39e64662e6ff55418108e6cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_821df979a199b97276c13c72f0431b2a = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_22917b335993e986c713ad2f4a72ee85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8392fd84106b4123119317bdf301067 = $(`<div id="html_c8392fd84106b4123119317bdf301067" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22917b335993e986c713ad2f4a72ee85.setContent(html_c8392fd84106b4123119317bdf301067);\\n \\n \\n\\n circle_marker_821df979a199b97276c13c72f0431b2a.bindPopup(popup_22917b335993e986c713ad2f4a72ee85)\\n ;\\n\\n \\n \\n \\n var circle_marker_b141f6c1a51ce24b8287aec1bb8fe2b6 = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.917270272703197, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_24f0444db4ad973de05757495c068830 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_885088039ad8c2099e7b633b0725b830 = $(`<div id="html_885088039ad8c2099e7b633b0725b830" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24f0444db4ad973de05757495c068830.setContent(html_885088039ad8c2099e7b633b0725b830);\\n \\n \\n\\n circle_marker_b141f6c1a51ce24b8287aec1bb8fe2b6.bindPopup(popup_24f0444db4ad973de05757495c068830)\\n ;\\n\\n \\n \\n \\n var circle_marker_0aede12da1fc91ebde796725054a7ba4 = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_54bc4866c2e7ee47934f4317cf18ffb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0ffbb48c127d1647e75bdf4ce1e6125 = $(`<div id="html_b0ffbb48c127d1647e75bdf4ce1e6125" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54bc4866c2e7ee47934f4317cf18ffb2.setContent(html_b0ffbb48c127d1647e75bdf4ce1e6125);\\n \\n \\n\\n circle_marker_0aede12da1fc91ebde796725054a7ba4.bindPopup(popup_54bc4866c2e7ee47934f4317cf18ffb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb0d04237b1b9d8dc2f4a3b6e8dff7e2 = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_28e3cce964c743f6f5cccaf0992f357d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_47c1e35c70ff727684d0fee5cb595650 = $(`<div id="html_47c1e35c70ff727684d0fee5cb595650" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_28e3cce964c743f6f5cccaf0992f357d.setContent(html_47c1e35c70ff727684d0fee5cb595650);\\n \\n \\n\\n circle_marker_eb0d04237b1b9d8dc2f4a3b6e8dff7e2.bindPopup(popup_28e3cce964c743f6f5cccaf0992f357d)\\n ;\\n\\n \\n \\n \\n var circle_marker_7875eb6a58c3c8d5cea76d59f70fb887 = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e1467cb85c466fec1840d54248787397 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_327b7fe330616122418692ca7987b5d5 = $(`<div id="html_327b7fe330616122418692ca7987b5d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1467cb85c466fec1840d54248787397.setContent(html_327b7fe330616122418692ca7987b5d5);\\n \\n \\n\\n circle_marker_7875eb6a58c3c8d5cea76d59f70fb887.bindPopup(popup_e1467cb85c466fec1840d54248787397)\\n ;\\n\\n \\n \\n \\n var circle_marker_99c94ee323ec92b1eeae12f4f920c57b = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.989422804014327, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_7f2afc8f2c9a80256614a846718ab3ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3cfbbec970869d6f1b9a8075f1587b6 = $(`<div id="html_a3cfbbec970869d6f1b9a8075f1587b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f2afc8f2c9a80256614a846718ab3ac.setContent(html_a3cfbbec970869d6f1b9a8075f1587b6);\\n \\n \\n\\n circle_marker_99c94ee323ec92b1eeae12f4f920c57b.bindPopup(popup_7f2afc8f2c9a80256614a846718ab3ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d96f2c18fef02f52ccb7d585a9c51c3 = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e5f0aa1691696ce3eb4a2a40504287e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_330166122bcceb31f0f74c6442e68d22 = $(`<div id="html_330166122bcceb31f0f74c6442e68d22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5f0aa1691696ce3eb4a2a40504287e9.setContent(html_330166122bcceb31f0f74c6442e68d22);\\n \\n \\n\\n circle_marker_0d96f2c18fef02f52ccb7d585a9c51c3.bindPopup(popup_e5f0aa1691696ce3eb4a2a40504287e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a40404ebde354d60825950ac91b9852 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_fbdc80b50dab882643bdbb1e7291504d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b97958ce52b95f10cab6e92117db7987 = $(`<div id="html_b97958ce52b95f10cab6e92117db7987" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fbdc80b50dab882643bdbb1e7291504d.setContent(html_b97958ce52b95f10cab6e92117db7987);\\n \\n \\n\\n circle_marker_5a40404ebde354d60825950ac91b9852.bindPopup(popup_fbdc80b50dab882643bdbb1e7291504d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b8a261dcfe7e8a1791660b23cad442c = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_2fc417439170448a9021dd624c1ffb1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c2061131531946c2028037fb436142b2 = $(`<div id="html_c2061131531946c2028037fb436142b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fc417439170448a9021dd624c1ffb1b.setContent(html_c2061131531946c2028037fb436142b2);\\n \\n \\n\\n circle_marker_0b8a261dcfe7e8a1791660b23cad442c.bindPopup(popup_2fc417439170448a9021dd624c1ffb1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba4f1962a5b61198e0e03c8db22477cf = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_1b3fc89ccffffa3932544edb940149c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04181472305403d3d2bd2b5ea771f50b = $(`<div id="html_04181472305403d3d2bd2b5ea771f50b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b3fc89ccffffa3932544edb940149c0.setContent(html_04181472305403d3d2bd2b5ea771f50b);\\n \\n \\n\\n circle_marker_ba4f1962a5b61198e0e03c8db22477cf.bindPopup(popup_1b3fc89ccffffa3932544edb940149c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_14bde6408151a40e02dfdd7637de57ee = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_ac3ed933718ad5640f3f47f6c811c954 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01e22a912a8d264409bd291ac243d7ae = $(`<div id="html_01e22a912a8d264409bd291ac243d7ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac3ed933718ad5640f3f47f6c811c954.setContent(html_01e22a912a8d264409bd291ac243d7ae);\\n \\n \\n\\n circle_marker_14bde6408151a40e02dfdd7637de57ee.bindPopup(popup_ac3ed933718ad5640f3f47f6c811c954)\\n ;\\n\\n \\n \\n \\n var circle_marker_36972553ce285bab206f462dd9556899 = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_565c0a4ec19f8085d7fe00172b15993e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09f5e2e7c1297db19bdb93df4921bbb0 = $(`<div id="html_09f5e2e7c1297db19bdb93df4921bbb0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_565c0a4ec19f8085d7fe00172b15993e.setContent(html_09f5e2e7c1297db19bdb93df4921bbb0);\\n \\n \\n\\n circle_marker_36972553ce285bab206f462dd9556899.bindPopup(popup_565c0a4ec19f8085d7fe00172b15993e)\\n ;\\n\\n \\n \\n \\n var circle_marker_427834275cf44e4803583e79696a89ac = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_f6e4b2df1425f50cc3369e902ed2a6ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a2c30e9fa3f8edd745a496e0a30385d = $(`<div id="html_0a2c30e9fa3f8edd745a496e0a30385d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6e4b2df1425f50cc3369e902ed2a6ec.setContent(html_0a2c30e9fa3f8edd745a496e0a30385d);\\n \\n \\n\\n circle_marker_427834275cf44e4803583e79696a89ac.bindPopup(popup_f6e4b2df1425f50cc3369e902ed2a6ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1bdaa9a1ad7e5b8a8b9ed27ae4af050 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_61c872cffa2921fb38f168c5f73f0795 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3fa7f1df6ca33a347ec92fc0d9873072 = $(`<div id="html_3fa7f1df6ca33a347ec92fc0d9873072" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61c872cffa2921fb38f168c5f73f0795.setContent(html_3fa7f1df6ca33a347ec92fc0d9873072);\\n \\n \\n\\n circle_marker_e1bdaa9a1ad7e5b8a8b9ed27ae4af050.bindPopup(popup_61c872cffa2921fb38f168c5f73f0795)\\n ;\\n\\n \\n \\n \\n var circle_marker_9441e5700688184af83d6de630ec0ffe = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_d3da3495ccaec522f268d7b70696a889 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7b6febfddfb9bbdabee96449537f1e1 = $(`<div id="html_f7b6febfddfb9bbdabee96449537f1e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3da3495ccaec522f268d7b70696a889.setContent(html_f7b6febfddfb9bbdabee96449537f1e1);\\n \\n \\n\\n circle_marker_9441e5700688184af83d6de630ec0ffe.bindPopup(popup_d3da3495ccaec522f268d7b70696a889)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d96f5558f288ba16686904fe5a0b682 = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_939d9236a7e2ec94bc792c53662cc134 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5d49536299b7e0d9e2c7c0be26fbdb8 = $(`<div id="html_e5d49536299b7e0d9e2c7c0be26fbdb8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_939d9236a7e2ec94bc792c53662cc134.setContent(html_e5d49536299b7e0d9e2c7c0be26fbdb8);\\n \\n \\n\\n circle_marker_5d96f5558f288ba16686904fe5a0b682.bindPopup(popup_939d9236a7e2ec94bc792c53662cc134)\\n ;\\n\\n \\n \\n \\n var circle_marker_5eb1d40e41d889b3a85c88fecd94069b = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_c8831a3fbd3002bc6624f3922f24bbc4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_843d71d604f3935e9be6953fd4c91427 = $(`<div id="html_843d71d604f3935e9be6953fd4c91427" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8831a3fbd3002bc6624f3922f24bbc4.setContent(html_843d71d604f3935e9be6953fd4c91427);\\n \\n \\n\\n circle_marker_5eb1d40e41d889b3a85c88fecd94069b.bindPopup(popup_c8831a3fbd3002bc6624f3922f24bbc4)\\n ;\\n\\n \\n \\n \\n var circle_marker_593c46ab694deacd0f0cf6fcccbae7c0 = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_73e4269a29662a529da076075aea0f0e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec306849f0f93e09a498af290b9a41ad = $(`<div id="html_ec306849f0f93e09a498af290b9a41ad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73e4269a29662a529da076075aea0f0e.setContent(html_ec306849f0f93e09a498af290b9a41ad);\\n \\n \\n\\n circle_marker_593c46ab694deacd0f0cf6fcccbae7c0.bindPopup(popup_73e4269a29662a529da076075aea0f0e)\\n ;\\n\\n \\n \\n \\n var circle_marker_0cf3444be06e4fa9deacff94e0dd403e = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_fd2b7e26c02244cbb79884235968504f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7217d64f652627e629d8d6cccdd4d527 = $(`<div id="html_7217d64f652627e629d8d6cccdd4d527" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd2b7e26c02244cbb79884235968504f.setContent(html_7217d64f652627e629d8d6cccdd4d527);\\n \\n \\n\\n circle_marker_0cf3444be06e4fa9deacff94e0dd403e.bindPopup(popup_fd2b7e26c02244cbb79884235968504f)\\n ;\\n\\n \\n \\n \\n var circle_marker_89ebfbb769609f2809d2c131c79c4980 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_86ba9f801450145fa1c2f7a3b400c824 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d95198da1547f8fc63675743194f4b1e = $(`<div id="html_d95198da1547f8fc63675743194f4b1e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86ba9f801450145fa1c2f7a3b400c824.setContent(html_d95198da1547f8fc63675743194f4b1e);\\n \\n \\n\\n circle_marker_89ebfbb769609f2809d2c131c79c4980.bindPopup(popup_86ba9f801450145fa1c2f7a3b400c824)\\n ;\\n\\n \\n \\n \\n var circle_marker_c161808de2a9dfeb1bd95249286af226 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_63984f7fb788e161522e1c8e87882430 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86a9f950f89cf24a2c90908818ab15bf = $(`<div id="html_86a9f950f89cf24a2c90908818ab15bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63984f7fb788e161522e1c8e87882430.setContent(html_86a9f950f89cf24a2c90908818ab15bf);\\n \\n \\n\\n circle_marker_c161808de2a9dfeb1bd95249286af226.bindPopup(popup_63984f7fb788e161522e1c8e87882430)\\n ;\\n\\n \\n \\n \\n var circle_marker_b72b63ae27488b267ecff615d2f09ece = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_8f2b57c580001636fcdfc25d1d2297a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b351fecff6a87991331ae166bdb9d1bc = $(`<div id="html_b351fecff6a87991331ae166bdb9d1bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f2b57c580001636fcdfc25d1d2297a2.setContent(html_b351fecff6a87991331ae166bdb9d1bc);\\n \\n \\n\\n circle_marker_b72b63ae27488b267ecff615d2f09ece.bindPopup(popup_8f2b57c580001636fcdfc25d1d2297a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_825a3cea138d8bec8ef7192a6fec5658 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_36ce13502a7442355996b6bef6411220 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5118f6ebb26238427f74ef02a92ea699 = $(`<div id="html_5118f6ebb26238427f74ef02a92ea699" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36ce13502a7442355996b6bef6411220.setContent(html_5118f6ebb26238427f74ef02a92ea699);\\n \\n \\n\\n circle_marker_825a3cea138d8bec8ef7192a6fec5658.bindPopup(popup_36ce13502a7442355996b6bef6411220)\\n ;\\n\\n \\n \\n \\n var circle_marker_d381ab0aa5cc4c5725d46e6999040c9b = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_de78384a8f5e899f1470c69b339714b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09eeeef04b7e83cc229186aba39f0517 = $(`<div id="html_09eeeef04b7e83cc229186aba39f0517" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de78384a8f5e899f1470c69b339714b6.setContent(html_09eeeef04b7e83cc229186aba39f0517);\\n \\n \\n\\n circle_marker_d381ab0aa5cc4c5725d46e6999040c9b.bindPopup(popup_de78384a8f5e899f1470c69b339714b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_11962a7cfe96eb1e5e932a4fd65b693f = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_c8f227679716b8d579358ce061c9abdc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d58a14b2e657500e1b2973671db8889 = $(`<div id="html_4d58a14b2e657500e1b2973671db8889" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8f227679716b8d579358ce061c9abdc.setContent(html_4d58a14b2e657500e1b2973671db8889);\\n \\n \\n\\n circle_marker_11962a7cfe96eb1e5e932a4fd65b693f.bindPopup(popup_c8f227679716b8d579358ce061c9abdc)\\n ;\\n\\n \\n \\n \\n var circle_marker_b29e448f24eeee5d9f34120b9ac33408 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_b0bf19c4d400640ceb9839af704da581 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf9e00966116d4f7e1dc4870cda08cfe = $(`<div id="html_cf9e00966116d4f7e1dc4870cda08cfe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0bf19c4d400640ceb9839af704da581.setContent(html_cf9e00966116d4f7e1dc4870cda08cfe);\\n \\n \\n\\n circle_marker_b29e448f24eeee5d9f34120b9ac33408.bindPopup(popup_b0bf19c4d400640ceb9839af704da581)\\n ;\\n\\n \\n \\n \\n var circle_marker_842d77143cd04c66fa2bb08413805b52 = L.circleMarker(\\n [42.7345450038305, -73.22673656679281],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_59e053bef4535d86365e8588a730289a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1612a6c54ed522a4aa3b995bf3f4f5dd = $(`<div id="html_1612a6c54ed522a4aa3b995bf3f4f5dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_59e053bef4535d86365e8588a730289a.setContent(html_1612a6c54ed522a4aa3b995bf3f4f5dd);\\n \\n \\n\\n circle_marker_842d77143cd04c66fa2bb08413805b52.bindPopup(popup_59e053bef4535d86365e8588a730289a)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ec08cf7b1ea3587dd9b544cde7b75f9 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_6f06af90b64bcc18e5f1c675e8c41d67 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3564625aa8adb34b70b0dda7100accd0 = $(`<div id="html_3564625aa8adb34b70b0dda7100accd0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f06af90b64bcc18e5f1c675e8c41d67.setContent(html_3564625aa8adb34b70b0dda7100accd0);\\n \\n \\n\\n circle_marker_9ec08cf7b1ea3587dd9b544cde7b75f9.bindPopup(popup_6f06af90b64bcc18e5f1c675e8c41d67)\\n ;\\n\\n \\n \\n \\n var circle_marker_24fbfcc536363acb04bd508f198fcd1a = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_63681e1f83890441d99cc8011cf08cd9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74eab1c4b6509b85273e6c3879a05d56 = $(`<div id="html_74eab1c4b6509b85273e6c3879a05d56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63681e1f83890441d99cc8011cf08cd9.setContent(html_74eab1c4b6509b85273e6c3879a05d56);\\n \\n \\n\\n circle_marker_24fbfcc536363acb04bd508f198fcd1a.bindPopup(popup_63681e1f83890441d99cc8011cf08cd9)\\n ;\\n\\n \\n \\n \\n var circle_marker_c92ea2fa1750bf6568dec1a91e69188a = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_88466f63cbc7cb331754459b44e455d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4c63859fb9613efecc80cd6c9389ddd1 = $(`<div id="html_4c63859fb9613efecc80cd6c9389ddd1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88466f63cbc7cb331754459b44e455d8.setContent(html_4c63859fb9613efecc80cd6c9389ddd1);\\n \\n \\n\\n circle_marker_c92ea2fa1750bf6568dec1a91e69188a.bindPopup(popup_88466f63cbc7cb331754459b44e455d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c511815d385da2ba459c1bbb1ecfa87 = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_cf4304795f2c7b4926143b03bb839221 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0c05c379e9bc727e00679c966a39468 = $(`<div id="html_c0c05c379e9bc727e00679c966a39468" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf4304795f2c7b4926143b03bb839221.setContent(html_c0c05c379e9bc727e00679c966a39468);\\n \\n \\n\\n circle_marker_2c511815d385da2ba459c1bbb1ecfa87.bindPopup(popup_cf4304795f2c7b4926143b03bb839221)\\n ;\\n\\n \\n \\n \\n var circle_marker_0f68409bac32e484cb254b5a8f41e413 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_62bf44e3dd76231e87753619f0b49510 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2481cdb5a88c6f29b3805fce15086a1b = $(`<div id="html_2481cdb5a88c6f29b3805fce15086a1b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62bf44e3dd76231e87753619f0b49510.setContent(html_2481cdb5a88c6f29b3805fce15086a1b);\\n \\n \\n\\n circle_marker_0f68409bac32e484cb254b5a8f41e413.bindPopup(popup_62bf44e3dd76231e87753619f0b49510)\\n ;\\n\\n \\n \\n \\n var circle_marker_b213f9712cc23950c400bdc3058018d8 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e13e20a02062de0275f251d5da227308 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_365e8ef2934bf1717e7e6754d2bba075 = $(`<div id="html_365e8ef2934bf1717e7e6754d2bba075" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e13e20a02062de0275f251d5da227308.setContent(html_365e8ef2934bf1717e7e6754d2bba075);\\n \\n \\n\\n circle_marker_b213f9712cc23950c400bdc3058018d8.bindPopup(popup_e13e20a02062de0275f251d5da227308)\\n ;\\n\\n \\n \\n \\n var circle_marker_94409e9dd0495a9818a0469f16cf77c3 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_05a4f0244ef61641e218c7ccfe33d194 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8b784ea44aadf513e67afb7f5214a2b = $(`<div id="html_a8b784ea44aadf513e67afb7f5214a2b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05a4f0244ef61641e218c7ccfe33d194.setContent(html_a8b784ea44aadf513e67afb7f5214a2b);\\n \\n \\n\\n circle_marker_94409e9dd0495a9818a0469f16cf77c3.bindPopup(popup_05a4f0244ef61641e218c7ccfe33d194)\\n ;\\n\\n \\n \\n \\n var circle_marker_35669ebc35d6ccdd8f87327b885e4189 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_9dd789847a0ff9c08f09bc7128d73c18 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3e1adcef31ceb3119ecfe4760810b2f = $(`<div id="html_a3e1adcef31ceb3119ecfe4760810b2f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9dd789847a0ff9c08f09bc7128d73c18.setContent(html_a3e1adcef31ceb3119ecfe4760810b2f);\\n \\n \\n\\n circle_marker_35669ebc35d6ccdd8f87327b885e4189.bindPopup(popup_9dd789847a0ff9c08f09bc7128d73c18)\\n ;\\n\\n \\n \\n \\n var circle_marker_13632ea994b3fd0314388de568c1bec3 = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_7868447accf1d4482c6e2f1b59737507 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7bf4e57994aa95e1a4a5a9627e10f5cd = $(`<div id="html_7bf4e57994aa95e1a4a5a9627e10f5cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7868447accf1d4482c6e2f1b59737507.setContent(html_7bf4e57994aa95e1a4a5a9627e10f5cd);\\n \\n \\n\\n circle_marker_13632ea994b3fd0314388de568c1bec3.bindPopup(popup_7868447accf1d4482c6e2f1b59737507)\\n ;\\n\\n \\n \\n \\n var circle_marker_82b4c3a39a0311deb10280463bbd008d = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_369718f1f88264024b83649205d17637 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fe1808046c10928f14e1f352482f2b1 = $(`<div id="html_8fe1808046c10928f14e1f352482f2b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_369718f1f88264024b83649205d17637.setContent(html_8fe1808046c10928f14e1f352482f2b1);\\n \\n \\n\\n circle_marker_82b4c3a39a0311deb10280463bbd008d.bindPopup(popup_369718f1f88264024b83649205d17637)\\n ;\\n\\n \\n \\n \\n var circle_marker_02f91ae6690964705017181c9ba9b2d3 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_80d624729f7de94ed8103eb75ec851e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f58c38f017b060c3a2645bbdfe00d46e = $(`<div id="html_f58c38f017b060c3a2645bbdfe00d46e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80d624729f7de94ed8103eb75ec851e3.setContent(html_f58c38f017b060c3a2645bbdfe00d46e);\\n \\n \\n\\n circle_marker_02f91ae6690964705017181c9ba9b2d3.bindPopup(popup_80d624729f7de94ed8103eb75ec851e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fa947d8f3072059c9606bb0789d4f68 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_625b2ff980d57d8700b54a67d2b27e94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ab1d129a827d2b7d8b2e88878ee72af = $(`<div id="html_6ab1d129a827d2b7d8b2e88878ee72af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_625b2ff980d57d8700b54a67d2b27e94.setContent(html_6ab1d129a827d2b7d8b2e88878ee72af);\\n \\n \\n\\n circle_marker_9fa947d8f3072059c9606bb0789d4f68.bindPopup(popup_625b2ff980d57d8700b54a67d2b27e94)\\n ;\\n\\n \\n \\n \\n var circle_marker_788dbdc2cb7d0da4a5315cf26bddd4cf = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_5f1359ddced37bf6a8770a1a8f26b93b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e0421ddff5b5b48e1ed5e57433241fc = $(`<div id="html_1e0421ddff5b5b48e1ed5e57433241fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f1359ddced37bf6a8770a1a8f26b93b.setContent(html_1e0421ddff5b5b48e1ed5e57433241fc);\\n \\n \\n\\n circle_marker_788dbdc2cb7d0da4a5315cf26bddd4cf.bindPopup(popup_5f1359ddced37bf6a8770a1a8f26b93b)\\n ;\\n\\n \\n \\n \\n var circle_marker_69c11d611e5470322052c2db4d1dfb27 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_d016c885d7a60d1edd05a122df28dbac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63002d686fb4ecc0a749515bce2a651c = $(`<div id="html_63002d686fb4ecc0a749515bce2a651c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d016c885d7a60d1edd05a122df28dbac.setContent(html_63002d686fb4ecc0a749515bce2a651c);\\n \\n \\n\\n circle_marker_69c11d611e5470322052c2db4d1dfb27.bindPopup(popup_d016c885d7a60d1edd05a122df28dbac)\\n ;\\n\\n \\n \\n \\n var circle_marker_05289dc081addba30a8d0ec019e2245d = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_68016747fc4f6276d2d6a6a7dfd89b77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9abcb57b2072d8e9936a1224c28fc41b = $(`<div id="html_9abcb57b2072d8e9936a1224c28fc41b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68016747fc4f6276d2d6a6a7dfd89b77.setContent(html_9abcb57b2072d8e9936a1224c28fc41b);\\n \\n \\n\\n circle_marker_05289dc081addba30a8d0ec019e2245d.bindPopup(popup_68016747fc4f6276d2d6a6a7dfd89b77)\\n ;\\n\\n \\n \\n \\n var circle_marker_9bce3fcf170852efd706c600e05ae45d = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_22062ee68f84204030d2728345f8b21a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b14317e47ca779f5d596151953c9960 = $(`<div id="html_5b14317e47ca779f5d596151953c9960" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22062ee68f84204030d2728345f8b21a.setContent(html_5b14317e47ca779f5d596151953c9960);\\n \\n \\n\\n circle_marker_9bce3fcf170852efd706c600e05ae45d.bindPopup(popup_22062ee68f84204030d2728345f8b21a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3906fce5fa9590296d4ea3a4e43b61d = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e6d63f0659534941f78d76c8d97c28bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7250d532733f9cfc9a8bbfdc4d676468 = $(`<div id="html_7250d532733f9cfc9a8bbfdc4d676468" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6d63f0659534941f78d76c8d97c28bc.setContent(html_7250d532733f9cfc9a8bbfdc4d676468);\\n \\n \\n\\n circle_marker_e3906fce5fa9590296d4ea3a4e43b61d.bindPopup(popup_e6d63f0659534941f78d76c8d97c28bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_1498967e8ff5c3e0ad1d046841749f58 = L.circleMarker(\\n [42.73093682684808, -73.27225242598358],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e08a3a06a4c31c84af1fe59528819f4a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78123c05dd8df00d4016d39d14b644f8 = $(`<div id="html_78123c05dd8df00d4016d39d14b644f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e08a3a06a4c31c84af1fe59528819f4a.setContent(html_78123c05dd8df00d4016d39d14b644f8);\\n \\n \\n\\n circle_marker_1498967e8ff5c3e0ad1d046841749f58.bindPopup(popup_e08a3a06a4c31c84af1fe59528819f4a)\\n ;\\n\\n \\n \\n \\n var circle_marker_861f2a974a6bc9e0db2c5aa1d75c392c = L.circleMarker(\\n [42.730936899260826, -73.2710223276323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_809b8900dd7a57b37887ac3a13df7991 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1813872e8b965d3e84d460ed3db2ac6 = $(`<div id="html_b1813872e8b965d3e84d460ed3db2ac6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_809b8900dd7a57b37887ac3a13df7991.setContent(html_b1813872e8b965d3e84d460ed3db2ac6);\\n \\n \\n\\n circle_marker_861f2a974a6bc9e0db2c5aa1d75c392c.bindPopup(popup_809b8900dd7a57b37887ac3a13df7991)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c6b1a39fb5a5500271e93772fe2e3fb = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_cc7baf4a7e469384aab0b6439e12fa88 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6edc0dab27e75c76ab1cc13ba6fea45 = $(`<div id="html_a6edc0dab27e75c76ab1cc13ba6fea45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc7baf4a7e469384aab0b6439e12fa88.setContent(html_a6edc0dab27e75c76ab1cc13ba6fea45);\\n \\n \\n\\n circle_marker_0c6b1a39fb5a5500271e93772fe2e3fb.bindPopup(popup_cc7baf4a7e469384aab0b6439e12fa88)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cec5780fa65164902b4dd33b0f4ae2b = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_51848cd5155405c279b68ffa0d51925e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ee400b1e9d72445a7e0a19c8d8d1879c = $(`<div id="html_ee400b1e9d72445a7e0a19c8d8d1879c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51848cd5155405c279b68ffa0d51925e.setContent(html_ee400b1e9d72445a7e0a19c8d8d1879c);\\n \\n \\n\\n circle_marker_3cec5780fa65164902b4dd33b0f4ae2b.bindPopup(popup_51848cd5155405c279b68ffa0d51925e)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e48f970cc50ac7a861d5e8aee19b4ff = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_a0a13262b1c64c01a4c42f2720235c13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b9faa5405ad6a5a643ff1de6428c045a = $(`<div id="html_b9faa5405ad6a5a643ff1de6428c045a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0a13262b1c64c01a4c42f2720235c13.setContent(html_b9faa5405ad6a5a643ff1de6428c045a);\\n \\n \\n\\n circle_marker_8e48f970cc50ac7a861d5e8aee19b4ff.bindPopup(popup_a0a13262b1c64c01a4c42f2720235c13)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c9e478a1424c00eb9879e037c0ea69f = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_f1dfa8de980bf69f27a40d9407f2346a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_460f37508132a0bab204ae25770da7f3 = $(`<div id="html_460f37508132a0bab204ae25770da7f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1dfa8de980bf69f27a40d9407f2346a.setContent(html_460f37508132a0bab204ae25770da7f3);\\n \\n \\n\\n circle_marker_1c9e478a1424c00eb9879e037c0ea69f.bindPopup(popup_f1dfa8de980bf69f27a40d9407f2346a)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5939f89c79691fc7309098f47fbd0db = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_46d9189bd8d3672b3d1ac7f9d499a892 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f37c158d3fdd03503f7932696856c6a = $(`<div id="html_0f37c158d3fdd03503f7932696856c6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46d9189bd8d3672b3d1ac7f9d499a892.setContent(html_0f37c158d3fdd03503f7932696856c6a);\\n \\n \\n\\n circle_marker_b5939f89c79691fc7309098f47fbd0db.bindPopup(popup_46d9189bd8d3672b3d1ac7f9d499a892)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b5bb61786f598269ebfc4a2294ffff7 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_bfeade08d9a5fa0434126b9d22d1f7db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8325e167581785f601a5f18d6b663ec3 = $(`<div id="html_8325e167581785f601a5f18d6b663ec3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfeade08d9a5fa0434126b9d22d1f7db.setContent(html_8325e167581785f601a5f18d6b663ec3);\\n \\n \\n\\n circle_marker_5b5bb61786f598269ebfc4a2294ffff7.bindPopup(popup_bfeade08d9a5fa0434126b9d22d1f7db)\\n ;\\n\\n \\n \\n \\n var circle_marker_6843c261188c7ca2bc8e1c2147a202e6 = L.circleMarker(\\n [42.73093113915656, -73.22796888742165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_b5a5df8580383503f42588e23625bab8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13e1c089e5f07a30142ad7b995a45482 = $(`<div id="html_13e1c089e5f07a30142ad7b995a45482" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5a5df8580383503f42588e23625bab8.setContent(html_13e1c089e5f07a30142ad7b995a45482);\\n \\n \\n\\n circle_marker_6843c261188c7ca2bc8e1c2147a202e6.bindPopup(popup_b5a5df8580383503f42588e23625bab8)\\n ;\\n\\n \\n \\n \\n var circle_marker_28033708a48aab295141da534b22b318 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_6f7ec3056081c5edf1437646e7e8fc83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f957e09f1f84f97827100b9994d459a = $(`<div id="html_5f957e09f1f84f97827100b9994d459a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f7ec3056081c5edf1437646e7e8fc83.setContent(html_5f957e09f1f84f97827100b9994d459a);\\n \\n \\n\\n circle_marker_28033708a48aab295141da534b22b318.bindPopup(popup_6f7ec3056081c5edf1437646e7e8fc83)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb6092ae4aebf81e7aca0f1204e68819 = L.circleMarker(\\n [42.73093032286755, -73.22550869120535],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_a9247c372275c2c02ed6e04401b3ad58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e98a3c1513df83344025b66d2f5eca3d = $(`<div id="html_e98a3c1513df83344025b66d2f5eca3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9247c372275c2c02ed6e04401b3ad58.setContent(html_e98a3c1513df83344025b66d2f5eca3d);\\n \\n \\n\\n circle_marker_fb6092ae4aebf81e7aca0f1204e68819.bindPopup(popup_a9247c372275c2c02ed6e04401b3ad58)\\n ;\\n\\n \\n \\n \\n var circle_marker_c7d61b0582b122033cf1d6901efe0fed = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_5ecaee2d5c105833ea85187aa4b9fe55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e61ab1dc9e96ff736a2e3820c367dbc = $(`<div id="html_8e61ab1dc9e96ff736a2e3820c367dbc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ecaee2d5c105833ea85187aa4b9fe55.setContent(html_8e61ab1dc9e96ff736a2e3820c367dbc);\\n \\n \\n\\n circle_marker_c7d61b0582b122033cf1d6901efe0fed.bindPopup(popup_5ecaee2d5c105833ea85187aa4b9fe55)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6ae6eb148ea5bcea9135049d36c3045 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_d9d61152b77522b11e6fc5594a951414 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e92b6ca5977720bf427ce7948cce0fb7 = $(`<div id="html_e92b6ca5977720bf427ce7948cce0fb7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d9d61152b77522b11e6fc5594a951414.setContent(html_e92b6ca5977720bf427ce7948cce0fb7);\\n \\n \\n\\n circle_marker_b6ae6eb148ea5bcea9135049d36c3045.bindPopup(popup_d9d61152b77522b11e6fc5594a951414)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ad1f172b7dfab7c4afe6d16fdb0a529 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_f6ec5c35003f2bc44a66e476445bba78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8e9e2f0e17c4accc0e83ff875a86ada = $(`<div id="html_d8e9e2f0e17c4accc0e83ff875a86ada" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6ec5c35003f2bc44a66e476445bba78.setContent(html_d8e9e2f0e17c4accc0e83ff875a86ada);\\n \\n \\n\\n circle_marker_7ad1f172b7dfab7c4afe6d16fdb0a529.bindPopup(popup_f6ec5c35003f2bc44a66e476445bba78)\\n ;\\n\\n \\n \\n \\n var circle_marker_1df3153be3524f88ad03d435a0df8776 = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_8925c5a08347974212debc2d0cc8f913 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21e78d4aed705723551d03ff8f212b0e = $(`<div id="html_21e78d4aed705723551d03ff8f212b0e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8925c5a08347974212debc2d0cc8f913.setContent(html_21e78d4aed705723551d03ff8f212b0e);\\n \\n \\n\\n circle_marker_1df3153be3524f88ad03d435a0df8776.bindPopup(popup_8925c5a08347974212debc2d0cc8f913)\\n ;\\n\\n \\n \\n \\n var circle_marker_f9a51ee3d11c4f285745e1ac7a9092eb = L.circleMarker(\\n [42.729129693483614, -73.27225221092779],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_cb1eaa79f9a87e8ea096de347a7ae0ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b5cf9cff72212d961e17285e011f1891 = $(`<div id="html_b5cf9cff72212d961e17285e011f1891" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb1eaa79f9a87e8ea096de347a7ae0ea.setContent(html_b5cf9cff72212d961e17285e011f1891);\\n \\n \\n\\n circle_marker_f9a51ee3d11c4f285745e1ac7a9092eb.bindPopup(popup_cb1eaa79f9a87e8ea096de347a7ae0ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7eab8535f9aa31708c3213e1cab9d5b = L.circleMarker(\\n [42.72912976589343, -73.27102214841915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e11aa3342f44a58cf2a8fc9d1829fa98 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0220777445b33dad807a0660b8fbf8cb = $(`<div id="html_0220777445b33dad807a0660b8fbf8cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e11aa3342f44a58cf2a8fc9d1829fa98.setContent(html_0220777445b33dad807a0660b8fbf8cb);\\n \\n \\n\\n circle_marker_f7eab8535f9aa31708c3213e1cab9d5b.bindPopup(popup_e11aa3342f44a58cf2a8fc9d1829fa98)\\n ;\\n\\n \\n \\n \\n var circle_marker_557d50ee8a8d7e769e3ddc0f13a350f3 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_07a754ac331f877f008927fe49263511 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3af07003ee56a9592c03ff0d84162a59 = $(`<div id="html_3af07003ee56a9592c03ff0d84162a59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07a754ac331f877f008927fe49263511.setContent(html_3af07003ee56a9592c03ff0d84162a59);\\n \\n \\n\\n circle_marker_557d50ee8a8d7e769e3ddc0f13a350f3.bindPopup(popup_07a754ac331f877f008927fe49263511)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a851f586aa4ecaaefdd98a9e34f14e0 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_0f51004daad678a927aa75ceb6525afa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e623b99816deb7c10ec36a5c00cd60d1 = $(`<div id="html_e623b99816deb7c10ec36a5c00cd60d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f51004daad678a927aa75ceb6525afa.setContent(html_e623b99816deb7c10ec36a5c00cd60d1);\\n \\n \\n\\n circle_marker_4a851f586aa4ecaaefdd98a9e34f14e0.bindPopup(popup_0f51004daad678a927aa75ceb6525afa)\\n ;\\n\\n \\n \\n \\n var circle_marker_990cdfc2e3c7a31c0aeb7e8c5029f3e7 = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_301b429c927a044bd57ff4adc3e35248 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40d788de37867c7f279e982d33e93e4d = $(`<div id="html_40d788de37867c7f279e982d33e93e4d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_301b429c927a044bd57ff4adc3e35248.setContent(html_40d788de37867c7f279e982d33e93e4d);\\n \\n \\n\\n circle_marker_990cdfc2e3c7a31c0aeb7e8c5029f3e7.bindPopup(popup_301b429c927a044bd57ff4adc3e35248)\\n ;\\n\\n \\n \\n \\n var circle_marker_9c055dd04a3109b67715cfbe6601fa73 = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_1ee7c7e48d86f056fe053cde795f5310 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3136d925fa9592373a4fe488cec678bd = $(`<div id="html_3136d925fa9592373a4fe488cec678bd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ee7c7e48d86f056fe053cde795f5310.setContent(html_3136d925fa9592373a4fe488cec678bd);\\n \\n \\n\\n circle_marker_9c055dd04a3109b67715cfbe6601fa73.bindPopup(popup_1ee7c7e48d86f056fe053cde795f5310)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c4830b7cb10a6cb5888804f5368f1b9 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_7bd43aa29c8cabd973ab855943ef2183 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9211c8c723546a5920015955b54d428e = $(`<div id="html_9211c8c723546a5920015955b54d428e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7bd43aa29c8cabd973ab855943ef2183.setContent(html_9211c8c723546a5920015955b54d428e);\\n \\n \\n\\n circle_marker_1c4830b7cb10a6cb5888804f5368f1b9.bindPopup(popup_7bd43aa29c8cabd973ab855943ef2183)\\n ;\\n\\n \\n \\n \\n var circle_marker_5214c9b01dd2672964b3ab532a36ffb5 = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_c46819e96a4f5c7bca848e47e0281f99 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a37baecc7a63d1e52b1080c8e78a363 = $(`<div id="html_7a37baecc7a63d1e52b1080c8e78a363" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c46819e96a4f5c7bca848e47e0281f99.setContent(html_7a37baecc7a63d1e52b1080c8e78a363);\\n \\n \\n\\n circle_marker_5214c9b01dd2672964b3ab532a36ffb5.bindPopup(popup_c46819e96a4f5c7bca848e47e0281f99)\\n ;\\n\\n \\n \\n \\n var circle_marker_b99bad55817b717b21904d535f51705d = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_9d78c9eff534f05a4547ce92a3efa762 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f16feb8e6d74c42d1763bd0edb7f563e = $(`<div id="html_f16feb8e6d74c42d1763bd0edb7f563e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d78c9eff534f05a4547ce92a3efa762.setContent(html_f16feb8e6d74c42d1763bd0edb7f563e);\\n \\n \\n\\n circle_marker_b99bad55817b717b21904d535f51705d.bindPopup(popup_9d78c9eff534f05a4547ce92a3efa762)\\n ;\\n\\n \\n \\n \\n var circle_marker_30a050ebde382be4dbe3d519b7205560 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_a425d41b34de900b46fa62f926ff3ea2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58321f2de6fa30eccdf8aa565fc24e5d = $(`<div id="html_58321f2de6fa30eccdf8aa565fc24e5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a425d41b34de900b46fa62f926ff3ea2.setContent(html_58321f2de6fa30eccdf8aa565fc24e5d);\\n \\n \\n\\n circle_marker_30a050ebde382be4dbe3d519b7205560.bindPopup(popup_a425d41b34de900b46fa62f926ff3ea2)\\n ;\\n\\n \\n \\n \\n var circle_marker_36a9abfc566b48af324621bf79794c78 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_9849093629827ec70147ca1bcafba179 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e872c01adbd32a46c6c2f63eb97b95b = $(`<div id="html_4e872c01adbd32a46c6c2f63eb97b95b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9849093629827ec70147ca1bcafba179.setContent(html_4e872c01adbd32a46c6c2f63eb97b95b);\\n \\n \\n\\n circle_marker_36a9abfc566b48af324621bf79794c78.bindPopup(popup_9849093629827ec70147ca1bcafba179)\\n ;\\n\\n \\n \\n \\n var circle_marker_362a6d3a7fd99d1dd321ad83b7183135 = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_95cf9e89f81656676fc0d4a9b168259f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6817d5104b073d328650df5866720af2 = $(`<div id="html_6817d5104b073d328650df5866720af2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95cf9e89f81656676fc0d4a9b168259f.setContent(html_6817d5104b073d328650df5866720af2);\\n \\n \\n\\n circle_marker_362a6d3a7fd99d1dd321ad83b7183135.bindPopup(popup_95cf9e89f81656676fc0d4a9b168259f)\\n ;\\n\\n \\n \\n \\n var circle_marker_5522c2d61c64cb32d2c0660022264a0f = L.circleMarker(\\n [42.72732269176802, -73.26979194254899],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_61defebea58445aafbbc2012b40c0772 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a92d4f6438f5c8a567cb5c1057e58bf = $(`<div id="html_3a92d4f6438f5c8a567cb5c1057e58bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61defebea58445aafbbc2012b40c0772.setContent(html_3a92d4f6438f5c8a567cb5c1057e58bf);\\n \\n \\n\\n circle_marker_5522c2d61c64cb32d2c0660022264a0f.bindPopup(popup_61defebea58445aafbbc2012b40c0772)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d44e1788a4290cc36e29bbb7f959bda = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_6a96a2b7d862399160f6d4eda6d9cb86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a877e32bb8f50def18b55fa2368d8b35 = $(`<div id="html_a877e32bb8f50def18b55fa2368d8b35" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a96a2b7d862399160f6d4eda6d9cb86.setContent(html_a877e32bb8f50def18b55fa2368d8b35);\\n \\n \\n\\n circle_marker_2d44e1788a4290cc36e29bbb7f959bda.bindPopup(popup_6a96a2b7d862399160f6d4eda6d9cb86)\\n ;\\n\\n \\n \\n \\n var circle_marker_bdec52ed57270b930c6c8f272be73109 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_dc954fa8ab01c72e8cbc373ed5a60f08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c9a3fc3d0f23d6bb0fb36e342367bab1 = $(`<div id="html_c9a3fc3d0f23d6bb0fb36e342367bab1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc954fa8ab01c72e8cbc373ed5a60f08.setContent(html_c9a3fc3d0f23d6bb0fb36e342367bab1);\\n \\n \\n\\n circle_marker_bdec52ed57270b930c6c8f272be73109.bindPopup(popup_dc954fa8ab01c72e8cbc373ed5a60f08)\\n ;\\n\\n \\n \\n \\n var circle_marker_25e324fe181cbecb91111677e0e2690f = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_94c8d581e65087934b3271d1bb8f6f08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e502ee7cd2ee8a149a23d2973c75e8d = $(`<div id="html_7e502ee7cd2ee8a149a23d2973c75e8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94c8d581e65087934b3271d1bb8f6f08.setContent(html_7e502ee7cd2ee8a149a23d2973c75e8d);\\n \\n \\n\\n circle_marker_25e324fe181cbecb91111677e0e2690f.bindPopup(popup_94c8d581e65087934b3271d1bb8f6f08)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff81e905cc6631ff48beedb6cc791895 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_2f3e2e007f36740dbdcee302e826c7e6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_933472a287d0d2619d50078c6f9c0f73 = $(`<div id="html_933472a287d0d2619d50078c6f9c0f73" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f3e2e007f36740dbdcee302e826c7e6.setContent(html_933472a287d0d2619d50078c6f9c0f73);\\n \\n \\n\\n circle_marker_ff81e905cc6631ff48beedb6cc791895.bindPopup(popup_2f3e2e007f36740dbdcee302e826c7e6)\\n ;\\n\\n \\n \\n \\n var circle_marker_d62bb0b7d9be28ca52bd300da41e3e13 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e28e659ede937d777991f6487b02609a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c7245da00f89814943477bc962c3b9c = $(`<div id="html_0c7245da00f89814943477bc962c3b9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e28e659ede937d777991f6487b02609a.setContent(html_0c7245da00f89814943477bc962c3b9c);\\n \\n \\n\\n circle_marker_d62bb0b7d9be28ca52bd300da41e3e13.bindPopup(popup_e28e659ede937d777991f6487b02609a)\\n ;\\n\\n \\n \\n \\n var circle_marker_970cbe76f06ba78730f0d185d78edd95 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_fb9bcea097ceb328400132148d64629a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6d337a8769e00b7fca87a0e160d44f7 = $(`<div id="html_c6d337a8769e00b7fca87a0e160d44f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb9bcea097ceb328400132148d64629a.setContent(html_c6d337a8769e00b7fca87a0e160d44f7);\\n \\n \\n\\n circle_marker_970cbe76f06ba78730f0d185d78edd95.bindPopup(popup_fb9bcea097ceb328400132148d64629a)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d0da5b3f6cc3badac00489f2fcba60d = L.circleMarker(\\n [42.72731518778323, -73.22305093225523],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_a68b2db9106dca52425eb844a3d46cd7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f1b1054345fcf92a36aabbd18499c8b = $(`<div id="html_6f1b1054345fcf92a36aabbd18499c8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a68b2db9106dca52425eb844a3d46cd7.setContent(html_6f1b1054345fcf92a36aabbd18499c8b);\\n \\n \\n\\n circle_marker_4d0da5b3f6cc3badac00489f2fcba60d.bindPopup(popup_a68b2db9106dca52425eb844a3d46cd7)\\n ;\\n\\n \\n \\n \\n var circle_marker_e70032dd5c5c8830ab8de0873ebb46b0 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_692c1df123967609be58e243a759a194 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_174f6b7528fec3a130676c3392962ffe = $(`<div id="html_174f6b7528fec3a130676c3392962ffe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_692c1df123967609be58e243a759a194.setContent(html_174f6b7528fec3a130676c3392962ffe);\\n \\n \\n\\n circle_marker_e70032dd5c5c8830ab8de0873ebb46b0.bindPopup(popup_692c1df123967609be58e243a759a194)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5d520e184cd17fbae7a84389c201306 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_e47cf52ccebe1bd403d899cb22eaa9f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a723e861e979839726289439641ebf9 = $(`<div id="html_1a723e861e979839726289439641ebf9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e47cf52ccebe1bd403d899cb22eaa9f0.setContent(html_1a723e861e979839726289439641ebf9);\\n \\n \\n\\n circle_marker_a5d520e184cd17fbae7a84389c201306.bindPopup(popup_e47cf52ccebe1bd403d899cb22eaa9f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_781164d150e42755698172287b4b3b93 = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_38d84a9ba0ea4a0a297137d43a04f7aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e06129876ed30703d2c68663d1674b4b = $(`<div id="html_e06129876ed30703d2c68663d1674b4b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38d84a9ba0ea4a0a297137d43a04f7aa.setContent(html_e06129876ed30703d2c68663d1674b4b);\\n \\n \\n\\n circle_marker_781164d150e42755698172287b4b3b93.bindPopup(popup_38d84a9ba0ea4a0a297137d43a04f7aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_96e399650dadeb4057423d3e63f3142d = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_2913b117ce1d5621adf2131deab8480f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99d748d3e98881a67ad5667a1ab135ca = $(`<div id="html_99d748d3e98881a67ad5667a1ab135ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2913b117ce1d5621adf2131deab8480f.setContent(html_99d748d3e98881a67ad5667a1ab135ca);\\n \\n \\n\\n circle_marker_96e399650dadeb4057423d3e63f3142d.bindPopup(popup_2913b117ce1d5621adf2131deab8480f)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b7b0518425abd19d83b2471662f4a86 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_5d162bf4714e267d467d1140c2d63b27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ac8121157a714fb2cfec1e68812a02f = $(`<div id="html_8ac8121157a714fb2cfec1e68812a02f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d162bf4714e267d467d1140c2d63b27.setContent(html_8ac8121157a714fb2cfec1e68812a02f);\\n \\n \\n\\n circle_marker_0b7b0518425abd19d83b2471662f4a86.bindPopup(popup_5d162bf4714e267d467d1140c2d63b27)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0d711947b79cf1804c02fec1afdb9c7 = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_cfa52cebb7f93e0ea01c1ca4d900b6f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec2a3ba570db2166b560672ba13069a2 = $(`<div id="html_ec2a3ba570db2166b560672ba13069a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfa52cebb7f93e0ea01c1ca4d900b6f5.setContent(html_ec2a3ba570db2166b560672ba13069a2);\\n \\n \\n\\n circle_marker_b0d711947b79cf1804c02fec1afdb9c7.bindPopup(popup_cfa52cebb7f93e0ea01c1ca4d900b6f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_90261a6e8ef41a65a9580cee62857289 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_440f6dc9fac4da925ab122256a8a8b0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53122c908382591f936b31bfff3fc14e = $(`<div id="html_53122c908382591f936b31bfff3fc14e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_440f6dc9fac4da925ab122256a8a8b0d.setContent(html_53122c908382591f936b31bfff3fc14e);\\n \\n \\n\\n circle_marker_90261a6e8ef41a65a9580cee62857289.bindPopup(popup_440f6dc9fac4da925ab122256a8a8b0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_a45a09a5a8263f1b340c89d9734ffca8 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_ad5c2830e287a4eb8d34fd617e8b9596 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_00001620e21d179539d67788486119f6 = $(`<div id="html_00001620e21d179539d67788486119f6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad5c2830e287a4eb8d34fd617e8b9596.setContent(html_00001620e21d179539d67788486119f6);\\n \\n \\n\\n circle_marker_a45a09a5a8263f1b340c89d9734ffca8.bindPopup(popup_ad5c2830e287a4eb8d34fd617e8b9596)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5b37c4f158fa7ce5fd7fb7c5fdf6e9a = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_1b547afd74de2147a3549b6579f42b29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a03438dc6186c9a27e090ca8b01d3de1 = $(`<div id="html_a03438dc6186c9a27e090ca8b01d3de1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b547afd74de2147a3549b6579f42b29.setContent(html_a03438dc6186c9a27e090ca8b01d3de1);\\n \\n \\n\\n circle_marker_a5b37c4f158fa7ce5fd7fb7c5fdf6e9a.bindPopup(popup_1b547afd74de2147a3549b6579f42b29)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c8e39e74672c4c36b7707a35b3f2d15 = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_2830254854e4619ff8de82d79fbff3e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7dd97c0ade01e5b1a33b00c72a81634c = $(`<div id="html_7dd97c0ade01e5b1a33b00c72a81634c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2830254854e4619ff8de82d79fbff3e7.setContent(html_7dd97c0ade01e5b1a33b00c72a81634c);\\n \\n \\n\\n circle_marker_7c8e39e74672c4c36b7707a35b3f2d15.bindPopup(popup_2830254854e4619ff8de82d79fbff3e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4a5e0395aab2581b9ce22a21623bc0b = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_b34ce04495f6071873e713ffea294a79 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_af59bff7a0dc28759698853d0953083b = $(`<div id="html_af59bff7a0dc28759698853d0953083b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b34ce04495f6071873e713ffea294a79.setContent(html_af59bff7a0dc28759698853d0953083b);\\n \\n \\n\\n circle_marker_c4a5e0395aab2581b9ce22a21623bc0b.bindPopup(popup_b34ce04495f6071873e713ffea294a79)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b7ff694ae4dbe1db97d2230d6da1a64 = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_58d694ed250808e17bc5a0fc866332bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4dd1344a7c74120980e0ab17df942a60 = $(`<div id="html_4dd1344a7c74120980e0ab17df942a60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58d694ed250808e17bc5a0fc866332bd.setContent(html_4dd1344a7c74120980e0ab17df942a60);\\n \\n \\n\\n circle_marker_3b7ff694ae4dbe1db97d2230d6da1a64.bindPopup(popup_58d694ed250808e17bc5a0fc866332bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_93c336c294c0c95515d4a3df0c623a4d = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_b1e17abcd9c3c0b2415effbf80a219f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3abd1922485d7d82f1f91f10dde0eeee = $(`<div id="html_3abd1922485d7d82f1f91f10dde0eeee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1e17abcd9c3c0b2415effbf80a219f6.setContent(html_3abd1922485d7d82f1f91f10dde0eeee);\\n \\n \\n\\n circle_marker_93c336c294c0c95515d4a3df0c623a4d.bindPopup(popup_b1e17abcd9c3c0b2415effbf80a219f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_e017d0e5dfc3cc56f40b05f69ac2a20f = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_4ed1f3f2572e7f8ae01288ae483971fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc64bdb88da4a23cba988bee7d3196b2 = $(`<div id="html_dc64bdb88da4a23cba988bee7d3196b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ed1f3f2572e7f8ae01288ae483971fc.setContent(html_dc64bdb88da4a23cba988bee7d3196b2);\\n \\n \\n\\n circle_marker_e017d0e5dfc3cc56f40b05f69ac2a20f.bindPopup(popup_4ed1f3f2572e7f8ae01288ae483971fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_97805634305535d33c39fde0363f9a2b = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_5cbedf25842242ccbd742522c5bdbf62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e427af4fdaa0d4ce9c649434c2459e3d = $(`<div id="html_e427af4fdaa0d4ce9c649434c2459e3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5cbedf25842242ccbd742522c5bdbf62.setContent(html_e427af4fdaa0d4ce9c649434c2459e3d);\\n \\n \\n\\n circle_marker_97805634305535d33c39fde0363f9a2b.bindPopup(popup_5cbedf25842242ccbd742522c5bdbf62)\\n ;\\n\\n \\n \\n \\n var circle_marker_78527535cd5e8cf173bee9530dba417e = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_48f427fb0acb47b6ad44a45391e921f7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32fc8cc214cb39a8931e83fe5bdbdfef = $(`<div id="html_32fc8cc214cb39a8931e83fe5bdbdfef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_48f427fb0acb47b6ad44a45391e921f7.setContent(html_32fc8cc214cb39a8931e83fe5bdbdfef);\\n \\n \\n\\n circle_marker_78527535cd5e8cf173bee9530dba417e.bindPopup(popup_48f427fb0acb47b6ad44a45391e921f7)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0e903c9f9d2288b455a24e8e1a812ba = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_455e04ec24606cdeaca59aaa1c39a976 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e4ceb30c86c68b17d78722b918d3ca5 = $(`<div id="html_7e4ceb30c86c68b17d78722b918d3ca5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_455e04ec24606cdeaca59aaa1c39a976.setContent(html_7e4ceb30c86c68b17d78722b918d3ca5);\\n \\n \\n\\n circle_marker_d0e903c9f9d2288b455a24e8e1a812ba.bindPopup(popup_455e04ec24606cdeaca59aaa1c39a976)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c7c0698434621eb7e9541bae0727641 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_a1f30c6a02b63ca87a7122dc3cfd1f45 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_933206e261f9082881046b4c6c2f6c06 = $(`<div id="html_933206e261f9082881046b4c6c2f6c06" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a1f30c6a02b63ca87a7122dc3cfd1f45.setContent(html_933206e261f9082881046b4c6c2f6c06);\\n \\n \\n\\n circle_marker_0c7c0698434621eb7e9541bae0727641.bindPopup(popup_a1f30c6a02b63ca87a7122dc3cfd1f45)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e959b400d050f5e5a32f471f2eca8e2 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_5edc971bb97973fd0e52fe539f6c25f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a88b7dcc8e4d5ffd629823d0fdfc07a9 = $(`<div id="html_a88b7dcc8e4d5ffd629823d0fdfc07a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5edc971bb97973fd0e52fe539f6c25f9.setContent(html_a88b7dcc8e4d5ffd629823d0fdfc07a9);\\n \\n \\n\\n circle_marker_5e959b400d050f5e5a32f471f2eca8e2.bindPopup(popup_5edc971bb97973fd0e52fe539f6c25f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d101366330273479c408dae7bc9dd73 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_f2f4d52e36e0f8060a1c373f1c3d6035);\\n \\n \\n var popup_26db1c382b9bd27ce285d78c49a0b25e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_434c648674049d4f4e2244e2b5ce55a5 = $(`<div id="html_434c648674049d4f4e2244e2b5ce55a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_26db1c382b9bd27ce285d78c49a0b25e.setContent(html_434c648674049d4f4e2244e2b5ce55a5);\\n \\n \\n\\n circle_marker_6d101366330273479c408dae7bc9dd73.bindPopup(popup_26db1c382b9bd27ce285d78c49a0b25e)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Cherry, choke\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_b0dc7769fd3a66043e647704a2450e84 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_b0dc7769fd3a66043e647704a2450e84" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_b0dc7769fd3a66043e647704a2450e84 = L.map(\\n "map_b0dc7769fd3a66043e647704a2450e84",\\n {\\n center: [42.73635569549022, -73.25503085222815],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_97578cc1be2bc80223e4d01ad3b6421f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_b0dc7769fd3a66043e647704a2450e84);\\n \\n \\n var circle_marker_d83497410bb9b2f1f419ec33a75d9410 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b0dc7769fd3a66043e647704a2450e84);\\n \\n \\n var popup_c8246d8288d0bbe1c17364f087557b1d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_160beb2b0c5eefe085a1d83a66076aad = $(`<div id="html_160beb2b0c5eefe085a1d83a66076aad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8246d8288d0bbe1c17364f087557b1d.setContent(html_160beb2b0c5eefe085a1d83a66076aad);\\n \\n \\n\\n circle_marker_d83497410bb9b2f1f419ec33a75d9410.bindPopup(popup_c8246d8288d0bbe1c17364f087557b1d)\\n ;\\n\\n \\n \\n \\n var circle_marker_9701db614556a7db76ba0abb3651cf21 = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b0dc7769fd3a66043e647704a2450e84);\\n \\n \\n var popup_163624c144f95da5119be2aa71e52b23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3af8bedf9b5f8361af9efbbe1e527cd3 = $(`<div id="html_3af8bedf9b5f8361af9efbbe1e527cd3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_163624c144f95da5119be2aa71e52b23.setContent(html_3af8bedf9b5f8361af9efbbe1e527cd3);\\n \\n \\n\\n circle_marker_9701db614556a7db76ba0abb3651cf21.bindPopup(popup_163624c144f95da5119be2aa71e52b23)\\n ;\\n\\n \\n \\n \\n var circle_marker_311e7648f377a94c6eb137987083e6ca = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b0dc7769fd3a66043e647704a2450e84);\\n \\n \\n var popup_8d44f7b48283496d272215e237c11e22 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ffb3d13f8d215efc59465b39cbc907af = $(`<div id="html_ffb3d13f8d215efc59465b39cbc907af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d44f7b48283496d272215e237c11e22.setContent(html_ffb3d13f8d215efc59465b39cbc907af);\\n \\n \\n\\n circle_marker_311e7648f377a94c6eb137987083e6ca.bindPopup(popup_8d44f7b48283496d272215e237c11e22)\\n ;\\n\\n \\n \\n \\n var circle_marker_239c82b30146ff4d81c3abedc3ae4439 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_b0dc7769fd3a66043e647704a2450e84);\\n \\n \\n var popup_e6d4559895c6c320351ff5c5fb42eba1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f3c2e8bce52207369bcdc05fe18b39c = $(`<div id="html_6f3c2e8bce52207369bcdc05fe18b39c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6d4559895c6c320351ff5c5fb42eba1.setContent(html_6f3c2e8bce52207369bcdc05fe18b39c);\\n \\n \\n\\n circle_marker_239c82b30146ff4d81c3abedc3ae4439.bindPopup(popup_e6d4559895c6c320351ff5c5fb42eba1)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Cherry, pin\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_cd9bf975a408d029bae39403882ab546 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_cd9bf975a408d029bae39403882ab546" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_cd9bf975a408d029bae39403882ab546 = L.map(\\n "map_cd9bf975a408d029bae39403882ab546",\\n {\\n center: [42.72551486726968, -73.27840173497155],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_0eb139f8033f4c59a4e59d0bb14446fe = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_cd9bf975a408d029bae39403882ab546);\\n \\n \\n var circle_marker_9965cd6dc8e0a196988dfab3e103fc90 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_cd9bf975a408d029bae39403882ab546);\\n \\n \\n var popup_d9d80b566e3293c096cb1543770d8c1d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bafa744c4089991378ba8b3443dbd8f2 = $(`<div id="html_bafa744c4089991378ba8b3443dbd8f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d9d80b566e3293c096cb1543770d8c1d.setContent(html_bafa744c4089991378ba8b3443dbd8f2);\\n \\n \\n\\n circle_marker_9965cd6dc8e0a196988dfab3e103fc90.bindPopup(popup_d9d80b566e3293c096cb1543770d8c1d)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Dogwood, alternate-leaved\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_afac8d3709152b5416dc89bd45e5b775 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_afac8d3709152b5416dc89bd45e5b775" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_afac8d3709152b5416dc89bd45e5b775 = L.map(\\n "map_afac8d3709152b5416dc89bd45e5b775",\\n {\\n center: [42.73003139060427, -73.25503090618253],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_06c164666c50bce185a33233202b228f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_afac8d3709152b5416dc89bd45e5b775);\\n \\n \\n var circle_marker_c1326cbe6dcfe58aaf6d42be57ebff25 = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_afac8d3709152b5416dc89bd45e5b775);\\n \\n \\n var popup_f809a24d5a36e60d75cc0135193565d0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_826507eeda0b644b727065048a1cab1f = $(`<div id="html_826507eeda0b644b727065048a1cab1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f809a24d5a36e60d75cc0135193565d0.setContent(html_826507eeda0b644b727065048a1cab1f);\\n \\n \\n\\n circle_marker_c1326cbe6dcfe58aaf6d42be57ebff25.bindPopup(popup_f809a24d5a36e60d75cc0135193565d0)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d5f411dcd1692eabb68e590714e7f10 = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_afac8d3709152b5416dc89bd45e5b775);\\n \\n \\n var popup_cf629a498e7af4e17cf338d31e531986 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_914d81a5f79a13b19772a920b062461f = $(`<div id="html_914d81a5f79a13b19772a920b062461f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf629a498e7af4e17cf338d31e531986.setContent(html_914d81a5f79a13b19772a920b062461f);\\n \\n \\n\\n circle_marker_3d5f411dcd1692eabb68e590714e7f10.bindPopup(popup_cf629a498e7af4e17cf338d31e531986)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Dogwood, gray\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_692f8ecef0676d81d0f13fcc8f3ce11a {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_692f8ecef0676d81d0f13fcc8f3ce11a" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_692f8ecef0676d81d0f13fcc8f3ce11a = L.map(\\n "map_692f8ecef0676d81d0f13fcc8f3ce11a",\\n {\\n center: [42.734546004747955, -73.23227338864854],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_82389d8bc18ec1e6719078fa6a0f5282 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_692f8ecef0676d81d0f13fcc8f3ce11a);\\n \\n \\n var circle_marker_f8b41d685d1a76bc4fc1d572a1f41181 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_692f8ecef0676d81d0f13fcc8f3ce11a);\\n \\n \\n var popup_94891b14ff7ed75c822c7e95cce406d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aca7dbf110745392368367fcdca374e1 = $(`<div id="html_aca7dbf110745392368367fcdca374e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94891b14ff7ed75c822c7e95cce406d7.setContent(html_aca7dbf110745392368367fcdca374e1);\\n \\n \\n\\n circle_marker_f8b41d685d1a76bc4fc1d572a1f41181.bindPopup(popup_94891b14ff7ed75c822c7e95cce406d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b153262e6973da1b7d8d1a45352ff50 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_692f8ecef0676d81d0f13fcc8f3ce11a);\\n \\n \\n var popup_a3a634938dd67bf0fc205419691dc4e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a544507f02e85a22cae6b3891e60a6fc = $(`<div id="html_a544507f02e85a22cae6b3891e60a6fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3a634938dd67bf0fc205419691dc4e4.setContent(html_a544507f02e85a22cae6b3891e60a6fc);\\n \\n \\n\\n circle_marker_3b153262e6973da1b7d8d1a45352ff50.bindPopup(popup_a3a634938dd67bf0fc205419691dc4e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f1d23629e53357eb1cce702ee6e01b2 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_692f8ecef0676d81d0f13fcc8f3ce11a);\\n \\n \\n var popup_9fb95293c3465419767dc530589dcac7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_91fd8b940e6af3e02a4d7a8db4a3b5a0 = $(`<div id="html_91fd8b940e6af3e02a4d7a8db4a3b5a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fb95293c3465419767dc530589dcac7.setContent(html_91fd8b940e6af3e02a4d7a8db4a3b5a0);\\n \\n \\n\\n circle_marker_2f1d23629e53357eb1cce702ee6e01b2.bindPopup(popup_9fb95293c3465419767dc530589dcac7)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa49b6d35776c5b58a553b3496f4b90b = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_692f8ecef0676d81d0f13fcc8f3ce11a);\\n \\n \\n var popup_0afde2bd8de720bf283ec79bd4f0ff34 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bec636240c70fdd062457ff2ad8cb7ad = $(`<div id="html_bec636240c70fdd062457ff2ad8cb7ad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0afde2bd8de720bf283ec79bd4f0ff34.setContent(html_bec636240c70fdd062457ff2ad8cb7ad);\\n \\n \\n\\n circle_marker_aa49b6d35776c5b58a553b3496f4b90b.bindPopup(popup_0afde2bd8de720bf283ec79bd4f0ff34)\\n ;\\n\\n \\n \\n \\n var circle_marker_8bc55728f5f6b40489404824b5103f38 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_692f8ecef0676d81d0f13fcc8f3ce11a);\\n \\n \\n var popup_ec1064744cb9665ceae29dd47fad7c6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_52b9239ce0c3813e9e1dd6791839a4cb = $(`<div id="html_52b9239ce0c3813e9e1dd6791839a4cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec1064744cb9665ceae29dd47fad7c6a.setContent(html_52b9239ce0c3813e9e1dd6791839a4cb);\\n \\n \\n\\n circle_marker_8bc55728f5f6b40489404824b5103f38.bindPopup(popup_ec1064744cb9665ceae29dd47fad7c6a)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Elderberry, red\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_751b11a0e6581d5ecfb9564fc0ed5c6e {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_751b11a0e6581d5ecfb9564fc0ed5c6e" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_751b11a0e6581d5ecfb9564fc0ed5c6e = L.map(\\n "map_751b11a0e6581d5ecfb9564fc0ed5c6e",\\n {\\n center: [42.73002630201289, -73.2242790050592],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_9cac4711f5ebf8f5c5fd5fa6dc30c524 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_751b11a0e6581d5ecfb9564fc0ed5c6e);\\n \\n \\n var circle_marker_48e66e832240c23cb5835b950978a28b = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_751b11a0e6581d5ecfb9564fc0ed5c6e);\\n \\n \\n var popup_d27515b0eff42b799f681fc73a8f20f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_93c73b9af9e19e23293f7326acff2132 = $(`<div id="html_93c73b9af9e19e23293f7326acff2132" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d27515b0eff42b799f681fc73a8f20f3.setContent(html_93c73b9af9e19e23293f7326acff2132);\\n \\n \\n\\n circle_marker_48e66e832240c23cb5835b950978a28b.bindPopup(popup_d27515b0eff42b799f681fc73a8f20f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fab49ebd364cab2e2741f7e132eaf8f = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_751b11a0e6581d5ecfb9564fc0ed5c6e);\\n \\n \\n var popup_60c1b273b3c5bb3f837bd2ac1117839f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b0fa39127a72736320c5c550a9ce2c7 = $(`<div id="html_6b0fa39127a72736320c5c550a9ce2c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_60c1b273b3c5bb3f837bd2ac1117839f.setContent(html_6b0fa39127a72736320c5c550a9ce2c7);\\n \\n \\n\\n circle_marker_3fab49ebd364cab2e2741f7e132eaf8f.bindPopup(popup_60c1b273b3c5bb3f837bd2ac1117839f)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Elm": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_b40872896c4f6f19d23843a9f8bf5f43 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_b40872896c4f6f19d23843a9f8bf5f43" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_b40872896c4f6f19d23843a9f8bf5f43 = L.map(\\n "map_b40872896c4f6f19d23843a9f8bf5f43",\\n {\\n center: [42.73183572636463, -73.23104182112704],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_78965b6e0f50da413c3e157db56268e9 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_b40872896c4f6f19d23843a9f8bf5f43);\\n \\n \\n var circle_marker_2b5ded98c9a7b270ae91e89312e651fa = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_b40872896c4f6f19d23843a9f8bf5f43);\\n \\n \\n var popup_e08fba8d85316bcad0dbcd0786b81859 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc413b1594f2ab5236827017b4aad1f1 = $(`<div id="html_bc413b1594f2ab5236827017b4aad1f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e08fba8d85316bcad0dbcd0786b81859.setContent(html_bc413b1594f2ab5236827017b4aad1f1);\\n \\n \\n\\n circle_marker_2b5ded98c9a7b270ae91e89312e651fa.bindPopup(popup_e08fba8d85316bcad0dbcd0786b81859)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6326e25f3dadf701565cb5469f31300 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_b40872896c4f6f19d23843a9f8bf5f43);\\n \\n \\n var popup_ebba50dcc1282fddcf7c1e427d6d6651 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48bf9579bdd1be0b3f4fc3c4ff2ed5db = $(`<div id="html_48bf9579bdd1be0b3f4fc3c4ff2ed5db" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebba50dcc1282fddcf7c1e427d6d6651.setContent(html_48bf9579bdd1be0b3f4fc3c4ff2ed5db);\\n \\n \\n\\n circle_marker_d6326e25f3dadf701565cb5469f31300.bindPopup(popup_ebba50dcc1282fddcf7c1e427d6d6651)\\n ;\\n\\n \\n \\n \\n var circle_marker_12e80a5dd6807738003bfb9a2b884e04 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b40872896c4f6f19d23843a9f8bf5f43);\\n \\n \\n var popup_dc5e4a59a38b835444e574d357c70acf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41417b59cdd704a64dc35432786bd0b9 = $(`<div id="html_41417b59cdd704a64dc35432786bd0b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc5e4a59a38b835444e574d357c70acf.setContent(html_41417b59cdd704a64dc35432786bd0b9);\\n \\n \\n\\n circle_marker_12e80a5dd6807738003bfb9a2b884e04.bindPopup(popup_dc5e4a59a38b835444e574d357c70acf)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4071eaafa39a1c9d08ac5ba2e6f7bc7 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_b40872896c4f6f19d23843a9f8bf5f43);\\n \\n \\n var popup_f75e66e0493509470fe877481ae8dad8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a21fdd9ecb7cb2bb9cae14a1d2f4c731 = $(`<div id="html_a21fdd9ecb7cb2bb9cae14a1d2f4c731" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f75e66e0493509470fe877481ae8dad8.setContent(html_a21fdd9ecb7cb2bb9cae14a1d2f4c731);\\n \\n \\n\\n circle_marker_a4071eaafa39a1c9d08ac5ba2e6f7bc7.bindPopup(popup_f75e66e0493509470fe877481ae8dad8)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a99354dc32a33c2b4b6bfde3c977ad7 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_b40872896c4f6f19d23843a9f8bf5f43);\\n \\n \\n var popup_66ebc47cf703ff662f91662c83afbd22 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_51fbdda64dc2e1dbf676bca7af7e13ab = $(`<div id="html_51fbdda64dc2e1dbf676bca7af7e13ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_66ebc47cf703ff662f91662c83afbd22.setContent(html_51fbdda64dc2e1dbf676bca7af7e13ab);\\n \\n \\n\\n circle_marker_3a99354dc32a33c2b4b6bfde3c977ad7.bindPopup(popup_66ebc47cf703ff662f91662c83afbd22)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Elm, American\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_24f35a98b352657854abbe774ba00d83 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_24f35a98b352657854abbe774ba00d83" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_24f35a98b352657854abbe774ba00d83 = L.map(\\n "map_24f35a98b352657854abbe774ba00d83",\\n {\\n center: [42.73183712183504, -73.23534980041066],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_2172baf4ac260a79ab43b3fed61f3b0a = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var circle_marker_154e1a6e7ac96ea4290421f62573744b = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_654936b164ec5a03ca2990c0275e420e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c2efd780d32b9ec34ea11c16c8e8c880 = $(`<div id="html_c2efd780d32b9ec34ea11c16c8e8c880" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_654936b164ec5a03ca2990c0275e420e.setContent(html_c2efd780d32b9ec34ea11c16c8e8c880);\\n \\n \\n\\n circle_marker_154e1a6e7ac96ea4290421f62573744b.bindPopup(popup_654936b164ec5a03ca2990c0275e420e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5570100369cd5d074fbe41cb7ed34cc = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_e8b6193aec48cfa6b6cc3b9cbde361aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_266b9fc1e3fb155f34cadd6036d5f349 = $(`<div id="html_266b9fc1e3fb155f34cadd6036d5f349" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8b6193aec48cfa6b6cc3b9cbde361aa.setContent(html_266b9fc1e3fb155f34cadd6036d5f349);\\n \\n \\n\\n circle_marker_a5570100369cd5d074fbe41cb7ed34cc.bindPopup(popup_e8b6193aec48cfa6b6cc3b9cbde361aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_0aab7e2efa6f3299b1b7873a54d859ae = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_375a5a2bf91674e8eb789b6730160b5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_27e5cfb06b5b7c3310b322fb745152cd = $(`<div id="html_27e5cfb06b5b7c3310b322fb745152cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_375a5a2bf91674e8eb789b6730160b5c.setContent(html_27e5cfb06b5b7c3310b322fb745152cd);\\n \\n \\n\\n circle_marker_0aab7e2efa6f3299b1b7873a54d859ae.bindPopup(popup_375a5a2bf91674e8eb789b6730160b5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_ae64c844f181ee1fc7a3bd91a332fe93 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_36a6b6226bc1c012f5fbaedbc8ce9707 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9f45c9b8c54fab44552941c5c3aa066 = $(`<div id="html_a9f45c9b8c54fab44552941c5c3aa066" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36a6b6226bc1c012f5fbaedbc8ce9707.setContent(html_a9f45c9b8c54fab44552941c5c3aa066);\\n \\n \\n\\n circle_marker_ae64c844f181ee1fc7a3bd91a332fe93.bindPopup(popup_36a6b6226bc1c012f5fbaedbc8ce9707)\\n ;\\n\\n \\n \\n \\n var circle_marker_aef6c60da406bab8b58d21f487a63b58 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_1f7ec85c36374463fbd34ad1acef9a0a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea518881c2d5bf05b93840c9cfc01fa5 = $(`<div id="html_ea518881c2d5bf05b93840c9cfc01fa5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f7ec85c36374463fbd34ad1acef9a0a.setContent(html_ea518881c2d5bf05b93840c9cfc01fa5);\\n \\n \\n\\n circle_marker_aef6c60da406bab8b58d21f487a63b58.bindPopup(popup_1f7ec85c36374463fbd34ad1acef9a0a)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b49334b35cfdb25a67d4db07978cf6c = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_a6023ecc3581110dfe7da9ff81943fcc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50fe5474b249b48f3e30637c8492bfa4 = $(`<div id="html_50fe5474b249b48f3e30637c8492bfa4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a6023ecc3581110dfe7da9ff81943fcc.setContent(html_50fe5474b249b48f3e30637c8492bfa4);\\n \\n \\n\\n circle_marker_4b49334b35cfdb25a67d4db07978cf6c.bindPopup(popup_a6023ecc3581110dfe7da9ff81943fcc)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d6f0b378355a405f4b7d842d0b861ef = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_b4873691ca44dd8bcdbcbe4b9926bda4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_601749e38229a4d3e899e96221eca73e = $(`<div id="html_601749e38229a4d3e899e96221eca73e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b4873691ca44dd8bcdbcbe4b9926bda4.setContent(html_601749e38229a4d3e899e96221eca73e);\\n \\n \\n\\n circle_marker_1d6f0b378355a405f4b7d842d0b861ef.bindPopup(popup_b4873691ca44dd8bcdbcbe4b9926bda4)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1284b45072241ab68f785f3a5300917 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_14ded92b223a7c3cac595728b5e5944a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c3d697b02eab71afb2b5ab27aa286f02 = $(`<div id="html_c3d697b02eab71afb2b5ab27aa286f02" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14ded92b223a7c3cac595728b5e5944a.setContent(html_c3d697b02eab71afb2b5ab27aa286f02);\\n \\n \\n\\n circle_marker_e1284b45072241ab68f785f3a5300917.bindPopup(popup_14ded92b223a7c3cac595728b5e5944a)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d23b9a8a1b78ea680ae536d11168182 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_4adba8319480c2b1021dd907673abb12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f039ff52ed3bf357728bed0abcb986a8 = $(`<div id="html_f039ff52ed3bf357728bed0abcb986a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4adba8319480c2b1021dd907673abb12.setContent(html_f039ff52ed3bf357728bed0abcb986a8);\\n \\n \\n\\n circle_marker_7d23b9a8a1b78ea680ae536d11168182.bindPopup(popup_4adba8319480c2b1021dd907673abb12)\\n ;\\n\\n \\n \\n \\n var circle_marker_36f9acfb387a1c81c2dd23c0990321b9 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_07b6b2a96d7c7fc47236437376588e9a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b29d9d829771021b6ded43e1669110f = $(`<div id="html_1b29d9d829771021b6ded43e1669110f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07b6b2a96d7c7fc47236437376588e9a.setContent(html_1b29d9d829771021b6ded43e1669110f);\\n \\n \\n\\n circle_marker_36f9acfb387a1c81c2dd23c0990321b9.bindPopup(popup_07b6b2a96d7c7fc47236437376588e9a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d3f95cc929efeb2bbe3e7fc52d6a02b = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_690d4119754b425bf1457c46d220a39c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3c075e54e2b6ebeef1dbcd8c80ba5e8 = $(`<div id="html_d3c075e54e2b6ebeef1dbcd8c80ba5e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_690d4119754b425bf1457c46d220a39c.setContent(html_d3c075e54e2b6ebeef1dbcd8c80ba5e8);\\n \\n \\n\\n circle_marker_8d3f95cc929efeb2bbe3e7fc52d6a02b.bindPopup(popup_690d4119754b425bf1457c46d220a39c)\\n ;\\n\\n \\n \\n \\n var circle_marker_d701bc9e5b60dab36ec4ab6662300799 = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_88029350daf2c171fe7a3fd443f4dc8a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_480487bbd651019afa26d66874a92012 = $(`<div id="html_480487bbd651019afa26d66874a92012" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88029350daf2c171fe7a3fd443f4dc8a.setContent(html_480487bbd651019afa26d66874a92012);\\n \\n \\n\\n circle_marker_d701bc9e5b60dab36ec4ab6662300799.bindPopup(popup_88029350daf2c171fe7a3fd443f4dc8a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6c1cb8733b24684517a734598c919fd = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_3cee1e76ce92e56f93c021e405597b82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_71ef6f237c41e37254d5f567f27d831e = $(`<div id="html_71ef6f237c41e37254d5f567f27d831e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3cee1e76ce92e56f93c021e405597b82.setContent(html_71ef6f237c41e37254d5f567f27d831e);\\n \\n \\n\\n circle_marker_f6c1cb8733b24684517a734598c919fd.bindPopup(popup_3cee1e76ce92e56f93c021e405597b82)\\n ;\\n\\n \\n \\n \\n var circle_marker_a65ff6ee833af45b4b329bf7d4a8722e = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_e583563a1111b73083f4af8a5dc3e6b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f5b0f71121cbc33b09c379cdd9b9994e = $(`<div id="html_f5b0f71121cbc33b09c379cdd9b9994e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e583563a1111b73083f4af8a5dc3e6b9.setContent(html_f5b0f71121cbc33b09c379cdd9b9994e);\\n \\n \\n\\n circle_marker_a65ff6ee833af45b4b329bf7d4a8722e.bindPopup(popup_e583563a1111b73083f4af8a5dc3e6b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_052bf28092942b7878792687bdcaace8 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_fe147e15e72c1feb909d5562d297cb4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db38bab56634b5400d75a39477cba803 = $(`<div id="html_db38bab56634b5400d75a39477cba803" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe147e15e72c1feb909d5562d297cb4e.setContent(html_db38bab56634b5400d75a39477cba803);\\n \\n \\n\\n circle_marker_052bf28092942b7878792687bdcaace8.bindPopup(popup_fe147e15e72c1feb909d5562d297cb4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_8eecbc734f70c17c61fc869f05be1269 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_8e207dd3eaac1783a1a935a393d3f0d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d0c71be53a24e180d070ff4e7d4a8775 = $(`<div id="html_d0c71be53a24e180d070ff4e7d4a8775" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e207dd3eaac1783a1a935a393d3f0d3.setContent(html_d0c71be53a24e180d070ff4e7d4a8775);\\n \\n \\n\\n circle_marker_8eecbc734f70c17c61fc869f05be1269.bindPopup(popup_8e207dd3eaac1783a1a935a393d3f0d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4ce69d669395b54ca389f233575b7db = L.circleMarker(\\n [42.73093113915656, -73.22796888742165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_1d06dde39f95ad7c6232f77f20775a4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c77fdc93d61ef87885e231ebe9afd01 = $(`<div id="html_2c77fdc93d61ef87885e231ebe9afd01" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d06dde39f95ad7c6232f77f20775a4c.setContent(html_2c77fdc93d61ef87885e231ebe9afd01);\\n \\n \\n\\n circle_marker_f4ce69d669395b54ca389f233575b7db.bindPopup(popup_1d06dde39f95ad7c6232f77f20775a4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_01e514938cd8020e0f45ef115a5739fe = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_aeb0ec95876f07ec7dc430e46722f1a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bcb11b8d43bb093a5de87f01cfc581a9 = $(`<div id="html_bcb11b8d43bb093a5de87f01cfc581a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aeb0ec95876f07ec7dc430e46722f1a0.setContent(html_bcb11b8d43bb093a5de87f01cfc581a9);\\n \\n \\n\\n circle_marker_01e514938cd8020e0f45ef115a5739fe.bindPopup(popup_aeb0ec95876f07ec7dc430e46722f1a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc2a3d438adc3d3bac44347e5f86015d = L.circleMarker(\\n [42.73093032286755, -73.22550869120535],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_f16de566c902dbfb058badd1f3ead5cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1883df44d0bdfb2d33233a7df22f2d2f = $(`<div id="html_1883df44d0bdfb2d33233a7df22f2d2f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f16de566c902dbfb058badd1f3ead5cd.setContent(html_1883df44d0bdfb2d33233a7df22f2d2f);\\n \\n \\n\\n circle_marker_fc2a3d438adc3d3bac44347e5f86015d.bindPopup(popup_f16de566c902dbfb058badd1f3ead5cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_68696fed4623f6bf80ed984f8a212368 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_11a93aea71725802735ac1d81d89c245 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aefb530c873438ab50e5ef237423b6be = $(`<div id="html_aefb530c873438ab50e5ef237423b6be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11a93aea71725802735ac1d81d89c245.setContent(html_aefb530c873438ab50e5ef237423b6be);\\n \\n \\n\\n circle_marker_68696fed4623f6bf80ed984f8a212368.bindPopup(popup_11a93aea71725802735ac1d81d89c245)\\n ;\\n\\n \\n \\n \\n var circle_marker_187b19bf30aa2d023a1b9639a0d2be51 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_0a37cfbe280462219ba28ba48afdc7f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c75d9c2d7551579c6839fb38fd1914a = $(`<div id="html_0c75d9c2d7551579c6839fb38fd1914a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a37cfbe280462219ba28ba48afdc7f4.setContent(html_0c75d9c2d7551579c6839fb38fd1914a);\\n \\n \\n\\n circle_marker_187b19bf30aa2d023a1b9639a0d2be51.bindPopup(popup_0a37cfbe280462219ba28ba48afdc7f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f17092387cf6b8f078948879fc2c87a = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_1aeb2837930b7d5403b6266b46b3e628 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd6bf0b105c40fb8105f1bcb6d574e94 = $(`<div id="html_cd6bf0b105c40fb8105f1bcb6d574e94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1aeb2837930b7d5403b6266b46b3e628.setContent(html_cd6bf0b105c40fb8105f1bcb6d574e94);\\n \\n \\n\\n circle_marker_3f17092387cf6b8f078948879fc2c87a.bindPopup(popup_1aeb2837930b7d5403b6266b46b3e628)\\n ;\\n\\n \\n \\n \\n var circle_marker_5dd4a4cb9004c1b7f6aaab89e50cdcac = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_269e149ab642ad0facf5de0b150b35ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e9f3f14b32127610df7c4979086881ef = $(`<div id="html_e9f3f14b32127610df7c4979086881ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_269e149ab642ad0facf5de0b150b35ef.setContent(html_e9f3f14b32127610df7c4979086881ef);\\n \\n \\n\\n circle_marker_5dd4a4cb9004c1b7f6aaab89e50cdcac.bindPopup(popup_269e149ab642ad0facf5de0b150b35ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_ddb29f3433d1a76d8d6190738ffecfc2 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_a390351a8a05ad3113f09155e87bccf7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9484f08e84708bc47656e0119cc69606 = $(`<div id="html_9484f08e84708bc47656e0119cc69606" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a390351a8a05ad3113f09155e87bccf7.setContent(html_9484f08e84708bc47656e0119cc69606);\\n \\n \\n\\n circle_marker_ddb29f3433d1a76d8d6190738ffecfc2.bindPopup(popup_a390351a8a05ad3113f09155e87bccf7)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8173d724da7c2fa309a44376464cf4f = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_2955ca6c20a04b24d68beff05f658bb5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_535aaa82072ea527d90fc821f8bdb5e3 = $(`<div id="html_535aaa82072ea527d90fc821f8bdb5e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2955ca6c20a04b24d68beff05f658bb5.setContent(html_535aaa82072ea527d90fc821f8bdb5e3);\\n \\n \\n\\n circle_marker_e8173d724da7c2fa309a44376464cf4f.bindPopup(popup_2955ca6c20a04b24d68beff05f658bb5)\\n ;\\n\\n \\n \\n \\n var circle_marker_aeba87c166fb81a3f62fcc77a51541a2 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_1984fe73c4cf2afad72d030850259ca5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a47885c0c04327c776e39a53ea4cb68 = $(`<div id="html_4a47885c0c04327c776e39a53ea4cb68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1984fe73c4cf2afad72d030850259ca5.setContent(html_4a47885c0c04327c776e39a53ea4cb68);\\n \\n \\n\\n circle_marker_aeba87c166fb81a3f62fcc77a51541a2.bindPopup(popup_1984fe73c4cf2afad72d030850259ca5)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d1df41a497ffc66dc8ae40df970edce = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_24d8ddcb5169c6219770a43ff1bcac30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_20f3cf9edd3675d65ea1904664d62fbb = $(`<div id="html_20f3cf9edd3675d65ea1904664d62fbb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24d8ddcb5169c6219770a43ff1bcac30.setContent(html_20f3cf9edd3675d65ea1904664d62fbb);\\n \\n \\n\\n circle_marker_8d1df41a497ffc66dc8ae40df970edce.bindPopup(popup_24d8ddcb5169c6219770a43ff1bcac30)\\n ;\\n\\n \\n \\n \\n var circle_marker_816fb2a8f52afcadf79c5cbba587882e = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_bfdee672680a6617f9b4e4d4948edf85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_59a40046163378fb776f522bdbfec21d = $(`<div id="html_59a40046163378fb776f522bdbfec21d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfdee672680a6617f9b4e4d4948edf85.setContent(html_59a40046163378fb776f522bdbfec21d);\\n \\n \\n\\n circle_marker_816fb2a8f52afcadf79c5cbba587882e.bindPopup(popup_bfdee672680a6617f9b4e4d4948edf85)\\n ;\\n\\n \\n \\n \\n var circle_marker_405b65eb05ebba06b518e2c5393132e2 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_e2eb8450c991c7d76717e2e58fd364e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_317beba1f08d69016ae98bd796661a05 = $(`<div id="html_317beba1f08d69016ae98bd796661a05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2eb8450c991c7d76717e2e58fd364e7.setContent(html_317beba1f08d69016ae98bd796661a05);\\n \\n \\n\\n circle_marker_405b65eb05ebba06b518e2c5393132e2.bindPopup(popup_e2eb8450c991c7d76717e2e58fd364e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_f30147815707638ce42e44cb2f89d88e = L.circleMarker(\\n [42.72731562880686, -73.22428095863906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_18f66bc3585f2c027519b958ee818b71 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d490957b504e170c9c1b5ddd775a4edb = $(`<div id="html_d490957b504e170c9c1b5ddd775a4edb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_18f66bc3585f2c027519b958ee818b71.setContent(html_d490957b504e170c9c1b5ddd775a4edb);\\n \\n \\n\\n circle_marker_f30147815707638ce42e44cb2f89d88e.bindPopup(popup_18f66bc3585f2c027519b958ee818b71)\\n ;\\n\\n \\n \\n \\n var circle_marker_a180b5ea33e9e9c9d220c9f87af4be5a = L.circleMarker(\\n [42.72731518778323, -73.22305093225523],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_8609176dc417cbd4d850ace9dae8d584 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3f1aef88c5bf08fd0d1ec9cf21eb737 = $(`<div id="html_d3f1aef88c5bf08fd0d1ec9cf21eb737" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8609176dc417cbd4d850ace9dae8d584.setContent(html_d3f1aef88c5bf08fd0d1ec9cf21eb737);\\n \\n \\n\\n circle_marker_a180b5ea33e9e9c9d220c9f87af4be5a.bindPopup(popup_8609176dc417cbd4d850ace9dae8d584)\\n ;\\n\\n \\n \\n \\n var circle_marker_9c1a7c8f776b5ee9f6b0901ba5fd2401 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_62e45c2214ca49c5da133040894aca49 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0971dbd5a7f6a70b5d8cbdfdd009ca26 = $(`<div id="html_0971dbd5a7f6a70b5d8cbdfdd009ca26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62e45c2214ca49c5da133040894aca49.setContent(html_0971dbd5a7f6a70b5d8cbdfdd009ca26);\\n \\n \\n\\n circle_marker_9c1a7c8f776b5ee9f6b0901ba5fd2401.bindPopup(popup_62e45c2214ca49c5da133040894aca49)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f301fa20e474466dbc890816abf8b7a = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_204376bc3898bd78b084c3575b300bb9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c06b07609f2f7ec5c97be407522b859d = $(`<div id="html_c06b07609f2f7ec5c97be407522b859d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_204376bc3898bd78b084c3575b300bb9.setContent(html_c06b07609f2f7ec5c97be407522b859d);\\n \\n \\n\\n circle_marker_8f301fa20e474466dbc890816abf8b7a.bindPopup(popup_204376bc3898bd78b084c3575b300bb9)\\n ;\\n\\n \\n \\n \\n var circle_marker_0340dda6910907a5cd0b157eb34fa284 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_e04703eea6845c8c894f8eb80e4cee5b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97026adc7a0f7167fbac2c038c57dc20 = $(`<div id="html_97026adc7a0f7167fbac2c038c57dc20" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e04703eea6845c8c894f8eb80e4cee5b.setContent(html_97026adc7a0f7167fbac2c038c57dc20);\\n \\n \\n\\n circle_marker_0340dda6910907a5cd0b157eb34fa284.bindPopup(popup_e04703eea6845c8c894f8eb80e4cee5b)\\n ;\\n\\n \\n \\n \\n var circle_marker_74496c2641627cc418aadff89029c0f5 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_24f35a98b352657854abbe774ba00d83);\\n \\n \\n var popup_f33eedfd45a8c16cb3ee6ac43c17d97b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0bfcdc2c5685ce6abc01b72742e270e6 = $(`<div id="html_0bfcdc2c5685ce6abc01b72742e270e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f33eedfd45a8c16cb3ee6ac43c17d97b.setContent(html_0bfcdc2c5685ce6abc01b72742e270e6);\\n \\n \\n\\n circle_marker_74496c2641627cc418aadff89029c0f5.bindPopup(popup_f33eedfd45a8c16cb3ee6ac43c17d97b)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Grape": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_cd6eb7135114287f1222881bc5b4b707 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_cd6eb7135114287f1222881bc5b4b707" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_cd6eb7135114287f1222881bc5b4b707 = L.map(\\n "map_cd6eb7135114287f1222881bc5b4b707",\\n {\\n center: [42.73183615105263, -73.23288852819861],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_c250b87b1a04682dabfa12474a7a0f5d = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var circle_marker_11b388845338898bfc1bf170bcc81a62 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_9d06ff1edf8b24a2b7c82f5835ec336e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0020f80a705f4014c274d610d2ad69b7 = $(`<div id="html_0020f80a705f4014c274d610d2ad69b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d06ff1edf8b24a2b7c82f5835ec336e.setContent(html_0020f80a705f4014c274d610d2ad69b7);\\n \\n \\n\\n circle_marker_11b388845338898bfc1bf170bcc81a62.bindPopup(popup_9d06ff1edf8b24a2b7c82f5835ec336e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8d66e78d6059abb77a82baafbdc8eb2 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_51257cbc6d044665a5380e4eef992d87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_154fd40e37e285ae38aca49e7b533daf = $(`<div id="html_154fd40e37e285ae38aca49e7b533daf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51257cbc6d044665a5380e4eef992d87.setContent(html_154fd40e37e285ae38aca49e7b533daf);\\n \\n \\n\\n circle_marker_e8d66e78d6059abb77a82baafbdc8eb2.bindPopup(popup_51257cbc6d044665a5380e4eef992d87)\\n ;\\n\\n \\n \\n \\n var circle_marker_ebdbde202d77f82bc1c78a7cd6efea73 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_6f8d119224c44dc2f7b9c297563b2d70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53b4404d60d7a26c0258d2e00b76b48b = $(`<div id="html_53b4404d60d7a26c0258d2e00b76b48b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f8d119224c44dc2f7b9c297563b2d70.setContent(html_53b4404d60d7a26c0258d2e00b76b48b);\\n \\n \\n\\n circle_marker_ebdbde202d77f82bc1c78a7cd6efea73.bindPopup(popup_6f8d119224c44dc2f7b9c297563b2d70)\\n ;\\n\\n \\n \\n \\n var circle_marker_abfde05e6263164f9de575715ba07a70 = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_0808a60de1e7a79d1c2c7ab6007e303d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9b6cbb202b859d04fbaf59a8029132c = $(`<div id="html_d9b6cbb202b859d04fbaf59a8029132c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0808a60de1e7a79d1c2c7ab6007e303d.setContent(html_d9b6cbb202b859d04fbaf59a8029132c);\\n \\n \\n\\n circle_marker_abfde05e6263164f9de575715ba07a70.bindPopup(popup_0808a60de1e7a79d1c2c7ab6007e303d)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d9913838add6bb91b7d18f3eae6c0b9 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_065e7639b9a9fba7dbfbf8f6fc13fe54 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b331f50a9dfd54efb7352c39b5804d30 = $(`<div id="html_b331f50a9dfd54efb7352c39b5804d30" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_065e7639b9a9fba7dbfbf8f6fc13fe54.setContent(html_b331f50a9dfd54efb7352c39b5804d30);\\n \\n \\n\\n circle_marker_4d9913838add6bb91b7d18f3eae6c0b9.bindPopup(popup_065e7639b9a9fba7dbfbf8f6fc13fe54)\\n ;\\n\\n \\n \\n \\n var circle_marker_065aa03e97f30d616d65ba30b12669b8 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.140011726956917, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_96b075cc61ffd9b6935d4024a2c0adc5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78e7668327aca0ce472b97d99a7bbb8c = $(`<div id="html_78e7668327aca0ce472b97d99a7bbb8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96b075cc61ffd9b6935d4024a2c0adc5.setContent(html_78e7668327aca0ce472b97d99a7bbb8c);\\n \\n \\n\\n circle_marker_065aa03e97f30d616d65ba30b12669b8.bindPopup(popup_96b075cc61ffd9b6935d4024a2c0adc5)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9bdecaf9180ed757511fcfb0581fa2b = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.8678889139475245, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_521c470fb4c24d1bc182b7adb6be6da4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f529ed360d5f59fe5eb31599fe1298e = $(`<div id="html_8f529ed360d5f59fe5eb31599fe1298e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_521c470fb4c24d1bc182b7adb6be6da4.setContent(html_8f529ed360d5f59fe5eb31599fe1298e);\\n \\n \\n\\n circle_marker_e9bdecaf9180ed757511fcfb0581fa2b.bindPopup(popup_521c470fb4c24d1bc182b7adb6be6da4)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0f5226ed5c372ed0d7cd2fd2c93995f = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_ac4921be1f748372792f3ddad622f74e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a7e91249cd03ec83b98b71b5352e2a0 = $(`<div id="html_1a7e91249cd03ec83b98b71b5352e2a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac4921be1f748372792f3ddad622f74e.setContent(html_1a7e91249cd03ec83b98b71b5352e2a0);\\n \\n \\n\\n circle_marker_c0f5226ed5c372ed0d7cd2fd2c93995f.bindPopup(popup_ac4921be1f748372792f3ddad622f74e)\\n ;\\n\\n \\n \\n \\n var circle_marker_71210ddb8a2256b96e5301c633263680 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_bcfb1e9d2926ae64e3893d8b7d023049 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ef69b41e218ddf7679414635fbc097ed = $(`<div id="html_ef69b41e218ddf7679414635fbc097ed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bcfb1e9d2926ae64e3893d8b7d023049.setContent(html_ef69b41e218ddf7679414635fbc097ed);\\n \\n \\n\\n circle_marker_71210ddb8a2256b96e5301c633263680.bindPopup(popup_bcfb1e9d2926ae64e3893d8b7d023049)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b74fb7e8c910a5fdd90922b916ecb8e = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_b54153f496aee7240e4f5b275ae1353e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_470800248a69fff34f3d21f1a8cd9c05 = $(`<div id="html_470800248a69fff34f3d21f1a8cd9c05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b54153f496aee7240e4f5b275ae1353e.setContent(html_470800248a69fff34f3d21f1a8cd9c05);\\n \\n \\n\\n circle_marker_4b74fb7e8c910a5fdd90922b916ecb8e.bindPopup(popup_b54153f496aee7240e4f5b275ae1353e)\\n ;\\n\\n \\n \\n \\n var circle_marker_12992b2f340a8f9cc1d525226f237c00 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_06dc4159feb5b1a74c662bca391ab884 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f407bc4626ce3062e6db415a043bb6ab = $(`<div id="html_f407bc4626ce3062e6db415a043bb6ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06dc4159feb5b1a74c662bca391ab884.setContent(html_f407bc4626ce3062e6db415a043bb6ab);\\n \\n \\n\\n circle_marker_12992b2f340a8f9cc1d525226f237c00.bindPopup(popup_06dc4159feb5b1a74c662bca391ab884)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c0214075ce634c23ee7fc762bb291a5 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_c6949e44c47da930284fb3dd35bd9770 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b3eb6f47eacd4ba9b8d74ae66ea815f2 = $(`<div id="html_b3eb6f47eacd4ba9b8d74ae66ea815f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6949e44c47da930284fb3dd35bd9770.setContent(html_b3eb6f47eacd4ba9b8d74ae66ea815f2);\\n \\n \\n\\n circle_marker_7c0214075ce634c23ee7fc762bb291a5.bindPopup(popup_c6949e44c47da930284fb3dd35bd9770)\\n ;\\n\\n \\n \\n \\n var circle_marker_db63c7767bd9d31ed2f83ae2b9f35262 = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_a451cad1db156e4953d656b8a98b7f20 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c76f53d4290393701a297c2782e70828 = $(`<div id="html_c76f53d4290393701a297c2782e70828" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a451cad1db156e4953d656b8a98b7f20.setContent(html_c76f53d4290393701a297c2782e70828);\\n \\n \\n\\n circle_marker_db63c7767bd9d31ed2f83ae2b9f35262.bindPopup(popup_a451cad1db156e4953d656b8a98b7f20)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d4a980f2d54c76b7e0164050f52baba = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_e9e896bb830f446088028c3f4e460847 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0645bfab18e7edafa2afed811d090964 = $(`<div id="html_0645bfab18e7edafa2afed811d090964" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9e896bb830f446088028c3f4e460847.setContent(html_0645bfab18e7edafa2afed811d090964);\\n \\n \\n\\n circle_marker_4d4a980f2d54c76b7e0164050f52baba.bindPopup(popup_e9e896bb830f446088028c3f4e460847)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d2d7961a2d7d12a404210d9d415dc4a = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.886025119029199, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_e205a65e66c986f23528bf46f2193e30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9075ac4688be982c93d328befdfdbd44 = $(`<div id="html_9075ac4688be982c93d328befdfdbd44" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e205a65e66c986f23528bf46f2193e30.setContent(html_9075ac4688be982c93d328befdfdbd44);\\n \\n \\n\\n circle_marker_9d2d7961a2d7d12a404210d9d415dc4a.bindPopup(popup_e205a65e66c986f23528bf46f2193e30)\\n ;\\n\\n \\n \\n \\n var circle_marker_d4daf3061eb844dd7fe6534ce6f244ed = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_a11e5c5390f999348adc2cdfa680468d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44114aa66cc7695200df6eff9a1f5f68 = $(`<div id="html_44114aa66cc7695200df6eff9a1f5f68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a11e5c5390f999348adc2cdfa680468d.setContent(html_44114aa66cc7695200df6eff9a1f5f68);\\n \\n \\n\\n circle_marker_d4daf3061eb844dd7fe6534ce6f244ed.bindPopup(popup_a11e5c5390f999348adc2cdfa680468d)\\n ;\\n\\n \\n \\n \\n var circle_marker_2076bcdbdc1d9b317a2a5caca2c7632e = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_3cfe0dd271b29048bb6f84798c029b9a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ec28bc97356edb9f86d79610f98c94b = $(`<div id="html_7ec28bc97356edb9f86d79610f98c94b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3cfe0dd271b29048bb6f84798c029b9a.setContent(html_7ec28bc97356edb9f86d79610f98c94b);\\n \\n \\n\\n circle_marker_2076bcdbdc1d9b317a2a5caca2c7632e.bindPopup(popup_3cfe0dd271b29048bb6f84798c029b9a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f2e62460dc7fc46daa8e2ecf44aae47 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_05d1fa0f444c02e3cab90cebbedaeb23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e926565ded868b575a179d12384b3e1b = $(`<div id="html_e926565ded868b575a179d12384b3e1b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05d1fa0f444c02e3cab90cebbedaeb23.setContent(html_e926565ded868b575a179d12384b3e1b);\\n \\n \\n\\n circle_marker_5f2e62460dc7fc46daa8e2ecf44aae47.bindPopup(popup_05d1fa0f444c02e3cab90cebbedaeb23)\\n ;\\n\\n \\n \\n \\n var circle_marker_69ed6b5ba8c8e952c129ec0a25632b8b = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_23d11b1e94b442d9e0e1ca50a5bbfaab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04f5a4f7a56962c9dd47dea1c3300f6b = $(`<div id="html_04f5a4f7a56962c9dd47dea1c3300f6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23d11b1e94b442d9e0e1ca50a5bbfaab.setContent(html_04f5a4f7a56962c9dd47dea1c3300f6b);\\n \\n \\n\\n circle_marker_69ed6b5ba8c8e952c129ec0a25632b8b.bindPopup(popup_23d11b1e94b442d9e0e1ca50a5bbfaab)\\n ;\\n\\n \\n \\n \\n var circle_marker_d8bfbb1fd1d5a8cafac1370f174261fd = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_ce37e332ef18e94c6529fca04f8247ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b87e536ceb3460bb2eef4fd595ac2ede = $(`<div id="html_b87e536ceb3460bb2eef4fd595ac2ede" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce37e332ef18e94c6529fca04f8247ff.setContent(html_b87e536ceb3460bb2eef4fd595ac2ede);\\n \\n \\n\\n circle_marker_d8bfbb1fd1d5a8cafac1370f174261fd.bindPopup(popup_ce37e332ef18e94c6529fca04f8247ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e5c4240fbc01a084efb3633e258e576 = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_2bea3f07538bd4c8850289adaae92d5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_699115afa3fb17721313080908be48b6 = $(`<div id="html_699115afa3fb17721313080908be48b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2bea3f07538bd4c8850289adaae92d5a.setContent(html_699115afa3fb17721313080908be48b6);\\n \\n \\n\\n circle_marker_7e5c4240fbc01a084efb3633e258e576.bindPopup(popup_2bea3f07538bd4c8850289adaae92d5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5def74a29bc2413dfe37e94ca27281e = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_aef6d77e45f381a09cbdf217b38a86b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da1f74c7ca5e1660c16ddc2d49317b3f = $(`<div id="html_da1f74c7ca5e1660c16ddc2d49317b3f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aef6d77e45f381a09cbdf217b38a86b3.setContent(html_da1f74c7ca5e1660c16ddc2d49317b3f);\\n \\n \\n\\n circle_marker_d5def74a29bc2413dfe37e94ca27281e.bindPopup(popup_aef6d77e45f381a09cbdf217b38a86b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_36f6ea62a28f0f8deb815a86057eacad = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_f13dfa44747dd0adce20fe76039c0576 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_23869ab272a6faa59978718dc48524af = $(`<div id="html_23869ab272a6faa59978718dc48524af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f13dfa44747dd0adce20fe76039c0576.setContent(html_23869ab272a6faa59978718dc48524af);\\n \\n \\n\\n circle_marker_36f6ea62a28f0f8deb815a86057eacad.bindPopup(popup_f13dfa44747dd0adce20fe76039c0576)\\n ;\\n\\n \\n \\n \\n var circle_marker_c2df55df32ec143b52a80a375bf32db7 = L.circleMarker(\\n [42.73635085311189, -73.22304483851624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_e2b74499462e623920443bb4d2353698 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7fe8e162932a373f0cf7a43763ad206 = $(`<div id="html_f7fe8e162932a373f0cf7a43763ad206" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2b74499462e623920443bb4d2353698.setContent(html_f7fe8e162932a373f0cf7a43763ad206);\\n \\n \\n\\n circle_marker_c2df55df32ec143b52a80a375bf32db7.bindPopup(popup_e2b74499462e623920443bb4d2353698)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb7e6e551cea30f182994e048f541040 = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_a1e3aaa69f415f485a56a72fd2cbb82a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2b8bfcbebe3662151f3587093fa6b6a = $(`<div id="html_b2b8bfcbebe3662151f3587093fa6b6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a1e3aaa69f415f485a56a72fd2cbb82a.setContent(html_b2b8bfcbebe3662151f3587093fa6b6a);\\n \\n \\n\\n circle_marker_fb7e6e551cea30f182994e048f541040.bindPopup(popup_a1e3aaa69f415f485a56a72fd2cbb82a)\\n ;\\n\\n \\n \\n \\n var circle_marker_df2a62fe6894023f0782ecd2d0968e8d = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_8aa6d0f35a6f6b2eeaf0fb377a29cf31 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6baefad6806f20b5de92cb027d6dd115 = $(`<div id="html_6baefad6806f20b5de92cb027d6dd115" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8aa6d0f35a6f6b2eeaf0fb377a29cf31.setContent(html_6baefad6806f20b5de92cb027d6dd115);\\n \\n \\n\\n circle_marker_df2a62fe6894023f0782ecd2d0968e8d.bindPopup(popup_8aa6d0f35a6f6b2eeaf0fb377a29cf31)\\n ;\\n\\n \\n \\n \\n var circle_marker_95611fca55b4d3debd699e8a62830a51 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_95f12181c5ff55f2b39df3916bb90d82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b1f755bba54e9a4236bc6252b08e549 = $(`<div id="html_1b1f755bba54e9a4236bc6252b08e549" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95f12181c5ff55f2b39df3916bb90d82.setContent(html_1b1f755bba54e9a4236bc6252b08e549);\\n \\n \\n\\n circle_marker_95611fca55b4d3debd699e8a62830a51.bindPopup(popup_95f12181c5ff55f2b39df3916bb90d82)\\n ;\\n\\n \\n \\n \\n var circle_marker_7797c7a2ab04549856ddf95baa9f9fe2 = L.circleMarker(\\n [42.7345450038305, -73.22673656679281],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_d239ef1391fbeb921f08ecd7b32e4514 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df5162aab28a8145422b2b8246ef5316 = $(`<div id="html_df5162aab28a8145422b2b8246ef5316" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d239ef1391fbeb921f08ecd7b32e4514.setContent(html_df5162aab28a8145422b2b8246ef5316);\\n \\n \\n\\n circle_marker_7797c7a2ab04549856ddf95baa9f9fe2.bindPopup(popup_d239ef1391fbeb921f08ecd7b32e4514)\\n ;\\n\\n \\n \\n \\n var circle_marker_8685a2a0665a3578c9e890a4a3cb3781 = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_00a4cc8e32ee294df8e8331a5229c9c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_335a5a6b1616d1970e5299a72210dbd4 = $(`<div id="html_335a5a6b1616d1970e5299a72210dbd4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00a4cc8e32ee294df8e8331a5229c9c4.setContent(html_335a5a6b1616d1970e5299a72210dbd4);\\n \\n \\n\\n circle_marker_8685a2a0665a3578c9e890a4a3cb3781.bindPopup(popup_00a4cc8e32ee294df8e8331a5229c9c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_2aeafc3d9ff9f836ece2d178e64b09e9 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_16fc6f7e7dae99243a07ae01b41176f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10fb79dc3d10cfa1f1e66c5cbe2906ee = $(`<div id="html_10fb79dc3d10cfa1f1e66c5cbe2906ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16fc6f7e7dae99243a07ae01b41176f3.setContent(html_10fb79dc3d10cfa1f1e66c5cbe2906ee);\\n \\n \\n\\n circle_marker_2aeafc3d9ff9f836ece2d178e64b09e9.bindPopup(popup_16fc6f7e7dae99243a07ae01b41176f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_37ab36161f67e5a6d2baba0c26fd4e7e = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.282549314314218, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_5cbdef5c3f5589405653547158bc45cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da37ede1cf10305e26688fd635087d3a = $(`<div id="html_da37ede1cf10305e26688fd635087d3a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5cbdef5c3f5589405653547158bc45cf.setContent(html_da37ede1cf10305e26688fd635087d3a);\\n \\n \\n\\n circle_marker_37ab36161f67e5a6d2baba0c26fd4e7e.bindPopup(popup_5cbdef5c3f5589405653547158bc45cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_84f262635f13a6490461f1d5cb7dd1f9 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_cf9f2691da33dfda1663ad76f9e615bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dde201389484bb83877d9b838fc9c991 = $(`<div id="html_dde201389484bb83877d9b838fc9c991" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf9f2691da33dfda1663ad76f9e615bd.setContent(html_dde201389484bb83877d9b838fc9c991);\\n \\n \\n\\n circle_marker_84f262635f13a6490461f1d5cb7dd1f9.bindPopup(popup_cf9f2691da33dfda1663ad76f9e615bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_b2c265ee9aefa9a6801dcada2e87df98 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_b1122c5a6fba39141d66b78ab2c6363d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07d08b78956acf07ec1d5ddca5f87fdc = $(`<div id="html_07d08b78956acf07ec1d5ddca5f87fdc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1122c5a6fba39141d66b78ab2c6363d.setContent(html_07d08b78956acf07ec1d5ddca5f87fdc);\\n \\n \\n\\n circle_marker_b2c265ee9aefa9a6801dcada2e87df98.bindPopup(popup_b1122c5a6fba39141d66b78ab2c6363d)\\n ;\\n\\n \\n \\n \\n var circle_marker_98261f91a1f18efee6cc55c75bd249ad = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_3bc2222e4745ec59276c31252d181b4a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ddd1f15e837e85a643eedaed968a5910 = $(`<div id="html_ddd1f15e837e85a643eedaed968a5910" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3bc2222e4745ec59276c31252d181b4a.setContent(html_ddd1f15e837e85a643eedaed968a5910);\\n \\n \\n\\n circle_marker_98261f91a1f18efee6cc55c75bd249ad.bindPopup(popup_3bc2222e4745ec59276c31252d181b4a)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d70a473660050d85d0444898a453d2b = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_ef29639bfb398dd6a5da819faf92e538 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ac85257f0901fe07dfd6d49707a403a = $(`<div id="html_4ac85257f0901fe07dfd6d49707a403a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef29639bfb398dd6a5da819faf92e538.setContent(html_4ac85257f0901fe07dfd6d49707a403a);\\n \\n \\n\\n circle_marker_7d70a473660050d85d0444898a453d2b.bindPopup(popup_ef29639bfb398dd6a5da819faf92e538)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb3b6dfd0bc4a734e6b3ae256855f07b = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_abf87def9698ad7d75302c73b576ffc2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_897bdb2d8149c85ef008fd6b4277d806 = $(`<div id="html_897bdb2d8149c85ef008fd6b4277d806" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_abf87def9698ad7d75302c73b576ffc2.setContent(html_897bdb2d8149c85ef008fd6b4277d806);\\n \\n \\n\\n circle_marker_eb3b6dfd0bc4a734e6b3ae256855f07b.bindPopup(popup_abf87def9698ad7d75302c73b576ffc2)\\n ;\\n\\n \\n \\n \\n var circle_marker_b200e7e0def87ae8dcce937d6a90ca44 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_e97a4dbc7683666d266ecccb78494bad = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a5edbc2f74a014e00b18a651ad60a22 = $(`<div id="html_4a5edbc2f74a014e00b18a651ad60a22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e97a4dbc7683666d266ecccb78494bad.setContent(html_4a5edbc2f74a014e00b18a651ad60a22);\\n \\n \\n\\n circle_marker_b200e7e0def87ae8dcce937d6a90ca44.bindPopup(popup_e97a4dbc7683666d266ecccb78494bad)\\n ;\\n\\n \\n \\n \\n var circle_marker_4f45dc8a61de3078bafd2bd779df20b1 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_a8c81fe22664243ebf005cf563770284 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6e0f40d2f8dde92fe3ad43fafb4819d = $(`<div id="html_a6e0f40d2f8dde92fe3ad43fafb4819d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8c81fe22664243ebf005cf563770284.setContent(html_a6e0f40d2f8dde92fe3ad43fafb4819d);\\n \\n \\n\\n circle_marker_4f45dc8a61de3078bafd2bd779df20b1.bindPopup(popup_a8c81fe22664243ebf005cf563770284)\\n ;\\n\\n \\n \\n \\n var circle_marker_31768a005463850727c52fd4c026b790 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_146224f33d410c414858f420fb851618 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e842394345bba204b726c77193c5cbd8 = $(`<div id="html_e842394345bba204b726c77193c5cbd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_146224f33d410c414858f420fb851618.setContent(html_e842394345bba204b726c77193c5cbd8);\\n \\n \\n\\n circle_marker_31768a005463850727c52fd4c026b790.bindPopup(popup_146224f33d410c414858f420fb851618)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf006cfcb36b1db90be34164d8f0d0ff = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_5ced52be8ab01704f0bdfb61f64e88f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e081ade1fa813a4e7a7dbf74ff398a1e = $(`<div id="html_e081ade1fa813a4e7a7dbf74ff398a1e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ced52be8ab01704f0bdfb61f64e88f3.setContent(html_e081ade1fa813a4e7a7dbf74ff398a1e);\\n \\n \\n\\n circle_marker_cf006cfcb36b1db90be34164d8f0d0ff.bindPopup(popup_5ced52be8ab01704f0bdfb61f64e88f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b157be309eeb23aded6b28c6e9302f2 = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_d4bdc4c6dded031f920d91113c60362c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e316bd7f0446f12fc2e27cb62a7df24f = $(`<div id="html_e316bd7f0446f12fc2e27cb62a7df24f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d4bdc4c6dded031f920d91113c60362c.setContent(html_e316bd7f0446f12fc2e27cb62a7df24f);\\n \\n \\n\\n circle_marker_0b157be309eeb23aded6b28c6e9302f2.bindPopup(popup_d4bdc4c6dded031f920d91113c60362c)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fbe774c35141aebfccdc1c2c8eac90a = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_d241de32cb0dc5fff839f95827212467 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d6c5983a9334186c90d5f3f2235494c = $(`<div id="html_0d6c5983a9334186c90d5f3f2235494c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d241de32cb0dc5fff839f95827212467.setContent(html_0d6c5983a9334186c90d5f3f2235494c);\\n \\n \\n\\n circle_marker_3fbe774c35141aebfccdc1c2c8eac90a.bindPopup(popup_d241de32cb0dc5fff839f95827212467)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e9a9037e3d1e674b7158cd2f77400c5 = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_3b91e3481b4ee4dc4aab2cce8f32f794 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e6ba2b8e5ed370a38123b982636cbd04 = $(`<div id="html_e6ba2b8e5ed370a38123b982636cbd04" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3b91e3481b4ee4dc4aab2cce8f32f794.setContent(html_e6ba2b8e5ed370a38123b982636cbd04);\\n \\n \\n\\n circle_marker_3e9a9037e3d1e674b7158cd2f77400c5.bindPopup(popup_3b91e3481b4ee4dc4aab2cce8f32f794)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe2435e444d8b7031e8ee55abc897dde = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_af6ffd0d8d99e672c11b49dfe2d2a4aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76c75e456270ee29aceb5220513ae5d5 = $(`<div id="html_76c75e456270ee29aceb5220513ae5d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af6ffd0d8d99e672c11b49dfe2d2a4aa.setContent(html_76c75e456270ee29aceb5220513ae5d5);\\n \\n \\n\\n circle_marker_fe2435e444d8b7031e8ee55abc897dde.bindPopup(popup_af6ffd0d8d99e672c11b49dfe2d2a4aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_4ea9426f9b4d1e826b5c5f66f1575d48 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_32a28f868009680963d0856ed4d397de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4eaba6270f56ecd2ec1b1c7d6c9688ae = $(`<div id="html_4eaba6270f56ecd2ec1b1c7d6c9688ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_32a28f868009680963d0856ed4d397de.setContent(html_4eaba6270f56ecd2ec1b1c7d6c9688ae);\\n \\n \\n\\n circle_marker_4ea9426f9b4d1e826b5c5f66f1575d48.bindPopup(popup_32a28f868009680963d0856ed4d397de)\\n ;\\n\\n \\n \\n \\n var circle_marker_858a671adebed3eb99734b8b42bb529e = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_898a974d9c936f723ea06391c3035550 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9d4ed22dfabb6064a264a279d7b8da3 = $(`<div id="html_d9d4ed22dfabb6064a264a279d7b8da3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_898a974d9c936f723ea06391c3035550.setContent(html_d9d4ed22dfabb6064a264a279d7b8da3);\\n \\n \\n\\n circle_marker_858a671adebed3eb99734b8b42bb529e.bindPopup(popup_898a974d9c936f723ea06391c3035550)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c7231324a9e51f6dde15203a1e45046 = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_9c7dd51711050b1f7ae318066d4510fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_23806b4d44b44cb777ef9edf0d31f4fa = $(`<div id="html_23806b4d44b44cb777ef9edf0d31f4fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9c7dd51711050b1f7ae318066d4510fc.setContent(html_23806b4d44b44cb777ef9edf0d31f4fa);\\n \\n \\n\\n circle_marker_8c7231324a9e51f6dde15203a1e45046.bindPopup(popup_9c7dd51711050b1f7ae318066d4510fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9d49100bac6e9a04e4207f53b14d405 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_8a8cfbb544330c3635da7a76650c2538 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e25ff08472a23af0a2d2162ffcac2266 = $(`<div id="html_e25ff08472a23af0a2d2162ffcac2266" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a8cfbb544330c3635da7a76650c2538.setContent(html_e25ff08472a23af0a2d2162ffcac2266);\\n \\n \\n\\n circle_marker_a9d49100bac6e9a04e4207f53b14d405.bindPopup(popup_8a8cfbb544330c3635da7a76650c2538)\\n ;\\n\\n \\n \\n \\n var circle_marker_d988abb94f1f75682ba1b1de29a16a01 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_04c732b8f594f61e06f000d66d7f5d4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd2d3c2fca2941ac3aa57f279cd1ec3b = $(`<div id="html_dd2d3c2fca2941ac3aa57f279cd1ec3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04c732b8f594f61e06f000d66d7f5d4f.setContent(html_dd2d3c2fca2941ac3aa57f279cd1ec3b);\\n \\n \\n\\n circle_marker_d988abb94f1f75682ba1b1de29a16a01.bindPopup(popup_04c732b8f594f61e06f000d66d7f5d4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_dfa5fea9e95c5390f8d1d0b5fe721355 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_9e8f5e22d5924193ba9e099bc1346ccf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2be51d1dec813cd0e8f273105cfc725f = $(`<div id="html_2be51d1dec813cd0e8f273105cfc725f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e8f5e22d5924193ba9e099bc1346ccf.setContent(html_2be51d1dec813cd0e8f273105cfc725f);\\n \\n \\n\\n circle_marker_dfa5fea9e95c5390f8d1d0b5fe721355.bindPopup(popup_9e8f5e22d5924193ba9e099bc1346ccf)\\n ;\\n\\n \\n \\n \\n var circle_marker_79276ae04d5c6fbf9d0ce64abedf9461 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_38581a3816c5a24e2be88d6011e4b1ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c05cfe769304950e52463e24b0a9fb4 = $(`<div id="html_2c05cfe769304950e52463e24b0a9fb4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38581a3816c5a24e2be88d6011e4b1ed.setContent(html_2c05cfe769304950e52463e24b0a9fb4);\\n \\n \\n\\n circle_marker_79276ae04d5c6fbf9d0ce64abedf9461.bindPopup(popup_38581a3816c5a24e2be88d6011e4b1ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_1fedca3be8c2fd8a65db8e8ead4fcc96 = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_175d3e4b91e93ae0f2d352d0ee44e2ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28b8e85af3b6c947920f1132d1c4d9b9 = $(`<div id="html_28b8e85af3b6c947920f1132d1c4d9b9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_175d3e4b91e93ae0f2d352d0ee44e2ed.setContent(html_28b8e85af3b6c947920f1132d1c4d9b9);\\n \\n \\n\\n circle_marker_1fedca3be8c2fd8a65db8e8ead4fcc96.bindPopup(popup_175d3e4b91e93ae0f2d352d0ee44e2ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa2871dad7370ff82d96b87b6585bc1f = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_1df3d8baf45f9555c699a7a68535fc4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a5b8fb0b64013bf6bc77d7dd7f300ee = $(`<div id="html_9a5b8fb0b64013bf6bc77d7dd7f300ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1df3d8baf45f9555c699a7a68535fc4c.setContent(html_9a5b8fb0b64013bf6bc77d7dd7f300ee);\\n \\n \\n\\n circle_marker_aa2871dad7370ff82d96b87b6585bc1f.bindPopup(popup_1df3d8baf45f9555c699a7a68535fc4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a42736b310fbf59e0f34a1afd310166 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_20c55a3ca7380785b6ebcefa621a6cab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5d847276052da5384f99b722e2f1fa25 = $(`<div id="html_5d847276052da5384f99b722e2f1fa25" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20c55a3ca7380785b6ebcefa621a6cab.setContent(html_5d847276052da5384f99b722e2f1fa25);\\n \\n \\n\\n circle_marker_4a42736b310fbf59e0f34a1afd310166.bindPopup(popup_20c55a3ca7380785b6ebcefa621a6cab)\\n ;\\n\\n \\n \\n \\n var circle_marker_f694419621b48187a6e97ba9f05c2cf5 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_7eb0bfcc679eba833a1600dd70996620 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_177f06b0183d68d200d200a69c065fa0 = $(`<div id="html_177f06b0183d68d200d200a69c065fa0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7eb0bfcc679eba833a1600dd70996620.setContent(html_177f06b0183d68d200d200a69c065fa0);\\n \\n \\n\\n circle_marker_f694419621b48187a6e97ba9f05c2cf5.bindPopup(popup_7eb0bfcc679eba833a1600dd70996620)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba9a894a82f92cbd11d4519dce8c8137 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_cf72a3d59b208cf784cc77febba6ee55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e72d9592e82f8333919464dc1a1f1b3a = $(`<div id="html_e72d9592e82f8333919464dc1a1f1b3a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf72a3d59b208cf784cc77febba6ee55.setContent(html_e72d9592e82f8333919464dc1a1f1b3a);\\n \\n \\n\\n circle_marker_ba9a894a82f92cbd11d4519dce8c8137.bindPopup(popup_cf72a3d59b208cf784cc77febba6ee55)\\n ;\\n\\n \\n \\n \\n var circle_marker_720ee2b9f3994adb259b81994ebe6f2c = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_cd6eb7135114287f1222881bc5b4b707);\\n \\n \\n var popup_0ec57d0cd36832ea89fd4e286caa6529 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b90136f8a29c4c3814b98736caaa7ed3 = $(`<div id="html_b90136f8a29c4c3814b98736caaa7ed3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0ec57d0cd36832ea89fd4e286caa6529.setContent(html_b90136f8a29c4c3814b98736caaa7ed3);\\n \\n \\n\\n circle_marker_720ee2b9f3994adb259b81994ebe6f2c.bindPopup(popup_0ec57d0cd36832ea89fd4e286caa6529)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Hawthorn": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_4106612fb14bcbe873c1b11f6252898f {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_4106612fb14bcbe873c1b11f6252898f" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_4106612fb14bcbe873c1b11f6252898f = L.map(\\n "map_4106612fb14bcbe873c1b11f6252898f",\\n {\\n center: [42.731836351800865, -73.24519064107557],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_4c8de13eb2737cf6b25b5707a940e6f6 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var circle_marker_df359f9eb88912acda2f4dc8da7fda73 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_a82ed0f9424d1b80420a30790e44223a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b97c76cd2e0c639e89cc7fb860b18fac = $(`<div id="html_b97c76cd2e0c639e89cc7fb860b18fac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a82ed0f9424d1b80420a30790e44223a.setContent(html_b97c76cd2e0c639e89cc7fb860b18fac);\\n \\n \\n\\n circle_marker_df359f9eb88912acda2f4dc8da7fda73.bindPopup(popup_a82ed0f9424d1b80420a30790e44223a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0d089f025d20650c8e1958675fb79f3 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_553f85702dde7f0fcaa2c14f2337c613 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f48ccd27b7f1170ff72a871cdf95d81b = $(`<div id="html_f48ccd27b7f1170ff72a871cdf95d81b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_553f85702dde7f0fcaa2c14f2337c613.setContent(html_f48ccd27b7f1170ff72a871cdf95d81b);\\n \\n \\n\\n circle_marker_e0d089f025d20650c8e1958675fb79f3.bindPopup(popup_553f85702dde7f0fcaa2c14f2337c613)\\n ;\\n\\n \\n \\n \\n var circle_marker_8fa8d9c5767959cddef92c60216698b1 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_241b493a2b04db18095068fb33dc1dbe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e72a750dea011536847b74a56188d53f = $(`<div id="html_e72a750dea011536847b74a56188d53f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_241b493a2b04db18095068fb33dc1dbe.setContent(html_e72a750dea011536847b74a56188d53f);\\n \\n \\n\\n circle_marker_8fa8d9c5767959cddef92c60216698b1.bindPopup(popup_241b493a2b04db18095068fb33dc1dbe)\\n ;\\n\\n \\n \\n \\n var circle_marker_6efe7dbc4602e912107eedcc14361a5e = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_d8139202b1ac87fe49d79326c714389d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3003d7431d9b96975c70acda38c23ad = $(`<div id="html_e3003d7431d9b96975c70acda38c23ad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8139202b1ac87fe49d79326c714389d.setContent(html_e3003d7431d9b96975c70acda38c23ad);\\n \\n \\n\\n circle_marker_6efe7dbc4602e912107eedcc14361a5e.bindPopup(popup_d8139202b1ac87fe49d79326c714389d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c025a25eee2efa68510d54899ad8f40 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_cadd54fb45cc94fe14e26ab9a330414e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_769a07e86746e01c3345149f390bfb56 = $(`<div id="html_769a07e86746e01c3345149f390bfb56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cadd54fb45cc94fe14e26ab9a330414e.setContent(html_769a07e86746e01c3345149f390bfb56);\\n \\n \\n\\n circle_marker_0c025a25eee2efa68510d54899ad8f40.bindPopup(popup_cadd54fb45cc94fe14e26ab9a330414e)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe8e39bf3485b699eb5bff03304f77b0 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_faccf9bbad9d0f251bee6c532dd1dcb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3247404e0bbe0d64714c6a6a91294fbb = $(`<div id="html_3247404e0bbe0d64714c6a6a91294fbb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_faccf9bbad9d0f251bee6c532dd1dcb8.setContent(html_3247404e0bbe0d64714c6a6a91294fbb);\\n \\n \\n\\n circle_marker_fe8e39bf3485b699eb5bff03304f77b0.bindPopup(popup_faccf9bbad9d0f251bee6c532dd1dcb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_644e743749b725d3ef183c44ab20bdcd = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_aec5a3b5bf634c64a018f2224dedab73 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eab39fc9b85d256882d2c130ea3a1ddb = $(`<div id="html_eab39fc9b85d256882d2c130ea3a1ddb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aec5a3b5bf634c64a018f2224dedab73.setContent(html_eab39fc9b85d256882d2c130ea3a1ddb);\\n \\n \\n\\n circle_marker_644e743749b725d3ef183c44ab20bdcd.bindPopup(popup_aec5a3b5bf634c64a018f2224dedab73)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d57aa64a443a97773dcca84686acafd = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_5dc00ea4c061f383563379a12753ee70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24854247f05bfd047a6ed7d0cbdcd94b = $(`<div id="html_24854247f05bfd047a6ed7d0cbdcd94b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5dc00ea4c061f383563379a12753ee70.setContent(html_24854247f05bfd047a6ed7d0cbdcd94b);\\n \\n \\n\\n circle_marker_6d57aa64a443a97773dcca84686acafd.bindPopup(popup_5dc00ea4c061f383563379a12753ee70)\\n ;\\n\\n \\n \\n \\n var circle_marker_4c162493c09cc8df3b9ca56b4920638f = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_58e75a2e8a8390696e71a177ed47b4e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_432e502d5b47b72cca69ed7f5e9ef953 = $(`<div id="html_432e502d5b47b72cca69ed7f5e9ef953" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58e75a2e8a8390696e71a177ed47b4e2.setContent(html_432e502d5b47b72cca69ed7f5e9ef953);\\n \\n \\n\\n circle_marker_4c162493c09cc8df3b9ca56b4920638f.bindPopup(popup_58e75a2e8a8390696e71a177ed47b4e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_29675df6d8828df16c7a65e6a750d1ae = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_8dd0bb893f5d66460ddbfe3f827f4885 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2869b7ca1d598fbefad036e8be2d4a0b = $(`<div id="html_2869b7ca1d598fbefad036e8be2d4a0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8dd0bb893f5d66460ddbfe3f827f4885.setContent(html_2869b7ca1d598fbefad036e8be2d4a0b);\\n \\n \\n\\n circle_marker_29675df6d8828df16c7a65e6a750d1ae.bindPopup(popup_8dd0bb893f5d66460ddbfe3f827f4885)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6d997a18d49037224bb1cda981731f0 = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_dd57bdd8a589f2b30062d2a5dff71990 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6114b926591cd4cb7718ad5ce9b2416f = $(`<div id="html_6114b926591cd4cb7718ad5ce9b2416f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd57bdd8a589f2b30062d2a5dff71990.setContent(html_6114b926591cd4cb7718ad5ce9b2416f);\\n \\n \\n\\n circle_marker_a6d997a18d49037224bb1cda981731f0.bindPopup(popup_dd57bdd8a589f2b30062d2a5dff71990)\\n ;\\n\\n \\n \\n \\n var circle_marker_fae99a128f426fdb197fe11085d0c9c9 = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_10aeb77ef5a24c137e08ee0dbd62485c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c349d08b44f7aa7800c0d2b47a03e91 = $(`<div id="html_8c349d08b44f7aa7800c0d2b47a03e91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10aeb77ef5a24c137e08ee0dbd62485c.setContent(html_8c349d08b44f7aa7800c0d2b47a03e91);\\n \\n \\n\\n circle_marker_fae99a128f426fdb197fe11085d0c9c9.bindPopup(popup_10aeb77ef5a24c137e08ee0dbd62485c)\\n ;\\n\\n \\n \\n \\n var circle_marker_66703c90ff1a1c2d82d89c0d0bfeeb93 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_41ffa6ebed28b5e57c521488d5269e44 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_707716c5dc4297225eaf322ff7b752b0 = $(`<div id="html_707716c5dc4297225eaf322ff7b752b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41ffa6ebed28b5e57c521488d5269e44.setContent(html_707716c5dc4297225eaf322ff7b752b0);\\n \\n \\n\\n circle_marker_66703c90ff1a1c2d82d89c0d0bfeeb93.bindPopup(popup_41ffa6ebed28b5e57c521488d5269e44)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e109211ddd9db66f7f2cd243ea03818 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_eb61860cfa7efa7d39398e8ae088d64c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50cbfe69d4e153987a98707cb10a17e8 = $(`<div id="html_50cbfe69d4e153987a98707cb10a17e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb61860cfa7efa7d39398e8ae088d64c.setContent(html_50cbfe69d4e153987a98707cb10a17e8);\\n \\n \\n\\n circle_marker_6e109211ddd9db66f7f2cd243ea03818.bindPopup(popup_eb61860cfa7efa7d39398e8ae088d64c)\\n ;\\n\\n \\n \\n \\n var circle_marker_27ae65f60a16473073b7fac3d564a8c5 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_d511625009d742eefffc4904774c2db6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f2eca2a126c07699597c8352c7690dd = $(`<div id="html_0f2eca2a126c07699597c8352c7690dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d511625009d742eefffc4904774c2db6.setContent(html_0f2eca2a126c07699597c8352c7690dd);\\n \\n \\n\\n circle_marker_27ae65f60a16473073b7fac3d564a8c5.bindPopup(popup_d511625009d742eefffc4904774c2db6)\\n ;\\n\\n \\n \\n \\n var circle_marker_583a7d78d1e455a5196fdf1ba13b6d22 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_3b3375ff93a7c228a6994a3ab2624842 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_72902ca78f039a46307e84fb18587ccf = $(`<div id="html_72902ca78f039a46307e84fb18587ccf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3b3375ff93a7c228a6994a3ab2624842.setContent(html_72902ca78f039a46307e84fb18587ccf);\\n \\n \\n\\n circle_marker_583a7d78d1e455a5196fdf1ba13b6d22.bindPopup(popup_3b3375ff93a7c228a6994a3ab2624842)\\n ;\\n\\n \\n \\n \\n var circle_marker_84f1f51867d6f18b339c601682b1c99c = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_e61dcebe93a360acb34a7bd60dab76b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ac4187e3bc3a5247d8e04b50c3b7eb7 = $(`<div id="html_7ac4187e3bc3a5247d8e04b50c3b7eb7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e61dcebe93a360acb34a7bd60dab76b4.setContent(html_7ac4187e3bc3a5247d8e04b50c3b7eb7);\\n \\n \\n\\n circle_marker_84f1f51867d6f18b339c601682b1c99c.bindPopup(popup_e61dcebe93a360acb34a7bd60dab76b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f8e74f55b1be3bba492c114e0f5f563 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_71626019d365f2f4b9d3dfa1f46e62da = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6905a7f1fefc07f8fdd6218907ebc81 = $(`<div id="html_a6905a7f1fefc07f8fdd6218907ebc81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71626019d365f2f4b9d3dfa1f46e62da.setContent(html_a6905a7f1fefc07f8fdd6218907ebc81);\\n \\n \\n\\n circle_marker_3f8e74f55b1be3bba492c114e0f5f563.bindPopup(popup_71626019d365f2f4b9d3dfa1f46e62da)\\n ;\\n\\n \\n \\n \\n var circle_marker_c930de9d16ee3f64e4b11fd46c965344 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_bca25ecf4d0c67dcf54e796ca60fc1a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_216589e4380e55018377ab7cc23be8e1 = $(`<div id="html_216589e4380e55018377ab7cc23be8e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bca25ecf4d0c67dcf54e796ca60fc1a8.setContent(html_216589e4380e55018377ab7cc23be8e1);\\n \\n \\n\\n circle_marker_c930de9d16ee3f64e4b11fd46c965344.bindPopup(popup_bca25ecf4d0c67dcf54e796ca60fc1a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_3632ffe1c5147d2130349a63630d5999 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_97dd9a7631e11e7a0dc9774435e8b9ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69265c4e762ee8332d1513bafb6b3765 = $(`<div id="html_69265c4e762ee8332d1513bafb6b3765" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97dd9a7631e11e7a0dc9774435e8b9ac.setContent(html_69265c4e762ee8332d1513bafb6b3765);\\n \\n \\n\\n circle_marker_3632ffe1c5147d2130349a63630d5999.bindPopup(popup_97dd9a7631e11e7a0dc9774435e8b9ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_03fe32685cacda611f1a1a486bfc2c5f = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_30c94a0eceb0b9243e797cfbc78926f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9e72b014cc5eb03975a3ac9fab09894 = $(`<div id="html_a9e72b014cc5eb03975a3ac9fab09894" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_30c94a0eceb0b9243e797cfbc78926f8.setContent(html_a9e72b014cc5eb03975a3ac9fab09894);\\n \\n \\n\\n circle_marker_03fe32685cacda611f1a1a486bfc2c5f.bindPopup(popup_30c94a0eceb0b9243e797cfbc78926f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_b934a039da2b6cf6fa55d6b737098df0 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_13e85c4ce162eea9ce05f372a97d0d2e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19ae424e63ea1d5b7f49876d542e9f5e = $(`<div id="html_19ae424e63ea1d5b7f49876d542e9f5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13e85c4ce162eea9ce05f372a97d0d2e.setContent(html_19ae424e63ea1d5b7f49876d542e9f5e);\\n \\n \\n\\n circle_marker_b934a039da2b6cf6fa55d6b737098df0.bindPopup(popup_13e85c4ce162eea9ce05f372a97d0d2e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f547777b84e403de563f5785ab8c2f88 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_786be8c2143022e069f035168b133850 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_90f249c2d09688a1cf8c737cf15fc787 = $(`<div id="html_90f249c2d09688a1cf8c737cf15fc787" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_786be8c2143022e069f035168b133850.setContent(html_90f249c2d09688a1cf8c737cf15fc787);\\n \\n \\n\\n circle_marker_f547777b84e403de563f5785ab8c2f88.bindPopup(popup_786be8c2143022e069f035168b133850)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cdf8be907920e800223061a29172dea = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_30a1b5a566a38043c171e57b7e267212 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a3d3f081995677afc4637c8a3494038 = $(`<div id="html_1a3d3f081995677afc4637c8a3494038" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_30a1b5a566a38043c171e57b7e267212.setContent(html_1a3d3f081995677afc4637c8a3494038);\\n \\n \\n\\n circle_marker_3cdf8be907920e800223061a29172dea.bindPopup(popup_30a1b5a566a38043c171e57b7e267212)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8c51e5a30f66625df823a0b161d4b85 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_6261920cba5736b6d8c841b46db2513f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e0a692ce582eea210bf417e11c768912 = $(`<div id="html_e0a692ce582eea210bf417e11c768912" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6261920cba5736b6d8c841b46db2513f.setContent(html_e0a692ce582eea210bf417e11c768912);\\n \\n \\n\\n circle_marker_a8c51e5a30f66625df823a0b161d4b85.bindPopup(popup_6261920cba5736b6d8c841b46db2513f)\\n ;\\n\\n \\n \\n \\n var circle_marker_55b6f9adea4c32752dcc7cf475ae8dd0 = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_f4b0fef157e8be6040f943cbe528922f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b9674db41074a2ec17d8e6e1ab8efee6 = $(`<div id="html_b9674db41074a2ec17d8e6e1ab8efee6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4b0fef157e8be6040f943cbe528922f.setContent(html_b9674db41074a2ec17d8e6e1ab8efee6);\\n \\n \\n\\n circle_marker_55b6f9adea4c32752dcc7cf475ae8dd0.bindPopup(popup_f4b0fef157e8be6040f943cbe528922f)\\n ;\\n\\n \\n \\n \\n var circle_marker_183f668b3537a9d6969f18ffffab1796 = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_0b2b4385b3f413ef63ab781da82d19d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0f6922e5fa48bb28de8e8e42115eb14 = $(`<div id="html_b0f6922e5fa48bb28de8e8e42115eb14" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b2b4385b3f413ef63ab781da82d19d5.setContent(html_b0f6922e5fa48bb28de8e8e42115eb14);\\n \\n \\n\\n circle_marker_183f668b3537a9d6969f18ffffab1796.bindPopup(popup_0b2b4385b3f413ef63ab781da82d19d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c82aa879097343a17792aedcf8d0ed90 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_4106612fb14bcbe873c1b11f6252898f);\\n \\n \\n var popup_eb39f19dcf6691c107eb00ed0edb44df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_207872e29ecca93e4b4c01879585ad8b = $(`<div id="html_207872e29ecca93e4b4c01879585ad8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb39f19dcf6691c107eb00ed0edb44df.setContent(html_207872e29ecca93e4b4c01879585ad8b);\\n \\n \\n\\n circle_marker_c82aa879097343a17792aedcf8d0ed90.bindPopup(popup_eb39f19dcf6691c107eb00ed0edb44df)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Hazelnut, American\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_4811b8a08d525ae3ccef08bd2ae59b25 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_4811b8a08d525ae3ccef08bd2ae59b25" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_4811b8a08d525ae3ccef08bd2ae59b25 = L.map(\\n "map_4811b8a08d525ae3ccef08bd2ae59b25",\\n {\\n center: [42.73454784790659, -73.23657792568366],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_6203e343369a9eab5c8e269e2bb54796 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_4811b8a08d525ae3ccef08bd2ae59b25);\\n \\n \\n var circle_marker_a270062f7d49cf390d76671d4fb770af = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_4811b8a08d525ae3ccef08bd2ae59b25);\\n \\n \\n var popup_9543ba08ea04cd7ac7869da47ff1c711 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d71935383b4efeb312015b0514ab9be8 = $(`<div id="html_d71935383b4efeb312015b0514ab9be8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9543ba08ea04cd7ac7869da47ff1c711.setContent(html_d71935383b4efeb312015b0514ab9be8);\\n \\n \\n\\n circle_marker_a270062f7d49cf390d76671d4fb770af.bindPopup(popup_9543ba08ea04cd7ac7869da47ff1c711)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Hemlock, eastern\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_88ae320d5ad55f2a627b82eec6e883a1 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_88ae320d5ad55f2a627b82eec6e883a1" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_88ae320d5ad55f2a627b82eec6e883a1 = L.map(\\n "map_88ae320d5ad55f2a627b82eec6e883a1",\\n {\\n center: [42.7363579603885, -73.2421151440802],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_def186b15d587af6e11495fe3a10fa5a = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var circle_marker_c6452d1465f8034b45f94fc8947d23f8 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_4412dbc8b118694678f008497b69884d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad2bedaa008192333b9c7faa2bbcc096 = $(`<div id="html_ad2bedaa008192333b9c7faa2bbcc096" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4412dbc8b118694678f008497b69884d.setContent(html_ad2bedaa008192333b9c7faa2bbcc096);\\n \\n \\n\\n circle_marker_c6452d1465f8034b45f94fc8947d23f8.bindPopup(popup_4412dbc8b118694678f008497b69884d)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc80a9845862f20659e143362e6d601d = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_e604e984100539035ba57193a7f0f50b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ebd8576a738b2dc2127f1606ffa99e5 = $(`<div id="html_4ebd8576a738b2dc2127f1606ffa99e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e604e984100539035ba57193a7f0f50b.setContent(html_4ebd8576a738b2dc2127f1606ffa99e5);\\n \\n \\n\\n circle_marker_fc80a9845862f20659e143362e6d601d.bindPopup(popup_e604e984100539035ba57193a7f0f50b)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc335952bc444bd8b1e6a1c7367e8c5a = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_86170fe41293701c3fcf8d63bbe879d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01f49311244277e490a93e2c685f7fba = $(`<div id="html_01f49311244277e490a93e2c685f7fba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86170fe41293701c3fcf8d63bbe879d1.setContent(html_01f49311244277e490a93e2c685f7fba);\\n \\n \\n\\n circle_marker_cc335952bc444bd8b1e6a1c7367e8c5a.bindPopup(popup_86170fe41293701c3fcf8d63bbe879d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9ac332c0a63648bea3fd34cc2d0f380 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_90a46da8bbef0153b91e70bdbb50de24 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a82e00fd9077ca400cae0b714d8d0aa = $(`<div id="html_8a82e00fd9077ca400cae0b714d8d0aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90a46da8bbef0153b91e70bdbb50de24.setContent(html_8a82e00fd9077ca400cae0b714d8d0aa);\\n \\n \\n\\n circle_marker_a9ac332c0a63648bea3fd34cc2d0f380.bindPopup(popup_90a46da8bbef0153b91e70bdbb50de24)\\n ;\\n\\n \\n \\n \\n var circle_marker_a637c00619a47a5cba15c63caa44e904 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_d82139e6c0e8f7b337c6bbd7d1d9c5a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_73c099d386b2b15203178523a0c78459 = $(`<div id="html_73c099d386b2b15203178523a0c78459" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d82139e6c0e8f7b337c6bbd7d1d9c5a1.setContent(html_73c099d386b2b15203178523a0c78459);\\n \\n \\n\\n circle_marker_a637c00619a47a5cba15c63caa44e904.bindPopup(popup_d82139e6c0e8f7b337c6bbd7d1d9c5a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_992a7137abfd1dab071bd800cf3e090b = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_13c47fa912b4aa4725423a24822b2e33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b59d4a852d9e6c4154278fc0600ce242 = $(`<div id="html_b59d4a852d9e6c4154278fc0600ce242" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13c47fa912b4aa4725423a24822b2e33.setContent(html_b59d4a852d9e6c4154278fc0600ce242);\\n \\n \\n\\n circle_marker_992a7137abfd1dab071bd800cf3e090b.bindPopup(popup_13c47fa912b4aa4725423a24822b2e33)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6ab7802eb86f783e223b370693d78ad = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_00b00ba6156df13029066dba77934d7e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54cadc9df581a5c2052f09633df4a735 = $(`<div id="html_54cadc9df581a5c2052f09633df4a735" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00b00ba6156df13029066dba77934d7e.setContent(html_54cadc9df581a5c2052f09633df4a735);\\n \\n \\n\\n circle_marker_a6ab7802eb86f783e223b370693d78ad.bindPopup(popup_00b00ba6156df13029066dba77934d7e)\\n ;\\n\\n \\n \\n \\n var circle_marker_227464878f988ac1749f5e31b84fb049 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_65b9e92ffa1aa43444b03fc340fcdb86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ba85971cdeef9c456ec702d53dbe823 = $(`<div id="html_6ba85971cdeef9c456ec702d53dbe823" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65b9e92ffa1aa43444b03fc340fcdb86.setContent(html_6ba85971cdeef9c456ec702d53dbe823);\\n \\n \\n\\n circle_marker_227464878f988ac1749f5e31b84fb049.bindPopup(popup_65b9e92ffa1aa43444b03fc340fcdb86)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c6003378a50714397433212e028a84d = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_007447c43c77ffbad117f9f2c51be78f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e1b96a627953c522f4e18ab87cd2f9a = $(`<div id="html_9e1b96a627953c522f4e18ab87cd2f9a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_007447c43c77ffbad117f9f2c51be78f.setContent(html_9e1b96a627953c522f4e18ab87cd2f9a);\\n \\n \\n\\n circle_marker_6c6003378a50714397433212e028a84d.bindPopup(popup_007447c43c77ffbad117f9f2c51be78f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1263a42e68016f72e080278caefe6b2b = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_18e465d61b911f7cb86e5cf6571c082a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d17f4a840da156bc98dd7e59ab260cfa = $(`<div id="html_d17f4a840da156bc98dd7e59ab260cfa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_18e465d61b911f7cb86e5cf6571c082a.setContent(html_d17f4a840da156bc98dd7e59ab260cfa);\\n \\n \\n\\n circle_marker_1263a42e68016f72e080278caefe6b2b.bindPopup(popup_18e465d61b911f7cb86e5cf6571c082a)\\n ;\\n\\n \\n \\n \\n var circle_marker_337202d9318c5483c6bfa30bda92bc8e = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_fcff32b13922cd2a04f6c45ddb0c14b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5cf757338da63214b16a7400ffa2787f = $(`<div id="html_5cf757338da63214b16a7400ffa2787f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fcff32b13922cd2a04f6c45ddb0c14b9.setContent(html_5cf757338da63214b16a7400ffa2787f);\\n \\n \\n\\n circle_marker_337202d9318c5483c6bfa30bda92bc8e.bindPopup(popup_fcff32b13922cd2a04f6c45ddb0c14b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_a02f1f871f10c0fc5e6ad5d4c7f29f19 = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_5caf264bdc762e6665562357a0090819 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9de5dddb3250af5d75976609751974a8 = $(`<div id="html_9de5dddb3250af5d75976609751974a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5caf264bdc762e6665562357a0090819.setContent(html_9de5dddb3250af5d75976609751974a8);\\n \\n \\n\\n circle_marker_a02f1f871f10c0fc5e6ad5d4c7f29f19.bindPopup(popup_5caf264bdc762e6665562357a0090819)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ff5216663aaaf27d1343bc17d94a5eb = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_e0f546bba31e720f8855eb505b2aef25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea52e407b7a76d33be32416fe44b380e = $(`<div id="html_ea52e407b7a76d33be32416fe44b380e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0f546bba31e720f8855eb505b2aef25.setContent(html_ea52e407b7a76d33be32416fe44b380e);\\n \\n \\n\\n circle_marker_0ff5216663aaaf27d1343bc17d94a5eb.bindPopup(popup_e0f546bba31e720f8855eb505b2aef25)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d6433b62ca3f18d9bcdac87a8d7a2f7 = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_8a0b7d50682eaeb8d00e9400787027f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea8aa4814f24c39fac05083139283398 = $(`<div id="html_ea8aa4814f24c39fac05083139283398" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a0b7d50682eaeb8d00e9400787027f9.setContent(html_ea8aa4814f24c39fac05083139283398);\\n \\n \\n\\n circle_marker_1d6433b62ca3f18d9bcdac87a8d7a2f7.bindPopup(popup_8a0b7d50682eaeb8d00e9400787027f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_ef45725c840734a2b85d67a95ff81fee = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_e329518c0d2d0906e2106c7c774239dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b57b0083373f1377496114d01fe53c50 = $(`<div id="html_b57b0083373f1377496114d01fe53c50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e329518c0d2d0906e2106c7c774239dd.setContent(html_b57b0083373f1377496114d01fe53c50);\\n \\n \\n\\n circle_marker_ef45725c840734a2b85d67a95ff81fee.bindPopup(popup_e329518c0d2d0906e2106c7c774239dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_e46c20de76377c89e826dfbf947e9bf6 = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_2ec97347a5e31201ea833bfc641ea953 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cbde0bcf31b583cfb9ad0a249f59d01f = $(`<div id="html_cbde0bcf31b583cfb9ad0a249f59d01f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ec97347a5e31201ea833bfc641ea953.setContent(html_cbde0bcf31b583cfb9ad0a249f59d01f);\\n \\n \\n\\n circle_marker_e46c20de76377c89e826dfbf947e9bf6.bindPopup(popup_2ec97347a5e31201ea833bfc641ea953)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9d4ed39dcf0287007dc540b917f76a0 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_88ae320d5ad55f2a627b82eec6e883a1);\\n \\n \\n var popup_769405be13bc7cfbcca2c166aca25a66 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b8cbbb12010ef78a41119e2a9ae88f6 = $(`<div id="html_1b8cbbb12010ef78a41119e2a9ae88f6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_769405be13bc7cfbcca2c166aca25a66.setContent(html_1b8cbbb12010ef78a41119e2a9ae88f6);\\n \\n \\n\\n circle_marker_e9d4ed39dcf0287007dc540b917f76a0.bindPopup(popup_769405be13bc7cfbcca2c166aca25a66)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Hickory, shagbark\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_3c26a340b13f64b5b45ea258f4d4a624 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_3c26a340b13f64b5b45ea258f4d4a624" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_3c26a340b13f64b5b45ea258f4d4a624 = L.map(\\n "map_3c26a340b13f64b5b45ea258f4d4a624",\\n {\\n center: [42.7399651192432, -73.22304240032922],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_4a8cdd69268abe01ba57fecdbf8c52fd = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_3c26a340b13f64b5b45ea258f4d4a624);\\n \\n \\n var circle_marker_83c66e25be6be40b03a51ce9a061e74d = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3c26a340b13f64b5b45ea258f4d4a624);\\n \\n \\n var popup_a63819a7df4e344491c8153b0e5ec1d0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab8afb002a0f7840a940b40ac744450c = $(`<div id="html_ab8afb002a0f7840a940b40ac744450c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a63819a7df4e344491c8153b0e5ec1d0.setContent(html_ab8afb002a0f7840a940b40ac744450c);\\n \\n \\n\\n circle_marker_83c66e25be6be40b03a51ce9a061e74d.bindPopup(popup_a63819a7df4e344491c8153b0e5ec1d0)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Holly, mountain\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_1fcf0103ee0fc7330c71c3fc0ea39580 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_1fcf0103ee0fc7330c71c3fc0ea39580" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_1fcf0103ee0fc7330c71c3fc0ea39580 = L.map(\\n "map_1fcf0103ee0fc7330c71c3fc0ea39580",\\n {\\n center: [42.72551559789129, -73.26856180836413],\\n crs: L.CRS.EPSG3857,\\n zoom: 18,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_d8583f19c68c201e56712126e9170cb4 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 20, "maxZoom": 20, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_1fcf0103ee0fc7330c71c3fc0ea39580);\\n \\n \\n var circle_marker_b26333c9e72ae9bb3556d3bc28b41bb3 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_1fcf0103ee0fc7330c71c3fc0ea39580);\\n \\n \\n var popup_61ddb13af21c64008598c892203e6e95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17300e47957a469631855fbfd7058144 = $(`<div id="html_17300e47957a469631855fbfd7058144" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61ddb13af21c64008598c892203e6e95.setContent(html_17300e47957a469631855fbfd7058144);\\n \\n \\n\\n circle_marker_b26333c9e72ae9bb3556d3bc28b41bb3.bindPopup(popup_61ddb13af21c64008598c892203e6e95)\\n ;\\n\\n \\n \\n \\n var circle_marker_448dbdff1528e35be8d0d2b824726577 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_1fcf0103ee0fc7330c71c3fc0ea39580);\\n \\n \\n var popup_8733dde098c840d579071e45ff508065 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13fb2cfc7493aba9ffef151930ad05de = $(`<div id="html_13fb2cfc7493aba9ffef151930ad05de" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8733dde098c840d579071e45ff508065.setContent(html_13fb2cfc7493aba9ffef151930ad05de);\\n \\n \\n\\n circle_marker_448dbdff1528e35be8d0d2b824726577.bindPopup(popup_8733dde098c840d579071e45ff508065)\\n ;\\n\\n \\n \\n \\n var circle_marker_0815b684412097c89487ad9983d2a094 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_1fcf0103ee0fc7330c71c3fc0ea39580);\\n \\n \\n var popup_19879865c6531055a9585db513d2f397 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d734cb781751dd0b73616351403383a9 = $(`<div id="html_d734cb781751dd0b73616351403383a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19879865c6531055a9585db513d2f397.setContent(html_d734cb781751dd0b73616351403383a9);\\n \\n \\n\\n circle_marker_0815b684412097c89487ad9983d2a094.bindPopup(popup_19879865c6531055a9585db513d2f397)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Honeysuckle": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_0c75cd49894c87e066bb2c9ba85b5f2f {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_0c75cd49894c87e066bb2c9ba85b5f2f" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_0c75cd49894c87e066bb2c9ba85b5f2f = L.map(\\n "map_0c75cd49894c87e066bb2c9ba85b5f2f",\\n {\\n center: [42.73454655783, -73.2322735507988],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_b393bdb28bd0806bfcadd8429e83b4af = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var circle_marker_5aff3b2e920e82d2f3416f758e55df01 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_79b0695092d30f626af48c963aa30ee3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd08f6d5eab7c15be6f53b9ec3d344d1 = $(`<div id="html_bd08f6d5eab7c15be6f53b9ec3d344d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79b0695092d30f626af48c963aa30ee3.setContent(html_bd08f6d5eab7c15be6f53b9ec3d344d1);\\n \\n \\n\\n circle_marker_5aff3b2e920e82d2f3416f758e55df01.bindPopup(popup_79b0695092d30f626af48c963aa30ee3)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e0803ceceeeb9c021ee12d3ff379384 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.651697090182843, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_f9f8a29234b2260fccc3740921dfe956 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2478ad0020c0aac7ad23c55cc4f0236 = $(`<div id="html_e2478ad0020c0aac7ad23c55cc4f0236" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9f8a29234b2260fccc3740921dfe956.setContent(html_e2478ad0020c0aac7ad23c55cc4f0236);\\n \\n \\n\\n circle_marker_2e0803ceceeeb9c021ee12d3ff379384.bindPopup(popup_f9f8a29234b2260fccc3740921dfe956)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4b9e6875214e841007b430565414d32 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.583497844237541, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_47c79f36e69e39b22460f9580e6d5ecb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d58bd33507c41817ce1b29117bf0a010 = $(`<div id="html_d58bd33507c41817ce1b29117bf0a010" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47c79f36e69e39b22460f9580e6d5ecb.setContent(html_d58bd33507c41817ce1b29117bf0a010);\\n \\n \\n\\n circle_marker_c4b9e6875214e841007b430565414d32.bindPopup(popup_47c79f36e69e39b22460f9580e6d5ecb)\\n ;\\n\\n \\n \\n \\n var circle_marker_40b5eb55be2308b3fd81b6a57e381fe8 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.997417539138688, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_2470c4fa131afff2fab752a7b282d4f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41bf55270dd3bc672344b38c260d0ff2 = $(`<div id="html_41bf55270dd3bc672344b38c260d0ff2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2470c4fa131afff2fab752a7b282d4f2.setContent(html_41bf55270dd3bc672344b38c260d0ff2);\\n \\n \\n\\n circle_marker_40b5eb55be2308b3fd81b6a57e381fe8.bindPopup(popup_2470c4fa131afff2fab752a7b282d4f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_b43562fe71eebe8bc40f5e9b678191b5 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_152cf7ed996958eff1f9a349d61273d2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fda41d8c211fd8404ab60cf8c14611b7 = $(`<div id="html_fda41d8c211fd8404ab60cf8c14611b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_152cf7ed996958eff1f9a349d61273d2.setContent(html_fda41d8c211fd8404ab60cf8c14611b7);\\n \\n \\n\\n circle_marker_b43562fe71eebe8bc40f5e9b678191b5.bindPopup(popup_152cf7ed996958eff1f9a349d61273d2)\\n ;\\n\\n \\n \\n \\n var circle_marker_668a69fe9b7d1389aa317ac915ee52fa = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_8a1c1659c8656ac469005e91a15ae317 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7c758b9c1b22b946b520d01a9fc81f8 = $(`<div id="html_e7c758b9c1b22b946b520d01a9fc81f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a1c1659c8656ac469005e91a15ae317.setContent(html_e7c758b9c1b22b946b520d01a9fc81f8);\\n \\n \\n\\n circle_marker_668a69fe9b7d1389aa317ac915ee52fa.bindPopup(popup_8a1c1659c8656ac469005e91a15ae317)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1112ff3965775359c5802dc9df1a510 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.918490759365935, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_d9d9f6ba947409dae2727c90e5d4f879 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ee434ed962c27b35ac3eb506a3f55f0 = $(`<div id="html_4ee434ed962c27b35ac3eb506a3f55f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d9d9f6ba947409dae2727c90e5d4f879.setContent(html_4ee434ed962c27b35ac3eb506a3f55f0);\\n \\n \\n\\n circle_marker_e1112ff3965775359c5802dc9df1a510.bindPopup(popup_d9d9f6ba947409dae2727c90e5d4f879)\\n ;\\n\\n \\n \\n \\n var circle_marker_34271dd8eb98802e91e0b407f5e1377e = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.836022431559495, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_9032c7490d21f5426d854d896b4533db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_caacd25cc1d9eadaf7b6246fc0be1c3b = $(`<div id="html_caacd25cc1d9eadaf7b6246fc0be1c3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9032c7490d21f5426d854d896b4533db.setContent(html_caacd25cc1d9eadaf7b6246fc0be1c3b);\\n \\n \\n\\n circle_marker_34271dd8eb98802e91e0b407f5e1377e.bindPopup(popup_9032c7490d21f5426d854d896b4533db)\\n ;\\n\\n \\n \\n \\n var circle_marker_aed81d1f0a685d893fa970d6f89a7290 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_88d1205ba22b57c63885b791f167b9c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07e63d653a5a5c53293a074bf8014e73 = $(`<div id="html_07e63d653a5a5c53293a074bf8014e73" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88d1205ba22b57c63885b791f167b9c0.setContent(html_07e63d653a5a5c53293a074bf8014e73);\\n \\n \\n\\n circle_marker_aed81d1f0a685d893fa970d6f89a7290.bindPopup(popup_88d1205ba22b57c63885b791f167b9c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_3749e6033a760f10f7e2d1c7b2d13d24 = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_dfd031836df9f2874b14028a4349a8a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb53ee561ca5d995a31eba279d296607 = $(`<div id="html_eb53ee561ca5d995a31eba279d296607" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dfd031836df9f2874b14028a4349a8a5.setContent(html_eb53ee561ca5d995a31eba279d296607);\\n \\n \\n\\n circle_marker_3749e6033a760f10f7e2d1c7b2d13d24.bindPopup(popup_dfd031836df9f2874b14028a4349a8a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_155d1ba3556d0811866fb580d9b808c9 = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_ce2f84a65201ac865ff7d865c37c9230 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ee15634aa69295fce4e2353a03455d2 = $(`<div id="html_8ee15634aa69295fce4e2353a03455d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce2f84a65201ac865ff7d865c37c9230.setContent(html_8ee15634aa69295fce4e2353a03455d2);\\n \\n \\n\\n circle_marker_155d1ba3556d0811866fb580d9b808c9.bindPopup(popup_ce2f84a65201ac865ff7d865c37c9230)\\n ;\\n\\n \\n \\n \\n var circle_marker_0f644e7abc6446f6a6a6d3291e31484b = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.753945931439391, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_c8094c0d4377cd13e101aaf9e16525fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ab25fca236aa41046db5b53df26e867 = $(`<div id="html_7ab25fca236aa41046db5b53df26e867" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8094c0d4377cd13e101aaf9e16525fc.setContent(html_7ab25fca236aa41046db5b53df26e867);\\n \\n \\n\\n circle_marker_0f644e7abc6446f6a6a6d3291e31484b.bindPopup(popup_c8094c0d4377cd13e101aaf9e16525fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_8789470f96e86442fe755144a44cc58e = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_bafbabad94a7d237626063c15e098335 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c57e8a8c4672dd1091f3212e4de391f = $(`<div id="html_8c57e8a8c4672dd1091f3212e4de391f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bafbabad94a7d237626063c15e098335.setContent(html_8c57e8a8c4672dd1091f3212e4de391f);\\n \\n \\n\\n circle_marker_8789470f96e86442fe755144a44cc58e.bindPopup(popup_bafbabad94a7d237626063c15e098335)\\n ;\\n\\n \\n \\n \\n var circle_marker_28a4346ab9c5183b4cdae02e04926a3c = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.203090409536582, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_8e01dd5d1178c2fd5a710fe437edb74e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1e4b5ac8435bdcc9fecc307e3d57f0d = $(`<div id="html_a1e4b5ac8435bdcc9fecc307e3d57f0d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e01dd5d1178c2fd5a710fe437edb74e.setContent(html_a1e4b5ac8435bdcc9fecc307e3d57f0d);\\n \\n \\n\\n circle_marker_28a4346ab9c5183b4cdae02e04926a3c.bindPopup(popup_8e01dd5d1178c2fd5a710fe437edb74e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e23bc958fff696fa19091699531f604f = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_111d7034df4e8b62714cb79d42ce6a9d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b442371d4b9ba35fda9f9313ecf1410 = $(`<div id="html_5b442371d4b9ba35fda9f9313ecf1410" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_111d7034df4e8b62714cb79d42ce6a9d.setContent(html_5b442371d4b9ba35fda9f9313ecf1410);\\n \\n \\n\\n circle_marker_e23bc958fff696fa19091699531f604f.bindPopup(popup_111d7034df4e8b62714cb79d42ce6a9d)\\n ;\\n\\n \\n \\n \\n var circle_marker_edfa7d1d2f423c9e5d2f430849ec9515 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_48784f032c46510bc1cce558303a1d55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa3dc44f490fc528878f55cb503326a2 = $(`<div id="html_aa3dc44f490fc528878f55cb503326a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_48784f032c46510bc1cce558303a1d55.setContent(html_aa3dc44f490fc528878f55cb503326a2);\\n \\n \\n\\n circle_marker_edfa7d1d2f423c9e5d2f430849ec9515.bindPopup(popup_48784f032c46510bc1cce558303a1d55)\\n ;\\n\\n \\n \\n \\n var circle_marker_29f3c4dd21c618d07e2832dd01c37142 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_5c24a00ab48febbd023cba3367a9a371 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_89f1d1a26441afd4212aab0070626356 = $(`<div id="html_89f1d1a26441afd4212aab0070626356" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c24a00ab48febbd023cba3367a9a371.setContent(html_89f1d1a26441afd4212aab0070626356);\\n \\n \\n\\n circle_marker_29f3c4dd21c618d07e2832dd01c37142.bindPopup(popup_5c24a00ab48febbd023cba3367a9a371)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a2d375977e523a78f1fd986590c9be0 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.61809077155419, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_5cf66c77eb9a2d73ecdf61776d42177a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b85a97eae0c3d55801200c713d9a8ded = $(`<div id="html_b85a97eae0c3d55801200c713d9a8ded" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5cf66c77eb9a2d73ecdf61776d42177a.setContent(html_b85a97eae0c3d55801200c713d9a8ded);\\n \\n \\n\\n circle_marker_2a2d375977e523a78f1fd986590c9be0.bindPopup(popup_5cf66c77eb9a2d73ecdf61776d42177a)\\n ;\\n\\n \\n \\n \\n var circle_marker_156d48f40485e063f19522069250c944 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.840435166640879, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_e9d2cbc802e46a9fee6c353dd73295c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0334e117bf744829595da0c84404ff49 = $(`<div id="html_0334e117bf744829595da0c84404ff49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9d2cbc802e46a9fee6c353dd73295c7.setContent(html_0334e117bf744829595da0c84404ff49);\\n \\n \\n\\n circle_marker_156d48f40485e063f19522069250c944.bindPopup(popup_e9d2cbc802e46a9fee6c353dd73295c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a3c19728f0c07699a1734b383e7fe9d = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_6afe14fdee47b52c8bb993b903b2e49e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f257e712da3834d1eb8e864647ae8fd = $(`<div id="html_4f257e712da3834d1eb8e864647ae8fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6afe14fdee47b52c8bb993b903b2e49e.setContent(html_4f257e712da3834d1eb8e864647ae8fd);\\n \\n \\n\\n circle_marker_9a3c19728f0c07699a1734b383e7fe9d.bindPopup(popup_6afe14fdee47b52c8bb993b903b2e49e)\\n ;\\n\\n \\n \\n \\n var circle_marker_320a45534c985a4b8867c29a764496f1 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_7a579eaa01ba38519b89dc121197bab0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_16ccf6e97b0df8bc73576c4efead5ee8 = $(`<div id="html_16ccf6e97b0df8bc73576c4efead5ee8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a579eaa01ba38519b89dc121197bab0.setContent(html_16ccf6e97b0df8bc73576c4efead5ee8);\\n \\n \\n\\n circle_marker_320a45534c985a4b8867c29a764496f1.bindPopup(popup_7a579eaa01ba38519b89dc121197bab0)\\n ;\\n\\n \\n \\n \\n var circle_marker_83441fb262e1163697ffa3c234ad7999 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_ecaf26a6e5d5adf5d21976db2b778e11 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97ac9715d111635f6d23195a1becb1a3 = $(`<div id="html_97ac9715d111635f6d23195a1becb1a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ecaf26a6e5d5adf5d21976db2b778e11.setContent(html_97ac9715d111635f6d23195a1becb1a3);\\n \\n \\n\\n circle_marker_83441fb262e1163697ffa3c234ad7999.bindPopup(popup_ecaf26a6e5d5adf5d21976db2b778e11)\\n ;\\n\\n \\n \\n \\n var circle_marker_71f520391e13bb24301240310983cef2 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_d5d388cf03f53fb0ed2c403bc67a7423 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33af5a3bbab1d2f72cc9268c7f7429dd = $(`<div id="html_33af5a3bbab1d2f72cc9268c7f7429dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5d388cf03f53fb0ed2c403bc67a7423.setContent(html_33af5a3bbab1d2f72cc9268c7f7429dd);\\n \\n \\n\\n circle_marker_71f520391e13bb24301240310983cef2.bindPopup(popup_d5d388cf03f53fb0ed2c403bc67a7423)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a6a6f178452b0ae542bc1b5c573384e = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_a7b87297f61fb1e28f23aa5913fcfb35 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_121db258ba052c007ee2b6b77fdc2a9d = $(`<div id="html_121db258ba052c007ee2b6b77fdc2a9d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7b87297f61fb1e28f23aa5913fcfb35.setContent(html_121db258ba052c007ee2b6b77fdc2a9d);\\n \\n \\n\\n circle_marker_7a6a6f178452b0ae542bc1b5c573384e.bindPopup(popup_a7b87297f61fb1e28f23aa5913fcfb35)\\n ;\\n\\n \\n \\n \\n var circle_marker_ab57b61ddf19dac34335a6a1aa33ed3f = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_ff43776e1484fe9b1165abe8f31b8621 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ecf946afbac195550bc8c34d2f01da4a = $(`<div id="html_ecf946afbac195550bc8c34d2f01da4a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff43776e1484fe9b1165abe8f31b8621.setContent(html_ecf946afbac195550bc8c34d2f01da4a);\\n \\n \\n\\n circle_marker_ab57b61ddf19dac34335a6a1aa33ed3f.bindPopup(popup_ff43776e1484fe9b1165abe8f31b8621)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4b817ac0e1fad562004c29634a01425 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_0c75cd49894c87e066bb2c9ba85b5f2f);\\n \\n \\n var popup_f3192ef48c1057e95cff7793ceb7e783 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0653ba14c0167ed6538af529affe057c = $(`<div id="html_0653ba14c0167ed6538af529affe057c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f3192ef48c1057e95cff7793ceb7e783.setContent(html_0653ba14c0167ed6538af529affe057c);\\n \\n \\n\\n circle_marker_c4b817ac0e1fad562004c29634a01425.bindPopup(popup_f3192ef48c1057e95cff7793ceb7e783)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Honeysuckle, European fly\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_d21c2cf9f5709bb54e0594dd9b9a6abf {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_d21c2cf9f5709bb54e0594dd9b9a6abf" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_d21c2cf9f5709bb54e0594dd9b9a6abf = L.map(\\n "map_d21c2cf9f5709bb54e0594dd9b9a6abf",\\n {\\n center: [42.731836279446675, -73.22919728337214],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_07eea45a15c76aa9a38dbc07b77099dc = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var circle_marker_18e3a57783a028cf5adcb42fcb5054b7 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_fad84d3bf3081028562eb609be6ef34d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74e20b09aaef21e47d18b1fcf4e3017e = $(`<div id="html_74e20b09aaef21e47d18b1fcf4e3017e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fad84d3bf3081028562eb609be6ef34d.setContent(html_74e20b09aaef21e47d18b1fcf4e3017e);\\n \\n \\n\\n circle_marker_18e3a57783a028cf5adcb42fcb5054b7.bindPopup(popup_fad84d3bf3081028562eb609be6ef34d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c9c0bbd15f8e80a041e653ae772cd7e9 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_f06a2d3bc137b6907b8dd7c9ecb0ce78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2502e2eddba3456d6e5940d6300ffa3d = $(`<div id="html_2502e2eddba3456d6e5940d6300ffa3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f06a2d3bc137b6907b8dd7c9ecb0ce78.setContent(html_2502e2eddba3456d6e5940d6300ffa3d);\\n \\n \\n\\n circle_marker_c9c0bbd15f8e80a041e653ae772cd7e9.bindPopup(popup_f06a2d3bc137b6907b8dd7c9ecb0ce78)\\n ;\\n\\n \\n \\n \\n var circle_marker_8949f2a416c755c5ee83541f44d72efc = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_39bb68e110a3e6e4f74777c9f5dcb804 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d33a10ba497f2e5b002bda3f9f70fbd = $(`<div id="html_7d33a10ba497f2e5b002bda3f9f70fbd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39bb68e110a3e6e4f74777c9f5dcb804.setContent(html_7d33a10ba497f2e5b002bda3f9f70fbd);\\n \\n \\n\\n circle_marker_8949f2a416c755c5ee83541f44d72efc.bindPopup(popup_39bb68e110a3e6e4f74777c9f5dcb804)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb12c20c37686f0cfa0342c23553ded1 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_4afdafb488338c5dc7bf3156f321461a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2f6e519a1b469fd0811dff890ded1bc8 = $(`<div id="html_2f6e519a1b469fd0811dff890ded1bc8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4afdafb488338c5dc7bf3156f321461a.setContent(html_2f6e519a1b469fd0811dff890ded1bc8);\\n \\n \\n\\n circle_marker_cb12c20c37686f0cfa0342c23553ded1.bindPopup(popup_4afdafb488338c5dc7bf3156f321461a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3c337116489631270d0107245572b5c = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.108953969950291, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_13bfa720647467bc95f2177e3f1b4f62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ef39485057905728e7dad42309b57b2 = $(`<div id="html_4ef39485057905728e7dad42309b57b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13bfa720647467bc95f2177e3f1b4f62.setContent(html_4ef39485057905728e7dad42309b57b2);\\n \\n \\n\\n circle_marker_c3c337116489631270d0107245572b5c.bindPopup(popup_13bfa720647467bc95f2177e3f1b4f62)\\n ;\\n\\n \\n \\n \\n var circle_marker_9403b3fc98cbe97a0d3559a40b37f4af = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.530966601401967, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_9b1f6aee0b6bca0eb8bad6b09b8edcea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac14d36e5e95d940b3d079ead2a5c57b = $(`<div id="html_ac14d36e5e95d940b3d079ead2a5c57b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b1f6aee0b6bca0eb8bad6b09b8edcea.setContent(html_ac14d36e5e95d940b3d079ead2a5c57b);\\n \\n \\n\\n circle_marker_9403b3fc98cbe97a0d3559a40b37f4af.bindPopup(popup_9b1f6aee0b6bca0eb8bad6b09b8edcea)\\n ;\\n\\n \\n \\n \\n var circle_marker_37f797ccc72b2162805104f335d7f7ab = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_ebdaa3fe3bc0d3ff67ccc793bbc59a86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c5274ddf7786b289a873c4115649a0d = $(`<div id="html_7c5274ddf7786b289a873c4115649a0d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebdaa3fe3bc0d3ff67ccc793bbc59a86.setContent(html_7c5274ddf7786b289a873c4115649a0d);\\n \\n \\n\\n circle_marker_37f797ccc72b2162805104f335d7f7ab.bindPopup(popup_ebdaa3fe3bc0d3ff67ccc793bbc59a86)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b1e1f976f330fd4cb5fa5ee91f33eea = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_24b5e20de3cb11aaca9f41130985fc39 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9bdce06892a36bc263b5cb01a686f17 = $(`<div id="html_f9bdce06892a36bc263b5cb01a686f17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24b5e20de3cb11aaca9f41130985fc39.setContent(html_f9bdce06892a36bc263b5cb01a686f17);\\n \\n \\n\\n circle_marker_2b1e1f976f330fd4cb5fa5ee91f33eea.bindPopup(popup_24b5e20de3cb11aaca9f41130985fc39)\\n ;\\n\\n \\n \\n \\n var circle_marker_5215e5b5d01689cbf011ad878af9bf34 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_65b377d904b3ee33d76fbade6db37513 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64c2110121de457213daf9cfc0cf988f = $(`<div id="html_64c2110121de457213daf9cfc0cf988f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65b377d904b3ee33d76fbade6db37513.setContent(html_64c2110121de457213daf9cfc0cf988f);\\n \\n \\n\\n circle_marker_5215e5b5d01689cbf011ad878af9bf34.bindPopup(popup_65b377d904b3ee33d76fbade6db37513)\\n ;\\n\\n \\n \\n \\n var circle_marker_61e3469141bb9451cc17f6b2562740df = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.997417539138688, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_ba45f4c62c57c0f63de20879ec5b1a74 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_abfa880b8c2f520b0db2e24293555cc3 = $(`<div id="html_abfa880b8c2f520b0db2e24293555cc3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba45f4c62c57c0f63de20879ec5b1a74.setContent(html_abfa880b8c2f520b0db2e24293555cc3);\\n \\n \\n\\n circle_marker_61e3469141bb9451cc17f6b2562740df.bindPopup(popup_ba45f4c62c57c0f63de20879ec5b1a74)\\n ;\\n\\n \\n \\n \\n var circle_marker_002d158fb9bd50df07d1fbb6408ac026 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_1a8a6f9d5aaa103019dfb58974735825 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10dac91587825a773fea0d1cccbdb91b = $(`<div id="html_10dac91587825a773fea0d1cccbdb91b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a8a6f9d5aaa103019dfb58974735825.setContent(html_10dac91587825a773fea0d1cccbdb91b);\\n \\n \\n\\n circle_marker_002d158fb9bd50df07d1fbb6408ac026.bindPopup(popup_1a8a6f9d5aaa103019dfb58974735825)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d2b97d71dcbb13c9ad643f20ae3b954 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_eed44c2c6291860d37133b416c27e41e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4bfc1634e8e91dca5fa51e76ab61455e = $(`<div id="html_4bfc1634e8e91dca5fa51e76ab61455e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eed44c2c6291860d37133b416c27e41e.setContent(html_4bfc1634e8e91dca5fa51e76ab61455e);\\n \\n \\n\\n circle_marker_1d2b97d71dcbb13c9ad643f20ae3b954.bindPopup(popup_eed44c2c6291860d37133b416c27e41e)\\n ;\\n\\n \\n \\n \\n var circle_marker_43f8d83a2236e8a3ca67e4d0adca34f7 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_6b0cd6f3115835887620cf0d1ffefe8c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b81a07ca4e52d3b6b78c321ad500baa = $(`<div id="html_2b81a07ca4e52d3b6b78c321ad500baa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b0cd6f3115835887620cf0d1ffefe8c.setContent(html_2b81a07ca4e52d3b6b78c321ad500baa);\\n \\n \\n\\n circle_marker_43f8d83a2236e8a3ca67e4d0adca34f7.bindPopup(popup_6b0cd6f3115835887620cf0d1ffefe8c)\\n ;\\n\\n \\n \\n \\n var circle_marker_dcc47c6521ab3c776292ac65fa79a767 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_796a599e7147392b77295193f28af95a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_235ae925977c762e59ee7a50734a6444 = $(`<div id="html_235ae925977c762e59ee7a50734a6444" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_796a599e7147392b77295193f28af95a.setContent(html_235ae925977c762e59ee7a50734a6444);\\n \\n \\n\\n circle_marker_dcc47c6521ab3c776292ac65fa79a767.bindPopup(popup_796a599e7147392b77295193f28af95a)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a94259ce2736e0865fccd3f5add0ced = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_2e3b86d98784b2b4b0e7d3d00025a3ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_788183bfd2b6d3832bf658ff33d68a5f = $(`<div id="html_788183bfd2b6d3832bf658ff33d68a5f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e3b86d98784b2b4b0e7d3d00025a3ee.setContent(html_788183bfd2b6d3832bf658ff33d68a5f);\\n \\n \\n\\n circle_marker_0a94259ce2736e0865fccd3f5add0ced.bindPopup(popup_2e3b86d98784b2b4b0e7d3d00025a3ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_c590a2e5f7774a460b2d976990d208e9 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_eacf47a39e7286e0f70497341d03e2dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f048c36ed3b20bf45f932a56421dcbc2 = $(`<div id="html_f048c36ed3b20bf45f932a56421dcbc2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eacf47a39e7286e0f70497341d03e2dd.setContent(html_f048c36ed3b20bf45f932a56421dcbc2);\\n \\n \\n\\n circle_marker_c590a2e5f7774a460b2d976990d208e9.bindPopup(popup_eacf47a39e7286e0f70497341d03e2dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_49817fd7c2261f63efe66ad6dbb6da5b = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_f7746d3c5716c70256ab9beb7428b334 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa575ee0a6f2fe439ba61db83536107a = $(`<div id="html_aa575ee0a6f2fe439ba61db83536107a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7746d3c5716c70256ab9beb7428b334.setContent(html_aa575ee0a6f2fe439ba61db83536107a);\\n \\n \\n\\n circle_marker_49817fd7c2261f63efe66ad6dbb6da5b.bindPopup(popup_f7746d3c5716c70256ab9beb7428b334)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4509c30501380665cfedcc07fc7bb26 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_cb47c68610c467a0135bdf0b441add30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1a987e64bbd9a56b230c60a40e5518f = $(`<div id="html_c1a987e64bbd9a56b230c60a40e5518f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb47c68610c467a0135bdf0b441add30.setContent(html_c1a987e64bbd9a56b230c60a40e5518f);\\n \\n \\n\\n circle_marker_a4509c30501380665cfedcc07fc7bb26.bindPopup(popup_cb47c68610c467a0135bdf0b441add30)\\n ;\\n\\n \\n \\n \\n var circle_marker_52d6eeccc0f38a3ac2b2b65a47ad4244 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_23670d13d2401a953985148f52842239 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94e91f33147fa18de765d5c0cdf5bdab = $(`<div id="html_94e91f33147fa18de765d5c0cdf5bdab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23670d13d2401a953985148f52842239.setContent(html_94e91f33147fa18de765d5c0cdf5bdab);\\n \\n \\n\\n circle_marker_52d6eeccc0f38a3ac2b2b65a47ad4244.bindPopup(popup_23670d13d2401a953985148f52842239)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d864171be11ee9b5daaace1e0053730 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.140011726956917, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_092a73aeaa07f7fd55ec244b4ee32dd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc187651cfba17d93bae5d71f99c091c = $(`<div id="html_cc187651cfba17d93bae5d71f99c091c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_092a73aeaa07f7fd55ec244b4ee32dd0.setContent(html_cc187651cfba17d93bae5d71f99c091c);\\n \\n \\n\\n circle_marker_5d864171be11ee9b5daaace1e0053730.bindPopup(popup_092a73aeaa07f7fd55ec244b4ee32dd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_bab9c83c55becd67215d0c1a76a89613 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_d21c2cf9f5709bb54e0594dd9b9a6abf);\\n \\n \\n var popup_7525c582d32089ce87513745432c699e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c3cec11acfa3fc9878fec1eb6a9e46e0 = $(`<div id="html_c3cec11acfa3fc9878fec1eb6a9e46e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7525c582d32089ce87513745432c699e.setContent(html_c3cec11acfa3fc9878fec1eb6a9e46e0);\\n \\n \\n\\n circle_marker_bab9c83c55becd67215d0c1a76a89613.bindPopup(popup_7525c582d32089ce87513745432c699e)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Honeysuckle, Morrow\'s\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_16a3911197768fa0ff3500c91bcfee7d {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_16a3911197768fa0ff3500c91bcfee7d" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_16a3911197768fa0ff3500c91bcfee7d = L.map(\\n "map_16a3911197768fa0ff3500c91bcfee7d",\\n {\\n center: [42.730932307889134, -73.22797001700748],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_7daf0b3229e11319957fbda49838e269 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var circle_marker_4af95ee44dc691aae9deb40507982186 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.90988298942671, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_84e5cf6f91d54370c05daf433f8ae285 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1e2b59754efd0ab8471bfba4a4f29e7 = $(`<div id="html_c1e2b59754efd0ab8471bfba4a4f29e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84e5cf6f91d54370c05daf433f8ae285.setContent(html_c1e2b59754efd0ab8471bfba4a4f29e7);\\n \\n \\n\\n circle_marker_4af95ee44dc691aae9deb40507982186.bindPopup(popup_84e5cf6f91d54370c05daf433f8ae285)\\n ;\\n\\n \\n \\n \\n var circle_marker_192bf1e1e5fa5b22deb99fc1118179ff = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.920620580763856, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_a0388c6664ae48af297b623052f54574 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c3c6c38a3c8dd990530258c3a43e751 = $(`<div id="html_5c3c6c38a3c8dd990530258c3a43e751" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0388c6664ae48af297b623052f54574.setContent(html_5c3c6c38a3c8dd990530258c3a43e751);\\n \\n \\n\\n circle_marker_192bf1e1e5fa5b22deb99fc1118179ff.bindPopup(popup_a0388c6664ae48af297b623052f54574)\\n ;\\n\\n \\n \\n \\n var circle_marker_a215cac7cd642c75cbdcc09fd470730c = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.673807981960539, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_e9a6e1d6107d7aaf77533938ac7df53f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49cce4da4eccca7db53416e242446969 = $(`<div id="html_49cce4da4eccca7db53416e242446969" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9a6e1d6107d7aaf77533938ac7df53f.setContent(html_49cce4da4eccca7db53416e242446969);\\n \\n \\n\\n circle_marker_a215cac7cd642c75cbdcc09fd470730c.bindPopup(popup_e9a6e1d6107d7aaf77533938ac7df53f)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc4e8c3df7a34c06d9e9fbc45d093b70 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.222008245644752, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_371f87d79326034aba1073148b3e7853 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd01d7887ba8e97793d4adc58d51f8c6 = $(`<div id="html_cd01d7887ba8e97793d4adc58d51f8c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_371f87d79326034aba1073148b3e7853.setContent(html_cd01d7887ba8e97793d4adc58d51f8c6);\\n \\n \\n\\n circle_marker_dc4e8c3df7a34c06d9e9fbc45d093b70.bindPopup(popup_371f87d79326034aba1073148b3e7853)\\n ;\\n\\n \\n \\n \\n var circle_marker_0530ed676b0fce89e526671fd8e88746 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_002a690bb4ab8f76b37642173fefed29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bcb40a7e0c9f722d4b94b3646c5a6c78 = $(`<div id="html_bcb40a7e0c9f722d4b94b3646c5a6c78" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_002a690bb4ab8f76b37642173fefed29.setContent(html_bcb40a7e0c9f722d4b94b3646c5a6c78);\\n \\n \\n\\n circle_marker_0530ed676b0fce89e526671fd8e88746.bindPopup(popup_002a690bb4ab8f76b37642173fefed29)\\n ;\\n\\n \\n \\n \\n var circle_marker_d845b9cd763f1ef806eba53760bf965d = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.530966601401967, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_5eaad8d7e3b9e9aa0b51b1af8ffa858b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bad215de30f04ef92a3ffccc8053290 = $(`<div id="html_1bad215de30f04ef92a3ffccc8053290" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5eaad8d7e3b9e9aa0b51b1af8ffa858b.setContent(html_1bad215de30f04ef92a3ffccc8053290);\\n \\n \\n\\n circle_marker_d845b9cd763f1ef806eba53760bf965d.bindPopup(popup_5eaad8d7e3b9e9aa0b51b1af8ffa858b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ce4fe7a5f17130f40a800b81b5dcb11 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.272643200907131, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_73dc89480cbfc30f20308e1053aa35f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa181938709802cf0f20e7fbb3f9a863 = $(`<div id="html_aa181938709802cf0f20e7fbb3f9a863" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73dc89480cbfc30f20308e1053aa35f9.setContent(html_aa181938709802cf0f20e7fbb3f9a863);\\n \\n \\n\\n circle_marker_9ce4fe7a5f17130f40a800b81b5dcb11.bindPopup(popup_73dc89480cbfc30f20308e1053aa35f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e0cefc2a09e9174a323916691cb549c = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_3768bae403e259380bfced94ddffeaf7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0cfadb8fc27629c972f03688b57ef44e = $(`<div id="html_0cfadb8fc27629c972f03688b57ef44e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3768bae403e259380bfced94ddffeaf7.setContent(html_0cfadb8fc27629c972f03688b57ef44e);\\n \\n \\n\\n circle_marker_2e0cefc2a09e9174a323916691cb549c.bindPopup(popup_3768bae403e259380bfced94ddffeaf7)\\n ;\\n\\n \\n \\n \\n var circle_marker_b68b52a024d0a8469aa3d2a67d576592 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_c3cd7861aae91d01390e7348443f5735 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de2a417bc7e8a59f14c3eab0d4209201 = $(`<div id="html_de2a417bc7e8a59f14c3eab0d4209201" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3cd7861aae91d01390e7348443f5735.setContent(html_de2a417bc7e8a59f14c3eab0d4209201);\\n \\n \\n\\n circle_marker_b68b52a024d0a8469aa3d2a67d576592.bindPopup(popup_c3cd7861aae91d01390e7348443f5735)\\n ;\\n\\n \\n \\n \\n var circle_marker_80f08ef12c343d1cc4bee14420faf789 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_ad98f79f10dbc45ad3c85eb45a6fbc9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_afb60949f25bf6cf191c3486f38318d0 = $(`<div id="html_afb60949f25bf6cf191c3486f38318d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad98f79f10dbc45ad3c85eb45a6fbc9c.setContent(html_afb60949f25bf6cf191c3486f38318d0);\\n \\n \\n\\n circle_marker_80f08ef12c343d1cc4bee14420faf789.bindPopup(popup_ad98f79f10dbc45ad3c85eb45a6fbc9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d032a952dae0ed55ab6159c5b763e2c = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_356c229e479abbaf9d3edca5ffcfd2b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cb591a8da51b25fe0920333b51a19e6 = $(`<div id="html_9cb591a8da51b25fe0920333b51a19e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_356c229e479abbaf9d3edca5ffcfd2b7.setContent(html_9cb591a8da51b25fe0920333b51a19e6);\\n \\n \\n\\n circle_marker_2d032a952dae0ed55ab6159c5b763e2c.bindPopup(popup_356c229e479abbaf9d3edca5ffcfd2b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_f20636d4ab4d9e86e5357b7cd6e8d0b9 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.982787485166879, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_2bdf3e2fe591f775caad684115fcc7a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_11d5ad8dff8c5020b2ec98a996fcc27a = $(`<div id="html_11d5ad8dff8c5020b2ec98a996fcc27a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2bdf3e2fe591f775caad684115fcc7a6.setContent(html_11d5ad8dff8c5020b2ec98a996fcc27a);\\n \\n \\n\\n circle_marker_f20636d4ab4d9e86e5357b7cd6e8d0b9.bindPopup(popup_2bdf3e2fe591f775caad684115fcc7a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_6570d84e504ed4939ecb81adee47c676 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.046265044040321, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_e02ba0ba0efbcc4c6f285c19fd5ffd6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f5ac649740ae44e2fa4d4f6c41df623a = $(`<div id="html_f5ac649740ae44e2fa4d4f6c41df623a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e02ba0ba0efbcc4c6f285c19fd5ffd6a.setContent(html_f5ac649740ae44e2fa4d4f6c41df623a);\\n \\n \\n\\n circle_marker_6570d84e504ed4939ecb81adee47c676.bindPopup(popup_e02ba0ba0efbcc4c6f285c19fd5ffd6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0b646572728e598b9d49f92014d1e71 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_276cbc7964c4d29ba209793d97fa9d19 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b87db8b8b196d534ab2499f49fee8d8 = $(`<div id="html_8b87db8b8b196d534ab2499f49fee8d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_276cbc7964c4d29ba209793d97fa9d19.setContent(html_8b87db8b8b196d534ab2499f49fee8d8);\\n \\n \\n\\n circle_marker_c0b646572728e598b9d49f92014d1e71.bindPopup(popup_276cbc7964c4d29ba209793d97fa9d19)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a9c21118e54eb1e528eee4581c2966d = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.282549314314218, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_fa4443f9905b1afc368b3443010954c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf4adbcaa9586b2caaab0725ea7ec88b = $(`<div id="html_cf4adbcaa9586b2caaab0725ea7ec88b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa4443f9905b1afc368b3443010954c8.setContent(html_cf4adbcaa9586b2caaab0725ea7ec88b);\\n \\n \\n\\n circle_marker_6a9c21118e54eb1e528eee4581c2966d.bindPopup(popup_fa4443f9905b1afc368b3443010954c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_fbb06bb68b6f79f8aacb3454d70dbd56 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_ca0bf67ce2debf9a2a2b61e04ba83f02 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc605f9a0e91389be58463573acc2f9c = $(`<div id="html_bc605f9a0e91389be58463573acc2f9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca0bf67ce2debf9a2a2b61e04ba83f02.setContent(html_bc605f9a0e91389be58463573acc2f9c);\\n \\n \\n\\n circle_marker_fbb06bb68b6f79f8aacb3454d70dbd56.bindPopup(popup_ca0bf67ce2debf9a2a2b61e04ba83f02)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5db822be8b7cb41473b7cc0924b181d = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.180387232371033, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_c8dcbf6aabd4f90528373c40152f2492 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76cefe02e5ca57e97e8b5ee683237bad = $(`<div id="html_76cefe02e5ca57e97e8b5ee683237bad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8dcbf6aabd4f90528373c40152f2492.setContent(html_76cefe02e5ca57e97e8b5ee683237bad);\\n \\n \\n\\n circle_marker_a5db822be8b7cb41473b7cc0924b181d.bindPopup(popup_c8dcbf6aabd4f90528373c40152f2492)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f6d4854b07c6bbf66dfe76beffd5d0b = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_94a57262d7a5ec6b501a34d8d938bb89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_072fd45dbc2b1ffc56e6359be55744e7 = $(`<div id="html_072fd45dbc2b1ffc56e6359be55744e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94a57262d7a5ec6b501a34d8d938bb89.setContent(html_072fd45dbc2b1ffc56e6359be55744e7);\\n \\n \\n\\n circle_marker_2f6d4854b07c6bbf66dfe76beffd5d0b.bindPopup(popup_94a57262d7a5ec6b501a34d8d938bb89)\\n ;\\n\\n \\n \\n \\n var circle_marker_56b98ab74dcfdafd958265a6b6ec2aeb = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_1f6759432413faed4450cfbb86841a53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f39da77bcd2c6fb1bb0e012b0e582b19 = $(`<div id="html_f39da77bcd2c6fb1bb0e012b0e582b19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f6759432413faed4450cfbb86841a53.setContent(html_f39da77bcd2c6fb1bb0e012b0e582b19);\\n \\n \\n\\n circle_marker_56b98ab74dcfdafd958265a6b6ec2aeb.bindPopup(popup_1f6759432413faed4450cfbb86841a53)\\n ;\\n\\n \\n \\n \\n var circle_marker_c462413748225cbba194cdbe258f8489 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_1f0466d6afe39e95205044c3089ad77c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6aea12456bf2fe28c0a68a5153ed759b = $(`<div id="html_6aea12456bf2fe28c0a68a5153ed759b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f0466d6afe39e95205044c3089ad77c.setContent(html_6aea12456bf2fe28c0a68a5153ed759b);\\n \\n \\n\\n circle_marker_c462413748225cbba194cdbe258f8489.bindPopup(popup_1f0466d6afe39e95205044c3089ad77c)\\n ;\\n\\n \\n \\n \\n var circle_marker_10657b90a982ec9e068f7544390b8800 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_03ee3d7bb3207bf2fb536481514f5bf2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4593ccaacee95831f696f691bf5e5211 = $(`<div id="html_4593ccaacee95831f696f691bf5e5211" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03ee3d7bb3207bf2fb536481514f5bf2.setContent(html_4593ccaacee95831f696f691bf5e5211);\\n \\n \\n\\n circle_marker_10657b90a982ec9e068f7544390b8800.bindPopup(popup_03ee3d7bb3207bf2fb536481514f5bf2)\\n ;\\n\\n \\n \\n \\n var circle_marker_6222adaf808181bc4bb755b20b0db8a5 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_16a3911197768fa0ff3500c91bcfee7d);\\n \\n \\n var popup_455506b2fffbf8f5513d9dddb6262449 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fcd0eb1addb8dc9408bda0e6e62978b0 = $(`<div id="html_fcd0eb1addb8dc9408bda0e6e62978b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_455506b2fffbf8f5513d9dddb6262449.setContent(html_fcd0eb1addb8dc9408bda0e6e62978b0);\\n \\n \\n\\n circle_marker_6222adaf808181bc4bb755b20b0db8a5.bindPopup(popup_455506b2fffbf8f5513d9dddb6262449)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Honeysuckle, Tartarian\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_ea2c205c8ad79d79328481a3ca5f7db2 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_ea2c205c8ad79d79328481a3ca5f7db2" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_ea2c205c8ad79d79328481a3ca5f7db2 = L.map(\\n "map_ea2c205c8ad79d79328481a3ca5f7db2",\\n {\\n center: [42.730934295709964, -73.23657849924581],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_4f6c89b5a78e0e0d205a383f3e033155 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var circle_marker_73e9b647348058bf2d5c3f3cb0d19783 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_e1ff996f0cbbda9d52476b8d2b5318ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eba00c5c3b62c28e2440714ad1db30f3 = $(`<div id="html_eba00c5c3b62c28e2440714ad1db30f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1ff996f0cbbda9d52476b8d2b5318ec.setContent(html_eba00c5c3b62c28e2440714ad1db30f3);\\n \\n \\n\\n circle_marker_73e9b647348058bf2d5c3f3cb0d19783.bindPopup(popup_e1ff996f0cbbda9d52476b8d2b5318ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_33d7559cad5bda3d453f531c0805fe3c = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_43c3d53fa8bbfca499a8cdd80e2b813d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41529e1d58a2f7a41a04982375990d72 = $(`<div id="html_41529e1d58a2f7a41a04982375990d72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43c3d53fa8bbfca499a8cdd80e2b813d.setContent(html_41529e1d58a2f7a41a04982375990d72);\\n \\n \\n\\n circle_marker_33d7559cad5bda3d453f531c0805fe3c.bindPopup(popup_43c3d53fa8bbfca499a8cdd80e2b813d)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca9f88804158ebda9b00d6b8c473aa12 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.180387232371033, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_cb356b90ddb42837ae865990bd5cac6f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5d12c7643c1f13ff0151892065b17fc7 = $(`<div id="html_5d12c7643c1f13ff0151892065b17fc7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb356b90ddb42837ae865990bd5cac6f.setContent(html_5d12c7643c1f13ff0151892065b17fc7);\\n \\n \\n\\n circle_marker_ca9f88804158ebda9b00d6b8c473aa12.bindPopup(popup_cb356b90ddb42837ae865990bd5cac6f)\\n ;\\n\\n \\n \\n \\n var circle_marker_404c37830aaa4df3a8a90a54f0504d68 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_cd02d88ab980601f749fe82a6d6e3bcd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8dfc3756ff459d8d8d5d0ac0f7a8e952 = $(`<div id="html_8dfc3756ff459d8d8d5d0ac0f7a8e952" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd02d88ab980601f749fe82a6d6e3bcd.setContent(html_8dfc3756ff459d8d8d5d0ac0f7a8e952);\\n \\n \\n\\n circle_marker_404c37830aaa4df3a8a90a54f0504d68.bindPopup(popup_cd02d88ab980601f749fe82a6d6e3bcd)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d88aa0ba1c11ec6b4a7b590f613e9db = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.51351666838205, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_18deec15852f2f39840719f78507c5a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_02ddf83d4cad241bc06039277bf494f2 = $(`<div id="html_02ddf83d4cad241bc06039277bf494f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_18deec15852f2f39840719f78507c5a6.setContent(html_02ddf83d4cad241bc06039277bf494f2);\\n \\n \\n\\n circle_marker_4d88aa0ba1c11ec6b4a7b590f613e9db.bindPopup(popup_18deec15852f2f39840719f78507c5a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_7221ca1a9b63493af96375bdbd6e87d1 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_e9162add472c3ae78da4bc7ab876bb05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0485a1e2a6a235b878a3d38832c57490 = $(`<div id="html_0485a1e2a6a235b878a3d38832c57490" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9162add472c3ae78da4bc7ab876bb05.setContent(html_0485a1e2a6a235b878a3d38832c57490);\\n \\n \\n\\n circle_marker_7221ca1a9b63493af96375bdbd6e87d1.bindPopup(popup_e9162add472c3ae78da4bc7ab876bb05)\\n ;\\n\\n \\n \\n \\n var circle_marker_1acc2876126555b7451466748e3f3d36 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_ea2c205c8ad79d79328481a3ca5f7db2);\\n \\n \\n var popup_8c134a34e47b0a721f9839acb2c8aa78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63bcc14b7bba6b9970b2cd42dbe98ecd = $(`<div id="html_63bcc14b7bba6b9970b2cd42dbe98ecd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c134a34e47b0a721f9839acb2c8aa78.setContent(html_63bcc14b7bba6b9970b2cd42dbe98ecd);\\n \\n \\n\\n circle_marker_1acc2876126555b7451466748e3f3d36.bindPopup(popup_8c134a34e47b0a721f9839acb2c8aa78)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Hophornbeam": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_bd0c3ccf1963089eb34dfcc8ffbd234c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_bd0c3ccf1963089eb34dfcc8ffbd234c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_bd0c3ccf1963089eb34dfcc8ffbd234c = L.map(\\n "map_bd0c3ccf1963089eb34dfcc8ffbd234c",\\n {\\n center: [42.73545468664926, -73.24765207473526],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_c4f7d89e1f19a9756f9d503bc4653933 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var circle_marker_ed0270ae19b762dc43ff424d684b329f = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_02656e21e9207a4e3264ea030752b03b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36750f71f75eb30326de1a9406772043 = $(`<div id="html_36750f71f75eb30326de1a9406772043" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_02656e21e9207a4e3264ea030752b03b.setContent(html_36750f71f75eb30326de1a9406772043);\\n \\n \\n\\n circle_marker_ed0270ae19b762dc43ff424d684b329f.bindPopup(popup_02656e21e9207a4e3264ea030752b03b)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d25fb06e10966aa8d00d9969c858bb1 = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2742db5e5203d63db626d97da9920812 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40a2cc6e1d7ae903d360314b521db813 = $(`<div id="html_40a2cc6e1d7ae903d360314b521db813" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2742db5e5203d63db626d97da9920812.setContent(html_40a2cc6e1d7ae903d360314b521db813);\\n \\n \\n\\n circle_marker_8d25fb06e10966aa8d00d9969c858bb1.bindPopup(popup_2742db5e5203d63db626d97da9920812)\\n ;\\n\\n \\n \\n \\n var circle_marker_1af793348731478f79f5a702c43d44c0 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f5be94d31ff3d4605de9313c80fca021 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec3f5ed924f8840aae1aaf8fecda31b7 = $(`<div id="html_ec3f5ed924f8840aae1aaf8fecda31b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5be94d31ff3d4605de9313c80fca021.setContent(html_ec3f5ed924f8840aae1aaf8fecda31b7);\\n \\n \\n\\n circle_marker_1af793348731478f79f5a702c43d44c0.bindPopup(popup_f5be94d31ff3d4605de9313c80fca021)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3b8ea188cf6114ba3eaf5af27f22ab2 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_21577ca09dc433cbc556823089ba9ce2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d522b9b9e0eb2bc0207d5e28df909f77 = $(`<div id="html_d522b9b9e0eb2bc0207d5e28df909f77" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_21577ca09dc433cbc556823089ba9ce2.setContent(html_d522b9b9e0eb2bc0207d5e28df909f77);\\n \\n \\n\\n circle_marker_f3b8ea188cf6114ba3eaf5af27f22ab2.bindPopup(popup_21577ca09dc433cbc556823089ba9ce2)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f2cdfefc93fd529fc5cc5740210feae = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f642939d8a669fc7c298bd4a08124801 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8be5b9645e738cfab17840a7815405e7 = $(`<div id="html_8be5b9645e738cfab17840a7815405e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f642939d8a669fc7c298bd4a08124801.setContent(html_8be5b9645e738cfab17840a7815405e7);\\n \\n \\n\\n circle_marker_6f2cdfefc93fd529fc5cc5740210feae.bindPopup(popup_f642939d8a669fc7c298bd4a08124801)\\n ;\\n\\n \\n \\n \\n var circle_marker_f708afa9df8a28c8f7f7c382a01635da = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_4e864b7cdba1b51188d56d73405dfa53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d87a6e9c3f716cc0af4cd31c3e6c481 = $(`<div id="html_0d87a6e9c3f716cc0af4cd31c3e6c481" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e864b7cdba1b51188d56d73405dfa53.setContent(html_0d87a6e9c3f716cc0af4cd31c3e6c481);\\n \\n \\n\\n circle_marker_f708afa9df8a28c8f7f7c382a01635da.bindPopup(popup_4e864b7cdba1b51188d56d73405dfa53)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e58aee24a62e1e68f2561cceacc692b = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_ba9fd88d435dfc09a2b59fe391cd5c8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a342325449962dc4dfbb448de558ddb = $(`<div id="html_9a342325449962dc4dfbb448de558ddb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba9fd88d435dfc09a2b59fe391cd5c8f.setContent(html_9a342325449962dc4dfbb448de558ddb);\\n \\n \\n\\n circle_marker_4e58aee24a62e1e68f2561cceacc692b.bindPopup(popup_ba9fd88d435dfc09a2b59fe391cd5c8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_227a8cef6e5b38a9b72df3af2ec0fc88 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6607729ca70c9957c5370231fc51f8a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e2abf29195b0646a448e5180cba4490 = $(`<div id="html_0e2abf29195b0646a448e5180cba4490" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6607729ca70c9957c5370231fc51f8a3.setContent(html_0e2abf29195b0646a448e5180cba4490);\\n \\n \\n\\n circle_marker_227a8cef6e5b38a9b72df3af2ec0fc88.bindPopup(popup_6607729ca70c9957c5370231fc51f8a3)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf2269eb5b541c33e1331b2fb91dd16e = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_3326afcb1710c8e183c928162c701756 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eeb271bd34ef9d8842c5ae8246929a55 = $(`<div id="html_eeb271bd34ef9d8842c5ae8246929a55" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3326afcb1710c8e183c928162c701756.setContent(html_eeb271bd34ef9d8842c5ae8246929a55);\\n \\n \\n\\n circle_marker_bf2269eb5b541c33e1331b2fb91dd16e.bindPopup(popup_3326afcb1710c8e183c928162c701756)\\n ;\\n\\n \\n \\n \\n var circle_marker_624800aae0f67cc744eae423fd8498e2 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9869d4150ef550a5b4dc9ec491504604 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2c32c00c0cdaae8a7b1ebe2700a931a = $(`<div id="html_b2c32c00c0cdaae8a7b1ebe2700a931a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9869d4150ef550a5b4dc9ec491504604.setContent(html_b2c32c00c0cdaae8a7b1ebe2700a931a);\\n \\n \\n\\n circle_marker_624800aae0f67cc744eae423fd8498e2.bindPopup(popup_9869d4150ef550a5b4dc9ec491504604)\\n ;\\n\\n \\n \\n \\n var circle_marker_00a9d180122b2e6fbba3f7e8cebe1fdd = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b1c9cce91dd36d12ecc5d342c798248d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf23e62a2476f52ccd93b241d30dc794 = $(`<div id="html_cf23e62a2476f52ccd93b241d30dc794" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1c9cce91dd36d12ecc5d342c798248d.setContent(html_cf23e62a2476f52ccd93b241d30dc794);\\n \\n \\n\\n circle_marker_00a9d180122b2e6fbba3f7e8cebe1fdd.bindPopup(popup_b1c9cce91dd36d12ecc5d342c798248d)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6ad0bf4fbc2498beebf5b303f77b65b = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f65dbaf42332245c263bcefb67d32113 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57b760c47d72a634f8147bc2c8261388 = $(`<div id="html_57b760c47d72a634f8147bc2c8261388" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f65dbaf42332245c263bcefb67d32113.setContent(html_57b760c47d72a634f8147bc2c8261388);\\n \\n \\n\\n circle_marker_f6ad0bf4fbc2498beebf5b303f77b65b.bindPopup(popup_f65dbaf42332245c263bcefb67d32113)\\n ;\\n\\n \\n \\n \\n var circle_marker_33852aac9d1511d186fc7cb1f2f2a42d = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0a76cd2df7f3f3047299e0d6ea8e83d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df6bc7250598da1b131e28af3c8827a5 = $(`<div id="html_df6bc7250598da1b131e28af3c8827a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a76cd2df7f3f3047299e0d6ea8e83d3.setContent(html_df6bc7250598da1b131e28af3c8827a5);\\n \\n \\n\\n circle_marker_33852aac9d1511d186fc7cb1f2f2a42d.bindPopup(popup_0a76cd2df7f3f3047299e0d6ea8e83d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_67bb8a236845ed26e3cc805caa204e3d = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_15e6323b764fd090c354d9a7d867cfd6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd732a13ac6d654fb56d4ebbc123bfe2 = $(`<div id="html_dd732a13ac6d654fb56d4ebbc123bfe2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_15e6323b764fd090c354d9a7d867cfd6.setContent(html_dd732a13ac6d654fb56d4ebbc123bfe2);\\n \\n \\n\\n circle_marker_67bb8a236845ed26e3cc805caa204e3d.bindPopup(popup_15e6323b764fd090c354d9a7d867cfd6)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc948cb3a7650c23a4fde1172d277377 = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6d3dabe279bbc1ea4eeddcf43f4d9e4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25f0b8dbe0de5cf1d48e423a86d982c8 = $(`<div id="html_25f0b8dbe0de5cf1d48e423a86d982c8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d3dabe279bbc1ea4eeddcf43f4d9e4b.setContent(html_25f0b8dbe0de5cf1d48e423a86d982c8);\\n \\n \\n\\n circle_marker_dc948cb3a7650c23a4fde1172d277377.bindPopup(popup_6d3dabe279bbc1ea4eeddcf43f4d9e4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9026b98bee90c4782b6ae0cb67441c42 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f42b38765c12788f005db8ca4cc9602a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64dde6b3208079c9141be799479b0292 = $(`<div id="html_64dde6b3208079c9141be799479b0292" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f42b38765c12788f005db8ca4cc9602a.setContent(html_64dde6b3208079c9141be799479b0292);\\n \\n \\n\\n circle_marker_9026b98bee90c4782b6ae0cb67441c42.bindPopup(popup_f42b38765c12788f005db8ca4cc9602a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b4641e5802923f2665ee73d51d46527 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_3fe9ee72867b6adc5374b972758b9222 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b5ac7a2f460f10a52cba6ab78cba559a = $(`<div id="html_b5ac7a2f460f10a52cba6ab78cba559a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3fe9ee72867b6adc5374b972758b9222.setContent(html_b5ac7a2f460f10a52cba6ab78cba559a);\\n \\n \\n\\n circle_marker_5b4641e5802923f2665ee73d51d46527.bindPopup(popup_3fe9ee72867b6adc5374b972758b9222)\\n ;\\n\\n \\n \\n \\n var circle_marker_45408a791e07bd08b2d8ce6c6d017a48 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_4a5a54520be7ee673e2f8596c4a3e180 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32e00e01d72bfa06a50d535130ad4150 = $(`<div id="html_32e00e01d72bfa06a50d535130ad4150" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a5a54520be7ee673e2f8596c4a3e180.setContent(html_32e00e01d72bfa06a50d535130ad4150);\\n \\n \\n\\n circle_marker_45408a791e07bd08b2d8ce6c6d017a48.bindPopup(popup_4a5a54520be7ee673e2f8596c4a3e180)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8a4b7b62a81944c1ee74babd6baeb86 = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f402e26f8706397420ee6073428b3693 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_caff98089a66529e5835ad7a51b0d2df = $(`<div id="html_caff98089a66529e5835ad7a51b0d2df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f402e26f8706397420ee6073428b3693.setContent(html_caff98089a66529e5835ad7a51b0d2df);\\n \\n \\n\\n circle_marker_e8a4b7b62a81944c1ee74babd6baeb86.bindPopup(popup_f402e26f8706397420ee6073428b3693)\\n ;\\n\\n \\n \\n \\n var circle_marker_39f728481bec931ddba0927b700fc4d7 = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_acca6219f1c43c87a33852c0ee04da6d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6edac4ef0d81c5bdcc076ae6e0502c15 = $(`<div id="html_6edac4ef0d81c5bdcc076ae6e0502c15" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_acca6219f1c43c87a33852c0ee04da6d.setContent(html_6edac4ef0d81c5bdcc076ae6e0502c15);\\n \\n \\n\\n circle_marker_39f728481bec931ddba0927b700fc4d7.bindPopup(popup_acca6219f1c43c87a33852c0ee04da6d)\\n ;\\n\\n \\n \\n \\n var circle_marker_54179dd5d20f7f66b70f7739cb4be056 = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8efe3fdb64747c8b335a275ed9173406 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58c35dafb5e871d9b50791b95bb27b2a = $(`<div id="html_58c35dafb5e871d9b50791b95bb27b2a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8efe3fdb64747c8b335a275ed9173406.setContent(html_58c35dafb5e871d9b50791b95bb27b2a);\\n \\n \\n\\n circle_marker_54179dd5d20f7f66b70f7739cb4be056.bindPopup(popup_8efe3fdb64747c8b335a275ed9173406)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3302431d59a7ff40fda7f82eab5c15c = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f3b00790eb3de4024883ebda52fcecbc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ca19ad7e8ce55d30d7b29ef65f7516d = $(`<div id="html_4ca19ad7e8ce55d30d7b29ef65f7516d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f3b00790eb3de4024883ebda52fcecbc.setContent(html_4ca19ad7e8ce55d30d7b29ef65f7516d);\\n \\n \\n\\n circle_marker_f3302431d59a7ff40fda7f82eab5c15c.bindPopup(popup_f3b00790eb3de4024883ebda52fcecbc)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b10afc31e7ea74359a86de631ece453 = L.circleMarker(\\n [42.74358689209615, -73.26979323319746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_fa0bd5f8392ab2b053e865ac89557062 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1d6c05045b67d4abb7bf25b7ac06378 = $(`<div id="html_d1d6c05045b67d4abb7bf25b7ac06378" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa0bd5f8392ab2b053e865ac89557062.setContent(html_d1d6c05045b67d4abb7bf25b7ac06378);\\n \\n \\n\\n circle_marker_3b10afc31e7ea74359a86de631ece453.bindPopup(popup_fa0bd5f8392ab2b053e865ac89557062)\\n ;\\n\\n \\n \\n \\n var circle_marker_b54598440672d2332fb78dd32c004214 = L.circleMarker(\\n [42.743586938190056, -73.26856288386173],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a8042e09671f2addab611abc145c0574 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_771e031cc842a0dae6a59f8bb8ebe9b5 = $(`<div id="html_771e031cc842a0dae6a59f8bb8ebe9b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8042e09671f2addab611abc145c0574.setContent(html_771e031cc842a0dae6a59f8bb8ebe9b5);\\n \\n \\n\\n circle_marker_b54598440672d2332fb78dd32c004214.bindPopup(popup_a8042e09671f2addab611abc145c0574)\\n ;\\n\\n \\n \\n \\n var circle_marker_c403dd841c9e0e169306a8c63de0d0aa = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8eac4eb88b10dd6384c1f7d92bc144fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_93540bcb4376bd3a9d3c929e0412c885 = $(`<div id="html_93540bcb4376bd3a9d3c929e0412c885" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8eac4eb88b10dd6384c1f7d92bc144fc.setContent(html_93540bcb4376bd3a9d3c929e0412c885);\\n \\n \\n\\n circle_marker_c403dd841c9e0e169306a8c63de0d0aa.bindPopup(popup_8eac4eb88b10dd6384c1f7d92bc144fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3711a608eeb8acb5718f92927816f0a = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0adf9f286acdea3015fa5bed1b7b8b99 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60ef0110b263fd677a8405a8188ace85 = $(`<div id="html_60ef0110b263fd677a8405a8188ace85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0adf9f286acdea3015fa5bed1b7b8b99.setContent(html_60ef0110b263fd677a8405a8188ace85);\\n \\n \\n\\n circle_marker_c3711a608eeb8acb5718f92927816f0a.bindPopup(popup_0adf9f286acdea3015fa5bed1b7b8b99)\\n ;\\n\\n \\n \\n \\n var circle_marker_5abf12d29f95d907d19e31d4502c07df = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2d4de16c712f7f2020d77aa9f8183daa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc84b71e3672931972e7f4525276eaf4 = $(`<div id="html_dc84b71e3672931972e7f4525276eaf4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d4de16c712f7f2020d77aa9f8183daa.setContent(html_dc84b71e3672931972e7f4525276eaf4);\\n \\n \\n\\n circle_marker_5abf12d29f95d907d19e31d4502c07df.bindPopup(popup_2d4de16c712f7f2020d77aa9f8183daa)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce15915445651599b6bd0b43dd5e4601 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b4718ef23a8874d3f1e05319772b0be3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2cb28bc090263dc37e2bb9e9f8bec94 = $(`<div id="html_b2cb28bc090263dc37e2bb9e9f8bec94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b4718ef23a8874d3f1e05319772b0be3.setContent(html_b2cb28bc090263dc37e2bb9e9f8bec94);\\n \\n \\n\\n circle_marker_ce15915445651599b6bd0b43dd5e4601.bindPopup(popup_b4718ef23a8874d3f1e05319772b0be3)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd0e8e1b049e018615d4a6b4dc7cf0f6 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_bb093888ba656aa1e5aeafdc1872fbbd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_16ccd0a2973d9cc3e26d5a3937bfb0f4 = $(`<div id="html_16ccd0a2973d9cc3e26d5a3937bfb0f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bb093888ba656aa1e5aeafdc1872fbbd.setContent(html_16ccd0a2973d9cc3e26d5a3937bfb0f4);\\n \\n \\n\\n circle_marker_dd0e8e1b049e018615d4a6b4dc7cf0f6.bindPopup(popup_bb093888ba656aa1e5aeafdc1872fbbd)\\n ;\\n\\n \\n \\n \\n var circle_marker_eec8f39115c6bf9ae6f7c6a675ba83a4 = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_dfdcc3b53fbc1355ca2461d14be665d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_466bd5764526c682b45c6c9576daf3f4 = $(`<div id="html_466bd5764526c682b45c6c9576daf3f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dfdcc3b53fbc1355ca2461d14be665d5.setContent(html_466bd5764526c682b45c6c9576daf3f4);\\n \\n \\n\\n circle_marker_eec8f39115c6bf9ae6f7c6a675ba83a4.bindPopup(popup_dfdcc3b53fbc1355ca2461d14be665d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9c3d6fa0ec7616e553bec34fbdd0bfb = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f912287d304b79a11af047662ebd5b72 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bdeb82c2cdbd6f2872cc90b2b955f729 = $(`<div id="html_bdeb82c2cdbd6f2872cc90b2b955f729" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f912287d304b79a11af047662ebd5b72.setContent(html_bdeb82c2cdbd6f2872cc90b2b955f729);\\n \\n \\n\\n circle_marker_a9c3d6fa0ec7616e553bec34fbdd0bfb.bindPopup(popup_f912287d304b79a11af047662ebd5b72)\\n ;\\n\\n \\n \\n \\n var circle_marker_77502cb52360754c1ebea38e9604be20 = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d3baadbfdb9c245edf5641eb109c917b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4629d0182b8b26f84628b28ca10b3874 = $(`<div id="html_4629d0182b8b26f84628b28ca10b3874" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3baadbfdb9c245edf5641eb109c917b.setContent(html_4629d0182b8b26f84628b28ca10b3874);\\n \\n \\n\\n circle_marker_77502cb52360754c1ebea38e9604be20.bindPopup(popup_d3baadbfdb9c245edf5641eb109c917b)\\n ;\\n\\n \\n \\n \\n var circle_marker_207083b5e3b0380193a31c21cab947f9 = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_bd73b62cbd21aef02fff08b9565083eb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15d119e57602d5c6c8817309c8f036e2 = $(`<div id="html_15d119e57602d5c6c8817309c8f036e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd73b62cbd21aef02fff08b9565083eb.setContent(html_15d119e57602d5c6c8817309c8f036e2);\\n \\n \\n\\n circle_marker_207083b5e3b0380193a31c21cab947f9.bindPopup(popup_bd73b62cbd21aef02fff08b9565083eb)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1404086b3debc88f7cbac9e5d0aee1f = L.circleMarker(\\n [42.7417798640796, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f622cdc632065e4906c283dae9f17bc8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3eceb533528d618c25cacae4a3dc26b = $(`<div id="html_e3eceb533528d618c25cacae4a3dc26b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f622cdc632065e4906c283dae9f17bc8.setContent(html_e3eceb533528d618c25cacae4a3dc26b);\\n \\n \\n\\n circle_marker_c1404086b3debc88f7cbac9e5d0aee1f.bindPopup(popup_f622cdc632065e4906c283dae9f17bc8)\\n ;\\n\\n \\n \\n \\n var circle_marker_537a973208335b42b7aab95335f85d6f = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2f0a9ebd1556b9515001dece814aebc7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_47fe32fc5e32bce76f2da9d25f034bde = $(`<div id="html_47fe32fc5e32bce76f2da9d25f034bde" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f0a9ebd1556b9515001dece814aebc7.setContent(html_47fe32fc5e32bce76f2da9d25f034bde);\\n \\n \\n\\n circle_marker_537a973208335b42b7aab95335f85d6f.bindPopup(popup_2f0a9ebd1556b9515001dece814aebc7)\\n ;\\n\\n \\n \\n \\n var circle_marker_63769c5aac6662f0620b94a2aedde349 = L.circleMarker(\\n [42.7417798048184, -73.2611808954216],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_3e0d2a0ce058e5bc7e63e011be9c4698 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb53ea40c0fdfe8d75e33560ed57c654 = $(`<div id="html_cb53ea40c0fdfe8d75e33560ed57c654" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e0d2a0ce058e5bc7e63e011be9c4698.setContent(html_cb53ea40c0fdfe8d75e33560ed57c654);\\n \\n \\n\\n circle_marker_63769c5aac6662f0620b94a2aedde349.bindPopup(popup_3e0d2a0ce058e5bc7e63e011be9c4698)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea3e8ed38716cd6223713d961f7dc925 = L.circleMarker(\\n [42.74177969946516, -73.25872026847817],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8e862c48e26538e530b7e7388c14ea25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d523f82dd09cf9a092ec50ab043495a2 = $(`<div id="html_d523f82dd09cf9a092ec50ab043495a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e862c48e26538e530b7e7388c14ea25.setContent(html_d523f82dd09cf9a092ec50ab043495a2);\\n \\n \\n\\n circle_marker_ea3e8ed38716cd6223713d961f7dc925.bindPopup(popup_8e862c48e26538e530b7e7388c14ea25)\\n ;\\n\\n \\n \\n \\n var circle_marker_b8c67a098f0cb044f3e710a21333ede7 = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d7a50651c448216e253529d166565d2c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06e2374e3930e1892bad6b20aefdc956 = $(`<div id="html_06e2374e3930e1892bad6b20aefdc956" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d7a50651c448216e253529d166565d2c.setContent(html_06e2374e3930e1892bad6b20aefdc956);\\n \\n \\n\\n circle_marker_b8c67a098f0cb044f3e710a21333ede7.bindPopup(popup_d7a50651c448216e253529d166565d2c)\\n ;\\n\\n \\n \\n \\n var circle_marker_10cef060efaa4a222ca6e1ad34f60f4f = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_3eb5795a921fcfbac475c4fb58c7ecfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_73f33d030ccd2b6c9b5c0d92757dd771 = $(`<div id="html_73f33d030ccd2b6c9b5c0d92757dd771" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3eb5795a921fcfbac475c4fb58c7ecfe.setContent(html_73f33d030ccd2b6c9b5c0d92757dd771);\\n \\n \\n\\n circle_marker_10cef060efaa4a222ca6e1ad34f60f4f.bindPopup(popup_3eb5795a921fcfbac475c4fb58c7ecfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_60a9f7dd14622655c9f55202b71a046b = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_1f2ea214a26f112ea9a0dfb73223985b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc3497b03fb376c6dc01356532396328 = $(`<div id="html_bc3497b03fb376c6dc01356532396328" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f2ea214a26f112ea9a0dfb73223985b.setContent(html_bc3497b03fb376c6dc01356532396328);\\n \\n \\n\\n circle_marker_60a9f7dd14622655c9f55202b71a046b.bindPopup(popup_1f2ea214a26f112ea9a0dfb73223985b)\\n ;\\n\\n \\n \\n \\n var circle_marker_324b015596eade9fc783af099a1203e7 = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5afd50dc6f5f8c077f4c16a4e4064a8a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a57a5712be7e6aedf945115744944df0 = $(`<div id="html_a57a5712be7e6aedf945115744944df0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5afd50dc6f5f8c077f4c16a4e4064a8a.setContent(html_a57a5712be7e6aedf945115744944df0);\\n \\n \\n\\n circle_marker_324b015596eade9fc783af099a1203e7.bindPopup(popup_5afd50dc6f5f8c077f4c16a4e4064a8a)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d4a233c325ee7677acc0d335096b6cb = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2cdd8b976f1e316d9adebcebf809de26 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b44d5dd9e1d746b69b8b30994708c4a = $(`<div id="html_3b44d5dd9e1d746b69b8b30994708c4a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2cdd8b976f1e316d9adebcebf809de26.setContent(html_3b44d5dd9e1d746b69b8b30994708c4a);\\n \\n \\n\\n circle_marker_4d4a233c325ee7677acc0d335096b6cb.bindPopup(popup_2cdd8b976f1e316d9adebcebf809de26)\\n ;\\n\\n \\n \\n \\n var circle_marker_b705b8670898fa375e78f0163061aaee = L.circleMarker(\\n [42.73997267144673, -73.26856266869262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2ee60d9e45867c0328b66d4232b5648f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba5fde696a8ba97ffbdcb493b29e7ae3 = $(`<div id="html_ba5fde696a8ba97ffbdcb493b29e7ae3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ee60d9e45867c0328b66d4232b5648f.setContent(html_ba5fde696a8ba97ffbdcb493b29e7ae3);\\n \\n \\n\\n circle_marker_b705b8670898fa375e78f0163061aaee.bindPopup(popup_2ee60d9e45867c0328b66d4232b5648f)\\n ;\\n\\n \\n \\n \\n var circle_marker_76fc80996d185f66fd1f7d403e0c8b1f = L.circleMarker(\\n [42.73997270436829, -73.26733239107837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a9b3dffd04ddc2c6fefb0e339b82f688 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b1ec5e033a94df86df928fe2809470a = $(`<div id="html_8b1ec5e033a94df86df928fe2809470a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9b3dffd04ddc2c6fefb0e339b82f688.setContent(html_8b1ec5e033a94df86df928fe2809470a);\\n \\n \\n\\n circle_marker_76fc80996d185f66fd1f7d403e0c8b1f.bindPopup(popup_a9b3dffd04ddc2c6fefb0e339b82f688)\\n ;\\n\\n \\n \\n \\n var circle_marker_e94518aac99919aaedcc2c124d04905f = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d50945ebbad557fa3e44ec8c186f4a81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1e36446cf3680a51a2543172a70ebc9 = $(`<div id="html_b1e36446cf3680a51a2543172a70ebc9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d50945ebbad557fa3e44ec8c186f4a81.setContent(html_b1e36446cf3680a51a2543172a70ebc9);\\n \\n \\n\\n circle_marker_e94518aac99919aaedcc2c124d04905f.bindPopup(popup_d50945ebbad557fa3e44ec8c186f4a81)\\n ;\\n\\n \\n \\n \\n var circle_marker_2911f3aa3fc0c4531e0ca58be6582894 = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_918a095a848e2c5403186f4d4128fb4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86a7a864a598f94a56cdfcca1ecaaab5 = $(`<div id="html_86a7a864a598f94a56cdfcca1ecaaab5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_918a095a848e2c5403186f4d4128fb4b.setContent(html_86a7a864a598f94a56cdfcca1ecaaab5);\\n \\n \\n\\n circle_marker_2911f3aa3fc0c4531e0ca58be6582894.bindPopup(popup_918a095a848e2c5403186f4d4128fb4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_911cdd0f882b5cb5a28c4c8dcbfe06b6 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_1b3c89be59d0986f27552c6b12786ea7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d166d5f6be5a34f81fe8086167caf1da = $(`<div id="html_d166d5f6be5a34f81fe8086167caf1da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b3c89be59d0986f27552c6b12786ea7.setContent(html_d166d5f6be5a34f81fe8086167caf1da);\\n \\n \\n\\n circle_marker_911cdd0f882b5cb5a28c4c8dcbfe06b6.bindPopup(popup_1b3c89be59d0986f27552c6b12786ea7)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa756de777db1c0c80478a8e4fc7f5ab = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_aa95246992858faa620bfdc5073d933d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3eb3171077c2a71c1cc8874bc6ffe7ff = $(`<div id="html_3eb3171077c2a71c1cc8874bc6ffe7ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aa95246992858faa620bfdc5073d933d.setContent(html_3eb3171077c2a71c1cc8874bc6ffe7ff);\\n \\n \\n\\n circle_marker_aa756de777db1c0c80478a8e4fc7f5ab.bindPopup(popup_aa95246992858faa620bfdc5073d933d)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb1f7a33ee190481b886f164e1a829b9 = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e64d58197d5d0eea5d273eb3d240ea52 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cbf498431fee5bd5cec17452680fc966 = $(`<div id="html_cbf498431fee5bd5cec17452680fc966" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e64d58197d5d0eea5d273eb3d240ea52.setContent(html_cbf498431fee5bd5cec17452680fc966);\\n \\n \\n\\n circle_marker_cb1f7a33ee190481b886f164e1a829b9.bindPopup(popup_e64d58197d5d0eea5d273eb3d240ea52)\\n ;\\n\\n \\n \\n \\n var circle_marker_afcfb357d66008a86ff1e290a621cce0 = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f7838bb01d319195ba2c4c408dfd708f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d23666aa55857afe899d35358c81f545 = $(`<div id="html_d23666aa55857afe899d35358c81f545" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7838bb01d319195ba2c4c408dfd708f.setContent(html_d23666aa55857afe899d35358c81f545);\\n \\n \\n\\n circle_marker_afcfb357d66008a86ff1e290a621cce0.bindPopup(popup_f7838bb01d319195ba2c4c408dfd708f)\\n ;\\n\\n \\n \\n \\n var circle_marker_d7a2a433bae45bfe24314294249d540d = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_1d800f948dad7d26cb171b735f5bfecd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39ee06b0e56cf45b41d73a2b5449e4cb = $(`<div id="html_39ee06b0e56cf45b41d73a2b5449e4cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d800f948dad7d26cb171b735f5bfecd.setContent(html_39ee06b0e56cf45b41d73a2b5449e4cb);\\n \\n \\n\\n circle_marker_d7a2a433bae45bfe24314294249d540d.bindPopup(popup_1d800f948dad7d26cb171b735f5bfecd)\\n ;\\n\\n \\n \\n \\n var circle_marker_fab722bbb73f417c3c22084ef90a4696 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e2c306ca47e37147f119d4315765b4c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4c85b37366ba0f3a5498cc0396767566 = $(`<div id="html_4c85b37366ba0f3a5498cc0396767566" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2c306ca47e37147f119d4315765b4c8.setContent(html_4c85b37366ba0f3a5498cc0396767566);\\n \\n \\n\\n circle_marker_fab722bbb73f417c3c22084ef90a4696.bindPopup(popup_e2c306ca47e37147f119d4315765b4c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_49526126e8034b683d86146ee10cf63f = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0c905fdb25602990d70d90d52da8df37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bc93e2f614515764a20fe59a4b111aa = $(`<div id="html_1bc93e2f614515764a20fe59a4b111aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c905fdb25602990d70d90d52da8df37.setContent(html_1bc93e2f614515764a20fe59a4b111aa);\\n \\n \\n\\n circle_marker_49526126e8034b683d86146ee10cf63f.bindPopup(popup_0c905fdb25602990d70d90d52da8df37)\\n ;\\n\\n \\n \\n \\n var circle_marker_30ffcf5ff2f375ea58918be9c651a20e = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_57e07922913d13afe26919ce461c31a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f04aec34af85ade74d41486ce136bc4e = $(`<div id="html_f04aec34af85ade74d41486ce136bc4e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_57e07922913d13afe26919ce461c31a6.setContent(html_f04aec34af85ade74d41486ce136bc4e);\\n \\n \\n\\n circle_marker_30ffcf5ff2f375ea58918be9c651a20e.bindPopup(popup_57e07922913d13afe26919ce461c31a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_56cddacc9d8fab1a2a54ae83742e5bea = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.8678889139475245, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_16570f75427abf468e63c2b261d72543 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_050fee1642b9326a09d35be343a7c672 = $(`<div id="html_050fee1642b9326a09d35be343a7c672" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16570f75427abf468e63c2b261d72543.setContent(html_050fee1642b9326a09d35be343a7c672);\\n \\n \\n\\n circle_marker_56cddacc9d8fab1a2a54ae83742e5bea.bindPopup(popup_16570f75427abf468e63c2b261d72543)\\n ;\\n\\n \\n \\n \\n var circle_marker_4278a530ce22c6ee87802918176f0bb6 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a7eac930b9859fb507b35e724d1f5f10 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_220f3cca3fcd948b682b0fdc443a2fda = $(`<div id="html_220f3cca3fcd948b682b0fdc443a2fda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7eac930b9859fb507b35e724d1f5f10.setContent(html_220f3cca3fcd948b682b0fdc443a2fda);\\n \\n \\n\\n circle_marker_4278a530ce22c6ee87802918176f0bb6.bindPopup(popup_a7eac930b9859fb507b35e724d1f5f10)\\n ;\\n\\n \\n \\n \\n var circle_marker_27203230d8c252a3b6bbdb7a20709bea = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0b890d0a34472b3b25a997f1db971db2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76afa232e803a1d41b8cc9e4fdef2d0f = $(`<div id="html_76afa232e803a1d41b8cc9e4fdef2d0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b890d0a34472b3b25a997f1db971db2.setContent(html_76afa232e803a1d41b8cc9e4fdef2d0f);\\n \\n \\n\\n circle_marker_27203230d8c252a3b6bbdb7a20709bea.bindPopup(popup_0b890d0a34472b3b25a997f1db971db2)\\n ;\\n\\n \\n \\n \\n var circle_marker_06fde114ca38876466368319d2228c24 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f6ad9549e5d0cb93bf3fa015d22743a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21fee6d9f5fc1e60a430586325ba07c5 = $(`<div id="html_21fee6d9f5fc1e60a430586325ba07c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6ad9549e5d0cb93bf3fa015d22743a6.setContent(html_21fee6d9f5fc1e60a430586325ba07c5);\\n \\n \\n\\n circle_marker_06fde114ca38876466368319d2228c24.bindPopup(popup_f6ad9549e5d0cb93bf3fa015d22743a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_16ca5da2727a543a807bdc33ac2ed8e7 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0b971eac2577f5c1eb70a580577934df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_572e520d8d76d3f962ccedd7fd5f3dd4 = $(`<div id="html_572e520d8d76d3f962ccedd7fd5f3dd4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b971eac2577f5c1eb70a580577934df.setContent(html_572e520d8d76d3f962ccedd7fd5f3dd4);\\n \\n \\n\\n circle_marker_16ca5da2727a543a807bdc33ac2ed8e7.bindPopup(popup_0b971eac2577f5c1eb70a580577934df)\\n ;\\n\\n \\n \\n \\n var circle_marker_540defa537ef99bb520b0b5b6b5655af = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6f2158a38efc5034c94a92d43e5fd192 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e499dabe1eab6b268f2410e01fff886a = $(`<div id="html_e499dabe1eab6b268f2410e01fff886a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f2158a38efc5034c94a92d43e5fd192.setContent(html_e499dabe1eab6b268f2410e01fff886a);\\n \\n \\n\\n circle_marker_540defa537ef99bb520b0b5b6b5655af.bindPopup(popup_6f2158a38efc5034c94a92d43e5fd192)\\n ;\\n\\n \\n \\n \\n var circle_marker_01a61cdab273273c0398bd21e90ddcd9 = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9b51ef232c6dea4533d28389182ba8f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_79e944342bd659adaff82e457d1778e3 = $(`<div id="html_79e944342bd659adaff82e457d1778e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b51ef232c6dea4533d28389182ba8f3.setContent(html_79e944342bd659adaff82e457d1778e3);\\n \\n \\n\\n circle_marker_01a61cdab273273c0398bd21e90ddcd9.bindPopup(popup_9b51ef232c6dea4533d28389182ba8f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_afa6833eed2ac3c15f9298e19d144ebb = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5c156b4888e02456d1ff16b367de3220 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e9e7704e484dd579088af91cd38b30b = $(`<div id="html_9e9e7704e484dd579088af91cd38b30b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c156b4888e02456d1ff16b367de3220.setContent(html_9e9e7704e484dd579088af91cd38b30b);\\n \\n \\n\\n circle_marker_afa6833eed2ac3c15f9298e19d144ebb.bindPopup(popup_5c156b4888e02456d1ff16b367de3220)\\n ;\\n\\n \\n \\n \\n var circle_marker_a282a48c31e1819d50596a6fb2e1d828 = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_31d065c8bfc6d24ab7060ef936bea3f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0c6957c9a933dff0e6addbd73a64da2f = $(`<div id="html_0c6957c9a933dff0e6addbd73a64da2f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31d065c8bfc6d24ab7060ef936bea3f5.setContent(html_0c6957c9a933dff0e6addbd73a64da2f);\\n \\n \\n\\n circle_marker_a282a48c31e1819d50596a6fb2e1d828.bindPopup(popup_31d065c8bfc6d24ab7060ef936bea3f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_42dfef70b8afffcbfa3a7467235725f5 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9b95c7e906b9e374b068637855c83f75 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d606f0478411b7973754bf0b95dac1bb = $(`<div id="html_d606f0478411b7973754bf0b95dac1bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b95c7e906b9e374b068637855c83f75.setContent(html_d606f0478411b7973754bf0b95dac1bb);\\n \\n \\n\\n circle_marker_42dfef70b8afffcbfa3a7467235725f5.bindPopup(popup_9b95c7e906b9e374b068637855c83f75)\\n ;\\n\\n \\n \\n \\n var circle_marker_a317d2f2484d89aef928ff013793d555 = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8c91b7285baf6c0d0a7564b9642aee89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58e5633b21040ce0845f44afc68befe1 = $(`<div id="html_58e5633b21040ce0845f44afc68befe1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c91b7285baf6c0d0a7564b9642aee89.setContent(html_58e5633b21040ce0845f44afc68befe1);\\n \\n \\n\\n circle_marker_a317d2f2484d89aef928ff013793d555.bindPopup(popup_8c91b7285baf6c0d0a7564b9642aee89)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6996dd217c23a38c7d43f94ac36b5ee = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_cc37180f708617043cc98a218c64ad78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38a6c84e77b38c654324e08f88e8fff8 = $(`<div id="html_38a6c84e77b38c654324e08f88e8fff8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc37180f708617043cc98a218c64ad78.setContent(html_38a6c84e77b38c654324e08f88e8fff8);\\n \\n \\n\\n circle_marker_e6996dd217c23a38c7d43f94ac36b5ee.bindPopup(popup_cc37180f708617043cc98a218c64ad78)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c1e31d3ecbaeb8c0639522e64b6d987 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5facec1bb68ee6482684d64d32487902 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ef18a8b95a916c124c3f09c43094c2b5 = $(`<div id="html_ef18a8b95a916c124c3f09c43094c2b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5facec1bb68ee6482684d64d32487902.setContent(html_ef18a8b95a916c124c3f09c43094c2b5);\\n \\n \\n\\n circle_marker_2c1e31d3ecbaeb8c0639522e64b6d987.bindPopup(popup_5facec1bb68ee6482684d64d32487902)\\n ;\\n\\n \\n \\n \\n var circle_marker_b233671e658455dd821d3b20730d6c22 = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_066fd0ab68a806a13a2a95af64a48add = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac96f44617b8a578637dcb77f89ecac7 = $(`<div id="html_ac96f44617b8a578637dcb77f89ecac7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_066fd0ab68a806a13a2a95af64a48add.setContent(html_ac96f44617b8a578637dcb77f89ecac7);\\n \\n \\n\\n circle_marker_b233671e658455dd821d3b20730d6c22.bindPopup(popup_066fd0ab68a806a13a2a95af64a48add)\\n ;\\n\\n \\n \\n \\n var circle_marker_e73b45583f8fad19d329acadf5f905df = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1073621666150775, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b2481c77c61cb22b9b29b4851370b162 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f56704090c21552bcb8963481abdcafc = $(`<div id="html_f56704090c21552bcb8963481abdcafc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2481c77c61cb22b9b29b4851370b162.setContent(html_f56704090c21552bcb8963481abdcafc);\\n \\n \\n\\n circle_marker_e73b45583f8fad19d329acadf5f905df.bindPopup(popup_b2481c77c61cb22b9b29b4851370b162)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b86e3f19d744e7ebc34668f9fd8dcde = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_86f3d4fa38d4c827ae707e524447011a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c28bce11544fba4f9fa7dcd806d4beb4 = $(`<div id="html_c28bce11544fba4f9fa7dcd806d4beb4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86f3d4fa38d4c827ae707e524447011a.setContent(html_c28bce11544fba4f9fa7dcd806d4beb4);\\n \\n \\n\\n circle_marker_2b86e3f19d744e7ebc34668f9fd8dcde.bindPopup(popup_86f3d4fa38d4c827ae707e524447011a)\\n ;\\n\\n \\n \\n \\n var circle_marker_10bf6d5d6fcd99c51c2e6ec2fe198202 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_90baff18be4348aeb1aa4137b5e5840b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd089d6906d406b37b3804983c909887 = $(`<div id="html_fd089d6906d406b37b3804983c909887" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90baff18be4348aeb1aa4137b5e5840b.setContent(html_fd089d6906d406b37b3804983c909887);\\n \\n \\n\\n circle_marker_10bf6d5d6fcd99c51c2e6ec2fe198202.bindPopup(popup_90baff18be4348aeb1aa4137b5e5840b)\\n ;\\n\\n \\n \\n \\n var circle_marker_4567e4e52467386440a3c888018b6a85 = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_95a817f4a6d9c81405dabc8bf5e42c96 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d1632cdc5e9866cd35176cfab9070c5 = $(`<div id="html_4d1632cdc5e9866cd35176cfab9070c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95a817f4a6d9c81405dabc8bf5e42c96.setContent(html_4d1632cdc5e9866cd35176cfab9070c5);\\n \\n \\n\\n circle_marker_4567e4e52467386440a3c888018b6a85.bindPopup(popup_95a817f4a6d9c81405dabc8bf5e42c96)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c4375756498722e439502b2cd45e284 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_7d03b64e9a84339147f53780330a9eab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f79a86d013d03babf6dc0a5a1d0c8d81 = $(`<div id="html_f79a86d013d03babf6dc0a5a1d0c8d81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d03b64e9a84339147f53780330a9eab.setContent(html_f79a86d013d03babf6dc0a5a1d0c8d81);\\n \\n \\n\\n circle_marker_1c4375756498722e439502b2cd45e284.bindPopup(popup_7d03b64e9a84339147f53780330a9eab)\\n ;\\n\\n \\n \\n \\n var circle_marker_4ee1a5a5c19f825632ee49c7f45b1d85 = L.circleMarker(\\n [42.73635822694145, -73.25749060043893],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_eb4725a441cbcbed425f58b7ba9bdf88 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70affcaa8fdf14d6f4ca240585dcb7cf = $(`<div id="html_70affcaa8fdf14d6f4ca240585dcb7cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb4725a441cbcbed425f58b7ba9bdf88.setContent(html_70affcaa8fdf14d6f4ca240585dcb7cf);\\n \\n \\n\\n circle_marker_4ee1a5a5c19f825632ee49c7f45b1d85.bindPopup(popup_eb4725a441cbcbed425f58b7ba9bdf88)\\n ;\\n\\n \\n \\n \\n var circle_marker_c626a9d585c1262994116fb869239756 = L.circleMarker(\\n [42.73635814135235, -73.25626039454548],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_fb4264d3b41e0de36e1866eaf5c399e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_819a5775c6934ad08bccc66e20d1b6a7 = $(`<div id="html_819a5775c6934ad08bccc66e20d1b6a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb4264d3b41e0de36e1866eaf5c399e4.setContent(html_819a5775c6934ad08bccc66e20d1b6a7);\\n \\n \\n\\n circle_marker_c626a9d585c1262994116fb869239756.bindPopup(popup_fb4264d3b41e0de36e1866eaf5c399e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_3f15c2d2de5ff732bd9d5f3eeb85acd5 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0908f5ab2f4280905798f59294ade064 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_893f9751fded40be371de98ec0fe54f7 = $(`<div id="html_893f9751fded40be371de98ec0fe54f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0908f5ab2f4280905798f59294ade064.setContent(html_893f9751fded40be371de98ec0fe54f7);\\n \\n \\n\\n circle_marker_3f15c2d2de5ff732bd9d5f3eeb85acd5.bindPopup(popup_0908f5ab2f4280905798f59294ade064)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7272a00b1a477ec85e58968876f0502 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b9b750b429da809e9984856c52b887dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38f793cbc19e88312945ab63dc2fafac = $(`<div id="html_38f793cbc19e88312945ab63dc2fafac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b9b750b429da809e9984856c52b887dd.setContent(html_38f793cbc19e88312945ab63dc2fafac);\\n \\n \\n\\n circle_marker_a7272a00b1a477ec85e58968876f0502.bindPopup(popup_b9b750b429da809e9984856c52b887dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_452fdf78af1d22ea7687f99065a814dd = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_bdbfcda58ca742a603fd2d825f809acb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_313b7160a029da90f6cf6483a87f5aa8 = $(`<div id="html_313b7160a029da90f6cf6483a87f5aa8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdbfcda58ca742a603fd2d825f809acb.setContent(html_313b7160a029da90f6cf6483a87f5aa8);\\n \\n \\n\\n circle_marker_452fdf78af1d22ea7687f99065a814dd.bindPopup(popup_bdbfcda58ca742a603fd2d825f809acb)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7730783855e45deeaca31905526fdef = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_1416b56092313e4653c3736992d7f7df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_beb4c264034179a86d2750edd226e081 = $(`<div id="html_beb4c264034179a86d2750edd226e081" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1416b56092313e4653c3736992d7f7df.setContent(html_beb4c264034179a86d2750edd226e081);\\n \\n \\n\\n circle_marker_a7730783855e45deeaca31905526fdef.bindPopup(popup_1416b56092313e4653c3736992d7f7df)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d9ea3f5a05e7a2f7ee15b520ba5b01a = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9d3a8d481a539e1057671879c5984e63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b804668ee63113c9ad387184e7c0a201 = $(`<div id="html_b804668ee63113c9ad387184e7c0a201" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d3a8d481a539e1057671879c5984e63.setContent(html_b804668ee63113c9ad387184e7c0a201);\\n \\n \\n\\n circle_marker_0d9ea3f5a05e7a2f7ee15b520ba5b01a.bindPopup(popup_9d3a8d481a539e1057671879c5984e63)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d1c7637d10df8a189a5e5279cc81745 = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e665ea428594f8f49fe7f5c75449672f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df5c29af7800de8a4846511ad9a0bd60 = $(`<div id="html_df5c29af7800de8a4846511ad9a0bd60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e665ea428594f8f49fe7f5c75449672f.setContent(html_df5c29af7800de8a4846511ad9a0bd60);\\n \\n \\n\\n circle_marker_0d1c7637d10df8a189a5e5279cc81745.bindPopup(popup_e665ea428594f8f49fe7f5c75449672f)\\n ;\\n\\n \\n \\n \\n var circle_marker_509e71b625bff53e399b153ad3e9dc0a = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d3b1b78eb8aad5bfa700fa40e0f497a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5481847745e974f6bc2fe7bc3b806848 = $(`<div id="html_5481847745e974f6bc2fe7bc3b806848" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3b1b78eb8aad5bfa700fa40e0f497a0.setContent(html_5481847745e974f6bc2fe7bc3b806848);\\n \\n \\n\\n circle_marker_509e71b625bff53e399b153ad3e9dc0a.bindPopup(popup_d3b1b78eb8aad5bfa700fa40e0f497a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_f13156b3ba28b130112fc592017f4636 = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_39de0241c19efae00be41c2cb9cf734e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b54026513667333e6c2f9cf017e4b488 = $(`<div id="html_b54026513667333e6c2f9cf017e4b488" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39de0241c19efae00be41c2cb9cf734e.setContent(html_b54026513667333e6c2f9cf017e4b488);\\n \\n \\n\\n circle_marker_f13156b3ba28b130112fc592017f4636.bindPopup(popup_39de0241c19efae00be41c2cb9cf734e)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0d1f5d1ffa817f51614d07a80d0f3a2 = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_34234862526c9ac9d82158188dbcc428 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44ffda183615d9c81dc194c2693ead2c = $(`<div id="html_44ffda183615d9c81dc194c2693ead2c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_34234862526c9ac9d82158188dbcc428.setContent(html_44ffda183615d9c81dc194c2693ead2c);\\n \\n \\n\\n circle_marker_c0d1f5d1ffa817f51614d07a80d0f3a2.bindPopup(popup_34234862526c9ac9d82158188dbcc428)\\n ;\\n\\n \\n \\n \\n var circle_marker_68a8bdd8807c8aa3b4ff75e694672cfd = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d30078faec64798ee025cfa9ff2af723 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_801d958da11adb06343980fdd7e7e28f = $(`<div id="html_801d958da11adb06343980fdd7e7e28f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d30078faec64798ee025cfa9ff2af723.setContent(html_801d958da11adb06343980fdd7e7e28f);\\n \\n \\n\\n circle_marker_68a8bdd8807c8aa3b4ff75e694672cfd.bindPopup(popup_d30078faec64798ee025cfa9ff2af723)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e6ca782433dd9efb1b0c0ec7ea58a96 = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_cd07da2cd5e69feb4a1556669cc939fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe790a9bf6a1318f53b3921cdd4c3f19 = $(`<div id="html_fe790a9bf6a1318f53b3921cdd4c3f19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd07da2cd5e69feb4a1556669cc939fa.setContent(html_fe790a9bf6a1318f53b3921cdd4c3f19);\\n \\n \\n\\n circle_marker_7e6ca782433dd9efb1b0c0ec7ea58a96.bindPopup(popup_cd07da2cd5e69feb4a1556669cc939fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_27c75f5027521c7710dda7269ac4178e = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f2e7f480f61f4945422aaf2588370070 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fbb1839018a4b7873adc189215e563a8 = $(`<div id="html_fbb1839018a4b7873adc189215e563a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2e7f480f61f4945422aaf2588370070.setContent(html_fbb1839018a4b7873adc189215e563a8);\\n \\n \\n\\n circle_marker_27c75f5027521c7710dda7269ac4178e.bindPopup(popup_f2e7f480f61f4945422aaf2588370070)\\n ;\\n\\n \\n \\n \\n var circle_marker_90d95fbba8d3ec84459794a20164da45 = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_c778d640df6c3d64ce7f04dd3158eebb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ced5f6a5425b39f20d07d36d28fa862 = $(`<div id="html_8ced5f6a5425b39f20d07d36d28fa862" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c778d640df6c3d64ce7f04dd3158eebb.setContent(html_8ced5f6a5425b39f20d07d36d28fa862);\\n \\n \\n\\n circle_marker_90d95fbba8d3ec84459794a20164da45.bindPopup(popup_c778d640df6c3d64ce7f04dd3158eebb)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5cd28c3133f32614c263e6073ebb0c3 = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f646fcfb7a515050320cd58b9ef69802 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ffb92224f87065a0e34689de9187cda = $(`<div id="html_5ffb92224f87065a0e34689de9187cda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f646fcfb7a515050320cd58b9ef69802.setContent(html_5ffb92224f87065a0e34689de9187cda);\\n \\n \\n\\n circle_marker_b5cd28c3133f32614c263e6073ebb0c3.bindPopup(popup_f646fcfb7a515050320cd58b9ef69802)\\n ;\\n\\n \\n \\n \\n var circle_marker_345b2523e98c5d488944dac2ac23f3a0 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_79763b2b2b7cb158ab4eed8114ad2578 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d432ef6da6697ed17473dbe92f58ee8b = $(`<div id="html_d432ef6da6697ed17473dbe92f58ee8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79763b2b2b7cb158ab4eed8114ad2578.setContent(html_d432ef6da6697ed17473dbe92f58ee8b);\\n \\n \\n\\n circle_marker_345b2523e98c5d488944dac2ac23f3a0.bindPopup(popup_79763b2b2b7cb158ab4eed8114ad2578)\\n ;\\n\\n \\n \\n \\n var circle_marker_7815f77ae8afd92adc6d9ab09a9528cc = L.circleMarker(\\n [42.7345512252472, -73.26979251605432],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f6a1b5c2cc54dc6da40b6618c2dfb504 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3b4b01dc721f006542d4f6b970e6e38 = $(`<div id="html_e3b4b01dc721f006542d4f6b970e6e38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6a1b5c2cc54dc6da40b6618c2dfb504.setContent(html_e3b4b01dc721f006542d4f6b970e6e38);\\n \\n \\n\\n circle_marker_7815f77ae8afd92adc6d9ab09a9528cc.bindPopup(popup_f6a1b5c2cc54dc6da40b6618c2dfb504)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3a00b99f9bf6568aece6fc8a1b19ac6 = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_ef7bb305ba8baf3cd06c2de810201afe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e01516009b813a7827577fd67507efb4 = $(`<div id="html_e01516009b813a7827577fd67507efb4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef7bb305ba8baf3cd06c2de810201afe.setContent(html_e01516009b813a7827577fd67507efb4);\\n \\n \\n\\n circle_marker_a3a00b99f9bf6568aece6fc8a1b19ac6.bindPopup(popup_ef7bb305ba8baf3cd06c2de810201afe)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa1d3222217f69923b8423b382098efa = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_bdb5bc1d0178f5d240d2079ab01331a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_34b6a89c295704bd7e68d6c37051f82b = $(`<div id="html_34b6a89c295704bd7e68d6c37051f82b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdb5bc1d0178f5d240d2079ab01331a5.setContent(html_34b6a89c295704bd7e68d6c37051f82b);\\n \\n \\n\\n circle_marker_fa1d3222217f69923b8423b382098efa.bindPopup(popup_bdb5bc1d0178f5d240d2079ab01331a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_005249a3c607d9693cdb45326895429a = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d6f89443d52df36b7ccf5b19f2ec577f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2496f7554511f0d08fd0de0533ae0930 = $(`<div id="html_2496f7554511f0d08fd0de0533ae0930" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d6f89443d52df36b7ccf5b19f2ec577f.setContent(html_2496f7554511f0d08fd0de0533ae0930);\\n \\n \\n\\n circle_marker_005249a3c607d9693cdb45326895429a.bindPopup(popup_d6f89443d52df36b7ccf5b19f2ec577f)\\n ;\\n\\n \\n \\n \\n var circle_marker_754d573bdf8719f23d1e50139ddbc0a7 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9f4a808ff087d9472ccb31bd7a1ee61a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c3a60e4e14ab3df2b5774cbdea35c0a = $(`<div id="html_7c3a60e4e14ab3df2b5774cbdea35c0a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f4a808ff087d9472ccb31bd7a1ee61a.setContent(html_7c3a60e4e14ab3df2b5774cbdea35c0a);\\n \\n \\n\\n circle_marker_754d573bdf8719f23d1e50139ddbc0a7.bindPopup(popup_9f4a808ff087d9472ccb31bd7a1ee61a)\\n ;\\n\\n \\n \\n \\n var circle_marker_03b32b12ab33e9792b599935e9ef7c02 = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_899745778c7cc60fde0855f8466050a7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e0d738fb2eeb53cd4389c3fc8203c09b = $(`<div id="html_e0d738fb2eeb53cd4389c3fc8203c09b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_899745778c7cc60fde0855f8466050a7.setContent(html_e0d738fb2eeb53cd4389c3fc8203c09b);\\n \\n \\n\\n circle_marker_03b32b12ab33e9792b599935e9ef7c02.bindPopup(popup_899745778c7cc60fde0855f8466050a7)\\n ;\\n\\n \\n \\n \\n var circle_marker_995d5d2970e4be7f7950aa10bc0bad20 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6426f6b1f62bd33d31a132b48861acc8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d55de0ef258491ecd9ba96ab86e168dc = $(`<div id="html_d55de0ef258491ecd9ba96ab86e168dc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6426f6b1f62bd33d31a132b48861acc8.setContent(html_d55de0ef258491ecd9ba96ab86e168dc);\\n \\n \\n\\n circle_marker_995d5d2970e4be7f7950aa10bc0bad20.bindPopup(popup_6426f6b1f62bd33d31a132b48861acc8)\\n ;\\n\\n \\n \\n \\n var circle_marker_1bcaea1ed49cf98a320f2f136d694339 = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0d5328c300e2d34886eb71660aa0d157 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f01630afda954579226cc336212c64ff = $(`<div id="html_f01630afda954579226cc336212c64ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d5328c300e2d34886eb71660aa0d157.setContent(html_f01630afda954579226cc336212c64ff);\\n \\n \\n\\n circle_marker_1bcaea1ed49cf98a320f2f136d694339.bindPopup(popup_0d5328c300e2d34886eb71660aa0d157)\\n ;\\n\\n \\n \\n \\n var circle_marker_7f45efd94e18a33ef43d053530e295b1 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_056963eb8bffeae66211bb43622a9203 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_85af9d2524743e4d8e75b3a539bfcfed = $(`<div id="html_85af9d2524743e4d8e75b3a539bfcfed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_056963eb8bffeae66211bb43622a9203.setContent(html_85af9d2524743e4d8e75b3a539bfcfed);\\n \\n \\n\\n circle_marker_7f45efd94e18a33ef43d053530e295b1.bindPopup(popup_056963eb8bffeae66211bb43622a9203)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d1454b0a72a752c8f4df279baadba56 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f92c9f84bd8dad697f96b098ae0939e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3a922a1d7b327e592b549e832a25883 = $(`<div id="html_e3a922a1d7b327e592b549e832a25883" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f92c9f84bd8dad697f96b098ae0939e7.setContent(html_e3a922a1d7b327e592b549e832a25883);\\n \\n \\n\\n circle_marker_6d1454b0a72a752c8f4df279baadba56.bindPopup(popup_f92c9f84bd8dad697f96b098ae0939e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_647697764c576abdd8442641a5bb99f2 = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_645ceb0d22f9e65b860c4e950bda0ab6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d32b19e6478806d6b6f2df22aa140f94 = $(`<div id="html_d32b19e6478806d6b6f2df22aa140f94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_645ceb0d22f9e65b860c4e950bda0ab6.setContent(html_d32b19e6478806d6b6f2df22aa140f94);\\n \\n \\n\\n circle_marker_647697764c576abdd8442641a5bb99f2.bindPopup(popup_645ceb0d22f9e65b860c4e950bda0ab6)\\n ;\\n\\n \\n \\n \\n var circle_marker_f16c26bb52e165b8a43af8272da9e7b2 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_cac742dfab7dfd3b7b3eefc7ed2128b2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92022d477b714f1f732c7d4d576d2362 = $(`<div id="html_92022d477b714f1f732c7d4d576d2362" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cac742dfab7dfd3b7b3eefc7ed2128b2.setContent(html_92022d477b714f1f732c7d4d576d2362);\\n \\n \\n\\n circle_marker_f16c26bb52e165b8a43af8272da9e7b2.bindPopup(popup_cac742dfab7dfd3b7b3eefc7ed2128b2)\\n ;\\n\\n \\n \\n \\n var circle_marker_89a9de9668129986c786a7c330b20dfe = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e047c1fac679734870c925629c2bec0f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_47d495f51066efe70aacb27fa308d62a = $(`<div id="html_47d495f51066efe70aacb27fa308d62a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e047c1fac679734870c925629c2bec0f.setContent(html_47d495f51066efe70aacb27fa308d62a);\\n \\n \\n\\n circle_marker_89a9de9668129986c786a7c330b20dfe.bindPopup(popup_e047c1fac679734870c925629c2bec0f)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ec56eaa5dd66f2d38dd15c4c3506d49 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_35c8597a34ec6fa707e8baa0e5c124d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dbb525af476d1eb648299435c91b14be = $(`<div id="html_dbb525af476d1eb648299435c91b14be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35c8597a34ec6fa707e8baa0e5c124d9.setContent(html_dbb525af476d1eb648299435c91b14be);\\n \\n \\n\\n circle_marker_2ec56eaa5dd66f2d38dd15c4c3506d49.bindPopup(popup_35c8597a34ec6fa707e8baa0e5c124d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_522e9a83b0e95c967910ad3808c51bcd = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8b69692b3ad21eb9c76994b0f93a2855 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09ff24395c3174238a75da2e41991da7 = $(`<div id="html_09ff24395c3174238a75da2e41991da7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b69692b3ad21eb9c76994b0f93a2855.setContent(html_09ff24395c3174238a75da2e41991da7);\\n \\n \\n\\n circle_marker_522e9a83b0e95c967910ad3808c51bcd.bindPopup(popup_8b69692b3ad21eb9c76994b0f93a2855)\\n ;\\n\\n \\n \\n \\n var circle_marker_52f03ef0bd77f531b4c16d552cc9791a = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e5257d1f9ed1fa4626a41ed23b117145 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bec93293f6a2dd895b7748ee78cea2c9 = $(`<div id="html_bec93293f6a2dd895b7748ee78cea2c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5257d1f9ed1fa4626a41ed23b117145.setContent(html_bec93293f6a2dd895b7748ee78cea2c9);\\n \\n \\n\\n circle_marker_52f03ef0bd77f531b4c16d552cc9791a.bindPopup(popup_e5257d1f9ed1fa4626a41ed23b117145)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ce53de8705f1dd7c79fdcd95a3946e7 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_db97c71bfa7d5919e4c320d7a2113597 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9d95b0929dc3054fcbdc0c2fe06e807 = $(`<div id="html_d9d95b0929dc3054fcbdc0c2fe06e807" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db97c71bfa7d5919e4c320d7a2113597.setContent(html_d9d95b0929dc3054fcbdc0c2fe06e807);\\n \\n \\n\\n circle_marker_9ce53de8705f1dd7c79fdcd95a3946e7.bindPopup(popup_db97c71bfa7d5919e4c320d7a2113597)\\n ;\\n\\n \\n \\n \\n var circle_marker_c470a6e64618b36dcc451e7b9abb805b = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_fb2c798dcfea3f068fd2c02e28384ff1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7b910bd5bae44f2366e0a9f30bfa670 = $(`<div id="html_e7b910bd5bae44f2366e0a9f30bfa670" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb2c798dcfea3f068fd2c02e28384ff1.setContent(html_e7b910bd5bae44f2366e0a9f30bfa670);\\n \\n \\n\\n circle_marker_c470a6e64618b36dcc451e7b9abb805b.bindPopup(popup_fb2c798dcfea3f068fd2c02e28384ff1)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e825615b08e6f849cc1a46d3781462b = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_de7b4c57e971d1c7b7e9f0908aad4ee6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c78ba3ff3080703b3bd8e558a89273c = $(`<div id="html_1c78ba3ff3080703b3bd8e558a89273c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de7b4c57e971d1c7b7e9f0908aad4ee6.setContent(html_1c78ba3ff3080703b3bd8e558a89273c);\\n \\n \\n\\n circle_marker_3e825615b08e6f849cc1a46d3781462b.bindPopup(popup_de7b4c57e971d1c7b7e9f0908aad4ee6)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e9032aed4fc609a069a6418c7b76fee = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8468f87d0395fda1b8b3b153d37b5e1d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d39b0eabe6909178dff179be4fb8cdb = $(`<div id="html_8d39b0eabe6909178dff179be4fb8cdb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8468f87d0395fda1b8b3b153d37b5e1d.setContent(html_8d39b0eabe6909178dff179be4fb8cdb);\\n \\n \\n\\n circle_marker_7e9032aed4fc609a069a6418c7b76fee.bindPopup(popup_8468f87d0395fda1b8b3b153d37b5e1d)\\n ;\\n\\n \\n \\n \\n var circle_marker_2bbd1014124118aa1344c8f1714d14d6 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9a943a3b0cf1038c25316534f11882ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43b1e99275c7f8f5dda57a61ff145fe2 = $(`<div id="html_43b1e99275c7f8f5dda57a61ff145fe2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a943a3b0cf1038c25316534f11882ec.setContent(html_43b1e99275c7f8f5dda57a61ff145fe2);\\n \\n \\n\\n circle_marker_2bbd1014124118aa1344c8f1714d14d6.bindPopup(popup_9a943a3b0cf1038c25316534f11882ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_47234dd288cf6aaa84765fef59add6ed = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_1d49d2fa14e7560bfafbd7598e25374d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8b93da91571de7d2736856211b28881 = $(`<div id="html_f8b93da91571de7d2736856211b28881" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d49d2fa14e7560bfafbd7598e25374d.setContent(html_f8b93da91571de7d2736856211b28881);\\n \\n \\n\\n circle_marker_47234dd288cf6aaa84765fef59add6ed.bindPopup(popup_1d49d2fa14e7560bfafbd7598e25374d)\\n ;\\n\\n \\n \\n \\n var circle_marker_a45c7b36ddcbaaae292708fad25c8f21 = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_dac44f72cf924c0b7bb7282d83c8ab7a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01c2143a0582da3455915db9608a211e = $(`<div id="html_01c2143a0582da3455915db9608a211e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dac44f72cf924c0b7bb7282d83c8ab7a.setContent(html_01c2143a0582da3455915db9608a211e);\\n \\n \\n\\n circle_marker_a45c7b36ddcbaaae292708fad25c8f21.bindPopup(popup_dac44f72cf924c0b7bb7282d83c8ab7a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c0e9b6aa0f38e46eac4a5a9879f3e86 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6ea155fd44ed7bf2e28f26788649dd35 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2421b6e148c10673af813f9f414f957e = $(`<div id="html_2421b6e148c10673af813f9f414f957e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ea155fd44ed7bf2e28f26788649dd35.setContent(html_2421b6e148c10673af813f9f414f957e);\\n \\n \\n\\n circle_marker_5c0e9b6aa0f38e46eac4a5a9879f3e86.bindPopup(popup_6ea155fd44ed7bf2e28f26788649dd35)\\n ;\\n\\n \\n \\n \\n var circle_marker_33007af597b16fb1d914494a6df3db0b = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_bd1ce3fcb9974c2d63eef1949373b2a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_023a3525c6f8e58ab43c278dd913cb50 = $(`<div id="html_023a3525c6f8e58ab43c278dd913cb50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd1ce3fcb9974c2d63eef1949373b2a5.setContent(html_023a3525c6f8e58ab43c278dd913cb50);\\n \\n \\n\\n circle_marker_33007af597b16fb1d914494a6df3db0b.bindPopup(popup_bd1ce3fcb9974c2d63eef1949373b2a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c7478cd9f6d3b06242fe47cbe26cec4 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_005971682174b2e043192df0210458c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f23af1954f8546fd03a36ec2a8316d86 = $(`<div id="html_f23af1954f8546fd03a36ec2a8316d86" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_005971682174b2e043192df0210458c8.setContent(html_f23af1954f8546fd03a36ec2a8316d86);\\n \\n \\n\\n circle_marker_8c7478cd9f6d3b06242fe47cbe26cec4.bindPopup(popup_005971682174b2e043192df0210458c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_b43beb806b5a55babdc41f909a4f8420 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6930d0b5c7568f95ba21a1b912e9a72e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1defaaa8af6bde28ba9cf1d275f06c7 = $(`<div id="html_b1defaaa8af6bde28ba9cf1d275f06c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6930d0b5c7568f95ba21a1b912e9a72e.setContent(html_b1defaaa8af6bde28ba9cf1d275f06c7);\\n \\n \\n\\n circle_marker_b43beb806b5a55babdc41f909a4f8420.bindPopup(popup_6930d0b5c7568f95ba21a1b912e9a72e)\\n ;\\n\\n \\n \\n \\n var circle_marker_87ae24a6eaa16d6d81ef5968571e383f = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d97ebeaf71692d2ed57bf85a64ea3ea6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab99ea0e4e14c4213b05eee569c24c7f = $(`<div id="html_ab99ea0e4e14c4213b05eee569c24c7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d97ebeaf71692d2ed57bf85a64ea3ea6.setContent(html_ab99ea0e4e14c4213b05eee569c24c7f);\\n \\n \\n\\n circle_marker_87ae24a6eaa16d6d81ef5968571e383f.bindPopup(popup_d97ebeaf71692d2ed57bf85a64ea3ea6)\\n ;\\n\\n \\n \\n \\n var circle_marker_74558f473d70fe0df272d2252b4dd8c9 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_89c773e7ebb295bbbf05c27a03a428ad = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d29e398e0f5774e60f718969b266b4eb = $(`<div id="html_d29e398e0f5774e60f718969b266b4eb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89c773e7ebb295bbbf05c27a03a428ad.setContent(html_d29e398e0f5774e60f718969b266b4eb);\\n \\n \\n\\n circle_marker_74558f473d70fe0df272d2252b4dd8c9.bindPopup(popup_89c773e7ebb295bbbf05c27a03a428ad)\\n ;\\n\\n \\n \\n \\n var circle_marker_c5cccd022671d73a644b638564bd38d6 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_04b4d48c47d215242ac4883cbc5e93f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da6b7f5b1ce90f2f2d77041eae454599 = $(`<div id="html_da6b7f5b1ce90f2f2d77041eae454599" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04b4d48c47d215242ac4883cbc5e93f8.setContent(html_da6b7f5b1ce90f2f2d77041eae454599);\\n \\n \\n\\n circle_marker_c5cccd022671d73a644b638564bd38d6.bindPopup(popup_04b4d48c47d215242ac4883cbc5e93f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a8caae6ff9b06ae51d0d4b69891b553 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8436598807ac6f4951845474b5ca2a3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8874b209c139cafbd87d4551796115d1 = $(`<div id="html_8874b209c139cafbd87d4551796115d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8436598807ac6f4951845474b5ca2a3e.setContent(html_8874b209c139cafbd87d4551796115d1);\\n \\n \\n\\n circle_marker_8a8caae6ff9b06ae51d0d4b69891b553.bindPopup(popup_8436598807ac6f4951845474b5ca2a3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b3793323930b18fa94d8c511798ec26d = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_7e2c67489c53055f23d0e0e17b9cb4c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_baedfabd4cb98876271c9c0b63a641e7 = $(`<div id="html_baedfabd4cb98876271c9c0b63a641e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e2c67489c53055f23d0e0e17b9cb4c6.setContent(html_baedfabd4cb98876271c9c0b63a641e7);\\n \\n \\n\\n circle_marker_b3793323930b18fa94d8c511798ec26d.bindPopup(popup_7e2c67489c53055f23d0e0e17b9cb4c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_b799f642120ce589cd3a34909562abd7 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d2a1c63694f3e780b015e69653ad8173 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a5d33e2f2190c8c9a62f5e51dc786ca = $(`<div id="html_0a5d33e2f2190c8c9a62f5e51dc786ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2a1c63694f3e780b015e69653ad8173.setContent(html_0a5d33e2f2190c8c9a62f5e51dc786ca);\\n \\n \\n\\n circle_marker_b799f642120ce589cd3a34909562abd7.bindPopup(popup_d2a1c63694f3e780b015e69653ad8173)\\n ;\\n\\n \\n \\n \\n var circle_marker_5bce370171a793730b43065602a75246 = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e4bf7dc189fa1f25a85a1ef68d2ff341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c86bd2833b42f3449183928a7a792bc7 = $(`<div id="html_c86bd2833b42f3449183928a7a792bc7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4bf7dc189fa1f25a85a1ef68d2ff341.setContent(html_c86bd2833b42f3449183928a7a792bc7);\\n \\n \\n\\n circle_marker_5bce370171a793730b43065602a75246.bindPopup(popup_e4bf7dc189fa1f25a85a1ef68d2ff341)\\n ;\\n\\n \\n \\n \\n var circle_marker_79a3281f22db01da4e65df27421b11f4 = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a18a911d15d9d6458f93b14a52a55420 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48c28db46281f41d2352b5cf7a36c9e9 = $(`<div id="html_48c28db46281f41d2352b5cf7a36c9e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a18a911d15d9d6458f93b14a52a55420.setContent(html_48c28db46281f41d2352b5cf7a36c9e9);\\n \\n \\n\\n circle_marker_79a3281f22db01da4e65df27421b11f4.bindPopup(popup_a18a911d15d9d6458f93b14a52a55420)\\n ;\\n\\n \\n \\n \\n var circle_marker_cbcf57e57eccb5bac99591caa29235f9 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_697853903ebcfde77007d96d2910b09b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6831092c0afcc0790794311b9198fc13 = $(`<div id="html_6831092c0afcc0790794311b9198fc13" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_697853903ebcfde77007d96d2910b09b.setContent(html_6831092c0afcc0790794311b9198fc13);\\n \\n \\n\\n circle_marker_cbcf57e57eccb5bac99591caa29235f9.bindPopup(popup_697853903ebcfde77007d96d2910b09b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ef43a9fe4316d716d1957d77e5206eb = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_23d3007992e5bc7a0933d98e5f8d9963 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fca9a2097e0230ae5637822b199f554 = $(`<div id="html_8fca9a2097e0230ae5637822b199f554" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23d3007992e5bc7a0933d98e5f8d9963.setContent(html_8fca9a2097e0230ae5637822b199f554);\\n \\n \\n\\n circle_marker_3ef43a9fe4316d716d1957d77e5206eb.bindPopup(popup_23d3007992e5bc7a0933d98e5f8d9963)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e3f953fb9be24a32bcc3e4c16dd3fca = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_ecbeafea383029e9b806c4ae34e056b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6e2675450c232bea0bae77a20425561 = $(`<div id="html_a6e2675450c232bea0bae77a20425561" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ecbeafea383029e9b806c4ae34e056b7.setContent(html_a6e2675450c232bea0bae77a20425561);\\n \\n \\n\\n circle_marker_6e3f953fb9be24a32bcc3e4c16dd3fca.bindPopup(popup_ecbeafea383029e9b806c4ae34e056b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_002a3204f99c77d75c42584dbe273af7 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_7d64e78dd358ab13fb5b99b17975a671 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc30055faad00c9b64d362076e02389a = $(`<div id="html_cc30055faad00c9b64d362076e02389a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d64e78dd358ab13fb5b99b17975a671.setContent(html_cc30055faad00c9b64d362076e02389a);\\n \\n \\n\\n circle_marker_002a3204f99c77d75c42584dbe273af7.bindPopup(popup_7d64e78dd358ab13fb5b99b17975a671)\\n ;\\n\\n \\n \\n \\n var circle_marker_c246455ec5f1887da4b125c39d5b33a2 = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e41ac62f90ea5bc195cdeb0889dd7e89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dabe66b30ceb025c92c7499ba8c9e91c = $(`<div id="html_dabe66b30ceb025c92c7499ba8c9e91c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e41ac62f90ea5bc195cdeb0889dd7e89.setContent(html_dabe66b30ceb025c92c7499ba8c9e91c);\\n \\n \\n\\n circle_marker_c246455ec5f1887da4b125c39d5b33a2.bindPopup(popup_e41ac62f90ea5bc195cdeb0889dd7e89)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec4bf866cfaa27acb4383b1f97da4264 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_072369f65131c5eb6fe6a1de44004a3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9eabd41d3c7fc852731991de5ad170c = $(`<div id="html_a9eabd41d3c7fc852731991de5ad170c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_072369f65131c5eb6fe6a1de44004a3e.setContent(html_a9eabd41d3c7fc852731991de5ad170c);\\n \\n \\n\\n circle_marker_ec4bf866cfaa27acb4383b1f97da4264.bindPopup(popup_072369f65131c5eb6fe6a1de44004a3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b1449f077ef9e93bb93e77b730e062b = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a73bc1d19425f6ae2ddb831ad76c3c66 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8bd0f652ff778cf7d18eeb369a29085c = $(`<div id="html_8bd0f652ff778cf7d18eeb369a29085c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a73bc1d19425f6ae2ddb831ad76c3c66.setContent(html_8bd0f652ff778cf7d18eeb369a29085c);\\n \\n \\n\\n circle_marker_6b1449f077ef9e93bb93e77b730e062b.bindPopup(popup_a73bc1d19425f6ae2ddb831ad76c3c66)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a509462a0dd7ccda89e5af76805f7c5 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_afd79b773e307902c390e7d204822bfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ebdba0f2b39f2fcb8148aa800744236 = $(`<div id="html_6ebdba0f2b39f2fcb8148aa800744236" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_afd79b773e307902c390e7d204822bfe.setContent(html_6ebdba0f2b39f2fcb8148aa800744236);\\n \\n \\n\\n circle_marker_2a509462a0dd7ccda89e5af76805f7c5.bindPopup(popup_afd79b773e307902c390e7d204822bfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9ca9d191fc156232f4955b04988dbf8 = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d0416e25309ff87e924a9aeca26a825e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cfc2a3f4762c7d7e843bb1cde754e79e = $(`<div id="html_cfc2a3f4762c7d7e843bb1cde754e79e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0416e25309ff87e924a9aeca26a825e.setContent(html_cfc2a3f4762c7d7e843bb1cde754e79e);\\n \\n \\n\\n circle_marker_e9ca9d191fc156232f4955b04988dbf8.bindPopup(popup_d0416e25309ff87e924a9aeca26a825e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ad84c107093eb4f392c055ef42b4941f = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8fc86ea823ceae690db54fae62f6714e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4aa253a2710d1c27cbc4b0f75165ce17 = $(`<div id="html_4aa253a2710d1c27cbc4b0f75165ce17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fc86ea823ceae690db54fae62f6714e.setContent(html_4aa253a2710d1c27cbc4b0f75165ce17);\\n \\n \\n\\n circle_marker_ad84c107093eb4f392c055ef42b4941f.bindPopup(popup_8fc86ea823ceae690db54fae62f6714e)\\n ;\\n\\n \\n \\n \\n var circle_marker_66ef5cf0363ef6a4e7f8f868d4d9ba17 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_977d39026c890ecc85e5ba9ca273d99f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_673c1fbfff64435733b58bf7beebf2cf = $(`<div id="html_673c1fbfff64435733b58bf7beebf2cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_977d39026c890ecc85e5ba9ca273d99f.setContent(html_673c1fbfff64435733b58bf7beebf2cf);\\n \\n \\n\\n circle_marker_66ef5cf0363ef6a4e7f8f868d4d9ba17.bindPopup(popup_977d39026c890ecc85e5ba9ca273d99f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6dd56c20716b78f3f4f61ac8c19157f5 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_c01c1cb29fd094511e533cdb0670f58a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30b1d27e0aa8ff4ab759cfb5c3d4c827 = $(`<div id="html_30b1d27e0aa8ff4ab759cfb5c3d4c827" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c01c1cb29fd094511e533cdb0670f58a.setContent(html_30b1d27e0aa8ff4ab759cfb5c3d4c827);\\n \\n \\n\\n circle_marker_6dd56c20716b78f3f4f61ac8c19157f5.bindPopup(popup_c01c1cb29fd094511e533cdb0670f58a)\\n ;\\n\\n \\n \\n \\n var circle_marker_d795676e82dc3913e7a8a79afcd624ac = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_44261eb2e2314827bd3200285a832443 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9527072fb37ce69ba7ba2ebf10196f85 = $(`<div id="html_9527072fb37ce69ba7ba2ebf10196f85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_44261eb2e2314827bd3200285a832443.setContent(html_9527072fb37ce69ba7ba2ebf10196f85);\\n \\n \\n\\n circle_marker_d795676e82dc3913e7a8a79afcd624ac.bindPopup(popup_44261eb2e2314827bd3200285a832443)\\n ;\\n\\n \\n \\n \\n var circle_marker_818f7fea025748821dd29e0d4249f7e3 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_cb65e0f00ff32987e170308a9f161ba2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_633ec3cb0d936406371a0b3d8a5f8c76 = $(`<div id="html_633ec3cb0d936406371a0b3d8a5f8c76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb65e0f00ff32987e170308a9f161ba2.setContent(html_633ec3cb0d936406371a0b3d8a5f8c76);\\n \\n \\n\\n circle_marker_818f7fea025748821dd29e0d4249f7e3.bindPopup(popup_cb65e0f00ff32987e170308a9f161ba2)\\n ;\\n\\n \\n \\n \\n var circle_marker_11014bb6632530fa5009e7a959499126 = L.circleMarker(\\n [42.73093032286755, -73.22550869120535],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5c7dd0dad2ebd347786ffd30bae06038 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_873c2f2c6f4177b67dedaf85eacd9411 = $(`<div id="html_873c2f2c6f4177b67dedaf85eacd9411" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c7dd0dad2ebd347786ffd30bae06038.setContent(html_873c2f2c6f4177b67dedaf85eacd9411);\\n \\n \\n\\n circle_marker_11014bb6632530fa5009e7a959499126.bindPopup(popup_5c7dd0dad2ebd347786ffd30bae06038)\\n ;\\n\\n \\n \\n \\n var circle_marker_806db9c539866f4d510cab98e3f0abc4 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e4c18c0ef6487ba884e4aad262e47bb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5213c70ef6e6e6aaacd81d63b4a754e9 = $(`<div id="html_5213c70ef6e6e6aaacd81d63b4a754e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4c18c0ef6487ba884e4aad262e47bb1.setContent(html_5213c70ef6e6e6aaacd81d63b4a754e9);\\n \\n \\n\\n circle_marker_806db9c539866f4d510cab98e3f0abc4.bindPopup(popup_e4c18c0ef6487ba884e4aad262e47bb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_ed6238b670d62497456ccd108621fb73 = L.circleMarker(\\n [42.73092945391477, -73.2230484950559],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_69e1f7af8eafe81252a77d94ea89fa87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5107001547570576a6f76ff4e1e8e839 = $(`<div id="html_5107001547570576a6f76ff4e1e8e839" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69e1f7af8eafe81252a77d94ea89fa87.setContent(html_5107001547570576a6f76ff4e1e8e839);\\n \\n \\n\\n circle_marker_ed6238b670d62497456ccd108621fb73.bindPopup(popup_69e1f7af8eafe81252a77d94ea89fa87)\\n ;\\n\\n \\n \\n \\n var circle_marker_38ec2380c0a253d76d8279adab26d6b6 = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_95c052331d058ae92e8b6eb732aaaf7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_021d0f267fac0f9655c2e6bab5eafc12 = $(`<div id="html_021d0f267fac0f9655c2e6bab5eafc12" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95c052331d058ae92e8b6eb732aaaf7b.setContent(html_021d0f267fac0f9655c2e6bab5eafc12);\\n \\n \\n\\n circle_marker_38ec2380c0a253d76d8279adab26d6b6.bindPopup(popup_95c052331d058ae92e8b6eb732aaaf7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb81829f3d54ac77460887715cc5a066 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_78cc6bb3cd191777c9378b8fa21379b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_121fb3bd1c5cb91c5dc733178ebaf386 = $(`<div id="html_121fb3bd1c5cb91c5dc733178ebaf386" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_78cc6bb3cd191777c9378b8fa21379b3.setContent(html_121fb3bd1c5cb91c5dc733178ebaf386);\\n \\n \\n\\n circle_marker_eb81829f3d54ac77460887715cc5a066.bindPopup(popup_78cc6bb3cd191777c9378b8fa21379b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b1ffaf1d3f92ed9ad716019e5147917 = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_909d6c27c99ab55af0a012dd236bae0f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e113c92a52c696f51015dcde859b072f = $(`<div id="html_e113c92a52c696f51015dcde859b072f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_909d6c27c99ab55af0a012dd236bae0f.setContent(html_e113c92a52c696f51015dcde859b072f);\\n \\n \\n\\n circle_marker_4b1ffaf1d3f92ed9ad716019e5147917.bindPopup(popup_909d6c27c99ab55af0a012dd236bae0f)\\n ;\\n\\n \\n \\n \\n var circle_marker_3fa7023c29b4961b296e651a94b4a15e = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_eebc17b4f2f93d938357e3556104d29a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_509fbfdaf4f9fb3147fd0857d1c77bf2 = $(`<div id="html_509fbfdaf4f9fb3147fd0857d1c77bf2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eebc17b4f2f93d938357e3556104d29a.setContent(html_509fbfdaf4f9fb3147fd0857d1c77bf2);\\n \\n \\n\\n circle_marker_3fa7023c29b4961b296e651a94b4a15e.bindPopup(popup_eebc17b4f2f93d938357e3556104d29a)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d7155e6b3753ad8e2ac115cea048fe6 = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_716c8092b108ac0e8d774b4d062d0c48 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f0791d2d05a046c0c7c140fb3553863 = $(`<div id="html_1f0791d2d05a046c0c7c140fb3553863" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_716c8092b108ac0e8d774b4d062d0c48.setContent(html_1f0791d2d05a046c0c7c140fb3553863);\\n \\n \\n\\n circle_marker_0d7155e6b3753ad8e2ac115cea048fe6.bindPopup(popup_716c8092b108ac0e8d774b4d062d0c48)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a46780c11fb42bdb71f0b410d91872b = L.circleMarker(\\n [42.729129693483614, -73.27225221092779],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_cbf4ed6bf24984f1fa68c0f6b200d6f7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_511defb60dacc6b96fb3acc6e415b913 = $(`<div id="html_511defb60dacc6b96fb3acc6e415b913" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cbf4ed6bf24984f1fa68c0f6b200d6f7.setContent(html_511defb60dacc6b96fb3acc6e415b913);\\n \\n \\n\\n circle_marker_2a46780c11fb42bdb71f0b410d91872b.bindPopup(popup_cbf4ed6bf24984f1fa68c0f6b200d6f7)\\n ;\\n\\n \\n \\n \\n var circle_marker_989c2d9758b40ae2519108853708d18f = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5834674fc8e2a0c47b068b78ccdf8b62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba463b52b36cc1cbd99bc44361bd2cbb = $(`<div id="html_ba463b52b36cc1cbd99bc44361bd2cbb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5834674fc8e2a0c47b068b78ccdf8b62.setContent(html_ba463b52b36cc1cbd99bc44361bd2cbb);\\n \\n \\n\\n circle_marker_989c2d9758b40ae2519108853708d18f.bindPopup(popup_5834674fc8e2a0c47b068b78ccdf8b62)\\n ;\\n\\n \\n \\n \\n var circle_marker_472e3322d40fdb84b120d474127ebb10 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0c1c6031efbe98187fef93477bce05b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49f240b741963ce12d19019a0ad08df2 = $(`<div id="html_49f240b741963ce12d19019a0ad08df2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c1c6031efbe98187fef93477bce05b5.setContent(html_49f240b741963ce12d19019a0ad08df2);\\n \\n \\n\\n circle_marker_472e3322d40fdb84b120d474127ebb10.bindPopup(popup_0c1c6031efbe98187fef93477bce05b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_deafb2be36bb77ccceaa9a16df48b0e7 = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b4a629b10297f8f678b1dcbef00e2670 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bfd50a3ebd98b1055659e6aaad8955b1 = $(`<div id="html_bfd50a3ebd98b1055659e6aaad8955b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b4a629b10297f8f678b1dcbef00e2670.setContent(html_bfd50a3ebd98b1055659e6aaad8955b1);\\n \\n \\n\\n circle_marker_deafb2be36bb77ccceaa9a16df48b0e7.bindPopup(popup_b4a629b10297f8f678b1dcbef00e2670)\\n ;\\n\\n \\n \\n \\n var circle_marker_aff279598593511e749ffeba43aefc17 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5429611b774bf4b687541bab33ec8989 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25f2a65716d631275ae22b9dad9fab0b = $(`<div id="html_25f2a65716d631275ae22b9dad9fab0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5429611b774bf4b687541bab33ec8989.setContent(html_25f2a65716d631275ae22b9dad9fab0b);\\n \\n \\n\\n circle_marker_aff279598593511e749ffeba43aefc17.bindPopup(popup_5429611b774bf4b687541bab33ec8989)\\n ;\\n\\n \\n \\n \\n var circle_marker_b664b3c69e7031010ec913650b3f3fa3 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_06d5f8a4e6286c2842e93f0ed7f05861 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6c907b238753242f105cea6e2488fc4d = $(`<div id="html_6c907b238753242f105cea6e2488fc4d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06d5f8a4e6286c2842e93f0ed7f05861.setContent(html_6c907b238753242f105cea6e2488fc4d);\\n \\n \\n\\n circle_marker_b664b3c69e7031010ec913650b3f3fa3.bindPopup(popup_06d5f8a4e6286c2842e93f0ed7f05861)\\n ;\\n\\n \\n \\n \\n var circle_marker_f44d937502ff72a19b76118a42557f15 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_86fb1730f0f0680f3192d30c14715b13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38b1fdbb1a2598d14709196c7ddd5584 = $(`<div id="html_38b1fdbb1a2598d14709196c7ddd5584" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86fb1730f0f0680f3192d30c14715b13.setContent(html_38b1fdbb1a2598d14709196c7ddd5584);\\n \\n \\n\\n circle_marker_f44d937502ff72a19b76118a42557f15.bindPopup(popup_86fb1730f0f0680f3192d30c14715b13)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8c407899f73fa88b9b02342d320533c = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0306337e507370bff9dbc033b86c2a38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_874208675e61b7bd92eccd32176a71e9 = $(`<div id="html_874208675e61b7bd92eccd32176a71e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0306337e507370bff9dbc033b86c2a38.setContent(html_874208675e61b7bd92eccd32176a71e9);\\n \\n \\n\\n circle_marker_e8c407899f73fa88b9b02342d320533c.bindPopup(popup_0306337e507370bff9dbc033b86c2a38)\\n ;\\n\\n \\n \\n \\n var circle_marker_e63e0d1725a24362d4dd0bb3e47f1d52 = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0693f5065b3ab73856914fb51b679036 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ab842e488c9efa202732ba96bf592ee = $(`<div id="html_3ab842e488c9efa202732ba96bf592ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0693f5065b3ab73856914fb51b679036.setContent(html_3ab842e488c9efa202732ba96bf592ee);\\n \\n \\n\\n circle_marker_e63e0d1725a24362d4dd0bb3e47f1d52.bindPopup(popup_0693f5065b3ab73856914fb51b679036)\\n ;\\n\\n \\n \\n \\n var circle_marker_473e5631a5650217aa7aa4f2e113e2ed = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e5a0d3d4fc446c6066ad0eb37807f68e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6de5299ae4d518c9439ebfa7038c402c = $(`<div id="html_6de5299ae4d518c9439ebfa7038c402c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5a0d3d4fc446c6066ad0eb37807f68e.setContent(html_6de5299ae4d518c9439ebfa7038c402c);\\n \\n \\n\\n circle_marker_473e5631a5650217aa7aa4f2e113e2ed.bindPopup(popup_e5a0d3d4fc446c6066ad0eb37807f68e)\\n ;\\n\\n \\n \\n \\n var circle_marker_19b06cf572ad7e768ab769a6ef12370f = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2813105f0d085e814804d7477ae18883 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd7153e399302049ccabfe3ce4508564 = $(`<div id="html_dd7153e399302049ccabfe3ce4508564" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2813105f0d085e814804d7477ae18883.setContent(html_dd7153e399302049ccabfe3ce4508564);\\n \\n \\n\\n circle_marker_19b06cf572ad7e768ab769a6ef12370f.bindPopup(popup_2813105f0d085e814804d7477ae18883)\\n ;\\n\\n \\n \\n \\n var circle_marker_e623db2ba357f78ef0b6b45b0a334ee9 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_75401ef2817cfe3c296e960241855202 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f43a8f7e7cc1162ab16ef934ea31dc62 = $(`<div id="html_f43a8f7e7cc1162ab16ef934ea31dc62" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75401ef2817cfe3c296e960241855202.setContent(html_f43a8f7e7cc1162ab16ef934ea31dc62);\\n \\n \\n\\n circle_marker_e623db2ba357f78ef0b6b45b0a334ee9.bindPopup(popup_75401ef2817cfe3c296e960241855202)\\n ;\\n\\n \\n \\n \\n var circle_marker_be8ccf3cd60562c657c97347eaa9abb4 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_e30097a1bd5e4dbf20948c4870295861 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc90c2d559b8e91311562dceed3e3171 = $(`<div id="html_bc90c2d559b8e91311562dceed3e3171" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e30097a1bd5e4dbf20948c4870295861.setContent(html_bc90c2d559b8e91311562dceed3e3171);\\n \\n \\n\\n circle_marker_be8ccf3cd60562c657c97347eaa9abb4.bindPopup(popup_e30097a1bd5e4dbf20948c4870295861)\\n ;\\n\\n \\n \\n \\n var circle_marker_b12136527bebfb14b0f85f6b0d780347 = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_13c79ad7d3532c476122051516161429 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e456a8bd9775f6bbda1ae5fba869a6c = $(`<div id="html_8e456a8bd9775f6bbda1ae5fba869a6c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13c79ad7d3532c476122051516161429.setContent(html_8e456a8bd9775f6bbda1ae5fba869a6c);\\n \\n \\n\\n circle_marker_b12136527bebfb14b0f85f6b0d780347.bindPopup(popup_13c79ad7d3532c476122051516161429)\\n ;\\n\\n \\n \\n \\n var circle_marker_d07ed76eca7e8ff0dcaf3558305d487f = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_33020a2a9309220593df99a12d27214d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c9a26f23476ab95f75d4f234e696bf4 = $(`<div id="html_7c9a26f23476ab95f75d4f234e696bf4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33020a2a9309220593df99a12d27214d.setContent(html_7c9a26f23476ab95f75d4f234e696bf4);\\n \\n \\n\\n circle_marker_d07ed76eca7e8ff0dcaf3558305d487f.bindPopup(popup_33020a2a9309220593df99a12d27214d)\\n ;\\n\\n \\n \\n \\n var circle_marker_9c7e538105c7e63ee7660540714db9cf = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_793c52909aa57d7e7052352bfc10c376 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2be9ec9737f3787a9f74cc402d7da27f = $(`<div id="html_2be9ec9737f3787a9f74cc402d7da27f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_793c52909aa57d7e7052352bfc10c376.setContent(html_2be9ec9737f3787a9f74cc402d7da27f);\\n \\n \\n\\n circle_marker_9c7e538105c7e63ee7660540714db9cf.bindPopup(popup_793c52909aa57d7e7052352bfc10c376)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b7a2c395374dad4753f2c2e592457be = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_62a1204f1885c73b1bbc53927a466078 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5bb3d085f078c4bc4e1e10bf995ed168 = $(`<div id="html_5bb3d085f078c4bc4e1e10bf995ed168" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62a1204f1885c73b1bbc53927a466078.setContent(html_5bb3d085f078c4bc4e1e10bf995ed168);\\n \\n \\n\\n circle_marker_6b7a2c395374dad4753f2c2e592457be.bindPopup(popup_62a1204f1885c73b1bbc53927a466078)\\n ;\\n\\n \\n \\n \\n var circle_marker_a37c4d33eee6f5eebe8e7c26e30e89f9 = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_9bfa70304ed5903cc52c20606011dacd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f348058236a0d9038f18576b65ce6345 = $(`<div id="html_f348058236a0d9038f18576b65ce6345" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9bfa70304ed5903cc52c20606011dacd.setContent(html_f348058236a0d9038f18576b65ce6345);\\n \\n \\n\\n circle_marker_a37c4d33eee6f5eebe8e7c26e30e89f9.bindPopup(popup_9bfa70304ed5903cc52c20606011dacd)\\n ;\\n\\n \\n \\n \\n var circle_marker_c5542eb7516db09dda75a6d50a04aef0 = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_46d4aed47677d510284d3add06f0bc9b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df5e0e2df51821aa8a924ab8a88b48be = $(`<div id="html_df5e0e2df51821aa8a924ab8a88b48be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46d4aed47677d510284d3add06f0bc9b.setContent(html_df5e0e2df51821aa8a924ab8a88b48be);\\n \\n \\n\\n circle_marker_c5542eb7516db09dda75a6d50a04aef0.bindPopup(popup_46d4aed47677d510284d3add06f0bc9b)\\n ;\\n\\n \\n \\n \\n var circle_marker_4219f84fb48dad1d802e282bde31e9a1 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_5427ecf8f96e9d699c2c348db4229b71 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd1b388c0970de396a33bf643f44960c = $(`<div id="html_fd1b388c0970de396a33bf643f44960c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5427ecf8f96e9d699c2c348db4229b71.setContent(html_fd1b388c0970de396a33bf643f44960c);\\n \\n \\n\\n circle_marker_4219f84fb48dad1d802e282bde31e9a1.bindPopup(popup_5427ecf8f96e9d699c2c348db4229b71)\\n ;\\n\\n \\n \\n \\n var circle_marker_7967d73774b07e46663ded084daed0d9 = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_149aafddce8587e2637dc3e559ac2fe9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b9d52c90b2e97d2e084024fe906f0e8 = $(`<div id="html_1b9d52c90b2e97d2e084024fe906f0e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_149aafddce8587e2637dc3e559ac2fe9.setContent(html_1b9d52c90b2e97d2e084024fe906f0e8);\\n \\n \\n\\n circle_marker_7967d73774b07e46663ded084daed0d9.bindPopup(popup_149aafddce8587e2637dc3e559ac2fe9)\\n ;\\n\\n \\n \\n \\n var circle_marker_77468501a9525cdc8c41fbbdd72fe1c3 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a0ceb99939fb91373788c10e5c159341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1cdf7b30ba0d467835c07d8c16ee4d10 = $(`<div id="html_1cdf7b30ba0d467835c07d8c16ee4d10" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0ceb99939fb91373788c10e5c159341.setContent(html_1cdf7b30ba0d467835c07d8c16ee4d10);\\n \\n \\n\\n circle_marker_77468501a9525cdc8c41fbbdd72fe1c3.bindPopup(popup_a0ceb99939fb91373788c10e5c159341)\\n ;\\n\\n \\n \\n \\n var circle_marker_d8cbbc331dd4b36e33163b86f9519379 = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6f1e3569cf00887f40c12644c6679c6f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_235931efba79650f17f897bfb74dafac = $(`<div id="html_235931efba79650f17f897bfb74dafac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f1e3569cf00887f40c12644c6679c6f.setContent(html_235931efba79650f17f897bfb74dafac);\\n \\n \\n\\n circle_marker_d8cbbc331dd4b36e33163b86f9519379.bindPopup(popup_6f1e3569cf00887f40c12644c6679c6f)\\n ;\\n\\n \\n \\n \\n var circle_marker_abc62862a152385b4c555569e63e2a0a = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b7383788561a048345d9cb0df7142c8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f6fd9fc89c51df3bad838b0e29d7d55 = $(`<div id="html_8f6fd9fc89c51df3bad838b0e29d7d55" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b7383788561a048345d9cb0df7142c8b.setContent(html_8f6fd9fc89c51df3bad838b0e29d7d55);\\n \\n \\n\\n circle_marker_abc62862a152385b4c555569e63e2a0a.bindPopup(popup_b7383788561a048345d9cb0df7142c8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_ebcc388f171b5ca37f000e30115c25b6 = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_4b1397fa469a7b43cff3c651aff6ce84 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2868a8b4d4b0f36e3b9c1d25fa9635b = $(`<div id="html_d2868a8b4d4b0f36e3b9c1d25fa9635b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4b1397fa469a7b43cff3c651aff6ce84.setContent(html_d2868a8b4d4b0f36e3b9c1d25fa9635b);\\n \\n \\n\\n circle_marker_ebcc388f171b5ca37f000e30115c25b6.bindPopup(popup_4b1397fa469a7b43cff3c651aff6ce84)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b63bde6822a1f07066afa5c45c8d1f4 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_7dd802ebef9b58f27d774eb199dd1737 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fa59d30fffd89e920bd065bbb1196aa3 = $(`<div id="html_fa59d30fffd89e920bd065bbb1196aa3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7dd802ebef9b58f27d774eb199dd1737.setContent(html_fa59d30fffd89e920bd065bbb1196aa3);\\n \\n \\n\\n circle_marker_0b63bde6822a1f07066afa5c45c8d1f4.bindPopup(popup_7dd802ebef9b58f27d774eb199dd1737)\\n ;\\n\\n \\n \\n \\n var circle_marker_d18a7d67856e171ce062022e14f77c26 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6217c6ee3ff89ac6e63e012e7f417aea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f292cb9794a76b15f95294ec1e58e84 = $(`<div id="html_0f292cb9794a76b15f95294ec1e58e84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6217c6ee3ff89ac6e63e012e7f417aea.setContent(html_0f292cb9794a76b15f95294ec1e58e84);\\n \\n \\n\\n circle_marker_d18a7d67856e171ce062022e14f77c26.bindPopup(popup_6217c6ee3ff89ac6e63e012e7f417aea)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee2be20595576dd16df5e4fb4d0382fe = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a3d4ddbdf40f28e0e67b1535d0666a78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2172dcf20e3d5ded200dcd29a545113b = $(`<div id="html_2172dcf20e3d5ded200dcd29a545113b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3d4ddbdf40f28e0e67b1535d0666a78.setContent(html_2172dcf20e3d5ded200dcd29a545113b);\\n \\n \\n\\n circle_marker_ee2be20595576dd16df5e4fb4d0382fe.bindPopup(popup_a3d4ddbdf40f28e0e67b1535d0666a78)\\n ;\\n\\n \\n \\n \\n var circle_marker_4236a042082c6d51f28697ce604db09e = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_ac12757a832e5ebd6d14a594e1c4ec97 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70b539ce5817488049bc7e4d73c7e02e = $(`<div id="html_70b539ce5817488049bc7e4d73c7e02e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac12757a832e5ebd6d14a594e1c4ec97.setContent(html_70b539ce5817488049bc7e4d73c7e02e);\\n \\n \\n\\n circle_marker_4236a042082c6d51f28697ce604db09e.bindPopup(popup_ac12757a832e5ebd6d14a594e1c4ec97)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ce164007981ccad0fc9db4a8c57a7fc = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_7767151fd48da6c22e62b5207302b3c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7960f790c0ed0758157e8a454e89527d = $(`<div id="html_7960f790c0ed0758157e8a454e89527d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7767151fd48da6c22e62b5207302b3c2.setContent(html_7960f790c0ed0758157e8a454e89527d);\\n \\n \\n\\n circle_marker_2ce164007981ccad0fc9db4a8c57a7fc.bindPopup(popup_7767151fd48da6c22e62b5207302b3c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_34403bf990910b950f5125766a8fb912 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f8611ef4d8340a10069d0fe82b41f486 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cbfe086f541d8dea04fda89ec728e1ce = $(`<div id="html_cbfe086f541d8dea04fda89ec728e1ce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f8611ef4d8340a10069d0fe82b41f486.setContent(html_cbfe086f541d8dea04fda89ec728e1ce);\\n \\n \\n\\n circle_marker_34403bf990910b950f5125766a8fb912.bindPopup(popup_f8611ef4d8340a10069d0fe82b41f486)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4a4bd7e345fbdd46ba4806b2f9e04ff = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_664d2b3cb6f58e914b93b93d45b0fc62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b82bdc1767122f63dc61e81152557b4d = $(`<div id="html_b82bdc1767122f63dc61e81152557b4d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_664d2b3cb6f58e914b93b93d45b0fc62.setContent(html_b82bdc1767122f63dc61e81152557b4d);\\n \\n \\n\\n circle_marker_c4a4bd7e345fbdd46ba4806b2f9e04ff.bindPopup(popup_664d2b3cb6f58e914b93b93d45b0fc62)\\n ;\\n\\n \\n \\n \\n var circle_marker_72a1fbe906837fe8623b8251243d5860 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_7ca74bc5363256a851974df981644da2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2813021ed21a326d4156e8107a98935 = $(`<div id="html_e2813021ed21a326d4156e8107a98935" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7ca74bc5363256a851974df981644da2.setContent(html_e2813021ed21a326d4156e8107a98935);\\n \\n \\n\\n circle_marker_72a1fbe906837fe8623b8251243d5860.bindPopup(popup_7ca74bc5363256a851974df981644da2)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc22d1efe6cbade0a1cf1d8c04d4e2ad = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_6fd58cc80e338fffd686babe486f8de2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a444733d981df265a8671275520c42e = $(`<div id="html_7a444733d981df265a8671275520c42e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6fd58cc80e338fffd686babe486f8de2.setContent(html_7a444733d981df265a8671275520c42e);\\n \\n \\n\\n circle_marker_cc22d1efe6cbade0a1cf1d8c04d4e2ad.bindPopup(popup_6fd58cc80e338fffd686babe486f8de2)\\n ;\\n\\n \\n \\n \\n var circle_marker_3300e057f512e05fa5df0047d036d6e4 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a3ca6449e9e6a237a078a13f605feda3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15ad343df0159d22e6449aa7cbcdb417 = $(`<div id="html_15ad343df0159d22e6449aa7cbcdb417" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3ca6449e9e6a237a078a13f605feda3.setContent(html_15ad343df0159d22e6449aa7cbcdb417);\\n \\n \\n\\n circle_marker_3300e057f512e05fa5df0047d036d6e4.bindPopup(popup_a3ca6449e9e6a237a078a13f605feda3)\\n ;\\n\\n \\n \\n \\n var circle_marker_985becf0eca436b2bdc8be27523815d1 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_0705ad45dc5a5f61058875d4293cf935 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4245c1eeeb5c7d380bd92abd76c6e224 = $(`<div id="html_4245c1eeeb5c7d380bd92abd76c6e224" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0705ad45dc5a5f61058875d4293cf935.setContent(html_4245c1eeeb5c7d380bd92abd76c6e224);\\n \\n \\n\\n circle_marker_985becf0eca436b2bdc8be27523815d1.bindPopup(popup_0705ad45dc5a5f61058875d4293cf935)\\n ;\\n\\n \\n \\n \\n var circle_marker_26d7a68d39f36b7ea7adc922d6088c14 = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_dcd91fc463d2ec04db1ac570a935c6cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_834cc172f80fbb5fb790ebaf5919cce0 = $(`<div id="html_834cc172f80fbb5fb790ebaf5919cce0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dcd91fc463d2ec04db1ac570a935c6cb.setContent(html_834cc172f80fbb5fb790ebaf5919cce0);\\n \\n \\n\\n circle_marker_26d7a68d39f36b7ea7adc922d6088c14.bindPopup(popup_dcd91fc463d2ec04db1ac570a935c6cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_391f55ffd79ee7010ee6817c1bab929d = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_757e9df39f11941d82f6699c657abd65 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6af1efeeb19708961bf159bd4516d2f0 = $(`<div id="html_6af1efeeb19708961bf159bd4516d2f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_757e9df39f11941d82f6699c657abd65.setContent(html_6af1efeeb19708961bf159bd4516d2f0);\\n \\n \\n\\n circle_marker_391f55ffd79ee7010ee6817c1bab929d.bindPopup(popup_757e9df39f11941d82f6699c657abd65)\\n ;\\n\\n \\n \\n \\n var circle_marker_429a298b530d5d0f9d1c7bea06c3af9b = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_2e1aafc83274d7dd93b5c01d62f83a3b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_954f672afab74273a358743046debe04 = $(`<div id="html_954f672afab74273a358743046debe04" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e1aafc83274d7dd93b5c01d62f83a3b.setContent(html_954f672afab74273a358743046debe04);\\n \\n \\n\\n circle_marker_429a298b530d5d0f9d1c7bea06c3af9b.bindPopup(popup_2e1aafc83274d7dd93b5c01d62f83a3b)\\n ;\\n\\n \\n \\n \\n var circle_marker_d212c222cf3e2911a8230c29a3435c12 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_900eeca47d199310e587d7c0c905bf4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c91af38f8eea64996dc200cc8da61b2 = $(`<div id="html_1c91af38f8eea64996dc200cc8da61b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_900eeca47d199310e587d7c0c905bf4c.setContent(html_1c91af38f8eea64996dc200cc8da61b2);\\n \\n \\n\\n circle_marker_d212c222cf3e2911a8230c29a3435c12.bindPopup(popup_900eeca47d199310e587d7c0c905bf4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_59d06a485d23dc53622bff06eea5468a = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b49217f596985395ae37a668741c348b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2d1f46bcb6c234a69e0b575b81fdf58e = $(`<div id="html_2d1f46bcb6c234a69e0b575b81fdf58e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b49217f596985395ae37a668741c348b.setContent(html_2d1f46bcb6c234a69e0b575b81fdf58e);\\n \\n \\n\\n circle_marker_59d06a485d23dc53622bff06eea5468a.bindPopup(popup_b49217f596985395ae37a668741c348b)\\n ;\\n\\n \\n \\n \\n var circle_marker_d466ef53252d5df515c57008793d9834 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_a6e2954241b7ddb8171619ab4f9a4341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c56cadc27d62a57eaf49e3f5f324f9db = $(`<div id="html_c56cadc27d62a57eaf49e3f5f324f9db" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a6e2954241b7ddb8171619ab4f9a4341.setContent(html_c56cadc27d62a57eaf49e3f5f324f9db);\\n \\n \\n\\n circle_marker_d466ef53252d5df515c57008793d9834.bindPopup(popup_a6e2954241b7ddb8171619ab4f9a4341)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c68154936582820bcfed56bc14c5d9f = L.circleMarker(\\n [42.725513531087856, -73.24273200123443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.3336224206596095, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_fdfda7e529f035297c1692338b68f41c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0dfd30e63bf7487659cfd1fe5dc1ff61 = $(`<div id="html_0dfd30e63bf7487659cfd1fe5dc1ff61" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fdfda7e529f035297c1692338b68f41c.setContent(html_0dfd30e63bf7487659cfd1fe5dc1ff61);\\n \\n \\n\\n circle_marker_3c68154936582820bcfed56bc14c5d9f.bindPopup(popup_fdfda7e529f035297c1692338b68f41c)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f92a0b89f39888676ef0bf7ba38c566 = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d7f17c5a802b65fc4fc47559dc4c8ffc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8bf2c3b642befca8bec2a5fec20f4eb2 = $(`<div id="html_8bf2c3b642befca8bec2a5fec20f4eb2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d7f17c5a802b65fc4fc47559dc4c8ffc.setContent(html_8bf2c3b642befca8bec2a5fec20f4eb2);\\n \\n \\n\\n circle_marker_8f92a0b89f39888676ef0bf7ba38c566.bindPopup(popup_d7f17c5a802b65fc4fc47559dc4c8ffc)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe62cddadae1882127e8eac016081142 = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_54902c897bd9158bff7d4b7719c6b6fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3bff6f03217f6555e48de9df1379fc03 = $(`<div id="html_3bff6f03217f6555e48de9df1379fc03" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54902c897bd9158bff7d4b7719c6b6fa.setContent(html_3bff6f03217f6555e48de9df1379fc03);\\n \\n \\n\\n circle_marker_fe62cddadae1882127e8eac016081142.bindPopup(popup_54902c897bd9158bff7d4b7719c6b6fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce40809775b455b90e5376916963f710 = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_af6289bd487c09f49baf5e332d7e7729 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a70e19d15081a389456a0df926ddb60a = $(`<div id="html_a70e19d15081a389456a0df926ddb60a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af6289bd487c09f49baf5e332d7e7729.setContent(html_a70e19d15081a389456a0df926ddb60a);\\n \\n \\n\\n circle_marker_ce40809775b455b90e5376916963f710.bindPopup(popup_af6289bd487c09f49baf5e332d7e7729)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8b9302c43fb26778da3b3c83b1265eb = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d7b508247ab0b6ea6d323a53ee0731b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_23f17b8325d6b589ed73577f571dda82 = $(`<div id="html_23f17b8325d6b589ed73577f571dda82" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d7b508247ab0b6ea6d323a53ee0731b6.setContent(html_23f17b8325d6b589ed73577f571dda82);\\n \\n \\n\\n circle_marker_a8b9302c43fb26778da3b3c83b1265eb.bindPopup(popup_d7b508247ab0b6ea6d323a53ee0731b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3a011edc7732bfe769119b39a9a91a9 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8e8d5a703e2cdd4edf846516f4e5d7d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f3d7d2b35f607bb02e135fef6f12425 = $(`<div id="html_6f3d7d2b35f607bb02e135fef6f12425" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e8d5a703e2cdd4edf846516f4e5d7d5.setContent(html_6f3d7d2b35f607bb02e135fef6f12425);\\n \\n \\n\\n circle_marker_c3a011edc7732bfe769119b39a9a91a9.bindPopup(popup_8e8d5a703e2cdd4edf846516f4e5d7d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_d09966e324223da9d6c6528b08ccc291 = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_73af49bea399d039598885572612e970 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a84d7394847086137780067cd9830d91 = $(`<div id="html_a84d7394847086137780067cd9830d91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73af49bea399d039598885572612e970.setContent(html_a84d7394847086137780067cd9830d91);\\n \\n \\n\\n circle_marker_d09966e324223da9d6c6528b08ccc291.bindPopup(popup_73af49bea399d039598885572612e970)\\n ;\\n\\n \\n \\n \\n var circle_marker_c2b45298ab2e33353a0b66e1e4d04df6 = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_d5ce62afc57505fe8d6f378c5db2ee16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f36f212fe81c214cdbeecdbed7f11cb1 = $(`<div id="html_f36f212fe81c214cdbeecdbed7f11cb1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5ce62afc57505fe8d6f378c5db2ee16.setContent(html_f36f212fe81c214cdbeecdbed7f11cb1);\\n \\n \\n\\n circle_marker_c2b45298ab2e33353a0b66e1e4d04df6.bindPopup(popup_d5ce62afc57505fe8d6f378c5db2ee16)\\n ;\\n\\n \\n \\n \\n var circle_marker_ac379bc3eb3287832f91d6a30e4964f9 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_8ef41d51a063e3668ae879313c8b804f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ec1795e6cabec19315da5ff2d2bd8d2 = $(`<div id="html_3ec1795e6cabec19315da5ff2d2bd8d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ef41d51a063e3668ae879313c8b804f.setContent(html_3ec1795e6cabec19315da5ff2d2bd8d2);\\n \\n \\n\\n circle_marker_ac379bc3eb3287832f91d6a30e4964f9.bindPopup(popup_8ef41d51a063e3668ae879313c8b804f)\\n ;\\n\\n \\n \\n \\n var circle_marker_cdd37bc0ddda6a5020a1020cd68f646d = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_f749e801ee0880b2db354cad7373195f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e11b8241eddcaa11dd1567b39f8ddfb3 = $(`<div id="html_e11b8241eddcaa11dd1567b39f8ddfb3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f749e801ee0880b2db354cad7373195f.setContent(html_e11b8241eddcaa11dd1567b39f8ddfb3);\\n \\n \\n\\n circle_marker_cdd37bc0ddda6a5020a1020cd68f646d.bindPopup(popup_f749e801ee0880b2db354cad7373195f)\\n ;\\n\\n \\n \\n \\n var circle_marker_bb4db77df3e8052ef8e4f2c54f776b95 = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_bd0c3ccf1963089eb34dfcc8ffbd234c);\\n \\n \\n var popup_b0a171394bc7b960aef799c068d889a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9bab18c0633f9a25bcd598e287ea8a5b = $(`<div id="html_9bab18c0633f9a25bcd598e287ea8a5b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0a171394bc7b960aef799c068d889a3.setContent(html_9bab18c0633f9a25bcd598e287ea8a5b);\\n \\n \\n\\n circle_marker_bb4db77df3e8052ef8e4f2c54f776b95.bindPopup(popup_b0a171394bc7b960aef799c068d889a3)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Japanese barberry": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_d6ec3315e307a44b760ffada97e7111e {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_d6ec3315e307a44b760ffada97e7111e" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_d6ec3315e307a44b760ffada97e7111e = L.map(\\n "map_d6ec3315e307a44b760ffada97e7111e",\\n {\\n center: [42.73273469100676, -73.21812674084454],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_12907ec649262abf4c0cae9d7a9aa9d3 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_d6ec3315e307a44b760ffada97e7111e);\\n \\n \\n var circle_marker_decaad1ed6d94e51a2b4ab6cd6ce9618 = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_d6ec3315e307a44b760ffada97e7111e);\\n \\n \\n var popup_27dda725efceb1ac8e1707bb375c1c20 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e730ba6c714e06abe30e1f3ebd7c05e0 = $(`<div id="html_e730ba6c714e06abe30e1f3ebd7c05e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_27dda725efceb1ac8e1707bb375c1c20.setContent(html_e730ba6c714e06abe30e1f3ebd7c05e0);\\n \\n \\n\\n circle_marker_decaad1ed6d94e51a2b4ab6cd6ce9618.bindPopup(popup_27dda725efceb1ac8e1707bb375c1c20)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Larch": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_db3c49ed4bc2059cebb3b1a8f51a0356 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_db3c49ed4bc2059cebb3b1a8f51a0356" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_db3c49ed4bc2059cebb3b1a8f51a0356 = L.map(\\n "map_db3c49ed4bc2059cebb3b1a8f51a0356",\\n {\\n center: [42.73274070811492, -73.23657882176398],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_80c79a22f97c47bd5004d1af77b6778b = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_db3c49ed4bc2059cebb3b1a8f51a0356);\\n \\n \\n var circle_marker_9d06c7d735f04dae70cd1157c98493bc = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_db3c49ed4bc2059cebb3b1a8f51a0356);\\n \\n \\n var popup_31da225f2593c07146a5914ea24e8f65 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_437427a873da0cb7560e4a6eca993c89 = $(`<div id="html_437427a873da0cb7560e4a6eca993c89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31da225f2593c07146a5914ea24e8f65.setContent(html_437427a873da0cb7560e4a6eca993c89);\\n \\n \\n\\n circle_marker_9d06c7d735f04dae70cd1157c98493bc.bindPopup(popup_31da225f2593c07146a5914ea24e8f65)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5ed3774184b9baa37ccfc0fe0edff97 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_db3c49ed4bc2059cebb3b1a8f51a0356);\\n \\n \\n var popup_421da8ed613a9af0ad159fe46b7bbb96 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6bcd7121a3a63683d2bf03c53724bc07 = $(`<div id="html_6bcd7121a3a63683d2bf03c53724bc07" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_421da8ed613a9af0ad159fe46b7bbb96.setContent(html_6bcd7121a3a63683d2bf03c53724bc07);\\n \\n \\n\\n circle_marker_b5ed3774184b9baa37ccfc0fe0edff97.bindPopup(popup_421da8ed613a9af0ad159fe46b7bbb96)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Locust, black\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_8989a74d0b0f209dc74186f7148d6a91 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_8989a74d0b0f209dc74186f7148d6a91" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_8989a74d0b0f209dc74186f7148d6a91 = L.map(\\n "map_8989a74d0b0f209dc74186f7148d6a91",\\n {\\n center: [42.72551027623974, -73.22981718851463],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_a22e6459a9e9b9da5a4c384762a3ff63 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_8989a74d0b0f209dc74186f7148d6a91);\\n \\n \\n var circle_marker_8218ab6b2bdcd700be395e2c67003203 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_8989a74d0b0f209dc74186f7148d6a91);\\n \\n \\n var popup_f6bded673c2b4ac02ea60360d0f93634 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd88f6e2fba312c9a3b847f04f54de75 = $(`<div id="html_cd88f6e2fba312c9a3b847f04f54de75" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6bded673c2b4ac02ea60360d0f93634.setContent(html_cd88f6e2fba312c9a3b847f04f54de75);\\n \\n \\n\\n circle_marker_8218ab6b2bdcd700be395e2c67003203.bindPopup(popup_f6bded673c2b4ac02ea60360d0f93634)\\n ;\\n\\n \\n \\n \\n var circle_marker_344a6df451c2b35e8fdd4c3c128e08d3 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_8989a74d0b0f209dc74186f7148d6a91);\\n \\n \\n var popup_81e4f17d08b9f87f157afa723506f4c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_efe7e5dc24f95df897366dd58d3c7384 = $(`<div id="html_efe7e5dc24f95df897366dd58d3c7384" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81e4f17d08b9f87f157afa723506f4c0.setContent(html_efe7e5dc24f95df897366dd58d3c7384);\\n \\n \\n\\n circle_marker_344a6df451c2b35e8fdd4c3c128e08d3.bindPopup(popup_81e4f17d08b9f87f157afa723506f4c0)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Maleberry": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_35160f185825934a47107144f919525b {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_35160f185825934a47107144f919525b" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_35160f185825934a47107144f919525b = L.map(\\n "map_35160f185825934a47107144f919525b",\\n {\\n center: [42.73364543014111, -73.24149833662852],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_7e72ae7a8ad1b0d36c74216a3b60a4d8 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var circle_marker_032a373c27c277bfc98a988bc04d82da = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var popup_6a4c78587b8df038899cbb8a7abe0468 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6125076c6493f24aac125f5bc68b10fa = $(`<div id="html_6125076c6493f24aac125f5bc68b10fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a4c78587b8df038899cbb8a7abe0468.setContent(html_6125076c6493f24aac125f5bc68b10fa);\\n \\n \\n\\n circle_marker_032a373c27c277bfc98a988bc04d82da.bindPopup(popup_6a4c78587b8df038899cbb8a7abe0468)\\n ;\\n\\n \\n \\n \\n var circle_marker_a74407a6c6be06a3b75b77068ff1f4f4 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var popup_ef7473855aae47809b054b0098c64e82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cacaaed79175598aa44de05c655a8e92 = $(`<div id="html_cacaaed79175598aa44de05c655a8e92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef7473855aae47809b054b0098c64e82.setContent(html_cacaaed79175598aa44de05c655a8e92);\\n \\n \\n\\n circle_marker_a74407a6c6be06a3b75b77068ff1f4f4.bindPopup(popup_ef7473855aae47809b054b0098c64e82)\\n ;\\n\\n \\n \\n \\n var circle_marker_065685a65081f8ff5659cd0bdfd8d8b5 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var popup_878047f88be2d0e725ea31239b5abc57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce9ec71bb8ec972e5823566ae9437d0e = $(`<div id="html_ce9ec71bb8ec972e5823566ae9437d0e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_878047f88be2d0e725ea31239b5abc57.setContent(html_ce9ec71bb8ec972e5823566ae9437d0e);\\n \\n \\n\\n circle_marker_065685a65081f8ff5659cd0bdfd8d8b5.bindPopup(popup_878047f88be2d0e725ea31239b5abc57)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf9b177ecae109f4fec777098e1dacde = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var popup_373f4f760cf8501e057e182aa830aed3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a8c2ae7162540d90a2cc7fa44bb8d41 = $(`<div id="html_0a8c2ae7162540d90a2cc7fa44bb8d41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_373f4f760cf8501e057e182aa830aed3.setContent(html_0a8c2ae7162540d90a2cc7fa44bb8d41);\\n \\n \\n\\n circle_marker_cf9b177ecae109f4fec777098e1dacde.bindPopup(popup_373f4f760cf8501e057e182aa830aed3)\\n ;\\n\\n \\n \\n \\n var circle_marker_7db05100de7c12f23fd372878fa78dc3 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var popup_79bc8da20b8435e1805bd44ac090df20 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2bd80aad826437661b5a8aa60513f66 = $(`<div id="html_e2bd80aad826437661b5a8aa60513f66" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79bc8da20b8435e1805bd44ac090df20.setContent(html_e2bd80aad826437661b5a8aa60513f66);\\n \\n \\n\\n circle_marker_7db05100de7c12f23fd372878fa78dc3.bindPopup(popup_79bc8da20b8435e1805bd44ac090df20)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc29bc108aba69dea31551823ab9e2d2 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_35160f185825934a47107144f919525b);\\n \\n \\n var popup_da484f2b580a102b6fe625e18f01a3eb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31d3cc5045bd2eb3cf18e248fc7338ff = $(`<div id="html_31d3cc5045bd2eb3cf18e248fc7338ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_da484f2b580a102b6fe625e18f01a3eb.setContent(html_31d3cc5045bd2eb3cf18e248fc7338ff);\\n \\n \\n\\n circle_marker_cc29bc108aba69dea31551823ab9e2d2.bindPopup(popup_da484f2b580a102b6fe625e18f01a3eb)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Maple, Norway\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_868df3bd90944f564103cd6feebd6428 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_868df3bd90944f564103cd6feebd6428" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_868df3bd90944f564103cd6feebd6428 = L.map(\\n "map_868df3bd90944f564103cd6feebd6428",\\n {\\n center: [42.72822130514125, -73.23104579941447],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_265189309d151294c256a33cd9c4b3ef = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_868df3bd90944f564103cd6feebd6428);\\n \\n \\n var circle_marker_2b6de6f33bd7b1c1960b1eff12357327 = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_868df3bd90944f564103cd6feebd6428);\\n \\n \\n var popup_fa85f77896df22a705a7db330151fce7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9dc7f445b3752885fdf10e8ee7bf60ed = $(`<div id="html_9dc7f445b3752885fdf10e8ee7bf60ed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa85f77896df22a705a7db330151fce7.setContent(html_9dc7f445b3752885fdf10e8ee7bf60ed);\\n \\n \\n\\n circle_marker_2b6de6f33bd7b1c1960b1eff12357327.bindPopup(popup_fa85f77896df22a705a7db330151fce7)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb0d73495f99cdb4760aca8287244b7c = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_868df3bd90944f564103cd6feebd6428);\\n \\n \\n var popup_0f01644550cc2fdb7be5e2a778312072 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f674a8e8953786d1e29fc588de6a6151 = $(`<div id="html_f674a8e8953786d1e29fc588de6a6151" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f01644550cc2fdb7be5e2a778312072.setContent(html_f674a8e8953786d1e29fc588de6a6151);\\n \\n \\n\\n circle_marker_eb0d73495f99cdb4760aca8287244b7c.bindPopup(popup_0f01644550cc2fdb7be5e2a778312072)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8d762cc092796e1da720d701a0c7571 = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_868df3bd90944f564103cd6feebd6428);\\n \\n \\n var popup_64ecc524efacb1f4ebf9fd64e4cfe993 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e48ba51a08770c954b175eddd1277dbb = $(`<div id="html_e48ba51a08770c954b175eddd1277dbb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64ecc524efacb1f4ebf9fd64e4cfe993.setContent(html_e48ba51a08770c954b175eddd1277dbb);\\n \\n \\n\\n circle_marker_e8d762cc092796e1da720d701a0c7571.bindPopup(popup_64ecc524efacb1f4ebf9fd64e4cfe993)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Maple, mountain\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_2d7688216a2168a0f6b1345215e427af {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_2d7688216a2168a0f6b1345215e427af" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_2d7688216a2168a0f6b1345215e427af = L.map(\\n "map_2d7688216a2168a0f6b1345215e427af",\\n {\\n center: [42.73816538339206, -73.24826411182724],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_abb9762faa1e6bd017868d7c33be28d9 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var circle_marker_a267ba05272e267d39f73bf9148531df = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var popup_e3bf1ef6b4fd0b4c0fa712f0ace5f7dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_88bdc3e05c9c82404a8954f47559baf8 = $(`<div id="html_88bdc3e05c9c82404a8954f47559baf8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3bf1ef6b4fd0b4c0fa712f0ace5f7dd.setContent(html_88bdc3e05c9c82404a8954f47559baf8);\\n \\n \\n\\n circle_marker_a267ba05272e267d39f73bf9148531df.bindPopup(popup_e3bf1ef6b4fd0b4c0fa712f0ace5f7dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_f2babfe46d2e8e1e3d4266e9cae359d6 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var popup_c37c6ef3b5b7d7f55b89d80b7698f857 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95c9be6423ca19fc5a16dbd9aeca19ea = $(`<div id="html_95c9be6423ca19fc5a16dbd9aeca19ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c37c6ef3b5b7d7f55b89d80b7698f857.setContent(html_95c9be6423ca19fc5a16dbd9aeca19ea);\\n \\n \\n\\n circle_marker_f2babfe46d2e8e1e3d4266e9cae359d6.bindPopup(popup_c37c6ef3b5b7d7f55b89d80b7698f857)\\n ;\\n\\n \\n \\n \\n var circle_marker_066d9a3f9199e23c277de2f22540f692 = L.circleMarker(\\n [42.73635085311189, -73.22304483851624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var popup_a70422411f75e2e75435879d40821f56 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a10f8c5afb06af50800dd0980bfa648f = $(`<div id="html_a10f8c5afb06af50800dd0980bfa648f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a70422411f75e2e75435879d40821f56.setContent(html_a10f8c5afb06af50800dd0980bfa648f);\\n \\n \\n\\n circle_marker_066d9a3f9199e23c277de2f22540f692.bindPopup(popup_a70422411f75e2e75435879d40821f56)\\n ;\\n\\n \\n \\n \\n var circle_marker_275df1c657261eef30dff0eef3f2f1a0 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var popup_4dbb0a0a822ba96c2ec33965bc7b0dca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96e1377b68bfcff5d81c61b1e5a0180c = $(`<div id="html_96e1377b68bfcff5d81c61b1e5a0180c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4dbb0a0a822ba96c2ec33965bc7b0dca.setContent(html_96e1377b68bfcff5d81c61b1e5a0180c);\\n \\n \\n\\n circle_marker_275df1c657261eef30dff0eef3f2f1a0.bindPopup(popup_4dbb0a0a822ba96c2ec33965bc7b0dca)\\n ;\\n\\n \\n \\n \\n var circle_marker_c106c49ac67a51b5300a9bad5d494ae0 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var popup_799e14acf7be6b0e8a82fc1613d74429 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ffd117f438e05f2f1739da9d20429fd = $(`<div id="html_1ffd117f438e05f2f1739da9d20429fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_799e14acf7be6b0e8a82fc1613d74429.setContent(html_1ffd117f438e05f2f1739da9d20429fd);\\n \\n \\n\\n circle_marker_c106c49ac67a51b5300a9bad5d494ae0.bindPopup(popup_799e14acf7be6b0e8a82fc1613d74429)\\n ;\\n\\n \\n \\n \\n var circle_marker_b4f32e63995394fd976cae8014cb8f5a = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.918490759365935, "stroke": true, "weight": 3}\\n ).addTo(map_2d7688216a2168a0f6b1345215e427af);\\n \\n \\n var popup_8ae80912f736ede5b9e5f4061a320877 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de6bb8a5a9b7743ecc82a3a63fe56554 = $(`<div id="html_de6bb8a5a9b7743ecc82a3a63fe56554" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ae80912f736ede5b9e5f4061a320877.setContent(html_de6bb8a5a9b7743ecc82a3a63fe56554);\\n \\n \\n\\n circle_marker_b4f32e63995394fd976cae8014cb8f5a.bindPopup(popup_8ae80912f736ede5b9e5f4061a320877)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Maple, red\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_3261d56ffb7664e1c2746248574c024b {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_3261d56ffb7664e1c2746248574c024b" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_3261d56ffb7664e1c2746248574c024b = L.map(\\n "map_3261d56ffb7664e1c2746248574c024b",\\n {\\n center: [42.73545173466282, -73.24764986998687],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_332e010cc36ddabe62759b8ebcde3741 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var circle_marker_f71e4d0556825477f741c076bb48851a = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_80069e23e0b96187dacffc1880b52158 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_485589442ceafbea951d433057723bdf = $(`<div id="html_485589442ceafbea951d433057723bdf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80069e23e0b96187dacffc1880b52158.setContent(html_485589442ceafbea951d433057723bdf);\\n \\n \\n\\n circle_marker_f71e4d0556825477f741c076bb48851a.bindPopup(popup_80069e23e0b96187dacffc1880b52158)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcb1e000d759fac0a1db087a3f09e188 = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6b83177778fae4ff330901195811eb1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b90dc9361e4ac4c9ad77a1d6d16b1dd = $(`<div id="html_0b90dc9361e4ac4c9ad77a1d6d16b1dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b83177778fae4ff330901195811eb1b.setContent(html_0b90dc9361e4ac4c9ad77a1d6d16b1dd);\\n \\n \\n\\n circle_marker_bcb1e000d759fac0a1db087a3f09e188.bindPopup(popup_6b83177778fae4ff330901195811eb1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_10ccbda16faa7f32ec06800b7aba0faf = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7a14bd862a7461540933173f96df0fcc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_524951eb2408abb8160789683b954542 = $(`<div id="html_524951eb2408abb8160789683b954542" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a14bd862a7461540933173f96df0fcc.setContent(html_524951eb2408abb8160789683b954542);\\n \\n \\n\\n circle_marker_10ccbda16faa7f32ec06800b7aba0faf.bindPopup(popup_7a14bd862a7461540933173f96df0fcc)\\n ;\\n\\n \\n \\n \\n var circle_marker_14c518d5faf237f4fa882919eb403dde = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_39cabc39ba486e8035cbc4026866e9e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_77052a47099eb8db1538c0d69b024e4b = $(`<div id="html_77052a47099eb8db1538c0d69b024e4b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39cabc39ba486e8035cbc4026866e9e0.setContent(html_77052a47099eb8db1538c0d69b024e4b);\\n \\n \\n\\n circle_marker_14c518d5faf237f4fa882919eb403dde.bindPopup(popup_39cabc39ba486e8035cbc4026866e9e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_92fb2f69fccaf7777b92f2acdc391192 = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2d0b161c389a5cd396d26ddee82978e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_572a574f2c0f06fa36d43351708fcdeb = $(`<div id="html_572a574f2c0f06fa36d43351708fcdeb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d0b161c389a5cd396d26ddee82978e3.setContent(html_572a574f2c0f06fa36d43351708fcdeb);\\n \\n \\n\\n circle_marker_92fb2f69fccaf7777b92f2acdc391192.bindPopup(popup_2d0b161c389a5cd396d26ddee82978e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_3105fbde80c89597e196c31cdfb349a2 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a01b27185cd1ed3d573afac024bfa3df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e697b8eceede2d3e8c69b44917f148a = $(`<div id="html_9e697b8eceede2d3e8c69b44917f148a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a01b27185cd1ed3d573afac024bfa3df.setContent(html_9e697b8eceede2d3e8c69b44917f148a);\\n \\n \\n\\n circle_marker_3105fbde80c89597e196c31cdfb349a2.bindPopup(popup_a01b27185cd1ed3d573afac024bfa3df)\\n ;\\n\\n \\n \\n \\n var circle_marker_788b6bfbabedcc9dabe67ec6a184d08b = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_616dff010468c1e46204312a82b3f583 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6725625c0e7181c334913e5f9b219de1 = $(`<div id="html_6725625c0e7181c334913e5f9b219de1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_616dff010468c1e46204312a82b3f583.setContent(html_6725625c0e7181c334913e5f9b219de1);\\n \\n \\n\\n circle_marker_788b6bfbabedcc9dabe67ec6a184d08b.bindPopup(popup_616dff010468c1e46204312a82b3f583)\\n ;\\n\\n \\n \\n \\n var circle_marker_cae2c4f05cff8b4b1526c684cae0ff20 = L.circleMarker(\\n [42.747201204933376, -73.2685630990657],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3098651044304e4b3dd27a13e2a2a43d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bbf9d3d478e04fb1ba30982cb1229c51 = $(`<div id="html_bbf9d3d478e04fb1ba30982cb1229c51" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3098651044304e4b3dd27a13e2a2a43d.setContent(html_bbf9d3d478e04fb1ba30982cb1229c51);\\n \\n \\n\\n circle_marker_cae2c4f05cff8b4b1526c684cae0ff20.bindPopup(popup_3098651044304e4b3dd27a13e2a2a43d)\\n ;\\n\\n \\n \\n \\n var circle_marker_a21e5d35733bade59c235c7d85853d2c = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9327c92c5ea1e52da0ee928a2d1c1197 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bdcdafc81826c0515c8de1b30acaabeb = $(`<div id="html_bdcdafc81826c0515c8de1b30acaabeb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9327c92c5ea1e52da0ee928a2d1c1197.setContent(html_bdcdafc81826c0515c8de1b30acaabeb);\\n \\n \\n\\n circle_marker_a21e5d35733bade59c235c7d85853d2c.bindPopup(popup_9327c92c5ea1e52da0ee928a2d1c1197)\\n ;\\n\\n \\n \\n \\n var circle_marker_c05d6b2ee22979b42d191aed0545f822 = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0a650b788c4605488f31261333741b93 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_459e4a51e87168c01ca413e3255a808d = $(`<div id="html_459e4a51e87168c01ca413e3255a808d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a650b788c4605488f31261333741b93.setContent(html_459e4a51e87168c01ca413e3255a808d);\\n \\n \\n\\n circle_marker_c05d6b2ee22979b42d191aed0545f822.bindPopup(popup_0a650b788c4605488f31261333741b93)\\n ;\\n\\n \\n \\n \\n var circle_marker_af46fb4fb80796ce216b7233e75bd4ca = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0c17a48f9828c2f9997b8475f9ba4878 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_45aee8e43c2e071c3221f85b96a40b6b = $(`<div id="html_45aee8e43c2e071c3221f85b96a40b6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c17a48f9828c2f9997b8475f9ba4878.setContent(html_45aee8e43c2e071c3221f85b96a40b6b);\\n \\n \\n\\n circle_marker_af46fb4fb80796ce216b7233e75bd4ca.bindPopup(popup_0c17a48f9828c2f9997b8475f9ba4878)\\n ;\\n\\n \\n \\n \\n var circle_marker_eae9521ea347de855ab9a9aaece5c4ec = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_72004ddc1bcb1c602ad73093e0591361 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da1efa6a7976a630643386fa897070bd = $(`<div id="html_da1efa6a7976a630643386fa897070bd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72004ddc1bcb1c602ad73093e0591361.setContent(html_da1efa6a7976a630643386fa897070bd);\\n \\n \\n\\n circle_marker_eae9521ea347de855ab9a9aaece5c4ec.bindPopup(popup_72004ddc1bcb1c602ad73093e0591361)\\n ;\\n\\n \\n \\n \\n var circle_marker_0238cf27632d83c706ea1481da921156 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_419cb02db14a404d3081a771a15ce5f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a95e8c3213f93105a6f962fd6d06aaff = $(`<div id="html_a95e8c3213f93105a6f962fd6d06aaff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_419cb02db14a404d3081a771a15ce5f2.setContent(html_a95e8c3213f93105a6f962fd6d06aaff);\\n \\n \\n\\n circle_marker_0238cf27632d83c706ea1481da921156.bindPopup(popup_419cb02db14a404d3081a771a15ce5f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_f99005c606080bd3f1b5d4cd3520cad9 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_32e0f6d6898af28bcd148c1427b12818 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_795c222e53e2f2306554d09c27214a65 = $(`<div id="html_795c222e53e2f2306554d09c27214a65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_32e0f6d6898af28bcd148c1427b12818.setContent(html_795c222e53e2f2306554d09c27214a65);\\n \\n \\n\\n circle_marker_f99005c606080bd3f1b5d4cd3520cad9.bindPopup(popup_32e0f6d6898af28bcd148c1427b12818)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d21617369d2dd0d924c80c0b1a4aacb = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6e292499763e31a9184c222d7a56ec6e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd5726412993c9eca4d31daebb7b2ad1 = $(`<div id="html_cd5726412993c9eca4d31daebb7b2ad1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e292499763e31a9184c222d7a56ec6e.setContent(html_cd5726412993c9eca4d31daebb7b2ad1);\\n \\n \\n\\n circle_marker_6d21617369d2dd0d924c80c0b1a4aacb.bindPopup(popup_6e292499763e31a9184c222d7a56ec6e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e6c7d2913394b4c0adeca262b3b7741 = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_55553336e63a30cecda44de2010a4f1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc227ec88862e1a55631de50ac6bacce = $(`<div id="html_cc227ec88862e1a55631de50ac6bacce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_55553336e63a30cecda44de2010a4f1b.setContent(html_cc227ec88862e1a55631de50ac6bacce);\\n \\n \\n\\n circle_marker_6e6c7d2913394b4c0adeca262b3b7741.bindPopup(popup_55553336e63a30cecda44de2010a4f1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_baed87699701fd4c3bfc6323707515ae = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e8b39c0d63a277b6f5da5970643ccbb0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63865eb0768f2fd5b8231a3c3ee17080 = $(`<div id="html_63865eb0768f2fd5b8231a3c3ee17080" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8b39c0d63a277b6f5da5970643ccbb0.setContent(html_63865eb0768f2fd5b8231a3c3ee17080);\\n \\n \\n\\n circle_marker_baed87699701fd4c3bfc6323707515ae.bindPopup(popup_e8b39c0d63a277b6f5da5970643ccbb0)\\n ;\\n\\n \\n \\n \\n var circle_marker_103e927aceaf5f677ee8ce3016000ec6 = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_903c63b59f29d9d48df170c2b87fa38c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44bbc1b20b347ce431beae1cc53c543f = $(`<div id="html_44bbc1b20b347ce431beae1cc53c543f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_903c63b59f29d9d48df170c2b87fa38c.setContent(html_44bbc1b20b347ce431beae1cc53c543f);\\n \\n \\n\\n circle_marker_103e927aceaf5f677ee8ce3016000ec6.bindPopup(popup_903c63b59f29d9d48df170c2b87fa38c)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1ab3aac953c03ead66b6a88b8784453 = L.circleMarker(\\n [42.74539347231661, -73.2771756878084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e4a0e77cec71457a941161a7a66793ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e158b030cc4669c333c24ceaf0d5cdd = $(`<div id="html_2e158b030cc4669c333c24ceaf0d5cdd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4a0e77cec71457a941161a7a66793ff.setContent(html_2e158b030cc4669c333c24ceaf0d5cdd);\\n \\n \\n\\n circle_marker_b1ab3aac953c03ead66b6a88b8784453.bindPopup(popup_e4a0e77cec71457a941161a7a66793ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e796050d4fda4bc374683ba3513bb18 = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6a27aad188093994235aa5bed04ab46b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_08c3834d9f09cdfcbee1926396651f82 = $(`<div id="html_08c3834d9f09cdfcbee1926396651f82" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a27aad188093994235aa5bed04ab46b.setContent(html_08c3834d9f09cdfcbee1926396651f82);\\n \\n \\n\\n circle_marker_7e796050d4fda4bc374683ba3513bb18.bindPopup(popup_6a27aad188093994235aa5bed04ab46b)\\n ;\\n\\n \\n \\n \\n var circle_marker_1981c70fc2c2f33402ac65e6c561e63c = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_da0f4f6ccfae5966a784b8ed409c144c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a60aed8d53f0ac26ad353edc45704d9e = $(`<div id="html_a60aed8d53f0ac26ad353edc45704d9e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_da0f4f6ccfae5966a784b8ed409c144c.setContent(html_a60aed8d53f0ac26ad353edc45704d9e);\\n \\n \\n\\n circle_marker_1981c70fc2c2f33402ac65e6c561e63c.bindPopup(popup_da0f4f6ccfae5966a784b8ed409c144c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b6175e2f8172831f47d04e28db79682 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_286fa5ace58e5cb4b7acf2b8468e1546 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6767c1030fb7f986b31134ad7cf7e19f = $(`<div id="html_6767c1030fb7f986b31134ad7cf7e19f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_286fa5ace58e5cb4b7acf2b8468e1546.setContent(html_6767c1030fb7f986b31134ad7cf7e19f);\\n \\n \\n\\n circle_marker_2b6175e2f8172831f47d04e28db79682.bindPopup(popup_286fa5ace58e5cb4b7acf2b8468e1546)\\n ;\\n\\n \\n \\n \\n var circle_marker_5dbb24200c18d9fab9e46bfdaeb01148 = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_54b3fcbed0173977d9716d50152605a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae7c7fb4c950a3db256be5ff10d3682a = $(`<div id="html_ae7c7fb4c950a3db256be5ff10d3682a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54b3fcbed0173977d9716d50152605a2.setContent(html_ae7c7fb4c950a3db256be5ff10d3682a);\\n \\n \\n\\n circle_marker_5dbb24200c18d9fab9e46bfdaeb01148.bindPopup(popup_54b3fcbed0173977d9716d50152605a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e69acbf7418bba80b750460e05bfa28 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ab4dd3c832522ee61ee3a506dfeea79e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fa082bc9a41bb86ee673c3bc5c3259e = $(`<div id="html_8fa082bc9a41bb86ee673c3bc5c3259e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ab4dd3c832522ee61ee3a506dfeea79e.setContent(html_8fa082bc9a41bb86ee673c3bc5c3259e);\\n \\n \\n\\n circle_marker_1e69acbf7418bba80b750460e05bfa28.bindPopup(popup_ab4dd3c832522ee61ee3a506dfeea79e)\\n ;\\n\\n \\n \\n \\n var circle_marker_77b4d9669bc57108b2767b0fa575d036 = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c4cd3769b941619b1bd7467b1cbbda63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce57b0dea0b17716b5ffb12dfc3724a2 = $(`<div id="html_ce57b0dea0b17716b5ffb12dfc3724a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4cd3769b941619b1bd7467b1cbbda63.setContent(html_ce57b0dea0b17716b5ffb12dfc3724a2);\\n \\n \\n\\n circle_marker_77b4d9669bc57108b2767b0fa575d036.bindPopup(popup_c4cd3769b941619b1bd7467b1cbbda63)\\n ;\\n\\n \\n \\n \\n var circle_marker_b2d7a55df0aebc42921ce267239cee54 = L.circleMarker(\\n [42.74539412424261, -73.26364145064247],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_52cb2e403f2972c85b0a2f3dda76f41c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60480dff5b657dc3efcb2ee771a2618a = $(`<div id="html_60480dff5b657dc3efcb2ee771a2618a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52cb2e403f2972c85b0a2f3dda76f41c.setContent(html_60480dff5b657dc3efcb2ee771a2618a);\\n \\n \\n\\n circle_marker_b2d7a55df0aebc42921ce267239cee54.bindPopup(popup_52cb2e403f2972c85b0a2f3dda76f41c)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a3d250ee7303f435f9e04648a9885ce = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0bc311e0df1b02b378b27e924f511575 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb9ce340ba252447f9455ea596385c2a = $(`<div id="html_eb9ce340ba252447f9455ea596385c2a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0bc311e0df1b02b378b27e924f511575.setContent(html_eb9ce340ba252447f9455ea596385c2a);\\n \\n \\n\\n circle_marker_9a3d250ee7303f435f9e04648a9885ce.bindPopup(popup_0bc311e0df1b02b378b27e924f511575)\\n ;\\n\\n \\n \\n \\n var circle_marker_d71e7c455f57b9ea90b61251b54da021 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c2cff4e07711bbcc8f274605ac42b320 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_829ae4d53d7847f567e0864865b9e12a = $(`<div id="html_829ae4d53d7847f567e0864865b9e12a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2cff4e07711bbcc8f274605ac42b320.setContent(html_829ae4d53d7847f567e0864865b9e12a);\\n \\n \\n\\n circle_marker_d71e7c455f57b9ea90b61251b54da021.bindPopup(popup_c2cff4e07711bbcc8f274605ac42b320)\\n ;\\n\\n \\n \\n \\n var circle_marker_fccd299cef449e0bf06ceee523fa8564 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_62b733b939ffb44e2ec94445710ea070 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_73b60c922a4ab711504d33a39671272f = $(`<div id="html_73b60c922a4ab711504d33a39671272f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62b733b939ffb44e2ec94445710ea070.setContent(html_73b60c922a4ab711504d33a39671272f);\\n \\n \\n\\n circle_marker_fccd299cef449e0bf06ceee523fa8564.bindPopup(popup_62b733b939ffb44e2ec94445710ea070)\\n ;\\n\\n \\n \\n \\n var circle_marker_104a2cf5aaaeee3705b3ca818b2f55ba = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_adff66931f242e0e5f6c7cb670f06ff6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83d0dad6b68aee90b538a67120b24b64 = $(`<div id="html_83d0dad6b68aee90b538a67120b24b64" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_adff66931f242e0e5f6c7cb670f06ff6.setContent(html_83d0dad6b68aee90b538a67120b24b64);\\n \\n \\n\\n circle_marker_104a2cf5aaaeee3705b3ca818b2f55ba.bindPopup(popup_adff66931f242e0e5f6c7cb670f06ff6)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c468b995915e172b84e108ea9f1f28f = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5357d48661fa4ec9a91fcf4345187449 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_713797ea1ac85312bc89fb089b4ef61f = $(`<div id="html_713797ea1ac85312bc89fb089b4ef61f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5357d48661fa4ec9a91fcf4345187449.setContent(html_713797ea1ac85312bc89fb089b4ef61f);\\n \\n \\n\\n circle_marker_5c468b995915e172b84e108ea9f1f28f.bindPopup(popup_5357d48661fa4ec9a91fcf4345187449)\\n ;\\n\\n \\n \\n \\n var circle_marker_0dab4fe65c3136092af4dc3bbb5b9849 = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7d8e73afa508808862f9179b79ebfa4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc7dd71d4e7c502e2ccad43f57c140f2 = $(`<div id="html_cc7dd71d4e7c502e2ccad43f57c140f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d8e73afa508808862f9179b79ebfa4c.setContent(html_cc7dd71d4e7c502e2ccad43f57c140f2);\\n \\n \\n\\n circle_marker_0dab4fe65c3136092af4dc3bbb5b9849.bindPopup(popup_7d8e73afa508808862f9179b79ebfa4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_91cb49627d61a27f00794ca6e0927cea = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_277cf81edc18ab34ae3a0c9b351775d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_34787f183c15a0db4f1b61fa73e31538 = $(`<div id="html_34787f183c15a0db4f1b61fa73e31538" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_277cf81edc18ab34ae3a0c9b351775d7.setContent(html_34787f183c15a0db4f1b61fa73e31538);\\n \\n \\n\\n circle_marker_91cb49627d61a27f00794ca6e0927cea.bindPopup(popup_277cf81edc18ab34ae3a0c9b351775d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_9c80bc63be283b1e2f52e88f9d1cb151 = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1837abc7bdce780e2c4f2a657a492c6e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bfa5a951bf3425632c556061ce82e4c2 = $(`<div id="html_bfa5a951bf3425632c556061ce82e4c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1837abc7bdce780e2c4f2a657a492c6e.setContent(html_bfa5a951bf3425632c556061ce82e4c2);\\n \\n \\n\\n circle_marker_9c80bc63be283b1e2f52e88f9d1cb151.bindPopup(popup_1837abc7bdce780e2c4f2a657a492c6e)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c5149a8beeecd6e45bf46ff6f1ce530 = L.circleMarker(\\n [42.74358667479628, -73.27348428119001],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_caeda9b0672a2a96d0092ca392d1b942 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_095de97e395095b1bebd954467537c24 = $(`<div id="html_095de97e395095b1bebd954467537c24" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_caeda9b0672a2a96d0092ca392d1b942.setContent(html_095de97e395095b1bebd954467537c24);\\n \\n \\n\\n circle_marker_5c5149a8beeecd6e45bf46ff6f1ce530.bindPopup(popup_caeda9b0672a2a96d0092ca392d1b942)\\n ;\\n\\n \\n \\n \\n var circle_marker_0298a1ecaa0e7f54e45a9c79745fb91a = L.circleMarker(\\n [42.74358676039927, -73.27225393186212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_daf2d8d8e64eda4b1bbede24b0dd499c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f55dcb7de222a0fb87aa59c1dc4ef95 = $(`<div id="html_4f55dcb7de222a0fb87aa59c1dc4ef95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_daf2d8d8e64eda4b1bbede24b0dd499c.setContent(html_4f55dcb7de222a0fb87aa59c1dc4ef95);\\n \\n \\n\\n circle_marker_0298a1ecaa0e7f54e45a9c79745fb91a.bindPopup(popup_daf2d8d8e64eda4b1bbede24b0dd499c)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a53a97b220a1477f434a72498827152 = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2c4d840392ed15588d758b04312ba239 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_549e68dda065e2d3257d34ba91cbb575 = $(`<div id="html_549e68dda065e2d3257d34ba91cbb575" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c4d840392ed15588d758b04312ba239.setContent(html_549e68dda065e2d3257d34ba91cbb575);\\n \\n \\n\\n circle_marker_4a53a97b220a1477f434a72498827152.bindPopup(popup_2c4d840392ed15588d758b04312ba239)\\n ;\\n\\n \\n \\n \\n var circle_marker_4183d810563551dff467b16025171d42 = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_566362e0cda6835125c6be25856cb66f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8791c8f46fe69f02218ebe70ce8dbd6b = $(`<div id="html_8791c8f46fe69f02218ebe70ce8dbd6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_566362e0cda6835125c6be25856cb66f.setContent(html_8791c8f46fe69f02218ebe70ce8dbd6b);\\n \\n \\n\\n circle_marker_4183d810563551dff467b16025171d42.bindPopup(popup_566362e0cda6835125c6be25856cb66f)\\n ;\\n\\n \\n \\n \\n var circle_marker_9047d4bce588f78d6c4c2d15d5eb037c = L.circleMarker(\\n [42.74358699086881, -73.26364148650833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b08d908b655787d6342c5cfa60120bdd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94e0ab063e682bdc49b4acafb2fa9b22 = $(`<div id="html_94e0ab063e682bdc49b4acafb2fa9b22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b08d908b655787d6342c5cfa60120bdd.setContent(html_94e0ab063e682bdc49b4acafb2fa9b22);\\n \\n \\n\\n circle_marker_9047d4bce588f78d6c4c2d15d5eb037c.bindPopup(popup_b08d908b655787d6342c5cfa60120bdd)\\n ;\\n\\n \\n \\n \\n var circle_marker_16cad1d45510ecee0687c0b0a2b6fea7 = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3a0b287138066893822be3261e6f9899 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_addf967bc55ea95d31d85924da190ae9 = $(`<div id="html_addf967bc55ea95d31d85924da190ae9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a0b287138066893822be3261e6f9899.setContent(html_addf967bc55ea95d31d85924da190ae9);\\n \\n \\n\\n circle_marker_16cad1d45510ecee0687c0b0a2b6fea7.bindPopup(popup_3a0b287138066893822be3261e6f9899)\\n ;\\n\\n \\n \\n \\n var circle_marker_76d45927c65d070bbe9952827376a442 = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9f9a448a13a99ea0460e189492161506 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db4a0e91eac83a3c35c1fe38d53c277b = $(`<div id="html_db4a0e91eac83a3c35c1fe38d53c277b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f9a448a13a99ea0460e189492161506.setContent(html_db4a0e91eac83a3c35c1fe38d53c277b);\\n \\n \\n\\n circle_marker_76d45927c65d070bbe9952827376a442.bindPopup(popup_9f9a448a13a99ea0460e189492161506)\\n ;\\n\\n \\n \\n \\n var circle_marker_b24f585719aa09059bc1f7e2325545ea = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bd77a2d728bd9b18bc18ce1eb6b204ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b353b242d4dd730f226d6b444345866 = $(`<div id="html_5b353b242d4dd730f226d6b444345866" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd77a2d728bd9b18bc18ce1eb6b204ec.setContent(html_5b353b242d4dd730f226d6b444345866);\\n \\n \\n\\n circle_marker_b24f585719aa09059bc1f7e2325545ea.bindPopup(popup_bd77a2d728bd9b18bc18ce1eb6b204ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_012b6034f0dd569c3a6bcb9a530fe016 = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_495a40ebc57ab2ff474e950a7db8a76f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9569d1b0d659fafdfefbb137cd609657 = $(`<div id="html_9569d1b0d659fafdfefbb137cd609657" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_495a40ebc57ab2ff474e950a7db8a76f.setContent(html_9569d1b0d659fafdfefbb137cd609657);\\n \\n \\n\\n circle_marker_012b6034f0dd569c3a6bcb9a530fe016.bindPopup(popup_495a40ebc57ab2ff474e950a7db8a76f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e954dda10cbc255c577e282fdb890775 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c45850440aebf36709a5ed3b893d9d46 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b4833a67d43f0a0faaebf01105822bcc = $(`<div id="html_b4833a67d43f0a0faaebf01105822bcc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c45850440aebf36709a5ed3b893d9d46.setContent(html_b4833a67d43f0a0faaebf01105822bcc);\\n \\n \\n\\n circle_marker_e954dda10cbc255c577e282fdb890775.bindPopup(popup_c45850440aebf36709a5ed3b893d9d46)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b5ef77cfc45f927a1926060453b4f50 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5a7969a8c34143e7b8f447f2e2fc714d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c4f29ca3dafe6149d3b75f513a22601 = $(`<div id="html_5c4f29ca3dafe6149d3b75f513a22601" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a7969a8c34143e7b8f447f2e2fc714d.setContent(html_5c4f29ca3dafe6149d3b75f513a22601);\\n \\n \\n\\n circle_marker_8b5ef77cfc45f927a1926060453b4f50.bindPopup(popup_5a7969a8c34143e7b8f447f2e2fc714d)\\n ;\\n\\n \\n \\n \\n var circle_marker_63f27eea6564c31e37d1d1196c204013 = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3041871724bed0ef59ce8792da46cbdf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09cb8d1b46214cc682c967e340c9948d = $(`<div id="html_09cb8d1b46214cc682c967e340c9948d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3041871724bed0ef59ce8792da46cbdf.setContent(html_09cb8d1b46214cc682c967e340c9948d);\\n \\n \\n\\n circle_marker_63f27eea6564c31e37d1d1196c204013.bindPopup(popup_3041871724bed0ef59ce8792da46cbdf)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ade65fc37a0d573a892b43495f68875 = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ae70aa5a56fe7286ecbde3c0397d5b22 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7bb0e7aada22dd6b888fcf8bf648b076 = $(`<div id="html_7bb0e7aada22dd6b888fcf8bf648b076" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ae70aa5a56fe7286ecbde3c0397d5b22.setContent(html_7bb0e7aada22dd6b888fcf8bf648b076);\\n \\n \\n\\n circle_marker_2ade65fc37a0d573a892b43495f68875.bindPopup(popup_ae70aa5a56fe7286ecbde3c0397d5b22)\\n ;\\n\\n \\n \\n \\n var circle_marker_2eee265459c1f92ede409df9f231a39a = L.circleMarker(\\n [42.74177983774129, -73.26733246279849],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_63e61f0e3bd5f4755d6f7d3becd6b9cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7cc6c0d14366db800630f7edf73f6c0 = $(`<div id="html_f7cc6c0d14366db800630f7edf73f6c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63e61f0e3bd5f4755d6f7d3becd6b9cb.setContent(html_f7cc6c0d14366db800630f7edf73f6c0);\\n \\n \\n\\n circle_marker_2eee265459c1f92ede409df9f231a39a.bindPopup(popup_63e61f0e3bd5f4755d6f7d3becd6b9cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f45f636ccdd724775e1e4b302a6ad10 = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_59869ece5420db5cfc2e4bca761e6ba8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6958966c6f5ec5c8eacc52d6062fc2eb = $(`<div id="html_6958966c6f5ec5c8eacc52d6062fc2eb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_59869ece5420db5cfc2e4bca761e6ba8.setContent(html_6958966c6f5ec5c8eacc52d6062fc2eb);\\n \\n \\n\\n circle_marker_8f45f636ccdd724775e1e4b302a6ad10.bindPopup(popup_59869ece5420db5cfc2e4bca761e6ba8)\\n ;\\n\\n \\n \\n \\n var circle_marker_f73858ea0ec9703c8c3877181949f40a = L.circleMarker(\\n [42.74177985749502, -73.26364152237132],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5c8c0cd84e2b1facfcdfc86ae2582458 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5824c52806efd5567987508595dc12a6 = $(`<div id="html_5824c52806efd5567987508595dc12a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c8c0cd84e2b1facfcdfc86ae2582458.setContent(html_5824c52806efd5567987508595dc12a6);\\n \\n \\n\\n circle_marker_f73858ea0ec9703c8c3877181949f40a.bindPopup(popup_5c8c0cd84e2b1facfcdfc86ae2582458)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d6c1cdda593aa956db886abc03f685c = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3a5b7d4ff298bd1314170287656e55be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd80d99478f9b1f58f2150f42e7c8d27 = $(`<div id="html_fd80d99478f9b1f58f2150f42e7c8d27" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a5b7d4ff298bd1314170287656e55be.setContent(html_fd80d99478f9b1f58f2150f42e7c8d27);\\n \\n \\n\\n circle_marker_2d6c1cdda593aa956db886abc03f685c.bindPopup(popup_3a5b7d4ff298bd1314170287656e55be)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ef96a3d05323298bce4c03976041709 = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b43769b7736f38acad7d0e3c1b855ba8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dad0ad7c59dfcdd8dc6dd20a551f6091 = $(`<div id="html_dad0ad7c59dfcdd8dc6dd20a551f6091" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b43769b7736f38acad7d0e3c1b855ba8.setContent(html_dad0ad7c59dfcdd8dc6dd20a551f6091);\\n \\n \\n\\n circle_marker_6ef96a3d05323298bce4c03976041709.bindPopup(popup_b43769b7736f38acad7d0e3c1b855ba8)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e0cbde665aa3245346ca093d6272e0c = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4dc3bc857ff27bbc8a6d72bf2255a864 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1fde72faf2331b4d5963d4bee787597 = $(`<div id="html_c1fde72faf2331b4d5963d4bee787597" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4dc3bc857ff27bbc8a6d72bf2255a864.setContent(html_c1fde72faf2331b4d5963d4bee787597);\\n \\n \\n\\n circle_marker_9e0cbde665aa3245346ca093d6272e0c.bindPopup(popup_4dc3bc857ff27bbc8a6d72bf2255a864)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f3ed1c1323f2204a1082a2c4c8a0766 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5edde0bb7239cbe80ca9a7bcc15b3498 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9445870dee198a139ec3d47677b4cbac = $(`<div id="html_9445870dee198a139ec3d47677b4cbac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5edde0bb7239cbe80ca9a7bcc15b3498.setContent(html_9445870dee198a139ec3d47677b4cbac);\\n \\n \\n\\n circle_marker_6f3ed1c1323f2204a1082a2c4c8a0766.bindPopup(popup_5edde0bb7239cbe80ca9a7bcc15b3498)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d6cbea32a6804c5bee71b487c3a7982 = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f8534462550e5a8424475149ea1a43d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f041454db23bb33be2273d153adb3ea = $(`<div id="html_6f041454db23bb33be2273d153adb3ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f8534462550e5a8424475149ea1a43d3.setContent(html_6f041454db23bb33be2273d153adb3ea);\\n \\n \\n\\n circle_marker_7d6cbea32a6804c5bee71b487c3a7982.bindPopup(popup_f8534462550e5a8424475149ea1a43d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_aeb7c6151439fe654b02ac2a78e84bb0 = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_962b6afb66e72c13197bd6b813f60011 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0fe6d927cd515b81c0dd6b714e2ff472 = $(`<div id="html_0fe6d927cd515b81c0dd6b714e2ff472" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_962b6afb66e72c13197bd6b813f60011.setContent(html_0fe6d927cd515b81c0dd6b714e2ff472);\\n \\n \\n\\n circle_marker_aeb7c6151439fe654b02ac2a78e84bb0.bindPopup(popup_962b6afb66e72c13197bd6b813f60011)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5420f72be8caa832d765ad4b7389878 = L.circleMarker(\\n [42.73997270436829, -73.26733239107837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2b74b1361df7819c79d5f81fd2d55deb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bafef2fd6720571e95aead7cb4033744 = $(`<div id="html_bafef2fd6720571e95aead7cb4033744" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b74b1361df7819c79d5f81fd2d55deb.setContent(html_bafef2fd6720571e95aead7cb4033744);\\n \\n \\n\\n circle_marker_f5420f72be8caa832d765ad4b7389878.bindPopup(popup_2b74b1361df7819c79d5f81fd2d55deb)\\n ;\\n\\n \\n \\n \\n var circle_marker_26296b407a1b96dd9519f1f9699626b9 = L.circleMarker(\\n [42.73997272412122, -73.26610211346305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e03b07adbb63194d740b03dc240c5715 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ac53dd0425688607c63ad92a8f7a54c = $(`<div id="html_6ac53dd0425688607c63ad92a8f7a54c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e03b07adbb63194d740b03dc240c5715.setContent(html_6ac53dd0425688607c63ad92a8f7a54c);\\n \\n \\n\\n circle_marker_26296b407a1b96dd9519f1f9699626b9.bindPopup(popup_e03b07adbb63194d740b03dc240c5715)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe79e4f33eeb2b5670a3d1315c0ed0da = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c75c4df19233ce6436bf2465f5befdc0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_582dfbdc66fae344763661d28414bab5 = $(`<div id="html_582dfbdc66fae344763661d28414bab5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c75c4df19233ce6436bf2465f5befdc0.setContent(html_582dfbdc66fae344763661d28414bab5);\\n \\n \\n\\n circle_marker_fe79e4f33eeb2b5670a3d1315c0ed0da.bindPopup(popup_c75c4df19233ce6436bf2465f5befdc0)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0f52d5b6a9c9df10f25d9e4a53e2a27 = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1392d1e4e46198f7bced6abbd6ebc8a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8dd94a30145f60a67ea623c2f9b75498 = $(`<div id="html_8dd94a30145f60a67ea623c2f9b75498" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1392d1e4e46198f7bced6abbd6ebc8a0.setContent(html_8dd94a30145f60a67ea623c2f9b75498);\\n \\n \\n\\n circle_marker_c0f52d5b6a9c9df10f25d9e4a53e2a27.bindPopup(popup_1392d1e4e46198f7bced6abbd6ebc8a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6a604f12d09c2fba31d8a3c24808edd = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_274f9146bc9f6faaecdee2b4f9f47dd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a957826c4de8714be8a75d973ccb6f65 = $(`<div id="html_a957826c4de8714be8a75d973ccb6f65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_274f9146bc9f6faaecdee2b4f9f47dd0.setContent(html_a957826c4de8714be8a75d973ccb6f65);\\n \\n \\n\\n circle_marker_f6a604f12d09c2fba31d8a3c24808edd.bindPopup(popup_274f9146bc9f6faaecdee2b4f9f47dd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_c42ee382a4802488058fe7b7996a2cbc = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_870f0a0bba976854de1295f6526d5cdc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3533c7bf01ebc2a170da9f2e836a5f5 = $(`<div id="html_f3533c7bf01ebc2a170da9f2e836a5f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_870f0a0bba976854de1295f6526d5cdc.setContent(html_f3533c7bf01ebc2a170da9f2e836a5f5);\\n \\n \\n\\n circle_marker_c42ee382a4802488058fe7b7996a2cbc.bindPopup(popup_870f0a0bba976854de1295f6526d5cdc)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c82303a060dedff195e8055fd01f48a = L.circleMarker(\\n [42.73997262535657, -73.25995072538909],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4652197f1f1a4abd8e2c99eaf1fc274d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3c5df384db1f242422573ed373924f41 = $(`<div id="html_3c5df384db1f242422573ed373924f41" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4652197f1f1a4abd8e2c99eaf1fc274d.setContent(html_3c5df384db1f242422573ed373924f41);\\n \\n \\n\\n circle_marker_0c82303a060dedff195e8055fd01f48a.bindPopup(popup_4652197f1f1a4abd8e2c99eaf1fc274d)\\n ;\\n\\n \\n \\n \\n var circle_marker_8dab5ed3d54935569aa9bc8d8db2b01e = L.circleMarker(\\n [42.73997256609777, -73.25872044777847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_66fd480d5ef7d4d82dc99270c692ab55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42363b85354bc4fad19b19a25c3d1023 = $(`<div id="html_42363b85354bc4fad19b19a25c3d1023" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_66fd480d5ef7d4d82dc99270c692ab55.setContent(html_42363b85354bc4fad19b19a25c3d1023);\\n \\n \\n\\n circle_marker_8dab5ed3d54935569aa9bc8d8db2b01e.bindPopup(popup_66fd480d5ef7d4d82dc99270c692ab55)\\n ;\\n\\n \\n \\n \\n var circle_marker_d18068682ea159aac253f3967a24acc1 = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_14cca811db52efc032faef94cea21eab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6138cad2c7f54c079fb052f219e03b8 = $(`<div id="html_d6138cad2c7f54c079fb052f219e03b8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14cca811db52efc032faef94cea21eab.setContent(html_d6138cad2c7f54c079fb052f219e03b8);\\n \\n \\n\\n circle_marker_d18068682ea159aac253f3967a24acc1.bindPopup(popup_14cca811db52efc032faef94cea21eab)\\n ;\\n\\n \\n \\n \\n var circle_marker_1688b6b73e246246addc9d93986cd5ab = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3de77f57ee4aea456a8ecf8789da6463 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9cd76dc220137bf1fdae9daf781b3e7 = $(`<div id="html_f9cd76dc220137bf1fdae9daf781b3e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3de77f57ee4aea456a8ecf8789da6463.setContent(html_f9cd76dc220137bf1fdae9daf781b3e7);\\n \\n \\n\\n circle_marker_1688b6b73e246246addc9d93986cd5ab.bindPopup(popup_3de77f57ee4aea456a8ecf8789da6463)\\n ;\\n\\n \\n \\n \\n var circle_marker_1391060526e45bfc3844984f7e8eb18f = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4b8a251239d10655ca533a2bdd516fce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28acacd283eb8d5b0ba4d151c291b537 = $(`<div id="html_28acacd283eb8d5b0ba4d151c291b537" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4b8a251239d10655ca533a2bdd516fce.setContent(html_28acacd283eb8d5b0ba4d151c291b537);\\n \\n \\n\\n circle_marker_1391060526e45bfc3844984f7e8eb18f.bindPopup(popup_4b8a251239d10655ca533a2bdd516fce)\\n ;\\n\\n \\n \\n \\n var circle_marker_a557f54488d22c211c99bdb16667e311 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d56b330c280a1e986b2d009edfb683bb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f4876523cfe3f2c85fe261db6e4c21b2 = $(`<div id="html_f4876523cfe3f2c85fe261db6e4c21b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d56b330c280a1e986b2d009edfb683bb.setContent(html_f4876523cfe3f2c85fe261db6e4c21b2);\\n \\n \\n\\n circle_marker_a557f54488d22c211c99bdb16667e311.bindPopup(popup_d56b330c280a1e986b2d009edfb683bb)\\n ;\\n\\n \\n \\n \\n var circle_marker_cbc7675b4842a140b5131a1b5a769182 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c141ffa1be4727e04aa12f74a9700fb3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_086d2da9596bcb2b484f09fee2b4f569 = $(`<div id="html_086d2da9596bcb2b484f09fee2b4f569" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c141ffa1be4727e04aa12f74a9700fb3.setContent(html_086d2da9596bcb2b484f09fee2b4f569);\\n \\n \\n\\n circle_marker_cbc7675b4842a140b5131a1b5a769182.bindPopup(popup_c141ffa1be4727e04aa12f74a9700fb3)\\n ;\\n\\n \\n \\n \\n var circle_marker_419a5f088ae34c206b2ebef0aa00d26c = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_fc2019664a8503d67ade364619446c27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ba86797e8bc4413938169e57d6c12a3 = $(`<div id="html_5ba86797e8bc4413938169e57d6c12a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc2019664a8503d67ade364619446c27.setContent(html_5ba86797e8bc4413938169e57d6c12a3);\\n \\n \\n\\n circle_marker_419a5f088ae34c206b2ebef0aa00d26c.bindPopup(popup_fc2019664a8503d67ade364619446c27)\\n ;\\n\\n \\n \\n \\n var circle_marker_969295ebe69b4d06bf8d2d074b1013d2 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4f5671162e381853c1fd6cb3c4c03fba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_230e6f74ae66088a2f9425d5fe0fa1a4 = $(`<div id="html_230e6f74ae66088a2f9425d5fe0fa1a4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f5671162e381853c1fd6cb3c4c03fba.setContent(html_230e6f74ae66088a2f9425d5fe0fa1a4);\\n \\n \\n\\n circle_marker_969295ebe69b4d06bf8d2d074b1013d2.bindPopup(popup_4f5671162e381853c1fd6cb3c4c03fba)\\n ;\\n\\n \\n \\n \\n var circle_marker_1044cb10e3300c388858e1623aab21ab = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9867bb5efaea7b3a425dabf54d225751 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2b4c8ccee47eff2ea882e8236c524a7 = $(`<div id="html_b2b4c8ccee47eff2ea882e8236c524a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9867bb5efaea7b3a425dabf54d225751.setContent(html_b2b4c8ccee47eff2ea882e8236c524a7);\\n \\n \\n\\n circle_marker_1044cb10e3300c388858e1623aab21ab.bindPopup(popup_9867bb5efaea7b3a425dabf54d225751)\\n ;\\n\\n \\n \\n \\n var circle_marker_ceada0804b22f7c0f0949088f9d022f6 = L.circleMarker(\\n [42.73996598837205, -73.22550295499178],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_94e9425a4a45a4df4ca9b38513ff5426 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5433f11bc75ecede2d1f82bbb7eb7018 = $(`<div id="html_5433f11bc75ecede2d1f82bbb7eb7018" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94e9425a4a45a4df4ca9b38513ff5426.setContent(html_5433f11bc75ecede2d1f82bbb7eb7018);\\n \\n \\n\\n circle_marker_ceada0804b22f7c0f0949088f9d022f6.bindPopup(popup_94e9425a4a45a4df4ca9b38513ff5426)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0eb5f3ee4332347e1f71c1b8476070e = L.circleMarker(\\n [42.73996556039193, -73.22427267765188],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2a0fe78aecf414953cae2428ed150533 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_662e69478d89c9d1082b151102618344 = $(`<div id="html_662e69478d89c9d1082b151102618344" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a0fe78aecf414953cae2428ed150533.setContent(html_662e69478d89c9d1082b151102618344);\\n \\n \\n\\n circle_marker_b0eb5f3ee4332347e1f71c1b8476070e.bindPopup(popup_2a0fe78aecf414953cae2428ed150533)\\n ;\\n\\n \\n \\n \\n var circle_marker_eca77f32228331aadb8afa89a89cbb98 = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f266dc51c9cfda264526365abf2be8d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b40190a8c4acbe7456acb2fa62cf0b4 = $(`<div id="html_9b40190a8c4acbe7456acb2fa62cf0b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f266dc51c9cfda264526365abf2be8d3.setContent(html_9b40190a8c4acbe7456acb2fa62cf0b4);\\n \\n \\n\\n circle_marker_eca77f32228331aadb8afa89a89cbb98.bindPopup(popup_f266dc51c9cfda264526365abf2be8d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_992b7f97f89e5d49ce34968b7f04cef3 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.296739856991561, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2e18989204a7973923bb15101a35a64f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b00474a78e6c52d3a5ce1cd0c15dd3c = $(`<div id="html_5b00474a78e6c52d3a5ce1cd0c15dd3c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e18989204a7973923bb15101a35a64f.setContent(html_5b00474a78e6c52d3a5ce1cd0c15dd3c);\\n \\n \\n\\n circle_marker_992b7f97f89e5d49ce34968b7f04cef3.bindPopup(popup_2e18989204a7973923bb15101a35a64f)\\n ;\\n\\n \\n \\n \\n var circle_marker_69eac39243c448f9629204c9ba2f98c3 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_044ce9a0bc05d993084d0983e32b9ce8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_45badff09f42ca8953c384930cf5d22a = $(`<div id="html_45badff09f42ca8953c384930cf5d22a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_044ce9a0bc05d993084d0983e32b9ce8.setContent(html_45badff09f42ca8953c384930cf5d22a);\\n \\n \\n\\n circle_marker_69eac39243c448f9629204c9ba2f98c3.bindPopup(popup_044ce9a0bc05d993084d0983e32b9ce8)\\n ;\\n\\n \\n \\n \\n var circle_marker_1625693f2bb35e7624c33450e17965cc = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6f8a68499a9e63ecae88b8a0a2918a28 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d54eb10f272e9c18d54d22df74a0efe7 = $(`<div id="html_d54eb10f272e9c18d54d22df74a0efe7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f8a68499a9e63ecae88b8a0a2918a28.setContent(html_d54eb10f272e9c18d54d22df74a0efe7);\\n \\n \\n\\n circle_marker_1625693f2bb35e7624c33450e17965cc.bindPopup(popup_6f8a68499a9e63ecae88b8a0a2918a28)\\n ;\\n\\n \\n \\n \\n var circle_marker_204c933a27a2b4c3d12279d87582de28 = L.circleMarker(\\n [42.73816527471333, -73.27348352812871],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f7fc0fdfbcad68e0329161b3a38c7353 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b2131066f965f8b6046059bf53bff92 = $(`<div id="html_8b2131066f965f8b6046059bf53bff92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7fc0fdfbcad68e0329161b3a38c7353.setContent(html_8b2131066f965f8b6046059bf53bff92);\\n \\n \\n\\n circle_marker_204c933a27a2b4c3d12279d87582de28.bindPopup(popup_f7fc0fdfbcad68e0329161b3a38c7353)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e8e9c0f587bf66c679ddee1ff45d9ed = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b92c18a635ca54fe84490f647f46b546 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_34f71a673c254197f9a3b9a056e2a1ff = $(`<div id="html_34f71a673c254197f9a3b9a056e2a1ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b92c18a635ca54fe84490f647f46b546.setContent(html_34f71a673c254197f9a3b9a056e2a1ff);\\n \\n \\n\\n circle_marker_2e8e9c0f587bf66c679ddee1ff45d9ed.bindPopup(popup_b92c18a635ca54fe84490f647f46b546)\\n ;\\n\\n \\n \\n \\n var circle_marker_691d08f79456450a5eb5838fe537997d = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_df15b45bb0b6f4aa09906771d0bc3f9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_363b7bebad2f26ff0f518c4bce978133 = $(`<div id="html_363b7bebad2f26ff0f518c4bce978133" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df15b45bb0b6f4aa09906771d0bc3f9c.setContent(html_363b7bebad2f26ff0f518c4bce978133);\\n \\n \\n\\n circle_marker_691d08f79456450a5eb5838fe537997d.bindPopup(popup_df15b45bb0b6f4aa09906771d0bc3f9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2224ea581b76dfdc10fa679f8b151826 = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_47ee9b6fbe70c80b6d5dbfdef230c2a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9356833422c3c56b2d9dcfe4eaef0142 = $(`<div id="html_9356833422c3c56b2d9dcfe4eaef0142" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47ee9b6fbe70c80b6d5dbfdef230c2a8.setContent(html_9356833422c3c56b2d9dcfe4eaef0142);\\n \\n \\n\\n circle_marker_2224ea581b76dfdc10fa679f8b151826.bindPopup(popup_47ee9b6fbe70c80b6d5dbfdef230c2a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f3ab26eb4babcd5c25c794c2ef78c30 = L.circleMarker(\\n [42.73816553807507, -73.26856256112116],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_be47cfe08a91c8ee0f9d320c2851f45f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41a30ced81705ab90c300170cc10f926 = $(`<div id="html_41a30ced81705ab90c300170cc10f926" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be47cfe08a91c8ee0f9d320c2851f45f.setContent(html_41a30ced81705ab90c300170cc10f926);\\n \\n \\n\\n circle_marker_2f3ab26eb4babcd5c25c794c2ef78c30.bindPopup(popup_be47cfe08a91c8ee0f9d320c2851f45f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b67f09e149a6662c12ac57e38b1fb4d = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e68969a2c785f9f5acc6c532fd7baa1c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b510c73f158f1930eca4aed044b552be = $(`<div id="html_b510c73f158f1930eca4aed044b552be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e68969a2c785f9f5acc6c532fd7baa1c.setContent(html_b510c73f158f1930eca4aed044b552be);\\n \\n \\n\\n circle_marker_1b67f09e149a6662c12ac57e38b1fb4d.bindPopup(popup_e68969a2c785f9f5acc6c532fd7baa1c)\\n ;\\n\\n \\n \\n \\n var circle_marker_99f60e66509167e77605d3d33e38fa6e = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_565d7f440eba8e7404d6cf9854a4b22f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56a1e576d3c30da3a5367f7006d7b830 = $(`<div id="html_56a1e576d3c30da3a5367f7006d7b830" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_565d7f440eba8e7404d6cf9854a4b22f.setContent(html_56a1e576d3c30da3a5367f7006d7b830);\\n \\n \\n\\n circle_marker_99f60e66509167e77605d3d33e38fa6e.bindPopup(popup_565d7f440eba8e7404d6cf9854a4b22f)\\n ;\\n\\n \\n \\n \\n var circle_marker_12b3e6512478412305356e80062a3a8d = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a493b4ea979e22a9972979718479b673 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae11a9c4a182de26ab3f181b79d7f00e = $(`<div id="html_ae11a9c4a182de26ab3f181b79d7f00e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a493b4ea979e22a9972979718479b673.setContent(html_ae11a9c4a182de26ab3f181b79d7f00e);\\n \\n \\n\\n circle_marker_12b3e6512478412305356e80062a3a8d.bindPopup(popup_a493b4ea979e22a9972979718479b673)\\n ;\\n\\n \\n \\n \\n var circle_marker_37a194190b453c4490c783ec2286680a = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8a6a6d5cbcb96242dd4b2d50a650fb83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e56b35f8d727e6d6e6536e3c33a7aaa = $(`<div id="html_4e56b35f8d727e6d6e6536e3c33a7aaa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a6a6d5cbcb96242dd4b2d50a650fb83.setContent(html_4e56b35f8d727e6d6e6536e3c33a7aaa);\\n \\n \\n\\n circle_marker_37a194190b453c4490c783ec2286680a.bindPopup(popup_8a6a6d5cbcb96242dd4b2d50a650fb83)\\n ;\\n\\n \\n \\n \\n var circle_marker_116e524fc2f1a73c51a3c98400d728a4 = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c61ecedfd75f761231297fe788037d9d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8aa5b822d2f8950b4c28278d64f4a4e5 = $(`<div id="html_8aa5b822d2f8950b4c28278d64f4a4e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c61ecedfd75f761231297fe788037d9d.setContent(html_8aa5b822d2f8950b4c28278d64f4a4e5);\\n \\n \\n\\n circle_marker_116e524fc2f1a73c51a3c98400d728a4.bindPopup(popup_c61ecedfd75f761231297fe788037d9d)\\n ;\\n\\n \\n \\n \\n var circle_marker_424f80a82ae73b6fe80ec8da665df150 = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a6e20e26ded886ae1f102f14dba05fc3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_98f7c5082a8ecb96cc333eb742c1c334 = $(`<div id="html_98f7c5082a8ecb96cc333eb742c1c334" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a6e20e26ded886ae1f102f14dba05fc3.setContent(html_98f7c5082a8ecb96cc333eb742c1c334);\\n \\n \\n\\n circle_marker_424f80a82ae73b6fe80ec8da665df150.bindPopup(popup_a6e20e26ded886ae1f102f14dba05fc3)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0c4be9a56f118ad5dc5851bcc2f78eb = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_68c85267846eb75d4f0d5f15e6aa9ae8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d37492cf12ced3ed9729b37c5fad64a0 = $(`<div id="html_d37492cf12ced3ed9729b37c5fad64a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68c85267846eb75d4f0d5f15e6aa9ae8.setContent(html_d37492cf12ced3ed9729b37c5fad64a0);\\n \\n \\n\\n circle_marker_b0c4be9a56f118ad5dc5851bcc2f78eb.bindPopup(popup_68c85267846eb75d4f0d5f15e6aa9ae8)\\n ;\\n\\n \\n \\n \\n var circle_marker_a30faf215d0ae51a1802591fb74adfe1 = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_aeef0eefdf3957d3ad160b7064f5eaf8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b5c4e4ac3cdbdf2792a1d2cd117fe598 = $(`<div id="html_b5c4e4ac3cdbdf2792a1d2cd117fe598" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aeef0eefdf3957d3ad160b7064f5eaf8.setContent(html_b5c4e4ac3cdbdf2792a1d2cd117fe598);\\n \\n \\n\\n circle_marker_a30faf215d0ae51a1802591fb74adfe1.bindPopup(popup_aeef0eefdf3957d3ad160b7064f5eaf8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b3ed9e3222a907574d8c88cde8cd5b5 = L.circleMarker(\\n [42.73816517595268, -73.25502990182166],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ef20f9b59193d2521b54d9eb0e31f978 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b96d4e52c49e02de090fb7c0dd6c320 = $(`<div id="html_9b96d4e52c49e02de090fb7c0dd6c320" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef20f9b59193d2521b54d9eb0e31f978.setContent(html_9b96d4e52c49e02de090fb7c0dd6c320);\\n \\n \\n\\n circle_marker_2b3ed9e3222a907574d8c88cde8cd5b5.bindPopup(popup_ef20f9b59193d2521b54d9eb0e31f978)\\n ;\\n\\n \\n \\n \\n var circle_marker_276d461f1155c12192ac8bfaabdeb1c3 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_de05ef1d4e5496fc1373244493b5cbce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_666d3c87b29541008a64a83714a2cae1 = $(`<div id="html_666d3c87b29541008a64a83714a2cae1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de05ef1d4e5496fc1373244493b5cbce.setContent(html_666d3c87b29541008a64a83714a2cae1);\\n \\n \\n\\n circle_marker_276d461f1155c12192ac8bfaabdeb1c3.bindPopup(popup_de05ef1d4e5496fc1373244493b5cbce)\\n ;\\n\\n \\n \\n \\n var circle_marker_24cbab96df2e4f2aed1762efd90829c8 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6cb8e265eea7a67c310cf51a0a80f3e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf73657e4aad053747e91a35756bee93 = $(`<div id="html_cf73657e4aad053747e91a35756bee93" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6cb8e265eea7a67c310cf51a0a80f3e7.setContent(html_cf73657e4aad053747e91a35756bee93);\\n \\n \\n\\n circle_marker_24cbab96df2e4f2aed1762efd90829c8.bindPopup(popup_6cb8e265eea7a67c310cf51a0a80f3e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_80b9474f7164e8f03e75a9d842e4f187 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2d83c4294c4a0de44d4e1767cd9061d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aded23314ba25e5f1d5cdea98c0cbe29 = $(`<div id="html_aded23314ba25e5f1d5cdea98c0cbe29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d83c4294c4a0de44d4e1767cd9061d8.setContent(html_aded23314ba25e5f1d5cdea98c0cbe29);\\n \\n \\n\\n circle_marker_80b9474f7164e8f03e75a9d842e4f187.bindPopup(popup_2d83c4294c4a0de44d4e1767cd9061d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ec71475529bfb08120eea0f79767c66 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e2cca3d2181407de5dcb922263c42979 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10329bcfaebecc8309d29b47537f06b1 = $(`<div id="html_10329bcfaebecc8309d29b47537f06b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2cca3d2181407de5dcb922263c42979.setContent(html_10329bcfaebecc8309d29b47537f06b1);\\n \\n \\n\\n circle_marker_7ec71475529bfb08120eea0f79767c66.bindPopup(popup_e2cca3d2181407de5dcb922263c42979)\\n ;\\n\\n \\n \\n \\n var circle_marker_a59aacd20062444f2efe7ea5fd22c755 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4c9a08fb5f9b11516b07c28d009c706f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d169ac77cce76ae82a0d038409e865b = $(`<div id="html_3d169ac77cce76ae82a0d038409e865b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c9a08fb5f9b11516b07c28d009c706f.setContent(html_3d169ac77cce76ae82a0d038409e865b);\\n \\n \\n\\n circle_marker_a59aacd20062444f2efe7ea5fd22c755.bindPopup(popup_4c9a08fb5f9b11516b07c28d009c706f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f74ff4e95022822bcd20386889847117 = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_32101cfed74834d50403473cc9a318fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a8a327e2b05a878eb7609a54faf6c50 = $(`<div id="html_5a8a327e2b05a878eb7609a54faf6c50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_32101cfed74834d50403473cc9a318fd.setContent(html_5a8a327e2b05a878eb7609a54faf6c50);\\n \\n \\n\\n circle_marker_f74ff4e95022822bcd20386889847117.bindPopup(popup_32101cfed74834d50403473cc9a318fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_d091b6330436027b09b0ed505a9eb755 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_98a692ef79c0452cacd2ddee3351f3b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ca5490878bed50b0aae258caee0c29a = $(`<div id="html_7ca5490878bed50b0aae258caee0c29a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98a692ef79c0452cacd2ddee3351f3b7.setContent(html_7ca5490878bed50b0aae258caee0c29a);\\n \\n \\n\\n circle_marker_d091b6330436027b09b0ed505a9eb755.bindPopup(popup_98a692ef79c0452cacd2ddee3351f3b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_323c6318f863b5ded473b13f26ecf84a = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d66ea042d61a9145c9472b8049cf727f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c82bc2eb1aa6aff6dea659a18c959bda = $(`<div id="html_c82bc2eb1aa6aff6dea659a18c959bda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d66ea042d61a9145c9472b8049cf727f.setContent(html_c82bc2eb1aa6aff6dea659a18c959bda);\\n \\n \\n\\n circle_marker_323c6318f863b5ded473b13f26ecf84a.bindPopup(popup_d66ea042d61a9145c9472b8049cf727f)\\n ;\\n\\n \\n \\n \\n var circle_marker_2296fb4b45e1a0688246e22657de4a79 = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c1fcad1da7b876d2b7c82e8eb85c5713 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d887c3ce1f7f7d52fa4b268d7a2af763 = $(`<div id="html_d887c3ce1f7f7d52fa4b268d7a2af763" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c1fcad1da7b876d2b7c82e8eb85c5713.setContent(html_d887c3ce1f7f7d52fa4b268d7a2af763);\\n \\n \\n\\n circle_marker_2296fb4b45e1a0688246e22657de4a79.bindPopup(popup_c1fcad1da7b876d2b7c82e8eb85c5713)\\n ;\\n\\n \\n \\n \\n var circle_marker_9cab6b96e830320545b4666e7e31a266 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a8be049a43a2bb9e775cc7556c2daf2a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7cdccc8fc95dd56236c024b8a45dbf06 = $(`<div id="html_7cdccc8fc95dd56236c024b8a45dbf06" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8be049a43a2bb9e775cc7556c2daf2a.setContent(html_7cdccc8fc95dd56236c024b8a45dbf06);\\n \\n \\n\\n circle_marker_9cab6b96e830320545b4666e7e31a266.bindPopup(popup_a8be049a43a2bb9e775cc7556c2daf2a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7fddbc2839403a51bf271c49ee107f0 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bae2827b519060ee25077088868aea82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db79b80ff1c7934de1599d0ac92f3885 = $(`<div id="html_db79b80ff1c7934de1599d0ac92f3885" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bae2827b519060ee25077088868aea82.setContent(html_db79b80ff1c7934de1599d0ac92f3885);\\n \\n \\n\\n circle_marker_f7fddbc2839403a51bf271c49ee107f0.bindPopup(popup_bae2827b519060ee25077088868aea82)\\n ;\\n\\n \\n \\n \\n var circle_marker_602c57391355cdd0848d6ce3b4078604 = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c22fbf66ec6f651a2dce6266ff4b08d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f2e940e80186f873f4240f0ceca73a3 = $(`<div id="html_8f2e940e80186f873f4240f0ceca73a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c22fbf66ec6f651a2dce6266ff4b08d7.setContent(html_8f2e940e80186f873f4240f0ceca73a3);\\n \\n \\n\\n circle_marker_602c57391355cdd0848d6ce3b4078604.bindPopup(popup_c22fbf66ec6f651a2dce6266ff4b08d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5db26ba6213a6f343cf1da67eb49211 = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_974f315323c819cd65e93fa7ec1b8781 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_61f07eec77c5c269a541d70baec49b7a = $(`<div id="html_61f07eec77c5c269a541d70baec49b7a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_974f315323c819cd65e93fa7ec1b8781.setContent(html_61f07eec77c5c269a541d70baec49b7a);\\n \\n \\n\\n circle_marker_d5db26ba6213a6f343cf1da67eb49211.bindPopup(popup_974f315323c819cd65e93fa7ec1b8781)\\n ;\\n\\n \\n \\n \\n var circle_marker_41ed913d344f26823b21b940848ca5df = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_626d11f198d60c4824a7f4a141a80e16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3427500168738972b35c0219373876f6 = $(`<div id="html_3427500168738972b35c0219373876f6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_626d11f198d60c4824a7f4a141a80e16.setContent(html_3427500168738972b35c0219373876f6);\\n \\n \\n\\n circle_marker_41ed913d344f26823b21b940848ca5df.bindPopup(popup_626d11f198d60c4824a7f4a141a80e16)\\n ;\\n\\n \\n \\n \\n var circle_marker_856c2a3e33420ee715feae58a0526096 = L.circleMarker(\\n [42.736358404703424, -73.2685624535584],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3114118f7d9a6ac79f89cfeb087810ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e8263fad8f3a645be3d454f5c122a46c = $(`<div id="html_e8263fad8f3a645be3d454f5c122a46c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3114118f7d9a6ac79f89cfeb087810ff.setContent(html_e8263fad8f3a645be3d454f5c122a46c);\\n \\n \\n\\n circle_marker_856c2a3e33420ee715feae58a0526096.bindPopup(popup_3114118f7d9a6ac79f89cfeb087810ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_64aedda44ea8df8f5960945d93a93de8 = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_dc6cd623b644bba81a97056db2a2ecff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6122315cb497bfaea5cfa4187e2dc2a = $(`<div id="html_f6122315cb497bfaea5cfa4187e2dc2a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc6cd623b644bba81a97056db2a2ecff.setContent(html_f6122315cb497bfaea5cfa4187e2dc2a);\\n \\n \\n\\n circle_marker_64aedda44ea8df8f5960945d93a93de8.bindPopup(popup_dc6cd623b644bba81a97056db2a2ecff)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7525fb990e6d8dae5cfbba006a7611d = L.circleMarker(\\n [42.73635845737364, -73.26610204175164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_617fbee8e72ff7ce24650a204ba9be8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e9ffa864d2c4cbd068524217e3385569 = $(`<div id="html_e9ffa864d2c4cbd068524217e3385569" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_617fbee8e72ff7ce24650a204ba9be8b.setContent(html_e9ffa864d2c4cbd068524217e3385569);\\n \\n \\n\\n circle_marker_e7525fb990e6d8dae5cfbba006a7611d.bindPopup(popup_617fbee8e72ff7ce24650a204ba9be8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a537fae0eac0e9aa6a9060545d3e8d3 = L.circleMarker(\\n [42.73635846395741, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_072364a9317b2413a7832701154eede1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6c5011647c5c98809468150acabc361c = $(`<div id="html_6c5011647c5c98809468150acabc361c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_072364a9317b2413a7832701154eede1.setContent(html_6c5011647c5c98809468150acabc361c);\\n \\n \\n\\n circle_marker_7a537fae0eac0e9aa6a9060545d3e8d3.bindPopup(popup_072364a9317b2413a7832701154eede1)\\n ;\\n\\n \\n \\n \\n var circle_marker_8830e7c2d47f645f56b3a38506d4b496 = L.circleMarker(\\n [42.73635845737364, -73.26364162994278],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_009c198674574e29d9c41cc55bc8c5e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_02555b2dca95c42ffdd348fe30de18bf = $(`<div id="html_02555b2dca95c42ffdd348fe30de18bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_009c198674574e29d9c41cc55bc8c5e1.setContent(html_02555b2dca95c42ffdd348fe30de18bf);\\n \\n \\n\\n circle_marker_8830e7c2d47f645f56b3a38506d4b496.bindPopup(popup_009c198674574e29d9c41cc55bc8c5e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1ceee694e43e5a390e96af65572176f = L.circleMarker(\\n [42.73635843762231, -73.26241142403887],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_35c48e4869b5169ab99416afbc7840b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed390ac8c3902c3db55a6636bdeb7990 = $(`<div id="html_ed390ac8c3902c3db55a6636bdeb7990" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35c48e4869b5169ab99416afbc7840b8.setContent(html_ed390ac8c3902c3db55a6636bdeb7990);\\n \\n \\n\\n circle_marker_d1ceee694e43e5a390e96af65572176f.bindPopup(popup_35c48e4869b5169ab99416afbc7840b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_f95244894ac0241d1327d558dd9206e9 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3581411afd08c1a31ffa27547a9166e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fde3d5ae940626a71459166cccd5232d = $(`<div id="html_fde3d5ae940626a71459166cccd5232d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3581411afd08c1a31ffa27547a9166e4.setContent(html_fde3d5ae940626a71459166cccd5232d);\\n \\n \\n\\n circle_marker_f95244894ac0241d1327d558dd9206e9.bindPopup(popup_3581411afd08c1a31ffa27547a9166e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_e07e793941e571a8f2f6f11478ac1879 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8c2ea7fd44c872ecedc35129e14d6ae1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c76b4c028963283c524ca389bff30b96 = $(`<div id="html_c76b4c028963283c524ca389bff30b96" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c2ea7fd44c872ecedc35129e14d6ae1.setContent(html_c76b4c028963283c524ca389bff30b96);\\n \\n \\n\\n circle_marker_e07e793941e571a8f2f6f11478ac1879.bindPopup(popup_8c2ea7fd44c872ecedc35129e14d6ae1)\\n ;\\n\\n \\n \\n \\n var circle_marker_dcf74d47d01f6344c60f2a6835656f72 = L.circleMarker(\\n [42.73635829936299, -73.25872080633552],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_821b96cb7c849eaa204d4d5b7a12990c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb0caacb8f76deb6e8cdfdd2dcbf5ab0 = $(`<div id="html_eb0caacb8f76deb6e8cdfdd2dcbf5ab0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_821b96cb7c849eaa204d4d5b7a12990c.setContent(html_eb0caacb8f76deb6e8cdfdd2dcbf5ab0);\\n \\n \\n\\n circle_marker_dcf74d47d01f6344c60f2a6835656f72.bindPopup(popup_821b96cb7c849eaa204d4d5b7a12990c)\\n ;\\n\\n \\n \\n \\n var circle_marker_eddecec67cbaf3adb982f2b192d8c289 = L.circleMarker(\\n [42.73635822694145, -73.25749060043893],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f8cb71d1300cf55d0be46798cdcd9135 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9bd354b5f51ca8a3c00fbb0e1c8c3f00 = $(`<div id="html_9bd354b5f51ca8a3c00fbb0e1c8c3f00" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f8cb71d1300cf55d0be46798cdcd9135.setContent(html_9bd354b5f51ca8a3c00fbb0e1c8c3f00);\\n \\n \\n\\n circle_marker_eddecec67cbaf3adb982f2b192d8c289.bindPopup(popup_f8cb71d1300cf55d0be46798cdcd9135)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea4229d82342e00aecfb2dfe1ff9df3f = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3858ae4b0d89c003456cfa0b9c0da96f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_684cd00b11f706e4185d53894f61ca92 = $(`<div id="html_684cd00b11f706e4185d53894f61ca92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3858ae4b0d89c003456cfa0b9c0da96f.setContent(html_684cd00b11f706e4185d53894f61ca92);\\n \\n \\n\\n circle_marker_ea4229d82342e00aecfb2dfe1ff9df3f.bindPopup(popup_3858ae4b0d89c003456cfa0b9c0da96f)\\n ;\\n\\n \\n \\n \\n var circle_marker_087f49aeda4ceea8eea3e8aac8c3d3d8 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_53e774b6607476375ac7700650587180 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e203be1b29b2bad2e6405548415d1741 = $(`<div id="html_e203be1b29b2bad2e6405548415d1741" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_53e774b6607476375ac7700650587180.setContent(html_e203be1b29b2bad2e6405548415d1741);\\n \\n \\n\\n circle_marker_087f49aeda4ceea8eea3e8aac8c3d3d8.bindPopup(popup_53e774b6607476375ac7700650587180)\\n ;\\n\\n \\n \\n \\n var circle_marker_b79bbeb1c69edfa38e99d09f730a1561 = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_448209888969c7707dfb3426a5883bcb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4db877a3694a1cb59b5518896b9a6ea6 = $(`<div id="html_4db877a3694a1cb59b5518896b9a6ea6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_448209888969c7707dfb3426a5883bcb.setContent(html_4db877a3694a1cb59b5518896b9a6ea6);\\n \\n \\n\\n circle_marker_b79bbeb1c69edfa38e99d09f730a1561.bindPopup(popup_448209888969c7707dfb3426a5883bcb)\\n ;\\n\\n \\n \\n \\n var circle_marker_36dbe3f090c4fc31cbab86e5cbe8bf32 = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b8d46cb2e14f1b5a834ebf7e330e45b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_34d06e187466f3cbc39599e1cb3cadb8 = $(`<div id="html_34d06e187466f3cbc39599e1cb3cadb8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b8d46cb2e14f1b5a834ebf7e330e45b6.setContent(html_34d06e187466f3cbc39599e1cb3cadb8);\\n \\n \\n\\n circle_marker_36dbe3f090c4fc31cbab86e5cbe8bf32.bindPopup(popup_b8d46cb2e14f1b5a834ebf7e330e45b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_066ad19480688397942769399efcbd58 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_cfae0ed5238cab722ee0aae7bde87012 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04b0b3e102ae03f2301dd97a8f735010 = $(`<div id="html_04b0b3e102ae03f2301dd97a8f735010" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfae0ed5238cab722ee0aae7bde87012.setContent(html_04b0b3e102ae03f2301dd97a8f735010);\\n \\n \\n\\n circle_marker_066ad19480688397942769399efcbd58.bindPopup(popup_cfae0ed5238cab722ee0aae7bde87012)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d6948794a0200328cdbe0837b66754e = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.808687281605183, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ca17a9e8246a051ee8ae47fa0f8ef559 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1d48b0778b38b289e42f4cb9ec964c2 = $(`<div id="html_d1d48b0778b38b289e42f4cb9ec964c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca17a9e8246a051ee8ae47fa0f8ef559.setContent(html_d1d48b0778b38b289e42f4cb9ec964c2);\\n \\n \\n\\n circle_marker_4d6948794a0200328cdbe0837b66754e.bindPopup(popup_ca17a9e8246a051ee8ae47fa0f8ef559)\\n ;\\n\\n \\n \\n \\n var circle_marker_8348fa0972f5a7a171c56a49973e0d90 = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.442433223290478, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_83b8d5dd88a4d1bf4daac481d543a7d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d91bd209d1fc8bc35d7e2b4e681e9b6 = $(`<div id="html_0d91bd209d1fc8bc35d7e2b4e681e9b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83b8d5dd88a4d1bf4daac481d543a7d8.setContent(html_0d91bd209d1fc8bc35d7e2b4e681e9b6);\\n \\n \\n\\n circle_marker_8348fa0972f5a7a171c56a49973e0d90.bindPopup(popup_83b8d5dd88a4d1bf4daac481d543a7d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_7781537e1162469c6d4e41b09dde9139 = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d5fdf3f4f7ea152557701c085d26130e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_271f9ee3490f6d72bb23188037136340 = $(`<div id="html_271f9ee3490f6d72bb23188037136340" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5fdf3f4f7ea152557701c085d26130e.setContent(html_271f9ee3490f6d72bb23188037136340);\\n \\n \\n\\n circle_marker_7781537e1162469c6d4e41b09dde9139.bindPopup(popup_d5fdf3f4f7ea152557701c085d26130e)\\n ;\\n\\n \\n \\n \\n var circle_marker_601af6ff4f4960f4fbba337679a9b7df = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.370193722368317, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b1f7a11d239ae8634514e7bb003694f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01e836b2d427472e2a4d05c13625f621 = $(`<div id="html_01e836b2d427472e2a4d05c13625f621" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1f7a11d239ae8634514e7bb003694f3.setContent(html_01e836b2d427472e2a4d05c13625f621);\\n \\n \\n\\n circle_marker_601af6ff4f4960f4fbba337679a9b7df.bindPopup(popup_b1f7a11d239ae8634514e7bb003694f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_fde7e3085e89052d30dbec4e6eed183a = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c3852d26e34c366634910e6b4f615714 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9f038a05d10f6266bbbe19a31268f7c = $(`<div id="html_a9f038a05d10f6266bbbe19a31268f7c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3852d26e34c366634910e6b4f615714.setContent(html_a9f038a05d10f6266bbbe19a31268f7c);\\n \\n \\n\\n circle_marker_fde7e3085e89052d30dbec4e6eed183a.bindPopup(popup_c3852d26e34c366634910e6b4f615714)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1a02ae756d89e59b476f83b8c4491a7 = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1073621666150775, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_83cb23f135ba5e64d253265b6fcd6751 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3dfd462b6c9dba8d91cd6e83b496a9ca = $(`<div id="html_3dfd462b6c9dba8d91cd6e83b496a9ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83cb23f135ba5e64d253265b6fcd6751.setContent(html_3dfd462b6c9dba8d91cd6e83b496a9ca);\\n \\n \\n\\n circle_marker_b1a02ae756d89e59b476f83b8c4491a7.bindPopup(popup_83cb23f135ba5e64d253265b6fcd6751)\\n ;\\n\\n \\n \\n \\n var circle_marker_3497291592b9bb07b299d7d0bfd55e60 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6e53ed3b78cf544525867e4f1f94cc37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb9b15c8f0220cc9bf121e01e92ad935 = $(`<div id="html_bb9b15c8f0220cc9bf121e01e92ad935" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e53ed3b78cf544525867e4f1f94cc37.setContent(html_bb9b15c8f0220cc9bf121e01e92ad935);\\n \\n \\n\\n circle_marker_3497291592b9bb07b299d7d0bfd55e60.bindPopup(popup_6e53ed3b78cf544525867e4f1f94cc37)\\n ;\\n\\n \\n \\n \\n var circle_marker_351a9c156eaf10cd5d835850fbcc73eb = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_47b85a64cbdcc7d34462edcf4f31a2f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c35dcb385cc511d7a028136c0d8bf484 = $(`<div id="html_c35dcb385cc511d7a028136c0d8bf484" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47b85a64cbdcc7d34462edcf4f31a2f2.setContent(html_c35dcb385cc511d7a028136c0d8bf484);\\n \\n \\n\\n circle_marker_351a9c156eaf10cd5d835850fbcc73eb.bindPopup(popup_47b85a64cbdcc7d34462edcf4f31a2f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a5106773583bd1dc6c3393db9a4def0 = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8c9c39a2d483ac5a5ff2800b2b17fd07 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_943dcb86cc2c5f499466741ea392dec4 = $(`<div id="html_943dcb86cc2c5f499466741ea392dec4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c9c39a2d483ac5a5ff2800b2b17fd07.setContent(html_943dcb86cc2c5f499466741ea392dec4);\\n \\n \\n\\n circle_marker_9a5106773583bd1dc6c3393db9a4def0.bindPopup(popup_8c9c39a2d483ac5a5ff2800b2b17fd07)\\n ;\\n\\n \\n \\n \\n var circle_marker_032292edc35e2b972d67a3626e1d8cdb = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.478115991081385, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c6af453a19bb1ba905dc0276b1793efd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc8e456f16e261094ffbc54d8a7b3164 = $(`<div id="html_fc8e456f16e261094ffbc54d8a7b3164" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6af453a19bb1ba905dc0276b1793efd.setContent(html_fc8e456f16e261094ffbc54d8a7b3164);\\n \\n \\n\\n circle_marker_032292edc35e2b972d67a3626e1d8cdb.bindPopup(popup_c6af453a19bb1ba905dc0276b1793efd)\\n ;\\n\\n \\n \\n \\n var circle_marker_393930009b7a79b4e59f28f344fed2f4 = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_49266f91a17a4095b1422c514f4b0af4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bc52676c24fd0a21139dd4c649f8e591 = $(`<div id="html_bc52676c24fd0a21139dd4c649f8e591" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_49266f91a17a4095b1422c514f4b0af4.setContent(html_bc52676c24fd0a21139dd4c649f8e591);\\n \\n \\n\\n circle_marker_393930009b7a79b4e59f28f344fed2f4.bindPopup(popup_49266f91a17a4095b1422c514f4b0af4)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd46842a3bdba87082bc1e43bde2b8aa = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_724ff5e76211551e5eb5e151195b90ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7ae3650c0488beca5fbe6722d95ccfc = $(`<div id="html_c7ae3650c0488beca5fbe6722d95ccfc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_724ff5e76211551e5eb5e151195b90ff.setContent(html_c7ae3650c0488beca5fbe6722d95ccfc);\\n \\n \\n\\n circle_marker_fd46842a3bdba87082bc1e43bde2b8aa.bindPopup(popup_724ff5e76211551e5eb5e151195b90ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7af9b1d9eed46634d5e9beae2d2ce9a = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b9d8020fdfc6ecc99ad6f86ecd013b49 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e62ac98cbff0fbc8d4845b92c6346c0 = $(`<div id="html_8e62ac98cbff0fbc8d4845b92c6346c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b9d8020fdfc6ecc99ad6f86ecd013b49.setContent(html_8e62ac98cbff0fbc8d4845b92c6346c0);\\n \\n \\n\\n circle_marker_b7af9b1d9eed46634d5e9beae2d2ce9a.bindPopup(popup_b9d8020fdfc6ecc99ad6f86ecd013b49)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a3c3eeff35ef4cbca674b08de9f1ad1 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c34d02c5a7dc0f790836ba8c60d64b53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f67245e71b9eea7baddab494473834a6 = $(`<div id="html_f67245e71b9eea7baddab494473834a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c34d02c5a7dc0f790836ba8c60d64b53.setContent(html_f67245e71b9eea7baddab494473834a6);\\n \\n \\n\\n circle_marker_9a3c3eeff35ef4cbca674b08de9f1ad1.bindPopup(popup_c34d02c5a7dc0f790836ba8c60d64b53)\\n ;\\n\\n \\n \\n \\n var circle_marker_380ee567cea90e468831bf18bb633c43 = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d526364d1e3ec2857dd8e06ca3573e5b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de5b448315b8cc05b047168909142e45 = $(`<div id="html_de5b448315b8cc05b047168909142e45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d526364d1e3ec2857dd8e06ca3573e5b.setContent(html_de5b448315b8cc05b047168909142e45);\\n \\n \\n\\n circle_marker_380ee567cea90e468831bf18bb633c43.bindPopup(popup_d526364d1e3ec2857dd8e06ca3573e5b)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3434493357d508ce681da9d9654f6c4 = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1454ed003e7ffd913d14ff7b01d0f176 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56b987309e3310978543ae72e494f525 = $(`<div id="html_56b987309e3310978543ae72e494f525" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1454ed003e7ffd913d14ff7b01d0f176.setContent(html_56b987309e3310978543ae72e494f525);\\n \\n \\n\\n circle_marker_f3434493357d508ce681da9d9654f6c4.bindPopup(popup_1454ed003e7ffd913d14ff7b01d0f176)\\n ;\\n\\n \\n \\n \\n var circle_marker_515c7cc5df2db6554348ab3275327424 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a7f9f023fe04bbaf9e687c1ba6243f58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95ba4a9b372cf878c11428299619e061 = $(`<div id="html_95ba4a9b372cf878c11428299619e061" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7f9f023fe04bbaf9e687c1ba6243f58.setContent(html_95ba4a9b372cf878c11428299619e061);\\n \\n \\n\\n circle_marker_515c7cc5df2db6554348ab3275327424.bindPopup(popup_a7f9f023fe04bbaf9e687c1ba6243f58)\\n ;\\n\\n \\n \\n \\n var circle_marker_0118121c4fde8c7d69c4d0911e66ccff = L.circleMarker(\\n [42.734551330583365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e52369fadaa628d091de80380d5660fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9b034488632118c991d5c05575bfea12 = $(`<div id="html_9b034488632118c991d5c05575bfea12" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e52369fadaa628d091de80380d5660fb.setContent(html_9b034488632118c991d5c05575bfea12);\\n \\n \\n\\n circle_marker_0118121c4fde8c7d69c4d0911e66ccff.bindPopup(popup_e52369fadaa628d091de80380d5660fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_41be6dc569ad5a93b4f81c68329d2c82 = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0f6a00cce17b303c20a668c3dbbfa2d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b006f8937b2d09f706c0d0d30aa2536 = $(`<div id="html_2b006f8937b2d09f706c0d0d30aa2536" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f6a00cce17b303c20a668c3dbbfa2d3.setContent(html_2b006f8937b2d09f706c0d0d30aa2536);\\n \\n \\n\\n circle_marker_41be6dc569ad5a93b4f81c68329d2c82.bindPopup(popup_0f6a00cce17b303c20a668c3dbbfa2d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_af746762b6741622a4e4b6a1b6371df7 = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0fdcbae8d26dc12340eec47716ad6e3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7dc7075f03b21cc15aba6f8a10f5ce3c = $(`<div id="html_7dc7075f03b21cc15aba6f8a10f5ce3c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0fdcbae8d26dc12340eec47716ad6e3f.setContent(html_7dc7075f03b21cc15aba6f8a10f5ce3c);\\n \\n \\n\\n circle_marker_af746762b6741622a4e4b6a1b6371df7.bindPopup(popup_0fdcbae8d26dc12340eec47716ad6e3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_98cc5c03d3cab3d78fea76c16e6b182d = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b168f821de3b851c16caea859bca0a94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd6464356e1e5d50b0edcdd59153f2ea = $(`<div id="html_bd6464356e1e5d50b0edcdd59153f2ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b168f821de3b851c16caea859bca0a94.setContent(html_bd6464356e1e5d50b0edcdd59153f2ea);\\n \\n \\n\\n circle_marker_98cc5c03d3cab3d78fea76c16e6b182d.bindPopup(popup_b168f821de3b851c16caea859bca0a94)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8eed9296d2694d74d43f401aba445e0 = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0cc5bf99b49fb64b25dcbcdefef64256 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48ead94eebf7b2bb137636abbceec402 = $(`<div id="html_48ead94eebf7b2bb137636abbceec402" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cc5bf99b49fb64b25dcbcdefef64256.setContent(html_48ead94eebf7b2bb137636abbceec402);\\n \\n \\n\\n circle_marker_f8eed9296d2694d74d43f401aba445e0.bindPopup(popup_0cc5bf99b49fb64b25dcbcdefef64256)\\n ;\\n\\n \\n \\n \\n var circle_marker_d61d21e254f74b233a839e47c5198267 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2654b3385ea1a254871db28a3593e961 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1c3b24f8819cd4fa3c6c96dc8317ed2 = $(`<div id="html_c1c3b24f8819cd4fa3c6c96dc8317ed2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2654b3385ea1a254871db28a3593e961.setContent(html_c1c3b24f8819cd4fa3c6c96dc8317ed2);\\n \\n \\n\\n circle_marker_d61d21e254f74b233a839e47c5198267.bindPopup(popup_2654b3385ea1a254871db28a3593e961)\\n ;\\n\\n \\n \\n \\n var circle_marker_3325b0779141016627e74e6381e67c6b = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a64741035e600d022a6076d5f5403e7a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25316d37415c9e109313203573bee545 = $(`<div id="html_25316d37415c9e109313203573bee545" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a64741035e600d022a6076d5f5403e7a.setContent(html_25316d37415c9e109313203573bee545);\\n \\n \\n\\n circle_marker_3325b0779141016627e74e6381e67c6b.bindPopup(popup_a64741035e600d022a6076d5f5403e7a)\\n ;\\n\\n \\n \\n \\n var circle_marker_b07edb7ed756704d34f3a26ac6889f31 = L.circleMarker(\\n [42.73455100799136, -73.25626064550488],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_64d8b32c208f0277c8e38c5fa0dd0309 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3133cc27c578b6682e23e94baaa3ba65 = $(`<div id="html_3133cc27c578b6682e23e94baaa3ba65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64d8b32c208f0277c8e38c5fa0dd0309.setContent(html_3133cc27c578b6682e23e94baaa3ba65);\\n \\n \\n\\n circle_marker_b07edb7ed756704d34f3a26ac6889f31.bindPopup(popup_64d8b32c208f0277c8e38c5fa0dd0309)\\n ;\\n\\n \\n \\n \\n var circle_marker_e2dfdf5fc704b4ec56df77ccafc8b5cf = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_606b9d10ab420f34c4a9fb3943c983bf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f569b1247649bbc458867df4bbab112c = $(`<div id="html_f569b1247649bbc458867df4bbab112c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_606b9d10ab420f34c4a9fb3943c983bf.setContent(html_f569b1247649bbc458867df4bbab112c);\\n \\n \\n\\n circle_marker_e2dfdf5fc704b4ec56df77ccafc8b5cf.bindPopup(popup_606b9d10ab420f34c4a9fb3943c983bf)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f949e0e4b755c8c933543e42e3a11c3 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5fda2c4af2c60cd85e90932e3bb3031c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c95d7a10a78135f39c237555324c3ad = $(`<div id="html_5c95d7a10a78135f39c237555324c3ad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5fda2c4af2c60cd85e90932e3bb3031c.setContent(html_5c95d7a10a78135f39c237555324c3ad);\\n \\n \\n\\n circle_marker_8f949e0e4b755c8c933543e42e3a11c3.bindPopup(popup_5fda2c4af2c60cd85e90932e3bb3031c)\\n ;\\n\\n \\n \\n \\n var circle_marker_bb72b7f294c3a7bb4c71e06d48d87d96 = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_271ec7d708072a53326fc2ed8fe571af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_531fba3b72c8bb9e206fe5142bfb1deb = $(`<div id="html_531fba3b72c8bb9e206fe5142bfb1deb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_271ec7d708072a53326fc2ed8fe571af.setContent(html_531fba3b72c8bb9e206fe5142bfb1deb);\\n \\n \\n\\n circle_marker_bb72b7f294c3a7bb4c71e06d48d87d96.bindPopup(popup_271ec7d708072a53326fc2ed8fe571af)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f9533dafff77dd0ad945c6af7ffddb6 = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0988be469641eba266777c38d86fe18a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d3ba6ce79bd62120cadc79faf5771e5 = $(`<div id="html_6d3ba6ce79bd62120cadc79faf5771e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0988be469641eba266777c38d86fe18a.setContent(html_6d3ba6ce79bd62120cadc79faf5771e5);\\n \\n \\n\\n circle_marker_9f9533dafff77dd0ad945c6af7ffddb6.bindPopup(popup_0988be469641eba266777c38d86fe18a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a428d0502949de0299cffc354ad5853d = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b4a7e9601fb5297653eaace6d990a222 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4a5f8f1a61975337c20ce3aabff8853 = $(`<div id="html_d4a5f8f1a61975337c20ce3aabff8853" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b4a7e9601fb5297653eaace6d990a222.setContent(html_d4a5f8f1a61975337c20ce3aabff8853);\\n \\n \\n\\n circle_marker_a428d0502949de0299cffc354ad5853d.bindPopup(popup_b4a7e9601fb5297653eaace6d990a222)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea7fd38ea9d499c7441b9c7da07119db = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8e44f7ea6d474908c51728e0c3133fe7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8cf799b075c1f0b6a42194a965d12f38 = $(`<div id="html_8cf799b075c1f0b6a42194a965d12f38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e44f7ea6d474908c51728e0c3133fe7.setContent(html_8cf799b075c1f0b6a42194a965d12f38);\\n \\n \\n\\n circle_marker_ea7fd38ea9d499c7441b9c7da07119db.bindPopup(popup_8e44f7ea6d474908c51728e0c3133fe7)\\n ;\\n\\n \\n \\n \\n var circle_marker_37e2bb9fbaad739730a7b76c8e515dce = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4c7963ed46467d936c9ef69231fa7f13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5054c7c0510a10f745829e42c2a80f0e = $(`<div id="html_5054c7c0510a10f745829e42c2a80f0e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c7963ed46467d936c9ef69231fa7f13.setContent(html_5054c7c0510a10f745829e42c2a80f0e);\\n \\n \\n\\n circle_marker_37e2bb9fbaad739730a7b76c8e515dce.bindPopup(popup_4c7963ed46467d936c9ef69231fa7f13)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c6a22bf071dbc796db428858ac65abe = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a3b2b2a005de5d06a924861b33f3849e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e4c4582e0ad4750734a073f6acaf7f07 = $(`<div id="html_e4c4582e0ad4750734a073f6acaf7f07" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3b2b2a005de5d06a924861b33f3849e.setContent(html_e4c4582e0ad4750734a073f6acaf7f07);\\n \\n \\n\\n circle_marker_7c6a22bf071dbc796db428858ac65abe.bindPopup(popup_a3b2b2a005de5d06a924861b33f3849e)\\n ;\\n\\n \\n \\n \\n var circle_marker_15c609457de3d1deb57a2435886a754f = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c4e87cb153effcc7de75710e4f3acbb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_118423f1cd5824fe0fa793d3b426de85 = $(`<div id="html_118423f1cd5824fe0fa793d3b426de85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4e87cb153effcc7de75710e4f3acbb8.setContent(html_118423f1cd5824fe0fa793d3b426de85);\\n \\n \\n\\n circle_marker_15c609457de3d1deb57a2435886a754f.bindPopup(popup_c4e87cb153effcc7de75710e4f3acbb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8472fbf690e9284c74d1077c9caea44 = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b6b8398b361b2760d08f9e92242e9e0f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_af414085d0f17cceb4f446767fde197f = $(`<div id="html_af414085d0f17cceb4f446767fde197f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b6b8398b361b2760d08f9e92242e9e0f.setContent(html_af414085d0f17cceb4f446767fde197f);\\n \\n \\n\\n circle_marker_f8472fbf690e9284c74d1077c9caea44.bindPopup(popup_b6b8398b361b2760d08f9e92242e9e0f)\\n ;\\n\\n \\n \\n \\n var circle_marker_3efea662cbd8482c103fb3a2aba692ea = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d6188ccd8e7a1a36e7d5c64517f60b66 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f04bb7cd4851303d5b57f205e49e686e = $(`<div id="html_f04bb7cd4851303d5b57f205e49e686e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d6188ccd8e7a1a36e7d5c64517f60b66.setContent(html_f04bb7cd4851303d5b57f205e49e686e);\\n \\n \\n\\n circle_marker_3efea662cbd8482c103fb3a2aba692ea.bindPopup(popup_d6188ccd8e7a1a36e7d5c64517f60b66)\\n ;\\n\\n \\n \\n \\n var circle_marker_c48da3c5f0c5b6859f111c22c8ef2962 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2a0b99f19f4486a57f7806ad75d08555 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7552f97531bd5cb705039a5e5e393946 = $(`<div id="html_7552f97531bd5cb705039a5e5e393946" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a0b99f19f4486a57f7806ad75d08555.setContent(html_7552f97531bd5cb705039a5e5e393946);\\n \\n \\n\\n circle_marker_c48da3c5f0c5b6859f111c22c8ef2962.bindPopup(popup_2a0b99f19f4486a57f7806ad75d08555)\\n ;\\n\\n \\n \\n \\n var circle_marker_966c7876c653f540e51d8d1bebb71fd2 = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_dd920f97b4044b87e936f053dd3f5baa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e503e92e0cff9b69b3b172cf54d053c = $(`<div id="html_7e503e92e0cff9b69b3b172cf54d053c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd920f97b4044b87e936f053dd3f5baa.setContent(html_7e503e92e0cff9b69b3b172cf54d053c);\\n \\n \\n\\n circle_marker_966c7876c653f540e51d8d1bebb71fd2.bindPopup(popup_dd920f97b4044b87e936f053dd3f5baa)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec284cba8edd9699292d467154322230 = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9f10ba266fb22750d78cf88d3b432ffb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f164217ee31cf27d260a32f13bc2857c = $(`<div id="html_f164217ee31cf27d260a32f13bc2857c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f10ba266fb22750d78cf88d3b432ffb.setContent(html_f164217ee31cf27d260a32f13bc2857c);\\n \\n \\n\\n circle_marker_ec284cba8edd9699292d467154322230.bindPopup(popup_9f10ba266fb22750d78cf88d3b432ffb)\\n ;\\n\\n \\n \\n \\n var circle_marker_f29a1cd5c055c73ecc1ca124259e8287 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d3fbd1d07dc5d21599dc55853fec0b8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0294eeeb6f047d9c85fe52c360fa59bf = $(`<div id="html_0294eeeb6f047d9c85fe52c360fa59bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d3fbd1d07dc5d21599dc55853fec0b8f.setContent(html_0294eeeb6f047d9c85fe52c360fa59bf);\\n \\n \\n\\n circle_marker_f29a1cd5c055c73ecc1ca124259e8287.bindPopup(popup_d3fbd1d07dc5d21599dc55853fec0b8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a51838800f61de41824f330dab544e4 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_fad49cdd90f43b5753d6d3a90fd9554e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de2c8c201ca4421b2317fcacf45b564d = $(`<div id="html_de2c8c201ca4421b2317fcacf45b564d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fad49cdd90f43b5753d6d3a90fd9554e.setContent(html_de2c8c201ca4421b2317fcacf45b564d);\\n \\n \\n\\n circle_marker_6a51838800f61de41824f330dab544e4.bindPopup(popup_fad49cdd90f43b5753d6d3a90fd9554e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1d32cdac4c2d68ef03cbf2a574cbab6 = L.circleMarker(\\n [42.7345450038305, -73.22673656679281],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.3336224206596095, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_14c0ae95204065d871219436e25a5268 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_189b462dc9952f30bbb350d487137c0f = $(`<div id="html_189b462dc9952f30bbb350d487137c0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14c0ae95204065d871219436e25a5268.setContent(html_189b462dc9952f30bbb350d487137c0f);\\n \\n \\n\\n circle_marker_d1d32cdac4c2d68ef03cbf2a574cbab6.bindPopup(popup_14c0ae95204065d871219436e25a5268)\\n ;\\n\\n \\n \\n \\n var circle_marker_24822e20c0d89aaeb2b1d9b766de8db1 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7c24b96e4ae14f2e9743d62d3a697748 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e92f22b3395b19b1ab0cc5edc9d7a780 = $(`<div id="html_e92f22b3395b19b1ab0cc5edc9d7a780" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c24b96e4ae14f2e9743d62d3a697748.setContent(html_e92f22b3395b19b1ab0cc5edc9d7a780);\\n \\n \\n\\n circle_marker_24822e20c0d89aaeb2b1d9b766de8db1.bindPopup(popup_7c24b96e4ae14f2e9743d62d3a697748)\\n ;\\n\\n \\n \\n \\n var circle_marker_199c3d79cf01e55d706942b6a249beee = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_36cb16a5562d34fb1a9523b24d3cecc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a582897a3d5e831cdef6f21c3cf9bb3 = $(`<div id="html_6a582897a3d5e831cdef6f21c3cf9bb3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36cb16a5562d34fb1a9523b24d3cecc1.setContent(html_6a582897a3d5e831cdef6f21c3cf9bb3);\\n \\n \\n\\n circle_marker_199c3d79cf01e55d706942b6a249beee.bindPopup(popup_36cb16a5562d34fb1a9523b24d3cecc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_fedd63d0b065a9fc3b8747653da8f3f2 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_258bba7a3a0c390a654b9fa85fac7abf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10bfe5d6d314edfbdfd9416fe703b2b8 = $(`<div id="html_10bfe5d6d314edfbdfd9416fe703b2b8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_258bba7a3a0c390a654b9fa85fac7abf.setContent(html_10bfe5d6d314edfbdfd9416fe703b2b8);\\n \\n \\n\\n circle_marker_fedd63d0b065a9fc3b8747653da8f3f2.bindPopup(popup_258bba7a3a0c390a654b9fa85fac7abf)\\n ;\\n\\n \\n \\n \\n var circle_marker_71236ef5568a3e334f2795ec019a5143 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4ac46e0a4c4995905f6dd3663eae7497 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92c0a9d85055aaf6cfbe756048d98104 = $(`<div id="html_92c0a9d85055aaf6cfbe756048d98104" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ac46e0a4c4995905f6dd3663eae7497.setContent(html_92c0a9d85055aaf6cfbe756048d98104);\\n \\n \\n\\n circle_marker_71236ef5568a3e334f2795ec019a5143.bindPopup(popup_4ac46e0a4c4995905f6dd3663eae7497)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec269ae3fc016dfe34446316480e8336 = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_08fbb4abceb6c2c5df8c09445b758032 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8909a9508ebaee3d91154f62457aba90 = $(`<div id="html_8909a9508ebaee3d91154f62457aba90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_08fbb4abceb6c2c5df8c09445b758032.setContent(html_8909a9508ebaee3d91154f62457aba90);\\n \\n \\n\\n circle_marker_ec269ae3fc016dfe34446316480e8336.bindPopup(popup_08fbb4abceb6c2c5df8c09445b758032)\\n ;\\n\\n \\n \\n \\n var circle_marker_10baa11e0c14de34343e92106f6698a3 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_fe477b341a63596224282b56d71dc8c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58e148912a435b58c405ab14a8e51b0f = $(`<div id="html_58e148912a435b58c405ab14a8e51b0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe477b341a63596224282b56d71dc8c1.setContent(html_58e148912a435b58c405ab14a8e51b0f);\\n \\n \\n\\n circle_marker_10baa11e0c14de34343e92106f6698a3.bindPopup(popup_fe477b341a63596224282b56d71dc8c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_df8b552a46897f5be2ff0bbe0c76b26d = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_09c4f31f92198211be89c297a1c65831 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a625337d0c6a64d6b934f9ef88e38ec = $(`<div id="html_7a625337d0c6a64d6b934f9ef88e38ec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09c4f31f92198211be89c297a1c65831.setContent(html_7a625337d0c6a64d6b934f9ef88e38ec);\\n \\n \\n\\n circle_marker_df8b552a46897f5be2ff0bbe0c76b26d.bindPopup(popup_09c4f31f92198211be89c297a1c65831)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b09f663183f40b90c423409068f3f96 = L.circleMarker(\\n [42.7327440918774, -73.26979237266056],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_125cb731ffabb0e7cf9fd4e7e517e929 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_263480035286df760e1fed0e9c7cc73f = $(`<div id="html_263480035286df760e1fed0e9c7cc73f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_125cb731ffabb0e7cf9fd4e7e517e929.setContent(html_263480035286df760e1fed0e9c7cc73f);\\n \\n \\n\\n circle_marker_5b09f663183f40b90c423409068f3f96.bindPopup(popup_125cb731ffabb0e7cf9fd4e7e517e929)\\n ;\\n\\n \\n \\n \\n var circle_marker_a92ad8cc5e5fa35c5237be04118da0fc = L.circleMarker(\\n [42.7327441379601, -73.26856223845904],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_43953a23a79430af5566a358b48da19a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6387d2b1acf4c3f42b8710c07df5dfa = $(`<div id="html_d6387d2b1acf4c3f42b8710c07df5dfa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43953a23a79430af5566a358b48da19a.setContent(html_d6387d2b1acf4c3f42b8710c07df5dfa);\\n \\n \\n\\n circle_marker_a92ad8cc5e5fa35c5237be04118da0fc.bindPopup(popup_43953a23a79430af5566a358b48da19a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3945cafc20126d28f654ca1a8b00a8e = L.circleMarker(\\n [42.73274417087632, -73.26733210425597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e02f9e9dc82d48167faca3f7a8919d30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1df0ac27333809a34ae96306ceba1148 = $(`<div id="html_1df0ac27333809a34ae96306ceba1148" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e02f9e9dc82d48167faca3f7a8919d30.setContent(html_1df0ac27333809a34ae96306ceba1148);\\n \\n \\n\\n circle_marker_f3945cafc20126d28f654ca1a8b00a8e.bindPopup(popup_e02f9e9dc82d48167faca3f7a8919d30)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea7279cdad4915c88836b12121b985d4 = L.circleMarker(\\n [42.73274419062606, -73.26610197005185],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2a5a5f2137bdb6c2041e0f24f76ddb4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aaceeabf2c332ade2c00476c15b66487 = $(`<div id="html_aaceeabf2c332ade2c00476c15b66487" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a5a5f2137bdb6c2041e0f24f76ddb4e.setContent(html_aaceeabf2c332ade2c00476c15b66487);\\n \\n \\n\\n circle_marker_ea7279cdad4915c88836b12121b985d4.bindPopup(popup_2a5a5f2137bdb6c2041e0f24f76ddb4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6e2210c45cc646825713f26a21e2fb3 = L.circleMarker(\\n [42.7327441972093, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_855b6eca4db0cbc19819a4120592db5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb5533dbf4ec4d5dd99cee7d6602d84b = $(`<div id="html_bb5533dbf4ec4d5dd99cee7d6602d84b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_855b6eca4db0cbc19819a4120592db5c.setContent(html_bb5533dbf4ec4d5dd99cee7d6602d84b);\\n \\n \\n\\n circle_marker_f6e2210c45cc646825713f26a21e2fb3.bindPopup(popup_855b6eca4db0cbc19819a4120592db5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_8135366100b062e39e377494016ded8f = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2e8c68d7023ac9e7a59f39d1e2be446f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e9bc97ce5a40cf3891b8a13bd366c970 = $(`<div id="html_e9bc97ce5a40cf3891b8a13bd366c970" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e8c68d7023ac9e7a59f39d1e2be446f.setContent(html_e9bc97ce5a40cf3891b8a13bd366c970);\\n \\n \\n\\n circle_marker_8135366100b062e39e377494016ded8f.bindPopup(popup_2e8c68d7023ac9e7a59f39d1e2be446f)\\n ;\\n\\n \\n \\n \\n var circle_marker_a68d3132de0fd93b450415a6fc35a1a3 = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d27c942b1946c852bb393b014a6ebf5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54c3a4316b2ba32bc4318d8e11366d44 = $(`<div id="html_54c3a4316b2ba32bc4318d8e11366d44" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d27c942b1946c852bb393b014a6ebf5e.setContent(html_54c3a4316b2ba32bc4318d8e11366d44);\\n \\n \\n\\n circle_marker_a68d3132de0fd93b450415a6fc35a1a3.bindPopup(popup_d27c942b1946c852bb393b014a6ebf5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_982dbbae32c92b4d9abf4660f5ebfb6a = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5a8646199f6a4033b237fb21cd44d7c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f550da9d50c591a57f4624c559bac91 = $(`<div id="html_3f550da9d50c591a57f4624c559bac91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a8646199f6a4033b237fb21cd44d7c6.setContent(html_3f550da9d50c591a57f4624c559bac91);\\n \\n \\n\\n circle_marker_982dbbae32c92b4d9abf4660f5ebfb6a.bindPopup(popup_5a8646199f6a4033b237fb21cd44d7c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_375b6ca843bf6cbd39f02b34843f11a1 = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9194f5f75bbb53c3bceb2d9c75186289 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_466e42557600f0aaac448b3391d773e3 = $(`<div id="html_466e42557600f0aaac448b3391d773e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9194f5f75bbb53c3bceb2d9c75186289.setContent(html_466e42557600f0aaac448b3391d773e3);\\n \\n \\n\\n circle_marker_375b6ca843bf6cbd39f02b34843f11a1.bindPopup(popup_9194f5f75bbb53c3bceb2d9c75186289)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3599def9fd9e6943da1f4fa13510f83 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_109a0cd33cadf17fa2e286e7f9774591 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_367423d848f29acac96429f97ebdbde2 = $(`<div id="html_367423d848f29acac96429f97ebdbde2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_109a0cd33cadf17fa2e286e7f9774591.setContent(html_367423d848f29acac96429f97ebdbde2);\\n \\n \\n\\n circle_marker_c3599def9fd9e6943da1f4fa13510f83.bindPopup(popup_109a0cd33cadf17fa2e286e7f9774591)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ec12283df2e75756642549dbee7a29f = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1f9bacea34ed4a78c602023ee5a4aed2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8242ca2c8d8443dc0c380915b8c8d2ab = $(`<div id="html_8242ca2c8d8443dc0c380915b8c8d2ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f9bacea34ed4a78c602023ee5a4aed2.setContent(html_8242ca2c8d8443dc0c380915b8c8d2ab);\\n \\n \\n\\n circle_marker_6ec12283df2e75756642549dbee7a29f.bindPopup(popup_1f9bacea34ed4a78c602023ee5a4aed2)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf5665edee9c1c8628394f0a3f581b69 = L.circleMarker(\\n [42.73274377588172, -73.25503076225394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2c61b635ee36fe95b09094566ae3937d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d257bc67aa13146b0502edfee7f2d85 = $(`<div id="html_3d257bc67aa13146b0502edfee7f2d85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c61b635ee36fe95b09094566ae3937d.setContent(html_3d257bc67aa13146b0502edfee7f2d85);\\n \\n \\n\\n circle_marker_cf5665edee9c1c8628394f0a3f581b69.bindPopup(popup_2c61b635ee36fe95b09094566ae3937d)\\n ;\\n\\n \\n \\n \\n var circle_marker_130d1c33d70537493aa94515c6fb5842 = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_76c2f46afd3cb53aee289b82d47d2817 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e1acabe9ffb98e4fa2f358047153fe5c = $(`<div id="html_e1acabe9ffb98e4fa2f358047153fe5c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76c2f46afd3cb53aee289b82d47d2817.setContent(html_e1acabe9ffb98e4fa2f358047153fe5c);\\n \\n \\n\\n circle_marker_130d1c33d70537493aa94515c6fb5842.bindPopup(popup_76c2f46afd3cb53aee289b82d47d2817)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c3c41d1eb4fb6594547bcc113ecc0cb = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bd62f82d579117586ecc6be14133d0fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a70becc352a9ce167f94649f4ad58544 = $(`<div id="html_a70becc352a9ce167f94649f4ad58544" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd62f82d579117586ecc6be14133d0fa.setContent(html_a70becc352a9ce167f94649f4ad58544);\\n \\n \\n\\n circle_marker_1c3c41d1eb4fb6594547bcc113ecc0cb.bindPopup(popup_bd62f82d579117586ecc6be14133d0fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a33e533ec18b7814e1e7859b2628930 = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1f609e87d65d264b1e1a2cede35b99d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9950ca8062852e34b83762b5262c39c1 = $(`<div id="html_9950ca8062852e34b83762b5262c39c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f609e87d65d264b1e1a2cede35b99d8.setContent(html_9950ca8062852e34b83762b5262c39c1);\\n \\n \\n\\n circle_marker_4a33e533ec18b7814e1e7859b2628930.bindPopup(popup_1f609e87d65d264b1e1a2cede35b99d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_d739f44a7f4a72add645c6552b7f2555 = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5a79877214fc8440efd44710b1245dee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e7920745abc616be107716f8aca0848 = $(`<div id="html_2e7920745abc616be107716f8aca0848" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a79877214fc8440efd44710b1245dee.setContent(html_2e7920745abc616be107716f8aca0848);\\n \\n \\n\\n circle_marker_d739f44a7f4a72add645c6552b7f2555.bindPopup(popup_5a79877214fc8440efd44710b1245dee)\\n ;\\n\\n \\n \\n \\n var circle_marker_80dc41703e02b51b71f4752e7b2ca4a7 = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.918490759365935, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2ef637763d48b9a3e578c3c87e652309 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6778e41a7f8573119e11dbafcf013f78 = $(`<div id="html_6778e41a7f8573119e11dbafcf013f78" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ef637763d48b9a3e578c3c87e652309.setContent(html_6778e41a7f8573119e11dbafcf013f78);\\n \\n \\n\\n circle_marker_80dc41703e02b51b71f4752e7b2ca4a7.bindPopup(popup_2ef637763d48b9a3e578c3c87e652309)\\n ;\\n\\n \\n \\n \\n var circle_marker_3484c491275fd8cf467c7b628cf3a720 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6aab442ed709715390bb7b071d81e6a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ff6023a26ab2185efe7594c7fad16f2 = $(`<div id="html_1ff6023a26ab2185efe7594c7fad16f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6aab442ed709715390bb7b071d81e6a8.setContent(html_1ff6023a26ab2185efe7594c7fad16f2);\\n \\n \\n\\n circle_marker_3484c491275fd8cf467c7b628cf3a720.bindPopup(popup_6aab442ed709715390bb7b071d81e6a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_43b0573035beb20deaaf4369112c5e56 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d09634978e68dd7b68f60ab30ecc98f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50572429c6e4691be682b399092ad41e = $(`<div id="html_50572429c6e4691be682b399092ad41e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d09634978e68dd7b68f60ab30ecc98f5.setContent(html_50572429c6e4691be682b399092ad41e);\\n \\n \\n\\n circle_marker_43b0573035beb20deaaf4369112c5e56.bindPopup(popup_d09634978e68dd7b68f60ab30ecc98f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_5996cbc053848232e6e388f45409f574 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c6aef01320ee49ffded39aca06dba582 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f807de43977e7203ceddfcb071915c09 = $(`<div id="html_f807de43977e7203ceddfcb071915c09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6aef01320ee49ffded39aca06dba582.setContent(html_f807de43977e7203ceddfcb071915c09);\\n \\n \\n\\n circle_marker_5996cbc053848232e6e388f45409f574.bindPopup(popup_c6aef01320ee49ffded39aca06dba582)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d5705dd0caa433518ed367018a6d410 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f0d8120c57aa3a4ddad9ef091d50295b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64dbb22a40aac2511b50ab68ad0d1df3 = $(`<div id="html_64dbb22a40aac2511b50ab68ad0d1df3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0d8120c57aa3a4ddad9ef091d50295b.setContent(html_64dbb22a40aac2511b50ab68ad0d1df3);\\n \\n \\n\\n circle_marker_4d5705dd0caa433518ed367018a6d410.bindPopup(popup_f0d8120c57aa3a4ddad9ef091d50295b)\\n ;\\n\\n \\n \\n \\n var circle_marker_f2a9c505c40e911d17cab91d98b7c5ec = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.259537945889915, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4f8f910bc5cfd15b6fb72911fdeb8f41 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc6dc29750943a0e478f4dda490e2eaf = $(`<div id="html_fc6dc29750943a0e478f4dda490e2eaf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f8f910bc5cfd15b6fb72911fdeb8f41.setContent(html_fc6dc29750943a0e478f4dda490e2eaf);\\n \\n \\n\\n circle_marker_f2a9c505c40e911d17cab91d98b7c5ec.bindPopup(popup_4f8f910bc5cfd15b6fb72911fdeb8f41)\\n ;\\n\\n \\n \\n \\n var circle_marker_339e8464bcbbb2482c84c1d041113ceb = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1e0a98108ba2f2af7ba48a7339bc3f78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_429145fb547afb8a3a3d9aed9e0de70f = $(`<div id="html_429145fb547afb8a3a3d9aed9e0de70f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1e0a98108ba2f2af7ba48a7339bc3f78.setContent(html_429145fb547afb8a3a3d9aed9e0de70f);\\n \\n \\n\\n circle_marker_339e8464bcbbb2482c84c1d041113ceb.bindPopup(popup_1e0a98108ba2f2af7ba48a7339bc3f78)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3041c56930af7c545bb010eaac781a0 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9e65b1f66b1f071cc7864054b103108d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_707ecf255c423bb81e358bfd25cbe087 = $(`<div id="html_707ecf255c423bb81e358bfd25cbe087" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e65b1f66b1f071cc7864054b103108d.setContent(html_707ecf255c423bb81e358bfd25cbe087);\\n \\n \\n\\n circle_marker_c3041c56930af7c545bb010eaac781a0.bindPopup(popup_9e65b1f66b1f071cc7864054b103108d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3331a3894c3f8c79c8a34f5e77f8301 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8cad93c2c2ae30f5812663a097d6d932 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a36cdb7005dc5e813df4ead8bfb79e31 = $(`<div id="html_a36cdb7005dc5e813df4ead8bfb79e31" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8cad93c2c2ae30f5812663a097d6d932.setContent(html_a36cdb7005dc5e813df4ead8bfb79e31);\\n \\n \\n\\n circle_marker_c3331a3894c3f8c79c8a34f5e77f8301.bindPopup(popup_8cad93c2c2ae30f5812663a097d6d932)\\n ;\\n\\n \\n \\n \\n var circle_marker_9254ad4e1841f871a2c2e30da528b0bb = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_dc85ea99fbc4a1aa882639f7bf13afed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a5832d79943c78ea336783885a2c453 = $(`<div id="html_3a5832d79943c78ea336783885a2c453" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc85ea99fbc4a1aa882639f7bf13afed.setContent(html_3a5832d79943c78ea336783885a2c453);\\n \\n \\n\\n circle_marker_9254ad4e1841f871a2c2e30da528b0bb.bindPopup(popup_dc85ea99fbc4a1aa882639f7bf13afed)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d49998cb7c1e03c603f73dad12d634f = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5843083b3a1e69954f96470275d9eb5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d8659dd1ecabd869aa855a3780b19dd = $(`<div id="html_6d8659dd1ecabd869aa855a3780b19dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5843083b3a1e69954f96470275d9eb5e.setContent(html_6d8659dd1ecabd869aa855a3780b19dd);\\n \\n \\n\\n circle_marker_3d49998cb7c1e03c603f73dad12d634f.bindPopup(popup_5843083b3a1e69954f96470275d9eb5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0cc4e6195eff9aabb49c2331e60b54f = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8d47b5a6402f1c5417ccd5b3807dcd68 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e0a57c50e28e9228bd51a78991a9e258 = $(`<div id="html_e0a57c50e28e9228bd51a78991a9e258" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d47b5a6402f1c5417ccd5b3807dcd68.setContent(html_e0a57c50e28e9228bd51a78991a9e258);\\n \\n \\n\\n circle_marker_e0cc4e6195eff9aabb49c2331e60b54f.bindPopup(popup_8d47b5a6402f1c5417ccd5b3807dcd68)\\n ;\\n\\n \\n \\n \\n var circle_marker_984fab35eaba1c2af1f81776a6191b25 = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_72f6c4f2c4f1cd4296c0531f768bb057 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_20a6836dc79197062ba8ef3009731cc1 = $(`<div id="html_20a6836dc79197062ba8ef3009731cc1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72f6c4f2c4f1cd4296c0531f768bb057.setContent(html_20a6836dc79197062ba8ef3009731cc1);\\n \\n \\n\\n circle_marker_984fab35eaba1c2af1f81776a6191b25.bindPopup(popup_72f6c4f2c4f1cd4296c0531f768bb057)\\n ;\\n\\n \\n \\n \\n var circle_marker_be7eab1fd54cdb73ee0c03cb7a951272 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7a2e43b8ed837ce558283635f40e0bf0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97b29e8d75e83e91f8a13d2eee0e6634 = $(`<div id="html_97b29e8d75e83e91f8a13d2eee0e6634" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a2e43b8ed837ce558283635f40e0bf0.setContent(html_97b29e8d75e83e91f8a13d2eee0e6634);\\n \\n \\n\\n circle_marker_be7eab1fd54cdb73ee0c03cb7a951272.bindPopup(popup_7a2e43b8ed837ce558283635f40e0bf0)\\n ;\\n\\n \\n \\n \\n var circle_marker_3404973a69eae99ac92e1a5ff2c5b99f = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c106bfa0fdb3a2819fcf64209e456525 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9efff003ff0d8bb16fb3c1bc0bef119 = $(`<div id="html_d9efff003ff0d8bb16fb3c1bc0bef119" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c106bfa0fdb3a2819fcf64209e456525.setContent(html_d9efff003ff0d8bb16fb3c1bc0bef119);\\n \\n \\n\\n circle_marker_3404973a69eae99ac92e1a5ff2c5b99f.bindPopup(popup_c106bfa0fdb3a2819fcf64209e456525)\\n ;\\n\\n \\n \\n \\n var circle_marker_e915b3b4a8b640ef67aeacf83ff6dd1c = L.circleMarker(\\n [42.73273469100676, -73.21812674084454],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8f9336c025654b5f0f18a81699aa8e51 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0aa78838e2c0298ebf5a028aba008348 = $(`<div id="html_0aa78838e2c0298ebf5a028aba008348" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f9336c025654b5f0f18a81699aa8e51.setContent(html_0aa78838e2c0298ebf5a028aba008348);\\n \\n \\n\\n circle_marker_e915b3b4a8b640ef67aeacf83ff6dd1c.bindPopup(popup_8f9336c025654b5f0f18a81699aa8e51)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d8ab35fe481a50b048eddd69e2d790f = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_cfaec823c2fe1299e118264957eea05b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d2c2eebb1a7369f4b7e762a871d97a6 = $(`<div id="html_0d2c2eebb1a7369f4b7e762a871d97a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfaec823c2fe1299e118264957eea05b.setContent(html_0d2c2eebb1a7369f4b7e762a871d97a6);\\n \\n \\n\\n circle_marker_3d8ab35fe481a50b048eddd69e2d790f.bindPopup(popup_cfaec823c2fe1299e118264957eea05b)\\n ;\\n\\n \\n \\n \\n var circle_marker_89a42f87ae658d0431a8509170a06e16 = L.circleMarker(\\n [42.73093703750333, -73.26733203256491],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4af93b713043afb11a690c6839986c92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38ec63825a2bb9fd4bc196957c397835 = $(`<div id="html_38ec63825a2bb9fd4bc196957c397835" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4af93b713043afb11a690c6839986c92.setContent(html_38ec63825a2bb9fd4bc196957c397835);\\n \\n \\n\\n circle_marker_89a42f87ae658d0431a8509170a06e16.bindPopup(popup_4af93b713043afb11a690c6839986c92)\\n ;\\n\\n \\n \\n \\n var circle_marker_15d208bb7820252de82c38a530481337 = L.circleMarker(\\n [42.73093705725226, -73.26610193420632],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3981fb9d6c060cc5803278d96b10f28f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c22e3777e9efbeca40cc6bcf8408eaee = $(`<div id="html_c22e3777e9efbeca40cc6bcf8408eaee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3981fb9d6c060cc5803278d96b10f28f.setContent(html_c22e3777e9efbeca40cc6bcf8408eaee);\\n \\n \\n\\n circle_marker_15d208bb7820252de82c38a530481337.bindPopup(popup_3981fb9d6c060cc5803278d96b10f28f)\\n ;\\n\\n \\n \\n \\n var circle_marker_25a96645c90bdc9ccc9d9ceea93ed338 = L.circleMarker(\\n [42.73093706383524, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4ea877a2c8340fd84b5341383f320a7f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_089985ea46bc46c9be6203834b6194a1 = $(`<div id="html_089985ea46bc46c9be6203834b6194a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ea877a2c8340fd84b5341383f320a7f.setContent(html_089985ea46bc46c9be6203834b6194a1);\\n \\n \\n\\n circle_marker_25a96645c90bdc9ccc9d9ceea93ed338.bindPopup(popup_4ea877a2c8340fd84b5341383f320a7f)\\n ;\\n\\n \\n \\n \\n var circle_marker_879a9ff90800b54aa0f9eb0be8c5d35c = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f3aaba6bd2175602f30212eef93eefc4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf71f03f2fb40d008cc72f6dbb80aa9f = $(`<div id="html_cf71f03f2fb40d008cc72f6dbb80aa9f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f3aaba6bd2175602f30212eef93eefc4.setContent(html_cf71f03f2fb40d008cc72f6dbb80aa9f);\\n \\n \\n\\n circle_marker_879a9ff90800b54aa0f9eb0be8c5d35c.bindPopup(popup_f3aaba6bd2175602f30212eef93eefc4)\\n ;\\n\\n \\n \\n \\n var circle_marker_79bcab54d377e0390fd9964bf60b6b39 = L.circleMarker(\\n [42.73093703750333, -73.26241163912951],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9e68d450875913a7e1fb6c25e101375f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97d6b94efa0a451cceff068ef75f9f9a = $(`<div id="html_97d6b94efa0a451cceff068ef75f9f9a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e68d450875913a7e1fb6c25e101375f.setContent(html_97d6b94efa0a451cceff068ef75f9f9a);\\n \\n \\n\\n circle_marker_79bcab54d377e0390fd9964bf60b6b39.bindPopup(popup_9e68d450875913a7e1fb6c25e101375f)\\n ;\\n\\n \\n \\n \\n var circle_marker_953628bbe205660ba15c9da680e6c9e2 = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_09b0b8e88a6d65f8e878cdf7907c172b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f7225b4bbc085bd2eab73820fe50b84 = $(`<div id="html_0f7225b4bbc085bd2eab73820fe50b84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09b0b8e88a6d65f8e878cdf7907c172b.setContent(html_0f7225b4bbc085bd2eab73820fe50b84);\\n \\n \\n\\n circle_marker_953628bbe205660ba15c9da680e6c9e2.bindPopup(popup_09b0b8e88a6d65f8e878cdf7907c172b)\\n ;\\n\\n \\n \\n \\n var circle_marker_959ed8a6ab955949bc586cc397d038bf = L.circleMarker(\\n [42.730936958507606, -73.259951442416],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5e6e51d8ddddff87970fc9043bbb915b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2016f47d3e72f4251e8b7ec852b98430 = $(`<div id="html_2016f47d3e72f4251e8b7ec852b98430" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e6e51d8ddddff87970fc9043bbb915b.setContent(html_2016f47d3e72f4251e8b7ec852b98430);\\n \\n \\n\\n circle_marker_959ed8a6ab955949bc586cc397d038bf.bindPopup(popup_5e6e51d8ddddff87970fc9043bbb915b)\\n ;\\n\\n \\n \\n \\n var circle_marker_12e4b4df5c0fda3a412389f8ba869443 = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c6f001230a81b40dfedd201eb1c4f096 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c605503b943172ef053a4df14e4dd7f9 = $(`<div id="html_c605503b943172ef053a4df14e4dd7f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6f001230a81b40dfedd201eb1c4f096.setContent(html_c605503b943172ef053a4df14e4dd7f9);\\n \\n \\n\\n circle_marker_12e4b4df5c0fda3a412389f8ba869443.bindPopup(popup_c6f001230a81b40dfedd201eb1c4f096)\\n ;\\n\\n \\n \\n \\n var circle_marker_580b730c5be7b4ada197bbd0a42a412b = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_97ef268396cb523ac4cd90a0e9f8dd9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74f46db81c45362218623fc866e9c513 = $(`<div id="html_74f46db81c45362218623fc866e9c513" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97ef268396cb523ac4cd90a0e9f8dd9c.setContent(html_74f46db81c45362218623fc866e9c513);\\n \\n \\n\\n circle_marker_580b730c5be7b4ada197bbd0a42a412b.bindPopup(popup_97ef268396cb523ac4cd90a0e9f8dd9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_dfcfa3c59733ba4cf14879502a22ec1a = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_dbf6dcf26181c1cd3227ae021dc8bcc9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_414cf601418f5320accb186eaca1eb09 = $(`<div id="html_414cf601418f5320accb186eaca1eb09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dbf6dcf26181c1cd3227ae021dc8bcc9.setContent(html_414cf601418f5320accb186eaca1eb09);\\n \\n \\n\\n circle_marker_dfcfa3c59733ba4cf14879502a22ec1a.bindPopup(popup_dbf6dcf26181c1cd3227ae021dc8bcc9)\\n ;\\n\\n \\n \\n \\n var circle_marker_29f8d657f13e88f89f1e52e760a87263 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_751c1489fb09251e1a0e7c53706cd367 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba4133a853b78c784f3523968efac131 = $(`<div id="html_ba4133a853b78c784f3523968efac131" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_751c1489fb09251e1a0e7c53706cd367.setContent(html_ba4133a853b78c784f3523968efac131);\\n \\n \\n\\n circle_marker_29f8d657f13e88f89f1e52e760a87263.bindPopup(popup_751c1489fb09251e1a0e7c53706cd367)\\n ;\\n\\n \\n \\n \\n var circle_marker_776d22fec622293b4c84f862bec1797a = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_eee07123d20fbd962fc81a4c59677205 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a929685ff3444a9d1c418d4108743460 = $(`<div id="html_a929685ff3444a9d1c418d4108743460" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eee07123d20fbd962fc81a4c59677205.setContent(html_a929685ff3444a9d1c418d4108743460);\\n \\n \\n\\n circle_marker_776d22fec622293b4c84f862bec1797a.bindPopup(popup_eee07123d20fbd962fc81a4c59677205)\\n ;\\n\\n \\n \\n \\n var circle_marker_e586feeb1312182eb5287dde3393898f = L.circleMarker(\\n [42.73093640553757, -73.2525708523423],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4411e44a7a779c58838a19bd6e57f46d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43307d0c533ff66209dc286debb679da = $(`<div id="html_43307d0c533ff66209dc286debb679da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4411e44a7a779c58838a19bd6e57f46d.setContent(html_43307d0c533ff66209dc286debb679da);\\n \\n \\n\\n circle_marker_e586feeb1312182eb5287dde3393898f.bindPopup(popup_4411e44a7a779c58838a19bd6e57f46d)\\n ;\\n\\n \\n \\n \\n var circle_marker_8595b1c39c5fa36d85dd08addaef5c1e = L.circleMarker(\\n [42.73093626729506, -73.25134075401192],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b56f4bf5b1bc6a7260eb1b90f16eef19 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5e0cfd92e0ed0c5e08baaf9a1bbb8f49 = $(`<div id="html_5e0cfd92e0ed0c5e08baaf9a1bbb8f49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b56f4bf5b1bc6a7260eb1b90f16eef19.setContent(html_5e0cfd92e0ed0c5e08baaf9a1bbb8f49);\\n \\n \\n\\n circle_marker_8595b1c39c5fa36d85dd08addaef5c1e.bindPopup(popup_b56f4bf5b1bc6a7260eb1b90f16eef19)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e48830a9cbfb2157b6266c7f7732ca8 = L.circleMarker(\\n [42.73093611588659, -73.2501106556873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6d0b29bfa1eae779877cf03140a70469 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_171c8cefcd9f18994d2ae47d945ae5ca = $(`<div id="html_171c8cefcd9f18994d2ae47d945ae5ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d0b29bfa1eae779877cf03140a70469.setContent(html_171c8cefcd9f18994d2ae47d945ae5ca);\\n \\n \\n\\n circle_marker_2e48830a9cbfb2157b6266c7f7732ca8.bindPopup(popup_6d0b29bfa1eae779877cf03140a70469)\\n ;\\n\\n \\n \\n \\n var circle_marker_2205e93f0d860f15b3b36f261d6b1c64 = L.circleMarker(\\n [42.73093595131219, -73.24888055736892],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0b2a7b215b6a7e11f50f0ed3ed9ff4e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec2b86e9a1c3006c2cea7dbde63b042b = $(`<div id="html_ec2b86e9a1c3006c2cea7dbde63b042b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b2a7b215b6a7e11f50f0ed3ed9ff4e9.setContent(html_ec2b86e9a1c3006c2cea7dbde63b042b);\\n \\n \\n\\n circle_marker_2205e93f0d860f15b3b36f261d6b1c64.bindPopup(popup_0b2a7b215b6a7e11f50f0ed3ed9ff4e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a773fba7eb80611f54be59996c4f455 = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d82629773a55ae79252a8323b5aeb197 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd21e81de340205900d2083d0b997a8a = $(`<div id="html_cd21e81de340205900d2083d0b997a8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d82629773a55ae79252a8323b5aeb197.setContent(html_cd21e81de340205900d2083d0b997a8a);\\n \\n \\n\\n circle_marker_6a773fba7eb80611f54be59996c4f455.bindPopup(popup_d82629773a55ae79252a8323b5aeb197)\\n ;\\n\\n \\n \\n \\n var circle_marker_ddb61cedc4e0c326bc8e0f7e9426702c = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_12ca637ecec118133c7f966b5b841ca0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c095a33277fbf058dc35e59dee1a8046 = $(`<div id="html_c095a33277fbf058dc35e59dee1a8046" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12ca637ecec118133c7f966b5b841ca0.setContent(html_c095a33277fbf058dc35e59dee1a8046);\\n \\n \\n\\n circle_marker_ddb61cedc4e0c326bc8e0f7e9426702c.bindPopup(popup_12ca637ecec118133c7f966b5b841ca0)\\n ;\\n\\n \\n \\n \\n var circle_marker_6417342513737994f6fd834b1a3f36ef = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bc12454dbeb663e62288f992e8d71af2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb427d367c9b67eed6f2ec8aeafcf9ac = $(`<div id="html_fb427d367c9b67eed6f2ec8aeafcf9ac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc12454dbeb663e62288f992e8d71af2.setContent(html_fb427d367c9b67eed6f2ec8aeafcf9ac);\\n \\n \\n\\n circle_marker_6417342513737994f6fd834b1a3f36ef.bindPopup(popup_bc12454dbeb663e62288f992e8d71af2)\\n ;\\n\\n \\n \\n \\n var circle_marker_56478e28a6148c9a094c7236d9f38108 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b5a8ea25c07abcd6ab786c7b398a94ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46f9f458ca8d7773b130947f6e90c2c4 = $(`<div id="html_46f9f458ca8d7773b130947f6e90c2c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5a8ea25c07abcd6ab786c7b398a94ab.setContent(html_46f9f458ca8d7773b130947f6e90c2c4);\\n \\n \\n\\n circle_marker_56478e28a6148c9a094c7236d9f38108.bindPopup(popup_b5a8ea25c07abcd6ab786c7b398a94ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_9109928d578b63c43783ac4e3c00d330 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_85ddbb356c0e26c29f81f2e9e68cc4c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ef10bb6e97e5365b67f4c01274f6c83 = $(`<div id="html_8ef10bb6e97e5365b67f4c01274f6c83" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_85ddbb356c0e26c29f81f2e9e68cc4c6.setContent(html_8ef10bb6e97e5365b67f4c01274f6c83);\\n \\n \\n\\n circle_marker_9109928d578b63c43783ac4e3c00d330.bindPopup(popup_85ddbb356c0e26c29f81f2e9e68cc4c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba57fee49f88e13ab37a23a5ba0fb1bd = L.circleMarker(\\n [42.730934687380696, -73.24149996761956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c628e4c4853ddb4d8633ed2435de0337 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_143fa465fe5ba6049a37984e0c4f47e4 = $(`<div id="html_143fa465fe5ba6049a37984e0c4f47e4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c628e4c4853ddb4d8633ed2435de0337.setContent(html_143fa465fe5ba6049a37984e0c4f47e4);\\n \\n \\n\\n circle_marker_ba57fee49f88e13ab37a23a5ba0fb1bd.bindPopup(popup_c628e4c4853ddb4d8633ed2435de0337)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ebbae7824f4cdd126bbdc8580ae45aa = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e6e66f7abe41e067df05c06a5b403c91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0c243d8168e99f7205fa3da6542fea2 = $(`<div id="html_c0c243d8168e99f7205fa3da6542fea2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6e66f7abe41e067df05c06a5b403c91.setContent(html_c0c243d8168e99f7205fa3da6542fea2);\\n \\n \\n\\n circle_marker_0ebbae7824f4cdd126bbdc8580ae45aa.bindPopup(popup_e6e66f7abe41e067df05c06a5b403c91)\\n ;\\n\\n \\n \\n \\n var circle_marker_aace11f1b4e9fa1bc6a8145c92469efd = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_204a2c53cbda99d96c7063d3611e3c31 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04002a8f7cfc1f63fdb702e52e3802c7 = $(`<div id="html_04002a8f7cfc1f63fdb702e52e3802c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_204a2c53cbda99d96c7063d3611e3c31.setContent(html_04002a8f7cfc1f63fdb702e52e3802c7);\\n \\n \\n\\n circle_marker_aace11f1b4e9fa1bc6a8145c92469efd.bindPopup(popup_204a2c53cbda99d96c7063d3611e3c31)\\n ;\\n\\n \\n \\n \\n var circle_marker_23a4fa889f0f3203dd69f2423ed79003 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_77d8d495bdc00854feb67fc97a349b30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67c4b22d3cd60eeed051d110d2e0e435 = $(`<div id="html_67c4b22d3cd60eeed051d110d2e0e435" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77d8d495bdc00854feb67fc97a349b30.setContent(html_67c4b22d3cd60eeed051d110d2e0e435);\\n \\n \\n\\n circle_marker_23a4fa889f0f3203dd69f2423ed79003.bindPopup(popup_77d8d495bdc00854feb67fc97a349b30)\\n ;\\n\\n \\n \\n \\n var circle_marker_87b33e7336b81d804db94d737bc59270 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_795b0291c328c9f94ef7ffddebbd9976 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eee4922a842a96d94f28d6f6037aed9c = $(`<div id="html_eee4922a842a96d94f28d6f6037aed9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_795b0291c328c9f94ef7ffddebbd9976.setContent(html_eee4922a842a96d94f28d6f6037aed9c);\\n \\n \\n\\n circle_marker_87b33e7336b81d804db94d737bc59270.bindPopup(popup_795b0291c328c9f94ef7ffddebbd9976)\\n ;\\n\\n \\n \\n \\n var circle_marker_31ffe415aa33b3362186753a68ccec09 = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7e0b49cbcb16a0a52cf7e53650703394 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d5a2b2be0d68de653bc30b06d71d632 = $(`<div id="html_8d5a2b2be0d68de653bc30b06d71d632" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e0b49cbcb16a0a52cf7e53650703394.setContent(html_8d5a2b2be0d68de653bc30b06d71d632);\\n \\n \\n\\n circle_marker_31ffe415aa33b3362186753a68ccec09.bindPopup(popup_7e0b49cbcb16a0a52cf7e53650703394)\\n ;\\n\\n \\n \\n \\n var circle_marker_e88b96e6130547a42a1c43d17586b4b3 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3ed8c8cf52600c78234170cddacf7123 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_556a8172584ade4b8110fe2ad344cb2d = $(`<div id="html_556a8172584ade4b8110fe2ad344cb2d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ed8c8cf52600c78234170cddacf7123.setContent(html_556a8172584ade4b8110fe2ad344cb2d);\\n \\n \\n\\n circle_marker_e88b96e6130547a42a1c43d17586b4b3.bindPopup(popup_3ed8c8cf52600c78234170cddacf7123)\\n ;\\n\\n \\n \\n \\n var circle_marker_21639087eed5664632115ebd47d12f60 = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e25eb919066a0346fb303af76b2b54df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0662c76ed3df2ffb27c2603e8786a3b7 = $(`<div id="html_0662c76ed3df2ffb27c2603e8786a3b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e25eb919066a0346fb303af76b2b54df.setContent(html_0662c76ed3df2ffb27c2603e8786a3b7);\\n \\n \\n\\n circle_marker_21639087eed5664632115ebd47d12f60.bindPopup(popup_e25eb919066a0346fb303af76b2b54df)\\n ;\\n\\n \\n \\n \\n var circle_marker_cac45ce8036cdb1987db2cf9465500df = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4ac25ed5b230d624a0283fff2849520a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53048558aea36a58824f6535d04d30cd = $(`<div id="html_53048558aea36a58824f6535d04d30cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ac25ed5b230d624a0283fff2849520a.setContent(html_53048558aea36a58824f6535d04d30cd);\\n \\n \\n\\n circle_marker_cac45ce8036cdb1987db2cf9465500df.bindPopup(popup_4ac25ed5b230d624a0283fff2849520a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e9b12d91a3eac79cebee8d0f2da9fb6 = L.circleMarker(\\n [42.73093113915656, -73.22796888742165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b544cdc7c094c05f3b56cf3a2e47dcba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14fed51b79d80bef1978475218eee78c = $(`<div id="html_14fed51b79d80bef1978475218eee78c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b544cdc7c094c05f3b56cf3a2e47dcba.setContent(html_14fed51b79d80bef1978475218eee78c);\\n \\n \\n\\n circle_marker_8e9b12d91a3eac79cebee8d0f2da9fb6.bindPopup(popup_b544cdc7c094c05f3b56cf3a2e47dcba)\\n ;\\n\\n \\n \\n \\n var circle_marker_c6c9ea6a118042bbe10057bf11d54f18 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2b0b9d27fb3255a1652963afb37b7096 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_08ad52b19e23261652b079cc9e497779 = $(`<div id="html_08ad52b19e23261652b079cc9e497779" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b0b9d27fb3255a1652963afb37b7096.setContent(html_08ad52b19e23261652b079cc9e497779);\\n \\n \\n\\n circle_marker_c6c9ea6a118042bbe10057bf11d54f18.bindPopup(popup_2b0b9d27fb3255a1652963afb37b7096)\\n ;\\n\\n \\n \\n \\n var circle_marker_4445a07b2b7b289f00f9ce90287f1592 = L.circleMarker(\\n [42.73093032286755, -73.22550869120535],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.406461512053774, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8b004314a82b4597cdcc8a47897655b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_51f8a9c505afaf02fd6514167b272fce = $(`<div id="html_51f8a9c505afaf02fd6514167b272fce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b004314a82b4597cdcc8a47897655b7.setContent(html_51f8a9c505afaf02fd6514167b272fce);\\n \\n \\n\\n circle_marker_4445a07b2b7b289f00f9ce90287f1592.bindPopup(popup_8b004314a82b4597cdcc8a47897655b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_2fda242b340c61399c8319834f909526 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_81edf0a350f11e7637556cb1b29171de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83aae5152a5ba193deddf567b4ff5b6a = $(`<div id="html_83aae5152a5ba193deddf567b4ff5b6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81edf0a350f11e7637556cb1b29171de.setContent(html_83aae5152a5ba193deddf567b4ff5b6a);\\n \\n \\n\\n circle_marker_2fda242b340c61399c8319834f909526.bindPopup(popup_81edf0a350f11e7637556cb1b29171de)\\n ;\\n\\n \\n \\n \\n var circle_marker_54ab77ca6d9b93df4d2c01de64fefd58 = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5621e4fa7ff3c1c098d10698bc5ba9a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6836fa15be59df8acf7d6fc1d9c894ea = $(`<div id="html_6836fa15be59df8acf7d6fc1d9c894ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5621e4fa7ff3c1c098d10698bc5ba9a8.setContent(html_6836fa15be59df8acf7d6fc1d9c894ea);\\n \\n \\n\\n circle_marker_54ab77ca6d9b93df4d2c01de64fefd58.bindPopup(popup_5621e4fa7ff3c1c098d10698bc5ba9a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec4bf8ed7b97ec083ae2d1419be9de17 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_78e93af79fac212390d973a7206f669e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f71e144161ea04fa69d62184a18ba3e7 = $(`<div id="html_f71e144161ea04fa69d62184a18ba3e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_78e93af79fac212390d973a7206f669e.setContent(html_f71e144161ea04fa69d62184a18ba3e7);\\n \\n \\n\\n circle_marker_ec4bf8ed7b97ec083ae2d1419be9de17.bindPopup(popup_78e93af79fac212390d973a7206f669e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca7771811f54d5fe5016bb8be89c40d8 = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_cd014f94224f6dc81f3db59bc2600221 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43abcd45e0a16250660c9689a9ff21f0 = $(`<div id="html_43abcd45e0a16250660c9689a9ff21f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd014f94224f6dc81f3db59bc2600221.setContent(html_43abcd45e0a16250660c9689a9ff21f0);\\n \\n \\n\\n circle_marker_ca7771811f54d5fe5016bb8be89c40d8.bindPopup(popup_cd014f94224f6dc81f3db59bc2600221)\\n ;\\n\\n \\n \\n \\n var circle_marker_bfb2359c19dd94b10b847d32dc119bbc = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_49b740fa480b641260f5f6709a4eccb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eae1f8caff6885fed4d927dabc8a4d34 = $(`<div id="html_eae1f8caff6885fed4d927dabc8a4d34" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_49b740fa480b641260f5f6709a4eccb8.setContent(html_eae1f8caff6885fed4d927dabc8a4d34);\\n \\n \\n\\n circle_marker_bfb2359c19dd94b10b847d32dc119bbc.bindPopup(popup_49b740fa480b641260f5f6709a4eccb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_008d1e20a52a906da26d61b425e112e9 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_01f887dbcf8eb634c605780e7e475e48 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31333bdaf624173da3d8ccbf3556afb2 = $(`<div id="html_31333bdaf624173da3d8ccbf3556afb2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_01f887dbcf8eb634c605780e7e475e48.setContent(html_31333bdaf624173da3d8ccbf3556afb2);\\n \\n \\n\\n circle_marker_008d1e20a52a906da26d61b425e112e9.bindPopup(popup_01f887dbcf8eb634c605780e7e475e48)\\n ;\\n\\n \\n \\n \\n var circle_marker_cbdf91269c52f7225d181eb2a2148077 = L.circleMarker(\\n [42.72912992387847, -73.26610189836369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_00e874398cccda9be2c560df47f47b9b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6103aee7745155def00afa741933babd = $(`<div id="html_6103aee7745155def00afa741933babd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00e874398cccda9be2c560df47f47b9b.setContent(html_6103aee7745155def00afa741933babd);\\n \\n \\n\\n circle_marker_cbdf91269c52f7225d181eb2a2148077.bindPopup(popup_00e874398cccda9be2c560df47f47b9b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ae04796596243c22eb2c494e38e9be5 = L.circleMarker(\\n [42.72912993046118, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2f8d5ce5625fa1ece2b973d09eb88f03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_247b48bcf463348c362c0316c83e270d = $(`<div id="html_247b48bcf463348c362c0316c83e270d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f8d5ce5625fa1ece2b973d09eb88f03.setContent(html_247b48bcf463348c362c0316c83e270d);\\n \\n \\n\\n circle_marker_9ae04796596243c22eb2c494e38e9be5.bindPopup(popup_2f8d5ce5625fa1ece2b973d09eb88f03)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8bb55008de5f463a662f0de80c9d6ba = L.circleMarker(\\n [42.72912992387847, -73.26364177333073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c945cd74c1ef839073a8c6f21e458c3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea2fb3bbd115849463c294d39ee86d86 = $(`<div id="html_ea2fb3bbd115849463c294d39ee86d86" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c945cd74c1ef839073a8c6f21e458c3f.setContent(html_ea2fb3bbd115849463c294d39ee86d86);\\n \\n \\n\\n circle_marker_a8bb55008de5f463a662f0de80c9d6ba.bindPopup(popup_c945cd74c1ef839073a8c6f21e458c3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f23075b161c3cb67b4bed8182241a93e = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c62cd27ee0cd461b6af95b0468895f57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_748c70967123a770cfb02d1f857dbaef = $(`<div id="html_748c70967123a770cfb02d1f857dbaef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c62cd27ee0cd461b6af95b0468895f57.setContent(html_748c70967123a770cfb02d1f857dbaef);\\n \\n \\n\\n circle_marker_f23075b161c3cb67b4bed8182241a93e.bindPopup(popup_c62cd27ee0cd461b6af95b0468895f57)\\n ;\\n\\n \\n \\n \\n var circle_marker_27b0eee984e28fdc0597cae5808cd01b = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f1a807d137f4b69cfc43b179ef251f67 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3856acedbcec32edcfe775f9dbf7dbd2 = $(`<div id="html_3856acedbcec32edcfe775f9dbf7dbd2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1a807d137f4b69cfc43b179ef251f67.setContent(html_3856acedbcec32edcfe775f9dbf7dbd2);\\n \\n \\n\\n circle_marker_27b0eee984e28fdc0597cae5808cd01b.bindPopup(popup_f1a807d137f4b69cfc43b179ef251f67)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0bfe8a913f791afa649009bf3a56467 = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1a9dadb20f1391f4ac9233afd81624c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c2051da83e74a1011eb606b7da42e5c = $(`<div id="html_2c2051da83e74a1011eb606b7da42e5c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a9dadb20f1391f4ac9233afd81624c6.setContent(html_2c2051da83e74a1011eb606b7da42e5c);\\n \\n \\n\\n circle_marker_c0bfe8a913f791afa649009bf3a56467.bindPopup(popup_1a9dadb20f1391f4ac9233afd81624c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_c84e4dfb28101aecf7831c06835fc090 = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a039d03de9976c25438e347a47965bd7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4877b1faf68d33ee41b4be9e3f0de04 = $(`<div id="html_a4877b1faf68d33ee41b4be9e3f0de04" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a039d03de9976c25438e347a47965bd7.setContent(html_a4877b1faf68d33ee41b4be9e3f0de04);\\n \\n \\n\\n circle_marker_c84e4dfb28101aecf7831c06835fc090.bindPopup(popup_a039d03de9976c25438e347a47965bd7)\\n ;\\n\\n \\n \\n \\n var circle_marker_4c8d5a71c445d36880a6c6b88b2c9c79 = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d991c31856e60aca3ca6144ed282e162 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec3c2bc3461e31c7b1af6f0f6a473c65 = $(`<div id="html_ec3c2bc3461e31c7b1af6f0f6a473c65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d991c31856e60aca3ca6144ed282e162.setContent(html_ec3c2bc3461e31c7b1af6f0f6a473c65);\\n \\n \\n\\n circle_marker_4c8d5a71c445d36880a6c6b88b2c9c79.bindPopup(popup_d991c31856e60aca3ca6144ed282e162)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a13e62c80c4bce83dd6b1e3e7df6f81 = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d61bd2d3e8aa02550a8a946ce3b8d7f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d97e4262ee1d464b4c3fea138056fa69 = $(`<div id="html_d97e4262ee1d464b4c3fea138056fa69" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d61bd2d3e8aa02550a8a946ce3b8d7f9.setContent(html_d97e4262ee1d464b4c3fea138056fa69);\\n \\n \\n\\n circle_marker_3a13e62c80c4bce83dd6b1e3e7df6f81.bindPopup(popup_d61bd2d3e8aa02550a8a946ce3b8d7f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6c0d6538068948b851b5406c469b190 = L.circleMarker(\\n [42.72912939726166, -73.25380127326159],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_44a28d8af0c2b82d069ab9e99e835d15 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50dbd485551d45f007f4a61763c8200d = $(`<div id="html_50dbd485551d45f007f4a61763c8200d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_44a28d8af0c2b82d069ab9e99e835d15.setContent(html_50dbd485551d45f007f4a61763c8200d);\\n \\n \\n\\n circle_marker_b6c0d6538068948b851b5406c469b190.bindPopup(popup_44a28d8af0c2b82d069ab9e99e835d15)\\n ;\\n\\n \\n \\n \\n var circle_marker_6431096bece67bbbe3989ff5d3032236 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b3d6c6b9e71489147578b3218dc45091 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba939e21a3733411064a4b4d1209fba0 = $(`<div id="html_ba939e21a3733411064a4b4d1209fba0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3d6c6b9e71489147578b3218dc45091.setContent(html_ba939e21a3733411064a4b4d1209fba0);\\n \\n \\n\\n circle_marker_6431096bece67bbbe3989ff5d3032236.bindPopup(popup_b3d6c6b9e71489147578b3218dc45091)\\n ;\\n\\n \\n \\n \\n var circle_marker_a284e461c4765c697f0c4f2158b9fb99 = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_03c46bb2177017536cb6d63d62347010 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2ac996efa2a67bb80f3b316cf46d023 = $(`<div id="html_d2ac996efa2a67bb80f3b316cf46d023" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03c46bb2177017536cb6d63d62347010.setContent(html_d2ac996efa2a67bb80f3b316cf46d023);\\n \\n \\n\\n circle_marker_a284e461c4765c697f0c4f2158b9fb99.bindPopup(popup_03c46bb2177017536cb6d63d62347010)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b75326cd889bfc6c78105741faa94bb = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d7a0f8cbc76521a3e537701d576bf50e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff1cc3131282109a2b22d89574688014 = $(`<div id="html_ff1cc3131282109a2b22d89574688014" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d7a0f8cbc76521a3e537701d576bf50e.setContent(html_ff1cc3131282109a2b22d89574688014);\\n \\n \\n\\n circle_marker_9b75326cd889bfc6c78105741faa94bb.bindPopup(popup_d7a0f8cbc76521a3e537701d576bf50e)\\n ;\\n\\n \\n \\n \\n var circle_marker_4764fc217f4bbf1af8f4306b3910527d = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bbfb2c589d0b917fdcaeb6c4c0694f50 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_722945a65c57af888209dae89395c453 = $(`<div id="html_722945a65c57af888209dae89395c453" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bbfb2c589d0b917fdcaeb6c4c0694f50.setContent(html_722945a65c57af888209dae89395c453);\\n \\n \\n\\n circle_marker_4764fc217f4bbf1af8f4306b3910527d.bindPopup(popup_bbfb2c589d0b917fdcaeb6c4c0694f50)\\n ;\\n\\n \\n \\n \\n var circle_marker_858b333a203da2add483614070bf2bf6 = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ce1ce008c1fc652790f3016080bee4fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aff56ef3b052590ef2b421908a4747f8 = $(`<div id="html_aff56ef3b052590ef2b421908a4747f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce1ce008c1fc652790f3016080bee4fd.setContent(html_aff56ef3b052590ef2b421908a4747f8);\\n \\n \\n\\n circle_marker_858b333a203da2add483614070bf2bf6.bindPopup(popup_ce1ce008c1fc652790f3016080bee4fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_ed3de1f39d4d4d679c49debfba79e1ec = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d85dfcf4b1b1c61afb4066e7ce35bc5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_141fec8e2badddf070e88711f1820f56 = $(`<div id="html_141fec8e2badddf070e88711f1820f56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d85dfcf4b1b1c61afb4066e7ce35bc5c.setContent(html_141fec8e2badddf070e88711f1820f56);\\n \\n \\n\\n circle_marker_ed3de1f39d4d4d679c49debfba79e1ec.bindPopup(popup_d85dfcf4b1b1c61afb4066e7ce35bc5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_955b2e30dd00f61bfa1d922ac252ee5b = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1b116c2f2e8fe5f95320dd9c21dd051f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1c1d314742d92dc516e33b2e442f6f5 = $(`<div id="html_f1c1d314742d92dc516e33b2e442f6f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b116c2f2e8fe5f95320dd9c21dd051f.setContent(html_f1c1d314742d92dc516e33b2e442f6f5);\\n \\n \\n\\n circle_marker_955b2e30dd00f61bfa1d922ac252ee5b.bindPopup(popup_1b116c2f2e8fe5f95320dd9c21dd051f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7fcc9bc2bc145e85809c1765df7ab39 = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_98c6192159bb0ad44fb65e859c4a3d89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17c09e0e62e1261ee69b7300ff917231 = $(`<div id="html_17c09e0e62e1261ee69b7300ff917231" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98c6192159bb0ad44fb65e859c4a3d89.setContent(html_17c09e0e62e1261ee69b7300ff917231);\\n \\n \\n\\n circle_marker_e7fcc9bc2bc145e85809c1765df7ab39.bindPopup(popup_98c6192159bb0ad44fb65e859c4a3d89)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e7b146c011739cc3c3d79eaa2f741cc = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_29b064f7808fbdbb5e772e91b3bf10e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_797ed756e9f1ebad4cf2c1176c9293b8 = $(`<div id="html_797ed756e9f1ebad4cf2c1176c9293b8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_29b064f7808fbdbb5e772e91b3bf10e4.setContent(html_797ed756e9f1ebad4cf2c1176c9293b8);\\n \\n \\n\\n circle_marker_2e7b146c011739cc3c3d79eaa2f741cc.bindPopup(popup_29b064f7808fbdbb5e772e91b3bf10e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_cd8a9b04b2900b7db89c41aaf9af988b = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a9ef07270ecbfd638b02991f9cf825c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c5bb0988d115ebd05b362b09f4b8d7f = $(`<div id="html_5c5bb0988d115ebd05b362b09f4b8d7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9ef07270ecbfd638b02991f9cf825c2.setContent(html_5c5bb0988d115ebd05b362b09f4b8d7f);\\n \\n \\n\\n circle_marker_cd8a9b04b2900b7db89c41aaf9af988b.bindPopup(popup_a9ef07270ecbfd638b02991f9cf825c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_b816cd6a558ba4876ae38634bc62dee7 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_8b18ca5ff2723ab459f638fc2e6c5264 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83998f15437b818071f37e7a0705c35d = $(`<div id="html_83998f15437b818071f37e7a0705c35d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b18ca5ff2723ab459f638fc2e6c5264.setContent(html_83998f15437b818071f37e7a0705c35d);\\n \\n \\n\\n circle_marker_b816cd6a558ba4876ae38634bc62dee7.bindPopup(popup_8b18ca5ff2723ab459f638fc2e6c5264)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3f616e4b9d152b08098f179c63916d7 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d84895220b77ada785d50d1bdb4c5bee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc8df319de148cb578fc3a849bcc2089 = $(`<div id="html_cc8df319de148cb578fc3a849bcc2089" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d84895220b77ada785d50d1bdb4c5bee.setContent(html_cc8df319de148cb578fc3a849bcc2089);\\n \\n \\n\\n circle_marker_e3f616e4b9d152b08098f179c63916d7.bindPopup(popup_d84895220b77ada785d50d1bdb4c5bee)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d5d136e702e3632efbefea9d658af30 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f60dd7be99faad685f30efefee8afdeb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f75a2031a03a5f73155695835770b472 = $(`<div id="html_f75a2031a03a5f73155695835770b472" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f60dd7be99faad685f30efefee8afdeb.setContent(html_f75a2031a03a5f73155695835770b472);\\n \\n \\n\\n circle_marker_6d5d136e702e3632efbefea9d658af30.bindPopup(popup_f60dd7be99faad685f30efefee8afdeb)\\n ;\\n\\n \\n \\n \\n var circle_marker_7badd8ff953e1511a9b5b9a251375ca1 = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_899e4195d7afc5d667239b669a376ea2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d71bec9782f3bb57837f088accee47a = $(`<div id="html_4d71bec9782f3bb57837f088accee47a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_899e4195d7afc5d667239b669a376ea2.setContent(html_4d71bec9782f3bb57837f088accee47a);\\n \\n \\n\\n circle_marker_7badd8ff953e1511a9b5b9a251375ca1.bindPopup(popup_899e4195d7afc5d667239b669a376ea2)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c738cee77bc1c4187966a763f827d40 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ee9a0a08f93521c90df016a2561d3479 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2225c1f4b8f533e2fa97b1a62432f51 = $(`<div id="html_e2225c1f4b8f533e2fa97b1a62432f51" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee9a0a08f93521c90df016a2561d3479.setContent(html_e2225c1f4b8f533e2fa97b1a62432f51);\\n \\n \\n\\n circle_marker_7c738cee77bc1c4187966a763f827d40.bindPopup(popup_ee9a0a08f93521c90df016a2561d3479)\\n ;\\n\\n \\n \\n \\n var circle_marker_41e8051afa5aa61f9c2af1af9798df38 = L.circleMarker(\\n [42.7291258162676, -73.23412027429306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0e1353e2a42fbb60079509014276467c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_579ae6072867046365d2cf3798b40531 = $(`<div id="html_579ae6072867046365d2cf3798b40531" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e1353e2a42fbb60079509014276467c.setContent(html_579ae6072867046365d2cf3798b40531);\\n \\n \\n\\n circle_marker_41e8051afa5aa61f9c2af1af9798df38.bindPopup(popup_0e1353e2a42fbb60079509014276467c)\\n ;\\n\\n \\n \\n \\n var circle_marker_c10f66f8ff232d38f19db5e64076ba9a = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c03fcfb6d0b550869393f69d91d78c47 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ebd589bcc4cc3e85a859519695a7b1f = $(`<div id="html_0ebd589bcc4cc3e85a859519695a7b1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c03fcfb6d0b550869393f69d91d78c47.setContent(html_0ebd589bcc4cc3e85a859519695a7b1f);\\n \\n \\n\\n circle_marker_c10f66f8ff232d38f19db5e64076ba9a.bindPopup(popup_c03fcfb6d0b550869393f69d91d78c47)\\n ;\\n\\n \\n \\n \\n var circle_marker_89afc8e674be9a20d9b87b03d4dbffff = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b3c4399f78ac2643232635d54f92c68f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a90a9b1785d2e0cdc4940d70684c5af = $(`<div id="html_8a90a9b1785d2e0cdc4940d70684c5af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3c4399f78ac2643232635d54f92c68f.setContent(html_8a90a9b1785d2e0cdc4940d70684c5af);\\n \\n \\n\\n circle_marker_89afc8e674be9a20d9b87b03d4dbffff.bindPopup(popup_b3c4399f78ac2643232635d54f92c68f)\\n ;\\n\\n \\n \\n \\n var circle_marker_7242c603b1ff813d94b79c64d12b17be = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6a95fbbf35d7d8aa3ebd017e1c573182 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_16c6b044d7de8c4d956cf845a48f8731 = $(`<div id="html_16c6b044d7de8c4d956cf845a48f8731" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a95fbbf35d7d8aa3ebd017e1c573182.setContent(html_16c6b044d7de8c4d956cf845a48f8731);\\n \\n \\n\\n circle_marker_7242c603b1ff813d94b79c64d12b17be.bindPopup(popup_6a95fbbf35d7d8aa3ebd017e1c573182)\\n ;\\n\\n \\n \\n \\n var circle_marker_4f16b51505da3ab074e5b47b110ecda1 = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e6bd76a3efd70e974e5ca6f4a440132d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0edcbffa94bca1f1ef1c3601869be58 = $(`<div id="html_c0edcbffa94bca1f1ef1c3601869be58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6bd76a3efd70e974e5ca6f4a440132d.setContent(html_c0edcbffa94bca1f1ef1c3601869be58);\\n \\n \\n\\n circle_marker_4f16b51505da3ab074e5b47b110ecda1.bindPopup(popup_e6bd76a3efd70e974e5ca6f4a440132d)\\n ;\\n\\n \\n \\n \\n var circle_marker_df688419a5291ac84f42f53d5ad28e59 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_68dff9e8a723cdf2a970be5ddf8dd96e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b3a6ad4c2ff4c242d789c70bc98824a6 = $(`<div id="html_b3a6ad4c2ff4c242d789c70bc98824a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68dff9e8a723cdf2a970be5ddf8dd96e.setContent(html_b3a6ad4c2ff4c242d789c70bc98824a6);\\n \\n \\n\\n circle_marker_df688419a5291ac84f42f53d5ad28e59.bindPopup(popup_68dff9e8a723cdf2a970be5ddf8dd96e)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a6a887400a80d29d2c2e5bd07df6ea1 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bc2994c1978d6a05d734301022ddfa05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8dd48304092f956ea12e6c82e8a37136 = $(`<div id="html_8dd48304092f956ea12e6c82e8a37136" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc2994c1978d6a05d734301022ddfa05.setContent(html_8dd48304092f956ea12e6c82e8a37136);\\n \\n \\n\\n circle_marker_8a6a887400a80d29d2c2e5bd07df6ea1.bindPopup(popup_bc2994c1978d6a05d734301022ddfa05)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea1edc98a0c5c33563dec2119ff5c522 = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0a553b1532c97401948cc9197cb19191 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9f8389fd3eefa6482e7de7edd41ae8d1 = $(`<div id="html_9f8389fd3eefa6482e7de7edd41ae8d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a553b1532c97401948cc9197cb19191.setContent(html_9f8389fd3eefa6482e7de7edd41ae8d1);\\n \\n \\n\\n circle_marker_ea1edc98a0c5c33563dec2119ff5c522.bindPopup(popup_0a553b1532c97401948cc9197cb19191)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd462628ca0fc36afe81135f93291a4f = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9fc40b42c93e5e15abbf8db0e13c8f2f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_584f254aef0ac9692def5a93f67f3444 = $(`<div id="html_584f254aef0ac9692def5a93f67f3444" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fc40b42c93e5e15abbf8db0e13c8f2f.setContent(html_584f254aef0ac9692def5a93f67f3444);\\n \\n \\n\\n circle_marker_dd462628ca0fc36afe81135f93291a4f.bindPopup(popup_9fc40b42c93e5e15abbf8db0e13c8f2f)\\n ;\\n\\n \\n \\n \\n var circle_marker_8227736f94c538b91d239575adc0646b = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f2bbdd93ebfd15e60620f76fa5177e03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac093297a96369bc0211d00c87657ad6 = $(`<div id="html_ac093297a96369bc0211d00c87657ad6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2bbdd93ebfd15e60620f76fa5177e03.setContent(html_ac093297a96369bc0211d00c87657ad6);\\n \\n \\n\\n circle_marker_8227736f94c538b91d239575adc0646b.bindPopup(popup_f2bbdd93ebfd15e60620f76fa5177e03)\\n ;\\n\\n \\n \\n \\n var circle_marker_fccf61fb3d9879bcba649ccc27be5f44 = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_08ff28ec57f8546acea924d2df575a3a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_226d42d1f2344caf324d4889c00df94a = $(`<div id="html_226d42d1f2344caf324d4889c00df94a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_08ff28ec57f8546acea924d2df575a3a.setContent(html_226d42d1f2344caf324d4889c00df94a);\\n \\n \\n\\n circle_marker_fccf61fb3d9879bcba649ccc27be5f44.bindPopup(popup_08ff28ec57f8546acea924d2df575a3a)\\n ;\\n\\n \\n \\n \\n var circle_marker_bd30ca655e969f2d40fdc7cabc0cefc6 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_42b76004d595453f0b19d4be4687b180 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_712d189dd42d0a19ec9f88ea8999ec03 = $(`<div id="html_712d189dd42d0a19ec9f88ea8999ec03" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_42b76004d595453f0b19d4be4687b180.setContent(html_712d189dd42d0a19ec9f88ea8999ec03);\\n \\n \\n\\n circle_marker_bd30ca655e969f2d40fdc7cabc0cefc6.bindPopup(popup_42b76004d595453f0b19d4be4687b180)\\n ;\\n\\n \\n \\n \\n var circle_marker_18a2bf8ca6f415238016be189010b790 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0e70c2d1af66238c0242de375d1c076e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ade03dbaa181e96c158f10fa78361ce = $(`<div id="html_0ade03dbaa181e96c158f10fa78361ce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e70c2d1af66238c0242de375d1c076e.setContent(html_0ade03dbaa181e96c158f10fa78361ce);\\n \\n \\n\\n circle_marker_18a2bf8ca6f415238016be189010b790.bindPopup(popup_0e70c2d1af66238c0242de375d1c076e)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca6d13bf999d11fe4c3c992612f8878f = L.circleMarker(\\n [42.72732247454739, -73.27348202255521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a06565758cae40dc9ac72ac26b558b58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd93e7ec1dc5dc5e011d1b57a75bb122 = $(`<div id="html_cd93e7ec1dc5dc5e011d1b57a75bb122" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a06565758cae40dc9ac72ac26b558b58.setContent(html_cd93e7ec1dc5dc5e011d1b57a75bb122);\\n \\n \\n\\n circle_marker_ca6d13bf999d11fe4c3c992612f8878f.bindPopup(popup_a06565758cae40dc9ac72ac26b558b58)\\n ;\\n\\n \\n \\n \\n var circle_marker_4bd6d47129f5bff07b8e267980f78638 = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_07e5c6c8346e905712fd907df2fd4cdd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e33c86da7912e238b06a08670b7dd492 = $(`<div id="html_e33c86da7912e238b06a08670b7dd492" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07e5c6c8346e905712fd907df2fd4cdd.setContent(html_e33c86da7912e238b06a08670b7dd492);\\n \\n \\n\\n circle_marker_4bd6d47129f5bff07b8e267980f78638.bindPopup(popup_07e5c6c8346e905712fd907df2fd4cdd)\\n ;\\n\\n \\n \\n \\n var circle_marker_082a5c68a79aed37bf9957bef3d41e1b = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d8b3e32caab3b93ab798b37e4c4a75e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f20d7adeeb5972468e0ca3b3ecc71820 = $(`<div id="html_f20d7adeeb5972468e0ca3b3ecc71820" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8b3e32caab3b93ab798b37e4c4a75e7.setContent(html_f20d7adeeb5972468e0ca3b3ecc71820);\\n \\n \\n\\n circle_marker_082a5c68a79aed37bf9957bef3d41e1b.bindPopup(popup_d8b3e32caab3b93ab798b37e4c4a75e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_d14d8db447ddf7c502aa8b5c320789cd = L.circleMarker(\\n [42.72732269176802, -73.26979194254899],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_319c1a8fe25c25b888ad79da1d9dfd3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f22456b73c457a4fb954d37ef254a997 = $(`<div id="html_f22456b73c457a4fb954d37ef254a997" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_319c1a8fe25c25b888ad79da1d9dfd3f.setContent(html_f22456b73c457a4fb954d37ef254a997);\\n \\n \\n\\n circle_marker_d14d8db447ddf7c502aa8b5c320789cd.bindPopup(popup_319c1a8fe25c25b888ad79da1d9dfd3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0763e855562d008b1cb374ed8d70e3a = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_bc3088819d360a74509bbdb7e7b4ce3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9243263ef37188925c933ce761750ea1 = $(`<div id="html_9243263ef37188925c933ce761750ea1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc3088819d360a74509bbdb7e7b4ce3f.setContent(html_9243263ef37188925c933ce761750ea1);\\n \\n \\n\\n circle_marker_e0763e855562d008b1cb374ed8d70e3a.bindPopup(popup_bc3088819d360a74509bbdb7e7b4ce3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1797601449beb48c22f528be21aed3ad = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ec88543f41655b5b907b624f618f8de8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04a9c9819a88cf4102869a34a0e285bc = $(`<div id="html_04a9c9819a88cf4102869a34a0e285bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec88543f41655b5b907b624f618f8de8.setContent(html_04a9c9819a88cf4102869a34a0e285bc);\\n \\n \\n\\n circle_marker_1797601449beb48c22f528be21aed3ad.bindPopup(popup_ec88543f41655b5b907b624f618f8de8)\\n ;\\n\\n \\n \\n \\n var circle_marker_03d589215bcb0c069de5cda4749c4521 = L.circleMarker(\\n [42.727322797087126, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1cdd701b2b99ff56ca6b6e9435e9c656 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae2248cd43168456fcd0ba220c34ffa5 = $(`<div id="html_ae2248cd43168456fcd0ba220c34ffa5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1cdd701b2b99ff56ca6b6e9435e9c656.setContent(html_ae2248cd43168456fcd0ba220c34ffa5);\\n \\n \\n\\n circle_marker_03d589215bcb0c069de5cda4749c4521.bindPopup(popup_1cdd701b2b99ff56ca6b6e9435e9c656)\\n ;\\n\\n \\n \\n \\n var circle_marker_57cf42df24738c29e19c4f74edafd0b0 = L.circleMarker(\\n [42.72732279050467, -73.26364180917045],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_65aedbe192032dc8e3985eb5dd404435 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8edb74807576d58f3b4cbd3e941427d2 = $(`<div id="html_8edb74807576d58f3b4cbd3e941427d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65aedbe192032dc8e3985eb5dd404435.setContent(html_8edb74807576d58f3b4cbd3e941427d2);\\n \\n \\n\\n circle_marker_57cf42df24738c29e19c4f74edafd0b0.bindPopup(popup_65aedbe192032dc8e3985eb5dd404435)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d563d2aac6ff26d98ea547f54d70b88 = L.circleMarker(\\n [42.72732269176802, -73.25995172914543],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ceb15d316c372fdada5619c1cb7e3247 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e195be8846d1ee1000e199cddefefdf0 = $(`<div id="html_e195be8846d1ee1000e199cddefefdf0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ceb15d316c372fdada5619c1cb7e3247.setContent(html_e195be8846d1ee1000e199cddefefdf0);\\n \\n \\n\\n circle_marker_7d563d2aac6ff26d98ea547f54d70b88.bindPopup(popup_ceb15d316c372fdada5619c1cb7e3247)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4418f0136806e01530ba9071f8f5a25 = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2d4b3d686bceb521a5a32f84b6ba49c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ee5456d34a929b04fa9543796727c93d = $(`<div id="html_ee5456d34a929b04fa9543796727c93d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d4b3d686bceb521a5a32f84b6ba49c4.setContent(html_ee5456d34a929b04fa9543796727c93d);\\n \\n \\n\\n circle_marker_f4418f0136806e01530ba9071f8f5a25.bindPopup(popup_2d4b3d686bceb521a5a32f84b6ba49c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a6c0b06de1c5f07f49a05af940cb08f = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f0793206c744010236eaf62d414d336f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a7ef91e4a75452452cbe7f4135dceac = $(`<div id="html_3a7ef91e4a75452452cbe7f4135dceac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0793206c744010236eaf62d414d336f.setContent(html_3a7ef91e4a75452452cbe7f4135dceac);\\n \\n \\n\\n circle_marker_1a6c0b06de1c5f07f49a05af940cb08f.bindPopup(popup_f0793206c744010236eaf62d414d336f)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d607483e9be944f008d26043e1ba70c = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6ce38f0b1764731ad5b1c6cdeabc0dba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4653cda52ec8e51a6116662610cbe945 = $(`<div id="html_4653cda52ec8e51a6116662610cbe945" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ce38f0b1764731ad5b1c6cdeabc0dba.setContent(html_4653cda52ec8e51a6116662610cbe945);\\n \\n \\n\\n circle_marker_0d607483e9be944f008d26043e1ba70c.bindPopup(popup_6ce38f0b1764731ad5b1c6cdeabc0dba)\\n ;\\n\\n \\n \\n \\n var circle_marker_6624b7163cda64ba99352321000a06cd = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c0a2df3a56a126489c82b111a7e339f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b79307e4647bd76fceed8013bec23153 = $(`<div id="html_b79307e4647bd76fceed8013bec23153" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0a2df3a56a126489c82b111a7e339f6.setContent(html_b79307e4647bd76fceed8013bec23153);\\n \\n \\n\\n circle_marker_6624b7163cda64ba99352321000a06cd.bindPopup(popup_c0a2df3a56a126489c82b111a7e339f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_95b6639dbdbfec7091e7db1738a320ca = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e8b6e85c39c5cba69b81ccdb89569d77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_747b09ab45e9135113ceb70d6d235a74 = $(`<div id="html_747b09ab45e9135113ceb70d6d235a74" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e8b6e85c39c5cba69b81ccdb89569d77.setContent(html_747b09ab45e9135113ceb70d6d235a74);\\n \\n \\n\\n circle_marker_95b6639dbdbfec7091e7db1738a320ca.bindPopup(popup_e8b6e85c39c5cba69b81ccdb89569d77)\\n ;\\n\\n \\n \\n \\n var circle_marker_0acdbedeafd8d461fd00ad61a67894c2 = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d1a71383a529cb3a56ec7c2ea677d819 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_881d51ba6a8d97320e6122ed7c066273 = $(`<div id="html_881d51ba6a8d97320e6122ed7c066273" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d1a71383a529cb3a56ec7c2ea677d819.setContent(html_881d51ba6a8d97320e6122ed7c066273);\\n \\n \\n\\n