Conditionals and Loops¶
from datascience import *
import numpy as np
%matplotlib inline
import matplotlib.pyplot as plots
plots.style.use('fivethirtyeight')
import warnings
warnings.simplefilter(action='ignore', category=np.VisibleDeprecationWarning)
Booleans and Comparison Operators¶
3 > 1
True
type(3 > 1)
bool
3 = 3
File "/var/folders/md/kwd9nc_d2ns0hw9wsvdrnt2c0000gn/T/ipykernel_4262/3802935557.py", line 1
3 = 3
^
SyntaxError: cannot assign to literal
3 == 3
True
x = 5
y = 12
x == 7
False
y - x
7
4 < y - x <= 6
False
4 < y - x
True
y - x <= 6
False
True and False
False
True or False
True
True and True
True
Monopoly¶
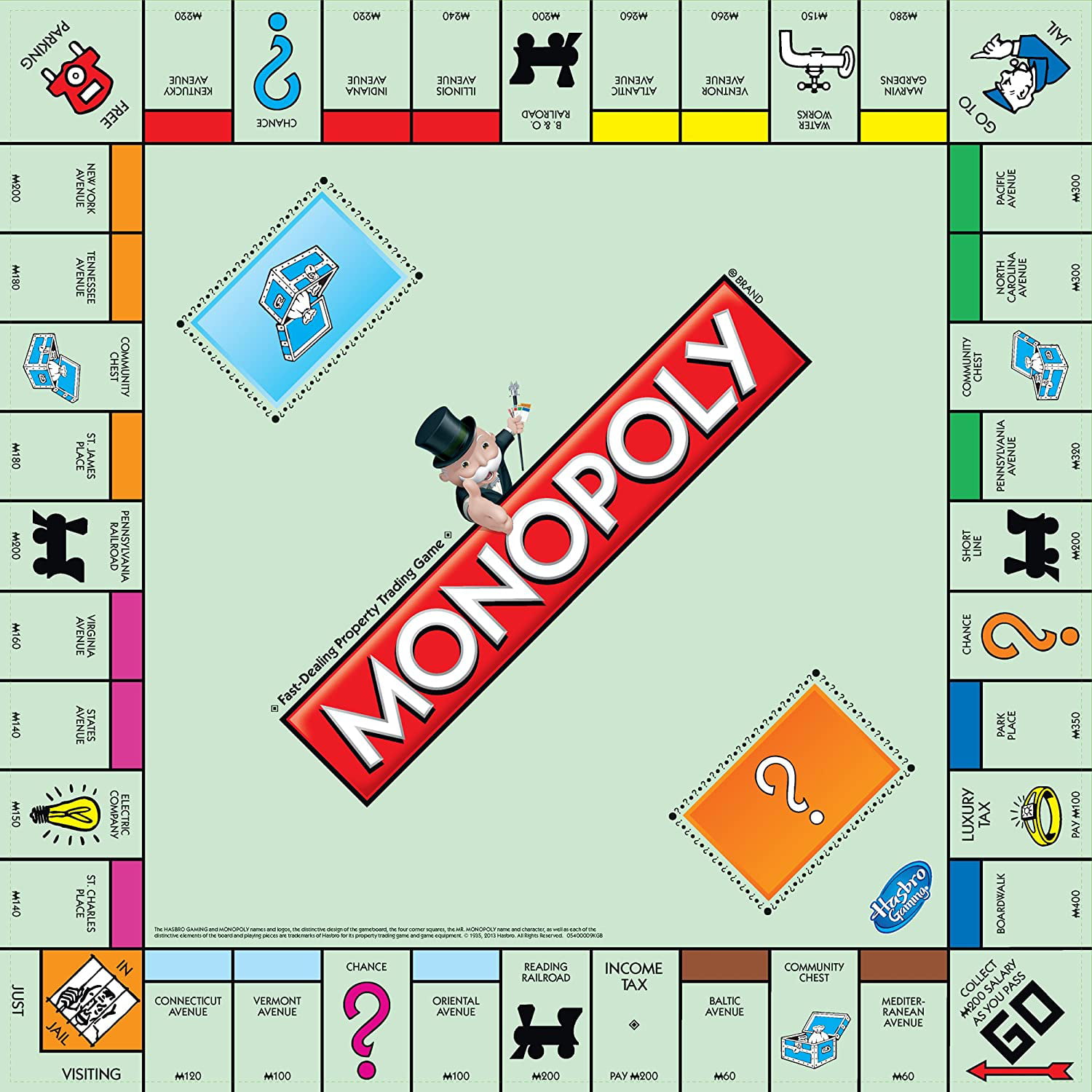
monopoly = Table().read_table("data/monopoly.csv")
monopoly
Name | Space | Color | Position | Price | PriceBuild | Rent | RentBuild1 | RentBuild2 | RentBuild3 | RentBuild4 | RentBuild5 | Number |
---|---|---|---|---|---|---|---|---|---|---|---|---|
Go | Go | None | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Mediterranean Avenue | Street | Brown | 1 | 60 | 50 | 2 | 10 | 30 | 90 | 160 | 250 | 2 |
Community Chest | Chest | None | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Baltic Avenue | Street | Brown | 3 | 60 | 50 | 4 | 20 | 60 | 180 | 320 | 450 | 2 |
Income Tax | Tax | None | 4 | 200 | 0 | 200 | 0 | 0 | 0 | 0 | 0 | 0 |
Reading Railroad | Railroad | None | 5 | 200 | 0 | 25 | 0 | 0 | 0 | 0 | 0 | 0 |
Oriental Avenue | Street | LightBlue | 6 | 100 | 50 | 6 | 30 | 90 | 270 | 400 | 550 | 3 |
Chance | Chance | None | 7 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
Vermont Avenue | Street | LightBlue | 8 | 100 | 50 | 6 | 30 | 90 | 270 | 400 | 550 | 3 |
Connecticut Avenue | Street | LightBlue | 9 | 120 | 50 | 8 | 40 | 100 | 300 | 450 | 600 | 3 |
... (30 rows omitted)
tiny_monopoly = monopoly.where('Color', are.not_equal_to('None'))
tiny_monopoly = tiny_monopoly.where('Space', are.containing('Street'))
tiny_monopoly = tiny_monopoly.select('Name', 'Color', 'Price')
tiny_monopoly = tiny_monopoly.sort('Name')
tiny_monopoly.show()
Name | Color | Price |
---|---|---|
Atlantic Avenue | Yellow | 260 |
Baltic Avenue | Brown | 60 |
Boardwalk | Blue | 400 |
Connecticut Avenue | LightBlue | 120 |
Illinois Avenue | Red | 240 |
Indiana Avenue | Red | 220 |
Kentucky Avenue | Red | 220 |
Marvin Gardens | Yellow | 280 |
Mediterranean Avenue | Brown | 60 |
New York Avenue | Orange | 200 |
North Carolina Avenue | Green | 300 |
Oriental Avenue | LightBlue | 100 |
Pacific Avenue | Green | 300 |
Park Place | Blue | 350 |
Pennsylvania Avenue | Green | 320 |
St. Charles Place | Pink | 140 |
St. James Place | Orange | 180 |
States Avenue | Pink | 140 |
Tennessee Avenue | Orange | 180 |
Ventnor Avenue | Yellow | 260 |
Vermont Avenue | LightBlue | 100 |
Virginia Avenue | Pink | 160 |
Suppose we only have 220 dollars. How many properties could we buy for exactly 220 dollars?
price = tiny_monopoly.column("Price")
price
array([260, 60, 400, 120, 240, 220, 220, 280, 60, 200, 300, 100, 300,
350, 320, 140, 180, 140, 180, 260, 100, 160])
price == 220
array([False, False, False, False, False, True, True, False, False,
False, False, False, False, False, False, False, False, False,
False, False, False, False])
sum(price==220)
2
np.count_nonzero(price == 220)
2
How many properties could we buy for less than or equal to 200 dollars?
price
array([260, 60, 400, 120, 240, 220, 220, 280, 60, 200, 300, 100, 300,
350, 320, 140, 180, 140, 180, 260, 100, 160])
price <= 200
array([False, True, False, True, False, False, False, False, True,
True, False, True, False, False, False, True, True, True,
True, False, True, True])
np.count_nonzero(price <= 200)
11
How many of the Monopoly spaces are light blue?
sum(monopoly.column("Color") == "LightBlue")
3
Conditional Statements¶
def price_rating(price):
if price < 100:
print("Inexpensive")
price_rating(50)
Inexpensive
price_rating(500)
def price_rating(price):
if price < 100:
print("Inexpensive")
else:
print("Expensive")
price_rating(500)
Expensive
# return a value instead of printing
def price_rating(price):
if price < 200:
return "Inexpensive"
elif price < 300:
return "Expensive"
elif price < 400:
return "Very Expensive"
else:
return "Outrageous"
ratings = tiny_monopoly.apply(price_rating, 'Price')
ratings
array(['Expensive', 'Inexpensive', 'Outrageous', 'Inexpensive',
'Expensive', 'Expensive', 'Expensive', 'Expensive', 'Inexpensive',
'Expensive', 'Very Expensive', 'Inexpensive', 'Very Expensive',
'Very Expensive', 'Very Expensive', 'Inexpensive', 'Inexpensive',
'Inexpensive', 'Inexpensive', 'Expensive', 'Inexpensive',
'Inexpensive'], dtype='<U14')
rated_monopoly = tiny_monopoly.with_columns("Cost Rating", ratings)
rated_monopoly
Name | Color | Price | Cost Rating |
---|---|---|---|
Atlantic Avenue | Yellow | 260 | Expensive |
Baltic Avenue | Brown | 60 | Inexpensive |
Boardwalk | Blue | 400 | Outrageous |
Connecticut Avenue | LightBlue | 120 | Inexpensive |
Illinois Avenue | Red | 240 | Expensive |
Indiana Avenue | Red | 220 | Expensive |
Kentucky Avenue | Red | 220 | Expensive |
Marvin Gardens | Yellow | 280 | Expensive |
Mediterranean Avenue | Brown | 60 | Inexpensive |
New York Avenue | Orange | 200 | Expensive |
... (12 rows omitted)
rated_monopoly.where('Cost Rating', 'Inexpensive')
Name | Color | Price | Cost Rating |
---|---|---|---|
Baltic Avenue | Brown | 60 | Inexpensive |
Connecticut Avenue | LightBlue | 120 | Inexpensive |
Mediterranean Avenue | Brown | 60 | Inexpensive |
Oriental Avenue | LightBlue | 100 | Inexpensive |
St. Charles Place | Pink | 140 | Inexpensive |
St. James Place | Orange | 180 | Inexpensive |
States Avenue | Pink | 140 | Inexpensive |
Tennessee Avenue | Orange | 180 | Inexpensive |
Vermont Avenue | LightBlue | 100 | Inexpensive |
Virginia Avenue | Pink | 160 | Inexpensive |
rated_monopoly.where('Cost Rating', 'Outrageous')
Name | Color | Price | Cost Rating |
---|---|---|---|
Boardwalk | Blue | 400 | Outrageous |