circle_marker_0acdbedeafd8d461fd00ad61a67894c2.bindPopup(popup_d1a71383a529cb3a56ec7c2ea677d819)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a478515f758a2a28301552ed970f471 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_10e487609f9ccdb735735adf1729814d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3eb133757f6b7bf1d80982bbf8a270c = $(`<div id="html_a3eb133757f6b7bf1d80982bbf8a270c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10e487609f9ccdb735735adf1729814d.setContent(html_a3eb133757f6b7bf1d80982bbf8a270c);\\n \\n \\n\\n circle_marker_0a478515f758a2a28301552ed970f471.bindPopup(popup_10e487609f9ccdb735735adf1729814d)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff523ba7b9a187df7c8c71fdef1da0f0 = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f1572f76569eed14793aed2c027e7786 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ebbe283339d12c3f67f8c098ce93ce2 = $(`<div id="html_8ebbe283339d12c3f67f8c098ce93ce2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1572f76569eed14793aed2c027e7786.setContent(html_8ebbe283339d12c3f67f8c098ce93ce2);\\n \\n \\n\\n circle_marker_ff523ba7b9a187df7c8c71fdef1da0f0.bindPopup(popup_f1572f76569eed14793aed2c027e7786)\\n ;\\n\\n \\n \\n \\n var circle_marker_379595013f75eabd2e888863a8ef1db6 = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_224902be167cdcade193dfd577457ebe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_35e017dea5a142915b2f64d6d13b242a = $(`<div id="html_35e017dea5a142915b2f64d6d13b242a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_224902be167cdcade193dfd577457ebe.setContent(html_35e017dea5a142915b2f64d6d13b242a);\\n \\n \\n\\n circle_marker_379595013f75eabd2e888863a8ef1db6.bindPopup(popup_224902be167cdcade193dfd577457ebe)\\n ;\\n\\n \\n \\n \\n var circle_marker_d40a3e8ebc4bf039366f44f86b2a04b7 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_28ea8cdaf838060c5879d7dfe482cfe9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e79853ad3bfb90ab02988b08e68901b1 = $(`<div id="html_e79853ad3bfb90ab02988b08e68901b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_28ea8cdaf838060c5879d7dfe482cfe9.setContent(html_e79853ad3bfb90ab02988b08e68901b1);\\n \\n \\n\\n circle_marker_d40a3e8ebc4bf039366f44f86b2a04b7.bindPopup(popup_28ea8cdaf838060c5879d7dfe482cfe9)\\n ;\\n\\n \\n \\n \\n var circle_marker_1447c5e0d66e26b837b164d153d8a4f9 = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a8f891fbb3693b1f0fda26723ea4d660 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_acbfab5715aaeef0597a32952ca5efe0 = $(`<div id="html_acbfab5715aaeef0597a32952ca5efe0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8f891fbb3693b1f0fda26723ea4d660.setContent(html_acbfab5715aaeef0597a32952ca5efe0);\\n \\n \\n\\n circle_marker_1447c5e0d66e26b837b164d153d8a4f9.bindPopup(popup_a8f891fbb3693b1f0fda26723ea4d660)\\n ;\\n\\n \\n \\n \\n var circle_marker_77c92d97f7cd9a073066b812a3c6322a = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4577def37f2585b9970800286ec0edb0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c57cc7749d421d6c1d23ba8360c5bfe2 = $(`<div id="html_c57cc7749d421d6c1d23ba8360c5bfe2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4577def37f2585b9970800286ec0edb0.setContent(html_c57cc7749d421d6c1d23ba8360c5bfe2);\\n \\n \\n\\n circle_marker_77c92d97f7cd9a073066b812a3c6322a.bindPopup(popup_4577def37f2585b9970800286ec0edb0)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e2e1f839d111153288a2775be2545ba = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4527344220b8809dcf6c2359db643235 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_102aee8d1674067e79092b06ab222188 = $(`<div id="html_102aee8d1674067e79092b06ab222188" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4527344220b8809dcf6c2359db643235.setContent(html_102aee8d1674067e79092b06ab222188);\\n \\n \\n\\n circle_marker_2e2e1f839d111153288a2775be2545ba.bindPopup(popup_4527344220b8809dcf6c2359db643235)\\n ;\\n\\n \\n \\n \\n var circle_marker_4ac5eaca90082d2c28544f4aa3466bb3 = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_2c5c04398e517df0abced3aee8489832 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0fcd9cc1ff00b7499f8dd2d53ab9d90 = $(`<div id="html_c0fcd9cc1ff00b7499f8dd2d53ab9d90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c5c04398e517df0abced3aee8489832.setContent(html_c0fcd9cc1ff00b7499f8dd2d53ab9d90);\\n \\n \\n\\n circle_marker_4ac5eaca90082d2c28544f4aa3466bb3.bindPopup(popup_2c5c04398e517df0abced3aee8489832)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee2a4ea00d194437f870a1d040b8e423 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b59df47f928c106a29be653b8b5cd712 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92098695daf4ef8e7eedeedd37524f29 = $(`<div id="html_92098695daf4ef8e7eedeedd37524f29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b59df47f928c106a29be653b8b5cd712.setContent(html_92098695daf4ef8e7eedeedd37524f29);\\n \\n \\n\\n circle_marker_ee2a4ea00d194437f870a1d040b8e423.bindPopup(popup_b59df47f928c106a29be653b8b5cd712)\\n ;\\n\\n \\n \\n \\n var circle_marker_8971b4af1f01de8861d96c6ba04ee434 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_cfb50bcc08e712592d4cc836a2dfc810 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37910d374ff29b19728883512ef1e6cd = $(`<div id="html_37910d374ff29b19728883512ef1e6cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfb50bcc08e712592d4cc836a2dfc810.setContent(html_37910d374ff29b19728883512ef1e6cd);\\n \\n \\n\\n circle_marker_8971b4af1f01de8861d96c6ba04ee434.bindPopup(popup_cfb50bcc08e712592d4cc836a2dfc810)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1c5b6117269232f32b3a7388b1bc31e = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3300d25a372a7d26329d562350c7eba3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3a3e93e2ee0f94f920dcdf5d38d8d68 = $(`<div id="html_d3a3e93e2ee0f94f920dcdf5d38d8d68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3300d25a372a7d26329d562350c7eba3.setContent(html_d3a3e93e2ee0f94f920dcdf5d38d8d68);\\n \\n \\n\\n circle_marker_c1c5b6117269232f32b3a7388b1bc31e.bindPopup(popup_3300d25a372a7d26329d562350c7eba3)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d62c3ab3917ddac48678c6ecde51048 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b425861406686ab8cc71c1d1f134fb48 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f5f450d98fa2e57dbb97b72bf6afaab = $(`<div id="html_1f5f450d98fa2e57dbb97b72bf6afaab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b425861406686ab8cc71c1d1f134fb48.setContent(html_1f5f450d98fa2e57dbb97b72bf6afaab);\\n \\n \\n\\n circle_marker_6d62c3ab3917ddac48678c6ecde51048.bindPopup(popup_b425861406686ab8cc71c1d1f134fb48)\\n ;\\n\\n \\n \\n \\n var circle_marker_8efa7b814bbc1f7e54c836e99117504f = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.51351666838205, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_f763f3f642da7bdcc3b829aac9940286 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f70c7920a21bf1711957834eb6ae1cd = $(`<div id="html_6f70c7920a21bf1711957834eb6ae1cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f763f3f642da7bdcc3b829aac9940286.setContent(html_6f70c7920a21bf1711957834eb6ae1cd);\\n \\n \\n\\n circle_marker_8efa7b814bbc1f7e54c836e99117504f.bindPopup(popup_f763f3f642da7bdcc3b829aac9940286)\\n ;\\n\\n \\n \\n \\n var circle_marker_70ac01fda6d018fe499b4d4f5bb54247 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d85f852e50337de50ef6a8231a499551 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87d8c9022f73d3693af47a1ae995f722 = $(`<div id="html_87d8c9022f73d3693af47a1ae995f722" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d85f852e50337de50ef6a8231a499551.setContent(html_87d8c9022f73d3693af47a1ae995f722);\\n \\n \\n\\n circle_marker_70ac01fda6d018fe499b4d4f5bb54247.bindPopup(popup_d85f852e50337de50ef6a8231a499551)\\n ;\\n\\n \\n \\n \\n var circle_marker_44659757650242b9620e14d3335a5fe5 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_236946773f2afc29711beb0265d02997 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c0d0679ef1f7a8ad8c6558fc6fff4ab = $(`<div id="html_5c0d0679ef1f7a8ad8c6558fc6fff4ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_236946773f2afc29711beb0265d02997.setContent(html_5c0d0679ef1f7a8ad8c6558fc6fff4ab);\\n \\n \\n\\n circle_marker_44659757650242b9620e14d3335a5fe5.bindPopup(popup_236946773f2afc29711beb0265d02997)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3df432aafc2f0648846170f07871499 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_06606f46084f82942b304cae36386523 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46137addadecefe908e4f03685c51c88 = $(`<div id="html_46137addadecefe908e4f03685c51c88" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06606f46084f82942b304cae36386523.setContent(html_46137addadecefe908e4f03685c51c88);\\n \\n \\n\\n circle_marker_f3df432aafc2f0648846170f07871499.bindPopup(popup_06606f46084f82942b304cae36386523)\\n ;\\n\\n \\n \\n \\n var circle_marker_10d361550825c13470ef8049c3f33913 = L.circleMarker(\\n [42.72731562880686, -73.22428095863906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.51351666838205, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_75bf56192d20ffccac8ed6c36fa405e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dfae749933b2e4fe5cdd55aacfc91643 = $(`<div id="html_dfae749933b2e4fe5cdd55aacfc91643" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75bf56192d20ffccac8ed6c36fa405e7.setContent(html_dfae749933b2e4fe5cdd55aacfc91643);\\n \\n \\n\\n circle_marker_10d361550825c13470ef8049c3f33913.bindPopup(popup_75bf56192d20ffccac8ed6c36fa405e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_570f7ce86a47dbde8a4d87316a65e64c = L.circleMarker(\\n [42.72731518778323, -73.22305093225523],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_cca055e11178559932e20c1d8a3ad2cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40a6903b5113a8de097789610bbd8515 = $(`<div id="html_40a6903b5113a8de097789610bbd8515" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cca055e11178559932e20c1d8a3ad2cd.setContent(html_40a6903b5113a8de097789610bbd8515);\\n \\n \\n\\n circle_marker_570f7ce86a47dbde8a4d87316a65e64c.bindPopup(popup_cca055e11178559932e20c1d8a3ad2cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_4afc8598ccd3e6f45af8cee6e5e36605 = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.656366395715726, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c0f9dbf2065d6172cfdf35cd986a86c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_495f84a77782b290bd9d08018273607b = $(`<div id="html_495f84a77782b290bd9d08018273607b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0f9dbf2065d6172cfdf35cd986a86c6.setContent(html_495f84a77782b290bd9d08018273607b);\\n \\n \\n\\n circle_marker_4afc8598ccd3e6f45af8cee6e5e36605.bindPopup(popup_c0f9dbf2065d6172cfdf35cd986a86c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_a0a3a93b52ea4c503371c92fe997d4a7 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_dd14bc0ab784ac6702179f85016a7010 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6023c7f644c78e3a6058b99dabe83986 = $(`<div id="html_6023c7f644c78e3a6058b99dabe83986" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd14bc0ab784ac6702179f85016a7010.setContent(html_6023c7f644c78e3a6058b99dabe83986);\\n \\n \\n\\n circle_marker_a0a3a93b52ea4c503371c92fe997d4a7.bindPopup(popup_dd14bc0ab784ac6702179f85016a7010)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b12258a3fc42a8f687cf93bdc306d10 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7fe47156dcc082b880ceeb5e5f3a4692 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5167688f83324bcad5033c7237ae9107 = $(`<div id="html_5167688f83324bcad5033c7237ae9107" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7fe47156dcc082b880ceeb5e5f3a4692.setContent(html_5167688f83324bcad5033c7237ae9107);\\n \\n \\n\\n circle_marker_5b12258a3fc42a8f687cf93bdc306d10.bindPopup(popup_7fe47156dcc082b880ceeb5e5f3a4692)\\n ;\\n\\n \\n \\n \\n var circle_marker_d20c94e4198cb8e02ecd2354f4a3c2c5 = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e1e8118016e8e5cbb19bd8f61f44c85b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28f894d1d8af742a8fe974711585e1c1 = $(`<div id="html_28f894d1d8af742a8fe974711585e1c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1e8118016e8e5cbb19bd8f61f44c85b.setContent(html_28f894d1d8af742a8fe974711585e1c1);\\n \\n \\n\\n circle_marker_d20c94e4198cb8e02ecd2354f4a3c2c5.bindPopup(popup_e1e8118016e8e5cbb19bd8f61f44c85b)\\n ;\\n\\n \\n \\n \\n var circle_marker_37783aa1b22e46c1215e4681a8184c88 = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_cd922cddb05406a3619f03065ff7350f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b51ff5b7e90d69b56fc2e092028b0da3 = $(`<div id="html_b51ff5b7e90d69b56fc2e092028b0da3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd922cddb05406a3619f03065ff7350f.setContent(html_b51ff5b7e90d69b56fc2e092028b0da3);\\n \\n \\n\\n circle_marker_37783aa1b22e46c1215e4681a8184c88.bindPopup(popup_cd922cddb05406a3619f03065ff7350f)\\n ;\\n\\n \\n \\n \\n var circle_marker_20ac0f25f0e2173fb635a3d0e9ae6f91 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e893f66922bf7df0ad754fb4cf1cde30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f4304c82a17512afa24371b720f09fa9 = $(`<div id="html_f4304c82a17512afa24371b720f09fa9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e893f66922bf7df0ad754fb4cf1cde30.setContent(html_f4304c82a17512afa24371b720f09fa9);\\n \\n \\n\\n circle_marker_20ac0f25f0e2173fb635a3d0e9ae6f91.bindPopup(popup_e893f66922bf7df0ad754fb4cf1cde30)\\n ;\\n\\n \\n \\n \\n var circle_marker_57a87b919d39f3a5a579e6a5372317f4 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_4cc811449806f8bdf4dffde6c571fcdc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d50e53dcde923bf9aed20274fcf98d2 = $(`<div id="html_6d50e53dcde923bf9aed20274fcf98d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4cc811449806f8bdf4dffde6c571fcdc.setContent(html_6d50e53dcde923bf9aed20274fcf98d2);\\n \\n \\n\\n circle_marker_57a87b919d39f3a5a579e6a5372317f4.bindPopup(popup_4cc811449806f8bdf4dffde6c571fcdc)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b945b8f1aa7bb57dff4327eebbcf897 = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_fbfdc0172e6c01d41110e3fbf6307785 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3650d1c2e84660e402bd18cffdb7fafa = $(`<div id="html_3650d1c2e84660e402bd18cffdb7fafa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fbfdc0172e6c01d41110e3fbf6307785.setContent(html_3650d1c2e84660e402bd18cffdb7fafa);\\n \\n \\n\\n circle_marker_5b945b8f1aa7bb57dff4327eebbcf897.bindPopup(popup_fbfdc0172e6c01d41110e3fbf6307785)\\n ;\\n\\n \\n \\n \\n var circle_marker_425db60b068b1abe8c2445b1f824e0d3 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c4694a3c70b5851546887fb45421fecf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_98a8317efa0d1bf0c4df56558585b9c3 = $(`<div id="html_98a8317efa0d1bf0c4df56558585b9c3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4694a3c70b5851546887fb45421fecf.setContent(html_98a8317efa0d1bf0c4df56558585b9c3);\\n \\n \\n\\n circle_marker_425db60b068b1abe8c2445b1f824e0d3.bindPopup(popup_c4694a3c70b5851546887fb45421fecf)\\n ;\\n\\n \\n \\n \\n var circle_marker_39e8b4685949a732eca977a1f6d89cd8 = L.circleMarker(\\n [42.72551563738435, -73.26241185416787],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7f1fd6b44ad81a8223d5297ab093a5f7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9872c77c1c81fa2059cd7c36a42b8574 = $(`<div id="html_9872c77c1c81fa2059cd7c36a42b8574" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f1fd6b44ad81a8223d5297ab093a5f7.setContent(html_9872c77c1c81fa2059cd7c36a42b8574);\\n \\n \\n\\n circle_marker_39e8b4685949a732eca977a1f6d89cd8.bindPopup(popup_7f1fd6b44ad81a8223d5297ab093a5f7)\\n ;\\n\\n \\n \\n \\n var circle_marker_28d391311a080455fe1a7c1a34173dec = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_52a89c63c0ecb9c53b6288778d2111ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba6d3e15278b983a5a6733400b5f68f1 = $(`<div id="html_ba6d3e15278b983a5a6733400b5f68f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52a89c63c0ecb9c53b6288778d2111ec.setContent(html_ba6d3e15278b983a5a6733400b5f68f1);\\n \\n \\n\\n circle_marker_28d391311a080455fe1a7c1a34173dec.bindPopup(popup_52a89c63c0ecb9c53b6288778d2111ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_b489498f094092618b21594a569f5ad9 = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_babfa3838aa79f03897737df1311e31f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3a1ba6f36d04876fb606da647041bcd = $(`<div id="html_d3a1ba6f36d04876fb606da647041bcd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_babfa3838aa79f03897737df1311e31f.setContent(html_d3a1ba6f36d04876fb606da647041bcd);\\n \\n \\n\\n circle_marker_b489498f094092618b21594a569f5ad9.bindPopup(popup_babfa3838aa79f03897737df1311e31f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b63a00a4f128b0653e080c1cb01e540c = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c5f1ec9d3a552f7e5dea5d585c0712d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82eb37c55a2f5443d905ff4747ddb209 = $(`<div id="html_82eb37c55a2f5443d905ff4747ddb209" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c5f1ec9d3a552f7e5dea5d585c0712d3.setContent(html_82eb37c55a2f5443d905ff4747ddb209);\\n \\n \\n\\n circle_marker_b63a00a4f128b0653e080c1cb01e540c.bindPopup(popup_c5f1ec9d3a552f7e5dea5d585c0712d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a7faf9ca48401a72308d80607dc53cd = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_0c6e516b3abe6783e980c6caaeb9da1e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44f504991a9f86503104123cd271b407 = $(`<div id="html_44f504991a9f86503104123cd271b407" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c6e516b3abe6783e980c6caaeb9da1e.setContent(html_44f504991a9f86503104123cd271b407);\\n \\n \\n\\n circle_marker_8a7faf9ca48401a72308d80607dc53cd.bindPopup(popup_0c6e516b3abe6783e980c6caaeb9da1e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8da5d1e401c89790394e816419ac054 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_05a72c1e2c97f582c4164e850fb9acad = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c99fdc637037d448030a6032b74c8914 = $(`<div id="html_c99fdc637037d448030a6032b74c8914" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05a72c1e2c97f582c4164e850fb9acad.setContent(html_c99fdc637037d448030a6032b74c8914);\\n \\n \\n\\n circle_marker_e8da5d1e401c89790394e816419ac054.bindPopup(popup_05a72c1e2c97f582c4164e850fb9acad)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cd6141b9d5a01f28dee2b33d62b4997 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_6f98acd3be164e8bd36f3528a7925eb7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df0da645711c6faf25756b00b053cd85 = $(`<div id="html_df0da645711c6faf25756b00b053cd85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f98acd3be164e8bd36f3528a7925eb7.setContent(html_df0da645711c6faf25756b00b053cd85);\\n \\n \\n\\n circle_marker_3cd6141b9d5a01f28dee2b33d62b4997.bindPopup(popup_6f98acd3be164e8bd36f3528a7925eb7)\\n ;\\n\\n \\n \\n \\n var circle_marker_e827fb8d4f2f40ba8102f2f7eeed699c = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e41172b31ca83531c01433b4bf5edf2d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b2caa20323815486303028d48708bc8 = $(`<div id="html_5b2caa20323815486303028d48708bc8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e41172b31ca83531c01433b4bf5edf2d.setContent(html_5b2caa20323815486303028d48708bc8);\\n \\n \\n\\n circle_marker_e827fb8d4f2f40ba8102f2f7eeed699c.bindPopup(popup_e41172b31ca83531c01433b4bf5edf2d)\\n ;\\n\\n \\n \\n \\n var circle_marker_40a9076d64408c5022de0f106f582d5a = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c9c6e38a8010f339be1f4205b7be82d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c4e854cc5ccff12c1875cb85ff46f2ee = $(`<div id="html_c4e854cc5ccff12c1875cb85ff46f2ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9c6e38a8010f339be1f4205b7be82d1.setContent(html_c4e854cc5ccff12c1875cb85ff46f2ee);\\n \\n \\n\\n circle_marker_40a9076d64408c5022de0f106f582d5a.bindPopup(popup_c9c6e38a8010f339be1f4205b7be82d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_1f458cddf297c397e0bb42fb782af56c = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_fd3ff014993d1fc5000c62933c6830c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9257d22d760f0b4e094da8cab60bc00 = $(`<div id="html_d9257d22d760f0b4e094da8cab60bc00" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd3ff014993d1fc5000c62933c6830c9.setContent(html_d9257d22d760f0b4e094da8cab60bc00);\\n \\n \\n\\n circle_marker_1f458cddf297c397e0bb42fb782af56c.bindPopup(popup_fd3ff014993d1fc5000c62933c6830c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9af6c95351e16f99333d476c45326935 = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d5b193fac621111f3baab977ef3eb3a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aea1a250cdc811399e6cc51b8408411f = $(`<div id="html_aea1a250cdc811399e6cc51b8408411f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5b193fac621111f3baab977ef3eb3a0.setContent(html_aea1a250cdc811399e6cc51b8408411f);\\n \\n \\n\\n circle_marker_9af6c95351e16f99333d476c45326935.bindPopup(popup_d5b193fac621111f3baab977ef3eb3a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6d742f40cb2bc1f19f61f17016718af = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1f1643d3a9739afc1ba8e3289b4ef1a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99d09ba79267a89579fa3f9e1d25ebdb = $(`<div id="html_99d09ba79267a89579fa3f9e1d25ebdb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f1643d3a9739afc1ba8e3289b4ef1a2.setContent(html_99d09ba79267a89579fa3f9e1d25ebdb);\\n \\n \\n\\n circle_marker_d6d742f40cb2bc1f19f61f17016718af.bindPopup(popup_1f1643d3a9739afc1ba8e3289b4ef1a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_10a3c1ba035c66c798327844b857a570 = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_9d516371afceb19f39ca08c245e2d605 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0159a53e034857c6b4ed6baf63bda398 = $(`<div id="html_0159a53e034857c6b4ed6baf63bda398" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d516371afceb19f39ca08c245e2d605.setContent(html_0159a53e034857c6b4ed6baf63bda398);\\n \\n \\n\\n circle_marker_10a3c1ba035c66c798327844b857a570.bindPopup(popup_9d516371afceb19f39ca08c245e2d605)\\n ;\\n\\n \\n \\n \\n var circle_marker_a39817d6ca9c95f06475209aa7d111fd = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_5d4e578174c5f9cc2c0e53ffccb5c079 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_657511c0e96023e2e24ba7ec9e6b6ec4 = $(`<div id="html_657511c0e96023e2e24ba7ec9e6b6ec4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d4e578174c5f9cc2c0e53ffccb5c079.setContent(html_657511c0e96023e2e24ba7ec9e6b6ec4);\\n \\n \\n\\n circle_marker_a39817d6ca9c95f06475209aa7d111fd.bindPopup(popup_5d4e578174c5f9cc2c0e53ffccb5c079)\\n ;\\n\\n \\n \\n \\n var circle_marker_9cb45288e70b3b7691a24358f8b0a462 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_94987bba2af6e19c0be7ec0f15ae123a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e1ad3b32cbb16edd76e2ab57e9849978 = $(`<div id="html_e1ad3b32cbb16edd76e2ab57e9849978" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94987bba2af6e19c0be7ec0f15ae123a.setContent(html_e1ad3b32cbb16edd76e2ab57e9849978);\\n \\n \\n\\n circle_marker_9cb45288e70b3b7691a24358f8b0a462.bindPopup(popup_94987bba2af6e19c0be7ec0f15ae123a)\\n ;\\n\\n \\n \\n \\n var circle_marker_6297bbffa1d075e9e36d6fe3795fe025 = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_fff0d8df26c6a7b1ebbf2cd55af6cf53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d9b868da0ac2dd0b8c8030d538244ee = $(`<div id="html_7d9b868da0ac2dd0b8c8030d538244ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fff0d8df26c6a7b1ebbf2cd55af6cf53.setContent(html_7d9b868da0ac2dd0b8c8030d538244ee);\\n \\n \\n\\n circle_marker_6297bbffa1d075e9e36d6fe3795fe025.bindPopup(popup_fff0d8df26c6a7b1ebbf2cd55af6cf53)\\n ;\\n\\n \\n \\n \\n var circle_marker_ecb9ac70739988ca86da212da0337784 = L.circleMarker(\\n [42.72551121416183, -73.23289207553623],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_b3d78b0b179231350fa26e82ce144e16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6898864285cc486c6880f182b7e894c6 = $(`<div id="html_6898864285cc486c6880f182b7e894c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3d78b0b179231350fa26e82ce144e16.setContent(html_6898864285cc486c6880f182b7e894c6);\\n \\n \\n\\n circle_marker_ecb9ac70739988ca86da212da0337784.bindPopup(popup_b3d78b0b179231350fa26e82ce144e16)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d676dd7ed9dd9e8ba86f404bf5ec6ea = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_7df5c8a944b93367752d0d702e41d1d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2d818d6405cec4de48cb4f2fb439c9b5 = $(`<div id="html_2d818d6405cec4de48cb4f2fb439c9b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7df5c8a944b93367752d0d702e41d1d8.setContent(html_2d818d6405cec4de48cb4f2fb439c9b5);\\n \\n \\n\\n circle_marker_5d676dd7ed9dd9e8ba86f404bf5ec6ea.bindPopup(popup_7df5c8a944b93367752d0d702e41d1d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_9507fc29087782d8421c1402a6104a6f = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.077706251929807, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_c8ce66a753f3e4a6a71588f8182ae3c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54144eab038147430dd0ff6064ea9730 = $(`<div id="html_54144eab038147430dd0ff6064ea9730" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8ce66a753f3e4a6a71588f8182ae3c0.setContent(html_54144eab038147430dd0ff6064ea9730);\\n \\n \\n\\n circle_marker_9507fc29087782d8421c1402a6104a6f.bindPopup(popup_c8ce66a753f3e4a6a71588f8182ae3c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f349189916231859cf3f481f7d7f265 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_106a7e9361906b7c71b2b0271f050c2e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b9c3e035a757c83f7825c8bbcdfcaec = $(`<div id="html_1b9c3e035a757c83f7825c8bbcdfcaec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_106a7e9361906b7c71b2b0271f050c2e.setContent(html_1b9c3e035a757c83f7825c8bbcdfcaec);\\n \\n \\n\\n circle_marker_6f349189916231859cf3f481f7d7f265.bindPopup(popup_106a7e9361906b7c71b2b0271f050c2e)\\n ;\\n\\n \\n \\n \\n var circle_marker_188ff4706e74f47035cdfc557bde4e55 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_ef84f8ee1ee25453035e17947fff6b46 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9dc3d6d9ceaa55d09c70095f86dd7bb9 = $(`<div id="html_9dc3d6d9ceaa55d09c70095f86dd7bb9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef84f8ee1ee25453035e17947fff6b46.setContent(html_9dc3d6d9ceaa55d09c70095f86dd7bb9);\\n \\n \\n\\n circle_marker_188ff4706e74f47035cdfc557bde4e55.bindPopup(popup_ef84f8ee1ee25453035e17947fff6b46)\\n ;\\n\\n \\n \\n \\n var circle_marker_910e9d9d1963a1a670e0881f0853f233 = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_3971c484670993d8a652677f1703e481 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1efab1d716ab7055970feece2bc7fc7c = $(`<div id="html_1efab1d716ab7055970feece2bc7fc7c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3971c484670993d8a652677f1703e481.setContent(html_1efab1d716ab7055970feece2bc7fc7c);\\n \\n \\n\\n circle_marker_910e9d9d1963a1a670e0881f0853f233.bindPopup(popup_3971c484670993d8a652677f1703e481)\\n ;\\n\\n \\n \\n \\n var circle_marker_673f676c5d03be5133c3decb46a539be = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_d0b6e71cccfbe6d116e6b856cb600a1c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4706a496c78d60f1a4fbeddf203a9d67 = $(`<div id="html_4706a496c78d60f1a4fbeddf203a9d67" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0b6e71cccfbe6d116e6b856cb600a1c.setContent(html_4706a496c78d60f1a4fbeddf203a9d67);\\n \\n \\n\\n circle_marker_673f676c5d03be5133c3decb46a539be.bindPopup(popup_d0b6e71cccfbe6d116e6b856cb600a1c)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ce81900329bdabc52d7b6b072e3a907 = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_1546e2c5c53d805eb88d84085e7de936 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e4232d28a077a2a5355d99c89da5eb9 = $(`<div id="html_8e4232d28a077a2a5355d99c89da5eb9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1546e2c5c53d805eb88d84085e7de936.setContent(html_8e4232d28a077a2a5355d99c89da5eb9);\\n \\n \\n\\n circle_marker_1ce81900329bdabc52d7b6b072e3a907.bindPopup(popup_1546e2c5c53d805eb88d84085e7de936)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e626778cebf29fe082c5855e742b269 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a8c3efb03b37164b8287bb249d03c0a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ebd9b85516cb16f96d5ff4ddabaa10a4 = $(`<div id="html_ebd9b85516cb16f96d5ff4ddabaa10a4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8c3efb03b37164b8287bb249d03c0a8.setContent(html_ebd9b85516cb16f96d5ff4ddabaa10a4);\\n \\n \\n\\n circle_marker_7e626778cebf29fe082c5855e742b269.bindPopup(popup_a8c3efb03b37164b8287bb249d03c0a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a9938c17e40d1c5025172b4677cb348 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_a19eeac25bd4705f6d59843da07df830 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e56ff9ed64245d43c7ced7c5ffe86fbe = $(`<div id="html_e56ff9ed64245d43c7ced7c5ffe86fbe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a19eeac25bd4705f6d59843da07df830.setContent(html_e56ff9ed64245d43c7ced7c5ffe86fbe);\\n \\n \\n\\n circle_marker_0a9938c17e40d1c5025172b4677cb348.bindPopup(popup_a19eeac25bd4705f6d59843da07df830)\\n ;\\n\\n \\n \\n \\n var circle_marker_dcb0125a71f0f93bff371396414ead19 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_e6df2d332790d9c8bb27509d56c7ff4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87409d2db60f0e24065161873f73e990 = $(`<div id="html_87409d2db60f0e24065161873f73e990" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6df2d332790d9c8bb27509d56c7ff4f.setContent(html_87409d2db60f0e24065161873f73e990);\\n \\n \\n\\n circle_marker_dcb0125a71f0f93bff371396414ead19.bindPopup(popup_e6df2d332790d9c8bb27509d56c7ff4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7b40553468196a6ac83d9eae0f5cf61 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_99311c7d57a2244d31d290929e418f23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_558f389726cc178141e360f1fe311f03 = $(`<div id="html_558f389726cc178141e360f1fe311f03" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_99311c7d57a2244d31d290929e418f23.setContent(html_558f389726cc178141e360f1fe311f03);\\n \\n \\n\\n circle_marker_b7b40553468196a6ac83d9eae0f5cf61.bindPopup(popup_99311c7d57a2244d31d290929e418f23)\\n ;\\n\\n \\n \\n \\n var circle_marker_7839bca28f5d0295c3333c6e45e8c50e = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_3261d56ffb7664e1c2746248574c024b);\\n \\n \\n var popup_91af093fb1bc866c506a7fb4e881cb2b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c58a830879772812b3cc6c228e01a209 = $(`<div id="html_c58a830879772812b3cc6c228e01a209" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91af093fb1bc866c506a7fb4e881cb2b.setContent(html_c58a830879772812b3cc6c228e01a209);\\n \\n \\n\\n circle_marker_7839bca28f5d0295c3333c6e45e8c50e.bindPopup(popup_91af093fb1bc866c506a7fb4e881cb2b)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Maple, striped\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_26545a85bb6c1aa612454c4160cb6eaf {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_26545a85bb6c1aa612454c4160cb6eaf" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_26545a85bb6c1aa612454c4160cb6eaf = L.map(\\n "map_26545a85bb6c1aa612454c4160cb6eaf",\\n {\\n center: [42.73545193541105, -73.24764986998687],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_afa7ce0b09fdffcdcd6e3786bae714cd = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var circle_marker_b4a3c3352b9c74971a03e2b96c253f6e = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ecf944bbfc3704f0c75f8e5368192fb3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b90fcc675a20440b481b03b5363d1ae2 = $(`<div id="html_b90fcc675a20440b481b03b5363d1ae2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ecf944bbfc3704f0c75f8e5368192fb3.setContent(html_b90fcc675a20440b481b03b5363d1ae2);\\n \\n \\n\\n circle_marker_b4a3c3352b9c74971a03e2b96c253f6e.bindPopup(popup_ecf944bbfc3704f0c75f8e5368192fb3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d69637379f2ff99fc5310c0b753902fd = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_302e18e682b3d96f54aae42e2b4a27f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50ac752c312dac07b87a658b3fce73ed = $(`<div id="html_50ac752c312dac07b87a658b3fce73ed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_302e18e682b3d96f54aae42e2b4a27f9.setContent(html_50ac752c312dac07b87a658b3fce73ed);\\n \\n \\n\\n circle_marker_d69637379f2ff99fc5310c0b753902fd.bindPopup(popup_302e18e682b3d96f54aae42e2b4a27f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ffc30e768241a6192e4cf1b68bd1ad0 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f1ca75810245d7a2cb8a5022a31b5aa4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_16097baa67ecf65b8bd4ebf56168d0a5 = $(`<div id="html_16097baa67ecf65b8bd4ebf56168d0a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1ca75810245d7a2cb8a5022a31b5aa4.setContent(html_16097baa67ecf65b8bd4ebf56168d0a5);\\n \\n \\n\\n circle_marker_7ffc30e768241a6192e4cf1b68bd1ad0.bindPopup(popup_f1ca75810245d7a2cb8a5022a31b5aa4)\\n ;\\n\\n \\n \\n \\n var circle_marker_912bb64db6239576b86b6981db17c896 = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d85d85a3a63e987fe9ffd25d067edef7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d552b9419efda2ea658510febf0a877 = $(`<div id="html_3d552b9419efda2ea658510febf0a877" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d85d85a3a63e987fe9ffd25d067edef7.setContent(html_3d552b9419efda2ea658510febf0a877);\\n \\n \\n\\n circle_marker_912bb64db6239576b86b6981db17c896.bindPopup(popup_d85d85a3a63e987fe9ffd25d067edef7)\\n ;\\n\\n \\n \\n \\n var circle_marker_c552240a8c2cc15b521aa8a77931c866 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7abde4cff7a0ddab5f04e96743185a91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf98c70c01f22d8a3c15f36abb959fec = $(`<div id="html_bf98c70c01f22d8a3c15f36abb959fec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7abde4cff7a0ddab5f04e96743185a91.setContent(html_bf98c70c01f22d8a3c15f36abb959fec);\\n \\n \\n\\n circle_marker_c552240a8c2cc15b521aa8a77931c866.bindPopup(popup_7abde4cff7a0ddab5f04e96743185a91)\\n ;\\n\\n \\n \\n \\n var circle_marker_3691fa2af6ceb5d0b8eb618b54acf4ef = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9615e544e65582305fcb3bdd9eb3c317 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3254c9182453f10ef6feabe7dbfc0049 = $(`<div id="html_3254c9182453f10ef6feabe7dbfc0049" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9615e544e65582305fcb3bdd9eb3c317.setContent(html_3254c9182453f10ef6feabe7dbfc0049);\\n \\n \\n\\n circle_marker_3691fa2af6ceb5d0b8eb618b54acf4ef.bindPopup(popup_9615e544e65582305fcb3bdd9eb3c317)\\n ;\\n\\n \\n \\n \\n var circle_marker_aabe10e12c1b170eb21fb18f5cfe5e43 = L.circleMarker(\\n [42.747201204933376, -73.2685630990657],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_95d521c401b0662566d9622add3c9fc7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5359c6ef21cb7bbe0b0cdfdf5dfbe8e4 = $(`<div id="html_5359c6ef21cb7bbe0b0cdfdf5dfbe8e4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95d521c401b0662566d9622add3c9fc7.setContent(html_5359c6ef21cb7bbe0b0cdfdf5dfbe8e4);\\n \\n \\n\\n circle_marker_aabe10e12c1b170eb21fb18f5cfe5e43.bindPopup(popup_95d521c401b0662566d9622add3c9fc7)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec791f8b0f131e9ae9884eb356734b31 = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_96a4b1faf6274ae420f368f77f9e3419 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99f604d18d683d2b07f34849531a879b = $(`<div id="html_99f604d18d683d2b07f34849531a879b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96a4b1faf6274ae420f368f77f9e3419.setContent(html_99f604d18d683d2b07f34849531a879b);\\n \\n \\n\\n circle_marker_ec791f8b0f131e9ae9884eb356734b31.bindPopup(popup_96a4b1faf6274ae420f368f77f9e3419)\\n ;\\n\\n \\n \\n \\n var circle_marker_59c917f76216187e2e97497c34ee6737 = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.257165172872317, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7e3861a48a7cede38e84ca90a062dd1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_995169d2f955ee1e5753c6c700a9ebc5 = $(`<div id="html_995169d2f955ee1e5753c6c700a9ebc5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e3861a48a7cede38e84ca90a062dd1b.setContent(html_995169d2f955ee1e5753c6c700a9ebc5);\\n \\n \\n\\n circle_marker_59c917f76216187e2e97497c34ee6737.bindPopup(popup_7e3861a48a7cede38e84ca90a062dd1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_c65e96a26eb60082141f38795292d466 = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.014626706796775, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c64126e645dcaf25af169a8634bd61b0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce4b4424b7bf303c769aa36d16114f64 = $(`<div id="html_ce4b4424b7bf303c769aa36d16114f64" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c64126e645dcaf25af169a8634bd61b0.setContent(html_ce4b4424b7bf303c769aa36d16114f64);\\n \\n \\n\\n circle_marker_c65e96a26eb60082141f38795292d466.bindPopup(popup_c64126e645dcaf25af169a8634bd61b0)\\n ;\\n\\n \\n \\n \\n var circle_marker_66b97ca81323259f166203f7106e7141 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.068428945128219, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9b33253179ed7e9172767d53e1754eb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f45f9245f63646c695bad72d858bc36 = $(`<div id="html_8f45f9245f63646c695bad72d858bc36" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b33253179ed7e9172767d53e1754eb8.setContent(html_8f45f9245f63646c695bad72d858bc36);\\n \\n \\n\\n circle_marker_66b97ca81323259f166203f7106e7141.bindPopup(popup_9b33253179ed7e9172767d53e1754eb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2216e31cce598f6f1c75d4bdda7581c1 = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b810d56e590b5799cd989481e66a3c4a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d82b7ffae2e24daa2b9139ef36bce3e = $(`<div id="html_6d82b7ffae2e24daa2b9139ef36bce3e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b810d56e590b5799cd989481e66a3c4a.setContent(html_6d82b7ffae2e24daa2b9139ef36bce3e);\\n \\n \\n\\n circle_marker_2216e31cce598f6f1c75d4bdda7581c1.bindPopup(popup_b810d56e590b5799cd989481e66a3c4a)\\n ;\\n\\n \\n \\n \\n var circle_marker_16483aad584b3de3f5098975957dbbc8 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_61f00cda32eb2a39b0369efbf20ef62e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_107c091567e655982c7c164d0dd8a698 = $(`<div id="html_107c091567e655982c7c164d0dd8a698" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61f00cda32eb2a39b0369efbf20ef62e.setContent(html_107c091567e655982c7c164d0dd8a698);\\n \\n \\n\\n circle_marker_16483aad584b3de3f5098975957dbbc8.bindPopup(popup_61f00cda32eb2a39b0369efbf20ef62e)\\n ;\\n\\n \\n \\n \\n var circle_marker_704fd27886ee2e59e9d286c22e447962 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c435e7e4464afa5a938c9ab9d3ef5663 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7d677ec6459ae64f0f2678eb6f86f89 = $(`<div id="html_e7d677ec6459ae64f0f2678eb6f86f89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c435e7e4464afa5a938c9ab9d3ef5663.setContent(html_e7d677ec6459ae64f0f2678eb6f86f89);\\n \\n \\n\\n circle_marker_704fd27886ee2e59e9d286c22e447962.bindPopup(popup_c435e7e4464afa5a938c9ab9d3ef5663)\\n ;\\n\\n \\n \\n \\n var circle_marker_02a9a1917216396786a69873383e9f67 = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_724da1e0914b5f1973b882ecbb9236a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db3cb8853445ee21f00e896da37cdbe7 = $(`<div id="html_db3cb8853445ee21f00e896da37cdbe7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_724da1e0914b5f1973b882ecbb9236a6.setContent(html_db3cb8853445ee21f00e896da37cdbe7);\\n \\n \\n\\n circle_marker_02a9a1917216396786a69873383e9f67.bindPopup(popup_724da1e0914b5f1973b882ecbb9236a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa86f9c0048a2d50684edddc3b2736e7 = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4e509d95dab9da4bee6f396a2144c43a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d5c11c6c7611dc91ebe715e4ed5c6df2 = $(`<div id="html_d5c11c6c7611dc91ebe715e4ed5c6df2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e509d95dab9da4bee6f396a2144c43a.setContent(html_d5c11c6c7611dc91ebe715e4ed5c6df2);\\n \\n \\n\\n circle_marker_aa86f9c0048a2d50684edddc3b2736e7.bindPopup(popup_4e509d95dab9da4bee6f396a2144c43a)\\n ;\\n\\n \\n \\n \\n var circle_marker_5588b40d689d7be4bc1ea1f188348845 = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0d03420f4fd0a7f07b6cec75c4b9b553 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43d8302c60dd84e648c6cc64e9249f60 = $(`<div id="html_43d8302c60dd84e648c6cc64e9249f60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d03420f4fd0a7f07b6cec75c4b9b553.setContent(html_43d8302c60dd84e648c6cc64e9249f60);\\n \\n \\n\\n circle_marker_5588b40d689d7be4bc1ea1f188348845.bindPopup(popup_0d03420f4fd0a7f07b6cec75c4b9b553)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c915b296ca089280172b2a510aa83f1 = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_16a06c4bd63dd568b583382c866f738e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56ff6d4a780700247863692fcd051325 = $(`<div id="html_56ff6d4a780700247863692fcd051325" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16a06c4bd63dd568b583382c866f738e.setContent(html_56ff6d4a780700247863692fcd051325);\\n \\n \\n\\n circle_marker_2c915b296ca089280172b2a510aa83f1.bindPopup(popup_16a06c4bd63dd568b583382c866f738e)\\n ;\\n\\n \\n \\n \\n var circle_marker_251850ba5184da498a85039267bfb77c = L.circleMarker(\\n [42.74539359743371, -73.27594530262718],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c19655671dfd63bf648b455b3d69407c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b1a0920963e86b4682ed5995c98a2b0 = $(`<div id="html_2b1a0920963e86b4682ed5995c98a2b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c19655671dfd63bf648b455b3d69407c.setContent(html_2b1a0920963e86b4682ed5995c98a2b0);\\n \\n \\n\\n circle_marker_251850ba5184da498a85039267bfb77c.bindPopup(popup_c19655671dfd63bf648b455b3d69407c)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d6aabffe1ffd1a2aaae5bba2a3656a1 = L.circleMarker(\\n [42.74539347231661, -73.2771756878084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0c65e196385dcba08564ddab55796768 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e75f61962701550130b5bd76853a2bf = $(`<div id="html_2e75f61962701550130b5bd76853a2bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c65e196385dcba08564ddab55796768.setContent(html_2e75f61962701550130b5bd76853a2bf);\\n \\n \\n\\n circle_marker_8d6aabffe1ffd1a2aaae5bba2a3656a1.bindPopup(popup_0c65e196385dcba08564ddab55796768)\\n ;\\n\\n \\n \\n \\n var circle_marker_bd414d1ba8477df12530b0cfcf879d71 = L.circleMarker(\\n [42.74539370938061, -73.27471491744124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.050259243299904, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_95099542771935f47ebad895c647f3a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6777189aa6a7e105705d0a9027057454 = $(`<div id="html_6777189aa6a7e105705d0a9027057454" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95099542771935f47ebad895c647f3a1.setContent(html_6777189aa6a7e105705d0a9027057454);\\n \\n \\n\\n circle_marker_bd414d1ba8477df12530b0cfcf879d71.bindPopup(popup_95099542771935f47ebad895c647f3a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_87cd847dd34efc9d21f2595b37d673de = L.circleMarker(\\n [42.74539380815728, -73.27348453225115],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3361e5155d6cebba0dbc5f47782a219d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c285f4800d311fa6fb6124071c02430a = $(`<div id="html_c285f4800d311fa6fb6124071c02430a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3361e5155d6cebba0dbc5f47782a219d.setContent(html_c285f4800d311fa6fb6124071c02430a);\\n \\n \\n\\n circle_marker_87cd847dd34efc9d21f2595b37d673de.bindPopup(popup_3361e5155d6cebba0dbc5f47782a219d)\\n ;\\n\\n \\n \\n \\n var circle_marker_60ebde28c899ef8ee452be109318e5e6 = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_445175abd259998900c6d38052829339 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a14bdb9ee725bef88639a6c2c14e9e72 = $(`<div id="html_a14bdb9ee725bef88639a6c2c14e9e72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_445175abd259998900c6d38052829339.setContent(html_a14bdb9ee725bef88639a6c2c14e9e72);\\n \\n \\n\\n circle_marker_60ebde28c899ef8ee452be109318e5e6.bindPopup(popup_445175abd259998900c6d38052829339)\\n ;\\n\\n \\n \\n \\n var circle_marker_823cd2f7c8efe8ade00df3c0d3197123 = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d47a0964229b6db368dd55a8dcca462f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_137dec80c7b9f1c8a97a4174b05a9c9f = $(`<div id="html_137dec80c7b9f1c8a97a4174b05a9c9f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d47a0964229b6db368dd55a8dcca462f.setContent(html_137dec80c7b9f1c8a97a4174b05a9c9f);\\n \\n \\n\\n circle_marker_823cd2f7c8efe8ade00df3c0d3197123.bindPopup(popup_d47a0964229b6db368dd55a8dcca462f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1604f6ebdb0ba474464a9f0d984a5e4f = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_52d29115e0ea6660f2e530d68505c8f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_02f70a25c161cf9c37b152b7d398a8c6 = $(`<div id="html_02f70a25c161cf9c37b152b7d398a8c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52d29115e0ea6660f2e530d68505c8f1.setContent(html_02f70a25c161cf9c37b152b7d398a8c6);\\n \\n \\n\\n circle_marker_1604f6ebdb0ba474464a9f0d984a5e4f.bindPopup(popup_52d29115e0ea6660f2e530d68505c8f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_7545de8064bfe37fb7c3467a861c9f87 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.068428945128219, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4a2c425ef5b75546e772074771d87ac5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3fb1eb746cdc5fe0f1028c125c7b29b4 = $(`<div id="html_3fb1eb746cdc5fe0f1028c125c7b29b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a2c425ef5b75546e772074771d87ac5.setContent(html_3fb1eb746cdc5fe0f1028c125c7b29b4);\\n \\n \\n\\n circle_marker_7545de8064bfe37fb7c3467a861c9f87.bindPopup(popup_4a2c425ef5b75546e772074771d87ac5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c9ac40ad0a0f4972629e734b0cf5a6fe = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e29a0eca52952d16ceec98906bfdbeda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fb1f1740803558cab5590288bc453e2 = $(`<div id="html_8fb1f1740803558cab5590288bc453e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e29a0eca52952d16ceec98906bfdbeda.setContent(html_8fb1f1740803558cab5590288bc453e2);\\n \\n \\n\\n circle_marker_c9ac40ad0a0f4972629e734b0cf5a6fe.bindPopup(popup_e29a0eca52952d16ceec98906bfdbeda)\\n ;\\n\\n \\n \\n \\n var circle_marker_8eb4473279b434cbb7629da725382b18 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8480a955942211b06aed01fe29aa868d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6efda57c7759752e849bd4d0a4342933 = $(`<div id="html_6efda57c7759752e849bd4d0a4342933" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8480a955942211b06aed01fe29aa868d.setContent(html_6efda57c7759752e849bd4d0a4342933);\\n \\n \\n\\n circle_marker_8eb4473279b434cbb7629da725382b18.bindPopup(popup_8480a955942211b06aed01fe29aa868d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0a6f90152c23e9caa6d6f11d416c5a4 = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cf9b87cfc318eae634351c4873d69315 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db2945cc5b1d63099dc8734a5fd0e066 = $(`<div id="html_db2945cc5b1d63099dc8734a5fd0e066" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf9b87cfc318eae634351c4873d69315.setContent(html_db2945cc5b1d63099dc8734a5fd0e066);\\n \\n \\n\\n circle_marker_c0a6f90152c23e9caa6d6f11d416c5a4.bindPopup(popup_cf9b87cfc318eae634351c4873d69315)\\n ;\\n\\n \\n \\n \\n var circle_marker_c24694a42fab373db259d05073856821 = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.442433223290478, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c4e89821890fe0fdc8cb34562da51920 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1abc4bb31ed1256beef4a4cbee63516 = $(`<div id="html_d1abc4bb31ed1256beef4a4cbee63516" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4e89821890fe0fdc8cb34562da51920.setContent(html_d1abc4bb31ed1256beef4a4cbee63516);\\n \\n \\n\\n circle_marker_c24694a42fab373db259d05073856821.bindPopup(popup_c4e89821890fe0fdc8cb34562da51920)\\n ;\\n\\n \\n \\n \\n var circle_marker_34d1d2c5ea4c83a06e79a8a9d4d838c7 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_119d3308b8e1fa6145203870e0094486 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d13230625f5ac94fa0041863c2b03157 = $(`<div id="html_d13230625f5ac94fa0041863c2b03157" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_119d3308b8e1fa6145203870e0094486.setContent(html_d13230625f5ac94fa0041863c2b03157);\\n \\n \\n\\n circle_marker_34d1d2c5ea4c83a06e79a8a9d4d838c7.bindPopup(popup_119d3308b8e1fa6145203870e0094486)\\n ;\\n\\n \\n \\n \\n var circle_marker_126520fe868942e50581b05130416564 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c750060a61aaa9ade88a75169769236d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1e72f2a5ec914b9f5ba43e0ff74c6c5 = $(`<div id="html_f1e72f2a5ec914b9f5ba43e0ff74c6c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c750060a61aaa9ade88a75169769236d.setContent(html_f1e72f2a5ec914b9f5ba43e0ff74c6c5);\\n \\n \\n\\n circle_marker_126520fe868942e50581b05130416564.bindPopup(popup_c750060a61aaa9ade88a75169769236d)\\n ;\\n\\n \\n \\n \\n var circle_marker_6473a3c628ad1d3c2090e9d000a14221 = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_85eb108ddfeeb85983926bc44eca71bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6dc1d134298293c2969dd23e29c1c14 = $(`<div id="html_c6dc1d134298293c2969dd23e29c1c14" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_85eb108ddfeeb85983926bc44eca71bd.setContent(html_c6dc1d134298293c2969dd23e29c1c14);\\n \\n \\n\\n circle_marker_6473a3c628ad1d3c2090e9d000a14221.bindPopup(popup_85eb108ddfeeb85983926bc44eca71bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_f8b6199112a034409bf092bad89b6470 = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_edde3472b12ccb0def4c384c249d0753 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28e2131dd9577c3df1c42484499ba2d1 = $(`<div id="html_28e2131dd9577c3df1c42484499ba2d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_edde3472b12ccb0def4c384c249d0753.setContent(html_28e2131dd9577c3df1c42484499ba2d1);\\n \\n \\n\\n circle_marker_f8b6199112a034409bf092bad89b6470.bindPopup(popup_edde3472b12ccb0def4c384c249d0753)\\n ;\\n\\n \\n \\n \\n var circle_marker_0bcee6c4631a749870ac65ee48ea5a83 = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.556622981616415, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_86ae34cb4956a3b0c8d259ee638401e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e41c6407217aa1853935a193663ee748 = $(`<div id="html_e41c6407217aa1853935a193663ee748" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86ae34cb4956a3b0c8d259ee638401e7.setContent(html_e41c6407217aa1853935a193663ee748);\\n \\n \\n\\n circle_marker_0bcee6c4631a749870ac65ee48ea5a83.bindPopup(popup_86ae34cb4956a3b0c8d259ee638401e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_2bb8175ac6a4b65b4e9294da9365b2a2 = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7f05b136bc2c2668be6f4935ed682d04 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f2d3d9100b50c115df110ccdb3f8244 = $(`<div id="html_6f2d3d9100b50c115df110ccdb3f8244" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f05b136bc2c2668be6f4935ed682d04.setContent(html_6f2d3d9100b50c115df110ccdb3f8244);\\n \\n \\n\\n circle_marker_2bb8175ac6a4b65b4e9294da9365b2a2.bindPopup(popup_7f05b136bc2c2668be6f4935ed682d04)\\n ;\\n\\n \\n \\n \\n var circle_marker_84ec32d4902c316fac302983670b35e5 = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_41c40b70c3ebbe5e000f367b8a31810b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cebcbaaca07ffe9dcb945c26f57c982b = $(`<div id="html_cebcbaaca07ffe9dcb945c26f57c982b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41c40b70c3ebbe5e000f367b8a31810b.setContent(html_cebcbaaca07ffe9dcb945c26f57c982b);\\n \\n \\n\\n circle_marker_84ec32d4902c316fac302983670b35e5.bindPopup(popup_41c40b70c3ebbe5e000f367b8a31810b)\\n ;\\n\\n \\n \\n \\n var circle_marker_aeaf6e7cb05208fa6eb0af22e51add46 = L.circleMarker(\\n [42.74358657602363, -73.27471463051425],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e66eda915cbc57792fb1f73326425ead = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fa41e2cc457f2414fdf53d893d67833d = $(`<div id="html_fa41e2cc457f2414fdf53d893d67833d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e66eda915cbc57792fb1f73326425ead.setContent(html_fa41e2cc457f2414fdf53d893d67833d);\\n \\n \\n\\n circle_marker_aeaf6e7cb05208fa6eb0af22e51add46.bindPopup(popup_e66eda915cbc57792fb1f73326425ead)\\n ;\\n\\n \\n \\n \\n var circle_marker_72c43d8d5e2044aecba7a2b6229f9f29 = L.circleMarker(\\n [42.74358667479628, -73.27348428119001],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a13ca68a5e60610870d600bf3aba5078 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7668e275596c59fc8667a0ad0e767277 = $(`<div id="html_7668e275596c59fc8667a0ad0e767277" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a13ca68a5e60610870d600bf3aba5078.setContent(html_7668e275596c59fc8667a0ad0e767277);\\n \\n \\n\\n circle_marker_72c43d8d5e2044aecba7a2b6229f9f29.bindPopup(popup_a13ca68a5e60610870d600bf3aba5078)\\n ;\\n\\n \\n \\n \\n var circle_marker_6bd0d9122402e556545e9a68d62f87ac = L.circleMarker(\\n [42.74358676039927, -73.27225393186212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d47e11fbbb78a9d19072c992dc2fc4ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_422b7879ae3d3917f6302cfdd840efa0 = $(`<div id="html_422b7879ae3d3917f6302cfdd840efa0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d47e11fbbb78a9d19072c992dc2fc4ab.setContent(html_422b7879ae3d3917f6302cfdd840efa0);\\n \\n \\n\\n circle_marker_6bd0d9122402e556545e9a68d62f87ac.bindPopup(popup_d47e11fbbb78a9d19072c992dc2fc4ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_f17e9715e8660c90f35b69a46a35ce25 = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3082f106cb47f05c916ad778b2822b7a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf0997ad7f4ce75cf9d9d2fe193d8ee6 = $(`<div id="html_bf0997ad7f4ce75cf9d9d2fe193d8ee6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3082f106cb47f05c916ad778b2822b7a.setContent(html_bf0997ad7f4ce75cf9d9d2fe193d8ee6);\\n \\n \\n\\n circle_marker_f17e9715e8660c90f35b69a46a35ce25.bindPopup(popup_3082f106cb47f05c916ad778b2822b7a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7523822e846efea5b65100131386358 = L.circleMarker(\\n [42.74358689209615, -73.26979323319746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2d695a77d9c890852402bc2f0d9712a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f5990753da5458f38e5506a06552252c = $(`<div id="html_f5990753da5458f38e5506a06552252c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d695a77d9c890852402bc2f0d9712a8.setContent(html_f5990753da5458f38e5506a06552252c);\\n \\n \\n\\n circle_marker_a7523822e846efea5b65100131386358.bindPopup(popup_2d695a77d9c890852402bc2f0d9712a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_e88b0b6a7f1aa8115739854abf1ebeba = L.circleMarker(\\n [42.743586938190056, -73.26856288386173],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4749fceead5c94370633a6158c603619 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1764ee1f99ceca4d54129aad1f62447 = $(`<div id="html_c1764ee1f99ceca4d54129aad1f62447" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4749fceead5c94370633a6158c603619.setContent(html_c1764ee1f99ceca4d54129aad1f62447);\\n \\n \\n\\n circle_marker_e88b0b6a7f1aa8115739854abf1ebeba.bindPopup(popup_4749fceead5c94370633a6158c603619)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e747def9aea824373e69681ce6be614 = L.circleMarker(\\n [42.74358697111428, -73.26733253452443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.046265044040321, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_845c76e48996feb6e0ac6ca16e945b4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6506faee2919d451d9bbb24ea36d1a6 = $(`<div id="html_f6506faee2919d451d9bbb24ea36d1a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_845c76e48996feb6e0ac6ca16e945b4e.setContent(html_f6506faee2919d451d9bbb24ea36d1a6);\\n \\n \\n\\n circle_marker_0e747def9aea824373e69681ce6be614.bindPopup(popup_845c76e48996feb6e0ac6ca16e945b4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d2ec64d01243ae4ee8211f7945b2107 = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8c79c0a56500d130a832fb4f1983c61e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67c98b0d6fef145be73609ebe79ac04d = $(`<div id="html_67c98b0d6fef145be73609ebe79ac04d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c79c0a56500d130a832fb4f1983c61e.setContent(html_67c98b0d6fef145be73609ebe79ac04d);\\n \\n \\n\\n circle_marker_1d2ec64d01243ae4ee8211f7945b2107.bindPopup(popup_8c79c0a56500d130a832fb4f1983c61e)\\n ;\\n\\n \\n \\n \\n var circle_marker_33f53701936c50b0d2b09bc3b55a6ac5 = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0910033654c886c771fe4133f2f65bcc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e2f71c68bd493e5c1c5abab333e8eaa = $(`<div id="html_4e2f71c68bd493e5c1c5abab333e8eaa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0910033654c886c771fe4133f2f65bcc.setContent(html_4e2f71c68bd493e5c1c5abab333e8eaa);\\n \\n \\n\\n circle_marker_33f53701936c50b0d2b09bc3b55a6ac5.bindPopup(popup_0910033654c886c771fe4133f2f65bcc)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d757b7bbcbb7959c2c3d71b5295028d = L.circleMarker(\\n [42.74358699086881, -73.26364148650833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_fdc7416120237e845a71634deb0dc2c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ae5835b2ca8c1df2b666c3082707d98 = $(`<div id="html_4ae5835b2ca8c1df2b666c3082707d98" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fdc7416120237e845a71634deb0dc2c7.setContent(html_4ae5835b2ca8c1df2b666c3082707d98);\\n \\n \\n\\n circle_marker_6d757b7bbcbb7959c2c3d71b5295028d.bindPopup(popup_fdc7416120237e845a71634deb0dc2c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_89fcfe8d907c1bcbcd67d64c43f1673c = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cf8752f03f060a4dde4d913a5f9dfedd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_124460b5ab22c839da6756ec7bc4a623 = $(`<div id="html_124460b5ab22c839da6756ec7bc4a623" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf8752f03f060a4dde4d913a5f9dfedd.setContent(html_124460b5ab22c839da6756ec7bc4a623);\\n \\n \\n\\n circle_marker_89fcfe8d907c1bcbcd67d64c43f1673c.bindPopup(popup_cf8752f03f060a4dde4d913a5f9dfedd)\\n ;\\n\\n \\n \\n \\n var circle_marker_c237a25a8a22fe39de3a800ad095ca9c = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_42703defce9680ad08d501890cedb2db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54fbfc812eda4e0fe7c24707cad558c7 = $(`<div id="html_54fbfc812eda4e0fe7c24707cad558c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_42703defce9680ad08d501890cedb2db.setContent(html_54fbfc812eda4e0fe7c24707cad558c7);\\n \\n \\n\\n circle_marker_c237a25a8a22fe39de3a800ad095ca9c.bindPopup(popup_42703defce9680ad08d501890cedb2db)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6edea39ccce1cdb110821c441c60bc0 = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_36432f0eb3cf8e0e637df5880979a0fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82b032a207f0597bcc65680e2029a12e = $(`<div id="html_82b032a207f0597bcc65680e2029a12e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36432f0eb3cf8e0e637df5880979a0fb.setContent(html_82b032a207f0597bcc65680e2029a12e);\\n \\n \\n\\n circle_marker_b6edea39ccce1cdb110821c441c60bc0.bindPopup(popup_36432f0eb3cf8e0e637df5880979a0fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_58ab2da2f8b39f33d85f2180532ebdfb = L.circleMarker(\\n [42.74358683283254, -73.25872008916333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0f3922dc1a6da9d3f471592ae6ba0242 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1abaf19cb70a5ff349d34fcb237675e0 = $(`<div id="html_1abaf19cb70a5ff349d34fcb237675e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f3922dc1a6da9d3f471592ae6ba0242.setContent(html_1abaf19cb70a5ff349d34fcb237675e0);\\n \\n \\n\\n circle_marker_58ab2da2f8b39f33d85f2180532ebdfb.bindPopup(popup_0f3922dc1a6da9d3f471592ae6ba0242)\\n ;\\n\\n \\n \\n \\n var circle_marker_973b05da5171d3dde17a84069ae29b30 = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.222008245644752, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b326e7126c499911594b865e0aabc00c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7f109d8c3023674bdefae4313c2bf2e = $(`<div id="html_f7f109d8c3023674bdefae4313c2bf2e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b326e7126c499911594b865e0aabc00c.setContent(html_f7f109d8c3023674bdefae4313c2bf2e);\\n \\n \\n\\n circle_marker_973b05da5171d3dde17a84069ae29b30.bindPopup(popup_b326e7126c499911594b865e0aabc00c)\\n ;\\n\\n \\n \\n \\n var circle_marker_f31b34f620517658edde83fccb6283b8 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4b58fb29c179806722c07f5d90251295 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83e8e08042663012817386923d5de52f = $(`<div id="html_83e8e08042663012817386923d5de52f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4b58fb29c179806722c07f5d90251295.setContent(html_83e8e08042663012817386923d5de52f);\\n \\n \\n\\n circle_marker_f31b34f620517658edde83fccb6283b8.bindPopup(popup_4b58fb29c179806722c07f5d90251295)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d01ff0b5dbe54550ac8712216ff7173 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.698035485213008, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_736b87905d879b5e05b81a1dd9c4b4f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e8718258edeb9eee32a71a1421bc7f0b = $(`<div id="html_e8718258edeb9eee32a71a1421bc7f0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_736b87905d879b5e05b81a1dd9c4b4f1.setContent(html_e8718258edeb9eee32a71a1421bc7f0b);\\n \\n \\n\\n circle_marker_9d01ff0b5dbe54550ac8712216ff7173.bindPopup(popup_736b87905d879b5e05b81a1dd9c4b4f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_1dc89c6ce6e3cb2da03d550c5beae9aa = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_61c73c7d2cad1ef11bfb2b8dab7c5d71 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b95348217c65572657a679020b777c4 = $(`<div id="html_2b95348217c65572657a679020b777c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61c73c7d2cad1ef11bfb2b8dab7c5d71.setContent(html_2b95348217c65572657a679020b777c4);\\n \\n \\n\\n circle_marker_1dc89c6ce6e3cb2da03d550c5beae9aa.bindPopup(popup_61c73c7d2cad1ef11bfb2b8dab7c5d71)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8b46cd42519c69fc4d0bb8984df768f = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cf922ec28d55a694e98fdcfb317ca2f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92a0d0ef86b6acc212f268bb6a1c813a = $(`<div id="html_92a0d0ef86b6acc212f268bb6a1c813a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf922ec28d55a694e98fdcfb317ca2f4.setContent(html_92a0d0ef86b6acc212f268bb6a1c813a);\\n \\n \\n\\n circle_marker_c8b46cd42519c69fc4d0bb8984df768f.bindPopup(popup_cf922ec28d55a694e98fdcfb317ca2f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_cccbca1d52e273d1ea27388bdf645a05 = L.circleMarker(\\n [42.7417795414353, -73.27348403014923],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cb05648b9e03138d9083346b7b295ebc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cdc4188543a4d950ba19467c42687963 = $(`<div id="html_cdc4188543a4d950ba19467c42687963" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb05648b9e03138d9083346b7b295ebc.setContent(html_cdc4188543a4d950ba19467c42687963);\\n \\n \\n\\n circle_marker_cccbca1d52e273d1ea27388bdf645a05.bindPopup(popup_cb05648b9e03138d9083346b7b295ebc)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5db5b9b4cdb45dd0d7c1dcf23608ab4 = L.circleMarker(\\n [42.74177962703481, -73.2722537166843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.8678889139475245, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c9054763f1811bb31e35b1d38497ebe1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_561519f8412812a0cb16140053d52dd8 = $(`<div id="html_561519f8412812a0cb16140053d52dd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9054763f1811bb31e35b1d38497ebe1.setContent(html_561519f8412812a0cb16140053d52dd8);\\n \\n \\n\\n circle_marker_d5db5b9b4cdb45dd0d7c1dcf23608ab4.bindPopup(popup_c9054763f1811bb31e35b1d38497ebe1)\\n ;\\n\\n \\n \\n \\n var circle_marker_c500662e456b54df1aded8c7c6b67116 = L.circleMarker(\\n [42.74177969946516, -73.27102340321625],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b3e9a2a8956ecbbaa79e76fd2886c4cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cdfd14cb86bae0b34dc92a66e0d43183 = $(`<div id="html_cdfd14cb86bae0b34dc92a66e0d43183" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3e9a2a8956ecbbaa79e76fd2886c4cb.setContent(html_cdfd14cb86bae0b34dc92a66e0d43183);\\n \\n \\n\\n circle_marker_c500662e456b54df1aded8c7c6b67116.bindPopup(popup_b3e9a2a8956ecbbaa79e76fd2886c4cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_264682f2b3b1615ed32b9d432cce79f9 = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_920a24a0c61aeb308aa0fcf29403cac9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d0e5f4c6cfeca8997a09dd0c9805ed85 = $(`<div id="html_d0e5f4c6cfeca8997a09dd0c9805ed85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_920a24a0c61aeb308aa0fcf29403cac9.setContent(html_d0e5f4c6cfeca8997a09dd0c9805ed85);\\n \\n \\n\\n circle_marker_264682f2b3b1615ed32b9d432cce79f9.bindPopup(popup_920a24a0c61aeb308aa0fcf29403cac9)\\n ;\\n\\n \\n \\n \\n var circle_marker_4650314fb499a674d0e35f38b14fa925 = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1683b010e362178cd7d8e600f4cf3589 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cef7a1faf540c3e4f7d5d9318a3f126 = $(`<div id="html_9cef7a1faf540c3e4f7d5d9318a3f126" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1683b010e362178cd7d8e600f4cf3589.setContent(html_9cef7a1faf540c3e4f7d5d9318a3f126);\\n \\n \\n\\n circle_marker_4650314fb499a674d0e35f38b14fa925.bindPopup(popup_1683b010e362178cd7d8e600f4cf3589)\\n ;\\n\\n \\n \\n \\n var circle_marker_d087a5d09b468e3262877ce2cbd0d49a = L.circleMarker(\\n [42.74177983774129, -73.26733246279849],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_605d6928970a51315f50c1ada64c71e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe67219a7923dec7f439b4e0c138a981 = $(`<div id="html_fe67219a7923dec7f439b4e0c138a981" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_605d6928970a51315f50c1ada64c71e2.setContent(html_fe67219a7923dec7f439b4e0c138a981);\\n \\n \\n\\n circle_marker_d087a5d09b468e3262877ce2cbd0d49a.bindPopup(popup_605d6928970a51315f50c1ada64c71e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_4fe828d33b99556ea8f4698d88d67b30 = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e1e9dd3da74ce522d12c59abc4011f75 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25165a609bcd1d49e71d205642a15b87 = $(`<div id="html_25165a609bcd1d49e71d205642a15b87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1e9dd3da74ce522d12c59abc4011f75.setContent(html_25165a609bcd1d49e71d205642a15b87);\\n \\n \\n\\n circle_marker_4fe828d33b99556ea8f4698d88d67b30.bindPopup(popup_e1e9dd3da74ce522d12c59abc4011f75)\\n ;\\n\\n \\n \\n \\n var circle_marker_09d9fc6eedbc0573b33920e60f9dfb89 = L.circleMarker(\\n [42.7417798640796, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.585191925620057, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_81398242dc870f3e55c5e3ebd0b055a8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a9398606b7e78d290f80fe7a06fcbae = $(`<div id="html_8a9398606b7e78d290f80fe7a06fcbae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81398242dc870f3e55c5e3ebd0b055a8.setContent(html_8a9398606b7e78d290f80fe7a06fcbae);\\n \\n \\n\\n circle_marker_09d9fc6eedbc0573b33920e60f9dfb89.bindPopup(popup_81398242dc870f3e55c5e3ebd0b055a8)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0ef1dee25c03bfbe8b11befaff65e4b = L.circleMarker(\\n [42.74177985749502, -73.26364152237132],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4c113ddba09474ebc1e2c088df0fce3d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc4132be6ee9da5f2cd41efac8307f38 = $(`<div id="html_cc4132be6ee9da5f2cd41efac8307f38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c113ddba09474ebc1e2c088df0fce3d.setContent(html_cc4132be6ee9da5f2cd41efac8307f38);\\n \\n \\n\\n circle_marker_d0ef1dee25c03bfbe8b11befaff65e4b.bindPopup(popup_4c113ddba09474ebc1e2c088df0fce3d)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa4080259ae5b773c220ae8c4851f83e = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d8c2f3bdac9d9bcdf26ce2743cd4b411 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6be692fd15e373f8e900b746f779de58 = $(`<div id="html_6be692fd15e373f8e900b746f779de58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8c2f3bdac9d9bcdf26ce2743cd4b411.setContent(html_6be692fd15e373f8e900b746f779de58);\\n \\n \\n\\n circle_marker_fa4080259ae5b773c220ae8c4851f83e.bindPopup(popup_d8c2f3bdac9d9bcdf26ce2743cd4b411)\\n ;\\n\\n \\n \\n \\n var circle_marker_bca80d7488d8016a483ad357f8dc6234 = L.circleMarker(\\n [42.7417798048184, -73.2611808954216],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_69e1b0f6d130f51541a3cdb219870a69 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d2e4d703ef4fdf69713488b1c52e990 = $(`<div id="html_7d2e4d703ef4fdf69713488b1c52e990" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69e1b0f6d130f51541a3cdb219870a69.setContent(html_7d2e4d703ef4fdf69713488b1c52e990);\\n \\n \\n\\n circle_marker_bca80d7488d8016a483ad357f8dc6234.bindPopup(popup_69e1b0f6d130f51541a3cdb219870a69)\\n ;\\n\\n \\n \\n \\n var circle_marker_779ad7233ec4c879d8f752312424554b = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.014626706796775, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f739b060a3f8e8d265bc6bfb16e25b11 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb222f43992e00ed910f892d036dfc6e = $(`<div id="html_bb222f43992e00ed910f892d036dfc6e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f739b060a3f8e8d265bc6bfb16e25b11.setContent(html_bb222f43992e00ed910f892d036dfc6e);\\n \\n \\n\\n circle_marker_779ad7233ec4c879d8f752312424554b.bindPopup(popup_f739b060a3f8e8d265bc6bfb16e25b11)\\n ;\\n\\n \\n \\n \\n var circle_marker_876ee55d80572b54978d918a579a7ef8 = L.circleMarker(\\n [42.74177969946516, -73.25872026847817],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e0d5ad44a310d0b457d2c918f860f9e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae834365f2c4edec78e057081e6de65a = $(`<div id="html_ae834365f2c4edec78e057081e6de65a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0d5ad44a310d0b457d2c918f860f9e2.setContent(html_ae834365f2c4edec78e057081e6de65a);\\n \\n \\n\\n circle_marker_876ee55d80572b54978d918a579a7ef8.bindPopup(popup_e0d5ad44a310d0b457d2c918f860f9e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_2420ef1e4a68cc81f09c35f124a5b8ba = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0d06515cdab971ffb06ad60e072f30e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_84fd10f5b7544d5f1ba226694dc5ced3 = $(`<div id="html_84fd10f5b7544d5f1ba226694dc5ced3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d06515cdab971ffb06ad60e072f30e7.setContent(html_84fd10f5b7544d5f1ba226694dc5ced3);\\n \\n \\n\\n circle_marker_2420ef1e4a68cc81f09c35f124a5b8ba.bindPopup(popup_0d06515cdab971ffb06ad60e072f30e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_0cb88109c0127269754fc9b31cfcd224 = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d970679af89002c0011eca177ba19466 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64b5eb7ffb1b405068a332e88267ef94 = $(`<div id="html_64b5eb7ffb1b405068a332e88267ef94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d970679af89002c0011eca177ba19466.setContent(html_64b5eb7ffb1b405068a332e88267ef94);\\n \\n \\n\\n circle_marker_0cb88109c0127269754fc9b31cfcd224.bindPopup(popup_d970679af89002c0011eca177ba19466)\\n ;\\n\\n \\n \\n \\n var circle_marker_33d1218f3d4e895525593cfc376944bf = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0a5e4e7792e97ed30f2f5c7af99f9958 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_596e013e87b25cbb86da64df41012ea5 = $(`<div id="html_596e013e87b25cbb86da64df41012ea5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a5e4e7792e97ed30f2f5c7af99f9958.setContent(html_596e013e87b25cbb86da64df41012ea5);\\n \\n \\n\\n circle_marker_33d1218f3d4e895525593cfc376944bf.bindPopup(popup_0a5e4e7792e97ed30f2f5c7af99f9958)\\n ;\\n\\n \\n \\n \\n var circle_marker_b675cffd4ab592cdd86b08a1f2597493 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_726244b2a053ea4c425fa8dd7f44686e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_093faf05edc5804bd4780fa42651e7ca = $(`<div id="html_093faf05edc5804bd4780fa42651e7ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_726244b2a053ea4c425fa8dd7f44686e.setContent(html_093faf05edc5804bd4780fa42651e7ca);\\n \\n \\n\\n circle_marker_b675cffd4ab592cdd86b08a1f2597493.bindPopup(popup_726244b2a053ea4c425fa8dd7f44686e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a94b8abede3429780e74af39e1a54e0e = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_de29019aa8942a72ba1bdb33b6928c30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1a5fe92a15292230fbd3fc1779df001 = $(`<div id="html_b1a5fe92a15292230fbd3fc1779df001" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de29019aa8942a72ba1bdb33b6928c30.setContent(html_b1a5fe92a15292230fbd3fc1779df001);\\n \\n \\n\\n circle_marker_a94b8abede3429780e74af39e1a54e0e.bindPopup(popup_de29019aa8942a72ba1bdb33b6928c30)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6b17386262d07d1ef2d8d2375e55038 = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_74f30baa360cb063e510b6a6556a9cc0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8cf6a3e282fb45341c731fc88ea3e9e1 = $(`<div id="html_8cf6a3e282fb45341c731fc88ea3e9e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_74f30baa360cb063e510b6a6556a9cc0.setContent(html_8cf6a3e282fb45341c731fc88ea3e9e1);\\n \\n \\n\\n circle_marker_e6b17386262d07d1ef2d8d2375e55038.bindPopup(popup_74f30baa360cb063e510b6a6556a9cc0)\\n ;\\n\\n \\n \\n \\n var circle_marker_c96306f3c6656e593a861b3d91a92a6c = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d57d4e2e67e15be9dca5ebb6676beb77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7033b41278d5ad2a10396668b0bb4b5 = $(`<div id="html_c7033b41278d5ad2a10396668b0bb4b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d57d4e2e67e15be9dca5ebb6676beb77.setContent(html_c7033b41278d5ad2a10396668b0bb4b5);\\n \\n \\n\\n circle_marker_c96306f3c6656e593a861b3d91a92a6c.bindPopup(popup_d57d4e2e67e15be9dca5ebb6676beb77)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc00e06ec257599e6415849b609b2f4a = L.circleMarker(\\n [42.73997267144673, -73.26856266869262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.970821321441846, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2382354d8d70ed5ef24ab270aa61575f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c16a7fba1f81e22edbc02c24930d6ff8 = $(`<div id="html_c16a7fba1f81e22edbc02c24930d6ff8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2382354d8d70ed5ef24ab270aa61575f.setContent(html_c16a7fba1f81e22edbc02c24930d6ff8);\\n \\n \\n\\n circle_marker_cc00e06ec257599e6415849b609b2f4a.bindPopup(popup_2382354d8d70ed5ef24ab270aa61575f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f55e00731a3898b8def48934b5634218 = L.circleMarker(\\n [42.73997270436829, -73.26733239107837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.583497844237541, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ed9370ea5dea39d518251c93b5b404f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1657ce35be61e81c454507b42e2d389 = $(`<div id="html_a1657ce35be61e81c454507b42e2d389" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed9370ea5dea39d518251c93b5b404f0.setContent(html_a1657ce35be61e81c454507b42e2d389);\\n \\n \\n\\n circle_marker_f55e00731a3898b8def48934b5634218.bindPopup(popup_ed9370ea5dea39d518251c93b5b404f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_89791de20c966c1f62d046c1daeaf370 = L.circleMarker(\\n [42.73997272412122, -73.26610211346305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_23225fbe7f2db0eb38588272f3202374 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_588089f66d8a4d93641bd50dbc322a8d = $(`<div id="html_588089f66d8a4d93641bd50dbc322a8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_23225fbe7f2db0eb38588272f3202374.setContent(html_588089f66d8a4d93641bd50dbc322a8d);\\n \\n \\n\\n circle_marker_89791de20c966c1f62d046c1daeaf370.bindPopup(popup_23225fbe7f2db0eb38588272f3202374)\\n ;\\n\\n \\n \\n \\n var circle_marker_a98a37f534268f9f930bc31d06309496 = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6c84665e424219613da5f2655f8805ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2f9af5df31f9c412f0eca587075d0e2 = $(`<div id="html_d2f9af5df31f9c412f0eca587075d0e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c84665e424219613da5f2655f8805ae.setContent(html_d2f9af5df31f9c412f0eca587075d0e2);\\n \\n \\n\\n circle_marker_a98a37f534268f9f930bc31d06309496.bindPopup(popup_6c84665e424219613da5f2655f8805ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_e5c2837356f69e2465bb48feb7129f67 = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9262fd35bc318179195481718d76230b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e93dcc14d9834227cb1c42789a97c61 = $(`<div id="html_8e93dcc14d9834227cb1c42789a97c61" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9262fd35bc318179195481718d76230b.setContent(html_8e93dcc14d9834227cb1c42789a97c61);\\n \\n \\n\\n circle_marker_e5c2837356f69e2465bb48feb7129f67.bindPopup(popup_9262fd35bc318179195481718d76230b)\\n ;\\n\\n \\n \\n \\n var circle_marker_af71e8cdc2c675852ee9d74a0cded027 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_467d38c5c0e01c56cc67aa6e4870c00d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf86e93dca9e6d032a9803421926bd89 = $(`<div id="html_cf86e93dca9e6d032a9803421926bd89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_467d38c5c0e01c56cc67aa6e4870c00d.setContent(html_cf86e93dca9e6d032a9803421926bd89);\\n \\n \\n\\n circle_marker_af71e8cdc2c675852ee9d74a0cded027.bindPopup(popup_467d38c5c0e01c56cc67aa6e4870c00d)\\n ;\\n\\n \\n \\n \\n var circle_marker_dae2af56738d07a124594a948b4d189a = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.583497844237541, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a230430a12efceb586244735a6d7689c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50eac048592538f49edf5773af848e6a = $(`<div id="html_50eac048592538f49edf5773af848e6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a230430a12efceb586244735a6d7689c.setContent(html_50eac048592538f49edf5773af848e6a);\\n \\n \\n\\n circle_marker_dae2af56738d07a124594a948b4d189a.bindPopup(popup_a230430a12efceb586244735a6d7689c)\\n ;\\n\\n \\n \\n \\n var circle_marker_775476ede0d0d00deb0ed8402e7cea05 = L.circleMarker(\\n [42.73997262535657, -73.25995072538909],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1d80d287a6a01fe876b9cce3a22da2a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4cfc5ddbc826f51181884ff13c62405 = $(`<div id="html_d4cfc5ddbc826f51181884ff13c62405" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d80d287a6a01fe876b9cce3a22da2a1.setContent(html_d4cfc5ddbc826f51181884ff13c62405);\\n \\n \\n\\n circle_marker_775476ede0d0d00deb0ed8402e7cea05.bindPopup(popup_1d80d287a6a01fe876b9cce3a22da2a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_a35803412625ff2fb0c3da1084a6fe68 = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.3336224206596095, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cae538fe2ba675067b3adb700806e4e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_851d8ec5cf5a3da82a217d1ab136bd11 = $(`<div id="html_851d8ec5cf5a3da82a217d1ab136bd11" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cae538fe2ba675067b3adb700806e4e5.setContent(html_851d8ec5cf5a3da82a217d1ab136bd11);\\n \\n \\n\\n circle_marker_a35803412625ff2fb0c3da1084a6fe68.bindPopup(popup_cae538fe2ba675067b3adb700806e4e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c2ee5c758211ff52313365c65b8bba9e = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.820437914901168, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9781f34ab5c3bf8101937ea059d34f29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7fe335e8ec56d152809cdb60b5b2ec0c = $(`<div id="html_7fe335e8ec56d152809cdb60b5b2ec0c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9781f34ab5c3bf8101937ea059d34f29.setContent(html_7fe335e8ec56d152809cdb60b5b2ec0c);\\n \\n \\n\\n circle_marker_c2ee5c758211ff52313365c65b8bba9e.bindPopup(popup_9781f34ab5c3bf8101937ea059d34f29)\\n ;\\n\\n \\n \\n \\n var circle_marker_f915d41ab2cea5191848deb97cc6e285 = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_42d1f83c96cd8212467b01fd0bf8adb9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3a2c155f01813e284b707e5ed9788dd = $(`<div id="html_e3a2c155f01813e284b707e5ed9788dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_42d1f83c96cd8212467b01fd0bf8adb9.setContent(html_e3a2c155f01813e284b707e5ed9788dd);\\n \\n \\n\\n circle_marker_f915d41ab2cea5191848deb97cc6e285.bindPopup(popup_42d1f83c96cd8212467b01fd0bf8adb9)\\n ;\\n\\n \\n \\n \\n var circle_marker_0db3a2523f58dd12bafe81fe175128a6 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5fbb6b57587823828fa79a6bde3a20b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_498614d134ee884d16da85079cf1dc47 = $(`<div id="html_498614d134ee884d16da85079cf1dc47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5fbb6b57587823828fa79a6bde3a20b9.setContent(html_498614d134ee884d16da85079cf1dc47);\\n \\n \\n\\n circle_marker_0db3a2523f58dd12bafe81fe175128a6.bindPopup(popup_5fbb6b57587823828fa79a6bde3a20b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c64a1c2aab34b179feb11c618c529d7 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_aa51a47fe0b3f102446630910894ebc3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86b0b5e3dff0bf7b3ef4861f339cddce = $(`<div id="html_86b0b5e3dff0bf7b3ef4861f339cddce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aa51a47fe0b3f102446630910894ebc3.setContent(html_86b0b5e3dff0bf7b3ef4861f339cddce);\\n \\n \\n\\n circle_marker_8c64a1c2aab34b179feb11c618c529d7.bindPopup(popup_aa51a47fe0b3f102446630910894ebc3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5440268d6fadcbc8e7b92d936ef78db = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_fd20dbe337f8f91de0f7a5b449c29cc9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41b3da6d95447bc791640bf0bf24bddc = $(`<div id="html_41b3da6d95447bc791640bf0bf24bddc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd20dbe337f8f91de0f7a5b449c29cc9.setContent(html_41b3da6d95447bc791640bf0bf24bddc);\\n \\n \\n\\n circle_marker_d5440268d6fadcbc8e7b92d936ef78db.bindPopup(popup_fd20dbe337f8f91de0f7a5b449c29cc9)\\n ;\\n\\n \\n \\n \\n var circle_marker_8247f3173c998985bcae723077b78ddd = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_eb8b1e7b0f847ae395ab06ee40b61f9f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e308196c5226b977e883f9305277d17 = $(`<div id="html_0e308196c5226b977e883f9305277d17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb8b1e7b0f847ae395ab06ee40b61f9f.setContent(html_0e308196c5226b977e883f9305277d17);\\n \\n \\n\\n circle_marker_8247f3173c998985bcae723077b78ddd.bindPopup(popup_eb8b1e7b0f847ae395ab06ee40b61f9f)\\n ;\\n\\n \\n \\n \\n var circle_marker_0685d7276ae1635c9444080b5722d086 = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1395e6a24c54b1ca2eb845a1478de2d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78ad4d1d72a4d9efa1d203a8d2ac3d4f = $(`<div id="html_78ad4d1d72a4d9efa1d203a8d2ac3d4f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1395e6a24c54b1ca2eb845a1478de2d9.setContent(html_78ad4d1d72a4d9efa1d203a8d2ac3d4f);\\n \\n \\n\\n circle_marker_0685d7276ae1635c9444080b5722d086.bindPopup(popup_1395e6a24c54b1ca2eb845a1478de2d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce4d0ba5a559e029917973e6809110b7 = L.circleMarker(\\n [42.73816553807507, -73.26856256112116],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.322553886092406, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9cadcef53d5a2f169873bc3c49b48d21 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_29c5ae3eb1d8cc120702c31b199fbf15 = $(`<div id="html_29c5ae3eb1d8cc120702c31b199fbf15" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9cadcef53d5a2f169873bc3c49b48d21.setContent(html_29c5ae3eb1d8cc120702c31b199fbf15);\\n \\n \\n\\n circle_marker_ce4d0ba5a559e029917973e6809110b7.bindPopup(popup_9cadcef53d5a2f169873bc3c49b48d21)\\n ;\\n\\n \\n \\n \\n var circle_marker_131aa3a7ca27f097a76e70a65776420b = L.circleMarker(\\n [42.7381655709953, -73.26733231936404],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.046265044040321, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_db171efa2c5cc5198808404961a9cdf1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57b8429495dff987a0e503c134511974 = $(`<div id="html_57b8429495dff987a0e503c134511974" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db171efa2c5cc5198808404961a9cdf1.setContent(html_57b8429495dff987a0e503c134511974);\\n \\n \\n\\n circle_marker_131aa3a7ca27f097a76e70a65776420b.bindPopup(popup_db171efa2c5cc5198808404961a9cdf1)\\n ;\\n\\n \\n \\n \\n var circle_marker_98128207f40ae737245013b3f9cfcca1 = L.circleMarker(\\n [42.73816559074744, -73.2661020776059],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.4820448144285745, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_729b1ca85f748eb7d9a058e736359b4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64f29f1bf8a9b63adf3ac3d9f52fc7b0 = $(`<div id="html_64f29f1bf8a9b63adf3ac3d9f52fc7b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_729b1ca85f748eb7d9a058e736359b4b.setContent(html_64f29f1bf8a9b63adf3ac3d9f52fc7b0);\\n \\n \\n\\n circle_marker_98128207f40ae737245013b3f9cfcca1.bindPopup(popup_729b1ca85f748eb7d9a058e736359b4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_230fc071a2071a48d2e106b426f1382b = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.527906391541368, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_efbf17d35039bdae0bf981e234269c43 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_daab2a4820d3f329838e7edbda7d42f4 = $(`<div id="html_daab2a4820d3f329838e7edbda7d42f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_efbf17d35039bdae0bf981e234269c43.setContent(html_daab2a4820d3f329838e7edbda7d42f4);\\n \\n \\n\\n circle_marker_230fc071a2071a48d2e106b426f1382b.bindPopup(popup_efbf17d35039bdae0bf981e234269c43)\\n ;\\n\\n \\n \\n \\n var circle_marker_a64122ccafaaad9cd53b725ff889bfe3 = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b48152dfb63383732be2eb5ecbd640f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f791ce75b1dfa9264e03943c11f3064c = $(`<div id="html_f791ce75b1dfa9264e03943c11f3064c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b48152dfb63383732be2eb5ecbd640f5.setContent(html_f791ce75b1dfa9264e03943c11f3064c);\\n \\n \\n\\n circle_marker_a64122ccafaaad9cd53b725ff889bfe3.bindPopup(popup_b48152dfb63383732be2eb5ecbd640f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_022a727ad91de7c2b4ca761cbf68972b = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1d465340ab1001f7017a461444698268 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68b8f3af8840a2a15bbad032df2dd9d2 = $(`<div id="html_68b8f3af8840a2a15bbad032df2dd9d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d465340ab1001f7017a461444698268.setContent(html_68b8f3af8840a2a15bbad032df2dd9d2);\\n \\n \\n\\n circle_marker_022a727ad91de7c2b4ca761cbf68972b.bindPopup(popup_1d465340ab1001f7017a461444698268)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a90915a0ff262835d81844b3b76cbb1 = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1ec5cf75d3c5653b0d0ec6118bf512d2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be0bdf295a9434b3a73a0a633744a412 = $(`<div id="html_be0bdf295a9434b3a73a0a633744a412" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ec5cf75d3c5653b0d0ec6118bf512d2.setContent(html_be0bdf295a9434b3a73a0a633744a412);\\n \\n \\n\\n circle_marker_1a90915a0ff262835d81844b3b76cbb1.bindPopup(popup_1ec5cf75d3c5653b0d0ec6118bf512d2)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1f85bec109096fff683845597415e76 = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_11aee231084a02fb1510209ac5a416ad = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c433493fbd572ea3a39cdffa37f53b8d = $(`<div id="html_c433493fbd572ea3a39cdffa37f53b8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11aee231084a02fb1510209ac5a416ad.setContent(html_c433493fbd572ea3a39cdffa37f53b8d);\\n \\n \\n\\n circle_marker_b1f85bec109096fff683845597415e76.bindPopup(popup_11aee231084a02fb1510209ac5a416ad)\\n ;\\n\\n \\n \\n \\n var circle_marker_0414e9eaf4b8b61a7443b307d00e1e35 = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0d0e0f67d9ca6a5d95be05461668ea70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6fa4cf9e84a3ca4ccd8e3605ba2aedd = $(`<div id="html_f6fa4cf9e84a3ca4ccd8e3605ba2aedd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d0e0f67d9ca6a5d95be05461668ea70.setContent(html_f6fa4cf9e84a3ca4ccd8e3605ba2aedd);\\n \\n \\n\\n circle_marker_0414e9eaf4b8b61a7443b307d00e1e35.bindPopup(popup_0d0e0f67d9ca6a5d95be05461668ea70)\\n ;\\n\\n \\n \\n \\n var circle_marker_b93d02848efad99001aa2f05348bedad = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f6a93e85d8ac534edce4e642be5c7469 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83955efad3b390984ece679aa89f834f = $(`<div id="html_83955efad3b390984ece679aa89f834f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6a93e85d8ac534edce4e642be5c7469.setContent(html_83955efad3b390984ece679aa89f834f);\\n \\n \\n\\n circle_marker_b93d02848efad99001aa2f05348bedad.bindPopup(popup_f6a93e85d8ac534edce4e642be5c7469)\\n ;\\n\\n \\n \\n \\n var circle_marker_9cb168a82266eb0df09db0e13b280099 = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_72e08157309352a60a1c4c52253c30f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e41b48f7a4a5ff8a16ea8d3603d495fe = $(`<div id="html_e41b48f7a4a5ff8a16ea8d3603d495fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72e08157309352a60a1c4c52253c30f2.setContent(html_e41b48f7a4a5ff8a16ea8d3603d495fe);\\n \\n \\n\\n circle_marker_9cb168a82266eb0df09db0e13b280099.bindPopup(popup_72e08157309352a60a1c4c52253c30f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_53e1a23f0d95a2b713a3bb1dd6253ea9 = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b1735fc41a4a9eedd80b0cd29cb42016 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f539891db632f1edf24c3e63d949609 = $(`<div id="html_6f539891db632f1edf24c3e63d949609" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1735fc41a4a9eedd80b0cd29cb42016.setContent(html_6f539891db632f1edf24c3e63d949609);\\n \\n \\n\\n circle_marker_53e1a23f0d95a2b713a3bb1dd6253ea9.bindPopup(popup_b1735fc41a4a9eedd80b0cd29cb42016)\\n ;\\n\\n \\n \\n \\n var circle_marker_04ff26c8e7e6bdf95d63b2665fb13591 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.420755373261929, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b3def08f6e8e18ec0df0aa565423e206 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c4a0ed614f27dec3095091eab10d4005 = $(`<div id="html_c4a0ed614f27dec3095091eab10d4005" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3def08f6e8e18ec0df0aa565423e206.setContent(html_c4a0ed614f27dec3095091eab10d4005);\\n \\n \\n\\n circle_marker_04ff26c8e7e6bdf95d63b2665fb13591.bindPopup(popup_b3def08f6e8e18ec0df0aa565423e206)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c2ff3e743ab16ea821d3fae300a48e0 = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4bb7d986b5e05a885fe3f6d6543070e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7402a9f8b25db1d144789ad8cf60261 = $(`<div id="html_c7402a9f8b25db1d144789ad8cf60261" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4bb7d986b5e05a885fe3f6d6543070e9.setContent(html_c7402a9f8b25db1d144789ad8cf60261);\\n \\n \\n\\n circle_marker_8c2ff3e743ab16ea821d3fae300a48e0.bindPopup(popup_4bb7d986b5e05a885fe3f6d6543070e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_1198711cd491e10d96cbf6e7e2161269 = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b7efbd4fcbad04ff692a9d42ededf0fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce637c6e55c4e9dac84ef4047ed9e5e5 = $(`<div id="html_ce637c6e55c4e9dac84ef4047ed9e5e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b7efbd4fcbad04ff692a9d42ededf0fc.setContent(html_ce637c6e55c4e9dac84ef4047ed9e5e5);\\n \\n \\n\\n circle_marker_1198711cd491e10d96cbf6e7e2161269.bindPopup(popup_b7efbd4fcbad04ff692a9d42ededf0fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea9c52929e272b5bc245caf5e60c0aa7 = L.circleMarker(\\n [42.736358404703424, -73.2685624535584],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f77e485f1b4e8ff0fd1740caa20cfa6d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_905162a0cf0a38e0b1f0683470a8a047 = $(`<div id="html_905162a0cf0a38e0b1f0683470a8a047" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f77e485f1b4e8ff0fd1740caa20cfa6d.setContent(html_905162a0cf0a38e0b1f0683470a8a047);\\n \\n \\n\\n circle_marker_ea9c52929e272b5bc245caf5e60c0aa7.bindPopup(popup_f77e485f1b4e8ff0fd1740caa20cfa6d)\\n ;\\n\\n \\n \\n \\n var circle_marker_4517b9899947a95cf57819c7f1debfdf = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2fa2a4469caa84d6f582c554b0008dea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_066f7e0f9b6d47867284a04a6c285556 = $(`<div id="html_066f7e0f9b6d47867284a04a6c285556" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fa2a4469caa84d6f582c554b0008dea.setContent(html_066f7e0f9b6d47867284a04a6c285556);\\n \\n \\n\\n circle_marker_4517b9899947a95cf57819c7f1debfdf.bindPopup(popup_2fa2a4469caa84d6f582c554b0008dea)\\n ;\\n\\n \\n \\n \\n var circle_marker_4de1b10568c49bb20c9019845503ed5f = L.circleMarker(\\n [42.73635846395741, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.949327084834294, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5e80c09f9d7d254cd1f2a4fc3b0e5828 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a5f1bd3e0104bf0ea82958e83740161 = $(`<div id="html_8a5f1bd3e0104bf0ea82958e83740161" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e80c09f9d7d254cd1f2a4fc3b0e5828.setContent(html_8a5f1bd3e0104bf0ea82958e83740161);\\n \\n \\n\\n circle_marker_4de1b10568c49bb20c9019845503ed5f.bindPopup(popup_5e80c09f9d7d254cd1f2a4fc3b0e5828)\\n ;\\n\\n \\n \\n \\n var circle_marker_2bb426d488aebfa8a2d4426f9a2bc85d = L.circleMarker(\\n [42.73635845737364, -73.26364162994278],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3c5f0a825f69b78d1d58880aa2fd6a57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2700b0298ac475ac6777d6b500c89b6 = $(`<div id="html_d2700b0298ac475ac6777d6b500c89b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c5f0a825f69b78d1d58880aa2fd6a57.setContent(html_d2700b0298ac475ac6777d6b500c89b6);\\n \\n \\n\\n circle_marker_2bb426d488aebfa8a2d4426f9a2bc85d.bindPopup(popup_3c5f0a825f69b78d1d58880aa2fd6a57)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e5d55e18be816f499722be91ad95259 = L.circleMarker(\\n [42.73635843762231, -73.26241142403887],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ace1ed80f4ace43f3dbe0b327167ddd5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d4a1ad7d4c14d4b5d4662659febe235 = $(`<div id="html_3d4a1ad7d4c14d4b5d4662659febe235" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ace1ed80f4ace43f3dbe0b327167ddd5.setContent(html_3d4a1ad7d4c14d4b5d4662659febe235);\\n \\n \\n\\n circle_marker_3e5d55e18be816f499722be91ad95259.bindPopup(popup_ace1ed80f4ace43f3dbe0b327167ddd5)\\n ;\\n\\n \\n \\n \\n var circle_marker_5ceeaec368fd50da3aae7325c5a34bb6 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a5edee02be1c657efb8ac82cda1baa00 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ace91012911f7b7f4322d4b9d32bdc8 = $(`<div id="html_7ace91012911f7b7f4322d4b9d32bdc8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a5edee02be1c657efb8ac82cda1baa00.setContent(html_7ace91012911f7b7f4322d4b9d32bdc8);\\n \\n \\n\\n circle_marker_5ceeaec368fd50da3aae7325c5a34bb6.bindPopup(popup_a5edee02be1c657efb8ac82cda1baa00)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c7c0e98c5c980adaa415316bbc764f7 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.652426491681278, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0c3cf9fb0833c1fbbbe7b74c13415a1a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37e133ca9917ea67a7a7945fc90bfdf6 = $(`<div id="html_37e133ca9917ea67a7a7945fc90bfdf6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c3cf9fb0833c1fbbbe7b74c13415a1a.setContent(html_37e133ca9917ea67a7a7945fc90bfdf6);\\n \\n \\n\\n circle_marker_7c7c0e98c5c980adaa415316bbc764f7.bindPopup(popup_0c3cf9fb0833c1fbbbe7b74c13415a1a)\\n ;\\n\\n \\n \\n \\n var circle_marker_bec54bc627daaf921c3f0b40bb5695fe = L.circleMarker(\\n [42.73635829936299, -73.25872080633552],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e6e5d92bf2d7165f161181948ab4024a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf8365aab8c0959380b4d2893f91e4ba = $(`<div id="html_cf8365aab8c0959380b4d2893f91e4ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6e5d92bf2d7165f161181948ab4024a.setContent(html_cf8365aab8c0959380b4d2893f91e4ba);\\n \\n \\n\\n circle_marker_bec54bc627daaf921c3f0b40bb5695fe.bindPopup(popup_e6e5d92bf2d7165f161181948ab4024a)\\n ;\\n\\n \\n \\n \\n var circle_marker_9188da38ecc115dc9f5f2d7f9fcbc5cc = L.circleMarker(\\n [42.73635822694145, -73.25749060043893],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_17b980f18c2fa966ef18de678ccd4e12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d053ef510291f0bdd0e61e122c9708e5 = $(`<div id="html_d053ef510291f0bdd0e61e122c9708e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_17b980f18c2fa966ef18de678ccd4e12.setContent(html_d053ef510291f0bdd0e61e122c9708e5);\\n \\n \\n\\n circle_marker_9188da38ecc115dc9f5f2d7f9fcbc5cc.bindPopup(popup_17b980f18c2fa966ef18de678ccd4e12)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d9afa32e55c5d2981569b7de1a23e91 = L.circleMarker(\\n [42.73635814135235, -73.25626039454548],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0a334cca0617245052741920edd0bf36 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e1b56c22436a1dd5fd1e745b85701b3d = $(`<div id="html_e1b56c22436a1dd5fd1e745b85701b3d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a334cca0617245052741920edd0bf36.setContent(html_e1b56c22436a1dd5fd1e745b85701b3d);\\n \\n \\n\\n circle_marker_3d9afa32e55c5d2981569b7de1a23e91.bindPopup(popup_0a334cca0617245052741920edd0bf36)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9ef62816a3deeb84f9bd92fa6c21bbb = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_81a5ca58d75db96c0ae04b432cc5b39b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ecdb3f324ee01e607c0396717256b08 = $(`<div id="html_1ecdb3f324ee01e607c0396717256b08" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81a5ca58d75db96c0ae04b432cc5b39b.setContent(html_1ecdb3f324ee01e607c0396717256b08);\\n \\n \\n\\n circle_marker_e9ef62816a3deeb84f9bd92fa6c21bbb.bindPopup(popup_81a5ca58d75db96c0ae04b432cc5b39b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6c4f7ced6775dc0b48fd9e74dc507b0 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_024da06978564c3888629e6ebde8c0ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53d4a33161cf5490b207e807eb597151 = $(`<div id="html_53d4a33161cf5490b207e807eb597151" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_024da06978564c3888629e6ebde8c0ff.setContent(html_53d4a33161cf5490b207e807eb597151);\\n \\n \\n\\n circle_marker_e6c4f7ced6775dc0b48fd9e74dc507b0.bindPopup(popup_024da06978564c3888629e6ebde8c0ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ee17ebe44d2a5d68a98eaea39952d27 = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.656366395715726, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f656ffb342304598e632a3d212c0c518 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f3f27e76fa4b45241d5aae588565e98 = $(`<div id="html_8f3f27e76fa4b45241d5aae588565e98" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f656ffb342304598e632a3d212c0c518.setContent(html_8f3f27e76fa4b45241d5aae588565e98);\\n \\n \\n\\n circle_marker_3ee17ebe44d2a5d68a98eaea39952d27.bindPopup(popup_f656ffb342304598e632a3d212c0c518)\\n ;\\n\\n \\n \\n \\n var circle_marker_672752fa524951ed4c4aec1ac0e5cef1 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.068428945128219, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6f88111bb65f71a8d14981d9cf955db8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b38acc35b3b1493d29208a48e9770768 = $(`<div id="html_b38acc35b3b1493d29208a48e9770768" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f88111bb65f71a8d14981d9cf955db8.setContent(html_b38acc35b3b1493d29208a48e9770768);\\n \\n \\n\\n circle_marker_672752fa524951ed4c4aec1ac0e5cef1.bindPopup(popup_6f88111bb65f71a8d14981d9cf955db8)\\n ;\\n\\n \\n \\n \\n var circle_marker_85ea3c7065e438792c41015b0c24b98f = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a6721ea654156353852dca98c8c36a38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d019f46b4cd4e4eafb2dff520f39c25 = $(`<div id="html_3d019f46b4cd4e4eafb2dff520f39c25" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a6721ea654156353852dca98c8c36a38.setContent(html_3d019f46b4cd4e4eafb2dff520f39c25);\\n \\n \\n\\n circle_marker_85ea3c7065e438792c41015b0c24b98f.bindPopup(popup_a6721ea654156353852dca98c8c36a38)\\n ;\\n\\n \\n \\n \\n var circle_marker_d2075db6be1039a8f64a9800f018c6c9 = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f87ab66f1c2a6a0447267752f0029beb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55f8d1558220db8a354e2d07586d9b78 = $(`<div id="html_55f8d1558220db8a354e2d07586d9b78" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f87ab66f1c2a6a0447267752f0029beb.setContent(html_55f8d1558220db8a354e2d07586d9b78);\\n \\n \\n\\n circle_marker_d2075db6be1039a8f64a9800f018c6c9.bindPopup(popup_f87ab66f1c2a6a0447267752f0029beb)\\n ;\\n\\n \\n \\n \\n var circle_marker_29e6edbe0030ace02119041a6be1b223 = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2f89bf9bd1ae79e70868dc21a35a2cbd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b8bbb5695eb18b898fa062ab1fce869 = $(`<div id="html_6b8bbb5695eb18b898fa062ab1fce869" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f89bf9bd1ae79e70868dc21a35a2cbd.setContent(html_6b8bbb5695eb18b898fa062ab1fce869);\\n \\n \\n\\n circle_marker_29e6edbe0030ace02119041a6be1b223.bindPopup(popup_2f89bf9bd1ae79e70868dc21a35a2cbd)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e8c12b25afbd9ca2e95a94538f5784e = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_631ec5d397393d72f8ddc818875e2549 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a4d6191dfa48005e53d7f2bce2d3424 = $(`<div id="html_8a4d6191dfa48005e53d7f2bce2d3424" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_631ec5d397393d72f8ddc818875e2549.setContent(html_8a4d6191dfa48005e53d7f2bce2d3424);\\n \\n \\n\\n circle_marker_0e8c12b25afbd9ca2e95a94538f5784e.bindPopup(popup_631ec5d397393d72f8ddc818875e2549)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a42bcd56bb4d64b90fe279b9ae2696e = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.3078313050504, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d28a80dadeb0d9455b021e77fdff9b3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a870ee9f36a49482aade4327905f9624 = $(`<div id="html_a870ee9f36a49482aade4327905f9624" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d28a80dadeb0d9455b021e77fdff9b3e.setContent(html_a870ee9f36a49482aade4327905f9624);\\n \\n \\n\\n circle_marker_6a42bcd56bb4d64b90fe279b9ae2696e.bindPopup(popup_d28a80dadeb0d9455b021e77fdff9b3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc079fad9a9610a85f4555fd308e8a4c = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_404d6ea00fb74c6e85f363698fc0e8be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ec513221df6d6999ae5810521f5ecfd = $(`<div id="html_4ec513221df6d6999ae5810521f5ecfd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_404d6ea00fb74c6e85f363698fc0e8be.setContent(html_4ec513221df6d6999ae5810521f5ecfd);\\n \\n \\n\\n circle_marker_fc079fad9a9610a85f4555fd308e8a4c.bindPopup(popup_404d6ea00fb74c6e85f363698fc0e8be)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6221cb4f2759d7287f4163710a981ee = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_90175b95b6b9a1a1189fa14fdf18e9ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fbfa682a6c212fc5d9068bb20a9db5ee = $(`<div id="html_fbfa682a6c212fc5d9068bb20a9db5ee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90175b95b6b9a1a1189fa14fdf18e9ca.setContent(html_fbfa682a6c212fc5d9068bb20a9db5ee);\\n \\n \\n\\n circle_marker_a6221cb4f2759d7287f4163710a981ee.bindPopup(popup_90175b95b6b9a1a1189fa14fdf18e9ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_df4df4cdb485c654a73e06e7a61316b4 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2255dde3adf4e8befe691e4f74125cdc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e85a4b7261c29e670e81d72873c270b = $(`<div id="html_0e85a4b7261c29e670e81d72873c270b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2255dde3adf4e8befe691e4f74125cdc.setContent(html_0e85a4b7261c29e670e81d72873c270b);\\n \\n \\n\\n circle_marker_df4df4cdb485c654a73e06e7a61316b4.bindPopup(popup_2255dde3adf4e8befe691e4f74125cdc)\\n ;\\n\\n \\n \\n \\n var circle_marker_94463254a1912b7935171e06d07da482 = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c4ef54d86245e81e1b071c0739fe24fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fbfdbe171a8d25c2b8b7299c569fe4f = $(`<div id="html_8fbfdbe171a8d25c2b8b7299c569fe4f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4ef54d86245e81e1b071c0739fe24fe.setContent(html_8fbfdbe171a8d25c2b8b7299c569fe4f);\\n \\n \\n\\n circle_marker_94463254a1912b7935171e06d07da482.bindPopup(popup_c4ef54d86245e81e1b071c0739fe24fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_f69b36221fe2e7b37010bff6b4fbdf0b = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_79ba48fb0bbaef5346b3d6460b7122a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5676343c75eb35ee6180d9f7ca4aebd6 = $(`<div id="html_5676343c75eb35ee6180d9f7ca4aebd6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79ba48fb0bbaef5346b3d6460b7122a1.setContent(html_5676343c75eb35ee6180d9f7ca4aebd6);\\n \\n \\n\\n circle_marker_f69b36221fe2e7b37010bff6b4fbdf0b.bindPopup(popup_79ba48fb0bbaef5346b3d6460b7122a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe6d2857205ecd7a306574afa4e11fab = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_573092364412e145ffb6e94406737df7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2ac6e7cd0e9cfb518433f8856faa6566 = $(`<div id="html_2ac6e7cd0e9cfb518433f8856faa6566" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_573092364412e145ffb6e94406737df7.setContent(html_2ac6e7cd0e9cfb518433f8856faa6566);\\n \\n \\n\\n circle_marker_fe6d2857205ecd7a306574afa4e11fab.bindPopup(popup_573092364412e145ffb6e94406737df7)\\n ;\\n\\n \\n \\n \\n var circle_marker_96372eecc3c4a25d7cedf08cbcf7fe28 = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_930894f25ad750f291f467826da29a4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dcf8b20e964170f032ec94f1395259ab = $(`<div id="html_dcf8b20e964170f032ec94f1395259ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_930894f25ad750f291f467826da29a4b.setContent(html_dcf8b20e964170f032ec94f1395259ab);\\n \\n \\n\\n circle_marker_96372eecc3c4a25d7cedf08cbcf7fe28.bindPopup(popup_930894f25ad750f291f467826da29a4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_79ef41fb2e95a6418885770b5da4fc95 = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_bf155a8b554afedaca7c63837bc8ad38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_29e13f394ee78a271b13f4c11f2c995e = $(`<div id="html_29e13f394ee78a271b13f4c11f2c995e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bf155a8b554afedaca7c63837bc8ad38.setContent(html_29e13f394ee78a271b13f4c11f2c995e);\\n \\n \\n\\n circle_marker_79ef41fb2e95a6418885770b5da4fc95.bindPopup(popup_bf155a8b554afedaca7c63837bc8ad38)\\n ;\\n\\n \\n \\n \\n var circle_marker_a585cb0f92b89365624d75fe031c2574 = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e44fd19d078ab467c4ef57f40edacb81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dea8817a565805af1fc653208717cd21 = $(`<div id="html_dea8817a565805af1fc653208717cd21" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e44fd19d078ab467c4ef57f40edacb81.setContent(html_dea8817a565805af1fc653208717cd21);\\n \\n \\n\\n circle_marker_a585cb0f92b89365624d75fe031c2574.bindPopup(popup_e44fd19d078ab467c4ef57f40edacb81)\\n ;\\n\\n \\n \\n \\n var circle_marker_114471b1d75fdaf0f42167926d0e5314 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1559495004a9898b1d38f86347367c03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f1d27629b3a1c781c24c010dc1e6377 = $(`<div id="html_0f1d27629b3a1c781c24c010dc1e6377" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1559495004a9898b1d38f86347367c03.setContent(html_0f1d27629b3a1c781c24c010dc1e6377);\\n \\n \\n\\n circle_marker_114471b1d75fdaf0f42167926d0e5314.bindPopup(popup_1559495004a9898b1d38f86347367c03)\\n ;\\n\\n \\n \\n \\n var circle_marker_c50aa5f092e5930db0487274de3cbb19 = L.circleMarker(\\n [42.7345512252472, -73.26979251605432],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0b5df9d88dca1b63bfb9f03b836760d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_89fbc659c63a2fed4fb72bbe2b765e90 = $(`<div id="html_89fbc659c63a2fed4fb72bbe2b765e90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b5df9d88dca1b63bfb9f03b836760d4.setContent(html_89fbc659c63a2fed4fb72bbe2b765e90);\\n \\n \\n\\n circle_marker_c50aa5f092e5930db0487274de3cbb19.bindPopup(popup_0b5df9d88dca1b63bfb9f03b836760d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_44606f9aefac7f2455ed1d79c05f8e07 = L.circleMarker(\\n [42.73455127133177, -73.26856234600437],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7a871c02207a166a2e9ff27a98578090 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b3c15228c078bfea1265964f6b61d638 = $(`<div id="html_b3c15228c078bfea1265964f6b61d638" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a871c02207a166a2e9ff27a98578090.setContent(html_b3c15228c078bfea1265964f6b61d638);\\n \\n \\n\\n circle_marker_44606f9aefac7f2455ed1d79c05f8e07.bindPopup(popup_7a871c02207a166a2e9ff27a98578090)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a057606323c26dfeae475e1187a8a4e = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1aa0862b295729be5a74bd9da40198aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_652c7e5c00559e83bbb72a5be1a06a48 = $(`<div id="html_652c7e5c00559e83bbb72a5be1a06a48" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1aa0862b295729be5a74bd9da40198aa.setContent(html_652c7e5c00559e83bbb72a5be1a06a48);\\n \\n \\n\\n circle_marker_9a057606323c26dfeae475e1187a8a4e.bindPopup(popup_1aa0862b295729be5a74bd9da40198aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_92eedf192c1ad83a8376cf9a0cd9ac7e = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_05b465bb5dae9de1fc22eb53da93e138 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b09d05ee06161798c2231b0ac2937260 = $(`<div id="html_b09d05ee06161798c2231b0ac2937260" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05b465bb5dae9de1fc22eb53da93e138.setContent(html_b09d05ee06161798c2231b0ac2937260);\\n \\n \\n\\n circle_marker_92eedf192c1ad83a8376cf9a0cd9ac7e.bindPopup(popup_05b465bb5dae9de1fc22eb53da93e138)\\n ;\\n\\n \\n \\n \\n var circle_marker_95ed26ee3e8dd71d6292a9ed09a457d6 = L.circleMarker(\\n [42.734551330583365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d087dcc38a3e334dc2e0cf60b0676156 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b7d19ce88929dec84f8538a39b8334c4 = $(`<div id="html_b7d19ce88929dec84f8538a39b8334c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d087dcc38a3e334dc2e0cf60b0676156.setContent(html_b7d19ce88929dec84f8538a39b8334c4);\\n \\n \\n\\n circle_marker_95ed26ee3e8dd71d6292a9ed09a457d6.bindPopup(popup_d087dcc38a3e334dc2e0cf60b0676156)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b905a384820414f9f4da4a742b6a99a = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_57b4f19e8c83d87ed4f08b67e8a2a62f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd31d56eeb42a5b79dbceca8d80e319e = $(`<div id="html_dd31d56eeb42a5b79dbceca8d80e319e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_57b4f19e8c83d87ed4f08b67e8a2a62f.setContent(html_dd31d56eeb42a5b79dbceca8d80e319e);\\n \\n \\n\\n circle_marker_1b905a384820414f9f4da4a742b6a99a.bindPopup(popup_57b4f19e8c83d87ed4f08b67e8a2a62f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b4cd5ae2481cea0bc6f49902af0dea20 = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c8e4861777ceadff302ee72f0c086b2e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d80fce09529d44bf277d7f9d4b6b3a25 = $(`<div id="html_d80fce09529d44bf277d7f9d4b6b3a25" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8e4861777ceadff302ee72f0c086b2e.setContent(html_d80fce09529d44bf277d7f9d4b6b3a25);\\n \\n \\n\\n circle_marker_b4cd5ae2481cea0bc6f49902af0dea20.bindPopup(popup_c8e4861777ceadff302ee72f0c086b2e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f348d564a78e9e309f1b10fb83ce3f62 = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_952184a1d2de02142276f70162e68fbf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2d361be40e2e4263411c49b80882496f = $(`<div id="html_2d361be40e2e4263411c49b80882496f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_952184a1d2de02142276f70162e68fbf.setContent(html_2d361be40e2e4263411c49b80882496f);\\n \\n \\n\\n circle_marker_f348d564a78e9e309f1b10fb83ce3f62.bindPopup(popup_952184a1d2de02142276f70162e68fbf)\\n ;\\n\\n \\n \\n \\n var circle_marker_507355161ae5fb22523b27c5bd8d20f3 = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8db6d435011c918007b1e81b22760bd2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d7f1ab808a446dec180a4926e888bf3 = $(`<div id="html_3d7f1ab808a446dec180a4926e888bf3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8db6d435011c918007b1e81b22760bd2.setContent(html_3d7f1ab808a446dec180a4926e888bf3);\\n \\n \\n\\n circle_marker_507355161ae5fb22523b27c5bd8d20f3.bindPopup(popup_8db6d435011c918007b1e81b22760bd2)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ea05fd6bfed8a2ac29cb337d77daac6 = L.circleMarker(\\n [42.73455100799136, -73.25626064550488],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f636c5860c0644866b8084ec312f2827 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c1f8f105dbb7a8dd6632b8cf8badf560 = $(`<div id="html_c1f8f105dbb7a8dd6632b8cf8badf560" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f636c5860c0644866b8084ec312f2827.setContent(html_c1f8f105dbb7a8dd6632b8cf8badf560);\\n \\n \\n\\n circle_marker_6ea05fd6bfed8a2ac29cb337d77daac6.bindPopup(popup_f636c5860c0644866b8084ec312f2827)\\n ;\\n\\n \\n \\n \\n var circle_marker_b089d2dd92df698391e69c1225a67658 = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_660607d35c6d4c9db50dd9dc57cdc450 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a8e144da87831c8721f3f0cc08d6fe9 = $(`<div id="html_6a8e144da87831c8721f3f0cc08d6fe9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_660607d35c6d4c9db50dd9dc57cdc450.setContent(html_6a8e144da87831c8721f3f0cc08d6fe9);\\n \\n \\n\\n circle_marker_b089d2dd92df698391e69c1225a67658.bindPopup(popup_660607d35c6d4c9db50dd9dc57cdc450)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1f0a0fa68e43d3529d04cbfaa8fec99 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0a8aafc6d87dd45b79cd69be3a18007a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8acb6e4948c1d6bc68c6766cde10213 = $(`<div id="html_f8acb6e4948c1d6bc68c6766cde10213" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a8aafc6d87dd45b79cd69be3a18007a.setContent(html_f8acb6e4948c1d6bc68c6766cde10213);\\n \\n \\n\\n circle_marker_f1f0a0fa68e43d3529d04cbfaa8fec99.bindPopup(popup_0a8aafc6d87dd45b79cd69be3a18007a)\\n ;\\n\\n \\n \\n \\n var circle_marker_68f998e5ec3c3eddfab6e152760d8005 = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_74562d97095d6aefc8de9a7c152e73d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf7a914eca6252df2cef657ae665345f = $(`<div id="html_cf7a914eca6252df2cef657ae665345f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_74562d97095d6aefc8de9a7c152e73d7.setContent(html_cf7a914eca6252df2cef657ae665345f);\\n \\n \\n\\n circle_marker_68f998e5ec3c3eddfab6e152760d8005.bindPopup(popup_74562d97095d6aefc8de9a7c152e73d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_eff331e324dbef676d52ea3d4ad58da8 = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3c38422e58421115b8df52e483e22053 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9b36951096eae9f51b2ed2bb7060f05 = $(`<div id="html_d9b36951096eae9f51b2ed2bb7060f05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c38422e58421115b8df52e483e22053.setContent(html_d9b36951096eae9f51b2ed2bb7060f05);\\n \\n \\n\\n circle_marker_eff331e324dbef676d52ea3d4ad58da8.bindPopup(popup_3c38422e58421115b8df52e483e22053)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d5c72eb3ea77b251d05e5117b5cb7be = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_71338deb93af0e0f8fef5caa619415c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_213c3295bd0ef2f0c2a2b670be63c6d8 = $(`<div id="html_213c3295bd0ef2f0c2a2b670be63c6d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71338deb93af0e0f8fef5caa619415c5.setContent(html_213c3295bd0ef2f0c2a2b670be63c6d8);\\n \\n \\n\\n circle_marker_8d5c72eb3ea77b251d05e5117b5cb7be.bindPopup(popup_71338deb93af0e0f8fef5caa619415c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8cd89c674ec681c047d6a3e9ab20a9e = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_36cc14af61f76b7f49b22d1fe81c4a38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c887964171e389ee79b919a1a05c1a30 = $(`<div id="html_c887964171e389ee79b919a1a05c1a30" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36cc14af61f76b7f49b22d1fe81c4a38.setContent(html_c887964171e389ee79b919a1a05c1a30);\\n \\n \\n\\n circle_marker_e8cd89c674ec681c047d6a3e9ab20a9e.bindPopup(popup_36cc14af61f76b7f49b22d1fe81c4a38)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b15aa473f4796a369783599c402b764 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_313806c74a93cf7f2a5399dabc226c60 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_803bca206ee593c40b12ded8986a83f5 = $(`<div id="html_803bca206ee593c40b12ded8986a83f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_313806c74a93cf7f2a5399dabc226c60.setContent(html_803bca206ee593c40b12ded8986a83f5);\\n \\n \\n\\n circle_marker_1b15aa473f4796a369783599c402b764.bindPopup(popup_313806c74a93cf7f2a5399dabc226c60)\\n ;\\n\\n \\n \\n \\n var circle_marker_54363a0159f95525b36260a06a50ff04 = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_914671566ffa7662927740cf32fd1979 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a650b901130cac83f38afde7ce13a8d = $(`<div id="html_1a650b901130cac83f38afde7ce13a8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_914671566ffa7662927740cf32fd1979.setContent(html_1a650b901130cac83f38afde7ce13a8d);\\n \\n \\n\\n circle_marker_54363a0159f95525b36260a06a50ff04.bindPopup(popup_914671566ffa7662927740cf32fd1979)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e2a6a00249eafc43c16e2c22a909dab = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.2909362219603215, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1343f4c27642099d5dd145bb2518e2c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2a0c955dc50385dbe89b1ac2090fe333 = $(`<div id="html_2a0c955dc50385dbe89b1ac2090fe333" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1343f4c27642099d5dd145bb2518e2c1.setContent(html_2a0c955dc50385dbe89b1ac2090fe333);\\n \\n \\n\\n circle_marker_0e2a6a00249eafc43c16e2c22a909dab.bindPopup(popup_1343f4c27642099d5dd145bb2518e2c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_873761b82e25c4224259178f07b0967b = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.170882945826411, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_59a6169ba6148d94233eb64f85fb9db8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5eccc21147244c93fae7642a32f43050 = $(`<div id="html_5eccc21147244c93fae7642a32f43050" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_59a6169ba6148d94233eb64f85fb9db8.setContent(html_5eccc21147244c93fae7642a32f43050);\\n \\n \\n\\n circle_marker_873761b82e25c4224259178f07b0967b.bindPopup(popup_59a6169ba6148d94233eb64f85fb9db8)\\n ;\\n\\n \\n \\n \\n var circle_marker_33e97736ab35d07d9279717f9a022c3a = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_857df92f7d0211c92e10ccc749e1be3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42dae7e1d6c383b2e5a09de1d0c7587d = $(`<div id="html_42dae7e1d6c383b2e5a09de1d0c7587d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_857df92f7d0211c92e10ccc749e1be3f.setContent(html_42dae7e1d6c383b2e5a09de1d0c7587d);\\n \\n \\n\\n circle_marker_33e97736ab35d07d9279717f9a022c3a.bindPopup(popup_857df92f7d0211c92e10ccc749e1be3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_aeac68854b5a62c78723b652d846443a = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_db9b85c724b8545c70852bc7c9512548 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7dd1e5c84db2f742f3f42cac899b95a9 = $(`<div id="html_7dd1e5c84db2f742f3f42cac899b95a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db9b85c724b8545c70852bc7c9512548.setContent(html_7dd1e5c84db2f742f3f42cac899b95a9);\\n \\n \\n\\n circle_marker_aeac68854b5a62c78723b652d846443a.bindPopup(popup_db9b85c724b8545c70852bc7c9512548)\\n ;\\n\\n \\n \\n \\n var circle_marker_1422da2a6832165ec047ec6d37009d69 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c185f27cf4fca251fd06106f45b92860 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc727138e2bfba814d9bc3c4202c8b0b = $(`<div id="html_fc727138e2bfba814d9bc3c4202c8b0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c185f27cf4fca251fd06106f45b92860.setContent(html_fc727138e2bfba814d9bc3c4202c8b0b);\\n \\n \\n\\n circle_marker_1422da2a6832165ec047ec6d37009d69.bindPopup(popup_c185f27cf4fca251fd06106f45b92860)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a3d68d166bb2c36993388f20cda6619 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e09d95fb02419aa22fac007fc42664ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_125f9d0a6caffff7abdd653fc94afec6 = $(`<div id="html_125f9d0a6caffff7abdd653fc94afec6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e09d95fb02419aa22fac007fc42664ba.setContent(html_125f9d0a6caffff7abdd653fc94afec6);\\n \\n \\n\\n circle_marker_7a3d68d166bb2c36993388f20cda6619.bindPopup(popup_e09d95fb02419aa22fac007fc42664ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_46205bee83a8328c30db6e90b3d15e3a = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8fe3b8f304a89e94c57984382bf25169 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3799584a69cc6df113160dbf75b338f8 = $(`<div id="html_3799584a69cc6df113160dbf75b338f8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fe3b8f304a89e94c57984382bf25169.setContent(html_3799584a69cc6df113160dbf75b338f8);\\n \\n \\n\\n circle_marker_46205bee83a8328c30db6e90b3d15e3a.bindPopup(popup_8fe3b8f304a89e94c57984382bf25169)\\n ;\\n\\n \\n \\n \\n var circle_marker_e39a576e0e615d94560414533bc928c3 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2ffd40dbb2db962d3e3fa0e417c96908 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc324dc7625a14d307fe55a07442fbb7 = $(`<div id="html_fc324dc7625a14d307fe55a07442fbb7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ffd40dbb2db962d3e3fa0e417c96908.setContent(html_fc324dc7625a14d307fe55a07442fbb7);\\n \\n \\n\\n circle_marker_e39a576e0e615d94560414533bc928c3.bindPopup(popup_2ffd40dbb2db962d3e3fa0e417c96908)\\n ;\\n\\n \\n \\n \\n var circle_marker_5cabedf056da7baf6530d1e81f6a0aee = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d04cef3a9330c32ecf89c0ff532a0e5b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6258a77dd747d7c10975017da2641b0 = $(`<div id="html_b6258a77dd747d7c10975017da2641b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d04cef3a9330c32ecf89c0ff532a0e5b.setContent(html_b6258a77dd747d7c10975017da2641b0);\\n \\n \\n\\n circle_marker_5cabedf056da7baf6530d1e81f6a0aee.bindPopup(popup_d04cef3a9330c32ecf89c0ff532a0e5b)\\n ;\\n\\n \\n \\n \\n var circle_marker_3498fecddc90fcdb17a7b4025fe03fee = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.890312181373172, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cf1339700edce2f4108e9690813056ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_646b8e844f9997d84b278dc8eec7cfb6 = $(`<div id="html_646b8e844f9997d84b278dc8eec7cfb6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf1339700edce2f4108e9690813056ab.setContent(html_646b8e844f9997d84b278dc8eec7cfb6);\\n \\n \\n\\n circle_marker_3498fecddc90fcdb17a7b4025fe03fee.bindPopup(popup_cf1339700edce2f4108e9690813056ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7d0b8964e5ce1d9423dbe0c1e214d43 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b7402a7cd0b95db682dd091c12497196 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d12072d85d6c1fe7bebdd9bb29a1ec7 = $(`<div id="html_7d12072d85d6c1fe7bebdd9bb29a1ec7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b7402a7cd0b95db682dd091c12497196.setContent(html_7d12072d85d6c1fe7bebdd9bb29a1ec7);\\n \\n \\n\\n circle_marker_a7d0b8964e5ce1d9423dbe0c1e214d43.bindPopup(popup_b7402a7cd0b95db682dd091c12497196)\\n ;\\n\\n \\n \\n \\n var circle_marker_45363d36777d489065674b64d6772048 = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_13eb03133ba43309a6f14c1c8170dbc3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94c6294be35706b14aae80e8aa636c6e = $(`<div id="html_94c6294be35706b14aae80e8aa636c6e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_13eb03133ba43309a6f14c1c8170dbc3.setContent(html_94c6294be35706b14aae80e8aa636c6e);\\n \\n \\n\\n circle_marker_45363d36777d489065674b64d6772048.bindPopup(popup_13eb03133ba43309a6f14c1c8170dbc3)\\n ;\\n\\n \\n \\n \\n var circle_marker_bbc2402a7a9ca3d098fdb042290467bd = L.circleMarker(\\n [42.7327440918774, -73.26979237266056],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cfceb52a19f9e4f7290a3eefac1cb01d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3992008d31845c6e2cc1fd9f21f41204 = $(`<div id="html_3992008d31845c6e2cc1fd9f21f41204" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfceb52a19f9e4f7290a3eefac1cb01d.setContent(html_3992008d31845c6e2cc1fd9f21f41204);\\n \\n \\n\\n circle_marker_bbc2402a7a9ca3d098fdb042290467bd.bindPopup(popup_cfceb52a19f9e4f7290a3eefac1cb01d)\\n ;\\n\\n \\n \\n \\n var circle_marker_717b0d67c15f5997379293cb7686af5f = L.circleMarker(\\n [42.7327441379601, -73.26856223845904],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_db8e218592445126104931c0269784a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6312a1d608b12cd087e0e47c3617afd8 = $(`<div id="html_6312a1d608b12cd087e0e47c3617afd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db8e218592445126104931c0269784a0.setContent(html_6312a1d608b12cd087e0e47c3617afd8);\\n \\n \\n\\n circle_marker_717b0d67c15f5997379293cb7686af5f.bindPopup(popup_db8e218592445126104931c0269784a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_0b64fb083ae2eac22ce53f081d0afb8a = L.circleMarker(\\n [42.73274417087632, -73.26733210425597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2aa0db39b54f5dfc483004954054c2c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0fea13e9bc44751432899dbba8704130 = $(`<div id="html_0fea13e9bc44751432899dbba8704130" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2aa0db39b54f5dfc483004954054c2c9.setContent(html_0fea13e9bc44751432899dbba8704130);\\n \\n \\n\\n circle_marker_0b64fb083ae2eac22ce53f081d0afb8a.bindPopup(popup_2aa0db39b54f5dfc483004954054c2c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_26324a1df84dc4d7f42a41d63482adff = L.circleMarker(\\n [42.73274419062606, -73.26610197005185],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6c702e382d99bd17aba34267653760b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1742b566fd57119300f2c0ae97ad37cf = $(`<div id="html_1742b566fd57119300f2c0ae97ad37cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c702e382d99bd17aba34267653760b7.setContent(html_1742b566fd57119300f2c0ae97ad37cf);\\n \\n \\n\\n circle_marker_26324a1df84dc4d7f42a41d63482adff.bindPopup(popup_6c702e382d99bd17aba34267653760b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_abf7fef020e304c23f1beb884c1f9c4a = L.circleMarker(\\n [42.7327441972093, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_096161f458fbecf607896454fbce1bd2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_951b9b8ebf1b0b52f6aa54ac95f66b44 = $(`<div id="html_951b9b8ebf1b0b52f6aa54ac95f66b44" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_096161f458fbecf607896454fbce1bd2.setContent(html_951b9b8ebf1b0b52f6aa54ac95f66b44);\\n \\n \\n\\n circle_marker_abf7fef020e304c23f1beb884c1f9c4a.bindPopup(popup_096161f458fbecf607896454fbce1bd2)\\n ;\\n\\n \\n \\n \\n var circle_marker_1687862a41ddddb4dd43b46d93547e8f = L.circleMarker(\\n [42.73274419062606, -73.26364170164257],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2ca00442fbbefe52a383123af1706a01 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4854dbffb0a3eb5bca587cc3a16b0233 = $(`<div id="html_4854dbffb0a3eb5bca587cc3a16b0233" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ca00442fbbefe52a383123af1706a01.setContent(html_4854dbffb0a3eb5bca587cc3a16b0233);\\n \\n \\n\\n circle_marker_1687862a41ddddb4dd43b46d93547e8f.bindPopup(popup_2ca00442fbbefe52a383123af1706a01)\\n ;\\n\\n \\n \\n \\n var circle_marker_707429018ccc871f3ba6cd59ce09e5eb = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5b0d18e561b31c9376c9f597d7a56c1c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_88ba749ba9119b4faedf0bbd4d945ccf = $(`<div id="html_88ba749ba9119b4faedf0bbd4d945ccf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5b0d18e561b31c9376c9f597d7a56c1c.setContent(html_88ba749ba9119b4faedf0bbd4d945ccf);\\n \\n \\n\\n circle_marker_707429018ccc871f3ba6cd59ce09e5eb.bindPopup(popup_5b0d18e561b31c9376c9f597d7a56c1c)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c9bf0c8fd605a515785c5b063e3e169 = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1841419359420025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_fa6511a39ffa53680b0c255856a5f947 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8000f53a99a11e2d756e62f8b9fb8d7 = $(`<div id="html_d8000f53a99a11e2d756e62f8b9fb8d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa6511a39ffa53680b0c255856a5f947.setContent(html_d8000f53a99a11e2d756e62f8b9fb8d7);\\n \\n \\n\\n circle_marker_5c9bf0c8fd605a515785c5b063e3e169.bindPopup(popup_fa6511a39ffa53680b0c255856a5f947)\\n ;\\n\\n \\n \\n \\n var circle_marker_02908e360de7bfd1b36fddd1a0491b90 = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2f8cdd3052085cbba30099cde5329129 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c0ecbb4b0fe265e9b78582a613534e3 = $(`<div id="html_9c0ecbb4b0fe265e9b78582a613534e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f8cdd3052085cbba30099cde5329129.setContent(html_9c0ecbb4b0fe265e9b78582a613534e3);\\n \\n \\n\\n circle_marker_02908e360de7bfd1b36fddd1a0491b90.bindPopup(popup_2f8cdd3052085cbba30099cde5329129)\\n ;\\n\\n \\n \\n \\n var circle_marker_0022351f3507555964d8327473a8c1db = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b82296f0df8ab402b7e33601ce8b555a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d334ba9a4702f651ee818d5a5a98bb24 = $(`<div id="html_d334ba9a4702f651ee818d5a5a98bb24" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b82296f0df8ab402b7e33601ce8b555a.setContent(html_d334ba9a4702f651ee818d5a5a98bb24);\\n \\n \\n\\n circle_marker_0022351f3507555964d8327473a8c1db.bindPopup(popup_b82296f0df8ab402b7e33601ce8b555a)\\n ;\\n\\n \\n \\n \\n var circle_marker_12e5345d39d26e75a58b162e56265228 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2440764487d0fd86a83cbf5fb8dd569d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_916eb7859d2e78a9d5ac1ff525e5a231 = $(`<div id="html_916eb7859d2e78a9d5ac1ff525e5a231" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2440764487d0fd86a83cbf5fb8dd569d.setContent(html_916eb7859d2e78a9d5ac1ff525e5a231);\\n \\n \\n\\n circle_marker_12e5345d39d26e75a58b162e56265228.bindPopup(popup_2440764487d0fd86a83cbf5fb8dd569d)\\n ;\\n\\n \\n \\n \\n var circle_marker_ab7ea52fce939a3755ab421d272f2162 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ee0d93bdf868d247db20404110027634 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e4560080c833dd40d5280c6375d3f8b = $(`<div id="html_7e4560080c833dd40d5280c6375d3f8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee0d93bdf868d247db20404110027634.setContent(html_7e4560080c833dd40d5280c6375d3f8b);\\n \\n \\n\\n circle_marker_ab7ea52fce939a3755ab421d272f2162.bindPopup(popup_ee0d93bdf868d247db20404110027634)\\n ;\\n\\n \\n \\n \\n var circle_marker_470f896e3db8aed02888b76fe91ea26d = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6c31646df1cca53b6a9be631f656cdfa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_abf93a6c5af628c09c9281b0340a579b = $(`<div id="html_abf93a6c5af628c09c9281b0340a579b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c31646df1cca53b6a9be631f656cdfa.setContent(html_abf93a6c5af628c09c9281b0340a579b);\\n \\n \\n\\n circle_marker_470f896e3db8aed02888b76fe91ea26d.bindPopup(popup_6c31646df1cca53b6a9be631f656cdfa)\\n ;\\n\\n \\n \\n \\n var circle_marker_50f404615f0e507de3b717d83facfbb0 = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ba1d3953aca540f3fa1952bf80e1b7ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_89ef2ff62facd7d2d9aebb4166520346 = $(`<div id="html_89ef2ff62facd7d2d9aebb4166520346" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba1d3953aca540f3fa1952bf80e1b7ef.setContent(html_89ef2ff62facd7d2d9aebb4166520346);\\n \\n \\n\\n circle_marker_50f404615f0e507de3b717d83facfbb0.bindPopup(popup_ba1d3953aca540f3fa1952bf80e1b7ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e1e46954cd09452d5994c8d78d7b68d = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.886025119029199, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3ca2c6c9eee7b0d61e4d2b8d80f36c7f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_16baaaef2c14c917bf56b39e1a40ef9c = $(`<div id="html_16baaaef2c14c917bf56b39e1a40ef9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ca2c6c9eee7b0d61e4d2b8d80f36c7f.setContent(html_16baaaef2c14c917bf56b39e1a40ef9c);\\n \\n \\n\\n circle_marker_5e1e46954cd09452d5994c8d78d7b68d.bindPopup(popup_3ca2c6c9eee7b0d61e4d2b8d80f36c7f)\\n ;\\n\\n \\n \\n \\n var circle_marker_2528e00b15508f36245113a0a5d7d967 = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0b5aae6ea3c07e7bb07cc4d941567144 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c453c04477e4f638cc326ce43716be45 = $(`<div id="html_c453c04477e4f638cc326ce43716be45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b5aae6ea3c07e7bb07cc4d941567144.setContent(html_c453c04477e4f638cc326ce43716be45);\\n \\n \\n\\n circle_marker_2528e00b15508f36245113a0a5d7d967.bindPopup(popup_0b5aae6ea3c07e7bb07cc4d941567144)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e2afa7192701e6a673aeb91521f7bde = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_389ca1fab4a15abc6dcc113695995374 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1576ff4ac525d6529153fc71a3f15c50 = $(`<div id="html_1576ff4ac525d6529153fc71a3f15c50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_389ca1fab4a15abc6dcc113695995374.setContent(html_1576ff4ac525d6529153fc71a3f15c50);\\n \\n \\n\\n circle_marker_3e2afa7192701e6a673aeb91521f7bde.bindPopup(popup_389ca1fab4a15abc6dcc113695995374)\\n ;\\n\\n \\n \\n \\n var circle_marker_c19808108f3b4239dd5f9643c4f30178 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_aaa277793cb57529b500ecf6e71f3906 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e209df1e25609e3f188a5d66f1509e7e = $(`<div id="html_e209df1e25609e3f188a5d66f1509e7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aaa277793cb57529b500ecf6e71f3906.setContent(html_e209df1e25609e3f188a5d66f1509e7e);\\n \\n \\n\\n circle_marker_c19808108f3b4239dd5f9643c4f30178.bindPopup(popup_aaa277793cb57529b500ecf6e71f3906)\\n ;\\n\\n \\n \\n \\n var circle_marker_f610d694c838f88f5114487c79daadf9 = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cd61066b9e9abeef3ac430fa719e2219 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_66b39ca6df09204ad770fa210aac025f = $(`<div id="html_66b39ca6df09204ad770fa210aac025f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd61066b9e9abeef3ac430fa719e2219.setContent(html_66b39ca6df09204ad770fa210aac025f);\\n \\n \\n\\n circle_marker_f610d694c838f88f5114487c79daadf9.bindPopup(popup_cd61066b9e9abeef3ac430fa719e2219)\\n ;\\n\\n \\n \\n \\n var circle_marker_9854aa90b32999d9105ca0168f4227b6 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e813f0971bfd4dc1408bd3abd650d88a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6c15cca5d2de66d32e966bcdf9177cf7 = $(`<div id="html_6c15cca5d2de66d32e966bcdf9177cf7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e813f0971bfd4dc1408bd3abd650d88a.setContent(html_6c15cca5d2de66d32e966bcdf9177cf7);\\n \\n \\n\\n circle_marker_9854aa90b32999d9105ca0168f4227b6.bindPopup(popup_e813f0971bfd4dc1408bd3abd650d88a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e81cb08b2cde177358691c6cbb3d3f5f = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c829370bfe5d8aac010cdaef40ad7ef4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06dbfd065c49e9ffc5cc31d4be5ba760 = $(`<div id="html_06dbfd065c49e9ffc5cc31d4be5ba760" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c829370bfe5d8aac010cdaef40ad7ef4.setContent(html_06dbfd065c49e9ffc5cc31d4be5ba760);\\n \\n \\n\\n circle_marker_e81cb08b2cde177358691c6cbb3d3f5f.bindPopup(popup_c829370bfe5d8aac010cdaef40ad7ef4)\\n ;\\n\\n \\n \\n \\n var circle_marker_31bea116bea63880d2a63b3de0fa8c9d = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.046265044040321, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9d59c30ab9398af393b6dbfe4a2a06fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d01a6e596f992376ebe4db0b7346aab1 = $(`<div id="html_d01a6e596f992376ebe4db0b7346aab1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d59c30ab9398af393b6dbfe4a2a06fc.setContent(html_d01a6e596f992376ebe4db0b7346aab1);\\n \\n \\n\\n circle_marker_31bea116bea63880d2a63b3de0fa8c9d.bindPopup(popup_9d59c30ab9398af393b6dbfe4a2a06fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d85080954fc5c4a8e81f3024ec44933 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4505adad36183d2fb2a4e5baa3c8372a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1fe7198f0a881139f647fdae375e453f = $(`<div id="html_1fe7198f0a881139f647fdae375e453f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4505adad36183d2fb2a4e5baa3c8372a.setContent(html_1fe7198f0a881139f647fdae375e453f);\\n \\n \\n\\n circle_marker_1d85080954fc5c4a8e81f3024ec44933.bindPopup(popup_4505adad36183d2fb2a4e5baa3c8372a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a25afad06c290a7a85e819e4444762d6 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9bbccbbcd972fef3aaab054559d0042c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0be1ff8677ab2154f6f2268b99a59a5d = $(`<div id="html_0be1ff8677ab2154f6f2268b99a59a5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9bbccbbcd972fef3aaab054559d0042c.setContent(html_0be1ff8677ab2154f6f2268b99a59a5d);\\n \\n \\n\\n circle_marker_a25afad06c290a7a85e819e4444762d6.bindPopup(popup_9bbccbbcd972fef3aaab054559d0042c)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1ddc0b531ab0dc271ced01e78f6244e = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_88a0830806d3a06eaaa5943117dde263 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3632dd44c8a9a6161652d20b32fa395e = $(`<div id="html_3632dd44c8a9a6161652d20b32fa395e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88a0830806d3a06eaaa5943117dde263.setContent(html_3632dd44c8a9a6161652d20b32fa395e);\\n \\n \\n\\n circle_marker_b1ddc0b531ab0dc271ced01e78f6244e.bindPopup(popup_88a0830806d3a06eaaa5943117dde263)\\n ;\\n\\n \\n \\n \\n var circle_marker_ddd48dc78f116f76b436220bca15dffe = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_05cc9f36592a6cddc2ccc7b37444eeee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5016407a0e8cbc4d0cffb89b423115ed = $(`<div id="html_5016407a0e8cbc4d0cffb89b423115ed" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05cc9f36592a6cddc2ccc7b37444eeee.setContent(html_5016407a0e8cbc4d0cffb89b423115ed);\\n \\n \\n\\n circle_marker_ddd48dc78f116f76b436220bca15dffe.bindPopup(popup_05cc9f36592a6cddc2ccc7b37444eeee)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a20239b728cea1db858cc4d97cb37d9 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3a2c273e8a50f520e7eed388159513b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b3a7eb7d1ff80ae883684f18450aee1d = $(`<div id="html_b3a7eb7d1ff80ae883684f18450aee1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3a2c273e8a50f520e7eed388159513b7.setContent(html_b3a7eb7d1ff80ae883684f18450aee1d);\\n \\n \\n\\n circle_marker_0a20239b728cea1db858cc4d97cb37d9.bindPopup(popup_3a2c273e8a50f520e7eed388159513b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c3cb05569007f3f2a9877453f598ebb = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ba2dbced3d22a2d9b662e6c0cd2df4cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6059aa08bfc9d1eb64795ebff4f5ac8a = $(`<div id="html_6059aa08bfc9d1eb64795ebff4f5ac8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba2dbced3d22a2d9b662e6c0cd2df4cb.setContent(html_6059aa08bfc9d1eb64795ebff4f5ac8a);\\n \\n \\n\\n circle_marker_5c3cb05569007f3f2a9877453f598ebb.bindPopup(popup_ba2dbced3d22a2d9b662e6c0cd2df4cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b641520a4b26f42c8f8473330f1e8d4 = L.circleMarker(\\n [42.730936899260826, -73.2710223276323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e9f9b425072e4d145062063b7e1ec2b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8bcbd0a95142d2d7dfde0e388ec0c66e = $(`<div id="html_8bcbd0a95142d2d7dfde0e388ec0c66e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e9f9b425072e4d145062063b7e1ec2b7.setContent(html_8bcbd0a95142d2d7dfde0e388ec0c66e);\\n \\n \\n\\n circle_marker_5b641520a4b26f42c8f8473330f1e8d4.bindPopup(popup_e9f9b425072e4d145062063b7e1ec2b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_ac70397c9bc748fc30fd7d28b4e37c03 = L.circleMarker(\\n [42.730936958507606, -73.26979222927841],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_05daa3a457e5aa1968f906d5e4d41767 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0700be806a7d5c35943a9ea6c6abd8a2 = $(`<div id="html_0700be806a7d5c35943a9ea6c6abd8a2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05daa3a457e5aa1968f906d5e4d41767.setContent(html_0700be806a7d5c35943a9ea6c6abd8a2);\\n \\n \\n\\n circle_marker_ac70397c9bc748fc30fd7d28b4e37c03.bindPopup(popup_05daa3a457e5aa1968f906d5e4d41767)\\n ;\\n\\n \\n \\n \\n var circle_marker_ee019dded65061264a6c8054e29fd5e9 = L.circleMarker(\\n [42.73093700458845, -73.26856213092245],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b252130f501c413f90586d70a82c0e71 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f24809e815d7f79239ec40235e0050f9 = $(`<div id="html_f24809e815d7f79239ec40235e0050f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b252130f501c413f90586d70a82c0e71.setContent(html_f24809e815d7f79239ec40235e0050f9);\\n \\n \\n\\n circle_marker_ee019dded65061264a6c8054e29fd5e9.bindPopup(popup_b252130f501c413f90586d70a82c0e71)\\n ;\\n\\n \\n \\n \\n var circle_marker_43c6983d65632c8b8499c8ecdcbc02dd = L.circleMarker(\\n [42.73093703750333, -73.26733203256491],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_df3bfa81ad57247a5d174b51742bfd7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_370897ad52e13aab7a61f1236b093485 = $(`<div id="html_370897ad52e13aab7a61f1236b093485" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df3bfa81ad57247a5d174b51742bfd7b.setContent(html_370897ad52e13aab7a61f1236b093485);\\n \\n \\n\\n circle_marker_43c6983d65632c8b8499c8ecdcbc02dd.bindPopup(popup_df3bfa81ad57247a5d174b51742bfd7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_a39e1ea67ed634df77bdd393502de398 = L.circleMarker(\\n [42.73093705725226, -73.26610193420632],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1d7d1b476aceef14a86fe9d1a54cc12d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81c3da8fc4d78984756f9ec05d2950e2 = $(`<div id="html_81c3da8fc4d78984756f9ec05d2950e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d7d1b476aceef14a86fe9d1a54cc12d.setContent(html_81c3da8fc4d78984756f9ec05d2950e2);\\n \\n \\n\\n circle_marker_a39e1ea67ed634df77bdd393502de398.bindPopup(popup_1d7d1b476aceef14a86fe9d1a54cc12d)\\n ;\\n\\n \\n \\n \\n var circle_marker_d9cc53b064be65e1dcc6306c20d980da = L.circleMarker(\\n [42.73093706383524, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9df2e65b4259ec53cd4b26ce79b494e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a7fa97372e60634a6ea94308409cd98 = $(`<div id="html_3a7fa97372e60634a6ea94308409cd98" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9df2e65b4259ec53cd4b26ce79b494e5.setContent(html_3a7fa97372e60634a6ea94308409cd98);\\n \\n \\n\\n circle_marker_d9cc53b064be65e1dcc6306c20d980da.bindPopup(popup_9df2e65b4259ec53cd4b26ce79b494e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_839be8d0c9e100aeb8aa621583f2125f = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_fe6acc02b26c40cca082ba5c61b6a5e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46665725b370f9391f352c3b04898506 = $(`<div id="html_46665725b370f9391f352c3b04898506" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe6acc02b26c40cca082ba5c61b6a5e9.setContent(html_46665725b370f9391f352c3b04898506);\\n \\n \\n\\n circle_marker_839be8d0c9e100aeb8aa621583f2125f.bindPopup(popup_fe6acc02b26c40cca082ba5c61b6a5e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4159b44f933743a66cfbdaa57ef3a01 = L.circleMarker(\\n [42.73093703750333, -73.26241163912951],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7054c3c41eb3b028bcb8e678533f129f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_670d1eaff9c584edac7552629caa3320 = $(`<div id="html_670d1eaff9c584edac7552629caa3320" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7054c3c41eb3b028bcb8e678533f129f.setContent(html_670d1eaff9c584edac7552629caa3320);\\n \\n \\n\\n circle_marker_a4159b44f933743a66cfbdaa57ef3a01.bindPopup(popup_7054c3c41eb3b028bcb8e678533f129f)\\n ;\\n\\n \\n \\n \\n var circle_marker_21b29f4b434beb738847ff595c2307c1 = L.circleMarker(\\n [42.730936958507606, -73.259951442416],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_04230e7cb74c44f65c2348525a83a0ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bbb3488e5449461b4d0f0424d3cd60b2 = $(`<div id="html_bbb3488e5449461b4d0f0424d3cd60b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04230e7cb74c44f65c2348525a83a0ba.setContent(html_bbb3488e5449461b4d0f0424d3cd60b2);\\n \\n \\n\\n circle_marker_21b29f4b434beb738847ff595c2307c1.bindPopup(popup_04230e7cb74c44f65c2348525a83a0ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f83724a9d6393aed5772449ca789b2b = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_35a88bc7c23b464c8df860b50a392b16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7061633f371358ab78d2688ee2b206ad = $(`<div id="html_7061633f371358ab78d2688ee2b206ad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35a88bc7c23b464c8df860b50a392b16.setContent(html_7061633f371358ab78d2688ee2b206ad);\\n \\n \\n\\n circle_marker_9f83724a9d6393aed5772449ca789b2b.bindPopup(popup_35a88bc7c23b464c8df860b50a392b16)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b9b32fa31f88f3b60ed7ff67a3d23c6 = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2a19db4892bd01d62a0a721f7f330e63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1757b16e896197a97a50cb7573b314a = $(`<div id="html_f1757b16e896197a97a50cb7573b314a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a19db4892bd01d62a0a721f7f330e63.setContent(html_f1757b16e896197a97a50cb7573b314a);\\n \\n \\n\\n circle_marker_6b9b32fa31f88f3b60ed7ff67a3d23c6.bindPopup(popup_2a19db4892bd01d62a0a721f7f330e63)\\n ;\\n\\n \\n \\n \\n var circle_marker_5de78b413248ad56d9a8b3122048bc3c = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_be7bf0c742b7b1395f78a0fc46357610 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81ca06bca26eb8be0b942632c4280cfe = $(`<div id="html_81ca06bca26eb8be0b942632c4280cfe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be7bf0c742b7b1395f78a0fc46357610.setContent(html_81ca06bca26eb8be0b942632c4280cfe);\\n \\n \\n\\n circle_marker_5de78b413248ad56d9a8b3122048bc3c.bindPopup(popup_be7bf0c742b7b1395f78a0fc46357610)\\n ;\\n\\n \\n \\n \\n var circle_marker_d52edbb82a1e23bed70fae2a5041185c = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ff4ddb25fc8be9dc07b24220d7010d58 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_511e41d55fe308fd594f51f1f8746631 = $(`<div id="html_511e41d55fe308fd594f51f1f8746631" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff4ddb25fc8be9dc07b24220d7010d58.setContent(html_511e41d55fe308fd594f51f1f8746631);\\n \\n \\n\\n circle_marker_d52edbb82a1e23bed70fae2a5041185c.bindPopup(popup_ff4ddb25fc8be9dc07b24220d7010d58)\\n ;\\n\\n \\n \\n \\n var circle_marker_14ebfeb60d2edf4f018b34da7b48249a = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cc584fe2c3a4f839e0fa2f43659f19ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_98a0484e6143286ff19f5cdb4956dcb6 = $(`<div id="html_98a0484e6143286ff19f5cdb4956dcb6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc584fe2c3a4f839e0fa2f43659f19ce.setContent(html_98a0484e6143286ff19f5cdb4956dcb6);\\n \\n \\n\\n circle_marker_14ebfeb60d2edf4f018b34da7b48249a.bindPopup(popup_cc584fe2c3a4f839e0fa2f43659f19ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_3995f16e107f5a886a13ab5b107975d0 = L.circleMarker(\\n [42.73093640553757, -73.2525708523423],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_01733c12ca9992e189291b1fc79d0150 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d85a60107741c3db119b3fe37cd1744f = $(`<div id="html_d85a60107741c3db119b3fe37cd1744f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_01733c12ca9992e189291b1fc79d0150.setContent(html_d85a60107741c3db119b3fe37cd1744f);\\n \\n \\n\\n circle_marker_3995f16e107f5a886a13ab5b107975d0.bindPopup(popup_01733c12ca9992e189291b1fc79d0150)\\n ;\\n\\n \\n \\n \\n var circle_marker_92c81e3fcb8e669d42c3641c0f537daa = L.circleMarker(\\n [42.73093626729506, -73.25134075401192],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d5736d8ecca9a8956ad48e6bde1c8beb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e819d3b26ba32db898b91e02b95f4d59 = $(`<div id="html_e819d3b26ba32db898b91e02b95f4d59" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5736d8ecca9a8956ad48e6bde1c8beb.setContent(html_e819d3b26ba32db898b91e02b95f4d59);\\n \\n \\n\\n circle_marker_92c81e3fcb8e669d42c3641c0f537daa.bindPopup(popup_d5736d8ecca9a8956ad48e6bde1c8beb)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba0bcff89bff3a093831855ccbce5a4c = L.circleMarker(\\n [42.73093595131219, -73.24888055736892],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e4b3f23de3efb7e4b9060eac6b78c3a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_010a381d540faf48c678982c44d82262 = $(`<div id="html_010a381d540faf48c678982c44d82262" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4b3f23de3efb7e4b9060eac6b78c3a6.setContent(html_010a381d540faf48c678982c44d82262);\\n \\n \\n\\n circle_marker_ba0bcff89bff3a093831855ccbce5a4c.bindPopup(popup_e4b3f23de3efb7e4b9060eac6b78c3a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_d513a68f46bd28e79123da05bfecae65 = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.585191925620057, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6b5cd34256bce0f327bc64f22b98987c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_338f3868776d1e6434bfe370a6c9ad17 = $(`<div id="html_338f3868776d1e6434bfe370a6c9ad17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b5cd34256bce0f327bc64f22b98987c.setContent(html_338f3868776d1e6434bfe370a6c9ad17);\\n \\n \\n\\n circle_marker_d513a68f46bd28e79123da05bfecae65.bindPopup(popup_6b5cd34256bce0f327bc64f22b98987c)\\n ;\\n\\n \\n \\n \\n var circle_marker_b2f75591e2db984a2355c363c9e02d78 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_678b14eb47a3420ed87be09302d86c77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_766d2edddeb82ebc4acb0491230431f9 = $(`<div id="html_766d2edddeb82ebc4acb0491230431f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_678b14eb47a3420ed87be09302d86c77.setContent(html_766d2edddeb82ebc4acb0491230431f9);\\n \\n \\n\\n circle_marker_b2f75591e2db984a2355c363c9e02d78.bindPopup(popup_678b14eb47a3420ed87be09302d86c77)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c72ab510c247e9b34b1f86910a27ea5 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_257d088316d2ec0c8f5989b9e00eb29b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76b904170cdb44c3909eb836e2b61248 = $(`<div id="html_76b904170cdb44c3909eb836e2b61248" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_257d088316d2ec0c8f5989b9e00eb29b.setContent(html_76b904170cdb44c3909eb836e2b61248);\\n \\n \\n\\n circle_marker_8c72ab510c247e9b34b1f86910a27ea5.bindPopup(popup_257d088316d2ec0c8f5989b9e00eb29b)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e041b7a04f75093f5b8032a8be73256 = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_400c0c94c6561f30f7cb3044e4a4ba03 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48a38612eb3b5684fe976934ed32bee3 = $(`<div id="html_48a38612eb3b5684fe976934ed32bee3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_400c0c94c6561f30f7cb3044e4a4ba03.setContent(html_48a38612eb3b5684fe976934ed32bee3);\\n \\n \\n\\n circle_marker_6e041b7a04f75093f5b8032a8be73256.bindPopup(popup_400c0c94c6561f30f7cb3044e4a4ba03)\\n ;\\n\\n \\n \\n \\n var circle_marker_67fb081bcc1d10a854a755bed61492ac = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_28c5cb9313236dc715a3c2cc12b9903a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d99d0e841353d014089faaa9f3b0038 = $(`<div id="html_4d99d0e841353d014089faaa9f3b0038" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_28c5cb9313236dc715a3c2cc12b9903a.setContent(html_4d99d0e841353d014089faaa9f3b0038);\\n \\n \\n\\n circle_marker_67fb081bcc1d10a854a755bed61492ac.bindPopup(popup_28c5cb9313236dc715a3c2cc12b9903a)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcc967b1a9e375644de566d6a6aad58f = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_86531106f9a02ff8bc96a4faf9f4cee6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d89f04a22b93f665aee2713e9460dc3f = $(`<div id="html_d89f04a22b93f665aee2713e9460dc3f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86531106f9a02ff8bc96a4faf9f4cee6.setContent(html_d89f04a22b93f665aee2713e9460dc3f);\\n \\n \\n\\n circle_marker_bcc967b1a9e375644de566d6a6aad58f.bindPopup(popup_86531106f9a02ff8bc96a4faf9f4cee6)\\n ;\\n\\n \\n \\n \\n var circle_marker_fdadda92c84d32dffb9e645bb562ae59 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2b9f5bbf28ca37ea48e451ef5302f4c4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41428e4135ecfe60e89ee25d80803840 = $(`<div id="html_41428e4135ecfe60e89ee25d80803840" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b9f5bbf28ca37ea48e451ef5302f4c4.setContent(html_41428e4135ecfe60e89ee25d80803840);\\n \\n \\n\\n circle_marker_fdadda92c84d32dffb9e645bb562ae59.bindPopup(popup_2b9f5bbf28ca37ea48e451ef5302f4c4)\\n ;\\n\\n \\n \\n \\n var circle_marker_6863a1984bb3e7e676f84007e34ebefc = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_858c01721ddede35f24eb228e47825b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7635d5a2496ca50cee4b5d0ecfe378cd = $(`<div id="html_7635d5a2496ca50cee4b5d0ecfe378cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_858c01721ddede35f24eb228e47825b9.setContent(html_7635d5a2496ca50cee4b5d0ecfe378cd);\\n \\n \\n\\n circle_marker_6863a1984bb3e7e676f84007e34ebefc.bindPopup(popup_858c01721ddede35f24eb228e47825b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_c2cb056e421c5ce36a6793bab1f3d781 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_79332ed3663342485650d4ea5fb3735e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3c64d5047412403c273b8cfef638fdb9 = $(`<div id="html_3c64d5047412403c273b8cfef638fdb9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79332ed3663342485650d4ea5fb3735e.setContent(html_3c64d5047412403c273b8cfef638fdb9);\\n \\n \\n\\n circle_marker_c2cb056e421c5ce36a6793bab1f3d781.bindPopup(popup_79332ed3663342485650d4ea5fb3735e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d03d20577431e065af15f1842f5f4d28 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0e7a5e29b55d674406e8f33adf5adb70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0335dd4cd2d62aae3d107f2a9459eac2 = $(`<div id="html_0335dd4cd2d62aae3d107f2a9459eac2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e7a5e29b55d674406e8f33adf5adb70.setContent(html_0335dd4cd2d62aae3d107f2a9459eac2);\\n \\n \\n\\n circle_marker_d03d20577431e065af15f1842f5f4d28.bindPopup(popup_0e7a5e29b55d674406e8f33adf5adb70)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4df7ee69054314da647adc09426d39e = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_61e0b42016cdaa7631c1501650c29487 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe82b7d9e68b7c87f85cb2ad8eb98b92 = $(`<div id="html_fe82b7d9e68b7c87f85cb2ad8eb98b92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61e0b42016cdaa7631c1501650c29487.setContent(html_fe82b7d9e68b7c87f85cb2ad8eb98b92);\\n \\n \\n\\n circle_marker_c4df7ee69054314da647adc09426d39e.bindPopup(popup_61e0b42016cdaa7631c1501650c29487)\\n ;\\n\\n \\n \\n \\n var circle_marker_29445954dc41f70a05ebab2374d21d8c = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_dd1f453d7a7fa78d38039612c033ff76 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2d31bc30ac8cea1334e5e948f444d6b = $(`<div id="html_b2d31bc30ac8cea1334e5e948f444d6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd1f453d7a7fa78d38039612c033ff76.setContent(html_b2d31bc30ac8cea1334e5e948f444d6b);\\n \\n \\n\\n circle_marker_29445954dc41f70a05ebab2374d21d8c.bindPopup(popup_dd1f453d7a7fa78d38039612c033ff76)\\n ;\\n\\n \\n \\n \\n var circle_marker_818fb2b712701b92c149d0f08f67901e = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1a3c89b45bc3fbcf8fef686e30aa8247 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a0f0cbd5efb464c86868afe9cb106f96 = $(`<div id="html_a0f0cbd5efb464c86868afe9cb106f96" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a3c89b45bc3fbcf8fef686e30aa8247.setContent(html_a0f0cbd5efb464c86868afe9cb106f96);\\n \\n \\n\\n circle_marker_818fb2b712701b92c149d0f08f67901e.bindPopup(popup_1a3c89b45bc3fbcf8fef686e30aa8247)\\n ;\\n\\n \\n \\n \\n var circle_marker_9fc93f48977dc1f98a2e1915a6e429e7 = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.583497844237541, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ca98603d0250b1006ced0f0f6b0e50cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed26437932d14b615df088eedcd74ae1 = $(`<div id="html_ed26437932d14b615df088eedcd74ae1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca98603d0250b1006ced0f0f6b0e50cc.setContent(html_ed26437932d14b615df088eedcd74ae1);\\n \\n \\n\\n circle_marker_9fc93f48977dc1f98a2e1915a6e429e7.bindPopup(popup_ca98603d0250b1006ced0f0f6b0e50cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_e582dcec7d9146d49a563fbe5bcd9ae7 = L.circleMarker(\\n [42.72912976589343, -73.27102214841915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d5d23c876182ca0d20f5f0b7f3010f9f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ccf0b1307ec65411ec0e3c03aada5ca1 = $(`<div id="html_ccf0b1307ec65411ec0e3c03aada5ca1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5d23c876182ca0d20f5f0b7f3010f9f.setContent(html_ccf0b1307ec65411ec0e3c03aada5ca1);\\n \\n \\n\\n circle_marker_e582dcec7d9146d49a563fbe5bcd9ae7.bindPopup(popup_d5d23c876182ca0d20f5f0b7f3010f9f)\\n ;\\n\\n \\n \\n \\n var circle_marker_20db23bca4dc3d8c4f38b846e383b94e = L.circleMarker(\\n [42.72912982513782, -73.2697920859079],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_889c44fa97df6d52e1ca20ef8af5b0de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c745173e307ea96ac08dd4f9992ac0ba = $(`<div id="html_c745173e307ea96ac08dd4f9992ac0ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_889c44fa97df6d52e1ca20ef8af5b0de.setContent(html_c745173e307ea96ac08dd4f9992ac0ba);\\n \\n \\n\\n circle_marker_20db23bca4dc3d8c4f38b846e383b94e.bindPopup(popup_889c44fa97df6d52e1ca20ef8af5b0de)\\n ;\\n\\n \\n \\n \\n var circle_marker_74eecb05708fb3254684e3560ae6d6af = L.circleMarker(\\n [42.729129871216784, -73.26856202339455],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_33e3d350b386c88e13c61020554b055d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_84008ee7099e7adaa8a718a8097bde79 = $(`<div id="html_84008ee7099e7adaa8a718a8097bde79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33e3d350b386c88e13c61020554b055d.setContent(html_84008ee7099e7adaa8a718a8097bde79);\\n \\n \\n\\n circle_marker_74eecb05708fb3254684e3560ae6d6af.bindPopup(popup_33e3d350b386c88e13c61020554b055d)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6a60d62b38cbbd5a2c9f16923323ec7 = L.circleMarker(\\n [42.72912990413034, -73.26733196087964],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f689f6ca8c333c126fdb406b76c2b674 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d708627dfd06e7ed5b05c108941c6161 = $(`<div id="html_d708627dfd06e7ed5b05c108941c6161" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f689f6ca8c333c126fdb406b76c2b674.setContent(html_d708627dfd06e7ed5b05c108941c6161);\\n \\n \\n\\n circle_marker_b6a60d62b38cbbd5a2c9f16923323ec7.bindPopup(popup_f689f6ca8c333c126fdb406b76c2b674)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e98b5821e07fa1deb82b93a72e60dfe = L.circleMarker(\\n [42.72912992387847, -73.26610189836369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b0785667901131f899aaf22db4388630 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2874b3a37f5e11591a43755fe8f77cf1 = $(`<div id="html_2874b3a37f5e11591a43755fe8f77cf1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0785667901131f899aaf22db4388630.setContent(html_2874b3a37f5e11591a43755fe8f77cf1);\\n \\n \\n\\n circle_marker_8e98b5821e07fa1deb82b93a72e60dfe.bindPopup(popup_b0785667901131f899aaf22db4388630)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d9b546d157eb5d60349634d4ad0e21d = L.circleMarker(\\n [42.72912993046118, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e0687d1cb6ca254b55931e96b4aaeaa1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac9d789b9458d982402835891d500ab9 = $(`<div id="html_ac9d789b9458d982402835891d500ab9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0687d1cb6ca254b55931e96b4aaeaa1.setContent(html_ac9d789b9458d982402835891d500ab9);\\n \\n \\n\\n circle_marker_1d9b546d157eb5d60349634d4ad0e21d.bindPopup(popup_e0687d1cb6ca254b55931e96b4aaeaa1)\\n ;\\n\\n \\n \\n \\n var circle_marker_23e4a43b8f52881c653cf06a21470259 = L.circleMarker(\\n [42.72912992387847, -73.26364177333073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f97f3be33dcb17f5f1312d0cf25e885f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6589fcde7771ab31b103ab578230f9b7 = $(`<div id="html_6589fcde7771ab31b103ab578230f9b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f97f3be33dcb17f5f1312d0cf25e885f.setContent(html_6589fcde7771ab31b103ab578230f9b7);\\n \\n \\n\\n circle_marker_23e4a43b8f52881c653cf06a21470259.bindPopup(popup_f97f3be33dcb17f5f1312d0cf25e885f)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c19b1dcaf361921e71183b8cc19cf6b = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_53547f5196318a214f7b530d01de29e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ce84a28a42c409abf5f57eb789911d8 = $(`<div id="html_9ce84a28a42c409abf5f57eb789911d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_53547f5196318a214f7b530d01de29e8.setContent(html_9ce84a28a42c409abf5f57eb789911d8);\\n \\n \\n\\n circle_marker_7c19b1dcaf361921e71183b8cc19cf6b.bindPopup(popup_53547f5196318a214f7b530d01de29e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_050fa210eae7b4ac323695520d678c7e = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1fe4c3bb8127a010bf211482a607c95d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc3ec6a13613bba0243c0c0f4e4a18a5 = $(`<div id="html_dc3ec6a13613bba0243c0c0f4e4a18a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1fe4c3bb8127a010bf211482a607c95d.setContent(html_dc3ec6a13613bba0243c0c0f4e4a18a5);\\n \\n \\n\\n circle_marker_050fa210eae7b4ac323695520d678c7e.bindPopup(popup_1fe4c3bb8127a010bf211482a607c95d)\\n ;\\n\\n \\n \\n \\n var circle_marker_90594c30a4bf51777fb60b0d70c2a613 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5e0511cf41b0ca02845ddc40957b5306 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed6fbb5ddee85c7d3b516ec0a8f6179a = $(`<div id="html_ed6fbb5ddee85c7d3b516ec0a8f6179a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e0511cf41b0ca02845ddc40957b5306.setContent(html_ed6fbb5ddee85c7d3b516ec0a8f6179a);\\n \\n \\n\\n circle_marker_90594c30a4bf51777fb60b0d70c2a613.bindPopup(popup_5e0511cf41b0ca02845ddc40957b5306)\\n ;\\n\\n \\n \\n \\n var circle_marker_3700a551ec095519c865481a5640f4f1 = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a45b1a05599cc2891fcc39770681279f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1dc321cd6e35f04c77e672ac75f28a94 = $(`<div id="html_1dc321cd6e35f04c77e672ac75f28a94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a45b1a05599cc2891fcc39770681279f.setContent(html_1dc321cd6e35f04c77e672ac75f28a94);\\n \\n \\n\\n circle_marker_3700a551ec095519c865481a5640f4f1.bindPopup(popup_a45b1a05599cc2891fcc39770681279f)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c022e3e11e6aa15a92ff784b4f5a62f = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e592d998d6f896aa5917755e0fdc0d95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99d0477fef1efece92b0c6ff6e6b7e1a = $(`<div id="html_99d0477fef1efece92b0c6ff6e6b7e1a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e592d998d6f896aa5917755e0fdc0d95.setContent(html_99d0477fef1efece92b0c6ff6e6b7e1a);\\n \\n \\n\\n circle_marker_0c022e3e11e6aa15a92ff784b4f5a62f.bindPopup(popup_e592d998d6f896aa5917755e0fdc0d95)\\n ;\\n\\n \\n \\n \\n var circle_marker_31e04b4a129b022e21b47d1db635ed1e = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_98316bd176b6a55450a43504c2dddfbd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_846a21b6174d055cb00517baa92c5b62 = $(`<div id="html_846a21b6174d055cb00517baa92c5b62" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98316bd176b6a55450a43504c2dddfbd.setContent(html_846a21b6174d055cb00517baa92c5b62);\\n \\n \\n\\n circle_marker_31e04b4a129b022e21b47d1db635ed1e.bindPopup(popup_98316bd176b6a55450a43504c2dddfbd)\\n ;\\n\\n \\n \\n \\n var circle_marker_95554a469218355f921347805422b41b = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_76b0421db10f6b6d52a1459159225ce1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8091175d7bb6b6655117a9f3fa10ef0 = $(`<div id="html_c8091175d7bb6b6655117a9f3fa10ef0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76b0421db10f6b6d52a1459159225ce1.setContent(html_c8091175d7bb6b6655117a9f3fa10ef0);\\n \\n \\n\\n circle_marker_95554a469218355f921347805422b41b.bindPopup(popup_76b0421db10f6b6d52a1459159225ce1)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe122a424eb8de031397efae713566fb = L.circleMarker(\\n [42.72912939726166, -73.25380127326159],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.753945931439391, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8714f1a6f8ce191912277837c3ac987a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ba4efaac005b725f1550014f5d45da1 = $(`<div id="html_9ba4efaac005b725f1550014f5d45da1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8714f1a6f8ce191912277837c3ac987a.setContent(html_9ba4efaac005b725f1550014f5d45da1);\\n \\n \\n\\n circle_marker_fe122a424eb8de031397efae713566fb.bindPopup(popup_8714f1a6f8ce191912277837c3ac987a)\\n ;\\n\\n \\n \\n \\n var circle_marker_828769af84a3ca1ac8c0a5308cb82d5a = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c90a71d9d838ecbd00727fb1892d01fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65810da2796b86bd72bb921e557df56d = $(`<div id="html_65810da2796b86bd72bb921e557df56d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c90a71d9d838ecbd00727fb1892d01fc.setContent(html_65810da2796b86bd72bb921e557df56d);\\n \\n \\n\\n circle_marker_828769af84a3ca1ac8c0a5308cb82d5a.bindPopup(popup_c90a71d9d838ecbd00727fb1892d01fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ed5c09ecfcc37247b133a4f674a35a1 = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.406461512053774, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_bc881d66de7de83544b82fb3b8a4cf7d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2aed15db0cf4bc5b7aca5c06dc878fb7 = $(`<div id="html_2aed15db0cf4bc5b7aca5c06dc878fb7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc881d66de7de83544b82fb3b8a4cf7d.setContent(html_2aed15db0cf4bc5b7aca5c06dc878fb7);\\n \\n \\n\\n circle_marker_0ed5c09ecfcc37247b133a4f674a35a1.bindPopup(popup_bc881d66de7de83544b82fb3b8a4cf7d)\\n ;\\n\\n \\n \\n \\n var circle_marker_bb184f7782dbc451b5673af1060636f5 = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4c6679cfa693fe033d89b0373edf5515 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95b6b33460d5b355ec535c1e9e7b0211 = $(`<div id="html_95b6b33460d5b355ec535c1e9e7b0211" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c6679cfa693fe033d89b0373edf5515.setContent(html_95b6b33460d5b355ec535c1e9e7b0211);\\n \\n \\n\\n circle_marker_bb184f7782dbc451b5673af1060636f5.bindPopup(popup_4c6679cfa693fe033d89b0373edf5515)\\n ;\\n\\n \\n \\n \\n var circle_marker_826bb340b1abb6caf2172016395fc0c4 = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c4ebcf81a241e7c10536fecbc9245172 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a0f3162e06819bb489059cacddda03cf = $(`<div id="html_a0f3162e06819bb489059cacddda03cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4ebcf81a241e7c10536fecbc9245172.setContent(html_a0f3162e06819bb489059cacddda03cf);\\n \\n \\n\\n circle_marker_826bb340b1abb6caf2172016395fc0c4.bindPopup(popup_c4ebcf81a241e7c10536fecbc9245172)\\n ;\\n\\n \\n \\n \\n var circle_marker_756952e184d67a6c8b2364d60e05c74a = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c23be9d0cfcf700e43f9188cc78807a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_253f2e20da48201ab56293261638a892 = $(`<div id="html_253f2e20da48201ab56293261638a892" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c23be9d0cfcf700e43f9188cc78807a5.setContent(html_253f2e20da48201ab56293261638a892);\\n \\n \\n\\n circle_marker_756952e184d67a6c8b2364d60e05c74a.bindPopup(popup_c23be9d0cfcf700e43f9188cc78807a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc30bdaf539de1f7c1e0b33b654073d9 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_40f7f9eb8bf896eac82e08b8e22a7e22 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5bda4d91ce5e15086ac20985a06617ca = $(`<div id="html_5bda4d91ce5e15086ac20985a06617ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_40f7f9eb8bf896eac82e08b8e22a7e22.setContent(html_5bda4d91ce5e15086ac20985a06617ca);\\n \\n \\n\\n circle_marker_cc30bdaf539de1f7c1e0b33b654073d9.bindPopup(popup_40f7f9eb8bf896eac82e08b8e22a7e22)\\n ;\\n\\n \\n \\n \\n var circle_marker_4397459fe160ebcde68eafd185d01806 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a644696d82796295c592c0d1503220bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_218460fd7f5e18b6f8168929ca26226c = $(`<div id="html_218460fd7f5e18b6f8168929ca26226c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a644696d82796295c592c0d1503220bc.setContent(html_218460fd7f5e18b6f8168929ca26226c);\\n \\n \\n\\n circle_marker_4397459fe160ebcde68eafd185d01806.bindPopup(popup_a644696d82796295c592c0d1503220bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_af96a1ad5bd9f356b0913c05e3e947e5 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b05cf130b1540a359a509ac1d25003bb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2b20c74508e6aaf2f9d18139c3738cc = $(`<div id="html_e2b20c74508e6aaf2f9d18139c3738cc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b05cf130b1540a359a509ac1d25003bb.setContent(html_e2b20c74508e6aaf2f9d18139c3738cc);\\n \\n \\n\\n circle_marker_af96a1ad5bd9f356b0913c05e3e947e5.bindPopup(popup_b05cf130b1540a359a509ac1d25003bb)\\n ;\\n\\n \\n \\n \\n var circle_marker_009eb16c0efc551793ffae85c8b523a1 = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_39460504175423d633fe32f1750191af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3a15cff1237380bf29ae92f63c92ea0 = $(`<div id="html_a3a15cff1237380bf29ae92f63c92ea0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39460504175423d633fe32f1750191af.setContent(html_a3a15cff1237380bf29ae92f63c92ea0);\\n \\n \\n\\n circle_marker_009eb16c0efc551793ffae85c8b523a1.bindPopup(popup_39460504175423d633fe32f1750191af)\\n ;\\n\\n \\n \\n \\n var circle_marker_e62371ffc655b962a35a1453aceec9cd = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f6645211d7a75d309f4f62edb1814494 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4043f25e4051885fad66338399f6231a = $(`<div id="html_4043f25e4051885fad66338399f6231a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6645211d7a75d309f4f62edb1814494.setContent(html_4043f25e4051885fad66338399f6231a);\\n \\n \\n\\n circle_marker_e62371ffc655b962a35a1453aceec9cd.bindPopup(popup_f6645211d7a75d309f4f62edb1814494)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa7e8f63703e4e03c55540163b8d9c80 = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7d5c28c8d213d0872d6a19ebd990ca59 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7067d46f20642638129c2b8a4f4faa7e = $(`<div id="html_7067d46f20642638129c2b8a4f4faa7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7d5c28c8d213d0872d6a19ebd990ca59.setContent(html_7067d46f20642638129c2b8a4f4faa7e);\\n \\n \\n\\n circle_marker_fa7e8f63703e4e03c55540163b8d9c80.bindPopup(popup_7d5c28c8d213d0872d6a19ebd990ca59)\\n ;\\n\\n \\n \\n \\n var circle_marker_feecb59d9fa333c030f2e111ea37dc48 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8fe258086e1418a52f4ace1039068750 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67517e9658b871bab0100893b732d562 = $(`<div id="html_67517e9658b871bab0100893b732d562" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fe258086e1418a52f4ace1039068750.setContent(html_67517e9658b871bab0100893b732d562);\\n \\n \\n\\n circle_marker_feecb59d9fa333c030f2e111ea37dc48.bindPopup(popup_8fe258086e1418a52f4ace1039068750)\\n ;\\n\\n \\n \\n \\n var circle_marker_20e0ed666a11a3392779111fc5650e21 = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2edebeaceb73ffe1c0951f77d2ea69ce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63146a81363ca46ea5413e99a68b54ea = $(`<div id="html_63146a81363ca46ea5413e99a68b54ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2edebeaceb73ffe1c0951f77d2ea69ce.setContent(html_63146a81363ca46ea5413e99a68b54ea);\\n \\n \\n\\n circle_marker_20e0ed666a11a3392779111fc5650e21.bindPopup(popup_2edebeaceb73ffe1c0951f77d2ea69ce)\\n ;\\n\\n \\n \\n \\n var circle_marker_4a97b531e9e5b4d33001916cd4c76acf = L.circleMarker(\\n [42.72732247454739, -73.27348202255521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6dccc4d67b90d1e0752e3e6427a2752a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_59f7897c3e4738e97774c30c543cc6de = $(`<div id="html_59f7897c3e4738e97774c30c543cc6de" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6dccc4d67b90d1e0752e3e6427a2752a.setContent(html_59f7897c3e4738e97774c30c543cc6de);\\n \\n \\n\\n circle_marker_4a97b531e9e5b4d33001916cd4c76acf.bindPopup(popup_6dccc4d67b90d1e0752e3e6427a2752a)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ac4969d93982e6b33f1a49495672364 = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5a3b80fbdc6b540d3e396498dd704a32 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_112ff5a401eb31102cf98a25cbe06f5d = $(`<div id="html_112ff5a401eb31102cf98a25cbe06f5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a3b80fbdc6b540d3e396498dd704a32.setContent(html_112ff5a401eb31102cf98a25cbe06f5d);\\n \\n \\n\\n circle_marker_2ac4969d93982e6b33f1a49495672364.bindPopup(popup_5a3b80fbdc6b540d3e396498dd704a32)\\n ;\\n\\n \\n \\n \\n var circle_marker_7cfbb696abdd1280d69eb4260f440e47 = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5ce3496384e3e2d9d98ab9d3cd99eaba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_621102fe084c255dd436b0ce45a1b625 = $(`<div id="html_621102fe084c255dd436b0ce45a1b625" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ce3496384e3e2d9d98ab9d3cd99eaba.setContent(html_621102fe084c255dd436b0ce45a1b625);\\n \\n \\n\\n circle_marker_7cfbb696abdd1280d69eb4260f440e47.bindPopup(popup_5ce3496384e3e2d9d98ab9d3cd99eaba)\\n ;\\n\\n \\n \\n \\n var circle_marker_a25710d879f43299ab8990c4ad4fba00 = L.circleMarker(\\n [42.727322737845135, -73.26856191587537],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_416cf851447e6789a59f25b5c7dc745b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_436621cc8c0ed9ac9303a672f8514d97 = $(`<div id="html_436621cc8c0ed9ac9303a672f8514d97" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_416cf851447e6789a59f25b5c7dc745b.setContent(html_436621cc8c0ed9ac9303a672f8514d97);\\n \\n \\n\\n circle_marker_a25710d879f43299ab8990c4ad4fba00.bindPopup(popup_416cf851447e6789a59f25b5c7dc745b)\\n ;\\n\\n \\n \\n \\n var circle_marker_7f3cb46e4cc9f8ad73590d0246c70710 = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_54ceb2591c88af9266c587093ca4fc7d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3899f9058948bf60f918726305fe908e = $(`<div id="html_3899f9058948bf60f918726305fe908e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54ceb2591c88af9266c587093ca4fc7d.setContent(html_3899f9058948bf60f918726305fe908e);\\n \\n \\n\\n circle_marker_7f3cb46e4cc9f8ad73590d0246c70710.bindPopup(popup_54ceb2591c88af9266c587093ca4fc7d)\\n ;\\n\\n \\n \\n \\n var circle_marker_20066055bb61a80aa9de73b4887b457d = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_86fa92228c95bed2f6b126a2e68667b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b079748e8a5af3094a5af9156eec582d = $(`<div id="html_b079748e8a5af3094a5af9156eec582d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86fa92228c95bed2f6b126a2e68667b8.setContent(html_b079748e8a5af3094a5af9156eec582d);\\n \\n \\n\\n circle_marker_20066055bb61a80aa9de73b4887b457d.bindPopup(popup_86fa92228c95bed2f6b126a2e68667b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_859b44792f2d24f96b898abcc0cbd741 = L.circleMarker(\\n [42.727322797087126, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.656366395715726, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1f27a9c25b14da8834e8e3ac7b7816c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14300af87d0d3c237ec5e9e0d3225ce0 = $(`<div id="html_14300af87d0d3c237ec5e9e0d3225ce0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f27a9c25b14da8834e8e3ac7b7816c9.setContent(html_14300af87d0d3c237ec5e9e0d3225ce0);\\n \\n \\n\\n circle_marker_859b44792f2d24f96b898abcc0cbd741.bindPopup(popup_1f27a9c25b14da8834e8e3ac7b7816c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_577ff5a03fe8066e908cc97ec7a18ba4 = L.circleMarker(\\n [42.72732279050467, -73.26364180917045],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_41619c2b7e4156d4f8415062984cb7b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ee67decbbc585bb3497b8902e2449128 = $(`<div id="html_ee67decbbc585bb3497b8902e2449128" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41619c2b7e4156d4f8415062984cb7b9.setContent(html_ee67decbbc585bb3497b8902e2449128);\\n \\n \\n\\n circle_marker_577ff5a03fe8066e908cc97ec7a18ba4.bindPopup(popup_41619c2b7e4156d4f8415062984cb7b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_bbcc1bfed4781b144bb9160a9f0ef638 = L.circleMarker(\\n [42.72732277075734, -73.26241178249424],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_11d1b26b17c5255983618075bb299e0f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_674cd53373c95ea4136da34dede1531c = $(`<div id="html_674cd53373c95ea4136da34dede1531c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11d1b26b17c5255983618075bb299e0f.setContent(html_674cd53373c95ea4136da34dede1531c);\\n \\n \\n\\n circle_marker_bbcc1bfed4781b144bb9160a9f0ef638.bindPopup(popup_11d1b26b17c5255983618075bb299e0f)\\n ;\\n\\n \\n \\n \\n var circle_marker_a50e492992e5431d947530611f2b5cbe = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ff1855b32f2dba91d0af78cc96580ca8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39f81d956cb246b87fc070f5e27e5e92 = $(`<div id="html_39f81d956cb246b87fc070f5e27e5e92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff1855b32f2dba91d0af78cc96580ca8.setContent(html_39f81d956cb246b87fc070f5e27e5e92);\\n \\n \\n\\n circle_marker_a50e492992e5431d947530611f2b5cbe.bindPopup(popup_ff1855b32f2dba91d0af78cc96580ca8)\\n ;\\n\\n \\n \\n \\n var circle_marker_626ca9e527b8d66b4d12f940d8e90407 = L.circleMarker(\\n [42.72732269176802, -73.25995172914543],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7e8fb70b715f1e60d09a1e127d997908 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_20ea9f2fc121e8fc3f06646be61ef177 = $(`<div id="html_20ea9f2fc121e8fc3f06646be61ef177" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e8fb70b715f1e60d09a1e127d997908.setContent(html_20ea9f2fc121e8fc3f06646be61ef177);\\n \\n \\n\\n circle_marker_626ca9e527b8d66b4d12f940d8e90407.bindPopup(popup_7e8fb70b715f1e60d09a1e127d997908)\\n ;\\n\\n \\n \\n \\n var circle_marker_e59463c74b265e20208e831a02bad81a = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_108b1e6397b510fd5de3b9f349f5394b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b2f2f0959fed655b99e0fd7744e834a = $(`<div id="html_8b2f2f0959fed655b99e0fd7744e834a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_108b1e6397b510fd5de3b9f349f5394b.setContent(html_8b2f2f0959fed655b99e0fd7744e834a);\\n \\n \\n\\n circle_marker_e59463c74b265e20208e831a02bad81a.bindPopup(popup_108b1e6397b510fd5de3b9f349f5394b)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d0cc8a256aa18e680c2265ed38f5bc1 = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9e9028f4e1ecab31d5c86083b4d1dfc3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17222fb282359665b0be62750b2c0d1a = $(`<div id="html_17222fb282359665b0be62750b2c0d1a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e9028f4e1ecab31d5c86083b4d1dfc3.setContent(html_17222fb282359665b0be62750b2c0d1a);\\n \\n \\n\\n circle_marker_8d0cc8a256aa18e680c2265ed38f5bc1.bindPopup(popup_9e9028f4e1ecab31d5c86083b4d1dfc3)\\n ;\\n\\n \\n \\n \\n var circle_marker_01b96645a4709ffc4ecc5dcbc04a27b4 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1b9b0a3777077107431cf95d61cc63c3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_039d56e7064557f191fbfe75e1a0fa81 = $(`<div id="html_039d56e7064557f191fbfe75e1a0fa81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b9b0a3777077107431cf95d61cc63c3.setContent(html_039d56e7064557f191fbfe75e1a0fa81);\\n \\n \\n\\n circle_marker_01b96645a4709ffc4ecc5dcbc04a27b4.bindPopup(popup_1b9b0a3777077107431cf95d61cc63c3)\\n ;\\n\\n \\n \\n \\n var circle_marker_222f742b1639c72dc8adc752f50f0d33 = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.3336224206596095, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_73027a887c3143412d36b46cfc5aba4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b147bec3c4542968a30be6d12d954cb3 = $(`<div id="html_b147bec3c4542968a30be6d12d954cb3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73027a887c3143412d36b46cfc5aba4f.setContent(html_b147bec3c4542968a30be6d12d954cb3);\\n \\n \\n\\n circle_marker_222f742b1639c72dc8adc752f50f0d33.bindPopup(popup_73027a887c3143412d36b46cfc5aba4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_0bef3b093f6f91dec26cf333a85fc359 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ebdd5b4ef912c0fb2209e15262d6591d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b7d75519734faa43f24a87917974e29c = $(`<div id="html_b7d75519734faa43f24a87917974e29c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebdd5b4ef912c0fb2209e15262d6591d.setContent(html_b7d75519734faa43f24a87917974e29c);\\n \\n \\n\\n circle_marker_0bef3b093f6f91dec26cf333a85fc359.bindPopup(popup_ebdd5b4ef912c0fb2209e15262d6591d)\\n ;\\n\\n \\n \\n \\n var circle_marker_10ad91a51366243a6b8484b1d6b4f2ff = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_cb6fff9768c852f250126b5f2f2aed98 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e5afe1cf706aeb90b4e8b06ce49b439 = $(`<div id="html_6e5afe1cf706aeb90b4e8b06ce49b439" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb6fff9768c852f250126b5f2f2aed98.setContent(html_6e5afe1cf706aeb90b4e8b06ce49b439);\\n \\n \\n\\n circle_marker_10ad91a51366243a6b8484b1d6b4f2ff.bindPopup(popup_cb6fff9768c852f250126b5f2f2aed98)\\n ;\\n\\n \\n \\n \\n var circle_marker_17224dc0bcc673cb7136f1b01780c885 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_976cc5dd4140f8e6fc7ee3608f3557fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cdbfaab12dc3cd281b2f27715fd4eed3 = $(`<div id="html_cdbfaab12dc3cd281b2f27715fd4eed3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_976cc5dd4140f8e6fc7ee3608f3557fd.setContent(html_cdbfaab12dc3cd281b2f27715fd4eed3);\\n \\n \\n\\n circle_marker_17224dc0bcc673cb7136f1b01780c885.bindPopup(popup_976cc5dd4140f8e6fc7ee3608f3557fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_2747dde87b86bd0d9a78ea9f1ec7c51f = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_72c9049874a5ad382e5e22210896b87a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ecdb2aa5ed1f0fde14bc75e4d87bc0b = $(`<div id="html_4ecdb2aa5ed1f0fde14bc75e4d87bc0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72c9049874a5ad382e5e22210896b87a.setContent(html_4ecdb2aa5ed1f0fde14bc75e4d87bc0b);\\n \\n \\n\\n circle_marker_2747dde87b86bd0d9a78ea9f1ec7c51f.bindPopup(popup_72c9049874a5ad382e5e22210896b87a)\\n ;\\n\\n \\n \\n \\n var circle_marker_05b0526d60076d3f8828f344d11216ae = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f987c6494bdc93a2b333bc79bacbbcb0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_817124a3e00abd840e60222a27d861c9 = $(`<div id="html_817124a3e00abd840e60222a27d861c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f987c6494bdc93a2b333bc79bacbbcb0.setContent(html_817124a3e00abd840e60222a27d861c9);\\n \\n \\n\\n circle_marker_05b0526d60076d3f8828f344d11216ae.bindPopup(popup_f987c6494bdc93a2b333bc79bacbbcb0)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1ebf5b9c745ba8721d45a1b87c4a73a = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_b399ab39fd19b73107f2444941d11d87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5cd97ecea9d9adb6bffe4387cd851191 = $(`<div id="html_5cd97ecea9d9adb6bffe4387cd851191" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b399ab39fd19b73107f2444941d11d87.setContent(html_5cd97ecea9d9adb6bffe4387cd851191);\\n \\n \\n\\n circle_marker_f1ebf5b9c745ba8721d45a1b87c4a73a.bindPopup(popup_b399ab39fd19b73107f2444941d11d87)\\n ;\\n\\n \\n \\n \\n var circle_marker_61d02251a2c3cec5bbf00e706c24f356 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_3bbb28041ae568cdfe48a381d1b9d1d0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57b81bdcbe911d72fb8def60a71ca79d = $(`<div id="html_57b81bdcbe911d72fb8def60a71ca79d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3bbb28041ae568cdfe48a381d1b9d1d0.setContent(html_57b81bdcbe911d72fb8def60a71ca79d);\\n \\n \\n\\n circle_marker_61d02251a2c3cec5bbf00e706c24f356.bindPopup(popup_3bbb28041ae568cdfe48a381d1b9d1d0)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe65c9772542b9e3b5a4aafb30bcca93 = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_1082b8540cd90cd8f7a79b06cd5bf37a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_580128c244d4ebd3bc62f46e6b9b0132 = $(`<div id="html_580128c244d4ebd3bc62f46e6b9b0132" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1082b8540cd90cd8f7a79b06cd5bf37a.setContent(html_580128c244d4ebd3bc62f46e6b9b0132);\\n \\n \\n\\n circle_marker_fe65c9772542b9e3b5a4aafb30bcca93.bindPopup(popup_1082b8540cd90cd8f7a79b06cd5bf37a)\\n ;\\n\\n \\n \\n \\n var circle_marker_b8dd9936a76dca2a1ecce607af184bc9 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_63897f691be55db392d6a5458793ae64 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2d6c9cddb8831137106676b4cb65d60 = $(`<div id="html_f2d6c9cddb8831137106676b4cb65d60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63897f691be55db392d6a5458793ae64.setContent(html_f2d6c9cddb8831137106676b4cb65d60);\\n \\n \\n\\n circle_marker_b8dd9936a76dca2a1ecce607af184bc9.bindPopup(popup_63897f691be55db392d6a5458793ae64)\\n ;\\n\\n \\n \\n \\n var circle_marker_0f6b64b10ba3c55b264958f745bba621 = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a8f962f9636a5cab70dc344853a8c6e6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a1ce158a0bb89cf21d5c5dff8a7ed07 = $(`<div id="html_4a1ce158a0bb89cf21d5c5dff8a7ed07" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8f962f9636a5cab70dc344853a8c6e6.setContent(html_4a1ce158a0bb89cf21d5c5dff8a7ed07);\\n \\n \\n\\n circle_marker_0f6b64b10ba3c55b264958f745bba621.bindPopup(popup_a8f962f9636a5cab70dc344853a8c6e6)\\n ;\\n\\n \\n \\n \\n var circle_marker_84939f8093f5ef7084e82452a04b7891 = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0ed018b65485aa3108fb774fc5ad7070 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd6b6e52f373c755111ef179a3c97cc6 = $(`<div id="html_fd6b6e52f373c755111ef179a3c97cc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0ed018b65485aa3108fb774fc5ad7070.setContent(html_fd6b6e52f373c755111ef179a3c97cc6);\\n \\n \\n\\n circle_marker_84939f8093f5ef7084e82452a04b7891.bindPopup(popup_0ed018b65485aa3108fb774fc5ad7070)\\n ;\\n\\n \\n \\n \\n var circle_marker_47570c090207ef3bada34cbb6af60c52 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_dbe0511694674742d65f5ecf385bc4f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f0d94d78cfb62fc16eb59608fb02623 = $(`<div id="html_1f0d94d78cfb62fc16eb59608fb02623" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dbe0511694674742d65f5ecf385bc4f9.setContent(html_1f0d94d78cfb62fc16eb59608fb02623);\\n \\n \\n\\n circle_marker_47570c090207ef3bada34cbb6af60c52.bindPopup(popup_dbe0511694674742d65f5ecf385bc4f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_646dcabf83b8aaf1140266e0539a3801 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_19ed3fc682b9c339f50e880bd18b83d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78aadd623cd527cd42bd9681fe47c7df = $(`<div id="html_78aadd623cd527cd42bd9681fe47c7df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19ed3fc682b9c339f50e880bd18b83d7.setContent(html_78aadd623cd527cd42bd9681fe47c7df);\\n \\n \\n\\n circle_marker_646dcabf83b8aaf1140266e0539a3801.bindPopup(popup_19ed3fc682b9c339f50e880bd18b83d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_70b49cdd380e7012594ca3c9ce1c05b2 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_8da49ae4810bcb399dd9f9126869851c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de422bbf032329506dc404441f9bbf87 = $(`<div id="html_de422bbf032329506dc404441f9bbf87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8da49ae4810bcb399dd9f9126869851c.setContent(html_de422bbf032329506dc404441f9bbf87);\\n \\n \\n\\n circle_marker_70b49cdd380e7012594ca3c9ce1c05b2.bindPopup(popup_8da49ae4810bcb399dd9f9126869851c)\\n ;\\n\\n \\n \\n \\n var circle_marker_aade0cba579ef49d59f46342551677f0 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c0943a101706de0fed1c424f6c9a43a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a92f295f636ed0571b9c84eecebf784 = $(`<div id="html_6a92f295f636ed0571b9c84eecebf784" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0943a101706de0fed1c424f6c9a43a6.setContent(html_6a92f295f636ed0571b9c84eecebf784);\\n \\n \\n\\n circle_marker_aade0cba579ef49d59f46342551677f0.bindPopup(popup_c0943a101706de0fed1c424f6c9a43a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_11ed41578b37986da3a588cae7e3d022 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.787307364817192, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_63691695147089d64e915e8aee23e2ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_058a01f5c7d8287a98499178d275b94f = $(`<div id="html_058a01f5c7d8287a98499178d275b94f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63691695147089d64e915e8aee23e2ea.setContent(html_058a01f5c7d8287a98499178d275b94f);\\n \\n \\n\\n circle_marker_11ed41578b37986da3a588cae7e3d022.bindPopup(popup_63691695147089d64e915e8aee23e2ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_457bf1b80f603bc1f4d7c9797cb89542 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ca68ad7170c4e46f65047a3bbec77216 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_680d676e8d298901672e298f18d5c65e = $(`<div id="html_680d676e8d298901672e298f18d5c65e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca68ad7170c4e46f65047a3bbec77216.setContent(html_680d676e8d298901672e298f18d5c65e);\\n \\n \\n\\n circle_marker_457bf1b80f603bc1f4d7c9797cb89542.bindPopup(popup_ca68ad7170c4e46f65047a3bbec77216)\\n ;\\n\\n \\n \\n \\n var circle_marker_4dc6967cffe8d1581704035568f6b474 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a8ce11d9e648649b23901412ac9689cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eeab8e31980d14d34bf22f64af627a19 = $(`<div id="html_eeab8e31980d14d34bf22f64af627a19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8ce11d9e648649b23901412ac9689cd.setContent(html_eeab8e31980d14d34bf22f64af627a19);\\n \\n \\n\\n circle_marker_4dc6967cffe8d1581704035568f6b474.bindPopup(popup_a8ce11d9e648649b23901412ac9689cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_56372f69d0ca71355042733ae6a62046 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4a5822303d3c3322dfe531e99c4d9c56 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92bd603df0b527483ae5d0d27d0c5719 = $(`<div id="html_92bd603df0b527483ae5d0d27d0c5719" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a5822303d3c3322dfe531e99c4d9c56.setContent(html_92bd603df0b527483ae5d0d27d0c5719);\\n \\n \\n\\n circle_marker_56372f69d0ca71355042733ae6a62046.bindPopup(popup_4a5822303d3c3322dfe531e99c4d9c56)\\n ;\\n\\n \\n \\n \\n var circle_marker_4dc6dab343a51062664bb01ef4715329 = L.circleMarker(\\n [42.725515657130885, -73.26610182668713],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_92a0d2165d069976d17292a89cf82ea4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_425513b66aeca3abbb4fc367dff8c40d = $(`<div id="html_425513b66aeca3abbb4fc367dff8c40d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_92a0d2165d069976d17292a89cf82ea4.setContent(html_425513b66aeca3abbb4fc367dff8c40d);\\n \\n \\n\\n circle_marker_4dc6dab343a51062664bb01ef4715329.bindPopup(popup_92a0d2165d069976d17292a89cf82ea4)\\n ;\\n\\n \\n \\n \\n var circle_marker_0fe446323093f92b98704cb7d9513868 = L.circleMarker(\\n [42.72551566371306, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_a85a44df8f28c4553cd4b7d1208b1772 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a6023101e4f0f9c6f20dfb589bb00c6 = $(`<div id="html_9a6023101e4f0f9c6f20dfb589bb00c6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a85a44df8f28c4553cd4b7d1208b1772.setContent(html_9a6023101e4f0f9c6f20dfb589bb00c6);\\n \\n \\n\\n circle_marker_0fe446323093f92b98704cb7d9513868.bindPopup(popup_a85a44df8f28c4553cd4b7d1208b1772)\\n ;\\n\\n \\n \\n \\n var circle_marker_84f26327f37a6c45edadbd8c579eaa79 = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2d047c2a7c62a32517ef6c4a615be15e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e91ff62a955bccd83405cafb9a67e5e = $(`<div id="html_8e91ff62a955bccd83405cafb9a67e5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d047c2a7c62a32517ef6c4a615be15e.setContent(html_8e91ff62a955bccd83405cafb9a67e5e);\\n \\n \\n\\n circle_marker_84f26327f37a6c45edadbd8c579eaa79.bindPopup(popup_2d047c2a7c62a32517ef6c4a615be15e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a564aef2a81d964a4a67a25cfec3f2b4 = L.circleMarker(\\n [42.72551563738435, -73.26241185416787],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_174ab1a84535904d20d2bbee6311b906 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83de4ba8e661f53bb0fcbf73d56b1149 = $(`<div id="html_83de4ba8e661f53bb0fcbf73d56b1149" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_174ab1a84535904d20d2bbee6311b906.setContent(html_83de4ba8e661f53bb0fcbf73d56b1149);\\n \\n \\n\\n circle_marker_a564aef2a81d964a4a67a25cfec3f2b4.bindPopup(popup_174ab1a84535904d20d2bbee6311b906)\\n ;\\n\\n \\n \\n \\n var circle_marker_851c6634ee9a459b2abfbc677f5b75c0 = L.circleMarker(\\n [42.72551560447347, -73.26118186332953],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c472139af125b2a25f5e2885d6d4974c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1770f9788d86264df791c983db020a92 = $(`<div id="html_1770f9788d86264df791c983db020a92" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c472139af125b2a25f5e2885d6d4974c.setContent(html_1770f9788d86264df791c983db020a92);\\n \\n \\n\\n circle_marker_851c6634ee9a459b2abfbc677f5b75c0.bindPopup(popup_c472139af125b2a25f5e2885d6d4974c)\\n ;\\n\\n \\n \\n \\n var circle_marker_95b1587abe4d3817e56970474a5bd001 = L.circleMarker(\\n [42.72551555839823, -73.25995187249272],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_5ec8dbd65bd408fa6d5e87aec6d8e4b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50fbd4989739c94b0d880eee1ecb4e15 = $(`<div id="html_50fbd4989739c94b0d880eee1ecb4e15" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ec8dbd65bd408fa6d5e87aec6d8e4b7.setContent(html_50fbd4989739c94b0d880eee1ecb4e15);\\n \\n \\n\\n circle_marker_95b1587abe4d3817e56970474a5bd001.bindPopup(popup_5ec8dbd65bd408fa6d5e87aec6d8e4b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5ca21fdb6d1841a889ad5f9f37b887d = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_03376fd39bf9cc75689a0e866134a14e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f6079a7618b2c7927464430ec7813d7 = $(`<div id="html_6f6079a7618b2c7927464430ec7813d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03376fd39bf9cc75689a0e866134a14e.setContent(html_6f6079a7618b2c7927464430ec7813d7);\\n \\n \\n\\n circle_marker_a5ca21fdb6d1841a889ad5f9f37b887d.bindPopup(popup_03376fd39bf9cc75689a0e866134a14e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d20d85cb1ee4fbe610c3b904fe7b252f = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_612781a339ec5aae58d1e76ac27bb775 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58800906f862dce1d12f39488b1fc97a = $(`<div id="html_58800906f862dce1d12f39488b1fc97a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_612781a339ec5aae58d1e76ac27bb775.setContent(html_58800906f862dce1d12f39488b1fc97a);\\n \\n \\n\\n circle_marker_d20d85cb1ee4fbe610c3b904fe7b252f.bindPopup(popup_612781a339ec5aae58d1e76ac27bb775)\\n ;\\n\\n \\n \\n \\n var circle_marker_eebe167b3b3bf890be1e143dac7297b2 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_03ea88abde45c7c015d6047a0e864a37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ffef3e250d43d929b7f8fd1c3d0f3b9d = $(`<div id="html_ffef3e250d43d929b7f8fd1c3d0f3b9d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03ea88abde45c7c015d6047a0e864a37.setContent(html_ffef3e250d43d929b7f8fd1c3d0f3b9d);\\n \\n \\n\\n circle_marker_eebe167b3b3bf890be1e143dac7297b2.bindPopup(popup_03ea88abde45c7c015d6047a0e864a37)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ac76108a969102757637918b1034dc5 = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.949327084834294, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f97a3fe7c1aed7e854d75273bffef7e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_869e95033390d5eaea9436b68be54602 = $(`<div id="html_869e95033390d5eaea9436b68be54602" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f97a3fe7c1aed7e854d75273bffef7e3.setContent(html_869e95033390d5eaea9436b68be54602);\\n \\n \\n\\n circle_marker_2ac76108a969102757637918b1034dc5.bindPopup(popup_f97a3fe7c1aed7e854d75273bffef7e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7ba3c978cf12961c97c4bc5e44e9059 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_044ccfb97857a6b2c65fa6af7d6fd024 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8484de1e0def715db2bcb7e209e24fe = $(`<div id="html_c8484de1e0def715db2bcb7e209e24fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_044ccfb97857a6b2c65fa6af7d6fd024.setContent(html_c8484de1e0def715db2bcb7e209e24fe);\\n \\n \\n\\n circle_marker_f7ba3c978cf12961c97c4bc5e44e9059.bindPopup(popup_044ccfb97857a6b2c65fa6af7d6fd024)\\n ;\\n\\n \\n \\n \\n var circle_marker_0946304c246895a1155435e00db34efe = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.440847306724618, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_2fff8afac3d8b5ff5ec86cfdd0d94d7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8279267b7686188959301a1497577272 = $(`<div id="html_8279267b7686188959301a1497577272" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2fff8afac3d8b5ff5ec86cfdd0d94d7b.setContent(html_8279267b7686188959301a1497577272);\\n \\n \\n\\n circle_marker_0946304c246895a1155435e00db34efe.bindPopup(popup_2fff8afac3d8b5ff5ec86cfdd0d94d7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6302cd4bd073e70de7ed0aa3f2e4056 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9fcb3d47b44f2aaf2295e8e079e807ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf12c22e9e5db2197219ff109daed496 = $(`<div id="html_bf12c22e9e5db2197219ff109daed496" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fcb3d47b44f2aaf2295e8e079e807ba.setContent(html_bf12c22e9e5db2197219ff109daed496);\\n \\n \\n\\n circle_marker_d6302cd4bd073e70de7ed0aa3f2e4056.bindPopup(popup_9fcb3d47b44f2aaf2295e8e079e807ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_5207d92afc980995632a8405f0bb1d70 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.232078956954491, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_c3eeb2d142db0fcf267ddc2d8e84aa5f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a85f7926a54e23adf21af7424e823a5 = $(`<div id="html_8a85f7926a54e23adf21af7424e823a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c3eeb2d142db0fcf267ddc2d8e84aa5f.setContent(html_8a85f7926a54e23adf21af7424e823a5);\\n \\n \\n\\n circle_marker_5207d92afc980995632a8405f0bb1d70.bindPopup(popup_c3eeb2d142db0fcf267ddc2d8e84aa5f)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d947a3b8e696d697204f04c8bfe50e2 = L.circleMarker(\\n [42.725513531087856, -73.24273200123443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4a8d30a9b2e4b544ab0fed7874c83dbf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82e9316057f96d18baa0681c7bb58ef6 = $(`<div id="html_82e9316057f96d18baa0681c7bb58ef6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a8d30a9b2e4b544ab0fed7874c83dbf.setContent(html_82e9316057f96d18baa0681c7bb58ef6);\\n \\n \\n\\n circle_marker_5d947a3b8e696d697204f04c8bfe50e2.bindPopup(popup_4a8d30a9b2e4b544ab0fed7874c83dbf)\\n ;\\n\\n \\n \\n \\n var circle_marker_7dd1bca9e79d34888e30de8bd5aa4f71 = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_70e8a65ce9f0486d700066113f6fc4b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b630fc0ae98612993806c5d19b2674c4 = $(`<div id="html_b630fc0ae98612993806c5d19b2674c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_70e8a65ce9f0486d700066113f6fc4b4.setContent(html_b630fc0ae98612993806c5d19b2674c4);\\n \\n \\n\\n circle_marker_7dd1bca9e79d34888e30de8bd5aa4f71.bindPopup(popup_70e8a65ce9f0486d700066113f6fc4b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_4638e5c0e37321c0c2a86604da5f756d = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_83cdc16d977c0632ddd23ba0ccefd68e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b190cfe6170b34687e5ae0cb2160bb42 = $(`<div id="html_b190cfe6170b34687e5ae0cb2160bb42" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_83cdc16d977c0632ddd23ba0ccefd68e.setContent(html_b190cfe6170b34687e5ae0cb2160bb42);\\n \\n \\n\\n circle_marker_4638e5c0e37321c0c2a86604da5f756d.bindPopup(popup_83cdc16d977c0632ddd23ba0ccefd68e)\\n ;\\n\\n \\n \\n \\n var circle_marker_54ebfa4665e5f3108906edbe833bca4a = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_de524941ea6b8365f206c42debb56a46 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_173805f483d29933066a7c05bf991e74 = $(`<div id="html_173805f483d29933066a7c05bf991e74" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de524941ea6b8365f206c42debb56a46.setContent(html_173805f483d29933066a7c05bf991e74);\\n \\n \\n\\n circle_marker_54ebfa4665e5f3108906edbe833bca4a.bindPopup(popup_de524941ea6b8365f206c42debb56a46)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b463388033d083092168a6d4cfe68cd = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9e758e63b4f00d8012a4cecdbaa086d2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10ebe0d185b740e51019a74ce6f1bf91 = $(`<div id="html_10ebe0d185b740e51019a74ce6f1bf91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e758e63b4f00d8012a4cecdbaa086d2.setContent(html_10ebe0d185b740e51019a74ce6f1bf91);\\n \\n \\n\\n circle_marker_2b463388033d083092168a6d4cfe68cd.bindPopup(popup_9e758e63b4f00d8012a4cecdbaa086d2)\\n ;\\n\\n \\n \\n \\n var circle_marker_e645d02bd4084cbfa551c85bb1f97a70 = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_dbb8abb92dc80555c027b2ef652ce448 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f2c4356644fe55d41a2d390af5414d5 = $(`<div id="html_3f2c4356644fe55d41a2d390af5414d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dbb8abb92dc80555c027b2ef652ce448.setContent(html_3f2c4356644fe55d41a2d390af5414d5);\\n \\n \\n\\n circle_marker_e645d02bd4084cbfa551c85bb1f97a70.bindPopup(popup_dbb8abb92dc80555c027b2ef652ce448)\\n ;\\n\\n \\n \\n \\n var circle_marker_339d0bd23a60a3430133927f407d7ceb = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_e6017be50b57c119941bc0f485052bd7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f5ce95e2c455cded8b2cbba34173385 = $(`<div id="html_3f5ce95e2c455cded8b2cbba34173385" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6017be50b57c119941bc0f485052bd7.setContent(html_3f5ce95e2c455cded8b2cbba34173385);\\n \\n \\n\\n circle_marker_339d0bd23a60a3430133927f407d7ceb.bindPopup(popup_e6017be50b57c119941bc0f485052bd7)\\n ;\\n\\n \\n \\n \\n var circle_marker_65c563af31abe6c39d8230e869a9574e = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_ef37724c50dd023314adc82da5f0ff43 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8aba6d13cccdd88cb8f69c0fa3094284 = $(`<div id="html_8aba6d13cccdd88cb8f69c0fa3094284" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef37724c50dd023314adc82da5f0ff43.setContent(html_8aba6d13cccdd88cb8f69c0fa3094284);\\n \\n \\n\\n circle_marker_65c563af31abe6c39d8230e869a9574e.bindPopup(popup_ef37724c50dd023314adc82da5f0ff43)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a80d3818fe95290780d049742f07658 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_4e6835b5ca4437aeb3af31aaa22d6bd4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76644ca910e3910eb0a0ed411a933ba7 = $(`<div id="html_76644ca910e3910eb0a0ed411a933ba7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e6835b5ca4437aeb3af31aaa22d6bd4.setContent(html_76644ca910e3910eb0a0ed411a933ba7);\\n \\n \\n\\n circle_marker_1a80d3818fe95290780d049742f07658.bindPopup(popup_4e6835b5ca4437aeb3af31aaa22d6bd4)\\n ;\\n\\n \\n \\n \\n var circle_marker_77b1d46a0d67c967fac40e7500451676 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_69ce107f7c467ef3060440a4dfa3c794 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e41b486c59c1bc0a475e223e1be5a24 = $(`<div id="html_9e41b486c59c1bc0a475e223e1be5a24" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69ce107f7c467ef3060440a4dfa3c794.setContent(html_9e41b486c59c1bc0a475e223e1be5a24);\\n \\n \\n\\n circle_marker_77b1d46a0d67c967fac40e7500451676.bindPopup(popup_69ce107f7c467ef3060440a4dfa3c794)\\n ;\\n\\n \\n \\n \\n var circle_marker_7520296dd0dda1bc627aefef0d2023f3 = L.circleMarker(\\n [42.72370842502844, -73.2599520158284],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.068428945128219, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_7317b6ca32d67420b117144e1ed4e927 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b7cbb14a3834488962dbabb1afa4e02 = $(`<div id="html_0b7cbb14a3834488962dbabb1afa4e02" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7317b6ca32d67420b117144e1ed4e927.setContent(html_0b7cbb14a3834488962dbabb1afa4e02);\\n \\n \\n\\n circle_marker_7520296dd0dda1bc627aefef0d2023f3.bindPopup(popup_7317b6ca32d67420b117144e1ed4e927)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e2f3239724e1a54877497af7e04a334 = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_385647348301700b66b6fc320741a6a5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c20578cc018943a114ba51632ec1b825 = $(`<div id="html_c20578cc018943a114ba51632ec1b825" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_385647348301700b66b6fc320741a6a5.setContent(html_c20578cc018943a114ba51632ec1b825);\\n \\n \\n\\n circle_marker_9e2f3239724e1a54877497af7e04a334.bindPopup(popup_385647348301700b66b6fc320741a6a5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5d1a98510032ab3f67e2026bafa82a2 = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_6a6220cb47bec71506c960381116979b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1201cb027fdc7a798749a64f0fe5ecea = $(`<div id="html_1201cb027fdc7a798749a64f0fe5ecea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a6220cb47bec71506c960381116979b.setContent(html_1201cb027fdc7a798749a64f0fe5ecea);\\n \\n \\n\\n circle_marker_a5d1a98510032ab3f67e2026bafa82a2.bindPopup(popup_6a6220cb47bec71506c960381116979b)\\n ;\\n\\n \\n \\n \\n var circle_marker_63dc08a8cc4b85f62728d8005373e3e4 = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9a2c356fba04a660d77ff6c6831b479a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3ce0bceceb421310ef20ff4c2a12ada0 = $(`<div id="html_3ce0bceceb421310ef20ff4c2a12ada0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a2c356fba04a660d77ff6c6831b479a.setContent(html_3ce0bceceb421310ef20ff4c2a12ada0);\\n \\n \\n\\n circle_marker_63dc08a8cc4b85f62728d8005373e3e4.bindPopup(popup_9a2c356fba04a660d77ff6c6831b479a)\\n ;\\n\\n \\n \\n \\n var circle_marker_472dff90023c3cf1f91c31e625dd07b2 = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.145929793656026, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_348ae968d6c4d425d5daa4f99d29e239 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48ca85d37aa6fab4049d84b94456ac3e = $(`<div id="html_48ca85d37aa6fab4049d84b94456ac3e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_348ae968d6c4d425d5daa4f99d29e239.setContent(html_48ca85d37aa6fab4049d84b94456ac3e);\\n \\n \\n\\n circle_marker_472dff90023c3cf1f91c31e625dd07b2.bindPopup(popup_348ae968d6c4d425d5daa4f99d29e239)\\n ;\\n\\n \\n \\n \\n var circle_marker_e2e788a4eea41bf5ae7700350e38b8a2 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_d10f37ddfac7ed60f895e3d6b3a6bed1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eed777ec931afb9717a039226d70db66 = $(`<div id="html_eed777ec931afb9717a039226d70db66" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d10f37ddfac7ed60f895e3d6b3a6bed1.setContent(html_eed777ec931afb9717a039226d70db66);\\n \\n \\n\\n circle_marker_e2e788a4eea41bf5ae7700350e38b8a2.bindPopup(popup_d10f37ddfac7ed60f895e3d6b3a6bed1)\\n ;\\n\\n \\n \\n \\n var circle_marker_209585b203ef1de7c38028c548ad63b9 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_9c146b734fad356347cfd35155691b6f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a43dbdab681af1bc33679e83bf6a288 = $(`<div id="html_9a43dbdab681af1bc33679e83bf6a288" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9c146b734fad356347cfd35155691b6f.setContent(html_9a43dbdab681af1bc33679e83bf6a288);\\n \\n \\n\\n circle_marker_209585b203ef1de7c38028c548ad63b9.bindPopup(popup_9c146b734fad356347cfd35155691b6f)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e5e600b5e3939df7bacc0721bede1f4 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_f365b004dbd3ef27108cbd4043a4827a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3619885f54dfdf6f5673d12e7f829b49 = $(`<div id="html_3619885f54dfdf6f5673d12e7f829b49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f365b004dbd3ef27108cbd4043a4827a.setContent(html_3619885f54dfdf6f5673d12e7f829b49);\\n \\n \\n\\n circle_marker_8e5e600b5e3939df7bacc0721bede1f4.bindPopup(popup_f365b004dbd3ef27108cbd4043a4827a)\\n ;\\n\\n \\n \\n \\n var circle_marker_468032566eb81509d829004646a3d597 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_26545a85bb6c1aa612454c4160cb6eaf);\\n \\n \\n var popup_0d091456f38915fe0cfa65db796f56fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b86faa24f4b30750fddccdb992cd693 = $(`<div id="html_0b86faa24f4b30750fddccdb992cd693" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d091456f38915fe0cfa65db796f56fe.setContent(html_0b86faa24f4b30750fddccdb992cd693);\\n \\n \\n\\n circle_marker_468032566eb81509d829004646a3d597.bindPopup(popup_0d091456f38915fe0cfa65db796f56fe)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Maple, sugar\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_91ef6e2ae17f39e5ddd0f1fa75ca7963 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_91ef6e2ae17f39e5ddd0f1fa75ca7963" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_91ef6e2ae17f39e5ddd0f1fa75ca7963 = L.map(\\n "map_91ef6e2ae17f39e5ddd0f1fa75ca7963",\\n {\\n center: [42.73545173466282, -73.24764986998687],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_3d93057248be1d744a52d5542a609366 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var circle_marker_e5927dc7f3576c89f2f07bde00b59ef0 = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_711da258fcbc3b781883cb6fcc08e418 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6ca0c8fe96353c7399282e55ab60009 = $(`<div id="html_f6ca0c8fe96353c7399282e55ab60009" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_711da258fcbc3b781883cb6fcc08e418.setContent(html_f6ca0c8fe96353c7399282e55ab60009);\\n \\n \\n\\n circle_marker_e5927dc7f3576c89f2f07bde00b59ef0.bindPopup(popup_711da258fcbc3b781883cb6fcc08e418)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd0f8b13fc37dd8b190dc5078da999ae = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_22df6e22ddef4be6153f3c38b7281872 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8691b985d412f7c17f346ab541b21c1e = $(`<div id="html_8691b985d412f7c17f346ab541b21c1e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22df6e22ddef4be6153f3c38b7281872.setContent(html_8691b985d412f7c17f346ab541b21c1e);\\n \\n \\n\\n circle_marker_fd0f8b13fc37dd8b190dc5078da999ae.bindPopup(popup_22df6e22ddef4be6153f3c38b7281872)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6aa9ddc88da17ac9dfaf95dc6182d5d = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_444c3952537dda8e600f2a82c125489d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92cff88cfca0085e9e5f4ce69ef99b16 = $(`<div id="html_92cff88cfca0085e9e5f4ce69ef99b16" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_444c3952537dda8e600f2a82c125489d.setContent(html_92cff88cfca0085e9e5f4ce69ef99b16);\\n \\n \\n\\n circle_marker_e6aa9ddc88da17ac9dfaf95dc6182d5d.bindPopup(popup_444c3952537dda8e600f2a82c125489d)\\n ;\\n\\n \\n \\n \\n var circle_marker_90dc30ba2dc2e0ef512ceb6d930a4f48 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1a865cc4e2ec2ce807f92cd4257a724a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78f30f99f6ce6e5c18fcfe0bf30dfe42 = $(`<div id="html_78f30f99f6ce6e5c18fcfe0bf30dfe42" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a865cc4e2ec2ce807f92cd4257a724a.setContent(html_78f30f99f6ce6e5c18fcfe0bf30dfe42);\\n \\n \\n\\n circle_marker_90dc30ba2dc2e0ef512ceb6d930a4f48.bindPopup(popup_1a865cc4e2ec2ce807f92cd4257a724a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c090f235cbb21ce3e6c7d158cf17db7b = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c99d33cbb958d3185d7c5476ecf4ea5e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4846bfc092bef57a5b835b55e91122b4 = $(`<div id="html_4846bfc092bef57a5b835b55e91122b4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c99d33cbb958d3185d7c5476ecf4ea5e.setContent(html_4846bfc092bef57a5b835b55e91122b4);\\n \\n \\n\\n circle_marker_c090f235cbb21ce3e6c7d158cf17db7b.bindPopup(popup_c99d33cbb958d3185d7c5476ecf4ea5e)\\n ;\\n\\n \\n \\n \\n var circle_marker_92350566814060da879262bac4939d76 = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_384b7784620cdccdc4a0422fa526719f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_72ddd58bfa2c73f14bb1e8646490ef60 = $(`<div id="html_72ddd58bfa2c73f14bb1e8646490ef60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_384b7784620cdccdc4a0422fa526719f.setContent(html_72ddd58bfa2c73f14bb1e8646490ef60);\\n \\n \\n\\n circle_marker_92350566814060da879262bac4939d76.bindPopup(popup_384b7784620cdccdc4a0422fa526719f)\\n ;\\n\\n \\n \\n \\n var circle_marker_1972f13e8cf37422edecfeeed5b78eba = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.61809077155419, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_69b752fba1dad4a7f4e38440e791579c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_279dcafe0a00ed9ba7876d84cdee964e = $(`<div id="html_279dcafe0a00ed9ba7876d84cdee964e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69b752fba1dad4a7f4e38440e791579c.setContent(html_279dcafe0a00ed9ba7876d84cdee964e);\\n \\n \\n\\n circle_marker_1972f13e8cf37422edecfeeed5b78eba.bindPopup(popup_69b752fba1dad4a7f4e38440e791579c)\\n ;\\n\\n \\n \\n \\n var circle_marker_d49bb5b908eaa2bf1e64a3000f2b5d7b = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_17f324f6918cb700a660d9e480936954 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b8812e4dc65386c029ba5a36444266d4 = $(`<div id="html_b8812e4dc65386c029ba5a36444266d4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_17f324f6918cb700a660d9e480936954.setContent(html_b8812e4dc65386c029ba5a36444266d4);\\n \\n \\n\\n circle_marker_d49bb5b908eaa2bf1e64a3000f2b5d7b.bindPopup(popup_17f324f6918cb700a660d9e480936954)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e63ea3435a71fd7a33545db4c466b33 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6f90b05931caa23391a712a4bad72881 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1237f6ac44f91bacd9acaa4bbce25cca = $(`<div id="html_1237f6ac44f91bacd9acaa4bbce25cca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f90b05931caa23391a712a4bad72881.setContent(html_1237f6ac44f91bacd9acaa4bbce25cca);\\n \\n \\n\\n circle_marker_1e63ea3435a71fd7a33545db4c466b33.bindPopup(popup_6f90b05931caa23391a712a4bad72881)\\n ;\\n\\n \\n \\n \\n var circle_marker_5fb47db4154e3908e0eeb35c39ff57a4 = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c89cc4f3fffde071061b65c97aab09e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1d2d13526f2197f44e33e76e9b2b799b = $(`<div id="html_1d2d13526f2197f44e33e76e9b2b799b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c89cc4f3fffde071061b65c97aab09e9.setContent(html_1d2d13526f2197f44e33e76e9b2b799b);\\n \\n \\n\\n circle_marker_5fb47db4154e3908e0eeb35c39ff57a4.bindPopup(popup_c89cc4f3fffde071061b65c97aab09e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ebb1f1f728f99cb7e5aedfbce7c7b78 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_098e8519c3d919181535c9221019c66e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_11a84032b618f7a9aaab09f0257e3b0a = $(`<div id="html_11a84032b618f7a9aaab09f0257e3b0a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_098e8519c3d919181535c9221019c66e.setContent(html_11a84032b618f7a9aaab09f0257e3b0a);\\n \\n \\n\\n circle_marker_7ebb1f1f728f99cb7e5aedfbce7c7b78.bindPopup(popup_098e8519c3d919181535c9221019c66e)\\n ;\\n\\n \\n \\n \\n var circle_marker_14704f88f46e7b49681da746bdb97939 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7f66253d0840936fc3cf0cc3e1805551 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f3315843e23703ba91c2eb63db477a3 = $(`<div id="html_8f3315843e23703ba91c2eb63db477a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f66253d0840936fc3cf0cc3e1805551.setContent(html_8f3315843e23703ba91c2eb63db477a3);\\n \\n \\n\\n circle_marker_14704f88f46e7b49681da746bdb97939.bindPopup(popup_7f66253d0840936fc3cf0cc3e1805551)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd68c80166ac6b8e73d88b35932d5b15 = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_65bfd7610894536b96151f0410d6080f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_746fd48816579edb5a26e5befd7a6eb8 = $(`<div id="html_746fd48816579edb5a26e5befd7a6eb8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65bfd7610894536b96151f0410d6080f.setContent(html_746fd48816579edb5a26e5befd7a6eb8);\\n \\n \\n\\n circle_marker_fd68c80166ac6b8e73d88b35932d5b15.bindPopup(popup_65bfd7610894536b96151f0410d6080f)\\n ;\\n\\n \\n \\n \\n var circle_marker_58ed9a14aa42b08a0a94f5c90fd211ef = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_77afa3654f7e5a25c3682fa747bbb10a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dfe4ef04b657139a8fc489919b498003 = $(`<div id="html_dfe4ef04b657139a8fc489919b498003" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77afa3654f7e5a25c3682fa747bbb10a.setContent(html_dfe4ef04b657139a8fc489919b498003);\\n \\n \\n\\n circle_marker_58ed9a14aa42b08a0a94f5c90fd211ef.bindPopup(popup_77afa3654f7e5a25c3682fa747bbb10a)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b209997903034c31983cdce77f1c849 = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e03f4b1971990a89d9c940ed03f178d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e7f71a8e87b1b85fffc75257f6faf38 = $(`<div id="html_1e7f71a8e87b1b85fffc75257f6faf38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e03f4b1971990a89d9c940ed03f178d3.setContent(html_1e7f71a8e87b1b85fffc75257f6faf38);\\n \\n \\n\\n circle_marker_2b209997903034c31983cdce77f1c849.bindPopup(popup_e03f4b1971990a89d9c940ed03f178d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ff554b2cc7af264bbfeace041568940 = L.circleMarker(\\n [42.74539359743371, -73.27594530262718],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ec98fef12688c82316d3625574a3fbcd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c4cb9cd7e57b4435fd504160a51ac77a = $(`<div id="html_c4cb9cd7e57b4435fd504160a51ac77a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec98fef12688c82316d3625574a3fbcd.setContent(html_c4cb9cd7e57b4435fd504160a51ac77a);\\n \\n \\n\\n circle_marker_0ff554b2cc7af264bbfeace041568940.bindPopup(popup_ec98fef12688c82316d3625574a3fbcd)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e8a7a4a3a3d07353574278d396b85fa = L.circleMarker(\\n [42.74539347231661, -73.2771756878084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_783f8a06ecc22bff941304be9112ce18 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ba0c218949e3ce75024e2ef4c3c8392 = $(`<div id="html_8ba0c218949e3ce75024e2ef4c3c8392" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_783f8a06ecc22bff941304be9112ce18.setContent(html_8ba0c218949e3ce75024e2ef4c3c8392);\\n \\n \\n\\n circle_marker_4e8a7a4a3a3d07353574278d396b85fa.bindPopup(popup_783f8a06ecc22bff941304be9112ce18)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1973556c39be879f722f1c2611bacb4 = L.circleMarker(\\n [42.74539370938061, -73.27471491744124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_68c79f32fcb4fe01f156da45de24b823 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28056e7f2b35f71559346cee21c8b6fc = $(`<div id="html_28056e7f2b35f71559346cee21c8b6fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68c79f32fcb4fe01f156da45de24b823.setContent(html_28056e7f2b35f71559346cee21c8b6fc);\\n \\n \\n\\n circle_marker_b1973556c39be879f722f1c2611bacb4.bindPopup(popup_68c79f32fcb4fe01f156da45de24b823)\\n ;\\n\\n \\n \\n \\n var circle_marker_b165582a7f570e1cf6cc2024dee3477d = L.circleMarker(\\n [42.74539380815728, -73.27348453225115],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_305a41a49091b434a4b653f857c6bf07 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ec6053e67a037cfffcca185d722dd3a = $(`<div id="html_8ec6053e67a037cfffcca185d722dd3a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_305a41a49091b434a4b653f857c6bf07.setContent(html_8ec6053e67a037cfffcca185d722dd3a);\\n \\n \\n\\n circle_marker_b165582a7f570e1cf6cc2024dee3477d.bindPopup(popup_305a41a49091b434a4b653f857c6bf07)\\n ;\\n\\n \\n \\n \\n var circle_marker_726eb68ed6f569fac38242e81bce2f97 = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e78f92829f1f5bf236775ad24a1d85aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f0ed69e93beb715ddcd36e6590e232af = $(`<div id="html_f0ed69e93beb715ddcd36e6590e232af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e78f92829f1f5bf236775ad24a1d85aa.setContent(html_f0ed69e93beb715ddcd36e6590e232af);\\n \\n \\n\\n circle_marker_726eb68ed6f569fac38242e81bce2f97.bindPopup(popup_e78f92829f1f5bf236775ad24a1d85aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ed9ca068b3d4867ef1b4bb7b096370c = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_96cf1cc6391719cc7462c568f5a50b6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_144b09e4c6e81e56036aad41462aaf43 = $(`<div id="html_144b09e4c6e81e56036aad41462aaf43" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96cf1cc6391719cc7462c568f5a50b6a.setContent(html_144b09e4c6e81e56036aad41462aaf43);\\n \\n \\n\\n circle_marker_0ed9ca068b3d4867ef1b4bb7b096370c.bindPopup(popup_96cf1cc6391719cc7462c568f5a50b6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_80e99a3d361c574f97b15185a031aae9 = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9dfa586fcd97b522137a006b2e4e621d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a0b3ebdb83bfb54de73e33fb61ea065 = $(`<div id="html_6a0b3ebdb83bfb54de73e33fb61ea065" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9dfa586fcd97b522137a006b2e4e621d.setContent(html_6a0b3ebdb83bfb54de73e33fb61ea065);\\n \\n \\n\\n circle_marker_80e99a3d361c574f97b15185a031aae9.bindPopup(popup_9dfa586fcd97b522137a006b2e4e621d)\\n ;\\n\\n \\n \\n \\n var circle_marker_24166792e40400442f0c36adbce4a904 = L.circleMarker(\\n [42.74539407156172, -73.26856299145935],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_5a5a9d55bc715846a1fca4ccfc055e5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dcff85f3bf3b95de5e6bef9344c664ba = $(`<div id="html_dcff85f3bf3b95de5e6bef9344c664ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5a5a9d55bc715846a1fca4ccfc055e5a.setContent(html_dcff85f3bf3b95de5e6bef9344c664ba);\\n \\n \\n\\n circle_marker_24166792e40400442f0c36adbce4a904.bindPopup(popup_5a5a9d55bc715846a1fca4ccfc055e5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4692b32061679fa04cc026298d21521 = L.circleMarker(\\n [42.74539410448727, -73.26733260625618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_eb46ffc0f20899c81246e64097b16250 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d36c29c50f587989b46ae57af2418e4e = $(`<div id="html_d36c29c50f587989b46ae57af2418e4e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb46ffc0f20899c81246e64097b16250.setContent(html_d36c29c50f587989b46ae57af2418e4e);\\n \\n \\n\\n circle_marker_a4692b32061679fa04cc026298d21521.bindPopup(popup_eb46ffc0f20899c81246e64097b16250)\\n ;\\n\\n \\n \\n \\n var circle_marker_27774e7bb2e26c9a3ad0e117994b4e50 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_aae1594f26139f3a5743926a12755c4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ee14a414ef42dd95f99caae9afaf992 = $(`<div id="html_6ee14a414ef42dd95f99caae9afaf992" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aae1594f26139f3a5743926a12755c4b.setContent(html_6ee14a414ef42dd95f99caae9afaf992);\\n \\n \\n\\n circle_marker_27774e7bb2e26c9a3ad0e117994b4e50.bindPopup(popup_aae1594f26139f3a5743926a12755c4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_160f8815bef17813acaf3efeecde8769 = L.circleMarker(\\n [42.74539413082771, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d97de5e94415aaf38501f6f01d873cea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4846a10d91ae55fd3cb71f00a5a349b = $(`<div id="html_d4846a10d91ae55fd3cb71f00a5a349b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d97de5e94415aaf38501f6f01d873cea.setContent(html_d4846a10d91ae55fd3cb71f00a5a349b);\\n \\n \\n\\n circle_marker_160f8815bef17813acaf3efeecde8769.bindPopup(popup_d97de5e94415aaf38501f6f01d873cea)\\n ;\\n\\n \\n \\n \\n var circle_marker_76faf345221d5c43657edbebaeadf0d9 = L.circleMarker(\\n [42.74539410448727, -73.26241106543824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f7348efd946ed7b1f79d295fe8ecc7a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d96866b190ca56167ce7b54fe8ede93 = $(`<div id="html_4d96866b190ca56167ce7b54fe8ede93" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7348efd946ed7b1f79d295fe8ecc7a9.setContent(html_4d96866b190ca56167ce7b54fe8ede93);\\n \\n \\n\\n circle_marker_76faf345221d5c43657edbebaeadf0d9.bindPopup(popup_f7348efd946ed7b1f79d295fe8ecc7a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_874f9de788a6d2aa2ec0cedd34c55f6f = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f347b3a30d9d48fc6749cb711bdcaf10 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_627e95bbf3541ab13e852890e42503b2 = $(`<div id="html_627e95bbf3541ab13e852890e42503b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f347b3a30d9d48fc6749cb711bdcaf10.setContent(html_627e95bbf3541ab13e852890e42503b2);\\n \\n \\n\\n circle_marker_874f9de788a6d2aa2ec0cedd34c55f6f.bindPopup(popup_f347b3a30d9d48fc6749cb711bdcaf10)\\n ;\\n\\n \\n \\n \\n var circle_marker_724b9440455f5ef56477e7602f550881 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0031b523afcb84c170f2b4d2c108b4a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_036d1cc1fbf2a5f0f6388af0811320bf = $(`<div id="html_036d1cc1fbf2a5f0f6388af0811320bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0031b523afcb84c170f2b4d2c108b4a9.setContent(html_036d1cc1fbf2a5f0f6388af0811320bf);\\n \\n \\n\\n circle_marker_724b9440455f5ef56477e7602f550881.bindPopup(popup_0031b523afcb84c170f2b4d2c108b4a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_70f7b7892a521bf5c6070b260dca52af = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_27f41d712a09c329d3bf1f5ab7d08783 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_178e8608c1344f36da9fc24109a0e448 = $(`<div id="html_178e8608c1344f36da9fc24109a0e448" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_27f41d712a09c329d3bf1f5ab7d08783.setContent(html_178e8608c1344f36da9fc24109a0e448);\\n \\n \\n\\n circle_marker_70f7b7892a521bf5c6070b260dca52af.bindPopup(popup_27f41d712a09c329d3bf1f5ab7d08783)\\n ;\\n\\n \\n \\n \\n var circle_marker_73236f12b56063b6e4d77badae16b14d = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d66b24fcef26fe8d9ccae703d66b683e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c4440a5efc7ecfa2a1a07dff127c5bc = $(`<div id="html_1c4440a5efc7ecfa2a1a07dff127c5bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d66b24fcef26fe8d9ccae703d66b683e.setContent(html_1c4440a5efc7ecfa2a1a07dff127c5bc);\\n \\n \\n\\n circle_marker_73236f12b56063b6e4d77badae16b14d.bindPopup(popup_d66b24fcef26fe8d9ccae703d66b683e)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e798173f5a94ba16affe32f08c28897 = L.circleMarker(\\n [42.74539380815728, -73.25625913944327],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0c7ede949ca2a67c53ff43f4c97b2b55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_90b170aa4a6ad6d31ac0064a8d9dd0f9 = $(`<div id="html_90b170aa4a6ad6d31ac0064a8d9dd0f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c7ede949ca2a67c53ff43f4c97b2b55.setContent(html_90b170aa4a6ad6d31ac0064a8d9dd0f9);\\n \\n \\n\\n circle_marker_2e798173f5a94ba16affe32f08c28897.bindPopup(popup_0c7ede949ca2a67c53ff43f4c97b2b55)\\n ;\\n\\n \\n \\n \\n var circle_marker_7fa6e6a5da9f5b9c508e7c4dd55ba2f4 = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_54b466664b746e3d519a6189ca734e54 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bf1329161f14a9dd6b5b1a096a4cf7e = $(`<div id="html_1bf1329161f14a9dd6b5b1a096a4cf7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54b466664b746e3d519a6189ca734e54.setContent(html_1bf1329161f14a9dd6b5b1a096a4cf7e);\\n \\n \\n\\n circle_marker_7fa6e6a5da9f5b9c508e7c4dd55ba2f4.bindPopup(popup_54b466664b746e3d519a6189ca734e54)\\n ;\\n\\n \\n \\n \\n var circle_marker_dad9a6ea43cd513be0fef88c8600b179 = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9f3d281877189f705a86c9c88521a8d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f41a1f9b0bbaf633ef2f1dd0846cccc0 = $(`<div id="html_f41a1f9b0bbaf633ef2f1dd0846cccc0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f3d281877189f705a86c9c88521a8d3.setContent(html_f41a1f9b0bbaf633ef2f1dd0846cccc0);\\n \\n \\n\\n circle_marker_dad9a6ea43cd513be0fef88c8600b179.bindPopup(popup_9f3d281877189f705a86c9c88521a8d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_95ede95fd941f23352942db4f5ac4032 = L.circleMarker(\\n [42.74358657602363, -73.27471463051425],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_82d5842c8c330e06f83b22c379ee0039 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9df965beb1d010206b7c652190b12f33 = $(`<div id="html_9df965beb1d010206b7c652190b12f33" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82d5842c8c330e06f83b22c379ee0039.setContent(html_9df965beb1d010206b7c652190b12f33);\\n \\n \\n\\n circle_marker_95ede95fd941f23352942db4f5ac4032.bindPopup(popup_82d5842c8c330e06f83b22c379ee0039)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6964708549a7727db6b0472ccd93086 = L.circleMarker(\\n [42.74358676039927, -73.27225393186212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_cb0dd2017c6331704fa5b572276e2c1e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07a9a863e25104bfee7876b6d8de11f4 = $(`<div id="html_07a9a863e25104bfee7876b6d8de11f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb0dd2017c6331704fa5b572276e2c1e.setContent(html_07a9a863e25104bfee7876b6d8de11f4);\\n \\n \\n\\n circle_marker_e6964708549a7727db6b0472ccd93086.bindPopup(popup_cb0dd2017c6331704fa5b572276e2c1e)\\n ;\\n\\n \\n \\n \\n var circle_marker_7419e02bcf4c4f4d9acd94e489fdfd69 = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.3377905890622728, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d75d96db6499ba21b91b1eee444c428b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e151270bfd0e4f7a6bc71745b45dd9b = $(`<div id="html_0e151270bfd0e4f7a6bc71745b45dd9b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d75d96db6499ba21b91b1eee444c428b.setContent(html_0e151270bfd0e4f7a6bc71745b45dd9b);\\n \\n \\n\\n circle_marker_7419e02bcf4c4f4d9acd94e489fdfd69.bindPopup(popup_d75d96db6499ba21b91b1eee444c428b)\\n ;\\n\\n \\n \\n \\n var circle_marker_b96250f4b673d3e84cbb93d37dcb314b = L.circleMarker(\\n [42.74358689209615, -73.26979323319746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_10312c6e303d80f51270a9cf7d18803d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7386ece7275cf82af7581de98bf65d90 = $(`<div id="html_7386ece7275cf82af7581de98bf65d90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10312c6e303d80f51270a9cf7d18803d.setContent(html_7386ece7275cf82af7581de98bf65d90);\\n \\n \\n\\n circle_marker_b96250f4b673d3e84cbb93d37dcb314b.bindPopup(popup_10312c6e303d80f51270a9cf7d18803d)\\n ;\\n\\n \\n \\n \\n var circle_marker_3aa8d8e8fb8ee0894f866aba0c7a3a74 = L.circleMarker(\\n [42.743586938190056, -73.26856288386173],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6dde7c8bbaf45079c08dce87e75c84cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5eaf604382e4370aed53cee9124a44b6 = $(`<div id="html_5eaf604382e4370aed53cee9124a44b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6dde7c8bbaf45079c08dce87e75c84cb.setContent(html_5eaf604382e4370aed53cee9124a44b6);\\n \\n \\n\\n circle_marker_3aa8d8e8fb8ee0894f866aba0c7a3a74.bindPopup(popup_6dde7c8bbaf45079c08dce87e75c84cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_1b8dff937789383821aca8c3e6da9022 = L.circleMarker(\\n [42.74358697111428, -73.26733253452443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4b7cd92c4ed064668ccef44ceb3a9c13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6777a7137671702b25c23d2fc22c837 = $(`<div id="html_c6777a7137671702b25c23d2fc22c837" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4b7cd92c4ed064668ccef44ceb3a9c13.setContent(html_c6777a7137671702b25c23d2fc22c837);\\n \\n \\n\\n circle_marker_1b8dff937789383821aca8c3e6da9022.bindPopup(popup_4b7cd92c4ed064668ccef44ceb3a9c13)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ed4e05d4c975387169cab5f0c95ebbf = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_07163b9dc263c33b8cbbcaf296b13177 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_27107728163658cdb4b399ffa8f3a517 = $(`<div id="html_27107728163658cdb4b399ffa8f3a517" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07163b9dc263c33b8cbbcaf296b13177.setContent(html_27107728163658cdb4b399ffa8f3a517);\\n \\n \\n\\n circle_marker_9ed4e05d4c975387169cab5f0c95ebbf.bindPopup(popup_07163b9dc263c33b8cbbcaf296b13177)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5cd8bad071baebe21e534430ed135f0 = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a7a784ef56432b84ccd9f3054a2122f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_068ec9c02f117699aa6f0f9a738a6bdd = $(`<div id="html_068ec9c02f117699aa6f0f9a738a6bdd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7a784ef56432b84ccd9f3054a2122f5.setContent(html_068ec9c02f117699aa6f0f9a738a6bdd);\\n \\n \\n\\n circle_marker_d5cd8bad071baebe21e534430ed135f0.bindPopup(popup_a7a784ef56432b84ccd9f3054a2122f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_7dc069fff3977f2fda458135a7b84ac6 = L.circleMarker(\\n [42.74358699086881, -73.26364148650833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a8fad5ee15608c0d5040f33a98734259 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83c6d477df0977fcfdd9bf4e633fe39d = $(`<div id="html_83c6d477df0977fcfdd9bf4e633fe39d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8fad5ee15608c0d5040f33a98734259.setContent(html_83c6d477df0977fcfdd9bf4e633fe39d);\\n \\n \\n\\n circle_marker_7dc069fff3977f2fda458135a7b84ac6.bindPopup(popup_a8fad5ee15608c0d5040f33a98734259)\\n ;\\n\\n \\n \\n \\n var circle_marker_d223114adbe3f7f9c05bb7d5dffcf004 = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_33919f3a3411973c4d39f58389831220 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_061078c18aca203a5c625dc359b58f14 = $(`<div id="html_061078c18aca203a5c625dc359b58f14" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33919f3a3411973c4d39f58389831220.setContent(html_061078c18aca203a5c625dc359b58f14);\\n \\n \\n\\n circle_marker_d223114adbe3f7f9c05bb7d5dffcf004.bindPopup(popup_33919f3a3411973c4d39f58389831220)\\n ;\\n\\n \\n \\n \\n var circle_marker_a859e09dbc6581b52ce53938f3536de7 = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_857fe58506e69990fc821b8c163a5bb1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74d2d5fac64da2979250d6a52c23c11c = $(`<div id="html_74d2d5fac64da2979250d6a52c23c11c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_857fe58506e69990fc821b8c163a5bb1.setContent(html_74d2d5fac64da2979250d6a52c23c11c);\\n \\n \\n\\n circle_marker_a859e09dbc6581b52ce53938f3536de7.bindPopup(popup_857fe58506e69990fc821b8c163a5bb1)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc88a44e77b3573be45dbe8a9074875f = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_da6a74f4c728d06468d4392515042379 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2d0e8baeb70c05f385624d0da704df29 = $(`<div id="html_2d0e8baeb70c05f385624d0da704df29" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_da6a74f4c728d06468d4392515042379.setContent(html_2d0e8baeb70c05f385624d0da704df29);\\n \\n \\n\\n circle_marker_dc88a44e77b3573be45dbe8a9074875f.bindPopup(popup_da6a74f4c728d06468d4392515042379)\\n ;\\n\\n \\n \\n \\n var circle_marker_96309d48aa155775228ff06a2d6180a3 = L.circleMarker(\\n [42.74358676039927, -73.2574897398323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_099ba12fe677bda6ff7d3f2db1f74f92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_03a09282b310bc03a54cd7e1d706e8e4 = $(`<div id="html_03a09282b310bc03a54cd7e1d706e8e4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_099ba12fe677bda6ff7d3f2db1f74f92.setContent(html_03a09282b310bc03a54cd7e1d706e8e4);\\n \\n \\n\\n circle_marker_96309d48aa155775228ff06a2d6180a3.bindPopup(popup_099ba12fe677bda6ff7d3f2db1f74f92)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc0ce648b6fbe3462ba328c832753664 = L.circleMarker(\\n [42.74358667479628, -73.25625939050441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_eee4e2d32aa7e5faa58775b01466d270 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1937b4837f0b4303886b6a335fc23624 = $(`<div id="html_1937b4837f0b4303886b6a335fc23624" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eee4e2d32aa7e5faa58775b01466d270.setContent(html_1937b4837f0b4303886b6a335fc23624);\\n \\n \\n\\n circle_marker_fc0ce648b6fbe3462ba328c832753664.bindPopup(popup_eee4e2d32aa7e5faa58775b01466d270)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6ff43f10686496c93279f025b44d2c5 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_584559f586c9c03f7c4f8a4f1db898dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f49e28ed3c3e0fde313b9e78b27523d7 = $(`<div id="html_f49e28ed3c3e0fde313b9e78b27523d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_584559f586c9c03f7c4f8a4f1db898dd.setContent(html_f49e28ed3c3e0fde313b9e78b27523d7);\\n \\n \\n\\n circle_marker_b6ff43f10686496c93279f025b44d2c5.bindPopup(popup_584559f586c9c03f7c4f8a4f1db898dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_7bf041567436b934cc4c57abc97804c3 = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_709cbae6f8d328cf1266fad0ae5f71e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ad114e0bf57923131a01999ae817454 = $(`<div id="html_5ad114e0bf57923131a01999ae817454" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_709cbae6f8d328cf1266fad0ae5f71e0.setContent(html_5ad114e0bf57923131a01999ae817454);\\n \\n \\n\\n circle_marker_7bf041567436b934cc4c57abc97804c3.bindPopup(popup_709cbae6f8d328cf1266fad0ae5f71e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_d58b33140be8b03b66c09fff2394087a = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.478115991081385, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4be441d5bc110758f46849b3031ed430 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44de358391ef6b8bd9171bb847020331 = $(`<div id="html_44de358391ef6b8bd9171bb847020331" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4be441d5bc110758f46849b3031ed430.setContent(html_44de358391ef6b8bd9171bb847020331);\\n \\n \\n\\n circle_marker_d58b33140be8b03b66c09fff2394087a.bindPopup(popup_4be441d5bc110758f46849b3031ed430)\\n ;\\n\\n \\n \\n \\n var circle_marker_f996e8f805fedde8b6774e8ccfa49300 = L.circleMarker(\\n [42.7417795414353, -73.27348403014923],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6888ba8fb5ee4f230769a6227abcba27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6f79e61e2f828c0b46de91eeead0f57 = $(`<div id="html_a6f79e61e2f828c0b46de91eeead0f57" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6888ba8fb5ee4f230769a6227abcba27.setContent(html_a6f79e61e2f828c0b46de91eeead0f57);\\n \\n \\n\\n circle_marker_f996e8f805fedde8b6774e8ccfa49300.bindPopup(popup_6888ba8fb5ee4f230769a6227abcba27)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0c7ab637fb06cc3e65ff8fa3a0f9ead = L.circleMarker(\\n [42.74177962703481, -73.2722537166843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6a60fa0b0b48d5e3a1fd545f49c6e8aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_915199fd69dfdc848d6bc3fec6c21c8b = $(`<div id="html_915199fd69dfdc848d6bc3fec6c21c8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a60fa0b0b48d5e3a1fd545f49c6e8aa.setContent(html_915199fd69dfdc848d6bc3fec6c21c8b);\\n \\n \\n\\n circle_marker_c0c7ab637fb06cc3e65ff8fa3a0f9ead.bindPopup(popup_6a60fa0b0b48d5e3a1fd545f49c6e8aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_f7d12141a399ade2a19ff00e5f44df28 = L.circleMarker(\\n [42.74177969946516, -73.27102340321625],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9057b9e2147a64167b52aaf31a844cbc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4d37fe0d60bba7c179db081d97ec8801 = $(`<div id="html_4d37fe0d60bba7c179db081d97ec8801" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9057b9e2147a64167b52aaf31a844cbc.setContent(html_4d37fe0d60bba7c179db081d97ec8801);\\n \\n \\n\\n circle_marker_f7d12141a399ade2a19ff00e5f44df28.bindPopup(popup_9057b9e2147a64167b52aaf31a844cbc)\\n ;\\n\\n \\n \\n \\n var circle_marker_51b39e138b6b2322045e430bfe9f8f8e = L.circleMarker(\\n [42.74177975872636, -73.26979308974558],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_dff5be84a9a5d318e924b57953207d9e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e792e12f40fa17aa1d03770ad79efbe9 = $(`<div id="html_e792e12f40fa17aa1d03770ad79efbe9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dff5be84a9a5d318e924b57953207d9e.setContent(html_e792e12f40fa17aa1d03770ad79efbe9);\\n \\n \\n\\n circle_marker_51b39e138b6b2322045e430bfe9f8f8e.bindPopup(popup_dff5be84a9a5d318e924b57953207d9e)\\n ;\\n\\n \\n \\n \\n var circle_marker_846f7c9321903dff66c77ab8d559e81e = L.circleMarker(\\n [42.7417798048184, -73.26856277627282],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7c6d89b0d413e5516677bd933b8415fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a9ee47f2984261c037dbfb320ac776a = $(`<div id="html_7a9ee47f2984261c037dbfb320ac776a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c6d89b0d413e5516677bd933b8415fc.setContent(html_7a9ee47f2984261c037dbfb320ac776a);\\n \\n \\n\\n circle_marker_846f7c9321903dff66c77ab8d559e81e.bindPopup(popup_7c6d89b0d413e5516677bd933b8415fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_121c4a5b0763f05e2fd46344067c3690 = L.circleMarker(\\n [42.7417798640796, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e008eac08d8b6c8b00c521838504ce1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_419f7516be0fd68d63713a0be6047c58 = $(`<div id="html_419f7516be0fd68d63713a0be6047c58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e008eac08d8b6c8b00c521838504ce1b.setContent(html_419f7516be0fd68d63713a0be6047c58);\\n \\n \\n\\n circle_marker_121c4a5b0763f05e2fd46344067c3690.bindPopup(popup_e008eac08d8b6c8b00c521838504ce1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e21cfc6dee59219cef11edac9cfcccb8 = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_35a7cb6cae9c7b12208f55d5c96ea155 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8429f60fbf67244077f5f25559e80b88 = $(`<div id="html_8429f60fbf67244077f5f25559e80b88" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35a7cb6cae9c7b12208f55d5c96ea155.setContent(html_8429f60fbf67244077f5f25559e80b88);\\n \\n \\n\\n circle_marker_e21cfc6dee59219cef11edac9cfcccb8.bindPopup(popup_35a7cb6cae9c7b12208f55d5c96ea155)\\n ;\\n\\n \\n \\n \\n var circle_marker_7b7586035e0a306e448f41f1fd932799 = L.circleMarker(\\n [42.7417798048184, -73.2611808954216],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1de1e40a14db507c8c1a19434bd20699 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_05ae3a0cfd84f4f3bb5e3dbc9cd91fec = $(`<div id="html_05ae3a0cfd84f4f3bb5e3dbc9cd91fec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1de1e40a14db507c8c1a19434bd20699.setContent(html_05ae3a0cfd84f4f3bb5e3dbc9cd91fec);\\n \\n \\n\\n circle_marker_7b7586035e0a306e448f41f1fd932799.bindPopup(popup_1de1e40a14db507c8c1a19434bd20699)\\n ;\\n\\n \\n \\n \\n var circle_marker_92f1e85f789e86588b7a01851d04c92d = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_72aed2d74c8a7ae30d4c971e12835c5c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_02dc6823fd337ad6fe6e316987bda09e = $(`<div id="html_02dc6823fd337ad6fe6e316987bda09e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72aed2d74c8a7ae30d4c971e12835c5c.setContent(html_02dc6823fd337ad6fe6e316987bda09e);\\n \\n \\n\\n circle_marker_92f1e85f789e86588b7a01851d04c92d.bindPopup(popup_72aed2d74c8a7ae30d4c971e12835c5c)\\n ;\\n\\n \\n \\n \\n var circle_marker_15410adcbf3c52506c29931069c4ddf3 = L.circleMarker(\\n [42.74177969946516, -73.25872026847817],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_37176c1dd5931f1c7f92b4ef4ec896a6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c23d8d6742196f4fe06acbdd5c9a52a3 = $(`<div id="html_c23d8d6742196f4fe06acbdd5c9a52a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_37176c1dd5931f1c7f92b4ef4ec896a6.setContent(html_c23d8d6742196f4fe06acbdd5c9a52a3);\\n \\n \\n\\n circle_marker_15410adcbf3c52506c29931069c4ddf3.bindPopup(popup_37176c1dd5931f1c7f92b4ef4ec896a6)\\n ;\\n\\n \\n \\n \\n var circle_marker_9538f04e58ba8c45315d1e06e150026d = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d8a985881c260d9a85200e8b7159d58c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b89a84c8158e9378b016b314e3af5df = $(`<div id="html_7b89a84c8158e9378b016b314e3af5df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8a985881c260d9a85200e8b7159d58c.setContent(html_7b89a84c8158e9378b016b314e3af5df);\\n \\n \\n\\n circle_marker_9538f04e58ba8c45315d1e06e150026d.bindPopup(popup_d8a985881c260d9a85200e8b7159d58c)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf21ec9f665eb5801792539f179f71fc = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0cd2ed5205c5cce34d4f05d14c891081 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15d020a2af42cd084600a4709f600953 = $(`<div id="html_15d020a2af42cd084600a4709f600953" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cd2ed5205c5cce34d4f05d14c891081.setContent(html_15d020a2af42cd084600a4709f600953);\\n \\n \\n\\n circle_marker_cf21ec9f665eb5801792539f179f71fc.bindPopup(popup_0cd2ed5205c5cce34d4f05d14c891081)\\n ;\\n\\n \\n \\n \\n var circle_marker_f25cd578315e43c72b664f2c0137cee1 = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fcfec01c22539fc9acbdd0556d31a1b1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8ae536d2bf17b5b1f61a7cc521dfa23 = $(`<div id="html_d8ae536d2bf17b5b1f61a7cc521dfa23" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fcfec01c22539fc9acbdd0556d31a1b1.setContent(html_d8ae536d2bf17b5b1f61a7cc521dfa23);\\n \\n \\n\\n circle_marker_f25cd578315e43c72b664f2c0137cee1.bindPopup(popup_fcfec01c22539fc9acbdd0556d31a1b1)\\n ;\\n\\n \\n \\n \\n var circle_marker_9632c2cb058124aa29fac952de049e6a = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_3daef46efa70ccccdef38a138776465a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a167c7335ca1ebf3caf607d70853e857 = $(`<div id="html_a167c7335ca1ebf3caf607d70853e857" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3daef46efa70ccccdef38a138776465a.setContent(html_a167c7335ca1ebf3caf607d70853e857);\\n \\n \\n\\n circle_marker_9632c2cb058124aa29fac952de049e6a.bindPopup(popup_3daef46efa70ccccdef38a138776465a)\\n ;\\n\\n \\n \\n \\n var circle_marker_dd3871f6b8bc68a782fffcbf1fc3ea70 = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_19f8770892f0ff09c6723ec846f5b530 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06dd3cbd15f5976782f47cd8d7b19c46 = $(`<div id="html_06dd3cbd15f5976782f47cd8d7b19c46" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19f8770892f0ff09c6723ec846f5b530.setContent(html_06dd3cbd15f5976782f47cd8d7b19c46);\\n \\n \\n\\n circle_marker_dd3871f6b8bc68a782fffcbf1fc3ea70.bindPopup(popup_19f8770892f0ff09c6723ec846f5b530)\\n ;\\n\\n \\n \\n \\n var circle_marker_461921b3a673a9af13c78c3cdb8106ee = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9c804b39bab5f6e85a8a6a55f47cf674 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8ad60b3b73d0639660889b032f6835b5 = $(`<div id="html_8ad60b3b73d0639660889b032f6835b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9c804b39bab5f6e85a8a6a55f47cf674.setContent(html_8ad60b3b73d0639660889b032f6835b5);\\n \\n \\n\\n circle_marker_461921b3a673a9af13c78c3cdb8106ee.bindPopup(popup_9c804b39bab5f6e85a8a6a55f47cf674)\\n ;\\n\\n \\n \\n \\n var circle_marker_7aa79fea9a32d3ffab45869a76c01076 = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4aa0e9090fe752baacc79cd2d886b849 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8da5d149767960bc6b57de5b7b9e865 = $(`<div id="html_a8da5d149767960bc6b57de5b7b9e865" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4aa0e9090fe752baacc79cd2d886b849.setContent(html_a8da5d149767960bc6b57de5b7b9e865);\\n \\n \\n\\n circle_marker_7aa79fea9a32d3ffab45869a76c01076.bindPopup(popup_4aa0e9090fe752baacc79cd2d886b849)\\n ;\\n\\n \\n \\n \\n var circle_marker_636a56adf4e67c775635d4655eb95ae5 = L.circleMarker(\\n [42.73997267144673, -73.26856266869262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e2c68b4e795853242518d689d3daafa1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54068d270291397686a69f569b92ce48 = $(`<div id="html_54068d270291397686a69f569b92ce48" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2c68b4e795853242518d689d3daafa1.setContent(html_54068d270291397686a69f569b92ce48);\\n \\n \\n\\n circle_marker_636a56adf4e67c775635d4655eb95ae5.bindPopup(popup_e2c68b4e795853242518d689d3daafa1)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d65d48b325c5bfe4e3638af430fe241 = L.circleMarker(\\n [42.73997270436829, -73.26733239107837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_dea5068c6589327c8f88fa11426b2857 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56654ef0e7f17368a9c88b95a2f82d0c = $(`<div id="html_56654ef0e7f17368a9c88b95a2f82d0c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dea5068c6589327c8f88fa11426b2857.setContent(html_56654ef0e7f17368a9c88b95a2f82d0c);\\n \\n \\n\\n circle_marker_9d65d48b325c5bfe4e3638af430fe241.bindPopup(popup_dea5068c6589327c8f88fa11426b2857)\\n ;\\n\\n \\n \\n \\n var circle_marker_fbacaf694e278a6de57670467e2c58bb = L.circleMarker(\\n [42.73997272412122, -73.26610211346305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c2df1be682e51bfd8418fb24f1055b6b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a119185d7e4d1e12eb937e7a04033282 = $(`<div id="html_a119185d7e4d1e12eb937e7a04033282" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2df1be682e51bfd8418fb24f1055b6b.setContent(html_a119185d7e4d1e12eb937e7a04033282);\\n \\n \\n\\n circle_marker_fbacaf694e278a6de57670467e2c58bb.bindPopup(popup_c2df1be682e51bfd8418fb24f1055b6b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e0ea375f35061be8d1df5b6472f28478 = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c7b8ad7f318f3c17b6c2fb53cf376db1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82aa03a1a8b4c78dc5da055074740ba4 = $(`<div id="html_82aa03a1a8b4c78dc5da055074740ba4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c7b8ad7f318f3c17b6c2fb53cf376db1.setContent(html_82aa03a1a8b4c78dc5da055074740ba4);\\n \\n \\n\\n circle_marker_e0ea375f35061be8d1df5b6472f28478.bindPopup(popup_c7b8ad7f318f3c17b6c2fb53cf376db1)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a14c1d9e438ca05c6cd1c485a7e5efe = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8bacb638c593533955de6502a9ab2ba6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_471017728c37e54c97d2705d5da1c361 = $(`<div id="html_471017728c37e54c97d2705d5da1c361" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8bacb638c593533955de6502a9ab2ba6.setContent(html_471017728c37e54c97d2705d5da1c361);\\n \\n \\n\\n circle_marker_3a14c1d9e438ca05c6cd1c485a7e5efe.bindPopup(popup_8bacb638c593533955de6502a9ab2ba6)\\n ;\\n\\n \\n \\n \\n var circle_marker_9efc7b290995f3b68a0a4510475adc81 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e019436696e19cf031af83c2fb074820 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8dfec8d9602518c2f0ac353cfba5c070 = $(`<div id="html_8dfec8d9602518c2f0ac353cfba5c070" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e019436696e19cf031af83c2fb074820.setContent(html_8dfec8d9602518c2f0ac353cfba5c070);\\n \\n \\n\\n circle_marker_9efc7b290995f3b68a0a4510475adc81.bindPopup(popup_e019436696e19cf031af83c2fb074820)\\n ;\\n\\n \\n \\n \\n var circle_marker_67a639946b81b6ae53f253b92feadffe = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ff7e714ba30e683940e35df911ed296c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_525c310c7bad4ebdfb15d46727ac6225 = $(`<div id="html_525c310c7bad4ebdfb15d46727ac6225" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ff7e714ba30e683940e35df911ed296c.setContent(html_525c310c7bad4ebdfb15d46727ac6225);\\n \\n \\n\\n circle_marker_67a639946b81b6ae53f253b92feadffe.bindPopup(popup_ff7e714ba30e683940e35df911ed296c)\\n ;\\n\\n \\n \\n \\n var circle_marker_3152469807823feceae423131a544028 = L.circleMarker(\\n [42.73997249367036, -73.25749017017048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8762085b62cbfdf5a1284faac9ced746 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_466bf185b13e80dee1a722e01a535b30 = $(`<div id="html_466bf185b13e80dee1a722e01a535b30" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8762085b62cbfdf5a1284faac9ced746.setContent(html_466bf185b13e80dee1a722e01a535b30);\\n \\n \\n\\n circle_marker_3152469807823feceae423131a544028.bindPopup(popup_8762085b62cbfdf5a1284faac9ced746)\\n ;\\n\\n \\n \\n \\n var circle_marker_057f21ea09072b054b2bb69919625688 = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a5171b5004666e722798c2a958a96f76 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d3dd503c3c7d60d30ee7681adbb33fe = $(`<div id="html_0d3dd503c3c7d60d30ee7681adbb33fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a5171b5004666e722798c2a958a96f76.setContent(html_0d3dd503c3c7d60d30ee7681adbb33fe);\\n \\n \\n\\n circle_marker_057f21ea09072b054b2bb69919625688.bindPopup(popup_a5171b5004666e722798c2a958a96f76)\\n ;\\n\\n \\n \\n \\n var circle_marker_45370f57ec372b8d44127f86d08c3b62 = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d46f812cb21e2a3bccb3a9281142953e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f6496cfeee9e472d4ebb735568bf1dd = $(`<div id="html_5f6496cfeee9e472d4ebb735568bf1dd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d46f812cb21e2a3bccb3a9281142953e.setContent(html_5f6496cfeee9e472d4ebb735568bf1dd);\\n \\n \\n\\n circle_marker_45370f57ec372b8d44127f86d08c3b62.bindPopup(popup_d46f812cb21e2a3bccb3a9281142953e)\\n ;\\n\\n \\n \\n \\n var circle_marker_c7ee72925c2dec6b8a1b0a0ffbbdb307 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_3e19707526dc19e9a7a86bcfcf759bd1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1b67a3270939eea0e702ef452c1372f = $(`<div id="html_b1b67a3270939eea0e702ef452c1372f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e19707526dc19e9a7a86bcfcf759bd1.setContent(html_b1b67a3270939eea0e702ef452c1372f);\\n \\n \\n\\n circle_marker_c7ee72925c2dec6b8a1b0a0ffbbdb307.bindPopup(popup_3e19707526dc19e9a7a86bcfcf759bd1)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1cea69a7947b92a575911a690ceb9d4 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_91ae1d5a4cc39e72735d24dc2fb1c5e6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc7cd3d215798388fcdaac6a47eea5d3 = $(`<div id="html_fc7cd3d215798388fcdaac6a47eea5d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91ae1d5a4cc39e72735d24dc2fb1c5e6.setContent(html_fc7cd3d215798388fcdaac6a47eea5d3);\\n \\n \\n\\n circle_marker_b1cea69a7947b92a575911a690ceb9d4.bindPopup(popup_91ae1d5a4cc39e72735d24dc2fb1c5e6)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb958e906b88e64e53f31f0fa8cba45c = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9508b2bd61cf7b8b8569a3256191bd74 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0d337836c165d3425471bde2845df5e = $(`<div id="html_b0d337836c165d3425471bde2845df5e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9508b2bd61cf7b8b8569a3256191bd74.setContent(html_b0d337836c165d3425471bde2845df5e);\\n \\n \\n\\n circle_marker_eb958e906b88e64e53f31f0fa8cba45c.bindPopup(popup_9508b2bd61cf7b8b8569a3256191bd74)\\n ;\\n\\n \\n \\n \\n var circle_marker_e587ca6ee35160d925b3ea7f54b6a369 = L.circleMarker(\\n [42.73996598837205, -73.22550295499178],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.431831258788849, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8e0456c1ca64adfce33bd8791a696c17 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4075f4d1050dc76f41f552e918aafcb1 = $(`<div id="html_4075f4d1050dc76f41f552e918aafcb1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e0456c1ca64adfce33bd8791a696c17.setContent(html_4075f4d1050dc76f41f552e918aafcb1);\\n \\n \\n\\n circle_marker_e587ca6ee35160d925b3ea7f54b6a369.bindPopup(popup_8e0456c1ca64adfce33bd8791a696c17)\\n ;\\n\\n \\n \\n \\n var circle_marker_307da0d86ead4e1fb09ef63574246056 = L.circleMarker(\\n [42.73996556039193, -73.22427267765188],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_84ffbdaa6be09d3c71ccf2be771dce81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c76ec882399d739a4a89e023df432259 = $(`<div id="html_c76ec882399d739a4a89e023df432259" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84ffbdaa6be09d3c71ccf2be771dce81.setContent(html_c76ec882399d739a4a89e023df432259);\\n \\n \\n\\n circle_marker_307da0d86ead4e1fb09ef63574246056.bindPopup(popup_84ffbdaa6be09d3c71ccf2be771dce81)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a512d31d43bcb8f9deb9210ccfa4552 = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_14ea2559dd941e77155dece5d1982e83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0fa2261ea30edb8d9d107e3b41c4f474 = $(`<div id="html_0fa2261ea30edb8d9d107e3b41c4f474" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14ea2559dd941e77155dece5d1982e83.setContent(html_0fa2261ea30edb8d9d107e3b41c4f474);\\n \\n \\n\\n circle_marker_8a512d31d43bcb8f9deb9210ccfa4552.bindPopup(popup_14ea2559dd941e77155dece5d1982e83)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb1dad0fd92b59ac4c59c59514dc3b84 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_86ac58e930a481ba1f98f58e266e4133 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb8b0aeadc23209a673a531c948e83b5 = $(`<div id="html_bb8b0aeadc23209a673a531c948e83b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86ac58e930a481ba1f98f58e266e4133.setContent(html_bb8b0aeadc23209a673a531c948e83b5);\\n \\n \\n\\n circle_marker_eb1dad0fd92b59ac4c59c59514dc3b84.bindPopup(popup_86ac58e930a481ba1f98f58e266e4133)\\n ;\\n\\n \\n \\n \\n var circle_marker_51109261d13426f9e525e63a6a70b0c2 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.982787485166879, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b5798685ca7e084f6ab16c9cf5e156d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5fdfbb798f28ad3011667a6c1a35a025 = $(`<div id="html_5fdfbb798f28ad3011667a6c1a35a025" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5798685ca7e084f6ab16c9cf5e156d7.setContent(html_5fdfbb798f28ad3011667a6c1a35a025);\\n \\n \\n\\n circle_marker_51109261d13426f9e525e63a6a70b0c2.bindPopup(popup_b5798685ca7e084f6ab16c9cf5e156d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_02d43fd297d0375dbe535c7eb17ee2bc = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_53bd66b38efcb5624338c303c5bd66de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f56406614bbe655025097c0c9495a90 = $(`<div id="html_0f56406614bbe655025097c0c9495a90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_53bd66b38efcb5624338c303c5bd66de.setContent(html_0f56406614bbe655025097c0c9495a90);\\n \\n \\n\\n circle_marker_02d43fd297d0375dbe535c7eb17ee2bc.bindPopup(popup_53bd66b38efcb5624338c303c5bd66de)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6db5d1c238df3c5ccedbc35e4596e81 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_62ce3c7a0ba2059886648044c04aa7ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2261cb9777b6060aab95914203589e95 = $(`<div id="html_2261cb9777b6060aab95914203589e95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62ce3c7a0ba2059886648044c04aa7ed.setContent(html_2261cb9777b6060aab95914203589e95);\\n \\n \\n\\n circle_marker_b6db5d1c238df3c5ccedbc35e4596e81.bindPopup(popup_62ce3c7a0ba2059886648044c04aa7ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_c68a52e9137145da3c40523705d8f977 = L.circleMarker(\\n [42.73816543273038, -73.27102304463016],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f4cbf6148821c5cd8b507a51a4363303 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ccbca64dacad209181964e667a870f7 = $(`<div id="html_0ccbca64dacad209181964e667a870f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f4cbf6148821c5cd8b507a51a4363303.setContent(html_0ccbca64dacad209181964e667a870f7);\\n \\n \\n\\n circle_marker_c68a52e9137145da3c40523705d8f977.bindPopup(popup_f4cbf6148821c5cd8b507a51a4363303)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4b55d337996dcf61ed4bc9682dd18bd = L.circleMarker(\\n [42.73816549198677, -73.26979280287671],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ebb52e9e016d0263b60902c2ffe88c59 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b658f7ce5b441739acc621bec0b8341 = $(`<div id="html_6b658f7ce5b441739acc621bec0b8341" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ebb52e9e016d0263b60902c2ffe88c59.setContent(html_6b658f7ce5b441739acc621bec0b8341);\\n \\n \\n\\n circle_marker_e4b55d337996dcf61ed4bc9682dd18bd.bindPopup(popup_ebb52e9e016d0263b60902c2ffe88c59)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e54b1e2cd3312d2c14ecd9878380f01 = L.circleMarker(\\n [42.73816553807507, -73.26856256112116],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_5c8516cb4f8048b383e8518c702f1cb0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3a923d3c8cc7621e9395f78d1bc869b = $(`<div id="html_a3a923d3c8cc7621e9395f78d1bc869b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c8516cb4f8048b383e8518c702f1cb0.setContent(html_a3a923d3c8cc7621e9395f78d1bc869b);\\n \\n \\n\\n circle_marker_0e54b1e2cd3312d2c14ecd9878380f01.bindPopup(popup_5c8516cb4f8048b383e8518c702f1cb0)\\n ;\\n\\n \\n \\n \\n var circle_marker_f31bcf49b5645cc600b32e7d584d74cf = L.circleMarker(\\n [42.7381655709953, -73.26733231936404],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_54729aac8a5b1fe0e7bb728af5e5a108 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8557c23c099c0bcbf937bfa7d3fd155 = $(`<div id="html_c8557c23c099c0bcbf937bfa7d3fd155" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54729aac8a5b1fe0e7bb728af5e5a108.setContent(html_c8557c23c099c0bcbf937bfa7d3fd155);\\n \\n \\n\\n circle_marker_f31bcf49b5645cc600b32e7d584d74cf.bindPopup(popup_54729aac8a5b1fe0e7bb728af5e5a108)\\n ;\\n\\n \\n \\n \\n var circle_marker_738e1029054eff238cd425f24e05339e = L.circleMarker(\\n [42.73816559074744, -73.2661020776059],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4d8c9f4cb99960de11aca5e7263fb787 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e0b800f7bcaff4e77a9ba2de1d113b6 = $(`<div id="html_0e0b800f7bcaff4e77a9ba2de1d113b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4d8c9f4cb99960de11aca5e7263fb787.setContent(html_0e0b800f7bcaff4e77a9ba2de1d113b6);\\n \\n \\n\\n circle_marker_738e1029054eff238cd425f24e05339e.bindPopup(popup_4d8c9f4cb99960de11aca5e7263fb787)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa1c22998484db799dc08cb90ed9c838 = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d40fca1cdd195756b5359436cfaf9ed0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9bf85db1aa925f0bf746f51c1a553d48 = $(`<div id="html_9bf85db1aa925f0bf746f51c1a553d48" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d40fca1cdd195756b5359436cfaf9ed0.setContent(html_9bf85db1aa925f0bf746f51c1a553d48);\\n \\n \\n\\n circle_marker_aa1c22998484db799dc08cb90ed9c838.bindPopup(popup_d40fca1cdd195756b5359436cfaf9ed0)\\n ;\\n\\n \\n \\n \\n var circle_marker_16eb73e97b92a2d11ee50dd3740d8262 = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_41367c9a651974dd34db80feddc98a7f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a71412b717160c49b05cbe78ceb737c = $(`<div id="html_8a71412b717160c49b05cbe78ceb737c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41367c9a651974dd34db80feddc98a7f.setContent(html_8a71412b717160c49b05cbe78ceb737c);\\n \\n \\n\\n circle_marker_16eb73e97b92a2d11ee50dd3740d8262.bindPopup(popup_41367c9a651974dd34db80feddc98a7f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6387d3545231b6fbdc9d1c69ff1131d9 = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2d634563ec8dd59680a9379d51a904cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31231dc777685661121e921319c987e1 = $(`<div id="html_31231dc777685661121e921319c987e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d634563ec8dd59680a9379d51a904cd.setContent(html_31231dc777685661121e921319c987e1);\\n \\n \\n\\n circle_marker_6387d3545231b6fbdc9d1c69ff1131d9.bindPopup(popup_2d634563ec8dd59680a9379d51a904cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_edb6e5569bd1d4594bfe6dbe764040dd = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6e05a5bff3464c05da54967460483fab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5d72dfa503f1be9ed0803e2657aa3fd7 = $(`<div id="html_5d72dfa503f1be9ed0803e2657aa3fd7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e05a5bff3464c05da54967460483fab.setContent(html_5d72dfa503f1be9ed0803e2657aa3fd7);\\n \\n \\n\\n circle_marker_edb6e5569bd1d4594bfe6dbe764040dd.bindPopup(popup_6e05a5bff3464c05da54967460483fab)\\n ;\\n\\n \\n \\n \\n var circle_marker_836a37e8fabd5550e7358ffabda4669d = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_dce50c55325698c5a305fefec648e67b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e704fe56bf83da766beabeec69ab7459 = $(`<div id="html_e704fe56bf83da766beabeec69ab7459" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dce50c55325698c5a305fefec648e67b.setContent(html_e704fe56bf83da766beabeec69ab7459);\\n \\n \\n\\n circle_marker_836a37e8fabd5550e7358ffabda4669d.bindPopup(popup_dce50c55325698c5a305fefec648e67b)\\n ;\\n\\n \\n \\n \\n var circle_marker_597883129a65cdace54d83958de7531a = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_890d03d3bddaa1c9adb9e9ef3ab2b620 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c189b92505c85772db072827551d9afa = $(`<div id="html_c189b92505c85772db072827551d9afa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_890d03d3bddaa1c9adb9e9ef3ab2b620.setContent(html_c189b92505c85772db072827551d9afa);\\n \\n \\n\\n circle_marker_597883129a65cdace54d83958de7531a.bindPopup(popup_890d03d3bddaa1c9adb9e9ef3ab2b620)\\n ;\\n\\n \\n \\n \\n var circle_marker_5cfa7c2e8a000d352bc0194f702ec770 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_363130c49f81a0017a869644649a519c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c2526ade71f793fa9350a42447160f0 = $(`<div id="html_1c2526ade71f793fa9350a42447160f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_363130c49f81a0017a869644649a519c.setContent(html_1c2526ade71f793fa9350a42447160f0);\\n \\n \\n\\n circle_marker_5cfa7c2e8a000d352bc0194f702ec770.bindPopup(popup_363130c49f81a0017a869644649a519c)\\n ;\\n\\n \\n \\n \\n var circle_marker_8949ea0634e5a26e7c5348609dfdefb7 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d2e2234f22abc3858ca27434b1e0342e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e635f3744eb7aca32ca538fc34ca61b1 = $(`<div id="html_e635f3744eb7aca32ca538fc34ca61b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2e2234f22abc3858ca27434b1e0342e.setContent(html_e635f3744eb7aca32ca538fc34ca61b1);\\n \\n \\n\\n circle_marker_8949ea0634e5a26e7c5348609dfdefb7.bindPopup(popup_d2e2234f22abc3858ca27434b1e0342e)\\n ;\\n\\n \\n \\n \\n var circle_marker_88d1aaa4d9486ac51e3e7cc2ec68f386 = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_37b96c1e82757aafd3e42636af7c2489 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb09e675cb5d9805a1c1c92bce2c0367 = $(`<div id="html_fb09e675cb5d9805a1c1c92bce2c0367" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_37b96c1e82757aafd3e42636af7c2489.setContent(html_fb09e675cb5d9805a1c1c92bce2c0367);\\n \\n \\n\\n circle_marker_88d1aaa4d9486ac51e3e7cc2ec68f386.bindPopup(popup_37b96c1e82757aafd3e42636af7c2489)\\n ;\\n\\n \\n \\n \\n var circle_marker_2bf023c10f04e2f8e882831ea228359a = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_88f9c37db8096de5bc7d16015892aa84 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_605a98aaff4ca0053a64e92eda4f3a89 = $(`<div id="html_605a98aaff4ca0053a64e92eda4f3a89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88f9c37db8096de5bc7d16015892aa84.setContent(html_605a98aaff4ca0053a64e92eda4f3a89);\\n \\n \\n\\n circle_marker_2bf023c10f04e2f8e882831ea228359a.bindPopup(popup_88f9c37db8096de5bc7d16015892aa84)\\n ;\\n\\n \\n \\n \\n var circle_marker_17f33409aafb2187338f92b151ecb66e = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d41cef384e5bbfe5e875084f66236250 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_df7b797bd39a077138d5768752ed6d10 = $(`<div id="html_df7b797bd39a077138d5768752ed6d10" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d41cef384e5bbfe5e875084f66236250.setContent(html_df7b797bd39a077138d5768752ed6d10);\\n \\n \\n\\n circle_marker_17f33409aafb2187338f92b151ecb66e.bindPopup(popup_d41cef384e5bbfe5e875084f66236250)\\n ;\\n\\n \\n \\n \\n var circle_marker_846a7a7e5ec384224a7ad930d67b125f = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_69fd1d86f117912b5ec7f9ebc11175ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a052440b3d15a61a98b8bf943759028 = $(`<div id="html_9a052440b3d15a61a98b8bf943759028" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_69fd1d86f117912b5ec7f9ebc11175ab.setContent(html_9a052440b3d15a61a98b8bf943759028);\\n \\n \\n\\n circle_marker_846a7a7e5ec384224a7ad930d67b125f.bindPopup(popup_69fd1d86f117912b5ec7f9ebc11175ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_14fa1dd1e2a612521783d73899d0f0ff = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_bc1e921161e001ca31769363898abb68 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_745566f69908d28f91682778063c1cb3 = $(`<div id="html_745566f69908d28f91682778063c1cb3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc1e921161e001ca31769363898abb68.setContent(html_745566f69908d28f91682778063c1cb3);\\n \\n \\n\\n circle_marker_14fa1dd1e2a612521783d73899d0f0ff.bindPopup(popup_bc1e921161e001ca31769363898abb68)\\n ;\\n\\n \\n \\n \\n var circle_marker_64177321d4777fb44e238b9a73e743ed = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_5e5f666bfceef77211521cb89c4872ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da9362aca1dc0ce810b4591e4f5ec7c0 = $(`<div id="html_da9362aca1dc0ce810b4591e4f5ec7c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e5f666bfceef77211521cb89c4872ac.setContent(html_da9362aca1dc0ce810b4591e4f5ec7c0);\\n \\n \\n\\n circle_marker_64177321d4777fb44e238b9a73e743ed.bindPopup(popup_5e5f666bfceef77211521cb89c4872ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_134c5d2af3bc1a40f558d1e9498f2400 = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8f0f6987123c2dbda3223fb9fc20c218 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c22d04723739d05915cc8a99a178d11 = $(`<div id="html_2c22d04723739d05915cc8a99a178d11" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f0f6987123c2dbda3223fb9fc20c218.setContent(html_2c22d04723739d05915cc8a99a178d11);\\n \\n \\n\\n circle_marker_134c5d2af3bc1a40f558d1e9498f2400.bindPopup(popup_8f0f6987123c2dbda3223fb9fc20c218)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c374fb3d002afd5ee09fc2cb30db63d = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.989422804014327, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a436f253dcacc373bddf30782ee31c34 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c8f4f955cdb92881a53822bae3092728 = $(`<div id="html_c8f4f955cdb92881a53822bae3092728" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a436f253dcacc373bddf30782ee31c34.setContent(html_c8f4f955cdb92881a53822bae3092728);\\n \\n \\n\\n circle_marker_2c374fb3d002afd5ee09fc2cb30db63d.bindPopup(popup_a436f253dcacc373bddf30782ee31c34)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f332c4ff5ca2274542a92d3493df100 = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1769b18f8924c607bcaa088479d9b97d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32f42bd569e2f5de302d07b379cd2b03 = $(`<div id="html_32f42bd569e2f5de302d07b379cd2b03" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1769b18f8924c607bcaa088479d9b97d.setContent(html_32f42bd569e2f5de302d07b379cd2b03);\\n \\n \\n\\n circle_marker_8f332c4ff5ca2274542a92d3493df100.bindPopup(popup_1769b18f8924c607bcaa088479d9b97d)\\n ;\\n\\n \\n \\n \\n var circle_marker_84d7ed598580b3d7b81b0971fc97a46b = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_38e887597552b60da0665c5110a7459c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db181481f7d4914fa13370c9f761e376 = $(`<div id="html_db181481f7d4914fa13370c9f761e376" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38e887597552b60da0665c5110a7459c.setContent(html_db181481f7d4914fa13370c9f761e376);\\n \\n \\n\\n circle_marker_84d7ed598580b3d7b81b0971fc97a46b.bindPopup(popup_38e887597552b60da0665c5110a7459c)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ddfb36380eac55091a0796598c97805 = L.circleMarker(\\n [42.736358404703424, -73.2685624535584],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_3088c1f3b36dd06ec1473a784a11ef2a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e95fb9992f0c5360c8a80be956ddb863 = $(`<div id="html_e95fb9992f0c5360c8a80be956ddb863" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3088c1f3b36dd06ec1473a784a11ef2a.setContent(html_e95fb9992f0c5360c8a80be956ddb863);\\n \\n \\n\\n circle_marker_9ddfb36380eac55091a0796598c97805.bindPopup(popup_3088c1f3b36dd06ec1473a784a11ef2a)\\n ;\\n\\n \\n \\n \\n var circle_marker_1bffe84b7d11a5335dae1624839d35bc = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_38db495b9be9961eb13e3eb100931b05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1495aa2ffff0576e3c079f5452619acd = $(`<div id="html_1495aa2ffff0576e3c079f5452619acd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38db495b9be9961eb13e3eb100931b05.setContent(html_1495aa2ffff0576e3c079f5452619acd);\\n \\n \\n\\n circle_marker_1bffe84b7d11a5335dae1624839d35bc.bindPopup(popup_38db495b9be9961eb13e3eb100931b05)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd7205b9efb938ce3cb8c55131651fde = L.circleMarker(\\n [42.73635846395741, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9ac967a3b844711bcc14927f203a269a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_932d0ef59f7d2b66b552fcae04c047f2 = $(`<div id="html_932d0ef59f7d2b66b552fcae04c047f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ac967a3b844711bcc14927f203a269a.setContent(html_932d0ef59f7d2b66b552fcae04c047f2);\\n \\n \\n\\n circle_marker_fd7205b9efb938ce3cb8c55131651fde.bindPopup(popup_9ac967a3b844711bcc14927f203a269a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c09be9a95fb186aa64e4c73d4030f558 = L.circleMarker(\\n [42.73635843762231, -73.26241142403887],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6f447b0095f04c25c018d86688afb687 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bb4de41c0f55ae7a7f7c5a65480b0b7 = $(`<div id="html_1bb4de41c0f55ae7a7f7c5a65480b0b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f447b0095f04c25c018d86688afb687.setContent(html_1bb4de41c0f55ae7a7f7c5a65480b0b7);\\n \\n \\n\\n circle_marker_c09be9a95fb186aa64e4c73d4030f558.bindPopup(popup_6f447b0095f04c25c018d86688afb687)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb49742ba15332fdbfe639666b2510c9 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_17235c84bb9887aa8d489245150e1b14 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48abc1413fdc52c68655a732d56eaee7 = $(`<div id="html_48abc1413fdc52c68655a732d56eaee7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_17235c84bb9887aa8d489245150e1b14.setContent(html_48abc1413fdc52c68655a732d56eaee7);\\n \\n \\n\\n circle_marker_eb49742ba15332fdbfe639666b2510c9.bindPopup(popup_17235c84bb9887aa8d489245150e1b14)\\n ;\\n\\n \\n \\n \\n var circle_marker_98b96da9a65af354bbc7e72c83275af7 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1ba3f10fb7391e5872b80136a992857b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a1515fd5e9d37575df546e5c5279f1d = $(`<div id="html_4a1515fd5e9d37575df546e5c5279f1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ba3f10fb7391e5872b80136a992857b.setContent(html_4a1515fd5e9d37575df546e5c5279f1d);\\n \\n \\n\\n circle_marker_98b96da9a65af354bbc7e72c83275af7.bindPopup(popup_1ba3f10fb7391e5872b80136a992857b)\\n ;\\n\\n \\n \\n \\n var circle_marker_c89c704a7af1ae8d769a78bc4922737b = L.circleMarker(\\n [42.73635829936299, -73.25872080633552],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ede7def2ffc2f1916c15b27bac433bce = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c1d5d3c46a8f9270ab35c4c899d6132 = $(`<div id="html_2c1d5d3c46a8f9270ab35c4c899d6132" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ede7def2ffc2f1916c15b27bac433bce.setContent(html_2c1d5d3c46a8f9270ab35c4c899d6132);\\n \\n \\n\\n circle_marker_c89c704a7af1ae8d769a78bc4922737b.bindPopup(popup_ede7def2ffc2f1916c15b27bac433bce)\\n ;\\n\\n \\n \\n \\n var circle_marker_01f8817b42309ed2abfb88299542a745 = L.circleMarker(\\n [42.73635822694145, -73.25749060043893],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d41293707bc2bbf782c4b29de49786c3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38cfd9221c8de039476638ad04250fa9 = $(`<div id="html_38cfd9221c8de039476638ad04250fa9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d41293707bc2bbf782c4b29de49786c3.setContent(html_38cfd9221c8de039476638ad04250fa9);\\n \\n \\n\\n circle_marker_01f8817b42309ed2abfb88299542a745.bindPopup(popup_d41293707bc2bbf782c4b29de49786c3)\\n ;\\n\\n \\n \\n \\n var circle_marker_583d78cf3161256ac6cccc38886bd7f7 = L.circleMarker(\\n [42.73635814135235, -73.25626039454548],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c5e80054b69a3241a7529e9710896f30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b05b682e93deb146e0c2c26955699a5 = $(`<div id="html_8b05b682e93deb146e0c2c26955699a5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c5e80054b69a3241a7529e9710896f30.setContent(html_8b05b682e93deb146e0c2c26955699a5);\\n \\n \\n\\n circle_marker_583d78cf3161256ac6cccc38886bd7f7.bindPopup(popup_c5e80054b69a3241a7529e9710896f30)\\n ;\\n\\n \\n \\n \\n var circle_marker_0998fa92b54da17dd5f31d8dd90cbfd0 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_64f1347e5ad28434a504e477318af047 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b58d874a538fc560b48a462f0fc4f7d = $(`<div id="html_6b58d874a538fc560b48a462f0fc4f7d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64f1347e5ad28434a504e477318af047.setContent(html_6b58d874a538fc560b48a462f0fc4f7d);\\n \\n \\n\\n circle_marker_0998fa92b54da17dd5f31d8dd90cbfd0.bindPopup(popup_64f1347e5ad28434a504e477318af047)\\n ;\\n\\n \\n \\n \\n var circle_marker_2795b7d0b5f3ad14b1ef94a4d28302ee = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_54f2cdba4521d8ccff0ac32a0b595757 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7467d32dc46045d786296183634fbb4 = $(`<div id="html_c7467d32dc46045d786296183634fbb4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54f2cdba4521d8ccff0ac32a0b595757.setContent(html_c7467d32dc46045d786296183634fbb4);\\n \\n \\n\\n circle_marker_2795b7d0b5f3ad14b1ef94a4d28302ee.bindPopup(popup_54f2cdba4521d8ccff0ac32a0b595757)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf6fe4c4083ae6c117df9f53dfc19e33 = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9208384aa9cdc3c7f4d2e5b54f159017 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f738226b547f994906abc9f94f0feca = $(`<div id="html_4f738226b547f994906abc9f94f0feca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9208384aa9cdc3c7f4d2e5b54f159017.setContent(html_4f738226b547f994906abc9f94f0feca);\\n \\n \\n\\n circle_marker_bf6fe4c4083ae6c117df9f53dfc19e33.bindPopup(popup_9208384aa9cdc3c7f4d2e5b54f159017)\\n ;\\n\\n \\n \\n \\n var circle_marker_8039fc775e948a46605b75ce62ee8aab = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_135289a5bd21a8475a4a5a0d5042aa98 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3c1a54d2eb45d1c15e13ba98287d470b = $(`<div id="html_3c1a54d2eb45d1c15e13ba98287d470b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_135289a5bd21a8475a4a5a0d5042aa98.setContent(html_3c1a54d2eb45d1c15e13ba98287d470b);\\n \\n \\n\\n circle_marker_8039fc775e948a46605b75ce62ee8aab.bindPopup(popup_135289a5bd21a8475a4a5a0d5042aa98)\\n ;\\n\\n \\n \\n \\n var circle_marker_9dad3c448ee45e72cf3a8ad75ea27a8b = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d5ca389472d653b8f8293d3a28107d05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dfb202c5096bf133861c60956cd5b5cd = $(`<div id="html_dfb202c5096bf133861c60956cd5b5cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d5ca389472d653b8f8293d3a28107d05.setContent(html_dfb202c5096bf133861c60956cd5b5cd);\\n \\n \\n\\n circle_marker_9dad3c448ee45e72cf3a8ad75ea27a8b.bindPopup(popup_d5ca389472d653b8f8293d3a28107d05)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6eb76f8f1ee96c16760f769c968da45 = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_235c85ece74642380f67d405525285d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ad51c06097505921bdc57c5302d6aba = $(`<div id="html_6ad51c06097505921bdc57c5302d6aba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_235c85ece74642380f67d405525285d5.setContent(html_6ad51c06097505921bdc57c5302d6aba);\\n \\n \\n\\n circle_marker_d6eb76f8f1ee96c16760f769c968da45.bindPopup(popup_235c85ece74642380f67d405525285d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a974efea3a6be404d0510555c6358143 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9bf42895a40a7fd6570392569e1b7fef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_060aae3088883e4fd66faf26242eede8 = $(`<div id="html_060aae3088883e4fd66faf26242eede8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9bf42895a40a7fd6570392569e1b7fef.setContent(html_060aae3088883e4fd66faf26242eede8);\\n \\n \\n\\n circle_marker_a974efea3a6be404d0510555c6358143.bindPopup(popup_9bf42895a40a7fd6570392569e1b7fef)\\n ;\\n\\n \\n \\n \\n var circle_marker_b0034e2094c101a24f1083557d19089b = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ad5292f66748e7b26a7397e6e733c86f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_414966bb321a7cd9752284818c32bc8f = $(`<div id="html_414966bb321a7cd9752284818c32bc8f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad5292f66748e7b26a7397e6e733c86f.setContent(html_414966bb321a7cd9752284818c32bc8f);\\n \\n \\n\\n circle_marker_b0034e2094c101a24f1083557d19089b.bindPopup(popup_ad5292f66748e7b26a7397e6e733c86f)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a9bacdb53541da562720fe4ba711ec1 = L.circleMarker(\\n [42.73635085311189, -73.22304483851624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.989422804014327, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_34e2c8a0b991756e82bc8bb5075ca0d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f04ead6ee738e554c2db641c3918e46 = $(`<div id="html_1f04ead6ee738e554c2db641c3918e46" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_34e2c8a0b991756e82bc8bb5075ca0d8.setContent(html_1f04ead6ee738e554c2db641c3918e46);\\n \\n \\n\\n circle_marker_6a9bacdb53541da562720fe4ba711ec1.bindPopup(popup_34e2c8a0b991756e82bc8bb5075ca0d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_63d41a53797d657ef03f965e81d51878 = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.8678889139475245, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8681cf6737a07ec7c7301120c609d07d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1fdae5cd1da583767fad40c2c13df42 = $(`<div id="html_b1fdae5cd1da583767fad40c2c13df42" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8681cf6737a07ec7c7301120c609d07d.setContent(html_b1fdae5cd1da583767fad40c2c13df42);\\n \\n \\n\\n circle_marker_63d41a53797d657ef03f965e81d51878.bindPopup(popup_8681cf6737a07ec7c7301120c609d07d)\\n ;\\n\\n \\n \\n \\n var circle_marker_974710bd8c9be20719968d61e0358145 = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_06ad0b0db1b70ce4b4deb671272ad817 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f1c1466c6229bc08ac8ce8f8e187abaf = $(`<div id="html_f1c1466c6229bc08ac8ce8f8e187abaf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06ad0b0db1b70ce4b4deb671272ad817.setContent(html_f1c1466c6229bc08ac8ce8f8e187abaf);\\n \\n \\n\\n circle_marker_974710bd8c9be20719968d61e0358145.bindPopup(popup_06ad0b0db1b70ce4b4deb671272ad817)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff09cb011ca2739043c3f90bae33cb5c = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e7ba39dd6568f929488cf8edea51abeb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_880ed4e56467d5fbba525ebcd90792fe = $(`<div id="html_880ed4e56467d5fbba525ebcd90792fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e7ba39dd6568f929488cf8edea51abeb.setContent(html_880ed4e56467d5fbba525ebcd90792fe);\\n \\n \\n\\n circle_marker_ff09cb011ca2739043c3f90bae33cb5c.bindPopup(popup_e7ba39dd6568f929488cf8edea51abeb)\\n ;\\n\\n \\n \\n \\n var circle_marker_99a5963da0c2e4be1c5ecd800bdd94e9 = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2023b053e57d9010b8d3d2e69e819bda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38022ec4f961e5e8eda83cbe6d20a7f3 = $(`<div id="html_38022ec4f961e5e8eda83cbe6d20a7f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2023b053e57d9010b8d3d2e69e819bda.setContent(html_38022ec4f961e5e8eda83cbe6d20a7f3);\\n \\n \\n\\n circle_marker_99a5963da0c2e4be1c5ecd800bdd94e9.bindPopup(popup_2023b053e57d9010b8d3d2e69e819bda)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9df94f9d312c3ee72b94a2e5e338584 = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7e08469a31795eaa5ee359b08444ac7e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8bcc3a7c62248e0fe692dcd6042020d3 = $(`<div id="html_8bcc3a7c62248e0fe692dcd6042020d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e08469a31795eaa5ee359b08444ac7e.setContent(html_8bcc3a7c62248e0fe692dcd6042020d3);\\n \\n \\n\\n circle_marker_a9df94f9d312c3ee72b94a2e5e338584.bindPopup(popup_7e08469a31795eaa5ee359b08444ac7e)\\n ;\\n\\n \\n \\n \\n var circle_marker_10a2e4c40e63fe3ec055dbe9da83bd44 = L.circleMarker(\\n [42.7345512252472, -73.26979251605432],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1c65ce9529589419b707a9c338950495 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9aef01c15bfa595ccaf5cdc4d25f850a = $(`<div id="html_9aef01c15bfa595ccaf5cdc4d25f850a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1c65ce9529589419b707a9c338950495.setContent(html_9aef01c15bfa595ccaf5cdc4d25f850a);\\n \\n \\n\\n circle_marker_10a2e4c40e63fe3ec055dbe9da83bd44.bindPopup(popup_1c65ce9529589419b707a9c338950495)\\n ;\\n\\n \\n \\n \\n var circle_marker_b239789cfb7d8028eba398148e2fad48 = L.circleMarker(\\n [42.73455127133177, -73.26856234600437],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_94b62aba305b6cbfeab397df5d168913 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9516562d37538234ef53e0be8cd915c8 = $(`<div id="html_9516562d37538234ef53e0be8cd915c8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94b62aba305b6cbfeab397df5d168913.setContent(html_9516562d37538234ef53e0be8cd915c8);\\n \\n \\n\\n circle_marker_b239789cfb7d8028eba398148e2fad48.bindPopup(popup_94b62aba305b6cbfeab397df5d168913)\\n ;\\n\\n \\n \\n \\n var circle_marker_5318b3c4f3328bdd2fc739652ce60960 = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6751dfef7ec20bfc4caa1102e4582156 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_660cbb58eb477558608a0eb7fcfb6a67 = $(`<div id="html_660cbb58eb477558608a0eb7fcfb6a67" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6751dfef7ec20bfc4caa1102e4582156.setContent(html_660cbb58eb477558608a0eb7fcfb6a67);\\n \\n \\n\\n circle_marker_5318b3c4f3328bdd2fc739652ce60960.bindPopup(popup_6751dfef7ec20bfc4caa1102e4582156)\\n ;\\n\\n \\n \\n \\n var circle_marker_a59872f8089e79955294e6c55acf8026 = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_04949290b333eab9dd7f79894a525750 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da58ebbf9b555e5c6b31e2d77a45570f = $(`<div id="html_da58ebbf9b555e5c6b31e2d77a45570f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_04949290b333eab9dd7f79894a525750.setContent(html_da58ebbf9b555e5c6b31e2d77a45570f);\\n \\n \\n\\n circle_marker_a59872f8089e79955294e6c55acf8026.bindPopup(popup_04949290b333eab9dd7f79894a525750)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8e36e4b221cf7d8f78467be44f86630 = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_900a4d6263f841de79d87c4fb003ec6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_44692481b8e61b3d758873d32e49155a = $(`<div id="html_44692481b8e61b3d758873d32e49155a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_900a4d6263f841de79d87c4fb003ec6a.setContent(html_44692481b8e61b3d758873d32e49155a);\\n \\n \\n\\n circle_marker_e8e36e4b221cf7d8f78467be44f86630.bindPopup(popup_900a4d6263f841de79d87c4fb003ec6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_958b4dd78fb766a56782a006aa431c3a = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fa5ccbb1ae6def54b5731df929d9027d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac57fe4eae6056c2eb333831bd7fa407 = $(`<div id="html_ac57fe4eae6056c2eb333831bd7fa407" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa5ccbb1ae6def54b5731df929d9027d.setContent(html_ac57fe4eae6056c2eb333831bd7fa407);\\n \\n \\n\\n circle_marker_958b4dd78fb766a56782a006aa431c3a.bindPopup(popup_fa5ccbb1ae6def54b5731df929d9027d)\\n ;\\n\\n \\n \\n \\n var circle_marker_051b11dbed15a7ddf853c53883feb4f2 = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1df9c3c9410e4ea66900b0ce44163071 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ffd17cf52f27462a61388a90da0be4e = $(`<div id="html_1ffd17cf52f27462a61388a90da0be4e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1df9c3c9410e4ea66900b0ce44163071.setContent(html_1ffd17cf52f27462a61388a90da0be4e);\\n \\n \\n\\n circle_marker_051b11dbed15a7ddf853c53883feb4f2.bindPopup(popup_1df9c3c9410e4ea66900b0ce44163071)\\n ;\\n\\n \\n \\n \\n var circle_marker_280e1be0f867bd7e80ea33f9689a2c67 = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4cdaaafb763dda7dedc626ca5d4f1463 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_427fd7eb45304a6d3d1b5a4116a53c97 = $(`<div id="html_427fd7eb45304a6d3d1b5a4116a53c97" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4cdaaafb763dda7dedc626ca5d4f1463.setContent(html_427fd7eb45304a6d3d1b5a4116a53c97);\\n \\n \\n\\n circle_marker_280e1be0f867bd7e80ea33f9689a2c67.bindPopup(popup_4cdaaafb763dda7dedc626ca5d4f1463)\\n ;\\n\\n \\n \\n \\n var circle_marker_42c8edd2b66fab699e04227c79e07f04 = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_174825c20cf4287a1288143c06939be8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d0c5f25f647d6e8247c708b5af97e5d3 = $(`<div id="html_d0c5f25f647d6e8247c708b5af97e5d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_174825c20cf4287a1288143c06939be8.setContent(html_d0c5f25f647d6e8247c708b5af97e5d3);\\n \\n \\n\\n circle_marker_42c8edd2b66fab699e04227c79e07f04.bindPopup(popup_174825c20cf4287a1288143c06939be8)\\n ;\\n\\n \\n \\n \\n var circle_marker_3a5bf16bf44b7167aabb271b2e4c68b1 = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_66e54565ce963776b181fac533166c53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f8f31043bab200b0b8835a5f63f00b6 = $(`<div id="html_0f8f31043bab200b0b8835a5f63f00b6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_66e54565ce963776b181fac533166c53.setContent(html_0f8f31043bab200b0b8835a5f63f00b6);\\n \\n \\n\\n circle_marker_3a5bf16bf44b7167aabb271b2e4c68b1.bindPopup(popup_66e54565ce963776b181fac533166c53)\\n ;\\n\\n \\n \\n \\n var circle_marker_6aec9c532db28ba9900449586560ca5a = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2d9dc7a5e8d34713025360946f1e0702 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39caf387871b116b8f85f141dba37bd9 = $(`<div id="html_39caf387871b116b8f85f141dba37bd9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d9dc7a5e8d34713025360946f1e0702.setContent(html_39caf387871b116b8f85f141dba37bd9);\\n \\n \\n\\n circle_marker_6aec9c532db28ba9900449586560ca5a.bindPopup(popup_2d9dc7a5e8d34713025360946f1e0702)\\n ;\\n\\n \\n \\n \\n var circle_marker_24328685e73200bb67d46d8864cbf53a = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1ac8d7fd5ddff9405f5f130523c09cc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e6c7671e9b4d298a8c90da3ad34f81a = $(`<div id="html_2e6c7671e9b4d298a8c90da3ad34f81a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ac8d7fd5ddff9405f5f130523c09cc1.setContent(html_2e6c7671e9b4d298a8c90da3ad34f81a);\\n \\n \\n\\n circle_marker_24328685e73200bb67d46d8864cbf53a.bindPopup(popup_1ac8d7fd5ddff9405f5f130523c09cc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_1c9a34dbf5eeffdb37b6804c228c1ea4 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a0b78e7a3c1c18a3e26bd84fa3068e5f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b81f9f55532a61e020a3ed27a1bab0a = $(`<div id="html_5b81f9f55532a61e020a3ed27a1bab0a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0b78e7a3c1c18a3e26bd84fa3068e5f.setContent(html_5b81f9f55532a61e020a3ed27a1bab0a);\\n \\n \\n\\n circle_marker_1c9a34dbf5eeffdb37b6804c228c1ea4.bindPopup(popup_a0b78e7a3c1c18a3e26bd84fa3068e5f)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d895037f67d2faa7de8cc7634d4720d = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b1ab70d489e3dc5f1061a32366033684 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_194e3349776227a4e2eff3801b5fb059 = $(`<div id="html_194e3349776227a4e2eff3801b5fb059" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1ab70d489e3dc5f1061a32366033684.setContent(html_194e3349776227a4e2eff3801b5fb059);\\n \\n \\n\\n circle_marker_7d895037f67d2faa7de8cc7634d4720d.bindPopup(popup_b1ab70d489e3dc5f1061a32366033684)\\n ;\\n\\n \\n \\n \\n var circle_marker_363ea1f90889960600fe0976b30e015e = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7313c0f441b4624bc87e026d139ed5a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37b5e38087cfbfe719d9f38545c87204 = $(`<div id="html_37b5e38087cfbfe719d9f38545c87204" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7313c0f441b4624bc87e026d139ed5a1.setContent(html_37b5e38087cfbfe719d9f38545c87204);\\n \\n \\n\\n circle_marker_363ea1f90889960600fe0976b30e015e.bindPopup(popup_7313c0f441b4624bc87e026d139ed5a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_5f5d22b9bce8b4e7d6cb39fa631da993 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d2ac401f189c6ee7f168637a3fdeeabd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f893b265e35bd14440e0de708dc61431 = $(`<div id="html_f893b265e35bd14440e0de708dc61431" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2ac401f189c6ee7f168637a3fdeeabd.setContent(html_f893b265e35bd14440e0de708dc61431);\\n \\n \\n\\n circle_marker_5f5d22b9bce8b4e7d6cb39fa631da993.bindPopup(popup_d2ac401f189c6ee7f168637a3fdeeabd)\\n ;\\n\\n \\n \\n \\n var circle_marker_d254e3e98bc640004e33ecf8e903d9c7 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b8073ae1f43ef9cf5b1f5368784f90d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_35a12d5a7f6d8cc18bb01564a220d04e = $(`<div id="html_35a12d5a7f6d8cc18bb01564a220d04e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b8073ae1f43ef9cf5b1f5368784f90d8.setContent(html_35a12d5a7f6d8cc18bb01564a220d04e);\\n \\n \\n\\n circle_marker_d254e3e98bc640004e33ecf8e903d9c7.bindPopup(popup_b8073ae1f43ef9cf5b1f5368784f90d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6be18522e3afadceed7d9d59890d7a7 = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c8ce6b91b9d9ddd5e49c0b10a811dcd3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c05de6383a83538f6c88d603e9f824b = $(`<div id="html_9c05de6383a83538f6c88d603e9f824b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8ce6b91b9d9ddd5e49c0b10a811dcd3.setContent(html_9c05de6383a83538f6c88d603e9f824b);\\n \\n \\n\\n circle_marker_d6be18522e3afadceed7d9d59890d7a7.bindPopup(popup_c8ce6b91b9d9ddd5e49c0b10a811dcd3)\\n ;\\n\\n \\n \\n \\n var circle_marker_4fe8ce9a1bc28d867be41b1d82edb1ea = L.circleMarker(\\n [42.7345450038305, -73.22673656679281],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6cb6a2f8124429250d9473ab22f4565b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb4d94ef1d21555263c0c22bebb7f76e = $(`<div id="html_bb4d94ef1d21555263c0c22bebb7f76e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6cb6a2f8124429250d9473ab22f4565b.setContent(html_bb4d94ef1d21555263c0c22bebb7f76e);\\n \\n \\n\\n circle_marker_4fe8ce9a1bc28d867be41b1d82edb1ea.bindPopup(popup_6cb6a2f8124429250d9473ab22f4565b)\\n ;\\n\\n \\n \\n \\n var circle_marker_985dfa861ebe69d24575fb066923128a = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e3ce1863110d1a698d4b1f44cd34c92a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_baac7c217b03905135a221c6126593e9 = $(`<div id="html_baac7c217b03905135a221c6126593e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3ce1863110d1a698d4b1f44cd34c92a.setContent(html_baac7c217b03905135a221c6126593e9);\\n \\n \\n\\n circle_marker_985dfa861ebe69d24575fb066923128a.bindPopup(popup_e3ce1863110d1a698d4b1f44cd34c92a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d0a30eb19700a1da4dd76ae03160b9b = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_18c6a0fa877acc6b405a2e322552b2ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7922817bf1f80e833e8e3fb2bb4fb74 = $(`<div id="html_e7922817bf1f80e833e8e3fb2bb4fb74" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_18c6a0fa877acc6b405a2e322552b2ca.setContent(html_e7922817bf1f80e833e8e3fb2bb4fb74);\\n \\n \\n\\n circle_marker_8d0a30eb19700a1da4dd76ae03160b9b.bindPopup(popup_18c6a0fa877acc6b405a2e322552b2ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d11e26aa3fb3d2c6367847f21543505 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.989422804014327, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_dbb7d40e09bd276f9912c882361656ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38042231474d98f4adfec1e456bbcd33 = $(`<div id="html_38042231474d98f4adfec1e456bbcd33" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dbb7d40e09bd276f9912c882361656ae.setContent(html_38042231474d98f4adfec1e456bbcd33);\\n \\n \\n\\n circle_marker_8d11e26aa3fb3d2c6367847f21543505.bindPopup(popup_dbb7d40e09bd276f9912c882361656ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_65c0733ea4860e4ba51d7b1d7c728ac8 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8369b2ed97d990ab89dc5e0d1b4a9b64 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_40d8b60dd7c6d69f983fa8579048a293 = $(`<div id="html_40d8b60dd7c6d69f983fa8579048a293" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8369b2ed97d990ab89dc5e0d1b4a9b64.setContent(html_40d8b60dd7c6d69f983fa8579048a293);\\n \\n \\n\\n circle_marker_65c0733ea4860e4ba51d7b1d7c728ac8.bindPopup(popup_8369b2ed97d990ab89dc5e0d1b4a9b64)\\n ;\\n\\n \\n \\n \\n var circle_marker_4bfbfb43f8c1b4c00fa21f796698ce82 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fb3f4c57c1e865812baa556c6685e1cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1882d06d18f7e3f66e6a36e37c643e0a = $(`<div id="html_1882d06d18f7e3f66e6a36e37c643e0a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb3f4c57c1e865812baa556c6685e1cd.setContent(html_1882d06d18f7e3f66e6a36e37c643e0a);\\n \\n \\n\\n circle_marker_4bfbfb43f8c1b4c00fa21f796698ce82.bindPopup(popup_fb3f4c57c1e865812baa556c6685e1cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_1122aa274c10713b7ee13ffeec46fe87 = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ba04aee690bf405a21bbd62b13d69d68 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1cf61976d152a71f43caa687abb5548f = $(`<div id="html_1cf61976d152a71f43caa687abb5548f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba04aee690bf405a21bbd62b13d69d68.setContent(html_1cf61976d152a71f43caa687abb5548f);\\n \\n \\n\\n circle_marker_1122aa274c10713b7ee13ffeec46fe87.bindPopup(popup_ba04aee690bf405a21bbd62b13d69d68)\\n ;\\n\\n \\n \\n \\n var circle_marker_c282e8149438c514a04a5576004b9d65 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f1e5b62a27bfecfd97f4e39bd972da89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c99f6026d55ab8fd3a2a22915bef485f = $(`<div id="html_c99f6026d55ab8fd3a2a22915bef485f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1e5b62a27bfecfd97f4e39bd972da89.setContent(html_c99f6026d55ab8fd3a2a22915bef485f);\\n \\n \\n\\n circle_marker_c282e8149438c514a04a5576004b9d65.bindPopup(popup_f1e5b62a27bfecfd97f4e39bd972da89)\\n ;\\n\\n \\n \\n \\n var circle_marker_e51c0d2270a2b777987b0c3ac7e8d45f = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_03a429a0311e581d0431e1ac004b229b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e4a69690db2159928c51960e375fb13e = $(`<div id="html_e4a69690db2159928c51960e375fb13e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_03a429a0311e581d0431e1ac004b229b.setContent(html_e4a69690db2159928c51960e375fb13e);\\n \\n \\n\\n circle_marker_e51c0d2270a2b777987b0c3ac7e8d45f.bindPopup(popup_03a429a0311e581d0431e1ac004b229b)\\n ;\\n\\n \\n \\n \\n var circle_marker_f12d6bb17ef1b67753306504a9e510eb = L.circleMarker(\\n [42.7327440918774, -73.26979237266056],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_26ce6eff476c5093fb07567934521fed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_936a05c44a26c2ba36af036c78ec181f = $(`<div id="html_936a05c44a26c2ba36af036c78ec181f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_26ce6eff476c5093fb07567934521fed.setContent(html_936a05c44a26c2ba36af036c78ec181f);\\n \\n \\n\\n circle_marker_f12d6bb17ef1b67753306504a9e510eb.bindPopup(popup_26ce6eff476c5093fb07567934521fed)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa55dc9833ecbb91129e28f65a1cd8e0 = L.circleMarker(\\n [42.73274419062606, -73.26610197005185],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a50414c2b9b5365701595d3968dcfcaf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ac56d5cee2b9cba5b15858e68d64fda = $(`<div id="html_7ac56d5cee2b9cba5b15858e68d64fda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a50414c2b9b5365701595d3968dcfcaf.setContent(html_7ac56d5cee2b9cba5b15858e68d64fda);\\n \\n \\n\\n circle_marker_fa55dc9833ecbb91129e28f65a1cd8e0.bindPopup(popup_a50414c2b9b5365701595d3968dcfcaf)\\n ;\\n\\n \\n \\n \\n var circle_marker_965a886cd833f9de720eb8308229f041 = L.circleMarker(\\n [42.7327441972093, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4bba149118b125fdd40aebfb72edb63d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63de9bce5b2aa2a71825969aa7a2c5f2 = $(`<div id="html_63de9bce5b2aa2a71825969aa7a2c5f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4bba149118b125fdd40aebfb72edb63d.setContent(html_63de9bce5b2aa2a71825969aa7a2c5f2);\\n \\n \\n\\n circle_marker_965a886cd833f9de720eb8308229f041.bindPopup(popup_4bba149118b125fdd40aebfb72edb63d)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3966b2cb53443eb7914dedca4983522 = L.circleMarker(\\n [42.73274419062606, -73.26364170164257],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d2d83aa976784618005b2f37dfa008d8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6968b3d305d83de7d74b11591fb861c = $(`<div id="html_b6968b3d305d83de7d74b11591fb861c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2d83aa976784618005b2f37dfa008d8.setContent(html_b6968b3d305d83de7d74b11591fb861c);\\n \\n \\n\\n circle_marker_f3966b2cb53443eb7914dedca4983522.bindPopup(popup_d2d83aa976784618005b2f37dfa008d8)\\n ;\\n\\n \\n \\n \\n var circle_marker_3cef7941995b0f80f9fdc79bf79d79cc = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_68f551044b8d4a2132508feeaa0d0fd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b0e244e3abccd1dd1f6a9917bfe08d0 = $(`<div id="html_8b0e244e3abccd1dd1f6a9917bfe08d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68f551044b8d4a2132508feeaa0d0fd0.setContent(html_8b0e244e3abccd1dd1f6a9917bfe08d0);\\n \\n \\n\\n circle_marker_3cef7941995b0f80f9fdc79bf79d79cc.bindPopup(popup_68f551044b8d4a2132508feeaa0d0fd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e71b78aee15d48c50ceb3c512f11d7a = L.circleMarker(\\n [42.7327440918774, -73.25995129903386],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_39eaf58edbe4197624ff32c3c0afa854 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_495e6c4ff14e7976e377d89d16a6fd17 = $(`<div id="html_495e6c4ff14e7976e377d89d16a6fd17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39eaf58edbe4197624ff32c3c0afa854.setContent(html_495e6c4ff14e7976e377d89d16a6fd17);\\n \\n \\n\\n circle_marker_5e71b78aee15d48c50ceb3c512f11d7a.bindPopup(popup_39eaf58edbe4197624ff32c3c0afa854)\\n ;\\n\\n \\n \\n \\n var circle_marker_bacf27bd25977d6c34443926682e5184 = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2977538f3ffa81a6f824fafd9c546c17 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de79c75569915a556ec636c8786fb17f = $(`<div id="html_de79c75569915a556ec636c8786fb17f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2977538f3ffa81a6f824fafd9c546c17.setContent(html_de79c75569915a556ec636c8786fb17f);\\n \\n \\n\\n circle_marker_bacf27bd25977d6c34443926682e5184.bindPopup(popup_2977538f3ffa81a6f824fafd9c546c17)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1edd0c06e5add463be495723a27deb5 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_849d32255c045ec4e50f66e22ffc8aed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1cf626979ee18b7ed02f9400c3c8ae6 = $(`<div id="html_b1cf626979ee18b7ed02f9400c3c8ae6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_849d32255c045ec4e50f66e22ffc8aed.setContent(html_b1cf626979ee18b7ed02f9400c3c8ae6);\\n \\n \\n\\n circle_marker_c1edd0c06e5add463be495723a27deb5.bindPopup(popup_849d32255c045ec4e50f66e22ffc8aed)\\n ;\\n\\n \\n \\n \\n var circle_marker_733b07a03ea6c0b0b4b12ba9fd6a374a = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6d2dea0e847989dc5f8e95fb196eb2ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_510d587df96009d5cee686d726cf8fae = $(`<div id="html_510d587df96009d5cee686d726cf8fae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d2dea0e847989dc5f8e95fb196eb2ef.setContent(html_510d587df96009d5cee686d726cf8fae);\\n \\n \\n\\n circle_marker_733b07a03ea6c0b0b4b12ba9fd6a374a.bindPopup(popup_6d2dea0e847989dc5f8e95fb196eb2ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_2692329bc6cdcf4d2a4da4f742c6eca7 = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b099b96b0cbe724642f76a9b1dda4fde = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f377979e884453e6ca2a88d05e3b665 = $(`<div id="html_7f377979e884453e6ca2a88d05e3b665" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b099b96b0cbe724642f76a9b1dda4fde.setContent(html_7f377979e884453e6ca2a88d05e3b665);\\n \\n \\n\\n circle_marker_2692329bc6cdcf4d2a4da4f742c6eca7.bindPopup(popup_b099b96b0cbe724642f76a9b1dda4fde)\\n ;\\n\\n \\n \\n \\n var circle_marker_108cda9bae8f84a21b4cbe5e154cc470 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_45049923f3b48349f899b1dfb038c1aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ad9f3304943d7b68acbcbcdfb13f492 = $(`<div id="html_0ad9f3304943d7b68acbcbcdfb13f492" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_45049923f3b48349f899b1dfb038c1aa.setContent(html_0ad9f3304943d7b68acbcbcdfb13f492);\\n \\n \\n\\n circle_marker_108cda9bae8f84a21b4cbe5e154cc470.bindPopup(popup_45049923f3b48349f899b1dfb038c1aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_36a43948979f72db6c57ba89f15a98d5 = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6ed823cb23e4d06c4cf1d1eb924d818d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f3bedfc4f7f617dcd7ce199e8af2410 = $(`<div id="html_6f3bedfc4f7f617dcd7ce199e8af2410" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ed823cb23e4d06c4cf1d1eb924d818d.setContent(html_6f3bedfc4f7f617dcd7ce199e8af2410);\\n \\n \\n\\n circle_marker_36a43948979f72db6c57ba89f15a98d5.bindPopup(popup_6ed823cb23e4d06c4cf1d1eb924d818d)\\n ;\\n\\n \\n \\n \\n var circle_marker_be72d67b47aaeb0ac7501ad3fbd1500d = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_82797928b43b6b282ecacdbeb6b1eb06 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f02931efa5004955e65e451cdebd5ece = $(`<div id="html_f02931efa5004955e65e451cdebd5ece" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82797928b43b6b282ecacdbeb6b1eb06.setContent(html_f02931efa5004955e65e451cdebd5ece);\\n \\n \\n\\n circle_marker_be72d67b47aaeb0ac7501ad3fbd1500d.bindPopup(popup_82797928b43b6b282ecacdbeb6b1eb06)\\n ;\\n\\n \\n \\n \\n var circle_marker_a2d388f368b887a2cfeaa395751a32a2 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b2dfd0e8f3e3ef0a2990bf0fe33ae79c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a03b42f065c738151e71f291846d34d8 = $(`<div id="html_a03b42f065c738151e71f291846d34d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2dfd0e8f3e3ef0a2990bf0fe33ae79c.setContent(html_a03b42f065c738151e71f291846d34d8);\\n \\n \\n\\n circle_marker_a2d388f368b887a2cfeaa395751a32a2.bindPopup(popup_b2dfd0e8f3e3ef0a2990bf0fe33ae79c)\\n ;\\n\\n \\n \\n \\n var circle_marker_11989ab0c1d8dee7cd36521443f465b9 = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e40ea27509b0fc782ab58311305d4d67 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1207ae3c95cb09611930c82934539871 = $(`<div id="html_1207ae3c95cb09611930c82934539871" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e40ea27509b0fc782ab58311305d4d67.setContent(html_1207ae3c95cb09611930c82934539871);\\n \\n \\n\\n circle_marker_11989ab0c1d8dee7cd36521443f465b9.bindPopup(popup_e40ea27509b0fc782ab58311305d4d67)\\n ;\\n\\n \\n \\n \\n var circle_marker_48d32f0474c786287ea57afea4d95973 = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_49476e15078a25e63c371915987d84c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01fdaa1677231af868c0863d195064a3 = $(`<div id="html_01fdaa1677231af868c0863d195064a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_49476e15078a25e63c371915987d84c9.setContent(html_01fdaa1677231af868c0863d195064a3);\\n \\n \\n\\n circle_marker_48d32f0474c786287ea57afea4d95973.bindPopup(popup_49476e15078a25e63c371915987d84c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6859028e90cafc62f72226982542f23 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0bbaa151028285ba726dd8217c872f50 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b89cb678a36a37d486c4bff9b4eb4b38 = $(`<div id="html_b89cb678a36a37d486c4bff9b4eb4b38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0bbaa151028285ba726dd8217c872f50.setContent(html_b89cb678a36a37d486c4bff9b4eb4b38);\\n \\n \\n\\n circle_marker_a6859028e90cafc62f72226982542f23.bindPopup(popup_0bbaa151028285ba726dd8217c872f50)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e6a0b3a55ecf79691a0a180bdde5abe = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fd50254696719a8e47190dc027e9e0c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d7ffe4adee342dba69e39fcb8c447d9a = $(`<div id="html_d7ffe4adee342dba69e39fcb8c447d9a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd50254696719a8e47190dc027e9e0c0.setContent(html_d7ffe4adee342dba69e39fcb8c447d9a);\\n \\n \\n\\n circle_marker_4e6a0b3a55ecf79691a0a180bdde5abe.bindPopup(popup_fd50254696719a8e47190dc027e9e0c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_150ce6f0ba4e05bea816a0284e9d23a6 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d73d04d36f4116cdd45f01bb6cd83bcb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1137cf07f39bca47b631be5aec75d93 = $(`<div id="html_d1137cf07f39bca47b631be5aec75d93" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d73d04d36f4116cdd45f01bb6cd83bcb.setContent(html_d1137cf07f39bca47b631be5aec75d93);\\n \\n \\n\\n circle_marker_150ce6f0ba4e05bea816a0284e9d23a6.bindPopup(popup_d73d04d36f4116cdd45f01bb6cd83bcb)\\n ;\\n\\n \\n \\n \\n var circle_marker_a03ee0738a4f3673402b247cba791488 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a7890db8dc5f3893bc0d93e76629c59d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_52680a706f235054fb198c1180f8d602 = $(`<div id="html_52680a706f235054fb198c1180f8d602" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7890db8dc5f3893bc0d93e76629c59d.setContent(html_52680a706f235054fb198c1180f8d602);\\n \\n \\n\\n circle_marker_a03ee0738a4f3673402b247cba791488.bindPopup(popup_a7890db8dc5f3893bc0d93e76629c59d)\\n ;\\n\\n \\n \\n \\n var circle_marker_4bc5c35808e79f846d3ea3584602c977 = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.583497844237541, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_af00d18dd254d5d8f68282e642b44b38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7801023dcb1ab08b90f63cbdbf7d188c = $(`<div id="html_7801023dcb1ab08b90f63cbdbf7d188c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af00d18dd254d5d8f68282e642b44b38.setContent(html_7801023dcb1ab08b90f63cbdbf7d188c);\\n \\n \\n\\n circle_marker_4bc5c35808e79f846d3ea3584602c977.bindPopup(popup_af00d18dd254d5d8f68282e642b44b38)\\n ;\\n\\n \\n \\n \\n var circle_marker_aea0892b07e284f17985c75be554f6a1 = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.886025119029199, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1e4a55a2d6814450c3de5392133fc034 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e426f2f0ce9d0c069f8ecd1e8ff1920 = $(`<div id="html_9e426f2f0ce9d0c069f8ecd1e8ff1920" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1e4a55a2d6814450c3de5392133fc034.setContent(html_9e426f2f0ce9d0c069f8ecd1e8ff1920);\\n \\n \\n\\n circle_marker_aea0892b07e284f17985c75be554f6a1.bindPopup(popup_1e4a55a2d6814450c3de5392133fc034)\\n ;\\n\\n \\n \\n \\n var circle_marker_33876a1f86af3f91b06e943db0448906 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_3aab17547339a727856cf57873411558 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a20bab92212d708a45b4ef17497c6168 = $(`<div id="html_a20bab92212d708a45b4ef17497c6168" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3aab17547339a727856cf57873411558.setContent(html_a20bab92212d708a45b4ef17497c6168);\\n \\n \\n\\n circle_marker_33876a1f86af3f91b06e943db0448906.bindPopup(popup_3aab17547339a727856cf57873411558)\\n ;\\n\\n \\n \\n \\n var circle_marker_8fa77d3c27b5b0b58fd5259b58dcb4c5 = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8360214cd3b0f0d59c59062bcf5b6e72 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a11797870ed8b201406524ef7048d4a = $(`<div id="html_1a11797870ed8b201406524ef7048d4a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8360214cd3b0f0d59c59062bcf5b6e72.setContent(html_1a11797870ed8b201406524ef7048d4a);\\n \\n \\n\\n circle_marker_8fa77d3c27b5b0b58fd5259b58dcb4c5.bindPopup(popup_8360214cd3b0f0d59c59062bcf5b6e72)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e84557ac6ababbb03e3eb3c026a6276 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_25093218f166cd17596fa6305677a4d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5de3b42ce25ebe30a94ad2b944947b5 = $(`<div id="html_a5de3b42ce25ebe30a94ad2b944947b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_25093218f166cd17596fa6305677a4d5.setContent(html_a5de3b42ce25ebe30a94ad2b944947b5);\\n \\n \\n\\n circle_marker_7e84557ac6ababbb03e3eb3c026a6276.bindPopup(popup_25093218f166cd17596fa6305677a4d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc945ae2d5f173d5fe1a8b25cad0d0a3 = L.circleMarker(\\n [42.73093682684808, -73.27225242598358],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fd91f0c3fcf9dcade950f5f787ca27af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_22f56e1566afc8f2243c08d4552a7da0 = $(`<div id="html_22f56e1566afc8f2243c08d4552a7da0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd91f0c3fcf9dcade950f5f787ca27af.setContent(html_22f56e1566afc8f2243c08d4552a7da0);\\n \\n \\n\\n circle_marker_fc945ae2d5f173d5fe1a8b25cad0d0a3.bindPopup(popup_fd91f0c3fcf9dcade950f5f787ca27af)\\n ;\\n\\n \\n \\n \\n var circle_marker_1047e425aebfc8dcc65cafd6abf991c7 = L.circleMarker(\\n [42.730936899260826, -73.2710223276323],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_153ded7e59946d824d8e1db924fc5cc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b8d3128ceefcfbf7325af87596b7e45 = $(`<div id="html_5b8d3128ceefcfbf7325af87596b7e45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_153ded7e59946d824d8e1db924fc5cc1.setContent(html_5b8d3128ceefcfbf7325af87596b7e45);\\n \\n \\n\\n circle_marker_1047e425aebfc8dcc65cafd6abf991c7.bindPopup(popup_153ded7e59946d824d8e1db924fc5cc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_43df82b0373cb1cedb1eec2c788e5dae = L.circleMarker(\\n [42.730936958507606, -73.26979222927841],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1b331cec49267619eeb71bd6cd238a79 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f1fb516939b397b1e299dbb1fe5a42d = $(`<div id="html_7f1fb516939b397b1e299dbb1fe5a42d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b331cec49267619eeb71bd6cd238a79.setContent(html_7f1fb516939b397b1e299dbb1fe5a42d);\\n \\n \\n\\n circle_marker_43df82b0373cb1cedb1eec2c788e5dae.bindPopup(popup_1b331cec49267619eeb71bd6cd238a79)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f300ad3501ca561129075ff178c314f = L.circleMarker(\\n [42.73093700458845, -73.26856213092245],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b2a93429e9c4425002f993c4c48b2a32 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6db297b10430880c44a3fcadcab96730 = $(`<div id="html_6db297b10430880c44a3fcadcab96730" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2a93429e9c4425002f993c4c48b2a32.setContent(html_6db297b10430880c44a3fcadcab96730);\\n \\n \\n\\n circle_marker_8f300ad3501ca561129075ff178c314f.bindPopup(popup_b2a93429e9c4425002f993c4c48b2a32)\\n ;\\n\\n \\n \\n \\n var circle_marker_44c1f6404ed471e72419e64e21071551 = L.circleMarker(\\n [42.73093703750333, -73.26733203256491],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_cdf661b96387887835af3d786b3f2df7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9773b0a0c40394aa7c630dd9c9ea4eb = $(`<div id="html_a9773b0a0c40394aa7c630dd9c9ea4eb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cdf661b96387887835af3d786b3f2df7.setContent(html_a9773b0a0c40394aa7c630dd9c9ea4eb);\\n \\n \\n\\n circle_marker_44c1f6404ed471e72419e64e21071551.bindPopup(popup_cdf661b96387887835af3d786b3f2df7)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3b80b2e390a3396760e297e9d863bd8 = L.circleMarker(\\n [42.73093706383524, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e43e5630dee60d75537641d0b5455afe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd8056c595d62d27c9ee09c0a33aeecf = $(`<div id="html_fd8056c595d62d27c9ee09c0a33aeecf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e43e5630dee60d75537641d0b5455afe.setContent(html_fd8056c595d62d27c9ee09c0a33aeecf);\\n \\n \\n\\n circle_marker_a3b80b2e390a3396760e297e9d863bd8.bindPopup(popup_e43e5630dee60d75537641d0b5455afe)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e310415eff01f49953537bedf7e2b46 = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d950c8bbbf5796977f6ac1ae4c818b13 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1130c3aaa83b594212573dbb44df9cf0 = $(`<div id="html_1130c3aaa83b594212573dbb44df9cf0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d950c8bbbf5796977f6ac1ae4c818b13.setContent(html_1130c3aaa83b594212573dbb44df9cf0);\\n \\n \\n\\n circle_marker_8e310415eff01f49953537bedf7e2b46.bindPopup(popup_d950c8bbbf5796977f6ac1ae4c818b13)\\n ;\\n\\n \\n \\n \\n var circle_marker_6852390cab20a35aea3ee913a7d72a22 = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_00d84076e70d5a81d6e53f77893a335e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_877b3ab520f05672f0fcb14ff037ecc2 = $(`<div id="html_877b3ab520f05672f0fcb14ff037ecc2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00d84076e70d5a81d6e53f77893a335e.setContent(html_877b3ab520f05672f0fcb14ff037ecc2);\\n \\n \\n\\n circle_marker_6852390cab20a35aea3ee913a7d72a22.bindPopup(popup_00d84076e70d5a81d6e53f77893a335e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3d4a95fb598f9a463dd8f3d2521e44a = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_3962cf41b320dec3c08d0b2d6ea3a924 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26e5f994a83c6af985533733389b49d2 = $(`<div id="html_26e5f994a83c6af985533733389b49d2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3962cf41b320dec3c08d0b2d6ea3a924.setContent(html_26e5f994a83c6af985533733389b49d2);\\n \\n \\n\\n circle_marker_a3d4a95fb598f9a463dd8f3d2521e44a.bindPopup(popup_3962cf41b320dec3c08d0b2d6ea3a924)\\n ;\\n\\n \\n \\n \\n var circle_marker_94649562ab75300b51e69f96df3715c3 = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_eb7bb47239f0d9394e0bad838585c08c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_581397977c3b070b05a6db2268ef7a26 = $(`<div id="html_581397977c3b070b05a6db2268ef7a26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eb7bb47239f0d9394e0bad838585c08c.setContent(html_581397977c3b070b05a6db2268ef7a26);\\n \\n \\n\\n circle_marker_94649562ab75300b51e69f96df3715c3.bindPopup(popup_eb7bb47239f0d9394e0bad838585c08c)\\n ;\\n\\n \\n \\n \\n var circle_marker_61fd80002c21bd7e8fdb980679a3cb3c = L.circleMarker(\\n [42.73093653061412, -73.25380095067791],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0ed41e315ae76c8f1c48e4af85cc64cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8741b24a03637470045c28a8aeb307df = $(`<div id="html_8741b24a03637470045c28a8aeb307df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0ed41e315ae76c8f1c48e4af85cc64cc.setContent(html_8741b24a03637470045c28a8aeb307df);\\n \\n \\n\\n circle_marker_61fd80002c21bd7e8fdb980679a3cb3c.bindPopup(popup_0ed41e315ae76c8f1c48e4af85cc64cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_238e6036447655f79f5ef96193d20a6d = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b74e0ab188aeff0ac6a8407c75d9172b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0970da9580a99b2ac5dc711d79c74881 = $(`<div id="html_0970da9580a99b2ac5dc711d79c74881" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b74e0ab188aeff0ac6a8407c75d9172b.setContent(html_0970da9580a99b2ac5dc711d79c74881);\\n \\n \\n\\n circle_marker_238e6036447655f79f5ef96193d20a6d.bindPopup(popup_b74e0ab188aeff0ac6a8407c75d9172b)\\n ;\\n\\n \\n \\n \\n var circle_marker_7b70056545fffd064279599cca4e1237 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9d71c5184a743efdbe58bec70290ed2d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07e59b5b69703ff834e47e150a9067a7 = $(`<div id="html_07e59b5b69703ff834e47e150a9067a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d71c5184a743efdbe58bec70290ed2d.setContent(html_07e59b5b69703ff834e47e150a9067a7);\\n \\n \\n\\n circle_marker_7b70056545fffd064279599cca4e1237.bindPopup(popup_9d71c5184a743efdbe58bec70290ed2d)\\n ;\\n\\n \\n \\n \\n var circle_marker_027a0e15c2263c3cf5e93ac13cfa0408 = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2a6d3936c0d3dce0c5a3a7da4ffb2c38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e4bb3582347450f7df0ab040d2469b5 = $(`<div id="html_6e4bb3582347450f7df0ab040d2469b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a6d3936c0d3dce0c5a3a7da4ffb2c38.setContent(html_6e4bb3582347450f7df0ab040d2469b5);\\n \\n \\n\\n circle_marker_027a0e15c2263c3cf5e93ac13cfa0408.bindPopup(popup_2a6d3936c0d3dce0c5a3a7da4ffb2c38)\\n ;\\n\\n \\n \\n \\n var circle_marker_12906efbda4f2e389b264b8da4a19332 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7ab72d272138a5c64359d4880c780098 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6805e9d689da0dc61df8396bc58cb0f0 = $(`<div id="html_6805e9d689da0dc61df8396bc58cb0f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7ab72d272138a5c64359d4880c780098.setContent(html_6805e9d689da0dc61df8396bc58cb0f0);\\n \\n \\n\\n circle_marker_12906efbda4f2e389b264b8da4a19332.bindPopup(popup_7ab72d272138a5c64359d4880c780098)\\n ;\\n\\n \\n \\n \\n var circle_marker_efbb069c598eaaa7bba4ca68584149a9 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_be57b07697ef95e5254ddfe2981be56f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2cfb7d509c9869da13b588437c9b15cb = $(`<div id="html_2cfb7d509c9869da13b588437c9b15cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be57b07697ef95e5254ddfe2981be56f.setContent(html_2cfb7d509c9869da13b588437c9b15cb);\\n \\n \\n\\n circle_marker_efbb069c598eaaa7bba4ca68584149a9.bindPopup(popup_be57b07697ef95e5254ddfe2981be56f)\\n ;\\n\\n \\n \\n \\n var circle_marker_be4421248014f03abd05209a02e70d02 = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fc14a0d6bb0b54c3c7f4ed4848c4245a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_799ad1bec3ec9545d94d3fd1053430d4 = $(`<div id="html_799ad1bec3ec9545d94d3fd1053430d4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc14a0d6bb0b54c3c7f4ed4848c4245a.setContent(html_799ad1bec3ec9545d94d3fd1053430d4);\\n \\n \\n\\n circle_marker_be4421248014f03abd05209a02e70d02.bindPopup(popup_fc14a0d6bb0b54c3c7f4ed4848c4245a)\\n ;\\n\\n \\n \\n \\n var circle_marker_978b0b68e1907b350bfa4bd3c89f45da = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ac226ff73046156e6035a2dde46a48c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b5145ecd17a31e5151b1326e34852f5 = $(`<div id="html_3b5145ecd17a31e5151b1326e34852f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac226ff73046156e6035a2dde46a48c1.setContent(html_3b5145ecd17a31e5151b1326e34852f5);\\n \\n \\n\\n circle_marker_978b0b68e1907b350bfa4bd3c89f45da.bindPopup(popup_ac226ff73046156e6035a2dde46a48c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_75e27610765130f21142a2f276325a56 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a709c76fdf3e449d6c28d130f2465808 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1b6715d9d59331b3f0e5cb18b9c66d27 = $(`<div id="html_1b6715d9d59331b3f0e5cb18b9c66d27" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a709c76fdf3e449d6c28d130f2465808.setContent(html_1b6715d9d59331b3f0e5cb18b9c66d27);\\n \\n \\n\\n circle_marker_75e27610765130f21142a2f276325a56.bindPopup(popup_a709c76fdf3e449d6c28d130f2465808)\\n ;\\n\\n \\n \\n \\n var circle_marker_07120159cc7380b448a60f170b4de93a = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f7dc2bf5e6ea51dce4dcc5d3302096f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db627edde6b4d4b6d1e96168d67c2b09 = $(`<div id="html_db627edde6b4d4b6d1e96168d67c2b09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f7dc2bf5e6ea51dce4dcc5d3302096f2.setContent(html_db627edde6b4d4b6d1e96168d67c2b09);\\n \\n \\n\\n circle_marker_07120159cc7380b448a60f170b4de93a.bindPopup(popup_f7dc2bf5e6ea51dce4dcc5d3302096f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_edf80d52dd6bb6367d2b9ea2065a973c = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_baadf4d17700a1be7c3222b939ee0133 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63be1da8cd8edfdb8f3ba396d5648ea3 = $(`<div id="html_63be1da8cd8edfdb8f3ba396d5648ea3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_baadf4d17700a1be7c3222b939ee0133.setContent(html_63be1da8cd8edfdb8f3ba396d5648ea3);\\n \\n \\n\\n circle_marker_edf80d52dd6bb6367d2b9ea2065a973c.bindPopup(popup_baadf4d17700a1be7c3222b939ee0133)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d9b269daa2bfa0fa60bfac5647bf754 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_79b728b09d9371857281dc61ed620381 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8385af8062ede91c2cdb4d34998d417a = $(`<div id="html_8385af8062ede91c2cdb4d34998d417a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79b728b09d9371857281dc61ed620381.setContent(html_8385af8062ede91c2cdb4d34998d417a);\\n \\n \\n\\n circle_marker_2d9b269daa2bfa0fa60bfac5647bf754.bindPopup(popup_79b728b09d9371857281dc61ed620381)\\n ;\\n\\n \\n \\n \\n var circle_marker_c20321a4ec8d6331b67818e7bd3fee42 = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b320566522449c3186f068580bd29228 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ab5db1b3b3884de3c3c4d778a6a7887a = $(`<div id="html_ab5db1b3b3884de3c3c4d778a6a7887a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b320566522449c3186f068580bd29228.setContent(html_ab5db1b3b3884de3c3c4d778a6a7887a);\\n \\n \\n\\n circle_marker_c20321a4ec8d6331b67818e7bd3fee42.bindPopup(popup_b320566522449c3186f068580bd29228)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ad76ca7d8fc46aa3c10bdc8259efbad = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_858e9bd9ed3229aa7bc2022479b8e216 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_25f09adfd51f7cc9c14d1fc6f7c43d78 = $(`<div id="html_25f09adfd51f7cc9c14d1fc6f7c43d78" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_858e9bd9ed3229aa7bc2022479b8e216.setContent(html_25f09adfd51f7cc9c14d1fc6f7c43d78);\\n \\n \\n\\n circle_marker_3ad76ca7d8fc46aa3c10bdc8259efbad.bindPopup(popup_858e9bd9ed3229aa7bc2022479b8e216)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e15a0da0bfae2afc29fc725d88fd075 = L.circleMarker(\\n [42.73093113915656, -73.22796888742165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.997417539138688, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9bee62d3b54efbd758cebd109002e37a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94503ec777728f0e783e9370c18b3646 = $(`<div id="html_94503ec777728f0e783e9370c18b3646" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9bee62d3b54efbd758cebd109002e37a.setContent(html_94503ec777728f0e783e9370c18b3646);\\n \\n \\n\\n circle_marker_6e15a0da0bfae2afc29fc725d88fd075.bindPopup(popup_9bee62d3b54efbd758cebd109002e37a)\\n ;\\n\\n \\n \\n \\n var circle_marker_628f340a60a652ca77881f6ffdcfc713 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2040f8dbf91eedef95dffbedd860369c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2e08cb62921cc9a5095b81e6d0c1eb93 = $(`<div id="html_2e08cb62921cc9a5095b81e6d0c1eb93" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2040f8dbf91eedef95dffbedd860369c.setContent(html_2e08cb62921cc9a5095b81e6d0c1eb93);\\n \\n \\n\\n circle_marker_628f340a60a652ca77881f6ffdcfc713.bindPopup(popup_2040f8dbf91eedef95dffbedd860369c)\\n ;\\n\\n \\n \\n \\n var circle_marker_856c441fae38978de217043055907722 = L.circleMarker(\\n [42.73093032286755, -73.22550869120535],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8849ce89d929e189a5f17f4c82b6162f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd5929b02bd0646688a0f2bf95ad56e4 = $(`<div id="html_fd5929b02bd0646688a0f2bf95ad56e4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8849ce89d929e189a5f17f4c82b6162f.setContent(html_fd5929b02bd0646688a0f2bf95ad56e4);\\n \\n \\n\\n circle_marker_856c441fae38978de217043055907722.bindPopup(popup_8849ce89d929e189a5f17f4c82b6162f)\\n ;\\n\\n \\n \\n \\n var circle_marker_4d1d00a0a3b7b6e240703e239b654985 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_132cca38d0a62d01ca67f2c9e6086da7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8dabb041d418dc096ddb455dda371ae = $(`<div id="html_a8dabb041d418dc096ddb455dda371ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_132cca38d0a62d01ca67f2c9e6086da7.setContent(html_a8dabb041d418dc096ddb455dda371ae);\\n \\n \\n\\n circle_marker_4d1d00a0a3b7b6e240703e239b654985.bindPopup(popup_132cca38d0a62d01ca67f2c9e6086da7)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3d607d5601f90604f5191201cca97aa = L.circleMarker(\\n [42.73092945391477, -73.2230484950559],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.627726932618989, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8db5c7312356304474a79918d1808ea6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5e032143c1796e3e5fba9e6ebe877561 = $(`<div id="html_5e032143c1796e3e5fba9e6ebe877561" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8db5c7312356304474a79918d1808ea6.setContent(html_5e032143c1796e3e5fba9e6ebe877561);\\n \\n \\n\\n circle_marker_a3d607d5601f90604f5191201cca97aa.bindPopup(popup_8db5c7312356304474a79918d1808ea6)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d7f24ea5f0dc279cb967f8a30ef5c4d = L.circleMarker(\\n [42.73092899968945, -73.22181839700757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e5eae00f215f2fcffcfc41fb0e038b23 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_779bfb1bceb8d9dd2441a6a4ab12aa77 = $(`<div id="html_779bfb1bceb8d9dd2441a6a4ab12aa77" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5eae00f215f2fcffcfc41fb0e038b23.setContent(html_779bfb1bceb8d9dd2441a6a4ab12aa77);\\n \\n \\n\\n circle_marker_9d7f24ea5f0dc279cb967f8a30ef5c4d.bindPopup(popup_e5eae00f215f2fcffcfc41fb0e038b23)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcc8d68796c2f9655b8db808c8c04fe6 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_96215e16704c202736c520a5f116719a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_880498ad1d75e15a4a3fed9cc26aac75 = $(`<div id="html_880498ad1d75e15a4a3fed9cc26aac75" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_96215e16704c202736c520a5f116719a.setContent(html_880498ad1d75e15a4a3fed9cc26aac75);\\n \\n \\n\\n circle_marker_bcc8d68796c2f9655b8db808c8c04fe6.bindPopup(popup_96215e16704c202736c520a5f116719a)\\n ;\\n\\n \\n \\n \\n var circle_marker_14ec7cad5b3a02c916fbcf8ab9b72a60 = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9d87d987213b276afbf2dfe1d66066dc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49bc5960cd6c75777b7e91203bde172f = $(`<div id="html_49bc5960cd6c75777b7e91203bde172f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d87d987213b276afbf2dfe1d66066dc.setContent(html_49bc5960cd6c75777b7e91203bde172f);\\n \\n \\n\\n circle_marker_14ec7cad5b3a02c916fbcf8ab9b72a60.bindPopup(popup_9d87d987213b276afbf2dfe1d66066dc)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec8017ff021b4f33899fd65fc83e11fd = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e2ccf3b13b92bcdcc69327b1507cf39e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82f627b4cd7b1143344fd492962489cf = $(`<div id="html_82f627b4cd7b1143344fd492962489cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2ccf3b13b92bcdcc69327b1507cf39e.setContent(html_82f627b4cd7b1143344fd492962489cf);\\n \\n \\n\\n circle_marker_ec8017ff021b4f33899fd65fc83e11fd.bindPopup(popup_e2ccf3b13b92bcdcc69327b1507cf39e)\\n ;\\n\\n \\n \\n \\n var circle_marker_32cefe14f8a52972f3a3911c946434bd = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_54b0095a7e799c70f52626ee6beb6ab7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b0f0f032505ea8ce33967851a0a7b69 = $(`<div id="html_3b0f0f032505ea8ce33967851a0a7b69" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54b0095a7e799c70f52626ee6beb6ab7.setContent(html_3b0f0f032505ea8ce33967851a0a7b69);\\n \\n \\n\\n circle_marker_32cefe14f8a52972f3a3911c946434bd.bindPopup(popup_54b0095a7e799c70f52626ee6beb6ab7)\\n ;\\n\\n \\n \\n \\n var circle_marker_e012ad43996a1a5c870d757652e628ab = L.circleMarker(\\n [42.72912950916773, -73.27471233593516],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c1621f5acb286c1259c897df93e22fd8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c7a7d1a6968069a3bd95ef649a0c9ff = $(`<div id="html_9c7a7d1a6968069a3bd95ef649a0c9ff" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c1621f5acb286c1259c897df93e22fd8.setContent(html_9c7a7d1a6968069a3bd95ef649a0c9ff);\\n \\n \\n\\n circle_marker_e012ad43996a1a5c870d757652e628ab.bindPopup(popup_c1621f5acb286c1259c897df93e22fd8)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d07865ed9ce4c2f1cda6cb235ade662 = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_177dcadce7d9a0d3db58116df5954852 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53c7d0d68bc0c9e39ad1c6c701e7ae8c = $(`<div id="html_53c7d0d68bc0c9e39ad1c6c701e7ae8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_177dcadce7d9a0d3db58116df5954852.setContent(html_53c7d0d68bc0c9e39ad1c6c701e7ae8c);\\n \\n \\n\\n circle_marker_2d07865ed9ce4c2f1cda6cb235ade662.bindPopup(popup_177dcadce7d9a0d3db58116df5954852)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb13697f5186be0827443879afe403e4 = L.circleMarker(\\n [42.729129693483614, -73.27225221092779],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_334389bd73f5e63e6a379be1b9f74b2f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ba627c245c6e99b2c90869dff26c4e8 = $(`<div id="html_1ba627c245c6e99b2c90869dff26c4e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_334389bd73f5e63e6a379be1b9f74b2f.setContent(html_1ba627c245c6e99b2c90869dff26c4e8);\\n \\n \\n\\n circle_marker_eb13697f5186be0827443879afe403e4.bindPopup(popup_334389bd73f5e63e6a379be1b9f74b2f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8d558c22def3602ceb2cb55012d43d0 = L.circleMarker(\\n [42.72912976589343, -73.27102214841915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_76c68d3c7432e7e10bdf8d1b1ddf6a95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e06dda08a0edc161ac369dba9ffe6de1 = $(`<div id="html_e06dda08a0edc161ac369dba9ffe6de1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_76c68d3c7432e7e10bdf8d1b1ddf6a95.setContent(html_e06dda08a0edc161ac369dba9ffe6de1);\\n \\n \\n\\n circle_marker_e8d558c22def3602ceb2cb55012d43d0.bindPopup(popup_76c68d3c7432e7e10bdf8d1b1ddf6a95)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d81ef6d87d6414e831d8ce9a31094f3 = L.circleMarker(\\n [42.72912982513782, -73.2697920859079],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b16bc5bae4420554cdb00db0de2bc68e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14ea84ae4ee6e825843468750467bcde = $(`<div id="html_14ea84ae4ee6e825843468750467bcde" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b16bc5bae4420554cdb00db0de2bc68e.setContent(html_14ea84ae4ee6e825843468750467bcde);\\n \\n \\n\\n circle_marker_9d81ef6d87d6414e831d8ce9a31094f3.bindPopup(popup_b16bc5bae4420554cdb00db0de2bc68e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6c6a6b9d19f9633140614eb6fc3ae967 = L.circleMarker(\\n [42.729129871216784, -73.26856202339455],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_00857a359c3fd8d58685e89318f84c0e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc498a370f39e7531123824c55cd357f = $(`<div id="html_fc498a370f39e7531123824c55cd357f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00857a359c3fd8d58685e89318f84c0e.setContent(html_fc498a370f39e7531123824c55cd357f);\\n \\n \\n\\n circle_marker_6c6a6b9d19f9633140614eb6fc3ae967.bindPopup(popup_00857a359c3fd8d58685e89318f84c0e)\\n ;\\n\\n \\n \\n \\n var circle_marker_959811599ff8d07d1951a73de831c67d = L.circleMarker(\\n [42.72912990413034, -73.26733196087964],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b0af485298c83ef01701585958f5df53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d3f2ab421b8c7704b39a76f745c4b09 = $(`<div id="html_0d3f2ab421b8c7704b39a76f745c4b09" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0af485298c83ef01701585958f5df53.setContent(html_0d3f2ab421b8c7704b39a76f745c4b09);\\n \\n \\n\\n circle_marker_959811599ff8d07d1951a73de831c67d.bindPopup(popup_b0af485298c83ef01701585958f5df53)\\n ;\\n\\n \\n \\n \\n var circle_marker_820c460f094e46a3b5c794bac28e4f36 = L.circleMarker(\\n [42.72912992387847, -73.26610189836369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8c4448d671a2aa2d3ef35825169f6130 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2f469fa0bf1be4b6e327642d2d6846ea = $(`<div id="html_2f469fa0bf1be4b6e327642d2d6846ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c4448d671a2aa2d3ef35825169f6130.setContent(html_2f469fa0bf1be4b6e327642d2d6846ea);\\n \\n \\n\\n circle_marker_820c460f094e46a3b5c794bac28e4f36.bindPopup(popup_8c4448d671a2aa2d3ef35825169f6130)\\n ;\\n\\n \\n \\n \\n var circle_marker_a0998a30ffd7b30781de69848a3df057 = L.circleMarker(\\n [42.72912993046118, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e61e9709ed33e36fa270bf28fea0a664 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a8c377fc2423224989788d8cb576ffa = $(`<div id="html_1a8c377fc2423224989788d8cb576ffa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e61e9709ed33e36fa270bf28fea0a664.setContent(html_1a8c377fc2423224989788d8cb576ffa);\\n \\n \\n\\n circle_marker_a0998a30ffd7b30781de69848a3df057.bindPopup(popup_e61e9709ed33e36fa270bf28fea0a664)\\n ;\\n\\n \\n \\n \\n var circle_marker_294205cc5a664b29d78cd6f5adbd85bb = L.circleMarker(\\n [42.72912992387847, -73.26364177333073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_cdead1d46fe092be131560eea06663fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c4433df394e429275aa39d9e8ca11dc3 = $(`<div id="html_c4433df394e429275aa39d9e8ca11dc3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cdead1d46fe092be131560eea06663fb.setContent(html_c4433df394e429275aa39d9e8ca11dc3);\\n \\n \\n\\n circle_marker_294205cc5a664b29d78cd6f5adbd85bb.bindPopup(popup_cdead1d46fe092be131560eea06663fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_bce33c1138a5ad9f9fcc6d59483351aa = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7f458e89b754ab57bf8037fb4e13c2dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a56a21511dc2a4731b77ac5f7c239d1f = $(`<div id="html_a56a21511dc2a4731b77ac5f7c239d1f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f458e89b754ab57bf8037fb4e13c2dd.setContent(html_a56a21511dc2a4731b77ac5f7c239d1f);\\n \\n \\n\\n circle_marker_bce33c1138a5ad9f9fcc6d59483351aa.bindPopup(popup_7f458e89b754ab57bf8037fb4e13c2dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b31ef198f2d4a1b29db0b35e35202f2 = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8c7a00faf95ffcd1c1e1508ce83e5fb7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f0a6f82a5fdbe48550206c125190c27 = $(`<div id="html_4f0a6f82a5fdbe48550206c125190c27" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c7a00faf95ffcd1c1e1508ce83e5fb7.setContent(html_4f0a6f82a5fdbe48550206c125190c27);\\n \\n \\n\\n circle_marker_8b31ef198f2d4a1b29db0b35e35202f2.bindPopup(popup_8c7a00faf95ffcd1c1e1508ce83e5fb7)\\n ;\\n\\n \\n \\n \\n var circle_marker_222c11d24dfb5a3939bb4112ed91ce55 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7cefa9712f6143f2069f54bf8e7c57d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed23bffc3635976887c38974f421aad3 = $(`<div id="html_ed23bffc3635976887c38974f421aad3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7cefa9712f6143f2069f54bf8e7c57d1.setContent(html_ed23bffc3635976887c38974f421aad3);\\n \\n \\n\\n circle_marker_222c11d24dfb5a3939bb4112ed91ce55.bindPopup(popup_7cefa9712f6143f2069f54bf8e7c57d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_b8f8842688c0ef235bd583a604b6342f = L.circleMarker(\\n [42.72912913395327, -73.25134114828086],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0c74ea993428b9b31792c1177c121266 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_45d6a5df2b28ce1d6dcc8ca3a282b4cb = $(`<div id="html_45d6a5df2b28ce1d6dcc8ca3a282b4cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c74ea993428b9b31792c1177c121266.setContent(html_45d6a5df2b28ce1d6dcc8ca3a282b4cb);\\n \\n \\n\\n circle_marker_b8f8842688c0ef235bd583a604b6342f.bindPopup(popup_0c74ea993428b9b31792c1177c121266)\\n ;\\n\\n \\n \\n \\n var circle_marker_20b5bd5295db1420001003e0ea9fd8e1 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_14d63b9571c4f2accdca9b6fca331685 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_78be8ad583d0699f8a99eb13d28d80db = $(`<div id="html_78be8ad583d0699f8a99eb13d28d80db" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14d63b9571c4f2accdca9b6fca331685.setContent(html_78be8ad583d0699f8a99eb13d28d80db);\\n \\n \\n\\n circle_marker_20b5bd5295db1420001003e0ea9fd8e1.bindPopup(popup_14d63b9571c4f2accdca9b6fca331685)\\n ;\\n\\n \\n \\n \\n var circle_marker_8ec93596294e4c78f8c369834fb748c9 = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a4569791d085aa21e3689c911276f426 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3801794a248bb325c8f109593e274bd0 = $(`<div id="html_3801794a248bb325c8f109593e274bd0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a4569791d085aa21e3689c911276f426.setContent(html_3801794a248bb325c8f109593e274bd0);\\n \\n \\n\\n circle_marker_8ec93596294e4c78f8c369834fb748c9.bindPopup(popup_a4569791d085aa21e3689c911276f426)\\n ;\\n\\n \\n \\n \\n var circle_marker_cc8123ff5dc1002ddffa7bfa451e7cfd = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b39e3a7d10e9da4970e41667f8cf7f31 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad7f9088ed854e77e280f893d564c009 = $(`<div id="html_ad7f9088ed854e77e280f893d564c009" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b39e3a7d10e9da4970e41667f8cf7f31.setContent(html_ad7f9088ed854e77e280f893d564c009);\\n \\n \\n\\n circle_marker_cc8123ff5dc1002ddffa7bfa451e7cfd.bindPopup(popup_b39e3a7d10e9da4970e41667f8cf7f31)\\n ;\\n\\n \\n \\n \\n var circle_marker_794f4b9bfba8396b9d5e7cd867c4fe3c = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9bbe85470085878f9daea7ad37dfee0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d09db19b0eaf12fd76329380ea110c27 = $(`<div id="html_d09db19b0eaf12fd76329380ea110c27" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9bbe85470085878f9daea7ad37dfee0d.setContent(html_d09db19b0eaf12fd76329380ea110c27);\\n \\n \\n\\n circle_marker_794f4b9bfba8396b9d5e7cd867c4fe3c.bindPopup(popup_9bbe85470085878f9daea7ad37dfee0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_b11b03960c1de20031c0709f7aec84aa = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.51351666838205, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0af516359d888e2c172ff0bfd06d734d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_51dbc0a437a4c3381ad15b6c3c033bfd = $(`<div id="html_51dbc0a437a4c3381ad15b6c3c033bfd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0af516359d888e2c172ff0bfd06d734d.setContent(html_51dbc0a437a4c3381ad15b6c3c033bfd);\\n \\n \\n\\n circle_marker_b11b03960c1de20031c0709f7aec84aa.bindPopup(popup_0af516359d888e2c172ff0bfd06d734d)\\n ;\\n\\n \\n \\n \\n var circle_marker_374c56447f52808fbc026e27301d1ecf = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_5c234de87eb5671374a746294bfeffbf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d8b58f563480e2cf7e1a2c63c1b0635 = $(`<div id="html_0d8b58f563480e2cf7e1a2c63c1b0635" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c234de87eb5671374a746294bfeffbf.setContent(html_0d8b58f563480e2cf7e1a2c63c1b0635);\\n \\n \\n\\n circle_marker_374c56447f52808fbc026e27301d1ecf.bindPopup(popup_5c234de87eb5671374a746294bfeffbf)\\n ;\\n\\n \\n \\n \\n var circle_marker_be58dd07b9f0142513a4eb4e69f7c4e3 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b6144b8d6a683752c530783401aa6558 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06568245da7a5cf2120b967a3f325f21 = $(`<div id="html_06568245da7a5cf2120b967a3f325f21" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b6144b8d6a683752c530783401aa6558.setContent(html_06568245da7a5cf2120b967a3f325f21);\\n \\n \\n\\n circle_marker_be58dd07b9f0142513a4eb4e69f7c4e3.bindPopup(popup_b6144b8d6a683752c530783401aa6558)\\n ;\\n\\n \\n \\n \\n var circle_marker_d977481f1be97f63ca6773be47583b0f = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_982ed5e88620eb3aaa4f26a2ce270a0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_79b53de9d29f0bb04b996b3279fdb239 = $(`<div id="html_79b53de9d29f0bb04b996b3279fdb239" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_982ed5e88620eb3aaa4f26a2ce270a0d.setContent(html_79b53de9d29f0bb04b996b3279fdb239);\\n \\n \\n\\n circle_marker_d977481f1be97f63ca6773be47583b0f.bindPopup(popup_982ed5e88620eb3aaa4f26a2ce270a0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_3378cb74c9a34c24b75d1a37447edba7 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.4700209598571305, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1311cd92697f8e5f3827d16c49b37e6f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b737e8752c6432ac3ec60333a382dea7 = $(`<div id="html_b737e8752c6432ac3ec60333a382dea7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1311cd92697f8e5f3827d16c49b37e6f.setContent(html_b737e8752c6432ac3ec60333a382dea7);\\n \\n \\n\\n circle_marker_3378cb74c9a34c24b75d1a37447edba7.bindPopup(popup_1311cd92697f8e5f3827d16c49b37e6f)\\n ;\\n\\n \\n \\n \\n var circle_marker_382dd62f8db9757fe92f9433cede04ba = L.circleMarker(\\n [42.729122320849, -73.22304971370495],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b18ac6e5654f1c333f689a05a599308d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95698381d94b80d697de1618e4ed5d56 = $(`<div id="html_95698381d94b80d697de1618e4ed5d56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b18ac6e5654f1c333f689a05a599308d.setContent(html_95698381d94b80d697de1618e4ed5d56);\\n \\n \\n\\n circle_marker_382dd62f8db9757fe92f9433cede04ba.bindPopup(popup_b18ac6e5654f1c333f689a05a599308d)\\n ;\\n\\n \\n \\n \\n var circle_marker_9363c801fd848eb41a522196b14f0104 = L.circleMarker(\\n [42.729121866642096, -73.22181965149919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.442433223290478, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_852d045ee18338344f3c5d2dd35cbac3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb6e315f16764872f949df4983313c99 = $(`<div id="html_fb6e315f16764872f949df4983313c99" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_852d045ee18338344f3c5d2dd35cbac3.setContent(html_fb6e315f16764872f949df4983313c99);\\n \\n \\n\\n circle_marker_9363c801fd848eb41a522196b14f0104.bindPopup(popup_852d045ee18338344f3c5d2dd35cbac3)\\n ;\\n\\n \\n \\n \\n var circle_marker_e5c44e83c189cd891f7b91b08348deef = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8fb7f833ef7f3ad03d04e238b543a4af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_35c0916ebf329fc74e160ac31da6985b = $(`<div id="html_35c0916ebf329fc74e160ac31da6985b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8fb7f833ef7f3ad03d04e238b543a4af.setContent(html_35c0916ebf329fc74e160ac31da6985b);\\n \\n \\n\\n circle_marker_e5c44e83c189cd891f7b91b08348deef.bindPopup(popup_8fb7f833ef7f3ad03d04e238b543a4af)\\n ;\\n\\n \\n \\n \\n var circle_marker_2aae035fb25514f57ea7d7b961aee801 = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c7a8bfddf5d005dacb000b1de72ec323 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_219f65408c9b4bc7d5079bba4f5849d7 = $(`<div id="html_219f65408c9b4bc7d5079bba4f5849d7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c7a8bfddf5d005dacb000b1de72ec323.setContent(html_219f65408c9b4bc7d5079bba4f5849d7);\\n \\n \\n\\n circle_marker_2aae035fb25514f57ea7d7b961aee801.bindPopup(popup_c7a8bfddf5d005dacb000b1de72ec323)\\n ;\\n\\n \\n \\n \\n var circle_marker_f393e847573ad248f83886ac8194f0c6 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4d6915314ba31f6154e3db2d65bf21d9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1d6a95ffa507fd2689fc9ee6a4f05442 = $(`<div id="html_1d6a95ffa507fd2689fc9ee6a4f05442" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4d6915314ba31f6154e3db2d65bf21d9.setContent(html_1d6a95ffa507fd2689fc9ee6a4f05442);\\n \\n \\n\\n circle_marker_f393e847573ad248f83886ac8194f0c6.bindPopup(popup_4d6915314ba31f6154e3db2d65bf21d9)\\n ;\\n\\n \\n \\n \\n var circle_marker_b00f81f0a1826fea9555e534c0eedc0e = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a29ce748d428520c982e118d39373cff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_420597ffa813dbed130272e7c265409f = $(`<div id="html_420597ffa813dbed130272e7c265409f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a29ce748d428520c982e118d39373cff.setContent(html_420597ffa813dbed130272e7c265409f);\\n \\n \\n\\n circle_marker_b00f81f0a1826fea9555e534c0eedc0e.bindPopup(popup_a29ce748d428520c982e118d39373cff)\\n ;\\n\\n \\n \\n \\n var circle_marker_28833b2afb0fd91791f9cec0fda7e688 = L.circleMarker(\\n [42.72732237581074, -73.27471204921736],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.54864184146723, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e30c73fbfb8a018f2fbf3acbf39c98bb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2d825b3a4eddd42abfdeafb3703c3fa = $(`<div id="html_d2d825b3a4eddd42abfdeafb3703c3fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e30c73fbfb8a018f2fbf3acbf39c98bb.setContent(html_d2d825b3a4eddd42abfdeafb3703c3fa);\\n \\n \\n\\n circle_marker_28833b2afb0fd91791f9cec0fda7e688.bindPopup(popup_e30c73fbfb8a018f2fbf3acbf39c98bb)\\n ;\\n\\n \\n \\n \\n var circle_marker_0916251c53399b42cd0f418a2b5fb59e = L.circleMarker(\\n [42.72732247454739, -73.27348202255521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_817c1c82baed85e23eaee62c58e02161 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edc3555a0e7775905c40e1ad3fc45c06 = $(`<div id="html_edc3555a0e7775905c40e1ad3fc45c06" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_817c1c82baed85e23eaee62c58e02161.setContent(html_edc3555a0e7775905c40e1ad3fc45c06);\\n \\n \\n\\n circle_marker_0916251c53399b42cd0f418a2b5fb59e.bindPopup(popup_817c1c82baed85e23eaee62c58e02161)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e6f95ac32fac186b9fac473002b077f = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_3ad201b04563d9aca21fdb777583678e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63ff67799b5a81fe8de9b814f198f130 = $(`<div id="html_63ff67799b5a81fe8de9b814f198f130" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ad201b04563d9aca21fdb777583678e.setContent(html_63ff67799b5a81fe8de9b814f198f130);\\n \\n \\n\\n circle_marker_2e6f95ac32fac186b9fac473002b077f.bindPopup(popup_3ad201b04563d9aca21fdb777583678e)\\n ;\\n\\n \\n \\n \\n var circle_marker_06d18ba793855f91e7d21ecc7ebc8058 = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_167b50c56cf8264847c0f2d9829c6a3d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e0f88d790ccce300ecf502fd591dfe94 = $(`<div id="html_e0f88d790ccce300ecf502fd591dfe94" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_167b50c56cf8264847c0f2d9829c6a3d.setContent(html_e0f88d790ccce300ecf502fd591dfe94);\\n \\n \\n\\n circle_marker_06d18ba793855f91e7d21ecc7ebc8058.bindPopup(popup_167b50c56cf8264847c0f2d9829c6a3d)\\n ;\\n\\n \\n \\n \\n var circle_marker_f878f9bbb61ad2f5b9adb3e030f40ec8 = L.circleMarker(\\n [42.727322737845135, -73.26856191587537],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6d79500df785e1b8209941c2523c29cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_690618e941c5a82f8bf9c88d050677c7 = $(`<div id="html_690618e941c5a82f8bf9c88d050677c7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d79500df785e1b8209941c2523c29cf.setContent(html_690618e941c5a82f8bf9c88d050677c7);\\n \\n \\n\\n circle_marker_f878f9bbb61ad2f5b9adb3e030f40ec8.bindPopup(popup_6d79500df785e1b8209941c2523c29cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4d88f7edacee9a3888ba3203f96697c = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b2376ba3909a6d1859004dd70e9e532d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_894eb0e019afb7a5abea2841aadee33c = $(`<div id="html_894eb0e019afb7a5abea2841aadee33c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2376ba3909a6d1859004dd70e9e532d.setContent(html_894eb0e019afb7a5abea2841aadee33c);\\n \\n \\n\\n circle_marker_e4d88f7edacee9a3888ba3203f96697c.bindPopup(popup_b2376ba3909a6d1859004dd70e9e532d)\\n ;\\n\\n \\n \\n \\n var circle_marker_76573e620d2b511723bbc461c8afecd6 = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f902ceb6fc742f1e49fba97256e9d9f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_48535d49cbd457132113906bf5df1173 = $(`<div id="html_48535d49cbd457132113906bf5df1173" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f902ceb6fc742f1e49fba97256e9d9f3.setContent(html_48535d49cbd457132113906bf5df1173);\\n \\n \\n\\n circle_marker_76573e620d2b511723bbc461c8afecd6.bindPopup(popup_f902ceb6fc742f1e49fba97256e9d9f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_98e5f92f252d2700139b8e2ebb94fa5f = L.circleMarker(\\n [42.727322797087126, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_679b7958ded200dbfbb77b59c7efb68a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b792940cb98ac303b17fc00098ddd397 = $(`<div id="html_b792940cb98ac303b17fc00098ddd397" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_679b7958ded200dbfbb77b59c7efb68a.setContent(html_b792940cb98ac303b17fc00098ddd397);\\n \\n \\n\\n circle_marker_98e5f92f252d2700139b8e2ebb94fa5f.bindPopup(popup_679b7958ded200dbfbb77b59c7efb68a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a2e50e27480e681c544151b77e24516 = L.circleMarker(\\n [42.72732279050467, -73.26364180917045],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_491b0eec9f47f3539c7f1e0364a17552 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f0a3258a6f763887a5a2ad5bd9283537 = $(`<div id="html_f0a3258a6f763887a5a2ad5bd9283537" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_491b0eec9f47f3539c7f1e0364a17552.setContent(html_f0a3258a6f763887a5a2ad5bd9283537);\\n \\n \\n\\n circle_marker_8a2e50e27480e681c544151b77e24516.bindPopup(popup_491b0eec9f47f3539c7f1e0364a17552)\\n ;\\n\\n \\n \\n \\n var circle_marker_7bd88dd71c4ba240b396fce7c42bb6a8 = L.circleMarker(\\n [42.72732277075734, -73.26241178249424],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_9cb63225e6194d1efb20aa02a1247009 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff3eaef90b68d809df4495b6460dacb6 = $(`<div id="html_ff3eaef90b68d809df4495b6460dacb6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9cb63225e6194d1efb20aa02a1247009.setContent(html_ff3eaef90b68d809df4495b6460dacb6);\\n \\n \\n\\n circle_marker_7bd88dd71c4ba240b396fce7c42bb6a8.bindPopup(popup_9cb63225e6194d1efb20aa02a1247009)\\n ;\\n\\n \\n \\n \\n var circle_marker_e3d2aed08276b869084761f62b2e2a83 = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_390b7f0580b2bc7b5e686192b4bfe7e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a50e5d208b8ef06a3e0cfb846ac27133 = $(`<div id="html_a50e5d208b8ef06a3e0cfb846ac27133" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_390b7f0580b2bc7b5e686192b4bfe7e4.setContent(html_a50e5d208b8ef06a3e0cfb846ac27133);\\n \\n \\n\\n circle_marker_e3d2aed08276b869084761f62b2e2a83.bindPopup(popup_390b7f0580b2bc7b5e686192b4bfe7e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c7604730a7f510a1708c0cff8a7b30b = L.circleMarker(\\n [42.72732269176802, -73.25995172914543],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_243efe1f0a8c0f11772e49bc225c0c6a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6329510ca9ebb11be58d7e7c654d7bc = $(`<div id="html_d6329510ca9ebb11be58d7e7c654d7bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_243efe1f0a8c0f11772e49bc225c0c6a.setContent(html_d6329510ca9ebb11be58d7e7c654d7bc);\\n \\n \\n\\n circle_marker_2c7604730a7f510a1708c0cff8a7b30b.bindPopup(popup_243efe1f0a8c0f11772e49bc225c0c6a)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c894a9c9e91df4b565f2452dd08fb2b = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c86fc3ed111b3dd401e0ffc41105770d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f5bcebc0eb6e26d8a6c5375d6978ae9 = $(`<div id="html_6f5bcebc0eb6e26d8a6c5375d6978ae9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c86fc3ed111b3dd401e0ffc41105770d.setContent(html_6f5bcebc0eb6e26d8a6c5375d6978ae9);\\n \\n \\n\\n circle_marker_0c894a9c9e91df4b565f2452dd08fb2b.bindPopup(popup_c86fc3ed111b3dd401e0ffc41105770d)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d988196471e2b2cb345737faf1c615b = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_41973f04ffa9023b1a58910603bb4ea6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a72bb108204b989fa17e2672e39e2a1 = $(`<div id="html_5a72bb108204b989fa17e2672e39e2a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41973f04ffa9023b1a58910603bb4ea6.setContent(html_5a72bb108204b989fa17e2672e39e2a1);\\n \\n \\n\\n circle_marker_0d988196471e2b2cb345737faf1c615b.bindPopup(popup_41973f04ffa9023b1a58910603bb4ea6)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1059169132f8145607a439ca9c3670d = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_c297995d4c5c090e0089f17e22e77618 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28526f3a0a11b8fc4439d882eb7db000 = $(`<div id="html_28526f3a0a11b8fc4439d882eb7db000" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c297995d4c5c090e0089f17e22e77618.setContent(html_28526f3a0a11b8fc4439d882eb7db000);\\n \\n \\n\\n circle_marker_e1059169132f8145607a439ca9c3670d.bindPopup(popup_c297995d4c5c090e0089f17e22e77618)\\n ;\\n\\n \\n \\n \\n var circle_marker_f95a3ad0176f302ac6432a24cb0b91cc = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e469600af2bca7133d9aa31e8d54d569 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_413053c5965582bbdba1b285a9638fc1 = $(`<div id="html_413053c5965582bbdba1b285a9638fc1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e469600af2bca7133d9aa31e8d54d569.setContent(html_413053c5965582bbdba1b285a9638fc1);\\n \\n \\n\\n circle_marker_f95a3ad0176f302ac6432a24cb0b91cc.bindPopup(popup_e469600af2bca7133d9aa31e8d54d569)\\n ;\\n\\n \\n \\n \\n var circle_marker_56f0ff23cc31fafd5431edf5079caceb = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e3e73eeafca48c28cc5bd75145aae790 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8799e4fa912eb5225fe9270fdef3eff9 = $(`<div id="html_8799e4fa912eb5225fe9270fdef3eff9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3e73eeafca48c28cc5bd75145aae790.setContent(html_8799e4fa912eb5225fe9270fdef3eff9);\\n \\n \\n\\n circle_marker_56f0ff23cc31fafd5431edf5079caceb.bindPopup(popup_e3e73eeafca48c28cc5bd75145aae790)\\n ;\\n\\n \\n \\n \\n var circle_marker_901bc266a0262cb101e427b08ff6affc = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_cd9c3bfb758cf1b92bb77659aa3315ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_560d50118b5446e9ae5b058a8c75a75e = $(`<div id="html_560d50118b5446e9ae5b058a8c75a75e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd9c3bfb758cf1b92bb77659aa3315ed.setContent(html_560d50118b5446e9ae5b058a8c75a75e);\\n \\n \\n\\n circle_marker_901bc266a0262cb101e427b08ff6affc.bindPopup(popup_cd9c3bfb758cf1b92bb77659aa3315ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_05f9e4d0ac95c7c47c5e82a3fd90db96 = L.circleMarker(\\n [42.72732111198165, -73.24519140937427],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.352372348458314, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e1ae5e9ae95487eaabadb2a3549c3834 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_262431b6192e571c416222cd6a1a27d8 = $(`<div id="html_262431b6192e571c416222cd6a1a27d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1ae5e9ae95487eaabadb2a3549c3834.setContent(html_262431b6192e571c416222cd6a1a27d8);\\n \\n \\n\\n circle_marker_05f9e4d0ac95c7c47c5e82a3fd90db96.bindPopup(popup_e1ae5e9ae95487eaabadb2a3549c3834)\\n ;\\n\\n \\n \\n \\n var circle_marker_632b51321db423369967ec262b49dbfe = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_5e2614bfc93ef7840eb936c2f69d1d01 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e888dc3869fca5e7a2ae36f1efe65cb4 = $(`<div id="html_e888dc3869fca5e7a2ae36f1efe65cb4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e2614bfc93ef7840eb936c2f69d1d01.setContent(html_e888dc3869fca5e7a2ae36f1efe65cb4);\\n \\n \\n\\n circle_marker_632b51321db423369967ec262b49dbfe.bindPopup(popup_5e2614bfc93ef7840eb936c2f69d1d01)\\n ;\\n\\n \\n \\n \\n var circle_marker_14884f79f81a0e0f261516c3309718a0 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_feeaa4243d7c3eed88289416a308be86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d00c83bb0b2c65e8f0ffcdbe4d65c788 = $(`<div id="html_d00c83bb0b2c65e8f0ffcdbe4d65c788" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_feeaa4243d7c3eed88289416a308be86.setContent(html_d00c83bb0b2c65e8f0ffcdbe4d65c788);\\n \\n \\n\\n circle_marker_14884f79f81a0e0f261516c3309718a0.bindPopup(popup_feeaa4243d7c3eed88289416a308be86)\\n ;\\n\\n \\n \\n \\n var circle_marker_554cdbd244602a3e14720ca3f94b8071 = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7526f51a329a9f3420047726db1f660d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f0dcf196a22c088f7d64d12bef2e461 = $(`<div id="html_8f0dcf196a22c088f7d64d12bef2e461" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7526f51a329a9f3420047726db1f660d.setContent(html_8f0dcf196a22c088f7d64d12bef2e461);\\n \\n \\n\\n circle_marker_554cdbd244602a3e14720ca3f94b8071.bindPopup(popup_7526f51a329a9f3420047726db1f660d)\\n ;\\n\\n \\n \\n \\n var circle_marker_dcdeb69f6b70e5792e16c76762ddc34c = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a7501950c16b9793049f82a22432798d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c832060e53b6f174a17283fb17672daf = $(`<div id="html_c832060e53b6f174a17283fb17672daf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7501950c16b9793049f82a22432798d.setContent(html_c832060e53b6f174a17283fb17672daf);\\n \\n \\n\\n circle_marker_dcdeb69f6b70e5792e16c76762ddc34c.bindPopup(popup_a7501950c16b9793049f82a22432798d)\\n ;\\n\\n \\n \\n \\n var circle_marker_daccf5c41265b1093070bac6a9e914f0 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0dd6693987e5c9bfdedec89c143738ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6d20edab75a38b28afa00029f7c90bf = $(`<div id="html_f6d20edab75a38b28afa00029f7c90bf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0dd6693987e5c9bfdedec89c143738ae.setContent(html_f6d20edab75a38b28afa00029f7c90bf);\\n \\n \\n\\n circle_marker_daccf5c41265b1093070bac6a9e914f0.bindPopup(popup_0dd6693987e5c9bfdedec89c143738ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_9dc2aee2918900dc2393d5a10d34a904 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4e7fd994ae5a6e0476d5b62624bc595d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54f19a2918301340e5dd12c0dc5ff0e2 = $(`<div id="html_54f19a2918301340e5dd12c0dc5ff0e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e7fd994ae5a6e0476d5b62624bc595d.setContent(html_54f19a2918301340e5dd12c0dc5ff0e2);\\n \\n \\n\\n circle_marker_9dc2aee2918900dc2393d5a10d34a904.bindPopup(popup_4e7fd994ae5a6e0476d5b62624bc595d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4f1d38a2c3b5ca11d250d646ba0b47b = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_05bb4277d8f8938e09c6eedb2ae54161 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd70965d401cec8fd6d6f8278a3df1e5 = $(`<div id="html_bd70965d401cec8fd6d6f8278a3df1e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05bb4277d8f8938e09c6eedb2ae54161.setContent(html_bd70965d401cec8fd6d6f8278a3df1e5);\\n \\n \\n\\n circle_marker_c4f1d38a2c3b5ca11d250d646ba0b47b.bindPopup(popup_05bb4277d8f8938e09c6eedb2ae54161)\\n ;\\n\\n \\n \\n \\n var circle_marker_2aaf5e8eaa6b1b07d0a36aee7b32c357 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0f5807ef3281cacfb0ab3239acc79e29 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30ebe9f69393b5b569bd0963d304c0b5 = $(`<div id="html_30ebe9f69393b5b569bd0963d304c0b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f5807ef3281cacfb0ab3239acc79e29.setContent(html_30ebe9f69393b5b569bd0963d304c0b5);\\n \\n \\n\\n circle_marker_2aaf5e8eaa6b1b07d0a36aee7b32c357.bindPopup(popup_0f5807ef3281cacfb0ab3239acc79e29)\\n ;\\n\\n \\n \\n \\n var circle_marker_f675b7409cbec233084e744949fa80d1 = L.circleMarker(\\n [42.727317636451815, -73.23043109080618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d8ce32c3c4690c5022b1c9c08dd10a2a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ff3ad91149fe9cedc13256587451595 = $(`<div id="html_6ff3ad91149fe9cedc13256587451595" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8ce32c3c4690c5022b1c9c08dd10a2a.setContent(html_6ff3ad91149fe9cedc13256587451595);\\n \\n \\n\\n circle_marker_f675b7409cbec233084e744949fa80d1.bindPopup(popup_d8ce32c3c4690c5022b1c9c08dd10a2a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e5c0d1788657e0271a2b0933bd1a08c7 = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_db9e2dc9a7384c74f79c8f9d97076554 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b9fb34f19646b9c50ff97aa443c4fc4 = $(`<div id="html_0b9fb34f19646b9c50ff97aa443c4fc4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db9e2dc9a7384c74f79c8f9d97076554.setContent(html_0b9fb34f19646b9c50ff97aa443c4fc4);\\n \\n \\n\\n circle_marker_e5c0d1788657e0271a2b0933bd1a08c7.bindPopup(popup_db9e2dc9a7384c74f79c8f9d97076554)\\n ;\\n\\n \\n \\n \\n var circle_marker_283247109b75b92bff4c9230f4a149d9 = L.circleMarker(\\n [42.72731605666562, -73.2255109850401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2e8c9a5da2161a59fe0a85687021be73 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74ee1a9c37484f3d050250676dfaab9e = $(`<div id="html_74ee1a9c37484f3d050250676dfaab9e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e8c9a5da2161a59fe0a85687021be73.setContent(html_74ee1a9c37484f3d050250676dfaab9e);\\n \\n \\n\\n circle_marker_283247109b75b92bff4c9230f4a149d9.bindPopup(popup_2e8c9a5da2161a59fe0a85687021be73)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d32ce68a45387dc8da24706eb62e1f1 = L.circleMarker(\\n [42.72731562880686, -73.22428095863906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.478115991081385, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_73c410f924ce97ebc0b1f0cadd299c76 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e007c209ca188fa6520bc3d81416b40f = $(`<div id="html_e007c209ca188fa6520bc3d81416b40f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73c410f924ce97ebc0b1f0cadd299c76.setContent(html_e007c209ca188fa6520bc3d81416b40f);\\n \\n \\n\\n circle_marker_8d32ce68a45387dc8da24706eb62e1f1.bindPopup(popup_73c410f924ce97ebc0b1f0cadd299c76)\\n ;\\n\\n \\n \\n \\n var circle_marker_24ac96d3ceaabda5a501d167e6cdd6c6 = L.circleMarker(\\n [42.72731518778323, -73.22305093225523],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_1e0cf9b5067eb1c312356a43d80b80df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb3caf9155594456cecc70f9157ba4ba = $(`<div id="html_fb3caf9155594456cecc70f9157ba4ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1e0cf9b5067eb1c312356a43d80b80df.setContent(html_fb3caf9155594456cecc70f9157ba4ba);\\n \\n \\n\\n circle_marker_24ac96d3ceaabda5a501d167e6cdd6c6.bindPopup(popup_1e0cf9b5067eb1c312356a43d80b80df)\\n ;\\n\\n \\n \\n \\n var circle_marker_bd288c9cb7e0db76fe1883af852f27d6 = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e32b2c884b929a541c14e5ec9eb90cf0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_723e325db05a061b19f14d0e8f70b320 = $(`<div id="html_723e325db05a061b19f14d0e8f70b320" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e32b2c884b929a541c14e5ec9eb90cf0.setContent(html_723e325db05a061b19f14d0e8f70b320);\\n \\n \\n\\n circle_marker_bd288c9cb7e0db76fe1883af852f27d6.bindPopup(popup_e32b2c884b929a541c14e5ec9eb90cf0)\\n ;\\n\\n \\n \\n \\n var circle_marker_ff567d0a9017b82a8f6146995973db64 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4c33917236bd7b3cad0bc5bcf218cce8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd6eda3f811044683ab43366462edadb = $(`<div id="html_fd6eda3f811044683ab43366462edadb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c33917236bd7b3cad0bc5bcf218cce8.setContent(html_fd6eda3f811044683ab43366462edadb);\\n \\n \\n\\n circle_marker_ff567d0a9017b82a8f6146995973db64.bindPopup(popup_4c33917236bd7b3cad0bc5bcf218cce8)\\n ;\\n\\n \\n \\n \\n var circle_marker_5502e6c91940ea15806a1018938a3f95 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d6b11fc2464be5f49e05448cbed692f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_188c768d8ec48e92ee6e9191bddad31d = $(`<div id="html_188c768d8ec48e92ee6e9191bddad31d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d6b11fc2464be5f49e05448cbed692f0.setContent(html_188c768d8ec48e92ee6e9191bddad31d);\\n \\n \\n\\n circle_marker_5502e6c91940ea15806a1018938a3f95.bindPopup(popup_d6b11fc2464be5f49e05448cbed692f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_45c801786f48f5b9b553c3f36a55872e = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_ae2bfb32e5ddea03d5b8cd12b6f18fc4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64c6ac71c8eadc70df7eb4ea8fd71f54 = $(`<div id="html_64c6ac71c8eadc70df7eb4ea8fd71f54" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ae2bfb32e5ddea03d5b8cd12b6f18fc4.setContent(html_64c6ac71c8eadc70df7eb4ea8fd71f54);\\n \\n \\n\\n circle_marker_45c801786f48f5b9b553c3f36a55872e.bindPopup(popup_ae2bfb32e5ddea03d5b8cd12b6f18fc4)\\n ;\\n\\n \\n \\n \\n var circle_marker_1aca782374fce0a9a8aa5d594de4e27c = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_a6ab8aff51a69641bc2225f75691398f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_108615ccbe67c1ee0cd098463478f36a = $(`<div id="html_108615ccbe67c1ee0cd098463478f36a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a6ab8aff51a69641bc2225f75691398f.setContent(html_108615ccbe67c1ee0cd098463478f36a);\\n \\n \\n\\n circle_marker_1aca782374fce0a9a8aa5d594de4e27c.bindPopup(popup_a6ab8aff51a69641bc2225f75691398f)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1958c0ca4375b13959dfead55c06740 = L.circleMarker(\\n [42.72551566371306, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_707ac7cfdab1d65ca583d6973de08a61 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_705df9813142dc426ca290c208c2ec28 = $(`<div id="html_705df9813142dc426ca290c208c2ec28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_707ac7cfdab1d65ca583d6973de08a61.setContent(html_705df9813142dc426ca290c208c2ec28);\\n \\n \\n\\n circle_marker_d1958c0ca4375b13959dfead55c06740.bindPopup(popup_707ac7cfdab1d65ca583d6973de08a61)\\n ;\\n\\n \\n \\n \\n var circle_marker_66ba314283a6bd6fd806ad2a9b27184d = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_743afdb82bd2e8d64855348648530d93 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a68d7498f3d163349776806c324ecf6 = $(`<div id="html_4a68d7498f3d163349776806c324ecf6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_743afdb82bd2e8d64855348648530d93.setContent(html_4a68d7498f3d163349776806c324ecf6);\\n \\n \\n\\n circle_marker_66ba314283a6bd6fd806ad2a9b27184d.bindPopup(popup_743afdb82bd2e8d64855348648530d93)\\n ;\\n\\n \\n \\n \\n var circle_marker_38f18056963f625842fc33aa0adc926e = L.circleMarker(\\n [42.72551563738435, -73.26241185416787],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_8d5d649b9166e52395590f72baf43984 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9bb8bab81b035e75f343902dabbd1ad1 = $(`<div id="html_9bb8bab81b035e75f343902dabbd1ad1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8d5d649b9166e52395590f72baf43984.setContent(html_9bb8bab81b035e75f343902dabbd1ad1);\\n \\n \\n\\n circle_marker_38f18056963f625842fc33aa0adc926e.bindPopup(popup_8d5d649b9166e52395590f72baf43984)\\n ;\\n\\n \\n \\n \\n var circle_marker_20b89cdef515a2287eee2965c6461995 = L.circleMarker(\\n [42.72551560447347, -73.26118186332953],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_e0da2b1a441f33de8bba2e54977c35c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f84eb0bd75f26bfcbc46ecfb16a598c1 = $(`<div id="html_f84eb0bd75f26bfcbc46ecfb16a598c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0da2b1a441f33de8bba2e54977c35c7.setContent(html_f84eb0bd75f26bfcbc46ecfb16a598c1);\\n \\n \\n\\n circle_marker_20b89cdef515a2287eee2965c6461995.bindPopup(popup_e0da2b1a441f33de8bba2e54977c35c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_e97a7138052b715ae544040159c0b97e = L.circleMarker(\\n [42.72551555839823, -73.25995187249272],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b72dca0ac37a6285a817838c7c073ead = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dcf91abe0b8bcb73e25c04ab0e93241b = $(`<div id="html_dcf91abe0b8bcb73e25c04ab0e93241b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b72dca0ac37a6285a817838c7c073ead.setContent(html_dcf91abe0b8bcb73e25c04ab0e93241b);\\n \\n \\n\\n circle_marker_e97a7138052b715ae544040159c0b97e.bindPopup(popup_b72dca0ac37a6285a817838c7c073ead)\\n ;\\n\\n \\n \\n \\n var circle_marker_9439880812b9d65f0196ca6539e6964a = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4f6e12c750d9ec422f098dbdfa6e1ccb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fab690d67c9c9df8f404f770e1b96e26 = $(`<div id="html_fab690d67c9c9df8f404f770e1b96e26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f6e12c750d9ec422f098dbdfa6e1ccb.setContent(html_fab690d67c9c9df8f404f770e1b96e26);\\n \\n \\n\\n circle_marker_9439880812b9d65f0196ca6539e6964a.bindPopup(popup_4f6e12c750d9ec422f098dbdfa6e1ccb)\\n ;\\n\\n \\n \\n \\n var circle_marker_472398fb542633ff79aca4d099138d44 = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_4871824c245a7c744b504e91d20acb83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_22beea30975ce812d24f698e96796c79 = $(`<div id="html_22beea30975ce812d24f698e96796c79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4871824c245a7c744b504e91d20acb83.setContent(html_22beea30975ce812d24f698e96796c79);\\n \\n \\n\\n circle_marker_472398fb542633ff79aca4d099138d44.bindPopup(popup_4871824c245a7c744b504e91d20acb83)\\n ;\\n\\n \\n \\n \\n var circle_marker_272d5b8e7c4e45cffb071a5986f6dc8c = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_0b246b4f2f3d6d2080bff36311fc2dda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d1d25f412f2d95ba4bf7f2ae6a1e6e45 = $(`<div id="html_d1d25f412f2d95ba4bf7f2ae6a1e6e45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b246b4f2f3d6d2080bff36311fc2dda.setContent(html_d1d25f412f2d95ba4bf7f2ae6a1e6e45);\\n \\n \\n\\n circle_marker_272d5b8e7c4e45cffb071a5986f6dc8c.bindPopup(popup_0b246b4f2f3d6d2080bff36311fc2dda)\\n ;\\n\\n \\n \\n \\n var circle_marker_f488482dba254332a231ff889b51e1e2 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_be3d32ce434df4b929f13e7154f3d8b1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f091740f2ca7b627ec99669aa4794e2b = $(`<div id="html_f091740f2ca7b627ec99669aa4794e2b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be3d32ce434df4b929f13e7154f3d8b1.setContent(html_f091740f2ca7b627ec99669aa4794e2b);\\n \\n \\n\\n circle_marker_f488482dba254332a231ff889b51e1e2.bindPopup(popup_be3d32ce434df4b929f13e7154f3d8b1)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec38d3dbcfa592bd686c7efa41431f53 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_95f6e124a1f4fce682bf577a75f6988f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8a142080b20a6628fff3809b934bffe = $(`<div id="html_f8a142080b20a6628fff3809b934bffe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95f6e124a1f4fce682bf577a75f6988f.setContent(html_f8a142080b20a6628fff3809b934bffe);\\n \\n \\n\\n circle_marker_ec38d3dbcfa592bd686c7efa41431f53.bindPopup(popup_95f6e124a1f4fce682bf577a75f6988f)\\n ;\\n\\n \\n \\n \\n var circle_marker_055b64f9cf2465f36533e406aced9c4e = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2a581993fe9659bec434e32602a2d212 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5c28f795523936ed87a59a389ea6303 = $(`<div id="html_a5c28f795523936ed87a59a389ea6303" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2a581993fe9659bec434e32602a2d212.setContent(html_a5c28f795523936ed87a59a389ea6303);\\n \\n \\n\\n circle_marker_055b64f9cf2465f36533e406aced9c4e.bindPopup(popup_2a581993fe9659bec434e32602a2d212)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ad05fa067e41cd495f53fb95b8d232c = L.circleMarker(\\n [42.725513531087856, -73.24273200123443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2329dbbd932a42cdac70f61ea78d2b85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf90c8b878e4143ddfafa67ca4406b4b = $(`<div id="html_cf90c8b878e4143ddfafa67ca4406b4b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2329dbbd932a42cdac70f61ea78d2b85.setContent(html_cf90c8b878e4143ddfafa67ca4406b4b);\\n \\n \\n\\n circle_marker_9ad05fa067e41cd495f53fb95b8d232c.bindPopup(popup_2329dbbd932a42cdac70f61ea78d2b85)\\n ;\\n\\n \\n \\n \\n var circle_marker_189c588ec9b7cb79e5b469e83a61179c = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.442433223290478, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6a98a1b98595741b7059a1828c5da0f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3cf25acd3497eb27174c9229895f80e4 = $(`<div id="html_3cf25acd3497eb27174c9229895f80e4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a98a1b98595741b7059a1828c5da0f5.setContent(html_3cf25acd3497eb27174c9229895f80e4);\\n \\n \\n\\n circle_marker_189c588ec9b7cb79e5b469e83a61179c.bindPopup(popup_6a98a1b98595741b7059a1828c5da0f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_426278a1cf104c1c86e5083e5496306b = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.918490759365935, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_67f6683f2ac4b871a6fca38e25fecd9a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d6a5c48c0c9282cccb7369f51d31597 = $(`<div id="html_3d6a5c48c0c9282cccb7369f51d31597" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_67f6683f2ac4b871a6fca38e25fecd9a.setContent(html_3d6a5c48c0c9282cccb7369f51d31597);\\n \\n \\n\\n circle_marker_426278a1cf104c1c86e5083e5496306b.bindPopup(popup_67f6683f2ac4b871a6fca38e25fecd9a)\\n ;\\n\\n \\n \\n \\n var circle_marker_2eae36bf51f6676fefd43d017d46fca3 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_61f79f59e1876fcc3fc8ef3843a13202 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_948973f1098528e1bd8f1307dfd19a6b = $(`<div id="html_948973f1098528e1bd8f1307dfd19a6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61f79f59e1876fcc3fc8ef3843a13202.setContent(html_948973f1098528e1bd8f1307dfd19a6b);\\n \\n \\n\\n circle_marker_2eae36bf51f6676fefd43d017d46fca3.bindPopup(popup_61f79f59e1876fcc3fc8ef3843a13202)\\n ;\\n\\n \\n \\n \\n var circle_marker_acf281117dc647c1261ae891401fd7cc = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.949327084834294, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_5f95bf3e3e305e47e2473728bce3a432 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e8fb787731721faf37edacaf451680ab = $(`<div id="html_e8fb787731721faf37edacaf451680ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f95bf3e3e305e47e2473728bce3a432.setContent(html_e8fb787731721faf37edacaf451680ab);\\n \\n \\n\\n circle_marker_acf281117dc647c1261ae891401fd7cc.bindPopup(popup_5f95bf3e3e305e47e2473728bce3a432)\\n ;\\n\\n \\n \\n \\n var circle_marker_41563e9a8b5fd5690a10404d6f79d73f = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_bc2dce8dc8d26970b1c51f7378fefc4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e1c45336ba9b6116d6e95f0f3a011fba = $(`<div id="html_e1c45336ba9b6116d6e95f0f3a011fba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bc2dce8dc8d26970b1c51f7378fefc4e.setContent(html_e1c45336ba9b6116d6e95f0f3a011fba);\\n \\n \\n\\n circle_marker_41563e9a8b5fd5690a10404d6f79d73f.bindPopup(popup_bc2dce8dc8d26970b1c51f7378fefc4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d7dc0084a90c41111270177077b26c84 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6256f0f1f6d6ea568db7e2d22091bd53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7cda6f4a9293e2eeee56901871be6e67 = $(`<div id="html_7cda6f4a9293e2eeee56901871be6e67" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6256f0f1f6d6ea568db7e2d22091bd53.setContent(html_7cda6f4a9293e2eeee56901871be6e67);\\n \\n \\n\\n circle_marker_d7dc0084a90c41111270177077b26c84.bindPopup(popup_6256f0f1f6d6ea568db7e2d22091bd53)\\n ;\\n\\n \\n \\n \\n var circle_marker_ef0078cdcc15a987e9fed7e533513368 = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_dcd14ee863cea792024bf58c4ac34c3b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_29f866e98c8a9f5039f00b3f49d9dbb8 = $(`<div id="html_29f866e98c8a9f5039f00b3f49d9dbb8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dcd14ee863cea792024bf58c4ac34c3b.setContent(html_29f866e98c8a9f5039f00b3f49d9dbb8);\\n \\n \\n\\n circle_marker_ef0078cdcc15a987e9fed7e533513368.bindPopup(popup_dcd14ee863cea792024bf58c4ac34c3b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1e751b9a79d9bf43503d773e15a022e = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_81406885029f38cf5b3bcdeaf39946b6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86244853b7de658f5b8e2cb8fde8237b = $(`<div id="html_86244853b7de658f5b8e2cb8fde8237b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81406885029f38cf5b3bcdeaf39946b6.setContent(html_86244853b7de658f5b8e2cb8fde8237b);\\n \\n \\n\\n circle_marker_e1e751b9a79d9bf43503d773e15a022e.bindPopup(popup_81406885029f38cf5b3bcdeaf39946b6)\\n ;\\n\\n \\n \\n \\n var circle_marker_02e4352e73c70a0db5dc0457f7dcc338 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_f271ae60475ac30859231af71949116b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba3f85f41f327dcbb792c18f50f9ec9e = $(`<div id="html_ba3f85f41f327dcbb792c18f50f9ec9e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f271ae60475ac30859231af71949116b.setContent(html_ba3f85f41f327dcbb792c18f50f9ec9e);\\n \\n \\n\\n circle_marker_02e4352e73c70a0db5dc0457f7dcc338.bindPopup(popup_f271ae60475ac30859231af71949116b)\\n ;\\n\\n \\n \\n \\n var circle_marker_b40353465f03b95fb845960059e1c4d5 = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.753945931439391, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_464f26a87a6016ffac8002d2409ea3ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aef05fedced3a0f36ad5067723598736 = $(`<div id="html_aef05fedced3a0f36ad5067723598736" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_464f26a87a6016ffac8002d2409ea3ba.setContent(html_aef05fedced3a0f36ad5067723598736);\\n \\n \\n\\n circle_marker_b40353465f03b95fb845960059e1c4d5.bindPopup(popup_464f26a87a6016ffac8002d2409ea3ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_084f1c100364ea4334cda94649484468 = L.circleMarker(\\n [42.72370842502844, -73.2599520158284],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_b35e99c29200c0a569a4f6a75dcdd9b8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_224d9c0bb38c53ae380fed4a69a5607e = $(`<div id="html_224d9c0bb38c53ae380fed4a69a5607e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b35e99c29200c0a569a4f6a75dcdd9b8.setContent(html_224d9c0bb38c53ae380fed4a69a5607e);\\n \\n \\n\\n circle_marker_084f1c100364ea4334cda94649484468.bindPopup(popup_b35e99c29200c0a569a4f6a75dcdd9b8)\\n ;\\n\\n \\n \\n \\n var circle_marker_03761a1100c4741ad802edce6c38f55d = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_7044dda13465c70c5363d0c82e0abd43 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aae484fd55c91c87a361660086153a4d = $(`<div id="html_aae484fd55c91c87a361660086153a4d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7044dda13465c70c5363d0c82e0abd43.setContent(html_aae484fd55c91c87a361660086153a4d);\\n \\n \\n\\n circle_marker_03761a1100c4741ad802edce6c38f55d.bindPopup(popup_7044dda13465c70c5363d0c82e0abd43)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b1577521b871c7334acf409de72cf22 = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fb2417d123c24ed502b412e05a66284a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f3c0dfa201c3de7f645a10246d76c9b = $(`<div id="html_5f3c0dfa201c3de7f645a10246d76c9b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb2417d123c24ed502b412e05a66284a.setContent(html_5f3c0dfa201c3de7f645a10246d76c9b);\\n \\n \\n\\n circle_marker_9b1577521b871c7334acf409de72cf22.bindPopup(popup_fb2417d123c24ed502b412e05a66284a)\\n ;\\n\\n \\n \\n \\n var circle_marker_bec98203db2b658c04e21cf5993a432a = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_07bdd1cba61e440eb6c2d93d0f8787a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ecc1784278f6306a96a9c2e234745d66 = $(`<div id="html_ecc1784278f6306a96a9c2e234745d66" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07bdd1cba61e440eb6c2d93d0f8787a9.setContent(html_ecc1784278f6306a96a9c2e234745d66);\\n \\n \\n\\n circle_marker_bec98203db2b658c04e21cf5993a432a.bindPopup(popup_07bdd1cba61e440eb6c2d93d0f8787a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d56940d3bbc4d8841d18e9c8eead1ef = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_fc9aa8fd89f737202882aaa9a571f5ea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7cba3f222e36423c06aa81626cc81fd4 = $(`<div id="html_7cba3f222e36423c06aa81626cc81fd4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc9aa8fd89f737202882aaa9a571f5ea.setContent(html_7cba3f222e36423c06aa81626cc81fd4);\\n \\n \\n\\n circle_marker_9d56940d3bbc4d8841d18e9c8eead1ef.bindPopup(popup_fc9aa8fd89f737202882aaa9a571f5ea)\\n ;\\n\\n \\n \\n \\n var circle_marker_b253223999c3e0839d29836585f0436e = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_d6eda37093f309f4c25f8929955d68f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_35882c884803bdd572caaf8c8f27c52e = $(`<div id="html_35882c884803bdd572caaf8c8f27c52e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d6eda37093f309f4c25f8929955d68f8.setContent(html_35882c884803bdd572caaf8c8f27c52e);\\n \\n \\n\\n circle_marker_b253223999c3e0839d29836585f0436e.bindPopup(popup_d6eda37093f309f4c25f8929955d68f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_66b020fbfb4863c3f9a5cad2c566cd74 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.902761462589227, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_07092fef371c27dccbe3769d74854e12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c54478feb4ff64a42174fd5296f2caab = $(`<div id="html_c54478feb4ff64a42174fd5296f2caab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07092fef371c27dccbe3769d74854e12.setContent(html_c54478feb4ff64a42174fd5296f2caab);\\n \\n \\n\\n circle_marker_66b020fbfb4863c3f9a5cad2c566cd74.bindPopup(popup_07092fef371c27dccbe3769d74854e12)\\n ;\\n\\n \\n \\n \\n var circle_marker_24392b92862b3ea04b0bac9d3dcbb797 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_2f9a8fe87f8f7ab2ee4b712ee4bed155 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c95f6b06dd48455b0ba8b45bb3233f7 = $(`<div id="html_8c95f6b06dd48455b0ba8b45bb3233f7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2f9a8fe87f8f7ab2ee4b712ee4bed155.setContent(html_8c95f6b06dd48455b0ba8b45bb3233f7);\\n \\n \\n\\n circle_marker_24392b92862b3ea04b0bac9d3dcbb797.bindPopup(popup_2f9a8fe87f8f7ab2ee4b712ee4bed155)\\n ;\\n\\n \\n \\n \\n var circle_marker_ef7f689578530ec212e32e518a8ee3a6 = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_6df292abb117d5ef1735338728969423 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b26160826906205ef20b004467b7f1fa = $(`<div id="html_b26160826906205ef20b004467b7f1fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6df292abb117d5ef1735338728969423.setContent(html_b26160826906205ef20b004467b7f1fa);\\n \\n \\n\\n circle_marker_ef7f689578530ec212e32e518a8ee3a6.bindPopup(popup_6df292abb117d5ef1735338728969423)\\n ;\\n\\n \\n \\n \\n var circle_marker_44ecf55196c855ec5799f86189e3b99f = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_91ef6e2ae17f39e5ddd0f1fa75ca7963);\\n \\n \\n var popup_bf4685d7aedf5d8abe1f5c9b80dc10b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19b45733aebd9ff6ad9553c8114e3fe4 = $(`<div id="html_19b45733aebd9ff6ad9553c8114e3fe4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bf4685d7aedf5d8abe1f5c9b80dc10b5.setContent(html_19b45733aebd9ff6ad9553c8114e3fe4);\\n \\n \\n\\n circle_marker_44ecf55196c855ec5799f86189e3b99f.bindPopup(popup_bf4685d7aedf5d8abe1f5c9b80dc10b5)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Musclewood": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_c963e198e2acbcda29cc40578aaa7d46 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_c963e198e2acbcda29cc40578aaa7d46" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_c963e198e2acbcda29cc40578aaa7d46 = L.map(\\n "map_c963e198e2acbcda29cc40578aaa7d46",\\n {\\n center: [42.73183698027237, -73.23596452701021],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_ca3d402681a774157c6560b1a5055134 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var circle_marker_d91d763616056a025cb0d4777e98c15a = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_c6c2dd09cd2d39a4658cbdb7f9ff60b1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0ea1321bcb304826601cc9b96ea43a3 = $(`<div id="html_c0ea1321bcb304826601cc9b96ea43a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c6c2dd09cd2d39a4658cbdb7f9ff60b1.setContent(html_c0ea1321bcb304826601cc9b96ea43a3);\\n \\n \\n\\n circle_marker_d91d763616056a025cb0d4777e98c15a.bindPopup(popup_c6c2dd09cd2d39a4658cbdb7f9ff60b1)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d24c91781f0fad5c6b686e2d298324a = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_1a76c9bdd81b8bc7921ef4357b62fa87 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_147bb66c7d3a18d80fb416e7262c350d = $(`<div id="html_147bb66c7d3a18d80fb416e7262c350d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1a76c9bdd81b8bc7921ef4357b62fa87.setContent(html_147bb66c7d3a18d80fb416e7262c350d);\\n \\n \\n\\n circle_marker_8d24c91781f0fad5c6b686e2d298324a.bindPopup(popup_1a76c9bdd81b8bc7921ef4357b62fa87)\\n ;\\n\\n \\n \\n \\n var circle_marker_81786b552f99daa59061008cef8ffb56 = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_3054ac5f696fe4371748771128662d0c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94416713b5af443aef92aeeffed90ffd = $(`<div id="html_94416713b5af443aef92aeeffed90ffd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3054ac5f696fe4371748771128662d0c.setContent(html_94416713b5af443aef92aeeffed90ffd);\\n \\n \\n\\n circle_marker_81786b552f99daa59061008cef8ffb56.bindPopup(popup_3054ac5f696fe4371748771128662d0c)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8c526c26401470c4849587907e58e11 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_f5d7df6c74ca77f8b8d1ad03bcb9c006 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fed763da713c1743c96c73581b68b6a7 = $(`<div id="html_fed763da713c1743c96c73581b68b6a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5d7df6c74ca77f8b8d1ad03bcb9c006.setContent(html_fed763da713c1743c96c73581b68b6a7);\\n \\n \\n\\n circle_marker_c8c526c26401470c4849587907e58e11.bindPopup(popup_f5d7df6c74ca77f8b8d1ad03bcb9c006)\\n ;\\n\\n \\n \\n \\n var circle_marker_6093bba146bb2b0bcb0ad83d59695f30 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_ee7785f1ba381a31a09b7b00a5822a8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_77c87205be001c1e48aad3730854512c = $(`<div id="html_77c87205be001c1e48aad3730854512c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee7785f1ba381a31a09b7b00a5822a8d.setContent(html_77c87205be001c1e48aad3730854512c);\\n \\n \\n\\n circle_marker_6093bba146bb2b0bcb0ad83d59695f30.bindPopup(popup_ee7785f1ba381a31a09b7b00a5822a8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_728f585f7d99b73cbe734c8c505653fb = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_16f391111e6e54301a4b764e9d59a20f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e66b1023b1cc8a7c1e3cdf36937fce5a = $(`<div id="html_e66b1023b1cc8a7c1e3cdf36937fce5a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16f391111e6e54301a4b764e9d59a20f.setContent(html_e66b1023b1cc8a7c1e3cdf36937fce5a);\\n \\n \\n\\n circle_marker_728f585f7d99b73cbe734c8c505653fb.bindPopup(popup_16f391111e6e54301a4b764e9d59a20f)\\n ;\\n\\n \\n \\n \\n var circle_marker_dcdb28b3bfd42b6eaa3536a691d3c62f = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_b2fec0e2e96c578904dcca04b19a3a70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ddbc06d13460ac9d14ce5902edf86884 = $(`<div id="html_ddbc06d13460ac9d14ce5902edf86884" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2fec0e2e96c578904dcca04b19a3a70.setContent(html_ddbc06d13460ac9d14ce5902edf86884);\\n \\n \\n\\n circle_marker_dcdb28b3bfd42b6eaa3536a691d3c62f.bindPopup(popup_b2fec0e2e96c578904dcca04b19a3a70)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d02d46cd67b0b16944d94845e9391f5 = L.circleMarker(\\n [42.73815658377651, -73.21935289518422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_8f1ccef83b817d4c790be989a14adcb9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4dac81c4fc31925978c51a00de5e007b = $(`<div id="html_4dac81c4fc31925978c51a00de5e007b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f1ccef83b817d4c790be989a14adcb9.setContent(html_4dac81c4fc31925978c51a00de5e007b);\\n \\n \\n\\n circle_marker_2d02d46cd67b0b16944d94845e9391f5.bindPopup(popup_8f1ccef83b817d4c790be989a14adcb9)\\n ;\\n\\n \\n \\n \\n var circle_marker_df96375439768c5914c6d7b9457ca81b = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_371d3751353960a997d61d2838dd57f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2bcd09ca66f61205015beb0a113f3be = $(`<div id="html_b2bcd09ca66f61205015beb0a113f3be" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_371d3751353960a997d61d2838dd57f8.setContent(html_b2bcd09ca66f61205015beb0a113f3be);\\n \\n \\n\\n circle_marker_df96375439768c5914c6d7b9457ca81b.bindPopup(popup_371d3751353960a997d61d2838dd57f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_446d746308833dfaf35eb8880e1c18ba = L.circleMarker(\\n [42.73635039883137, -73.2218146329227],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_54569c92634d6e39556ad799721b38ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_337894ca0ad69f25291889dd98e1e01a = $(`<div id="html_337894ca0ad69f25291889dd98e1e01a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54569c92634d6e39556ad799721b38ab.setContent(html_337894ca0ad69f25291889dd98e1e01a);\\n \\n \\n\\n circle_marker_446d746308833dfaf35eb8880e1c18ba.bindPopup(popup_54569c92634d6e39556ad799721b38ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_b233516ee61496990c456c3db289cedd = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_f42b18a1e7ab9f051c5d2292e5cc1c92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26a33d0a95e935bcebc12710d3f72879 = $(`<div id="html_26a33d0a95e935bcebc12710d3f72879" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f42b18a1e7ab9f051c5d2292e5cc1c92.setContent(html_26a33d0a95e935bcebc12710d3f72879);\\n \\n \\n\\n circle_marker_b233516ee61496990c456c3db289cedd.bindPopup(popup_f42b18a1e7ab9f051c5d2292e5cc1c92)\\n ;\\n\\n \\n \\n \\n var circle_marker_9955bb475780c4f4259b191181f2331f = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_824259a08b2fbd9f6bba30730558543e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8be542f555840921194f6bfc73f9a31e = $(`<div id="html_8be542f555840921194f6bfc73f9a31e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_824259a08b2fbd9f6bba30730558543e.setContent(html_8be542f555840921194f6bfc73f9a31e);\\n \\n \\n\\n circle_marker_9955bb475780c4f4259b191181f2331f.bindPopup(popup_824259a08b2fbd9f6bba30730558543e)\\n ;\\n\\n \\n \\n \\n var circle_marker_47be430a516ffc5be6c1748ca3e89c84 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_e105370d2e56ae54cd87d6ee5f897d8a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b09569ac66ab34d01ada674228871591 = $(`<div id="html_b09569ac66ab34d01ada674228871591" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e105370d2e56ae54cd87d6ee5f897d8a.setContent(html_b09569ac66ab34d01ada674228871591);\\n \\n \\n\\n circle_marker_47be430a516ffc5be6c1748ca3e89c84.bindPopup(popup_e105370d2e56ae54cd87d6ee5f897d8a)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ea977f1392541faf5cf3aec470f2668 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_f6457131c90f8aefadf4176e3c94e134 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4bbf9103e38cb9fbc35bce0f76446b1c = $(`<div id="html_4bbf9103e38cb9fbc35bce0f76446b1c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f6457131c90f8aefadf4176e3c94e134.setContent(html_4bbf9103e38cb9fbc35bce0f76446b1c);\\n \\n \\n\\n circle_marker_3ea977f1392541faf5cf3aec470f2668.bindPopup(popup_f6457131c90f8aefadf4176e3c94e134)\\n ;\\n\\n \\n \\n \\n var circle_marker_b8bbfe79a088eb18f47757ed22c00a02 = L.circleMarker(\\n [42.73454326578408, -73.22181588771933],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.296739856991561, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_b5d1eb566156e6c79b80c236eacad5e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8dd5ac81cc4892fcb94a23a6cfe0db2f = $(`<div id="html_8dd5ac81cc4892fcb94a23a6cfe0db2f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5d1eb566156e6c79b80c236eacad5e2.setContent(html_8dd5ac81cc4892fcb94a23a6cfe0db2f);\\n \\n \\n\\n circle_marker_b8bbfe79a088eb18f47757ed22c00a02.bindPopup(popup_b5d1eb566156e6c79b80c236eacad5e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_a13cddd00d196d573bfcefb5c7ac2bae = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_4c7460532be2c4ae223ea85a02f6cdde = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ecd0ae9f9df261a1eb5a8f4be91d943 = $(`<div id="html_0ecd0ae9f9df261a1eb5a8f4be91d943" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c7460532be2c4ae223ea85a02f6cdde.setContent(html_0ecd0ae9f9df261a1eb5a8f4be91d943);\\n \\n \\n\\n circle_marker_a13cddd00d196d573bfcefb5c7ac2bae.bindPopup(popup_4c7460532be2c4ae223ea85a02f6cdde)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba374020db3442bf5a14a572e78d326e = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_0855824dda47fb252c170890dc1d2b45 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9e817cd0440724bd773a92fc78f362f9 = $(`<div id="html_9e817cd0440724bd773a92fc78f362f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0855824dda47fb252c170890dc1d2b45.setContent(html_9e817cd0440724bd773a92fc78f362f9);\\n \\n \\n\\n circle_marker_ba374020db3442bf5a14a572e78d326e.bindPopup(popup_0855824dda47fb252c170890dc1d2b45)\\n ;\\n\\n \\n \\n \\n var circle_marker_dada2a1308a9d4cfbff34817488b8fe3 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_6b532f135843895db7f269a42fc264d0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_274c0f84c95716cd8098a098eafb0f0b = $(`<div id="html_274c0f84c95716cd8098a098eafb0f0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b532f135843895db7f269a42fc264d0.setContent(html_274c0f84c95716cd8098a098eafb0f0b);\\n \\n \\n\\n circle_marker_dada2a1308a9d4cfbff34817488b8fe3.bindPopup(popup_6b532f135843895db7f269a42fc264d0)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec02958aeed410dfe8e366683d4fc22e = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_fd64a91c78c2b9acbcadf03da1645bf4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94a70cfbd31fe25b494fe07dc9e4d219 = $(`<div id="html_94a70cfbd31fe25b494fe07dc9e4d219" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd64a91c78c2b9acbcadf03da1645bf4.setContent(html_94a70cfbd31fe25b494fe07dc9e4d219);\\n \\n \\n\\n circle_marker_ec02958aeed410dfe8e366683d4fc22e.bindPopup(popup_fd64a91c78c2b9acbcadf03da1645bf4)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e2d24ff0c96eca198b58fad9c92d0a1 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_859f84e2de74ea38153b2e95e92fc0e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_494e523e243d6439e13e81bea2b95948 = $(`<div id="html_494e523e243d6439e13e81bea2b95948" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_859f84e2de74ea38153b2e95e92fc0e1.setContent(html_494e523e243d6439e13e81bea2b95948);\\n \\n \\n\\n circle_marker_9e2d24ff0c96eca198b58fad9c92d0a1.bindPopup(popup_859f84e2de74ea38153b2e95e92fc0e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_5532a7fc950980babfeaad2e572f00bc = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_29241e981a85c789777c6fba1168162c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e19077aa3578094107092dea2571bbf6 = $(`<div id="html_e19077aa3578094107092dea2571bbf6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_29241e981a85c789777c6fba1168162c.setContent(html_e19077aa3578094107092dea2571bbf6);\\n \\n \\n\\n circle_marker_5532a7fc950980babfeaad2e572f00bc.bindPopup(popup_29241e981a85c789777c6fba1168162c)\\n ;\\n\\n \\n \\n \\n var circle_marker_142a325b4b2d2387b73afeecc75e8a37 = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_15faeccc2057966c54711effee50f957 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_164c5ccba112965e2b1a7d3d1bba5731 = $(`<div id="html_164c5ccba112965e2b1a7d3d1bba5731" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_15faeccc2057966c54711effee50f957.setContent(html_164c5ccba112965e2b1a7d3d1bba5731);\\n \\n \\n\\n circle_marker_142a325b4b2d2387b73afeecc75e8a37.bindPopup(popup_15faeccc2057966c54711effee50f957)\\n ;\\n\\n \\n \\n \\n var circle_marker_912bd5e9491c5a1149eb02f792746e56 = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_689019cbf538e0b88061fe96cdc634e6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b1409d87e35162315bb82336d2f81d3 = $(`<div id="html_7b1409d87e35162315bb82336d2f81d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_689019cbf538e0b88061fe96cdc634e6.setContent(html_7b1409d87e35162315bb82336d2f81d3);\\n \\n \\n\\n circle_marker_912bd5e9491c5a1149eb02f792746e56.bindPopup(popup_689019cbf538e0b88061fe96cdc634e6)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b7b85086f0f844fe3115e4f5d1c1125 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_a609b6041ba86f6395d5508fbf73efd7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5bd905a3bf8e0675a1b230d82c4b65f0 = $(`<div id="html_5bd905a3bf8e0675a1b230d82c4b65f0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a609b6041ba86f6395d5508fbf73efd7.setContent(html_5bd905a3bf8e0675a1b230d82c4b65f0);\\n \\n \\n\\n circle_marker_5b7b85086f0f844fe3115e4f5d1c1125.bindPopup(popup_a609b6041ba86f6395d5508fbf73efd7)\\n ;\\n\\n \\n \\n \\n var circle_marker_4015e55c529d0b3c9f4df5cdf50eaf65 = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_5830d3847c019118355f9eac4a471ba6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_79c37e4b1c638efde0fef2c7ee06e742 = $(`<div id="html_79c37e4b1c638efde0fef2c7ee06e742" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5830d3847c019118355f9eac4a471ba6.setContent(html_79c37e4b1c638efde0fef2c7ee06e742);\\n \\n \\n\\n circle_marker_4015e55c529d0b3c9f4df5cdf50eaf65.bindPopup(popup_5830d3847c019118355f9eac4a471ba6)\\n ;\\n\\n \\n \\n \\n var circle_marker_619c3f21f789c6896817035a893dced4 = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_779f083fd22ab81db2685243a62afeea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0b2ac33b30aaa23584f53638feac91b = $(`<div id="html_b0b2ac33b30aaa23584f53638feac91b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_779f083fd22ab81db2685243a62afeea.setContent(html_b0b2ac33b30aaa23584f53638feac91b);\\n \\n \\n\\n circle_marker_619c3f21f789c6896817035a893dced4.bindPopup(popup_779f083fd22ab81db2685243a62afeea)\\n ;\\n\\n \\n \\n \\n var circle_marker_214f88f25e553322140361e422b03f48 = L.circleMarker(\\n [42.73092705112874, -73.21689800500221],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_8b9379f19383b82eb7d29096ce67bf6f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2a22570c53276f7c997c82498d2aa22 = $(`<div id="html_b2a22570c53276f7c997c82498d2aa22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b9379f19383b82eb7d29096ce67bf6f.setContent(html_b2a22570c53276f7c997c82498d2aa22);\\n \\n \\n\\n circle_marker_214f88f25e553322140361e422b03f48.bindPopup(popup_8b9379f19383b82eb7d29096ce67bf6f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e31bdd7ae4ac81e529c199fe44dd58b6 = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_8a7a49d30b8c77e2f7863eb5aac127f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7834ba37e3045030c2dcddf86ae2903c = $(`<div id="html_7834ba37e3045030c2dcddf86ae2903c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a7a49d30b8c77e2f7863eb5aac127f4.setContent(html_7834ba37e3045030c2dcddf86ae2903c);\\n \\n \\n\\n circle_marker_e31bdd7ae4ac81e529c199fe44dd58b6.bindPopup(popup_8a7a49d30b8c77e2f7863eb5aac127f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_750aee3fe29b2ca4295c27beff2b9ba2 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_d0ce01564caaabb3951f6f794935e3ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1a763dd72d69c675b6901c7376e0e698 = $(`<div id="html_1a763dd72d69c675b6901c7376e0e698" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d0ce01564caaabb3951f6f794935e3ef.setContent(html_1a763dd72d69c675b6901c7376e0e698);\\n \\n \\n\\n circle_marker_750aee3fe29b2ca4295c27beff2b9ba2.bindPopup(popup_d0ce01564caaabb3951f6f794935e3ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_93d44d34209a04aebc8a71f750f78c54 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_44ee1415cf9b2a9a542b4e79827cdb3c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3a20c9d48424b7035c4b5b338216e510 = $(`<div id="html_3a20c9d48424b7035c4b5b338216e510" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_44ee1415cf9b2a9a542b4e79827cdb3c.setContent(html_3a20c9d48424b7035c4b5b338216e510);\\n \\n \\n\\n circle_marker_93d44d34209a04aebc8a71f750f78c54.bindPopup(popup_44ee1415cf9b2a9a542b4e79827cdb3c)\\n ;\\n\\n \\n \\n \\n var circle_marker_ae98ee0245242db22a4b835666dbc370 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_c0854a1e962f9ecfe8db3e636036bfa7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b6620e3a9f66cb9ce721b5a32a888ba4 = $(`<div id="html_b6620e3a9f66cb9ce721b5a32a888ba4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c0854a1e962f9ecfe8db3e636036bfa7.setContent(html_b6620e3a9f66cb9ce721b5a32a888ba4);\\n \\n \\n\\n circle_marker_ae98ee0245242db22a4b835666dbc370.bindPopup(popup_c0854a1e962f9ecfe8db3e636036bfa7)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0727770be5f10f683668ad5fb460115 = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_65da53b2b3a49aea929f10e885c299c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55d2577e2a1e3effd2c412afe2172947 = $(`<div id="html_55d2577e2a1e3effd2c412afe2172947" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65da53b2b3a49aea929f10e885c299c2.setContent(html_55d2577e2a1e3effd2c412afe2172947);\\n \\n \\n\\n circle_marker_c0727770be5f10f683668ad5fb460115.bindPopup(popup_65da53b2b3a49aea929f10e885c299c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e732eef263e213838aaa0211708a0a9 = L.circleMarker(\\n [42.72912439440237, -73.22920002498961],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_9b36d44724c0c9f7afee4a5cc7421a62 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e93657e9336ecf76575cb0a796791176 = $(`<div id="html_e93657e9336ecf76575cb0a796791176" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b36d44724c0c9f7afee4a5cc7421a62.setContent(html_e93657e9336ecf76575cb0a796791176);\\n \\n \\n\\n circle_marker_8e732eef263e213838aaa0211708a0a9.bindPopup(popup_9b36d44724c0c9f7afee4a5cc7421a62)\\n ;\\n\\n \\n \\n \\n var circle_marker_12afbdcf096c21ed825f3397f4bbf500 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.7846987830302403, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_11c539fc2fb4a74e9d1edb6de2068659 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_108bebe812d0d5910ec65ee953a15ba9 = $(`<div id="html_108bebe812d0d5910ec65ee953a15ba9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_11c539fc2fb4a74e9d1edb6de2068659.setContent(html_108bebe812d0d5910ec65ee953a15ba9);\\n \\n \\n\\n circle_marker_12afbdcf096c21ed825f3397f4bbf500.bindPopup(popup_11c539fc2fb4a74e9d1edb6de2068659)\\n ;\\n\\n \\n \\n \\n var circle_marker_00aad0174be2c9b1808e89cbcdbe4fc4 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_ecd61399d4f26530372cbcf820934443 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3867f981b83da65b96a210b3e1b96ed6 = $(`<div id="html_3867f981b83da65b96a210b3e1b96ed6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ecd61399d4f26530372cbcf820934443.setContent(html_3867f981b83da65b96a210b3e1b96ed6);\\n \\n \\n\\n circle_marker_00aad0174be2c9b1808e89cbcdbe4fc4.bindPopup(popup_ecd61399d4f26530372cbcf820934443)\\n ;\\n\\n \\n \\n \\n var circle_marker_166a135eeeab121c90adbb013aa967fd = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_f2551b1806351dd7c9a64aa882a03d33 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e40dd006db91f426ab274e22b9e05f5d = $(`<div id="html_e40dd006db91f426ab274e22b9e05f5d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2551b1806351dd7c9a64aa882a03d33.setContent(html_e40dd006db91f426ab274e22b9e05f5d);\\n \\n \\n\\n circle_marker_166a135eeeab121c90adbb013aa967fd.bindPopup(popup_f2551b1806351dd7c9a64aa882a03d33)\\n ;\\n\\n \\n \\n \\n var circle_marker_61b9a49b1fa3086abd58d817d022b611 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_e6d6fdd0f9c7ee9f6f5f76b813de3880 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2619d0f787e56bf119a7cfefaf6cb599 = $(`<div id="html_2619d0f787e56bf119a7cfefaf6cb599" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6d6fdd0f9c7ee9f6f5f76b813de3880.setContent(html_2619d0f787e56bf119a7cfefaf6cb599);\\n \\n \\n\\n circle_marker_61b9a49b1fa3086abd58d817d022b611.bindPopup(popup_e6d6fdd0f9c7ee9f6f5f76b813de3880)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f3b3d9810d9d1196b7b60c3137a84a9 = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_53d4a46d171c7328638f1724bcc95f53 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_11b5ce55ac58a3ed1fd69756f27dcc11 = $(`<div id="html_11b5ce55ac58a3ed1fd69756f27dcc11" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_53d4a46d171c7328638f1724bcc95f53.setContent(html_11b5ce55ac58a3ed1fd69756f27dcc11);\\n \\n \\n\\n circle_marker_8f3b3d9810d9d1196b7b60c3137a84a9.bindPopup(popup_53d4a46d171c7328638f1724bcc95f53)\\n ;\\n\\n \\n \\n \\n var circle_marker_961bb3e005e0381696a37ec649e08e19 = L.circleMarker(\\n [42.72732066437552, -73.24273135617167],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_4e65949753d817abf4064f6857886e0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b665455846be463dd7995573887a312 = $(`<div id="html_6b665455846be463dd7995573887a312" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e65949753d817abf4064f6857886e0d.setContent(html_6b665455846be463dd7995573887a312);\\n \\n \\n\\n circle_marker_961bb3e005e0381696a37ec649e08e19.bindPopup(popup_4e65949753d817abf4064f6857886e0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8b8b8cbd24880e52819ed8d5fcbac70 = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_cb63668f6831f1ebbad56102bdea5d27 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b2e49a1027e1696b23c61be4ce16bcd = $(`<div id="html_5b2e49a1027e1696b23c61be4ce16bcd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb63668f6831f1ebbad56102bdea5d27.setContent(html_5b2e49a1027e1696b23c61be4ce16bcd);\\n \\n \\n\\n circle_marker_c8b8b8cbd24880e52819ed8d5fcbac70.bindPopup(popup_cb63668f6831f1ebbad56102bdea5d27)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb907078e3bb60c6eff242e01d770dc9 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_dd57d54d9ed60ad3d612625eb5c308ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24c4e9701bfadc440733b731e76d2ef9 = $(`<div id="html_24c4e9701bfadc440733b731e76d2ef9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd57d54d9ed60ad3d612625eb5c308ca.setContent(html_24c4e9701bfadc440733b731e76d2ef9);\\n \\n \\n\\n circle_marker_fb907078e3bb60c6eff242e01d770dc9.bindPopup(popup_dd57d54d9ed60ad3d612625eb5c308ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b91bee12a0e67a128f76d2b6067e05c = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_f9c31d5662adf4b1d4c5edf71d8a843f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_062e5039e19a53268129e31ad28c2a56 = $(`<div id="html_062e5039e19a53268129e31ad28c2a56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9c31d5662adf4b1d4c5edf71d8a843f.setContent(html_062e5039e19a53268129e31ad28c2a56);\\n \\n \\n\\n circle_marker_4b91bee12a0e67a128f76d2b6067e05c.bindPopup(popup_f9c31d5662adf4b1d4c5edf71d8a843f)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7228b1ab8e8677fb7024e43d30aa3e3 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_77b7ccafa373aa43607d16e20e23387c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_468c0c00e4b8bf0ad929bacb60ad47e8 = $(`<div id="html_468c0c00e4b8bf0ad929bacb60ad47e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_77b7ccafa373aa43607d16e20e23387c.setContent(html_468c0c00e4b8bf0ad929bacb60ad47e8);\\n \\n \\n\\n circle_marker_e7228b1ab8e8677fb7024e43d30aa3e3.bindPopup(popup_77b7ccafa373aa43607d16e20e23387c)\\n ;\\n\\n \\n \\n \\n var circle_marker_56f4f950bbea1b1125caf02c2c9afbe7 = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_d13f638d86fff27cc6aecd8d064692ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c448913e2ee61282b4c18d6cb3636855 = $(`<div id="html_c448913e2ee61282b4c18d6cb3636855" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d13f638d86fff27cc6aecd8d064692ab.setContent(html_c448913e2ee61282b4c18d6cb3636855);\\n \\n \\n\\n circle_marker_56f4f950bbea1b1125caf02c2c9afbe7.bindPopup(popup_d13f638d86fff27cc6aecd8d064692ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_f749b2972d126649bb8fd962a302652a = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_7495451b61c92a0e512227f556bb0cad = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d36523264b7ad030e98bcba610203916 = $(`<div id="html_d36523264b7ad030e98bcba610203916" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7495451b61c92a0e512227f556bb0cad.setContent(html_d36523264b7ad030e98bcba610203916);\\n \\n \\n\\n circle_marker_f749b2972d126649bb8fd962a302652a.bindPopup(popup_7495451b61c92a0e512227f556bb0cad)\\n ;\\n\\n \\n \\n \\n var circle_marker_5629f2e4f1bfd923da02e502b3da2461 = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_fcbec588e2ef0daf67d9e3c91c37cb5d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f730c68b0eb5d84fa56e2897a8827940 = $(`<div id="html_f730c68b0eb5d84fa56e2897a8827940" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fcbec588e2ef0daf67d9e3c91c37cb5d.setContent(html_f730c68b0eb5d84fa56e2897a8827940);\\n \\n \\n\\n circle_marker_5629f2e4f1bfd923da02e502b3da2461.bindPopup(popup_fcbec588e2ef0daf67d9e3c91c37cb5d)\\n ;\\n\\n \\n \\n \\n var circle_marker_803d750291462d739ae94319b64efc09 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_a12fec868bdab640b077f48df90c1f25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_082223ffc971e2532939e551a8a437f9 = $(`<div id="html_082223ffc971e2532939e551a8a437f9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a12fec868bdab640b077f48df90c1f25.setContent(html_082223ffc971e2532939e551a8a437f9);\\n \\n \\n\\n circle_marker_803d750291462d739ae94319b64efc09.bindPopup(popup_a12fec868bdab640b077f48df90c1f25)\\n ;\\n\\n \\n \\n \\n var circle_marker_f14da262ed88c45f15a18d69066671e5 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_c86087ca47bd80421eee3c63b707dbee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2e1dcb32c55f3e27b578360ccfd4912 = $(`<div id="html_f2e1dcb32c55f3e27b578360ccfd4912" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c86087ca47bd80421eee3c63b707dbee.setContent(html_f2e1dcb32c55f3e27b578360ccfd4912);\\n \\n \\n\\n circle_marker_f14da262ed88c45f15a18d69066671e5.bindPopup(popup_c86087ca47bd80421eee3c63b707dbee)\\n ;\\n\\n \\n \\n \\n var circle_marker_dcb216ad6febc91261f85da5d46084ed = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_efe213902a0b4b896d9d3dcc3a8a98a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e31248c4c189c63fbcd2e02f31e1a0d3 = $(`<div id="html_e31248c4c189c63fbcd2e02f31e1a0d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_efe213902a0b4b896d9d3dcc3a8a98a3.setContent(html_e31248c4c189c63fbcd2e02f31e1a0d3);\\n \\n \\n\\n circle_marker_dcb216ad6febc91261f85da5d46084ed.bindPopup(popup_efe213902a0b4b896d9d3dcc3a8a98a3)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba5134b8b9e3fe80e609a99e8c575df2 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_b67dd4988cf4b91891d07ff0e45ab7ac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc5178f8c09355ff019017ff3e897c1d = $(`<div id="html_cc5178f8c09355ff019017ff3e897c1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b67dd4988cf4b91891d07ff0e45ab7ac.setContent(html_cc5178f8c09355ff019017ff3e897c1d);\\n \\n \\n\\n circle_marker_ba5134b8b9e3fe80e609a99e8c575df2.bindPopup(popup_b67dd4988cf4b91891d07ff0e45ab7ac)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9e2df2e5902d17c8d102c7c8567240c = L.circleMarker(\\n [42.72551247793964, -73.23781203829343],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_c1461f60609047c1429b397c81470bbe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c7ea9acbc7caded3d5e61fb4c7027e17 = $(`<div id="html_c7ea9acbc7caded3d5e61fb4c7027e17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c1461f60609047c1429b397c81470bbe.setContent(html_c7ea9acbc7caded3d5e61fb4c7027e17);\\n \\n \\n\\n circle_marker_a9e2df2e5902d17c8d102c7c8567240c.bindPopup(popup_c1461f60609047c1429b397c81470bbe)\\n ;\\n\\n \\n \\n \\n var circle_marker_1afce7855b3602dadb8bfab5d7d27f86 = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_b2ba82c1f19353e46ca7c7a27a8acc16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9bb96c8d2a43dcd8722109dacf0a120c = $(`<div id="html_9bb96c8d2a43dcd8722109dacf0a120c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2ba82c1f19353e46ca7c7a27a8acc16.setContent(html_9bb96c8d2a43dcd8722109dacf0a120c);\\n \\n \\n\\n circle_marker_1afce7855b3602dadb8bfab5d7d27f86.bindPopup(popup_b2ba82c1f19353e46ca7c7a27a8acc16)\\n ;\\n\\n \\n \\n \\n var circle_marker_b7e6ce96cfc5c744ca57a200021a9989 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_17ad6551d560509d254618e1c252d227 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b3352de8e85e884a186c6471a760a27 = $(`<div id="html_0b3352de8e85e884a186c6471a760a27" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_17ad6551d560509d254618e1c252d227.setContent(html_0b3352de8e85e884a186c6471a760a27);\\n \\n \\n\\n circle_marker_b7e6ce96cfc5c744ca57a200021a9989.bindPopup(popup_17ad6551d560509d254618e1c252d227)\\n ;\\n\\n \\n \\n \\n var circle_marker_69659752cc34b1dd60cffa6f64645a41 = L.circleMarker(\\n [42.72551154985281, -73.23412206620647],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_3ed42f709e16859199ebc19903298640 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42aacd969cb015d7bd851630307dea1e = $(`<div id="html_42aacd969cb015d7bd851630307dea1e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ed42f709e16859199ebc19903298640.setContent(html_42aacd969cb015d7bd851630307dea1e);\\n \\n \\n\\n circle_marker_69659752cc34b1dd60cffa6f64645a41.bindPopup(popup_3ed42f709e16859199ebc19903298640)\\n ;\\n\\n \\n \\n \\n var circle_marker_b9291de772e5d7da4dcff15b6a5d7c87 = L.circleMarker(\\n [42.7255108653065, -73.23166208487956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_dd48e204b78425f8b8a2cee505ae25c0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a99238d0e1ad29363a1ced335eff9e47 = $(`<div id="html_a99238d0e1ad29363a1ced335eff9e47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd48e204b78425f8b8a2cee505ae25c0.setContent(html_a99238d0e1ad29363a1ced335eff9e47);\\n \\n \\n\\n circle_marker_b9291de772e5d7da4dcff15b6a5d7c87.bindPopup(popup_dd48e204b78425f8b8a2cee505ae25c0)\\n ;\\n\\n \\n \\n \\n var circle_marker_226dc1ae5bf32975fb4c7d64bd05a60f = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c963e198e2acbcda29cc40578aaa7d46);\\n \\n \\n var popup_98bf9dea14bd49c273e3e91cdba03252 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_88ba66736d3c0ada0733de8813101882 = $(`<div id="html_88ba66736d3c0ada0733de8813101882" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98bf9dea14bd49c273e3e91cdba03252.setContent(html_88ba66736d3c0ada0733de8813101882);\\n \\n \\n\\n circle_marker_226dc1ae5bf32975fb4c7d64bd05a60f.bindPopup(popup_98bf9dea14bd49c273e3e91cdba03252)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Nannyberry": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_8cd6950d46ea99ab717d1aa987bc877c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_8cd6950d46ea99ab717d1aa987bc877c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_8cd6950d46ea99ab717d1aa987bc877c = L.map(\\n "map_8cd6950d46ea99ab717d1aa987bc877c",\\n {\\n center: [42.731837727370745, -73.23719446217768],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_47289a4718a122f113975eee6a5edfea = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var circle_marker_2269c25ddd8af111560c0daf08573f67 = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var popup_2ee940a0d38b6408027a41ce0577d6e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9147e5584bea2a354df72832d60118c = $(`<div id="html_d9147e5584bea2a354df72832d60118c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ee940a0d38b6408027a41ce0577d6e9.setContent(html_d9147e5584bea2a354df72832d60118c);\\n \\n \\n\\n circle_marker_2269c25ddd8af111560c0daf08573f67.bindPopup(popup_2ee940a0d38b6408027a41ce0577d6e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_e23c7126f6716e436502d620810c114a = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var popup_3644355e9b14883651c79e590762283e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6bba29df8a25b47789c47265dad405e7 = $(`<div id="html_6bba29df8a25b47789c47265dad405e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3644355e9b14883651c79e590762283e.setContent(html_6bba29df8a25b47789c47265dad405e7);\\n \\n \\n\\n circle_marker_e23c7126f6716e436502d620810c114a.bindPopup(popup_3644355e9b14883651c79e590762283e)\\n ;\\n\\n \\n \\n \\n var circle_marker_d52df27d8fbe2db7e921a085626bdc4d = L.circleMarker(\\n [42.73454814416453, -73.23780809560456],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var popup_16f065cfe505aaf156218797bafc45f7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad7bf87085b5903fe04b47032f19276d = $(`<div id="html_ad7bf87085b5903fe04b47032f19276d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16f065cfe505aaf156218797bafc45f7.setContent(html_ad7bf87085b5903fe04b47032f19276d);\\n \\n \\n\\n circle_marker_d52df27d8fbe2db7e921a085626bdc4d.bindPopup(popup_16f065cfe505aaf156218797bafc45f7)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a9b381400341138b7154831d6640da4 = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var popup_b3c4160400c996339264eb508c4b306d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43b307fe20db3e5cd1ab155b9b02fdd9 = $(`<div id="html_43b307fe20db3e5cd1ab155b9b02fdd9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3c4160400c996339264eb508c4b306d.setContent(html_43b307fe20db3e5cd1ab155b9b02fdd9);\\n \\n \\n\\n circle_marker_6a9b381400341138b7154831d6640da4.bindPopup(popup_b3c4160400c996339264eb508c4b306d)\\n ;\\n\\n \\n \\n \\n var circle_marker_9696c4fb1c884e5a332735694607aa1c = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var popup_dfbb4a65faf858de26ca4b06f3f64a86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55c04ccfbf1deaac591b18c4378d8820 = $(`<div id="html_55c04ccfbf1deaac591b18c4378d8820" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dfbb4a65faf858de26ca4b06f3f64a86.setContent(html_55c04ccfbf1deaac591b18c4378d8820);\\n \\n \\n\\n circle_marker_9696c4fb1c884e5a332735694607aa1c.bindPopup(popup_dfbb4a65faf858de26ca4b06f3f64a86)\\n ;\\n\\n \\n \\n \\n var circle_marker_25906b93367dc3a41d1bbaf5256573c8 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_8cd6950d46ea99ab717d1aa987bc877c);\\n \\n \\n var popup_cf9c29c9f20a921b8fd575b16fbfe818 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f7c6c5b45d1ef00fe0cb41cdebcb7305 = $(`<div id="html_f7c6c5b45d1ef00fe0cb41cdebcb7305" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf9c29c9f20a921b8fd575b16fbfe818.setContent(html_f7c6c5b45d1ef00fe0cb41cdebcb7305);\\n \\n \\n\\n circle_marker_25906b93367dc3a41d1bbaf5256573c8.bindPopup(popup_cf9c29c9f20a921b8fd575b16fbfe818)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Oak, black\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_fcf78228cc1211d269d1230c940d9635 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_fcf78228cc1211d269d1230c940d9635" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_fcf78228cc1211d269d1230c940d9635 = L.map(\\n "map_fcf78228cc1211d269d1230c940d9635",\\n {\\n center: [42.73635805253136, -73.24949193359234],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_b15d86267534a646d5480f7904bd0069 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_fcf78228cc1211d269d1230c940d9635);\\n \\n \\n var circle_marker_4fd0255d2da1e442006d61876ce40a51 = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_fcf78228cc1211d269d1230c940d9635);\\n \\n \\n var popup_df3ca7ac8244746ea266dc8cba290d85 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ae8108e8abd653b317204dc43a10c54 = $(`<div id="html_4ae8108e8abd653b317204dc43a10c54" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df3ca7ac8244746ea266dc8cba290d85.setContent(html_4ae8108e8abd653b317204dc43a10c54);\\n \\n \\n\\n circle_marker_4fd0255d2da1e442006d61876ce40a51.bindPopup(popup_df3ca7ac8244746ea266dc8cba290d85)\\n ;\\n\\n \\n \\n \\n var circle_marker_2261e75362fd89bd26a64af6705fb6fc = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_fcf78228cc1211d269d1230c940d9635);\\n \\n \\n var popup_644c55042b969ae585374bacc4c6d017 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_04ae765f48b853212c534c612ca623fa = $(`<div id="html_04ae765f48b853212c534c612ca623fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_644c55042b969ae585374bacc4c6d017.setContent(html_04ae765f48b853212c534c612ca623fa);\\n \\n \\n\\n circle_marker_2261e75362fd89bd26a64af6705fb6fc.bindPopup(popup_644c55042b969ae585374bacc4c6d017)\\n ;\\n\\n \\n \\n \\n var circle_marker_66693277184185bf29cb7fe0083e6ede = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_fcf78228cc1211d269d1230c940d9635);\\n \\n \\n var popup_63cdfab57770b07e19d55972a63ca9e2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_73dc925bc0188b07393ee2e634c23f28 = $(`<div id="html_73dc925bc0188b07393ee2e634c23f28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63cdfab57770b07e19d55972a63ca9e2.setContent(html_73dc925bc0188b07393ee2e634c23f28);\\n \\n \\n\\n circle_marker_66693277184185bf29cb7fe0083e6ede.bindPopup(popup_63cdfab57770b07e19d55972a63ca9e2)\\n ;\\n\\n \\n \\n \\n var circle_marker_97aff6da20cc622aa6eb7e7d301ec163 = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_fcf78228cc1211d269d1230c940d9635);\\n \\n \\n var popup_39da630da4d97953d9908f700aec77ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e9e2225fe2f65acac164abb85abb390 = $(`<div id="html_8e9e2225fe2f65acac164abb85abb390" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39da630da4d97953d9908f700aec77ec.setContent(html_8e9e2225fe2f65acac164abb85abb390);\\n \\n \\n\\n circle_marker_97aff6da20cc622aa6eb7e7d301ec163.bindPopup(popup_39da630da4d97953d9908f700aec77ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_47430eb0ecf435d76d209955e55e3a62 = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_fcf78228cc1211d269d1230c940d9635);\\n \\n \\n var popup_0032f266a9346e618a69557e1ef758ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1facb6bc880b14064caf623cab51e083 = $(`<div id="html_1facb6bc880b14064caf623cab51e083" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0032f266a9346e618a69557e1ef758ae.setContent(html_1facb6bc880b14064caf623cab51e083);\\n \\n \\n\\n circle_marker_47430eb0ecf435d76d209955e55e3a62.bindPopup(popup_0032f266a9346e618a69557e1ef758ae)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Oak, red\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_32b180e8965d68b62502b147e1b13201 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_32b180e8965d68b62502b147e1b13201" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_32b180e8965d68b62502b147e1b13201 = L.map(\\n "map_32b180e8965d68b62502b147e1b13201",\\n {\\n center: [42.73545173466282, -73.24887665172076],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_d8525f267337fda57a57e225543f9c2f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var circle_marker_1c14f7c147ef4b6080feb562d0027bff = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7e41742f751cd954dc48ee8d1c24c0af = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b73e32d23ff164467f6ec29adff0ade = $(`<div id="html_3b73e32d23ff164467f6ec29adff0ade" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e41742f751cd954dc48ee8d1c24c0af.setContent(html_3b73e32d23ff164467f6ec29adff0ade);\\n \\n \\n\\n circle_marker_1c14f7c147ef4b6080feb562d0027bff.bindPopup(popup_7e41742f751cd954dc48ee8d1c24c0af)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e29649c6c4581f21fb891ad67562546 = L.circleMarker(\\n [42.74720060566399, -73.27717604649621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b13ddc444ddaf7a100e50e761b0a00a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3e6d36c9265a156b87837c202abd220e = $(`<div id="html_3e6d36c9265a156b87837c202abd220e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b13ddc444ddaf7a100e50e761b0a00a0.setContent(html_3e6d36c9265a156b87837c202abd220e);\\n \\n \\n\\n circle_marker_6e29649c6c4581f21fb891ad67562546.bindPopup(popup_b13ddc444ddaf7a100e50e761b0a00a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_6dfb18fbe52dae7b24a16da6f46fdac1 = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_fc179eb2607e65eb0bb1022485dbf5aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_650a2586f8e3ce27ccc8631b04f5db49 = $(`<div id="html_650a2586f8e3ce27ccc8631b04f5db49" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc179eb2607e65eb0bb1022485dbf5aa.setContent(html_650a2586f8e3ce27ccc8631b04f5db49);\\n \\n \\n\\n circle_marker_6dfb18fbe52dae7b24a16da6f46fdac1.bindPopup(popup_fc179eb2607e65eb0bb1022485dbf5aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_61fe6f8c47c8f24e66aaea38361a35b3 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9ff2f425d220909a823346687066ed4a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_823c08cc15040f5d72f4c6a5881854e8 = $(`<div id="html_823c08cc15040f5d72f4c6a5881854e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ff2f425d220909a823346687066ed4a.setContent(html_823c08cc15040f5d72f4c6a5881854e8);\\n \\n \\n\\n circle_marker_61fe6f8c47c8f24e66aaea38361a35b3.bindPopup(popup_9ff2f425d220909a823346687066ed4a)\\n ;\\n\\n \\n \\n \\n var circle_marker_be991d9a3453def17afe00e90dc4df01 = L.circleMarker(\\n [42.74720102712816, -73.27225436227008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_63b75daacbab06d90ac8cd9d9e519dfe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eafe5432c20b802ab54d828fabac60a9 = $(`<div id="html_eafe5432c20b802ab54d828fabac60a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63b75daacbab06d90ac8cd9d9e519dfe.setContent(html_eafe5432c20b802ab54d828fabac60a9);\\n \\n \\n\\n circle_marker_be991d9a3453def17afe00e90dc4df01.bindPopup(popup_63b75daacbab06d90ac8cd9d9e519dfe)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a1214a50488b92dfe184ee39c72c3db = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d2aa0ee800dacffec0316dfb236d2556 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c47675358fc3027f1dc911039663034 = $(`<div id="html_1c47675358fc3027f1dc911039663034" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2aa0ee800dacffec0316dfb236d2556.setContent(html_1c47675358fc3027f1dc911039663034);\\n \\n \\n\\n circle_marker_9a1214a50488b92dfe184ee39c72c3db.bindPopup(popup_d2aa0ee800dacffec0316dfb236d2556)\\n ;\\n\\n \\n \\n \\n var circle_marker_3d314fd01ee96944e7336b044327dc72 = L.circleMarker(\\n [42.74720115883572, -73.26979352013609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_358e3f159b971e72a59f259bfa13389b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dd796142a3f11df1821b9449271b23ab = $(`<div id="html_dd796142a3f11df1821b9449271b23ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_358e3f159b971e72a59f259bfa13389b.setContent(html_dd796142a3f11df1821b9449271b23ab);\\n \\n \\n\\n circle_marker_3d314fd01ee96944e7336b044327dc72.bindPopup(popup_358e3f159b971e72a59f259bfa13389b)\\n ;\\n\\n \\n \\n \\n var circle_marker_27910e50e695680ee8309c78ed728ed3 = L.circleMarker(\\n [42.747201204933376, -73.2685630990657],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_19d04bee62b65fc2ea606fcbfe1e49b3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e699d0326d4d468746e0123ed3f1f35 = $(`<div id="html_4e699d0326d4d468746e0123ed3f1f35" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19d04bee62b65fc2ea606fcbfe1e49b3.setContent(html_4e699d0326d4d468746e0123ed3f1f35);\\n \\n \\n\\n circle_marker_27910e50e695680ee8309c78ed728ed3.bindPopup(popup_19d04bee62b65fc2ea606fcbfe1e49b3)\\n ;\\n\\n \\n \\n \\n var circle_marker_54ec41f5ebac8e0dcc933d2561e43308 = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6b90333e8757db291df2e1e4b16a0462 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1d6eea1545cffc120706c0a5602e45c2 = $(`<div id="html_1d6eea1545cffc120706c0a5602e45c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b90333e8757db291df2e1e4b16a0462.setContent(html_1d6eea1545cffc120706c0a5602e45c2);\\n \\n \\n\\n circle_marker_54ec41f5ebac8e0dcc933d2561e43308.bindPopup(popup_6b90333e8757db291df2e1e4b16a0462)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd1a2ac85764138f5969a7779b9b0a14 = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_818000b4a32704620d6f0bc65b541b8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f49b514345b8243da2c6297250a12e6 = $(`<div id="html_4f49b514345b8243da2c6297250a12e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_818000b4a32704620d6f0bc65b541b8d.setContent(html_4f49b514345b8243da2c6297250a12e6);\\n \\n \\n\\n circle_marker_fd1a2ac85764138f5969a7779b9b0a14.bindPopup(popup_818000b4a32704620d6f0bc65b541b8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_7471139e9044c63e4cb2aa2cdcae7211 = L.circleMarker(\\n [42.74720126420177, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3dc7a505bab6f9498430924a006dd7fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ecac93e19ba51d7700c587f9527fd37 = $(`<div id="html_9ecac93e19ba51d7700c587f9527fd37" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3dc7a505bab6f9498430924a006dd7fc.setContent(html_9ecac93e19ba51d7700c587f9527fd37);\\n \\n \\n\\n circle_marker_7471139e9044c63e4cb2aa2cdcae7211.bindPopup(popup_3dc7a505bab6f9498430924a006dd7fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_372a35e85cc84a6de316774d2d5fe7d9 = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9edf5a34163dcf031e019025ed6124d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d5f2a8c4ff2d5748db4bb76a8805718 = $(`<div id="html_0d5f2a8c4ff2d5748db4bb76a8805718" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9edf5a34163dcf031e019025ed6124d3.setContent(html_0d5f2a8c4ff2d5748db4bb76a8805718);\\n \\n \\n\\n circle_marker_372a35e85cc84a6de316774d2d5fe7d9.bindPopup(popup_9edf5a34163dcf031e019025ed6124d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_667d24463c35985d819cd2a13d35dd79 = L.circleMarker(\\n [42.747201204933376, -73.26118057262872],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c762dfe4ea7dd8c3de2ad2ae6dd81deb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38bf835b9ec716d6ccf04917bba15c16 = $(`<div id="html_38bf835b9ec716d6ccf04917bba15c16" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c762dfe4ea7dd8c3de2ad2ae6dd81deb.setContent(html_38bf835b9ec716d6ccf04917bba15c16);\\n \\n \\n\\n circle_marker_667d24463c35985d819cd2a13d35dd79.bindPopup(popup_c762dfe4ea7dd8c3de2ad2ae6dd81deb)\\n ;\\n\\n \\n \\n \\n var circle_marker_caeeff6a2f771405fbd7e5fb598680c4 = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b2ac2f4aff92f599a6ad9c039552493e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_839ab6826eb800e4cd8d478ed5d6ef70 = $(`<div id="html_839ab6826eb800e4cd8d478ed5d6ef70" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2ac2f4aff92f599a6ad9c039552493e.setContent(html_839ab6826eb800e4cd8d478ed5d6ef70);\\n \\n \\n\\n circle_marker_caeeff6a2f771405fbd7e5fb598680c4.bindPopup(popup_b2ac2f4aff92f599a6ad9c039552493e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4f1f9e076b99efbdf535e86e228f1d3 = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d346a0d920dd869ef88a7cf6bc7c2ef1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc2ac79d95070e2f870e1af566a52b1c = $(`<div id="html_dc2ac79d95070e2f870e1af566a52b1c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d346a0d920dd869ef88a7cf6bc7c2ef1.setContent(html_dc2ac79d95070e2f870e1af566a52b1c);\\n \\n \\n\\n circle_marker_a4f1f9e076b99efbdf535e86e228f1d3.bindPopup(popup_d346a0d920dd869ef88a7cf6bc7c2ef1)\\n ;\\n\\n \\n \\n \\n var circle_marker_75ad50e10137f9410c856fc332d1e3f9 = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5fc13b04bd39dfea0cba9b096fc488b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70856e735fff2ccd20798dd1a731a40f = $(`<div id="html_70856e735fff2ccd20798dd1a731a40f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5fc13b04bd39dfea0cba9b096fc488b4.setContent(html_70856e735fff2ccd20798dd1a731a40f);\\n \\n \\n\\n circle_marker_75ad50e10137f9410c856fc332d1e3f9.bindPopup(popup_5fc13b04bd39dfea0cba9b096fc488b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_59aa2f0bef124480534ceabce7d43bfa = L.circleMarker(\\n [42.74539347231661, -73.2771756878084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_105f8b0c1a73a53d04036a22d643e1e8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8801af4e0820d426315ab4d3a5a8a33f = $(`<div id="html_8801af4e0820d426315ab4d3a5a8a33f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_105f8b0c1a73a53d04036a22d643e1e8.setContent(html_8801af4e0820d426315ab4d3a5a8a33f);\\n \\n \\n\\n circle_marker_59aa2f0bef124480534ceabce7d43bfa.bindPopup(popup_105f8b0c1a73a53d04036a22d643e1e8)\\n ;\\n\\n \\n \\n \\n var circle_marker_03332240b883de647ffabc65b4c819dc = L.circleMarker(\\n [42.74539389376372, -73.27225414705738],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_80113cc91e6f37c117f58f3dfcd26208 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_724a2810d74752f3c6236314bb7befda = $(`<div id="html_724a2810d74752f3c6236314bb7befda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_80113cc91e6f37c117f58f3dfcd26208.setContent(html_724a2810d74752f3c6236314bb7befda);\\n \\n \\n\\n circle_marker_03332240b883de647ffabc65b4c819dc.bindPopup(popup_80113cc91e6f37c117f58f3dfcd26208)\\n ;\\n\\n \\n \\n \\n var circle_marker_a99bc15ecb968d350746914dad7dd27b = L.circleMarker(\\n [42.745393966199934, -73.27102376186048],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f38217394fe11584fae02c2c798431dc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_380b93b723295ecaffd86e54b73349b1 = $(`<div id="html_380b93b723295ecaffd86e54b73349b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f38217394fe11584fae02c2c798431dc.setContent(html_380b93b723295ecaffd86e54b73349b1);\\n \\n \\n\\n circle_marker_a99bc15ecb968d350746914dad7dd27b.bindPopup(popup_f38217394fe11584fae02c2c798431dc)\\n ;\\n\\n \\n \\n \\n var circle_marker_98bc5782f35b371e159c58f9d46c9d69 = L.circleMarker(\\n [42.745394025465934, -73.26979337666096],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_003d5e012a8f336db1c24986fc99b5d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f34c2c8d4be112ecb0ae103d8ac93fc = $(`<div id="html_1f34c2c8d4be112ecb0ae103d8ac93fc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_003d5e012a8f336db1c24986fc99b5d1.setContent(html_1f34c2c8d4be112ecb0ae103d8ac93fc);\\n \\n \\n\\n circle_marker_98bc5782f35b371e159c58f9d46c9d69.bindPopup(popup_003d5e012a8f336db1c24986fc99b5d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1a6b1095937764037a07dc3bddad952 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_35a68d94b1a6b93689c15d10044e43bb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_83f23c87c5e600622d2f8fa3b7f53b2d = $(`<div id="html_83f23c87c5e600622d2f8fa3b7f53b2d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35a68d94b1a6b93689c15d10044e43bb.setContent(html_83f23c87c5e600622d2f8fa3b7f53b2d);\\n \\n \\n\\n circle_marker_e1a6b1095937764037a07dc3bddad952.bindPopup(popup_35a68d94b1a6b93689c15d10044e43bb)\\n ;\\n\\n \\n \\n \\n var circle_marker_76709d28d2d021125c571c8d543b4397 = L.circleMarker(\\n [42.74539412424261, -73.26364145064247],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b1742147c60292e4dabd555fbe642faf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f82607604a69ed5dd6b1cbe041f51ce1 = $(`<div id="html_f82607604a69ed5dd6b1cbe041f51ce1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1742147c60292e4dabd555fbe642faf.setContent(html_f82607604a69ed5dd6b1cbe041f51ce1);\\n \\n \\n\\n circle_marker_76709d28d2d021125c571c8d543b4397.bindPopup(popup_b1742147c60292e4dabd555fbe642faf)\\n ;\\n\\n \\n \\n \\n var circle_marker_75310dd1dea9c9b5efcd88291b070541 = L.circleMarker(\\n [42.74539407156172, -73.26118068023507],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3bef51a153bc58a852349082fe1a298a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f6a0d59cec9bec2823c1a7574b815b1b = $(`<div id="html_f6a0d59cec9bec2823c1a7574b815b1b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3bef51a153bc58a852349082fe1a298a.setContent(html_f6a0d59cec9bec2823c1a7574b815b1b);\\n \\n \\n\\n circle_marker_75310dd1dea9c9b5efcd88291b070541.bindPopup(popup_3bef51a153bc58a852349082fe1a298a)\\n ;\\n\\n \\n \\n \\n var circle_marker_8928ef8b83655e6f8d2d396b95605d48 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f523fca8c6a528f432564dca0e9b3cfc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6119507666078417761a53569adccca = $(`<div id="html_a6119507666078417761a53569adccca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f523fca8c6a528f432564dca0e9b3cfc.setContent(html_a6119507666078417761a53569adccca);\\n \\n \\n\\n circle_marker_8928ef8b83655e6f8d2d396b95605d48.bindPopup(popup_f523fca8c6a528f432564dca0e9b3cfc)\\n ;\\n\\n \\n \\n \\n var circle_marker_762cd7906a4e7ac0662f2652a1ed0ccc = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_86c874cd6ae7acc9becf34a0c7ccc417 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_30e45aa494906bdfda3e1996b83b8e01 = $(`<div id="html_30e45aa494906bdfda3e1996b83b8e01" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86c874cd6ae7acc9becf34a0c7ccc417.setContent(html_30e45aa494906bdfda3e1996b83b8e01);\\n \\n \\n\\n circle_marker_762cd7906a4e7ac0662f2652a1ed0ccc.bindPopup(popup_86c874cd6ae7acc9becf34a0c7ccc417)\\n ;\\n\\n \\n \\n \\n var circle_marker_5295a6edf0878b26382b17e682eed79c = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_43143e581fd070306cc970dc008c94bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_79d66ee6e97140c59538fc8353f5459b = $(`<div id="html_79d66ee6e97140c59538fc8353f5459b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43143e581fd070306cc970dc008c94bd.setContent(html_79d66ee6e97140c59538fc8353f5459b);\\n \\n \\n\\n circle_marker_5295a6edf0878b26382b17e682eed79c.bindPopup(popup_43143e581fd070306cc970dc008c94bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e3b70deb680fff65436fde93e291eaa = L.circleMarker(\\n [42.74358667479628, -73.27348428119001],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_fb68292d9d34f83aa0fbee2e4e648779 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8bb04d00370dc9ba62d06a63bae632a9 = $(`<div id="html_8bb04d00370dc9ba62d06a63bae632a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fb68292d9d34f83aa0fbee2e4e648779.setContent(html_8bb04d00370dc9ba62d06a63bae632a9);\\n \\n \\n\\n circle_marker_0e3b70deb680fff65436fde93e291eaa.bindPopup(popup_fb68292d9d34f83aa0fbee2e4e648779)\\n ;\\n\\n \\n \\n \\n var circle_marker_36d55875be7236a09a6d56bd953412a0 = L.circleMarker(\\n [42.74358683283254, -73.27102358253109],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_64a0b77f01aa4871138d624c3aa3dc46 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7529af112ea995ffc4c0337061c0a19f = $(`<div id="html_7529af112ea995ffc4c0337061c0a19f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64a0b77f01aa4871138d624c3aa3dc46.setContent(html_7529af112ea995ffc4c0337061c0a19f);\\n \\n \\n\\n circle_marker_36d55875be7236a09a6d56bd953412a0.bindPopup(popup_64a0b77f01aa4871138d624c3aa3dc46)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4774f8c142fee35705200855440049e = L.circleMarker(\\n [42.74358689209615, -73.26979323319746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_67b907cb69b5563fdbcaf7438f9cbb77 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3debc3425cc3224ee2d77e70f6dbedca = $(`<div id="html_3debc3425cc3224ee2d77e70f6dbedca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_67b907cb69b5563fdbcaf7438f9cbb77.setContent(html_3debc3425cc3224ee2d77e70f6dbedca);\\n \\n \\n\\n circle_marker_a4774f8c142fee35705200855440049e.bindPopup(popup_67b907cb69b5563fdbcaf7438f9cbb77)\\n ;\\n\\n \\n \\n \\n var circle_marker_15fb3b631fbdf943f0d9895c2b5d34b7 = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1f7be24b59933e61e604dce3af7ae15c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b0b5c5ac2864d3cb404846c44c0b63c = $(`<div id="html_3b0b5c5ac2864d3cb404846c44c0b63c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f7be24b59933e61e604dce3af7ae15c.setContent(html_3b0b5c5ac2864d3cb404846c44c0b63c);\\n \\n \\n\\n circle_marker_15fb3b631fbdf943f0d9895c2b5d34b7.bindPopup(popup_1f7be24b59933e61e604dce3af7ae15c)\\n ;\\n\\n \\n \\n \\n var circle_marker_d32e4873441a41dbb46efae1c459cc38 = L.circleMarker(\\n [42.74358699745365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_39547b134006c5092efdfbef4ebe5dc9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_418e1829f26b30527f837fdca19f2864 = $(`<div id="html_418e1829f26b30527f837fdca19f2864" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_39547b134006c5092efdfbef4ebe5dc9.setContent(html_418e1829f26b30527f837fdca19f2864);\\n \\n \\n\\n circle_marker_d32e4873441a41dbb46efae1c459cc38.bindPopup(popup_39547b134006c5092efdfbef4ebe5dc9)\\n ;\\n\\n \\n \\n \\n var circle_marker_55a3ce7ed3ce6af50e70055ae6d475df = L.circleMarker(\\n [42.74358699086881, -73.26364148650833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_feaf18a3526fc4495f2612cc90705666 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14bf86d3ad6a8f94ee90d4fcabd0a959 = $(`<div id="html_14bf86d3ad6a8f94ee90d4fcabd0a959" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_feaf18a3526fc4495f2612cc90705666.setContent(html_14bf86d3ad6a8f94ee90d4fcabd0a959);\\n \\n \\n\\n circle_marker_55a3ce7ed3ce6af50e70055ae6d475df.bindPopup(popup_feaf18a3526fc4495f2612cc90705666)\\n ;\\n\\n \\n \\n \\n var circle_marker_8d5c63ce0406f621a18b198b90c0292e = L.circleMarker(\\n [42.74358697111428, -73.26241113716999],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_65dcfbe7c63770a09240926610f94898 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf762fde534fc52091d65e0bd0c0d869 = $(`<div id="html_bf762fde534fc52091d65e0bd0c0d869" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65dcfbe7c63770a09240926610f94898.setContent(html_bf762fde534fc52091d65e0bd0c0d869);\\n \\n \\n\\n circle_marker_8d5c63ce0406f621a18b198b90c0292e.bindPopup(popup_65dcfbe7c63770a09240926610f94898)\\n ;\\n\\n \\n \\n \\n var circle_marker_bb8b13535f9642ff208384375064805d = L.circleMarker(\\n [42.743586938190056, -73.26118078783269],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6c84977f3fac81f19f56b31cf3a023f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ebfb9db2a42efc7561e88f3d98772b65 = $(`<div id="html_ebfb9db2a42efc7561e88f3d98772b65" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c84977f3fac81f19f56b31cf3a023f1.setContent(html_ebfb9db2a42efc7561e88f3d98772b65);\\n \\n \\n\\n circle_marker_bb8b13535f9642ff208384375064805d.bindPopup(popup_6c84977f3fac81f19f56b31cf3a023f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_205cd270e1ba9a71fe480c52f593b69a = L.circleMarker(\\n [42.74358689209615, -73.25995043849696],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8672b52cd4968484bcd97c8ac1b3c486 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e57e22354a4a23a0c89cb2467248955 = $(`<div id="html_6e57e22354a4a23a0c89cb2467248955" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8672b52cd4968484bcd97c8ac1b3c486.setContent(html_6e57e22354a4a23a0c89cb2467248955);\\n \\n \\n\\n circle_marker_205cd270e1ba9a71fe480c52f593b69a.bindPopup(popup_8672b52cd4968484bcd97c8ac1b3c486)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe629db3b4100c3ed9a5f0cf3ef72046 = L.circleMarker(\\n [42.74358657602363, -73.25502904118017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_510e317d227849e6dd06f015ffbbb9b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8dd6c8fabb0a593f092d2634aac95a83 = $(`<div id="html_8dd6c8fabb0a593f092d2634aac95a83" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_510e317d227849e6dd06f015ffbbb9b7.setContent(html_8dd6c8fabb0a593f092d2634aac95a83);\\n \\n \\n\\n circle_marker_fe629db3b4100c3ed9a5f0cf3ef72046.bindPopup(popup_510e317d227849e6dd06f015ffbbb9b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f73036eb03684206f97325727660234 = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e3c3be172b4412e3c18d4348f7e2d3d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e41bb9dd03763d62afcf531f0c6457d6 = $(`<div id="html_e41bb9dd03763d62afcf531f0c6457d6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3c3be172b4412e3c18d4348f7e2d3d5.setContent(html_e41bb9dd03763d62afcf531f0c6457d6);\\n \\n \\n\\n circle_marker_2f73036eb03684206f97325727660234.bindPopup(popup_e3c3be172b4412e3c18d4348f7e2d3d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ceb471896f8219a7de49b6033db1af2 = L.circleMarker(\\n [42.74177944266665, -73.2747143436105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ec9609c7339f023d71660d6b6c5f7a3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2694b2c9cf4c1d60e660c87181571c8a = $(`<div id="html_2694b2c9cf4c1d60e660c87181571c8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec9609c7339f023d71660d6b6c5f7a3e.setContent(html_2694b2c9cf4c1d60e660c87181571c8a);\\n \\n \\n\\n circle_marker_1ceb471896f8219a7de49b6033db1af2.bindPopup(popup_ec9609c7339f023d71660d6b6c5f7a3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_5fdf1cc01ce1c96eaedd39e8c201ecf9 = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7b619020bec5be5ff69edec7221ae56f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14cc2c095aba9d566436961c51f0ef85 = $(`<div id="html_14cc2c095aba9d566436961c51f0ef85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7b619020bec5be5ff69edec7221ae56f.setContent(html_14cc2c095aba9d566436961c51f0ef85);\\n \\n \\n\\n circle_marker_5fdf1cc01ce1c96eaedd39e8c201ecf9.bindPopup(popup_7b619020bec5be5ff69edec7221ae56f)\\n ;\\n\\n \\n \\n \\n var circle_marker_252a96663438eab5eda6cd19dad3e675 = L.circleMarker(\\n [42.74177985749502, -73.26364152237132],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_226d0c605be23f65b00205e9ee157e25 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e679928e5725cfe2ec77a09872640f5b = $(`<div id="html_e679928e5725cfe2ec77a09872640f5b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_226d0c605be23f65b00205e9ee157e25.setContent(html_e679928e5725cfe2ec77a09872640f5b);\\n \\n \\n\\n circle_marker_252a96663438eab5eda6cd19dad3e675.bindPopup(popup_226d0c605be23f65b00205e9ee157e25)\\n ;\\n\\n \\n \\n \\n var circle_marker_e34ec3537280c7b0b449f34bfcf911f9 = L.circleMarker(\\n [42.74177983774129, -73.26241120889593],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_feeb3295630b9b822dea240030e538c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_785409ab705608d711b4a3b8fa63e9fa = $(`<div id="html_785409ab705608d711b4a3b8fa63e9fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_feeb3295630b9b822dea240030e538c7.setContent(html_785409ab705608d711b4a3b8fa63e9fa);\\n \\n \\n\\n circle_marker_e34ec3537280c7b0b449f34bfcf911f9.bindPopup(popup_feeb3295630b9b822dea240030e538c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_3ced7b9f647dbf3a30775b4392973d3d = L.circleMarker(\\n [42.74177975872636, -73.25995058194884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f8fea3ba24aeaf971b693ad9082b1daf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_865ab5c03616611c0f413b256dd92538 = $(`<div id="html_865ab5c03616611c0f413b256dd92538" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f8fea3ba24aeaf971b693ad9082b1daf.setContent(html_865ab5c03616611c0f413b256dd92538);\\n \\n \\n\\n circle_marker_3ced7b9f647dbf3a30775b4392973d3d.bindPopup(popup_f8fea3ba24aeaf971b693ad9082b1daf)\\n ;\\n\\n \\n \\n \\n var circle_marker_8241848e5374e6240d4797c7f5647e06 = L.circleMarker(\\n [42.74177969946516, -73.25872026847817],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5ceb69433f01bd0f6e65163ea42968ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5bb09f74c55a54f3d9b53ebb24aedf9c = $(`<div id="html_5bb09f74c55a54f3d9b53ebb24aedf9c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ceb69433f01bd0f6e65163ea42968ff.setContent(html_5bb09f74c55a54f3d9b53ebb24aedf9c);\\n \\n \\n\\n circle_marker_8241848e5374e6240d4797c7f5647e06.bindPopup(popup_5ceb69433f01bd0f6e65163ea42968ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb7e829d7950682d1baaa5c5302f9ef2 = L.circleMarker(\\n [42.74177962703481, -73.25748995501012],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c07698c2ae163fe29e10ec96d54ca6a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_69498cde3a60b9fdcde6723e169af244 = $(`<div id="html_69498cde3a60b9fdcde6723e169af244" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c07698c2ae163fe29e10ec96d54ca6a2.setContent(html_69498cde3a60b9fdcde6723e169af244);\\n \\n \\n\\n circle_marker_cb7e829d7950682d1baaa5c5302f9ef2.bindPopup(popup_c07698c2ae163fe29e10ec96d54ca6a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_fccf26c42832c23702de4e520b158c45 = L.circleMarker(\\n [42.7417795414353, -73.25625964154518],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6fdec3fb02ffd6fc95054408eefbe545 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_425be29c49b41dbc2e350bbf2c59ea1d = $(`<div id="html_425be29c49b41dbc2e350bbf2c59ea1d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6fdec3fb02ffd6fc95054408eefbe545.setContent(html_425be29c49b41dbc2e350bbf2c59ea1d);\\n \\n \\n\\n circle_marker_fccf26c42832c23702de4e520b158c45.bindPopup(popup_6fdec3fb02ffd6fc95054408eefbe545)\\n ;\\n\\n \\n \\n \\n var circle_marker_650f9baf79a061cf715372bda509b7ca = L.circleMarker(\\n [42.74177944266665, -73.25502932808392],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b53edc365e23dc93ff79fce555fa2b24 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b57f756d1b98d78847a9518e9c64093 = $(`<div id="html_5b57f756d1b98d78847a9518e9c64093" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b53edc365e23dc93ff79fce555fa2b24.setContent(html_5b57f756d1b98d78847a9518e9c64093);\\n \\n \\n\\n circle_marker_650f9baf79a061cf715372bda509b7ca.bindPopup(popup_b53edc365e23dc93ff79fce555fa2b24)\\n ;\\n\\n \\n \\n \\n var circle_marker_893312b37495e06e6632a1c54126b533 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bdd5aa83c9c69ed39ba64d17094f5650 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_616ade0c3eeef178e2d77daf31a51ec9 = $(`<div id="html_616ade0c3eeef178e2d77daf31a51ec9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdd5aa83c9c69ed39ba64d17094f5650.setContent(html_616ade0c3eeef178e2d77daf31a51ec9);\\n \\n \\n\\n circle_marker_893312b37495e06e6632a1c54126b533.bindPopup(popup_bdd5aa83c9c69ed39ba64d17094f5650)\\n ;\\n\\n \\n \\n \\n var circle_marker_75c9f4a092188846f7757b1a7e1de2b1 = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b0248f63ae3317edc214dafa26151c8b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9fecd5bb1ac0778f75ade8fbd9b00964 = $(`<div id="html_9fecd5bb1ac0778f75ade8fbd9b00964" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0248f63ae3317edc214dafa26151c8b.setContent(html_9fecd5bb1ac0778f75ade8fbd9b00964);\\n \\n \\n\\n circle_marker_75c9f4a092188846f7757b1a7e1de2b1.bindPopup(popup_b0248f63ae3317edc214dafa26151c8b)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0dd16f37ea263cc2dc4ba591de014c2 = L.circleMarker(\\n [42.73997256609777, -73.27102322391595],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_68089fc2c078b693511f185e0d949eb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9a06362194ee8dece787e25729a05df2 = $(`<div id="html_9a06362194ee8dece787e25729a05df2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68089fc2c078b693511f185e0d949eb8.setContent(html_9a06362194ee8dece787e25729a05df2);\\n \\n \\n\\n circle_marker_d0dd16f37ea263cc2dc4ba591de014c2.bindPopup(popup_68089fc2c078b693511f185e0d949eb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa535a2b820f02c59b4f4af7443ab3c8 = L.circleMarker(\\n [42.73997262535657, -73.26979294630533],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_668648284a0c6767c17ddee32639ac4b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b1b0a59efa39f1eb6b7e280b81d6452 = $(`<div id="html_4b1b0a59efa39f1eb6b7e280b81d6452" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_668648284a0c6767c17ddee32639ac4b.setContent(html_4b1b0a59efa39f1eb6b7e280b81d6452);\\n \\n \\n\\n circle_marker_fa535a2b820f02c59b4f4af7443ab3c8.bindPopup(popup_668648284a0c6767c17ddee32639ac4b)\\n ;\\n\\n \\n \\n \\n var circle_marker_412bf4755809cda48711ba53f59e2af4 = L.circleMarker(\\n [42.73997272412122, -73.26610211346305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_59cc4e38f793b468a1c691c27b374b8a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe098ba5189cf304037f1b667e5c3182 = $(`<div id="html_fe098ba5189cf304037f1b667e5c3182" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_59cc4e38f793b468a1c691c27b374b8a.setContent(html_fe098ba5189cf304037f1b667e5c3182);\\n \\n \\n\\n circle_marker_412bf4755809cda48711ba53f59e2af4.bindPopup(popup_59cc4e38f793b468a1c691c27b374b8a)\\n ;\\n\\n \\n \\n \\n var circle_marker_10911671c897c11a75b35c3018f3e122 = L.circleMarker(\\n [42.73997273070553, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bd9653f000944386b4c225a6ef066c9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f3a2ce2547b01203021a65ba06c11350 = $(`<div id="html_f3a2ce2547b01203021a65ba06c11350" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd9653f000944386b4c225a6ef066c9c.setContent(html_f3a2ce2547b01203021a65ba06c11350);\\n \\n \\n\\n circle_marker_10911671c897c11a75b35c3018f3e122.bindPopup(popup_bd9653f000944386b4c225a6ef066c9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a30ae0947dc67d9e18b79d1bf0d7084 = L.circleMarker(\\n [42.73997272412122, -73.26364155823137],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9f9fec42b9526f5fabdf3b543f26a55a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_897f1a96683993d2b0962a48495e54c5 = $(`<div id="html_897f1a96683993d2b0962a48495e54c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f9fec42b9526f5fabdf3b543f26a55a.setContent(html_897f1a96683993d2b0962a48495e54c5);\\n \\n \\n\\n circle_marker_5a30ae0947dc67d9e18b79d1bf0d7084.bindPopup(popup_9f9fec42b9526f5fabdf3b543f26a55a)\\n ;\\n\\n \\n \\n \\n var circle_marker_864d0fc54dfae39800fd8ee9c945b9c3 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cbf55d3fee0969970a3c5898aa5e024e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6ad20119c193c283123a3540f7768dd8 = $(`<div id="html_6ad20119c193c283123a3540f7768dd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cbf55d3fee0969970a3c5898aa5e024e.setContent(html_6ad20119c193c283123a3540f7768dd8);\\n \\n \\n\\n circle_marker_864d0fc54dfae39800fd8ee9c945b9c3.bindPopup(popup_cbf55d3fee0969970a3c5898aa5e024e)\\n ;\\n\\n \\n \\n \\n var circle_marker_63a85804ca076ceb3aa23d9fa3c4a6e9 = L.circleMarker(\\n [42.73997267144673, -73.2611810030018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f8c12b52d23f1e823583d7924fc5d6ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f72dd0945668b88e7d720b48c56d6bfb = $(`<div id="html_f72dd0945668b88e7d720b48c56d6bfb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f8c12b52d23f1e823583d7924fc5d6ee.setContent(html_f72dd0945668b88e7d720b48c56d6bfb);\\n \\n \\n\\n circle_marker_63a85804ca076ceb3aa23d9fa3c4a6e9.bindPopup(popup_f8c12b52d23f1e823583d7924fc5d6ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_8cbad9da81386781c9e90cd5179102b0 = L.circleMarker(\\n [42.73997262535657, -73.25995072538909],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9b8ec511990a78974e82cdf9fcb22c57 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0365471178e490af50d642432875f30a = $(`<div id="html_0365471178e490af50d642432875f30a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b8ec511990a78974e82cdf9fcb22c57.setContent(html_0365471178e490af50d642432875f30a);\\n \\n \\n\\n circle_marker_8cbad9da81386781c9e90cd5179102b0.bindPopup(popup_9b8ec511990a78974e82cdf9fcb22c57)\\n ;\\n\\n \\n \\n \\n var circle_marker_7025c44088eb92a57d6333f63ee91242 = L.circleMarker(\\n [42.73997256609777, -73.25872044777847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7b3f4a72f42740f12d8b9e0b5489a305 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5c9699583d4131754e57ae18d2a9f000 = $(`<div id="html_5c9699583d4131754e57ae18d2a9f000" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7b3f4a72f42740f12d8b9e0b5489a305.setContent(html_5c9699583d4131754e57ae18d2a9f000);\\n \\n \\n\\n circle_marker_7025c44088eb92a57d6333f63ee91242.bindPopup(popup_7b3f4a72f42740f12d8b9e0b5489a305)\\n ;\\n\\n \\n \\n \\n var circle_marker_4797fc1dd26fe5906cc6b5b899d57e07 = L.circleMarker(\\n [42.73997240807432, -73.25625989256562],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ef23b55950a4d3db20fd799c0985ee89 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e2b366d787b61f7944f2fa151f18ae70 = $(`<div id="html_e2b366d787b61f7944f2fa151f18ae70" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ef23b55950a4d3db20fd799c0985ee89.setContent(html_e2b366d787b61f7944f2fa151f18ae70);\\n \\n \\n\\n circle_marker_4797fc1dd26fe5906cc6b5b899d57e07.bindPopup(popup_ef23b55950a4d3db20fd799c0985ee89)\\n ;\\n\\n \\n \\n \\n var circle_marker_b02553b328c317fcd6523dcc278d7cc9 = L.circleMarker(\\n [42.73997230930966, -73.25502961496441],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1843de427e1d9514c6e2ed679ae11189 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bcbe0e74b5f0033c4a14edcf8d4ebec6 = $(`<div id="html_bcbe0e74b5f0033c4a14edcf8d4ebec6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1843de427e1d9514c6e2ed679ae11189.setContent(html_bcbe0e74b5f0033c4a14edcf8d4ebec6);\\n \\n \\n\\n circle_marker_b02553b328c317fcd6523dcc278d7cc9.bindPopup(popup_1843de427e1d9514c6e2ed679ae11189)\\n ;\\n\\n \\n \\n \\n var circle_marker_0838e91ea096dbe1212a94965fd21c2c = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_66439aed845f36868f87eef35376704a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fce23c049c11ed187396b2a53a74bbcd = $(`<div id="html_fce23c049c11ed187396b2a53a74bbcd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_66439aed845f36868f87eef35376704a.setContent(html_fce23c049c11ed187396b2a53a74bbcd);\\n \\n \\n\\n circle_marker_0838e91ea096dbe1212a94965fd21c2c.bindPopup(popup_66439aed845f36868f87eef35376704a)\\n ;\\n\\n \\n \\n \\n var circle_marker_a8f7927ba33542cb2bfb7a977a096054 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9599188122723d5dcdc4e4b3fbbe3029 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4299e2273e8e200f7d17ae010ede8706 = $(`<div id="html_4299e2273e8e200f7d17ae010ede8706" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9599188122723d5dcdc4e4b3fbbe3029.setContent(html_4299e2273e8e200f7d17ae010ede8706);\\n \\n \\n\\n circle_marker_a8f7927ba33542cb2bfb7a977a096054.bindPopup(popup_9599188122723d5dcdc4e4b3fbbe3029)\\n ;\\n\\n \\n \\n \\n var circle_marker_0040f1ff85c176d55e48e29534d86109 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9ecfcfa1f01806b66eeb0cde58c09118 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36d737b55483cc391871e8b8b890bdfe = $(`<div id="html_36d737b55483cc391871e8b8b890bdfe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ecfcfa1f01806b66eeb0cde58c09118.setContent(html_36d737b55483cc391871e8b8b890bdfe);\\n \\n \\n\\n circle_marker_0040f1ff85c176d55e48e29534d86109.bindPopup(popup_9ecfcfa1f01806b66eeb0cde58c09118)\\n ;\\n\\n \\n \\n \\n var circle_marker_2a16ea51efc5f007d8fd1e04b1370053 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_729179648916b8fab8c70c1b40fd9a0d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_675f0cdf4119c21806c977e122079de3 = $(`<div id="html_675f0cdf4119c21806c977e122079de3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_729179648916b8fab8c70c1b40fd9a0d.setContent(html_675f0cdf4119c21806c977e122079de3);\\n \\n \\n\\n circle_marker_2a16ea51efc5f007d8fd1e04b1370053.bindPopup(popup_729179648916b8fab8c70c1b40fd9a0d)\\n ;\\n\\n \\n \\n \\n var circle_marker_5ccd303062806ac4f95f5654d079c82f = L.circleMarker(\\n [42.73996556039193, -73.22427267765188],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3de23727fb5a86e14f2acdd17ff2f5de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c3f31de895a911b082c18fc3c4e73b95 = $(`<div id="html_c3f31de895a911b082c18fc3c4e73b95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3de23727fb5a86e14f2acdd17ff2f5de.setContent(html_c3f31de895a911b082c18fc3c4e73b95);\\n \\n \\n\\n circle_marker_5ccd303062806ac4f95f5654d079c82f.bindPopup(popup_3de23727fb5a86e14f2acdd17ff2f5de)\\n ;\\n\\n \\n \\n \\n var circle_marker_d53bf6b3320f15c63b3de0ee62e5c8ae = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cba52adb3b2ddc3a3637408085fcfa60 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8e85209690eb974822f6df5097a1a95 = $(`<div id="html_f8e85209690eb974822f6df5097a1a95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cba52adb3b2ddc3a3637408085fcfa60.setContent(html_f8e85209690eb974822f6df5097a1a95);\\n \\n \\n\\n circle_marker_d53bf6b3320f15c63b3de0ee62e5c8ae.bindPopup(popup_cba52adb3b2ddc3a3637408085fcfa60)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba1bc7f6e2642f67f3eb430435ffba47 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2287c39e9c8ae64677d33c4b07ec5dd9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_acda34f931d28103200b2d76cf0d5737 = $(`<div id="html_acda34f931d28103200b2d76cf0d5737" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2287c39e9c8ae64677d33c4b07ec5dd9.setContent(html_acda34f931d28103200b2d76cf0d5737);\\n \\n \\n\\n circle_marker_ba1bc7f6e2642f67f3eb430435ffba47.bindPopup(popup_2287c39e9c8ae64677d33c4b07ec5dd9)\\n ;\\n\\n \\n \\n \\n var circle_marker_98b47abe8f7b616fdba523d1d8b8f50e = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_35c2e65e4cfcb60a76547e93bc15fb34 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13b61a6164d6571a8cea779a9ba6d430 = $(`<div id="html_13b61a6164d6571a8cea779a9ba6d430" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35c2e65e4cfcb60a76547e93bc15fb34.setContent(html_13b61a6164d6571a8cea779a9ba6d430);\\n \\n \\n\\n circle_marker_98b47abe8f7b616fdba523d1d8b8f50e.bindPopup(popup_35c2e65e4cfcb60a76547e93bc15fb34)\\n ;\\n\\n \\n \\n \\n var circle_marker_5da5ed8e9e0e7dd863e3535699f89a24 = L.circleMarker(\\n [42.73816527471333, -73.27348352812871],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_38e52af454fc0cdd154c050aa60f1ad1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_387798a149974f82e727a5ecca1124e0 = $(`<div id="html_387798a149974f82e727a5ecca1124e0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38e52af454fc0cdd154c050aa60f1ad1.setContent(html_387798a149974f82e727a5ecca1124e0);\\n \\n \\n\\n circle_marker_5da5ed8e9e0e7dd863e3535699f89a24.bindPopup(popup_38e52af454fc0cdd154c050aa60f1ad1)\\n ;\\n\\n \\n \\n \\n var circle_marker_97d83aae379f8412f17ecfb557311bdf = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f51e0606889c35d59d7461732b4a68f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8d29b5aacc307740da2911885bc10724 = $(`<div id="html_8d29b5aacc307740da2911885bc10724" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f51e0606889c35d59d7461732b4a68f4.setContent(html_8d29b5aacc307740da2911885bc10724);\\n \\n \\n\\n circle_marker_97d83aae379f8412f17ecfb557311bdf.bindPopup(popup_f51e0606889c35d59d7461732b4a68f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_45c04f554d477be617b96d93fff58200 = L.circleMarker(\\n [42.7381655709953, -73.26241135233037],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8a3755cbf42d133f91b9c3f082dbb738 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_225d2d9e183b2305fc34d90577f8c81b = $(`<div id="html_225d2d9e183b2305fc34d90577f8c81b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a3755cbf42d133f91b9c3f082dbb738.setContent(html_225d2d9e183b2305fc34d90577f8c81b);\\n \\n \\n\\n circle_marker_45c04f554d477be617b96d93fff58200.bindPopup(popup_8a3755cbf42d133f91b9c3f082dbb738)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b49d8e3cfa8ba8da896bbe69a776f2a = L.circleMarker(\\n [42.73816553807507, -73.26118111057326],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a024b5495f2bb211a3ee3523d1739f63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26cc34465f3de4081f46a06bd6d79236 = $(`<div id="html_26cc34465f3de4081f46a06bd6d79236" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a024b5495f2bb211a3ee3523d1739f63.setContent(html_26cc34465f3de4081f46a06bd6d79236);\\n \\n \\n\\n circle_marker_2b49d8e3cfa8ba8da896bbe69a776f2a.bindPopup(popup_a024b5495f2bb211a3ee3523d1739f63)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1cd2eaf96a7175a849cbb76fc8efae7 = L.circleMarker(\\n [42.73816549198677, -73.25995086881771],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a657e3defc567c98cba8f7dd721804ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e303c603829429948ec958c0d74d60c0 = $(`<div id="html_e303c603829429948ec958c0d74d60c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a657e3defc567c98cba8f7dd721804ca.setContent(html_e303c603829429948ec958c0d74d60c0);\\n \\n \\n\\n circle_marker_e1cd2eaf96a7175a849cbb76fc8efae7.bindPopup(popup_a657e3defc567c98cba8f7dd721804ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_c4cbdd4866022432bf47d315656b0780 = L.circleMarker(\\n [42.73816543273038, -73.25872062706426],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b508abac52d9b88f13db6d8e09be095f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d25f79ee2a2dc551d75c6e5770400db9 = $(`<div id="html_d25f79ee2a2dc551d75c6e5770400db9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b508abac52d9b88f13db6d8e09be095f.setContent(html_d25f79ee2a2dc551d75c6e5770400db9);\\n \\n \\n\\n circle_marker_c4cbdd4866022432bf47d315656b0780.bindPopup(popup_b508abac52d9b88f13db6d8e09be095f)\\n ;\\n\\n \\n \\n \\n var circle_marker_95c0f09fc991c8b9883de5f02a13a0e2 = L.circleMarker(\\n [42.7381653603059, -73.25749038531342],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_dd70a3890ac7f939be5e65162d405316 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1218f01a3567962c10802f0c7f636a84 = $(`<div id="html_1218f01a3567962c10802f0c7f636a84" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd70a3890ac7f939be5e65162d405316.setContent(html_1218f01a3567962c10802f0c7f636a84);\\n \\n \\n\\n circle_marker_95c0f09fc991c8b9883de5f02a13a0e2.bindPopup(popup_dd70a3890ac7f939be5e65162d405316)\\n ;\\n\\n \\n \\n \\n var circle_marker_6900067b99e42c14f650240c2ac39b76 = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_43fda2db5fdc2205908b776efa11dc68 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_348cd3860e9e97c60077f19960b4a19f = $(`<div id="html_348cd3860e9e97c60077f19960b4a19f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43fda2db5fdc2205908b776efa11dc68.setContent(html_348cd3860e9e97c60077f19960b4a19f);\\n \\n \\n\\n circle_marker_6900067b99e42c14f650240c2ac39b76.bindPopup(popup_43fda2db5fdc2205908b776efa11dc68)\\n ;\\n\\n \\n \\n \\n var circle_marker_d244157ff03fe43f93b00bcf9c3167f3 = L.circleMarker(\\n [42.73816517595268, -73.25502990182166],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.477898169151024, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9e5958cc4e1ebd18a1fd3554716ed4a7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c50405f2dd5171bf00df7e79559a0f4 = $(`<div id="html_1c50405f2dd5171bf00df7e79559a0f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e5958cc4e1ebd18a1fd3554716ed4a7.setContent(html_1c50405f2dd5171bf00df7e79559a0f4);\\n \\n \\n\\n circle_marker_d244157ff03fe43f93b00bcf9c3167f3.bindPopup(popup_9e5958cc4e1ebd18a1fd3554716ed4a7)\\n ;\\n\\n \\n \\n \\n var circle_marker_4735083aedfee83d08918f435208edc0 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_323b0cd2a3e4147a56e220a9314a4cdb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01032d3639ce2eabd03e6ffd8d93fc6d = $(`<div id="html_01032d3639ce2eabd03e6ffd8d93fc6d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_323b0cd2a3e4147a56e220a9314a4cdb.setContent(html_01032d3639ce2eabd03e6ffd8d93fc6d);\\n \\n \\n\\n circle_marker_4735083aedfee83d08918f435208edc0.bindPopup(popup_323b0cd2a3e4147a56e220a9314a4cdb)\\n ;\\n\\n \\n \\n \\n var circle_marker_993dc2de91493bd5029d339ec4b2061f = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_db15a25dbe97eef967b356df69ae67db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_724d12b39347ff30a4e67def737b4a2e = $(`<div id="html_724d12b39347ff30a4e67def737b4a2e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db15a25dbe97eef967b356df69ae67db.setContent(html_724d12b39347ff30a4e67def737b4a2e);\\n \\n \\n\\n circle_marker_993dc2de91493bd5029d339ec4b2061f.bindPopup(popup_db15a25dbe97eef967b356df69ae67db)\\n ;\\n\\n \\n \\n \\n var circle_marker_fdde5d5f01a0c67cb793f7fcc812c9bd = L.circleMarker(\\n [42.73815842730842, -73.22427386093767],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0ae66e83a00854a4f2668689527b22d3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31bd83ad051dc85277067155de215f05 = $(`<div id="html_31bd83ad051dc85277067155de215f05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0ae66e83a00854a4f2668689527b22d3.setContent(html_31bd83ad051dc85277067155de215f05);\\n \\n \\n\\n circle_marker_fdde5d5f01a0c67cb793f7fcc812c9bd.bindPopup(popup_0ae66e83a00854a4f2668689527b22d3)\\n ;\\n\\n \\n \\n \\n var circle_marker_d8b04a547b1722c8102e34ab0e9953db = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_35d9c91df7b441bd885c37834044559e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e4619aa9b0d8d4e8439ffc8eb6f78f6b = $(`<div id="html_e4619aa9b0d8d4e8439ffc8eb6f78f6b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35d9c91df7b441bd885c37834044559e.setContent(html_e4619aa9b0d8d4e8439ffc8eb6f78f6b);\\n \\n \\n\\n circle_marker_d8b04a547b1722c8102e34ab0e9953db.bindPopup(popup_35d9c91df7b441bd885c37834044559e)\\n ;\\n\\n \\n \\n \\n var circle_marker_fab06d6bb45a39df466e0c8e72deb9da = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5be2ec00e0b74fef0839d660b4ca97ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f9ce51b2ec7e258d483aed976f1ec83 = $(`<div id="html_1f9ce51b2ec7e258d483aed976f1ec83" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5be2ec00e0b74fef0839d660b4ca97ff.setContent(html_1f9ce51b2ec7e258d483aed976f1ec83);\\n \\n \\n\\n circle_marker_fab06d6bb45a39df466e0c8e72deb9da.bindPopup(popup_5be2ec00e0b74fef0839d660b4ca97ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_1f5cf75245752d17fb8604113c8b1e5f = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6b80f4f2b96ebe48f53de1722891495a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6719805ea9f5d71cc5d6f07e91fb40e3 = $(`<div id="html_6719805ea9f5d71cc5d6f07e91fb40e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b80f4f2b96ebe48f53de1722891495a.setContent(html_6719805ea9f5d71cc5d6f07e91fb40e3);\\n \\n \\n\\n circle_marker_1f5cf75245752d17fb8604113c8b1e5f.bindPopup(popup_6b80f4f2b96ebe48f53de1722891495a)\\n ;\\n\\n \\n \\n \\n var circle_marker_658caf47ecc674c697efa5c8dc46cb73 = L.circleMarker(\\n [42.736358404703424, -73.2685624535584],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bf5b306f8ff58d12db554a593df23a12 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2a98110186f4fc6ca154c1f144d051a4 = $(`<div id="html_2a98110186f4fc6ca154c1f144d051a4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bf5b306f8ff58d12db554a593df23a12.setContent(html_2a98110186f4fc6ca154c1f144d051a4);\\n \\n \\n\\n circle_marker_658caf47ecc674c697efa5c8dc46cb73.bindPopup(popup_bf5b306f8ff58d12db554a593df23a12)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a11290824b3ec2f0547fdfd07f51610 = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_17b09ebbc4c33839adf6eeea3aa0fa80 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a34b550365c1240549f7874b6a29b604 = $(`<div id="html_a34b550365c1240549f7874b6a29b604" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_17b09ebbc4c33839adf6eeea3aa0fa80.setContent(html_a34b550365c1240549f7874b6a29b604);\\n \\n \\n\\n circle_marker_0a11290824b3ec2f0547fdfd07f51610.bindPopup(popup_17b09ebbc4c33839adf6eeea3aa0fa80)\\n ;\\n\\n \\n \\n \\n var circle_marker_2e213a3f3ac149974f33f14faf71478d = L.circleMarker(\\n [42.73635845737364, -73.26610204175164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f5add071ebfca91d19bf511db1d04434 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d96ace95f3ddfa17a657b05766f3259a = $(`<div id="html_d96ace95f3ddfa17a657b05766f3259a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5add071ebfca91d19bf511db1d04434.setContent(html_d96ace95f3ddfa17a657b05766f3259a);\\n \\n \\n\\n circle_marker_2e213a3f3ac149974f33f14faf71478d.bindPopup(popup_f5add071ebfca91d19bf511db1d04434)\\n ;\\n\\n \\n \\n \\n var circle_marker_5c3513bb90736691d707ef2796a45711 = L.circleMarker(\\n [42.73635846395741, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_db3726bf17d8870e18132848fe67f8c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a52a4e7ba9ca125552eb2df139dea0a7 = $(`<div id="html_a52a4e7ba9ca125552eb2df139dea0a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db3726bf17d8870e18132848fe67f8c8.setContent(html_a52a4e7ba9ca125552eb2df139dea0a7);\\n \\n \\n\\n circle_marker_5c3513bb90736691d707ef2796a45711.bindPopup(popup_db3726bf17d8870e18132848fe67f8c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e8819b0a65737ab8ce4e876ae7c8c81 = L.circleMarker(\\n [42.73635845737364, -73.26364162994278],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7b7a47b68f5c45ff56d94e4d3022a83e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_29fbec35a8db020b9c6eac93c2555f5b = $(`<div id="html_29fbec35a8db020b9c6eac93c2555f5b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7b7a47b68f5c45ff56d94e4d3022a83e.setContent(html_29fbec35a8db020b9c6eac93c2555f5b);\\n \\n \\n\\n circle_marker_8e8819b0a65737ab8ce4e876ae7c8c81.bindPopup(popup_7b7a47b68f5c45ff56d94e4d3022a83e)\\n ;\\n\\n \\n \\n \\n var circle_marker_c13062461f73df7843f4e57d9cb868db = L.circleMarker(\\n [42.73635843762231, -73.26241142403887],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_33999d60b42fcc1fc75bf56d42ccc237 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07f1cccdadb40ceb8fd7dc7364589e87 = $(`<div id="html_07f1cccdadb40ceb8fd7dc7364589e87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33999d60b42fcc1fc75bf56d42ccc237.setContent(html_07f1cccdadb40ceb8fd7dc7364589e87);\\n \\n \\n\\n circle_marker_c13062461f73df7843f4e57d9cb868db.bindPopup(popup_33999d60b42fcc1fc75bf56d42ccc237)\\n ;\\n\\n \\n \\n \\n var circle_marker_15ee7cd123e7eb9d2bc89bf380066798 = L.circleMarker(\\n [42.736358404703424, -73.26118121813602],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_75bf572f1d7db1c928433eb542eab50d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6976021a9cbbeb356be83fbab4802975 = $(`<div id="html_6976021a9cbbeb356be83fbab4802975" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75bf572f1d7db1c928433eb542eab50d.setContent(html_6976021a9cbbeb356be83fbab4802975);\\n \\n \\n\\n circle_marker_15ee7cd123e7eb9d2bc89bf380066798.bindPopup(popup_75bf572f1d7db1c928433eb542eab50d)\\n ;\\n\\n \\n \\n \\n var circle_marker_1902bd02041a463c38c497f63ba70588 = L.circleMarker(\\n [42.73635835861699, -73.25995101223472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4fefc6852e1483c8695e574b6a316945 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b75bd2cbd1e4f18dd599cf477a521193 = $(`<div id="html_b75bd2cbd1e4f18dd599cf477a521193" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4fefc6852e1483c8695e574b6a316945.setContent(html_b75bd2cbd1e4f18dd599cf477a521193);\\n \\n \\n\\n circle_marker_1902bd02041a463c38c497f63ba70588.bindPopup(popup_4fefc6852e1483c8695e574b6a316945)\\n ;\\n\\n \\n \\n \\n var circle_marker_e706162927fd88c1a8563c9eac4c54fe = L.circleMarker(\\n [42.73635829936299, -73.25872080633552],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2978c0e8846c2b81443ce4799adcfe8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd8e0e4f423c816955b8098a246825cb = $(`<div id="html_cd8e0e4f423c816955b8098a246825cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2978c0e8846c2b81443ce4799adcfe8f.setContent(html_cd8e0e4f423c816955b8098a246825cb);\\n \\n \\n\\n circle_marker_e706162927fd88c1a8563c9eac4c54fe.bindPopup(popup_2978c0e8846c2b81443ce4799adcfe8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_7d20b4406d55b7fe44eae824a40aa968 = L.circleMarker(\\n [42.73635822694145, -73.25749060043893],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2b271d1289ce63a630104491a7ce3cd9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d75e770b73d247757ec76d04243bbf72 = $(`<div id="html_d75e770b73d247757ec76d04243bbf72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b271d1289ce63a630104491a7ce3cd9.setContent(html_d75e770b73d247757ec76d04243bbf72);\\n \\n \\n\\n circle_marker_7d20b4406d55b7fe44eae824a40aa968.bindPopup(popup_2b271d1289ce63a630104491a7ce3cd9)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6ec453eca15eac5948517f949d45b1f = L.circleMarker(\\n [42.73635814135235, -73.25626039454548],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1f997ba4cf6689d17908a1a633fd44c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56d98320e6bdc5c8eb11e3742f85a815 = $(`<div id="html_56d98320e6bdc5c8eb11e3742f85a815" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f997ba4cf6689d17908a1a633fd44c5.setContent(html_56d98320e6bdc5c8eb11e3742f85a815);\\n \\n \\n\\n circle_marker_d6ec453eca15eac5948517f949d45b1f.bindPopup(popup_1f997ba4cf6689d17908a1a633fd44c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_df174668003a680c64feaea3999ada45 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bba671f365fd333297a548caf1dbe810 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57a47177b76806da9bb1fade004d6763 = $(`<div id="html_57a47177b76806da9bb1fade004d6763" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bba671f365fd333297a548caf1dbe810.setContent(html_57a47177b76806da9bb1fade004d6763);\\n \\n \\n\\n circle_marker_df174668003a680c64feaea3999ada45.bindPopup(popup_bba671f365fd333297a548caf1dbe810)\\n ;\\n\\n \\n \\n \\n var circle_marker_7da774395b8a789e160acfcb90ed4882 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_676980695e655a08e44919aef5376d55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be295e67ece59ea96c7e4cf757765f47 = $(`<div id="html_be295e67ece59ea96c7e4cf757765f47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_676980695e655a08e44919aef5376d55.setContent(html_be295e67ece59ea96c7e4cf757765f47);\\n \\n \\n\\n circle_marker_7da774395b8a789e160acfcb90ed4882.bindPopup(popup_676980695e655a08e44919aef5376d55)\\n ;\\n\\n \\n \\n \\n var circle_marker_0e09c9e1661374ff9db1612b255bf563 = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8615c8a81778658a335fcbcd6ac5700a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a97f940327a0c16313c4b96a43555bd = $(`<div id="html_4a97f940327a0c16313c4b96a43555bd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8615c8a81778658a335fcbcd6ac5700a.setContent(html_4a97f940327a0c16313c4b96a43555bd);\\n \\n \\n\\n circle_marker_0e09c9e1661374ff9db1612b255bf563.bindPopup(popup_8615c8a81778658a335fcbcd6ac5700a)\\n ;\\n\\n \\n \\n \\n var circle_marker_33c73f3f0e130e7d1e6ffb39b7b6ac81 = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.949327084834294, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4012d38f517b15e4119cee874f5f2cac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e8f079a5c2124656bf1dec5ca0828073 = $(`<div id="html_e8f079a5c2124656bf1dec5ca0828073" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4012d38f517b15e4119cee874f5f2cac.setContent(html_e8f079a5c2124656bf1dec5ca0828073);\\n \\n \\n\\n circle_marker_33c73f3f0e130e7d1e6ffb39b7b6ac81.bindPopup(popup_4012d38f517b15e4119cee874f5f2cac)\\n ;\\n\\n \\n \\n \\n var circle_marker_30fd9265e3151c3673b06f9007dd21c2 = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5ab79890f6a6835be88997d9aa350936 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ad5f7665edaca2e728ff93189730ae45 = $(`<div id="html_ad5f7665edaca2e728ff93189730ae45" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ab79890f6a6835be88997d9aa350936.setContent(html_ad5f7665edaca2e728ff93189730ae45);\\n \\n \\n\\n circle_marker_30fd9265e3151c3673b06f9007dd21c2.bindPopup(popup_5ab79890f6a6835be88997d9aa350936)\\n ;\\n\\n \\n \\n \\n var circle_marker_be77bb7bbca51226f035ed84f1aa52d2 = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_45ac77f1735f7ab9ba998e64bd53e11f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e48f0925afce5b1b53c7bf65e729159c = $(`<div id="html_e48f0925afce5b1b53c7bf65e729159c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_45ac77f1735f7ab9ba998e64bd53e11f.setContent(html_e48f0925afce5b1b53c7bf65e729159c);\\n \\n \\n\\n circle_marker_be77bb7bbca51226f035ed84f1aa52d2.bindPopup(popup_45ac77f1735f7ab9ba998e64bd53e11f)\\n ;\\n\\n \\n \\n \\n var circle_marker_397ccd06ba0e928324ac3e296274a2bb = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7f4d857dc0aac126a9dd46d31cf49888 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_daa5000a329427a9e736ff8cfec36b0c = $(`<div id="html_daa5000a329427a9e736ff8cfec36b0c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7f4d857dc0aac126a9dd46d31cf49888.setContent(html_daa5000a329427a9e736ff8cfec36b0c);\\n \\n \\n\\n circle_marker_397ccd06ba0e928324ac3e296274a2bb.bindPopup(popup_7f4d857dc0aac126a9dd46d31cf49888)\\n ;\\n\\n \\n \\n \\n var circle_marker_8986e472437b95c36c7651f5ca3ada19 = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b994d5d5f654e15e3d61732245140a94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c7d3ecda563e5b70c7209a17090b113 = $(`<div id="html_1c7d3ecda563e5b70c7209a17090b113" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b994d5d5f654e15e3d61732245140a94.setContent(html_1c7d3ecda563e5b70c7209a17090b113);\\n \\n \\n\\n circle_marker_8986e472437b95c36c7651f5ca3ada19.bindPopup(popup_b994d5d5f654e15e3d61732245140a94)\\n ;\\n\\n \\n \\n \\n var circle_marker_72ec7e2f0beebdf1175e483171f2ce7a = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_26729cb120893f43db303d28908796bc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a4cf88d6c7fbb78515d06b29ffa43f4 = $(`<div id="html_6a4cf88d6c7fbb78515d06b29ffa43f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_26729cb120893f43db303d28908796bc.setContent(html_6a4cf88d6c7fbb78515d06b29ffa43f4);\\n \\n \\n\\n circle_marker_72ec7e2f0beebdf1175e483171f2ce7a.bindPopup(popup_26729cb120893f43db303d28908796bc)\\n ;\\n\\n \\n \\n \\n var circle_marker_86403ad136574cc748a56ba540cb116b = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bd85e6ee975c763d5612defb23bc1b3f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_93841b78d622f902a23d9e84fbf7dc17 = $(`<div id="html_93841b78d622f902a23d9e84fbf7dc17" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bd85e6ee975c763d5612defb23bc1b3f.setContent(html_93841b78d622f902a23d9e84fbf7dc17);\\n \\n \\n\\n circle_marker_86403ad136574cc748a56ba540cb116b.bindPopup(popup_bd85e6ee975c763d5612defb23bc1b3f)\\n ;\\n\\n \\n \\n \\n var circle_marker_baeb345aec101b1687c90e8ca307271a = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f439beec31ca024f6c52b2cf8a657988 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d1ee4508b2c3c915306e69b06705d32 = $(`<div id="html_6d1ee4508b2c3c915306e69b06705d32" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f439beec31ca024f6c52b2cf8a657988.setContent(html_6d1ee4508b2c3c915306e69b06705d32);\\n \\n \\n\\n circle_marker_baeb345aec101b1687c90e8ca307271a.bindPopup(popup_f439beec31ca024f6c52b2cf8a657988)\\n ;\\n\\n \\n \\n \\n var circle_marker_cccb89eae45436729d3995ddeefe8efa = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5345bd9c11973b62366145dc83f728ff = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fb9ab87513eb1af55f3a9f0596eb4d44 = $(`<div id="html_fb9ab87513eb1af55f3a9f0596eb4d44" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5345bd9c11973b62366145dc83f728ff.setContent(html_fb9ab87513eb1af55f3a9f0596eb4d44);\\n \\n \\n\\n circle_marker_cccb89eae45436729d3995ddeefe8efa.bindPopup(popup_5345bd9c11973b62366145dc83f728ff)\\n ;\\n\\n \\n \\n \\n var circle_marker_936ee891cff9a9bdcacf40ed7e26af7d = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2b06be4fd2ec659628ed7d2fea83316f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e17eb6eb37f66bd63dc55f1e698123e = $(`<div id="html_6e17eb6eb37f66bd63dc55f1e698123e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b06be4fd2ec659628ed7d2fea83316f.setContent(html_6e17eb6eb37f66bd63dc55f1e698123e);\\n \\n \\n\\n circle_marker_936ee891cff9a9bdcacf40ed7e26af7d.bindPopup(popup_2b06be4fd2ec659628ed7d2fea83316f)\\n ;\\n\\n \\n \\n \\n var circle_marker_55f99e5aa2a100cc31dfd3bb0b21cf34 = L.circleMarker(\\n [42.73635556051186, -73.23903751265883],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6ac68a78a9f017a6b290ca1a9f2a23c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b207c18a7466027d009a94c6d696a32 = $(`<div id="html_5b207c18a7466027d009a94c6d696a32" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ac68a78a9f017a6b290ca1a9f2a23c7.setContent(html_5b207c18a7466027d009a94c6d696a32);\\n \\n \\n\\n circle_marker_55f99e5aa2a100cc31dfd3bb0b21cf34.bindPopup(popup_6ac68a78a9f017a6b290ca1a9f2a23c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_462bcf6f8426afdf9318b0341d1b8a73 = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c659b9c7ab39ed0e70f316049e257102 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edce614689b216838759007f9c187d0b = $(`<div id="html_edce614689b216838759007f9c187d0b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c659b9c7ab39ed0e70f316049e257102.setContent(html_edce614689b216838759007f9c187d0b);\\n \\n \\n\\n circle_marker_462bcf6f8426afdf9318b0341d1b8a73.bindPopup(popup_c659b9c7ab39ed0e70f316049e257102)\\n ;\\n\\n \\n \\n \\n var circle_marker_7a8eadec000b2bbb11f63f44307475c6 = L.circleMarker(\\n [42.73635129422489, -73.22427504412757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2cdbc1d9459e7111a61b872b7ae1b1be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b9a3d9d4757e338b00d2aede2d2605a6 = $(`<div id="html_b9a3d9d4757e338b00d2aede2d2605a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2cdbc1d9459e7111a61b872b7ae1b1be.setContent(html_b9a3d9d4757e338b00d2aede2d2605a6);\\n \\n \\n\\n circle_marker_7a8eadec000b2bbb11f63f44307475c6.bindPopup(popup_2cdbc1d9459e7111a61b872b7ae1b1be)\\n ;\\n\\n \\n \\n \\n var circle_marker_d8e6065818b5b8a93e5fb0b82e4ab41f = L.circleMarker(\\n [42.73635085311189, -73.22304483851624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_653172c422a3226c88599dce5ddb0626 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ca8f4b3d05c83a8323c1e70b4e93c8cf = $(`<div id="html_ca8f4b3d05c83a8323c1e70b4e93c8cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_653172c422a3226c88599dce5ddb0626.setContent(html_ca8f4b3d05c83a8323c1e70b4e93c8cf);\\n \\n \\n\\n circle_marker_d8e6065818b5b8a93e5fb0b82e4ab41f.bindPopup(popup_653172c422a3226c88599dce5ddb0626)\\n ;\\n\\n \\n \\n \\n var circle_marker_edc1dbd4442f9a5a34887b06e71fb201 = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6ae72d44fe85de84ddb1b903a4aed112 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_adfeb864457584084365897a2d68bc96 = $(`<div id="html_adfeb864457584084365897a2d68bc96" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ae72d44fe85de84ddb1b903a4aed112.setContent(html_adfeb864457584084365897a2d68bc96);\\n \\n \\n\\n circle_marker_edc1dbd4442f9a5a34887b06e71fb201.bindPopup(popup_6ae72d44fe85de84ddb1b903a4aed112)\\n ;\\n\\n \\n \\n \\n var circle_marker_fbb9122420c251e273d702ae743734ca = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_206ec71c3d62a93ef87cb0ec1ad2017c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e07e22ae763583d10bb96b6de7392fc6 = $(`<div id="html_e07e22ae763583d10bb96b6de7392fc6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_206ec71c3d62a93ef87cb0ec1ad2017c.setContent(html_e07e22ae763583d10bb96b6de7392fc6);\\n \\n \\n\\n circle_marker_fbb9122420c251e273d702ae743734ca.bindPopup(popup_206ec71c3d62a93ef87cb0ec1ad2017c)\\n ;\\n\\n \\n \\n \\n var circle_marker_ad2b82917f8aa090cebe9a743aceebfa = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_216b8fd193844b0c07416d1d6026347a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e61f3edfa659f2fd392aeee83b6c62b = $(`<div id="html_1e61f3edfa659f2fd392aeee83b6c62b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_216b8fd193844b0c07416d1d6026347a.setContent(html_1e61f3edfa659f2fd392aeee83b6c62b);\\n \\n \\n\\n circle_marker_ad2b82917f8aa090cebe9a743aceebfa.bindPopup(popup_216b8fd193844b0c07416d1d6026347a)\\n ;\\n\\n \\n \\n \\n var circle_marker_380edb2b6392191ffda5b4573746b669 = L.circleMarker(\\n [42.73455127133177, -73.26856234600437],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9db4d3120e867677b5968e197a1851be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f79efbddff2a8a8b1baf4b1f0867efc = $(`<div id="html_0f79efbddff2a8a8b1baf4b1f0867efc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9db4d3120e867677b5968e197a1851be.setContent(html_0f79efbddff2a8a8b1baf4b1f0867efc);\\n \\n \\n\\n circle_marker_380edb2b6392191ffda5b4573746b669.bindPopup(popup_9db4d3120e867677b5968e197a1851be)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf10d34d9074dcd16b7c2df1383c7287 = L.circleMarker(\\n [42.73455130424932, -73.26733217595286],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f59a3b6c8d76bd275ce33df90943fbae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b4c8f0d9488efe62d1df3574b508e6c5 = $(`<div id="html_b4c8f0d9488efe62d1df3574b508e6c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f59a3b6c8d76bd275ce33df90943fbae.setContent(html_b4c8f0d9488efe62d1df3574b508e6c5);\\n \\n \\n\\n circle_marker_cf10d34d9074dcd16b7c2df1383c7287.bindPopup(popup_f59a3b6c8d76bd275ce33df90943fbae)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f11199c686d0b9aa7795d34c3dc4240 = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b2df9c0079790190c85ca6d2245d4b75 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_786c1e293f34061628dcd1db04b5c678 = $(`<div id="html_786c1e293f34061628dcd1db04b5c678" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2df9c0079790190c85ca6d2245d4b75.setContent(html_786c1e293f34061628dcd1db04b5c678);\\n \\n \\n\\n circle_marker_6f11199c686d0b9aa7795d34c3dc4240.bindPopup(popup_b2df9c0079790190c85ca6d2245d4b75)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6d7e4421566f3d96796762833377b34 = L.circleMarker(\\n [42.734551330583365, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4703056815aafcfc6df9320601397acd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2a60d01845b58129b5b05c18d4db9670 = $(`<div id="html_2a60d01845b58129b5b05c18d4db9670" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4703056815aafcfc6df9320601397acd.setContent(html_2a60d01845b58129b5b05c18d4db9670);\\n \\n \\n\\n circle_marker_a6d7e4421566f3d96796762833377b34.bindPopup(popup_4703056815aafcfc6df9320601397acd)\\n ;\\n\\n \\n \\n \\n var circle_marker_09338328f5941ce1405f067ddfc61d1f = L.circleMarker(\\n [42.73455132399984, -73.26364166579413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6d7063681a9f7f41232645f34c85f77e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb8eff749917e43dc55d47978a9ee69d = $(`<div id="html_eb8eff749917e43dc55d47978a9ee69d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d7063681a9f7f41232645f34c85f77e.setContent(html_eb8eff749917e43dc55d47978a9ee69d);\\n \\n \\n\\n circle_marker_09338328f5941ce1405f067ddfc61d1f.bindPopup(popup_6d7063681a9f7f41232645f34c85f77e)\\n ;\\n\\n \\n \\n \\n var circle_marker_a00382ad3e0e5b13e16dc0e1c7e598d1 = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_24d7ccb7f187a3a183dbf4ab8f67130a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4e131f48c6763ed6d435f56b4c62f02 = $(`<div id="html_d4e131f48c6763ed6d435f56b4c62f02" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24d7ccb7f187a3a183dbf4ab8f67130a.setContent(html_d4e131f48c6763ed6d435f56b4c62f02);\\n \\n \\n\\n circle_marker_a00382ad3e0e5b13e16dc0e1c7e598d1.bindPopup(popup_24d7ccb7f187a3a183dbf4ab8f67130a)\\n ;\\n\\n \\n \\n \\n var circle_marker_b4660abaa0b3a7bb9e31ae378117641c = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8e5b4e5c7bf2d9c68108aab3b11440cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46c3be3e8132f526675ac5ae4b09f7ea = $(`<div id="html_46c3be3e8132f526675ac5ae4b09f7ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8e5b4e5c7bf2d9c68108aab3b11440cd.setContent(html_46c3be3e8132f526675ac5ae4b09f7ea);\\n \\n \\n\\n circle_marker_b4660abaa0b3a7bb9e31ae378117641c.bindPopup(popup_8e5b4e5c7bf2d9c68108aab3b11440cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_55e8ceb5321b3e9544842ffa61c6f531 = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_35dab324733e6f51c96b233bf73d5bb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_55b1d202c3ffef7ebef3b1657a5dd522 = $(`<div id="html_55b1d202c3ffef7ebef3b1657a5dd522" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35dab324733e6f51c96b233bf73d5bb8.setContent(html_55b1d202c3ffef7ebef3b1657a5dd522);\\n \\n \\n\\n circle_marker_55e8ceb5321b3e9544842ffa61c6f531.bindPopup(popup_35dab324733e6f51c96b233bf73d5bb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca3f5e81412821a756221b6717a50b2b = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_da98c3e34cadc4da4c831be075e4e661 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_96edeff63009813f473f4f94e7b89e21 = $(`<div id="html_96edeff63009813f473f4f94e7b89e21" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_da98c3e34cadc4da4c831be075e4e661.setContent(html_96edeff63009813f473f4f94e7b89e21);\\n \\n \\n\\n circle_marker_ca3f5e81412821a756221b6717a50b2b.bindPopup(popup_da98c3e34cadc4da4c831be075e4e661)\\n ;\\n\\n \\n \\n \\n var circle_marker_202bf317e59c25b6e24d2260d44a0abf = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0288f36468446859000c36473cb7401a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a043ac0a37d992fc55dcf19e9499a838 = $(`<div id="html_a043ac0a37d992fc55dcf19e9499a838" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0288f36468446859000c36473cb7401a.setContent(html_a043ac0a37d992fc55dcf19e9499a838);\\n \\n \\n\\n circle_marker_202bf317e59c25b6e24d2260d44a0abf.bindPopup(popup_0288f36468446859000c36473cb7401a)\\n ;\\n\\n \\n \\n \\n var circle_marker_16724fb4a225f155bce03da5c4bedb49 = L.circleMarker(\\n [42.73455100799136, -73.25626064550488],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_341e5a8b7817074bc0305575b48cbbab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2841460d263a6fa13b462f92caaadc3 = $(`<div id="html_a2841460d263a6fa13b462f92caaadc3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_341e5a8b7817074bc0305575b48cbbab.setContent(html_a2841460d263a6fa13b462f92caaadc3);\\n \\n \\n\\n circle_marker_16724fb4a225f155bce03da5c4bedb49.bindPopup(popup_341e5a8b7817074bc0305575b48cbbab)\\n ;\\n\\n \\n \\n \\n var circle_marker_289b0c5c0f1a82269c74acf396d5ba33 = L.circleMarker(\\n [42.73455090923871, -73.25503047546643],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ac9629900df3e92cb3a63593088b2943 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_809275a3527d29a2367235037e3b0021 = $(`<div id="html_809275a3527d29a2367235037e3b0021" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ac9629900df3e92cb3a63593088b2943.setContent(html_809275a3527d29a2367235037e3b0021);\\n \\n \\n\\n circle_marker_289b0c5c0f1a82269c74acf396d5ba33.bindPopup(popup_ac9629900df3e92cb3a63593088b2943)\\n ;\\n\\n \\n \\n \\n var circle_marker_85751467283f989f192a014cc7bd449f = L.circleMarker(\\n [42.73455079731904, -73.25380030543215],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_36621137c20785da5d78ae6036aaa6f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f67c24acd8f64b34a22fa5602e82aea0 = $(`<div id="html_f67c24acd8f64b34a22fa5602e82aea0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_36621137c20785da5d78ae6036aaa6f5.setContent(html_f67c24acd8f64b34a22fa5602e82aea0);\\n \\n \\n\\n circle_marker_85751467283f989f192a014cc7bd449f.bindPopup(popup_36621137c20785da5d78ae6036aaa6f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a2399f5944b43311f1d6258e22b16326 = L.circleMarker(\\n [42.73455067223233, -73.25257013540256],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7bc5089ee4442589852470130cc9559f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4bf8da04a2685483c53cd678066c640 = $(`<div id="html_a4bf8da04a2685483c53cd678066c640" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7bc5089ee4442589852470130cc9559f.setContent(html_a4bf8da04a2685483c53cd678066c640);\\n \\n \\n\\n circle_marker_a2399f5944b43311f1d6258e22b16326.bindPopup(popup_7bc5089ee4442589852470130cc9559f)\\n ;\\n\\n \\n \\n \\n var circle_marker_5b5272684c296a50e49e1d200b8c4498 = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bf15baf106a4065c34b0625f503dc028 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33f904249e4384d2e2c7c61f565ccb61 = $(`<div id="html_33f904249e4384d2e2c7c61f565ccb61" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bf15baf106a4065c34b0625f503dc028.setContent(html_33f904249e4384d2e2c7c61f565ccb61);\\n \\n \\n\\n circle_marker_5b5272684c296a50e49e1d200b8c4498.bindPopup(popup_bf15baf106a4065c34b0625f503dc028)\\n ;\\n\\n \\n \\n \\n var circle_marker_ab66c7e2fec4d54e6e4634963b88de7a = L.circleMarker(\\n [42.7345503825579, -73.25010979535962],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b20ea996be5f59c71c54736076df9de7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09030e0fa68114a98260bd683970cf22 = $(`<div id="html_09030e0fa68114a98260bd683970cf22" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b20ea996be5f59c71c54736076df9de7.setContent(html_09030e0fa68114a98260bd683970cf22);\\n \\n \\n\\n circle_marker_ab66c7e2fec4d54e6e4634963b88de7a.bindPopup(popup_b20ea996be5f59c71c54736076df9de7)\\n ;\\n\\n \\n \\n \\n var circle_marker_ba55cc543c8e7d2113f743d0d1d0ace1 = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1875889617caf67e90e322efabc9bcb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5ba40f23ffcf8010c9238b80847e118a = $(`<div id="html_5ba40f23ffcf8010c9238b80847e118a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1875889617caf67e90e322efabc9bcb2.setContent(html_5ba40f23ffcf8010c9238b80847e118a);\\n \\n \\n\\n circle_marker_ba55cc543c8e7d2113f743d0d1d0ace1.bindPopup(popup_1875889617caf67e90e322efabc9bcb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_5885cad3da143311dbe9bde1291e791b = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d8abebca3b97e4ccff65a6634f825086 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff49d6aff93ed38a58ca0316b8cfe09d = $(`<div id="html_ff49d6aff93ed38a58ca0316b8cfe09d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8abebca3b97e4ccff65a6634f825086.setContent(html_ff49d6aff93ed38a58ca0316b8cfe09d);\\n \\n \\n\\n circle_marker_5885cad3da143311dbe9bde1291e791b.bindPopup(popup_d8abebca3b97e4ccff65a6634f825086)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6b2d22a6524b68c1d153402fe290dc5 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a8682f45fc8a5847695311f279384492 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a45ef2e844094d77c62b438e3c143506 = $(`<div id="html_a45ef2e844094d77c62b438e3c143506" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8682f45fc8a5847695311f279384492.setContent(html_a45ef2e844094d77c62b438e3c143506);\\n \\n \\n\\n circle_marker_f6b2d22a6524b68c1d153402fe290dc5.bindPopup(popup_a8682f45fc8a5847695311f279384492)\\n ;\\n\\n \\n \\n \\n var circle_marker_7144c2564d0af768555f1703caab7c19 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.826519928662906, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ffa4bf6d4c780a07b82c3c9b059c1eb5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_19fa093dea25b710d2eee642d6d195c3 = $(`<div id="html_19fa093dea25b710d2eee642d6d195c3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ffa4bf6d4c780a07b82c3c9b059c1eb5.setContent(html_19fa093dea25b710d2eee642d6d195c3);\\n \\n \\n\\n circle_marker_7144c2564d0af768555f1703caab7c19.bindPopup(popup_ffa4bf6d4c780a07b82c3c9b059c1eb5)\\n ;\\n\\n \\n \\n \\n var circle_marker_529aa17cc3a228db9eeefdf3a39edc5d = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3fe2fe6b8eabf7a167e7eebe90e40f98 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_951ec6c3f7264fa06a41d6fcfe7b9b46 = $(`<div id="html_951ec6c3f7264fa06a41d6fcfe7b9b46" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3fe2fe6b8eabf7a167e7eebe90e40f98.setContent(html_951ec6c3f7264fa06a41d6fcfe7b9b46);\\n \\n \\n\\n circle_marker_529aa17cc3a228db9eeefdf3a39edc5d.bindPopup(popup_3fe2fe6b8eabf7a167e7eebe90e40f98)\\n ;\\n\\n \\n \\n \\n var circle_marker_195a6e624344fd89f3d5c9438cea2b18 = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8521946a1cb9e83ebf2f96164894514f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e85f3e424948460865b76a09c0e5def5 = $(`<div id="html_e85f3e424948460865b76a09c0e5def5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8521946a1cb9e83ebf2f96164894514f.setContent(html_e85f3e424948460865b76a09c0e5def5);\\n \\n \\n\\n circle_marker_195a6e624344fd89f3d5c9438cea2b18.bindPopup(popup_8521946a1cb9e83ebf2f96164894514f)\\n ;\\n\\n \\n \\n \\n var circle_marker_7450833c99cae73c63f76301bbf22b9a = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_62def0d2a36d9ee9be7b4f0cb602ac95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_263e3d57bd1b85a24bd1e316e5b2092c = $(`<div id="html_263e3d57bd1b85a24bd1e316e5b2092c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_62def0d2a36d9ee9be7b4f0cb602ac95.setContent(html_263e3d57bd1b85a24bd1e316e5b2092c);\\n \\n \\n\\n circle_marker_7450833c99cae73c63f76301bbf22b9a.bindPopup(popup_62def0d2a36d9ee9be7b4f0cb602ac95)\\n ;\\n\\n \\n \\n \\n var circle_marker_43e1f8a079ab6767caf869c41d1c5546 = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_12e8ee166fef45ac8e12e8ba209aafe6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36d284fcbd2d361ce8c06a86b1427a0f = $(`<div id="html_36d284fcbd2d361ce8c06a86b1427a0f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_12e8ee166fef45ac8e12e8ba209aafe6.setContent(html_36d284fcbd2d361ce8c06a86b1427a0f);\\n \\n \\n\\n circle_marker_43e1f8a079ab6767caf869c41d1c5546.bindPopup(popup_12e8ee166fef45ac8e12e8ba209aafe6)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e3a6f8eb811b365b3ca0f35a867c90d = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_71f512325901918e16b1038c7d4a125b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7640d2f5c352390a510afe65167d1c19 = $(`<div id="html_7640d2f5c352390a510afe65167d1c19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71f512325901918e16b1038c7d4a125b.setContent(html_7640d2f5c352390a510afe65167d1c19);\\n \\n \\n\\n circle_marker_7e3a6f8eb811b365b3ca0f35a867c90d.bindPopup(popup_71f512325901918e16b1038c7d4a125b)\\n ;\\n\\n \\n \\n \\n var circle_marker_4136ce0f7e54381a95b42d86fdfd85dd = L.circleMarker(\\n [42.73454540542456, -73.22796673660297],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_64a5f017f2c2c44b6bb796c978d0b6e9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37106974258f857e3e175801daf96da2 = $(`<div id="html_37106974258f857e3e175801daf96da2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64a5f017f2c2c44b6bb796c978d0b6e9.setContent(html_37106974258f857e3e175801daf96da2);\\n \\n \\n\\n circle_marker_4136ce0f7e54381a95b42d86fdfd85dd.bindPopup(popup_64a5f017f2c2c44b6bb796c978d0b6e9)\\n ;\\n\\n \\n \\n \\n var circle_marker_7279c5ed71aa8c33be4491f17402737d = L.circleMarker(\\n [42.7345450038305, -73.22673656679281],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f99c7c988f4efe794a7bdb9bebc3eac9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8864cdf8523f74289640f281877891d0 = $(`<div id="html_8864cdf8523f74289640f281877891d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f99c7c988f4efe794a7bdb9bebc3eac9.setContent(html_8864cdf8523f74289640f281877891d0);\\n \\n \\n\\n circle_marker_7279c5ed71aa8c33be4491f17402737d.bindPopup(popup_f99c7c988f4efe794a7bdb9bebc3eac9)\\n ;\\n\\n \\n \\n \\n var circle_marker_04f82e44ee0142d654e2d2c95a064509 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_355f49cc4ea17892b9542eb7952d84f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97aa4333eb16f6e0d51acf51bdc2eca4 = $(`<div id="html_97aa4333eb16f6e0d51acf51bdc2eca4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_355f49cc4ea17892b9542eb7952d84f8.setContent(html_97aa4333eb16f6e0d51acf51bdc2eca4);\\n \\n \\n\\n circle_marker_04f82e44ee0142d654e2d2c95a064509.bindPopup(popup_355f49cc4ea17892b9542eb7952d84f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb484d495b13b388abd750d6f01843fd = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e6055bc4f5dc4edfdce9df08dea15ba2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c2412aefcad24f8aa070b43ae6a8f65a = $(`<div id="html_c2412aefcad24f8aa070b43ae6a8f65a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e6055bc4f5dc4edfdce9df08dea15ba2.setContent(html_c2412aefcad24f8aa070b43ae6a8f65a);\\n \\n \\n\\n circle_marker_eb484d495b13b388abd750d6f01843fd.bindPopup(popup_e6055bc4f5dc4edfdce9df08dea15ba2)\\n ;\\n\\n \\n \\n \\n var circle_marker_f30b659f78da5ebb3823b84ede4e373a = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_55eca942b3c479867b2a6729c492a12e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8debb5fa92cc65fe99dac3ae5ceda2ae = $(`<div id="html_8debb5fa92cc65fe99dac3ae5ceda2ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_55eca942b3c479867b2a6729c492a12e.setContent(html_8debb5fa92cc65fe99dac3ae5ceda2ae);\\n \\n \\n\\n circle_marker_f30b659f78da5ebb3823b84ede4e373a.bindPopup(popup_55eca942b3c479867b2a6729c492a12e)\\n ;\\n\\n \\n \\n \\n var circle_marker_e8f775eb41eca7cf49d37a2fc9573ef7 = L.circleMarker(\\n [42.73454279835495, -73.22058571799538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a3ec1ff84e6de0a2787ff391f8d8d73a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b0c6a3c1e198f01bd029b5c7a7629f5 = $(`<div id="html_8b0c6a3c1e198f01bd029b5c7a7629f5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a3ec1ff84e6de0a2787ff391f8d8d73a.setContent(html_8b0c6a3c1e198f01bd029b5c7a7629f5);\\n \\n \\n\\n circle_marker_e8f775eb41eca7cf49d37a2fc9573ef7.bindPopup(popup_a3ec1ff84e6de0a2787ff391f8d8d73a)\\n ;\\n\\n \\n \\n \\n var circle_marker_3496605a0b7dc2e9eb99e36f0a62dc0b = L.circleMarker(\\n [42.73274387463036, -73.27348277525046],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4cb134d2ef52c2e54aee761c1a178341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0a6922bc9164559b82a56821ad5c68de = $(`<div id="html_0a6922bc9164559b82a56821ad5c68de" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4cb134d2ef52c2e54aee761c1a178341.setContent(html_0a6922bc9164559b82a56821ad5c68de);\\n \\n \\n\\n circle_marker_3496605a0b7dc2e9eb99e36f0a62dc0b.bindPopup(popup_4cb134d2ef52c2e54aee761c1a178341)\\n ;\\n\\n \\n \\n \\n var circle_marker_5706dd703e13861df5461db9458be188 = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6ce647e3582e8868ceac778d58440159 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21c14eeb09a56d980e3667342796074c = $(`<div id="html_21c14eeb09a56d980e3667342796074c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ce647e3582e8868ceac778d58440159.setContent(html_21c14eeb09a56d980e3667342796074c);\\n \\n \\n\\n circle_marker_5706dd703e13861df5461db9458be188.bindPopup(popup_6ce647e3582e8868ceac778d58440159)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ef4c4b7090fc95ec0aa776111a2ff57 = L.circleMarker(\\n [42.7327440918774, -73.26979237266056],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7fddbad346b97d3125d3c3d4902222f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7323724563ce399e55153bd43dfd1131 = $(`<div id="html_7323724563ce399e55153bd43dfd1131" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7fddbad346b97d3125d3c3d4902222f6.setContent(html_7323724563ce399e55153bd43dfd1131);\\n \\n \\n\\n circle_marker_6ef4c4b7090fc95ec0aa776111a2ff57.bindPopup(popup_7fddbad346b97d3125d3c3d4902222f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_7f4b5bf9b5d5027e054d59d9072eaad3 = L.circleMarker(\\n [42.7327441379601, -73.26856223845904],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_566cc8c1870ec6ba86d8c6fbe11ee0d6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b58c0d9cb4a61fe8c4672f97c70508a = $(`<div id="html_8b58c0d9cb4a61fe8c4672f97c70508a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_566cc8c1870ec6ba86d8c6fbe11ee0d6.setContent(html_8b58c0d9cb4a61fe8c4672f97c70508a);\\n \\n \\n\\n circle_marker_7f4b5bf9b5d5027e054d59d9072eaad3.bindPopup(popup_566cc8c1870ec6ba86d8c6fbe11ee0d6)\\n ;\\n\\n \\n \\n \\n var circle_marker_e461675a37da8506fd5fe40e9712882e = L.circleMarker(\\n [42.73274417087632, -73.26733210425597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_08df69db26cedfc81d41dc6401105063 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_075ab3181ff9236d59dc2bf77eab7a70 = $(`<div id="html_075ab3181ff9236d59dc2bf77eab7a70" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_08df69db26cedfc81d41dc6401105063.setContent(html_075ab3181ff9236d59dc2bf77eab7a70);\\n \\n \\n\\n circle_marker_e461675a37da8506fd5fe40e9712882e.bindPopup(popup_08df69db26cedfc81d41dc6401105063)\\n ;\\n\\n \\n \\n \\n var circle_marker_e433dfce00e3c8ea93676b27b39bdbc2 = L.circleMarker(\\n [42.73274419062606, -73.26610197005185],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_67600fb369e19ea488764439a98d2437 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49ff4752e9c809300d8a469d08786dd8 = $(`<div id="html_49ff4752e9c809300d8a469d08786dd8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_67600fb369e19ea488764439a98d2437.setContent(html_49ff4752e9c809300d8a469d08786dd8);\\n \\n \\n\\n circle_marker_e433dfce00e3c8ea93676b27b39bdbc2.bindPopup(popup_67600fb369e19ea488764439a98d2437)\\n ;\\n\\n \\n \\n \\n var circle_marker_8cd94995075bf63dc02854b1725d4adf = L.circleMarker(\\n [42.7327441972093, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cdf6678066c31dd96b67e115068e9b90 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f63bb5b56420ccb067b601a5e83cad19 = $(`<div id="html_f63bb5b56420ccb067b601a5e83cad19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cdf6678066c31dd96b67e115068e9b90.setContent(html_f63bb5b56420ccb067b601a5e83cad19);\\n \\n \\n\\n circle_marker_8cd94995075bf63dc02854b1725d4adf.bindPopup(popup_cdf6678066c31dd96b67e115068e9b90)\\n ;\\n\\n \\n \\n \\n var circle_marker_797fcb3b4e169b576f12f1524ec19672 = L.circleMarker(\\n [42.73274419062606, -73.26364170164257],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7477a099fc81c0b159b385e85602bb69 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aff8b7dd60520c9cd93d1ab703ff616f = $(`<div id="html_aff8b7dd60520c9cd93d1ab703ff616f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7477a099fc81c0b159b385e85602bb69.setContent(html_aff8b7dd60520c9cd93d1ab703ff616f);\\n \\n \\n\\n circle_marker_797fcb3b4e169b576f12f1524ec19672.bindPopup(popup_7477a099fc81c0b159b385e85602bb69)\\n ;\\n\\n \\n \\n \\n var circle_marker_c6af3e3c4fe094cea5d5f8cad792e2ae = L.circleMarker(\\n [42.73274417087632, -73.26241156743845],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_47748f4b24d385eb030f8cb37a65e3df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38f668a41c8e96f884977198fb7bc05d = $(`<div id="html_38f668a41c8e96f884977198fb7bc05d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47748f4b24d385eb030f8cb37a65e3df.setContent(html_38f668a41c8e96f884977198fb7bc05d);\\n \\n \\n\\n circle_marker_c6af3e3c4fe094cea5d5f8cad792e2ae.bindPopup(popup_47748f4b24d385eb030f8cb37a65e3df)\\n ;\\n\\n \\n \\n \\n var circle_marker_cf373156b966faec1bce2224790e98a0 = L.circleMarker(\\n [42.7327441379601, -73.26118143323538],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3823a32da9080a0f35bfc77259691b19 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f18a4ea4cdc838b7f4f2ff6c21b3b386 = $(`<div id="html_f18a4ea4cdc838b7f4f2ff6c21b3b386" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3823a32da9080a0f35bfc77259691b19.setContent(html_f18a4ea4cdc838b7f4f2ff6c21b3b386);\\n \\n \\n\\n circle_marker_cf373156b966faec1bce2224790e98a0.bindPopup(popup_3823a32da9080a0f35bfc77259691b19)\\n ;\\n\\n \\n \\n \\n var circle_marker_7e78e34dad3ee87c0494de8829eb36e3 = L.circleMarker(\\n [42.73274403262821, -73.25872116483444],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4821e70967582d1077a32468cfedc878 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_332de755971b66a3a3b7a8002ffbac48 = $(`<div id="html_332de755971b66a3a3b7a8002ffbac48" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4821e70967582d1077a32468cfedc878.setContent(html_332de755971b66a3a3b7a8002ffbac48);\\n \\n \\n\\n circle_marker_7e78e34dad3ee87c0494de8829eb36e3.bindPopup(popup_4821e70967582d1077a32468cfedc878)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e7bd3bca44c8e2ece65ca414405774a = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_126f7c2bd9c65cec220418d86a157044 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3829347ab6fb2aa5b2c82d3e499a476d = $(`<div id="html_3829347ab6fb2aa5b2c82d3e499a476d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_126f7c2bd9c65cec220418d86a157044.setContent(html_3829347ab6fb2aa5b2c82d3e499a476d);\\n \\n \\n\\n circle_marker_9e7bd3bca44c8e2ece65ca414405774a.bindPopup(popup_126f7c2bd9c65cec220418d86a157044)\\n ;\\n\\n \\n \\n \\n var circle_marker_516ec49c628a0db273c66f5e9fbfb2e8 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9517b9797dbfc14c4a58838b028ca240 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50e01c402c3e9383be6317f91989c4da = $(`<div id="html_50e01c402c3e9383be6317f91989c4da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9517b9797dbfc14c4a58838b028ca240.setContent(html_50e01c402c3e9383be6317f91989c4da);\\n \\n \\n\\n circle_marker_516ec49c628a0db273c66f5e9fbfb2e8.bindPopup(popup_9517b9797dbfc14c4a58838b028ca240)\\n ;\\n\\n \\n \\n \\n var circle_marker_7423bbc9250892351f7702ce03c0ddba = L.circleMarker(\\n [42.73274377588172, -73.25503076225394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_defe12ff603aafedf8897735d9e9c255 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94cf2dc06ae0cce93b7ebab1def88f68 = $(`<div id="html_94cf2dc06ae0cce93b7ebab1def88f68" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_defe12ff603aafedf8897735d9e9c255.setContent(html_94cf2dc06ae0cce93b7ebab1def88f68);\\n \\n \\n\\n circle_marker_7423bbc9250892351f7702ce03c0ddba.bindPopup(popup_defe12ff603aafedf8897735d9e9c255)\\n ;\\n\\n \\n \\n \\n var circle_marker_02dbebaf0aa47b2a7f57e3c69135f177 = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d597428a200b45b9d73b9dccd4a24c1b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99748250ec65cdc313bf29ddeb8e5dc8 = $(`<div id="html_99748250ec65cdc313bf29ddeb8e5dc8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d597428a200b45b9d73b9dccd4a24c1b.setContent(html_99748250ec65cdc313bf29ddeb8e5dc8);\\n \\n \\n\\n circle_marker_02dbebaf0aa47b2a7f57e3c69135f177.bindPopup(popup_d597428a200b45b9d73b9dccd4a24c1b)\\n ;\\n\\n \\n \\n \\n var circle_marker_a03ba718e0136f19d62bc9ebee2941f3 = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_baea9bd3c93dee03dffbcaf0f3735fd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3244e0dab5b25427e501acc13623c704 = $(`<div id="html_3244e0dab5b25427e501acc13623c704" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_baea9bd3c93dee03dffbcaf0f3735fd0.setContent(html_3244e0dab5b25427e501acc13623c704);\\n \\n \\n\\n circle_marker_a03ba718e0136f19d62bc9ebee2941f3.bindPopup(popup_baea9bd3c93dee03dffbcaf0f3735fd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca00a83b958883b6b2b08bf03593c8e4 = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6e683b08d012b13a4dc4f0e8ce5ca848 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0dbe23ea75d644d226b5c22990ebc98e = $(`<div id="html_0dbe23ea75d644d226b5c22990ebc98e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e683b08d012b13a4dc4f0e8ce5ca848.setContent(html_0dbe23ea75d644d226b5c22990ebc98e);\\n \\n \\n\\n circle_marker_ca00a83b958883b6b2b08bf03593c8e4.bindPopup(popup_6e683b08d012b13a4dc4f0e8ce5ca848)\\n ;\\n\\n \\n \\n \\n var circle_marker_cfa494c07419bff4df68fb4405e49d5f = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_72cdf1826b01b3bd87e21ed130e28a88 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b7c210a83fe481e359e7d23e293fb05 = $(`<div id="html_2b7c210a83fe481e359e7d23e293fb05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72cdf1826b01b3bd87e21ed130e28a88.setContent(html_2b7c210a83fe481e359e7d23e293fb05);\\n \\n \\n\\n circle_marker_cfa494c07419bff4df68fb4405e49d5f.bindPopup(popup_72cdf1826b01b3bd87e21ed130e28a88)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3a0c03a31e5cda39416ae1c402801cb = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_91b35a3d32099b1e85620f30ba5c1832 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70c7755206cc459da939406eb3924984 = $(`<div id="html_70c7755206cc459da939406eb3924984" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91b35a3d32099b1e85620f30ba5c1832.setContent(html_70c7755206cc459da939406eb3924984);\\n \\n \\n\\n circle_marker_a3a0c03a31e5cda39416ae1c402801cb.bindPopup(popup_91b35a3d32099b1e85620f30ba5c1832)\\n ;\\n\\n \\n \\n \\n var circle_marker_bc41f145040b731d709aeae4b24bbb2a = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_65cae366525c2ec2f48b1f742e4f87ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5f41bb737d88671142b0e78b3fdd005 = $(`<div id="html_a5f41bb737d88671142b0e78b3fdd005" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_65cae366525c2ec2f48b1f742e4f87ee.setContent(html_a5f41bb737d88671142b0e78b3fdd005);\\n \\n \\n\\n circle_marker_bc41f145040b731d709aeae4b24bbb2a.bindPopup(popup_65cae366525c2ec2f48b1f742e4f87ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c60bc7973e496f67db3589efda6a101 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8263c856a8d8d86a3d231df8332fc8dd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2df06d4ddfef4f5190314053b52864b2 = $(`<div id="html_2df06d4ddfef4f5190314053b52864b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8263c856a8d8d86a3d231df8332fc8dd.setContent(html_2df06d4ddfef4f5190314053b52864b2);\\n \\n \\n\\n circle_marker_8c60bc7973e496f67db3589efda6a101.bindPopup(popup_8263c856a8d8d86a3d231df8332fc8dd)\\n ;\\n\\n \\n \\n \\n var circle_marker_ad272e9d3ee819b12122759c87bba64d = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2eb90a4ca8a0ac872bc25afdfd1f23c9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b0c19af275875ebdc8b3eff9fdf2401 = $(`<div id="html_2b0c19af275875ebdc8b3eff9fdf2401" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2eb90a4ca8a0ac872bc25afdfd1f23c9.setContent(html_2b0c19af275875ebdc8b3eff9fdf2401);\\n \\n \\n\\n circle_marker_ad272e9d3ee819b12122759c87bba64d.bindPopup(popup_2eb90a4ca8a0ac872bc25afdfd1f23c9)\\n ;\\n\\n \\n \\n \\n var circle_marker_d07fcca52e8df32a9e78b773b0ede4b4 = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a0e7704a1439fe85b0412de434e171c8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d71460a543ce399826bc5e953aca4c0e = $(`<div id="html_d71460a543ce399826bc5e953aca4c0e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a0e7704a1439fe85b0412de434e171c8.setContent(html_d71460a543ce399826bc5e953aca4c0e);\\n \\n \\n\\n circle_marker_d07fcca52e8df32a9e78b773b0ede4b4.bindPopup(popup_a0e7704a1439fe85b0412de434e171c8)\\n ;\\n\\n \\n \\n \\n var circle_marker_1cc223381625400c1f2471eec7dc7b76 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6b9d382f4b278b7fa3aba3d699955ed8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d9778c718d809639c332709b26910d1 = $(`<div id="html_3d9778c718d809639c332709b26910d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b9d382f4b278b7fa3aba3d699955ed8.setContent(html_3d9778c718d809639c332709b26910d1);\\n \\n \\n\\n circle_marker_1cc223381625400c1f2471eec7dc7b76.bindPopup(popup_6b9d382f4b278b7fa3aba3d699955ed8)\\n ;\\n\\n \\n \\n \\n var circle_marker_c775d12a1467794f629dca5d2e45f2a5 = L.circleMarker(\\n [42.73274129399903, -73.23903901835412],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8a06b117a535dd348234438236d3479d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8949726b13b5fc8f1330b652b7aad4d0 = $(`<div id="html_8949726b13b5fc8f1330b652b7aad4d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a06b117a535dd348234438236d3479d.setContent(html_8949726b13b5fc8f1330b652b7aad4d0);\\n \\n \\n\\n circle_marker_c775d12a1467794f629dca5d2e45f2a5.bindPopup(popup_8a06b117a535dd348234438236d3479d)\\n ;\\n\\n \\n \\n \\n var circle_marker_009515ccff897880a341c716371c6ed4 = L.circleMarker(\\n [42.73273702805773, -73.22427741021973],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ce209b1d461784d8d5ce7699bb2d0a0b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9b4aebb7b06072e001e99e47b9df69b = $(`<div id="html_f9b4aebb7b06072e001e99e47b9df69b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ce209b1d461784d8d5ce7699bb2d0a0b.setContent(html_f9b4aebb7b06072e001e99e47b9df69b);\\n \\n \\n\\n circle_marker_009515ccff897880a341c716371c6ed4.bindPopup(popup_ce209b1d461784d8d5ce7699bb2d0a0b)\\n ;\\n\\n \\n \\n \\n var circle_marker_1651e7e5876fa1123dae0a1d938af2ef = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cc49a105465e2056a26e2ea824de4c30 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9763b2fd309816ce3efc46617f0b097d = $(`<div id="html_9763b2fd309816ce3efc46617f0b097d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc49a105465e2056a26e2ea824de4c30.setContent(html_9763b2fd309816ce3efc46617f0b097d);\\n \\n \\n\\n circle_marker_1651e7e5876fa1123dae0a1d938af2ef.bindPopup(popup_cc49a105465e2056a26e2ea824de4c30)\\n ;\\n\\n \\n \\n \\n var circle_marker_b62762a55086f59d557753438e678ae9 = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_68f96675abeefbc4e957396878153597 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3d78d04b64c9d4f1b31b505f1be0510 = $(`<div id="html_d3d78d04b64c9d4f1b31b505f1be0510" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_68f96675abeefbc4e957396878153597.setContent(html_d3d78d04b64c9d4f1b31b505f1be0510);\\n \\n \\n\\n circle_marker_b62762a55086f59d557753438e678ae9.bindPopup(popup_68f96675abeefbc4e957396878153597)\\n ;\\n\\n \\n \\n \\n var circle_marker_34a51c3a373de44cb06b6357eebe8e93 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3e78948f55561ecc079143b93e94d5b9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c70dedd0d2dd1c4744905ae7768c165e = $(`<div id="html_c70dedd0d2dd1c4744905ae7768c165e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3e78948f55561ecc079143b93e94d5b9.setContent(html_c70dedd0d2dd1c4744905ae7768c165e);\\n \\n \\n\\n circle_marker_34a51c3a373de44cb06b6357eebe8e93.bindPopup(popup_3e78948f55561ecc079143b93e94d5b9)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0665e7dd6534abe412fda43b5567724 = L.circleMarker(\\n [42.73093705725226, -73.26610193420632],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1593488de465e2f96a1c6779f1e8c122 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3900d77405975e09a8aad84db3e3201 = $(`<div id="html_d3900d77405975e09a8aad84db3e3201" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1593488de465e2f96a1c6779f1e8c122.setContent(html_d3900d77405975e09a8aad84db3e3201);\\n \\n \\n\\n circle_marker_d0665e7dd6534abe412fda43b5567724.bindPopup(popup_1593488de465e2f96a1c6779f1e8c122)\\n ;\\n\\n \\n \\n \\n var circle_marker_f986ab838923bf398b0241d3291be3fe = L.circleMarker(\\n [42.73093706383524, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6cda674232ec6f0fbd379c88f0210b31 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d2f62f46511f8035b1ab61d4fe1a44c = $(`<div id="html_0d2f62f46511f8035b1ab61d4fe1a44c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6cda674232ec6f0fbd379c88f0210b31.setContent(html_0d2f62f46511f8035b1ab61d4fe1a44c);\\n \\n \\n\\n circle_marker_f986ab838923bf398b0241d3291be3fe.bindPopup(popup_6cda674232ec6f0fbd379c88f0210b31)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d2b38be59381f6ee5c75bfb42bc5524 = L.circleMarker(\\n [42.73093705725226, -73.2636417374881],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0b30fb0f9df31d4a61ef4f9104738d65 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_511f2640167ed4980815c4ac27ba4b25 = $(`<div id="html_511f2640167ed4980815c4ac27ba4b25" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b30fb0f9df31d4a61ef4f9104738d65.setContent(html_511f2640167ed4980815c4ac27ba4b25);\\n \\n \\n\\n circle_marker_0d2b38be59381f6ee5c75bfb42bc5524.bindPopup(popup_0b30fb0f9df31d4a61ef4f9104738d65)\\n ;\\n\\n \\n \\n \\n var circle_marker_c950c6f14ec1a446363374987bd0d6a0 = L.circleMarker(\\n [42.73093703750333, -73.26241163912951],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1e4cc373c58d1568b8ae54f7e27ca4e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a10ee0dd6e21d47374be36ff23b771a = $(`<div id="html_4a10ee0dd6e21d47374be36ff23b771a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1e4cc373c58d1568b8ae54f7e27ca4e4.setContent(html_4a10ee0dd6e21d47374be36ff23b771a);\\n \\n \\n\\n circle_marker_c950c6f14ec1a446363374987bd0d6a0.bindPopup(popup_1e4cc373c58d1568b8ae54f7e27ca4e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_fea4056f276040fa98b70c74d36c46c5 = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3af2352e7079d90d98b3e3caeac7f01f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_956d5a6afe0e00df127def715516eae1 = $(`<div id="html_956d5a6afe0e00df127def715516eae1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3af2352e7079d90d98b3e3caeac7f01f.setContent(html_956d5a6afe0e00df127def715516eae1);\\n \\n \\n\\n circle_marker_fea4056f276040fa98b70c74d36c46c5.bindPopup(popup_3af2352e7079d90d98b3e3caeac7f01f)\\n ;\\n\\n \\n \\n \\n var circle_marker_c7ad453ffab716503bab1e3f113b0cd5 = L.circleMarker(\\n [42.730936958507606, -73.259951442416],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ec6f43e96c2767bb51a5d4ad3ebdd549 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_540ca5687cb99fd3049d20f6bb414252 = $(`<div id="html_540ca5687cb99fd3049d20f6bb414252" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec6f43e96c2767bb51a5d4ad3ebdd549.setContent(html_540ca5687cb99fd3049d20f6bb414252);\\n \\n \\n\\n circle_marker_c7ad453ffab716503bab1e3f113b0cd5.bindPopup(popup_ec6f43e96c2767bb51a5d4ad3ebdd549)\\n ;\\n\\n \\n \\n \\n var circle_marker_947225f0f7f86c28c94a039be0f54466 = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e1429df410fc517a77944080e508be3e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_542d4281ee2d0d0929108a1f7605db6a = $(`<div id="html_542d4281ee2d0d0929108a1f7605db6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1429df410fc517a77944080e508be3e.setContent(html_542d4281ee2d0d0929108a1f7605db6a);\\n \\n \\n\\n circle_marker_947225f0f7f86c28c94a039be0f54466.bindPopup(popup_e1429df410fc517a77944080e508be3e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d105d682866ad2f84e6b69f105df3fd = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7839f127b2f2d94bc71f9dbaa9183173 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e82b5ea6cdade1c24c4e3ab37a29a367 = $(`<div id="html_e82b5ea6cdade1c24c4e3ab37a29a367" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7839f127b2f2d94bc71f9dbaa9183173.setContent(html_e82b5ea6cdade1c24c4e3ab37a29a367);\\n \\n \\n\\n circle_marker_6d105d682866ad2f84e6b69f105df3fd.bindPopup(popup_7839f127b2f2d94bc71f9dbaa9183173)\\n ;\\n\\n \\n \\n \\n var circle_marker_2178fe35bf27e0a0823229f6d8ed4ae9 = L.circleMarker(\\n [42.73093674126938, -73.25626114736271],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5f05f3cf837f6860b29627bcd7bf28ae = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0e03e3bf882583eb51d97b1ba6f5840c = $(`<div id="html_0e03e3bf882583eb51d97b1ba6f5840c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5f05f3cf837f6860b29627bcd7bf28ae.setContent(html_0e03e3bf882583eb51d97b1ba6f5840c);\\n \\n \\n\\n circle_marker_2178fe35bf27e0a0823229f6d8ed4ae9.bindPopup(popup_5f05f3cf837f6860b29627bcd7bf28ae)\\n ;\\n\\n \\n \\n \\n var circle_marker_98b4463aaa1bdb188061a250ef7aa4ee = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c698c4ecd117887c9c7ea7b8ca15c4ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_266feca3c8c1f32e3fb13a0808934ac2 = $(`<div id="html_266feca3c8c1f32e3fb13a0808934ac2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c698c4ecd117887c9c7ea7b8ca15c4ed.setContent(html_266feca3c8c1f32e3fb13a0808934ac2);\\n \\n \\n\\n circle_marker_98b4463aaa1bdb188061a250ef7aa4ee.bindPopup(popup_c698c4ecd117887c9c7ea7b8ca15c4ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc59a79ec79d265788316c48fd4673b7 = L.circleMarker(\\n [42.73093640553757, -73.2525708523423],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1dbadaecdc422908b58a3d936ce5cc16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5af2d175fcdd677c276432fc579d2e36 = $(`<div id="html_5af2d175fcdd677c276432fc579d2e36" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1dbadaecdc422908b58a3d936ce5cc16.setContent(html_5af2d175fcdd677c276432fc579d2e36);\\n \\n \\n\\n circle_marker_dc59a79ec79d265788316c48fd4673b7.bindPopup(popup_1dbadaecdc422908b58a3d936ce5cc16)\\n ;\\n\\n \\n \\n \\n var circle_marker_56103f61868fac8462f12247c2733dfb = L.circleMarker(\\n [42.73093626729506, -73.25134075401192],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_97b810ab6a05499eb852086857992af5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9ededced9f0348e9700c20610ef2195b = $(`<div id="html_9ededced9f0348e9700c20610ef2195b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97b810ab6a05499eb852086857992af5.setContent(html_9ededced9f0348e9700c20610ef2195b);\\n \\n \\n\\n circle_marker_56103f61868fac8462f12247c2733dfb.bindPopup(popup_97b810ab6a05499eb852086857992af5)\\n ;\\n\\n \\n \\n \\n var circle_marker_f6a2862e5ed9de00287cb2f513a7d0a9 = L.circleMarker(\\n [42.73093611588659, -73.2501106556873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7b594dfe47e5905574c4b6caddb1d1d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_26b797bfced97d5741020e401b9e8255 = $(`<div id="html_26b797bfced97d5741020e401b9e8255" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7b594dfe47e5905574c4b6caddb1d1d7.setContent(html_26b797bfced97d5741020e401b9e8255);\\n \\n \\n\\n circle_marker_f6a2862e5ed9de00287cb2f513a7d0a9.bindPopup(popup_7b594dfe47e5905574c4b6caddb1d1d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_b86907e393b9f1ced2c91264c8c2bb78 = L.circleMarker(\\n [42.73093595131219, -73.24888055736892],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_93d2ce2566e695de457869b17d376064 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ca8e4df701f18ae6ef52e40c7ea3f947 = $(`<div id="html_ca8e4df701f18ae6ef52e40c7ea3f947" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93d2ce2566e695de457869b17d376064.setContent(html_ca8e4df701f18ae6ef52e40c7ea3f947);\\n \\n \\n\\n circle_marker_b86907e393b9f1ced2c91264c8c2bb78.bindPopup(popup_93d2ce2566e695de457869b17d376064)\\n ;\\n\\n \\n \\n \\n var circle_marker_f71f5edc9db2f8293fb780c3a148801c = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_16f510969cc814643625f21feadbd876 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09d8e0828445ec8817c023f9ee688921 = $(`<div id="html_09d8e0828445ec8817c023f9ee688921" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16f510969cc814643625f21feadbd876.setContent(html_09d8e0828445ec8817c023f9ee688921);\\n \\n \\n\\n circle_marker_f71f5edc9db2f8293fb780c3a148801c.bindPopup(popup_16f510969cc814643625f21feadbd876)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f9e5323a799470f99ef52a1c0817736 = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_64c2a2d5de0d33eaf7ab0b49db128812 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b76518ec17a485394dd8dedaebc0437 = $(`<div id="html_4b76518ec17a485394dd8dedaebc0437" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64c2a2d5de0d33eaf7ab0b49db128812.setContent(html_4b76518ec17a485394dd8dedaebc0437);\\n \\n \\n\\n circle_marker_9f9e5323a799470f99ef52a1c0817736.bindPopup(popup_64c2a2d5de0d33eaf7ab0b49db128812)\\n ;\\n\\n \\n \\n \\n var circle_marker_31fb4d7ae07e9867f475d397b11fa759 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ae11819eacb6ea029f1b54f38c2941ee = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_facbc926084916ef4dd0b94400829f91 = $(`<div id="html_facbc926084916ef4dd0b94400829f91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ae11819eacb6ea029f1b54f38c2941ee.setContent(html_facbc926084916ef4dd0b94400829f91);\\n \\n \\n\\n circle_marker_31fb4d7ae07e9867f475d397b11fa759.bindPopup(popup_ae11819eacb6ea029f1b54f38c2941ee)\\n ;\\n\\n \\n \\n \\n var circle_marker_f0eb94c5aa8fd73dbba9d20835dc6e37 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_58860a4722478c100193a442b963c0a7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ecfb8495d4c53308f06d4563d6dfc38 = $(`<div id="html_1ecfb8495d4c53308f06d4563d6dfc38" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58860a4722478c100193a442b963c0a7.setContent(html_1ecfb8495d4c53308f06d4563d6dfc38);\\n \\n \\n\\n circle_marker_f0eb94c5aa8fd73dbba9d20835dc6e37.bindPopup(popup_58860a4722478c100193a442b963c0a7)\\n ;\\n\\n \\n \\n \\n var circle_marker_7263306fe9a6e563141ef18070bb571a = L.circleMarker(\\n [42.730934687380696, -73.24149996761956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_703c404d52408c765fe1be8fa0a069e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_125d31f8167813590ae5339116e6efce = $(`<div id="html_125d31f8167813590ae5339116e6efce" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_703c404d52408c765fe1be8fa0a069e4.setContent(html_125d31f8167813590ae5339116e6efce);\\n \\n \\n\\n circle_marker_7263306fe9a6e563141ef18070bb571a.bindPopup(popup_703c404d52408c765fe1be8fa0a069e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f2b6eb3b5b9db21028d1155f50992d5 = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e2ecb4aff2a7e09a18d6a0f9cc93e32a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58fe2de7c0bef447ae46aaeb19b45f20 = $(`<div id="html_58fe2de7c0bef447ae46aaeb19b45f20" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2ecb4aff2a7e09a18d6a0f9cc93e32a.setContent(html_58fe2de7c0bef447ae46aaeb19b45f20);\\n \\n \\n\\n circle_marker_6f2b6eb3b5b9db21028d1155f50992d5.bindPopup(popup_e2ecb4aff2a7e09a18d6a0f9cc93e32a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c07c8e091e4ae776529fdde5cbc425c8 = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_30c815c97f56293ed0abbcfb4877eed5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2428c8c6de4951ad9e1161de4c83809e = $(`<div id="html_2428c8c6de4951ad9e1161de4c83809e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_30c815c97f56293ed0abbcfb4877eed5.setContent(html_2428c8c6de4951ad9e1161de4c83809e);\\n \\n \\n\\n circle_marker_c07c8e091e4ae776529fdde5cbc425c8.bindPopup(popup_30c815c97f56293ed0abbcfb4877eed5)\\n ;\\n\\n \\n \\n \\n var circle_marker_dda8d4fca8e698f05826ae40a20a9b10 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3728ca2d4d0a7f4d56cee56d5cd37066 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_056659d3584dbf352b361319d97d9340 = $(`<div id="html_056659d3584dbf352b361319d97d9340" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3728ca2d4d0a7f4d56cee56d5cd37066.setContent(html_056659d3584dbf352b361319d97d9340);\\n \\n \\n\\n circle_marker_dda8d4fca8e698f05826ae40a20a9b10.bindPopup(popup_3728ca2d4d0a7f4d56cee56d5cd37066)\\n ;\\n\\n \\n \\n \\n var circle_marker_fc26feff34920608425898afc83a0883 = L.circleMarker(\\n [42.73093358144067, -73.23657957464485],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_47b62db7254cc8899d3226eddd0388bf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ccccb5575be5212e52705bc933f9c546 = $(`<div id="html_ccccb5575be5212e52705bc933f9c546" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_47b62db7254cc8899d3226eddd0388bf.setContent(html_ccccb5575be5212e52705bc933f9c546);\\n \\n \\n\\n circle_marker_fc26feff34920608425898afc83a0883.bindPopup(popup_47b62db7254cc8899d3226eddd0388bf)\\n ;\\n\\n \\n \\n \\n var circle_marker_316b3bad8e9924f89f13f6062ae9e329 = L.circleMarker(\\n [42.7309332720408, -73.23534947642989],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_be0b4d32ad8f150cb5b003ea8c461469 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_63ef7180e2f19be5d02927fbbabab1b3 = $(`<div id="html_63ef7180e2f19be5d02927fbbabab1b3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_be0b4d32ad8f150cb5b003ea8c461469.setContent(html_63ef7180e2f19be5d02927fbbabab1b3);\\n \\n \\n\\n circle_marker_316b3bad8e9924f89f13f6062ae9e329.bindPopup(popup_be0b4d32ad8f150cb5b003ea8c461469)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d6cfb4820a03f02193f53197a2af6bf = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8a09fc21c256b48ba13fed47b1bd8afa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2cc52f1be8c212299c18666e0dd5dc6f = $(`<div id="html_2cc52f1be8c212299c18666e0dd5dc6f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a09fc21c256b48ba13fed47b1bd8afa.setContent(html_2cc52f1be8c212299c18666e0dd5dc6f);\\n \\n \\n\\n circle_marker_5d6cfb4820a03f02193f53197a2af6bf.bindPopup(popup_8a09fc21c256b48ba13fed47b1bd8afa)\\n ;\\n\\n \\n \\n \\n var circle_marker_901353ac0f7f840a55e12aba3d5d152b = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a76ebb0bbab4d8a6839ff07a3a1dddd6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf924655bec723535fedc0071f1ec91c = $(`<div id="html_bf924655bec723535fedc0071f1ec91c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a76ebb0bbab4d8a6839ff07a3a1dddd6.setContent(html_bf924655bec723535fedc0071f1ec91c);\\n \\n \\n\\n circle_marker_901353ac0f7f840a55e12aba3d5d152b.bindPopup(popup_a76ebb0bbab4d8a6839ff07a3a1dddd6)\\n ;\\n\\n \\n \\n \\n var circle_marker_0d5a5c1b06439afccfb4eefafd07d7d6 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8ed0345ff87ca34ce23ea1fe24587fa4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_796a9c9e07d8c59c1d19e91e347768b5 = $(`<div id="html_796a9c9e07d8c59c1d19e91e347768b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ed0345ff87ca34ce23ea1fe24587fa4.setContent(html_796a9c9e07d8c59c1d19e91e347768b5);\\n \\n \\n\\n circle_marker_0d5a5c1b06439afccfb4eefafd07d7d6.bindPopup(popup_8ed0345ff87ca34ce23ea1fe24587fa4)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ae6c02cb985d62a70628278c7fed4c8 = L.circleMarker(\\n [42.73093073759504, -73.2267387893054],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_14e0fa3b6cc76ec8570f7f77fa30d78c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7dd9444becbffc809d23ec44a4c04e05 = $(`<div id="html_7dd9444becbffc809d23ec44a4c04e05" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_14e0fa3b6cc76ec8570f7f77fa30d78c.setContent(html_7dd9444becbffc809d23ec44a4c04e05);\\n \\n \\n\\n circle_marker_6ae6c02cb985d62a70628278c7fed4c8.bindPopup(popup_14e0fa3b6cc76ec8570f7f77fa30d78c)\\n ;\\n\\n \\n \\n \\n var circle_marker_0cd9300ca3e38d6889d7a9f197c4b191 = L.circleMarker(\\n [42.73092989497414, -73.22427859312202],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5b7adfcba3978d38cd1b1b4a29226c3b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_43a88cf70e300198b8218cf127709d26 = $(`<div id="html_43a88cf70e300198b8218cf127709d26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5b7adfcba3978d38cd1b1b4a29226c3b.setContent(html_43a88cf70e300198b8218cf127709d26);\\n \\n \\n\\n circle_marker_0cd9300ca3e38d6889d7a9f197c4b191.bindPopup(popup_5b7adfcba3978d38cd1b1b4a29226c3b)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4c47845840aadb702f97027b89d7c5b = L.circleMarker(\\n [42.73092945391477, -73.2230484950559],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2c202c9c83fefe413f3b7e6fe00e8331 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_54291b69e139a2d829d03fef397e0d81 = $(`<div id="html_54291b69e139a2d829d03fef397e0d81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2c202c9c83fefe413f3b7e6fe00e8331.setContent(html_54291b69e139a2d829d03fef397e0d81);\\n \\n \\n\\n circle_marker_e4c47845840aadb702f97027b89d7c5b.bindPopup(popup_2c202c9c83fefe413f3b7e6fe00e8331)\\n ;\\n\\n \\n \\n \\n var circle_marker_026f4e36f6def2e8abe6fa2038e67a4b = L.circleMarker(\\n [42.73092853229819, -73.2205882989775],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ae27f3c6b4a951ca591feaf7d6c69534 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e842732ab36ffabda7ae13d131ce77a = $(`<div id="html_8e842732ab36ffabda7ae13d131ce77a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ae27f3c6b4a951ca591feaf7d6c69534.setContent(html_8e842732ab36ffabda7ae13d131ce77a);\\n \\n \\n\\n circle_marker_026f4e36f6def2e8abe6fa2038e67a4b.bindPopup(popup_ae27f3c6b4a951ca591feaf7d6c69534)\\n ;\\n\\n \\n \\n \\n var circle_marker_79618f4c727358833efd0650fa3c1e0f = L.circleMarker(\\n [42.73092805174098, -73.21935820096624],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3fc874ebd3cda92487c2ab0bc3f62b72 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_65499537e5eb6d447837753d815908b7 = $(`<div id="html_65499537e5eb6d447837753d815908b7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3fc874ebd3cda92487c2ab0bc3f62b72.setContent(html_65499537e5eb6d447837753d815908b7);\\n \\n \\n\\n circle_marker_79618f4c727358833efd0650fa3c1e0f.bindPopup(popup_3fc874ebd3cda92487c2ab0bc3f62b72)\\n ;\\n\\n \\n \\n \\n var circle_marker_f9cea6119a4278f62732bc2e58b221ac = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4f7bab988daf6d384f770382cdf5244c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d234d7e995d61e9fd0807bd8e99ecbdf = $(`<div id="html_d234d7e995d61e9fd0807bd8e99ecbdf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f7bab988daf6d384f770382cdf5244c.setContent(html_d234d7e995d61e9fd0807bd8e99ecbdf);\\n \\n \\n\\n circle_marker_f9cea6119a4278f62732bc2e58b221ac.bindPopup(popup_4f7bab988daf6d384f770382cdf5244c)\\n ;\\n\\n \\n \\n \\n var circle_marker_da2626dbc4922d67d7735270697b09e7 = L.circleMarker(\\n [42.729129693483614, -73.27225221092779],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a65dd284c155a016836dc3af4b407960 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31bfb3f8f673684f98a78a60d52215bc = $(`<div id="html_31bfb3f8f673684f98a78a60d52215bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a65dd284c155a016836dc3af4b407960.setContent(html_31bfb3f8f673684f98a78a60d52215bc);\\n \\n \\n\\n circle_marker_da2626dbc4922d67d7735270697b09e7.bindPopup(popup_a65dd284c155a016836dc3af4b407960)\\n ;\\n\\n \\n \\n \\n var circle_marker_a69bd50de9c9603784a225fdf3f69089 = L.circleMarker(\\n [42.72912992387847, -73.26610189836369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_44b77c1a88ce6be58973b46f26ee25cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b87af03c34bfb1ea8ca2cd990d3639b = $(`<div id="html_2b87af03c34bfb1ea8ca2cd990d3639b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_44b77c1a88ce6be58973b46f26ee25cf.setContent(html_2b87af03c34bfb1ea8ca2cd990d3639b);\\n \\n \\n\\n circle_marker_a69bd50de9c9603784a225fdf3f69089.bindPopup(popup_44b77c1a88ce6be58973b46f26ee25cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb6c8685086d4b3d7f7d77352fae79e9 = L.circleMarker(\\n [42.72912992387847, -73.26364177333073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_31d8accc4d68eb29e2bb4c52738a5005 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4491f82a1315ccf8f14311f4748af9e2 = $(`<div id="html_4491f82a1315ccf8f14311f4748af9e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31d8accc4d68eb29e2bb4c52738a5005.setContent(html_4491f82a1315ccf8f14311f4748af9e2);\\n \\n \\n\\n circle_marker_fb6c8685086d4b3d7f7d77352fae79e9.bindPopup(popup_31d8accc4d68eb29e2bb4c52738a5005)\\n ;\\n\\n \\n \\n \\n var circle_marker_99c7baeac89b2db22dde64003ed17dce = L.circleMarker(\\n [42.72912990413034, -73.26241171081477],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b3b64207212265d09df82c1b6fe468b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c4cb21279e50b03bccc1fe19f706f8b = $(`<div id="html_1c4cb21279e50b03bccc1fe19f706f8b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b3b64207212265d09df82c1b6fe468b5.setContent(html_1c4cb21279e50b03bccc1fe19f706f8b);\\n \\n \\n\\n circle_marker_99c7baeac89b2db22dde64003ed17dce.bindPopup(popup_b3b64207212265d09df82c1b6fe468b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_9685d9aabc126d574a2f20f70ca1aebc = L.circleMarker(\\n [42.729129871216784, -73.26118164829987],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d2f24b0838ceb01c46f2ef81b8feb073 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0f84b59eba272a552442c90930aeb196 = $(`<div id="html_0f84b59eba272a552442c90930aeb196" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d2f24b0838ceb01c46f2ef81b8feb073.setContent(html_0f84b59eba272a552442c90930aeb196);\\n \\n \\n\\n circle_marker_9685d9aabc126d574a2f20f70ca1aebc.bindPopup(popup_d2f24b0838ceb01c46f2ef81b8feb073)\\n ;\\n\\n \\n \\n \\n var circle_marker_03a2c769b2f143ab3e9e6c46d0290480 = L.circleMarker(\\n [42.72912982513782, -73.25995158578652],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_52085af5377136556e7c273db8b447fc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24bfc6da6709410c1bc64de07a466f58 = $(`<div id="html_24bfc6da6709410c1bc64de07a466f58" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_52085af5377136556e7c273db8b447fc.setContent(html_24bfc6da6709410c1bc64de07a466f58);\\n \\n \\n\\n circle_marker_03a2c769b2f143ab3e9e6c46d0290480.bindPopup(popup_52085af5377136556e7c273db8b447fc)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9b33adfaa7ba4753acab4ac8ca8ca5b = L.circleMarker(\\n [42.72912976589343, -73.25872152327527],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_31d378846a61143baab149a1cbb0d8a3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8ced4745f2e028f416f151f8d7c42a7 = $(`<div id="html_f8ced4745f2e028f416f151f8d7c42a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_31d378846a61143baab149a1cbb0d8a3.setContent(html_f8ced4745f2e028f416f151f8d7c42a7);\\n \\n \\n\\n circle_marker_a9b33adfaa7ba4753acab4ac8ca8ca5b.bindPopup(popup_31d378846a61143baab149a1cbb0d8a3)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f4e83082fb328ec5ccb6f28fd76eaa1 = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5363614516c0e02c75a930770c69e2f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d462d9fe6682ca4747358422da9e3d6f = $(`<div id="html_d462d9fe6682ca4747358422da9e3d6f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5363614516c0e02c75a930770c69e2f4.setContent(html_d462d9fe6682ca4747358422da9e3d6f);\\n \\n \\n\\n circle_marker_2f4e83082fb328ec5ccb6f28fd76eaa1.bindPopup(popup_5363614516c0e02c75a930770c69e2f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_67c75a0fbdd294947a6bcc243579c307 = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e41fd70f1cff4921662dcc998af5cf07 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_059232c60f9d7c52840b51a6f85081df = $(`<div id="html_059232c60f9d7c52840b51a6f85081df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e41fd70f1cff4921662dcc998af5cf07.setContent(html_059232c60f9d7c52840b51a6f85081df);\\n \\n \\n\\n circle_marker_67c75a0fbdd294947a6bcc243579c307.bindPopup(popup_e41fd70f1cff4921662dcc998af5cf07)\\n ;\\n\\n \\n \\n \\n var circle_marker_51ebc6c37156131de7ce88c04ad96956 = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8a2dab04fdbb12e2cf8e7cbe5162d3ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_24cb10fa36df4ce2081b06487a07073c = $(`<div id="html_24cb10fa36df4ce2081b06487a07073c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8a2dab04fdbb12e2cf8e7cbe5162d3ed.setContent(html_24cb10fa36df4ce2081b06487a07073c);\\n \\n \\n\\n circle_marker_51ebc6c37156131de7ce88c04ad96956.bindPopup(popup_8a2dab04fdbb12e2cf8e7cbe5162d3ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_62c00b1a4e569a0cf1c422016791df1f = L.circleMarker(\\n [42.72912939726166, -73.25380127326159],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_072001bf588be9256c05f7021f3c763c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3dbcc865eb31ec28ace1c1933503bcad = $(`<div id="html_3dbcc865eb31ec28ace1c1933503bcad" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_072001bf588be9256c05f7021f3c763c.setContent(html_3dbcc865eb31ec28ace1c1933503bcad);\\n \\n \\n\\n circle_marker_62c00b1a4e569a0cf1c422016791df1f.bindPopup(popup_072001bf588be9256c05f7021f3c763c)\\n ;\\n\\n \\n \\n \\n var circle_marker_a3dcf911eeace1701ed1cc7cbf0f7767 = L.circleMarker(\\n [42.72912927219017, -73.25257121076861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_01fcc9ee1e7e1932b04683b9d7906029 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a994d93d83e4a4366d49aa4570db82f1 = $(`<div id="html_a994d93d83e4a4366d49aa4570db82f1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_01fcc9ee1e7e1932b04683b9d7906029.setContent(html_a994d93d83e4a4366d49aa4570db82f1);\\n \\n \\n\\n circle_marker_a3dcf911eeace1701ed1cc7cbf0f7767.bindPopup(popup_01fcc9ee1e7e1932b04683b9d7906029)\\n ;\\n\\n \\n \\n \\n var circle_marker_22e9b274821d60fba9ee9c5585097261 = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0e0c8cf18c2dfdb631e49a7c1a00b0ca = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_556436ddd47bc0625cd201e66c5677bb = $(`<div id="html_556436ddd47bc0625cd201e66c5677bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e0c8cf18c2dfdb631e49a7c1a00b0ca.setContent(html_556436ddd47bc0625cd201e66c5677bb);\\n \\n \\n\\n circle_marker_22e9b274821d60fba9ee9c5585097261.bindPopup(popup_0e0c8cf18c2dfdb631e49a7c1a00b0ca)\\n ;\\n\\n \\n \\n \\n var circle_marker_6a0e07e5db4aa4497e69315efcfac3c6 = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a777f23b6a8eff598594787940f0ea73 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7aa194d6056044ce30832712ff2c6259 = $(`<div id="html_7aa194d6056044ce30832712ff2c6259" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a777f23b6a8eff598594787940f0ea73.setContent(html_7aa194d6056044ce30832712ff2c6259);\\n \\n \\n\\n circle_marker_6a0e07e5db4aa4497e69315efcfac3c6.bindPopup(popup_a777f23b6a8eff598594787940f0ea73)\\n ;\\n\\n \\n \\n \\n var circle_marker_9ad554b54951bc0c0f30da89cc4597dd = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_42898ad26babb0075ffda801a0fe5ed0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5e4ca057b87a2d90c3dd25a0de805ec8 = $(`<div id="html_5e4ca057b87a2d90c3dd25a0de805ec8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_42898ad26babb0075ffda801a0fe5ed0.setContent(html_5e4ca057b87a2d90c3dd25a0de805ec8);\\n \\n \\n\\n circle_marker_9ad554b54951bc0c0f30da89cc4597dd.bindPopup(popup_42898ad26babb0075ffda801a0fe5ed0)\\n ;\\n\\n \\n \\n \\n var circle_marker_03878a4296fe9925dd5b2065f1f95567 = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d64bfe0f8ab5f3e97d62fa86c3c7fbcc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2c72dd3c73bc38c7e45effd1af19e54 = $(`<div id="html_f2c72dd3c73bc38c7e45effd1af19e54" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d64bfe0f8ab5f3e97d62fa86c3c7fbcc.setContent(html_f2c72dd3c73bc38c7e45effd1af19e54);\\n \\n \\n\\n circle_marker_03878a4296fe9925dd5b2065f1f95567.bindPopup(popup_d64bfe0f8ab5f3e97d62fa86c3c7fbcc)\\n ;\\n\\n \\n \\n \\n var circle_marker_46c9a13438860bfc2682e532013fd431 = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6d63ebe809e4cac14fd273b55bddbc7a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7b7e1557bba447aebae70b0ffe5a9db2 = $(`<div id="html_7b7e1557bba447aebae70b0ffe5a9db2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d63ebe809e4cac14fd273b55bddbc7a.setContent(html_7b7e1557bba447aebae70b0ffe5a9db2);\\n \\n \\n\\n circle_marker_46c9a13438860bfc2682e532013fd431.bindPopup(popup_6d63ebe809e4cac14fd273b55bddbc7a)\\n ;\\n\\n \\n \\n \\n var circle_marker_065705600345801b06cc5d695e25dc82 = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bdfac85313f3e7abf62fca27c49f2734 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fa0b277d33178040d9f86a3d3ec68a2b = $(`<div id="html_fa0b277d33178040d9f86a3d3ec68a2b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdfac85313f3e7abf62fca27c49f2734.setContent(html_fa0b277d33178040d9f86a3d3ec68a2b);\\n \\n \\n\\n circle_marker_065705600345801b06cc5d695e25dc82.bindPopup(popup_bdfac85313f3e7abf62fca27c49f2734)\\n ;\\n\\n \\n \\n \\n var circle_marker_000ffa6cac3ed4c0b1598233a9b1aa99 = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b5e9e9f7d7cc6cffe4e2c94f0cd08426 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec27f1380b32750c0a4fd34e0937a00a = $(`<div id="html_ec27f1380b32750c0a4fd34e0937a00a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5e9e9f7d7cc6cffe4e2c94f0cd08426.setContent(html_ec27f1380b32750c0a4fd34e0937a00a);\\n \\n \\n\\n circle_marker_000ffa6cac3ed4c0b1598233a9b1aa99.bindPopup(popup_b5e9e9f7d7cc6cffe4e2c94f0cd08426)\\n ;\\n\\n \\n \\n \\n var circle_marker_716c8c9687b965a31dc8e14cfca411ba = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4e67e70d64ec089063cb7b576536d2f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6db62446b82a6c9999222daa378dbdbb = $(`<div id="html_6db62446b82a6c9999222daa378dbdbb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e67e70d64ec089063cb7b576536d2f0.setContent(html_6db62446b82a6c9999222daa378dbdbb);\\n \\n \\n\\n circle_marker_716c8c9687b965a31dc8e14cfca411ba.bindPopup(popup_4e67e70d64ec089063cb7b576536d2f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_a7fdc778788b1ff067efb9ead4eedb4e = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1c87b0fa18a2f9d2af42c8396f75bdac = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a36b5ff6f29b1485266152f48452969b = $(`<div id="html_a36b5ff6f29b1485266152f48452969b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1c87b0fa18a2f9d2af42c8396f75bdac.setContent(html_a36b5ff6f29b1485266152f48452969b);\\n \\n \\n\\n circle_marker_a7fdc778788b1ff067efb9ead4eedb4e.bindPopup(popup_1c87b0fa18a2f9d2af42c8396f75bdac)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d42be4cc9a7ccbf081c0d9b80ba45b7 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bcadc8400f81f3ea539f13615019d372 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a9189170edc5249cb7c917f191c5d4f2 = $(`<div id="html_a9189170edc5249cb7c917f191c5d4f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bcadc8400f81f3ea539f13615019d372.setContent(html_a9189170edc5249cb7c917f191c5d4f2);\\n \\n \\n\\n circle_marker_9d42be4cc9a7ccbf081c0d9b80ba45b7.bindPopup(popup_bcadc8400f81f3ea539f13615019d372)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4588569cff77d7ce033ab21d3a08211 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_19b9436e38ea4f3b97803f3625a5babf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_28f9768d38e00fe56060712c1ae292e8 = $(`<div id="html_28f9768d38e00fe56060712c1ae292e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19b9436e38ea4f3b97803f3625a5babf.setContent(html_28f9768d38e00fe56060712c1ae292e8);\\n \\n \\n\\n circle_marker_f4588569cff77d7ce033ab21d3a08211.bindPopup(popup_19b9436e38ea4f3b97803f3625a5babf)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e6ae3fa49ca1ce5ef4981b2f8f5a9ff = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4ca2154f4a4dcc11ff131f38b75c10a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2eccb62bc03210553f07bb425ceec619 = $(`<div id="html_2eccb62bc03210553f07bb425ceec619" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ca2154f4a4dcc11ff131f38b75c10a4.setContent(html_2eccb62bc03210553f07bb425ceec619);\\n \\n \\n\\n circle_marker_1e6ae3fa49ca1ce5ef4981b2f8f5a9ff.bindPopup(popup_4ca2154f4a4dcc11ff131f38b75c10a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_30a289a2d822541c9aefe5b04062e840 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b611c68216d8769271b2a7f3c234814e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1bf7d8be9dca5d293eaa61aedcbda86e = $(`<div id="html_1bf7d8be9dca5d293eaa61aedcbda86e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b611c68216d8769271b2a7f3c234814e.setContent(html_1bf7d8be9dca5d293eaa61aedcbda86e);\\n \\n \\n\\n circle_marker_30a289a2d822541c9aefe5b04062e840.bindPopup(popup_b611c68216d8769271b2a7f3c234814e)\\n ;\\n\\n \\n \\n \\n var circle_marker_468d5cd15dcf8d6614ef87a11f886cb7 = L.circleMarker(\\n [42.7291258162676, -73.23412027429306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ba3e7800652b9eb6272e20d3cfaee994 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb9e9e6839e2ec82011c72e9ecd304bc = $(`<div id="html_cb9e9e6839e2ec82011c72e9ecd304bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ba3e7800652b9eb6272e20d3cfaee994.setContent(html_cb9e9e6839e2ec82011c72e9ecd304bc);\\n \\n \\n\\n circle_marker_468d5cd15dcf8d6614ef87a11f886cb7.bindPopup(popup_ba3e7800652b9eb6272e20d3cfaee994)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c2a49708094e2a755666b4bddc5e1cd = L.circleMarker(\\n [42.72912548054941, -73.23289021194631],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ea18b718d44ddbf310e7e29e9e0dc9e3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7aa68cb04d4e3a2a63b23409a12ae9e8 = $(`<div id="html_7aa68cb04d4e3a2a63b23409a12ae9e8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ea18b718d44ddbf310e7e29e9e0dc9e3.setContent(html_7aa68cb04d4e3a2a63b23409a12ae9e8);\\n \\n \\n\\n circle_marker_2c2a49708094e2a755666b4bddc5e1cd.bindPopup(popup_ea18b718d44ddbf310e7e29e9e0dc9e3)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e76196fe991ec7f6d9475d862fdd565 = L.circleMarker(\\n [42.72912513166581, -73.23166014961313],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_90847f3f2a71e829d1b248117e505250 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_51250dbedc60c1138e197262ba22f318 = $(`<div id="html_51250dbedc60c1138e197262ba22f318" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90847f3f2a71e829d1b248117e505250.setContent(html_51250dbedc60c1138e197262ba22f318);\\n \\n \\n\\n circle_marker_5e76196fe991ec7f6d9475d862fdd565.bindPopup(popup_90847f3f2a71e829d1b248117e505250)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d8e5d4f91392e72c518341f355ad639 = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6c5723cbb740aa59041989764ca494aa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_50fa93ab5a7388398414a489f6cfe502 = $(`<div id="html_50fa93ab5a7388398414a489f6cfe502" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c5723cbb740aa59041989764ca494aa.setContent(html_50fa93ab5a7388398414a489f6cfe502);\\n \\n \\n\\n circle_marker_1d8e5d4f91392e72c518341f355ad639.bindPopup(popup_6c5723cbb740aa59041989764ca494aa)\\n ;\\n\\n \\n \\n \\n var circle_marker_ed1f488e68eb0dc7391c966bea5e70ff = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0123024c9ac0781c10d98ed8cc3b25a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a44621e1ae1f41bccef2ee30b34bf85 = $(`<div id="html_8a44621e1ae1f41bccef2ee30b34bf85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0123024c9ac0781c10d98ed8cc3b25a4.setContent(html_8a44621e1ae1f41bccef2ee30b34bf85);\\n \\n \\n\\n circle_marker_ed1f488e68eb0dc7391c966bea5e70ff.bindPopup(popup_0123024c9ac0781c10d98ed8cc3b25a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_4fbd01ac2b8bd1a3009b37507e832476 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7c620b7a632502008851419077c56d75 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41f3e922091c892fbf19ac7a0915e916 = $(`<div id="html_41f3e922091c892fbf19ac7a0915e916" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c620b7a632502008851419077c56d75.setContent(html_41f3e922091c892fbf19ac7a0915e916);\\n \\n \\n\\n circle_marker_4fbd01ac2b8bd1a3009b37507e832476.bindPopup(popup_7c620b7a632502008851419077c56d75)\\n ;\\n\\n \\n \\n \\n var circle_marker_f60117bcbba2587ce0918d8b31e2cefe = L.circleMarker(\\n [42.72732247454739, -73.27348202255521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_191d684a0e41edd9ee51294e34238480 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_101e7c77641da2377dbcb3538f0cc1f2 = $(`<div id="html_101e7c77641da2377dbcb3538f0cc1f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_191d684a0e41edd9ee51294e34238480.setContent(html_101e7c77641da2377dbcb3538f0cc1f2);\\n \\n \\n\\n circle_marker_f60117bcbba2587ce0918d8b31e2cefe.bindPopup(popup_191d684a0e41edd9ee51294e34238480)\\n ;\\n\\n \\n \\n \\n var circle_marker_80006ed0e31ab9c0c32783868b46dd55 = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e687c6e0292e87763f1a5e37572fa96a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6bf2fbd4ca0dfae890d9272478ba48c2 = $(`<div id="html_6bf2fbd4ca0dfae890d9272478ba48c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e687c6e0292e87763f1a5e37572fa96a.setContent(html_6bf2fbd4ca0dfae890d9272478ba48c2);\\n \\n \\n\\n circle_marker_80006ed0e31ab9c0c32783868b46dd55.bindPopup(popup_e687c6e0292e87763f1a5e37572fa96a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f50b9dcab782d75df0d0a0a0d58b1425 = L.circleMarker(\\n [42.72732263252603, -73.27102196922051],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0b631e9689c57f14673c28cc38da3b82 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e99425249ddb1875cef57f5a62cfecfb = $(`<div id="html_e99425249ddb1875cef57f5a62cfecfb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0b631e9689c57f14673c28cc38da3b82.setContent(html_e99425249ddb1875cef57f5a62cfecfb);\\n \\n \\n\\n circle_marker_f50b9dcab782d75df0d0a0a0d58b1425.bindPopup(popup_0b631e9689c57f14673c28cc38da3b82)\\n ;\\n\\n \\n \\n \\n var circle_marker_d0f2789aa16634322d03d3ad489ac84c = L.circleMarker(\\n [42.72732269176802, -73.26979194254899],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.9316150714175193, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_28d553553235039c833ccc43a8bf4f38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_851b42b667746538f4eecef4bad5a33b = $(`<div id="html_851b42b667746538f4eecef4bad5a33b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_28d553553235039c833ccc43a8bf4f38.setContent(html_851b42b667746538f4eecef4bad5a33b);\\n \\n \\n\\n circle_marker_d0f2789aa16634322d03d3ad489ac84c.bindPopup(popup_28d553553235039c833ccc43a8bf4f38)\\n ;\\n\\n \\n \\n \\n var circle_marker_03bfc984878061c643737935dc0a4161 = L.circleMarker(\\n [42.72732277075734, -73.26733188920018],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_122121022dbbd0ff1f21128b5bc68f69 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_deb99e47fb89576179fb5ff0134fc0ae = $(`<div id="html_deb99e47fb89576179fb5ff0134fc0ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_122121022dbbd0ff1f21128b5bc68f69.setContent(html_deb99e47fb89576179fb5ff0134fc0ae);\\n \\n \\n\\n circle_marker_03bfc984878061c643737935dc0a4161.bindPopup(popup_122121022dbbd0ff1f21128b5bc68f69)\\n ;\\n\\n \\n \\n \\n var circle_marker_6e3f3328c4684ccc9f536b043d32e5b6 = L.circleMarker(\\n [42.72732279050467, -73.26610186252397],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ea5354c06c845a7f3b7cf93570e79b47 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2fc4065cf514bee208368342d612bda2 = $(`<div id="html_2fc4065cf514bee208368342d612bda2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ea5354c06c845a7f3b7cf93570e79b47.setContent(html_2fc4065cf514bee208368342d612bda2);\\n \\n \\n\\n circle_marker_6e3f3328c4684ccc9f536b043d32e5b6.bindPopup(popup_ea5354c06c845a7f3b7cf93570e79b47)\\n ;\\n\\n \\n \\n \\n var circle_marker_db1d08e225f32d27d6a82cf8450ef406 = L.circleMarker(\\n [42.727322797087126, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7743a4c4c32ae62b5db4f8b4612b6a6b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f462339ecbccee9a7b4b51a8dbb75a7b = $(`<div id="html_f462339ecbccee9a7b4b51a8dbb75a7b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7743a4c4c32ae62b5db4f8b4612b6a6b.setContent(html_f462339ecbccee9a7b4b51a8dbb75a7b);\\n \\n \\n\\n circle_marker_db1d08e225f32d27d6a82cf8450ef406.bindPopup(popup_7743a4c4c32ae62b5db4f8b4612b6a6b)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd2552399666b2eb63b7714fe53276f6 = L.circleMarker(\\n [42.72732279050467, -73.26364180917045],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0f764bf6fddb197b7ef2dddd344e4945 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_75a0b107f1ed6018dd532689e40b5ae9 = $(`<div id="html_75a0b107f1ed6018dd532689e40b5ae9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0f764bf6fddb197b7ef2dddd344e4945.setContent(html_75a0b107f1ed6018dd532689e40b5ae9);\\n \\n \\n\\n circle_marker_fd2552399666b2eb63b7714fe53276f6.bindPopup(popup_0f764bf6fddb197b7ef2dddd344e4945)\\n ;\\n\\n \\n \\n \\n var circle_marker_2c47b07c095218f47460083104a631dc = L.circleMarker(\\n [42.72732277075734, -73.26241178249424],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0e655e14fab9495b60b2403486255f17 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_56b6abdcc2f7d5478fbddd1814d81a2c = $(`<div id="html_56b6abdcc2f7d5478fbddd1814d81a2c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0e655e14fab9495b60b2403486255f17.setContent(html_56b6abdcc2f7d5478fbddd1814d81a2c);\\n \\n \\n\\n circle_marker_2c47b07c095218f47460083104a631dc.bindPopup(popup_0e655e14fab9495b60b2403486255f17)\\n ;\\n\\n \\n \\n \\n var circle_marker_ca77b968a7fc99bed804abb65528c13c = L.circleMarker(\\n [42.727322737845135, -73.26118175581905],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d1519fa66387360b514f0573497c8b49 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_64b6d40780a7a096c3d65e9918efd484 = $(`<div id="html_64b6d40780a7a096c3d65e9918efd484" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d1519fa66387360b514f0573497c8b49.setContent(html_64b6d40780a7a096c3d65e9918efd484);\\n \\n \\n\\n circle_marker_ca77b968a7fc99bed804abb65528c13c.bindPopup(popup_d1519fa66387360b514f0573497c8b49)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa5c8059cc474a24db7f6676aec2f08c = L.circleMarker(\\n [42.72732269176802, -73.25995172914543],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ab9d45a3a9605bfae690cf407632a802 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ecbda048fd7a5b055bb80ad929dc7ee9 = $(`<div id="html_ecbda048fd7a5b055bb80ad929dc7ee9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ab9d45a3a9605bfae690cf407632a802.setContent(html_ecbda048fd7a5b055bb80ad929dc7ee9);\\n \\n \\n\\n circle_marker_aa5c8059cc474a24db7f6676aec2f08c.bindPopup(popup_ab9d45a3a9605bfae690cf407632a802)\\n ;\\n\\n \\n \\n \\n var circle_marker_5adad205228e1164e5613de59b4b60dc = L.circleMarker(\\n [42.72732263252603, -73.2587217024739],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_aeb0c7b5cdeafef69bebd85488af31a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_809df1318cb2548de4204ffe14a045f2 = $(`<div id="html_809df1318cb2548de4204ffe14a045f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aeb0c7b5cdeafef69bebd85488af31a0.setContent(html_809df1318cb2548de4204ffe14a045f2);\\n \\n \\n\\n circle_marker_5adad205228e1164e5613de59b4b60dc.bindPopup(popup_aeb0c7b5cdeafef69bebd85488af31a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_dab1b088b19d97f733ece9060acee008 = L.circleMarker(\\n [42.72732256011916, -73.25749167580499],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_baaf9434d9e52778c867e44ca819cb91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2286e792d3a9a48e008da48edd704a6a = $(`<div id="html_2286e792d3a9a48e008da48edd704a6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_baaf9434d9e52778c867e44ca819cb91.setContent(html_2286e792d3a9a48e008da48edd704a6a);\\n \\n \\n\\n circle_marker_dab1b088b19d97f733ece9060acee008.bindPopup(popup_baaf9434d9e52778c867e44ca819cb91)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e9fda447d32298c1c7f23eedf5feda8 = L.circleMarker(\\n [42.72732247454739, -73.25626164913919],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_547c79eb49cac75ff21723a46c9324d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0bece3b81a7f5606c43b2e36094afd6a = $(`<div id="html_0bece3b81a7f5606c43b2e36094afd6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_547c79eb49cac75ff21723a46c9324d4.setContent(html_0bece3b81a7f5606c43b2e36094afd6a);\\n \\n \\n\\n circle_marker_3e9fda447d32298c1c7f23eedf5feda8.bindPopup(popup_547c79eb49cac75ff21723a46c9324d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_93db1a36cbdff84273a8d2588f10585a = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_aca682a1c3ffdbc3f741414d7e075f4e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2215b67ae3ba3bcd1d840a7f2c0b4420 = $(`<div id="html_2215b67ae3ba3bcd1d840a7f2c0b4420" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aca682a1c3ffdbc3f741414d7e075f4e.setContent(html_2215b67ae3ba3bcd1d840a7f2c0b4420);\\n \\n \\n\\n circle_marker_93db1a36cbdff84273a8d2588f10585a.bindPopup(popup_aca682a1c3ffdbc3f741414d7e075f4e)\\n ;\\n\\n \\n \\n \\n var circle_marker_552c8abb52c708aa2c8d806273b0ea1a = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5e92666e51b2987e259ae86823882209 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ca2de97d067d3d6d70720cdeab39dc6a = $(`<div id="html_ca2de97d067d3d6d70720cdeab39dc6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e92666e51b2987e259ae86823882209.setContent(html_ca2de97d067d3d6d70720cdeab39dc6a);\\n \\n \\n\\n circle_marker_552c8abb52c708aa2c8d806273b0ea1a.bindPopup(popup_5e92666e51b2987e259ae86823882209)\\n ;\\n\\n \\n \\n \\n var circle_marker_8f0414097773faf01b662184f75b2bf2 = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c052ec8adda0ebae44115223ac306f47 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc6cd2d960bdf1cc648b2e6d370d4250 = $(`<div id="html_cc6cd2d960bdf1cc648b2e6d370d4250" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c052ec8adda0ebae44115223ac306f47.setContent(html_cc6cd2d960bdf1cc648b2e6d370d4250);\\n \\n \\n\\n circle_marker_8f0414097773faf01b662184f75b2bf2.bindPopup(popup_c052ec8adda0ebae44115223ac306f47)\\n ;\\n\\n \\n \\n \\n var circle_marker_094bc3d042abddd4fd635fcc47e30aaf = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b557b4948dbebc32bbe31862042b678f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9296c81b0430f81529d939f705db1cd5 = $(`<div id="html_9296c81b0430f81529d939f705db1cd5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b557b4948dbebc32bbe31862042b678f.setContent(html_9296c81b0430f81529d939f705db1cd5);\\n \\n \\n\\n circle_marker_094bc3d042abddd4fd635fcc47e30aaf.bindPopup(popup_b557b4948dbebc32bbe31862042b678f)\\n ;\\n\\n \\n \\n \\n var circle_marker_201c64c0b0ec2324c83465769343b72a = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f34de31c8cbafd20a6ead90e90d4b48e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ec4b7573de9e115cca697ffc8cd669ea = $(`<div id="html_ec4b7573de9e115cca697ffc8cd669ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f34de31c8cbafd20a6ead90e90d4b48e.setContent(html_ec4b7573de9e115cca697ffc8cd669ea);\\n \\n \\n\\n circle_marker_201c64c0b0ec2324c83465769343b72a.bindPopup(popup_f34de31c8cbafd20a6ead90e90d4b48e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b14944998531e8a7a801ad6dd54386a8 = L.circleMarker(\\n [42.7273216846542, -73.24888148923952],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_8ed4dd2fcd5023403b0509a3f86c51c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6328cd148c449d894a8bb9e1d5cfa2d8 = $(`<div id="html_6328cd148c449d894a8bb9e1d5cfa2d8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ed4dd2fcd5023403b0509a3f86c51c2.setContent(html_6328cd148c449d894a8bb9e1d5cfa2d8);\\n \\n \\n\\n circle_marker_b14944998531e8a7a801ad6dd54386a8.bindPopup(popup_8ed4dd2fcd5023403b0509a3f86c51c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_6ba00392b8a8274e19392f8f6c397e81 = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6a1dfa8d40060ab0eac0f1c9b6abceb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6879e6b5d9b549d2b0cff56229e3aab4 = $(`<div id="html_6879e6b5d9b549d2b0cff56229e3aab4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a1dfa8d40060ab0eac0f1c9b6abceb8.setContent(html_6879e6b5d9b549d2b0cff56229e3aab4);\\n \\n \\n\\n circle_marker_6ba00392b8a8274e19392f8f6c397e81.bindPopup(popup_6a1dfa8d40060ab0eac0f1c9b6abceb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_d9341a0dcafbbd6f28549da7b7d54f63 = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_06c1b0a1d73800306ed9c483ca526021 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e7ffe65a76f437a260e4693d8a3bb4d1 = $(`<div id="html_e7ffe65a76f437a260e4693d8a3bb4d1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_06c1b0a1d73800306ed9c483ca526021.setContent(html_e7ffe65a76f437a260e4693d8a3bb4d1);\\n \\n \\n\\n circle_marker_d9341a0dcafbbd6f28549da7b7d54f63.bindPopup(popup_06c1b0a1d73800306ed9c483ca526021)\\n ;\\n\\n \\n \\n \\n var circle_marker_3b3dcf8468a99f358dff96a006d259fc = L.circleMarker(\\n [42.72732089476102, -73.24396138276853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5b5bc2cc801a6b66cb65de4559c165d6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_483faabcb7a04383984d682a99b5c419 = $(`<div id="html_483faabcb7a04383984d682a99b5c419" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5b5bc2cc801a6b66cb65de4559c165d6.setContent(html_483faabcb7a04383984d682a99b5c419);\\n \\n \\n\\n circle_marker_3b3dcf8468a99f358dff96a006d259fc.bindPopup(popup_5b5bc2cc801a6b66cb65de4559c165d6)\\n ;\\n\\n \\n \\n \\n var circle_marker_802246785387d1c048720f48bf6a0d5e = L.circleMarker(\\n [42.72732042082513, -73.24150132958422],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3505ed545c1fa8be6c2e625a3c7f697c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5213fe7ff091e347ee9468688d728a91 = $(`<div id="html_5213fe7ff091e347ee9468688d728a91" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3505ed545c1fa8be6c2e625a3c7f697c.setContent(html_5213fe7ff091e347ee9468688d728a91);\\n \\n \\n\\n circle_marker_802246785387d1c048720f48bf6a0d5e.bindPopup(popup_3505ed545c1fa8be6c2e625a3c7f697c)\\n ;\\n\\n \\n \\n \\n var circle_marker_c29f9690433e3f567b28a1f61468c86b = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_0c9037f30a4e7d00b4d49b16bb6dcce3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1236f0c52d996f34de551e309ee77b3f = $(`<div id="html_1236f0c52d996f34de551e309ee77b3f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0c9037f30a4e7d00b4d49b16bb6dcce3.setContent(html_1236f0c52d996f34de551e309ee77b3f);\\n \\n \\n\\n circle_marker_c29f9690433e3f567b28a1f61468c86b.bindPopup(popup_0c9037f30a4e7d00b4d49b16bb6dcce3)\\n ;\\n\\n \\n \\n \\n var circle_marker_8ee3cee934d5fa41b8364437516ecbc5 = L.circleMarker(\\n [42.72731961118464, -73.23781124988345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cebf56f4d962e06fa01f62cd998ee1a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a529cbfb71a33402279800efb9c650f = $(`<div id="html_5a529cbfb71a33402279800efb9c650f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cebf56f4d962e06fa01f62cd998ee1a2.setContent(html_5a529cbfb71a33402279800efb9c650f);\\n \\n \\n\\n circle_marker_8ee3cee934d5fa41b8364437516ecbc5.bindPopup(popup_cebf56f4d962e06fa01f62cd998ee1a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_e7730d5c6104838792daee1e23386243 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_fbe72abb42b9a2c97e02591e05648363 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5630711c4ca93a0d3e9cfe064177799b = $(`<div id="html_5630711c4ca93a0d3e9cfe064177799b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fbe72abb42b9a2c97e02591e05648363.setContent(html_5630711c4ca93a0d3e9cfe064177799b);\\n \\n \\n\\n circle_marker_e7730d5c6104838792daee1e23386243.bindPopup(popup_fbe72abb42b9a2c97e02591e05648363)\\n ;\\n\\n \\n \\n \\n var circle_marker_6755001f2e7c4a6bf11b2836d66a9e71 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_43923d6ff31f09f1fe44807277d5fac1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_901bf5570e5eda34ed589f76f0b6cb72 = $(`<div id="html_901bf5570e5eda34ed589f76f0b6cb72" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_43923d6ff31f09f1fe44807277d5fac1.setContent(html_901bf5570e5eda34ed589f76f0b6cb72);\\n \\n \\n\\n circle_marker_6755001f2e7c4a6bf11b2836d66a9e71.bindPopup(popup_43923d6ff31f09f1fe44807277d5fac1)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf2eaf1ccd7f97641936df7bb5bf6144 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e465d498943cd6458bf95512e23528f2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bdfad7d4019c1ee8c7be03f430ccf1a8 = $(`<div id="html_bdfad7d4019c1ee8c7be03f430ccf1a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e465d498943cd6458bf95512e23528f2.setContent(html_bdfad7d4019c1ee8c7be03f430ccf1a8);\\n \\n \\n\\n circle_marker_bf2eaf1ccd7f97641936df7bb5bf6144.bindPopup(popup_e465d498943cd6458bf95512e23528f2)\\n ;\\n\\n \\n \\n \\n var circle_marker_bcff04397551ecad358388527ebb839f = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_3ceb53b8b5b8d9e6fdb2424a09108996 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e47ef0a9acbf882bdda7f0021267ec15 = $(`<div id="html_e47ef0a9acbf882bdda7f0021267ec15" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3ceb53b8b5b8d9e6fdb2424a09108996.setContent(html_e47ef0a9acbf882bdda7f0021267ec15);\\n \\n \\n\\n circle_marker_bcff04397551ecad358388527ebb839f.bindPopup(popup_3ceb53b8b5b8d9e6fdb2424a09108996)\\n ;\\n\\n \\n \\n \\n var circle_marker_4bb231dcd237bd2d21965d0ee10f2e65 = L.circleMarker(\\n [42.72731799848616, -73.23166111728555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_82cf8c0824344a3bdb79633ae601c5d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_10faa804eee0722e46711e8ad847341b = $(`<div id="html_10faa804eee0722e46711e8ad847341b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_82cf8c0824344a3bdb79633ae601c5d7.setContent(html_10faa804eee0722e46711e8ad847341b);\\n \\n \\n\\n circle_marker_4bb231dcd237bd2d21965d0ee10f2e65.bindPopup(popup_82cf8c0824344a3bdb79633ae601c5d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_24468ffc64c8233056fd0734b7d9da25 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_e5ffa1427b43ad7f4a1b40951259b84e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a2b18f5ad6fb1fbce11ec224c5f29991 = $(`<div id="html_a2b18f5ad6fb1fbce11ec224c5f29991" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e5ffa1427b43ad7f4a1b40951259b84e.setContent(html_a2b18f5ad6fb1fbce11ec224c5f29991);\\n \\n \\n\\n circle_marker_24468ffc64c8233056fd0734b7d9da25.bindPopup(popup_e5ffa1427b43ad7f4a1b40951259b84e)\\n ;\\n\\n \\n \\n \\n var circle_marker_8a68f3f124fa08c3578661802a4e0759 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_7087a2254d23c8d3518fe921cccf0f38 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_57f2326986755afd5e3edd034115f637 = $(`<div id="html_57f2326986755afd5e3edd034115f637" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7087a2254d23c8d3518fe921cccf0f38.setContent(html_57f2326986755afd5e3edd034115f637);\\n \\n \\n\\n circle_marker_8a68f3f124fa08c3578661802a4e0759.bindPopup(popup_7087a2254d23c8d3518fe921cccf0f38)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c6accc99793dc52478fe62653205947 = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ad2c646cada0f8fca60959ea81b20085 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_13055e24ad9ef91d7f544811954a7986 = $(`<div id="html_13055e24ad9ef91d7f544811954a7986" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ad2c646cada0f8fca60959ea81b20085.setContent(html_13055e24ad9ef91d7f544811954a7986);\\n \\n \\n\\n circle_marker_7c6accc99793dc52478fe62653205947.bindPopup(popup_ad2c646cada0f8fca60959ea81b20085)\\n ;\\n\\n \\n \\n \\n var circle_marker_a52ed8e85d1e4487423468c22a28515c = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c2b02f905d7819081c6e7d1ce7fc1aa1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c5c9001ee667e515ec06807a1f5d1618 = $(`<div id="html_c5c9001ee667e515ec06807a1f5d1618" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c2b02f905d7819081c6e7d1ce7fc1aa1.setContent(html_c5c9001ee667e515ec06807a1f5d1618);\\n \\n \\n\\n circle_marker_a52ed8e85d1e4487423468c22a28515c.bindPopup(popup_c2b02f905d7819081c6e7d1ce7fc1aa1)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe05d17482f6ce415629d65f2acf8056 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.5682482323055424, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_24506ad754b637918a1c1b91c8eda037 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_530c714919312c75c30ad410f05083fd = $(`<div id="html_530c714919312c75c30ad410f05083fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24506ad754b637918a1c1b91c8eda037.setContent(html_530c714919312c75c30ad410f05083fd);\\n \\n \\n\\n circle_marker_fe05d17482f6ce415629d65f2acf8056.bindPopup(popup_24506ad754b637918a1c1b91c8eda037)\\n ;\\n\\n \\n \\n \\n var circle_marker_91ba5630c916d2e421bf5fcd58b6b978 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.853342309955121, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f30ace955bd13942c46d52bfb5aeac40 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c04263ace73400987ed0c68372e1a011 = $(`<div id="html_c04263ace73400987ed0c68372e1a011" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f30ace955bd13942c46d52bfb5aeac40.setContent(html_c04263ace73400987ed0c68372e1a011);\\n \\n \\n\\n circle_marker_91ba5630c916d2e421bf5fcd58b6b978.bindPopup(popup_f30ace955bd13942c46d52bfb5aeac40)\\n ;\\n\\n \\n \\n \\n var circle_marker_c3ca2af62b2c496a2a46fe508409880b = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ceb97dc908420de865b948d662cb6c26 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8f3e640f8dcab28f357485b71dd13b32 = $(`<div id="html_8f3e640f8dcab28f357485b71dd13b32" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ceb97dc908420de865b948d662cb6c26.setContent(html_8f3e640f8dcab28f357485b71dd13b32);\\n \\n \\n\\n circle_marker_c3ca2af62b2c496a2a46fe508409880b.bindPopup(popup_ceb97dc908420de865b948d662cb6c26)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c2a047adc835bada17b5d397ba08638 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_d380b1c14d96fdbc160481e0a6a69b92 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d5a9535e44fb8af95568f38968f0d182 = $(`<div id="html_d5a9535e44fb8af95568f38968f0d182" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d380b1c14d96fdbc160481e0a6a69b92.setContent(html_d5a9535e44fb8af95568f38968f0d182);\\n \\n \\n\\n circle_marker_3c2a047adc835bada17b5d397ba08638.bindPopup(popup_d380b1c14d96fdbc160481e0a6a69b92)\\n ;\\n\\n \\n \\n \\n var circle_marker_93a08173a533a573a19f37548185441d = L.circleMarker(\\n [42.725515657130885, -73.26610182668713],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_accf75aa0d8647add6181fd5d2c72c45 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_791d872d7a633ef4a052dd57b54958c4 = $(`<div id="html_791d872d7a633ef4a052dd57b54958c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_accf75aa0d8647add6181fd5d2c72c45.setContent(html_791d872d7a633ef4a052dd57b54958c4);\\n \\n \\n\\n circle_marker_93a08173a533a573a19f37548185441d.bindPopup(popup_accf75aa0d8647add6181fd5d2c72c45)\\n ;\\n\\n \\n \\n \\n var circle_marker_548afee56fc49354832822885ac42566 = L.circleMarker(\\n [42.72551566371306, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_91d9b2502806138c59684355475fe5e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_611ac754d8fa0f5eddfa0e1cd304e679 = $(`<div id="html_611ac754d8fa0f5eddfa0e1cd304e679" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_91d9b2502806138c59684355475fe5e4.setContent(html_611ac754d8fa0f5eddfa0e1cd304e679);\\n \\n \\n\\n circle_marker_548afee56fc49354832822885ac42566.bindPopup(popup_91d9b2502806138c59684355475fe5e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_d9250817c4f2550f6c3ea25cf8170147 = L.circleMarker(\\n [42.725515657130885, -73.26364184500729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_20e6bf54dce73b63dba85aee11a5cbc1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7d10d784007c8f3a0eb668d7eb88efee = $(`<div id="html_7d10d784007c8f3a0eb668d7eb88efee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_20e6bf54dce73b63dba85aee11a5cbc1.setContent(html_7d10d784007c8f3a0eb668d7eb88efee);\\n \\n \\n\\n circle_marker_d9250817c4f2550f6c3ea25cf8170147.bindPopup(popup_20e6bf54dce73b63dba85aee11a5cbc1)\\n ;\\n\\n \\n \\n \\n var circle_marker_b500ad665a8b9c221a87cc5bde135492 = L.circleMarker(\\n [42.72551563738435, -73.26241185416787],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cfa62cc394ac509a66ff015661c2d975 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_82b50d34ef97052d5eb5b8b45fc4c82d = $(`<div id="html_82b50d34ef97052d5eb5b8b45fc4c82d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfa62cc394ac509a66ff015661c2d975.setContent(html_82b50d34ef97052d5eb5b8b45fc4c82d);\\n \\n \\n\\n circle_marker_b500ad665a8b9c221a87cc5bde135492.bindPopup(popup_cfa62cc394ac509a66ff015661c2d975)\\n ;\\n\\n \\n \\n \\n var circle_marker_20e72641aebb60444e3d8dedacc9e472 = L.circleMarker(\\n [42.72551560447347, -73.26118186332953],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_131a257086487a7dda50e37e302c675d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f1342cd689908111600af1103163e8f = $(`<div id="html_6f1342cd689908111600af1103163e8f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_131a257086487a7dda50e37e302c675d.setContent(html_6f1342cd689908111600af1103163e8f);\\n \\n \\n\\n circle_marker_20e72641aebb60444e3d8dedacc9e472.bindPopup(popup_131a257086487a7dda50e37e302c675d)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9283aae93c3dfbd2ae417b426a4c9fa = L.circleMarker(\\n [42.72551555839823, -73.25995187249272],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_af530d9823b9c29430ccdb96d3f7ca9b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cd9fbfc73425323cf9cd221860c5b4e5 = $(`<div id="html_cd9fbfc73425323cf9cd221860c5b4e5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_af530d9823b9c29430ccdb96d3f7ca9b.setContent(html_cd9fbfc73425323cf9cd221860c5b4e5);\\n \\n \\n\\n circle_marker_e9283aae93c3dfbd2ae417b426a4c9fa.bindPopup(popup_af530d9823b9c29430ccdb96d3f7ca9b)\\n ;\\n\\n \\n \\n \\n var circle_marker_eabf7db45a3a2f8377b52145070ff51c = L.circleMarker(\\n [42.72551549915864, -73.25872188165802],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_ca75f8952bfc854d96642695ef75b99a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d2de33aa276d82e36a85027a07b88501 = $(`<div id="html_d2de33aa276d82e36a85027a07b88501" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca75f8952bfc854d96642695ef75b99a.setContent(html_d2de33aa276d82e36a85027a07b88501);\\n \\n \\n\\n circle_marker_eabf7db45a3a2f8377b52145070ff51c.bindPopup(popup_ca75f8952bfc854d96642695ef75b99a)\\n ;\\n\\n \\n \\n \\n var circle_marker_abad33718609212978ac732b69606a43 = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_960611bb453b40bc70095506f60dee80 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da21c498534b8591829d771540d648bc = $(`<div id="html_da21c498534b8591829d771540d648bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_960611bb453b40bc70095506f60dee80.setContent(html_da21c498534b8591829d771540d648bc);\\n \\n \\n\\n circle_marker_abad33718609212978ac732b69606a43.bindPopup(popup_960611bb453b40bc70095506f60dee80)\\n ;\\n\\n \\n \\n \\n var circle_marker_4dd2f30e01686f40afabec2d23c4140e = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9ff79182e832b2412db3ce9e840d523b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_403723555ee3061504f808aeaceb8afe = $(`<div id="html_403723555ee3061504f808aeaceb8afe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ff79182e832b2412db3ce9e840d523b.setContent(html_403723555ee3061504f808aeaceb8afe);\\n \\n \\n\\n circle_marker_4dd2f30e01686f40afabec2d23c4140e.bindPopup(popup_9ff79182e832b2412db3ce9e840d523b)\\n ;\\n\\n \\n \\n \\n var circle_marker_53ce239351543800dc0cfb0a6c5b7554 = L.circleMarker(\\n [42.72551513055674, -73.25380191835052],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b2acb454ef1120edfa9aee1241716d24 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4c5addccb1201acb6d98e8917c052a6 = $(`<div id="html_d4c5addccb1201acb6d98e8917c052a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b2acb454ef1120edfa9aee1241716d24.setContent(html_d4c5addccb1201acb6d98e8917c052a6);\\n \\n \\n\\n circle_marker_53ce239351543800dc0cfb0a6c5b7554.bindPopup(popup_b2acb454ef1120edfa9aee1241716d24)\\n ;\\n\\n \\n \\n \\n var circle_marker_5691059ef1048955af504f8d418f6962 = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6c31ed00e0876a485ee5f1d002500e96 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bdba61d2e41f3a81851e3997453f09fd = $(`<div id="html_bdba61d2e41f3a81851e3997453f09fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6c31ed00e0876a485ee5f1d002500e96.setContent(html_bdba61d2e41f3a81851e3997453f09fd);\\n \\n \\n\\n circle_marker_5691059ef1048955af504f8d418f6962.bindPopup(popup_6c31ed00e0876a485ee5f1d002500e96)\\n ;\\n\\n \\n \\n \\n var circle_marker_392190ae92d04a3b3f373108c1268c06 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6bc37a58b534f793eabbe85639efc68d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4223a91d88ba1e0501d12d1bdbc17a8 = $(`<div id="html_a4223a91d88ba1e0501d12d1bdbc17a8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6bc37a58b534f793eabbe85639efc68d.setContent(html_a4223a91d88ba1e0501d12d1bdbc17a8);\\n \\n \\n\\n circle_marker_392190ae92d04a3b3f373108c1268c06.bindPopup(popup_6bc37a58b534f793eabbe85639efc68d)\\n ;\\n\\n \\n \\n \\n var circle_marker_b618aa14daa51c8f01d61e575d611721 = L.circleMarker(\\n [42.72551376146403, -73.24396199199447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_c1224e994819db6f9c8825eb8cccd3ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14e96abea63422f8889a8fa8761e3ae3 = $(`<div id="html_14e96abea63422f8889a8fa8761e3ae3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c1224e994819db6f9c8825eb8cccd3ec.setContent(html_14e96abea63422f8889a8fa8761e3ae3);\\n \\n \\n\\n circle_marker_b618aa14daa51c8f01d61e575d611721.bindPopup(popup_c1224e994819db6f9c8825eb8cccd3ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_2df8c664f4efd088e5938e12d72a6e7b = L.circleMarker(\\n [42.725513531087856, -73.24273200123443],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a9d1f4c2774b4af48cfb97d6ab7c0924 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f473cca34b229552adcf83834ceab8a7 = $(`<div id="html_f473cca34b229552adcf83834ceab8a7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9d1f4c2774b4af48cfb97d6ab7c0924.setContent(html_f473cca34b229552adcf83834ceab8a7);\\n \\n \\n\\n circle_marker_2df8c664f4efd088e5938e12d72a6e7b.bindPopup(popup_a9d1f4c2774b4af48cfb97d6ab7c0924)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e07b04acfc4949775cb0169aab2233b = L.circleMarker(\\n [42.72551303084245, -73.24027201974305],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_930dcdd2e4215563f4c1561a3df2585d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87d2ca4a0fd5692806b6a086c893f99f = $(`<div id="html_87d2ca4a0fd5692806b6a086c893f99f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_930dcdd2e4215563f4c1561a3df2585d.setContent(html_87d2ca4a0fd5692806b6a086c893f99f);\\n \\n \\n\\n circle_marker_3e07b04acfc4949775cb0169aab2233b.bindPopup(popup_930dcdd2e4215563f4c1561a3df2585d)\\n ;\\n\\n \\n \\n \\n var circle_marker_144a33c7e93ab34e5bd91b604e36bed7 = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_cfc5f0866cc1b2aed2302d3096cf79d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ea3c492cd82cb8be4a4f8090aea6856f = $(`<div id="html_ea3c492cd82cb8be4a4f8090aea6856f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cfc5f0866cc1b2aed2302d3096cf79d4.setContent(html_ea3c492cd82cb8be4a4f8090aea6856f);\\n \\n \\n\\n circle_marker_144a33c7e93ab34e5bd91b604e36bed7.bindPopup(popup_cfc5f0866cc1b2aed2302d3096cf79d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_a052759867cec1644da30b4198957b07 = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_9100d5232a3f655d2668a32d3272d89a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bea40886b8ae3bf9c148a2230d854ce5 = $(`<div id="html_bea40886b8ae3bf9c148a2230d854ce5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9100d5232a3f655d2668a32d3272d89a.setContent(html_bea40886b8ae3bf9c148a2230d854ce5);\\n \\n \\n\\n circle_marker_a052759867cec1644da30b4198957b07.bindPopup(popup_9100d5232a3f655d2668a32d3272d89a)\\n ;\\n\\n \\n \\n \\n var circle_marker_fa838c2d93c3232dd230201389e294a9 = L.circleMarker(\\n [42.72551121416183, -73.23289207553623],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_1f047fc2de0a515ff8e05ec14e86a907 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4f338e03a3696ad54c68e238916c1cd2 = $(`<div id="html_4f338e03a3696ad54c68e238916c1cd2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f047fc2de0a515ff8e05ec14e86a907.setContent(html_4f338e03a3696ad54c68e238916c1cd2);\\n \\n \\n\\n circle_marker_fa838c2d93c3232dd230201389e294a9.bindPopup(popup_1f047fc2de0a515ff8e05ec14e86a907)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb544528f266b3adcde0a9423b6a252a = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_5130662e2cea7c59819136efc090cfbe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_583bfed12466750b7fd216fbe8ab6a7f = $(`<div id="html_583bfed12466750b7fd216fbe8ab6a7f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5130662e2cea7c59819136efc090cfbe.setContent(html_583bfed12466750b7fd216fbe8ab6a7f);\\n \\n \\n\\n circle_marker_fb544528f266b3adcde0a9423b6a252a.bindPopup(popup_5130662e2cea7c59819136efc090cfbe)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e5f06e416b79859be4dc5555a4a5fd6 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_89a13b8715983aa00704a3d547806cc9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b51137cac7a68f3fc7b639f1efdc469a = $(`<div id="html_b51137cac7a68f3fc7b639f1efdc469a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_89a13b8715983aa00704a3d547806cc9.setContent(html_b51137cac7a68f3fc7b639f1efdc469a);\\n \\n \\n\\n circle_marker_4e5f06e416b79859be4dc5555a4a5fd6.bindPopup(popup_89a13b8715983aa00704a3d547806cc9)\\n ;\\n\\n \\n \\n \\n var circle_marker_84a3766446b782dbfa85e9bfd01499d3 = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_2b56aaf616eff30cc2e6e908a3a066f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06e8ee63af585c5aec9bd23916b9dab9 = $(`<div id="html_06e8ee63af585c5aec9bd23916b9dab9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b56aaf616eff30cc2e6e908a3a066f9.setContent(html_06e8ee63af585c5aec9bd23916b9dab9);\\n \\n \\n\\n circle_marker_84a3766446b782dbfa85e9bfd01499d3.bindPopup(popup_2b56aaf616eff30cc2e6e908a3a066f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_3523f1d24ffe91645a4384bb0a24e310 = L.circleMarker(\\n [42.72370842502844, -73.2599520158284],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a7e5d5929cf537364d996f75dcbdbfd0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f526030131e6e58e95b02bb07191bfdb = $(`<div id="html_f526030131e6e58e95b02bb07191bfdb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a7e5d5929cf537364d996f75dcbdbfd0.setContent(html_f526030131e6e58e95b02bb07191bfdb);\\n \\n \\n\\n circle_marker_3523f1d24ffe91645a4384bb0a24e310.bindPopup(popup_a7e5d5929cf537364d996f75dcbdbfd0)\\n ;\\n\\n \\n \\n \\n var circle_marker_68d34b9de169dc342fee1bf6fa9da822 = L.circleMarker(\\n [42.723708365791246, -73.25872206082761],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_6373e13127de615b538d17f8a574ea5a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d9cc3ea4aa85fb8ec103d6e19cc9c0ec = $(`<div id="html_d9cc3ea4aa85fb8ec103d6e19cc9c0ec" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6373e13127de615b538d17f8a574ea5a.setContent(html_d9cc3ea4aa85fb8ec103d6e19cc9c0ec);\\n \\n \\n\\n circle_marker_68d34b9de169dc342fee1bf6fa9da822.bindPopup(popup_6373e13127de615b538d17f8a574ea5a)\\n ;\\n\\n \\n \\n \\n var circle_marker_216044ecc75d32455afa0da316d428fd = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b635ae1e21fc522a73cc90d5706dc5c2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07326c4ec90580dcb4a7255776d600f4 = $(`<div id="html_07326c4ec90580dcb4a7255776d600f4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b635ae1e21fc522a73cc90d5706dc5c2.setContent(html_07326c4ec90580dcb4a7255776d600f4);\\n \\n \\n\\n circle_marker_216044ecc75d32455afa0da316d428fd.bindPopup(popup_b635ae1e21fc522a73cc90d5706dc5c2)\\n ;\\n\\n \\n \\n \\n var circle_marker_0cf42b525308d28e2baac83ac001787f = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_bde3002cd190642ee4b3c4d1f5e8da20 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a0671c9442775617adeaf3e36cfc5007 = $(`<div id="html_a0671c9442775617adeaf3e36cfc5007" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bde3002cd190642ee4b3c4d1f5e8da20.setContent(html_a0671c9442775617adeaf3e36cfc5007);\\n \\n \\n\\n circle_marker_0cf42b525308d28e2baac83ac001787f.bindPopup(popup_bde3002cd190642ee4b3c4d1f5e8da20)\\n ;\\n\\n \\n \\n \\n var circle_marker_1593f32ad48d735914a33404f9838d5a = L.circleMarker(\\n [42.72370810909676, -73.255032195843],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_f72d8fe0785a886d7947832c593509fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f2e9f9aa795c2b5597be670de1297fb3 = $(`<div id="html_f2e9f9aa795c2b5597be670de1297fb3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f72d8fe0785a886d7947832c593509fe.setContent(html_f2e9f9aa795c2b5597be670de1297fb3);\\n \\n \\n\\n circle_marker_1593f32ad48d735914a33404f9838d5a.bindPopup(popup_f72d8fe0785a886d7947832c593509fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_472272cf4abe4a8f04b42785df446847 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_4e57c64272243d35d358fb7af67fcbe8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a97463d657f7a8d9ccbae7d07b263256 = $(`<div id="html_a97463d657f7a8d9ccbae7d07b263256" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4e57c64272243d35d358fb7af67fcbe8.setContent(html_a97463d657f7a8d9ccbae7d07b263256);\\n \\n \\n\\n circle_marker_472272cf4abe4a8f04b42785df446847.bindPopup(popup_4e57c64272243d35d358fb7af67fcbe8)\\n ;\\n\\n \\n \\n \\n var circle_marker_32533c86352038b73def7f90ad4901d8 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_357881f47c6011c1f5d78c1227eaa714 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6251b7c3fedf6c03bd16584c0a0a2d5 = $(`<div id="html_a6251b7c3fedf6c03bd16584c0a0a2d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_357881f47c6011c1f5d78c1227eaa714.setContent(html_a6251b7c3fedf6c03bd16584c0a0a2d5);\\n \\n \\n\\n circle_marker_32533c86352038b73def7f90ad4901d8.bindPopup(popup_357881f47c6011c1f5d78c1227eaa714)\\n ;\\n\\n \\n \\n \\n var circle_marker_204d822d12a717b3041733e625f95297 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_a04113d029a86accc859e2cfba5b034a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_75b55596a1bf26a6d5fc847ca0149fb6 = $(`<div id="html_75b55596a1bf26a6d5fc847ca0149fb6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a04113d029a86accc859e2cfba5b034a.setContent(html_75b55596a1bf26a6d5fc847ca0149fb6);\\n \\n \\n\\n circle_marker_204d822d12a717b3041733e625f95297.bindPopup(popup_a04113d029a86accc859e2cfba5b034a)\\n ;\\n\\n \\n \\n \\n var circle_marker_f37e6bcde03103cf9fea3f9af27ff2fc = L.circleMarker(\\n [42.72370260662033, -73.22797318801351],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_b648e5438408792019b8e48c6f4d1a45 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e5bdb3b01162b919174ba0682a50ae60 = $(`<div id="html_e5bdb3b01162b919174ba0682a50ae60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b648e5438408792019b8e48c6f4d1a45.setContent(html_e5bdb3b01162b919174ba0682a50ae60);\\n \\n \\n\\n circle_marker_f37e6bcde03103cf9fea3f9af27ff2fc.bindPopup(popup_b648e5438408792019b8e48c6f4d1a45)\\n ;\\n\\n \\n \\n \\n var circle_marker_e920545eb7e418f663bfacb849c88e54 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_32b180e8965d68b62502b147e1b13201);\\n \\n \\n var popup_738ffb7733afaec6a291692c688330f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c2af500cb1a3e0cad09a878ba0669ffd = $(`<div id="html_c2af500cb1a3e0cad09a878ba0669ffd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_738ffb7733afaec6a291692c688330f9.setContent(html_c2af500cb1a3e0cad09a878ba0669ffd);\\n \\n \\n\\n circle_marker_e920545eb7e418f663bfacb849c88e54.bindPopup(popup_738ffb7733afaec6a291692c688330f9)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Oak, white\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_1bf50892cc49230657cfd758f7dbd79b {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_1bf50892cc49230657cfd758f7dbd79b" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_1bf50892cc49230657cfd758f7dbd79b = L.map(\\n "map_1bf50892cc49230657cfd758f7dbd79b",\\n {\\n center: [42.73996465834155, -73.22181212303349],\\n crs: L.CRS.EPSG3857,\\n zoom: 18,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_7eec90a972d6c18ce7327a053befd47f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 20, "maxZoom": 20, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_1bf50892cc49230657cfd758f7dbd79b);\\n \\n \\n var circle_marker_e6a0b138b8af6ad57d23d499456df3ec = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_1bf50892cc49230657cfd758f7dbd79b);\\n \\n \\n var popup_cf7056d85d13b6e1f28189c2974a9a0f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f36a95573f5816c5b52c8c3187457fa = $(`<div id="html_1f36a95573f5816c5b52c8c3187457fa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cf7056d85d13b6e1f28189c2974a9a0f.setContent(html_1f36a95573f5816c5b52c8c3187457fa);\\n \\n \\n\\n circle_marker_e6a0b138b8af6ad57d23d499456df3ec.bindPopup(popup_cf7056d85d13b6e1f28189c2974a9a0f)\\n ;\\n\\n \\n \\n \\n var circle_marker_56d05d6af91897c23294756b275d71f3 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_1bf50892cc49230657cfd758f7dbd79b);\\n \\n \\n var popup_fd680f316f829914ea2036d6ce8314c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ae2c21d2ddaad52a06cf9e0158ba52c9 = $(`<div id="html_ae2c21d2ddaad52a06cf9e0158ba52c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd680f316f829914ea2036d6ce8314c6.setContent(html_ae2c21d2ddaad52a06cf9e0158ba52c9);\\n \\n \\n\\n circle_marker_56d05d6af91897c23294756b275d71f3.bindPopup(popup_fd680f316f829914ea2036d6ce8314c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_69b4aa454f3ee29afa79a067871218eb = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_1bf50892cc49230657cfd758f7dbd79b);\\n \\n \\n var popup_f5b33f4a42f23a70b977beb6ef7eaecf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d199671aceb8c5b2e486ca34af2bd0e = $(`<div id="html_9d199671aceb8c5b2e486ca34af2bd0e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f5b33f4a42f23a70b977beb6ef7eaecf.setContent(html_9d199671aceb8c5b2e486ca34af2bd0e);\\n \\n \\n\\n circle_marker_69b4aa454f3ee29afa79a067871218eb.bindPopup(popup_f5b33f4a42f23a70b977beb6ef7eaecf)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Olive, Russian\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_ef1164b00b7c29cf55ecbf3def9441f5 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_ef1164b00b7c29cf55ecbf3def9441f5" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_ef1164b00b7c29cf55ecbf3def9441f5 = L.map(\\n "map_ef1164b00b7c29cf55ecbf3def9441f5",\\n {\\n center: [42.73906582913591, -73.23719059048253],\\n crs: L.CRS.EPSG3857,\\n zoom: 15,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_0b0148058305ba9e9603150715ffd0fb = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_ef1164b00b7c29cf55ecbf3def9441f5);\\n \\n \\n var circle_marker_738d23b94bf2c3d69ca648d30037233a = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ef1164b00b7c29cf55ecbf3def9441f5);\\n \\n \\n var popup_0bad690a5c0ef1efea0089a76237f544 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edf2819d46b4e0e2bd1795209ef36c32 = $(`<div id="html_edf2819d46b4e0e2bd1795209ef36c32" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0bad690a5c0ef1efea0089a76237f544.setContent(html_edf2819d46b4e0e2bd1795209ef36c32);\\n \\n \\n\\n circle_marker_738d23b94bf2c3d69ca648d30037233a.bindPopup(popup_0bad690a5c0ef1efea0089a76237f544)\\n ;\\n\\n \\n \\n \\n var circle_marker_b52321a34ea7390a96cbdd9756eb48e2 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_ef1164b00b7c29cf55ecbf3def9441f5);\\n \\n \\n var popup_19dd6cd60aba6aba0dea01f6a6c52559 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9cd53be88c4573ae1e207518b9890299 = $(`<div id="html_9cd53be88c4573ae1e207518b9890299" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_19dd6cd60aba6aba0dea01f6a6c52559.setContent(html_9cd53be88c4573ae1e207518b9890299);\\n \\n \\n\\n circle_marker_b52321a34ea7390a96cbdd9756eb48e2.bindPopup(popup_19dd6cd60aba6aba0dea01f6a6c52559)\\n ;\\n\\n \\n \\n \\n var circle_marker_3603557e9751bb0c7cc74e0d01281eca = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_ef1164b00b7c29cf55ecbf3def9441f5);\\n \\n \\n var popup_93550188e0a9d6fd794631b344664b05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33995e0dda18f02f0608e16cb66ee87f = $(`<div id="html_33995e0dda18f02f0608e16cb66ee87f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93550188e0a9d6fd794631b344664b05.setContent(html_33995e0dda18f02f0608e16cb66ee87f);\\n \\n \\n\\n circle_marker_3603557e9751bb0c7cc74e0d01281eca.bindPopup(popup_93550188e0a9d6fd794631b344664b05)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Pine, red\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_8306fff8c169352f4d9c1bfb2cfe2c56 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_8306fff8c169352f4d9c1bfb2cfe2c56" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_8306fff8c169352f4d9c1bfb2cfe2c56 = L.map(\\n "map_8306fff8c169352f4d9c1bfb2cfe2c56",\\n {\\n center: [42.731835005502106, -73.24765171511044],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_d917cf5a24e52e34c872077515ff1fee = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_8306fff8c169352f4d9c1bfb2cfe2c56);\\n \\n \\n var circle_marker_0307f042c787400382c0514e087efaf0 = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_8306fff8c169352f4d9c1bfb2cfe2c56);\\n \\n \\n var popup_98bb491af0064cad8aba000059c28df2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_570092cfebdf03050f63f1d8b6834fc9 = $(`<div id="html_570092cfebdf03050f63f1d8b6834fc9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98bb491af0064cad8aba000059c28df2.setContent(html_570092cfebdf03050f63f1d8b6834fc9);\\n \\n \\n\\n circle_marker_0307f042c787400382c0514e087efaf0.bindPopup(popup_98bb491af0064cad8aba000059c28df2)\\n ;\\n\\n \\n \\n \\n var circle_marker_2867c1a364b558ee774339f89f7ff549 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_8306fff8c169352f4d9c1bfb2cfe2c56);\\n \\n \\n var popup_5526596624f4d905a3b480e02cba5023 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0b900e35293de97e6d65775301747c7c = $(`<div id="html_0b900e35293de97e6d65775301747c7c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5526596624f4d905a3b480e02cba5023.setContent(html_0b900e35293de97e6d65775301747c7c);\\n \\n \\n\\n circle_marker_2867c1a364b558ee774339f89f7ff549.bindPopup(popup_5526596624f4d905a3b480e02cba5023)\\n ;\\n\\n \\n \\n \\n var circle_marker_825db79d9450fb4a71521d16d7d04caa = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_8306fff8c169352f4d9c1bfb2cfe2c56);\\n \\n \\n var popup_81ee4c49cd1e8f9b1b52ee6db2faafe7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3e71efb540a374bb7c06d08f45f2c72b = $(`<div id="html_3e71efb540a374bb7c06d08f45f2c72b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81ee4c49cd1e8f9b1b52ee6db2faafe7.setContent(html_3e71efb540a374bb7c06d08f45f2c72b);\\n \\n \\n\\n circle_marker_825db79d9450fb4a71521d16d7d04caa.bindPopup(popup_81ee4c49cd1e8f9b1b52ee6db2faafe7)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Pine, white\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_c2a5229c225a480e978c0aec68cbf89c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_c2a5229c225a480e978c0aec68cbf89c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_c2a5229c225a480e978c0aec68cbf89c = L.map(\\n "map_c2a5229c225a480e978c0aec68cbf89c",\\n {\\n center: [42.73545165234559, -73.24519137568734],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_6bfc9aeab2a682730ce2a5d2423a526d = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var circle_marker_6744826175464d4c0bd0a8c919335f3b = L.circleMarker(\\n [42.74720109956733, -73.25871973049003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_7c09d0a270ab3b1a30b7a6e31676d61d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_02c235bd4b44bc569b3bc6c199c26b11 = $(`<div id="html_02c235bd4b44bc569b3bc6c199c26b11" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c09d0a270ab3b1a30b7a6e31676d61d.setContent(html_02c235bd4b44bc569b3bc6c199c26b11);\\n \\n \\n\\n circle_marker_6744826175464d4c0bd0a8c919335f3b.bindPopup(popup_7c09d0a270ab3b1a30b7a6e31676d61d)\\n ;\\n\\n \\n \\n \\n var circle_marker_3026af7b1fb9d9849858231ed542a60f = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_dc12064f7d6d324de64089c5bca99ad9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8297fd9670611ce6ee22763bb922f941 = $(`<div id="html_8297fd9670611ce6ee22763bb922f941" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dc12064f7d6d324de64089c5bca99ad9.setContent(html_8297fd9670611ce6ee22763bb922f941);\\n \\n \\n\\n circle_marker_3026af7b1fb9d9849858231ed542a60f.bindPopup(popup_dc12064f7d6d324de64089c5bca99ad9)\\n ;\\n\\n \\n \\n \\n var circle_marker_52d6c89162817d287129ecb6e4d91fe4 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_7eee120a467ebdc95cd9653148c8dc9e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_da079b24be4655ba479875f12533f410 = $(`<div id="html_da079b24be4655ba479875f12533f410" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7eee120a467ebdc95cd9653148c8dc9e.setContent(html_da079b24be4655ba479875f12533f410);\\n \\n \\n\\n circle_marker_52d6c89162817d287129ecb6e4d91fe4.bindPopup(popup_7eee120a467ebdc95cd9653148c8dc9e)\\n ;\\n\\n \\n \\n \\n var circle_marker_036e6d1889de3856ce36dd358ad76da3 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_461a2d7b6af812f30714284526b12f64 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42527add828856389324782a8b91a9d5 = $(`<div id="html_42527add828856389324782a8b91a9d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_461a2d7b6af812f30714284526b12f64.setContent(html_42527add828856389324782a8b91a9d5);\\n \\n \\n\\n circle_marker_036e6d1889de3856ce36dd358ad76da3.bindPopup(popup_461a2d7b6af812f30714284526b12f64)\\n ;\\n\\n \\n \\n \\n var circle_marker_f85084a613cec4388a5c69ebc7fc111d = L.circleMarker(\\n [42.73816517595268, -73.25502990182166],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_726b5e11f459e88ddcfb711ae9268fb3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff80783a15087b509a1869ac8389d2b2 = $(`<div id="html_ff80783a15087b509a1869ac8389d2b2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_726b5e11f459e88ddcfb711ae9268fb3.setContent(html_ff80783a15087b509a1869ac8389d2b2);\\n \\n \\n\\n circle_marker_f85084a613cec4388a5c69ebc7fc111d.bindPopup(popup_726b5e11f459e88ddcfb711ae9268fb3)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd17c3b64ed58607d1011910c7531d19 = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_f28831dc9224f42299eda41f298d93fd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cb644c2f51d44b5f0506e102fa8bda63 = $(`<div id="html_cb644c2f51d44b5f0506e102fa8bda63" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f28831dc9224f42299eda41f298d93fd.setContent(html_cb644c2f51d44b5f0506e102fa8bda63);\\n \\n \\n\\n circle_marker_fd17c3b64ed58607d1011910c7531d19.bindPopup(popup_f28831dc9224f42299eda41f298d93fd)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6f93941f2278df9ae51129547e0036d = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_6d978c174b7d7893cea9d18b4333b0ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21fbe7950b722fd4218766f0a600307d = $(`<div id="html_21fbe7950b722fd4218766f0a600307d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d978c174b7d7893cea9d18b4333b0ab.setContent(html_21fbe7950b722fd4218766f0a600307d);\\n \\n \\n\\n circle_marker_a6f93941f2278df9ae51129547e0036d.bindPopup(popup_6d978c174b7d7893cea9d18b4333b0ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_5309baca6782c233bb3274b401d8e6b8 = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_9508df6596ff79e79b9c0890b1d871eb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_61bbf5dd0d8e48bee591a24a3bec57c4 = $(`<div id="html_61bbf5dd0d8e48bee591a24a3bec57c4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9508df6596ff79e79b9c0890b1d871eb.setContent(html_61bbf5dd0d8e48bee591a24a3bec57c4);\\n \\n \\n\\n circle_marker_5309baca6782c233bb3274b401d8e6b8.bindPopup(popup_9508df6596ff79e79b9c0890b1d871eb)\\n ;\\n\\n \\n \\n \\n var circle_marker_96ff9dcb9e3f07382f77449964ffc4f4 = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_2e0f864a9a047c07bb5af10d4eb3cba7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_edb225c920d7cc36fd111bfb102fb4ab = $(`<div id="html_edb225c920d7cc36fd111bfb102fb4ab" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e0f864a9a047c07bb5af10d4eb3cba7.setContent(html_edb225c920d7cc36fd111bfb102fb4ab);\\n \\n \\n\\n circle_marker_96ff9dcb9e3f07382f77449964ffc4f4.bindPopup(popup_2e0f864a9a047c07bb5af10d4eb3cba7)\\n ;\\n\\n \\n \\n \\n var circle_marker_8020f2ec6a0c29702eb309001f1fae8d = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_164661d4893d10978b935a82e47622b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38ceeb6850fc0dd8791ab4fead6b07fe = $(`<div id="html_38ceeb6850fc0dd8791ab4fead6b07fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_164661d4893d10978b935a82e47622b5.setContent(html_38ceeb6850fc0dd8791ab4fead6b07fe);\\n \\n \\n\\n circle_marker_8020f2ec6a0c29702eb309001f1fae8d.bindPopup(popup_164661d4893d10978b935a82e47622b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_a9065249774affe11ce311c49716f000 = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_6b2e740a6202984b0d20d35c29fc8634 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6a32cd3900d3e00fcb80d9335f07fa3e = $(`<div id="html_6a32cd3900d3e00fcb80d9335f07fa3e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6b2e740a6202984b0d20d35c29fc8634.setContent(html_6a32cd3900d3e00fcb80d9335f07fa3e);\\n \\n \\n\\n circle_marker_a9065249774affe11ce311c49716f000.bindPopup(popup_6b2e740a6202984b0d20d35c29fc8634)\\n ;\\n\\n \\n \\n \\n var circle_marker_a4b2c02f381e9be4e91cf61d6f1f7923 = L.circleMarker(\\n [42.73635717353716, -73.24764895342292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_b95f0560533e1ec67fedb67449074ce5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9feb961913dc23e4827700cc72088f8e = $(`<div id="html_9feb961913dc23e4827700cc72088f8e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b95f0560533e1ec67fedb67449074ce5.setContent(html_9feb961913dc23e4827700cc72088f8e);\\n \\n \\n\\n circle_marker_a4b2c02f381e9be4e91cf61d6f1f7923.bindPopup(popup_b95f0560533e1ec67fedb67449074ce5)\\n ;\\n\\n \\n \\n \\n var circle_marker_dc06f914349ebc228b1b454a59d2a9fd = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_58a6f72e00f121a8640e35d300138e8c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a5d5069122eb9303646d54a0c7a12448 = $(`<div id="html_a5d5069122eb9303646d54a0c7a12448" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_58a6f72e00f121a8640e35d300138e8c.setContent(html_a5d5069122eb9303646d54a0c7a12448);\\n \\n \\n\\n circle_marker_dc06f914349ebc228b1b454a59d2a9fd.bindPopup(popup_58a6f72e00f121a8640e35d300138e8c)\\n ;\\n\\n \\n \\n \\n var circle_marker_9227acd6da49b6b602abc44bdbb90b1c = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_fc27f038cc0d56d87053dbea40274f72 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0dc43ffb41bb3e214078549018705322 = $(`<div id="html_0dc43ffb41bb3e214078549018705322" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc27f038cc0d56d87053dbea40274f72.setContent(html_0dc43ffb41bb3e214078549018705322);\\n \\n \\n\\n circle_marker_9227acd6da49b6b602abc44bdbb90b1c.bindPopup(popup_fc27f038cc0d56d87053dbea40274f72)\\n ;\\n\\n \\n \\n \\n var circle_marker_4825c11e5fa558170ae2775f024592e7 = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_6a18de01fb4c9beaf83acf7a719720d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d682cf1c6b7425675c90d5592de47af = $(`<div id="html_3d682cf1c6b7425675c90d5592de47af" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6a18de01fb4c9beaf83acf7a719720d5.setContent(html_3d682cf1c6b7425675c90d5592de47af);\\n \\n \\n\\n circle_marker_4825c11e5fa558170ae2775f024592e7.bindPopup(popup_6a18de01fb4c9beaf83acf7a719720d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_67584863a5c91634dd3c715413db8845 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_07d593b3a9893bc9a1f9f917c45c46a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e637496dd827f3327259c8c4baf3181 = $(`<div id="html_8e637496dd827f3327259c8c4baf3181" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07d593b3a9893bc9a1f9f917c45c46a4.setContent(html_8e637496dd827f3327259c8c4baf3181);\\n \\n \\n\\n circle_marker_67584863a5c91634dd3c715413db8845.bindPopup(popup_07d593b3a9893bc9a1f9f917c45c46a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_37b45cba231bb32d6488a7567d7ccdfb = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_db8f610a1f04d5757b851cd947941411 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38c050da29b4010b174696c67ac3efd6 = $(`<div id="html_38c050da29b4010b174696c67ac3efd6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db8f610a1f04d5757b851cd947941411.setContent(html_38c050da29b4010b174696c67ac3efd6);\\n \\n \\n\\n circle_marker_37b45cba231bb32d6488a7567d7ccdfb.bindPopup(popup_db8f610a1f04d5757b851cd947941411)\\n ;\\n\\n \\n \\n \\n var circle_marker_821135abea5d4a077389f6ed189ae3a4 = L.circleMarker(\\n [42.73454458906941, -73.22550639699884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_f84e3cde3e33062c5ee894c760611810 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a5128f3114cf94a03fc7c36fed742bb = $(`<div id="html_4a5128f3114cf94a03fc7c36fed742bb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f84e3cde3e33062c5ee894c760611810.setContent(html_4a5128f3114cf94a03fc7c36fed742bb);\\n \\n \\n\\n circle_marker_821135abea5d4a077389f6ed189ae3a4.bindPopup(popup_f84e3cde3e33062c5ee894c760611810)\\n ;\\n\\n \\n \\n \\n var circle_marker_738e428fd572039872ea319aff7c2b31 = L.circleMarker(\\n [42.73274324922224, -73.25011022554088],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_e4891624f7082e0a2333da84355fe851 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db20fd608b1a44b65afc5bb62f9a47e9 = $(`<div id="html_db20fd608b1a44b65afc5bb62f9a47e9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e4891624f7082e0a2333da84355fe851.setContent(html_db20fd608b1a44b65afc5bb62f9a47e9);\\n \\n \\n\\n circle_marker_738e428fd572039872ea319aff7c2b31.bindPopup(popup_e4891624f7082e0a2333da84355fe851)\\n ;\\n\\n \\n \\n \\n var circle_marker_ccc5d2bf3660c96b24e441cda5f4b120 = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_796df56c43939dceca5894a3f7c728e5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7ddcad52353cded69de5e0645e2dce50 = $(`<div id="html_7ddcad52353cded69de5e0645e2dce50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_796df56c43939dceca5894a3f7c728e5.setContent(html_7ddcad52353cded69de5e0645e2dce50);\\n \\n \\n\\n circle_marker_ccc5d2bf3660c96b24e441cda5f4b120.bindPopup(popup_796df56c43939dceca5894a3f7c728e5)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b23efbf3921b2668a6e3083d8621e00 = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_de9ebae29e1ba5d5e3ede6c019753c4f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_afa3f88f45726bc2699d18efba92eda8 = $(`<div id="html_afa3f88f45726bc2699d18efba92eda8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de9ebae29e1ba5d5e3ede6c019753c4f.setContent(html_afa3f88f45726bc2699d18efba92eda8);\\n \\n \\n\\n circle_marker_4b23efbf3921b2668a6e3083d8621e00.bindPopup(popup_de9ebae29e1ba5d5e3ede6c019753c4f)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5e62dc0b9123aac22a8cb5035eb591c = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_4de493f0d0b553be80367a6f5b4eb6df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_22d6e9c4bd2da892c6776a54bec8c856 = $(`<div id="html_22d6e9c4bd2da892c6776a54bec8c856" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4de493f0d0b553be80367a6f5b4eb6df.setContent(html_22d6e9c4bd2da892c6776a54bec8c856);\\n \\n \\n\\n circle_marker_a5e62dc0b9123aac22a8cb5035eb591c.bindPopup(popup_4de493f0d0b553be80367a6f5b4eb6df)\\n ;\\n\\n \\n \\n \\n var circle_marker_c2f8df5fdbc7d48c941d397082b97cfe = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_21e32ab13e4a4c2c9927fe27e3e67679 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_03a2027d1e0ab4290c155b3715edee47 = $(`<div id="html_03a2027d1e0ab4290c155b3715edee47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_21e32ab13e4a4c2c9927fe27e3e67679.setContent(html_03a2027d1e0ab4290c155b3715edee47);\\n \\n \\n\\n circle_marker_c2f8df5fdbc7d48c941d397082b97cfe.bindPopup(popup_21e32ab13e4a4c2c9927fe27e3e67679)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d1050cdeaaddf1275262e9aee4886f9 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_adfe1d89c1af01f923687ab32b91b16f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f39dfa9c9659dab0ccc3ff2957bf8e90 = $(`<div id="html_f39dfa9c9659dab0ccc3ff2957bf8e90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_adfe1d89c1af01f923687ab32b91b16f.setContent(html_f39dfa9c9659dab0ccc3ff2957bf8e90);\\n \\n \\n\\n circle_marker_5d1050cdeaaddf1275262e9aee4886f9.bindPopup(popup_adfe1d89c1af01f923687ab32b91b16f)\\n ;\\n\\n \\n \\n \\n var circle_marker_8abc4bad19ac4f2f6b627f36ed83b36c = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_95af478b7565e2b256dcc209de8cefb8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b10ff8f99eee08d2a87a6eb2de55b8e1 = $(`<div id="html_b10ff8f99eee08d2a87a6eb2de55b8e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_95af478b7565e2b256dcc209de8cefb8.setContent(html_b10ff8f99eee08d2a87a6eb2de55b8e1);\\n \\n \\n\\n circle_marker_8abc4bad19ac4f2f6b627f36ed83b36c.bindPopup(popup_95af478b7565e2b256dcc209de8cefb8)\\n ;\\n\\n \\n \\n \\n var circle_marker_956f8b2be4031ccf5fa28b1ff9b7830c = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_1659be6e50bcfe897ecccca47ec64117 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a24725427b28eaf40e26fff63cc5944 = $(`<div id="html_4a24725427b28eaf40e26fff63cc5944" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1659be6e50bcfe897ecccca47ec64117.setContent(html_4a24725427b28eaf40e26fff63cc5944);\\n \\n \\n\\n circle_marker_956f8b2be4031ccf5fa28b1ff9b7830c.bindPopup(popup_1659be6e50bcfe897ecccca47ec64117)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d86e0fbc8e319ae00b3ba3fd47569f3 = L.circleMarker(\\n [42.730934687380696, -73.24149996761956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_35921a58c5eb03fe8caf080344662b39 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c31c828af0da008a1d9aa2495fafc9fb = $(`<div id="html_c31c828af0da008a1d9aa2495fafc9fb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_35921a58c5eb03fe8caf080344662b39.setContent(html_c31c828af0da008a1d9aa2495fafc9fb);\\n \\n \\n\\n circle_marker_6d86e0fbc8e319ae00b3ba3fd47569f3.bindPopup(popup_35921a58c5eb03fe8caf080344662b39)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c7eae7604d5611af655db86e2ea9f10 = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_758aee3a9f7a84cead07d440c94543cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b893523a036684fa6dee27c736d037a0 = $(`<div id="html_b893523a036684fa6dee27c736d037a0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_758aee3a9f7a84cead07d440c94543cc.setContent(html_b893523a036684fa6dee27c736d037a0);\\n \\n \\n\\n circle_marker_7c7eae7604d5611af655db86e2ea9f10.bindPopup(popup_758aee3a9f7a84cead07d440c94543cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_e466e54d911c6c4bae5ae5bd1c8692e7 = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_b78330c1e99a2477c8b2516c367c72be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_75d02d8fabd002149f72e5a8ccb6a45b = $(`<div id="html_75d02d8fabd002149f72e5a8ccb6a45b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b78330c1e99a2477c8b2516c367c72be.setContent(html_75d02d8fabd002149f72e5a8ccb6a45b);\\n \\n \\n\\n circle_marker_e466e54d911c6c4bae5ae5bd1c8692e7.bindPopup(popup_b78330c1e99a2477c8b2516c367c72be)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ecb05b1b0edb704f7ca64300acac0d1 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_ab9188920dba027053d557e04480e757 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ff50cf660adc18798c98696cb10e249f = $(`<div id="html_ff50cf660adc18798c98696cb10e249f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ab9188920dba027053d557e04480e757.setContent(html_ff50cf660adc18798c98696cb10e249f);\\n \\n \\n\\n circle_marker_7ecb05b1b0edb704f7ca64300acac0d1.bindPopup(popup_ab9188920dba027053d557e04480e757)\\n ;\\n\\n \\n \\n \\n var circle_marker_e4a9a29e129bf246a868c69831276147 = L.circleMarker(\\n [42.72912674442964, -73.23781046140957],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_9d1182d16dffc87dfb0e7b7008a341df = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5611d9f91805b0481ba090611131db6a = $(`<div id="html_5611d9f91805b0481ba090611131db6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9d1182d16dffc87dfb0e7b7008a341df.setContent(html_5611d9f91805b0481ba090611131db6a);\\n \\n \\n\\n circle_marker_e4a9a29e129bf246a868c69831276147.bindPopup(popup_9d1182d16dffc87dfb0e7b7008a341df)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e3cab6a9d118bbe3dcd32c8f1a2b983 = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_eab475068d456fde3e32513974cef6e0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3e52cbf683f6d9648d123336c1a0c61 = $(`<div id="html_a3e52cbf683f6d9648d123336c1a0c61" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eab475068d456fde3e32513974cef6e0.setContent(html_a3e52cbf683f6d9648d123336c1a0c61);\\n \\n \\n\\n circle_marker_4e3cab6a9d118bbe3dcd32c8f1a2b983.bindPopup(popup_eab475068d456fde3e32513974cef6e0)\\n ;\\n\\n \\n \\n \\n var circle_marker_cb0b59d7797d1da883319a103be02fc0 = L.circleMarker(\\n [42.72912360447727, -73.22673990042665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_6599bdab862fb61edaac6885824b6445 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aba253b88ce6ff68ea3b62ec815ed8c9 = $(`<div id="html_aba253b88ce6ff68ea3b62ec815ed8c9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6599bdab862fb61edaac6885824b6445.setContent(html_aba253b88ce6ff68ea3b62ec815ed8c9);\\n \\n \\n\\n circle_marker_cb0b59d7797d1da883319a103be02fc0.bindPopup(popup_6599bdab862fb61edaac6885824b6445)\\n ;\\n\\n \\n \\n \\n var circle_marker_19ce953e6af35c5e295e20908b58d1ea = L.circleMarker(\\n [42.7291231897666, -73.2255098381692],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_cd428b02db921000ef46bada254ead5f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_80617a6bb6adff2887bee781b75073e6 = $(`<div id="html_80617a6bb6adff2887bee781b75073e6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cd428b02db921000ef46bada254ead5f.setContent(html_80617a6bb6adff2887bee781b75073e6);\\n \\n \\n\\n circle_marker_19ce953e6af35c5e295e20908b58d1ea.bindPopup(popup_cd428b02db921000ef46bada254ead5f)\\n ;\\n\\n \\n \\n \\n var circle_marker_a2a2e06ef0674d925b82a399e782e909 = L.circleMarker(\\n [42.72912276189051, -73.22427977592847],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_8c8991b0d2f7139bded6ceb4e3bc519c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc891e96741dab65b42dcfb920a0d483 = $(`<div id="html_cc891e96741dab65b42dcfb920a0d483" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c8991b0d2f7139bded6ceb4e3bc519c.setContent(html_cc891e96741dab65b42dcfb920a0d483);\\n \\n \\n\\n circle_marker_a2a2e06ef0674d925b82a399e782e909.bindPopup(popup_8c8991b0d2f7139bded6ceb4e3bc519c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2411de233b2f6e858282dc6c68450866 = L.circleMarker(\\n [42.72912139926977, -73.22058958931171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_a9dabfba69609f14947c33c0b28c4b65 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21f84687aa1b413c267b40b17571846f = $(`<div id="html_21f84687aa1b413c267b40b17571846f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9dabfba69609f14947c33c0b28c4b65.setContent(html_21f84687aa1b413c267b40b17571846f);\\n \\n \\n\\n circle_marker_2411de233b2f6e858282dc6c68450866.bindPopup(popup_a9dabfba69609f14947c33c0b28c4b65)\\n ;\\n\\n \\n \\n \\n var circle_marker_94a2c810f1395698ed1b9970f0dfcd2e = L.circleMarker(\\n [42.72912091873204, -73.21935952714304],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_2b30a0dc7a980c9ccf098de56b10610e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6be7b618d59d28af68dfe04c908d9e3b = $(`<div id="html_6be7b618d59d28af68dfe04c908d9e3b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2b30a0dc7a980c9ccf098de56b10610e.setContent(html_6be7b618d59d28af68dfe04c908d9e3b);\\n \\n \\n\\n circle_marker_94a2c810f1395698ed1b9970f0dfcd2e.bindPopup(popup_2b30a0dc7a980c9ccf098de56b10610e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1cac2c317d94bcfa2ef6984454db7dc = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_79d01564911ccd134359d9536990aac6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_94778390e2da62880429443a44cd8d97 = $(`<div id="html_94778390e2da62880429443a44cd8d97" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_79d01564911ccd134359d9536990aac6.setContent(html_94778390e2da62880429443a44cd8d97);\\n \\n \\n\\n circle_marker_b1cac2c317d94bcfa2ef6984454db7dc.bindPopup(popup_79d01564911ccd134359d9536990aac6)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d0220d7fd7c057baf29417145294724 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_c228259428051b33254b5e79fc19af55 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_323a3104191cdfaa21d6b04389851ee4 = $(`<div id="html_323a3104191cdfaa21d6b04389851ee4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c228259428051b33254b5e79fc19af55.setContent(html_323a3104191cdfaa21d6b04389851ee4);\\n \\n \\n\\n circle_marker_2d0220d7fd7c057baf29417145294724.bindPopup(popup_c228259428051b33254b5e79fc19af55)\\n ;\\n\\n \\n \\n \\n var circle_marker_c21c681fa3a727f6006851b4d5288e5f = L.circleMarker(\\n [42.72731562880686, -73.22428095863906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_b1581c75ec5917423d9a031561baa4e1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92933b6a2412e365162db9781ed0ebe4 = $(`<div id="html_92933b6a2412e365162db9781ed0ebe4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b1581c75ec5917423d9a031561baa4e1.setContent(html_92933b6a2412e365162db9781ed0ebe4);\\n \\n \\n\\n circle_marker_c21c681fa3a727f6006851b4d5288e5f.bindPopup(popup_b1581c75ec5917423d9a031561baa4e1)\\n ;\\n\\n \\n \\n \\n var circle_marker_91a5d4dfce8d1257a067b9c106841f81 = L.circleMarker(\\n [42.72731518778323, -73.22305093225523],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_afdb092a5d4348262af65414a2c03f8c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_01d617b3329df77a300275a3003c1bb0 = $(`<div id="html_01d617b3329df77a300275a3003c1bb0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_afdb092a5d4348262af65414a2c03f8c.setContent(html_01d617b3329df77a300275a3003c1bb0);\\n \\n \\n\\n circle_marker_91a5d4dfce8d1257a067b9c106841f81.bindPopup(popup_afdb092a5d4348262af65414a2c03f8c)\\n ;\\n\\n \\n \\n \\n var circle_marker_5af06e875fb2221cb8cf1eb31049937d = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_b37df804718ff906f821c8658729d7d4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32508a415aa13dfe35d07476285d8fea = $(`<div id="html_32508a415aa13dfe35d07476285d8fea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b37df804718ff906f821c8658729d7d4.setContent(html_32508a415aa13dfe35d07476285d8fea);\\n \\n \\n\\n circle_marker_5af06e875fb2221cb8cf1eb31049937d.bindPopup(popup_b37df804718ff906f821c8658729d7d4)\\n ;\\n\\n \\n \\n \\n var circle_marker_c347f98cf4f68e43932ce1b0e38bfdd4 = L.circleMarker(\\n [42.727314266241336, -73.22059087954139],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_b25987e04ab624d114ce90cc1811e587 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_95ea48e27460fdce80a3ca47c902f62c = $(`<div id="html_95ea48e27460fdce80a3ca47c902f62c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b25987e04ab624d114ce90cc1811e587.setContent(html_95ea48e27460fdce80a3ca47c902f62c);\\n \\n \\n\\n circle_marker_c347f98cf4f68e43932ce1b0e38bfdd4.bindPopup(popup_b25987e04ab624d114ce90cc1811e587)\\n ;\\n\\n \\n \\n \\n var circle_marker_98fd06d0c7a8c1dd5ef160d75520e5fd = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_8aa9765e57924eeb17da01d45102de70 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d63fe3e1a3081f2cd83208592d23e910 = $(`<div id="html_d63fe3e1a3081f2cd83208592d23e910" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8aa9765e57924eeb17da01d45102de70.setContent(html_d63fe3e1a3081f2cd83208592d23e910);\\n \\n \\n\\n circle_marker_98fd06d0c7a8c1dd5ef160d75520e5fd.bindPopup(popup_8aa9765e57924eeb17da01d45102de70)\\n ;\\n\\n \\n \\n \\n var circle_marker_d45cd0119949bd029dc54cfc6a30df1a = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_38fa61d78c74b4f2ff18462aa10ec787 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_caa9d5e0e71d971a87ab1087119e75d5 = $(`<div id="html_caa9d5e0e71d971a87ab1087119e75d5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38fa61d78c74b4f2ff18462aa10ec787.setContent(html_caa9d5e0e71d971a87ab1087119e75d5);\\n \\n \\n\\n circle_marker_d45cd0119949bd029dc54cfc6a30df1a.bindPopup(popup_38fa61d78c74b4f2ff18462aa10ec787)\\n ;\\n\\n \\n \\n \\n var circle_marker_31bc3000ddbb61caeaa8688244463872 = L.circleMarker(\\n [42.72370220512386, -73.22674323325023],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_c2a5229c225a480e978c0aec68cbf89c);\\n \\n \\n var popup_32d2fa504ef54c17133c4ae25a8ee15c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e88070166e38a183743bb5c9f671411b = $(`<div id="html_e88070166e38a183743bb5c9f671411b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_32d2fa504ef54c17133c4ae25a8ee15c.setContent(html_e88070166e38a183743bb5c9f671411b);\\n \\n \\n\\n circle_marker_31bc3000ddbb61caeaa8688244463872.bindPopup(popup_32d2fa504ef54c17133c4ae25a8ee15c)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Plantation spruce": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_211122d68656370f1a7c842881cc155c {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_211122d68656370f1a7c842881cc155c" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_211122d68656370f1a7c842881cc155c = L.map(\\n "map_211122d68656370f1a7c842881cc155c",\\n {\\n center: [42.72731834735562, -73.23289114377901],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_46ec512d12be5262da2dc8a50ca90fe5 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_211122d68656370f1a7c842881cc155c);\\n \\n \\n var circle_marker_7c67f5383b91d817e65a739a910d17be = L.circleMarker(\\n [42.72731834735562, -73.23289114377901],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_211122d68656370f1a7c842881cc155c);\\n \\n \\n var popup_8c90d31a57f7ac0e922343849f9dea9a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36c29d375498b6928d03390c6a547e79 = $(`<div id="html_36c29d375498b6928d03390c6a547e79" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c90d31a57f7ac0e922343849f9dea9a.setContent(html_36c29d375498b6928d03390c6a547e79);\\n \\n \\n\\n circle_marker_7c67f5383b91d817e65a739a910d17be.bindPopup(popup_8c90d31a57f7ac0e922343849f9dea9a)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Plot converted to meadow": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_4ec78e8e15dfc383b5ca4bc3368316b5 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_4ec78e8e15dfc383b5ca4bc3368316b5" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_4ec78e8e15dfc383b5ca4bc3368316b5 = L.map(\\n "map_4ec78e8e15dfc383b5ca4bc3368316b5",\\n {\\n center: [42.72731706707053, -73.22858605111662],\\n crs: L.CRS.EPSG3857,\\n zoom: 18,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_d8ad45f534ec6f77fdbaaeb1ce728766 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 20, "maxZoom": 20, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_4ec78e8e15dfc383b5ca4bc3368316b5);\\n \\n \\n var circle_marker_e6319002bb1c5182d45c4cac0f5cf8e4 = L.circleMarker(\\n [42.72731726125259, -73.22920106434142],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4ec78e8e15dfc383b5ca4bc3368316b5);\\n \\n \\n var popup_c9f62aad1629e206d145ca290a7facd5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1d3b109f17191d1e1cd746d38abc3e7 = $(`<div id="html_a1d3b109f17191d1e1cd746d38abc3e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9f62aad1629e206d145ca290a7facd5.setContent(html_a1d3b109f17191d1e1cd746d38abc3e7);\\n \\n \\n\\n circle_marker_e6319002bb1c5182d45c4cac0f5cf8e4.bindPopup(popup_c9f62aad1629e206d145ca290a7facd5)\\n ;\\n\\n \\n \\n \\n var circle_marker_dee49904d54301ebc77e0f9f497548c3 = L.circleMarker(\\n [42.72731687288848, -73.2279710378918],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_4ec78e8e15dfc383b5ca4bc3368316b5);\\n \\n \\n var popup_5c613aa2be5a9711d68cd0e28cf5d1fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f48e4f005ccbfbaeecc6d206a0b7c1c2 = $(`<div id="html_f48e4f005ccbfbaeecc6d206a0b7c1c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c613aa2be5a9711d68cd0e28cf5d1fa.setContent(html_f48e4f005ccbfbaeecc6d206a0b7c1c2);\\n \\n \\n\\n circle_marker_dee49904d54301ebc77e0f9f497548c3.bindPopup(popup_5c613aa2be5a9711d68cd0e28cf5d1fa)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Poplar species": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_2cb6f01edd0d163f78187e3e8dcdc14e {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_2cb6f01edd0d163f78187e3e8dcdc14e" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_2cb6f01edd0d163f78187e3e8dcdc14e = L.map(\\n "map_2cb6f01edd0d163f78187e3e8dcdc14e",\\n {\\n center: [42.72551012810279, -73.22920210360903],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_7230581a6cb997ede2ef3ba07902f281 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_2cb6f01edd0d163f78187e3e8dcdc14e);\\n \\n \\n var circle_marker_886e0ee22db0bbecea4a76f3f26195b7 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_2cb6f01edd0d163f78187e3e8dcdc14e);\\n \\n \\n var popup_4a10f16ccfa0be5f16879fcb966325a7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1fcf1103b682ed11e879236c21840e32 = $(`<div id="html_1fcf1103b682ed11e879236c21840e32" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a10f16ccfa0be5f16879fcb966325a7.setContent(html_1fcf1103b682ed11e879236c21840e32);\\n \\n \\n\\n circle_marker_886e0ee22db0bbecea4a76f3f26195b7.bindPopup(popup_4a10f16ccfa0be5f16879fcb966325a7)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Poplar, hybrid in plantation\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_21c6eb52fd914dafd628b1622f6aaeb5 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_21c6eb52fd914dafd628b1622f6aaeb5" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_21c6eb52fd914dafd628b1622f6aaeb5 = L.map(\\n "map_21c6eb52fd914dafd628b1622f6aaeb5",\\n {\\n center: [42.725509338241686, -73.22674212239906],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_f1583fb70cf6baf195b479b22cd8bc0b = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_21c6eb52fd914dafd628b1622f6aaeb5);\\n \\n \\n var circle_marker_383e5e16a725848ab63ddbfb54d33797 = L.circleMarker(\\n [42.725509338241686, -73.22674212239906],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_21c6eb52fd914dafd628b1622f6aaeb5);\\n \\n \\n var popup_f9d5ad78abd7fc5258c1b0194deb4117 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ee94c01c2c6f4aa46fe4e4a88c533c8 = $(`<div id="html_4ee94c01c2c6f4aa46fe4e4a88c533c8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9d5ad78abd7fc5258c1b0194deb4117.setContent(html_4ee94c01c2c6f4aa46fe4e4a88c533c8);\\n \\n \\n\\n circle_marker_383e5e16a725848ab63ddbfb54d33797.bindPopup(popup_f9d5ad78abd7fc5258c1b0194deb4117)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Privet": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_f1717268793dc63265166904c8ad8300 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_f1717268793dc63265166904c8ad8300" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_f1717268793dc63265166904c8ad8300 = L.map(\\n "map_f1717268793dc63265166904c8ad8300",\\n {\\n center: [42.73906582913591, -73.23719100283978],\\n crs: L.CRS.EPSG3857,\\n zoom: 15,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_8ea9e58f5036d29a85b33f10aa6513b8 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_f1717268793dc63265166904c8ad8300);\\n \\n \\n var circle_marker_a17d90355c357bd58d915112364d6e2a = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f1717268793dc63265166904c8ad8300);\\n \\n \\n var popup_214593bc54fd8b1cf5acd44c9c42d5ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_be782d5d88d13a5c51625c4d1008ba1c = $(`<div id="html_be782d5d88d13a5c51625c4d1008ba1c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_214593bc54fd8b1cf5acd44c9c42d5ed.setContent(html_be782d5d88d13a5c51625c4d1008ba1c);\\n \\n \\n\\n circle_marker_a17d90355c357bd58d915112364d6e2a.bindPopup(popup_214593bc54fd8b1cf5acd44c9c42d5ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_da8393bab9987632b22c87f2c5cb01d6 = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f1717268793dc63265166904c8ad8300);\\n \\n \\n var popup_8c24a2aa25f0148aa7dfca47658f0034 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_12fe52b0c6acd5a86dfebd40df77a3b8 = $(`<div id="html_12fe52b0c6acd5a86dfebd40df77a3b8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8c24a2aa25f0148aa7dfca47658f0034.setContent(html_12fe52b0c6acd5a86dfebd40df77a3b8);\\n \\n \\n\\n circle_marker_da8393bab9987632b22c87f2c5cb01d6.bindPopup(popup_8c24a2aa25f0148aa7dfca47658f0034)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Rose": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_c0f3b170adb674cae5316a0c408d7658 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_c0f3b170adb674cae5316a0c408d7658" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_c0f3b170adb674cae5316a0c408d7658 = L.map(\\n "map_c0f3b170adb674cae5316a0c408d7658",\\n {\\n center: [42.736354671702045, -73.23534689534263],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_3382c7f48a6d5e66dab1face2d4f7392 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_c0f3b170adb674cae5316a0c408d7658);\\n \\n \\n var circle_marker_1cc60f8857c82867f809e31cae5e6e1f = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5231325220201604, "stroke": true, "weight": 3}\\n ).addTo(map_c0f3b170adb674cae5316a0c408d7658);\\n \\n \\n var popup_f63e1598ac6cbcd88a66fa8821924781 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_66ae5986de3981e5419494ec37787766 = $(`<div id="html_66ae5986de3981e5419494ec37787766" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f63e1598ac6cbcd88a66fa8821924781.setContent(html_66ae5986de3981e5419494ec37787766);\\n \\n \\n\\n circle_marker_1cc60f8857c82867f809e31cae5e6e1f.bindPopup(popup_f63e1598ac6cbcd88a66fa8821924781)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Rose, multiflora\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_fc13842f3e2195b419a2292212ebbfb1 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_fc13842f3e2195b419a2292212ebbfb1" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_fc13842f3e2195b419a2292212ebbfb1 = L.map(\\n "map_fc13842f3e2195b419a2292212ebbfb1",\\n {\\n center: [42.735452115722055, -73.23842163893437],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_636ef061d2e1da57bdf0ebdd5ef5e53e = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var circle_marker_e8fa4a0bd851a8825f6422d05005cff2 = L.circleMarker(\\n [42.73997035376949, -73.24149656174208],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_a9d411ef0dbee5c9081dfa559492ce78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_92c7753565c9459cc7fd16c578e1bc2b = $(`<div id="html_92c7753565c9459cc7fd16c578e1bc2b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9d411ef0dbee5c9081dfa559492ce78.setContent(html_92c7753565c9459cc7fd16c578e1bc2b);\\n \\n \\n\\n circle_marker_e8fa4a0bd851a8825f6422d05005cff2.bindPopup(popup_a9d411ef0dbee5c9081dfa559492ce78)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e9e2141df51df474b930919dd301f24 = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.753627391751592, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_9f035f8d052247b2ba63e79bbfc0c4de = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f722ed9cc9ca69c7e15f9d2f0f3e6ea = $(`<div id="html_3f722ed9cc9ca69c7e15f9d2f0f3e6ea" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f035f8d052247b2ba63e79bbfc0c4de.setContent(html_3f722ed9cc9ca69c7e15f9d2f0f3e6ea);\\n \\n \\n\\n circle_marker_1e9e2141df51df474b930919dd301f24.bindPopup(popup_9f035f8d052247b2ba63e79bbfc0c4de)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b3543458fe2e39b4cfff2a5fc919693 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.820437914901168, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_f0c06c6e701a6382a5c18a6dcbdba87d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4b9813f5eb48f4955ee16a455664d339 = $(`<div id="html_4b9813f5eb48f4955ee16a455664d339" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f0c06c6e701a6382a5c18a6dcbdba87d.setContent(html_4b9813f5eb48f4955ee16a455664d339);\\n \\n \\n\\n circle_marker_6b3543458fe2e39b4cfff2a5fc919693.bindPopup(popup_f0c06c6e701a6382a5c18a6dcbdba87d)\\n ;\\n\\n \\n \\n \\n var circle_marker_1d65b14d693734b600c1a9d213bed014 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_33b46412b12b6920b21d99454e0b62cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38416a37fa72807367eff37326222e26 = $(`<div id="html_38416a37fa72807367eff37326222e26" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_33b46412b12b6920b21d99454e0b62cd.setContent(html_38416a37fa72807367eff37326222e26);\\n \\n \\n\\n circle_marker_1d65b14d693734b600c1a9d213bed014.bindPopup(popup_33b46412b12b6920b21d99454e0b62cd)\\n ;\\n\\n \\n \\n \\n var circle_marker_6d90ad9ef869745dcde196931a314c35 = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.029119531035698, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_c041effce50c83321e95c40b9a86fd5f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c42fbe7a5468062b67a24aeb678d1695 = $(`<div id="html_c42fbe7a5468062b67a24aeb678d1695" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c041effce50c83321e95c40b9a86fd5f.setContent(html_c42fbe7a5468062b67a24aeb678d1695);\\n \\n \\n\\n circle_marker_6d90ad9ef869745dcde196931a314c35.bindPopup(popup_c041effce50c83321e95c40b9a86fd5f)\\n ;\\n\\n \\n \\n \\n var circle_marker_f59fcc1185e6e4199c73dc40311f7e53 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_8ead5a8e401ed364104b684e5baa69cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6662345251984ac227b84e84e24f9098 = $(`<div id="html_6662345251984ac227b84e84e24f9098" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ead5a8e401ed364104b684e5baa69cf.setContent(html_6662345251984ac227b84e84e24f9098);\\n \\n \\n\\n circle_marker_f59fcc1185e6e4199c73dc40311f7e53.bindPopup(popup_8ead5a8e401ed364104b684e5baa69cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_bad68e32f20f0c58f73b48b6b9f77ad9 = L.circleMarker(\\n [42.73816296371405, -73.24026700136861],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.840435166640879, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_2e3c1ab3ecf4a6c98c1b6e97cdcb0960 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c6ee64ab2f3c30acc4ca5f20ee984333 = $(`<div id="html_c6ee64ab2f3c30acc4ca5f20ee984333" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e3c1ab3ecf4a6c98c1b6e97cdcb0960.setContent(html_c6ee64ab2f3c30acc4ca5f20ee984333);\\n \\n \\n\\n circle_marker_bad68e32f20f0c58f73b48b6b9f77ad9.bindPopup(popup_2e3c1ab3ecf4a6c98c1b6e97cdcb0960)\\n ;\\n\\n \\n \\n \\n var circle_marker_a0970b0523f00ab0029b0796fd93624e = L.circleMarker(\\n [42.73816269376828, -73.23903675971965],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 9.0270333367641, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_e198f45c287142edee84b1c09c289ff0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49b111bb495baa98ee6693934299e788 = $(`<div id="html_49b111bb495baa98ee6693934299e788" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e198f45c287142edee84b1c09c289ff0.setContent(html_49b111bb495baa98ee6693934299e788);\\n \\n \\n\\n circle_marker_a0970b0523f00ab0029b0796fd93624e.bindPopup(popup_e198f45c287142edee84b1c09c289ff0)\\n ;\\n\\n \\n \\n \\n var circle_marker_302e02522362b34970bb5b7cbc7cd68b = L.circleMarker(\\n [42.73816241065441, -73.23780651808168],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 8.234075889688375, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_a2bd08bcf14761806db50e447e7988eb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_846e4910c71afa9825b6b034fa22d71d = $(`<div id="html_846e4910c71afa9825b6b034fa22d71d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a2bd08bcf14761806db50e447e7988eb.setContent(html_846e4910c71afa9825b6b034fa22d71d);\\n \\n \\n\\n circle_marker_302e02522362b34970bb5b7cbc7cd68b.bindPopup(popup_a2bd08bcf14761806db50e447e7988eb)\\n ;\\n\\n \\n \\n \\n var circle_marker_024fe1e38eadc895577f8ca16292d1ea = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_4ddf814a97b5defcb369c39f00724dfc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4dd75079e3e745313c8035223d891ee6 = $(`<div id="html_4dd75079e3e745313c8035223d891ee6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4ddf814a97b5defcb369c39f00724dfc.setContent(html_4dd75079e3e745313c8035223d891ee6);\\n \\n \\n\\n circle_marker_024fe1e38eadc895577f8ca16292d1ea.bindPopup(popup_4ddf814a97b5defcb369c39f00724dfc)\\n ;\\n\\n \\n \\n \\n var circle_marker_d5ee7e93fc70fcc8c29ea50df17aa793 = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_41b58486aa5b15fa9ef09b79d4ff28e7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_84d0adf8c8b0cf4c07b0987489775bd6 = $(`<div id="html_84d0adf8c8b0cf4c07b0987489775bd6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41b58486aa5b15fa9ef09b79d4ff28e7.setContent(html_84d0adf8c8b0cf4c07b0987489775bd6);\\n \\n \\n\\n circle_marker_d5ee7e93fc70fcc8c29ea50df17aa793.bindPopup(popup_41b58486aa5b15fa9ef09b79d4ff28e7)\\n ;\\n\\n \\n \\n \\n var circle_marker_014c48219d80d314f9736a4314bc920e = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_7ae35c3f40e4d004ace23159260cc6f4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_aa616f65ed073ebfb55f978fd183251a = $(`<div id="html_aa616f65ed073ebfb55f978fd183251a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7ae35c3f40e4d004ace23159260cc6f4.setContent(html_aa616f65ed073ebfb55f978fd183251a);\\n \\n \\n\\n circle_marker_014c48219d80d314f9736a4314bc920e.bindPopup(popup_7ae35c3f40e4d004ace23159260cc6f4)\\n ;\\n\\n \\n \\n \\n var circle_marker_215d27e7c6f6472424cc3d03498c056f = L.circleMarker(\\n [42.734548427255454, -73.23903826553698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.393653682408596, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_49e97cb6e5ac6972ec74d4e56deb4926 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_11a8d5daa7c1b610e822a920bc877ead = $(`<div id="html_11a8d5daa7c1b610e822a920bc877ead" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_49e97cb6e5ac6972ec74d4e56deb4926.setContent(html_11a8d5daa7c1b610e822a920bc877ead);\\n \\n \\n\\n circle_marker_215d27e7c6f6472424cc3d03498c056f.bindPopup(popup_49e97cb6e5ac6972ec74d4e56deb4926)\\n ;\\n\\n \\n \\n \\n var circle_marker_74204103a1829084fd9aacf826d7af56 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_ddd980ec22f245ce53617561c131679b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f0348b7a70b4249967232c82d2f0af1 = $(`<div id="html_3f0348b7a70b4249967232c82d2f0af1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ddd980ec22f245ce53617561c131679b.setContent(html_3f0348b7a70b4249967232c82d2f0af1);\\n \\n \\n\\n circle_marker_74204103a1829084fd9aacf826d7af56.bindPopup(popup_ddd980ec22f245ce53617561c131679b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9cf73a73a06c3a83f04c58b1bc69c06c = L.circleMarker(\\n [42.73274101091957, -73.23780888427015],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_6e92cf65b92091731e39e586f2bc7b3b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7bcb906770527465c31a2bd8de143964 = $(`<div id="html_7bcb906770527465c31a2bd8de143964" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e92cf65b92091731e39e586f2bc7b3b.setContent(html_7bcb906770527465c31a2bd8de143964);\\n \\n \\n\\n circle_marker_9cf73a73a06c3a83f04c58b1bc69c06c.bindPopup(popup_6e92cf65b92091731e39e586f2bc7b3b)\\n ;\\n\\n \\n \\n \\n var circle_marker_515ca6b28a3e124f244bedec90b08303 = L.circleMarker(\\n [42.73274071467364, -73.23657875019765],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.9544100476116797, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_df5bdd055f8cc218fc961ed935f07bb3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_806a10f23c9603f9c2a411fa23fe764b = $(`<div id="html_806a10f23c9603f9c2a411fa23fe764b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_df5bdd055f8cc218fc961ed935f07bb3.setContent(html_806a10f23c9603f9c2a411fa23fe764b);\\n \\n \\n\\n circle_marker_515ca6b28a3e124f244bedec90b08303.bindPopup(popup_df5bdd055f8cc218fc961ed935f07bb3)\\n ;\\n\\n \\n \\n \\n var circle_marker_4b2da5c583d8df618e462f952e8f7fb5 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_fc13842f3e2195b419a2292212ebbfb1);\\n \\n \\n var popup_1b6c02a307a4e020cdb7cb803defd458 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2a4c202c57cddf7f393cad1771b54a6d = $(`<div id="html_2a4c202c57cddf7f393cad1771b54a6d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b6c02a307a4e020cdb7cb803defd458.setContent(html_2a4c202c57cddf7f393cad1771b54a6d);\\n \\n \\n\\n circle_marker_4b2da5c583d8df618e462f952e8f7fb5.bindPopup(popup_1b6c02a307a4e020cdb7cb803defd458)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Saphireberry": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_660819e22dc52385496a2e8d4ad2ad27 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_660819e22dc52385496a2e8d4ad2ad27" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_660819e22dc52385496a2e8d4ad2ad27 = L.map(\\n "map_660819e22dc52385496a2e8d4ad2ad27",\\n {\\n center: [42.72551050328681, -73.23043209423697],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_a6c67ea749de55edee100a41b1c4664f = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_660819e22dc52385496a2e8d4ad2ad27);\\n \\n \\n var circle_marker_06a10d5dd327f7de4276aca24f3a3acd = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_660819e22dc52385496a2e8d4ad2ad27);\\n \\n \\n var popup_8b65b10c5b54656b1e6c23eefe1d7338 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8b42afefc4eb8a1299d6762c32122b6f = $(`<div id="html_8b42afefc4eb8a1299d6762c32122b6f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b65b10c5b54656b1e6c23eefe1d7338.setContent(html_8b42afefc4eb8a1299d6762c32122b6f);\\n \\n \\n\\n circle_marker_06a10d5dd327f7de4276aca24f3a3acd.bindPopup(popup_8b65b10c5b54656b1e6c23eefe1d7338)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Shadbush": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_f63e623fcf6aa61a0d3c6da4c4eeff8d {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_f63e623fcf6aa61a0d3c6da4c4eeff8d" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_f63e623fcf6aa61a0d3c6da4c4eeff8d = L.map(\\n "map_f63e623fcf6aa61a0d3c6da4c4eeff8d",\\n {\\n center: [42.735452126284684, -73.24887665172076],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_f8e9d7513172b15ac1421afdb88a48d5 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var circle_marker_77976bbedd8c4200abfb02c356a82ddc = L.circleMarker(\\n [42.74720073078616, -73.27594562544621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_aa77d5e29dce94cae359a6466230c1d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7dec32503cb7c8b162f102f7fd2b2e43 = $(`<div id="html_7dec32503cb7c8b162f102f7fd2b2e43" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aa77d5e29dce94cae359a6466230c1d1.setContent(html_7dec32503cb7c8b162f102f7fd2b2e43);\\n \\n \\n\\n circle_marker_77976bbedd8c4200abfb02c356a82ddc.bindPopup(popup_aa77d5e29dce94cae359a6466230c1d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c3bfa90a68b8143a4128147b63c3660 = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_984e90433c07d3c143aaf5fb256ba0a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9dd9cb66279fa402b29817735e4ae806 = $(`<div id="html_9dd9cb66279fa402b29817735e4ae806" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_984e90433c07d3c143aaf5fb256ba0a1.setContent(html_9dd9cb66279fa402b29817735e4ae806);\\n \\n \\n\\n circle_marker_0c3bfa90a68b8143a4128147b63c3660.bindPopup(popup_984e90433c07d3c143aaf5fb256ba0a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e6b532c2995aef833fdbba72bdb41e2 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.763953195770684, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a09b1c820e45d3563147ac74cc67c352 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1f4cfb146f57376940f7f3a0ffde485 = $(`<div id="html_a1f4cfb146f57376940f7f3a0ffde485" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a09b1c820e45d3563147ac74cc67c352.setContent(html_a1f4cfb146f57376940f7f3a0ffde485);\\n \\n \\n\\n circle_marker_4e6b532c2995aef833fdbba72bdb41e2.bindPopup(popup_a09b1c820e45d3563147ac74cc67c352)\\n ;\\n\\n \\n \\n \\n var circle_marker_d14d4916793cde1c0cdd968f329257bb = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_e3e84ad3bc0a5b3ac0f82ffc805354c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_300a38dc60e93406f4e318c76446fce0 = $(`<div id="html_300a38dc60e93406f4e318c76446fce0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e3e84ad3bc0a5b3ac0f82ffc805354c5.setContent(html_300a38dc60e93406f4e318c76446fce0);\\n \\n \\n\\n circle_marker_d14d4916793cde1c0cdd968f329257bb.bindPopup(popup_e3e84ad3bc0a5b3ac0f82ffc805354c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_113146239d82187680486580a1096f32 = L.circleMarker(\\n [42.74720123786026, -73.26733267799375],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_dd9033f30b7b59a00e10b9a799d6c37b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0174687b0d525b0dd079d4c277283b28 = $(`<div id="html_0174687b0d525b0dd079d4c277283b28" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd9033f30b7b59a00e10b9a799d6c37b.setContent(html_0174687b0d525b0dd079d4c277283b28);\\n \\n \\n\\n circle_marker_113146239d82187680486580a1096f32.bindPopup(popup_dd9033f30b7b59a00e10b9a799d6c37b)\\n ;\\n\\n \\n \\n \\n var circle_marker_a6bd6783c62fa08a86aeb9a24cda337b = L.circleMarker(\\n [42.74720125761639, -73.26610225692073],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_2258b0213c98cd9fbbf3750d9f402fb2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_595f5355abf53b71a747025419bb0ec9 = $(`<div id="html_595f5355abf53b71a747025419bb0ec9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2258b0213c98cd9fbbf3750d9f402fb2.setContent(html_595f5355abf53b71a747025419bb0ec9);\\n \\n \\n\\n circle_marker_a6bd6783c62fa08a86aeb9a24cda337b.bindPopup(popup_2258b0213c98cd9fbbf3750d9f402fb2)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c8115f3de56f29aaec11ecf131ffcdc = L.circleMarker(\\n [42.74720115883572, -73.25995015155833],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_8f5b04d7828ae63a442da99af7242da7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7862c5949d1467cb6053c9219f28a977 = $(`<div id="html_7862c5949d1467cb6053c9219f28a977" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f5b04d7828ae63a442da99af7242da7.setContent(html_7862c5949d1467cb6053c9219f28a977);\\n \\n \\n\\n circle_marker_7c8115f3de56f29aaec11ecf131ffcdc.bindPopup(popup_8f5b04d7828ae63a442da99af7242da7)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e8c2726f497a7f348cdfbe0cf6cb0b1 = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_37ff4992f4b2d0e0e0b088245398506d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7137f355082d2741de525615248374b5 = $(`<div id="html_7137f355082d2741de525615248374b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_37ff4992f4b2d0e0e0b088245398506d.setContent(html_7137f355082d2741de525615248374b5);\\n \\n \\n\\n circle_marker_5e8c2726f497a7f348cdfbe0cf6cb0b1.bindPopup(popup_37ff4992f4b2d0e0e0b088245398506d)\\n ;\\n\\n \\n \\n \\n var circle_marker_8af37fcb4f9bc04fee18541b768c7538 = L.circleMarker(\\n [42.74720094151825, -73.2562588883618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_b950b609d4cf55218de81c4394efe7a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bb6d14af7e6575f3a049f2ccf769c936 = $(`<div id="html_bb6d14af7e6575f3a049f2ccf769c936" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b950b609d4cf55218de81c4394efe7a0.setContent(html_bb6d14af7e6575f3a049f2ccf769c936);\\n \\n \\n\\n circle_marker_8af37fcb4f9bc04fee18541b768c7538.bindPopup(popup_b950b609d4cf55218de81c4394efe7a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_085a2df6e2b52875af9c3255c3b7a2ab = L.circleMarker(\\n [42.747200842737584, -73.25502846730292],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_8949e3c409b6cc6fe6e8c44838932176 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a1227a0ba084dd87a64fcc5f0825112b = $(`<div id="html_a1227a0ba084dd87a64fcc5f0825112b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8949e3c409b6cc6fe6e8c44838932176.setContent(html_a1227a0ba084dd87a64fcc5f0825112b);\\n \\n \\n\\n circle_marker_085a2df6e2b52875af9c3255c3b7a2ab.bindPopup(popup_8949e3c409b6cc6fe6e8c44838932176)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa727f68572f95a0a9cdf28764212f75 = L.circleMarker(\\n [42.74539412424261, -73.26610222105195],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_7089df1b57bc00d2ed97ad7658516858 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b2202dac241c588832f37b833545fee8 = $(`<div id="html_b2202dac241c588832f37b833545fee8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7089df1b57bc00d2ed97ad7658516858.setContent(html_b2202dac241c588832f37b833545fee8);\\n \\n \\n\\n circle_marker_aa727f68572f95a0a9cdf28764212f75.bindPopup(popup_7089df1b57bc00d2ed97ad7658516858)\\n ;\\n\\n \\n \\n \\n var circle_marker_fb113a3e5ee91d3799d8703a8a38bc86 = L.circleMarker(\\n [42.745394025465934, -73.25995029503346],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_37023da4bcedf80896846d26a1ed622d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6b316621a3ab7c038bab4416086f52cc = $(`<div id="html_6b316621a3ab7c038bab4416086f52cc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_37023da4bcedf80896846d26a1ed622d.setContent(html_6b316621a3ab7c038bab4416086f52cc);\\n \\n \\n\\n circle_marker_fb113a3e5ee91d3799d8703a8a38bc86.bindPopup(popup_37023da4bcedf80896846d26a1ed622d)\\n ;\\n\\n \\n \\n \\n var circle_marker_00837f35d3dc37ae3190efd1f960f418 = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_9e54d0a2c7c63cccca8b389a8c304809 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a49dd0f3ce7b9b99773f2801e4c1476 = $(`<div id="html_8a49dd0f3ce7b9b99773f2801e4c1476" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9e54d0a2c7c63cccca8b389a8c304809.setContent(html_8a49dd0f3ce7b9b99773f2801e4c1476);\\n \\n \\n\\n circle_marker_00837f35d3dc37ae3190efd1f960f418.bindPopup(popup_9e54d0a2c7c63cccca8b389a8c304809)\\n ;\\n\\n \\n \\n \\n var circle_marker_02b56840e15de335572c8f1e4a642361 = L.circleMarker(\\n [42.74539370938061, -73.25502875425317],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_4f7395c289b4235c485bec1d1f12dc16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3fb79a04b6c3280c242b4751e892942e = $(`<div id="html_3fb79a04b6c3280c242b4751e892942e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4f7395c289b4235c485bec1d1f12dc16.setContent(html_3fb79a04b6c3280c242b4751e892942e);\\n \\n \\n\\n circle_marker_02b56840e15de335572c8f1e4a642361.bindPopup(popup_4f7395c289b4235c485bec1d1f12dc16)\\n ;\\n\\n \\n \\n \\n var circle_marker_34a79cd131ccb354d3d0c217714bf9ea = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_22507d0ea8a4549aafb1b9cd27daabe9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0abe9832c239e1c7c1ebf4c4148c3b90 = $(`<div id="html_0abe9832c239e1c7c1ebf4c4148c3b90" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_22507d0ea8a4549aafb1b9cd27daabe9.setContent(html_0abe9832c239e1c7c1ebf4c4148c3b90);\\n \\n \\n\\n circle_marker_34a79cd131ccb354d3d0c217714bf9ea.bindPopup(popup_22507d0ea8a4549aafb1b9cd27daabe9)\\n ;\\n\\n \\n \\n \\n var circle_marker_42315c1642c6bd87954ef6a161d5283a = L.circleMarker(\\n [42.74358699086881, -73.26610218518609],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_902ec290baf1b9f610f3e09059224f16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_958341041bb62c03f3dcc1d0e14feb8c = $(`<div id="html_958341041bb62c03f3dcc1d0e14feb8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_902ec290baf1b9f610f3e09059224f16.setContent(html_958341041bb62c03f3dcc1d0e14feb8c);\\n \\n \\n\\n circle_marker_42315c1642c6bd87954ef6a161d5283a.bindPopup(popup_902ec290baf1b9f610f3e09059224f16)\\n ;\\n\\n \\n \\n \\n var circle_marker_b64d81045902cc6f3736db47e4c83eb6 = L.circleMarker(\\n [42.741779330728825, -73.27594465706757],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_f9cf8b413915a108e0181c528fe6f20d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b55dbb7cf425ab21fd7f8e950575206f = $(`<div id="html_b55dbb7cf425ab21fd7f8e950575206f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f9cf8b413915a108e0181c528fe6f20d.setContent(html_b55dbb7cf425ab21fd7f8e950575206f);\\n \\n \\n\\n circle_marker_b64d81045902cc6f3736db47e4c83eb6.bindPopup(popup_f9cf8b413915a108e0181c528fe6f20d)\\n ;\\n\\n \\n \\n \\n var circle_marker_13e80b33c8481a996e73c257ce789782 = L.circleMarker(\\n [42.74177985749502, -73.2661021493231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_984be08c252848b2e2adac3f4b163eec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07d4e1f8711282cab5a3b21318e22dda = $(`<div id="html_07d4e1f8711282cab5a3b21318e22dda" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_984be08c252848b2e2adac3f4b163eec.setContent(html_07d4e1f8711282cab5a3b21318e22dda);\\n \\n \\n\\n circle_marker_13e80b33c8481a996e73c257ce789782.bindPopup(popup_984be08c252848b2e2adac3f4b163eec)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3fbc2739e089df05865130e22142792 = L.circleMarker(\\n [42.73997240807432, -73.2734837791288],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_71d5cb202769961cecbd76d4526af709 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8eb8b81ec4c5c0d1ad363bc74cf82597 = $(`<div id="html_8eb8b81ec4c5c0d1ad363bc74cf82597" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71d5cb202769961cecbd76d4526af709.setContent(html_8eb8b81ec4c5c0d1ad363bc74cf82597);\\n \\n \\n\\n circle_marker_f3fbc2739e089df05865130e22142792.bindPopup(popup_71d5cb202769961cecbd76d4526af709)\\n ;\\n\\n \\n \\n \\n var circle_marker_f1211c4028e36f5f42c4db5355245060 = L.circleMarker(\\n [42.73997249367036, -73.27225350152393],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_dafef290fad5beebf29b4afb598e23c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2c449065a2742961fe3806479eec9439 = $(`<div id="html_2c449065a2742961fe3806479eec9439" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dafef290fad5beebf29b4afb598e23c5.setContent(html_2c449065a2742961fe3806479eec9439);\\n \\n \\n\\n circle_marker_f1211c4028e36f5f42c4db5355245060.bindPopup(popup_dafef290fad5beebf29b4afb598e23c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_0ae69805a1bf5c0b91371e38b92fd726 = L.circleMarker(\\n [42.73997270436829, -73.26241128061605],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_2ca478e6362eb84a20c664905d6ecdef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ef5ea9bccc5124d8ea43d3b20d8a2fac = $(`<div id="html_ef5ea9bccc5124d8ea43d3b20d8a2fac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ca478e6362eb84a20c664905d6ecdef.setContent(html_ef5ea9bccc5124d8ea43d3b20d8a2fac);\\n \\n \\n\\n circle_marker_0ae69805a1bf5c0b91371e38b92fd726.bindPopup(popup_2ca478e6362eb84a20c664905d6ecdef)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ead5fcdc30ad6fee174210740ab42d7 = L.circleMarker(\\n [42.73996982702469, -73.23903600671946],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_fab10ebeee8a7b269e3c0598136bce9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4ba11c04e005919a711671e9932bd96a = $(`<div id="html_4ba11c04e005919a711671e9932bd96a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fab10ebeee8a7b269e3c0598136bce9c.setContent(html_4ba11c04e005919a711671e9932bd96a);\\n \\n \\n\\n circle_marker_7ead5fcdc30ad6fee174210740ab42d7.bindPopup(popup_fab10ebeee8a7b269e3c0598136bce9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_eed8c82d117a36d06f59634d307ca961 = L.circleMarker(\\n [42.73996954389935, -73.23780572922435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_7e8e77e84c41d37cc0a5b746ce345cf7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f12d1d41edd1fa7b739a39cdb99c85e7 = $(`<div id="html_f12d1d41edd1fa7b739a39cdb99c85e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7e8e77e84c41d37cc0a5b746ce345cf7.setContent(html_f12d1d41edd1fa7b739a39cdb99c85e7);\\n \\n \\n\\n circle_marker_eed8c82d117a36d06f59634d307ca961.bindPopup(popup_7e8e77e84c41d37cc0a5b746ce345cf7)\\n ;\\n\\n \\n \\n \\n var circle_marker_5d974b8b0a1e51bed78441dcfce5656a = L.circleMarker(\\n [42.7399692476054, -73.23657545174072],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_40791f8dc13576132d353a7cc2138a00 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a817de3feb3b93ff490d8ae7a42a0b4e = $(`<div id="html_a817de3feb3b93ff490d8ae7a42a0b4e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_40791f8dc13576132d353a7cc2138a00.setContent(html_a817de3feb3b93ff490d8ae7a42a0b4e);\\n \\n \\n\\n circle_marker_5d974b8b0a1e51bed78441dcfce5656a.bindPopup(popup_40791f8dc13576132d353a7cc2138a00)\\n ;\\n\\n \\n \\n \\n var circle_marker_4ae2f4456bd2c53b8c3990a98e0548bf = L.circleMarker(\\n [42.73996556039193, -73.22427267765188],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_894eb29600c9b7968563c67bebc1e607 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_db009c2267aa9b9676884250be44305a = $(`<div id="html_db009c2267aa9b9676884250be44305a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_894eb29600c9b7968563c67bebc1e607.setContent(html_db009c2267aa9b9676884250be44305a);\\n \\n \\n\\n circle_marker_4ae2f4456bd2c53b8c3990a98e0548bf.bindPopup(popup_894eb29600c9b7968563c67bebc1e607)\\n ;\\n\\n \\n \\n \\n var circle_marker_5e092037acc59414273712691cf12e94 = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_e2dd85362463623f7ea287534d77c4a4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba849266602702fdaae3425de39c9e60 = $(`<div id="html_ba849266602702fdaae3425de39c9e60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e2dd85362463623f7ea287534d77c4a4.setContent(html_ba849266602702fdaae3425de39c9e60);\\n \\n \\n\\n circle_marker_5e092037acc59414273712691cf12e94.bindPopup(popup_e2dd85362463623f7ea287534d77c4a4)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a1e4075ac506f561a951f794fb262af = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_782cd4e85f5cec1c3c9c6cbcf68f415e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2d7982ae57cd954c2f11192d17a417c1 = $(`<div id="html_2d7982ae57cd954c2f11192d17a417c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_782cd4e85f5cec1c3c9c6cbcf68f415e.setContent(html_2d7982ae57cd954c2f11192d17a417c1);\\n \\n \\n\\n circle_marker_9a1e4075ac506f561a951f794fb262af.bindPopup(popup_782cd4e85f5cec1c3c9c6cbcf68f415e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f600a87082e9b218789883c4eeab3c3c = L.circleMarker(\\n [42.73996371678531, -73.21935156846997],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5029f04bfa7b8385707fa40a014ff3ed = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7a26a31d5576354fae32f4cabbf45b6d = $(`<div id="html_7a26a31d5576354fae32f4cabbf45b6d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5029f04bfa7b8385707fa40a014ff3ed.setContent(html_7a26a31d5576354fae32f4cabbf45b6d);\\n \\n \\n\\n circle_marker_f600a87082e9b218789883c4eeab3c3c.bindPopup(popup_5029f04bfa7b8385707fa40a014ff3ed)\\n ;\\n\\n \\n \\n \\n var circle_marker_ac1deda1bdd4504f88e0faf550180773 = L.circleMarker(\\n [42.73816527471333, -73.27348352812871],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_2d3591d199f9299c65ef9ee4ccac14d1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b31580110b69ae991326776ee1d671f3 = $(`<div id="html_b31580110b69ae991326776ee1d671f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2d3591d199f9299c65ef9ee4ccac14d1.setContent(html_b31580110b69ae991326776ee1d671f3);\\n \\n \\n\\n circle_marker_ac1deda1bdd4504f88e0faf550180773.bindPopup(popup_2d3591d199f9299c65ef9ee4ccac14d1)\\n ;\\n\\n \\n \\n \\n var circle_marker_591e4b4439c156116c264c72f0b8cff8 = L.circleMarker(\\n [42.7381653603059, -73.272253286381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5423d693e5e3aad34be5992078cd61b7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a5b43334f06ebb7807f8fb29de763a4 = $(`<div id="html_4a5b43334f06ebb7807f8fb29de763a4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5423d693e5e3aad34be5992078cd61b7.setContent(html_4a5b43334f06ebb7807f8fb29de763a4);\\n \\n \\n\\n circle_marker_591e4b4439c156116c264c72f0b8cff8.bindPopup(popup_5423d693e5e3aad34be5992078cd61b7)\\n ;\\n\\n \\n \\n \\n var circle_marker_f0613601889038c2ce078f84f153f65d = L.circleMarker(\\n [42.73816559733148, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_93f6a7994e3e7b9c20568e0644cdd987 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ce19dfac7bfd3db449b5cf40f075c371 = $(`<div id="html_ce19dfac7bfd3db449b5cf40f075c371" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93f6a7994e3e7b9c20568e0644cdd987.setContent(html_ce19dfac7bfd3db449b5cf40f075c371);\\n \\n \\n\\n circle_marker_f0613601889038c2ce078f84f153f65d.bindPopup(popup_93f6a7994e3e7b9c20568e0644cdd987)\\n ;\\n\\n \\n \\n \\n var circle_marker_314b9b448e05fd2a733b6a66b333088d = L.circleMarker(\\n [42.73816559074744, -73.26364159408853],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a9179e26cd6d7cca5a0fb0840dffc899 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0d7b6a5a492fbae22f5dbbd8d70fef20 = $(`<div id="html_0d7b6a5a492fbae22f5dbbd8d70fef20" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a9179e26cd6d7cca5a0fb0840dffc899.setContent(html_0d7b6a5a492fbae22f5dbbd8d70fef20);\\n \\n \\n\\n circle_marker_314b9b448e05fd2a733b6a66b333088d.bindPopup(popup_a9179e26cd6d7cca5a0fb0840dffc899)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ef063553a3885b03cb95e9c86e55de3 = L.circleMarker(\\n [42.73816527471333, -73.25626014356571],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_0cd305411fcae0e1c61fa7ff8643c85b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_679fe7d0628f44bc5761f2d90eaa6b8a = $(`<div id="html_679fe7d0628f44bc5761f2d90eaa6b8a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cd305411fcae0e1c61fa7ff8643c85b.setContent(html_679fe7d0628f44bc5761f2d90eaa6b8a);\\n \\n \\n\\n circle_marker_7ef063553a3885b03cb95e9c86e55de3.bindPopup(popup_0cd305411fcae0e1c61fa7ff8643c85b)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b0280dff1280a49c8d9c628127a27f3 = L.circleMarker(\\n [42.73816517595268, -73.25502990182166],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_4df90b400daa6b74160e5807ca0c1c66 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5b132956567c0e63ad5dd6ca32ef8737 = $(`<div id="html_5b132956567c0e63ad5dd6ca32ef8737" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4df90b400daa6b74160e5807ca0c1c66.setContent(html_5b132956567c0e63ad5dd6ca32ef8737);\\n \\n \\n\\n circle_marker_9b0280dff1280a49c8d9c628127a27f3.bindPopup(popup_4df90b400daa6b74160e5807ca0c1c66)\\n ;\\n\\n \\n \\n \\n var circle_marker_785dc2918378b8a9397ce81aa55402b7 = L.circleMarker(\\n [42.73816322049174, -73.241497243028],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_00d38d63699ca295eb7b8f3c5f4ede16 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_81c7df0e85ed742786f0b0dde69ea87d = $(`<div id="html_81c7df0e85ed742786f0b0dde69ea87d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_00d38d63699ca295eb7b8f3c5f4ede16.setContent(html_81c7df0e85ed742786f0b0dde69ea87d);\\n \\n \\n\\n circle_marker_785dc2918378b8a9397ce81aa55402b7.bindPopup(popup_00d38d63699ca295eb7b8f3c5f4ede16)\\n ;\\n\\n \\n \\n \\n var circle_marker_f2da2477506cc939c8c9a2a9a91b01af = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_9f85c351d21db159142d9ee2009a7731 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ab4194572e860feb4914b037845662e = $(`<div id="html_1ab4194572e860feb4914b037845662e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f85c351d21db159142d9ee2009a7731.setContent(html_1ab4194572e860feb4914b037845662e);\\n \\n \\n\\n circle_marker_f2da2477506cc939c8c9a2a9a91b01af.bindPopup(popup_9f85c351d21db159142d9ee2009a7731)\\n ;\\n\\n \\n \\n \\n var circle_marker_e5a9add2c320beb180be9552d523663a = L.circleMarker(\\n [42.73816180492244, -73.23534603484075],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_8251c4772dbd648da3be4c4c0837ceb6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_411110e39abe120d5cfbf42464b24398 = $(`<div id="html_411110e39abe120d5cfbf42464b24398" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8251c4772dbd648da3be4c4c0837ceb6.setContent(html_411110e39abe120d5cfbf42464b24398);\\n \\n \\n\\n circle_marker_e5a9add2c320beb180be9552d523663a.bindPopup(popup_8251c4772dbd648da3be4c4c0837ceb6)\\n ;\\n\\n \\n \\n \\n var circle_marker_19b4e62cd3ecc60c6314b9d64666782f = L.circleMarker(\\n [42.7381588552712, -73.22550410242044],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_ab721432b9e97fb1ab9ee6b7f1dfdb3a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f8cf23579bd058bfe2aef9bbe96d774e = $(`<div id="html_f8cf23579bd058bfe2aef9bbe96d774e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ab721432b9e97fb1ab9ee6b7f1dfdb3a.setContent(html_f8cf23579bd058bfe2aef9bbe96d774e);\\n \\n \\n\\n circle_marker_19b4e62cd3ecc60c6314b9d64666782f.bindPopup(popup_ab721432b9e97fb1ab9ee6b7f1dfdb3a)\\n ;\\n\\n \\n \\n \\n var circle_marker_c5820c432ca1e2d316b59422161a7b7f = L.circleMarker(\\n [42.73635814135235, -73.27348327714894],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.876813695875796, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_470fb060ad35419f8e60bc915f6e6c02 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4fdd4258c1264ea5c8019f9b9289581a = $(`<div id="html_4fdd4258c1264ea5c8019f9b9289581a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_470fb060ad35419f8e60bc915f6e6c02.setContent(html_4fdd4258c1264ea5c8019f9b9289581a);\\n \\n \\n\\n circle_marker_c5820c432ca1e2d316b59422161a7b7f.bindPopup(popup_470fb060ad35419f8e60bc915f6e6c02)\\n ;\\n\\n \\n \\n \\n var circle_marker_e40d2694d2429057eef665a5aea5dee6 = L.circleMarker(\\n [42.73635822694145, -73.27225307125549],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_4c69a9ba4049cdc5b9ca885a645d4468 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_607086f99b6e8235c911b92ca452576c = $(`<div id="html_607086f99b6e8235c911b92ca452576c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4c69a9ba4049cdc5b9ca885a645d4468.setContent(html_607086f99b6e8235c911b92ca452576c);\\n \\n \\n\\n circle_marker_e40d2694d2429057eef665a5aea5dee6.bindPopup(popup_4c69a9ba4049cdc5b9ca885a645d4468)\\n ;\\n\\n \\n \\n \\n var circle_marker_bdc6bd6181670294cb7ae9124f88fbd9 = L.circleMarker(\\n [42.73635829936299, -73.2710228653589],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.170882945826411, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_44651ef769134cfc71f7a485c5773013 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c604d3411546e270663ea9aeb7f43f47 = $(`<div id="html_c604d3411546e270663ea9aeb7f43f47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_44651ef769134cfc71f7a485c5773013.setContent(html_c604d3411546e270663ea9aeb7f43f47);\\n \\n \\n\\n circle_marker_bdc6bd6181670294cb7ae9124f88fbd9.bindPopup(popup_44651ef769134cfc71f7a485c5773013)\\n ;\\n\\n \\n \\n \\n var circle_marker_6f0e93b860023a7a3404578b4feb0a6b = L.circleMarker(\\n [42.73635835861699, -73.2697926594597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_10e11b67c3314c23f17fb5205c8d62b4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1f6ad71b1ed6a89b541ab4d9b38c0778 = $(`<div id="html_1f6ad71b1ed6a89b541ab4d9b38c0778" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10e11b67c3314c23f17fb5205c8d62b4.setContent(html_1f6ad71b1ed6a89b541ab4d9b38c0778);\\n \\n \\n\\n circle_marker_6f0e93b860023a7a3404578b4feb0a6b.bindPopup(popup_10e11b67c3314c23f17fb5205c8d62b4)\\n ;\\n\\n \\n \\n \\n var circle_marker_03b6cedaae33392c8327c783f96a4fbd = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.7057581899030048, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_46acc520d7215b6d88490f87995d2ff5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ab92d80f9655c9aedf93630f0ad401a = $(`<div id="html_1ab92d80f9655c9aedf93630f0ad401a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_46acc520d7215b6d88490f87995d2ff5.setContent(html_1ab92d80f9655c9aedf93630f0ad401a);\\n \\n \\n\\n circle_marker_03b6cedaae33392c8327c783f96a4fbd.bindPopup(popup_46acc520d7215b6d88490f87995d2ff5)\\n ;\\n\\n \\n \\n \\n var circle_marker_9a4b093ba2c99dbdf5e37f0ca5d0ea1c = L.circleMarker(\\n [42.73635845737364, -73.26364162994278],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_c045122b68d823e5536c01a47d83a6ec = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e3cf1b33f4a638c95f47e6f08f09f8cd = $(`<div id="html_e3cf1b33f4a638c95f47e6f08f09f8cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c045122b68d823e5536c01a47d83a6ec.setContent(html_e3cf1b33f4a638c95f47e6f08f09f8cd);\\n \\n \\n\\n circle_marker_9a4b093ba2c99dbdf5e37f0ca5d0ea1c.bindPopup(popup_c045122b68d823e5536c01a47d83a6ec)\\n ;\\n\\n \\n \\n \\n var circle_marker_9dab53bd49cf3c2b6be660e49c0aa267 = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_bfb18149ac2a0639c75182819d94914c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3391da6616660a66d7d0416f58582841 = $(`<div id="html_3391da6616660a66d7d0416f58582841" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfb18149ac2a0639c75182819d94914c.setContent(html_3391da6616660a66d7d0416f58582841);\\n \\n \\n\\n circle_marker_9dab53bd49cf3c2b6be660e49c0aa267.bindPopup(popup_bfb18149ac2a0639c75182819d94914c)\\n ;\\n\\n \\n \\n \\n var circle_marker_1a0e4e29ba1cf89e7f9b3a6b1853cd1e = L.circleMarker(\\n [42.73635780557973, -73.25256977688913],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_4b937ddcf8b085c9cdc591605480283d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6f2db2cefa1283b24485dcf356a6a4b0 = $(`<div id="html_6f2db2cefa1283b24485dcf356a6a4b0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4b937ddcf8b085c9cdc591605480283d.setContent(html_6f2db2cefa1283b24485dcf356a6a4b0);\\n \\n \\n\\n circle_marker_1a0e4e29ba1cf89e7f9b3a6b1853cd1e.bindPopup(popup_4b937ddcf8b085c9cdc591605480283d)\\n ;\\n\\n \\n \\n \\n var circle_marker_817960b31a4df18a2d1eafd12a90a9fc = L.circleMarker(\\n [42.73635766732041, -73.25133957101345],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 6.90988298942671, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_71177121894e6a2b703ca6e101d3f29b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ac513c93b9a5b43df295335117a170e1 = $(`<div id="html_ac513c93b9a5b43df295335117a170e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_71177121894e6a2b703ca6e101d3f29b.setContent(html_ac513c93b9a5b43df295335117a170e1);\\n \\n \\n\\n circle_marker_817960b31a4df18a2d1eafd12a90a9fc.bindPopup(popup_71177121894e6a2b703ca6e101d3f29b)\\n ;\\n\\n \\n \\n \\n var circle_marker_aa75c62529f658cd870fb1f88053091a = L.circleMarker(\\n [42.73635751589355, -73.25010936514349],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_629ee1cce94e74c17ddc8aea4ba329f0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_868306a2f120f76bbb36e749856a45f3 = $(`<div id="html_868306a2f120f76bbb36e749856a45f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_629ee1cce94e74c17ddc8aea4ba329f0.setContent(html_868306a2f120f76bbb36e749856a45f3);\\n \\n \\n\\n circle_marker_aa75c62529f658cd870fb1f88053091a.bindPopup(popup_629ee1cce94e74c17ddc8aea4ba329f0)\\n ;\\n\\n \\n \\n \\n var circle_marker_6fec552424a604609b9d44d86a1b78b5 = L.circleMarker(\\n [42.73635698260761, -73.24641874757337],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_f48f11746185f1ee49aab654a289e4f5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1fe6586d90b5b17899e52c055245bdf3 = $(`<div id="html_1fe6586d90b5b17899e52c055245bdf3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f48f11746185f1ee49aab654a289e4f5.setContent(html_1fe6586d90b5b17899e52c055245bdf3);\\n \\n \\n\\n circle_marker_6fec552424a604609b9d44d86a1b78b5.bindPopup(popup_f48f11746185f1ee49aab654a289e4f5)\\n ;\\n\\n \\n \\n \\n var circle_marker_031d3fc4505c88005a386b32f573138d = L.circleMarker(\\n [42.73635677851055, -73.24518854173164],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_1ca33deb3056a1973b6f87fc02f16650 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_89296dade0599f29a43ae53f5789aa3c = $(`<div id="html_89296dade0599f29a43ae53f5789aa3c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ca33deb3056a1973b6f87fc02f16650.setContent(html_89296dade0599f29a43ae53f5789aa3c);\\n \\n \\n\\n circle_marker_031d3fc4505c88005a386b32f573138d.bindPopup(popup_1ca33deb3056a1973b6f87fc02f16650)\\n ;\\n\\n \\n \\n \\n var circle_marker_54e33a2e9f7bc87a0b35dbc2f76f85c8 = L.circleMarker(\\n [42.73635656124591, -73.24395833589827],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_86bd2bbc63422928bb1e2fd8636b0505 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_739baab041f7b7653e0fadf28e515b6a = $(`<div id="html_739baab041f7b7653e0fadf28e515b6a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86bd2bbc63422928bb1e2fd8636b0505.setContent(html_739baab041f7b7653e0fadf28e515b6a);\\n \\n \\n\\n circle_marker_54e33a2e9f7bc87a0b35dbc2f76f85c8.bindPopup(popup_86bd2bbc63422928bb1e2fd8636b0505)\\n ;\\n\\n \\n \\n \\n var circle_marker_fe0815de565ea521c1dd68773c65980b = L.circleMarker(\\n [42.73635633081373, -73.24272813007379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_467e3756654fb488baf64242dd9eef8f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b0fdd47a3bdfcfcc8ced2f2e62d9fdc4 = $(`<div id="html_b0fdd47a3bdfcfcc8ced2f2e62d9fdc4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_467e3756654fb488baf64242dd9eef8f.setContent(html_b0fdd47a3bdfcfcc8ced2f2e62d9fdc4);\\n \\n \\n\\n circle_marker_fe0815de565ea521c1dd68773c65980b.bindPopup(popup_467e3756654fb488baf64242dd9eef8f)\\n ;\\n\\n \\n \\n \\n var circle_marker_b6328aa93e340fb40cde9f50200fff44 = L.circleMarker(\\n [42.736356087214006, -73.24149792425868],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_ee4ea01a5d1db541a780bd41b3ee9c9d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ed3cd5831b50bdefa15746e16b8415a = $(`<div id="html_0ed3cd5831b50bdefa15746e16b8415a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ee4ea01a5d1db541a780bd41b3ee9c9d.setContent(html_0ed3cd5831b50bdefa15746e16b8415a);\\n \\n \\n\\n circle_marker_b6328aa93e340fb40cde9f50200fff44.bindPopup(popup_ee4ea01a5d1db541a780bd41b3ee9c9d)\\n ;\\n\\n \\n \\n \\n var circle_marker_5976f36e1771fd0cf68980f6f181d724 = L.circleMarker(\\n [42.73635583044671, -73.24026771845354],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_1f9fcece0db088a12cf010421f952028 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e26dab0a0ecdc0695ee7713bc4015b76 = $(`<div id="html_e26dab0a0ecdc0695ee7713bc4015b76" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1f9fcece0db088a12cf010421f952028.setContent(html_e26dab0a0ecdc0695ee7713bc4015b76);\\n \\n \\n\\n circle_marker_5976f36e1771fd0cf68980f6f181d724.bindPopup(popup_1f9fcece0db088a12cf010421f952028)\\n ;\\n\\n \\n \\n \\n var circle_marker_e52d8eea2adcc1909c56e6f6a2aa63cb = L.circleMarker(\\n [42.736354981139534, -73.23657710110285],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_e799b3e527c9b155cebac007cf86e94b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bfd6c775c483f3d16fed1a8f22a4a89d = $(`<div id="html_bfd6c775c483f3d16fed1a8f22a4a89d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e799b3e527c9b155cebac007cf86e94b.setContent(html_bfd6c775c483f3d16fed1a8f22a4a89d);\\n \\n \\n\\n circle_marker_e52d8eea2adcc1909c56e6f6a2aa63cb.bindPopup(popup_e799b3e527c9b155cebac007cf86e94b)\\n ;\\n\\n \\n \\n \\n var circle_marker_2653fb152234f9da1f1d55683c86d39f = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_e1cfb6fdf5cb9148016e61ab8fe43d95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_789a8bc1240e8be957880e6eb3dacce4 = $(`<div id="html_789a8bc1240e8be957880e6eb3dacce4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e1cfb6fdf5cb9148016e61ab8fe43d95.setContent(html_789a8bc1240e8be957880e6eb3dacce4);\\n \\n \\n\\n circle_marker_2653fb152234f9da1f1d55683c86d39f.bindPopup(popup_e1cfb6fdf5cb9148016e61ab8fe43d95)\\n ;\\n\\n \\n \\n \\n var circle_marker_363f368d1a06411cfc6b40fc522a7d6f = L.circleMarker(\\n [42.73455100799136, -73.27348302618954],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_64f9b4d30ed07c856ef65068b63b2841 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2441c5a8b5c25276771e687dc19fbffa = $(`<div id="html_2441c5a8b5c25276771e687dc19fbffa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64f9b4d30ed07c856ef65068b63b2841.setContent(html_2441c5a8b5c25276771e687dc19fbffa);\\n \\n \\n\\n circle_marker_363f368d1a06411cfc6b40fc522a7d6f.bindPopup(popup_64f9b4d30ed07c856ef65068b63b2841)\\n ;\\n\\n \\n \\n \\n var circle_marker_15f6031df3f5fb2ab48a3e302cc371b1 = L.circleMarker(\\n [42.73455109357698, -73.27225285614743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.6462837142006137, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_81ee23bdd76d1cf7bb9cfa6066357baa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b01e3c4d3dc16a49dce0be1443d45d51 = $(`<div id="html_b01e3c4d3dc16a49dce0be1443d45d51" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_81ee23bdd76d1cf7bb9cfa6066357baa.setContent(html_b01e3c4d3dc16a49dce0be1443d45d51);\\n \\n \\n\\n circle_marker_15f6031df3f5fb2ab48a3e302cc371b1.bindPopup(popup_81ee23bdd76d1cf7bb9cfa6066357baa)\\n ;\\n\\n \\n \\n \\n var circle_marker_c0901b1e85fbb342fe5827e843c5502b = L.circleMarker(\\n [42.734551165995605, -73.27102268610217],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a5c1c8e8382e344f89e39afcdc1fadcc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_49436bd832fbe33e2842a8f51d5ebad7 = $(`<div id="html_49436bd832fbe33e2842a8f51d5ebad7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a5c1c8e8382e344f89e39afcdc1fadcc.setContent(html_49436bd832fbe33e2842a8f51d5ebad7);\\n \\n \\n\\n circle_marker_c0901b1e85fbb342fe5827e843c5502b.bindPopup(popup_a5c1c8e8382e344f89e39afcdc1fadcc)\\n ;\\n\\n \\n \\n \\n var circle_marker_436d9980ccd8bd8782e4845f227f04a9 = L.circleMarker(\\n [42.73455127133177, -73.26118132569005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_9f559531f7336451b6ac922094b79010 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_67bd1c019bd003d9d44799638d09b86b = $(`<div id="html_67bd1c019bd003d9d44799638d09b86b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f559531f7336451b6ac922094b79010.setContent(html_67bd1c019bd003d9d44799638d09b86b);\\n \\n \\n\\n circle_marker_436d9980ccd8bd8782e4845f227f04a9.bindPopup(popup_9f559531f7336451b6ac922094b79010)\\n ;\\n\\n \\n \\n \\n var circle_marker_7912cf7ef8be1a033afc30ed6e9e7ba2 = L.circleMarker(\\n [42.7345512252472, -73.2599511556401],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_de2b27b4a9665de13beb525d2ee46eea = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86e16faabb0e35603ae467e65705a407 = $(`<div id="html_86e16faabb0e35603ae467e65705a407" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de2b27b4a9665de13beb525d2ee46eea.setContent(html_86e16faabb0e35603ae467e65705a407);\\n \\n \\n\\n circle_marker_7912cf7ef8be1a033afc30ed6e9e7ba2.bindPopup(popup_de2b27b4a9665de13beb525d2ee46eea)\\n ;\\n\\n \\n \\n \\n var circle_marker_8edc5448ec021ebc0db9a9873b4dc8d0 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0382538898732494, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_b9f7f91124711e163441502e7cc16db0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc54d8ec0bcb35b133647217fc969aa8 = $(`<div id="html_dc54d8ec0bcb35b133647217fc969aa8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b9f7f91124711e163441502e7cc16db0.setContent(html_dc54d8ec0bcb35b133647217fc969aa8);\\n \\n \\n\\n circle_marker_8edc5448ec021ebc0db9a9873b4dc8d0.bindPopup(popup_b9f7f91124711e163441502e7cc16db0)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b4705d51853906c7f2b10b470c5b017 = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_72a7e82f5673a1dc9d364993547fa855 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_61729e0d6ee17783be03343533de2dd2 = $(`<div id="html_61729e0d6ee17783be03343533de2dd2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72a7e82f5673a1dc9d364993547fa855.setContent(html_61729e0d6ee17783be03343533de2dd2);\\n \\n \\n\\n circle_marker_8b4705d51853906c7f2b10b470c5b017.bindPopup(popup_72a7e82f5673a1dc9d364993547fa855)\\n ;\\n\\n \\n \\n \\n var circle_marker_c02da0c62e255e73fc7773c90d6cf02c = L.circleMarker(\\n [42.73455100799136, -73.25626064550488],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_1b319b92740ec98d5198b7f8b2b4ddb7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1482d59c726647b1e2ea4ff5fd138595 = $(`<div id="html_1482d59c726647b1e2ea4ff5fd138595" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b319b92740ec98d5198b7f8b2b4ddb7.setContent(html_1482d59c726647b1e2ea4ff5fd138595);\\n \\n \\n\\n circle_marker_c02da0c62e255e73fc7773c90d6cf02c.bindPopup(popup_1b319b92740ec98d5198b7f8b2b4ddb7)\\n ;\\n\\n \\n \\n \\n var circle_marker_b1115cbc5325b0756796b51e1062d40e = L.circleMarker(\\n [42.73455053397864, -73.25133996537824],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_e029c4a5ce09b798c15b635566824317 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2a193cafb7fa39b3a6209b9472a97610 = $(`<div id="html_2a193cafb7fa39b3a6209b9472a97610" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e029c4a5ce09b798c15b635566824317.setContent(html_2a193cafb7fa39b3a6209b9472a97610);\\n \\n \\n\\n circle_marker_b1115cbc5325b0756796b51e1062d40e.bindPopup(popup_e029c4a5ce09b798c15b635566824317)\\n ;\\n\\n \\n \\n \\n var circle_marker_24bd199c7008d054ee3902aa494cef1a = L.circleMarker(\\n [42.73455021797015, -73.24887962534729],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_ddc0dd84728539219c263d1c9a55d1a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f386c28d44528a00f92f020721587542 = $(`<div id="html_f386c28d44528a00f92f020721587542" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ddc0dd84728539219c263d1c9a55d1a2.setContent(html_f386c28d44528a00f92f020721587542);\\n \\n \\n\\n circle_marker_24bd199c7008d054ee3902aa494cef1a.bindPopup(popup_ddc0dd84728539219c263d1c9a55d1a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_2ff80b857dfe99722901793cea3099a5 = L.circleMarker(\\n [42.734550040215375, -73.24764945534174],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_172f729368ba61cbffd00c3c19de39bd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e32292aaa9b80eb3484bb728ada4009 = $(`<div id="html_7e32292aaa9b80eb3484bb728ada4009" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_172f729368ba61cbffd00c3c19de39bd.setContent(html_7e32292aaa9b80eb3484bb728ada4009);\\n \\n \\n\\n circle_marker_2ff80b857dfe99722901793cea3099a5.bindPopup(popup_172f729368ba61cbffd00c3c19de39bd)\\n ;\\n\\n \\n \\n \\n var circle_marker_0a6eaf36906bb698ff1424586beb2c75 = L.circleMarker(\\n [42.73454984929359, -73.2464192853435],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_f20940746f71a81f2473b9cc45539fd3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d68d0f7cfb6ccc312e670e39c7e0daf = $(`<div id="html_6d68d0f7cfb6ccc312e670e39c7e0daf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f20940746f71a81f2473b9cc45539fd3.setContent(html_6d68d0f7cfb6ccc312e670e39c7e0daf);\\n \\n \\n\\n circle_marker_0a6eaf36906bb698ff1424586beb2c75.bindPopup(popup_f20940746f71a81f2473b9cc45539fd3)\\n ;\\n\\n \\n \\n \\n var circle_marker_963fa447e789ed9c96c4dd16cb5ad877 = L.circleMarker(\\n [42.73454964520478, -73.24518911535311],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_319c751fffef14970bb36f9a890b763e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b6251665696467b98dd23298256442f = $(`<div id="html_3b6251665696467b98dd23298256442f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_319c751fffef14970bb36f9a890b763e.setContent(html_3b6251665696467b98dd23298256442f);\\n \\n \\n\\n circle_marker_963fa447e789ed9c96c4dd16cb5ad877.bindPopup(popup_319c751fffef14970bb36f9a890b763e)\\n ;\\n\\n \\n \\n \\n var circle_marker_3227787212764e4b93aeda9afca10f72 = L.circleMarker(\\n [42.7345491975261, -73.24272877539794],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_27cb25b7801b62d9203cd9d34c3b7ebb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09e22897fef0845aad4100d4174e55a9 = $(`<div id="html_09e22897fef0845aad4100d4174e55a9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_27cb25b7801b62d9203cd9d34c3b7ebb.setContent(html_09e22897fef0845aad4100d4174e55a9);\\n \\n \\n\\n circle_marker_3227787212764e4b93aeda9afca10f72.bindPopup(popup_27cb25b7801b62d9203cd9d34c3b7ebb)\\n ;\\n\\n \\n \\n \\n var circle_marker_38b2c3dcc9951063093cc860d684f413 = L.circleMarker(\\n [42.73454895393624, -73.24149860543417],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_bfc7666cc65dfe6d8ea50706bd8d4956 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb287f2fdaf5baa8ae765d7d2918ff64 = $(`<div id="html_eb287f2fdaf5baa8ae765d7d2918ff64" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfc7666cc65dfe6d8ea50706bd8d4956.setContent(html_eb287f2fdaf5baa8ae765d7d2918ff64);\\n \\n \\n\\n circle_marker_38b2c3dcc9951063093cc860d684f413.bindPopup(popup_bfc7666cc65dfe6d8ea50706bd8d4956)\\n ;\\n\\n \\n \\n \\n var circle_marker_586c69ffa2e332df4806c5c1d00f344c = L.circleMarker(\\n [42.73454869717936, -73.24026843548036],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5d3df12c8cec2beb2f37595fbc32f72e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b05269ad0fef005f4e1150feee3146fe = $(`<div id="html_b05269ad0fef005f4e1150feee3146fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d3df12c8cec2beb2f37595fbc32f72e.setContent(html_b05269ad0fef005f4e1150feee3146fe);\\n \\n \\n\\n circle_marker_586c69ffa2e332df4806c5c1d00f344c.bindPopup(popup_5d3df12c8cec2beb2f37595fbc32f72e)\\n ;\\n\\n \\n \\n \\n var circle_marker_16a56dc148f4a6ba00501de7dfeca223 = L.circleMarker(\\n [42.73454784790659, -73.23657792568366],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_10d67a6276af7c88b2538ecfd4b81a81 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c29fda90a78be1657741902857d422a1 = $(`<div id="html_c29fda90a78be1657741902857d422a1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_10d67a6276af7c88b2538ecfd4b81a81.setContent(html_c29fda90a78be1657741902857d422a1);\\n \\n \\n\\n circle_marker_16a56dc148f4a6ba00501de7dfeca223.bindPopup(popup_10d67a6276af7c88b2538ecfd4b81a81)\\n ;\\n\\n \\n \\n \\n var circle_marker_34c9d26a1dc9f3c56db9e958f5f6e5d8 = L.circleMarker(\\n [42.73454416114132, -73.22427622722158],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_16718609437978d037ec0a96be0afa9c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_09a803cd3dd07edc6bcb6c1cdbf86920 = $(`<div id="html_09a803cd3dd07edc6bcb6c1cdbf86920" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_16718609437978d037ec0a96be0afa9c.setContent(html_09a803cd3dd07edc6bcb6c1cdbf86920);\\n \\n \\n\\n circle_marker_34c9d26a1dc9f3c56db9e958f5f6e5d8.bindPopup(popup_16718609437978d037ec0a96be0afa9c)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b5f296150938224564cc889678c6670 = L.circleMarker(\\n [42.73274396021253, -73.27225264105678],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_b0719ac46ec6cb097529f910d673308f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_46b75e8e8a24d4eacd78c6aaf2f3351c = $(`<div id="html_46b75e8e8a24d4eacd78c6aaf2f3351c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b0719ac46ec6cb097529f910d673308f.setContent(html_46b75e8e8a24d4eacd78c6aaf2f3351c);\\n \\n \\n\\n circle_marker_2b5f296150938224564cc889678c6670.bindPopup(popup_b0719ac46ec6cb097529f910d673308f)\\n ;\\n\\n \\n \\n \\n var circle_marker_37bac6fa39cf7cc5233ff5df9e6171ce = L.circleMarker(\\n [42.73274403262821, -73.27102250685998],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_6799658724a672f63285f9986a6a9d37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7953ed0d7d13914a2db4f9cc28b007e7 = $(`<div id="html_7953ed0d7d13914a2db4f9cc28b007e7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6799658724a672f63285f9986a6a9d37.setContent(html_7953ed0d7d13914a2db4f9cc28b007e7);\\n \\n \\n\\n circle_marker_37bac6fa39cf7cc5233ff5df9e6171ce.bindPopup(popup_6799658724a672f63285f9986a6a9d37)\\n ;\\n\\n \\n \\n \\n var circle_marker_d6a26781a48b65808adc060a24627750 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_8274ecd6742eb48ba40b5c13405b3cd1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4a678302c3bea128e92cb53004457b1c = $(`<div id="html_4a678302c3bea128e92cb53004457b1c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8274ecd6742eb48ba40b5c13405b3cd1.setContent(html_4a678302c3bea128e92cb53004457b1c);\\n \\n \\n\\n circle_marker_d6a26781a48b65808adc060a24627750.bindPopup(popup_8274ecd6742eb48ba40b5c13405b3cd1)\\n ;\\n\\n \\n \\n \\n var circle_marker_c6009075a46d25e7b603421eb9e58272 = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_8ccf5517e470c5b0e3b9bbc1c7ea86cb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cc830a4fbfe82fa46f07775a4fc8fd85 = $(`<div id="html_cc830a4fbfe82fa46f07775a4fc8fd85" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8ccf5517e470c5b0e3b9bbc1c7ea86cb.setContent(html_cc830a4fbfe82fa46f07775a4fc8fd85);\\n \\n \\n\\n circle_marker_c6009075a46d25e7b603421eb9e58272.bindPopup(popup_8ccf5517e470c5b0e3b9bbc1c7ea86cb)\\n ;\\n\\n \\n \\n \\n var circle_marker_578ea5c36c4f4be54d3ba1e93a198fa5 = L.circleMarker(\\n [42.73274353888496, -73.25257049388698],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_6d8bc36e7f5546a5e88868ee4cdf01ab = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b1b3495cf508ea98eea511b3628c7d7e = $(`<div id="html_b1b3495cf508ea98eea511b3628c7d7e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6d8bc36e7f5546a5e88868ee4cdf01ab.setContent(html_b1b3495cf508ea98eea511b3628c7d7e);\\n \\n \\n\\n circle_marker_578ea5c36c4f4be54d3ba1e93a198fa5.bindPopup(popup_6d8bc36e7f5546a5e88868ee4cdf01ab)\\n ;\\n\\n \\n \\n \\n var circle_marker_a555dee2cd74baeaaa4830b6bcd4d6c8 = L.circleMarker(\\n [42.73274340063685, -73.25134035971105],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_d8b8885eebe7559d865c0845e7eeab7c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e66075ff08bc54f4f052fc2c8b697acf = $(`<div id="html_e66075ff08bc54f4f052fc2c8b697acf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8b8885eebe7559d865c0845e7eeab7c.setContent(html_e66075ff08bc54f4f052fc2c8b697acf);\\n \\n \\n\\n circle_marker_a555dee2cd74baeaaa4830b6bcd4d6c8.bindPopup(popup_d8b8885eebe7559d865c0845e7eeab7c)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb6012513df8f3a290a5fbcb08383730 = L.circleMarker(\\n [42.73274308464117, -73.24888009137699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_b025a946645cf66dd2935b9e716387d2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_91450d67de036dd459fe81728cecfe50 = $(`<div id="html_91450d67de036dd459fe81728cecfe50" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b025a946645cf66dd2935b9e716387d2.setContent(html_91450d67de036dd459fe81728cecfe50);\\n \\n \\n\\n circle_marker_eb6012513df8f3a290a5fbcb08383730.bindPopup(popup_b025a946645cf66dd2935b9e716387d2)\\n ;\\n\\n \\n \\n \\n var circle_marker_818fc92f89ee2b08b098f3b6a008bccb = L.circleMarker(\\n [42.732742906893606, -73.24764995721988],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_3c4a2b209e6e91083e5ad078981be372 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc99465bdcae18e203f85f68ac2cc974 = $(`<div id="html_fc99465bdcae18e203f85f68ac2cc974" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c4a2b209e6e91083e5ad078981be372.setContent(html_fc99465bdcae18e203f85f68ac2cc974);\\n \\n \\n\\n circle_marker_818fc92f89ee2b08b098f3b6a008bccb.bindPopup(popup_3c4a2b209e6e91083e5ad078981be372)\\n ;\\n\\n \\n \\n \\n var circle_marker_2dc3691ceb9fa00f9edb46381b131daf = L.circleMarker(\\n [42.732742511899, -73.24518968892811],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_bdf854478c6c348ae8c4058ed98c0c08 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a8ce72ca33c6a8e7194e9aa5123200a3 = $(`<div id="html_a8ce72ca33c6a8e7194e9aa5123200a3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdf854478c6c348ae8c4058ed98c0c08.setContent(html_a8ce72ca33c6a8e7194e9aa5123200a3);\\n \\n \\n\\n circle_marker_2dc3691ceb9fa00f9edb46381b131daf.bindPopup(popup_bdf854478c6c348ae8c4058ed98c0c08)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8cd454d1e071302a34db5b2d01de086 = L.circleMarker(\\n [42.73274229465196, -73.24395955479451],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_93e0b9760d06a98f0e55533b3ee7b386 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8e55a177a4e9a810a1a0f5a30c82a25a = $(`<div id="html_8e55a177a4e9a810a1a0f5a30c82a25a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_93e0b9760d06a98f0e55533b3ee7b386.setContent(html_8e55a177a4e9a810a1a0f5a30c82a25a);\\n \\n \\n\\n circle_marker_c8cd454d1e071302a34db5b2d01de086.bindPopup(popup_93e0b9760d06a98f0e55533b3ee7b386)\\n ;\\n\\n \\n \\n \\n var circle_marker_aab0c596b4c766784e6d4a42de59c7c8 = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_8f5980ae4fe7946a5350cc177a342435 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37eb2377be200c60e3c60dec64d83872 = $(`<div id="html_37eb2377be200c60e3c60dec64d83872" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8f5980ae4fe7946a5350cc177a342435.setContent(html_37eb2377be200c60e3c60dec64d83872);\\n \\n \\n\\n circle_marker_aab0c596b4c766784e6d4a42de59c7c8.bindPopup(popup_8f5980ae4fe7946a5350cc177a342435)\\n ;\\n\\n \\n \\n \\n var circle_marker_ce497ba61d50b88639e296936117fff7 = L.circleMarker(\\n [42.73274156391199, -73.24026915244907],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_9fd450b57a7a4571b5dd197a9e2444d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c40994a8fe5a4ea32a8faec30dc8b937 = $(`<div id="html_c40994a8fe5a4ea32a8faec30dc8b937" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9fd450b57a7a4571b5dd197a9e2444d7.setContent(html_c40994a8fe5a4ea32a8faec30dc8b937);\\n \\n \\n\\n circle_marker_ce497ba61d50b88639e296936117fff7.bindPopup(popup_9fd450b57a7a4571b5dd197a9e2444d7)\\n ;\\n\\n \\n \\n \\n var circle_marker_3648cfb489c4b5659ce8ea14aa819300 = L.circleMarker(\\n [42.73273518474991, -73.21935687468196],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_c4a186f20588013c2f75ae755c9d60ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_abbbc435ac89e829829226626a4e5f56 = $(`<div id="html_abbbc435ac89e829829226626a4e5f56" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c4a186f20588013c2f75ae755c9d60ef.setContent(html_abbbc435ac89e829829226626a4e5f56);\\n \\n \\n\\n circle_marker_3648cfb489c4b5659ce8ea14aa819300.bindPopup(popup_c4a186f20588013c2f75ae755c9d60ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c26dda6573f7dd22a0e40a3ef945441 = L.circleMarker(\\n [42.73093674126938, -73.27348252433171],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_88dc62d08dc61b4e0cb2d07429d0bcf6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f08ed06b4099e46b946b94d0f525c0a6 = $(`<div id="html_f08ed06b4099e46b946b94d0f525c0a6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_88dc62d08dc61b4e0cb2d07429d0bcf6.setContent(html_f08ed06b4099e46b946b94d0f525c0a6);\\n \\n \\n\\n circle_marker_7c26dda6573f7dd22a0e40a3ef945441.bindPopup(popup_88dc62d08dc61b4e0cb2d07429d0bcf6)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5e753ee19028d072047ed77947990c4 = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_cba336683345a5f81b16414f58f6ac3c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a411efb02436ea9f5727dce76e89c705 = $(`<div id="html_a411efb02436ea9f5727dce76e89c705" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cba336683345a5f81b16414f58f6ac3c.setContent(html_a411efb02436ea9f5727dce76e89c705);\\n \\n \\n\\n circle_marker_f5e753ee19028d072047ed77947990c4.bindPopup(popup_cba336683345a5f81b16414f58f6ac3c)\\n ;\\n\\n \\n \\n \\n var circle_marker_714843c86a5a5a6099b54913e7a6949f = L.circleMarker(\\n [42.730936899260826, -73.25872134406212],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5c2229e877bbe228b1629c36ea087fdf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c3e32dc5213407c023b7400b0c0b42e1 = $(`<div id="html_c3e32dc5213407c023b7400b0c0b42e1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c2229e877bbe228b1629c36ea087fdf.setContent(html_c3e32dc5213407c023b7400b0c0b42e1);\\n \\n \\n\\n circle_marker_714843c86a5a5a6099b54913e7a6949f.bindPopup(popup_5c2229e877bbe228b1629c36ea087fdf)\\n ;\\n\\n \\n \\n \\n var circle_marker_84f471839a0d462ba9d7809efe202729 = L.circleMarker(\\n [42.73093682684808, -73.25749124571084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_eedb8fc1718bd777f355a99652156c37 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_102f95026cfc652168469b84e6b96594 = $(`<div id="html_102f95026cfc652168469b84e6b96594" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_eedb8fc1718bd777f355a99652156c37.setContent(html_102f95026cfc652168469b84e6b96594);\\n \\n \\n\\n circle_marker_84f471839a0d462ba9d7809efe202729.bindPopup(popup_eedb8fc1718bd777f355a99652156c37)\\n ;\\n\\n \\n \\n \\n var circle_marker_606e9744d10f69150d2ae9b0deb91a0f = L.circleMarker(\\n [42.730936642524725, -73.25503104901821],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_490b860a120f560ae183328651b091a0 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_70ad4d5f61cc0c3c64a697d52bbe3ba1 = $(`<div id="html_70ad4d5f61cc0c3c64a697d52bbe3ba1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_490b860a120f560ae183328651b091a0.setContent(html_70ad4d5f61cc0c3c64a697d52bbe3ba1);\\n \\n \\n\\n circle_marker_606e9744d10f69150d2ae9b0deb91a0f.bindPopup(popup_490b860a120f560ae183328651b091a0)\\n ;\\n\\n \\n \\n \\n var circle_marker_77e008397eabe419d2f770b22f79612a = L.circleMarker(\\n [42.73093611588659, -73.2501106556873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_de80e3e65ff6775c22e5ec5981929701 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a73b0287baab90469ebb1e7c482c2cee = $(`<div id="html_a73b0287baab90469ebb1e7c482c2cee" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_de80e3e65ff6775c22e5ec5981929701.setContent(html_a73b0287baab90469ebb1e7c482c2cee);\\n \\n \\n\\n circle_marker_77e008397eabe419d2f770b22f79612a.bindPopup(popup_de80e3e65ff6775c22e5ec5981929701)\\n ;\\n\\n \\n \\n \\n var circle_marker_d3450972914605646a1a15f29e7a5ac1 = L.circleMarker(\\n [42.730935773571815, -73.24765045905734],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_d74aff08b5a72b02d30a1f1daa64487e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fe20c112ad623059754e23cdfeb06e8d = $(`<div id="html_fe20c112ad623059754e23cdfeb06e8d" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d74aff08b5a72b02d30a1f1daa64487e.setContent(html_fe20c112ad623059754e23cdfeb06e8d);\\n \\n \\n\\n circle_marker_d3450972914605646a1a15f29e7a5ac1.bindPopup(popup_d74aff08b5a72b02d30a1f1daa64487e)\\n ;\\n\\n \\n \\n \\n var circle_marker_750b3c8e9d68c2534832053aaa499180 = L.circleMarker(\\n [42.730935582665495, -73.24642036075306],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_2211692c41a09228046e848ee7ffbccb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b318f59c3531fde117cfb0427383425e = $(`<div id="html_b318f59c3531fde117cfb0427383425e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2211692c41a09228046e848ee7ffbccb.setContent(html_b318f59c3531fde117cfb0427383425e);\\n \\n \\n\\n circle_marker_750b3c8e9d68c2534832053aaa499180.bindPopup(popup_2211692c41a09228046e848ee7ffbccb)\\n ;\\n\\n \\n \\n \\n var circle_marker_113d577039d1adf60fbab5a21a18a718 = L.circleMarker(\\n [42.73093537859322, -73.24519026245663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_776a8b05b21bd5b2d59bc6db09dac41a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8fa9088cb33be0f4d4d603ca12970447 = $(`<div id="html_8fa9088cb33be0f4d4d603ca12970447" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_776a8b05b21bd5b2d59bc6db09dac41a.setContent(html_8fa9088cb33be0f4d4d603ca12970447);\\n \\n \\n\\n circle_marker_113d577039d1adf60fbab5a21a18a718.bindPopup(popup_776a8b05b21bd5b2d59bc6db09dac41a)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f02ace3b0c7d8a0e2d779ca88391ebe = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_7c9f8ddf83e9d02e6945e1333189547c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_15bc60463da1bc3c8f7429bd35098086 = $(`<div id="html_15bc60463da1bc3c8f7429bd35098086" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7c9f8ddf83e9d02e6945e1333189547c.setContent(html_15bc60463da1bc3c8f7429bd35098086);\\n \\n \\n\\n circle_marker_2f02ace3b0c7d8a0e2d779ca88391ebe.bindPopup(popup_7c9f8ddf83e9d02e6945e1333189547c)\\n ;\\n\\n \\n \\n \\n var circle_marker_c6b18045789b2386adabe88b9d3e2db1 = L.circleMarker(\\n [42.73093493095083, -73.24273006588936],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_4791d0f422d4c3d9c98494de35c64130 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_36e8162c09c6067e78cff5ff8920f2cf = $(`<div id="html_36e8162c09c6067e78cff5ff8920f2cf" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4791d0f422d4c3d9c98494de35c64130.setContent(html_36e8162c09c6067e78cff5ff8920f2cf);\\n \\n \\n\\n circle_marker_c6b18045789b2386adabe88b9d3e2db1.bindPopup(popup_4791d0f422d4c3d9c98494de35c64130)\\n ;\\n\\n \\n \\n \\n var circle_marker_2d9679888cb96c0e0fe9bc4891192683 = L.circleMarker(\\n [42.730934687380696, -73.24149996761956],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.5957691216057308, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5716c9288b13ed6315924e1d522a3ee5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9c8d3a55b8f96a53130abb6fd9c0621a = $(`<div id="html_9c8d3a55b8f96a53130abb6fd9c0621a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5716c9288b13ed6315924e1d522a3ee5.setContent(html_9c8d3a55b8f96a53130abb6fd9c0621a);\\n \\n \\n\\n circle_marker_2d9679888cb96c0e0fe9bc4891192683.bindPopup(popup_5716c9288b13ed6315924e1d522a3ee5)\\n ;\\n\\n \\n \\n \\n var circle_marker_7643335e0fd891eca39568e8846af035 = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_61f1eb2fe7271420134c6847d99be68a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14b8fdd9a734e90c84820dbf312bdc4f = $(`<div id="html_14b8fdd9a734e90c84820dbf312bdc4f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_61f1eb2fe7271420134c6847d99be68a.setContent(html_14b8fdd9a734e90c84820dbf312bdc4f);\\n \\n \\n\\n circle_marker_7643335e0fd891eca39568e8846af035.bindPopup(popup_61f1eb2fe7271420134c6847d99be68a)\\n ;\\n\\n \\n \\n \\n var circle_marker_2f041c208c4d7461e3e276f481dad390 = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_086c2ce0f9bcbc2fde128d2294123ed6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1db53a1bb487b06beab0df871e0df97e = $(`<div id="html_1db53a1bb487b06beab0df871e0df97e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_086c2ce0f9bcbc2fde128d2294123ed6.setContent(html_1db53a1bb487b06beab0df871e0df97e);\\n \\n \\n\\n circle_marker_2f041c208c4d7461e3e276f481dad390.bindPopup(popup_086c2ce0f9bcbc2fde128d2294123ed6)\\n ;\\n\\n \\n \\n \\n var circle_marker_c8d0f04d7b7a697733de07f783388896 = L.circleMarker(\\n [42.73093387767461, -73.23780967287182],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_0d695ef51914e21429ca57bffa8aef86 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d75d9198149c3bba14395df93fdd4059 = $(`<div id="html_d75d9198149c3bba14395df93fdd4059" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0d695ef51914e21429ca57bffa8aef86.setContent(html_d75d9198149c3bba14395df93fdd4059);\\n \\n \\n\\n circle_marker_c8d0f04d7b7a697733de07f783388896.bindPopup(popup_0d695ef51914e21429ca57bffa8aef86)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1fa821e2d59a499c37e689eaec551d5 = L.circleMarker(\\n [42.73093226484545, -73.23165918186231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_2e472ba14e63f4870fb5060871dc3b7b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_99c3b2460d7b6a7155836baef4d7b845 = $(`<div id="html_99c3b2460d7b6a7155836baef4d7b845" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2e472ba14e63f4870fb5060871dc3b7b.setContent(html_99c3b2460d7b6a7155836baef4d7b845);\\n \\n \\n\\n circle_marker_d1fa821e2d59a499c37e689eaec551d5.bindPopup(popup_2e472ba14e63f4870fb5060871dc3b7b)\\n ;\\n\\n \\n \\n \\n var circle_marker_7b7854c557ca9c4b07db673b77f5f923 = L.circleMarker(\\n [42.729129693483614, -73.25749146076663],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_cb7e1be8c8d8762e23de0897a20ad2d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f49cfb0b1924b0e8a862112fc598ca89 = $(`<div id="html_f49cfb0b1924b0e8a862112fc598ca89" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cb7e1be8c8d8762e23de0897a20ad2d5.setContent(html_f49cfb0b1924b0e8a862112fc598ca89);\\n \\n \\n\\n circle_marker_7b7854c557ca9c4b07db673b77f5f923.bindPopup(popup_cb7e1be8c8d8762e23de0897a20ad2d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_501a757f397a070e60e44bca37bf1ea1 = L.circleMarker(\\n [42.72912960790838, -73.25626139826112],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_9ff8881bb46fb39bf41686c1d17b2d36 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1c0bdd5d45e4c2f5458db9e75d9d01ae = $(`<div id="html_1c0bdd5d45e4c2f5458db9e75d9d01ae" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9ff8881bb46fb39bf41686c1d17b2d36.setContent(html_1c0bdd5d45e4c2f5458db9e75d9d01ae);\\n \\n \\n\\n circle_marker_501a757f397a070e60e44bca37bf1ea1.bindPopup(popup_9ff8881bb46fb39bf41686c1d17b2d36)\\n ;\\n\\n \\n \\n \\n var circle_marker_189ccde6a4283c46f010e633224038c9 = L.circleMarker(\\n [42.72912950916773, -73.25503133575926],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.111004122822376, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_0589f342fe6abf55604b7dceb1fae220 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fdf1ddd1829f1cb2d45cd19f35bc41b8 = $(`<div id="html_fdf1ddd1829f1cb2d45cd19f35bc41b8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0589f342fe6abf55604b7dceb1fae220.setContent(html_fdf1ddd1829f1cb2d45cd19f35bc41b8);\\n \\n \\n\\n circle_marker_189ccde6a4283c46f010e633224038c9.bindPopup(popup_0589f342fe6abf55604b7dceb1fae220)\\n ;\\n\\n \\n \\n \\n var circle_marker_3c4422fbdb9fc1ec495484bfd96e924b = L.circleMarker(\\n [42.72912864025003, -73.24765096085413],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a517fa7a9e1c09acecc6cc3f104e885a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_32e98afe44d8164035a7f830b14f7369 = $(`<div id="html_32e98afe44d8164035a7f830b14f7369" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a517fa7a9e1c09acecc6cc3f104e885a.setContent(html_32e98afe44d8164035a7f830b14f7369);\\n \\n \\n\\n circle_marker_3c4422fbdb9fc1ec495484bfd96e924b.bindPopup(popup_a517fa7a9e1c09acecc6cc3f104e885a)\\n ;\\n\\n \\n \\n \\n var circle_marker_15de3d6ad5fe833484aee35cc37a8a7c = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_689483babce19b216ac19aeb0a5b2d95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b52e36f085d40d46d5e0eb16393553c3 = $(`<div id="html_b52e36f085d40d46d5e0eb16393553c3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_689483babce19b216ac19aeb0a5b2d95.setContent(html_b52e36f085d40d46d5e0eb16393553c3);\\n \\n \\n\\n circle_marker_15de3d6ad5fe833484aee35cc37a8a7c.bindPopup(popup_689483babce19b216ac19aeb0a5b2d95)\\n ;\\n\\n \\n \\n \\n var circle_marker_48bb8eac70d6d1b19370083920378b76 = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.7841241161527712, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_50dff7e50fbc7e6ca02f0ab6d765e3f8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8a42fcd413b0f69de06a5f7ee687eda9 = $(`<div id="html_8a42fcd413b0f69de06a5f7ee687eda9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_50dff7e50fbc7e6ca02f0ab6d765e3f8.setContent(html_8a42fcd413b0f69de06a5f7ee687eda9);\\n \\n \\n\\n circle_marker_48bb8eac70d6d1b19370083920378b76.bindPopup(popup_50dff7e50fbc7e6ca02f0ab6d765e3f8)\\n ;\\n\\n \\n \\n \\n var circle_marker_99add87bbefef213f3f04cb87d13e02b = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_234f3081de3a3604c8a493da5a486a78 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f80bab94727ddea5284a44f339ea2282 = $(`<div id="html_f80bab94727ddea5284a44f339ea2282" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_234f3081de3a3604c8a493da5a486a78.setContent(html_f80bab94727ddea5284a44f339ea2282);\\n \\n \\n\\n circle_marker_99add87bbefef213f3f04cb87d13e02b.bindPopup(popup_234f3081de3a3604c8a493da5a486a78)\\n ;\\n\\n \\n \\n \\n var circle_marker_ed4252e33c0dbc4ca039ab27e5baea62 = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_6e10564545230e2a3146bb8e992ba50e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0bccda16832df17f565735dc0774b3d9 = $(`<div id="html_0bccda16832df17f565735dc0774b3d9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6e10564545230e2a3146bb8e992ba50e.setContent(html_0bccda16832df17f565735dc0774b3d9);\\n \\n \\n\\n circle_marker_ed4252e33c0dbc4ca039ab27e5baea62.bindPopup(popup_6e10564545230e2a3146bb8e992ba50e)\\n ;\\n\\n \\n \\n \\n var circle_marker_23d6c6955bd51d541080650095c2649d = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_aef3da1ed2f6d0d99e9566b5c9b0df32 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b06df1d2c6783b916d64da46dc4375f6 = $(`<div id="html_b06df1d2c6783b916d64da46dc4375f6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_aef3da1ed2f6d0d99e9566b5c9b0df32.setContent(html_b06df1d2c6783b916d64da46dc4375f6);\\n \\n \\n\\n circle_marker_23d6c6955bd51d541080650095c2649d.bindPopup(popup_aef3da1ed2f6d0d99e9566b5c9b0df32)\\n ;\\n\\n \\n \\n \\n var circle_marker_5af78ea3596259fe1617717e15ba7c38 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_e07373f38a4ed12781d9e302c4a61636 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7f869f42bc4251403686a2daa5e14fc2 = $(`<div id="html_7f869f42bc4251403686a2daa5e14fc2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e07373f38a4ed12781d9e302c4a61636.setContent(html_7f869f42bc4251403686a2daa5e14fc2);\\n \\n \\n\\n circle_marker_5af78ea3596259fe1617717e15ba7c38.bindPopup(popup_e07373f38a4ed12781d9e302c4a61636)\\n ;\\n\\n \\n \\n \\n var circle_marker_8b63b90caf1c684f4bf5ab688c5c6df0 = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_63c7872925c58bd49a8476ba9e61caba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_259e42d5a1befc8e6b89baa42eb8c1cd = $(`<div id="html_259e42d5a1befc8e6b89baa42eb8c1cd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63c7872925c58bd49a8476ba9e61caba.setContent(html_259e42d5a1befc8e6b89baa42eb8c1cd);\\n \\n \\n\\n circle_marker_8b63b90caf1c684f4bf5ab688c5c6df0.bindPopup(popup_63c7872925c58bd49a8476ba9e61caba)\\n ;\\n\\n \\n \\n \\n var circle_marker_eb5d5545fbda6546b451f71e462107f1 = L.circleMarker(\\n [42.729126448207694, -73.23658039902521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5ef6fbd920371e38c98f0eac56bd5403 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b9437b21d86845a33ca04169711f365b = $(`<div id="html_b9437b21d86845a33ca04169711f365b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5ef6fbd920371e38c98f0eac56bd5403.setContent(html_b9437b21d86845a33ca04169711f365b);\\n \\n \\n\\n circle_marker_eb5d5545fbda6546b451f71e462107f1.bindPopup(popup_5ef6fbd920371e38c98f0eac56bd5403)\\n ;\\n\\n \\n \\n \\n var circle_marker_af6a65946e7bafd229843845f91c20e3 = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_7bff61038aa2fa8b187be8da8618ff63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07bb3f7101222bb7822ec573aed1ec19 = $(`<div id="html_07bb3f7101222bb7822ec573aed1ec19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7bff61038aa2fa8b187be8da8618ff63.setContent(html_07bb3f7101222bb7822ec573aed1ec19);\\n \\n \\n\\n circle_marker_af6a65946e7bafd229843845f91c20e3.bindPopup(popup_7bff61038aa2fa8b187be8da8618ff63)\\n ;\\n\\n \\n \\n \\n var circle_marker_2322fa9310fac0246baa8d21a4227beb = L.circleMarker(\\n [42.72912400602253, -73.2279699627003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_49fc0728399ac2d4068e0a3209d5c67b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_74bc1190dc4449b4520d6e9c29f3c65a = $(`<div id="html_74bc1190dc4449b4520d6e9c29f3c65a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_49fc0728399ac2d4068e0a3209d5c67b.setContent(html_74bc1190dc4449b4520d6e9c29f3c65a);\\n \\n \\n\\n circle_marker_2322fa9310fac0246baa8d21a4227beb.bindPopup(popup_49fc0728399ac2d4068e0a3209d5c67b)\\n ;\\n\\n \\n \\n \\n var circle_marker_792fb909518091a183d01f6dc01bbd84 = L.circleMarker(\\n [42.72732226390921, -73.2759420758753],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_1ac6f004848e0494eb7e96ab1f3100f1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6e35bdcca178e9c2ca70056a58fde241 = $(`<div id="html_6e35bdcca178e9c2ca70056a58fde241" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1ac6f004848e0494eb7e96ab1f3100f1.setContent(html_6e35bdcca178e9c2ca70056a58fde241);\\n \\n \\n\\n circle_marker_792fb909518091a183d01f6dc01bbd84.bindPopup(popup_1ac6f004848e0494eb7e96ab1f3100f1)\\n ;\\n\\n \\n \\n \\n var circle_marker_fca05581a6870a05c28f9ceee650a08a = L.circleMarker(\\n [42.72732269176802, -73.26979194254899],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1073621666150775, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_c9d13939dea550c437ed5527550ae45c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2368f936a27fa68856fb87685d43532e = $(`<div id="html_2368f936a27fa68856fb87685d43532e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c9d13939dea550c437ed5527550ae45c.setContent(html_2368f936a27fa68856fb87685d43532e);\\n \\n \\n\\n circle_marker_fca05581a6870a05c28f9ceee650a08a.bindPopup(popup_c9d13939dea550c437ed5527550ae45c)\\n ;\\n\\n \\n \\n \\n var circle_marker_42b219989217938765f024a50902b68b = L.circleMarker(\\n [42.727322797087126, -73.2648718358472],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a2609f7fe67f6e9afef2f3d77ec97097 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed83a35604cb5e444fbfa10e888682b1 = $(`<div id="html_ed83a35604cb5e444fbfa10e888682b1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a2609f7fe67f6e9afef2f3d77ec97097.setContent(html_ed83a35604cb5e444fbfa10e888682b1);\\n \\n \\n\\n circle_marker_42b219989217938765f024a50902b68b.bindPopup(popup_a2609f7fe67f6e9afef2f3d77ec97097)\\n ;\\n\\n \\n \\n \\n var circle_marker_f382dc7fe0b4557ed9a0f0a35485404b = L.circleMarker(\\n [42.72732237581074, -73.25503162247706],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_94b14e1c4df44e8013507994b52550c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bd4f7b404cf0983beb9cac5d33ce53e3 = $(`<div id="html_bd4f7b404cf0983beb9cac5d33ce53e3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_94b14e1c4df44e8013507994b52550c1.setContent(html_bd4f7b404cf0983beb9cac5d33ce53e3);\\n \\n \\n\\n circle_marker_f382dc7fe0b4557ed9a0f0a35485404b.bindPopup(popup_94b14e1c4df44e8013507994b52550c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_b67d3c0bfe2c9aac660baa6acb793825 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_ca7e32ff358c32d036c52259702b5ec8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0dea27ebf25b0b2eb1e8e274ef0feced = $(`<div id="html_0dea27ebf25b0b2eb1e8e274ef0feced" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ca7e32ff358c32d036c52259702b5ec8.setContent(html_0dea27ebf25b0b2eb1e8e274ef0feced);\\n \\n \\n\\n circle_marker_b67d3c0bfe2c9aac660baa6acb793825.bindPopup(popup_ca7e32ff358c32d036c52259702b5ec8)\\n ;\\n\\n \\n \\n \\n var circle_marker_e9d6df9e02885c93b99bf67af0cccdad = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_50f4aa7d5281e513ff273e45efbf3ca5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d318f56bcd17d271ef52696487a3c398 = $(`<div id="html_d318f56bcd17d271ef52696487a3c398" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_50f4aa7d5281e513ff273e45efbf3ca5.setContent(html_d318f56bcd17d271ef52696487a3c398);\\n \\n \\n\\n circle_marker_e9d6df9e02885c93b99bf67af0cccdad.bindPopup(popup_50f4aa7d5281e513ff273e45efbf3ca5)\\n ;\\n\\n \\n \\n \\n var circle_marker_16a52a6adffa182543b77f70edcef025 = L.circleMarker(\\n [42.727321849215286, -73.25011151587555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_38f224c57e99d23eb964380ae86867ef = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0718eb1a3e2775251ed9a5a2478f7d77 = $(`<div id="html_0718eb1a3e2775251ed9a5a2478f7d77" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_38f224c57e99d23eb964380ae86867ef.setContent(html_0718eb1a3e2775251ed9a5a2478f7d77);\\n \\n \\n\\n circle_marker_16a52a6adffa182543b77f70edcef025.bindPopup(popup_38f224c57e99d23eb964380ae86867ef)\\n ;\\n\\n \\n \\n \\n var circle_marker_1ad73fbe6e8e3967b064644a752e283a = L.circleMarker(\\n [42.72732131603739, -73.24642143598837],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_fc9ced9a9e2a887912af7f75c674f094 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d8b49a14b204bca0607c40658bab5830 = $(`<div id="html_d8b49a14b204bca0607c40658bab5830" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc9ced9a9e2a887912af7f75c674f094.setContent(html_d8b49a14b204bca0607c40658bab5830);\\n \\n \\n\\n circle_marker_1ad73fbe6e8e3967b064644a752e283a.bindPopup(popup_fc9ced9a9e2a887912af7f75c674f094)\\n ;\\n\\n \\n \\n \\n var circle_marker_acda0b95214219586fd829fe2c5ce58b = L.circleMarker(\\n [42.72732016410985, -73.24027130300668],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_54075e76d12a9ef4c3ca837a504e319d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_86b9b1e6e52d3a9cdf78f36e63784dac = $(`<div id="html_86b9b1e6e52d3a9cdf78f36e63784dac" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_54075e76d12a9ef4c3ca837a504e319d.setContent(html_86b9b1e6e52d3a9cdf78f36e63784dac);\\n \\n \\n\\n circle_marker_acda0b95214219586fd829fe2c5ce58b.bindPopup(popup_54075e76d12a9ef4c3ca837a504e319d)\\n ;\\n\\n \\n \\n \\n var circle_marker_e2a2b96ce668987d11ad6fe42bece60c = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_fa5d233deb7d8578220fe2c34beb9016 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7da02f809c1e29ed019f9d3372ea0c44 = $(`<div id="html_7da02f809c1e29ed019f9d3372ea0c44" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fa5d233deb7d8578220fe2c34beb9016.setContent(html_7da02f809c1e29ed019f9d3372ea0c44);\\n \\n \\n\\n circle_marker_e2a2b96ce668987d11ad6fe42bece60c.bindPopup(popup_fa5d233deb7d8578220fe2c34beb9016)\\n ;\\n\\n \\n \\n \\n var circle_marker_f2aa33b8ebe41f44a84b11792b04db87 = L.circleMarker(\\n [42.7273190055999, -73.23535119680618],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_18dd42ffcc4dc988a9ab002ef642d3ba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0cb630a3ae5369fe5469ba6423777b70 = $(`<div id="html_0cb630a3ae5369fe5469ba6423777b70" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_18dd42ffcc4dc988a9ab002ef642d3ba.setContent(html_0cb630a3ae5369fe5469ba6423777b70);\\n \\n \\n\\n circle_marker_f2aa33b8ebe41f44a84b11792b04db87.bindPopup(popup_18dd42ffcc4dc988a9ab002ef642d3ba)\\n ;\\n\\n \\n \\n \\n var circle_marker_bf9b523a1f2d26a0769a3d09154e8501 = L.circleMarker(\\n [42.72731868306021, -73.23412117028607],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_66334fd0d3dc8a1c9de4e7525b118f2c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7c5ce78b0859f136dd10bb2e14b8f905 = $(`<div id="html_7c5ce78b0859f136dd10bb2e14b8f905" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_66334fd0d3dc8a1c9de4e7525b118f2c.setContent(html_7c5ce78b0859f136dd10bb2e14b8f905);\\n \\n \\n\\n circle_marker_bf9b523a1f2d26a0769a3d09154e8501.bindPopup(popup_66334fd0d3dc8a1c9de4e7525b118f2c)\\n ;\\n\\n \\n \\n \\n var circle_marker_0bee0fe537f582732a1d2a9238c9ebe2 = L.circleMarker(\\n [42.72731647135949, -73.22674101145786],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_1d5669c308d101a94a8884110b769bf8 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_425809b500682beb99a9888547bf9ce4 = $(`<div id="html_425809b500682beb99a9888547bf9ce4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1d5669c308d101a94a8884110b769bf8.setContent(html_425809b500682beb99a9888547bf9ce4);\\n \\n \\n\\n circle_marker_0bee0fe537f582732a1d2a9238c9ebe2.bindPopup(popup_1d5669c308d101a94a8884110b769bf8)\\n ;\\n\\n \\n \\n \\n var circle_marker_57f6b4a23a425b924bc6586b6cd08003 = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_cab7b24518176af4c09d93d4d2536976 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_587794c09231918a041e6874fe282af8 = $(`<div id="html_587794c09231918a041e6874fe282af8" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cab7b24518176af4c09d93d4d2536976.setContent(html_587794c09231918a041e6874fe282af8);\\n \\n \\n\\n circle_marker_57f6b4a23a425b924bc6586b6cd08003.bindPopup(popup_cab7b24518176af4c09d93d4d2536976)\\n ;\\n\\n \\n \\n \\n var circle_marker_ccdf5fd1f7577b8e14d47ae62b3be658 = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.949327084834294, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a53de200c0f54bead4aa3434ada8a201 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb0190c770186d80107cb94ea7c9252e = $(`<div id="html_eb0190c770186d80107cb94ea7c9252e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a53de200c0f54bead4aa3434ada8a201.setContent(html_eb0190c770186d80107cb94ea7c9252e);\\n \\n \\n\\n circle_marker_ccdf5fd1f7577b8e14d47ae62b3be658.bindPopup(popup_a53de200c0f54bead4aa3434ada8a201)\\n ;\\n\\n \\n \\n \\n var circle_marker_35cef1ed2cb5c949e368468d24e18412 = L.circleMarker(\\n [42.72551555839823, -73.2697917992017],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.997417539138688, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_634eef446d2b321ef966e4047af11341 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5300a19e2c5637d07e7399bcefb3df08 = $(`<div id="html_5300a19e2c5637d07e7399bcefb3df08" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_634eef446d2b321ef966e4047af11341.setContent(html_5300a19e2c5637d07e7399bcefb3df08);\\n \\n \\n\\n circle_marker_35cef1ed2cb5c949e368468d24e18412.bindPopup(popup_634eef446d2b321ef966e4047af11341)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b2062204139db6413d382e8c1892e4e = L.circleMarker(\\n [42.72551560447347, -73.2685618083649],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.725898905580716, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_cc9dfefe5bb8ed6ca427b25e1c81a392 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_14f4109606b1f68650e48d28ff75cae4 = $(`<div id="html_14f4109606b1f68650e48d28ff75cae4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_cc9dfefe5bb8ed6ca427b25e1c81a392.setContent(html_14f4109606b1f68650e48d28ff75cae4);\\n \\n \\n\\n circle_marker_6b2062204139db6413d382e8c1892e4e.bindPopup(popup_cc9dfefe5bb8ed6ca427b25e1c81a392)\\n ;\\n\\n \\n \\n \\n var circle_marker_c95d7f8a219b5702b188ca3a63cc7aa3 = L.circleMarker(\\n [42.72551563738435, -73.26733181752655],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_050a9135008a7521a46ca53f63623fa2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dbe3ea15dc8b0a87dfdccc740fc7e52a = $(`<div id="html_dbe3ea15dc8b0a87dfdccc740fc7e52a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_050a9135008a7521a46ca53f63623fa2.setContent(html_dbe3ea15dc8b0a87dfdccc740fc7e52a);\\n \\n \\n\\n circle_marker_c95d7f8a219b5702b188ca3a63cc7aa3.bindPopup(popup_050a9135008a7521a46ca53f63623fa2)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b0f837837447a5d5af50bff9f144b84 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a24211237f4757f3149875eafb7a20a9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_39ae3835428ec154320607fa8539c523 = $(`<div id="html_39ae3835428ec154320607fa8539c523" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a24211237f4757f3149875eafb7a20a9.setContent(html_39ae3835428ec154320607fa8539c523);\\n \\n \\n\\n circle_marker_2b0f837837447a5d5af50bff9f144b84.bindPopup(popup_a24211237f4757f3149875eafb7a20a9)\\n ;\\n\\n \\n \\n \\n var circle_marker_968c76fb63bd70acdfa985529b0e96fb = L.circleMarker(\\n [42.72551524245375, -73.25503190917165],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_daea66add0fef91b293d7991c378fdf5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_60f124f132f652ece4ce2992f1ba6cb5 = $(`<div id="html_60f124f132f652ece4ce2992f1ba6cb5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_daea66add0fef91b293d7991c378fdf5.setContent(html_60f124f132f652ece4ce2992f1ba6cb5);\\n \\n \\n\\n circle_marker_968c76fb63bd70acdfa985529b0e96fb.bindPopup(popup_daea66add0fef91b293d7991c378fdf5)\\n ;\\n\\n \\n \\n \\n var circle_marker_2b5ff0490b1a17790c2b700d37340ccd = L.circleMarker(\\n [42.72551500549539, -73.25257192753408],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_fd4cf059870a0465eb6e291b9da15369 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7115414d3d351e5e3ca8a72cf5049395 = $(`<div id="html_7115414d3d351e5e3ca8a72cf5049395" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fd4cf059870a0465eb6e291b9da15369.setContent(html_7115414d3d351e5e3ca8a72cf5049395);\\n \\n \\n\\n circle_marker_2b5ff0490b1a17790c2b700d37340ccd.bindPopup(popup_fd4cf059870a0465eb6e291b9da15369)\\n ;\\n\\n \\n \\n \\n var circle_marker_010a6b10f0e7c37adb41b967815482a7 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_db175358c03dc450a78b5bc9bccfeeda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_323d893d9b9b7a88f31cc4587630ee47 = $(`<div id="html_323d893d9b9b7a88f31cc4587630ee47" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_db175358c03dc450a78b5bc9bccfeeda.setContent(html_323d893d9b9b7a88f31cc4587630ee47);\\n \\n \\n\\n circle_marker_010a6b10f0e7c37adb41b967815482a7.bindPopup(popup_db175358c03dc450a78b5bc9bccfeeda)\\n ;\\n\\n \\n \\n \\n var circle_marker_0c117c8a45b2e35141c35f305c898404 = L.circleMarker(\\n [42.72551328754733, -73.24150201048379],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_9b5e057b73a6a23fe59559f8803d41f6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d6810c3bd46eebe5c1708bb7e3426ce4 = $(`<div id="html_d6810c3bd46eebe5c1708bb7e3426ce4" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9b5e057b73a6a23fe59559f8803d41f6.setContent(html_d6810c3bd46eebe5c1708bb7e3426ce4);\\n \\n \\n\\n circle_marker_0c117c8a45b2e35141c35f305c898404.bindPopup(popup_9b5e057b73a6a23fe59559f8803d41f6)\\n ;\\n\\n \\n \\n \\n var circle_marker_fdc86e3d00ddba7ee7318ca2da4647bb = L.circleMarker(\\n [42.72551276097322, -73.23904202901275],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_5d1b1a853a522dbd579f43c9f4d42077 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_68c1b55e7c2e3dfd5b30fc86bfc68875 = $(`<div id="html_68c1b55e7c2e3dfd5b30fc86bfc68875" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5d1b1a853a522dbd579f43c9f4d42077.setContent(html_68c1b55e7c2e3dfd5b30fc86bfc68875);\\n \\n \\n\\n circle_marker_fdc86e3d00ddba7ee7318ca2da4647bb.bindPopup(popup_5d1b1a853a522dbd579f43c9f4d42077)\\n ;\\n\\n \\n \\n \\n var circle_marker_c5f4b7b1f04e29a75489686bd0cbe825 = L.circleMarker(\\n [42.72551187237944, -73.23535205688977],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_329c9b5dbbbcbdcc7082b5e61749ad63 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_85c69eaf02097b2c37870ad93e169b81 = $(`<div id="html_85c69eaf02097b2c37870ad93e169b81" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_329c9b5dbbbcbdcc7082b5e61749ad63.setContent(html_85c69eaf02097b2c37870ad93e169b81);\\n \\n \\n\\n circle_marker_c5f4b7b1f04e29a75489686bd0cbe825.bindPopup(popup_329c9b5dbbbcbdcc7082b5e61749ad63)\\n ;\\n\\n \\n \\n \\n var circle_marker_3940cfe38c7392dd48d75aa30a07af88 = L.circleMarker(\\n [42.72551121416183, -73.23289207553623],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_bdd154023fe89b91205ab502f3776b60 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c864efa924ce95195e7848caea277ce5 = $(`<div id="html_c864efa924ce95195e7848caea277ce5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdd154023fe89b91205ab502f3776b60.setContent(html_c864efa924ce95195e7848caea277ce5);\\n \\n \\n\\n circle_marker_3940cfe38c7392dd48d75aa30a07af88.bindPopup(popup_bdd154023fe89b91205ab502f3776b60)\\n ;\\n\\n \\n \\n \\n var circle_marker_5a8db1a3084c346491b487ca2a15f17b = L.circleMarker(\\n [42.72551050328681, -73.23043209423697],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a5870a34fdb861839356d7c6380ed8a2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b31dc7b5f21356fb018a7749480aed61 = $(`<div id="html_b31dc7b5f21356fb018a7749480aed61" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a5870a34fdb861839356d7c6380ed8a2.setContent(html_b31dc7b5f21356fb018a7749480aed61);\\n \\n \\n\\n circle_marker_5a8db1a3084c346491b487ca2a15f17b.bindPopup(popup_a5870a34fdb861839356d7c6380ed8a2)\\n ;\\n\\n \\n \\n \\n var circle_marker_f3e34d62546a69d8d036834eb299aca2 = L.circleMarker(\\n [42.72551012810279, -73.22920210360903],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_879d8b3c039eff3e79ec19db2f8cfa2f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_9d39049145e1bbcc3a0d332260489945 = $(`<div id="html_9d39049145e1bbcc3a0d332260489945" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_879d8b3c039eff3e79ec19db2f8cfa2f.setContent(html_9d39049145e1bbcc3a0d332260489945);\\n \\n \\n\\n circle_marker_f3e34d62546a69d8d036834eb299aca2.bindPopup(popup_879d8b3c039eff3e79ec19db2f8cfa2f)\\n ;\\n\\n \\n \\n \\n var circle_marker_3e3b3e6bb258b480eb07282654426d2d = L.circleMarker(\\n [42.72550973975441, -73.22797211299621],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_98ae393f8ac7c0bcf77dda2d7d5c3f0e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4a283916bbfc7642a2259beb6dbc4ef = $(`<div id="html_d4a283916bbfc7642a2259beb6dbc4ef" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_98ae393f8ac7c0bcf77dda2d7d5c3f0e.setContent(html_d4a283916bbfc7642a2259beb6dbc4ef);\\n \\n \\n\\n circle_marker_3e3b3e6bb258b480eb07282654426d2d.bindPopup(popup_98ae393f8ac7c0bcf77dda2d7d5c3f0e)\\n ;\\n\\n \\n \\n \\n var circle_marker_41b333e88b2224a90f7f669ec6a69807 = L.circleMarker(\\n [42.72370829339024, -73.25749210582943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_1b0df9a6526c00526c6b2096169f13c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_97d00aba65eda9fc895b6a1bc3bcad4e = $(`<div id="html_97d00aba65eda9fc895b6a1bc3bcad4e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1b0df9a6526c00526c6b2096169f13c5.setContent(html_97d00aba65eda9fc895b6a1bc3bcad4e);\\n \\n \\n\\n circle_marker_41b333e88b2224a90f7f669ec6a69807.bindPopup(popup_1b0df9a6526c00526c6b2096169f13c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_00cb311dae0e3f3b9531618749009b45 = L.circleMarker(\\n [42.723707997204286, -73.25380224085578],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_a709fdd3291c0e430043429614de8958 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d058460f220bcd4d512ed0e49c8c0233 = $(`<div id="html_d058460f220bcd4d512ed0e49c8c0233" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a709fdd3291c0e430043429614de8958.setContent(html_d058460f220bcd4d512ed0e49c8c0233);\\n \\n \\n\\n circle_marker_00cb311dae0e3f3b9531618749009b45.bindPopup(popup_a709fdd3291c0e430043429614de8958)\\n ;\\n\\n \\n \\n \\n var circle_marker_9b8988a8b1ca5b9ceecaf1a28a42d2b5 = L.circleMarker(\\n [42.72370441664539, -73.23412296205429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_968a347ae49c49909047d51f70af142a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e4e761b0a30e9ef1ed69d3f81197939 = $(`<div id="html_1e4e761b0a30e9ef1ed69d3f81197939" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_968a347ae49c49909047d51f70af142a.setContent(html_1e4e761b0a30e9ef1ed69d3f81197939);\\n \\n \\n\\n circle_marker_9b8988a8b1ca5b9ceecaf1a28a42d2b5.bindPopup(popup_968a347ae49c49909047d51f70af142a)\\n ;\\n\\n \\n \\n \\n var circle_marker_b5875b12c994b68e59c33eccb4c329c7 = L.circleMarker(\\n [42.72370299495297, -73.22920314279241],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f63e623fcf6aa61a0d3c6da4c4eeff8d);\\n \\n \\n var popup_64faf1d257f144cce041f129b6e4c5cd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a3c1e036c4482f1abf822a70aab88be9 = $(`<div id="html_a3c1e036c4482f1abf822a70aab88be9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64faf1d257f144cce041f129b6e4c5cd.setContent(html_a3c1e036c4482f1abf822a70aab88be9);\\n \\n \\n\\n circle_marker_b5875b12c994b68e59c33eccb4c329c7.bindPopup(popup_64faf1d257f144cce041f129b6e4c5cd)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Spicebush": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_09574e477bea7bf154538fc555250d4f {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_09574e477bea7bf154538fc555250d4f" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_09574e477bea7bf154538fc555250d4f = L.map(\\n "map_09574e477bea7bf154538fc555250d4f",\\n {\\n center: [42.730935161355, -73.24396016416856],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_72299b88a5e05a6610155968529b82fd = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_09574e477bea7bf154538fc555250d4f);\\n \\n \\n var circle_marker_f498482a9abbef79faa30fbcea56da74 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_09574e477bea7bf154538fc555250d4f);\\n \\n \\n var popup_775cdf49d37b4aef73d3d614626aba28 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_528f1d2d0129dcec5f40bfaeeb6a2ca6 = $(`<div id="html_528f1d2d0129dcec5f40bfaeeb6a2ca6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_775cdf49d37b4aef73d3d614626aba28.setContent(html_528f1d2d0129dcec5f40bfaeeb6a2ca6);\\n \\n \\n\\n circle_marker_f498482a9abbef79faa30fbcea56da74.bindPopup(popup_775cdf49d37b4aef73d3d614626aba28)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Spruce, Norway\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_bc9ffcf7984570d9a02bd9e4f85027d5 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_bc9ffcf7984570d9a02bd9e4f85027d5" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_bc9ffcf7984570d9a02bd9e4f85027d5 = L.map(\\n "map_bc9ffcf7984570d9a02bd9e4f85027d5",\\n {\\n center: [42.728220436207536, -73.22858562127104],\\n crs: L.CRS.EPSG3857,\\n zoom: 13,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_3172d3fdc840eda9407c7efd45f8d5bf = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_bc9ffcf7984570d9a02bd9e4f85027d5);\\n \\n \\n var circle_marker_de33dc617237c20a68cc1e943eb005ac = L.circleMarker(\\n [42.72912613882035, -73.23535033665289],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_bc9ffcf7984570d9a02bd9e4f85027d5);\\n \\n \\n var popup_a90b2f1d9e13274b5a0dda739e51b7c1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fd2cc25f7525a1ec7bda0c2ba08bd1da = $(`<div id="html_fd2cc25f7525a1ec7bda0c2ba08bd1da" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a90b2f1d9e13274b5a0dda739e51b7c1.setContent(html_fd2cc25f7525a1ec7bda0c2ba08bd1da);\\n \\n \\n\\n circle_marker_de33dc617237c20a68cc1e943eb005ac.bindPopup(popup_a90b2f1d9e13274b5a0dda739e51b7c1)\\n ;\\n\\n \\n \\n \\n var circle_marker_c52b9df7c00464752f00c2131b075fe9 = L.circleMarker(\\n [42.72731473359472, -73.22182090588917],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.985410660720923, "stroke": true, "weight": 3}\\n ).addTo(map_bc9ffcf7984570d9a02bd9e4f85027d5);\\n \\n \\n var popup_869d7aa1ac2f76c4a17905a29a4ca16d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e29b0dddaf6c7752790fd655e409b12a = $(`<div id="html_e29b0dddaf6c7752790fd655e409b12a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_869d7aa1ac2f76c4a17905a29a4ca16d.setContent(html_e29b0dddaf6c7752790fd655e409b12a);\\n \\n \\n\\n circle_marker_c52b9df7c00464752f00c2131b075fe9.bindPopup(popup_869d7aa1ac2f76c4a17905a29a4ca16d)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Spruce, red\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_e9f90aa5a903d81454d316e53f61b68b {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_e9f90aa5a903d81454d316e53f61b68b" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_e9f90aa5a903d81454d316e53f61b68b = L.map(\\n "map_e9f90aa5a903d81454d316e53f61b68b",\\n {\\n center: [42.73364733595334, -73.25687614335469],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_62d06d85e8a14e5437a3ca6b447d9a55 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var circle_marker_335a4fb9c36f103d2b7b5d60ba8457b7 = L.circleMarker(\\n [42.74358646408128, -73.27594497983429],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_198245bff0a020af536a7999f434ec84 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c0b7c3901f4940c81973022e20c44971 = $(`<div id="html_c0b7c3901f4940c81973022e20c44971" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_198245bff0a020af536a7999f434ec84.setContent(html_c0b7c3901f4940c81973022e20c44971);\\n \\n \\n\\n circle_marker_335a4fb9c36f103d2b7b5d60ba8457b7.bindPopup(popup_198245bff0a020af536a7999f434ec84)\\n ;\\n\\n \\n \\n \\n var circle_marker_9f9f9b6619b1ee9d1f1f11a4b95ab8a6 = L.circleMarker(\\n [42.7381655709953, -73.26733231936404],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_a137fd919b624e62859a7dc5760085a1 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_37a4bfea3f2c94d3545599a5001926d6 = $(`<div id="html_37a4bfea3f2c94d3545599a5001926d6" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a137fd919b624e62859a7dc5760085a1.setContent(html_37a4bfea3f2c94d3545599a5001926d6);\\n \\n \\n\\n circle_marker_9f9f9b6619b1ee9d1f1f11a4b95ab8a6.bindPopup(popup_a137fd919b624e62859a7dc5760085a1)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1e324ecbff337d1f286d88f2b5bda51 = L.circleMarker(\\n [42.73635843762231, -73.26733224765555],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.970821321441846, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_b454dbf5236bc151612e7fd372572ba5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_41a5fbff39f43634247cd0f830a1b486 = $(`<div id="html_41a5fbff39f43634247cd0f830a1b486" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b454dbf5236bc151612e7fd372572ba5.setContent(html_41a5fbff39f43634247cd0f830a1b486);\\n \\n \\n\\n circle_marker_c1e324ecbff337d1f286d88f2b5bda51.bindPopup(popup_b454dbf5236bc151612e7fd372572ba5)\\n ;\\n\\n \\n \\n \\n var circle_marker_ea7f467234989795faec4282f08dd12a = L.circleMarker(\\n [42.73635735129912, -73.24887915927982],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_0cba0ce0f2fecf67e71ecd1bc4242d64 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a6e3dbc3a28f9f99df17b5ea36c76374 = $(`<div id="html_a6e3dbc3a28f9f99df17b5ea36c76374" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0cba0ce0f2fecf67e71ecd1bc4242d64.setContent(html_a6e3dbc3a28f9f99df17b5ea36c76374);\\n \\n \\n\\n circle_marker_ea7f467234989795faec4282f08dd12a.bindPopup(popup_0cba0ce0f2fecf67e71ecd1bc4242d64)\\n ;\\n\\n \\n \\n \\n var circle_marker_c1ac26346e244a68a65799c294a98b2a = L.circleMarker(\\n [42.73635527740949, -73.23780730687508],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_e75a0f6978c5a6b0f7b1353e19a78f6e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4cad36b8df115c8b31f8c353cabf8947 = $(`<div id="html_4cad36b8df115c8b31f8c353cabf8947" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e75a0f6978c5a6b0f7b1353e19a78f6e.setContent(html_4cad36b8df115c8b31f8c353cabf8947);\\n \\n \\n\\n circle_marker_c1ac26346e244a68a65799c294a98b2a.bindPopup(popup_e75a0f6978c5a6b0f7b1353e19a78f6e)\\n ;\\n\\n \\n \\n \\n var circle_marker_4e05dbc2c34f9ae4a32a044b38d44265 = L.circleMarker(\\n [42.734551165995605, -73.25872098559225],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_63542304e234f2161acab6466548069a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_a4ba4b3041af0a3ef95c01b658d8f2c1 = $(`<div id="html_a4ba4b3041af0a3ef95c01b658d8f2c1" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_63542304e234f2161acab6466548069a.setContent(html_a4ba4b3041af0a3ef95c01b658d8f2c1);\\n \\n \\n\\n circle_marker_4e05dbc2c34f9ae4a32a044b38d44265.bindPopup(popup_63542304e234f2161acab6466548069a)\\n ;\\n\\n \\n \\n \\n var circle_marker_57632c287f99036083eef75c71ee27cd = L.circleMarker(\\n [42.73454942794894, -73.24395894537108],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_987f6390506692438dc18d8f3eea17c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d4fadb6d10c2ac78af4380ea4304c584 = $(`<div id="html_d4fadb6d10c2ac78af4380ea4304c584" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_987f6390506692438dc18d8f3eea17c6.setContent(html_d4fadb6d10c2ac78af4380ea4304c584);\\n \\n \\n\\n circle_marker_57632c287f99036083eef75c71ee27cd.bindPopup(popup_987f6390506692438dc18d8f3eea17c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_4cd1f8b5f89e6b0c71562c824d319c35 = L.circleMarker(\\n [42.73274387463036, -73.25626089644396],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_0a683fda7c5b7342153c388793855814 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ba3ae8141b21851a6ec95cf03e05b892 = $(`<div id="html_ba3ae8141b21851a6ec95cf03e05b892" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_0a683fda7c5b7342153c388793855814.setContent(html_ba3ae8141b21851a6ec95cf03e05b892);\\n \\n \\n\\n circle_marker_4cd1f8b5f89e6b0c71562c824d319c35.bindPopup(popup_0a683fda7c5b7342153c388793855814)\\n ;\\n\\n \\n \\n \\n var circle_marker_577893b8f7098b25124882e58cdc24ce = L.circleMarker(\\n [42.73274366396657, -73.2538006280681],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_c8570e99867ee23f345af30adb2ca20e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e30807bdde33e02f467576e5d61b0503 = $(`<div id="html_e30807bdde33e02f467576e5d61b0503" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c8570e99867ee23f345af30adb2ca20e.setContent(html_e30807bdde33e02f467576e5d61b0503);\\n \\n \\n\\n circle_marker_577893b8f7098b25124882e58cdc24ce.bindPopup(popup_c8570e99867ee23f345af30adb2ca20e)\\n ;\\n\\n \\n \\n \\n var circle_marker_6b2090e04716f1cfbe8ff5ea754a92ce = L.circleMarker(\\n [42.73274271597954, -73.24641982307008],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_53426d0e2735d959dc5c853a297387cf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_fc1eb5229ef4d3388eed008d8d7608aa = $(`<div id="html_fc1eb5229ef4d3388eed008d8d7608aa" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_53426d0e2735d959dc5c853a297387cf.setContent(html_fc1eb5229ef4d3388eed008d8d7608aa);\\n \\n \\n\\n circle_marker_6b2090e04716f1cfbe8ff5ea754a92ce.bindPopup(popup_53426d0e2735d959dc5c853a297387cf)\\n ;\\n\\n \\n \\n \\n var circle_marker_66d19338d8430f7ddeb9cde910b8d27d = L.circleMarker(\\n [42.73274206423847, -73.24272942066979],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_9cc061da1c0e966563d178996ca5d988 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5aa187543172eac4455221489cca8995 = $(`<div id="html_5aa187543172eac4455221489cca8995" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9cc061da1c0e966563d178996ca5d988.setContent(html_5aa187543172eac4455221489cca8995);\\n \\n \\n\\n circle_marker_66d19338d8430f7ddeb9cde910b8d27d.bindPopup(popup_9cc061da1c0e966563d178996ca5d988)\\n ;\\n\\n \\n \\n \\n var circle_marker_ec70d2ee3f971934077694f5f2d62cce = L.circleMarker(\\n [42.73093700458845, -73.26118154077197],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_84d2dd7a50a638853c9eee380d873ae5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_791283ae340e360ff32fe83ca42eddd3 = $(`<div id="html_791283ae340e360ff32fe83ca42eddd3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_84d2dd7a50a638853c9eee380d873ae5.setContent(html_791283ae340e360ff32fe83ca42eddd3);\\n \\n \\n\\n circle_marker_ec70d2ee3f971934077694f5f2d62cce.bindPopup(popup_84d2dd7a50a638853c9eee380d873ae5)\\n ;\\n\\n \\n \\n \\n var circle_marker_d805e8312e9a227cab12b8100bceb319 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_2939fdf1b1177698d426c4caa357fa83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7e2854e95c3103f804c1a74522edc270 = $(`<div id="html_7e2854e95c3103f804c1a74522edc270" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2939fdf1b1177698d426c4caa357fa83.setContent(html_7e2854e95c3103f804c1a74522edc270);\\n \\n \\n\\n circle_marker_d805e8312e9a227cab12b8100bceb319.bindPopup(popup_2939fdf1b1177698d426c4caa357fa83)\\n ;\\n\\n \\n \\n \\n var circle_marker_e6db9cf907e1c0b5f0ab58eb4aaeb1e1 = L.circleMarker(\\n [42.730934160742585, -73.23903977111027],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_60e37c43f83ed536f8c1d1649edbb499 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3b12b175fc1a4bb52476f4fbca562189 = $(`<div id="html_3b12b175fc1a4bb52476f4fbca562189" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_60e37c43f83ed536f8c1d1649edbb499.setContent(html_3b12b175fc1a4bb52476f4fbca562189);\\n \\n \\n\\n circle_marker_e6db9cf907e1c0b5f0ab58eb4aaeb1e1.bindPopup(popup_60e37c43f83ed536f8c1d1649edbb499)\\n ;\\n\\n \\n \\n \\n var circle_marker_bedddbd4cb4cb8ca32f4d2abfa01918c = L.circleMarker(\\n [42.7291288179832, -73.2488810233231],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_b5f471df4fe7e5b94429382db847c0b5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_98b8f8c8b0e01825836affd18083648c = $(`<div id="html_98b8f8c8b0e01825836affd18083648c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5f471df4fe7e5b94429382db847c0b5.setContent(html_98b8f8c8b0e01825836affd18083648c);\\n \\n \\n\\n circle_marker_bedddbd4cb4cb8ca32f4d2abfa01918c.bindPopup(popup_b5f471df4fe7e5b94429382db847c0b5)\\n ;\\n\\n \\n \\n \\n var circle_marker_26b3721e89425746c4f8abc528462cd9 = L.circleMarker(\\n [42.72912844935144, -73.2464208983925],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_51fb06cde5b55a10a6b7c739d72c95be = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_17f8e1d75aaebdb192fc6ef68172d6fd = $(`<div id="html_17f8e1d75aaebdb192fc6ef68172d6fd" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_51fb06cde5b55a10a6b7c739d72c95be.setContent(html_17f8e1d75aaebdb192fc6ef68172d6fd);\\n \\n \\n\\n circle_marker_26b3721e89425746c4f8abc528462cd9.bindPopup(popup_51fb06cde5b55a10a6b7c739d72c95be)\\n ;\\n\\n \\n \\n \\n var circle_marker_8e12d5c75754269dba92b477ff000c6c = L.circleMarker(\\n [42.72912824528744, -73.24519083593869],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_bdd703bac105f9a359afe302cde07679 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c587ca923040e83ccb74573845085f8c = $(`<div id="html_c587ca923040e83ccb74573845085f8c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bdd703bac105f9a359afe302cde07679.setContent(html_c587ca923040e83ccb74573845085f8c);\\n \\n \\n\\n circle_marker_8e12d5c75754269dba92b477ff000c6c.bindPopup(popup_bdd703bac105f9a359afe302cde07679)\\n ;\\n\\n \\n \\n \\n var circle_marker_6fe68b0f03d17db585e2fa347b2445e5 = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_a121948fd4ec05215a2b081b30d3effd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_c826f074af9607817b2b90196a386ac7 = $(`<div id="html_c826f074af9607817b2b90196a386ac7" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a121948fd4ec05215a2b081b30d3effd.setContent(html_c826f074af9607817b2b90196a386ac7);\\n \\n \\n\\n circle_marker_6fe68b0f03d17db585e2fa347b2445e5.bindPopup(popup_a121948fd4ec05215a2b081b30d3effd)\\n ;\\n\\n \\n \\n \\n var circle_marker_d1087b4a883380054f3e011f97d856cf = L.circleMarker(\\n [42.72551513055674, -73.2759417533439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_e0cb9e5a90dae7b266d7f11ba7204e8e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f51c3509939c0c2d81f91f7e693209c2 = $(`<div id="html_f51c3509939c0c2d81f91f7e693209c2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_e0cb9e5a90dae7b266d7f11ba7204e8e.setContent(html_f51c3509939c0c2d81f91f7e693209c2);\\n \\n \\n\\n circle_marker_d1087b4a883380054f3e011f97d856cf.bindPopup(popup_e0cb9e5a90dae7b266d7f11ba7204e8e)\\n ;\\n\\n \\n \\n \\n var circle_marker_f5bde0c49dc0a58125387b9287f5d530 = L.circleMarker(\\n [42.7255154267547, -73.25749189082592],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_a76fe83f4903b506dcbab35f24f0231b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_20ac5cb8b2beb73598cca9be57591a86 = $(`<div id="html_20ac5cb8b2beb73598cca9be57591a86" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a76fe83f4903b506dcbab35f24f0231b.setContent(html_20ac5cb8b2beb73598cca9be57591a86);\\n \\n \\n\\n circle_marker_f5bde0c49dc0a58125387b9287f5d530.bindPopup(popup_a76fe83f4903b506dcbab35f24f0231b)\\n ;\\n\\n \\n \\n \\n var circle_marker_77f5556ff6dc79c8fb46e33a516467a3 = L.circleMarker(\\n [42.72551486726968, -73.25134193672287],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_c58fbd423b1e55e702cae9c2206f578a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_07c6f5888513018f8c5b7c968767326c = $(`<div id="html_07c6f5888513018f8c5b7c968767326c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c58fbd423b1e55e702cae9c2206f578a.setContent(html_07c6f5888513018f8c5b7c968767326c);\\n \\n \\n\\n circle_marker_77f5556ff6dc79c8fb46e33a516467a3.bindPopup(popup_c58fbd423b1e55e702cae9c2206f578a)\\n ;\\n\\n \\n \\n \\n var circle_marker_e2cb3f02a33268474cdd434ef6fa53ca = L.circleMarker(\\n [42.7237082078254, -73.25626215083439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_e9f90aa5a903d81454d316e53f61b68b);\\n \\n \\n var popup_2676b613c613b31cb4a67e3c4fea1ddd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_33c9750740a2f226f54d30bd8ec2f1ba = $(`<div id="html_33c9750740a2f226f54d30bd8ec2f1ba" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2676b613c613b31cb4a67e3c4fea1ddd.setContent(html_33c9750740a2f226f54d30bd8ec2f1ba);\\n \\n \\n\\n circle_marker_e2cb3f02a33268474cdd434ef6fa53ca.bindPopup(popup_2676b613c613b31cb4a67e3c4fea1ddd)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Virginia creeper": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_bbe09b0f4b89c3c63dc927a644fcaf33 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_bbe09b0f4b89c3c63dc927a644fcaf33" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_bbe09b0f4b89c3c63dc927a644fcaf33 = L.map(\\n "map_bbe09b0f4b89c3c63dc927a644fcaf33",\\n {\\n center: [42.7399700969814, -73.24026628422554],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_c64d2b864a5707a8f8a0fcde463d41d7 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_bbe09b0f4b89c3c63dc927a644fcaf33);\\n \\n \\n var circle_marker_d41632428da768a8cee0a1a205bb9eec = L.circleMarker(\\n [42.7399700969814, -73.24026628422554],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_bbe09b0f4b89c3c63dc927a644fcaf33);\\n \\n \\n var popup_75ee2cb6d3afc813607e0405cc4745d7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_76c4d9f73b68db4add37c1aa70ad9064 = $(`<div id="html_76c4d9f73b68db4add37c1aa70ad9064" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_75ee2cb6d3afc813607e0405cc4745d7.setContent(html_76c4d9f73b68db4add37c1aa70ad9064);\\n \\n \\n\\n circle_marker_d41632428da768a8cee0a1a205bb9eec.bindPopup(popup_75ee2cb6d3afc813607e0405cc4745d7)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "\\"Willow, black\\"": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_7276e6cfe69d7582812e92b388fb3759 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_7276e6cfe69d7582812e92b388fb3759" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_7276e6cfe69d7582812e92b388fb3759 = L.map(\\n "map_7276e6cfe69d7582812e92b388fb3759",\\n {\\n center: [42.736354671702045, -73.23534689534263],\\n crs: L.CRS.EPSG3857,\\n zoom: 12,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_6ad8784011b3bab1a7ef23f54c2dd34b = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 10, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_7276e6cfe69d7582812e92b388fb3759);\\n \\n \\n var circle_marker_484b3827fa51624727abf8e4705eb30e = L.circleMarker(\\n [42.736354671702045, -73.23534689534263],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_7276e6cfe69d7582812e92b388fb3759);\\n \\n \\n var popup_1eabf30354573563be747c20fe8fadf2 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_4e3e735e711d3ade79be35ced7dbe58f = $(`<div id="html_4e3e735e711d3ade79be35ced7dbe58f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1eabf30354573563be747c20fe8fadf2.setContent(html_4e3e735e711d3ade79be35ced7dbe58f);\\n \\n \\n\\n circle_marker_484b3827fa51624727abf8e4705eb30e.bindPopup(popup_1eabf30354573563be747c20fe8fadf2)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Witch-hazel": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_f8adf017e5e89e47349dfd03ccc2c586 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_f8adf017e5e89e47349dfd03ccc2c586" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_f8adf017e5e89e47349dfd03ccc2c586 = L.map(\\n "map_f8adf017e5e89e47349dfd03ccc2c586",\\n {\\n center: [42.73635671967905, -73.24765189539136],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_794c2cbad8645a9168687668e2ef2643 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var circle_marker_1a38b5dd026f8e30286ff781438fc72f = L.circleMarker(\\n [42.747200842737584, -73.2747152043915],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_ed101f884af0d662b00f29a47716208e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_b897e3971bfe2ecb802457c97dd4db60 = $(`<div id="html_b897e3971bfe2ecb802457c97dd4db60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ed101f884af0d662b00f29a47716208e.setContent(html_b897e3971bfe2ecb802457c97dd4db60);\\n \\n \\n\\n circle_marker_1a38b5dd026f8e30286ff781438fc72f.bindPopup(popup_ed101f884af0d662b00f29a47716208e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b11826274869ad171029ccb503a14c79 = L.circleMarker(\\n [42.74720094151825, -73.27348478333262],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.1073621666150775, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_07daa1a549dd44294200708947a6fad6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_2b897c863fb0ba79deb2fbeea306c18c = $(`<div id="html_2b897c863fb0ba79deb2fbeea306c18c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_07daa1a549dd44294200708947a6fad6.setContent(html_2b897c863fb0ba79deb2fbeea306c18c);\\n \\n \\n\\n circle_marker_b11826274869ad171029ccb503a14c79.bindPopup(popup_07daa1a549dd44294200708947a6fad6)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c7dbdf79d9ac31445da4e2e3a630529 = L.circleMarker(\\n [42.74720109956733, -73.27102394120439],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_3c62c800f4c3b6becb5651775e923bda = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1ff929dd65207e1fd20c95eaf507b75f = $(`<div id="html_1ff929dd65207e1fd20c95eaf507b75f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3c62c800f4c3b6becb5651775e923bda.setContent(html_1ff929dd65207e1fd20c95eaf507b75f);\\n \\n \\n\\n circle_marker_7c7dbdf79d9ac31445da4e2e3a630529.bindPopup(popup_3c62c800f4c3b6becb5651775e923bda)\\n ;\\n\\n \\n \\n \\n var circle_marker_d075fbc74de4e744cb70791a0e7028aa = L.circleMarker(\\n [42.74720125761639, -73.26364141477369],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.4927053303604616, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_3baa00ddb37ce8c2b95972e7ecccaf91 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5131a3c0500b433730c8ef3c5f1ec00e = $(`<div id="html_5131a3c0500b433730c8ef3c5f1ec00e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3baa00ddb37ce8c2b95972e7ecccaf91.setContent(html_5131a3c0500b433730c8ef3c5f1ec00e);\\n \\n \\n\\n circle_marker_d075fbc74de4e744cb70791a0e7028aa.bindPopup(popup_3baa00ddb37ce8c2b95972e7ecccaf91)\\n ;\\n\\n \\n \\n \\n var circle_marker_02b80242f87593a34fbd77141adae42e = L.circleMarker(\\n [42.74720123786026, -73.26241099370067],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_d60eb67dab2d6582d3ccb1811b38a380 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_701d56efdc50e3ab2f5b07aef4228394 = $(`<div id="html_701d56efdc50e3ab2f5b07aef4228394" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d60eb67dab2d6582d3ccb1811b38a380.setContent(html_701d56efdc50e3ab2f5b07aef4228394);\\n \\n \\n\\n circle_marker_02b80242f87593a34fbd77141adae42e.bindPopup(popup_d60eb67dab2d6582d3ccb1811b38a380)\\n ;\\n\\n \\n \\n \\n var circle_marker_58319eaab1bcfe15c5c12169bee35760 = L.circleMarker(\\n [42.74720102712816, -73.25748930942434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.8209479177387813, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_90831443c63f804bf6ae7eedc42bce34 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_345157b22dfcb18568737c5727eea4bc = $(`<div id="html_345157b22dfcb18568737c5727eea4bc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_90831443c63f804bf6ae7eedc42bce34.setContent(html_345157b22dfcb18568737c5727eea4bc);\\n \\n \\n\\n circle_marker_58319eaab1bcfe15c5c12169bee35760.bindPopup(popup_90831443c63f804bf6ae7eedc42bce34)\\n ;\\n\\n \\n \\n \\n var circle_marker_9d9b4b317333aaa762ff63b54356e35d = L.circleMarker(\\n [42.74539347231661, -73.2771756878084],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_64ebb5d2fd088808c8ed1dd45ce0cc4c = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f60dcbf1d0ea1e6cba3072dc741fb6c5 = $(`<div id="html_f60dcbf1d0ea1e6cba3072dc741fb6c5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_64ebb5d2fd088808c8ed1dd45ce0cc4c.setContent(html_f60dcbf1d0ea1e6cba3072dc741fb6c5);\\n \\n \\n\\n circle_marker_9d9b4b317333aaa762ff63b54356e35d.bindPopup(popup_64ebb5d2fd088808c8ed1dd45ce0cc4c)\\n ;\\n\\n \\n \\n \\n var circle_marker_17d2a5f7e8cdff80593174c746e941b4 = L.circleMarker(\\n [42.745393966199934, -73.25871990983394],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.1915382432114616, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_18090a22ad6cb08fc4585e4b8e1b11dc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_226da38af74302d920ccf1c7867bf1c0 = $(`<div id="html_226da38af74302d920ccf1c7867bf1c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_18090a22ad6cb08fc4585e4b8e1b11dc.setContent(html_226da38af74302d920ccf1c7867bf1c0);\\n \\n \\n\\n circle_marker_17d2a5f7e8cdff80593174c746e941b4.bindPopup(popup_18090a22ad6cb08fc4585e4b8e1b11dc)\\n ;\\n\\n \\n \\n \\n var circle_marker_7c7b7c74d273e43dae65e2e01282d2e2 = L.circleMarker(\\n [42.74539389376372, -73.25748952463704],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 7.001408606295148, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_2878e3db6b4ab199e7b658308c682fbf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3726eeefdbcafbbbf8ff466ac3865ca9 = $(`<div id="html_3726eeefdbcafbbbf8ff466ac3865ca9" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2878e3db6b4ab199e7b658308c682fbf.setContent(html_3726eeefdbcafbbbf8ff466ac3865ca9);\\n \\n \\n\\n circle_marker_7c7b7c74d273e43dae65e2e01282d2e2.bindPopup(popup_2878e3db6b4ab199e7b658308c682fbf)\\n ;\\n\\n \\n \\n \\n var circle_marker_1fe0026e0da04f7fdd4f0aa0ba4e9e70 = L.circleMarker(\\n [42.73996598837205, -73.22550295499178],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_9a22ca91ce1b211183a6e8a192a367f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf6498b1b5940a25a4c6b43d43313aa2 = $(`<div id="html_bf6498b1b5940a25a4c6b43d43313aa2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9a22ca91ce1b211183a6e8a192a367f3.setContent(html_bf6498b1b5940a25a4c6b43d43313aa2);\\n \\n \\n\\n circle_marker_1fe0026e0da04f7fdd4f0aa0ba4e9e70.bindPopup(popup_9a22ca91ce1b211183a6e8a192a367f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_da3926bdef53b166d0f74f2c816ce13a = L.circleMarker(\\n [42.7399651192432, -73.22304240032922],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.5854414729132054, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_afdec5acea916050e36e31f833a676fa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_58574a5771e314eba5d2e214b09f72d0 = $(`<div id="html_58574a5771e314eba5d2e214b09f72d0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_afdec5acea916050e36e31f833a676fa.setContent(html_58574a5771e314eba5d2e214b09f72d0);\\n \\n \\n\\n circle_marker_da3926bdef53b166d0f74f2c816ce13a.bindPopup(popup_afdec5acea916050e36e31f833a676fa)\\n ;\\n\\n \\n \\n \\n var circle_marker_d7ff791817c7e3cfb54739f9102d74ac = L.circleMarker(\\n [42.73996466492586, -73.22181212302434],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.51351666838205, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_05b0a2a61f95be899400719b73f4a717 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0bf4c8dec460346f6f5cb0b6b70803ca = $(`<div id="html_0bf4c8dec460346f6f5cb0b6b70803ca" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_05b0a2a61f95be899400719b73f4a717.setContent(html_0bf4c8dec460346f6f5cb0b6b70803ca);\\n \\n \\n\\n circle_marker_d7ff791817c7e3cfb54739f9102d74ac.bindPopup(popup_05b0a2a61f95be899400719b73f4a717)\\n ;\\n\\n \\n \\n \\n var circle_marker_18137f59b49bc1a5846f193a4873aa02 = L.circleMarker(\\n [42.73996419743989, -73.22058184573774],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_4a406e925e42beac4c0c56127ff75e2f = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_21dc29d57dc825a2692531174b24c453 = $(`<div id="html_21dc29d57dc825a2692531174b24c453" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4a406e925e42beac4c0c56127ff75e2f.setContent(html_21dc29d57dc825a2692531174b24c453);\\n \\n \\n\\n circle_marker_18137f59b49bc1a5846f193a4873aa02.bindPopup(popup_4a406e925e42beac4c0c56127ff75e2f)\\n ;\\n\\n \\n \\n \\n var circle_marker_30b4bf33690ea1fa2f29c27d2b073162 = L.circleMarker(\\n [42.73815798617756, -73.22304361947214],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_f2670628e7c36dbef82ca8251220c301 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_31bcac0c4450595cfd27791760d5fe87 = $(`<div id="html_31bcac0c4450595cfd27791760d5fe87" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f2670628e7c36dbef82ca8251220c301.setContent(html_31bcac0c4450595cfd27791760d5fe87);\\n \\n \\n\\n circle_marker_30b4bf33690ea1fa2f29c27d2b073162.bindPopup(popup_f2670628e7c36dbef82ca8251220c301)\\n ;\\n\\n \\n \\n \\n var circle_marker_71674105c191af5acb565832b7826f1e = L.circleMarker(\\n [42.73815753187863, -73.22181337802438],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_24a7fa346c922f6edaa82fd07de434fb = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_6d867396f5665a8d7ff96ca250ad72f2 = $(`<div id="html_6d867396f5665a8d7ff96ca250ad72f2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_24a7fa346c922f6edaa82fd07de434fb.setContent(html_6d867396f5665a8d7ff96ca250ad72f2);\\n \\n \\n\\n circle_marker_71674105c191af5acb565832b7826f1e.bindPopup(popup_24a7fa346c922f6edaa82fd07de434fb)\\n ;\\n\\n \\n \\n \\n var circle_marker_64527b5a19528948194d0fd4eb94c322 = L.circleMarker(\\n [42.7381570644116, -73.2205831365949],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.1850968611841584, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_09e40e0eabd42c5a7db3403d1a264894 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f708776867e56a51a6b9f7287d0b62e = $(`<div id="html_5f708776867e56a51a6b9f7287d0b62e" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_09e40e0eabd42c5a7db3403d1a264894.setContent(html_5f708776867e56a51a6b9f7287d0b62e);\\n \\n \\n\\n circle_marker_64527b5a19528948194d0fd4eb94c322.bindPopup(popup_09e40e0eabd42c5a7db3403d1a264894)\\n ;\\n\\n \\n \\n \\n var circle_marker_817a1764e892646d3000bd480e3f1553 = L.circleMarker(\\n [42.7363580425957, -73.25503018865567],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_5e0247b1a262fd76308e33366029d48a = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_835b92c92f17b8d334d4a869d9fe3ff3 = $(`<div id="html_835b92c92f17b8d334d4a869d9fe3ff3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5e0247b1a262fd76308e33366029d48a.setContent(html_835b92c92f17b8d334d4a869d9fe3ff3);\\n \\n \\n\\n circle_marker_817a1764e892646d3000bd480e3f1553.bindPopup(popup_5e0247b1a262fd76308e33366029d48a)\\n ;\\n\\n \\n \\n \\n var circle_marker_1e3e506b7b07fe5b26d945cb3037fbac = L.circleMarker(\\n [42.73635793067149, -73.25379998277005],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_a75eca38a3e4900639fdce5526a919c7 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_bf76e43915471646f12bdfef8e422612 = $(`<div id="html_bf76e43915471646f12bdfef8e422612" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a75eca38a3e4900639fdce5526a919c7.setContent(html_bf76e43915471646f12bdfef8e422612);\\n \\n \\n\\n circle_marker_1e3e506b7b07fe5b26d945cb3037fbac.bindPopup(popup_a75eca38a3e4900639fdce5526a919c7)\\n ;\\n\\n \\n \\n \\n var circle_marker_b9a7472bc68e94c8d9fbf7b220fbd15e = L.circleMarker(\\n [42.736349931383295, -73.22058442734742],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_fe11c23bed388df4ed1ece4fd64ef058 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_de47085f8cb01eecbb3dfbdd3833bb19 = $(`<div id="html_de47085f8cb01eecbb3dfbdd3833bb19" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fe11c23bed388df4ed1ece4fd64ef058.setContent(html_de47085f8cb01eecbb3dfbdd3833bb19);\\n \\n \\n\\n circle_marker_b9a7472bc68e94c8d9fbf7b220fbd15e.bindPopup(popup_fe11c23bed388df4ed1ece4fd64ef058)\\n ;\\n\\n \\n \\n \\n var circle_marker_ffdd60c0604f39747570ea6b62488e4f = L.circleMarker(\\n [42.73455132399984, -73.2661020059003],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_4fca65f1382f1cd1b8547d12d542639e = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_faccb7e0a8aac322ef17666eb0605de3 = $(`<div id="html_faccb7e0a8aac322ef17666eb0605de3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4fca65f1382f1cd1b8547d12d542639e.setContent(html_faccb7e0a8aac322ef17666eb0605de3);\\n \\n \\n\\n circle_marker_ffdd60c0604f39747570ea6b62488e4f.bindPopup(popup_4fca65f1382f1cd1b8547d12d542639e)\\n ;\\n\\n \\n \\n \\n var circle_marker_b8b2bb4c5e0a206f1d15bb7bd0b6799c = L.circleMarker(\\n [42.73455130424932, -73.26241149574156],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.523362819972964, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_6f58fa2f0890edb42653c6b39eff1aba = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ca018b3ce38e4a56d10c0d1261660a95 = $(`<div id="html_ca018b3ce38e4a56d10c0d1261660a95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6f58fa2f0890edb42653c6b39eff1aba.setContent(html_ca018b3ce38e4a56d10c0d1261660a95);\\n \\n \\n\\n circle_marker_b8b2bb4c5e0a206f1d15bb7bd0b6799c.bindPopup(popup_6f58fa2f0890edb42653c6b39eff1aba)\\n ;\\n\\n \\n \\n \\n var circle_marker_99d035596d2da697f60ff819d3f6e47e = L.circleMarker(\\n [42.73455109357698, -73.25749081554699],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_ae867f8e8d29537d325b0d496d5f1ef5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3f8ff61485809124674f54e6555373dc = $(`<div id="html_3f8ff61485809124674f54e6555373dc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ae867f8e8d29537d325b0d496d5f1ef5.setContent(html_3f8ff61485809124674f54e6555373dc);\\n \\n \\n\\n circle_marker_99d035596d2da697f60ff819d3f6e47e.bindPopup(popup_ae867f8e8d29537d325b0d496d5f1ef5)\\n ;\\n\\n \\n \\n \\n var circle_marker_e70ec4bba46b26b65b7fb67fcd7d1ec2 = L.circleMarker(\\n [42.73454372004621, -73.22304605746157],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.141274657157109, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_fc8412408839190d5b320079d951e039 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f9c5556e921cc4e023f22c83e3828313 = $(`<div id="html_f9c5556e921cc4e023f22c83e3828313" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc8412408839190d5b320079d951e039.setContent(html_f9c5556e921cc4e023f22c83e3828313);\\n \\n \\n\\n circle_marker_e70ec4bba46b26b65b7fb67fcd7d1ec2.bindPopup(popup_fc8412408839190d5b320079d951e039)\\n ;\\n\\n \\n \\n \\n var circle_marker_6be77ded435480277439ce06098fe409 = L.circleMarker(\\n [42.73274396021253, -73.25749103063764],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_6ba65e865d8dba57b61dc4651a499785 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_06cf7ed0fd4be1675f895f2d3c9c4ac0 = $(`<div id="html_06cf7ed0fd4be1675f895f2d3c9c4ac0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_6ba65e865d8dba57b61dc4651a499785.setContent(html_06cf7ed0fd4be1675f895f2d3c9c4ac0);\\n \\n \\n\\n circle_marker_6be77ded435480277439ce06098fe409.bindPopup(popup_6ba65e865d8dba57b61dc4651a499785)\\n ;\\n\\n \\n \\n \\n var circle_marker_9235a192d51b76f3e0d53f6ff746e339 = L.circleMarker(\\n [42.73274182065848, -73.24149928655447],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.9772050238058398, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_dd02ae35e8806e934305475ca0598ac5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_362c875442599910481d026e79ebab67 = $(`<div id="html_362c875442599910481d026e79ebab67" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd02ae35e8806e934305475ca0598ac5.setContent(html_362c875442599910481d026e79ebab67);\\n \\n \\n\\n circle_marker_9235a192d51b76f3e0d53f6ff746e339.bindPopup(popup_dd02ae35e8806e934305475ca0598ac5)\\n ;\\n\\n \\n \\n \\n var circle_marker_c039457fc97244cd0885a86dcd9c60fd = L.circleMarker(\\n [42.7327365869805, -73.22304727630814],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_7413a47955535564b3c4377d6303bd47 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5f79d351375dc21a70d5dbd0bab80854 = $(`<div id="html_5f79d351375dc21a70d5dbd0bab80854" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7413a47955535564b3c4377d6303bd47.setContent(html_5f79d351375dc21a70d5dbd0bab80854);\\n \\n \\n\\n circle_marker_c039457fc97244cd0885a86dcd9c60fd.bindPopup(popup_7413a47955535564b3c4377d6303bd47)\\n ;\\n\\n \\n \\n \\n var circle_marker_a5b242b215be2e55af04ab6064a6ca96 = L.circleMarker(\\n [42.73273613273678, -73.22181714241428],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.4592453796829674, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_50e6e8c892a77e2e299de797bb2e4901 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cf1b3e8f71dc481278ebb53f9590836b = $(`<div id="html_cf1b3e8f71dc481278ebb53f9590836b" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_50e6e8c892a77e2e299de797bb2e4901.setContent(html_cf1b3e8f71dc481278ebb53f9590836b);\\n \\n \\n\\n circle_marker_a5b242b215be2e55af04ab6064a6ca96.bindPopup(popup_50e6e8c892a77e2e299de797bb2e4901)\\n ;\\n\\n \\n \\n \\n var circle_marker_2986462b6031a2a73cb7541bba050c9b = L.circleMarker(\\n [42.73273566532658, -73.22058700853873],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_2ff550ad00da02f536392ee8a0ef9cbe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8106f71f932d486b9f15ef94d5aba67a = $(`<div id="html_8106f71f932d486b9f15ef94d5aba67a" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_2ff550ad00da02f536392ee8a0ef9cbe.setContent(html_8106f71f932d486b9f15ef94d5aba67a);\\n \\n \\n\\n circle_marker_2986462b6031a2a73cb7541bba050c9b.bindPopup(popup_2ff550ad00da02f536392ee8a0ef9cbe)\\n ;\\n\\n \\n \\n \\n var circle_marker_f4678d4ad5f81c8911763ab76abb29a5 = L.circleMarker(\\n [42.73093626729506, -73.25134075401192],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.0342144725641096, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_d47705c4e54f492190a1300e552ce56b = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_84cf4ef8fa295b96124dd49a404b93c0 = $(`<div id="html_84cf4ef8fa295b96124dd49a404b93c0" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d47705c4e54f492190a1300e552ce56b.setContent(html_84cf4ef8fa295b96124dd49a404b93c0);\\n \\n \\n\\n circle_marker_f4678d4ad5f81c8911763ab76abb29a5.bindPopup(popup_d47705c4e54f492190a1300e552ce56b)\\n ;\\n\\n \\n \\n \\n var circle_marker_29bf3e6bcbd1740f5dfeee799bca27d8 = L.circleMarker(\\n [42.730935161355, -73.24396016416856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.9088200952233594, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_86f408ef43f6c9209eeaf735aeeee2c6 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_87ee4930a07ef9565537ff3b17738438 = $(`<div id="html_87ee4930a07ef9565537ff3b17738438" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_86f408ef43f6c9209eeaf735aeeee2c6.setContent(html_87ee4930a07ef9565537ff3b17738438);\\n \\n \\n\\n circle_marker_29bf3e6bcbd1740f5dfeee799bca27d8.bindPopup(popup_86f408ef43f6c9209eeaf735aeeee2c6)\\n ;\\n\\n \\n \\n \\n var circle_marker_fd783edd7a545f5c206934095dac4200 = L.circleMarker(\\n [42.73093443064462, -73.2402698693597],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_1bfc41ffdd62dba1c2b37c3ea3b4a113 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cdcb2dfdc11cc8d4210f8dee005bfa98 = $(`<div id="html_cdcb2dfdc11cc8d4210f8dee005bfa98" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_1bfc41ffdd62dba1c2b37c3ea3b4a113.setContent(html_cdcb2dfdc11cc8d4210f8dee005bfa98);\\n \\n \\n\\n circle_marker_fd783edd7a545f5c206934095dac4200.bindPopup(popup_1bfc41ffdd62dba1c2b37c3ea3b4a113)\\n ;\\n\\n \\n \\n \\n var circle_marker_60aefa879fee3a519b19dcf8999df54e = L.circleMarker(\\n [42.73093294947496, -73.23411937822746],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_bfe2e35cc6be8fc51a422abc7faea0f9 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_dc2defacd56c72f608e5f549c461504c = $(`<div id="html_dc2defacd56c72f608e5f549c461504c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_bfe2e35cc6be8fc51a422abc7faea0f9.setContent(html_dc2defacd56c72f608e5f549c461504c);\\n \\n \\n\\n circle_marker_60aefa879fee3a519b19dcf8999df54e.bindPopup(popup_bfe2e35cc6be8fc51a422abc7faea0f9)\\n ;\\n\\n \\n \\n \\n var circle_marker_38cc23507df1b707daee69d13e2c0375 = L.circleMarker(\\n [42.73093261374318, -73.2328892800381],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_5c8683ea252d5874b235d040cd96d671 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0737c2935b0c81442e6fec355eff9b78 = $(`<div id="html_0737c2935b0c81442e6fec355eff9b78" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_5c8683ea252d5874b235d040cd96d671.setContent(html_0737c2935b0c81442e6fec355eff9b78);\\n \\n \\n\\n circle_marker_38cc23507df1b707daee69d13e2c0375.bindPopup(popup_5c8683ea252d5874b235d040cd96d671)\\n ;\\n\\n \\n \\n \\n var circle_marker_7ae0e845bd258319d06701880b5f365e = L.circleMarker(\\n [42.73092755801783, -73.2181281029743],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_7a4f62294ebfb2d2838594521365c956 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5a308477991966068a14e31d623ae7cc = $(`<div id="html_5a308477991966068a14e31d623ae7cc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_7a4f62294ebfb2d2838594521365c956.setContent(html_5a308477991966068a14e31d623ae7cc);\\n \\n \\n\\n circle_marker_7ae0e845bd258319d06701880b5f365e.bindPopup(popup_7a4f62294ebfb2d2838594521365c956)\\n ;\\n\\n \\n \\n \\n var circle_marker_4ac57e2a35e5caf413ee14b98795f986 = L.circleMarker(\\n [42.72912960790838, -73.2734822734333],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.381976597885342, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_8b770dd7055a27fa1dc11f7dfbf6d385 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_38255756c571f60854aaf3c0d8a42931 = $(`<div id="html_38255756c571f60854aaf3c0d8a42931" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_8b770dd7055a27fa1dc11f7dfbf6d385.setContent(html_38255756c571f60854aaf3c0d8a42931);\\n \\n \\n\\n circle_marker_4ac57e2a35e5caf413ee14b98795f986.bindPopup(popup_8b770dd7055a27fa1dc11f7dfbf6d385)\\n ;\\n\\n \\n \\n \\n var circle_marker_946a6d5e83161d2f47622de397a527d7 = L.circleMarker(\\n [42.72912898255094, -73.25011108579884],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_3d244fc63419d91db7484456a89b0132 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_cbb561e09fb756683f9d32938e25ea60 = $(`<div id="html_cbb561e09fb756683f9d32938e25ea60" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d244fc63419d91db7484456a89b0132.setContent(html_cbb561e09fb756683f9d32938e25ea60);\\n \\n \\n\\n circle_marker_946a6d5e83161d2f47622de397a527d7.bindPopup(popup_3d244fc63419d91db7484456a89b0132)\\n ;\\n\\n \\n \\n \\n var circle_marker_20ef24ccd6f810c85337f339b2780301 = L.circleMarker(\\n [42.729128028058014, -73.24396077349324],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_a380406930f65982492846fb87571d8d = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_eb50bc11dd5cfd5854308050002bf17f = $(`<div id="html_eb50bc11dd5cfd5854308050002bf17f" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a380406930f65982492846fb87571d8d.setContent(html_eb50bc11dd5cfd5854308050002bf17f);\\n \\n \\n\\n circle_marker_20ef24ccd6f810c85337f339b2780301.bindPopup(popup_a380406930f65982492846fb87571d8d)\\n ;\\n\\n \\n \\n \\n var circle_marker_053dcb90a37613f3734e7199830a31fe = L.circleMarker(\\n [42.729127797663175, -73.24273071105665],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.0901936161855166, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_3f5fc5c8e5b645477a607ba2064e5ac5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_3d6cc897b95dd8a4e4e0eedf4e7481df = $(`<div id="html_3d6cc897b95dd8a4e4e0eedf4e7481df" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3f5fc5c8e5b645477a607ba2064e5ac5.setContent(html_3d6cc897b95dd8a4e4e0eedf4e7481df);\\n \\n \\n\\n circle_marker_053dcb90a37613f3734e7199830a31fe.bindPopup(popup_3f5fc5c8e5b645477a607ba2064e5ac5)\\n ;\\n\\n \\n \\n \\n var circle_marker_e1a37c63d69f06663b91da06adf1b3dc = L.circleMarker(\\n [42.72912755410291, -73.24150064862948],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 4.3336224206596095, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_f1aec5d88689dfd95e5af627f6c7db05 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d3ecec49b13ae0e6d0fabef54b11c859 = $(`<div id="html_d3ecec49b13ae0e6d0fabef54b11c859" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_f1aec5d88689dfd95e5af627f6c7db05.setContent(html_d3ecec49b13ae0e6d0fabef54b11c859);\\n \\n \\n\\n circle_marker_e1a37c63d69f06663b91da06adf1b3dc.bindPopup(popup_f1aec5d88689dfd95e5af627f6c7db05)\\n ;\\n\\n \\n \\n \\n var circle_marker_87f2e1da75b56822d222d1d6ee2253a4 = L.circleMarker(\\n [42.72912729737724, -73.24027058621223],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.385137501286538, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_73ec68b3fca9f925ba654224b692a6e4 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_ed5805a0038c650694be262d48fab8fe = $(`<div id="html_ed5805a0038c650694be262d48fab8fe" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_73ec68b3fca9f925ba654224b692a6e4.setContent(html_ed5805a0038c650694be262d48fab8fe);\\n \\n \\n\\n circle_marker_87f2e1da75b56822d222d1d6ee2253a4.bindPopup(popup_73ec68b3fca9f925ba654224b692a6e4)\\n ;\\n\\n \\n \\n \\n var circle_marker_145c8d96a83454a803d6ff08053b42fa = L.circleMarker(\\n [42.729127027486136, -73.23904052380541],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2897623212397704, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_746353f9011b4f1f30f6202cb23662cc = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_400b4a512ce8562ebf482a89b8a4a9e2 = $(`<div id="html_400b4a512ce8562ebf482a89b8a4a9e2" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_746353f9011b4f1f30f6202cb23662cc.setContent(html_400b4a512ce8562ebf482a89b8a4a9e2);\\n \\n \\n\\n circle_marker_145c8d96a83454a803d6ff08053b42fa.bindPopup(popup_746353f9011b4f1f30f6202cb23662cc)\\n ;\\n\\n \\n \\n \\n var circle_marker_142ec3f16ec1b351fef3cb9e9dd4b7d2 = L.circleMarker(\\n [42.7291247696168, -73.23043008729405],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.8712051592547776, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_b5982792cb21ee48770600d77b72eacd = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7581d7be14af7587cbc100247e332ffb = $(`<div id="html_7581d7be14af7587cbc100247e332ffb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_b5982792cb21ee48770600d77b72eacd.setContent(html_7581d7be14af7587cbc100247e332ffb);\\n \\n \\n\\n circle_marker_142ec3f16ec1b351fef3cb9e9dd4b7d2.bindPopup(popup_b5982792cb21ee48770600d77b72eacd)\\n ;\\n\\n \\n \\n \\n var circle_marker_020e8092a88e38d02fec95a2fececb08 = L.circleMarker(\\n [42.72912042502888, -73.2181294649937],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 5.201570947860099, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_41e3428659d600da2f549648e2b199fe = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0139ca115dbb232d3c9291d1fca4bdcb = $(`<div id="html_0139ca115dbb232d3c9291d1fca4bdcb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_41e3428659d600da2f549648e2b199fe.setContent(html_0139ca115dbb232d3c9291d1fca4bdcb);\\n \\n \\n\\n circle_marker_020e8092a88e38d02fec95a2fececb08.bindPopup(popup_41e3428659d600da2f549648e2b199fe)\\n ;\\n\\n \\n \\n \\n var circle_marker_a0d127626b1d64d30501aeb1bbd201ff = L.circleMarker(\\n [42.72732256011916, -73.27225199588943],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_d8d2f22c32603300060723a99d951e54 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_256fe782c6ccf7998063e206445c7c6c = $(`<div id="html_256fe782c6ccf7998063e206445c7c6c" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_d8d2f22c32603300060723a99d951e54.setContent(html_256fe782c6ccf7998063e206445c7c6c);\\n \\n \\n\\n circle_marker_a0d127626b1d64d30501aeb1bbd201ff.bindPopup(popup_d8d2f22c32603300060723a99d951e54)\\n ;\\n\\n \\n \\n \\n var circle_marker_c6b1215d444ad7efa5d7e08213402d33 = L.circleMarker(\\n [42.72732226390921, -73.25380159581911],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6996385101659595, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_dd1b8c828caf591d99a719f9c164a832 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_5be04a289ae364b33a2531c37a9e9652 = $(`<div id="html_5be04a289ae364b33a2531c37a9e9652" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_dd1b8c828caf591d99a719f9c164a832.setContent(html_5be04a289ae364b33a2531c37a9e9652);\\n \\n \\n\\n circle_marker_c6b1215d444ad7efa5d7e08213402d33.bindPopup(popup_dd1b8c828caf591d99a719f9c164a832)\\n ;\\n\\n \\n \\n \\n var circle_marker_af4de2beca6bf24011702efb2b38af37 = L.circleMarker(\\n [42.72732213884279, -73.25257156916585],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.256758334191025, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_9f34c165d290b00bffcdf85ccdc546c5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_e455f1d37e521a5d1d8259db47dda2cc = $(`<div id="html_e455f1d37e521a5d1d8259db47dda2cc" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_9f34c165d290b00bffcdf85ccdc546c5.setContent(html_e455f1d37e521a5d1d8259db47dda2cc);\\n \\n \\n\\n circle_marker_af4de2beca6bf24011702efb2b38af37.bindPopup(popup_9f34c165d290b00bffcdf85ccdc546c5)\\n ;\\n\\n \\n \\n \\n var circle_marker_8c43e6bf0db35949e47d6223710e6ad9 = L.circleMarker(\\n [42.72732200061147, -73.25134154251784],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.2615662610100802, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_a683a8d47b26500f182e1df26e0b9daf = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_d35cbeae4aa74a9ac4c5bae84a891e95 = $(`<div id="html_d35cbeae4aa74a9ac4c5bae84a891e95" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a683a8d47b26500f182e1df26e0b9daf.setContent(html_d35cbeae4aa74a9ac4c5bae84a891e95);\\n \\n \\n\\n circle_marker_8c43e6bf0db35949e47d6223710e6ad9.bindPopup(popup_a683a8d47b26500f182e1df26e0b9daf)\\n ;\\n\\n \\n \\n \\n var circle_marker_e179d13ec02385ed7217756a61d2fb3e = L.circleMarker(\\n [42.72732150692823, -73.24765146261029],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.692568750643269, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_c896d6ed7598a57ecb9a0044f5044c95 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_7bc131ba1c560a6daed67a30e88d65d3 = $(`<div id="html_7bc131ba1c560a6daed67a30e88d65d3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_c896d6ed7598a57ecb9a0044f5044c95.setContent(html_7bc131ba1c560a6daed67a30e88d65d3);\\n \\n \\n\\n circle_marker_e179d13ec02385ed7217756a61d2fb3e.bindPopup(popup_c896d6ed7598a57ecb9a0044f5044c95)\\n ;\\n\\n \\n \\n \\n var circle_marker_2343e10447f975544d237ec718aec5d5 = L.circleMarker(\\n [42.72731989422968, -73.23904127643958],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.742410318509555, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_72bdfaed14e6d24d9e3c46409a7cfe83 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_8c486966a34f180153c0076fb02bd3f3 = $(`<div id="html_8c486966a34f180153c0076fb02bd3f3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_72bdfaed14e6d24d9e3c46409a7cfe83.setContent(html_8c486966a34f180153c0076fb02bd3f3);\\n \\n \\n\\n circle_marker_2343e10447f975544d237ec718aec5d5.bindPopup(popup_72bdfaed14e6d24d9e3c46409a7cfe83)\\n ;\\n\\n \\n \\n \\n var circle_marker_f724db48b058f156e02154a7d8c070f3 = L.circleMarker(\\n [42.72731931497472, -73.2365812233388],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.7978845608028654, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_4fb68a35bf47a111a4ff36eca17975f3 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_601e2513984b5cd335dac413e20e69b5 = $(`<div id="html_601e2513984b5cd335dac413e20e69b5" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_4fb68a35bf47a111a4ff36eca17975f3.setContent(html_601e2513984b5cd335dac413e20e69b5);\\n \\n \\n\\n circle_marker_f724db48b058f156e02154a7d8c070f3.bindPopup(popup_4fb68a35bf47a111a4ff36eca17975f3)\\n ;\\n\\n \\n \\n \\n var circle_marker_9e69f34415d5b1bc9096f9ce9b784ac6 = L.circleMarker(\\n [42.727313785723055, -73.2193608532124],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_a8de5124285b9e0eff99a60984a2e8d5 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_42d2cc895b37c5db07a6d6958b2369c3 = $(`<div id="html_42d2cc895b37c5db07a6d6958b2369c3" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_a8de5124285b9e0eff99a60984a2e8d5.setContent(html_42d2cc895b37c5db07a6d6958b2369c3);\\n \\n \\n\\n circle_marker_9e69f34415d5b1bc9096f9ce9b784ac6.bindPopup(popup_a8de5124285b9e0eff99a60984a2e8d5)\\n ;\\n\\n \\n \\n \\n var circle_marker_70a932c1acd958c2fd934b2b52086c08 = L.circleMarker(\\n [42.72551500549539, -73.27717174416034],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.2410224072142872, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_ec36f750408534dcb3e2c6c6469be4db = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_f895201ed0529e2a0130ce74f2a41348 = $(`<div id="html_f895201ed0529e2a0130ce74f2a41348" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_ec36f750408534dcb3e2c6c6469be4db.setContent(html_f895201ed0529e2a0130ce74f2a41348);\\n \\n \\n\\n circle_marker_70a932c1acd958c2fd934b2b52086c08.bindPopup(popup_ec36f750408534dcb3e2c6c6469be4db)\\n ;\\n\\n \\n \\n \\n var circle_marker_87f23113cc866f44b5ded4c5cb496a90 = L.circleMarker(\\n [42.7255153411864, -73.25626189999694],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 0.5641895835477563, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_3d10dd13781a71690dc54c15d008cdfa = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_0ce28443211a70adc22496f68e13d3cb = $(`<div id="html_0ce28443211a70adc22496f68e13d3cb" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_3d10dd13781a71690dc54c15d008cdfa.setContent(html_0ce28443211a70adc22496f68e13d3cb);\\n \\n \\n\\n circle_marker_87f23113cc866f44b5ded4c5cb496a90.bindPopup(popup_3d10dd13781a71690dc54c15d008cdfa)\\n ;\\n\\n \\n \\n \\n var circle_marker_9cbd49e87f841bcb1d3d9a8f7a33078e = L.circleMarker(\\n [42.72551218174171, -73.2365820475856],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 3.6125759969217834, "stroke": true, "weight": 3}\\n ).addTo(map_f8adf017e5e89e47349dfd03ccc2c586);\\n \\n \\n var popup_97feb0feed4d6540544dafad48ea8183 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_85c5b926f19e0dbb3efc2f2197e61e97 = $(`<div id="html_85c5b926f19e0dbb3efc2f2197e61e97" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_97feb0feed4d6540544dafad48ea8183.setContent(html_85c5b926f19e0dbb3efc2f2197e61e97);\\n \\n \\n\\n circle_marker_9cbd49e87f841bcb1d3d9a8f7a33078e.bindPopup(popup_97feb0feed4d6540544dafad48ea8183)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>",\n "Withe-rod": "<div style=\\"width:100%;\\"><div style=\\"position:relative;width:100%;height:0;padding-bottom:60%;\\"><span style=\\"color:#565656\\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\\"<!DOCTYPE html>\\n<html>\\n<head>\\n \\n <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\\n \\n <script>\\n L_NO_TOUCH = false;\\n L_DISABLE_3D = false;\\n </script>\\n \\n <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\\n <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\\n <script src="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.js"></script>\\n <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\\n <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"></script>\\n <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.9.3/dist/leaflet.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@6.2.0/css/all.min.css"/>\\n <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\\n <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\\n \\n <meta name="viewport" content="width=device-width,\\n initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\\n <style>\\n #map_bbcf56ce380d41956815216ba8c7fa46 {\\n position: relative;\\n width: 720.0px;\\n height: 375.0px;\\n left: 0.0%;\\n top: 0.0%;\\n }\\n .leaflet-container { font-size: 1rem; }\\n </style>\\n \\n</head>\\n<body>\\n \\n \\n <div class="folium-map" id="map_bbcf56ce380d41956815216ba8c7fa46" ></div>\\n \\n</body>\\n<script>\\n \\n \\n var map_bbcf56ce380d41956815216ba8c7fa46 = L.map(\\n "map_bbcf56ce380d41956815216ba8c7fa46",\\n {\\n center: [42.731838490821076, -73.25748900571338],\\n crs: L.CRS.EPSG3857,\\n zoom: 11,\\n zoomControl: true,\\n preferCanvas: false,\\n clusteredMarker: false,\\n includeColorScaleOutliers: true,\\n radiusInMeters: false,\\n }\\n );\\n\\n \\n\\n \\n \\n var tile_layer_f995470fe8a1b23b1b1c1257f309e767 = L.tileLayer(\\n "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\\n {"attribution": "Data by \\\\u0026copy; \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://openstreetmap.org\\\\"\\\\u003eOpenStreetMap\\\\u003c/a\\\\u003e, under \\\\u003ca target=\\\\"_blank\\\\" href=\\\\"http://www.openstreetmap.org/copyright\\\\"\\\\u003eODbL\\\\u003c/a\\\\u003e.", "detectRetina": false, "maxNativeZoom": 17, "maxZoom": 17, "minZoom": 9, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\\n ).addTo(map_bbcf56ce380d41956815216ba8c7fa46);\\n \\n \\n var circle_marker_22fff0d5fce2933807dbff6177094f9f = L.circleMarker(\\n [42.73816211437248, -73.23657627645521],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 2.326213245840639, "stroke": true, "weight": 3}\\n ).addTo(map_bbcf56ce380d41956815216ba8c7fa46);\\n \\n \\n var popup_350cd6795d908fa26e415b432f126f94 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_1e16adc35f551d4367c5bdc5b0936585 = $(`<div id="html_1e16adc35f551d4367c5bdc5b0936585" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_350cd6795d908fa26e415b432f126f94.setContent(html_1e16adc35f551d4367c5bdc5b0936585);\\n \\n \\n\\n circle_marker_22fff0d5fce2933807dbff6177094f9f.bindPopup(popup_350cd6795d908fa26e415b432f126f94)\\n ;\\n\\n \\n \\n \\n var circle_marker_8487533319e91510a274be1b4536fc3b = L.circleMarker(\\n [42.72551486726968, -73.27840173497155],\\n {"bubblingMouseEvents": true, "color": "blue", "dashArray": null, "dashOffset": null, "fill": true, "fillColor": "blue", "fillOpacity": 0.2, "fillRule": "evenodd", "lineCap": "round", "lineJoin": "round", "opacity": 1.0, "radius": 1.1283791670955126, "stroke": true, "weight": 3}\\n ).addTo(map_bbcf56ce380d41956815216ba8c7fa46);\\n \\n \\n var popup_fc8c91aa26c6fa9ac07f1d86b12a6167 = L.popup({"maxWidth": "100%"});\\n\\n \\n \\n var html_53ca52ebab5a426eac7fa4a51b169975 = $(`<div id="html_53ca52ebab5a426eac7fa4a51b169975" style="width: 100.0%; height: 100.0%;"></div>`)[0];\\n popup_fc8c91aa26c6fa9ac07f1d86b12a6167.setContent(html_53ca52ebab5a426eac7fa4a51b169975);\\n \\n \\n\\n circle_marker_8487533319e91510a274be1b4536fc3b.bindPopup(popup_fc8c91aa26c6fa9ac07f1d86b12a6167)\\n ;\\n\\n \\n \\n</script>\\n</html>\\" style=\\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>"\n};\n\n function update_i_2() {\n var text = createCSVLine([i_1_value()]);\n _output_i_2.innerHTML = _cache_i_2[text];\n } \n update_i_2();\n\n\n _choice_i_1.oninput = function() { update_i_2(); }\n </script>\n'
Even More Visualization#
def population_choropleth(tree_name):
counts = trees_with_lat_lon.where("common name", tree_name).select("plot", "count")
return HopkinsForest.map_table(counts)
population_choropleth("Maple, red")
Make this Notebook Trusted to load map: File -> Trust Notebook